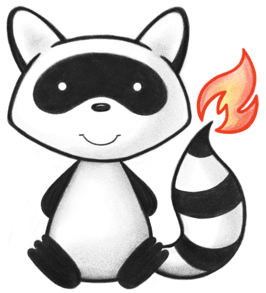
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.Description; 041import ca.uhn.fhir.model.api.annotation.ResourceDef; 042import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 043 044/** 045 * This resource provides the status of the payment for goods and services 046 * rendered, and the request and response resource references. 047 */ 048@ResourceDef(name = "PaymentNotice", profile = "http://hl7.org/fhir/StructureDefinition/PaymentNotice") 049public class PaymentNotice extends DomainResource { 050 051 public enum PaymentNoticeStatus { 052 /** 053 * The instance is currently in-force. 054 */ 055 ACTIVE, 056 /** 057 * The instance is withdrawn, rescinded or reversed. 058 */ 059 CANCELLED, 060 /** 061 * A new instance the contents of which is not complete. 062 */ 063 DRAFT, 064 /** 065 * The instance was entered in error. 066 */ 067 ENTEREDINERROR, 068 /** 069 * added to help the parsers with the generic types 070 */ 071 NULL; 072 073 public static PaymentNoticeStatus fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("active".equals(codeString)) 077 return ACTIVE; 078 if ("cancelled".equals(codeString)) 079 return CANCELLED; 080 if ("draft".equals(codeString)) 081 return DRAFT; 082 if ("entered-in-error".equals(codeString)) 083 return ENTEREDINERROR; 084 if (Configuration.isAcceptInvalidEnums()) 085 return null; 086 else 087 throw new FHIRException("Unknown PaymentNoticeStatus code '" + codeString + "'"); 088 } 089 090 public String toCode() { 091 switch (this) { 092 case ACTIVE: 093 return "active"; 094 case CANCELLED: 095 return "cancelled"; 096 case DRAFT: 097 return "draft"; 098 case ENTEREDINERROR: 099 return "entered-in-error"; 100 case NULL: 101 return null; 102 default: 103 return "?"; 104 } 105 } 106 107 public String getSystem() { 108 switch (this) { 109 case ACTIVE: 110 return "http://hl7.org/fhir/fm-status"; 111 case CANCELLED: 112 return "http://hl7.org/fhir/fm-status"; 113 case DRAFT: 114 return "http://hl7.org/fhir/fm-status"; 115 case ENTEREDINERROR: 116 return "http://hl7.org/fhir/fm-status"; 117 case NULL: 118 return null; 119 default: 120 return "?"; 121 } 122 } 123 124 public String getDefinition() { 125 switch (this) { 126 case ACTIVE: 127 return "The instance is currently in-force."; 128 case CANCELLED: 129 return "The instance is withdrawn, rescinded or reversed."; 130 case DRAFT: 131 return "A new instance the contents of which is not complete."; 132 case ENTEREDINERROR: 133 return "The instance was entered in error."; 134 case NULL: 135 return null; 136 default: 137 return "?"; 138 } 139 } 140 141 public String getDisplay() { 142 switch (this) { 143 case ACTIVE: 144 return "Active"; 145 case CANCELLED: 146 return "Cancelled"; 147 case DRAFT: 148 return "Draft"; 149 case ENTEREDINERROR: 150 return "Entered in Error"; 151 case NULL: 152 return null; 153 default: 154 return "?"; 155 } 156 } 157 } 158 159 public static class PaymentNoticeStatusEnumFactory implements EnumFactory<PaymentNoticeStatus> { 160 public PaymentNoticeStatus fromCode(String codeString) throws IllegalArgumentException { 161 if (codeString == null || "".equals(codeString)) 162 if (codeString == null || "".equals(codeString)) 163 return null; 164 if ("active".equals(codeString)) 165 return PaymentNoticeStatus.ACTIVE; 166 if ("cancelled".equals(codeString)) 167 return PaymentNoticeStatus.CANCELLED; 168 if ("draft".equals(codeString)) 169 return PaymentNoticeStatus.DRAFT; 170 if ("entered-in-error".equals(codeString)) 171 return PaymentNoticeStatus.ENTEREDINERROR; 172 throw new IllegalArgumentException("Unknown PaymentNoticeStatus code '" + codeString + "'"); 173 } 174 175 public Enumeration<PaymentNoticeStatus> fromType(PrimitiveType<?> code) throws FHIRException { 176 if (code == null) 177 return null; 178 if (code.isEmpty()) 179 return new Enumeration<PaymentNoticeStatus>(this, PaymentNoticeStatus.NULL, code); 180 String codeString = code.asStringValue(); 181 if (codeString == null || "".equals(codeString)) 182 return new Enumeration<PaymentNoticeStatus>(this, PaymentNoticeStatus.NULL, code); 183 if ("active".equals(codeString)) 184 return new Enumeration<PaymentNoticeStatus>(this, PaymentNoticeStatus.ACTIVE, code); 185 if ("cancelled".equals(codeString)) 186 return new Enumeration<PaymentNoticeStatus>(this, PaymentNoticeStatus.CANCELLED, code); 187 if ("draft".equals(codeString)) 188 return new Enumeration<PaymentNoticeStatus>(this, PaymentNoticeStatus.DRAFT, code); 189 if ("entered-in-error".equals(codeString)) 190 return new Enumeration<PaymentNoticeStatus>(this, PaymentNoticeStatus.ENTEREDINERROR, code); 191 throw new FHIRException("Unknown PaymentNoticeStatus code '" + codeString + "'"); 192 } 193 194 public String toCode(PaymentNoticeStatus code) { 195 if (code == PaymentNoticeStatus.NULL) 196 return null; 197 if (code == PaymentNoticeStatus.ACTIVE) 198 return "active"; 199 if (code == PaymentNoticeStatus.CANCELLED) 200 return "cancelled"; 201 if (code == PaymentNoticeStatus.DRAFT) 202 return "draft"; 203 if (code == PaymentNoticeStatus.ENTEREDINERROR) 204 return "entered-in-error"; 205 return "?"; 206 } 207 208 public String toSystem(PaymentNoticeStatus code) { 209 return code.getSystem(); 210 } 211 } 212 213 /** 214 * A unique identifier assigned to this payment notice. 215 */ 216 @Child(name = "identifier", type = { 217 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 218 @Description(shortDefinition = "Business Identifier for the payment noctice", formalDefinition = "A unique identifier assigned to this payment notice.") 219 protected List<Identifier> identifier; 220 221 /** 222 * The status of the resource instance. 223 */ 224 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 225 @Description(shortDefinition = "active | cancelled | draft | entered-in-error", formalDefinition = "The status of the resource instance.") 226 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/fm-status") 227 protected Enumeration<PaymentNoticeStatus> status; 228 229 /** 230 * Reference of resource for which payment is being made. 231 */ 232 @Child(name = "request", type = { Reference.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 233 @Description(shortDefinition = "Request reference", formalDefinition = "Reference of resource for which payment is being made.") 234 protected Reference request; 235 236 /** 237 * The actual object that is the target of the reference (Reference of resource 238 * for which payment is being made.) 239 */ 240 protected Resource requestTarget; 241 242 /** 243 * Reference of response to resource for which payment is being made. 244 */ 245 @Child(name = "response", type = { Reference.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 246 @Description(shortDefinition = "Response reference", formalDefinition = "Reference of response to resource for which payment is being made.") 247 protected Reference response; 248 249 /** 250 * The actual object that is the target of the reference (Reference of response 251 * to resource for which payment is being made.) 252 */ 253 protected Resource responseTarget; 254 255 /** 256 * The date when this resource was created. 257 */ 258 @Child(name = "created", type = { DateTimeType.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 259 @Description(shortDefinition = "Creation date", formalDefinition = "The date when this resource was created.") 260 protected DateTimeType created; 261 262 /** 263 * The practitioner who is responsible for the services rendered to the patient. 264 */ 265 @Child(name = "provider", type = { Practitioner.class, PractitionerRole.class, 266 Organization.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 267 @Description(shortDefinition = "Responsible practitioner", formalDefinition = "The practitioner who is responsible for the services rendered to the patient.") 268 protected Reference provider; 269 270 /** 271 * The actual object that is the target of the reference (The practitioner who 272 * is responsible for the services rendered to the patient.) 273 */ 274 protected Resource providerTarget; 275 276 /** 277 * A reference to the payment which is the subject of this notice. 278 */ 279 @Child(name = "payment", type = { 280 PaymentReconciliation.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 281 @Description(shortDefinition = "Payment reference", formalDefinition = "A reference to the payment which is the subject of this notice.") 282 protected Reference payment; 283 284 /** 285 * The actual object that is the target of the reference (A reference to the 286 * payment which is the subject of this notice.) 287 */ 288 protected PaymentReconciliation paymentTarget; 289 290 /** 291 * The date when the above payment action occurred. 292 */ 293 @Child(name = "paymentDate", type = { 294 DateType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 295 @Description(shortDefinition = "Payment or clearing date", formalDefinition = "The date when the above payment action occurred.") 296 protected DateType paymentDate; 297 298 /** 299 * The party who will receive or has received payment that is the subject of 300 * this notification. 301 */ 302 @Child(name = "payee", type = { Practitioner.class, PractitionerRole.class, 303 Organization.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 304 @Description(shortDefinition = "Party being paid", formalDefinition = "The party who will receive or has received payment that is the subject of this notification.") 305 protected Reference payee; 306 307 /** 308 * The actual object that is the target of the reference (The party who will 309 * receive or has received payment that is the subject of this notification.) 310 */ 311 protected Resource payeeTarget; 312 313 /** 314 * The party who is notified of the payment status. 315 */ 316 @Child(name = "recipient", type = { 317 Organization.class }, order = 9, min = 1, max = 1, modifier = false, summary = true) 318 @Description(shortDefinition = "Party being notified", formalDefinition = "The party who is notified of the payment status.") 319 protected Reference recipient; 320 321 /** 322 * The actual object that is the target of the reference (The party who is 323 * notified of the payment status.) 324 */ 325 protected Organization recipientTarget; 326 327 /** 328 * The amount sent to the payee. 329 */ 330 @Child(name = "amount", type = { Money.class }, order = 10, min = 1, max = 1, modifier = false, summary = true) 331 @Description(shortDefinition = "Monetary amount of the payment", formalDefinition = "The amount sent to the payee.") 332 protected Money amount; 333 334 /** 335 * A code indicating whether payment has been sent or cleared. 336 */ 337 @Child(name = "paymentStatus", type = { 338 CodeableConcept.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 339 @Description(shortDefinition = "Issued or cleared Status of the payment", formalDefinition = "A code indicating whether payment has been sent or cleared.") 340 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/payment-status") 341 protected CodeableConcept paymentStatus; 342 343 private static final long serialVersionUID = -545198613L; 344 345 /** 346 * Constructor 347 */ 348 public PaymentNotice() { 349 super(); 350 } 351 352 /** 353 * Constructor 354 */ 355 public PaymentNotice(Enumeration<PaymentNoticeStatus> status, DateTimeType created, Reference payment, 356 Reference recipient, Money amount) { 357 super(); 358 this.status = status; 359 this.created = created; 360 this.payment = payment; 361 this.recipient = recipient; 362 this.amount = amount; 363 } 364 365 /** 366 * @return {@link #identifier} (A unique identifier assigned to this payment 367 * notice.) 368 */ 369 public List<Identifier> getIdentifier() { 370 if (this.identifier == null) 371 this.identifier = new ArrayList<Identifier>(); 372 return this.identifier; 373 } 374 375 /** 376 * @return Returns a reference to <code>this</code> for easy method chaining 377 */ 378 public PaymentNotice setIdentifier(List<Identifier> theIdentifier) { 379 this.identifier = theIdentifier; 380 return this; 381 } 382 383 public boolean hasIdentifier() { 384 if (this.identifier == null) 385 return false; 386 for (Identifier item : this.identifier) 387 if (!item.isEmpty()) 388 return true; 389 return false; 390 } 391 392 public Identifier addIdentifier() { // 3 393 Identifier t = new Identifier(); 394 if (this.identifier == null) 395 this.identifier = new ArrayList<Identifier>(); 396 this.identifier.add(t); 397 return t; 398 } 399 400 public PaymentNotice addIdentifier(Identifier t) { // 3 401 if (t == null) 402 return this; 403 if (this.identifier == null) 404 this.identifier = new ArrayList<Identifier>(); 405 this.identifier.add(t); 406 return this; 407 } 408 409 /** 410 * @return The first repetition of repeating field {@link #identifier}, creating 411 * it if it does not already exist 412 */ 413 public Identifier getIdentifierFirstRep() { 414 if (getIdentifier().isEmpty()) { 415 addIdentifier(); 416 } 417 return getIdentifier().get(0); 418 } 419 420 /** 421 * @return {@link #status} (The status of the resource instance.). This is the 422 * underlying object with id, value and extensions. The accessor 423 * "getStatus" gives direct access to the value 424 */ 425 public Enumeration<PaymentNoticeStatus> getStatusElement() { 426 if (this.status == null) 427 if (Configuration.errorOnAutoCreate()) 428 throw new Error("Attempt to auto-create PaymentNotice.status"); 429 else if (Configuration.doAutoCreate()) 430 this.status = new Enumeration<PaymentNoticeStatus>(new PaymentNoticeStatusEnumFactory()); // bb 431 return this.status; 432 } 433 434 public boolean hasStatusElement() { 435 return this.status != null && !this.status.isEmpty(); 436 } 437 438 public boolean hasStatus() { 439 return this.status != null && !this.status.isEmpty(); 440 } 441 442 /** 443 * @param value {@link #status} (The status of the resource instance.). This is 444 * the underlying object with id, value and extensions. The 445 * accessor "getStatus" gives direct access to the value 446 */ 447 public PaymentNotice setStatusElement(Enumeration<PaymentNoticeStatus> value) { 448 this.status = value; 449 return this; 450 } 451 452 /** 453 * @return The status of the resource instance. 454 */ 455 public PaymentNoticeStatus getStatus() { 456 return this.status == null ? null : this.status.getValue(); 457 } 458 459 /** 460 * @param value The status of the resource instance. 461 */ 462 public PaymentNotice setStatus(PaymentNoticeStatus value) { 463 if (this.status == null) 464 this.status = new Enumeration<PaymentNoticeStatus>(new PaymentNoticeStatusEnumFactory()); 465 this.status.setValue(value); 466 return this; 467 } 468 469 /** 470 * @return {@link #request} (Reference of resource for which payment is being 471 * made.) 472 */ 473 public Reference getRequest() { 474 if (this.request == null) 475 if (Configuration.errorOnAutoCreate()) 476 throw new Error("Attempt to auto-create PaymentNotice.request"); 477 else if (Configuration.doAutoCreate()) 478 this.request = new Reference(); // cc 479 return this.request; 480 } 481 482 public boolean hasRequest() { 483 return this.request != null && !this.request.isEmpty(); 484 } 485 486 /** 487 * @param value {@link #request} (Reference of resource for which payment is 488 * being made.) 489 */ 490 public PaymentNotice setRequest(Reference value) { 491 this.request = value; 492 return this; 493 } 494 495 /** 496 * @return {@link #request} The actual object that is the target of the 497 * reference. The reference library doesn't populate this, but you can 498 * use it to hold the resource if you resolve it. (Reference of resource 499 * for which payment is being made.) 500 */ 501 public Resource getRequestTarget() { 502 return this.requestTarget; 503 } 504 505 /** 506 * @param value {@link #request} The actual object that is the target of the 507 * reference. The reference library doesn't use these, but you can 508 * use it to hold the resource if you resolve it. (Reference of 509 * resource for which payment is being made.) 510 */ 511 public PaymentNotice setRequestTarget(Resource value) { 512 this.requestTarget = value; 513 return this; 514 } 515 516 /** 517 * @return {@link #response} (Reference of response to resource for which 518 * payment is being made.) 519 */ 520 public Reference getResponse() { 521 if (this.response == null) 522 if (Configuration.errorOnAutoCreate()) 523 throw new Error("Attempt to auto-create PaymentNotice.response"); 524 else if (Configuration.doAutoCreate()) 525 this.response = new Reference(); // cc 526 return this.response; 527 } 528 529 public boolean hasResponse() { 530 return this.response != null && !this.response.isEmpty(); 531 } 532 533 /** 534 * @param value {@link #response} (Reference of response to resource for which 535 * payment is being made.) 536 */ 537 public PaymentNotice setResponse(Reference value) { 538 this.response = value; 539 return this; 540 } 541 542 /** 543 * @return {@link #response} The actual object that is the target of the 544 * reference. The reference library doesn't populate this, but you can 545 * use it to hold the resource if you resolve it. (Reference of response 546 * to resource for which payment is being made.) 547 */ 548 public Resource getResponseTarget() { 549 return this.responseTarget; 550 } 551 552 /** 553 * @param value {@link #response} The actual object that is the target of the 554 * reference. The reference library doesn't use these, but you can 555 * use it to hold the resource if you resolve it. (Reference of 556 * response to resource for which payment is being made.) 557 */ 558 public PaymentNotice setResponseTarget(Resource value) { 559 this.responseTarget = value; 560 return this; 561 } 562 563 /** 564 * @return {@link #created} (The date when this resource was created.). This is 565 * the underlying object with id, value and extensions. The accessor 566 * "getCreated" gives direct access to the value 567 */ 568 public DateTimeType getCreatedElement() { 569 if (this.created == null) 570 if (Configuration.errorOnAutoCreate()) 571 throw new Error("Attempt to auto-create PaymentNotice.created"); 572 else if (Configuration.doAutoCreate()) 573 this.created = new DateTimeType(); // bb 574 return this.created; 575 } 576 577 public boolean hasCreatedElement() { 578 return this.created != null && !this.created.isEmpty(); 579 } 580 581 public boolean hasCreated() { 582 return this.created != null && !this.created.isEmpty(); 583 } 584 585 /** 586 * @param value {@link #created} (The date when this resource was created.). 587 * This is the underlying object with id, value and extensions. The 588 * accessor "getCreated" gives direct access to the value 589 */ 590 public PaymentNotice setCreatedElement(DateTimeType value) { 591 this.created = value; 592 return this; 593 } 594 595 /** 596 * @return The date when this resource was created. 597 */ 598 public Date getCreated() { 599 return this.created == null ? null : this.created.getValue(); 600 } 601 602 /** 603 * @param value The date when this resource was created. 604 */ 605 public PaymentNotice setCreated(Date value) { 606 if (this.created == null) 607 this.created = new DateTimeType(); 608 this.created.setValue(value); 609 return this; 610 } 611 612 /** 613 * @return {@link #provider} (The practitioner who is responsible for the 614 * services rendered to the patient.) 615 */ 616 public Reference getProvider() { 617 if (this.provider == null) 618 if (Configuration.errorOnAutoCreate()) 619 throw new Error("Attempt to auto-create PaymentNotice.provider"); 620 else if (Configuration.doAutoCreate()) 621 this.provider = new Reference(); // cc 622 return this.provider; 623 } 624 625 public boolean hasProvider() { 626 return this.provider != null && !this.provider.isEmpty(); 627 } 628 629 /** 630 * @param value {@link #provider} (The practitioner who is responsible for the 631 * services rendered to the patient.) 632 */ 633 public PaymentNotice setProvider(Reference value) { 634 this.provider = value; 635 return this; 636 } 637 638 /** 639 * @return {@link #provider} The actual object that is the target of the 640 * reference. The reference library doesn't populate this, but you can 641 * use it to hold the resource if you resolve it. (The practitioner who 642 * is responsible for the services rendered to the patient.) 643 */ 644 public Resource getProviderTarget() { 645 return this.providerTarget; 646 } 647 648 /** 649 * @param value {@link #provider} The actual object that is the target of the 650 * reference. The reference library doesn't use these, but you can 651 * use it to hold the resource if you resolve it. (The practitioner 652 * who is responsible for the services rendered to the patient.) 653 */ 654 public PaymentNotice setProviderTarget(Resource value) { 655 this.providerTarget = value; 656 return this; 657 } 658 659 /** 660 * @return {@link #payment} (A reference to the payment which is the subject of 661 * this notice.) 662 */ 663 public Reference getPayment() { 664 if (this.payment == null) 665 if (Configuration.errorOnAutoCreate()) 666 throw new Error("Attempt to auto-create PaymentNotice.payment"); 667 else if (Configuration.doAutoCreate()) 668 this.payment = new Reference(); // cc 669 return this.payment; 670 } 671 672 public boolean hasPayment() { 673 return this.payment != null && !this.payment.isEmpty(); 674 } 675 676 /** 677 * @param value {@link #payment} (A reference to the payment which is the 678 * subject of this notice.) 679 */ 680 public PaymentNotice setPayment(Reference value) { 681 this.payment = value; 682 return this; 683 } 684 685 /** 686 * @return {@link #payment} The actual object that is the target of the 687 * reference. The reference library doesn't populate this, but you can 688 * use it to hold the resource if you resolve it. (A reference to the 689 * payment which is the subject of this notice.) 690 */ 691 public PaymentReconciliation getPaymentTarget() { 692 if (this.paymentTarget == null) 693 if (Configuration.errorOnAutoCreate()) 694 throw new Error("Attempt to auto-create PaymentNotice.payment"); 695 else if (Configuration.doAutoCreate()) 696 this.paymentTarget = new PaymentReconciliation(); // aa 697 return this.paymentTarget; 698 } 699 700 /** 701 * @param value {@link #payment} The actual object that is the target of the 702 * reference. The reference library doesn't use these, but you can 703 * use it to hold the resource if you resolve it. (A reference to 704 * the payment which is the subject of this notice.) 705 */ 706 public PaymentNotice setPaymentTarget(PaymentReconciliation value) { 707 this.paymentTarget = value; 708 return this; 709 } 710 711 /** 712 * @return {@link #paymentDate} (The date when the above payment action 713 * occurred.). This is the underlying object with id, value and 714 * extensions. The accessor "getPaymentDate" gives direct access to the 715 * value 716 */ 717 public DateType getPaymentDateElement() { 718 if (this.paymentDate == null) 719 if (Configuration.errorOnAutoCreate()) 720 throw new Error("Attempt to auto-create PaymentNotice.paymentDate"); 721 else if (Configuration.doAutoCreate()) 722 this.paymentDate = new DateType(); // bb 723 return this.paymentDate; 724 } 725 726 public boolean hasPaymentDateElement() { 727 return this.paymentDate != null && !this.paymentDate.isEmpty(); 728 } 729 730 public boolean hasPaymentDate() { 731 return this.paymentDate != null && !this.paymentDate.isEmpty(); 732 } 733 734 /** 735 * @param value {@link #paymentDate} (The date when the above payment action 736 * occurred.). This is the underlying object with id, value and 737 * extensions. The accessor "getPaymentDate" gives direct access to 738 * the value 739 */ 740 public PaymentNotice setPaymentDateElement(DateType value) { 741 this.paymentDate = value; 742 return this; 743 } 744 745 /** 746 * @return The date when the above payment action occurred. 747 */ 748 public Date getPaymentDate() { 749 return this.paymentDate == null ? null : this.paymentDate.getValue(); 750 } 751 752 /** 753 * @param value The date when the above payment action occurred. 754 */ 755 public PaymentNotice setPaymentDate(Date value) { 756 if (value == null) 757 this.paymentDate = null; 758 else { 759 if (this.paymentDate == null) 760 this.paymentDate = new DateType(); 761 this.paymentDate.setValue(value); 762 } 763 return this; 764 } 765 766 /** 767 * @return {@link #payee} (The party who will receive or has received payment 768 * that is the subject of this notification.) 769 */ 770 public Reference getPayee() { 771 if (this.payee == null) 772 if (Configuration.errorOnAutoCreate()) 773 throw new Error("Attempt to auto-create PaymentNotice.payee"); 774 else if (Configuration.doAutoCreate()) 775 this.payee = new Reference(); // cc 776 return this.payee; 777 } 778 779 public boolean hasPayee() { 780 return this.payee != null && !this.payee.isEmpty(); 781 } 782 783 /** 784 * @param value {@link #payee} (The party who will receive or has received 785 * payment that is the subject of this notification.) 786 */ 787 public PaymentNotice setPayee(Reference value) { 788 this.payee = value; 789 return this; 790 } 791 792 /** 793 * @return {@link #payee} The actual object that is the target of the reference. 794 * The reference library doesn't populate this, but you can use it to 795 * hold the resource if you resolve it. (The party who will receive or 796 * has received payment that is the subject of this notification.) 797 */ 798 public Resource getPayeeTarget() { 799 return this.payeeTarget; 800 } 801 802 /** 803 * @param value {@link #payee} The actual object that is the target of the 804 * reference. The reference library doesn't use these, but you can 805 * use it to hold the resource if you resolve it. (The party who 806 * will receive or has received payment that is the subject of this 807 * notification.) 808 */ 809 public PaymentNotice setPayeeTarget(Resource value) { 810 this.payeeTarget = value; 811 return this; 812 } 813 814 /** 815 * @return {@link #recipient} (The party who is notified of the payment status.) 816 */ 817 public Reference getRecipient() { 818 if (this.recipient == null) 819 if (Configuration.errorOnAutoCreate()) 820 throw new Error("Attempt to auto-create PaymentNotice.recipient"); 821 else if (Configuration.doAutoCreate()) 822 this.recipient = new Reference(); // cc 823 return this.recipient; 824 } 825 826 public boolean hasRecipient() { 827 return this.recipient != null && !this.recipient.isEmpty(); 828 } 829 830 /** 831 * @param value {@link #recipient} (The party who is notified of the payment 832 * status.) 833 */ 834 public PaymentNotice setRecipient(Reference value) { 835 this.recipient = value; 836 return this; 837 } 838 839 /** 840 * @return {@link #recipient} The actual object that is the target of the 841 * reference. The reference library doesn't populate this, but you can 842 * use it to hold the resource if you resolve it. (The party who is 843 * notified of the payment status.) 844 */ 845 public Organization getRecipientTarget() { 846 if (this.recipientTarget == null) 847 if (Configuration.errorOnAutoCreate()) 848 throw new Error("Attempt to auto-create PaymentNotice.recipient"); 849 else if (Configuration.doAutoCreate()) 850 this.recipientTarget = new Organization(); // aa 851 return this.recipientTarget; 852 } 853 854 /** 855 * @param value {@link #recipient} The actual object that is the target of the 856 * reference. The reference library doesn't use these, but you can 857 * use it to hold the resource if you resolve it. (The party who is 858 * notified of the payment status.) 859 */ 860 public PaymentNotice setRecipientTarget(Organization value) { 861 this.recipientTarget = value; 862 return this; 863 } 864 865 /** 866 * @return {@link #amount} (The amount sent to the payee.) 867 */ 868 public Money getAmount() { 869 if (this.amount == null) 870 if (Configuration.errorOnAutoCreate()) 871 throw new Error("Attempt to auto-create PaymentNotice.amount"); 872 else if (Configuration.doAutoCreate()) 873 this.amount = new Money(); // cc 874 return this.amount; 875 } 876 877 public boolean hasAmount() { 878 return this.amount != null && !this.amount.isEmpty(); 879 } 880 881 /** 882 * @param value {@link #amount} (The amount sent to the payee.) 883 */ 884 public PaymentNotice setAmount(Money value) { 885 this.amount = value; 886 return this; 887 } 888 889 /** 890 * @return {@link #paymentStatus} (A code indicating whether payment has been 891 * sent or cleared.) 892 */ 893 public CodeableConcept getPaymentStatus() { 894 if (this.paymentStatus == null) 895 if (Configuration.errorOnAutoCreate()) 896 throw new Error("Attempt to auto-create PaymentNotice.paymentStatus"); 897 else if (Configuration.doAutoCreate()) 898 this.paymentStatus = new CodeableConcept(); // cc 899 return this.paymentStatus; 900 } 901 902 public boolean hasPaymentStatus() { 903 return this.paymentStatus != null && !this.paymentStatus.isEmpty(); 904 } 905 906 /** 907 * @param value {@link #paymentStatus} (A code indicating whether payment has 908 * been sent or cleared.) 909 */ 910 public PaymentNotice setPaymentStatus(CodeableConcept value) { 911 this.paymentStatus = value; 912 return this; 913 } 914 915 protected void listChildren(List<Property> children) { 916 super.listChildren(children); 917 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this payment notice.", 0, 918 java.lang.Integer.MAX_VALUE, identifier)); 919 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 920 children.add(new Property("request", "Reference(Any)", "Reference of resource for which payment is being made.", 0, 921 1, request)); 922 children.add(new Property("response", "Reference(Any)", 923 "Reference of response to resource for which payment is being made.", 0, 1, response)); 924 children.add(new Property("created", "dateTime", "The date when this resource was created.", 0, 1, created)); 925 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", 926 "The practitioner who is responsible for the services rendered to the patient.", 0, 1, provider)); 927 children.add(new Property("payment", "Reference(PaymentReconciliation)", 928 "A reference to the payment which is the subject of this notice.", 0, 1, payment)); 929 children.add( 930 new Property("paymentDate", "date", "The date when the above payment action occurred.", 0, 1, paymentDate)); 931 children.add(new Property("payee", "Reference(Practitioner|PractitionerRole|Organization)", 932 "The party who will receive or has received payment that is the subject of this notification.", 0, 1, payee)); 933 children.add(new Property("recipient", "Reference(Organization)", 934 "The party who is notified of the payment status.", 0, 1, recipient)); 935 children.add(new Property("amount", "Money", "The amount sent to the payee.", 0, 1, amount)); 936 children.add(new Property("paymentStatus", "CodeableConcept", 937 "A code indicating whether payment has been sent or cleared.", 0, 1, paymentStatus)); 938 } 939 940 @Override 941 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 942 switch (_hash) { 943 case -1618432855: 944 /* identifier */ return new Property("identifier", "Identifier", 945 "A unique identifier assigned to this payment notice.", 0, java.lang.Integer.MAX_VALUE, identifier); 946 case -892481550: 947 /* status */ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 948 case 1095692943: 949 /* request */ return new Property("request", "Reference(Any)", 950 "Reference of resource for which payment is being made.", 0, 1, request); 951 case -340323263: 952 /* response */ return new Property("response", "Reference(Any)", 953 "Reference of response to resource for which payment is being made.", 0, 1, response); 954 case 1028554472: 955 /* created */ return new Property("created", "dateTime", "The date when this resource was created.", 0, 1, 956 created); 957 case -987494927: 958 /* provider */ return new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", 959 "The practitioner who is responsible for the services rendered to the patient.", 0, 1, provider); 960 case -786681338: 961 /* payment */ return new Property("payment", "Reference(PaymentReconciliation)", 962 "A reference to the payment which is the subject of this notice.", 0, 1, payment); 963 case -1540873516: 964 /* paymentDate */ return new Property("paymentDate", "date", "The date when the above payment action occurred.", 965 0, 1, paymentDate); 966 case 106443592: 967 /* payee */ return new Property("payee", "Reference(Practitioner|PractitionerRole|Organization)", 968 "The party who will receive or has received payment that is the subject of this notification.", 0, 1, payee); 969 case 820081177: 970 /* recipient */ return new Property("recipient", "Reference(Organization)", 971 "The party who is notified of the payment status.", 0, 1, recipient); 972 case -1413853096: 973 /* amount */ return new Property("amount", "Money", "The amount sent to the payee.", 0, 1, amount); 974 case 1430704536: 975 /* paymentStatus */ return new Property("paymentStatus", "CodeableConcept", 976 "A code indicating whether payment has been sent or cleared.", 0, 1, paymentStatus); 977 default: 978 return super.getNamedProperty(_hash, _name, _checkValid); 979 } 980 981 } 982 983 @Override 984 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 985 switch (hash) { 986 case -1618432855: 987 /* identifier */ return this.identifier == null ? new Base[0] 988 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 989 case -892481550: 990 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PaymentNoticeStatus> 991 case 1095692943: 992 /* request */ return this.request == null ? new Base[0] : new Base[] { this.request }; // Reference 993 case -340323263: 994 /* response */ return this.response == null ? new Base[0] : new Base[] { this.response }; // Reference 995 case 1028554472: 996 /* created */ return this.created == null ? new Base[0] : new Base[] { this.created }; // DateTimeType 997 case -987494927: 998 /* provider */ return this.provider == null ? new Base[0] : new Base[] { this.provider }; // Reference 999 case -786681338: 1000 /* payment */ return this.payment == null ? new Base[0] : new Base[] { this.payment }; // Reference 1001 case -1540873516: 1002 /* paymentDate */ return this.paymentDate == null ? new Base[0] : new Base[] { this.paymentDate }; // DateType 1003 case 106443592: 1004 /* payee */ return this.payee == null ? new Base[0] : new Base[] { this.payee }; // Reference 1005 case 820081177: 1006 /* recipient */ return this.recipient == null ? new Base[0] : new Base[] { this.recipient }; // Reference 1007 case -1413853096: 1008 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // Money 1009 case 1430704536: 1010 /* paymentStatus */ return this.paymentStatus == null ? new Base[0] : new Base[] { this.paymentStatus }; // CodeableConcept 1011 default: 1012 return super.getProperty(hash, name, checkValid); 1013 } 1014 1015 } 1016 1017 @Override 1018 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1019 switch (hash) { 1020 case -1618432855: // identifier 1021 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1022 return value; 1023 case -892481550: // status 1024 value = new PaymentNoticeStatusEnumFactory().fromType(castToCode(value)); 1025 this.status = (Enumeration) value; // Enumeration<PaymentNoticeStatus> 1026 return value; 1027 case 1095692943: // request 1028 this.request = castToReference(value); // Reference 1029 return value; 1030 case -340323263: // response 1031 this.response = castToReference(value); // Reference 1032 return value; 1033 case 1028554472: // created 1034 this.created = castToDateTime(value); // DateTimeType 1035 return value; 1036 case -987494927: // provider 1037 this.provider = castToReference(value); // Reference 1038 return value; 1039 case -786681338: // payment 1040 this.payment = castToReference(value); // Reference 1041 return value; 1042 case -1540873516: // paymentDate 1043 this.paymentDate = castToDate(value); // DateType 1044 return value; 1045 case 106443592: // payee 1046 this.payee = castToReference(value); // Reference 1047 return value; 1048 case 820081177: // recipient 1049 this.recipient = castToReference(value); // Reference 1050 return value; 1051 case -1413853096: // amount 1052 this.amount = castToMoney(value); // Money 1053 return value; 1054 case 1430704536: // paymentStatus 1055 this.paymentStatus = castToCodeableConcept(value); // CodeableConcept 1056 return value; 1057 default: 1058 return super.setProperty(hash, name, value); 1059 } 1060 1061 } 1062 1063 @Override 1064 public Base setProperty(String name, Base value) throws FHIRException { 1065 if (name.equals("identifier")) { 1066 this.getIdentifier().add(castToIdentifier(value)); 1067 } else if (name.equals("status")) { 1068 value = new PaymentNoticeStatusEnumFactory().fromType(castToCode(value)); 1069 this.status = (Enumeration) value; // Enumeration<PaymentNoticeStatus> 1070 } else if (name.equals("request")) { 1071 this.request = castToReference(value); // Reference 1072 } else if (name.equals("response")) { 1073 this.response = castToReference(value); // Reference 1074 } else if (name.equals("created")) { 1075 this.created = castToDateTime(value); // DateTimeType 1076 } else if (name.equals("provider")) { 1077 this.provider = castToReference(value); // Reference 1078 } else if (name.equals("payment")) { 1079 this.payment = castToReference(value); // Reference 1080 } else if (name.equals("paymentDate")) { 1081 this.paymentDate = castToDate(value); // DateType 1082 } else if (name.equals("payee")) { 1083 this.payee = castToReference(value); // Reference 1084 } else if (name.equals("recipient")) { 1085 this.recipient = castToReference(value); // Reference 1086 } else if (name.equals("amount")) { 1087 this.amount = castToMoney(value); // Money 1088 } else if (name.equals("paymentStatus")) { 1089 this.paymentStatus = castToCodeableConcept(value); // CodeableConcept 1090 } else 1091 return super.setProperty(name, value); 1092 return value; 1093 } 1094 1095 @Override 1096 public void removeChild(String name, Base value) throws FHIRException { 1097 if (name.equals("identifier")) { 1098 this.getIdentifier().remove(castToIdentifier(value)); 1099 } else if (name.equals("status")) { 1100 this.status = null; 1101 } else if (name.equals("request")) { 1102 this.request = null; 1103 } else if (name.equals("response")) { 1104 this.response = null; 1105 } else if (name.equals("created")) { 1106 this.created = null; 1107 } else if (name.equals("provider")) { 1108 this.provider = null; 1109 } else if (name.equals("payment")) { 1110 this.payment = null; 1111 } else if (name.equals("paymentDate")) { 1112 this.paymentDate = null; 1113 } else if (name.equals("payee")) { 1114 this.payee = null; 1115 } else if (name.equals("recipient")) { 1116 this.recipient = null; 1117 } else if (name.equals("amount")) { 1118 this.amount = null; 1119 } else if (name.equals("paymentStatus")) { 1120 this.paymentStatus = null; 1121 } else 1122 super.removeChild(name, value); 1123 1124 } 1125 1126 @Override 1127 public Base makeProperty(int hash, String name) throws FHIRException { 1128 switch (hash) { 1129 case -1618432855: 1130 return addIdentifier(); 1131 case -892481550: 1132 return getStatusElement(); 1133 case 1095692943: 1134 return getRequest(); 1135 case -340323263: 1136 return getResponse(); 1137 case 1028554472: 1138 return getCreatedElement(); 1139 case -987494927: 1140 return getProvider(); 1141 case -786681338: 1142 return getPayment(); 1143 case -1540873516: 1144 return getPaymentDateElement(); 1145 case 106443592: 1146 return getPayee(); 1147 case 820081177: 1148 return getRecipient(); 1149 case -1413853096: 1150 return getAmount(); 1151 case 1430704536: 1152 return getPaymentStatus(); 1153 default: 1154 return super.makeProperty(hash, name); 1155 } 1156 1157 } 1158 1159 @Override 1160 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1161 switch (hash) { 1162 case -1618432855: 1163 /* identifier */ return new String[] { "Identifier" }; 1164 case -892481550: 1165 /* status */ return new String[] { "code" }; 1166 case 1095692943: 1167 /* request */ return new String[] { "Reference" }; 1168 case -340323263: 1169 /* response */ return new String[] { "Reference" }; 1170 case 1028554472: 1171 /* created */ return new String[] { "dateTime" }; 1172 case -987494927: 1173 /* provider */ return new String[] { "Reference" }; 1174 case -786681338: 1175 /* payment */ return new String[] { "Reference" }; 1176 case -1540873516: 1177 /* paymentDate */ return new String[] { "date" }; 1178 case 106443592: 1179 /* payee */ return new String[] { "Reference" }; 1180 case 820081177: 1181 /* recipient */ return new String[] { "Reference" }; 1182 case -1413853096: 1183 /* amount */ return new String[] { "Money" }; 1184 case 1430704536: 1185 /* paymentStatus */ return new String[] { "CodeableConcept" }; 1186 default: 1187 return super.getTypesForProperty(hash, name); 1188 } 1189 1190 } 1191 1192 @Override 1193 public Base addChild(String name) throws FHIRException { 1194 if (name.equals("identifier")) { 1195 return addIdentifier(); 1196 } else if (name.equals("status")) { 1197 throw new FHIRException("Cannot call addChild on a singleton property PaymentNotice.status"); 1198 } else if (name.equals("request")) { 1199 this.request = new Reference(); 1200 return this.request; 1201 } else if (name.equals("response")) { 1202 this.response = new Reference(); 1203 return this.response; 1204 } else if (name.equals("created")) { 1205 throw new FHIRException("Cannot call addChild on a singleton property PaymentNotice.created"); 1206 } else if (name.equals("provider")) { 1207 this.provider = new Reference(); 1208 return this.provider; 1209 } else if (name.equals("payment")) { 1210 this.payment = new Reference(); 1211 return this.payment; 1212 } else if (name.equals("paymentDate")) { 1213 throw new FHIRException("Cannot call addChild on a singleton property PaymentNotice.paymentDate"); 1214 } else if (name.equals("payee")) { 1215 this.payee = new Reference(); 1216 return this.payee; 1217 } else if (name.equals("recipient")) { 1218 this.recipient = new Reference(); 1219 return this.recipient; 1220 } else if (name.equals("amount")) { 1221 this.amount = new Money(); 1222 return this.amount; 1223 } else if (name.equals("paymentStatus")) { 1224 this.paymentStatus = new CodeableConcept(); 1225 return this.paymentStatus; 1226 } else 1227 return super.addChild(name); 1228 } 1229 1230 public String fhirType() { 1231 return "PaymentNotice"; 1232 1233 } 1234 1235 public PaymentNotice copy() { 1236 PaymentNotice dst = new PaymentNotice(); 1237 copyValues(dst); 1238 return dst; 1239 } 1240 1241 public void copyValues(PaymentNotice dst) { 1242 super.copyValues(dst); 1243 if (identifier != null) { 1244 dst.identifier = new ArrayList<Identifier>(); 1245 for (Identifier i : identifier) 1246 dst.identifier.add(i.copy()); 1247 } 1248 ; 1249 dst.status = status == null ? null : status.copy(); 1250 dst.request = request == null ? null : request.copy(); 1251 dst.response = response == null ? null : response.copy(); 1252 dst.created = created == null ? null : created.copy(); 1253 dst.provider = provider == null ? null : provider.copy(); 1254 dst.payment = payment == null ? null : payment.copy(); 1255 dst.paymentDate = paymentDate == null ? null : paymentDate.copy(); 1256 dst.payee = payee == null ? null : payee.copy(); 1257 dst.recipient = recipient == null ? null : recipient.copy(); 1258 dst.amount = amount == null ? null : amount.copy(); 1259 dst.paymentStatus = paymentStatus == null ? null : paymentStatus.copy(); 1260 } 1261 1262 protected PaymentNotice typedCopy() { 1263 return copy(); 1264 } 1265 1266 @Override 1267 public boolean equalsDeep(Base other_) { 1268 if (!super.equalsDeep(other_)) 1269 return false; 1270 if (!(other_ instanceof PaymentNotice)) 1271 return false; 1272 PaymentNotice o = (PaymentNotice) other_; 1273 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 1274 && compareDeep(request, o.request, true) && compareDeep(response, o.response, true) 1275 && compareDeep(created, o.created, true) && compareDeep(provider, o.provider, true) 1276 && compareDeep(payment, o.payment, true) && compareDeep(paymentDate, o.paymentDate, true) 1277 && compareDeep(payee, o.payee, true) && compareDeep(recipient, o.recipient, true) 1278 && compareDeep(amount, o.amount, true) && compareDeep(paymentStatus, o.paymentStatus, true); 1279 } 1280 1281 @Override 1282 public boolean equalsShallow(Base other_) { 1283 if (!super.equalsShallow(other_)) 1284 return false; 1285 if (!(other_ instanceof PaymentNotice)) 1286 return false; 1287 PaymentNotice o = (PaymentNotice) other_; 1288 return compareValues(status, o.status, true) && compareValues(created, o.created, true) 1289 && compareValues(paymentDate, o.paymentDate, true); 1290 } 1291 1292 public boolean isEmpty() { 1293 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, request, response, created, 1294 provider, payment, paymentDate, payee, recipient, amount, paymentStatus); 1295 } 1296 1297 @Override 1298 public ResourceType getResourceType() { 1299 return ResourceType.PaymentNotice; 1300 } 1301 1302 /** 1303 * Search parameter: <b>identifier</b> 1304 * <p> 1305 * Description: <b>The business identifier of the notice</b><br> 1306 * Type: <b>token</b><br> 1307 * Path: <b>PaymentNotice.identifier</b><br> 1308 * </p> 1309 */ 1310 @SearchParamDefinition(name = "identifier", path = "PaymentNotice.identifier", description = "The business identifier of the notice", type = "token") 1311 public static final String SP_IDENTIFIER = "identifier"; 1312 /** 1313 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1314 * <p> 1315 * Description: <b>The business identifier of the notice</b><br> 1316 * Type: <b>token</b><br> 1317 * Path: <b>PaymentNotice.identifier</b><br> 1318 * </p> 1319 */ 1320 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1321 SP_IDENTIFIER); 1322 1323 /** 1324 * Search parameter: <b>request</b> 1325 * <p> 1326 * Description: <b>The Claim</b><br> 1327 * Type: <b>reference</b><br> 1328 * Path: <b>PaymentNotice.request</b><br> 1329 * </p> 1330 */ 1331 @SearchParamDefinition(name = "request", path = "PaymentNotice.request", description = "The Claim", type = "reference") 1332 public static final String SP_REQUEST = "request"; 1333 /** 1334 * <b>Fluent Client</b> search parameter constant for <b>request</b> 1335 * <p> 1336 * Description: <b>The Claim</b><br> 1337 * Type: <b>reference</b><br> 1338 * Path: <b>PaymentNotice.request</b><br> 1339 * </p> 1340 */ 1341 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUEST = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1342 SP_REQUEST); 1343 1344 /** 1345 * Constant for fluent queries to be used to add include statements. Specifies 1346 * the path value of "<b>PaymentNotice:request</b>". 1347 */ 1348 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUEST = new ca.uhn.fhir.model.api.Include( 1349 "PaymentNotice:request").toLocked(); 1350 1351 /** 1352 * Search parameter: <b>provider</b> 1353 * <p> 1354 * Description: <b>The reference to the provider</b><br> 1355 * Type: <b>reference</b><br> 1356 * Path: <b>PaymentNotice.provider</b><br> 1357 * </p> 1358 */ 1359 @SearchParamDefinition(name = "provider", path = "PaymentNotice.provider", description = "The reference to the provider", type = "reference", providesMembershipIn = { 1360 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Organization.class, 1361 Practitioner.class, PractitionerRole.class }) 1362 public static final String SP_PROVIDER = "provider"; 1363 /** 1364 * <b>Fluent Client</b> search parameter constant for <b>provider</b> 1365 * <p> 1366 * Description: <b>The reference to the provider</b><br> 1367 * Type: <b>reference</b><br> 1368 * Path: <b>PaymentNotice.provider</b><br> 1369 * </p> 1370 */ 1371 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1372 SP_PROVIDER); 1373 1374 /** 1375 * Constant for fluent queries to be used to add include statements. Specifies 1376 * the path value of "<b>PaymentNotice:provider</b>". 1377 */ 1378 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROVIDER = new ca.uhn.fhir.model.api.Include( 1379 "PaymentNotice:provider").toLocked(); 1380 1381 /** 1382 * Search parameter: <b>created</b> 1383 * <p> 1384 * Description: <b>Creation date fro the notice</b><br> 1385 * Type: <b>date</b><br> 1386 * Path: <b>PaymentNotice.created</b><br> 1387 * </p> 1388 */ 1389 @SearchParamDefinition(name = "created", path = "PaymentNotice.created", description = "Creation date fro the notice", type = "date") 1390 public static final String SP_CREATED = "created"; 1391 /** 1392 * <b>Fluent Client</b> search parameter constant for <b>created</b> 1393 * <p> 1394 * Description: <b>Creation date fro the notice</b><br> 1395 * Type: <b>date</b><br> 1396 * Path: <b>PaymentNotice.created</b><br> 1397 * </p> 1398 */ 1399 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam( 1400 SP_CREATED); 1401 1402 /** 1403 * Search parameter: <b>response</b> 1404 * <p> 1405 * Description: <b>The ClaimResponse</b><br> 1406 * Type: <b>reference</b><br> 1407 * Path: <b>PaymentNotice.response</b><br> 1408 * </p> 1409 */ 1410 @SearchParamDefinition(name = "response", path = "PaymentNotice.response", description = "The ClaimResponse", type = "reference") 1411 public static final String SP_RESPONSE = "response"; 1412 /** 1413 * <b>Fluent Client</b> search parameter constant for <b>response</b> 1414 * <p> 1415 * Description: <b>The ClaimResponse</b><br> 1416 * Type: <b>reference</b><br> 1417 * Path: <b>PaymentNotice.response</b><br> 1418 * </p> 1419 */ 1420 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RESPONSE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1421 SP_RESPONSE); 1422 1423 /** 1424 * Constant for fluent queries to be used to add include statements. Specifies 1425 * the path value of "<b>PaymentNotice:response</b>". 1426 */ 1427 public static final ca.uhn.fhir.model.api.Include INCLUDE_RESPONSE = new ca.uhn.fhir.model.api.Include( 1428 "PaymentNotice:response").toLocked(); 1429 1430 /** 1431 * Search parameter: <b>payment-status</b> 1432 * <p> 1433 * Description: <b>The type of payment notice</b><br> 1434 * Type: <b>token</b><br> 1435 * Path: <b>PaymentNotice.paymentStatus</b><br> 1436 * </p> 1437 */ 1438 @SearchParamDefinition(name = "payment-status", path = "PaymentNotice.paymentStatus", description = "The type of payment notice", type = "token") 1439 public static final String SP_PAYMENT_STATUS = "payment-status"; 1440 /** 1441 * <b>Fluent Client</b> search parameter constant for <b>payment-status</b> 1442 * <p> 1443 * Description: <b>The type of payment notice</b><br> 1444 * Type: <b>token</b><br> 1445 * Path: <b>PaymentNotice.paymentStatus</b><br> 1446 * </p> 1447 */ 1448 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PAYMENT_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1449 SP_PAYMENT_STATUS); 1450 1451 /** 1452 * Search parameter: <b>status</b> 1453 * <p> 1454 * Description: <b>The status of the payment notice</b><br> 1455 * Type: <b>token</b><br> 1456 * Path: <b>PaymentNotice.status</b><br> 1457 * </p> 1458 */ 1459 @SearchParamDefinition(name = "status", path = "PaymentNotice.status", description = "The status of the payment notice", type = "token") 1460 public static final String SP_STATUS = "status"; 1461 /** 1462 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1463 * <p> 1464 * Description: <b>The status of the payment notice</b><br> 1465 * Type: <b>token</b><br> 1466 * Path: <b>PaymentNotice.status</b><br> 1467 * </p> 1468 */ 1469 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1470 SP_STATUS); 1471 1472}