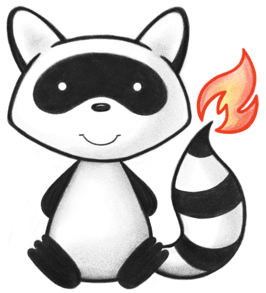
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.instance.model.api.IBaseOperationOutcome; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045 046/** 047 * A collection of error, warning, or information messages that result from a 048 * system action. 049 */ 050@ResourceDef(name = "OperationOutcome", profile = "http://hl7.org/fhir/StructureDefinition/OperationOutcome") 051public class OperationOutcome extends DomainResource implements IBaseOperationOutcome { 052 053 public enum IssueSeverity { 054 /** 055 * The issue caused the action to fail and no further checking could be 056 * performed. 057 */ 058 FATAL, 059 /** 060 * The issue is sufficiently important to cause the action to fail. 061 */ 062 ERROR, 063 /** 064 * The issue is not important enough to cause the action to fail but may cause 065 * it to be performed suboptimally or in a way that is not as desired. 066 */ 067 WARNING, 068 /** 069 * The issue has no relation to the degree of success of the action. 070 */ 071 INFORMATION, 072 /** 073 * added to help the parsers with the generic types 074 */ 075 NULL; 076 077 public static IssueSeverity fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("fatal".equals(codeString)) 081 return FATAL; 082 if ("error".equals(codeString)) 083 return ERROR; 084 if ("warning".equals(codeString)) 085 return WARNING; 086 if ("information".equals(codeString)) 087 return INFORMATION; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown IssueSeverity code '" + codeString + "'"); 092 } 093 094 public String toCode() { 095 switch (this) { 096 case FATAL: 097 return "fatal"; 098 case ERROR: 099 return "error"; 100 case WARNING: 101 return "warning"; 102 case INFORMATION: 103 return "information"; 104 case NULL: 105 return null; 106 default: 107 return "?"; 108 } 109 } 110 111 public String getSystem() { 112 switch (this) { 113 case FATAL: 114 return "http://hl7.org/fhir/issue-severity"; 115 case ERROR: 116 return "http://hl7.org/fhir/issue-severity"; 117 case WARNING: 118 return "http://hl7.org/fhir/issue-severity"; 119 case INFORMATION: 120 return "http://hl7.org/fhir/issue-severity"; 121 case NULL: 122 return null; 123 default: 124 return "?"; 125 } 126 } 127 128 public String getDefinition() { 129 switch (this) { 130 case FATAL: 131 return "The issue caused the action to fail and no further checking could be performed."; 132 case ERROR: 133 return "The issue is sufficiently important to cause the action to fail."; 134 case WARNING: 135 return "The issue is not important enough to cause the action to fail but may cause it to be performed suboptimally or in a way that is not as desired."; 136 case INFORMATION: 137 return "The issue has no relation to the degree of success of the action."; 138 case NULL: 139 return null; 140 default: 141 return "?"; 142 } 143 } 144 145 public String getDisplay() { 146 switch (this) { 147 case FATAL: 148 return "Fatal"; 149 case ERROR: 150 return "Error"; 151 case WARNING: 152 return "Warning"; 153 case INFORMATION: 154 return "Information"; 155 case NULL: 156 return null; 157 default: 158 return "?"; 159 } 160 } 161 } 162 163 public static class IssueSeverityEnumFactory implements EnumFactory<IssueSeverity> { 164 public IssueSeverity fromCode(String codeString) throws IllegalArgumentException { 165 if (codeString == null || "".equals(codeString)) 166 if (codeString == null || "".equals(codeString)) 167 return null; 168 if ("fatal".equals(codeString)) 169 return IssueSeverity.FATAL; 170 if ("error".equals(codeString)) 171 return IssueSeverity.ERROR; 172 if ("warning".equals(codeString)) 173 return IssueSeverity.WARNING; 174 if ("information".equals(codeString)) 175 return IssueSeverity.INFORMATION; 176 throw new IllegalArgumentException("Unknown IssueSeverity code '" + codeString + "'"); 177 } 178 179 public Enumeration<IssueSeverity> fromType(PrimitiveType<?> code) throws FHIRException { 180 if (code == null) 181 return null; 182 if (code.isEmpty()) 183 return new Enumeration<IssueSeverity>(this, IssueSeverity.NULL, code); 184 String codeString = code.asStringValue(); 185 if (codeString == null || "".equals(codeString)) 186 return new Enumeration<IssueSeverity>(this, IssueSeverity.NULL, code); 187 if ("fatal".equals(codeString)) 188 return new Enumeration<IssueSeverity>(this, IssueSeverity.FATAL, code); 189 if ("error".equals(codeString)) 190 return new Enumeration<IssueSeverity>(this, IssueSeverity.ERROR, code); 191 if ("warning".equals(codeString)) 192 return new Enumeration<IssueSeverity>(this, IssueSeverity.WARNING, code); 193 if ("information".equals(codeString)) 194 return new Enumeration<IssueSeverity>(this, IssueSeverity.INFORMATION, code); 195 throw new FHIRException("Unknown IssueSeverity code '" + codeString + "'"); 196 } 197 198 public String toCode(IssueSeverity code) { 199 if (code == IssueSeverity.NULL) 200 return null; 201 if (code == IssueSeverity.FATAL) 202 return "fatal"; 203 if (code == IssueSeverity.ERROR) 204 return "error"; 205 if (code == IssueSeverity.WARNING) 206 return "warning"; 207 if (code == IssueSeverity.INFORMATION) 208 return "information"; 209 return "?"; 210 } 211 212 public String toSystem(IssueSeverity code) { 213 return code.getSystem(); 214 } 215 } 216 217 public enum IssueType { 218 /** 219 * Content invalid against the specification or a profile. 220 */ 221 INVALID, 222 /** 223 * A structural issue in the content such as wrong namespace, unable to parse 224 * the content completely, invalid syntax, etc. 225 */ 226 STRUCTURE, 227 /** 228 * A required element is missing. 229 */ 230 REQUIRED, 231 /** 232 * An element or header value is invalid. 233 */ 234 VALUE, 235 /** 236 * A content validation rule failed - e.g. a schematron rule. 237 */ 238 INVARIANT, 239 /** 240 * An authentication/authorization/permissions issue of some kind. 241 */ 242 SECURITY, 243 /** 244 * The client needs to initiate an authentication process. 245 */ 246 LOGIN, 247 /** 248 * The user or system was not able to be authenticated (either there is no 249 * process, or the proferred token is unacceptable). 250 */ 251 UNKNOWN, 252 /** 253 * User session expired; a login may be required. 254 */ 255 EXPIRED, 256 /** 257 * The user does not have the rights to perform this action. 258 */ 259 FORBIDDEN, 260 /** 261 * Some information was not or might not have been returned due to business 262 * rules, consent or privacy rules, or access permission constraints. This 263 * information may be accessible through alternate processes. 264 */ 265 SUPPRESSED, 266 /** 267 * Processing issues. These are expected to be final e.g. there is no point 268 * resubmitting the same content unchanged. 269 */ 270 PROCESSING, 271 /** 272 * The interaction, operation, resource or profile is not supported. 273 */ 274 NOTSUPPORTED, 275 /** 276 * An attempt was made to create a duplicate record. 277 */ 278 DUPLICATE, 279 /** 280 * Multiple matching records were found when the operation required only one 281 * match. 282 */ 283 MULTIPLEMATCHES, 284 /** 285 * The reference provided was not found. In a pure RESTful environment, this 286 * would be an HTTP 404 error, but this code may be used where the content is 287 * not found further into the application architecture. 288 */ 289 NOTFOUND, 290 /** 291 * The reference pointed to content (usually a resource) that has been deleted. 292 */ 293 DELETED, 294 /** 295 * Provided content is too long (typically, this is a denial of service 296 * protection type of error). 297 */ 298 TOOLONG, 299 /** 300 * The code or system could not be understood, or it was not valid in the 301 * context of a particular ValueSet.code. 302 */ 303 CODEINVALID, 304 /** 305 * An extension was found that was not acceptable, could not be resolved, or a 306 * modifierExtension was not recognized. 307 */ 308 EXTENSION, 309 /** 310 * The operation was stopped to protect server resources; e.g. a request for a 311 * value set expansion on all of SNOMED CT. 312 */ 313 TOOCOSTLY, 314 /** 315 * The content/operation failed to pass some business rule and so could not 316 * proceed. 317 */ 318 BUSINESSRULE, 319 /** 320 * Content could not be accepted because of an edit conflict (i.e. version aware 321 * updates). (In a pure RESTful environment, this would be an HTTP 409 error, 322 * but this code may be used where the conflict is discovered further into the 323 * application architecture.). 324 */ 325 CONFLICT, 326 /** 327 * Transient processing issues. The system receiving the message may be able to 328 * resubmit the same content once an underlying issue is resolved. 329 */ 330 TRANSIENT, 331 /** 332 * A resource/record locking failure (usually in an underlying database). 333 */ 334 LOCKERROR, 335 /** 336 * The persistent store is unavailable; e.g. the database is down for 337 * maintenance or similar action, and the interaction or operation cannot be 338 * processed. 339 */ 340 NOSTORE, 341 /** 342 * An unexpected internal error has occurred. 343 */ 344 EXCEPTION, 345 /** 346 * An internal timeout has occurred. 347 */ 348 TIMEOUT, 349 /** 350 * Not all data sources typically accessed could be reached or responded in 351 * time, so the returned information might not be complete (applies to search 352 * interactions and some operations). 353 */ 354 INCOMPLETE, 355 /** 356 * The system is not prepared to handle this request due to load management. 357 */ 358 THROTTLED, 359 /** 360 * A message unrelated to the processing success of the completed operation 361 * (examples of the latter include things like reminders of password expiry, 362 * system maintenance times, etc.). 363 */ 364 INFORMATIONAL, 365 /** 366 * added to help the parsers with the generic types 367 */ 368 NULL; 369 370 public static IssueType fromCode(String codeString) throws FHIRException { 371 if (codeString == null || "".equals(codeString)) 372 return null; 373 if ("invalid".equals(codeString)) 374 return INVALID; 375 if ("structure".equals(codeString)) 376 return STRUCTURE; 377 if ("required".equals(codeString)) 378 return REQUIRED; 379 if ("value".equals(codeString)) 380 return VALUE; 381 if ("invariant".equals(codeString)) 382 return INVARIANT; 383 if ("security".equals(codeString)) 384 return SECURITY; 385 if ("login".equals(codeString)) 386 return LOGIN; 387 if ("unknown".equals(codeString)) 388 return UNKNOWN; 389 if ("expired".equals(codeString)) 390 return EXPIRED; 391 if ("forbidden".equals(codeString)) 392 return FORBIDDEN; 393 if ("suppressed".equals(codeString)) 394 return SUPPRESSED; 395 if ("processing".equals(codeString)) 396 return PROCESSING; 397 if ("not-supported".equals(codeString)) 398 return NOTSUPPORTED; 399 if ("duplicate".equals(codeString)) 400 return DUPLICATE; 401 if ("multiple-matches".equals(codeString)) 402 return MULTIPLEMATCHES; 403 if ("not-found".equals(codeString)) 404 return NOTFOUND; 405 if ("deleted".equals(codeString)) 406 return DELETED; 407 if ("too-long".equals(codeString)) 408 return TOOLONG; 409 if ("code-invalid".equals(codeString)) 410 return CODEINVALID; 411 if ("extension".equals(codeString)) 412 return EXTENSION; 413 if ("too-costly".equals(codeString)) 414 return TOOCOSTLY; 415 if ("business-rule".equals(codeString)) 416 return BUSINESSRULE; 417 if ("conflict".equals(codeString)) 418 return CONFLICT; 419 if ("transient".equals(codeString)) 420 return TRANSIENT; 421 if ("lock-error".equals(codeString)) 422 return LOCKERROR; 423 if ("no-store".equals(codeString)) 424 return NOSTORE; 425 if ("exception".equals(codeString)) 426 return EXCEPTION; 427 if ("timeout".equals(codeString)) 428 return TIMEOUT; 429 if ("incomplete".equals(codeString)) 430 return INCOMPLETE; 431 if ("throttled".equals(codeString)) 432 return THROTTLED; 433 if ("informational".equals(codeString)) 434 return INFORMATIONAL; 435 if (Configuration.isAcceptInvalidEnums()) 436 return null; 437 else 438 throw new FHIRException("Unknown IssueType code '" + codeString + "'"); 439 } 440 441 public String toCode() { 442 switch (this) { 443 case INVALID: 444 return "invalid"; 445 case STRUCTURE: 446 return "structure"; 447 case REQUIRED: 448 return "required"; 449 case VALUE: 450 return "value"; 451 case INVARIANT: 452 return "invariant"; 453 case SECURITY: 454 return "security"; 455 case LOGIN: 456 return "login"; 457 case UNKNOWN: 458 return "unknown"; 459 case EXPIRED: 460 return "expired"; 461 case FORBIDDEN: 462 return "forbidden"; 463 case SUPPRESSED: 464 return "suppressed"; 465 case PROCESSING: 466 return "processing"; 467 case NOTSUPPORTED: 468 return "not-supported"; 469 case DUPLICATE: 470 return "duplicate"; 471 case MULTIPLEMATCHES: 472 return "multiple-matches"; 473 case NOTFOUND: 474 return "not-found"; 475 case DELETED: 476 return "deleted"; 477 case TOOLONG: 478 return "too-long"; 479 case CODEINVALID: 480 return "code-invalid"; 481 case EXTENSION: 482 return "extension"; 483 case TOOCOSTLY: 484 return "too-costly"; 485 case BUSINESSRULE: 486 return "business-rule"; 487 case CONFLICT: 488 return "conflict"; 489 case TRANSIENT: 490 return "transient"; 491 case LOCKERROR: 492 return "lock-error"; 493 case NOSTORE: 494 return "no-store"; 495 case EXCEPTION: 496 return "exception"; 497 case TIMEOUT: 498 return "timeout"; 499 case INCOMPLETE: 500 return "incomplete"; 501 case THROTTLED: 502 return "throttled"; 503 case INFORMATIONAL: 504 return "informational"; 505 case NULL: 506 return null; 507 default: 508 return "?"; 509 } 510 } 511 512 public String getSystem() { 513 switch (this) { 514 case INVALID: 515 return "http://hl7.org/fhir/issue-type"; 516 case STRUCTURE: 517 return "http://hl7.org/fhir/issue-type"; 518 case REQUIRED: 519 return "http://hl7.org/fhir/issue-type"; 520 case VALUE: 521 return "http://hl7.org/fhir/issue-type"; 522 case INVARIANT: 523 return "http://hl7.org/fhir/issue-type"; 524 case SECURITY: 525 return "http://hl7.org/fhir/issue-type"; 526 case LOGIN: 527 return "http://hl7.org/fhir/issue-type"; 528 case UNKNOWN: 529 return "http://hl7.org/fhir/issue-type"; 530 case EXPIRED: 531 return "http://hl7.org/fhir/issue-type"; 532 case FORBIDDEN: 533 return "http://hl7.org/fhir/issue-type"; 534 case SUPPRESSED: 535 return "http://hl7.org/fhir/issue-type"; 536 case PROCESSING: 537 return "http://hl7.org/fhir/issue-type"; 538 case NOTSUPPORTED: 539 return "http://hl7.org/fhir/issue-type"; 540 case DUPLICATE: 541 return "http://hl7.org/fhir/issue-type"; 542 case MULTIPLEMATCHES: 543 return "http://hl7.org/fhir/issue-type"; 544 case NOTFOUND: 545 return "http://hl7.org/fhir/issue-type"; 546 case DELETED: 547 return "http://hl7.org/fhir/issue-type"; 548 case TOOLONG: 549 return "http://hl7.org/fhir/issue-type"; 550 case CODEINVALID: 551 return "http://hl7.org/fhir/issue-type"; 552 case EXTENSION: 553 return "http://hl7.org/fhir/issue-type"; 554 case TOOCOSTLY: 555 return "http://hl7.org/fhir/issue-type"; 556 case BUSINESSRULE: 557 return "http://hl7.org/fhir/issue-type"; 558 case CONFLICT: 559 return "http://hl7.org/fhir/issue-type"; 560 case TRANSIENT: 561 return "http://hl7.org/fhir/issue-type"; 562 case LOCKERROR: 563 return "http://hl7.org/fhir/issue-type"; 564 case NOSTORE: 565 return "http://hl7.org/fhir/issue-type"; 566 case EXCEPTION: 567 return "http://hl7.org/fhir/issue-type"; 568 case TIMEOUT: 569 return "http://hl7.org/fhir/issue-type"; 570 case INCOMPLETE: 571 return "http://hl7.org/fhir/issue-type"; 572 case THROTTLED: 573 return "http://hl7.org/fhir/issue-type"; 574 case INFORMATIONAL: 575 return "http://hl7.org/fhir/issue-type"; 576 case NULL: 577 return null; 578 default: 579 return "?"; 580 } 581 } 582 583 public String getDefinition() { 584 switch (this) { 585 case INVALID: 586 return "Content invalid against the specification or a profile."; 587 case STRUCTURE: 588 return "A structural issue in the content such as wrong namespace, unable to parse the content completely, invalid syntax, etc."; 589 case REQUIRED: 590 return "A required element is missing."; 591 case VALUE: 592 return "An element or header value is invalid."; 593 case INVARIANT: 594 return "A content validation rule failed - e.g. a schematron rule."; 595 case SECURITY: 596 return "An authentication/authorization/permissions issue of some kind."; 597 case LOGIN: 598 return "The client needs to initiate an authentication process."; 599 case UNKNOWN: 600 return "The user or system was not able to be authenticated (either there is no process, or the proferred token is unacceptable)."; 601 case EXPIRED: 602 return "User session expired; a login may be required."; 603 case FORBIDDEN: 604 return "The user does not have the rights to perform this action."; 605 case SUPPRESSED: 606 return "Some information was not or might not have been returned due to business rules, consent or privacy rules, or access permission constraints. This information may be accessible through alternate processes."; 607 case PROCESSING: 608 return "Processing issues. These are expected to be final e.g. there is no point resubmitting the same content unchanged."; 609 case NOTSUPPORTED: 610 return "The interaction, operation, resource or profile is not supported."; 611 case DUPLICATE: 612 return "An attempt was made to create a duplicate record."; 613 case MULTIPLEMATCHES: 614 return "Multiple matching records were found when the operation required only one match."; 615 case NOTFOUND: 616 return "The reference provided was not found. In a pure RESTful environment, this would be an HTTP 404 error, but this code may be used where the content is not found further into the application architecture."; 617 case DELETED: 618 return "The reference pointed to content (usually a resource) that has been deleted."; 619 case TOOLONG: 620 return "Provided content is too long (typically, this is a denial of service protection type of error)."; 621 case CODEINVALID: 622 return "The code or system could not be understood, or it was not valid in the context of a particular ValueSet.code."; 623 case EXTENSION: 624 return "An extension was found that was not acceptable, could not be resolved, or a modifierExtension was not recognized."; 625 case TOOCOSTLY: 626 return "The operation was stopped to protect server resources; e.g. a request for a value set expansion on all of SNOMED CT."; 627 case BUSINESSRULE: 628 return "The content/operation failed to pass some business rule and so could not proceed."; 629 case CONFLICT: 630 return "Content could not be accepted because of an edit conflict (i.e. version aware updates). (In a pure RESTful environment, this would be an HTTP 409 error, but this code may be used where the conflict is discovered further into the application architecture.)."; 631 case TRANSIENT: 632 return "Transient processing issues. The system receiving the message may be able to resubmit the same content once an underlying issue is resolved."; 633 case LOCKERROR: 634 return "A resource/record locking failure (usually in an underlying database)."; 635 case NOSTORE: 636 return "The persistent store is unavailable; e.g. the database is down for maintenance or similar action, and the interaction or operation cannot be processed."; 637 case EXCEPTION: 638 return "An unexpected internal error has occurred."; 639 case TIMEOUT: 640 return "An internal timeout has occurred."; 641 case INCOMPLETE: 642 return "Not all data sources typically accessed could be reached or responded in time, so the returned information might not be complete (applies to search interactions and some operations)."; 643 case THROTTLED: 644 return "The system is not prepared to handle this request due to load management."; 645 case INFORMATIONAL: 646 return "A message unrelated to the processing success of the completed operation (examples of the latter include things like reminders of password expiry, system maintenance times, etc.)."; 647 case NULL: 648 return null; 649 default: 650 return "?"; 651 } 652 } 653 654 public String getDisplay() { 655 switch (this) { 656 case INVALID: 657 return "Invalid Content"; 658 case STRUCTURE: 659 return "Structural Issue"; 660 case REQUIRED: 661 return "Required element missing"; 662 case VALUE: 663 return "Element value invalid"; 664 case INVARIANT: 665 return "Validation rule failed"; 666 case SECURITY: 667 return "Security Problem"; 668 case LOGIN: 669 return "Login Required"; 670 case UNKNOWN: 671 return "Unknown User"; 672 case EXPIRED: 673 return "Session Expired"; 674 case FORBIDDEN: 675 return "Forbidden"; 676 case SUPPRESSED: 677 return "Information Suppressed"; 678 case PROCESSING: 679 return "Processing Failure"; 680 case NOTSUPPORTED: 681 return "Content not supported"; 682 case DUPLICATE: 683 return "Duplicate"; 684 case MULTIPLEMATCHES: 685 return "Multiple Matches"; 686 case NOTFOUND: 687 return "Not Found"; 688 case DELETED: 689 return "Deleted"; 690 case TOOLONG: 691 return "Content Too Long"; 692 case CODEINVALID: 693 return "Invalid Code"; 694 case EXTENSION: 695 return "Unacceptable Extension"; 696 case TOOCOSTLY: 697 return "Operation Too Costly"; 698 case BUSINESSRULE: 699 return "Business Rule Violation"; 700 case CONFLICT: 701 return "Edit Version Conflict"; 702 case TRANSIENT: 703 return "Transient Issue"; 704 case LOCKERROR: 705 return "Lock Error"; 706 case NOSTORE: 707 return "No Store Available"; 708 case EXCEPTION: 709 return "Exception"; 710 case TIMEOUT: 711 return "Timeout"; 712 case INCOMPLETE: 713 return "Incomplete Results"; 714 case THROTTLED: 715 return "Throttled"; 716 case INFORMATIONAL: 717 return "Informational Note"; 718 case NULL: 719 return null; 720 default: 721 return "?"; 722 } 723 } 724 } 725 726 public static class IssueTypeEnumFactory implements EnumFactory<IssueType> { 727 public IssueType fromCode(String codeString) throws IllegalArgumentException { 728 if (codeString == null || "".equals(codeString)) 729 if (codeString == null || "".equals(codeString)) 730 return null; 731 if ("invalid".equals(codeString)) 732 return IssueType.INVALID; 733 if ("structure".equals(codeString)) 734 return IssueType.STRUCTURE; 735 if ("required".equals(codeString)) 736 return IssueType.REQUIRED; 737 if ("value".equals(codeString)) 738 return IssueType.VALUE; 739 if ("invariant".equals(codeString)) 740 return IssueType.INVARIANT; 741 if ("security".equals(codeString)) 742 return IssueType.SECURITY; 743 if ("login".equals(codeString)) 744 return IssueType.LOGIN; 745 if ("unknown".equals(codeString)) 746 return IssueType.UNKNOWN; 747 if ("expired".equals(codeString)) 748 return IssueType.EXPIRED; 749 if ("forbidden".equals(codeString)) 750 return IssueType.FORBIDDEN; 751 if ("suppressed".equals(codeString)) 752 return IssueType.SUPPRESSED; 753 if ("processing".equals(codeString)) 754 return IssueType.PROCESSING; 755 if ("not-supported".equals(codeString)) 756 return IssueType.NOTSUPPORTED; 757 if ("duplicate".equals(codeString)) 758 return IssueType.DUPLICATE; 759 if ("multiple-matches".equals(codeString)) 760 return IssueType.MULTIPLEMATCHES; 761 if ("not-found".equals(codeString)) 762 return IssueType.NOTFOUND; 763 if ("deleted".equals(codeString)) 764 return IssueType.DELETED; 765 if ("too-long".equals(codeString)) 766 return IssueType.TOOLONG; 767 if ("code-invalid".equals(codeString)) 768 return IssueType.CODEINVALID; 769 if ("extension".equals(codeString)) 770 return IssueType.EXTENSION; 771 if ("too-costly".equals(codeString)) 772 return IssueType.TOOCOSTLY; 773 if ("business-rule".equals(codeString)) 774 return IssueType.BUSINESSRULE; 775 if ("conflict".equals(codeString)) 776 return IssueType.CONFLICT; 777 if ("transient".equals(codeString)) 778 return IssueType.TRANSIENT; 779 if ("lock-error".equals(codeString)) 780 return IssueType.LOCKERROR; 781 if ("no-store".equals(codeString)) 782 return IssueType.NOSTORE; 783 if ("exception".equals(codeString)) 784 return IssueType.EXCEPTION; 785 if ("timeout".equals(codeString)) 786 return IssueType.TIMEOUT; 787 if ("incomplete".equals(codeString)) 788 return IssueType.INCOMPLETE; 789 if ("throttled".equals(codeString)) 790 return IssueType.THROTTLED; 791 if ("informational".equals(codeString)) 792 return IssueType.INFORMATIONAL; 793 throw new IllegalArgumentException("Unknown IssueType code '" + codeString + "'"); 794 } 795 796 public Enumeration<IssueType> fromType(PrimitiveType<?> code) throws FHIRException { 797 if (code == null) 798 return null; 799 if (code.isEmpty()) 800 return new Enumeration<IssueType>(this, IssueType.NULL, code); 801 String codeString = code.asStringValue(); 802 if (codeString == null || "".equals(codeString)) 803 return new Enumeration<IssueType>(this, IssueType.NULL, code); 804 if ("invalid".equals(codeString)) 805 return new Enumeration<IssueType>(this, IssueType.INVALID, code); 806 if ("structure".equals(codeString)) 807 return new Enumeration<IssueType>(this, IssueType.STRUCTURE, code); 808 if ("required".equals(codeString)) 809 return new Enumeration<IssueType>(this, IssueType.REQUIRED, code); 810 if ("value".equals(codeString)) 811 return new Enumeration<IssueType>(this, IssueType.VALUE, code); 812 if ("invariant".equals(codeString)) 813 return new Enumeration<IssueType>(this, IssueType.INVARIANT, code); 814 if ("security".equals(codeString)) 815 return new Enumeration<IssueType>(this, IssueType.SECURITY, code); 816 if ("login".equals(codeString)) 817 return new Enumeration<IssueType>(this, IssueType.LOGIN, code); 818 if ("unknown".equals(codeString)) 819 return new Enumeration<IssueType>(this, IssueType.UNKNOWN, code); 820 if ("expired".equals(codeString)) 821 return new Enumeration<IssueType>(this, IssueType.EXPIRED, code); 822 if ("forbidden".equals(codeString)) 823 return new Enumeration<IssueType>(this, IssueType.FORBIDDEN, code); 824 if ("suppressed".equals(codeString)) 825 return new Enumeration<IssueType>(this, IssueType.SUPPRESSED, code); 826 if ("processing".equals(codeString)) 827 return new Enumeration<IssueType>(this, IssueType.PROCESSING, code); 828 if ("not-supported".equals(codeString)) 829 return new Enumeration<IssueType>(this, IssueType.NOTSUPPORTED, code); 830 if ("duplicate".equals(codeString)) 831 return new Enumeration<IssueType>(this, IssueType.DUPLICATE, code); 832 if ("multiple-matches".equals(codeString)) 833 return new Enumeration<IssueType>(this, IssueType.MULTIPLEMATCHES, code); 834 if ("not-found".equals(codeString)) 835 return new Enumeration<IssueType>(this, IssueType.NOTFOUND, code); 836 if ("deleted".equals(codeString)) 837 return new Enumeration<IssueType>(this, IssueType.DELETED, code); 838 if ("too-long".equals(codeString)) 839 return new Enumeration<IssueType>(this, IssueType.TOOLONG, code); 840 if ("code-invalid".equals(codeString)) 841 return new Enumeration<IssueType>(this, IssueType.CODEINVALID, code); 842 if ("extension".equals(codeString)) 843 return new Enumeration<IssueType>(this, IssueType.EXTENSION, code); 844 if ("too-costly".equals(codeString)) 845 return new Enumeration<IssueType>(this, IssueType.TOOCOSTLY, code); 846 if ("business-rule".equals(codeString)) 847 return new Enumeration<IssueType>(this, IssueType.BUSINESSRULE, code); 848 if ("conflict".equals(codeString)) 849 return new Enumeration<IssueType>(this, IssueType.CONFLICT, code); 850 if ("transient".equals(codeString)) 851 return new Enumeration<IssueType>(this, IssueType.TRANSIENT, code); 852 if ("lock-error".equals(codeString)) 853 return new Enumeration<IssueType>(this, IssueType.LOCKERROR, code); 854 if ("no-store".equals(codeString)) 855 return new Enumeration<IssueType>(this, IssueType.NOSTORE, code); 856 if ("exception".equals(codeString)) 857 return new Enumeration<IssueType>(this, IssueType.EXCEPTION, code); 858 if ("timeout".equals(codeString)) 859 return new Enumeration<IssueType>(this, IssueType.TIMEOUT, code); 860 if ("incomplete".equals(codeString)) 861 return new Enumeration<IssueType>(this, IssueType.INCOMPLETE, code); 862 if ("throttled".equals(codeString)) 863 return new Enumeration<IssueType>(this, IssueType.THROTTLED, code); 864 if ("informational".equals(codeString)) 865 return new Enumeration<IssueType>(this, IssueType.INFORMATIONAL, code); 866 throw new FHIRException("Unknown IssueType code '" + codeString + "'"); 867 } 868 869 public String toCode(IssueType code) { 870 if (code == IssueType.NULL) 871 return null; 872 if (code == IssueType.INVALID) 873 return "invalid"; 874 if (code == IssueType.STRUCTURE) 875 return "structure"; 876 if (code == IssueType.REQUIRED) 877 return "required"; 878 if (code == IssueType.VALUE) 879 return "value"; 880 if (code == IssueType.INVARIANT) 881 return "invariant"; 882 if (code == IssueType.SECURITY) 883 return "security"; 884 if (code == IssueType.LOGIN) 885 return "login"; 886 if (code == IssueType.UNKNOWN) 887 return "unknown"; 888 if (code == IssueType.EXPIRED) 889 return "expired"; 890 if (code == IssueType.FORBIDDEN) 891 return "forbidden"; 892 if (code == IssueType.SUPPRESSED) 893 return "suppressed"; 894 if (code == IssueType.PROCESSING) 895 return "processing"; 896 if (code == IssueType.NOTSUPPORTED) 897 return "not-supported"; 898 if (code == IssueType.DUPLICATE) 899 return "duplicate"; 900 if (code == IssueType.MULTIPLEMATCHES) 901 return "multiple-matches"; 902 if (code == IssueType.NOTFOUND) 903 return "not-found"; 904 if (code == IssueType.DELETED) 905 return "deleted"; 906 if (code == IssueType.TOOLONG) 907 return "too-long"; 908 if (code == IssueType.CODEINVALID) 909 return "code-invalid"; 910 if (code == IssueType.EXTENSION) 911 return "extension"; 912 if (code == IssueType.TOOCOSTLY) 913 return "too-costly"; 914 if (code == IssueType.BUSINESSRULE) 915 return "business-rule"; 916 if (code == IssueType.CONFLICT) 917 return "conflict"; 918 if (code == IssueType.TRANSIENT) 919 return "transient"; 920 if (code == IssueType.LOCKERROR) 921 return "lock-error"; 922 if (code == IssueType.NOSTORE) 923 return "no-store"; 924 if (code == IssueType.EXCEPTION) 925 return "exception"; 926 if (code == IssueType.TIMEOUT) 927 return "timeout"; 928 if (code == IssueType.INCOMPLETE) 929 return "incomplete"; 930 if (code == IssueType.THROTTLED) 931 return "throttled"; 932 if (code == IssueType.INFORMATIONAL) 933 return "informational"; 934 return "?"; 935 } 936 937 public String toSystem(IssueType code) { 938 return code.getSystem(); 939 } 940 } 941 942 @Block() 943 public static class OperationOutcomeIssueComponent extends BackboneElement implements IBaseBackboneElement { 944 /** 945 * Indicates whether the issue indicates a variation from successful processing. 946 */ 947 @Child(name = "severity", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 948 @Description(shortDefinition = "fatal | error | warning | information", formalDefinition = "Indicates whether the issue indicates a variation from successful processing.") 949 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/issue-severity") 950 protected Enumeration<IssueSeverity> severity; 951 952 /** 953 * Describes the type of the issue. The system that creates an OperationOutcome 954 * SHALL choose the most applicable code from the IssueType value set, and may 955 * additional provide its own code for the error in the details element. 956 */ 957 @Child(name = "code", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 958 @Description(shortDefinition = "Error or warning code", formalDefinition = "Describes the type of the issue. The system that creates an OperationOutcome SHALL choose the most applicable code from the IssueType value set, and may additional provide its own code for the error in the details element.") 959 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/issue-type") 960 protected Enumeration<IssueType> code; 961 962 /** 963 * Additional details about the error. This may be a text description of the 964 * error or a system code that identifies the error. 965 */ 966 @Child(name = "details", type = { 967 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 968 @Description(shortDefinition = "Additional details about the error", formalDefinition = "Additional details about the error. This may be a text description of the error or a system code that identifies the error.") 969 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/operation-outcome") 970 protected CodeableConcept details; 971 972 /** 973 * Additional diagnostic information about the issue. 974 */ 975 @Child(name = "diagnostics", type = { 976 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 977 @Description(shortDefinition = "Additional diagnostic information about the issue", formalDefinition = "Additional diagnostic information about the issue.") 978 protected StringType diagnostics; 979 980 /** 981 * This element is deprecated because it is XML specific. It is replaced by 982 * issue.expression, which is format independent, and simpler to parse. 983 * 984 * For resource issues, this will be a simple XPath limited to element names, 985 * repetition indicators and the default child accessor that identifies one of 986 * the elements in the resource that caused this issue to be raised. For HTTP 987 * errors, will be "http." + the parameter name. 988 */ 989 @Child(name = "location", type = { 990 StringType.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 991 @Description(shortDefinition = "Deprecated: Path of element(s) related to issue", formalDefinition = "This element is deprecated because it is XML specific. It is replaced by issue.expression, which is format independent, and simpler to parse. \n\nFor resource issues, this will be a simple XPath limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised. For HTTP errors, will be \"http.\" + the parameter name.") 992 protected List<StringType> location; 993 994 /** 995 * A [simple subset of FHIRPath](fhirpath.html#simple) limited to element names, 996 * repetition indicators and the default child accessor that identifies one of 997 * the elements in the resource that caused this issue to be raised. 998 */ 999 @Child(name = "expression", type = { 1000 StringType.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1001 @Description(shortDefinition = "FHIRPath of element(s) related to issue", formalDefinition = "A [simple subset of FHIRPath](fhirpath.html#simple) limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised.") 1002 protected List<StringType> expression; 1003 1004 private static final long serialVersionUID = -1681095438L; 1005 1006 /** 1007 * Constructor 1008 */ 1009 public OperationOutcomeIssueComponent() { 1010 super(); 1011 } 1012 1013 /** 1014 * Constructor 1015 */ 1016 public OperationOutcomeIssueComponent(Enumeration<IssueSeverity> severity, Enumeration<IssueType> code) { 1017 super(); 1018 this.severity = severity; 1019 this.code = code; 1020 } 1021 1022 /** 1023 * @return {@link #severity} (Indicates whether the issue indicates a variation 1024 * from successful processing.). This is the underlying object with id, 1025 * value and extensions. The accessor "getSeverity" gives direct access 1026 * to the value 1027 */ 1028 public Enumeration<IssueSeverity> getSeverityElement() { 1029 if (this.severity == null) 1030 if (Configuration.errorOnAutoCreate()) 1031 throw new Error("Attempt to auto-create OperationOutcomeIssueComponent.severity"); 1032 else if (Configuration.doAutoCreate()) 1033 this.severity = new Enumeration<IssueSeverity>(new IssueSeverityEnumFactory()); // bb 1034 return this.severity; 1035 } 1036 1037 public boolean hasSeverityElement() { 1038 return this.severity != null && !this.severity.isEmpty(); 1039 } 1040 1041 public boolean hasSeverity() { 1042 return this.severity != null && !this.severity.isEmpty(); 1043 } 1044 1045 /** 1046 * @param value {@link #severity} (Indicates whether the issue indicates a 1047 * variation from successful processing.). This is the underlying 1048 * object with id, value and extensions. The accessor "getSeverity" 1049 * gives direct access to the value 1050 */ 1051 public OperationOutcomeIssueComponent setSeverityElement(Enumeration<IssueSeverity> value) { 1052 this.severity = value; 1053 return this; 1054 } 1055 1056 /** 1057 * @return Indicates whether the issue indicates a variation from successful 1058 * processing. 1059 */ 1060 public IssueSeverity getSeverity() { 1061 return this.severity == null ? null : this.severity.getValue(); 1062 } 1063 1064 /** 1065 * @param value Indicates whether the issue indicates a variation from 1066 * successful processing. 1067 */ 1068 public OperationOutcomeIssueComponent setSeverity(IssueSeverity value) { 1069 if (this.severity == null) 1070 this.severity = new Enumeration<IssueSeverity>(new IssueSeverityEnumFactory()); 1071 this.severity.setValue(value); 1072 return this; 1073 } 1074 1075 /** 1076 * @return {@link #code} (Describes the type of the issue. The system that 1077 * creates an OperationOutcome SHALL choose the most applicable code 1078 * from the IssueType value set, and may additional provide its own code 1079 * for the error in the details element.). This is the underlying object 1080 * with id, value and extensions. The accessor "getCode" gives direct 1081 * access to the value 1082 */ 1083 public Enumeration<IssueType> getCodeElement() { 1084 if (this.code == null) 1085 if (Configuration.errorOnAutoCreate()) 1086 throw new Error("Attempt to auto-create OperationOutcomeIssueComponent.code"); 1087 else if (Configuration.doAutoCreate()) 1088 this.code = new Enumeration<IssueType>(new IssueTypeEnumFactory()); // bb 1089 return this.code; 1090 } 1091 1092 public boolean hasCodeElement() { 1093 return this.code != null && !this.code.isEmpty(); 1094 } 1095 1096 public boolean hasCode() { 1097 return this.code != null && !this.code.isEmpty(); 1098 } 1099 1100 /** 1101 * @param value {@link #code} (Describes the type of the issue. The system that 1102 * creates an OperationOutcome SHALL choose the most applicable 1103 * code from the IssueType value set, and may additional provide 1104 * its own code for the error in the details element.). This is the 1105 * underlying object with id, value and extensions. The accessor 1106 * "getCode" gives direct access to the value 1107 */ 1108 public OperationOutcomeIssueComponent setCodeElement(Enumeration<IssueType> value) { 1109 this.code = value; 1110 return this; 1111 } 1112 1113 /** 1114 * @return Describes the type of the issue. The system that creates an 1115 * OperationOutcome SHALL choose the most applicable code from the 1116 * IssueType value set, and may additional provide its own code for the 1117 * error in the details element. 1118 */ 1119 public IssueType getCode() { 1120 return this.code == null ? null : this.code.getValue(); 1121 } 1122 1123 /** 1124 * @param value Describes the type of the issue. The system that creates an 1125 * OperationOutcome SHALL choose the most applicable code from the 1126 * IssueType value set, and may additional provide its own code for 1127 * the error in the details element. 1128 */ 1129 public OperationOutcomeIssueComponent setCode(IssueType value) { 1130 if (this.code == null) 1131 this.code = new Enumeration<IssueType>(new IssueTypeEnumFactory()); 1132 this.code.setValue(value); 1133 return this; 1134 } 1135 1136 /** 1137 * @return {@link #details} (Additional details about the error. This may be a 1138 * text description of the error or a system code that identifies the 1139 * error.) 1140 */ 1141 public CodeableConcept getDetails() { 1142 if (this.details == null) 1143 if (Configuration.errorOnAutoCreate()) 1144 throw new Error("Attempt to auto-create OperationOutcomeIssueComponent.details"); 1145 else if (Configuration.doAutoCreate()) 1146 this.details = new CodeableConcept(); // cc 1147 return this.details; 1148 } 1149 1150 public boolean hasDetails() { 1151 return this.details != null && !this.details.isEmpty(); 1152 } 1153 1154 /** 1155 * @param value {@link #details} (Additional details about the error. This may 1156 * be a text description of the error or a system code that 1157 * identifies the error.) 1158 */ 1159 public OperationOutcomeIssueComponent setDetails(CodeableConcept value) { 1160 this.details = value; 1161 return this; 1162 } 1163 1164 /** 1165 * @return {@link #diagnostics} (Additional diagnostic information about the 1166 * issue.). This is the underlying object with id, value and extensions. 1167 * The accessor "getDiagnostics" gives direct access to the value 1168 */ 1169 public StringType getDiagnosticsElement() { 1170 if (this.diagnostics == null) 1171 if (Configuration.errorOnAutoCreate()) 1172 throw new Error("Attempt to auto-create OperationOutcomeIssueComponent.diagnostics"); 1173 else if (Configuration.doAutoCreate()) 1174 this.diagnostics = new StringType(); // bb 1175 return this.diagnostics; 1176 } 1177 1178 public boolean hasDiagnosticsElement() { 1179 return this.diagnostics != null && !this.diagnostics.isEmpty(); 1180 } 1181 1182 public boolean hasDiagnostics() { 1183 return this.diagnostics != null && !this.diagnostics.isEmpty(); 1184 } 1185 1186 /** 1187 * @param value {@link #diagnostics} (Additional diagnostic information about 1188 * the issue.). This is the underlying object with id, value and 1189 * extensions. The accessor "getDiagnostics" gives direct access to 1190 * the value 1191 */ 1192 public OperationOutcomeIssueComponent setDiagnosticsElement(StringType value) { 1193 this.diagnostics = value; 1194 return this; 1195 } 1196 1197 /** 1198 * @return Additional diagnostic information about the issue. 1199 */ 1200 public String getDiagnostics() { 1201 return this.diagnostics == null ? null : this.diagnostics.getValue(); 1202 } 1203 1204 /** 1205 * @param value Additional diagnostic information about the issue. 1206 */ 1207 public OperationOutcomeIssueComponent setDiagnostics(String value) { 1208 if (Utilities.noString(value)) 1209 this.diagnostics = null; 1210 else { 1211 if (this.diagnostics == null) 1212 this.diagnostics = new StringType(); 1213 this.diagnostics.setValue(value); 1214 } 1215 return this; 1216 } 1217 1218 /** 1219 * @return {@link #location} (This element is deprecated because it is XML 1220 * specific. It is replaced by issue.expression, which is format 1221 * independent, and simpler to parse. 1222 * 1223 * For resource issues, this will be a simple XPath limited to element 1224 * names, repetition indicators and the default child accessor that 1225 * identifies one of the elements in the resource that caused this issue 1226 * to be raised. For HTTP errors, will be "http." + the parameter name.) 1227 */ 1228 public List<StringType> getLocation() { 1229 if (this.location == null) 1230 this.location = new ArrayList<StringType>(); 1231 return this.location; 1232 } 1233 1234 /** 1235 * @return Returns a reference to <code>this</code> for easy method chaining 1236 */ 1237 public OperationOutcomeIssueComponent setLocation(List<StringType> theLocation) { 1238 this.location = theLocation; 1239 return this; 1240 } 1241 1242 public boolean hasLocation() { 1243 if (this.location == null) 1244 return false; 1245 for (StringType item : this.location) 1246 if (!item.isEmpty()) 1247 return true; 1248 return false; 1249 } 1250 1251 /** 1252 * @return {@link #location} (This element is deprecated because it is XML 1253 * specific. It is replaced by issue.expression, which is format 1254 * independent, and simpler to parse. 1255 * 1256 * For resource issues, this will be a simple XPath limited to element 1257 * names, repetition indicators and the default child accessor that 1258 * identifies one of the elements in the resource that caused this issue 1259 * to be raised. For HTTP errors, will be "http." + the parameter name.) 1260 */ 1261 public StringType addLocationElement() {// 2 1262 StringType t = new StringType(); 1263 if (this.location == null) 1264 this.location = new ArrayList<StringType>(); 1265 this.location.add(t); 1266 return t; 1267 } 1268 1269 /** 1270 * @param value {@link #location} (This element is deprecated because it is XML 1271 * specific. It is replaced by issue.expression, which is format 1272 * independent, and simpler to parse. 1273 * 1274 * For resource issues, this will be a simple XPath limited to 1275 * element names, repetition indicators and the default child 1276 * accessor that identifies one of the elements in the resource 1277 * that caused this issue to be raised. For HTTP errors, will be 1278 * "http." + the parameter name.) 1279 */ 1280 public OperationOutcomeIssueComponent addLocation(String value) { // 1 1281 StringType t = new StringType(); 1282 t.setValue(value); 1283 if (this.location == null) 1284 this.location = new ArrayList<StringType>(); 1285 this.location.add(t); 1286 return this; 1287 } 1288 1289 /** 1290 * @param value {@link #location} (This element is deprecated because it is XML 1291 * specific. It is replaced by issue.expression, which is format 1292 * independent, and simpler to parse. 1293 * 1294 * For resource issues, this will be a simple XPath limited to 1295 * element names, repetition indicators and the default child 1296 * accessor that identifies one of the elements in the resource 1297 * that caused this issue to be raised. For HTTP errors, will be 1298 * "http." + the parameter name.) 1299 */ 1300 public boolean hasLocation(String value) { 1301 if (this.location == null) 1302 return false; 1303 for (StringType v : this.location) 1304 if (v.getValue().equals(value)) // string 1305 return true; 1306 return false; 1307 } 1308 1309 /** 1310 * @return {@link #expression} (A [simple subset of 1311 * FHIRPath](fhirpath.html#simple) limited to element names, repetition 1312 * indicators and the default child accessor that identifies one of the 1313 * elements in the resource that caused this issue to be raised.) 1314 */ 1315 public List<StringType> getExpression() { 1316 if (this.expression == null) 1317 this.expression = new ArrayList<StringType>(); 1318 return this.expression; 1319 } 1320 1321 /** 1322 * @return Returns a reference to <code>this</code> for easy method chaining 1323 */ 1324 public OperationOutcomeIssueComponent setExpression(List<StringType> theExpression) { 1325 this.expression = theExpression; 1326 return this; 1327 } 1328 1329 public boolean hasExpression() { 1330 if (this.expression == null) 1331 return false; 1332 for (StringType item : this.expression) 1333 if (!item.isEmpty()) 1334 return true; 1335 return false; 1336 } 1337 1338 /** 1339 * @return {@link #expression} (A [simple subset of 1340 * FHIRPath](fhirpath.html#simple) limited to element names, repetition 1341 * indicators and the default child accessor that identifies one of the 1342 * elements in the resource that caused this issue to be raised.) 1343 */ 1344 public StringType addExpressionElement() {// 2 1345 StringType t = new StringType(); 1346 if (this.expression == null) 1347 this.expression = new ArrayList<StringType>(); 1348 this.expression.add(t); 1349 return t; 1350 } 1351 1352 /** 1353 * @param value {@link #expression} (A [simple subset of 1354 * FHIRPath](fhirpath.html#simple) limited to element names, 1355 * repetition indicators and the default child accessor that 1356 * identifies one of the elements in the resource that caused this 1357 * issue to be raised.) 1358 */ 1359 public OperationOutcomeIssueComponent addExpression(String value) { // 1 1360 StringType t = new StringType(); 1361 t.setValue(value); 1362 if (this.expression == null) 1363 this.expression = new ArrayList<StringType>(); 1364 this.expression.add(t); 1365 return this; 1366 } 1367 1368 /** 1369 * @param value {@link #expression} (A [simple subset of 1370 * FHIRPath](fhirpath.html#simple) limited to element names, 1371 * repetition indicators and the default child accessor that 1372 * identifies one of the elements in the resource that caused this 1373 * issue to be raised.) 1374 */ 1375 public boolean hasExpression(String value) { 1376 if (this.expression == null) 1377 return false; 1378 for (StringType v : this.expression) 1379 if (v.getValue().equals(value)) // string 1380 return true; 1381 return false; 1382 } 1383 1384 protected void listChildren(List<Property> children) { 1385 super.listChildren(children); 1386 children.add(new Property("severity", "code", 1387 "Indicates whether the issue indicates a variation from successful processing.", 0, 1, severity)); 1388 children.add(new Property("code", "code", 1389 "Describes the type of the issue. The system that creates an OperationOutcome SHALL choose the most applicable code from the IssueType value set, and may additional provide its own code for the error in the details element.", 1390 0, 1, code)); 1391 children.add(new Property("details", "CodeableConcept", 1392 "Additional details about the error. This may be a text description of the error or a system code that identifies the error.", 1393 0, 1, details)); 1394 children.add(new Property("diagnostics", "string", "Additional diagnostic information about the issue.", 0, 1, 1395 diagnostics)); 1396 children.add(new Property("location", "string", 1397 "This element is deprecated because it is XML specific. It is replaced by issue.expression, which is format independent, and simpler to parse. \n\nFor resource issues, this will be a simple XPath limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised. For HTTP errors, will be \"http.\" + the parameter name.", 1398 0, java.lang.Integer.MAX_VALUE, location)); 1399 children.add(new Property("expression", "string", 1400 "A [simple subset of FHIRPath](fhirpath.html#simple) limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised.", 1401 0, java.lang.Integer.MAX_VALUE, expression)); 1402 } 1403 1404 @Override 1405 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1406 switch (_hash) { 1407 case 1478300413: 1408 /* severity */ return new Property("severity", "code", 1409 "Indicates whether the issue indicates a variation from successful processing.", 0, 1, severity); 1410 case 3059181: 1411 /* code */ return new Property("code", "code", 1412 "Describes the type of the issue. The system that creates an OperationOutcome SHALL choose the most applicable code from the IssueType value set, and may additional provide its own code for the error in the details element.", 1413 0, 1, code); 1414 case 1557721666: 1415 /* details */ return new Property("details", "CodeableConcept", 1416 "Additional details about the error. This may be a text description of the error or a system code that identifies the error.", 1417 0, 1, details); 1418 case -740386388: 1419 /* diagnostics */ return new Property("diagnostics", "string", 1420 "Additional diagnostic information about the issue.", 0, 1, diagnostics); 1421 case 1901043637: 1422 /* location */ return new Property("location", "string", 1423 "This element is deprecated because it is XML specific. It is replaced by issue.expression, which is format independent, and simpler to parse. \n\nFor resource issues, this will be a simple XPath limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised. For HTTP errors, will be \"http.\" + the parameter name.", 1424 0, java.lang.Integer.MAX_VALUE, location); 1425 case -1795452264: 1426 /* expression */ return new Property("expression", "string", 1427 "A [simple subset of FHIRPath](fhirpath.html#simple) limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised.", 1428 0, java.lang.Integer.MAX_VALUE, expression); 1429 default: 1430 return super.getNamedProperty(_hash, _name, _checkValid); 1431 } 1432 1433 } 1434 1435 @Override 1436 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1437 switch (hash) { 1438 case 1478300413: 1439 /* severity */ return this.severity == null ? new Base[0] : new Base[] { this.severity }; // Enumeration<IssueSeverity> 1440 case 3059181: 1441 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // Enumeration<IssueType> 1442 case 1557721666: 1443 /* details */ return this.details == null ? new Base[0] : new Base[] { this.details }; // CodeableConcept 1444 case -740386388: 1445 /* diagnostics */ return this.diagnostics == null ? new Base[0] : new Base[] { this.diagnostics }; // StringType 1446 case 1901043637: 1447 /* location */ return this.location == null ? new Base[0] 1448 : this.location.toArray(new Base[this.location.size()]); // StringType 1449 case -1795452264: 1450 /* expression */ return this.expression == null ? new Base[0] 1451 : this.expression.toArray(new Base[this.expression.size()]); // StringType 1452 default: 1453 return super.getProperty(hash, name, checkValid); 1454 } 1455 1456 } 1457 1458 @Override 1459 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1460 switch (hash) { 1461 case 1478300413: // severity 1462 value = new IssueSeverityEnumFactory().fromType(castToCode(value)); 1463 this.severity = (Enumeration) value; // Enumeration<IssueSeverity> 1464 return value; 1465 case 3059181: // code 1466 value = new IssueTypeEnumFactory().fromType(castToCode(value)); 1467 this.code = (Enumeration) value; // Enumeration<IssueType> 1468 return value; 1469 case 1557721666: // details 1470 this.details = castToCodeableConcept(value); // CodeableConcept 1471 return value; 1472 case -740386388: // diagnostics 1473 this.diagnostics = castToString(value); // StringType 1474 return value; 1475 case 1901043637: // location 1476 this.getLocation().add(castToString(value)); // StringType 1477 return value; 1478 case -1795452264: // expression 1479 this.getExpression().add(castToString(value)); // StringType 1480 return value; 1481 default: 1482 return super.setProperty(hash, name, value); 1483 } 1484 1485 } 1486 1487 @Override 1488 public Base setProperty(String name, Base value) throws FHIRException { 1489 if (name.equals("severity")) { 1490 value = new IssueSeverityEnumFactory().fromType(castToCode(value)); 1491 this.severity = (Enumeration) value; // Enumeration<IssueSeverity> 1492 } else if (name.equals("code")) { 1493 value = new IssueTypeEnumFactory().fromType(castToCode(value)); 1494 this.code = (Enumeration) value; // Enumeration<IssueType> 1495 } else if (name.equals("details")) { 1496 this.details = castToCodeableConcept(value); // CodeableConcept 1497 } else if (name.equals("diagnostics")) { 1498 this.diagnostics = castToString(value); // StringType 1499 } else if (name.equals("location")) { 1500 this.getLocation().add(castToString(value)); 1501 } else if (name.equals("expression")) { 1502 this.getExpression().add(castToString(value)); 1503 } else 1504 return super.setProperty(name, value); 1505 return value; 1506 } 1507 1508 @Override 1509 public void removeChild(String name, Base value) throws FHIRException { 1510 if (name.equals("severity")) { 1511 this.severity = null; 1512 } else if (name.equals("code")) { 1513 this.code = null; 1514 } else if (name.equals("details")) { 1515 this.details = null; 1516 } else if (name.equals("diagnostics")) { 1517 this.diagnostics = null; 1518 } else if (name.equals("location")) { 1519 this.getLocation().remove(castToString(value)); 1520 } else if (name.equals("expression")) { 1521 this.getExpression().remove(castToString(value)); 1522 } else 1523 super.removeChild(name, value); 1524 1525 } 1526 1527 @Override 1528 public Base makeProperty(int hash, String name) throws FHIRException { 1529 switch (hash) { 1530 case 1478300413: 1531 return getSeverityElement(); 1532 case 3059181: 1533 return getCodeElement(); 1534 case 1557721666: 1535 return getDetails(); 1536 case -740386388: 1537 return getDiagnosticsElement(); 1538 case 1901043637: 1539 return addLocationElement(); 1540 case -1795452264: 1541 return addExpressionElement(); 1542 default: 1543 return super.makeProperty(hash, name); 1544 } 1545 1546 } 1547 1548 @Override 1549 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1550 switch (hash) { 1551 case 1478300413: 1552 /* severity */ return new String[] { "code" }; 1553 case 3059181: 1554 /* code */ return new String[] { "code" }; 1555 case 1557721666: 1556 /* details */ return new String[] { "CodeableConcept" }; 1557 case -740386388: 1558 /* diagnostics */ return new String[] { "string" }; 1559 case 1901043637: 1560 /* location */ return new String[] { "string" }; 1561 case -1795452264: 1562 /* expression */ return new String[] { "string" }; 1563 default: 1564 return super.getTypesForProperty(hash, name); 1565 } 1566 1567 } 1568 1569 @Override 1570 public Base addChild(String name) throws FHIRException { 1571 if (name.equals("severity")) { 1572 throw new FHIRException("Cannot call addChild on a singleton property OperationOutcome.severity"); 1573 } else if (name.equals("code")) { 1574 throw new FHIRException("Cannot call addChild on a singleton property OperationOutcome.code"); 1575 } else if (name.equals("details")) { 1576 this.details = new CodeableConcept(); 1577 return this.details; 1578 } else if (name.equals("diagnostics")) { 1579 throw new FHIRException("Cannot call addChild on a singleton property OperationOutcome.diagnostics"); 1580 } else if (name.equals("location")) { 1581 throw new FHIRException("Cannot call addChild on a singleton property OperationOutcome.location"); 1582 } else if (name.equals("expression")) { 1583 throw new FHIRException("Cannot call addChild on a singleton property OperationOutcome.expression"); 1584 } else 1585 return super.addChild(name); 1586 } 1587 1588 public OperationOutcomeIssueComponent copy() { 1589 OperationOutcomeIssueComponent dst = new OperationOutcomeIssueComponent(); 1590 copyValues(dst); 1591 return dst; 1592 } 1593 1594 public void copyValues(OperationOutcomeIssueComponent dst) { 1595 super.copyValues(dst); 1596 dst.severity = severity == null ? null : severity.copy(); 1597 dst.code = code == null ? null : code.copy(); 1598 dst.details = details == null ? null : details.copy(); 1599 dst.diagnostics = diagnostics == null ? null : diagnostics.copy(); 1600 if (location != null) { 1601 dst.location = new ArrayList<StringType>(); 1602 for (StringType i : location) 1603 dst.location.add(i.copy()); 1604 } 1605 ; 1606 if (expression != null) { 1607 dst.expression = new ArrayList<StringType>(); 1608 for (StringType i : expression) 1609 dst.expression.add(i.copy()); 1610 } 1611 ; 1612 } 1613 1614 @Override 1615 public boolean equalsDeep(Base other_) { 1616 if (!super.equalsDeep(other_)) 1617 return false; 1618 if (!(other_ instanceof OperationOutcomeIssueComponent)) 1619 return false; 1620 OperationOutcomeIssueComponent o = (OperationOutcomeIssueComponent) other_; 1621 return compareDeep(severity, o.severity, true) && compareDeep(code, o.code, true) 1622 && compareDeep(details, o.details, true) && compareDeep(diagnostics, o.diagnostics, true) 1623 && compareDeep(location, o.location, true) && compareDeep(expression, o.expression, true); 1624 } 1625 1626 @Override 1627 public boolean equalsShallow(Base other_) { 1628 if (!super.equalsShallow(other_)) 1629 return false; 1630 if (!(other_ instanceof OperationOutcomeIssueComponent)) 1631 return false; 1632 OperationOutcomeIssueComponent o = (OperationOutcomeIssueComponent) other_; 1633 return compareValues(severity, o.severity, true) && compareValues(code, o.code, true) 1634 && compareValues(diagnostics, o.diagnostics, true) && compareValues(location, o.location, true) 1635 && compareValues(expression, o.expression, true); 1636 } 1637 1638 public boolean isEmpty() { 1639 return super.isEmpty() 1640 && ca.uhn.fhir.util.ElementUtil.isEmpty(severity, code, details, diagnostics, location, expression); 1641 } 1642 1643 public String fhirType() { 1644 return "OperationOutcome.issue"; 1645 1646 } 1647 1648 } 1649 1650 /** 1651 * An error, warning, or information message that results from a system action. 1652 */ 1653 @Child(name = "issue", type = {}, order = 0, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1654 @Description(shortDefinition = "A single issue associated with the action", formalDefinition = "An error, warning, or information message that results from a system action.") 1655 protected List<OperationOutcomeIssueComponent> issue; 1656 1657 private static final long serialVersionUID = -152150052L; 1658 1659 /** 1660 * Constructor 1661 */ 1662 public OperationOutcome() { 1663 super(); 1664 } 1665 1666 /** 1667 * @return {@link #issue} (An error, warning, or information message that 1668 * results from a system action.) 1669 */ 1670 public List<OperationOutcomeIssueComponent> getIssue() { 1671 if (this.issue == null) 1672 this.issue = new ArrayList<OperationOutcomeIssueComponent>(); 1673 return this.issue; 1674 } 1675 1676 /** 1677 * @return Returns a reference to <code>this</code> for easy method chaining 1678 */ 1679 public OperationOutcome setIssue(List<OperationOutcomeIssueComponent> theIssue) { 1680 this.issue = theIssue; 1681 return this; 1682 } 1683 1684 public boolean hasIssue() { 1685 if (this.issue == null) 1686 return false; 1687 for (OperationOutcomeIssueComponent item : this.issue) 1688 if (!item.isEmpty()) 1689 return true; 1690 return false; 1691 } 1692 1693 public OperationOutcomeIssueComponent addIssue() { // 3 1694 OperationOutcomeIssueComponent t = new OperationOutcomeIssueComponent(); 1695 if (this.issue == null) 1696 this.issue = new ArrayList<OperationOutcomeIssueComponent>(); 1697 this.issue.add(t); 1698 return t; 1699 } 1700 1701 public OperationOutcome addIssue(OperationOutcomeIssueComponent t) { // 3 1702 if (t == null) 1703 return this; 1704 if (this.issue == null) 1705 this.issue = new ArrayList<OperationOutcomeIssueComponent>(); 1706 this.issue.add(t); 1707 return this; 1708 } 1709 1710 /** 1711 * @return The first repetition of repeating field {@link #issue}, creating it 1712 * if it does not already exist 1713 */ 1714 public OperationOutcomeIssueComponent getIssueFirstRep() { 1715 if (getIssue().isEmpty()) { 1716 addIssue(); 1717 } 1718 return getIssue().get(0); 1719 } 1720 1721 protected void listChildren(List<Property> children) { 1722 super.listChildren(children); 1723 children 1724 .add(new Property("issue", "", "An error, warning, or information message that results from a system action.", 1725 0, java.lang.Integer.MAX_VALUE, issue)); 1726 } 1727 1728 @Override 1729 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1730 switch (_hash) { 1731 case 100509913: 1732 /* issue */ return new Property("issue", "", 1733 "An error, warning, or information message that results from a system action.", 0, 1734 java.lang.Integer.MAX_VALUE, issue); 1735 default: 1736 return super.getNamedProperty(_hash, _name, _checkValid); 1737 } 1738 1739 } 1740 1741 @Override 1742 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1743 switch (hash) { 1744 case 100509913: 1745 /* issue */ return this.issue == null ? new Base[0] : this.issue.toArray(new Base[this.issue.size()]); // OperationOutcomeIssueComponent 1746 default: 1747 return super.getProperty(hash, name, checkValid); 1748 } 1749 1750 } 1751 1752 @Override 1753 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1754 switch (hash) { 1755 case 100509913: // issue 1756 this.getIssue().add((OperationOutcomeIssueComponent) value); // OperationOutcomeIssueComponent 1757 return value; 1758 default: 1759 return super.setProperty(hash, name, value); 1760 } 1761 1762 } 1763 1764 @Override 1765 public Base setProperty(String name, Base value) throws FHIRException { 1766 if (name.equals("issue")) { 1767 this.getIssue().add((OperationOutcomeIssueComponent) value); 1768 } else 1769 return super.setProperty(name, value); 1770 return value; 1771 } 1772 1773 @Override 1774 public void removeChild(String name, Base value) throws FHIRException { 1775 if (name.equals("issue")) { 1776 this.getIssue().remove((OperationOutcomeIssueComponent) value); 1777 } else 1778 super.removeChild(name, value); 1779 1780 } 1781 1782 @Override 1783 public Base makeProperty(int hash, String name) throws FHIRException { 1784 switch (hash) { 1785 case 100509913: 1786 return addIssue(); 1787 default: 1788 return super.makeProperty(hash, name); 1789 } 1790 1791 } 1792 1793 @Override 1794 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1795 switch (hash) { 1796 case 100509913: 1797 /* issue */ return new String[] {}; 1798 default: 1799 return super.getTypesForProperty(hash, name); 1800 } 1801 1802 } 1803 1804 @Override 1805 public Base addChild(String name) throws FHIRException { 1806 if (name.equals("issue")) { 1807 return addIssue(); 1808 } else 1809 return super.addChild(name); 1810 } 1811 1812 public String fhirType() { 1813 return "OperationOutcome"; 1814 1815 } 1816 1817 public OperationOutcome copy() { 1818 OperationOutcome dst = new OperationOutcome(); 1819 copyValues(dst); 1820 return dst; 1821 } 1822 1823 public void copyValues(OperationOutcome dst) { 1824 super.copyValues(dst); 1825 if (issue != null) { 1826 dst.issue = new ArrayList<OperationOutcomeIssueComponent>(); 1827 for (OperationOutcomeIssueComponent i : issue) 1828 dst.issue.add(i.copy()); 1829 } 1830 ; 1831 } 1832 1833 protected OperationOutcome typedCopy() { 1834 return copy(); 1835 } 1836 1837 @Override 1838 public boolean equalsDeep(Base other_) { 1839 if (!super.equalsDeep(other_)) 1840 return false; 1841 if (!(other_ instanceof OperationOutcome)) 1842 return false; 1843 OperationOutcome o = (OperationOutcome) other_; 1844 return compareDeep(issue, o.issue, true); 1845 } 1846 1847 @Override 1848 public boolean equalsShallow(Base other_) { 1849 if (!super.equalsShallow(other_)) 1850 return false; 1851 if (!(other_ instanceof OperationOutcome)) 1852 return false; 1853 OperationOutcome o = (OperationOutcome) other_; 1854 return true; 1855 } 1856 1857 public boolean isEmpty() { 1858 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(issue); 1859 } 1860 1861 @Override 1862 public ResourceType getResourceType() { 1863 return ResourceType.OperationOutcome; 1864 } 1865 1866}