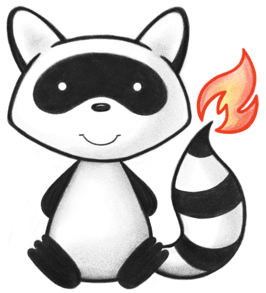
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.AdministrativeGender; 040import org.hl7.fhir.r4.model.Enumerations.AdministrativeGenderEnumFactory; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047 048/** 049 * Demographics and other administrative information about an individual or 050 * animal receiving care or other health-related services. 051 */ 052@ResourceDef(name = "Patient", profile = "http://hl7.org/fhir/StructureDefinition/Patient") 053public class Patient extends DomainResource { 054 055 public enum LinkType { 056 /** 057 * The patient resource containing this link must no longer be used. The link 058 * points forward to another patient resource that must be used in lieu of the 059 * patient resource that contains this link. 060 */ 061 REPLACEDBY, 062 /** 063 * The patient resource containing this link is the current active patient 064 * record. The link points back to an inactive patient resource that has been 065 * merged into this resource, and should be consulted to retrieve additional 066 * referenced information. 067 */ 068 REPLACES, 069 /** 070 * The patient resource containing this link is in use and valid but not 071 * considered the main source of information about a patient. The link points 072 * forward to another patient resource that should be consulted to retrieve 073 * additional patient information. 074 */ 075 REFER, 076 /** 077 * The patient resource containing this link is in use and valid, but points to 078 * another patient resource that is known to contain data about the same person. 079 * Data in this resource might overlap or contradict information found in the 080 * other patient resource. This link does not indicate any relative importance 081 * of the resources concerned, and both should be regarded as equally valid. 082 */ 083 SEEALSO, 084 /** 085 * added to help the parsers with the generic types 086 */ 087 NULL; 088 089 public static LinkType fromCode(String codeString) throws FHIRException { 090 if (codeString == null || "".equals(codeString)) 091 return null; 092 if ("replaced-by".equals(codeString)) 093 return REPLACEDBY; 094 if ("replaces".equals(codeString)) 095 return REPLACES; 096 if ("refer".equals(codeString)) 097 return REFER; 098 if ("seealso".equals(codeString)) 099 return SEEALSO; 100 if (Configuration.isAcceptInvalidEnums()) 101 return null; 102 else 103 throw new FHIRException("Unknown LinkType code '" + codeString + "'"); 104 } 105 106 public String toCode() { 107 switch (this) { 108 case REPLACEDBY: 109 return "replaced-by"; 110 case REPLACES: 111 return "replaces"; 112 case REFER: 113 return "refer"; 114 case SEEALSO: 115 return "seealso"; 116 case NULL: 117 return null; 118 default: 119 return "?"; 120 } 121 } 122 123 public String getSystem() { 124 switch (this) { 125 case REPLACEDBY: 126 return "http://hl7.org/fhir/link-type"; 127 case REPLACES: 128 return "http://hl7.org/fhir/link-type"; 129 case REFER: 130 return "http://hl7.org/fhir/link-type"; 131 case SEEALSO: 132 return "http://hl7.org/fhir/link-type"; 133 case NULL: 134 return null; 135 default: 136 return "?"; 137 } 138 } 139 140 public String getDefinition() { 141 switch (this) { 142 case REPLACEDBY: 143 return "The patient resource containing this link must no longer be used. The link points forward to another patient resource that must be used in lieu of the patient resource that contains this link."; 144 case REPLACES: 145 return "The patient resource containing this link is the current active patient record. The link points back to an inactive patient resource that has been merged into this resource, and should be consulted to retrieve additional referenced information."; 146 case REFER: 147 return "The patient resource containing this link is in use and valid but not considered the main source of information about a patient. The link points forward to another patient resource that should be consulted to retrieve additional patient information."; 148 case SEEALSO: 149 return "The patient resource containing this link is in use and valid, but points to another patient resource that is known to contain data about the same person. Data in this resource might overlap or contradict information found in the other patient resource. This link does not indicate any relative importance of the resources concerned, and both should be regarded as equally valid."; 150 case NULL: 151 return null; 152 default: 153 return "?"; 154 } 155 } 156 157 public String getDisplay() { 158 switch (this) { 159 case REPLACEDBY: 160 return "Replaced-by"; 161 case REPLACES: 162 return "Replaces"; 163 case REFER: 164 return "Refer"; 165 case SEEALSO: 166 return "See also"; 167 case NULL: 168 return null; 169 default: 170 return "?"; 171 } 172 } 173 } 174 175 public static class LinkTypeEnumFactory implements EnumFactory<LinkType> { 176 public LinkType fromCode(String codeString) throws IllegalArgumentException { 177 if (codeString == null || "".equals(codeString)) 178 if (codeString == null || "".equals(codeString)) 179 return null; 180 if ("replaced-by".equals(codeString)) 181 return LinkType.REPLACEDBY; 182 if ("replaces".equals(codeString)) 183 return LinkType.REPLACES; 184 if ("refer".equals(codeString)) 185 return LinkType.REFER; 186 if ("seealso".equals(codeString)) 187 return LinkType.SEEALSO; 188 throw new IllegalArgumentException("Unknown LinkType code '" + codeString + "'"); 189 } 190 191 public Enumeration<LinkType> fromType(PrimitiveType<?> code) throws FHIRException { 192 if (code == null) 193 return null; 194 if (code.isEmpty()) 195 return new Enumeration<LinkType>(this, LinkType.NULL, code); 196 String codeString = code.asStringValue(); 197 if (codeString == null || "".equals(codeString)) 198 return new Enumeration<LinkType>(this, LinkType.NULL, code); 199 if ("replaced-by".equals(codeString)) 200 return new Enumeration<LinkType>(this, LinkType.REPLACEDBY, code); 201 if ("replaces".equals(codeString)) 202 return new Enumeration<LinkType>(this, LinkType.REPLACES, code); 203 if ("refer".equals(codeString)) 204 return new Enumeration<LinkType>(this, LinkType.REFER, code); 205 if ("seealso".equals(codeString)) 206 return new Enumeration<LinkType>(this, LinkType.SEEALSO, code); 207 throw new FHIRException("Unknown LinkType code '" + codeString + "'"); 208 } 209 210 public String toCode(LinkType code) { 211 if (code == LinkType.NULL) 212 return null; 213 if (code == LinkType.REPLACEDBY) 214 return "replaced-by"; 215 if (code == LinkType.REPLACES) 216 return "replaces"; 217 if (code == LinkType.REFER) 218 return "refer"; 219 if (code == LinkType.SEEALSO) 220 return "seealso"; 221 return "?"; 222 } 223 224 public String toSystem(LinkType code) { 225 return code.getSystem(); 226 } 227 } 228 229 @Block() 230 public static class ContactComponent extends BackboneElement implements IBaseBackboneElement { 231 /** 232 * The nature of the relationship between the patient and the contact person. 233 */ 234 @Child(name = "relationship", type = { 235 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 236 @Description(shortDefinition = "The kind of relationship", formalDefinition = "The nature of the relationship between the patient and the contact person.") 237 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/patient-contactrelationship") 238 protected List<CodeableConcept> relationship; 239 240 /** 241 * A name associated with the contact person. 242 */ 243 @Child(name = "name", type = { HumanName.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 244 @Description(shortDefinition = "A name associated with the contact person", formalDefinition = "A name associated with the contact person.") 245 protected HumanName name; 246 247 /** 248 * A contact detail for the person, e.g. a telephone number or an email address. 249 */ 250 @Child(name = "telecom", type = { 251 ContactPoint.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 252 @Description(shortDefinition = "A contact detail for the person", formalDefinition = "A contact detail for the person, e.g. a telephone number or an email address.") 253 protected List<ContactPoint> telecom; 254 255 /** 256 * Address for the contact person. 257 */ 258 @Child(name = "address", type = { Address.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 259 @Description(shortDefinition = "Address for the contact person", formalDefinition = "Address for the contact person.") 260 protected Address address; 261 262 /** 263 * Administrative Gender - the gender that the contact person is considered to 264 * have for administration and record keeping purposes. 265 */ 266 @Child(name = "gender", type = { CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 267 @Description(shortDefinition = "male | female | other | unknown", formalDefinition = "Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes.") 268 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/administrative-gender") 269 protected Enumeration<AdministrativeGender> gender; 270 271 /** 272 * Organization on behalf of which the contact is acting or for which the 273 * contact is working. 274 */ 275 @Child(name = "organization", type = { 276 Organization.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 277 @Description(shortDefinition = "Organization that is associated with the contact", formalDefinition = "Organization on behalf of which the contact is acting or for which the contact is working.") 278 protected Reference organization; 279 280 /** 281 * The actual object that is the target of the reference (Organization on behalf 282 * of which the contact is acting or for which the contact is working.) 283 */ 284 protected Organization organizationTarget; 285 286 /** 287 * The period during which this contact person or organization is valid to be 288 * contacted relating to this patient. 289 */ 290 @Child(name = "period", type = { Period.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 291 @Description(shortDefinition = "The period during which this contact person or organization is valid to be contacted relating to this patient", formalDefinition = "The period during which this contact person or organization is valid to be contacted relating to this patient.") 292 protected Period period; 293 294 private static final long serialVersionUID = 364269017L; 295 296 /** 297 * Constructor 298 */ 299 public ContactComponent() { 300 super(); 301 } 302 303 /** 304 * @return {@link #relationship} (The nature of the relationship between the 305 * patient and the contact person.) 306 */ 307 public List<CodeableConcept> getRelationship() { 308 if (this.relationship == null) 309 this.relationship = new ArrayList<CodeableConcept>(); 310 return this.relationship; 311 } 312 313 /** 314 * @return Returns a reference to <code>this</code> for easy method chaining 315 */ 316 public ContactComponent setRelationship(List<CodeableConcept> theRelationship) { 317 this.relationship = theRelationship; 318 return this; 319 } 320 321 public boolean hasRelationship() { 322 if (this.relationship == null) 323 return false; 324 for (CodeableConcept item : this.relationship) 325 if (!item.isEmpty()) 326 return true; 327 return false; 328 } 329 330 public CodeableConcept addRelationship() { // 3 331 CodeableConcept t = new CodeableConcept(); 332 if (this.relationship == null) 333 this.relationship = new ArrayList<CodeableConcept>(); 334 this.relationship.add(t); 335 return t; 336 } 337 338 public ContactComponent addRelationship(CodeableConcept t) { // 3 339 if (t == null) 340 return this; 341 if (this.relationship == null) 342 this.relationship = new ArrayList<CodeableConcept>(); 343 this.relationship.add(t); 344 return this; 345 } 346 347 /** 348 * @return The first repetition of repeating field {@link #relationship}, 349 * creating it if it does not already exist 350 */ 351 public CodeableConcept getRelationshipFirstRep() { 352 if (getRelationship().isEmpty()) { 353 addRelationship(); 354 } 355 return getRelationship().get(0); 356 } 357 358 /** 359 * @return {@link #name} (A name associated with the contact person.) 360 */ 361 public HumanName getName() { 362 if (this.name == null) 363 if (Configuration.errorOnAutoCreate()) 364 throw new Error("Attempt to auto-create ContactComponent.name"); 365 else if (Configuration.doAutoCreate()) 366 this.name = new HumanName(); // cc 367 return this.name; 368 } 369 370 public boolean hasName() { 371 return this.name != null && !this.name.isEmpty(); 372 } 373 374 /** 375 * @param value {@link #name} (A name associated with the contact person.) 376 */ 377 public ContactComponent setName(HumanName value) { 378 this.name = value; 379 return this; 380 } 381 382 /** 383 * @return {@link #telecom} (A contact detail for the person, e.g. a telephone 384 * number or an email address.) 385 */ 386 public List<ContactPoint> getTelecom() { 387 if (this.telecom == null) 388 this.telecom = new ArrayList<ContactPoint>(); 389 return this.telecom; 390 } 391 392 /** 393 * @return Returns a reference to <code>this</code> for easy method chaining 394 */ 395 public ContactComponent setTelecom(List<ContactPoint> theTelecom) { 396 this.telecom = theTelecom; 397 return this; 398 } 399 400 public boolean hasTelecom() { 401 if (this.telecom == null) 402 return false; 403 for (ContactPoint item : this.telecom) 404 if (!item.isEmpty()) 405 return true; 406 return false; 407 } 408 409 public ContactPoint addTelecom() { // 3 410 ContactPoint t = new ContactPoint(); 411 if (this.telecom == null) 412 this.telecom = new ArrayList<ContactPoint>(); 413 this.telecom.add(t); 414 return t; 415 } 416 417 public ContactComponent addTelecom(ContactPoint t) { // 3 418 if (t == null) 419 return this; 420 if (this.telecom == null) 421 this.telecom = new ArrayList<ContactPoint>(); 422 this.telecom.add(t); 423 return this; 424 } 425 426 /** 427 * @return The first repetition of repeating field {@link #telecom}, creating it 428 * if it does not already exist 429 */ 430 public ContactPoint getTelecomFirstRep() { 431 if (getTelecom().isEmpty()) { 432 addTelecom(); 433 } 434 return getTelecom().get(0); 435 } 436 437 /** 438 * @return {@link #address} (Address for the contact person.) 439 */ 440 public Address getAddress() { 441 if (this.address == null) 442 if (Configuration.errorOnAutoCreate()) 443 throw new Error("Attempt to auto-create ContactComponent.address"); 444 else if (Configuration.doAutoCreate()) 445 this.address = new Address(); // cc 446 return this.address; 447 } 448 449 public boolean hasAddress() { 450 return this.address != null && !this.address.isEmpty(); 451 } 452 453 /** 454 * @param value {@link #address} (Address for the contact person.) 455 */ 456 public ContactComponent setAddress(Address value) { 457 this.address = value; 458 return this; 459 } 460 461 /** 462 * @return {@link #gender} (Administrative Gender - the gender that the contact 463 * person is considered to have for administration and record keeping 464 * purposes.). This is the underlying object with id, value and 465 * extensions. The accessor "getGender" gives direct access to the value 466 */ 467 public Enumeration<AdministrativeGender> getGenderElement() { 468 if (this.gender == null) 469 if (Configuration.errorOnAutoCreate()) 470 throw new Error("Attempt to auto-create ContactComponent.gender"); 471 else if (Configuration.doAutoCreate()) 472 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 473 return this.gender; 474 } 475 476 public boolean hasGenderElement() { 477 return this.gender != null && !this.gender.isEmpty(); 478 } 479 480 public boolean hasGender() { 481 return this.gender != null && !this.gender.isEmpty(); 482 } 483 484 /** 485 * @param value {@link #gender} (Administrative Gender - the gender that the 486 * contact person is considered to have for administration and 487 * record keeping purposes.). This is the underlying object with 488 * id, value and extensions. The accessor "getGender" gives direct 489 * access to the value 490 */ 491 public ContactComponent setGenderElement(Enumeration<AdministrativeGender> value) { 492 this.gender = value; 493 return this; 494 } 495 496 /** 497 * @return Administrative Gender - the gender that the contact person is 498 * considered to have for administration and record keeping purposes. 499 */ 500 public AdministrativeGender getGender() { 501 return this.gender == null ? null : this.gender.getValue(); 502 } 503 504 /** 505 * @param value Administrative Gender - the gender that the contact person is 506 * considered to have for administration and record keeping 507 * purposes. 508 */ 509 public ContactComponent setGender(AdministrativeGender value) { 510 if (value == null) 511 this.gender = null; 512 else { 513 if (this.gender == null) 514 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 515 this.gender.setValue(value); 516 } 517 return this; 518 } 519 520 /** 521 * @return {@link #organization} (Organization on behalf of which the contact is 522 * acting or for which the contact is working.) 523 */ 524 public Reference getOrganization() { 525 if (this.organization == null) 526 if (Configuration.errorOnAutoCreate()) 527 throw new Error("Attempt to auto-create ContactComponent.organization"); 528 else if (Configuration.doAutoCreate()) 529 this.organization = new Reference(); // cc 530 return this.organization; 531 } 532 533 public boolean hasOrganization() { 534 return this.organization != null && !this.organization.isEmpty(); 535 } 536 537 /** 538 * @param value {@link #organization} (Organization on behalf of which the 539 * contact is acting or for which the contact is working.) 540 */ 541 public ContactComponent setOrganization(Reference value) { 542 this.organization = value; 543 return this; 544 } 545 546 /** 547 * @return {@link #organization} The actual object that is the target of the 548 * reference. The reference library doesn't populate this, but you can 549 * use it to hold the resource if you resolve it. (Organization on 550 * behalf of which the contact is acting or for which the contact is 551 * working.) 552 */ 553 public Organization getOrganizationTarget() { 554 if (this.organizationTarget == null) 555 if (Configuration.errorOnAutoCreate()) 556 throw new Error("Attempt to auto-create ContactComponent.organization"); 557 else if (Configuration.doAutoCreate()) 558 this.organizationTarget = new Organization(); // aa 559 return this.organizationTarget; 560 } 561 562 /** 563 * @param value {@link #organization} The actual object that is the target of 564 * the reference. The reference library doesn't use these, but you 565 * can use it to hold the resource if you resolve it. (Organization 566 * on behalf of which the contact is acting or for which the 567 * contact is working.) 568 */ 569 public ContactComponent setOrganizationTarget(Organization value) { 570 this.organizationTarget = value; 571 return this; 572 } 573 574 /** 575 * @return {@link #period} (The period during which this contact person or 576 * organization is valid to be contacted relating to this patient.) 577 */ 578 public Period getPeriod() { 579 if (this.period == null) 580 if (Configuration.errorOnAutoCreate()) 581 throw new Error("Attempt to auto-create ContactComponent.period"); 582 else if (Configuration.doAutoCreate()) 583 this.period = new Period(); // cc 584 return this.period; 585 } 586 587 public boolean hasPeriod() { 588 return this.period != null && !this.period.isEmpty(); 589 } 590 591 /** 592 * @param value {@link #period} (The period during which this contact person or 593 * organization is valid to be contacted relating to this patient.) 594 */ 595 public ContactComponent setPeriod(Period value) { 596 this.period = value; 597 return this; 598 } 599 600 protected void listChildren(List<Property> children) { 601 super.listChildren(children); 602 children.add(new Property("relationship", "CodeableConcept", 603 "The nature of the relationship between the patient and the contact person.", 0, java.lang.Integer.MAX_VALUE, 604 relationship)); 605 children.add(new Property("name", "HumanName", "A name associated with the contact person.", 0, 1, name)); 606 children.add(new Property("telecom", "ContactPoint", 607 "A contact detail for the person, e.g. a telephone number or an email address.", 0, 608 java.lang.Integer.MAX_VALUE, telecom)); 609 children.add(new Property("address", "Address", "Address for the contact person.", 0, 1, address)); 610 children.add(new Property("gender", "code", 611 "Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes.", 612 0, 1, gender)); 613 children.add(new Property("organization", "Reference(Organization)", 614 "Organization on behalf of which the contact is acting or for which the contact is working.", 0, 1, 615 organization)); 616 children.add(new Property("period", "Period", 617 "The period during which this contact person or organization is valid to be contacted relating to this patient.", 618 0, 1, period)); 619 } 620 621 @Override 622 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 623 switch (_hash) { 624 case -261851592: 625 /* relationship */ return new Property("relationship", "CodeableConcept", 626 "The nature of the relationship between the patient and the contact person.", 0, 627 java.lang.Integer.MAX_VALUE, relationship); 628 case 3373707: 629 /* name */ return new Property("name", "HumanName", "A name associated with the contact person.", 0, 1, name); 630 case -1429363305: 631 /* telecom */ return new Property("telecom", "ContactPoint", 632 "A contact detail for the person, e.g. a telephone number or an email address.", 0, 633 java.lang.Integer.MAX_VALUE, telecom); 634 case -1147692044: 635 /* address */ return new Property("address", "Address", "Address for the contact person.", 0, 1, address); 636 case -1249512767: 637 /* gender */ return new Property("gender", "code", 638 "Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes.", 639 0, 1, gender); 640 case 1178922291: 641 /* organization */ return new Property("organization", "Reference(Organization)", 642 "Organization on behalf of which the contact is acting or for which the contact is working.", 0, 1, 643 organization); 644 case -991726143: 645 /* period */ return new Property("period", "Period", 646 "The period during which this contact person or organization is valid to be contacted relating to this patient.", 647 0, 1, period); 648 default: 649 return super.getNamedProperty(_hash, _name, _checkValid); 650 } 651 652 } 653 654 @Override 655 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 656 switch (hash) { 657 case -261851592: 658 /* relationship */ return this.relationship == null ? new Base[0] 659 : this.relationship.toArray(new Base[this.relationship.size()]); // CodeableConcept 660 case 3373707: 661 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // HumanName 662 case -1429363305: 663 /* telecom */ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 664 case -1147692044: 665 /* address */ return this.address == null ? new Base[0] : new Base[] { this.address }; // Address 666 case -1249512767: 667 /* gender */ return this.gender == null ? new Base[0] : new Base[] { this.gender }; // Enumeration<AdministrativeGender> 668 case 1178922291: 669 /* organization */ return this.organization == null ? new Base[0] : new Base[] { this.organization }; // Reference 670 case -991726143: 671 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 672 default: 673 return super.getProperty(hash, name, checkValid); 674 } 675 676 } 677 678 @Override 679 public Base setProperty(int hash, String name, Base value) throws FHIRException { 680 switch (hash) { 681 case -261851592: // relationship 682 this.getRelationship().add(castToCodeableConcept(value)); // CodeableConcept 683 return value; 684 case 3373707: // name 685 this.name = castToHumanName(value); // HumanName 686 return value; 687 case -1429363305: // telecom 688 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 689 return value; 690 case -1147692044: // address 691 this.address = castToAddress(value); // Address 692 return value; 693 case -1249512767: // gender 694 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 695 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 696 return value; 697 case 1178922291: // organization 698 this.organization = castToReference(value); // Reference 699 return value; 700 case -991726143: // period 701 this.period = castToPeriod(value); // Period 702 return value; 703 default: 704 return super.setProperty(hash, name, value); 705 } 706 707 } 708 709 @Override 710 public Base setProperty(String name, Base value) throws FHIRException { 711 if (name.equals("relationship")) { 712 this.getRelationship().add(castToCodeableConcept(value)); 713 } else if (name.equals("name")) { 714 this.name = castToHumanName(value); // HumanName 715 } else if (name.equals("telecom")) { 716 this.getTelecom().add(castToContactPoint(value)); 717 } else if (name.equals("address")) { 718 this.address = castToAddress(value); // Address 719 } else if (name.equals("gender")) { 720 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 721 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 722 } else if (name.equals("organization")) { 723 this.organization = castToReference(value); // Reference 724 } else if (name.equals("period")) { 725 this.period = castToPeriod(value); // Period 726 } else 727 return super.setProperty(name, value); 728 return value; 729 } 730 731 @Override 732 public void removeChild(String name, Base value) throws FHIRException { 733 if (name.equals("relationship")) { 734 this.getRelationship().remove(castToCodeableConcept(value)); 735 } else if (name.equals("name")) { 736 this.name = null; 737 } else if (name.equals("telecom")) { 738 this.getTelecom().remove(castToContactPoint(value)); 739 } else if (name.equals("address")) { 740 this.address = null; 741 } else if (name.equals("gender")) { 742 this.gender = null; 743 } else if (name.equals("organization")) { 744 this.organization = null; 745 } else if (name.equals("period")) { 746 this.period = null; 747 } else 748 super.removeChild(name, value); 749 750 } 751 752 @Override 753 public Base makeProperty(int hash, String name) throws FHIRException { 754 switch (hash) { 755 case -261851592: 756 return addRelationship(); 757 case 3373707: 758 return getName(); 759 case -1429363305: 760 return addTelecom(); 761 case -1147692044: 762 return getAddress(); 763 case -1249512767: 764 return getGenderElement(); 765 case 1178922291: 766 return getOrganization(); 767 case -991726143: 768 return getPeriod(); 769 default: 770 return super.makeProperty(hash, name); 771 } 772 773 } 774 775 @Override 776 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 777 switch (hash) { 778 case -261851592: 779 /* relationship */ return new String[] { "CodeableConcept" }; 780 case 3373707: 781 /* name */ return new String[] { "HumanName" }; 782 case -1429363305: 783 /* telecom */ return new String[] { "ContactPoint" }; 784 case -1147692044: 785 /* address */ return new String[] { "Address" }; 786 case -1249512767: 787 /* gender */ return new String[] { "code" }; 788 case 1178922291: 789 /* organization */ return new String[] { "Reference" }; 790 case -991726143: 791 /* period */ return new String[] { "Period" }; 792 default: 793 return super.getTypesForProperty(hash, name); 794 } 795 796 } 797 798 @Override 799 public Base addChild(String name) throws FHIRException { 800 if (name.equals("relationship")) { 801 return addRelationship(); 802 } else if (name.equals("name")) { 803 this.name = new HumanName(); 804 return this.name; 805 } else if (name.equals("telecom")) { 806 return addTelecom(); 807 } else if (name.equals("address")) { 808 this.address = new Address(); 809 return this.address; 810 } else if (name.equals("gender")) { 811 throw new FHIRException("Cannot call addChild on a singleton property Patient.gender"); 812 } else if (name.equals("organization")) { 813 this.organization = new Reference(); 814 return this.organization; 815 } else if (name.equals("period")) { 816 this.period = new Period(); 817 return this.period; 818 } else 819 return super.addChild(name); 820 } 821 822 public ContactComponent copy() { 823 ContactComponent dst = new ContactComponent(); 824 copyValues(dst); 825 return dst; 826 } 827 828 public void copyValues(ContactComponent dst) { 829 super.copyValues(dst); 830 if (relationship != null) { 831 dst.relationship = new ArrayList<CodeableConcept>(); 832 for (CodeableConcept i : relationship) 833 dst.relationship.add(i.copy()); 834 } 835 ; 836 dst.name = name == null ? null : name.copy(); 837 if (telecom != null) { 838 dst.telecom = new ArrayList<ContactPoint>(); 839 for (ContactPoint i : telecom) 840 dst.telecom.add(i.copy()); 841 } 842 ; 843 dst.address = address == null ? null : address.copy(); 844 dst.gender = gender == null ? null : gender.copy(); 845 dst.organization = organization == null ? null : organization.copy(); 846 dst.period = period == null ? null : period.copy(); 847 } 848 849 @Override 850 public boolean equalsDeep(Base other_) { 851 if (!super.equalsDeep(other_)) 852 return false; 853 if (!(other_ instanceof ContactComponent)) 854 return false; 855 ContactComponent o = (ContactComponent) other_; 856 return compareDeep(relationship, o.relationship, true) && compareDeep(name, o.name, true) 857 && compareDeep(telecom, o.telecom, true) && compareDeep(address, o.address, true) 858 && compareDeep(gender, o.gender, true) && compareDeep(organization, o.organization, true) 859 && compareDeep(period, o.period, true); 860 } 861 862 @Override 863 public boolean equalsShallow(Base other_) { 864 if (!super.equalsShallow(other_)) 865 return false; 866 if (!(other_ instanceof ContactComponent)) 867 return false; 868 ContactComponent o = (ContactComponent) other_; 869 return compareValues(gender, o.gender, true); 870 } 871 872 public boolean isEmpty() { 873 return super.isEmpty() 874 && ca.uhn.fhir.util.ElementUtil.isEmpty(relationship, name, telecom, address, gender, organization, period); 875 } 876 877 public String fhirType() { 878 return "Patient.contact"; 879 880 } 881 882 } 883 884 @Block() 885 public static class PatientCommunicationComponent extends BackboneElement implements IBaseBackboneElement { 886 /** 887 * The ISO-639-1 alpha 2 code in lower case for the language, optionally 888 * followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper 889 * case; e.g. "en" for English, or "en-US" for American English versus "en-EN" 890 * for England English. 891 */ 892 @Child(name = "language", type = { 893 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 894 @Description(shortDefinition = "The language which can be used to communicate with the patient about his or her health", formalDefinition = "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-EN\" for England English.") 895 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/languages") 896 protected CodeableConcept language; 897 898 /** 899 * Indicates whether or not the patient prefers this language (over other 900 * languages he masters up a certain level). 901 */ 902 @Child(name = "preferred", type = { 903 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 904 @Description(shortDefinition = "Language preference indicator", formalDefinition = "Indicates whether or not the patient prefers this language (over other languages he masters up a certain level).") 905 protected BooleanType preferred; 906 907 private static final long serialVersionUID = 633792918L; 908 909 /** 910 * Constructor 911 */ 912 public PatientCommunicationComponent() { 913 super(); 914 } 915 916 /** 917 * Constructor 918 */ 919 public PatientCommunicationComponent(CodeableConcept language) { 920 super(); 921 this.language = language; 922 } 923 924 /** 925 * @return {@link #language} (The ISO-639-1 alpha 2 code in lower case for the 926 * language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 927 * code for the region in upper case; e.g. "en" for English, or "en-US" 928 * for American English versus "en-EN" for England English.) 929 */ 930 public CodeableConcept getLanguage() { 931 if (this.language == null) 932 if (Configuration.errorOnAutoCreate()) 933 throw new Error("Attempt to auto-create PatientCommunicationComponent.language"); 934 else if (Configuration.doAutoCreate()) 935 this.language = new CodeableConcept(); // cc 936 return this.language; 937 } 938 939 public boolean hasLanguage() { 940 return this.language != null && !this.language.isEmpty(); 941 } 942 943 /** 944 * @param value {@link #language} (The ISO-639-1 alpha 2 code in lower case for 945 * the language, optionally followed by a hyphen and the ISO-3166-1 946 * alpha 2 code for the region in upper case; e.g. "en" for 947 * English, or "en-US" for American English versus "en-EN" for 948 * England English.) 949 */ 950 public PatientCommunicationComponent setLanguage(CodeableConcept value) { 951 this.language = value; 952 return this; 953 } 954 955 /** 956 * @return {@link #preferred} (Indicates whether or not the patient prefers this 957 * language (over other languages he masters up a certain level).). This 958 * is the underlying object with id, value and extensions. The accessor 959 * "getPreferred" gives direct access to the value 960 */ 961 public BooleanType getPreferredElement() { 962 if (this.preferred == null) 963 if (Configuration.errorOnAutoCreate()) 964 throw new Error("Attempt to auto-create PatientCommunicationComponent.preferred"); 965 else if (Configuration.doAutoCreate()) 966 this.preferred = new BooleanType(); // bb 967 return this.preferred; 968 } 969 970 public boolean hasPreferredElement() { 971 return this.preferred != null && !this.preferred.isEmpty(); 972 } 973 974 public boolean hasPreferred() { 975 return this.preferred != null && !this.preferred.isEmpty(); 976 } 977 978 /** 979 * @param value {@link #preferred} (Indicates whether or not the patient prefers 980 * this language (over other languages he masters up a certain 981 * level).). This is the underlying object with id, value and 982 * extensions. The accessor "getPreferred" gives direct access to 983 * the value 984 */ 985 public PatientCommunicationComponent setPreferredElement(BooleanType value) { 986 this.preferred = value; 987 return this; 988 } 989 990 /** 991 * @return Indicates whether or not the patient prefers this language (over 992 * other languages he masters up a certain level). 993 */ 994 public boolean getPreferred() { 995 return this.preferred == null || this.preferred.isEmpty() ? false : this.preferred.getValue(); 996 } 997 998 /** 999 * @param value Indicates whether or not the patient prefers this language (over 1000 * other languages he masters up a certain level). 1001 */ 1002 public PatientCommunicationComponent setPreferred(boolean value) { 1003 if (this.preferred == null) 1004 this.preferred = new BooleanType(); 1005 this.preferred.setValue(value); 1006 return this; 1007 } 1008 1009 protected void listChildren(List<Property> children) { 1010 super.listChildren(children); 1011 children.add(new Property("language", "CodeableConcept", 1012 "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-EN\" for England English.", 1013 0, 1, language)); 1014 children.add(new Property("preferred", "boolean", 1015 "Indicates whether or not the patient prefers this language (over other languages he masters up a certain level).", 1016 0, 1, preferred)); 1017 } 1018 1019 @Override 1020 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1021 switch (_hash) { 1022 case -1613589672: 1023 /* language */ return new Property("language", "CodeableConcept", 1024 "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-EN\" for England English.", 1025 0, 1, language); 1026 case -1294005119: 1027 /* preferred */ return new Property("preferred", "boolean", 1028 "Indicates whether or not the patient prefers this language (over other languages he masters up a certain level).", 1029 0, 1, preferred); 1030 default: 1031 return super.getNamedProperty(_hash, _name, _checkValid); 1032 } 1033 1034 } 1035 1036 @Override 1037 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1038 switch (hash) { 1039 case -1613589672: 1040 /* language */ return this.language == null ? new Base[0] : new Base[] { this.language }; // CodeableConcept 1041 case -1294005119: 1042 /* preferred */ return this.preferred == null ? new Base[0] : new Base[] { this.preferred }; // BooleanType 1043 default: 1044 return super.getProperty(hash, name, checkValid); 1045 } 1046 1047 } 1048 1049 @Override 1050 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1051 switch (hash) { 1052 case -1613589672: // language 1053 this.language = castToCodeableConcept(value); // CodeableConcept 1054 return value; 1055 case -1294005119: // preferred 1056 this.preferred = castToBoolean(value); // BooleanType 1057 return value; 1058 default: 1059 return super.setProperty(hash, name, value); 1060 } 1061 1062 } 1063 1064 @Override 1065 public Base setProperty(String name, Base value) throws FHIRException { 1066 if (name.equals("language")) { 1067 this.language = castToCodeableConcept(value); // CodeableConcept 1068 } else if (name.equals("preferred")) { 1069 this.preferred = castToBoolean(value); // BooleanType 1070 } else 1071 return super.setProperty(name, value); 1072 return value; 1073 } 1074 1075 @Override 1076 public void removeChild(String name, Base value) throws FHIRException { 1077 if (name.equals("language")) { 1078 this.language = null; 1079 } else if (name.equals("preferred")) { 1080 this.preferred = null; 1081 } else 1082 super.removeChild(name, value); 1083 1084 } 1085 1086 @Override 1087 public Base makeProperty(int hash, String name) throws FHIRException { 1088 switch (hash) { 1089 case -1613589672: 1090 return getLanguage(); 1091 case -1294005119: 1092 return getPreferredElement(); 1093 default: 1094 return super.makeProperty(hash, name); 1095 } 1096 1097 } 1098 1099 @Override 1100 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1101 switch (hash) { 1102 case -1613589672: 1103 /* language */ return new String[] { "CodeableConcept" }; 1104 case -1294005119: 1105 /* preferred */ return new String[] { "boolean" }; 1106 default: 1107 return super.getTypesForProperty(hash, name); 1108 } 1109 1110 } 1111 1112 @Override 1113 public Base addChild(String name) throws FHIRException { 1114 if (name.equals("language")) { 1115 this.language = new CodeableConcept(); 1116 return this.language; 1117 } else if (name.equals("preferred")) { 1118 throw new FHIRException("Cannot call addChild on a singleton property Patient.preferred"); 1119 } else 1120 return super.addChild(name); 1121 } 1122 1123 public PatientCommunicationComponent copy() { 1124 PatientCommunicationComponent dst = new PatientCommunicationComponent(); 1125 copyValues(dst); 1126 return dst; 1127 } 1128 1129 public void copyValues(PatientCommunicationComponent dst) { 1130 super.copyValues(dst); 1131 dst.language = language == null ? null : language.copy(); 1132 dst.preferred = preferred == null ? null : preferred.copy(); 1133 } 1134 1135 @Override 1136 public boolean equalsDeep(Base other_) { 1137 if (!super.equalsDeep(other_)) 1138 return false; 1139 if (!(other_ instanceof PatientCommunicationComponent)) 1140 return false; 1141 PatientCommunicationComponent o = (PatientCommunicationComponent) other_; 1142 return compareDeep(language, o.language, true) && compareDeep(preferred, o.preferred, true); 1143 } 1144 1145 @Override 1146 public boolean equalsShallow(Base other_) { 1147 if (!super.equalsShallow(other_)) 1148 return false; 1149 if (!(other_ instanceof PatientCommunicationComponent)) 1150 return false; 1151 PatientCommunicationComponent o = (PatientCommunicationComponent) other_; 1152 return compareValues(preferred, o.preferred, true); 1153 } 1154 1155 public boolean isEmpty() { 1156 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(language, preferred); 1157 } 1158 1159 public String fhirType() { 1160 return "Patient.communication"; 1161 1162 } 1163 1164 } 1165 1166 @Block() 1167 public static class PatientLinkComponent extends BackboneElement implements IBaseBackboneElement { 1168 /** 1169 * The other patient resource that the link refers to. 1170 */ 1171 @Child(name = "other", type = { Patient.class, 1172 RelatedPerson.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1173 @Description(shortDefinition = "The other patient or related person resource that the link refers to", formalDefinition = "The other patient resource that the link refers to.") 1174 protected Reference other; 1175 1176 /** 1177 * The actual object that is the target of the reference (The other patient 1178 * resource that the link refers to.) 1179 */ 1180 protected Resource otherTarget; 1181 1182 /** 1183 * The type of link between this patient resource and another patient resource. 1184 */ 1185 @Child(name = "type", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1186 @Description(shortDefinition = "replaced-by | replaces | refer | seealso", formalDefinition = "The type of link between this patient resource and another patient resource.") 1187 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/link-type") 1188 protected Enumeration<LinkType> type; 1189 1190 private static final long serialVersionUID = 1083576633L; 1191 1192 /** 1193 * Constructor 1194 */ 1195 public PatientLinkComponent() { 1196 super(); 1197 } 1198 1199 /** 1200 * Constructor 1201 */ 1202 public PatientLinkComponent(Reference other, Enumeration<LinkType> type) { 1203 super(); 1204 this.other = other; 1205 this.type = type; 1206 } 1207 1208 /** 1209 * @return {@link #other} (The other patient resource that the link refers to.) 1210 */ 1211 public Reference getOther() { 1212 if (this.other == null) 1213 if (Configuration.errorOnAutoCreate()) 1214 throw new Error("Attempt to auto-create PatientLinkComponent.other"); 1215 else if (Configuration.doAutoCreate()) 1216 this.other = new Reference(); // cc 1217 return this.other; 1218 } 1219 1220 public boolean hasOther() { 1221 return this.other != null && !this.other.isEmpty(); 1222 } 1223 1224 /** 1225 * @param value {@link #other} (The other patient resource that the link refers 1226 * to.) 1227 */ 1228 public PatientLinkComponent setOther(Reference value) { 1229 this.other = value; 1230 return this; 1231 } 1232 1233 /** 1234 * @return {@link #other} The actual object that is the target of the reference. 1235 * The reference library doesn't populate this, but you can use it to 1236 * hold the resource if you resolve it. (The other patient resource that 1237 * the link refers to.) 1238 */ 1239 public Resource getOtherTarget() { 1240 return this.otherTarget; 1241 } 1242 1243 /** 1244 * @param value {@link #other} The actual object that is the target of the 1245 * reference. The reference library doesn't use these, but you can 1246 * use it to hold the resource if you resolve it. (The other 1247 * patient resource that the link refers to.) 1248 */ 1249 public PatientLinkComponent setOtherTarget(Resource value) { 1250 this.otherTarget = value; 1251 return this; 1252 } 1253 1254 /** 1255 * @return {@link #type} (The type of link between this patient resource and 1256 * another patient resource.). This is the underlying object with id, 1257 * value and extensions. The accessor "getType" gives direct access to 1258 * the value 1259 */ 1260 public Enumeration<LinkType> getTypeElement() { 1261 if (this.type == null) 1262 if (Configuration.errorOnAutoCreate()) 1263 throw new Error("Attempt to auto-create PatientLinkComponent.type"); 1264 else if (Configuration.doAutoCreate()) 1265 this.type = new Enumeration<LinkType>(new LinkTypeEnumFactory()); // bb 1266 return this.type; 1267 } 1268 1269 public boolean hasTypeElement() { 1270 return this.type != null && !this.type.isEmpty(); 1271 } 1272 1273 public boolean hasType() { 1274 return this.type != null && !this.type.isEmpty(); 1275 } 1276 1277 /** 1278 * @param value {@link #type} (The type of link between this patient resource 1279 * and another patient resource.). This is the underlying object 1280 * with id, value and extensions. The accessor "getType" gives 1281 * direct access to the value 1282 */ 1283 public PatientLinkComponent setTypeElement(Enumeration<LinkType> value) { 1284 this.type = value; 1285 return this; 1286 } 1287 1288 /** 1289 * @return The type of link between this patient resource and another patient 1290 * resource. 1291 */ 1292 public LinkType getType() { 1293 return this.type == null ? null : this.type.getValue(); 1294 } 1295 1296 /** 1297 * @param value The type of link between this patient resource and another 1298 * patient resource. 1299 */ 1300 public PatientLinkComponent setType(LinkType value) { 1301 if (this.type == null) 1302 this.type = new Enumeration<LinkType>(new LinkTypeEnumFactory()); 1303 this.type.setValue(value); 1304 return this; 1305 } 1306 1307 protected void listChildren(List<Property> children) { 1308 super.listChildren(children); 1309 children.add(new Property("other", "Reference(Patient|RelatedPerson)", 1310 "The other patient resource that the link refers to.", 0, 1, other)); 1311 children.add(new Property("type", "code", 1312 "The type of link between this patient resource and another patient resource.", 0, 1, type)); 1313 } 1314 1315 @Override 1316 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1317 switch (_hash) { 1318 case 106069776: 1319 /* other */ return new Property("other", "Reference(Patient|RelatedPerson)", 1320 "The other patient resource that the link refers to.", 0, 1, other); 1321 case 3575610: 1322 /* type */ return new Property("type", "code", 1323 "The type of link between this patient resource and another patient resource.", 0, 1, type); 1324 default: 1325 return super.getNamedProperty(_hash, _name, _checkValid); 1326 } 1327 1328 } 1329 1330 @Override 1331 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1332 switch (hash) { 1333 case 106069776: 1334 /* other */ return this.other == null ? new Base[0] : new Base[] { this.other }; // Reference 1335 case 3575610: 1336 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<LinkType> 1337 default: 1338 return super.getProperty(hash, name, checkValid); 1339 } 1340 1341 } 1342 1343 @Override 1344 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1345 switch (hash) { 1346 case 106069776: // other 1347 this.other = castToReference(value); // Reference 1348 return value; 1349 case 3575610: // type 1350 value = new LinkTypeEnumFactory().fromType(castToCode(value)); 1351 this.type = (Enumeration) value; // Enumeration<LinkType> 1352 return value; 1353 default: 1354 return super.setProperty(hash, name, value); 1355 } 1356 1357 } 1358 1359 @Override 1360 public Base setProperty(String name, Base value) throws FHIRException { 1361 if (name.equals("other")) { 1362 this.other = castToReference(value); // Reference 1363 } else if (name.equals("type")) { 1364 value = new LinkTypeEnumFactory().fromType(castToCode(value)); 1365 this.type = (Enumeration) value; // Enumeration<LinkType> 1366 } else 1367 return super.setProperty(name, value); 1368 return value; 1369 } 1370 1371 @Override 1372 public void removeChild(String name, Base value) throws FHIRException { 1373 if (name.equals("other")) { 1374 this.other = null; 1375 } else if (name.equals("type")) { 1376 this.type = null; 1377 } else 1378 super.removeChild(name, value); 1379 1380 } 1381 1382 @Override 1383 public Base makeProperty(int hash, String name) throws FHIRException { 1384 switch (hash) { 1385 case 106069776: 1386 return getOther(); 1387 case 3575610: 1388 return getTypeElement(); 1389 default: 1390 return super.makeProperty(hash, name); 1391 } 1392 1393 } 1394 1395 @Override 1396 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1397 switch (hash) { 1398 case 106069776: 1399 /* other */ return new String[] { "Reference" }; 1400 case 3575610: 1401 /* type */ return new String[] { "code" }; 1402 default: 1403 return super.getTypesForProperty(hash, name); 1404 } 1405 1406 } 1407 1408 @Override 1409 public Base addChild(String name) throws FHIRException { 1410 if (name.equals("other")) { 1411 this.other = new Reference(); 1412 return this.other; 1413 } else if (name.equals("type")) { 1414 throw new FHIRException("Cannot call addChild on a singleton property Patient.type"); 1415 } else 1416 return super.addChild(name); 1417 } 1418 1419 public PatientLinkComponent copy() { 1420 PatientLinkComponent dst = new PatientLinkComponent(); 1421 copyValues(dst); 1422 return dst; 1423 } 1424 1425 public void copyValues(PatientLinkComponent dst) { 1426 super.copyValues(dst); 1427 dst.other = other == null ? null : other.copy(); 1428 dst.type = type == null ? null : type.copy(); 1429 } 1430 1431 @Override 1432 public boolean equalsDeep(Base other_) { 1433 if (!super.equalsDeep(other_)) 1434 return false; 1435 if (!(other_ instanceof PatientLinkComponent)) 1436 return false; 1437 PatientLinkComponent o = (PatientLinkComponent) other_; 1438 return compareDeep(other, o.other, true) && compareDeep(type, o.type, true); 1439 } 1440 1441 @Override 1442 public boolean equalsShallow(Base other_) { 1443 if (!super.equalsShallow(other_)) 1444 return false; 1445 if (!(other_ instanceof PatientLinkComponent)) 1446 return false; 1447 PatientLinkComponent o = (PatientLinkComponent) other_; 1448 return compareValues(type, o.type, true); 1449 } 1450 1451 public boolean isEmpty() { 1452 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(other, type); 1453 } 1454 1455 public String fhirType() { 1456 return "Patient.link"; 1457 1458 } 1459 1460 } 1461 1462 /** 1463 * An identifier for this patient. 1464 */ 1465 @Child(name = "identifier", type = { 1466 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1467 @Description(shortDefinition = "An identifier for this patient", formalDefinition = "An identifier for this patient.") 1468 protected List<Identifier> identifier; 1469 1470 /** 1471 * Whether this patient record is in active use. Many systems use this property 1472 * to mark as non-current patients, such as those that have not been seen for a 1473 * period of time based on an organization's business rules. 1474 * 1475 * It is often used to filter patient lists to exclude inactive patients 1476 * 1477 * Deceased patients may also be marked as inactive for the same reasons, but 1478 * may be active for some time after death. 1479 */ 1480 @Child(name = "active", type = { BooleanType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 1481 @Description(shortDefinition = "Whether this patient's record is in active use", formalDefinition = "Whether this patient record is in active use. \nMany systems use this property to mark as non-current patients, such as those that have not been seen for a period of time based on an organization's business rules.\n\nIt is often used to filter patient lists to exclude inactive patients\n\nDeceased patients may also be marked as inactive for the same reasons, but may be active for some time after death.") 1482 protected BooleanType active; 1483 1484 /** 1485 * A name associated with the individual. 1486 */ 1487 @Child(name = "name", type = { 1488 HumanName.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1489 @Description(shortDefinition = "A name associated with the patient", formalDefinition = "A name associated with the individual.") 1490 protected List<HumanName> name; 1491 1492 /** 1493 * A contact detail (e.g. a telephone number or an email address) by which the 1494 * individual may be contacted. 1495 */ 1496 @Child(name = "telecom", type = { 1497 ContactPoint.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1498 @Description(shortDefinition = "A contact detail for the individual", formalDefinition = "A contact detail (e.g. a telephone number or an email address) by which the individual may be contacted.") 1499 protected List<ContactPoint> telecom; 1500 1501 /** 1502 * Administrative Gender - the gender that the patient is considered to have for 1503 * administration and record keeping purposes. 1504 */ 1505 @Child(name = "gender", type = { CodeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1506 @Description(shortDefinition = "male | female | other | unknown", formalDefinition = "Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes.") 1507 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/administrative-gender") 1508 protected Enumeration<AdministrativeGender> gender; 1509 1510 /** 1511 * The date of birth for the individual. 1512 */ 1513 @Child(name = "birthDate", type = { DateType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1514 @Description(shortDefinition = "The date of birth for the individual", formalDefinition = "The date of birth for the individual.") 1515 protected DateType birthDate; 1516 1517 /** 1518 * Indicates if the individual is deceased or not. 1519 */ 1520 @Child(name = "deceased", type = { BooleanType.class, 1521 DateTimeType.class }, order = 6, min = 0, max = 1, modifier = true, summary = true) 1522 @Description(shortDefinition = "Indicates if the individual is deceased or not", formalDefinition = "Indicates if the individual is deceased or not.") 1523 protected Type deceased; 1524 1525 /** 1526 * An address for the individual. 1527 */ 1528 @Child(name = "address", type = { 1529 Address.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1530 @Description(shortDefinition = "An address for the individual", formalDefinition = "An address for the individual.") 1531 protected List<Address> address; 1532 1533 /** 1534 * This field contains a patient's most recent marital (civil) status. 1535 */ 1536 @Child(name = "maritalStatus", type = { 1537 CodeableConcept.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 1538 @Description(shortDefinition = "Marital (civil) status of a patient", formalDefinition = "This field contains a patient's most recent marital (civil) status.") 1539 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/marital-status") 1540 protected CodeableConcept maritalStatus; 1541 1542 /** 1543 * Indicates whether the patient is part of a multiple (boolean) or indicates 1544 * the actual birth order (integer). 1545 */ 1546 @Child(name = "multipleBirth", type = { BooleanType.class, 1547 IntegerType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1548 @Description(shortDefinition = "Whether patient is part of a multiple birth", formalDefinition = "Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer).") 1549 protected Type multipleBirth; 1550 1551 /** 1552 * Image of the patient. 1553 */ 1554 @Child(name = "photo", type = { 1555 Attachment.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1556 @Description(shortDefinition = "Image of the patient", formalDefinition = "Image of the patient.") 1557 protected List<Attachment> photo; 1558 1559 /** 1560 * A contact party (e.g. guardian, partner, friend) for the patient. 1561 */ 1562 @Child(name = "contact", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1563 @Description(shortDefinition = "A contact party (e.g. guardian, partner, friend) for the patient", formalDefinition = "A contact party (e.g. guardian, partner, friend) for the patient.") 1564 protected List<ContactComponent> contact; 1565 1566 /** 1567 * A language which may be used to communicate with the patient about his or her 1568 * health. 1569 */ 1570 @Child(name = "communication", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1571 @Description(shortDefinition = "A language which may be used to communicate with the patient about his or her health", formalDefinition = "A language which may be used to communicate with the patient about his or her health.") 1572 protected List<PatientCommunicationComponent> communication; 1573 1574 /** 1575 * Patient's nominated care provider. 1576 */ 1577 @Child(name = "generalPractitioner", type = { Organization.class, Practitioner.class, 1578 PractitionerRole.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1579 @Description(shortDefinition = "Patient's nominated primary care provider", formalDefinition = "Patient's nominated care provider.") 1580 protected List<Reference> generalPractitioner; 1581 /** 1582 * The actual objects that are the target of the reference (Patient's nominated 1583 * care provider.) 1584 */ 1585 protected List<Resource> generalPractitionerTarget; 1586 1587 /** 1588 * Organization that is the custodian of the patient record. 1589 */ 1590 @Child(name = "managingOrganization", type = { 1591 Organization.class }, order = 14, min = 0, max = 1, modifier = false, summary = true) 1592 @Description(shortDefinition = "Organization that is the custodian of the patient record", formalDefinition = "Organization that is the custodian of the patient record.") 1593 protected Reference managingOrganization; 1594 1595 /** 1596 * The actual object that is the target of the reference (Organization that is 1597 * the custodian of the patient record.) 1598 */ 1599 protected Organization managingOrganizationTarget; 1600 1601 /** 1602 * Link to another patient resource that concerns the same actual patient. 1603 */ 1604 @Child(name = "link", type = {}, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = true, summary = true) 1605 @Description(shortDefinition = "Link to another patient resource that concerns the same actual person", formalDefinition = "Link to another patient resource that concerns the same actual patient.") 1606 protected List<PatientLinkComponent> link; 1607 1608 private static final long serialVersionUID = 2138656939L; 1609 1610 /** 1611 * Constructor 1612 */ 1613 public Patient() { 1614 super(); 1615 } 1616 1617 /** 1618 * @return {@link #identifier} (An identifier for this patient.) 1619 */ 1620 public List<Identifier> getIdentifier() { 1621 if (this.identifier == null) 1622 this.identifier = new ArrayList<Identifier>(); 1623 return this.identifier; 1624 } 1625 1626 /** 1627 * @return Returns a reference to <code>this</code> for easy method chaining 1628 */ 1629 public Patient setIdentifier(List<Identifier> theIdentifier) { 1630 this.identifier = theIdentifier; 1631 return this; 1632 } 1633 1634 public boolean hasIdentifier() { 1635 if (this.identifier == null) 1636 return false; 1637 for (Identifier item : this.identifier) 1638 if (!item.isEmpty()) 1639 return true; 1640 return false; 1641 } 1642 1643 public Identifier addIdentifier() { // 3 1644 Identifier t = new Identifier(); 1645 if (this.identifier == null) 1646 this.identifier = new ArrayList<Identifier>(); 1647 this.identifier.add(t); 1648 return t; 1649 } 1650 1651 public Patient addIdentifier(Identifier t) { // 3 1652 if (t == null) 1653 return this; 1654 if (this.identifier == null) 1655 this.identifier = new ArrayList<Identifier>(); 1656 this.identifier.add(t); 1657 return this; 1658 } 1659 1660 /** 1661 * @return The first repetition of repeating field {@link #identifier}, creating 1662 * it if it does not already exist 1663 */ 1664 public Identifier getIdentifierFirstRep() { 1665 if (getIdentifier().isEmpty()) { 1666 addIdentifier(); 1667 } 1668 return getIdentifier().get(0); 1669 } 1670 1671 /** 1672 * @return {@link #active} (Whether this patient record is in active use. Many 1673 * systems use this property to mark as non-current patients, such as 1674 * those that have not been seen for a period of time based on an 1675 * organization's business rules. 1676 * 1677 * It is often used to filter patient lists to exclude inactive patients 1678 * 1679 * Deceased patients may also be marked as inactive for the same 1680 * reasons, but may be active for some time after death.). This is the 1681 * underlying object with id, value and extensions. The accessor 1682 * "getActive" gives direct access to the value 1683 */ 1684 public BooleanType getActiveElement() { 1685 if (this.active == null) 1686 if (Configuration.errorOnAutoCreate()) 1687 throw new Error("Attempt to auto-create Patient.active"); 1688 else if (Configuration.doAutoCreate()) 1689 this.active = new BooleanType(); // bb 1690 return this.active; 1691 } 1692 1693 public boolean hasActiveElement() { 1694 return this.active != null && !this.active.isEmpty(); 1695 } 1696 1697 public boolean hasActive() { 1698 return this.active != null && !this.active.isEmpty(); 1699 } 1700 1701 /** 1702 * @param value {@link #active} (Whether this patient record is in active use. 1703 * Many systems use this property to mark as non-current patients, 1704 * such as those that have not been seen for a period of time based 1705 * on an organization's business rules. 1706 * 1707 * It is often used to filter patient lists to exclude inactive 1708 * patients 1709 * 1710 * Deceased patients may also be marked as inactive for the same 1711 * reasons, but may be active for some time after death.). This is 1712 * the underlying object with id, value and extensions. The 1713 * accessor "getActive" gives direct access to the value 1714 */ 1715 public Patient setActiveElement(BooleanType value) { 1716 this.active = value; 1717 return this; 1718 } 1719 1720 /** 1721 * @return Whether this patient record is in active use. Many systems use this 1722 * property to mark as non-current patients, such as those that have not 1723 * been seen for a period of time based on an organization's business 1724 * rules. 1725 * 1726 * It is often used to filter patient lists to exclude inactive patients 1727 * 1728 * Deceased patients may also be marked as inactive for the same 1729 * reasons, but may be active for some time after death. 1730 */ 1731 public boolean getActive() { 1732 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 1733 } 1734 1735 /** 1736 * @param value Whether this patient record is in active use. Many systems use 1737 * this property to mark as non-current patients, such as those 1738 * that have not been seen for a period of time based on an 1739 * organization's business rules. 1740 * 1741 * It is often used to filter patient lists to exclude inactive 1742 * patients 1743 * 1744 * Deceased patients may also be marked as inactive for the same 1745 * reasons, but may be active for some time after death. 1746 */ 1747 public Patient setActive(boolean value) { 1748 if (this.active == null) 1749 this.active = new BooleanType(); 1750 this.active.setValue(value); 1751 return this; 1752 } 1753 1754 /** 1755 * @return {@link #name} (A name associated with the individual.) 1756 */ 1757 public List<HumanName> getName() { 1758 if (this.name == null) 1759 this.name = new ArrayList<HumanName>(); 1760 return this.name; 1761 } 1762 1763 /** 1764 * @return Returns a reference to <code>this</code> for easy method chaining 1765 */ 1766 public Patient setName(List<HumanName> theName) { 1767 this.name = theName; 1768 return this; 1769 } 1770 1771 public boolean hasName() { 1772 if (this.name == null) 1773 return false; 1774 for (HumanName item : this.name) 1775 if (!item.isEmpty()) 1776 return true; 1777 return false; 1778 } 1779 1780 public HumanName addName() { // 3 1781 HumanName t = new HumanName(); 1782 if (this.name == null) 1783 this.name = new ArrayList<HumanName>(); 1784 this.name.add(t); 1785 return t; 1786 } 1787 1788 public Patient addName(HumanName t) { // 3 1789 if (t == null) 1790 return this; 1791 if (this.name == null) 1792 this.name = new ArrayList<HumanName>(); 1793 this.name.add(t); 1794 return this; 1795 } 1796 1797 /** 1798 * @return The first repetition of repeating field {@link #name}, creating it if 1799 * it does not already exist 1800 */ 1801 public HumanName getNameFirstRep() { 1802 if (getName().isEmpty()) { 1803 addName(); 1804 } 1805 return getName().get(0); 1806 } 1807 1808 /** 1809 * @return {@link #telecom} (A contact detail (e.g. a telephone number or an 1810 * email address) by which the individual may be contacted.) 1811 */ 1812 public List<ContactPoint> getTelecom() { 1813 if (this.telecom == null) 1814 this.telecom = new ArrayList<ContactPoint>(); 1815 return this.telecom; 1816 } 1817 1818 /** 1819 * @return Returns a reference to <code>this</code> for easy method chaining 1820 */ 1821 public Patient setTelecom(List<ContactPoint> theTelecom) { 1822 this.telecom = theTelecom; 1823 return this; 1824 } 1825 1826 public boolean hasTelecom() { 1827 if (this.telecom == null) 1828 return false; 1829 for (ContactPoint item : this.telecom) 1830 if (!item.isEmpty()) 1831 return true; 1832 return false; 1833 } 1834 1835 public ContactPoint addTelecom() { // 3 1836 ContactPoint t = new ContactPoint(); 1837 if (this.telecom == null) 1838 this.telecom = new ArrayList<ContactPoint>(); 1839 this.telecom.add(t); 1840 return t; 1841 } 1842 1843 public Patient addTelecom(ContactPoint t) { // 3 1844 if (t == null) 1845 return this; 1846 if (this.telecom == null) 1847 this.telecom = new ArrayList<ContactPoint>(); 1848 this.telecom.add(t); 1849 return this; 1850 } 1851 1852 /** 1853 * @return The first repetition of repeating field {@link #telecom}, creating it 1854 * if it does not already exist 1855 */ 1856 public ContactPoint getTelecomFirstRep() { 1857 if (getTelecom().isEmpty()) { 1858 addTelecom(); 1859 } 1860 return getTelecom().get(0); 1861 } 1862 1863 /** 1864 * @return {@link #gender} (Administrative Gender - the gender that the patient 1865 * is considered to have for administration and record keeping 1866 * purposes.). This is the underlying object with id, value and 1867 * extensions. The accessor "getGender" gives direct access to the value 1868 */ 1869 public Enumeration<AdministrativeGender> getGenderElement() { 1870 if (this.gender == null) 1871 if (Configuration.errorOnAutoCreate()) 1872 throw new Error("Attempt to auto-create Patient.gender"); 1873 else if (Configuration.doAutoCreate()) 1874 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 1875 return this.gender; 1876 } 1877 1878 public boolean hasGenderElement() { 1879 return this.gender != null && !this.gender.isEmpty(); 1880 } 1881 1882 public boolean hasGender() { 1883 return this.gender != null && !this.gender.isEmpty(); 1884 } 1885 1886 /** 1887 * @param value {@link #gender} (Administrative Gender - the gender that the 1888 * patient is considered to have for administration and record 1889 * keeping purposes.). This is the underlying object with id, value 1890 * and extensions. The accessor "getGender" gives direct access to 1891 * the value 1892 */ 1893 public Patient setGenderElement(Enumeration<AdministrativeGender> value) { 1894 this.gender = value; 1895 return this; 1896 } 1897 1898 /** 1899 * @return Administrative Gender - the gender that the patient is considered to 1900 * have for administration and record keeping purposes. 1901 */ 1902 public AdministrativeGender getGender() { 1903 return this.gender == null ? null : this.gender.getValue(); 1904 } 1905 1906 /** 1907 * @param value Administrative Gender - the gender that the patient is 1908 * considered to have for administration and record keeping 1909 * purposes. 1910 */ 1911 public Patient setGender(AdministrativeGender value) { 1912 if (value == null) 1913 this.gender = null; 1914 else { 1915 if (this.gender == null) 1916 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 1917 this.gender.setValue(value); 1918 } 1919 return this; 1920 } 1921 1922 /** 1923 * @return {@link #birthDate} (The date of birth for the individual.). This is 1924 * the underlying object with id, value and extensions. The accessor 1925 * "getBirthDate" gives direct access to the value 1926 */ 1927 public DateType getBirthDateElement() { 1928 if (this.birthDate == null) 1929 if (Configuration.errorOnAutoCreate()) 1930 throw new Error("Attempt to auto-create Patient.birthDate"); 1931 else if (Configuration.doAutoCreate()) 1932 this.birthDate = new DateType(); // bb 1933 return this.birthDate; 1934 } 1935 1936 public boolean hasBirthDateElement() { 1937 return this.birthDate != null && !this.birthDate.isEmpty(); 1938 } 1939 1940 public boolean hasBirthDate() { 1941 return this.birthDate != null && !this.birthDate.isEmpty(); 1942 } 1943 1944 /** 1945 * @param value {@link #birthDate} (The date of birth for the individual.). This 1946 * is the underlying object with id, value and extensions. The 1947 * accessor "getBirthDate" gives direct access to the value 1948 */ 1949 public Patient setBirthDateElement(DateType value) { 1950 this.birthDate = value; 1951 return this; 1952 } 1953 1954 /** 1955 * @return The date of birth for the individual. 1956 */ 1957 public Date getBirthDate() { 1958 return this.birthDate == null ? null : this.birthDate.getValue(); 1959 } 1960 1961 /** 1962 * @param value The date of birth for the individual. 1963 */ 1964 public Patient setBirthDate(Date value) { 1965 if (value == null) 1966 this.birthDate = null; 1967 else { 1968 if (this.birthDate == null) 1969 this.birthDate = new DateType(); 1970 this.birthDate.setValue(value); 1971 } 1972 return this; 1973 } 1974 1975 /** 1976 * @return {@link #deceased} (Indicates if the individual is deceased or not.) 1977 */ 1978 public Type getDeceased() { 1979 return this.deceased; 1980 } 1981 1982 /** 1983 * @return {@link #deceased} (Indicates if the individual is deceased or not.) 1984 */ 1985 public BooleanType getDeceasedBooleanType() throws FHIRException { 1986 if (this.deceased == null) 1987 this.deceased = new BooleanType(); 1988 if (!(this.deceased instanceof BooleanType)) 1989 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 1990 + this.deceased.getClass().getName() + " was encountered"); 1991 return (BooleanType) this.deceased; 1992 } 1993 1994 public boolean hasDeceasedBooleanType() { 1995 return this.deceased instanceof BooleanType; 1996 } 1997 1998 /** 1999 * @return {@link #deceased} (Indicates if the individual is deceased or not.) 2000 */ 2001 public DateTimeType getDeceasedDateTimeType() throws FHIRException { 2002 if (this.deceased == null) 2003 this.deceased = new DateTimeType(); 2004 if (!(this.deceased instanceof DateTimeType)) 2005 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 2006 + this.deceased.getClass().getName() + " was encountered"); 2007 return (DateTimeType) this.deceased; 2008 } 2009 2010 public boolean hasDeceasedDateTimeType() { 2011 return this.deceased instanceof DateTimeType; 2012 } 2013 2014 public boolean hasDeceased() { 2015 return this.deceased != null && !this.deceased.isEmpty(); 2016 } 2017 2018 /** 2019 * @param value {@link #deceased} (Indicates if the individual is deceased or 2020 * not.) 2021 */ 2022 public Patient setDeceased(Type value) { 2023 if (value != null && !(value instanceof BooleanType || value instanceof DateTimeType)) 2024 throw new Error("Not the right type for Patient.deceased[x]: " + value.fhirType()); 2025 this.deceased = value; 2026 return this; 2027 } 2028 2029 /** 2030 * @return {@link #address} (An address for the individual.) 2031 */ 2032 public List<Address> getAddress() { 2033 if (this.address == null) 2034 this.address = new ArrayList<Address>(); 2035 return this.address; 2036 } 2037 2038 /** 2039 * @return Returns a reference to <code>this</code> for easy method chaining 2040 */ 2041 public Patient setAddress(List<Address> theAddress) { 2042 this.address = theAddress; 2043 return this; 2044 } 2045 2046 public boolean hasAddress() { 2047 if (this.address == null) 2048 return false; 2049 for (Address item : this.address) 2050 if (!item.isEmpty()) 2051 return true; 2052 return false; 2053 } 2054 2055 public Address addAddress() { // 3 2056 Address t = new Address(); 2057 if (this.address == null) 2058 this.address = new ArrayList<Address>(); 2059 this.address.add(t); 2060 return t; 2061 } 2062 2063 public Patient addAddress(Address t) { // 3 2064 if (t == null) 2065 return this; 2066 if (this.address == null) 2067 this.address = new ArrayList<Address>(); 2068 this.address.add(t); 2069 return this; 2070 } 2071 2072 /** 2073 * @return The first repetition of repeating field {@link #address}, creating it 2074 * if it does not already exist 2075 */ 2076 public Address getAddressFirstRep() { 2077 if (getAddress().isEmpty()) { 2078 addAddress(); 2079 } 2080 return getAddress().get(0); 2081 } 2082 2083 /** 2084 * @return {@link #maritalStatus} (This field contains a patient's most recent 2085 * marital (civil) status.) 2086 */ 2087 public CodeableConcept getMaritalStatus() { 2088 if (this.maritalStatus == null) 2089 if (Configuration.errorOnAutoCreate()) 2090 throw new Error("Attempt to auto-create Patient.maritalStatus"); 2091 else if (Configuration.doAutoCreate()) 2092 this.maritalStatus = new CodeableConcept(); // cc 2093 return this.maritalStatus; 2094 } 2095 2096 public boolean hasMaritalStatus() { 2097 return this.maritalStatus != null && !this.maritalStatus.isEmpty(); 2098 } 2099 2100 /** 2101 * @param value {@link #maritalStatus} (This field contains a patient's most 2102 * recent marital (civil) status.) 2103 */ 2104 public Patient setMaritalStatus(CodeableConcept value) { 2105 this.maritalStatus = value; 2106 return this; 2107 } 2108 2109 /** 2110 * @return {@link #multipleBirth} (Indicates whether the patient is part of a 2111 * multiple (boolean) or indicates the actual birth order (integer).) 2112 */ 2113 public Type getMultipleBirth() { 2114 return this.multipleBirth; 2115 } 2116 2117 /** 2118 * @return {@link #multipleBirth} (Indicates whether the patient is part of a 2119 * multiple (boolean) or indicates the actual birth order (integer).) 2120 */ 2121 public BooleanType getMultipleBirthBooleanType() throws FHIRException { 2122 if (this.multipleBirth == null) 2123 this.multipleBirth = new BooleanType(); 2124 if (!(this.multipleBirth instanceof BooleanType)) 2125 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 2126 + this.multipleBirth.getClass().getName() + " was encountered"); 2127 return (BooleanType) this.multipleBirth; 2128 } 2129 2130 public boolean hasMultipleBirthBooleanType() { 2131 return this.multipleBirth instanceof BooleanType; 2132 } 2133 2134 /** 2135 * @return {@link #multipleBirth} (Indicates whether the patient is part of a 2136 * multiple (boolean) or indicates the actual birth order (integer).) 2137 */ 2138 public IntegerType getMultipleBirthIntegerType() throws FHIRException { 2139 if (this.multipleBirth == null) 2140 this.multipleBirth = new IntegerType(); 2141 if (!(this.multipleBirth instanceof IntegerType)) 2142 throw new FHIRException("Type mismatch: the type IntegerType was expected, but " 2143 + this.multipleBirth.getClass().getName() + " was encountered"); 2144 return (IntegerType) this.multipleBirth; 2145 } 2146 2147 public boolean hasMultipleBirthIntegerType() { 2148 return this.multipleBirth instanceof IntegerType; 2149 } 2150 2151 public boolean hasMultipleBirth() { 2152 return this.multipleBirth != null && !this.multipleBirth.isEmpty(); 2153 } 2154 2155 /** 2156 * @param value {@link #multipleBirth} (Indicates whether the patient is part of 2157 * a multiple (boolean) or indicates the actual birth order 2158 * (integer).) 2159 */ 2160 public Patient setMultipleBirth(Type value) { 2161 if (value != null && !(value instanceof BooleanType || value instanceof IntegerType)) 2162 throw new Error("Not the right type for Patient.multipleBirth[x]: " + value.fhirType()); 2163 this.multipleBirth = value; 2164 return this; 2165 } 2166 2167 /** 2168 * @return {@link #photo} (Image of the patient.) 2169 */ 2170 public List<Attachment> getPhoto() { 2171 if (this.photo == null) 2172 this.photo = new ArrayList<Attachment>(); 2173 return this.photo; 2174 } 2175 2176 /** 2177 * @return Returns a reference to <code>this</code> for easy method chaining 2178 */ 2179 public Patient setPhoto(List<Attachment> thePhoto) { 2180 this.photo = thePhoto; 2181 return this; 2182 } 2183 2184 public boolean hasPhoto() { 2185 if (this.photo == null) 2186 return false; 2187 for (Attachment item : this.photo) 2188 if (!item.isEmpty()) 2189 return true; 2190 return false; 2191 } 2192 2193 public Attachment addPhoto() { // 3 2194 Attachment t = new Attachment(); 2195 if (this.photo == null) 2196 this.photo = new ArrayList<Attachment>(); 2197 this.photo.add(t); 2198 return t; 2199 } 2200 2201 public Patient addPhoto(Attachment t) { // 3 2202 if (t == null) 2203 return this; 2204 if (this.photo == null) 2205 this.photo = new ArrayList<Attachment>(); 2206 this.photo.add(t); 2207 return this; 2208 } 2209 2210 /** 2211 * @return The first repetition of repeating field {@link #photo}, creating it 2212 * if it does not already exist 2213 */ 2214 public Attachment getPhotoFirstRep() { 2215 if (getPhoto().isEmpty()) { 2216 addPhoto(); 2217 } 2218 return getPhoto().get(0); 2219 } 2220 2221 /** 2222 * @return {@link #contact} (A contact party (e.g. guardian, partner, friend) 2223 * for the patient.) 2224 */ 2225 public List<ContactComponent> getContact() { 2226 if (this.contact == null) 2227 this.contact = new ArrayList<ContactComponent>(); 2228 return this.contact; 2229 } 2230 2231 /** 2232 * @return Returns a reference to <code>this</code> for easy method chaining 2233 */ 2234 public Patient setContact(List<ContactComponent> theContact) { 2235 this.contact = theContact; 2236 return this; 2237 } 2238 2239 public boolean hasContact() { 2240 if (this.contact == null) 2241 return false; 2242 for (ContactComponent item : this.contact) 2243 if (!item.isEmpty()) 2244 return true; 2245 return false; 2246 } 2247 2248 public ContactComponent addContact() { // 3 2249 ContactComponent t = new ContactComponent(); 2250 if (this.contact == null) 2251 this.contact = new ArrayList<ContactComponent>(); 2252 this.contact.add(t); 2253 return t; 2254 } 2255 2256 public Patient addContact(ContactComponent t) { // 3 2257 if (t == null) 2258 return this; 2259 if (this.contact == null) 2260 this.contact = new ArrayList<ContactComponent>(); 2261 this.contact.add(t); 2262 return this; 2263 } 2264 2265 /** 2266 * @return The first repetition of repeating field {@link #contact}, creating it 2267 * if it does not already exist 2268 */ 2269 public ContactComponent getContactFirstRep() { 2270 if (getContact().isEmpty()) { 2271 addContact(); 2272 } 2273 return getContact().get(0); 2274 } 2275 2276 /** 2277 * @return {@link #communication} (A language which may be used to communicate 2278 * with the patient about his or her health.) 2279 */ 2280 public List<PatientCommunicationComponent> getCommunication() { 2281 if (this.communication == null) 2282 this.communication = new ArrayList<PatientCommunicationComponent>(); 2283 return this.communication; 2284 } 2285 2286 /** 2287 * @return Returns a reference to <code>this</code> for easy method chaining 2288 */ 2289 public Patient setCommunication(List<PatientCommunicationComponent> theCommunication) { 2290 this.communication = theCommunication; 2291 return this; 2292 } 2293 2294 public boolean hasCommunication() { 2295 if (this.communication == null) 2296 return false; 2297 for (PatientCommunicationComponent item : this.communication) 2298 if (!item.isEmpty()) 2299 return true; 2300 return false; 2301 } 2302 2303 public PatientCommunicationComponent addCommunication() { // 3 2304 PatientCommunicationComponent t = new PatientCommunicationComponent(); 2305 if (this.communication == null) 2306 this.communication = new ArrayList<PatientCommunicationComponent>(); 2307 this.communication.add(t); 2308 return t; 2309 } 2310 2311 public Patient addCommunication(PatientCommunicationComponent t) { // 3 2312 if (t == null) 2313 return this; 2314 if (this.communication == null) 2315 this.communication = new ArrayList<PatientCommunicationComponent>(); 2316 this.communication.add(t); 2317 return this; 2318 } 2319 2320 /** 2321 * @return The first repetition of repeating field {@link #communication}, 2322 * creating it if it does not already exist 2323 */ 2324 public PatientCommunicationComponent getCommunicationFirstRep() { 2325 if (getCommunication().isEmpty()) { 2326 addCommunication(); 2327 } 2328 return getCommunication().get(0); 2329 } 2330 2331 /** 2332 * @return {@link #generalPractitioner} (Patient's nominated care provider.) 2333 */ 2334 public List<Reference> getGeneralPractitioner() { 2335 if (this.generalPractitioner == null) 2336 this.generalPractitioner = new ArrayList<Reference>(); 2337 return this.generalPractitioner; 2338 } 2339 2340 /** 2341 * @return Returns a reference to <code>this</code> for easy method chaining 2342 */ 2343 public Patient setGeneralPractitioner(List<Reference> theGeneralPractitioner) { 2344 this.generalPractitioner = theGeneralPractitioner; 2345 return this; 2346 } 2347 2348 public boolean hasGeneralPractitioner() { 2349 if (this.generalPractitioner == null) 2350 return false; 2351 for (Reference item : this.generalPractitioner) 2352 if (!item.isEmpty()) 2353 return true; 2354 return false; 2355 } 2356 2357 public Reference addGeneralPractitioner() { // 3 2358 Reference t = new Reference(); 2359 if (this.generalPractitioner == null) 2360 this.generalPractitioner = new ArrayList<Reference>(); 2361 this.generalPractitioner.add(t); 2362 return t; 2363 } 2364 2365 public Patient addGeneralPractitioner(Reference t) { // 3 2366 if (t == null) 2367 return this; 2368 if (this.generalPractitioner == null) 2369 this.generalPractitioner = new ArrayList<Reference>(); 2370 this.generalPractitioner.add(t); 2371 return this; 2372 } 2373 2374 /** 2375 * @return The first repetition of repeating field {@link #generalPractitioner}, 2376 * creating it if it does not already exist 2377 */ 2378 public Reference getGeneralPractitionerFirstRep() { 2379 if (getGeneralPractitioner().isEmpty()) { 2380 addGeneralPractitioner(); 2381 } 2382 return getGeneralPractitioner().get(0); 2383 } 2384 2385 /** 2386 * @return {@link #managingOrganization} (Organization that is the custodian of 2387 * the patient record.) 2388 */ 2389 public Reference getManagingOrganization() { 2390 if (this.managingOrganization == null) 2391 if (Configuration.errorOnAutoCreate()) 2392 throw new Error("Attempt to auto-create Patient.managingOrganization"); 2393 else if (Configuration.doAutoCreate()) 2394 this.managingOrganization = new Reference(); // cc 2395 return this.managingOrganization; 2396 } 2397 2398 public boolean hasManagingOrganization() { 2399 return this.managingOrganization != null && !this.managingOrganization.isEmpty(); 2400 } 2401 2402 /** 2403 * @param value {@link #managingOrganization} (Organization that is the 2404 * custodian of the patient record.) 2405 */ 2406 public Patient setManagingOrganization(Reference value) { 2407 this.managingOrganization = value; 2408 return this; 2409 } 2410 2411 /** 2412 * @return {@link #managingOrganization} The actual object that is the target of 2413 * the reference. The reference library doesn't populate this, but you 2414 * can use it to hold the resource if you resolve it. (Organization that 2415 * is the custodian of the patient record.) 2416 */ 2417 public Organization getManagingOrganizationTarget() { 2418 if (this.managingOrganizationTarget == null) 2419 if (Configuration.errorOnAutoCreate()) 2420 throw new Error("Attempt to auto-create Patient.managingOrganization"); 2421 else if (Configuration.doAutoCreate()) 2422 this.managingOrganizationTarget = new Organization(); // aa 2423 return this.managingOrganizationTarget; 2424 } 2425 2426 /** 2427 * @param value {@link #managingOrganization} The actual object that is the 2428 * target of the reference. The reference library doesn't use 2429 * these, but you can use it to hold the resource if you resolve 2430 * it. (Organization that is the custodian of the patient record.) 2431 */ 2432 public Patient setManagingOrganizationTarget(Organization value) { 2433 this.managingOrganizationTarget = value; 2434 return this; 2435 } 2436 2437 /** 2438 * @return {@link #link} (Link to another patient resource that concerns the 2439 * same actual patient.) 2440 */ 2441 public List<PatientLinkComponent> getLink() { 2442 if (this.link == null) 2443 this.link = new ArrayList<PatientLinkComponent>(); 2444 return this.link; 2445 } 2446 2447 /** 2448 * @return Returns a reference to <code>this</code> for easy method chaining 2449 */ 2450 public Patient setLink(List<PatientLinkComponent> theLink) { 2451 this.link = theLink; 2452 return this; 2453 } 2454 2455 public boolean hasLink() { 2456 if (this.link == null) 2457 return false; 2458 for (PatientLinkComponent item : this.link) 2459 if (!item.isEmpty()) 2460 return true; 2461 return false; 2462 } 2463 2464 public PatientLinkComponent addLink() { // 3 2465 PatientLinkComponent t = new PatientLinkComponent(); 2466 if (this.link == null) 2467 this.link = new ArrayList<PatientLinkComponent>(); 2468 this.link.add(t); 2469 return t; 2470 } 2471 2472 public Patient addLink(PatientLinkComponent t) { // 3 2473 if (t == null) 2474 return this; 2475 if (this.link == null) 2476 this.link = new ArrayList<PatientLinkComponent>(); 2477 this.link.add(t); 2478 return this; 2479 } 2480 2481 /** 2482 * @return The first repetition of repeating field {@link #link}, creating it if 2483 * it does not already exist 2484 */ 2485 public PatientLinkComponent getLinkFirstRep() { 2486 if (getLink().isEmpty()) { 2487 addLink(); 2488 } 2489 return getLink().get(0); 2490 } 2491 2492 protected void listChildren(List<Property> children) { 2493 super.listChildren(children); 2494 children.add(new Property("identifier", "Identifier", "An identifier for this patient.", 0, 2495 java.lang.Integer.MAX_VALUE, identifier)); 2496 children.add(new Property("active", "boolean", 2497 "Whether this patient record is in active use. \nMany systems use this property to mark as non-current patients, such as those that have not been seen for a period of time based on an organization's business rules.\n\nIt is often used to filter patient lists to exclude inactive patients\n\nDeceased patients may also be marked as inactive for the same reasons, but may be active for some time after death.", 2498 0, 1, active)); 2499 children.add(new Property("name", "HumanName", "A name associated with the individual.", 0, 2500 java.lang.Integer.MAX_VALUE, name)); 2501 children.add(new Property("telecom", "ContactPoint", 2502 "A contact detail (e.g. a telephone number or an email address) by which the individual may be contacted.", 0, 2503 java.lang.Integer.MAX_VALUE, telecom)); 2504 children.add(new Property("gender", "code", 2505 "Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes.", 2506 0, 1, gender)); 2507 children.add(new Property("birthDate", "date", "The date of birth for the individual.", 0, 1, birthDate)); 2508 children.add(new Property("deceased[x]", "boolean|dateTime", "Indicates if the individual is deceased or not.", 0, 2509 1, deceased)); 2510 children.add( 2511 new Property("address", "Address", "An address for the individual.", 0, java.lang.Integer.MAX_VALUE, address)); 2512 children.add(new Property("maritalStatus", "CodeableConcept", 2513 "This field contains a patient's most recent marital (civil) status.", 0, 1, maritalStatus)); 2514 children.add(new Property("multipleBirth[x]", "boolean|integer", 2515 "Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer).", 2516 0, 1, multipleBirth)); 2517 children.add(new Property("photo", "Attachment", "Image of the patient.", 0, java.lang.Integer.MAX_VALUE, photo)); 2518 children.add(new Property("contact", "", "A contact party (e.g. guardian, partner, friend) for the patient.", 0, 2519 java.lang.Integer.MAX_VALUE, contact)); 2520 children.add(new Property("communication", "", 2521 "A language which may be used to communicate with the patient about his or her health.", 0, 2522 java.lang.Integer.MAX_VALUE, communication)); 2523 children.add(new Property("generalPractitioner", "Reference(Organization|Practitioner|PractitionerRole)", 2524 "Patient's nominated care provider.", 0, java.lang.Integer.MAX_VALUE, generalPractitioner)); 2525 children.add(new Property("managingOrganization", "Reference(Organization)", 2526 "Organization that is the custodian of the patient record.", 0, 1, managingOrganization)); 2527 children.add(new Property("link", "", "Link to another patient resource that concerns the same actual patient.", 0, 2528 java.lang.Integer.MAX_VALUE, link)); 2529 } 2530 2531 @Override 2532 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2533 switch (_hash) { 2534 case -1618432855: 2535 /* identifier */ return new Property("identifier", "Identifier", "An identifier for this patient.", 0, 2536 java.lang.Integer.MAX_VALUE, identifier); 2537 case -1422950650: 2538 /* active */ return new Property("active", "boolean", 2539 "Whether this patient record is in active use. \nMany systems use this property to mark as non-current patients, such as those that have not been seen for a period of time based on an organization's business rules.\n\nIt is often used to filter patient lists to exclude inactive patients\n\nDeceased patients may also be marked as inactive for the same reasons, but may be active for some time after death.", 2540 0, 1, active); 2541 case 3373707: 2542 /* name */ return new Property("name", "HumanName", "A name associated with the individual.", 0, 2543 java.lang.Integer.MAX_VALUE, name); 2544 case -1429363305: 2545 /* telecom */ return new Property("telecom", "ContactPoint", 2546 "A contact detail (e.g. a telephone number or an email address) by which the individual may be contacted.", 0, 2547 java.lang.Integer.MAX_VALUE, telecom); 2548 case -1249512767: 2549 /* gender */ return new Property("gender", "code", 2550 "Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes.", 2551 0, 1, gender); 2552 case -1210031859: 2553 /* birthDate */ return new Property("birthDate", "date", "The date of birth for the individual.", 0, 1, 2554 birthDate); 2555 case -1311442804: 2556 /* deceased[x] */ return new Property("deceased[x]", "boolean|dateTime", 2557 "Indicates if the individual is deceased or not.", 0, 1, deceased); 2558 case 561497972: 2559 /* deceased */ return new Property("deceased[x]", "boolean|dateTime", 2560 "Indicates if the individual is deceased or not.", 0, 1, deceased); 2561 case 497463828: 2562 /* deceasedBoolean */ return new Property("deceased[x]", "boolean|dateTime", 2563 "Indicates if the individual is deceased or not.", 0, 1, deceased); 2564 case -1971804369: 2565 /* deceasedDateTime */ return new Property("deceased[x]", "boolean|dateTime", 2566 "Indicates if the individual is deceased or not.", 0, 1, deceased); 2567 case -1147692044: 2568 /* address */ return new Property("address", "Address", "An address for the individual.", 0, 2569 java.lang.Integer.MAX_VALUE, address); 2570 case 1756919302: 2571 /* maritalStatus */ return new Property("maritalStatus", "CodeableConcept", 2572 "This field contains a patient's most recent marital (civil) status.", 0, 1, maritalStatus); 2573 case -1764672111: 2574 /* multipleBirth[x] */ return new Property("multipleBirth[x]", "boolean|integer", 2575 "Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer).", 2576 0, 1, multipleBirth); 2577 case -677369713: 2578 /* multipleBirth */ return new Property("multipleBirth[x]", "boolean|integer", 2579 "Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer).", 2580 0, 1, multipleBirth); 2581 case -247534439: 2582 /* multipleBirthBoolean */ return new Property("multipleBirth[x]", "boolean|integer", 2583 "Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer).", 2584 0, 1, multipleBirth); 2585 case 1645805999: 2586 /* multipleBirthInteger */ return new Property("multipleBirth[x]", "boolean|integer", 2587 "Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer).", 2588 0, 1, multipleBirth); 2589 case 106642994: 2590 /* photo */ return new Property("photo", "Attachment", "Image of the patient.", 0, java.lang.Integer.MAX_VALUE, 2591 photo); 2592 case 951526432: 2593 /* contact */ return new Property("contact", "", 2594 "A contact party (e.g. guardian, partner, friend) for the patient.", 0, java.lang.Integer.MAX_VALUE, contact); 2595 case -1035284522: 2596 /* communication */ return new Property("communication", "", 2597 "A language which may be used to communicate with the patient about his or her health.", 0, 2598 java.lang.Integer.MAX_VALUE, communication); 2599 case 1488292898: 2600 /* generalPractitioner */ return new Property("generalPractitioner", 2601 "Reference(Organization|Practitioner|PractitionerRole)", "Patient's nominated care provider.", 0, 2602 java.lang.Integer.MAX_VALUE, generalPractitioner); 2603 case -2058947787: 2604 /* managingOrganization */ return new Property("managingOrganization", "Reference(Organization)", 2605 "Organization that is the custodian of the patient record.", 0, 1, managingOrganization); 2606 case 3321850: 2607 /* link */ return new Property("link", "", 2608 "Link to another patient resource that concerns the same actual patient.", 0, java.lang.Integer.MAX_VALUE, 2609 link); 2610 default: 2611 return super.getNamedProperty(_hash, _name, _checkValid); 2612 } 2613 2614 } 2615 2616 @Override 2617 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2618 switch (hash) { 2619 case -1618432855: 2620 /* identifier */ return this.identifier == null ? new Base[0] 2621 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2622 case -1422950650: 2623 /* active */ return this.active == null ? new Base[0] : new Base[] { this.active }; // BooleanType 2624 case 3373707: 2625 /* name */ return this.name == null ? new Base[0] : this.name.toArray(new Base[this.name.size()]); // HumanName 2626 case -1429363305: 2627 /* telecom */ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 2628 case -1249512767: 2629 /* gender */ return this.gender == null ? new Base[0] : new Base[] { this.gender }; // Enumeration<AdministrativeGender> 2630 case -1210031859: 2631 /* birthDate */ return this.birthDate == null ? new Base[0] : new Base[] { this.birthDate }; // DateType 2632 case 561497972: 2633 /* deceased */ return this.deceased == null ? new Base[0] : new Base[] { this.deceased }; // Type 2634 case -1147692044: 2635 /* address */ return this.address == null ? new Base[0] : this.address.toArray(new Base[this.address.size()]); // Address 2636 case 1756919302: 2637 /* maritalStatus */ return this.maritalStatus == null ? new Base[0] : new Base[] { this.maritalStatus }; // CodeableConcept 2638 case -677369713: 2639 /* multipleBirth */ return this.multipleBirth == null ? new Base[0] : new Base[] { this.multipleBirth }; // Type 2640 case 106642994: 2641 /* photo */ return this.photo == null ? new Base[0] : this.photo.toArray(new Base[this.photo.size()]); // Attachment 2642 case 951526432: 2643 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactComponent 2644 case -1035284522: 2645 /* communication */ return this.communication == null ? new Base[0] 2646 : this.communication.toArray(new Base[this.communication.size()]); // PatientCommunicationComponent 2647 case 1488292898: 2648 /* generalPractitioner */ return this.generalPractitioner == null ? new Base[0] 2649 : this.generalPractitioner.toArray(new Base[this.generalPractitioner.size()]); // Reference 2650 case -2058947787: 2651 /* managingOrganization */ return this.managingOrganization == null ? new Base[0] 2652 : new Base[] { this.managingOrganization }; // Reference 2653 case 3321850: 2654 /* link */ return this.link == null ? new Base[0] : this.link.toArray(new Base[this.link.size()]); // PatientLinkComponent 2655 default: 2656 return super.getProperty(hash, name, checkValid); 2657 } 2658 2659 } 2660 2661 @Override 2662 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2663 switch (hash) { 2664 case -1618432855: // identifier 2665 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2666 return value; 2667 case -1422950650: // active 2668 this.active = castToBoolean(value); // BooleanType 2669 return value; 2670 case 3373707: // name 2671 this.getName().add(castToHumanName(value)); // HumanName 2672 return value; 2673 case -1429363305: // telecom 2674 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 2675 return value; 2676 case -1249512767: // gender 2677 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 2678 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 2679 return value; 2680 case -1210031859: // birthDate 2681 this.birthDate = castToDate(value); // DateType 2682 return value; 2683 case 561497972: // deceased 2684 this.deceased = castToType(value); // Type 2685 return value; 2686 case -1147692044: // address 2687 this.getAddress().add(castToAddress(value)); // Address 2688 return value; 2689 case 1756919302: // maritalStatus 2690 this.maritalStatus = castToCodeableConcept(value); // CodeableConcept 2691 return value; 2692 case -677369713: // multipleBirth 2693 this.multipleBirth = castToType(value); // Type 2694 return value; 2695 case 106642994: // photo 2696 this.getPhoto().add(castToAttachment(value)); // Attachment 2697 return value; 2698 case 951526432: // contact 2699 this.getContact().add((ContactComponent) value); // ContactComponent 2700 return value; 2701 case -1035284522: // communication 2702 this.getCommunication().add((PatientCommunicationComponent) value); // PatientCommunicationComponent 2703 return value; 2704 case 1488292898: // generalPractitioner 2705 this.getGeneralPractitioner().add(castToReference(value)); // Reference 2706 return value; 2707 case -2058947787: // managingOrganization 2708 this.managingOrganization = castToReference(value); // Reference 2709 return value; 2710 case 3321850: // link 2711 this.getLink().add((PatientLinkComponent) value); // PatientLinkComponent 2712 return value; 2713 default: 2714 return super.setProperty(hash, name, value); 2715 } 2716 2717 } 2718 2719 @Override 2720 public Base setProperty(String name, Base value) throws FHIRException { 2721 if (name.equals("identifier")) { 2722 this.getIdentifier().add(castToIdentifier(value)); 2723 } else if (name.equals("active")) { 2724 this.active = castToBoolean(value); // BooleanType 2725 } else if (name.equals("name")) { 2726 this.getName().add(castToHumanName(value)); 2727 } else if (name.equals("telecom")) { 2728 this.getTelecom().add(castToContactPoint(value)); 2729 } else if (name.equals("gender")) { 2730 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 2731 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 2732 } else if (name.equals("birthDate")) { 2733 this.birthDate = castToDate(value); // DateType 2734 } else if (name.equals("deceased[x]")) { 2735 this.deceased = castToType(value); // Type 2736 } else if (name.equals("address")) { 2737 this.getAddress().add(castToAddress(value)); 2738 } else if (name.equals("maritalStatus")) { 2739 this.maritalStatus = castToCodeableConcept(value); // CodeableConcept 2740 } else if (name.equals("multipleBirth[x]")) { 2741 this.multipleBirth = castToType(value); // Type 2742 } else if (name.equals("photo")) { 2743 this.getPhoto().add(castToAttachment(value)); 2744 } else if (name.equals("contact")) { 2745 this.getContact().add((ContactComponent) value); 2746 } else if (name.equals("communication")) { 2747 this.getCommunication().add((PatientCommunicationComponent) value); 2748 } else if (name.equals("generalPractitioner")) { 2749 this.getGeneralPractitioner().add(castToReference(value)); 2750 } else if (name.equals("managingOrganization")) { 2751 this.managingOrganization = castToReference(value); // Reference 2752 } else if (name.equals("link")) { 2753 this.getLink().add((PatientLinkComponent) value); 2754 } else 2755 return super.setProperty(name, value); 2756 return value; 2757 } 2758 2759 @Override 2760 public void removeChild(String name, Base value) throws FHIRException { 2761 if (name.equals("identifier")) { 2762 this.getIdentifier().remove(castToIdentifier(value)); 2763 } else if (name.equals("active")) { 2764 this.active = null; 2765 } else if (name.equals("name")) { 2766 this.getName().remove(castToHumanName(value)); 2767 } else if (name.equals("telecom")) { 2768 this.getTelecom().remove(castToContactPoint(value)); 2769 } else if (name.equals("gender")) { 2770 this.gender = null; 2771 } else if (name.equals("birthDate")) { 2772 this.birthDate = null; 2773 } else if (name.equals("deceased[x]")) { 2774 this.deceased = null; 2775 } else if (name.equals("address")) { 2776 this.getAddress().remove(castToAddress(value)); 2777 } else if (name.equals("maritalStatus")) { 2778 this.maritalStatus = null; 2779 } else if (name.equals("multipleBirth[x]")) { 2780 this.multipleBirth = null; 2781 } else if (name.equals("photo")) { 2782 this.getPhoto().remove(castToAttachment(value)); 2783 } else if (name.equals("contact")) { 2784 this.getContact().remove((ContactComponent) value); 2785 } else if (name.equals("communication")) { 2786 this.getCommunication().remove((PatientCommunicationComponent) value); 2787 } else if (name.equals("generalPractitioner")) { 2788 this.getGeneralPractitioner().remove(castToReference(value)); 2789 } else if (name.equals("managingOrganization")) { 2790 this.managingOrganization = null; 2791 } else if (name.equals("link")) { 2792 this.getLink().remove((PatientLinkComponent) value); 2793 } else 2794 super.removeChild(name, value); 2795 2796 } 2797 2798 @Override 2799 public Base makeProperty(int hash, String name) throws FHIRException { 2800 switch (hash) { 2801 case -1618432855: 2802 return addIdentifier(); 2803 case -1422950650: 2804 return getActiveElement(); 2805 case 3373707: 2806 return addName(); 2807 case -1429363305: 2808 return addTelecom(); 2809 case -1249512767: 2810 return getGenderElement(); 2811 case -1210031859: 2812 return getBirthDateElement(); 2813 case -1311442804: 2814 return getDeceased(); 2815 case 561497972: 2816 return getDeceased(); 2817 case -1147692044: 2818 return addAddress(); 2819 case 1756919302: 2820 return getMaritalStatus(); 2821 case -1764672111: 2822 return getMultipleBirth(); 2823 case -677369713: 2824 return getMultipleBirth(); 2825 case 106642994: 2826 return addPhoto(); 2827 case 951526432: 2828 return addContact(); 2829 case -1035284522: 2830 return addCommunication(); 2831 case 1488292898: 2832 return addGeneralPractitioner(); 2833 case -2058947787: 2834 return getManagingOrganization(); 2835 case 3321850: 2836 return addLink(); 2837 default: 2838 return super.makeProperty(hash, name); 2839 } 2840 2841 } 2842 2843 @Override 2844 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2845 switch (hash) { 2846 case -1618432855: 2847 /* identifier */ return new String[] { "Identifier" }; 2848 case -1422950650: 2849 /* active */ return new String[] { "boolean" }; 2850 case 3373707: 2851 /* name */ return new String[] { "HumanName" }; 2852 case -1429363305: 2853 /* telecom */ return new String[] { "ContactPoint" }; 2854 case -1249512767: 2855 /* gender */ return new String[] { "code" }; 2856 case -1210031859: 2857 /* birthDate */ return new String[] { "date" }; 2858 case 561497972: 2859 /* deceased */ return new String[] { "boolean", "dateTime" }; 2860 case -1147692044: 2861 /* address */ return new String[] { "Address" }; 2862 case 1756919302: 2863 /* maritalStatus */ return new String[] { "CodeableConcept" }; 2864 case -677369713: 2865 /* multipleBirth */ return new String[] { "boolean", "integer" }; 2866 case 106642994: 2867 /* photo */ return new String[] { "Attachment" }; 2868 case 951526432: 2869 /* contact */ return new String[] {}; 2870 case -1035284522: 2871 /* communication */ return new String[] {}; 2872 case 1488292898: 2873 /* generalPractitioner */ return new String[] { "Reference" }; 2874 case -2058947787: 2875 /* managingOrganization */ return new String[] { "Reference" }; 2876 case 3321850: 2877 /* link */ return new String[] {}; 2878 default: 2879 return super.getTypesForProperty(hash, name); 2880 } 2881 2882 } 2883 2884 @Override 2885 public Base addChild(String name) throws FHIRException { 2886 if (name.equals("identifier")) { 2887 return addIdentifier(); 2888 } else if (name.equals("active")) { 2889 throw new FHIRException("Cannot call addChild on a singleton property Patient.active"); 2890 } else if (name.equals("name")) { 2891 return addName(); 2892 } else if (name.equals("telecom")) { 2893 return addTelecom(); 2894 } else if (name.equals("gender")) { 2895 throw new FHIRException("Cannot call addChild on a singleton property Patient.gender"); 2896 } else if (name.equals("birthDate")) { 2897 throw new FHIRException("Cannot call addChild on a singleton property Patient.birthDate"); 2898 } else if (name.equals("deceasedBoolean")) { 2899 this.deceased = new BooleanType(); 2900 return this.deceased; 2901 } else if (name.equals("deceasedDateTime")) { 2902 this.deceased = new DateTimeType(); 2903 return this.deceased; 2904 } else if (name.equals("address")) { 2905 return addAddress(); 2906 } else if (name.equals("maritalStatus")) { 2907 this.maritalStatus = new CodeableConcept(); 2908 return this.maritalStatus; 2909 } else if (name.equals("multipleBirthBoolean")) { 2910 this.multipleBirth = new BooleanType(); 2911 return this.multipleBirth; 2912 } else if (name.equals("multipleBirthInteger")) { 2913 this.multipleBirth = new IntegerType(); 2914 return this.multipleBirth; 2915 } else if (name.equals("photo")) { 2916 return addPhoto(); 2917 } else if (name.equals("contact")) { 2918 return addContact(); 2919 } else if (name.equals("communication")) { 2920 return addCommunication(); 2921 } else if (name.equals("generalPractitioner")) { 2922 return addGeneralPractitioner(); 2923 } else if (name.equals("managingOrganization")) { 2924 this.managingOrganization = new Reference(); 2925 return this.managingOrganization; 2926 } else if (name.equals("link")) { 2927 return addLink(); 2928 } else 2929 return super.addChild(name); 2930 } 2931 2932 public String fhirType() { 2933 return "Patient"; 2934 2935 } 2936 2937 public Patient copy() { 2938 Patient dst = new Patient(); 2939 copyValues(dst); 2940 return dst; 2941 } 2942 2943 public void copyValues(Patient dst) { 2944 super.copyValues(dst); 2945 if (identifier != null) { 2946 dst.identifier = new ArrayList<Identifier>(); 2947 for (Identifier i : identifier) 2948 dst.identifier.add(i.copy()); 2949 } 2950 ; 2951 dst.active = active == null ? null : active.copy(); 2952 if (name != null) { 2953 dst.name = new ArrayList<HumanName>(); 2954 for (HumanName i : name) 2955 dst.name.add(i.copy()); 2956 } 2957 ; 2958 if (telecom != null) { 2959 dst.telecom = new ArrayList<ContactPoint>(); 2960 for (ContactPoint i : telecom) 2961 dst.telecom.add(i.copy()); 2962 } 2963 ; 2964 dst.gender = gender == null ? null : gender.copy(); 2965 dst.birthDate = birthDate == null ? null : birthDate.copy(); 2966 dst.deceased = deceased == null ? null : deceased.copy(); 2967 if (address != null) { 2968 dst.address = new ArrayList<Address>(); 2969 for (Address i : address) 2970 dst.address.add(i.copy()); 2971 } 2972 ; 2973 dst.maritalStatus = maritalStatus == null ? null : maritalStatus.copy(); 2974 dst.multipleBirth = multipleBirth == null ? null : multipleBirth.copy(); 2975 if (photo != null) { 2976 dst.photo = new ArrayList<Attachment>(); 2977 for (Attachment i : photo) 2978 dst.photo.add(i.copy()); 2979 } 2980 ; 2981 if (contact != null) { 2982 dst.contact = new ArrayList<ContactComponent>(); 2983 for (ContactComponent i : contact) 2984 dst.contact.add(i.copy()); 2985 } 2986 ; 2987 if (communication != null) { 2988 dst.communication = new ArrayList<PatientCommunicationComponent>(); 2989 for (PatientCommunicationComponent i : communication) 2990 dst.communication.add(i.copy()); 2991 } 2992 ; 2993 if (generalPractitioner != null) { 2994 dst.generalPractitioner = new ArrayList<Reference>(); 2995 for (Reference i : generalPractitioner) 2996 dst.generalPractitioner.add(i.copy()); 2997 } 2998 ; 2999 dst.managingOrganization = managingOrganization == null ? null : managingOrganization.copy(); 3000 if (link != null) { 3001 dst.link = new ArrayList<PatientLinkComponent>(); 3002 for (PatientLinkComponent i : link) 3003 dst.link.add(i.copy()); 3004 } 3005 ; 3006 } 3007 3008 protected Patient typedCopy() { 3009 return copy(); 3010 } 3011 3012 @Override 3013 public boolean equalsDeep(Base other_) { 3014 if (!super.equalsDeep(other_)) 3015 return false; 3016 if (!(other_ instanceof Patient)) 3017 return false; 3018 Patient o = (Patient) other_; 3019 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) 3020 && compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true) 3021 && compareDeep(gender, o.gender, true) && compareDeep(birthDate, o.birthDate, true) 3022 && compareDeep(deceased, o.deceased, true) && compareDeep(address, o.address, true) 3023 && compareDeep(maritalStatus, o.maritalStatus, true) && compareDeep(multipleBirth, o.multipleBirth, true) 3024 && compareDeep(photo, o.photo, true) && compareDeep(contact, o.contact, true) 3025 && compareDeep(communication, o.communication, true) 3026 && compareDeep(generalPractitioner, o.generalPractitioner, true) 3027 && compareDeep(managingOrganization, o.managingOrganization, true) && compareDeep(link, o.link, true); 3028 } 3029 3030 @Override 3031 public boolean equalsShallow(Base other_) { 3032 if (!super.equalsShallow(other_)) 3033 return false; 3034 if (!(other_ instanceof Patient)) 3035 return false; 3036 Patient o = (Patient) other_; 3037 return compareValues(active, o.active, true) && compareValues(gender, o.gender, true) 3038 && compareValues(birthDate, o.birthDate, true); 3039 } 3040 3041 public boolean isEmpty() { 3042 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, name, telecom, gender, birthDate, 3043 deceased, address, maritalStatus, multipleBirth, photo, contact, communication, generalPractitioner, 3044 managingOrganization, link); 3045 } 3046 3047 @Override 3048 public ResourceType getResourceType() { 3049 return ResourceType.Patient; 3050 } 3051 3052 /** 3053 * Search parameter: <b>identifier</b> 3054 * <p> 3055 * Description: <b>A patient identifier</b><br> 3056 * Type: <b>token</b><br> 3057 * Path: <b>Patient.identifier</b><br> 3058 * </p> 3059 */ 3060 @SearchParamDefinition(name = "identifier", path = "Patient.identifier", description = "A patient identifier", type = "token") 3061 public static final String SP_IDENTIFIER = "identifier"; 3062 /** 3063 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3064 * <p> 3065 * Description: <b>A patient identifier</b><br> 3066 * Type: <b>token</b><br> 3067 * Path: <b>Patient.identifier</b><br> 3068 * </p> 3069 */ 3070 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3071 SP_IDENTIFIER); 3072 3073 /** 3074 * Search parameter: <b>given</b> 3075 * <p> 3076 * Description: <b>A portion of the given name of the patient</b><br> 3077 * Type: <b>string</b><br> 3078 * Path: <b>Patient.name.given</b><br> 3079 * </p> 3080 */ 3081 @SearchParamDefinition(name = "given", path = "Patient.name.given", description = "A portion of the given name of the patient", type = "string") 3082 public static final String SP_GIVEN = "given"; 3083 /** 3084 * <b>Fluent Client</b> search parameter constant for <b>given</b> 3085 * <p> 3086 * Description: <b>A portion of the given name of the patient</b><br> 3087 * Type: <b>string</b><br> 3088 * Path: <b>Patient.name.given</b><br> 3089 * </p> 3090 */ 3091 public static final ca.uhn.fhir.rest.gclient.StringClientParam GIVEN = new ca.uhn.fhir.rest.gclient.StringClientParam( 3092 SP_GIVEN); 3093 3094 /** 3095 * Search parameter: <b>address</b> 3096 * <p> 3097 * Description: <b>A server defined search that may match any of the string 3098 * fields in the Address, including line, city, district, state, country, 3099 * postalCode, and/or text</b><br> 3100 * Type: <b>string</b><br> 3101 * Path: <b>Patient.address</b><br> 3102 * </p> 3103 */ 3104 @SearchParamDefinition(name = "address", path = "Patient.address", description = "A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text", type = "string") 3105 public static final String SP_ADDRESS = "address"; 3106 /** 3107 * <b>Fluent Client</b> search parameter constant for <b>address</b> 3108 * <p> 3109 * Description: <b>A server defined search that may match any of the string 3110 * fields in the Address, including line, city, district, state, country, 3111 * postalCode, and/or text</b><br> 3112 * Type: <b>string</b><br> 3113 * Path: <b>Patient.address</b><br> 3114 * </p> 3115 */ 3116 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS = new ca.uhn.fhir.rest.gclient.StringClientParam( 3117 SP_ADDRESS); 3118 3119 /** 3120 * Search parameter: <b>birthdate</b> 3121 * <p> 3122 * Description: <b>The patient's date of birth</b><br> 3123 * Type: <b>date</b><br> 3124 * Path: <b>Patient.birthDate</b><br> 3125 * </p> 3126 */ 3127 @SearchParamDefinition(name = "birthdate", path = "Patient.birthDate", description = "The patient's date of birth", type = "date") 3128 public static final String SP_BIRTHDATE = "birthdate"; 3129 /** 3130 * <b>Fluent Client</b> search parameter constant for <b>birthdate</b> 3131 * <p> 3132 * Description: <b>The patient's date of birth</b><br> 3133 * Type: <b>date</b><br> 3134 * Path: <b>Patient.birthDate</b><br> 3135 * </p> 3136 */ 3137 public static final ca.uhn.fhir.rest.gclient.DateClientParam BIRTHDATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3138 SP_BIRTHDATE); 3139 3140 /** 3141 * Search parameter: <b>deceased</b> 3142 * <p> 3143 * Description: <b>This patient has been marked as deceased, or as a death date 3144 * entered</b><br> 3145 * Type: <b>token</b><br> 3146 * Path: <b>Patient.deceased[x]</b><br> 3147 * </p> 3148 */ 3149 @SearchParamDefinition(name = "deceased", path = "Patient.deceased.exists() and Patient.deceased != false", description = "This patient has been marked as deceased, or as a death date entered", type = "token") 3150 public static final String SP_DECEASED = "deceased"; 3151 /** 3152 * <b>Fluent Client</b> search parameter constant for <b>deceased</b> 3153 * <p> 3154 * Description: <b>This patient has been marked as deceased, or as a death date 3155 * entered</b><br> 3156 * Type: <b>token</b><br> 3157 * Path: <b>Patient.deceased[x]</b><br> 3158 * </p> 3159 */ 3160 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DECEASED = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3161 SP_DECEASED); 3162 3163 /** 3164 * Search parameter: <b>address-state</b> 3165 * <p> 3166 * Description: <b>A state specified in an address</b><br> 3167 * Type: <b>string</b><br> 3168 * Path: <b>Patient.address.state</b><br> 3169 * </p> 3170 */ 3171 @SearchParamDefinition(name = "address-state", path = "Patient.address.state", description = "A state specified in an address", type = "string") 3172 public static final String SP_ADDRESS_STATE = "address-state"; 3173 /** 3174 * <b>Fluent Client</b> search parameter constant for <b>address-state</b> 3175 * <p> 3176 * Description: <b>A state specified in an address</b><br> 3177 * Type: <b>string</b><br> 3178 * Path: <b>Patient.address.state</b><br> 3179 * </p> 3180 */ 3181 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_STATE = new ca.uhn.fhir.rest.gclient.StringClientParam( 3182 SP_ADDRESS_STATE); 3183 3184 /** 3185 * Search parameter: <b>gender</b> 3186 * <p> 3187 * Description: <b>Gender of the patient</b><br> 3188 * Type: <b>token</b><br> 3189 * Path: <b>Patient.gender</b><br> 3190 * </p> 3191 */ 3192 @SearchParamDefinition(name = "gender", path = "Patient.gender", description = "Gender of the patient", type = "token") 3193 public static final String SP_GENDER = "gender"; 3194 /** 3195 * <b>Fluent Client</b> search parameter constant for <b>gender</b> 3196 * <p> 3197 * Description: <b>Gender of the patient</b><br> 3198 * Type: <b>token</b><br> 3199 * Path: <b>Patient.gender</b><br> 3200 * </p> 3201 */ 3202 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GENDER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3203 SP_GENDER); 3204 3205 /** 3206 * Search parameter: <b>general-practitioner</b> 3207 * <p> 3208 * Description: <b>Patient's nominated general practitioner, not the 3209 * organization that manages the record</b><br> 3210 * Type: <b>reference</b><br> 3211 * Path: <b>Patient.generalPractitioner</b><br> 3212 * </p> 3213 */ 3214 @SearchParamDefinition(name = "general-practitioner", path = "Patient.generalPractitioner", description = "Patient's nominated general practitioner, not the organization that manages the record", type = "reference", providesMembershipIn = { 3215 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Organization.class, 3216 Practitioner.class, PractitionerRole.class }) 3217 public static final String SP_GENERAL_PRACTITIONER = "general-practitioner"; 3218 /** 3219 * <b>Fluent Client</b> search parameter constant for 3220 * <b>general-practitioner</b> 3221 * <p> 3222 * Description: <b>Patient's nominated general practitioner, not the 3223 * organization that manages the record</b><br> 3224 * Type: <b>reference</b><br> 3225 * Path: <b>Patient.generalPractitioner</b><br> 3226 * </p> 3227 */ 3228 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam GENERAL_PRACTITIONER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3229 SP_GENERAL_PRACTITIONER); 3230 3231 /** 3232 * Constant for fluent queries to be used to add include statements. Specifies 3233 * the path value of "<b>Patient:general-practitioner</b>". 3234 */ 3235 public static final ca.uhn.fhir.model.api.Include INCLUDE_GENERAL_PRACTITIONER = new ca.uhn.fhir.model.api.Include( 3236 "Patient:general-practitioner").toLocked(); 3237 3238 /** 3239 * Search parameter: <b>link</b> 3240 * <p> 3241 * Description: <b>All patients linked to the given patient</b><br> 3242 * Type: <b>reference</b><br> 3243 * Path: <b>Patient.link.other</b><br> 3244 * </p> 3245 */ 3246 @SearchParamDefinition(name = "link", path = "Patient.link.other", description = "All patients linked to the given patient", type = "reference", providesMembershipIn = { 3247 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3248 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Patient.class, 3249 RelatedPerson.class }) 3250 public static final String SP_LINK = "link"; 3251 /** 3252 * <b>Fluent Client</b> search parameter constant for <b>link</b> 3253 * <p> 3254 * Description: <b>All patients linked to the given patient</b><br> 3255 * Type: <b>reference</b><br> 3256 * Path: <b>Patient.link.other</b><br> 3257 * </p> 3258 */ 3259 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LINK = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3260 SP_LINK); 3261 3262 /** 3263 * Constant for fluent queries to be used to add include statements. Specifies 3264 * the path value of "<b>Patient:link</b>". 3265 */ 3266 public static final ca.uhn.fhir.model.api.Include INCLUDE_LINK = new ca.uhn.fhir.model.api.Include("Patient:link") 3267 .toLocked(); 3268 3269 /** 3270 * Search parameter: <b>active</b> 3271 * <p> 3272 * Description: <b>Whether the patient record is active</b><br> 3273 * Type: <b>token</b><br> 3274 * Path: <b>Patient.active</b><br> 3275 * </p> 3276 */ 3277 @SearchParamDefinition(name = "active", path = "Patient.active", description = "Whether the patient record is active", type = "token") 3278 public static final String SP_ACTIVE = "active"; 3279 /** 3280 * <b>Fluent Client</b> search parameter constant for <b>active</b> 3281 * <p> 3282 * Description: <b>Whether the patient record is active</b><br> 3283 * Type: <b>token</b><br> 3284 * Path: <b>Patient.active</b><br> 3285 * </p> 3286 */ 3287 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3288 SP_ACTIVE); 3289 3290 /** 3291 * Search parameter: <b>language</b> 3292 * <p> 3293 * Description: <b>Language code (irrespective of use value)</b><br> 3294 * Type: <b>token</b><br> 3295 * Path: <b>Patient.communication.language</b><br> 3296 * </p> 3297 */ 3298 @SearchParamDefinition(name = "language", path = "Patient.communication.language", description = "Language code (irrespective of use value)", type = "token") 3299 public static final String SP_LANGUAGE = "language"; 3300 /** 3301 * <b>Fluent Client</b> search parameter constant for <b>language</b> 3302 * <p> 3303 * Description: <b>Language code (irrespective of use value)</b><br> 3304 * Type: <b>token</b><br> 3305 * Path: <b>Patient.communication.language</b><br> 3306 * </p> 3307 */ 3308 public static final ca.uhn.fhir.rest.gclient.TokenClientParam LANGUAGE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3309 SP_LANGUAGE); 3310 3311 /** 3312 * Search parameter: <b>address-postalcode</b> 3313 * <p> 3314 * Description: <b>A postalCode specified in an address</b><br> 3315 * Type: <b>string</b><br> 3316 * Path: <b>Patient.address.postalCode</b><br> 3317 * </p> 3318 */ 3319 @SearchParamDefinition(name = "address-postalcode", path = "Patient.address.postalCode", description = "A postalCode specified in an address", type = "string") 3320 public static final String SP_ADDRESS_POSTALCODE = "address-postalcode"; 3321 /** 3322 * <b>Fluent Client</b> search parameter constant for <b>address-postalcode</b> 3323 * <p> 3324 * Description: <b>A postalCode specified in an address</b><br> 3325 * Type: <b>string</b><br> 3326 * Path: <b>Patient.address.postalCode</b><br> 3327 * </p> 3328 */ 3329 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_POSTALCODE = new ca.uhn.fhir.rest.gclient.StringClientParam( 3330 SP_ADDRESS_POSTALCODE); 3331 3332 /** 3333 * Search parameter: <b>address-country</b> 3334 * <p> 3335 * Description: <b>A country specified in an address</b><br> 3336 * Type: <b>string</b><br> 3337 * Path: <b>Patient.address.country</b><br> 3338 * </p> 3339 */ 3340 @SearchParamDefinition(name = "address-country", path = "Patient.address.country", description = "A country specified in an address", type = "string") 3341 public static final String SP_ADDRESS_COUNTRY = "address-country"; 3342 /** 3343 * <b>Fluent Client</b> search parameter constant for <b>address-country</b> 3344 * <p> 3345 * Description: <b>A country specified in an address</b><br> 3346 * Type: <b>string</b><br> 3347 * Path: <b>Patient.address.country</b><br> 3348 * </p> 3349 */ 3350 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_COUNTRY = new ca.uhn.fhir.rest.gclient.StringClientParam( 3351 SP_ADDRESS_COUNTRY); 3352 3353 /** 3354 * Search parameter: <b>death-date</b> 3355 * <p> 3356 * Description: <b>The date of death has been provided and satisfies this search 3357 * value</b><br> 3358 * Type: <b>date</b><br> 3359 * Path: <b>Patient.deceasedDateTime</b><br> 3360 * </p> 3361 */ 3362 @SearchParamDefinition(name = "death-date", path = "(Patient.deceased as dateTime)", description = "The date of death has been provided and satisfies this search value", type = "date") 3363 public static final String SP_DEATH_DATE = "death-date"; 3364 /** 3365 * <b>Fluent Client</b> search parameter constant for <b>death-date</b> 3366 * <p> 3367 * Description: <b>The date of death has been provided and satisfies this search 3368 * value</b><br> 3369 * Type: <b>date</b><br> 3370 * Path: <b>Patient.deceasedDateTime</b><br> 3371 * </p> 3372 */ 3373 public static final ca.uhn.fhir.rest.gclient.DateClientParam DEATH_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3374 SP_DEATH_DATE); 3375 3376 /** 3377 * Search parameter: <b>phonetic</b> 3378 * <p> 3379 * Description: <b>A portion of either family or given name using some kind of 3380 * phonetic matching algorithm</b><br> 3381 * Type: <b>string</b><br> 3382 * Path: <b>Patient.name</b><br> 3383 * </p> 3384 */ 3385 @SearchParamDefinition(name = "phonetic", path = "Patient.name", description = "A portion of either family or given name using some kind of phonetic matching algorithm", type = "string") 3386 public static final String SP_PHONETIC = "phonetic"; 3387 /** 3388 * <b>Fluent Client</b> search parameter constant for <b>phonetic</b> 3389 * <p> 3390 * Description: <b>A portion of either family or given name using some kind of 3391 * phonetic matching algorithm</b><br> 3392 * Type: <b>string</b><br> 3393 * Path: <b>Patient.name</b><br> 3394 * </p> 3395 */ 3396 public static final ca.uhn.fhir.rest.gclient.StringClientParam PHONETIC = new ca.uhn.fhir.rest.gclient.StringClientParam( 3397 SP_PHONETIC); 3398 3399 /** 3400 * Search parameter: <b>phone</b> 3401 * <p> 3402 * Description: <b>A value in a phone contact</b><br> 3403 * Type: <b>token</b><br> 3404 * Path: <b>Patient.telecom(system=phone)</b><br> 3405 * </p> 3406 */ 3407 @SearchParamDefinition(name = "phone", path = "Patient.telecom.where(system='phone')", description = "A value in a phone contact", type = "token") 3408 public static final String SP_PHONE = "phone"; 3409 /** 3410 * <b>Fluent Client</b> search parameter constant for <b>phone</b> 3411 * <p> 3412 * Description: <b>A value in a phone contact</b><br> 3413 * Type: <b>token</b><br> 3414 * Path: <b>Patient.telecom(system=phone)</b><br> 3415 * </p> 3416 */ 3417 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PHONE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3418 SP_PHONE); 3419 3420 /** 3421 * Search parameter: <b>organization</b> 3422 * <p> 3423 * Description: <b>The organization that is the custodian of the patient 3424 * record</b><br> 3425 * Type: <b>reference</b><br> 3426 * Path: <b>Patient.managingOrganization</b><br> 3427 * </p> 3428 */ 3429 @SearchParamDefinition(name = "organization", path = "Patient.managingOrganization", description = "The organization that is the custodian of the patient record", type = "reference", target = { 3430 Organization.class }) 3431 public static final String SP_ORGANIZATION = "organization"; 3432 /** 3433 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 3434 * <p> 3435 * Description: <b>The organization that is the custodian of the patient 3436 * record</b><br> 3437 * Type: <b>reference</b><br> 3438 * Path: <b>Patient.managingOrganization</b><br> 3439 * </p> 3440 */ 3441 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3442 SP_ORGANIZATION); 3443 3444 /** 3445 * Constant for fluent queries to be used to add include statements. Specifies 3446 * the path value of "<b>Patient:organization</b>". 3447 */ 3448 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include( 3449 "Patient:organization").toLocked(); 3450 3451 /** 3452 * Search parameter: <b>name</b> 3453 * <p> 3454 * Description: <b>A server defined search that may match any of the string 3455 * fields in the HumanName, including family, give, prefix, suffix, suffix, 3456 * and/or text</b><br> 3457 * Type: <b>string</b><br> 3458 * Path: <b>Patient.name</b><br> 3459 * </p> 3460 */ 3461 @SearchParamDefinition(name = "name", path = "Patient.name", description = "A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text", type = "string") 3462 public static final String SP_NAME = "name"; 3463 /** 3464 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3465 * <p> 3466 * Description: <b>A server defined search that may match any of the string 3467 * fields in the HumanName, including family, give, prefix, suffix, suffix, 3468 * and/or text</b><br> 3469 * Type: <b>string</b><br> 3470 * Path: <b>Patient.name</b><br> 3471 * </p> 3472 */ 3473 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 3474 SP_NAME); 3475 3476 /** 3477 * Search parameter: <b>address-use</b> 3478 * <p> 3479 * Description: <b>A use code specified in an address</b><br> 3480 * Type: <b>token</b><br> 3481 * Path: <b>Patient.address.use</b><br> 3482 * </p> 3483 */ 3484 @SearchParamDefinition(name = "address-use", path = "Patient.address.use", description = "A use code specified in an address", type = "token") 3485 public static final String SP_ADDRESS_USE = "address-use"; 3486 /** 3487 * <b>Fluent Client</b> search parameter constant for <b>address-use</b> 3488 * <p> 3489 * Description: <b>A use code specified in an address</b><br> 3490 * Type: <b>token</b><br> 3491 * Path: <b>Patient.address.use</b><br> 3492 * </p> 3493 */ 3494 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADDRESS_USE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3495 SP_ADDRESS_USE); 3496 3497 /** 3498 * Search parameter: <b>telecom</b> 3499 * <p> 3500 * Description: <b>The value in any kind of telecom details of the 3501 * patient</b><br> 3502 * Type: <b>token</b><br> 3503 * Path: <b>Patient.telecom</b><br> 3504 * </p> 3505 */ 3506 @SearchParamDefinition(name = "telecom", path = "Patient.telecom", description = "The value in any kind of telecom details of the patient", type = "token") 3507 public static final String SP_TELECOM = "telecom"; 3508 /** 3509 * <b>Fluent Client</b> search parameter constant for <b>telecom</b> 3510 * <p> 3511 * Description: <b>The value in any kind of telecom details of the 3512 * patient</b><br> 3513 * Type: <b>token</b><br> 3514 * Path: <b>Patient.telecom</b><br> 3515 * </p> 3516 */ 3517 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TELECOM = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3518 SP_TELECOM); 3519 3520 /** 3521 * Search parameter: <b>family</b> 3522 * <p> 3523 * Description: <b>A portion of the family name of the patient</b><br> 3524 * Type: <b>string</b><br> 3525 * Path: <b>Patient.name.family</b><br> 3526 * </p> 3527 */ 3528 @SearchParamDefinition(name = "family", path = "Patient.name.family", description = "A portion of the family name of the patient", type = "string") 3529 public static final String SP_FAMILY = "family"; 3530 /** 3531 * <b>Fluent Client</b> search parameter constant for <b>family</b> 3532 * <p> 3533 * Description: <b>A portion of the family name of the patient</b><br> 3534 * Type: <b>string</b><br> 3535 * Path: <b>Patient.name.family</b><br> 3536 * </p> 3537 */ 3538 public static final ca.uhn.fhir.rest.gclient.StringClientParam FAMILY = new ca.uhn.fhir.rest.gclient.StringClientParam( 3539 SP_FAMILY); 3540 3541 /** 3542 * Search parameter: <b>address-city</b> 3543 * <p> 3544 * Description: <b>A city specified in an address</b><br> 3545 * Type: <b>string</b><br> 3546 * Path: <b>Patient.address.city</b><br> 3547 * </p> 3548 */ 3549 @SearchParamDefinition(name = "address-city", path = "Patient.address.city", description = "A city specified in an address", type = "string") 3550 public static final String SP_ADDRESS_CITY = "address-city"; 3551 /** 3552 * <b>Fluent Client</b> search parameter constant for <b>address-city</b> 3553 * <p> 3554 * Description: <b>A city specified in an address</b><br> 3555 * Type: <b>string</b><br> 3556 * Path: <b>Patient.address.city</b><br> 3557 * </p> 3558 */ 3559 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_CITY = new ca.uhn.fhir.rest.gclient.StringClientParam( 3560 SP_ADDRESS_CITY); 3561 3562 /** 3563 * Search parameter: <b>email</b> 3564 * <p> 3565 * Description: <b>A value in an email contact</b><br> 3566 * Type: <b>token</b><br> 3567 * Path: <b>Patient.telecom(system=email)</b><br> 3568 * </p> 3569 */ 3570 @SearchParamDefinition(name = "email", path = "Patient.telecom.where(system='email')", description = "A value in an email contact", type = "token") 3571 public static final String SP_EMAIL = "email"; 3572 /** 3573 * <b>Fluent Client</b> search parameter constant for <b>email</b> 3574 * <p> 3575 * Description: <b>A value in an email contact</b><br> 3576 * Type: <b>token</b><br> 3577 * Path: <b>Patient.telecom(system=email)</b><br> 3578 * </p> 3579 */ 3580 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EMAIL = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3581 SP_EMAIL); 3582 3583}