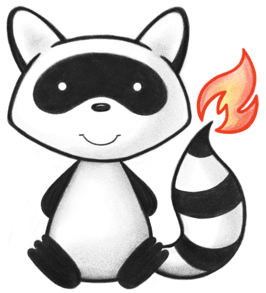
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042 043/** 044 * Defines an affiliation/assotiation/relationship between 2 distinct 045 * oganizations, that is not a part-of relationship/sub-division relationship. 046 */ 047@ResourceDef(name = "OrganizationAffiliation", profile = "http://hl7.org/fhir/StructureDefinition/OrganizationAffiliation") 048public class OrganizationAffiliation extends DomainResource { 049 050 /** 051 * Business identifiers that are specific to this role. 052 */ 053 @Child(name = "identifier", type = { 054 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 055 @Description(shortDefinition = "Business identifiers that are specific to this role", formalDefinition = "Business identifiers that are specific to this role.") 056 protected List<Identifier> identifier; 057 058 /** 059 * Whether this organization affiliation record is in active use. 060 */ 061 @Child(name = "active", type = { BooleanType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 062 @Description(shortDefinition = "Whether this organization affiliation record is in active use", formalDefinition = "Whether this organization affiliation record is in active use.") 063 protected BooleanType active; 064 065 /** 066 * The period during which the participatingOrganization is affiliated with the 067 * primary organization. 068 */ 069 @Child(name = "period", type = { Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 070 @Description(shortDefinition = "The period during which the participatingOrganization is affiliated with the primary organization", formalDefinition = "The period during which the participatingOrganization is affiliated with the primary organization.") 071 protected Period period; 072 073 /** 074 * Organization where the role is available (primary organization/has members). 075 */ 076 @Child(name = "organization", type = { 077 Organization.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 078 @Description(shortDefinition = "Organization where the role is available", formalDefinition = "Organization where the role is available (primary organization/has members).") 079 protected Reference organization; 080 081 /** 082 * The actual object that is the target of the reference (Organization where the 083 * role is available (primary organization/has members).) 084 */ 085 protected Organization organizationTarget; 086 087 /** 088 * The Participating Organization provides/performs the role(s) defined by the 089 * code to the Primary Organization (e.g. providing services or is a member of). 090 */ 091 @Child(name = "participatingOrganization", type = { 092 Organization.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 093 @Description(shortDefinition = "Organization that provides/performs the role (e.g. providing services or is a member of)", formalDefinition = "The Participating Organization provides/performs the role(s) defined by the code to the Primary Organization (e.g. providing services or is a member of).") 094 protected Reference participatingOrganization; 095 096 /** 097 * The actual object that is the target of the reference (The Participating 098 * Organization provides/performs the role(s) defined by the code to the Primary 099 * Organization (e.g. providing services or is a member of).) 100 */ 101 protected Organization participatingOrganizationTarget; 102 103 /** 104 * Health insurance provider network in which the participatingOrganization 105 * provides the role's services (if defined) at the indicated locations (if 106 * defined). 107 */ 108 @Child(name = "network", type = { 109 Organization.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 110 @Description(shortDefinition = "Health insurance provider network in which the participatingOrganization provides the role's services (if defined) at the indicated locations (if defined)", formalDefinition = "Health insurance provider network in which the participatingOrganization provides the role's services (if defined) at the indicated locations (if defined).") 111 protected List<Reference> network; 112 /** 113 * The actual objects that are the target of the reference (Health insurance 114 * provider network in which the participatingOrganization provides the role's 115 * services (if defined) at the indicated locations (if defined).) 116 */ 117 protected List<Organization> networkTarget; 118 119 /** 120 * Definition of the role the participatingOrganization plays in the 121 * association. 122 */ 123 @Child(name = "code", type = { 124 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 125 @Description(shortDefinition = "Definition of the role the participatingOrganization plays", formalDefinition = "Definition of the role the participatingOrganization plays in the association.") 126 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/organization-role") 127 protected List<CodeableConcept> code; 128 129 /** 130 * Specific specialty of the participatingOrganization in the context of the 131 * role. 132 */ 133 @Child(name = "specialty", type = { 134 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 135 @Description(shortDefinition = "Specific specialty of the participatingOrganization in the context of the role", formalDefinition = "Specific specialty of the participatingOrganization in the context of the role.") 136 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/c80-practice-codes") 137 protected List<CodeableConcept> specialty; 138 139 /** 140 * The location(s) at which the role occurs. 141 */ 142 @Child(name = "location", type = { 143 Location.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 144 @Description(shortDefinition = "The location(s) at which the role occurs", formalDefinition = "The location(s) at which the role occurs.") 145 protected List<Reference> location; 146 /** 147 * The actual objects that are the target of the reference (The location(s) at 148 * which the role occurs.) 149 */ 150 protected List<Location> locationTarget; 151 152 /** 153 * Healthcare services provided through the role. 154 */ 155 @Child(name = "healthcareService", type = { 156 HealthcareService.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 157 @Description(shortDefinition = "Healthcare services provided through the role", formalDefinition = "Healthcare services provided through the role.") 158 protected List<Reference> healthcareService; 159 /** 160 * The actual objects that are the target of the reference (Healthcare services 161 * provided through the role.) 162 */ 163 protected List<HealthcareService> healthcareServiceTarget; 164 165 /** 166 * Contact details at the participatingOrganization relevant to this 167 * Affiliation. 168 */ 169 @Child(name = "telecom", type = { 170 ContactPoint.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 171 @Description(shortDefinition = "Contact details at the participatingOrganization relevant to this Affiliation", formalDefinition = "Contact details at the participatingOrganization relevant to this Affiliation.") 172 protected List<ContactPoint> telecom; 173 174 /** 175 * Technical endpoints providing access to services operated for this role. 176 */ 177 @Child(name = "endpoint", type = { 178 Endpoint.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 179 @Description(shortDefinition = "Technical endpoints providing access to services operated for this role", formalDefinition = "Technical endpoints providing access to services operated for this role.") 180 protected List<Reference> endpoint; 181 /** 182 * The actual objects that are the target of the reference (Technical endpoints 183 * providing access to services operated for this role.) 184 */ 185 protected List<Endpoint> endpointTarget; 186 187 private static final long serialVersionUID = -62510821L; 188 189 /** 190 * Constructor 191 */ 192 public OrganizationAffiliation() { 193 super(); 194 } 195 196 /** 197 * @return {@link #identifier} (Business identifiers that are specific to this 198 * role.) 199 */ 200 public List<Identifier> getIdentifier() { 201 if (this.identifier == null) 202 this.identifier = new ArrayList<Identifier>(); 203 return this.identifier; 204 } 205 206 /** 207 * @return Returns a reference to <code>this</code> for easy method chaining 208 */ 209 public OrganizationAffiliation setIdentifier(List<Identifier> theIdentifier) { 210 this.identifier = theIdentifier; 211 return this; 212 } 213 214 public boolean hasIdentifier() { 215 if (this.identifier == null) 216 return false; 217 for (Identifier item : this.identifier) 218 if (!item.isEmpty()) 219 return true; 220 return false; 221 } 222 223 public Identifier addIdentifier() { // 3 224 Identifier t = new Identifier(); 225 if (this.identifier == null) 226 this.identifier = new ArrayList<Identifier>(); 227 this.identifier.add(t); 228 return t; 229 } 230 231 public OrganizationAffiliation addIdentifier(Identifier t) { // 3 232 if (t == null) 233 return this; 234 if (this.identifier == null) 235 this.identifier = new ArrayList<Identifier>(); 236 this.identifier.add(t); 237 return this; 238 } 239 240 /** 241 * @return The first repetition of repeating field {@link #identifier}, creating 242 * it if it does not already exist 243 */ 244 public Identifier getIdentifierFirstRep() { 245 if (getIdentifier().isEmpty()) { 246 addIdentifier(); 247 } 248 return getIdentifier().get(0); 249 } 250 251 /** 252 * @return {@link #active} (Whether this organization affiliation record is in 253 * active use.). This is the underlying object with id, value and 254 * extensions. The accessor "getActive" gives direct access to the value 255 */ 256 public BooleanType getActiveElement() { 257 if (this.active == null) 258 if (Configuration.errorOnAutoCreate()) 259 throw new Error("Attempt to auto-create OrganizationAffiliation.active"); 260 else if (Configuration.doAutoCreate()) 261 this.active = new BooleanType(); // bb 262 return this.active; 263 } 264 265 public boolean hasActiveElement() { 266 return this.active != null && !this.active.isEmpty(); 267 } 268 269 public boolean hasActive() { 270 return this.active != null && !this.active.isEmpty(); 271 } 272 273 /** 274 * @param value {@link #active} (Whether this organization affiliation record is 275 * in active use.). This is the underlying object with id, value 276 * and extensions. The accessor "getActive" gives direct access to 277 * the value 278 */ 279 public OrganizationAffiliation setActiveElement(BooleanType value) { 280 this.active = value; 281 return this; 282 } 283 284 /** 285 * @return Whether this organization affiliation record is in active use. 286 */ 287 public boolean getActive() { 288 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 289 } 290 291 /** 292 * @param value Whether this organization affiliation record is in active use. 293 */ 294 public OrganizationAffiliation setActive(boolean value) { 295 if (this.active == null) 296 this.active = new BooleanType(); 297 this.active.setValue(value); 298 return this; 299 } 300 301 /** 302 * @return {@link #period} (The period during which the 303 * participatingOrganization is affiliated with the primary 304 * organization.) 305 */ 306 public Period getPeriod() { 307 if (this.period == null) 308 if (Configuration.errorOnAutoCreate()) 309 throw new Error("Attempt to auto-create OrganizationAffiliation.period"); 310 else if (Configuration.doAutoCreate()) 311 this.period = new Period(); // cc 312 return this.period; 313 } 314 315 public boolean hasPeriod() { 316 return this.period != null && !this.period.isEmpty(); 317 } 318 319 /** 320 * @param value {@link #period} (The period during which the 321 * participatingOrganization is affiliated with the primary 322 * organization.) 323 */ 324 public OrganizationAffiliation setPeriod(Period value) { 325 this.period = value; 326 return this; 327 } 328 329 /** 330 * @return {@link #organization} (Organization where the role is available 331 * (primary organization/has members).) 332 */ 333 public Reference getOrganization() { 334 if (this.organization == null) 335 if (Configuration.errorOnAutoCreate()) 336 throw new Error("Attempt to auto-create OrganizationAffiliation.organization"); 337 else if (Configuration.doAutoCreate()) 338 this.organization = new Reference(); // cc 339 return this.organization; 340 } 341 342 public boolean hasOrganization() { 343 return this.organization != null && !this.organization.isEmpty(); 344 } 345 346 /** 347 * @param value {@link #organization} (Organization where the role is available 348 * (primary organization/has members).) 349 */ 350 public OrganizationAffiliation setOrganization(Reference value) { 351 this.organization = value; 352 return this; 353 } 354 355 /** 356 * @return {@link #organization} The actual object that is the target of the 357 * reference. The reference library doesn't populate this, but you can 358 * use it to hold the resource if you resolve it. (Organization where 359 * the role is available (primary organization/has members).) 360 */ 361 public Organization getOrganizationTarget() { 362 if (this.organizationTarget == null) 363 if (Configuration.errorOnAutoCreate()) 364 throw new Error("Attempt to auto-create OrganizationAffiliation.organization"); 365 else if (Configuration.doAutoCreate()) 366 this.organizationTarget = new Organization(); // aa 367 return this.organizationTarget; 368 } 369 370 /** 371 * @param value {@link #organization} The actual object that is the target of 372 * the reference. The reference library doesn't use these, but you 373 * can use it to hold the resource if you resolve it. (Organization 374 * where the role is available (primary organization/has members).) 375 */ 376 public OrganizationAffiliation setOrganizationTarget(Organization value) { 377 this.organizationTarget = value; 378 return this; 379 } 380 381 /** 382 * @return {@link #participatingOrganization} (The Participating Organization 383 * provides/performs the role(s) defined by the code to the Primary 384 * Organization (e.g. providing services or is a member of).) 385 */ 386 public Reference getParticipatingOrganization() { 387 if (this.participatingOrganization == null) 388 if (Configuration.errorOnAutoCreate()) 389 throw new Error("Attempt to auto-create OrganizationAffiliation.participatingOrganization"); 390 else if (Configuration.doAutoCreate()) 391 this.participatingOrganization = new Reference(); // cc 392 return this.participatingOrganization; 393 } 394 395 public boolean hasParticipatingOrganization() { 396 return this.participatingOrganization != null && !this.participatingOrganization.isEmpty(); 397 } 398 399 /** 400 * @param value {@link #participatingOrganization} (The Participating 401 * Organization provides/performs the role(s) defined by the code 402 * to the Primary Organization (e.g. providing services or is a 403 * member of).) 404 */ 405 public OrganizationAffiliation setParticipatingOrganization(Reference value) { 406 this.participatingOrganization = value; 407 return this; 408 } 409 410 /** 411 * @return {@link #participatingOrganization} The actual object that is the 412 * target of the reference. The reference library doesn't populate this, 413 * but you can use it to hold the resource if you resolve it. (The 414 * Participating Organization provides/performs the role(s) defined by 415 * the code to the Primary Organization (e.g. providing services or is a 416 * member of).) 417 */ 418 public Organization getParticipatingOrganizationTarget() { 419 if (this.participatingOrganizationTarget == null) 420 if (Configuration.errorOnAutoCreate()) 421 throw new Error("Attempt to auto-create OrganizationAffiliation.participatingOrganization"); 422 else if (Configuration.doAutoCreate()) 423 this.participatingOrganizationTarget = new Organization(); // aa 424 return this.participatingOrganizationTarget; 425 } 426 427 /** 428 * @param value {@link #participatingOrganization} The actual object that is the 429 * target of the reference. The reference library doesn't use 430 * these, but you can use it to hold the resource if you resolve 431 * it. (The Participating Organization provides/performs the 432 * role(s) defined by the code to the Primary Organization (e.g. 433 * providing services or is a member of).) 434 */ 435 public OrganizationAffiliation setParticipatingOrganizationTarget(Organization value) { 436 this.participatingOrganizationTarget = value; 437 return this; 438 } 439 440 /** 441 * @return {@link #network} (Health insurance provider network in which the 442 * participatingOrganization provides the role's services (if defined) 443 * at the indicated locations (if defined).) 444 */ 445 public List<Reference> getNetwork() { 446 if (this.network == null) 447 this.network = new ArrayList<Reference>(); 448 return this.network; 449 } 450 451 /** 452 * @return Returns a reference to <code>this</code> for easy method chaining 453 */ 454 public OrganizationAffiliation setNetwork(List<Reference> theNetwork) { 455 this.network = theNetwork; 456 return this; 457 } 458 459 public boolean hasNetwork() { 460 if (this.network == null) 461 return false; 462 for (Reference item : this.network) 463 if (!item.isEmpty()) 464 return true; 465 return false; 466 } 467 468 public Reference addNetwork() { // 3 469 Reference t = new Reference(); 470 if (this.network == null) 471 this.network = new ArrayList<Reference>(); 472 this.network.add(t); 473 return t; 474 } 475 476 public OrganizationAffiliation addNetwork(Reference t) { // 3 477 if (t == null) 478 return this; 479 if (this.network == null) 480 this.network = new ArrayList<Reference>(); 481 this.network.add(t); 482 return this; 483 } 484 485 /** 486 * @return The first repetition of repeating field {@link #network}, creating it 487 * if it does not already exist 488 */ 489 public Reference getNetworkFirstRep() { 490 if (getNetwork().isEmpty()) { 491 addNetwork(); 492 } 493 return getNetwork().get(0); 494 } 495 496 /** 497 * @deprecated Use Reference#setResource(IBaseResource) instead 498 */ 499 @Deprecated 500 public List<Organization> getNetworkTarget() { 501 if (this.networkTarget == null) 502 this.networkTarget = new ArrayList<Organization>(); 503 return this.networkTarget; 504 } 505 506 /** 507 * @deprecated Use Reference#setResource(IBaseResource) instead 508 */ 509 @Deprecated 510 public Organization addNetworkTarget() { 511 Organization r = new Organization(); 512 if (this.networkTarget == null) 513 this.networkTarget = new ArrayList<Organization>(); 514 this.networkTarget.add(r); 515 return r; 516 } 517 518 /** 519 * @return {@link #code} (Definition of the role the participatingOrganization 520 * plays in the association.) 521 */ 522 public List<CodeableConcept> getCode() { 523 if (this.code == null) 524 this.code = new ArrayList<CodeableConcept>(); 525 return this.code; 526 } 527 528 /** 529 * @return Returns a reference to <code>this</code> for easy method chaining 530 */ 531 public OrganizationAffiliation setCode(List<CodeableConcept> theCode) { 532 this.code = theCode; 533 return this; 534 } 535 536 public boolean hasCode() { 537 if (this.code == null) 538 return false; 539 for (CodeableConcept item : this.code) 540 if (!item.isEmpty()) 541 return true; 542 return false; 543 } 544 545 public CodeableConcept addCode() { // 3 546 CodeableConcept t = new CodeableConcept(); 547 if (this.code == null) 548 this.code = new ArrayList<CodeableConcept>(); 549 this.code.add(t); 550 return t; 551 } 552 553 public OrganizationAffiliation addCode(CodeableConcept t) { // 3 554 if (t == null) 555 return this; 556 if (this.code == null) 557 this.code = new ArrayList<CodeableConcept>(); 558 this.code.add(t); 559 return this; 560 } 561 562 /** 563 * @return The first repetition of repeating field {@link #code}, creating it if 564 * it does not already exist 565 */ 566 public CodeableConcept getCodeFirstRep() { 567 if (getCode().isEmpty()) { 568 addCode(); 569 } 570 return getCode().get(0); 571 } 572 573 /** 574 * @return {@link #specialty} (Specific specialty of the 575 * participatingOrganization in the context of the role.) 576 */ 577 public List<CodeableConcept> getSpecialty() { 578 if (this.specialty == null) 579 this.specialty = new ArrayList<CodeableConcept>(); 580 return this.specialty; 581 } 582 583 /** 584 * @return Returns a reference to <code>this</code> for easy method chaining 585 */ 586 public OrganizationAffiliation setSpecialty(List<CodeableConcept> theSpecialty) { 587 this.specialty = theSpecialty; 588 return this; 589 } 590 591 public boolean hasSpecialty() { 592 if (this.specialty == null) 593 return false; 594 for (CodeableConcept item : this.specialty) 595 if (!item.isEmpty()) 596 return true; 597 return false; 598 } 599 600 public CodeableConcept addSpecialty() { // 3 601 CodeableConcept t = new CodeableConcept(); 602 if (this.specialty == null) 603 this.specialty = new ArrayList<CodeableConcept>(); 604 this.specialty.add(t); 605 return t; 606 } 607 608 public OrganizationAffiliation addSpecialty(CodeableConcept t) { // 3 609 if (t == null) 610 return this; 611 if (this.specialty == null) 612 this.specialty = new ArrayList<CodeableConcept>(); 613 this.specialty.add(t); 614 return this; 615 } 616 617 /** 618 * @return The first repetition of repeating field {@link #specialty}, creating 619 * it if it does not already exist 620 */ 621 public CodeableConcept getSpecialtyFirstRep() { 622 if (getSpecialty().isEmpty()) { 623 addSpecialty(); 624 } 625 return getSpecialty().get(0); 626 } 627 628 /** 629 * @return {@link #location} (The location(s) at which the role occurs.) 630 */ 631 public List<Reference> getLocation() { 632 if (this.location == null) 633 this.location = new ArrayList<Reference>(); 634 return this.location; 635 } 636 637 /** 638 * @return Returns a reference to <code>this</code> for easy method chaining 639 */ 640 public OrganizationAffiliation setLocation(List<Reference> theLocation) { 641 this.location = theLocation; 642 return this; 643 } 644 645 public boolean hasLocation() { 646 if (this.location == null) 647 return false; 648 for (Reference item : this.location) 649 if (!item.isEmpty()) 650 return true; 651 return false; 652 } 653 654 public Reference addLocation() { // 3 655 Reference t = new Reference(); 656 if (this.location == null) 657 this.location = new ArrayList<Reference>(); 658 this.location.add(t); 659 return t; 660 } 661 662 public OrganizationAffiliation addLocation(Reference t) { // 3 663 if (t == null) 664 return this; 665 if (this.location == null) 666 this.location = new ArrayList<Reference>(); 667 this.location.add(t); 668 return this; 669 } 670 671 /** 672 * @return The first repetition of repeating field {@link #location}, creating 673 * it if it does not already exist 674 */ 675 public Reference getLocationFirstRep() { 676 if (getLocation().isEmpty()) { 677 addLocation(); 678 } 679 return getLocation().get(0); 680 } 681 682 /** 683 * @deprecated Use Reference#setResource(IBaseResource) instead 684 */ 685 @Deprecated 686 public List<Location> getLocationTarget() { 687 if (this.locationTarget == null) 688 this.locationTarget = new ArrayList<Location>(); 689 return this.locationTarget; 690 } 691 692 /** 693 * @deprecated Use Reference#setResource(IBaseResource) instead 694 */ 695 @Deprecated 696 public Location addLocationTarget() { 697 Location r = new Location(); 698 if (this.locationTarget == null) 699 this.locationTarget = new ArrayList<Location>(); 700 this.locationTarget.add(r); 701 return r; 702 } 703 704 /** 705 * @return {@link #healthcareService} (Healthcare services provided through the 706 * role.) 707 */ 708 public List<Reference> getHealthcareService() { 709 if (this.healthcareService == null) 710 this.healthcareService = new ArrayList<Reference>(); 711 return this.healthcareService; 712 } 713 714 /** 715 * @return Returns a reference to <code>this</code> for easy method chaining 716 */ 717 public OrganizationAffiliation setHealthcareService(List<Reference> theHealthcareService) { 718 this.healthcareService = theHealthcareService; 719 return this; 720 } 721 722 public boolean hasHealthcareService() { 723 if (this.healthcareService == null) 724 return false; 725 for (Reference item : this.healthcareService) 726 if (!item.isEmpty()) 727 return true; 728 return false; 729 } 730 731 public Reference addHealthcareService() { // 3 732 Reference t = new Reference(); 733 if (this.healthcareService == null) 734 this.healthcareService = new ArrayList<Reference>(); 735 this.healthcareService.add(t); 736 return t; 737 } 738 739 public OrganizationAffiliation addHealthcareService(Reference t) { // 3 740 if (t == null) 741 return this; 742 if (this.healthcareService == null) 743 this.healthcareService = new ArrayList<Reference>(); 744 this.healthcareService.add(t); 745 return this; 746 } 747 748 /** 749 * @return The first repetition of repeating field {@link #healthcareService}, 750 * creating it if it does not already exist 751 */ 752 public Reference getHealthcareServiceFirstRep() { 753 if (getHealthcareService().isEmpty()) { 754 addHealthcareService(); 755 } 756 return getHealthcareService().get(0); 757 } 758 759 /** 760 * @deprecated Use Reference#setResource(IBaseResource) instead 761 */ 762 @Deprecated 763 public List<HealthcareService> getHealthcareServiceTarget() { 764 if (this.healthcareServiceTarget == null) 765 this.healthcareServiceTarget = new ArrayList<HealthcareService>(); 766 return this.healthcareServiceTarget; 767 } 768 769 /** 770 * @deprecated Use Reference#setResource(IBaseResource) instead 771 */ 772 @Deprecated 773 public HealthcareService addHealthcareServiceTarget() { 774 HealthcareService r = new HealthcareService(); 775 if (this.healthcareServiceTarget == null) 776 this.healthcareServiceTarget = new ArrayList<HealthcareService>(); 777 this.healthcareServiceTarget.add(r); 778 return r; 779 } 780 781 /** 782 * @return {@link #telecom} (Contact details at the participatingOrganization 783 * relevant to this Affiliation.) 784 */ 785 public List<ContactPoint> getTelecom() { 786 if (this.telecom == null) 787 this.telecom = new ArrayList<ContactPoint>(); 788 return this.telecom; 789 } 790 791 /** 792 * @return Returns a reference to <code>this</code> for easy method chaining 793 */ 794 public OrganizationAffiliation setTelecom(List<ContactPoint> theTelecom) { 795 this.telecom = theTelecom; 796 return this; 797 } 798 799 public boolean hasTelecom() { 800 if (this.telecom == null) 801 return false; 802 for (ContactPoint item : this.telecom) 803 if (!item.isEmpty()) 804 return true; 805 return false; 806 } 807 808 public ContactPoint addTelecom() { // 3 809 ContactPoint t = new ContactPoint(); 810 if (this.telecom == null) 811 this.telecom = new ArrayList<ContactPoint>(); 812 this.telecom.add(t); 813 return t; 814 } 815 816 public OrganizationAffiliation addTelecom(ContactPoint t) { // 3 817 if (t == null) 818 return this; 819 if (this.telecom == null) 820 this.telecom = new ArrayList<ContactPoint>(); 821 this.telecom.add(t); 822 return this; 823 } 824 825 /** 826 * @return The first repetition of repeating field {@link #telecom}, creating it 827 * if it does not already exist 828 */ 829 public ContactPoint getTelecomFirstRep() { 830 if (getTelecom().isEmpty()) { 831 addTelecom(); 832 } 833 return getTelecom().get(0); 834 } 835 836 /** 837 * @return {@link #endpoint} (Technical endpoints providing access to services 838 * operated for this role.) 839 */ 840 public List<Reference> getEndpoint() { 841 if (this.endpoint == null) 842 this.endpoint = new ArrayList<Reference>(); 843 return this.endpoint; 844 } 845 846 /** 847 * @return Returns a reference to <code>this</code> for easy method chaining 848 */ 849 public OrganizationAffiliation setEndpoint(List<Reference> theEndpoint) { 850 this.endpoint = theEndpoint; 851 return this; 852 } 853 854 public boolean hasEndpoint() { 855 if (this.endpoint == null) 856 return false; 857 for (Reference item : this.endpoint) 858 if (!item.isEmpty()) 859 return true; 860 return false; 861 } 862 863 public Reference addEndpoint() { // 3 864 Reference t = new Reference(); 865 if (this.endpoint == null) 866 this.endpoint = new ArrayList<Reference>(); 867 this.endpoint.add(t); 868 return t; 869 } 870 871 public OrganizationAffiliation addEndpoint(Reference t) { // 3 872 if (t == null) 873 return this; 874 if (this.endpoint == null) 875 this.endpoint = new ArrayList<Reference>(); 876 this.endpoint.add(t); 877 return this; 878 } 879 880 /** 881 * @return The first repetition of repeating field {@link #endpoint}, creating 882 * it if it does not already exist 883 */ 884 public Reference getEndpointFirstRep() { 885 if (getEndpoint().isEmpty()) { 886 addEndpoint(); 887 } 888 return getEndpoint().get(0); 889 } 890 891 /** 892 * @deprecated Use Reference#setResource(IBaseResource) instead 893 */ 894 @Deprecated 895 public List<Endpoint> getEndpointTarget() { 896 if (this.endpointTarget == null) 897 this.endpointTarget = new ArrayList<Endpoint>(); 898 return this.endpointTarget; 899 } 900 901 /** 902 * @deprecated Use Reference#setResource(IBaseResource) instead 903 */ 904 @Deprecated 905 public Endpoint addEndpointTarget() { 906 Endpoint r = new Endpoint(); 907 if (this.endpointTarget == null) 908 this.endpointTarget = new ArrayList<Endpoint>(); 909 this.endpointTarget.add(r); 910 return r; 911 } 912 913 protected void listChildren(List<Property> children) { 914 super.listChildren(children); 915 children.add(new Property("identifier", "Identifier", "Business identifiers that are specific to this role.", 0, 916 java.lang.Integer.MAX_VALUE, identifier)); 917 children.add(new Property("active", "boolean", "Whether this organization affiliation record is in active use.", 0, 918 1, active)); 919 children.add(new Property("period", "Period", 920 "The period during which the participatingOrganization is affiliated with the primary organization.", 0, 1, 921 period)); 922 children.add(new Property("organization", "Reference(Organization)", 923 "Organization where the role is available (primary organization/has members).", 0, 1, organization)); 924 children.add(new Property("participatingOrganization", "Reference(Organization)", 925 "The Participating Organization provides/performs the role(s) defined by the code to the Primary Organization (e.g. providing services or is a member of).", 926 0, 1, participatingOrganization)); 927 children.add(new Property("network", "Reference(Organization)", 928 "Health insurance provider network in which the participatingOrganization provides the role's services (if defined) at the indicated locations (if defined).", 929 0, java.lang.Integer.MAX_VALUE, network)); 930 children.add(new Property("code", "CodeableConcept", 931 "Definition of the role the participatingOrganization plays in the association.", 0, 932 java.lang.Integer.MAX_VALUE, code)); 933 children.add(new Property("specialty", "CodeableConcept", 934 "Specific specialty of the participatingOrganization in the context of the role.", 0, 935 java.lang.Integer.MAX_VALUE, specialty)); 936 children.add(new Property("location", "Reference(Location)", "The location(s) at which the role occurs.", 0, 937 java.lang.Integer.MAX_VALUE, location)); 938 children.add(new Property("healthcareService", "Reference(HealthcareService)", 939 "Healthcare services provided through the role.", 0, java.lang.Integer.MAX_VALUE, healthcareService)); 940 children.add(new Property("telecom", "ContactPoint", 941 "Contact details at the participatingOrganization relevant to this Affiliation.", 0, 942 java.lang.Integer.MAX_VALUE, telecom)); 943 children.add(new Property("endpoint", "Reference(Endpoint)", 944 "Technical endpoints providing access to services operated for this role.", 0, java.lang.Integer.MAX_VALUE, 945 endpoint)); 946 } 947 948 @Override 949 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 950 switch (_hash) { 951 case -1618432855: 952 /* identifier */ return new Property("identifier", "Identifier", 953 "Business identifiers that are specific to this role.", 0, java.lang.Integer.MAX_VALUE, identifier); 954 case -1422950650: 955 /* active */ return new Property("active", "boolean", 956 "Whether this organization affiliation record is in active use.", 0, 1, active); 957 case -991726143: 958 /* period */ return new Property("period", "Period", 959 "The period during which the participatingOrganization is affiliated with the primary organization.", 0, 1, 960 period); 961 case 1178922291: 962 /* organization */ return new Property("organization", "Reference(Organization)", 963 "Organization where the role is available (primary organization/has members).", 0, 1, organization); 964 case 1593310702: 965 /* participatingOrganization */ return new Property("participatingOrganization", "Reference(Organization)", 966 "The Participating Organization provides/performs the role(s) defined by the code to the Primary Organization (e.g. providing services or is a member of).", 967 0, 1, participatingOrganization); 968 case 1843485230: 969 /* network */ return new Property("network", "Reference(Organization)", 970 "Health insurance provider network in which the participatingOrganization provides the role's services (if defined) at the indicated locations (if defined).", 971 0, java.lang.Integer.MAX_VALUE, network); 972 case 3059181: 973 /* code */ return new Property("code", "CodeableConcept", 974 "Definition of the role the participatingOrganization plays in the association.", 0, 975 java.lang.Integer.MAX_VALUE, code); 976 case -1694759682: 977 /* specialty */ return new Property("specialty", "CodeableConcept", 978 "Specific specialty of the participatingOrganization in the context of the role.", 0, 979 java.lang.Integer.MAX_VALUE, specialty); 980 case 1901043637: 981 /* location */ return new Property("location", "Reference(Location)", "The location(s) at which the role occurs.", 982 0, java.lang.Integer.MAX_VALUE, location); 983 case 1289661064: 984 /* healthcareService */ return new Property("healthcareService", "Reference(HealthcareService)", 985 "Healthcare services provided through the role.", 0, java.lang.Integer.MAX_VALUE, healthcareService); 986 case -1429363305: 987 /* telecom */ return new Property("telecom", "ContactPoint", 988 "Contact details at the participatingOrganization relevant to this Affiliation.", 0, 989 java.lang.Integer.MAX_VALUE, telecom); 990 case 1741102485: 991 /* endpoint */ return new Property("endpoint", "Reference(Endpoint)", 992 "Technical endpoints providing access to services operated for this role.", 0, java.lang.Integer.MAX_VALUE, 993 endpoint); 994 default: 995 return super.getNamedProperty(_hash, _name, _checkValid); 996 } 997 998 } 999 1000 @Override 1001 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1002 switch (hash) { 1003 case -1618432855: 1004 /* identifier */ return this.identifier == null ? new Base[0] 1005 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1006 case -1422950650: 1007 /* active */ return this.active == null ? new Base[0] : new Base[] { this.active }; // BooleanType 1008 case -991726143: 1009 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 1010 case 1178922291: 1011 /* organization */ return this.organization == null ? new Base[0] : new Base[] { this.organization }; // Reference 1012 case 1593310702: 1013 /* participatingOrganization */ return this.participatingOrganization == null ? new Base[0] 1014 : new Base[] { this.participatingOrganization }; // Reference 1015 case 1843485230: 1016 /* network */ return this.network == null ? new Base[0] : this.network.toArray(new Base[this.network.size()]); // Reference 1017 case 3059181: 1018 /* code */ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 1019 case -1694759682: 1020 /* specialty */ return this.specialty == null ? new Base[0] 1021 : this.specialty.toArray(new Base[this.specialty.size()]); // CodeableConcept 1022 case 1901043637: 1023 /* location */ return this.location == null ? new Base[0] : this.location.toArray(new Base[this.location.size()]); // Reference 1024 case 1289661064: 1025 /* healthcareService */ return this.healthcareService == null ? new Base[0] 1026 : this.healthcareService.toArray(new Base[this.healthcareService.size()]); // Reference 1027 case -1429363305: 1028 /* telecom */ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 1029 case 1741102485: 1030 /* endpoint */ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 1031 default: 1032 return super.getProperty(hash, name, checkValid); 1033 } 1034 1035 } 1036 1037 @Override 1038 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1039 switch (hash) { 1040 case -1618432855: // identifier 1041 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1042 return value; 1043 case -1422950650: // active 1044 this.active = castToBoolean(value); // BooleanType 1045 return value; 1046 case -991726143: // period 1047 this.period = castToPeriod(value); // Period 1048 return value; 1049 case 1178922291: // organization 1050 this.organization = castToReference(value); // Reference 1051 return value; 1052 case 1593310702: // participatingOrganization 1053 this.participatingOrganization = castToReference(value); // Reference 1054 return value; 1055 case 1843485230: // network 1056 this.getNetwork().add(castToReference(value)); // Reference 1057 return value; 1058 case 3059181: // code 1059 this.getCode().add(castToCodeableConcept(value)); // CodeableConcept 1060 return value; 1061 case -1694759682: // specialty 1062 this.getSpecialty().add(castToCodeableConcept(value)); // CodeableConcept 1063 return value; 1064 case 1901043637: // location 1065 this.getLocation().add(castToReference(value)); // Reference 1066 return value; 1067 case 1289661064: // healthcareService 1068 this.getHealthcareService().add(castToReference(value)); // Reference 1069 return value; 1070 case -1429363305: // telecom 1071 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 1072 return value; 1073 case 1741102485: // endpoint 1074 this.getEndpoint().add(castToReference(value)); // Reference 1075 return value; 1076 default: 1077 return super.setProperty(hash, name, value); 1078 } 1079 1080 } 1081 1082 @Override 1083 public Base setProperty(String name, Base value) throws FHIRException { 1084 if (name.equals("identifier")) { 1085 this.getIdentifier().add(castToIdentifier(value)); 1086 } else if (name.equals("active")) { 1087 this.active = castToBoolean(value); // BooleanType 1088 } else if (name.equals("period")) { 1089 this.period = castToPeriod(value); // Period 1090 } else if (name.equals("organization")) { 1091 this.organization = castToReference(value); // Reference 1092 } else if (name.equals("participatingOrganization")) { 1093 this.participatingOrganization = castToReference(value); // Reference 1094 } else if (name.equals("network")) { 1095 this.getNetwork().add(castToReference(value)); 1096 } else if (name.equals("code")) { 1097 this.getCode().add(castToCodeableConcept(value)); 1098 } else if (name.equals("specialty")) { 1099 this.getSpecialty().add(castToCodeableConcept(value)); 1100 } else if (name.equals("location")) { 1101 this.getLocation().add(castToReference(value)); 1102 } else if (name.equals("healthcareService")) { 1103 this.getHealthcareService().add(castToReference(value)); 1104 } else if (name.equals("telecom")) { 1105 this.getTelecom().add(castToContactPoint(value)); 1106 } else if (name.equals("endpoint")) { 1107 this.getEndpoint().add(castToReference(value)); 1108 } else 1109 return super.setProperty(name, value); 1110 return value; 1111 } 1112 1113 @Override 1114 public void removeChild(String name, Base value) throws FHIRException { 1115 if (name.equals("identifier")) { 1116 this.getIdentifier().remove(castToIdentifier(value)); 1117 } else if (name.equals("active")) { 1118 this.active = null; 1119 } else if (name.equals("period")) { 1120 this.period = null; 1121 } else if (name.equals("organization")) { 1122 this.organization = null; 1123 } else if (name.equals("participatingOrganization")) { 1124 this.participatingOrganization = null; 1125 } else if (name.equals("network")) { 1126 this.getNetwork().remove(castToReference(value)); 1127 } else if (name.equals("code")) { 1128 this.getCode().remove(castToCodeableConcept(value)); 1129 } else if (name.equals("specialty")) { 1130 this.getSpecialty().remove(castToCodeableConcept(value)); 1131 } else if (name.equals("location")) { 1132 this.getLocation().remove(castToReference(value)); 1133 } else if (name.equals("healthcareService")) { 1134 this.getHealthcareService().remove(castToReference(value)); 1135 } else if (name.equals("telecom")) { 1136 this.getTelecom().remove(castToContactPoint(value)); 1137 } else if (name.equals("endpoint")) { 1138 this.getEndpoint().remove(castToReference(value)); 1139 } else 1140 super.removeChild(name, value); 1141 1142 } 1143 1144 @Override 1145 public Base makeProperty(int hash, String name) throws FHIRException { 1146 switch (hash) { 1147 case -1618432855: 1148 return addIdentifier(); 1149 case -1422950650: 1150 return getActiveElement(); 1151 case -991726143: 1152 return getPeriod(); 1153 case 1178922291: 1154 return getOrganization(); 1155 case 1593310702: 1156 return getParticipatingOrganization(); 1157 case 1843485230: 1158 return addNetwork(); 1159 case 3059181: 1160 return addCode(); 1161 case -1694759682: 1162 return addSpecialty(); 1163 case 1901043637: 1164 return addLocation(); 1165 case 1289661064: 1166 return addHealthcareService(); 1167 case -1429363305: 1168 return addTelecom(); 1169 case 1741102485: 1170 return addEndpoint(); 1171 default: 1172 return super.makeProperty(hash, name); 1173 } 1174 1175 } 1176 1177 @Override 1178 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1179 switch (hash) { 1180 case -1618432855: 1181 /* identifier */ return new String[] { "Identifier" }; 1182 case -1422950650: 1183 /* active */ return new String[] { "boolean" }; 1184 case -991726143: 1185 /* period */ return new String[] { "Period" }; 1186 case 1178922291: 1187 /* organization */ return new String[] { "Reference" }; 1188 case 1593310702: 1189 /* participatingOrganization */ return new String[] { "Reference" }; 1190 case 1843485230: 1191 /* network */ return new String[] { "Reference" }; 1192 case 3059181: 1193 /* code */ return new String[] { "CodeableConcept" }; 1194 case -1694759682: 1195 /* specialty */ return new String[] { "CodeableConcept" }; 1196 case 1901043637: 1197 /* location */ return new String[] { "Reference" }; 1198 case 1289661064: 1199 /* healthcareService */ return new String[] { "Reference" }; 1200 case -1429363305: 1201 /* telecom */ return new String[] { "ContactPoint" }; 1202 case 1741102485: 1203 /* endpoint */ return new String[] { "Reference" }; 1204 default: 1205 return super.getTypesForProperty(hash, name); 1206 } 1207 1208 } 1209 1210 @Override 1211 public Base addChild(String name) throws FHIRException { 1212 if (name.equals("identifier")) { 1213 return addIdentifier(); 1214 } else if (name.equals("active")) { 1215 throw new FHIRException("Cannot call addChild on a singleton property OrganizationAffiliation.active"); 1216 } else if (name.equals("period")) { 1217 this.period = new Period(); 1218 return this.period; 1219 } else if (name.equals("organization")) { 1220 this.organization = new Reference(); 1221 return this.organization; 1222 } else if (name.equals("participatingOrganization")) { 1223 this.participatingOrganization = new Reference(); 1224 return this.participatingOrganization; 1225 } else if (name.equals("network")) { 1226 return addNetwork(); 1227 } else if (name.equals("code")) { 1228 return addCode(); 1229 } else if (name.equals("specialty")) { 1230 return addSpecialty(); 1231 } else if (name.equals("location")) { 1232 return addLocation(); 1233 } else if (name.equals("healthcareService")) { 1234 return addHealthcareService(); 1235 } else if (name.equals("telecom")) { 1236 return addTelecom(); 1237 } else if (name.equals("endpoint")) { 1238 return addEndpoint(); 1239 } else 1240 return super.addChild(name); 1241 } 1242 1243 public String fhirType() { 1244 return "OrganizationAffiliation"; 1245 1246 } 1247 1248 public OrganizationAffiliation copy() { 1249 OrganizationAffiliation dst = new OrganizationAffiliation(); 1250 copyValues(dst); 1251 return dst; 1252 } 1253 1254 public void copyValues(OrganizationAffiliation dst) { 1255 super.copyValues(dst); 1256 if (identifier != null) { 1257 dst.identifier = new ArrayList<Identifier>(); 1258 for (Identifier i : identifier) 1259 dst.identifier.add(i.copy()); 1260 } 1261 ; 1262 dst.active = active == null ? null : active.copy(); 1263 dst.period = period == null ? null : period.copy(); 1264 dst.organization = organization == null ? null : organization.copy(); 1265 dst.participatingOrganization = participatingOrganization == null ? null : participatingOrganization.copy(); 1266 if (network != null) { 1267 dst.network = new ArrayList<Reference>(); 1268 for (Reference i : network) 1269 dst.network.add(i.copy()); 1270 } 1271 ; 1272 if (code != null) { 1273 dst.code = new ArrayList<CodeableConcept>(); 1274 for (CodeableConcept i : code) 1275 dst.code.add(i.copy()); 1276 } 1277 ; 1278 if (specialty != null) { 1279 dst.specialty = new ArrayList<CodeableConcept>(); 1280 for (CodeableConcept i : specialty) 1281 dst.specialty.add(i.copy()); 1282 } 1283 ; 1284 if (location != null) { 1285 dst.location = new ArrayList<Reference>(); 1286 for (Reference i : location) 1287 dst.location.add(i.copy()); 1288 } 1289 ; 1290 if (healthcareService != null) { 1291 dst.healthcareService = new ArrayList<Reference>(); 1292 for (Reference i : healthcareService) 1293 dst.healthcareService.add(i.copy()); 1294 } 1295 ; 1296 if (telecom != null) { 1297 dst.telecom = new ArrayList<ContactPoint>(); 1298 for (ContactPoint i : telecom) 1299 dst.telecom.add(i.copy()); 1300 } 1301 ; 1302 if (endpoint != null) { 1303 dst.endpoint = new ArrayList<Reference>(); 1304 for (Reference i : endpoint) 1305 dst.endpoint.add(i.copy()); 1306 } 1307 ; 1308 } 1309 1310 protected OrganizationAffiliation typedCopy() { 1311 return copy(); 1312 } 1313 1314 @Override 1315 public boolean equalsDeep(Base other_) { 1316 if (!super.equalsDeep(other_)) 1317 return false; 1318 if (!(other_ instanceof OrganizationAffiliation)) 1319 return false; 1320 OrganizationAffiliation o = (OrganizationAffiliation) other_; 1321 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) 1322 && compareDeep(period, o.period, true) && compareDeep(organization, o.organization, true) 1323 && compareDeep(participatingOrganization, o.participatingOrganization, true) 1324 && compareDeep(network, o.network, true) && compareDeep(code, o.code, true) 1325 && compareDeep(specialty, o.specialty, true) && compareDeep(location, o.location, true) 1326 && compareDeep(healthcareService, o.healthcareService, true) && compareDeep(telecom, o.telecom, true) 1327 && compareDeep(endpoint, o.endpoint, true); 1328 } 1329 1330 @Override 1331 public boolean equalsShallow(Base other_) { 1332 if (!super.equalsShallow(other_)) 1333 return false; 1334 if (!(other_ instanceof OrganizationAffiliation)) 1335 return false; 1336 OrganizationAffiliation o = (OrganizationAffiliation) other_; 1337 return compareValues(active, o.active, true); 1338 } 1339 1340 public boolean isEmpty() { 1341 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, period, organization, 1342 participatingOrganization, network, code, specialty, location, healthcareService, telecom, endpoint); 1343 } 1344 1345 @Override 1346 public ResourceType getResourceType() { 1347 return ResourceType.OrganizationAffiliation; 1348 } 1349 1350 /** 1351 * Search parameter: <b>date</b> 1352 * <p> 1353 * Description: <b>The period during which the participatingOrganization is 1354 * affiliated with the primary organization</b><br> 1355 * Type: <b>date</b><br> 1356 * Path: <b>OrganizationAffiliation.period</b><br> 1357 * </p> 1358 */ 1359 @SearchParamDefinition(name = "date", path = "OrganizationAffiliation.period", description = "The period during which the participatingOrganization is affiliated with the primary organization", type = "date") 1360 public static final String SP_DATE = "date"; 1361 /** 1362 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1363 * <p> 1364 * Description: <b>The period during which the participatingOrganization is 1365 * affiliated with the primary organization</b><br> 1366 * Type: <b>date</b><br> 1367 * Path: <b>OrganizationAffiliation.period</b><br> 1368 * </p> 1369 */ 1370 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 1371 SP_DATE); 1372 1373 /** 1374 * Search parameter: <b>identifier</b> 1375 * <p> 1376 * Description: <b>An organization affiliation's Identifier</b><br> 1377 * Type: <b>token</b><br> 1378 * Path: <b>OrganizationAffiliation.identifier</b><br> 1379 * </p> 1380 */ 1381 @SearchParamDefinition(name = "identifier", path = "OrganizationAffiliation.identifier", description = "An organization affiliation's Identifier", type = "token") 1382 public static final String SP_IDENTIFIER = "identifier"; 1383 /** 1384 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1385 * <p> 1386 * Description: <b>An organization affiliation's Identifier</b><br> 1387 * Type: <b>token</b><br> 1388 * Path: <b>OrganizationAffiliation.identifier</b><br> 1389 * </p> 1390 */ 1391 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1392 SP_IDENTIFIER); 1393 1394 /** 1395 * Search parameter: <b>specialty</b> 1396 * <p> 1397 * Description: <b>Specific specialty of the participatingOrganization in the 1398 * context of the role</b><br> 1399 * Type: <b>token</b><br> 1400 * Path: <b>OrganizationAffiliation.specialty</b><br> 1401 * </p> 1402 */ 1403 @SearchParamDefinition(name = "specialty", path = "OrganizationAffiliation.specialty", description = "Specific specialty of the participatingOrganization in the context of the role", type = "token") 1404 public static final String SP_SPECIALTY = "specialty"; 1405 /** 1406 * <b>Fluent Client</b> search parameter constant for <b>specialty</b> 1407 * <p> 1408 * Description: <b>Specific specialty of the participatingOrganization in the 1409 * context of the role</b><br> 1410 * Type: <b>token</b><br> 1411 * Path: <b>OrganizationAffiliation.specialty</b><br> 1412 * </p> 1413 */ 1414 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SPECIALTY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1415 SP_SPECIALTY); 1416 1417 /** 1418 * Search parameter: <b>role</b> 1419 * <p> 1420 * Description: <b>Definition of the role the participatingOrganization 1421 * plays</b><br> 1422 * Type: <b>token</b><br> 1423 * Path: <b>OrganizationAffiliation.code</b><br> 1424 * </p> 1425 */ 1426 @SearchParamDefinition(name = "role", path = "OrganizationAffiliation.code", description = "Definition of the role the participatingOrganization plays", type = "token") 1427 public static final String SP_ROLE = "role"; 1428 /** 1429 * <b>Fluent Client</b> search parameter constant for <b>role</b> 1430 * <p> 1431 * Description: <b>Definition of the role the participatingOrganization 1432 * plays</b><br> 1433 * Type: <b>token</b><br> 1434 * Path: <b>OrganizationAffiliation.code</b><br> 1435 * </p> 1436 */ 1437 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ROLE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1438 SP_ROLE); 1439 1440 /** 1441 * Search parameter: <b>active</b> 1442 * <p> 1443 * Description: <b>Whether this organization affiliation record is in active 1444 * use</b><br> 1445 * Type: <b>token</b><br> 1446 * Path: <b>OrganizationAffiliation.active</b><br> 1447 * </p> 1448 */ 1449 @SearchParamDefinition(name = "active", path = "OrganizationAffiliation.active", description = "Whether this organization affiliation record is in active use", type = "token") 1450 public static final String SP_ACTIVE = "active"; 1451 /** 1452 * <b>Fluent Client</b> search parameter constant for <b>active</b> 1453 * <p> 1454 * Description: <b>Whether this organization affiliation record is in active 1455 * use</b><br> 1456 * Type: <b>token</b><br> 1457 * Path: <b>OrganizationAffiliation.active</b><br> 1458 * </p> 1459 */ 1460 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1461 SP_ACTIVE); 1462 1463 /** 1464 * Search parameter: <b>primary-organization</b> 1465 * <p> 1466 * Description: <b>The organization that receives the services from the 1467 * participating organization</b><br> 1468 * Type: <b>reference</b><br> 1469 * Path: <b>OrganizationAffiliation.organization</b><br> 1470 * </p> 1471 */ 1472 @SearchParamDefinition(name = "primary-organization", path = "OrganizationAffiliation.organization", description = "The organization that receives the services from the participating organization", type = "reference", target = { 1473 Organization.class }) 1474 public static final String SP_PRIMARY_ORGANIZATION = "primary-organization"; 1475 /** 1476 * <b>Fluent Client</b> search parameter constant for 1477 * <b>primary-organization</b> 1478 * <p> 1479 * Description: <b>The organization that receives the services from the 1480 * participating organization</b><br> 1481 * Type: <b>reference</b><br> 1482 * Path: <b>OrganizationAffiliation.organization</b><br> 1483 * </p> 1484 */ 1485 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRIMARY_ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1486 SP_PRIMARY_ORGANIZATION); 1487 1488 /** 1489 * Constant for fluent queries to be used to add include statements. Specifies 1490 * the path value of "<b>OrganizationAffiliation:primary-organization</b>". 1491 */ 1492 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRIMARY_ORGANIZATION = new ca.uhn.fhir.model.api.Include( 1493 "OrganizationAffiliation:primary-organization").toLocked(); 1494 1495 /** 1496 * Search parameter: <b>network</b> 1497 * <p> 1498 * Description: <b>Health insurance provider network in which the 1499 * participatingOrganization provides the role's services (if defined) at the 1500 * indicated locations (if defined)</b><br> 1501 * Type: <b>reference</b><br> 1502 * Path: <b>OrganizationAffiliation.network</b><br> 1503 * </p> 1504 */ 1505 @SearchParamDefinition(name = "network", path = "OrganizationAffiliation.network", description = "Health insurance provider network in which the participatingOrganization provides the role's services (if defined) at the indicated locations (if defined)", type = "reference", target = { 1506 Organization.class }) 1507 public static final String SP_NETWORK = "network"; 1508 /** 1509 * <b>Fluent Client</b> search parameter constant for <b>network</b> 1510 * <p> 1511 * Description: <b>Health insurance provider network in which the 1512 * participatingOrganization provides the role's services (if defined) at the 1513 * indicated locations (if defined)</b><br> 1514 * Type: <b>reference</b><br> 1515 * Path: <b>OrganizationAffiliation.network</b><br> 1516 * </p> 1517 */ 1518 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam NETWORK = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1519 SP_NETWORK); 1520 1521 /** 1522 * Constant for fluent queries to be used to add include statements. Specifies 1523 * the path value of "<b>OrganizationAffiliation:network</b>". 1524 */ 1525 public static final ca.uhn.fhir.model.api.Include INCLUDE_NETWORK = new ca.uhn.fhir.model.api.Include( 1526 "OrganizationAffiliation:network").toLocked(); 1527 1528 /** 1529 * Search parameter: <b>endpoint</b> 1530 * <p> 1531 * Description: <b>Technical endpoints providing access to services operated for 1532 * this role</b><br> 1533 * Type: <b>reference</b><br> 1534 * Path: <b>OrganizationAffiliation.endpoint</b><br> 1535 * </p> 1536 */ 1537 @SearchParamDefinition(name = "endpoint", path = "OrganizationAffiliation.endpoint", description = "Technical endpoints providing access to services operated for this role", type = "reference", target = { 1538 Endpoint.class }) 1539 public static final String SP_ENDPOINT = "endpoint"; 1540 /** 1541 * <b>Fluent Client</b> search parameter constant for <b>endpoint</b> 1542 * <p> 1543 * Description: <b>Technical endpoints providing access to services operated for 1544 * this role</b><br> 1545 * Type: <b>reference</b><br> 1546 * Path: <b>OrganizationAffiliation.endpoint</b><br> 1547 * </p> 1548 */ 1549 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENDPOINT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1550 SP_ENDPOINT); 1551 1552 /** 1553 * Constant for fluent queries to be used to add include statements. Specifies 1554 * the path value of "<b>OrganizationAffiliation:endpoint</b>". 1555 */ 1556 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENDPOINT = new ca.uhn.fhir.model.api.Include( 1557 "OrganizationAffiliation:endpoint").toLocked(); 1558 1559 /** 1560 * Search parameter: <b>phone</b> 1561 * <p> 1562 * Description: <b>A value in a phone contact</b><br> 1563 * Type: <b>token</b><br> 1564 * Path: <b>OrganizationAffiliation.telecom(system=phone)</b><br> 1565 * </p> 1566 */ 1567 @SearchParamDefinition(name = "phone", path = "OrganizationAffiliation.telecom.where(system='phone')", description = "A value in a phone contact", type = "token") 1568 public static final String SP_PHONE = "phone"; 1569 /** 1570 * <b>Fluent Client</b> search parameter constant for <b>phone</b> 1571 * <p> 1572 * Description: <b>A value in a phone contact</b><br> 1573 * Type: <b>token</b><br> 1574 * Path: <b>OrganizationAffiliation.telecom(system=phone)</b><br> 1575 * </p> 1576 */ 1577 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PHONE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1578 SP_PHONE); 1579 1580 /** 1581 * Search parameter: <b>service</b> 1582 * <p> 1583 * Description: <b>Healthcare services provided through the role</b><br> 1584 * Type: <b>reference</b><br> 1585 * Path: <b>OrganizationAffiliation.healthcareService</b><br> 1586 * </p> 1587 */ 1588 @SearchParamDefinition(name = "service", path = "OrganizationAffiliation.healthcareService", description = "Healthcare services provided through the role", type = "reference", target = { 1589 HealthcareService.class }) 1590 public static final String SP_SERVICE = "service"; 1591 /** 1592 * <b>Fluent Client</b> search parameter constant for <b>service</b> 1593 * <p> 1594 * Description: <b>Healthcare services provided through the role</b><br> 1595 * Type: <b>reference</b><br> 1596 * Path: <b>OrganizationAffiliation.healthcareService</b><br> 1597 * </p> 1598 */ 1599 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SERVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1600 SP_SERVICE); 1601 1602 /** 1603 * Constant for fluent queries to be used to add include statements. Specifies 1604 * the path value of "<b>OrganizationAffiliation:service</b>". 1605 */ 1606 public static final ca.uhn.fhir.model.api.Include INCLUDE_SERVICE = new ca.uhn.fhir.model.api.Include( 1607 "OrganizationAffiliation:service").toLocked(); 1608 1609 /** 1610 * Search parameter: <b>participating-organization</b> 1611 * <p> 1612 * Description: <b>The organization that provides services to the primary 1613 * organization</b><br> 1614 * Type: <b>reference</b><br> 1615 * Path: <b>OrganizationAffiliation.participatingOrganization</b><br> 1616 * </p> 1617 */ 1618 @SearchParamDefinition(name = "participating-organization", path = "OrganizationAffiliation.participatingOrganization", description = "The organization that provides services to the primary organization", type = "reference", target = { 1619 Organization.class }) 1620 public static final String SP_PARTICIPATING_ORGANIZATION = "participating-organization"; 1621 /** 1622 * <b>Fluent Client</b> search parameter constant for 1623 * <b>participating-organization</b> 1624 * <p> 1625 * Description: <b>The organization that provides services to the primary 1626 * organization</b><br> 1627 * Type: <b>reference</b><br> 1628 * Path: <b>OrganizationAffiliation.participatingOrganization</b><br> 1629 * </p> 1630 */ 1631 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTICIPATING_ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1632 SP_PARTICIPATING_ORGANIZATION); 1633 1634 /** 1635 * Constant for fluent queries to be used to add include statements. Specifies 1636 * the path value of 1637 * "<b>OrganizationAffiliation:participating-organization</b>". 1638 */ 1639 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTICIPATING_ORGANIZATION = new ca.uhn.fhir.model.api.Include( 1640 "OrganizationAffiliation:participating-organization").toLocked(); 1641 1642 /** 1643 * Search parameter: <b>telecom</b> 1644 * <p> 1645 * Description: <b>The value in any kind of contact</b><br> 1646 * Type: <b>token</b><br> 1647 * Path: <b>OrganizationAffiliation.telecom</b><br> 1648 * </p> 1649 */ 1650 @SearchParamDefinition(name = "telecom", path = "OrganizationAffiliation.telecom", description = "The value in any kind of contact", type = "token") 1651 public static final String SP_TELECOM = "telecom"; 1652 /** 1653 * <b>Fluent Client</b> search parameter constant for <b>telecom</b> 1654 * <p> 1655 * Description: <b>The value in any kind of contact</b><br> 1656 * Type: <b>token</b><br> 1657 * Path: <b>OrganizationAffiliation.telecom</b><br> 1658 * </p> 1659 */ 1660 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TELECOM = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1661 SP_TELECOM); 1662 1663 /** 1664 * Search parameter: <b>location</b> 1665 * <p> 1666 * Description: <b>The location(s) at which the role occurs</b><br> 1667 * Type: <b>reference</b><br> 1668 * Path: <b>OrganizationAffiliation.location</b><br> 1669 * </p> 1670 */ 1671 @SearchParamDefinition(name = "location", path = "OrganizationAffiliation.location", description = "The location(s) at which the role occurs", type = "reference", target = { 1672 Location.class }) 1673 public static final String SP_LOCATION = "location"; 1674 /** 1675 * <b>Fluent Client</b> search parameter constant for <b>location</b> 1676 * <p> 1677 * Description: <b>The location(s) at which the role occurs</b><br> 1678 * Type: <b>reference</b><br> 1679 * Path: <b>OrganizationAffiliation.location</b><br> 1680 * </p> 1681 */ 1682 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1683 SP_LOCATION); 1684 1685 /** 1686 * Constant for fluent queries to be used to add include statements. Specifies 1687 * the path value of "<b>OrganizationAffiliation:location</b>". 1688 */ 1689 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include( 1690 "OrganizationAffiliation:location").toLocked(); 1691 1692 /** 1693 * Search parameter: <b>email</b> 1694 * <p> 1695 * Description: <b>A value in an email contact</b><br> 1696 * Type: <b>token</b><br> 1697 * Path: <b>OrganizationAffiliation.telecom(system=email)</b><br> 1698 * </p> 1699 */ 1700 @SearchParamDefinition(name = "email", path = "OrganizationAffiliation.telecom.where(system='email')", description = "A value in an email contact", type = "token") 1701 public static final String SP_EMAIL = "email"; 1702 /** 1703 * <b>Fluent Client</b> search parameter constant for <b>email</b> 1704 * <p> 1705 * Description: <b>A value in an email contact</b><br> 1706 * Type: <b>token</b><br> 1707 * Path: <b>OrganizationAffiliation.telecom(system=email)</b><br> 1708 * </p> 1709 */ 1710 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EMAIL = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1711 SP_EMAIL); 1712 1713}