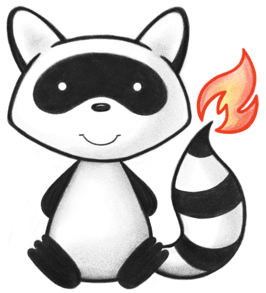
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * A formally or informally recognized grouping of people or organizations 048 * formed for the purpose of achieving some form of collective action. Includes 049 * companies, institutions, corporations, departments, community groups, 050 * healthcare practice groups, payer/insurer, etc. 051 */ 052@ResourceDef(name = "Organization", profile = "http://hl7.org/fhir/StructureDefinition/Organization") 053public class Organization extends DomainResource { 054 055 @Block() 056 public static class OrganizationContactComponent extends BackboneElement implements IBaseBackboneElement { 057 /** 058 * Indicates a purpose for which the contact can be reached. 059 */ 060 @Child(name = "purpose", type = { 061 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 062 @Description(shortDefinition = "The type of contact", formalDefinition = "Indicates a purpose for which the contact can be reached.") 063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contactentity-type") 064 protected CodeableConcept purpose; 065 066 /** 067 * A name associated with the contact. 068 */ 069 @Child(name = "name", type = { HumanName.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 070 @Description(shortDefinition = "A name associated with the contact", formalDefinition = "A name associated with the contact.") 071 protected HumanName name; 072 073 /** 074 * A contact detail (e.g. a telephone number or an email address) by which the 075 * party may be contacted. 076 */ 077 @Child(name = "telecom", type = { 078 ContactPoint.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 079 @Description(shortDefinition = "Contact details (telephone, email, etc.) for a contact", formalDefinition = "A contact detail (e.g. a telephone number or an email address) by which the party may be contacted.") 080 protected List<ContactPoint> telecom; 081 082 /** 083 * Visiting or postal addresses for the contact. 084 */ 085 @Child(name = "address", type = { Address.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 086 @Description(shortDefinition = "Visiting or postal addresses for the contact", formalDefinition = "Visiting or postal addresses for the contact.") 087 protected Address address; 088 089 private static final long serialVersionUID = 1831121305L; 090 091 /** 092 * Constructor 093 */ 094 public OrganizationContactComponent() { 095 super(); 096 } 097 098 /** 099 * @return {@link #purpose} (Indicates a purpose for which the contact can be 100 * reached.) 101 */ 102 public CodeableConcept getPurpose() { 103 if (this.purpose == null) 104 if (Configuration.errorOnAutoCreate()) 105 throw new Error("Attempt to auto-create OrganizationContactComponent.purpose"); 106 else if (Configuration.doAutoCreate()) 107 this.purpose = new CodeableConcept(); // cc 108 return this.purpose; 109 } 110 111 public boolean hasPurpose() { 112 return this.purpose != null && !this.purpose.isEmpty(); 113 } 114 115 /** 116 * @param value {@link #purpose} (Indicates a purpose for which the contact can 117 * be reached.) 118 */ 119 public OrganizationContactComponent setPurpose(CodeableConcept value) { 120 this.purpose = value; 121 return this; 122 } 123 124 /** 125 * @return {@link #name} (A name associated with the contact.) 126 */ 127 public HumanName getName() { 128 if (this.name == null) 129 if (Configuration.errorOnAutoCreate()) 130 throw new Error("Attempt to auto-create OrganizationContactComponent.name"); 131 else if (Configuration.doAutoCreate()) 132 this.name = new HumanName(); // cc 133 return this.name; 134 } 135 136 public boolean hasName() { 137 return this.name != null && !this.name.isEmpty(); 138 } 139 140 /** 141 * @param value {@link #name} (A name associated with the contact.) 142 */ 143 public OrganizationContactComponent setName(HumanName value) { 144 this.name = value; 145 return this; 146 } 147 148 /** 149 * @return {@link #telecom} (A contact detail (e.g. a telephone number or an 150 * email address) by which the party may be contacted.) 151 */ 152 public List<ContactPoint> getTelecom() { 153 if (this.telecom == null) 154 this.telecom = new ArrayList<ContactPoint>(); 155 return this.telecom; 156 } 157 158 /** 159 * @return Returns a reference to <code>this</code> for easy method chaining 160 */ 161 public OrganizationContactComponent setTelecom(List<ContactPoint> theTelecom) { 162 this.telecom = theTelecom; 163 return this; 164 } 165 166 public boolean hasTelecom() { 167 if (this.telecom == null) 168 return false; 169 for (ContactPoint item : this.telecom) 170 if (!item.isEmpty()) 171 return true; 172 return false; 173 } 174 175 public ContactPoint addTelecom() { // 3 176 ContactPoint t = new ContactPoint(); 177 if (this.telecom == null) 178 this.telecom = new ArrayList<ContactPoint>(); 179 this.telecom.add(t); 180 return t; 181 } 182 183 public OrganizationContactComponent addTelecom(ContactPoint t) { // 3 184 if (t == null) 185 return this; 186 if (this.telecom == null) 187 this.telecom = new ArrayList<ContactPoint>(); 188 this.telecom.add(t); 189 return this; 190 } 191 192 /** 193 * @return The first repetition of repeating field {@link #telecom}, creating it 194 * if it does not already exist 195 */ 196 public ContactPoint getTelecomFirstRep() { 197 if (getTelecom().isEmpty()) { 198 addTelecom(); 199 } 200 return getTelecom().get(0); 201 } 202 203 /** 204 * @return {@link #address} (Visiting or postal addresses for the contact.) 205 */ 206 public Address getAddress() { 207 if (this.address == null) 208 if (Configuration.errorOnAutoCreate()) 209 throw new Error("Attempt to auto-create OrganizationContactComponent.address"); 210 else if (Configuration.doAutoCreate()) 211 this.address = new Address(); // cc 212 return this.address; 213 } 214 215 public boolean hasAddress() { 216 return this.address != null && !this.address.isEmpty(); 217 } 218 219 /** 220 * @param value {@link #address} (Visiting or postal addresses for the contact.) 221 */ 222 public OrganizationContactComponent setAddress(Address value) { 223 this.address = value; 224 return this; 225 } 226 227 protected void listChildren(List<Property> children) { 228 super.listChildren(children); 229 children.add(new Property("purpose", "CodeableConcept", 230 "Indicates a purpose for which the contact can be reached.", 0, 1, purpose)); 231 children.add(new Property("name", "HumanName", "A name associated with the contact.", 0, 1, name)); 232 children.add(new Property("telecom", "ContactPoint", 233 "A contact detail (e.g. a telephone number or an email address) by which the party may be contacted.", 0, 234 java.lang.Integer.MAX_VALUE, telecom)); 235 children.add(new Property("address", "Address", "Visiting or postal addresses for the contact.", 0, 1, address)); 236 } 237 238 @Override 239 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 240 switch (_hash) { 241 case -220463842: 242 /* purpose */ return new Property("purpose", "CodeableConcept", 243 "Indicates a purpose for which the contact can be reached.", 0, 1, purpose); 244 case 3373707: 245 /* name */ return new Property("name", "HumanName", "A name associated with the contact.", 0, 1, name); 246 case -1429363305: 247 /* telecom */ return new Property("telecom", "ContactPoint", 248 "A contact detail (e.g. a telephone number or an email address) by which the party may be contacted.", 0, 249 java.lang.Integer.MAX_VALUE, telecom); 250 case -1147692044: 251 /* address */ return new Property("address", "Address", "Visiting or postal addresses for the contact.", 0, 1, 252 address); 253 default: 254 return super.getNamedProperty(_hash, _name, _checkValid); 255 } 256 257 } 258 259 @Override 260 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 261 switch (hash) { 262 case -220463842: 263 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // CodeableConcept 264 case 3373707: 265 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // HumanName 266 case -1429363305: 267 /* telecom */ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 268 case -1147692044: 269 /* address */ return this.address == null ? new Base[0] : new Base[] { this.address }; // Address 270 default: 271 return super.getProperty(hash, name, checkValid); 272 } 273 274 } 275 276 @Override 277 public Base setProperty(int hash, String name, Base value) throws FHIRException { 278 switch (hash) { 279 case -220463842: // purpose 280 this.purpose = castToCodeableConcept(value); // CodeableConcept 281 return value; 282 case 3373707: // name 283 this.name = castToHumanName(value); // HumanName 284 return value; 285 case -1429363305: // telecom 286 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 287 return value; 288 case -1147692044: // address 289 this.address = castToAddress(value); // Address 290 return value; 291 default: 292 return super.setProperty(hash, name, value); 293 } 294 295 } 296 297 @Override 298 public Base setProperty(String name, Base value) throws FHIRException { 299 if (name.equals("purpose")) { 300 this.purpose = castToCodeableConcept(value); // CodeableConcept 301 } else if (name.equals("name")) { 302 this.name = castToHumanName(value); // HumanName 303 } else if (name.equals("telecom")) { 304 this.getTelecom().add(castToContactPoint(value)); 305 } else if (name.equals("address")) { 306 this.address = castToAddress(value); // Address 307 } else 308 return super.setProperty(name, value); 309 return value; 310 } 311 312 @Override 313 public void removeChild(String name, Base value) throws FHIRException { 314 if (name.equals("purpose")) { 315 this.purpose = null; 316 } else if (name.equals("name")) { 317 this.name = null; 318 } else if (name.equals("telecom")) { 319 this.getTelecom().remove(castToContactPoint(value)); 320 } else if (name.equals("address")) { 321 this.address = null; 322 } else 323 super.removeChild(name, value); 324 325 } 326 327 @Override 328 public Base makeProperty(int hash, String name) throws FHIRException { 329 switch (hash) { 330 case -220463842: 331 return getPurpose(); 332 case 3373707: 333 return getName(); 334 case -1429363305: 335 return addTelecom(); 336 case -1147692044: 337 return getAddress(); 338 default: 339 return super.makeProperty(hash, name); 340 } 341 342 } 343 344 @Override 345 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 346 switch (hash) { 347 case -220463842: 348 /* purpose */ return new String[] { "CodeableConcept" }; 349 case 3373707: 350 /* name */ return new String[] { "HumanName" }; 351 case -1429363305: 352 /* telecom */ return new String[] { "ContactPoint" }; 353 case -1147692044: 354 /* address */ return new String[] { "Address" }; 355 default: 356 return super.getTypesForProperty(hash, name); 357 } 358 359 } 360 361 @Override 362 public Base addChild(String name) throws FHIRException { 363 if (name.equals("purpose")) { 364 this.purpose = new CodeableConcept(); 365 return this.purpose; 366 } else if (name.equals("name")) { 367 this.name = new HumanName(); 368 return this.name; 369 } else if (name.equals("telecom")) { 370 return addTelecom(); 371 } else if (name.equals("address")) { 372 this.address = new Address(); 373 return this.address; 374 } else 375 return super.addChild(name); 376 } 377 378 public OrganizationContactComponent copy() { 379 OrganizationContactComponent dst = new OrganizationContactComponent(); 380 copyValues(dst); 381 return dst; 382 } 383 384 public void copyValues(OrganizationContactComponent dst) { 385 super.copyValues(dst); 386 dst.purpose = purpose == null ? null : purpose.copy(); 387 dst.name = name == null ? null : name.copy(); 388 if (telecom != null) { 389 dst.telecom = new ArrayList<ContactPoint>(); 390 for (ContactPoint i : telecom) 391 dst.telecom.add(i.copy()); 392 } 393 ; 394 dst.address = address == null ? null : address.copy(); 395 } 396 397 @Override 398 public boolean equalsDeep(Base other_) { 399 if (!super.equalsDeep(other_)) 400 return false; 401 if (!(other_ instanceof OrganizationContactComponent)) 402 return false; 403 OrganizationContactComponent o = (OrganizationContactComponent) other_; 404 return compareDeep(purpose, o.purpose, true) && compareDeep(name, o.name, true) 405 && compareDeep(telecom, o.telecom, true) && compareDeep(address, o.address, true); 406 } 407 408 @Override 409 public boolean equalsShallow(Base other_) { 410 if (!super.equalsShallow(other_)) 411 return false; 412 if (!(other_ instanceof OrganizationContactComponent)) 413 return false; 414 OrganizationContactComponent o = (OrganizationContactComponent) other_; 415 return true; 416 } 417 418 public boolean isEmpty() { 419 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(purpose, name, telecom, address); 420 } 421 422 public String fhirType() { 423 return "Organization.contact"; 424 425 } 426 427 } 428 429 /** 430 * Identifier for the organization that is used to identify the organization 431 * across multiple disparate systems. 432 */ 433 @Child(name = "identifier", type = { 434 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 435 @Description(shortDefinition = "Identifies this organization across multiple systems", formalDefinition = "Identifier for the organization that is used to identify the organization across multiple disparate systems.") 436 protected List<Identifier> identifier; 437 438 /** 439 * Whether the organization's record is still in active use. 440 */ 441 @Child(name = "active", type = { BooleanType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 442 @Description(shortDefinition = "Whether the organization's record is still in active use", formalDefinition = "Whether the organization's record is still in active use.") 443 protected BooleanType active; 444 445 /** 446 * The kind(s) of organization that this is. 447 */ 448 @Child(name = "type", type = { 449 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 450 @Description(shortDefinition = "Kind of organization", formalDefinition = "The kind(s) of organization that this is.") 451 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/organization-type") 452 protected List<CodeableConcept> type; 453 454 /** 455 * A name associated with the organization. 456 */ 457 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 458 @Description(shortDefinition = "Name used for the organization", formalDefinition = "A name associated with the organization.") 459 protected StringType name; 460 461 /** 462 * A list of alternate names that the organization is known as, or was known as 463 * in the past. 464 */ 465 @Child(name = "alias", type = { 466 StringType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 467 @Description(shortDefinition = "A list of alternate names that the organization is known as, or was known as in the past", formalDefinition = "A list of alternate names that the organization is known as, or was known as in the past.") 468 protected List<StringType> alias; 469 470 /** 471 * A contact detail for the organization. 472 */ 473 @Child(name = "telecom", type = { 474 ContactPoint.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 475 @Description(shortDefinition = "A contact detail for the organization", formalDefinition = "A contact detail for the organization.") 476 protected List<ContactPoint> telecom; 477 478 /** 479 * An address for the organization. 480 */ 481 @Child(name = "address", type = { 482 Address.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 483 @Description(shortDefinition = "An address for the organization", formalDefinition = "An address for the organization.") 484 protected List<Address> address; 485 486 /** 487 * The organization of which this organization forms a part. 488 */ 489 @Child(name = "partOf", type = { Organization.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 490 @Description(shortDefinition = "The organization of which this organization forms a part", formalDefinition = "The organization of which this organization forms a part.") 491 protected Reference partOf; 492 493 /** 494 * The actual object that is the target of the reference (The organization of 495 * which this organization forms a part.) 496 */ 497 protected Organization partOfTarget; 498 499 /** 500 * Contact for the organization for a certain purpose. 501 */ 502 @Child(name = "contact", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 503 @Description(shortDefinition = "Contact for the organization for a certain purpose", formalDefinition = "Contact for the organization for a certain purpose.") 504 protected List<OrganizationContactComponent> contact; 505 506 /** 507 * Technical endpoints providing access to services operated for the 508 * organization. 509 */ 510 @Child(name = "endpoint", type = { 511 Endpoint.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 512 @Description(shortDefinition = "Technical endpoints providing access to services operated for the organization", formalDefinition = "Technical endpoints providing access to services operated for the organization.") 513 protected List<Reference> endpoint; 514 /** 515 * The actual objects that are the target of the reference (Technical endpoints 516 * providing access to services operated for the organization.) 517 */ 518 protected List<Endpoint> endpointTarget; 519 520 private static final long serialVersionUID = -2113244111L; 521 522 /** 523 * Constructor 524 */ 525 public Organization() { 526 super(); 527 } 528 529 /** 530 * @return {@link #identifier} (Identifier for the organization that is used to 531 * identify the organization across multiple disparate systems.) 532 */ 533 public List<Identifier> getIdentifier() { 534 if (this.identifier == null) 535 this.identifier = new ArrayList<Identifier>(); 536 return this.identifier; 537 } 538 539 /** 540 * @return Returns a reference to <code>this</code> for easy method chaining 541 */ 542 public Organization setIdentifier(List<Identifier> theIdentifier) { 543 this.identifier = theIdentifier; 544 return this; 545 } 546 547 public boolean hasIdentifier() { 548 if (this.identifier == null) 549 return false; 550 for (Identifier item : this.identifier) 551 if (!item.isEmpty()) 552 return true; 553 return false; 554 } 555 556 public Identifier addIdentifier() { // 3 557 Identifier t = new Identifier(); 558 if (this.identifier == null) 559 this.identifier = new ArrayList<Identifier>(); 560 this.identifier.add(t); 561 return t; 562 } 563 564 public Organization addIdentifier(Identifier t) { // 3 565 if (t == null) 566 return this; 567 if (this.identifier == null) 568 this.identifier = new ArrayList<Identifier>(); 569 this.identifier.add(t); 570 return this; 571 } 572 573 /** 574 * @return The first repetition of repeating field {@link #identifier}, creating 575 * it if it does not already exist 576 */ 577 public Identifier getIdentifierFirstRep() { 578 if (getIdentifier().isEmpty()) { 579 addIdentifier(); 580 } 581 return getIdentifier().get(0); 582 } 583 584 /** 585 * @return {@link #active} (Whether the organization's record is still in active 586 * use.). This is the underlying object with id, value and extensions. 587 * The accessor "getActive" gives direct access to the value 588 */ 589 public BooleanType getActiveElement() { 590 if (this.active == null) 591 if (Configuration.errorOnAutoCreate()) 592 throw new Error("Attempt to auto-create Organization.active"); 593 else if (Configuration.doAutoCreate()) 594 this.active = new BooleanType(); // bb 595 return this.active; 596 } 597 598 public boolean hasActiveElement() { 599 return this.active != null && !this.active.isEmpty(); 600 } 601 602 public boolean hasActive() { 603 return this.active != null && !this.active.isEmpty(); 604 } 605 606 /** 607 * @param value {@link #active} (Whether the organization's record is still in 608 * active use.). This is the underlying object with id, value and 609 * extensions. The accessor "getActive" gives direct access to the 610 * value 611 */ 612 public Organization setActiveElement(BooleanType value) { 613 this.active = value; 614 return this; 615 } 616 617 /** 618 * @return Whether the organization's record is still in active use. 619 */ 620 public boolean getActive() { 621 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 622 } 623 624 /** 625 * @param value Whether the organization's record is still in active use. 626 */ 627 public Organization setActive(boolean value) { 628 if (this.active == null) 629 this.active = new BooleanType(); 630 this.active.setValue(value); 631 return this; 632 } 633 634 /** 635 * @return {@link #type} (The kind(s) of organization that this is.) 636 */ 637 public List<CodeableConcept> getType() { 638 if (this.type == null) 639 this.type = new ArrayList<CodeableConcept>(); 640 return this.type; 641 } 642 643 /** 644 * @return Returns a reference to <code>this</code> for easy method chaining 645 */ 646 public Organization setType(List<CodeableConcept> theType) { 647 this.type = theType; 648 return this; 649 } 650 651 public boolean hasType() { 652 if (this.type == null) 653 return false; 654 for (CodeableConcept item : this.type) 655 if (!item.isEmpty()) 656 return true; 657 return false; 658 } 659 660 public CodeableConcept addType() { // 3 661 CodeableConcept t = new CodeableConcept(); 662 if (this.type == null) 663 this.type = new ArrayList<CodeableConcept>(); 664 this.type.add(t); 665 return t; 666 } 667 668 public Organization addType(CodeableConcept t) { // 3 669 if (t == null) 670 return this; 671 if (this.type == null) 672 this.type = new ArrayList<CodeableConcept>(); 673 this.type.add(t); 674 return this; 675 } 676 677 /** 678 * @return The first repetition of repeating field {@link #type}, creating it if 679 * it does not already exist 680 */ 681 public CodeableConcept getTypeFirstRep() { 682 if (getType().isEmpty()) { 683 addType(); 684 } 685 return getType().get(0); 686 } 687 688 /** 689 * @return {@link #name} (A name associated with the organization.). This is the 690 * underlying object with id, value and extensions. The accessor 691 * "getName" gives direct access to the value 692 */ 693 public StringType getNameElement() { 694 if (this.name == null) 695 if (Configuration.errorOnAutoCreate()) 696 throw new Error("Attempt to auto-create Organization.name"); 697 else if (Configuration.doAutoCreate()) 698 this.name = new StringType(); // bb 699 return this.name; 700 } 701 702 public boolean hasNameElement() { 703 return this.name != null && !this.name.isEmpty(); 704 } 705 706 public boolean hasName() { 707 return this.name != null && !this.name.isEmpty(); 708 } 709 710 /** 711 * @param value {@link #name} (A name associated with the organization.). This 712 * is the underlying object with id, value and extensions. The 713 * accessor "getName" gives direct access to the value 714 */ 715 public Organization setNameElement(StringType value) { 716 this.name = value; 717 return this; 718 } 719 720 /** 721 * @return A name associated with the organization. 722 */ 723 public String getName() { 724 return this.name == null ? null : this.name.getValue(); 725 } 726 727 /** 728 * @param value A name associated with the organization. 729 */ 730 public Organization setName(String value) { 731 if (Utilities.noString(value)) 732 this.name = null; 733 else { 734 if (this.name == null) 735 this.name = new StringType(); 736 this.name.setValue(value); 737 } 738 return this; 739 } 740 741 /** 742 * @return {@link #alias} (A list of alternate names that the organization is 743 * known as, or was known as in the past.) 744 */ 745 public List<StringType> getAlias() { 746 if (this.alias == null) 747 this.alias = new ArrayList<StringType>(); 748 return this.alias; 749 } 750 751 /** 752 * @return Returns a reference to <code>this</code> for easy method chaining 753 */ 754 public Organization setAlias(List<StringType> theAlias) { 755 this.alias = theAlias; 756 return this; 757 } 758 759 public boolean hasAlias() { 760 if (this.alias == null) 761 return false; 762 for (StringType item : this.alias) 763 if (!item.isEmpty()) 764 return true; 765 return false; 766 } 767 768 /** 769 * @return {@link #alias} (A list of alternate names that the organization is 770 * known as, or was known as in the past.) 771 */ 772 public StringType addAliasElement() {// 2 773 StringType t = new StringType(); 774 if (this.alias == null) 775 this.alias = new ArrayList<StringType>(); 776 this.alias.add(t); 777 return t; 778 } 779 780 /** 781 * @param value {@link #alias} (A list of alternate names that the organization 782 * is known as, or was known as in the past.) 783 */ 784 public Organization addAlias(String value) { // 1 785 StringType t = new StringType(); 786 t.setValue(value); 787 if (this.alias == null) 788 this.alias = new ArrayList<StringType>(); 789 this.alias.add(t); 790 return this; 791 } 792 793 /** 794 * @param value {@link #alias} (A list of alternate names that the organization 795 * is known as, or was known as in the past.) 796 */ 797 public boolean hasAlias(String value) { 798 if (this.alias == null) 799 return false; 800 for (StringType v : this.alias) 801 if (v.getValue().equals(value)) // string 802 return true; 803 return false; 804 } 805 806 /** 807 * @return {@link #telecom} (A contact detail for the organization.) 808 */ 809 public List<ContactPoint> getTelecom() { 810 if (this.telecom == null) 811 this.telecom = new ArrayList<ContactPoint>(); 812 return this.telecom; 813 } 814 815 /** 816 * @return Returns a reference to <code>this</code> for easy method chaining 817 */ 818 public Organization setTelecom(List<ContactPoint> theTelecom) { 819 this.telecom = theTelecom; 820 return this; 821 } 822 823 public boolean hasTelecom() { 824 if (this.telecom == null) 825 return false; 826 for (ContactPoint item : this.telecom) 827 if (!item.isEmpty()) 828 return true; 829 return false; 830 } 831 832 public ContactPoint addTelecom() { // 3 833 ContactPoint t = new ContactPoint(); 834 if (this.telecom == null) 835 this.telecom = new ArrayList<ContactPoint>(); 836 this.telecom.add(t); 837 return t; 838 } 839 840 public Organization addTelecom(ContactPoint t) { // 3 841 if (t == null) 842 return this; 843 if (this.telecom == null) 844 this.telecom = new ArrayList<ContactPoint>(); 845 this.telecom.add(t); 846 return this; 847 } 848 849 /** 850 * @return The first repetition of repeating field {@link #telecom}, creating it 851 * if it does not already exist 852 */ 853 public ContactPoint getTelecomFirstRep() { 854 if (getTelecom().isEmpty()) { 855 addTelecom(); 856 } 857 return getTelecom().get(0); 858 } 859 860 /** 861 * @return {@link #address} (An address for the organization.) 862 */ 863 public List<Address> getAddress() { 864 if (this.address == null) 865 this.address = new ArrayList<Address>(); 866 return this.address; 867 } 868 869 /** 870 * @return Returns a reference to <code>this</code> for easy method chaining 871 */ 872 public Organization setAddress(List<Address> theAddress) { 873 this.address = theAddress; 874 return this; 875 } 876 877 public boolean hasAddress() { 878 if (this.address == null) 879 return false; 880 for (Address item : this.address) 881 if (!item.isEmpty()) 882 return true; 883 return false; 884 } 885 886 public Address addAddress() { // 3 887 Address t = new Address(); 888 if (this.address == null) 889 this.address = new ArrayList<Address>(); 890 this.address.add(t); 891 return t; 892 } 893 894 public Organization addAddress(Address t) { // 3 895 if (t == null) 896 return this; 897 if (this.address == null) 898 this.address = new ArrayList<Address>(); 899 this.address.add(t); 900 return this; 901 } 902 903 /** 904 * @return The first repetition of repeating field {@link #address}, creating it 905 * if it does not already exist 906 */ 907 public Address getAddressFirstRep() { 908 if (getAddress().isEmpty()) { 909 addAddress(); 910 } 911 return getAddress().get(0); 912 } 913 914 /** 915 * @return {@link #partOf} (The organization of which this organization forms a 916 * part.) 917 */ 918 public Reference getPartOf() { 919 if (this.partOf == null) 920 if (Configuration.errorOnAutoCreate()) 921 throw new Error("Attempt to auto-create Organization.partOf"); 922 else if (Configuration.doAutoCreate()) 923 this.partOf = new Reference(); // cc 924 return this.partOf; 925 } 926 927 public boolean hasPartOf() { 928 return this.partOf != null && !this.partOf.isEmpty(); 929 } 930 931 /** 932 * @param value {@link #partOf} (The organization of which this organization 933 * forms a part.) 934 */ 935 public Organization setPartOf(Reference value) { 936 this.partOf = value; 937 return this; 938 } 939 940 /** 941 * @return {@link #partOf} The actual object that is the target of the 942 * reference. The reference library doesn't populate this, but you can 943 * use it to hold the resource if you resolve it. (The organization of 944 * which this organization forms a part.) 945 */ 946 public Organization getPartOfTarget() { 947 if (this.partOfTarget == null) 948 if (Configuration.errorOnAutoCreate()) 949 throw new Error("Attempt to auto-create Organization.partOf"); 950 else if (Configuration.doAutoCreate()) 951 this.partOfTarget = new Organization(); // aa 952 return this.partOfTarget; 953 } 954 955 /** 956 * @param value {@link #partOf} The actual object that is the target of the 957 * reference. The reference library doesn't use these, but you can 958 * use it to hold the resource if you resolve it. (The organization 959 * of which this organization forms a part.) 960 */ 961 public Organization setPartOfTarget(Organization value) { 962 this.partOfTarget = value; 963 return this; 964 } 965 966 /** 967 * @return {@link #contact} (Contact for the organization for a certain 968 * purpose.) 969 */ 970 public List<OrganizationContactComponent> getContact() { 971 if (this.contact == null) 972 this.contact = new ArrayList<OrganizationContactComponent>(); 973 return this.contact; 974 } 975 976 /** 977 * @return Returns a reference to <code>this</code> for easy method chaining 978 */ 979 public Organization setContact(List<OrganizationContactComponent> theContact) { 980 this.contact = theContact; 981 return this; 982 } 983 984 public boolean hasContact() { 985 if (this.contact == null) 986 return false; 987 for (OrganizationContactComponent item : this.contact) 988 if (!item.isEmpty()) 989 return true; 990 return false; 991 } 992 993 public OrganizationContactComponent addContact() { // 3 994 OrganizationContactComponent t = new OrganizationContactComponent(); 995 if (this.contact == null) 996 this.contact = new ArrayList<OrganizationContactComponent>(); 997 this.contact.add(t); 998 return t; 999 } 1000 1001 public Organization addContact(OrganizationContactComponent t) { // 3 1002 if (t == null) 1003 return this; 1004 if (this.contact == null) 1005 this.contact = new ArrayList<OrganizationContactComponent>(); 1006 this.contact.add(t); 1007 return this; 1008 } 1009 1010 /** 1011 * @return The first repetition of repeating field {@link #contact}, creating it 1012 * if it does not already exist 1013 */ 1014 public OrganizationContactComponent getContactFirstRep() { 1015 if (getContact().isEmpty()) { 1016 addContact(); 1017 } 1018 return getContact().get(0); 1019 } 1020 1021 /** 1022 * @return {@link #endpoint} (Technical endpoints providing access to services 1023 * operated for the organization.) 1024 */ 1025 public List<Reference> getEndpoint() { 1026 if (this.endpoint == null) 1027 this.endpoint = new ArrayList<Reference>(); 1028 return this.endpoint; 1029 } 1030 1031 /** 1032 * @return Returns a reference to <code>this</code> for easy method chaining 1033 */ 1034 public Organization setEndpoint(List<Reference> theEndpoint) { 1035 this.endpoint = theEndpoint; 1036 return this; 1037 } 1038 1039 public boolean hasEndpoint() { 1040 if (this.endpoint == null) 1041 return false; 1042 for (Reference item : this.endpoint) 1043 if (!item.isEmpty()) 1044 return true; 1045 return false; 1046 } 1047 1048 public Reference addEndpoint() { // 3 1049 Reference t = new Reference(); 1050 if (this.endpoint == null) 1051 this.endpoint = new ArrayList<Reference>(); 1052 this.endpoint.add(t); 1053 return t; 1054 } 1055 1056 public Organization addEndpoint(Reference t) { // 3 1057 if (t == null) 1058 return this; 1059 if (this.endpoint == null) 1060 this.endpoint = new ArrayList<Reference>(); 1061 this.endpoint.add(t); 1062 return this; 1063 } 1064 1065 /** 1066 * @return The first repetition of repeating field {@link #endpoint}, creating 1067 * it if it does not already exist 1068 */ 1069 public Reference getEndpointFirstRep() { 1070 if (getEndpoint().isEmpty()) { 1071 addEndpoint(); 1072 } 1073 return getEndpoint().get(0); 1074 } 1075 1076 protected void listChildren(List<Property> children) { 1077 super.listChildren(children); 1078 children.add(new Property("identifier", "Identifier", 1079 "Identifier for the organization that is used to identify the organization across multiple disparate systems.", 1080 0, java.lang.Integer.MAX_VALUE, identifier)); 1081 children.add( 1082 new Property("active", "boolean", "Whether the organization's record is still in active use.", 0, 1, active)); 1083 children.add(new Property("type", "CodeableConcept", "The kind(s) of organization that this is.", 0, 1084 java.lang.Integer.MAX_VALUE, type)); 1085 children.add(new Property("name", "string", "A name associated with the organization.", 0, 1, name)); 1086 children.add(new Property("alias", "string", 1087 "A list of alternate names that the organization is known as, or was known as in the past.", 0, 1088 java.lang.Integer.MAX_VALUE, alias)); 1089 children.add(new Property("telecom", "ContactPoint", "A contact detail for the organization.", 0, 1090 java.lang.Integer.MAX_VALUE, telecom)); 1091 children.add(new Property("address", "Address", "An address for the organization.", 0, java.lang.Integer.MAX_VALUE, 1092 address)); 1093 children.add(new Property("partOf", "Reference(Organization)", 1094 "The organization of which this organization forms a part.", 0, 1, partOf)); 1095 children.add(new Property("contact", "", "Contact for the organization for a certain purpose.", 0, 1096 java.lang.Integer.MAX_VALUE, contact)); 1097 children.add(new Property("endpoint", "Reference(Endpoint)", 1098 "Technical endpoints providing access to services operated for the organization.", 0, 1099 java.lang.Integer.MAX_VALUE, endpoint)); 1100 } 1101 1102 @Override 1103 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1104 switch (_hash) { 1105 case -1618432855: 1106 /* identifier */ return new Property("identifier", "Identifier", 1107 "Identifier for the organization that is used to identify the organization across multiple disparate systems.", 1108 0, java.lang.Integer.MAX_VALUE, identifier); 1109 case -1422950650: 1110 /* active */ return new Property("active", "boolean", "Whether the organization's record is still in active use.", 1111 0, 1, active); 1112 case 3575610: 1113 /* type */ return new Property("type", "CodeableConcept", "The kind(s) of organization that this is.", 0, 1114 java.lang.Integer.MAX_VALUE, type); 1115 case 3373707: 1116 /* name */ return new Property("name", "string", "A name associated with the organization.", 0, 1, name); 1117 case 92902992: 1118 /* alias */ return new Property("alias", "string", 1119 "A list of alternate names that the organization is known as, or was known as in the past.", 0, 1120 java.lang.Integer.MAX_VALUE, alias); 1121 case -1429363305: 1122 /* telecom */ return new Property("telecom", "ContactPoint", "A contact detail for the organization.", 0, 1123 java.lang.Integer.MAX_VALUE, telecom); 1124 case -1147692044: 1125 /* address */ return new Property("address", "Address", "An address for the organization.", 0, 1126 java.lang.Integer.MAX_VALUE, address); 1127 case -995410646: 1128 /* partOf */ return new Property("partOf", "Reference(Organization)", 1129 "The organization of which this organization forms a part.", 0, 1, partOf); 1130 case 951526432: 1131 /* contact */ return new Property("contact", "", "Contact for the organization for a certain purpose.", 0, 1132 java.lang.Integer.MAX_VALUE, contact); 1133 case 1741102485: 1134 /* endpoint */ return new Property("endpoint", "Reference(Endpoint)", 1135 "Technical endpoints providing access to services operated for the organization.", 0, 1136 java.lang.Integer.MAX_VALUE, endpoint); 1137 default: 1138 return super.getNamedProperty(_hash, _name, _checkValid); 1139 } 1140 1141 } 1142 1143 @Override 1144 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1145 switch (hash) { 1146 case -1618432855: 1147 /* identifier */ return this.identifier == null ? new Base[0] 1148 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1149 case -1422950650: 1150 /* active */ return this.active == null ? new Base[0] : new Base[] { this.active }; // BooleanType 1151 case 3575610: 1152 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 1153 case 3373707: 1154 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 1155 case 92902992: 1156 /* alias */ return this.alias == null ? new Base[0] : this.alias.toArray(new Base[this.alias.size()]); // StringType 1157 case -1429363305: 1158 /* telecom */ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 1159 case -1147692044: 1160 /* address */ return this.address == null ? new Base[0] : this.address.toArray(new Base[this.address.size()]); // Address 1161 case -995410646: 1162 /* partOf */ return this.partOf == null ? new Base[0] : new Base[] { this.partOf }; // Reference 1163 case 951526432: 1164 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // OrganizationContactComponent 1165 case 1741102485: 1166 /* endpoint */ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 1167 default: 1168 return super.getProperty(hash, name, checkValid); 1169 } 1170 1171 } 1172 1173 @Override 1174 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1175 switch (hash) { 1176 case -1618432855: // identifier 1177 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1178 return value; 1179 case -1422950650: // active 1180 this.active = castToBoolean(value); // BooleanType 1181 return value; 1182 case 3575610: // type 1183 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 1184 return value; 1185 case 3373707: // name 1186 this.name = castToString(value); // StringType 1187 return value; 1188 case 92902992: // alias 1189 this.getAlias().add(castToString(value)); // StringType 1190 return value; 1191 case -1429363305: // telecom 1192 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 1193 return value; 1194 case -1147692044: // address 1195 this.getAddress().add(castToAddress(value)); // Address 1196 return value; 1197 case -995410646: // partOf 1198 this.partOf = castToReference(value); // Reference 1199 return value; 1200 case 951526432: // contact 1201 this.getContact().add((OrganizationContactComponent) value); // OrganizationContactComponent 1202 return value; 1203 case 1741102485: // endpoint 1204 this.getEndpoint().add(castToReference(value)); // Reference 1205 return value; 1206 default: 1207 return super.setProperty(hash, name, value); 1208 } 1209 1210 } 1211 1212 @Override 1213 public Base setProperty(String name, Base value) throws FHIRException { 1214 if (name.equals("identifier")) { 1215 this.getIdentifier().add(castToIdentifier(value)); 1216 } else if (name.equals("active")) { 1217 this.active = castToBoolean(value); // BooleanType 1218 } else if (name.equals("type")) { 1219 this.getType().add(castToCodeableConcept(value)); 1220 } else if (name.equals("name")) { 1221 this.name = castToString(value); // StringType 1222 } else if (name.equals("alias")) { 1223 this.getAlias().add(castToString(value)); 1224 } else if (name.equals("telecom")) { 1225 this.getTelecom().add(castToContactPoint(value)); 1226 } else if (name.equals("address")) { 1227 this.getAddress().add(castToAddress(value)); 1228 } else if (name.equals("partOf")) { 1229 this.partOf = castToReference(value); // Reference 1230 } else if (name.equals("contact")) { 1231 this.getContact().add((OrganizationContactComponent) value); 1232 } else if (name.equals("endpoint")) { 1233 this.getEndpoint().add(castToReference(value)); 1234 } else 1235 return super.setProperty(name, value); 1236 return value; 1237 } 1238 1239 @Override 1240 public void removeChild(String name, Base value) throws FHIRException { 1241 if (name.equals("identifier")) { 1242 this.getIdentifier().remove(castToIdentifier(value)); 1243 } else if (name.equals("active")) { 1244 this.active = null; 1245 } else if (name.equals("type")) { 1246 this.getType().remove(castToCodeableConcept(value)); 1247 } else if (name.equals("name")) { 1248 this.name = null; 1249 } else if (name.equals("alias")) { 1250 this.getAlias().remove(castToString(value)); 1251 } else if (name.equals("telecom")) { 1252 this.getTelecom().remove(castToContactPoint(value)); 1253 } else if (name.equals("address")) { 1254 this.getAddress().remove(castToAddress(value)); 1255 } else if (name.equals("partOf")) { 1256 this.partOf = null; 1257 } else if (name.equals("contact")) { 1258 this.getContact().remove((OrganizationContactComponent) value); 1259 } else if (name.equals("endpoint")) { 1260 this.getEndpoint().remove(castToReference(value)); 1261 } else 1262 super.removeChild(name, value); 1263 1264 } 1265 1266 @Override 1267 public Base makeProperty(int hash, String name) throws FHIRException { 1268 switch (hash) { 1269 case -1618432855: 1270 return addIdentifier(); 1271 case -1422950650: 1272 return getActiveElement(); 1273 case 3575610: 1274 return addType(); 1275 case 3373707: 1276 return getNameElement(); 1277 case 92902992: 1278 return addAliasElement(); 1279 case -1429363305: 1280 return addTelecom(); 1281 case -1147692044: 1282 return addAddress(); 1283 case -995410646: 1284 return getPartOf(); 1285 case 951526432: 1286 return addContact(); 1287 case 1741102485: 1288 return addEndpoint(); 1289 default: 1290 return super.makeProperty(hash, name); 1291 } 1292 1293 } 1294 1295 @Override 1296 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1297 switch (hash) { 1298 case -1618432855: 1299 /* identifier */ return new String[] { "Identifier" }; 1300 case -1422950650: 1301 /* active */ return new String[] { "boolean" }; 1302 case 3575610: 1303 /* type */ return new String[] { "CodeableConcept" }; 1304 case 3373707: 1305 /* name */ return new String[] { "string" }; 1306 case 92902992: 1307 /* alias */ return new String[] { "string" }; 1308 case -1429363305: 1309 /* telecom */ return new String[] { "ContactPoint" }; 1310 case -1147692044: 1311 /* address */ return new String[] { "Address" }; 1312 case -995410646: 1313 /* partOf */ return new String[] { "Reference" }; 1314 case 951526432: 1315 /* contact */ return new String[] {}; 1316 case 1741102485: 1317 /* endpoint */ return new String[] { "Reference" }; 1318 default: 1319 return super.getTypesForProperty(hash, name); 1320 } 1321 1322 } 1323 1324 @Override 1325 public Base addChild(String name) throws FHIRException { 1326 if (name.equals("identifier")) { 1327 return addIdentifier(); 1328 } else if (name.equals("active")) { 1329 throw new FHIRException("Cannot call addChild on a singleton property Organization.active"); 1330 } else if (name.equals("type")) { 1331 return addType(); 1332 } else if (name.equals("name")) { 1333 throw new FHIRException("Cannot call addChild on a singleton property Organization.name"); 1334 } else if (name.equals("alias")) { 1335 throw new FHIRException("Cannot call addChild on a singleton property Organization.alias"); 1336 } else if (name.equals("telecom")) { 1337 return addTelecom(); 1338 } else if (name.equals("address")) { 1339 return addAddress(); 1340 } else if (name.equals("partOf")) { 1341 this.partOf = new Reference(); 1342 return this.partOf; 1343 } else if (name.equals("contact")) { 1344 return addContact(); 1345 } else if (name.equals("endpoint")) { 1346 return addEndpoint(); 1347 } else 1348 return super.addChild(name); 1349 } 1350 1351 public String fhirType() { 1352 return "Organization"; 1353 1354 } 1355 1356 public Organization copy() { 1357 Organization dst = new Organization(); 1358 copyValues(dst); 1359 return dst; 1360 } 1361 1362 public void copyValues(Organization dst) { 1363 super.copyValues(dst); 1364 if (identifier != null) { 1365 dst.identifier = new ArrayList<Identifier>(); 1366 for (Identifier i : identifier) 1367 dst.identifier.add(i.copy()); 1368 } 1369 ; 1370 dst.active = active == null ? null : active.copy(); 1371 if (type != null) { 1372 dst.type = new ArrayList<CodeableConcept>(); 1373 for (CodeableConcept i : type) 1374 dst.type.add(i.copy()); 1375 } 1376 ; 1377 dst.name = name == null ? null : name.copy(); 1378 if (alias != null) { 1379 dst.alias = new ArrayList<StringType>(); 1380 for (StringType i : alias) 1381 dst.alias.add(i.copy()); 1382 } 1383 ; 1384 if (telecom != null) { 1385 dst.telecom = new ArrayList<ContactPoint>(); 1386 for (ContactPoint i : telecom) 1387 dst.telecom.add(i.copy()); 1388 } 1389 ; 1390 if (address != null) { 1391 dst.address = new ArrayList<Address>(); 1392 for (Address i : address) 1393 dst.address.add(i.copy()); 1394 } 1395 ; 1396 dst.partOf = partOf == null ? null : partOf.copy(); 1397 if (contact != null) { 1398 dst.contact = new ArrayList<OrganizationContactComponent>(); 1399 for (OrganizationContactComponent i : contact) 1400 dst.contact.add(i.copy()); 1401 } 1402 ; 1403 if (endpoint != null) { 1404 dst.endpoint = new ArrayList<Reference>(); 1405 for (Reference i : endpoint) 1406 dst.endpoint.add(i.copy()); 1407 } 1408 ; 1409 } 1410 1411 protected Organization typedCopy() { 1412 return copy(); 1413 } 1414 1415 @Override 1416 public boolean equalsDeep(Base other_) { 1417 if (!super.equalsDeep(other_)) 1418 return false; 1419 if (!(other_ instanceof Organization)) 1420 return false; 1421 Organization o = (Organization) other_; 1422 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) 1423 && compareDeep(type, o.type, true) && compareDeep(name, o.name, true) && compareDeep(alias, o.alias, true) 1424 && compareDeep(telecom, o.telecom, true) && compareDeep(address, o.address, true) 1425 && compareDeep(partOf, o.partOf, true) && compareDeep(contact, o.contact, true) 1426 && compareDeep(endpoint, o.endpoint, true); 1427 } 1428 1429 @Override 1430 public boolean equalsShallow(Base other_) { 1431 if (!super.equalsShallow(other_)) 1432 return false; 1433 if (!(other_ instanceof Organization)) 1434 return false; 1435 Organization o = (Organization) other_; 1436 return compareValues(active, o.active, true) && compareValues(name, o.name, true) 1437 && compareValues(alias, o.alias, true); 1438 } 1439 1440 public boolean isEmpty() { 1441 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, type, name, alias, telecom, 1442 address, partOf, contact, endpoint); 1443 } 1444 1445 @Override 1446 public ResourceType getResourceType() { 1447 return ResourceType.Organization; 1448 } 1449 1450 /** 1451 * Search parameter: <b>identifier</b> 1452 * <p> 1453 * Description: <b>Any identifier for the organization (not the accreditation 1454 * issuer's identifier)</b><br> 1455 * Type: <b>token</b><br> 1456 * Path: <b>Organization.identifier</b><br> 1457 * </p> 1458 */ 1459 @SearchParamDefinition(name = "identifier", path = "Organization.identifier", description = "Any identifier for the organization (not the accreditation issuer's identifier)", type = "token") 1460 public static final String SP_IDENTIFIER = "identifier"; 1461 /** 1462 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1463 * <p> 1464 * Description: <b>Any identifier for the organization (not the accreditation 1465 * issuer's identifier)</b><br> 1466 * Type: <b>token</b><br> 1467 * Path: <b>Organization.identifier</b><br> 1468 * </p> 1469 */ 1470 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1471 SP_IDENTIFIER); 1472 1473 /** 1474 * Search parameter: <b>partof</b> 1475 * <p> 1476 * Description: <b>An organization of which this organization forms a 1477 * part</b><br> 1478 * Type: <b>reference</b><br> 1479 * Path: <b>Organization.partOf</b><br> 1480 * </p> 1481 */ 1482 @SearchParamDefinition(name = "partof", path = "Organization.partOf", description = "An organization of which this organization forms a part", type = "reference", target = { 1483 Organization.class }) 1484 public static final String SP_PARTOF = "partof"; 1485 /** 1486 * <b>Fluent Client</b> search parameter constant for <b>partof</b> 1487 * <p> 1488 * Description: <b>An organization of which this organization forms a 1489 * part</b><br> 1490 * Type: <b>reference</b><br> 1491 * Path: <b>Organization.partOf</b><br> 1492 * </p> 1493 */ 1494 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTOF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1495 SP_PARTOF); 1496 1497 /** 1498 * Constant for fluent queries to be used to add include statements. Specifies 1499 * the path value of "<b>Organization:partof</b>". 1500 */ 1501 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTOF = new ca.uhn.fhir.model.api.Include( 1502 "Organization:partof").toLocked(); 1503 1504 /** 1505 * Search parameter: <b>address</b> 1506 * <p> 1507 * Description: <b>A server defined search that may match any of the string 1508 * fields in the Address, including line, city, district, state, country, 1509 * postalCode, and/or text</b><br> 1510 * Type: <b>string</b><br> 1511 * Path: <b>Organization.address</b><br> 1512 * </p> 1513 */ 1514 @SearchParamDefinition(name = "address", path = "Organization.address", description = "A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text", type = "string") 1515 public static final String SP_ADDRESS = "address"; 1516 /** 1517 * <b>Fluent Client</b> search parameter constant for <b>address</b> 1518 * <p> 1519 * Description: <b>A server defined search that may match any of the string 1520 * fields in the Address, including line, city, district, state, country, 1521 * postalCode, and/or text</b><br> 1522 * Type: <b>string</b><br> 1523 * Path: <b>Organization.address</b><br> 1524 * </p> 1525 */ 1526 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS = new ca.uhn.fhir.rest.gclient.StringClientParam( 1527 SP_ADDRESS); 1528 1529 /** 1530 * Search parameter: <b>address-state</b> 1531 * <p> 1532 * Description: <b>A state specified in an address</b><br> 1533 * Type: <b>string</b><br> 1534 * Path: <b>Organization.address.state</b><br> 1535 * </p> 1536 */ 1537 @SearchParamDefinition(name = "address-state", path = "Organization.address.state", description = "A state specified in an address", type = "string") 1538 public static final String SP_ADDRESS_STATE = "address-state"; 1539 /** 1540 * <b>Fluent Client</b> search parameter constant for <b>address-state</b> 1541 * <p> 1542 * Description: <b>A state specified in an address</b><br> 1543 * Type: <b>string</b><br> 1544 * Path: <b>Organization.address.state</b><br> 1545 * </p> 1546 */ 1547 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_STATE = new ca.uhn.fhir.rest.gclient.StringClientParam( 1548 SP_ADDRESS_STATE); 1549 1550 /** 1551 * Search parameter: <b>active</b> 1552 * <p> 1553 * Description: <b>Is the Organization record active</b><br> 1554 * Type: <b>token</b><br> 1555 * Path: <b>Organization.active</b><br> 1556 * </p> 1557 */ 1558 @SearchParamDefinition(name = "active", path = "Organization.active", description = "Is the Organization record active", type = "token") 1559 public static final String SP_ACTIVE = "active"; 1560 /** 1561 * <b>Fluent Client</b> search parameter constant for <b>active</b> 1562 * <p> 1563 * Description: <b>Is the Organization record active</b><br> 1564 * Type: <b>token</b><br> 1565 * Path: <b>Organization.active</b><br> 1566 * </p> 1567 */ 1568 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1569 SP_ACTIVE); 1570 1571 /** 1572 * Search parameter: <b>type</b> 1573 * <p> 1574 * Description: <b>A code for the type of organization</b><br> 1575 * Type: <b>token</b><br> 1576 * Path: <b>Organization.type</b><br> 1577 * </p> 1578 */ 1579 @SearchParamDefinition(name = "type", path = "Organization.type", description = "A code for the type of organization", type = "token") 1580 public static final String SP_TYPE = "type"; 1581 /** 1582 * <b>Fluent Client</b> search parameter constant for <b>type</b> 1583 * <p> 1584 * Description: <b>A code for the type of organization</b><br> 1585 * Type: <b>token</b><br> 1586 * Path: <b>Organization.type</b><br> 1587 * </p> 1588 */ 1589 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1590 SP_TYPE); 1591 1592 /** 1593 * Search parameter: <b>address-postalcode</b> 1594 * <p> 1595 * Description: <b>A postal code specified in an address</b><br> 1596 * Type: <b>string</b><br> 1597 * Path: <b>Organization.address.postalCode</b><br> 1598 * </p> 1599 */ 1600 @SearchParamDefinition(name = "address-postalcode", path = "Organization.address.postalCode", description = "A postal code specified in an address", type = "string") 1601 public static final String SP_ADDRESS_POSTALCODE = "address-postalcode"; 1602 /** 1603 * <b>Fluent Client</b> search parameter constant for <b>address-postalcode</b> 1604 * <p> 1605 * Description: <b>A postal code specified in an address</b><br> 1606 * Type: <b>string</b><br> 1607 * Path: <b>Organization.address.postalCode</b><br> 1608 * </p> 1609 */ 1610 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_POSTALCODE = new ca.uhn.fhir.rest.gclient.StringClientParam( 1611 SP_ADDRESS_POSTALCODE); 1612 1613 /** 1614 * Search parameter: <b>address-country</b> 1615 * <p> 1616 * Description: <b>A country specified in an address</b><br> 1617 * Type: <b>string</b><br> 1618 * Path: <b>Organization.address.country</b><br> 1619 * </p> 1620 */ 1621 @SearchParamDefinition(name = "address-country", path = "Organization.address.country", description = "A country specified in an address", type = "string") 1622 public static final String SP_ADDRESS_COUNTRY = "address-country"; 1623 /** 1624 * <b>Fluent Client</b> search parameter constant for <b>address-country</b> 1625 * <p> 1626 * Description: <b>A country specified in an address</b><br> 1627 * Type: <b>string</b><br> 1628 * Path: <b>Organization.address.country</b><br> 1629 * </p> 1630 */ 1631 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_COUNTRY = new ca.uhn.fhir.rest.gclient.StringClientParam( 1632 SP_ADDRESS_COUNTRY); 1633 1634 /** 1635 * Search parameter: <b>endpoint</b> 1636 * <p> 1637 * Description: <b>Technical endpoints providing access to services operated for 1638 * the organization</b><br> 1639 * Type: <b>reference</b><br> 1640 * Path: <b>Organization.endpoint</b><br> 1641 * </p> 1642 */ 1643 @SearchParamDefinition(name = "endpoint", path = "Organization.endpoint", description = "Technical endpoints providing access to services operated for the organization", type = "reference", target = { 1644 Endpoint.class }) 1645 public static final String SP_ENDPOINT = "endpoint"; 1646 /** 1647 * <b>Fluent Client</b> search parameter constant for <b>endpoint</b> 1648 * <p> 1649 * Description: <b>Technical endpoints providing access to services operated for 1650 * the organization</b><br> 1651 * Type: <b>reference</b><br> 1652 * Path: <b>Organization.endpoint</b><br> 1653 * </p> 1654 */ 1655 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENDPOINT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1656 SP_ENDPOINT); 1657 1658 /** 1659 * Constant for fluent queries to be used to add include statements. Specifies 1660 * the path value of "<b>Organization:endpoint</b>". 1661 */ 1662 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENDPOINT = new ca.uhn.fhir.model.api.Include( 1663 "Organization:endpoint").toLocked(); 1664 1665 /** 1666 * Search parameter: <b>phonetic</b> 1667 * <p> 1668 * Description: <b>A portion of the organization's name using some kind of 1669 * phonetic matching algorithm</b><br> 1670 * Type: <b>string</b><br> 1671 * Path: <b>Organization.name</b><br> 1672 * </p> 1673 */ 1674 @SearchParamDefinition(name = "phonetic", path = "Organization.name", description = "A portion of the organization's name using some kind of phonetic matching algorithm", type = "string") 1675 public static final String SP_PHONETIC = "phonetic"; 1676 /** 1677 * <b>Fluent Client</b> search parameter constant for <b>phonetic</b> 1678 * <p> 1679 * Description: <b>A portion of the organization's name using some kind of 1680 * phonetic matching algorithm</b><br> 1681 * Type: <b>string</b><br> 1682 * Path: <b>Organization.name</b><br> 1683 * </p> 1684 */ 1685 public static final ca.uhn.fhir.rest.gclient.StringClientParam PHONETIC = new ca.uhn.fhir.rest.gclient.StringClientParam( 1686 SP_PHONETIC); 1687 1688 /** 1689 * Search parameter: <b>name</b> 1690 * <p> 1691 * Description: <b>A portion of the organization's name or alias</b><br> 1692 * Type: <b>string</b><br> 1693 * Path: <b>Organization.name, Organization.alias</b><br> 1694 * </p> 1695 */ 1696 @SearchParamDefinition(name = "name", path = "Organization.name | Organization.alias", description = "A portion of the organization's name or alias", type = "string") 1697 public static final String SP_NAME = "name"; 1698 /** 1699 * <b>Fluent Client</b> search parameter constant for <b>name</b> 1700 * <p> 1701 * Description: <b>A portion of the organization's name or alias</b><br> 1702 * Type: <b>string</b><br> 1703 * Path: <b>Organization.name, Organization.alias</b><br> 1704 * </p> 1705 */ 1706 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 1707 SP_NAME); 1708 1709 /** 1710 * Search parameter: <b>address-use</b> 1711 * <p> 1712 * Description: <b>A use code specified in an address</b><br> 1713 * Type: <b>token</b><br> 1714 * Path: <b>Organization.address.use</b><br> 1715 * </p> 1716 */ 1717 @SearchParamDefinition(name = "address-use", path = "Organization.address.use", description = "A use code specified in an address", type = "token") 1718 public static final String SP_ADDRESS_USE = "address-use"; 1719 /** 1720 * <b>Fluent Client</b> search parameter constant for <b>address-use</b> 1721 * <p> 1722 * Description: <b>A use code specified in an address</b><br> 1723 * Type: <b>token</b><br> 1724 * Path: <b>Organization.address.use</b><br> 1725 * </p> 1726 */ 1727 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADDRESS_USE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1728 SP_ADDRESS_USE); 1729 1730 /** 1731 * Search parameter: <b>address-city</b> 1732 * <p> 1733 * Description: <b>A city specified in an address</b><br> 1734 * Type: <b>string</b><br> 1735 * Path: <b>Organization.address.city</b><br> 1736 * </p> 1737 */ 1738 @SearchParamDefinition(name = "address-city", path = "Organization.address.city", description = "A city specified in an address", type = "string") 1739 public static final String SP_ADDRESS_CITY = "address-city"; 1740 /** 1741 * <b>Fluent Client</b> search parameter constant for <b>address-city</b> 1742 * <p> 1743 * Description: <b>A city specified in an address</b><br> 1744 * Type: <b>string</b><br> 1745 * Path: <b>Organization.address.city</b><br> 1746 * </p> 1747 */ 1748 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_CITY = new ca.uhn.fhir.rest.gclient.StringClientParam( 1749 SP_ADDRESS_CITY); 1750 1751}