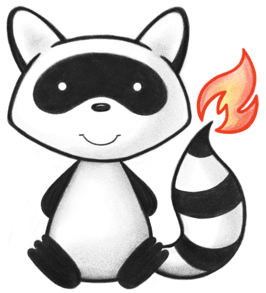
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.NoteType; 040import org.hl7.fhir.r4.model.Enumerations.NoteTypeEnumFactory; 041import org.hl7.fhir.r4.model.Enumerations.RemittanceOutcome; 042import org.hl7.fhir.r4.model.Enumerations.RemittanceOutcomeEnumFactory; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.ResourceDef; 049import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 050 051/** 052 * This resource provides the details including amount of a payment and 053 * allocates the payment items being paid. 054 */ 055@ResourceDef(name = "PaymentReconciliation", profile = "http://hl7.org/fhir/StructureDefinition/PaymentReconciliation") 056public class PaymentReconciliation extends DomainResource { 057 058 public enum PaymentReconciliationStatus { 059 /** 060 * The instance is currently in-force. 061 */ 062 ACTIVE, 063 /** 064 * The instance is withdrawn, rescinded or reversed. 065 */ 066 CANCELLED, 067 /** 068 * A new instance the contents of which is not complete. 069 */ 070 DRAFT, 071 /** 072 * The instance was entered in error. 073 */ 074 ENTEREDINERROR, 075 /** 076 * added to help the parsers with the generic types 077 */ 078 NULL; 079 080 public static PaymentReconciliationStatus fromCode(String codeString) throws FHIRException { 081 if (codeString == null || "".equals(codeString)) 082 return null; 083 if ("active".equals(codeString)) 084 return ACTIVE; 085 if ("cancelled".equals(codeString)) 086 return CANCELLED; 087 if ("draft".equals(codeString)) 088 return DRAFT; 089 if ("entered-in-error".equals(codeString)) 090 return ENTEREDINERROR; 091 if (Configuration.isAcceptInvalidEnums()) 092 return null; 093 else 094 throw new FHIRException("Unknown PaymentReconciliationStatus code '" + codeString + "'"); 095 } 096 097 public String toCode() { 098 switch (this) { 099 case ACTIVE: 100 return "active"; 101 case CANCELLED: 102 return "cancelled"; 103 case DRAFT: 104 return "draft"; 105 case ENTEREDINERROR: 106 return "entered-in-error"; 107 case NULL: 108 return null; 109 default: 110 return "?"; 111 } 112 } 113 114 public String getSystem() { 115 switch (this) { 116 case ACTIVE: 117 return "http://hl7.org/fhir/fm-status"; 118 case CANCELLED: 119 return "http://hl7.org/fhir/fm-status"; 120 case DRAFT: 121 return "http://hl7.org/fhir/fm-status"; 122 case ENTEREDINERROR: 123 return "http://hl7.org/fhir/fm-status"; 124 case NULL: 125 return null; 126 default: 127 return "?"; 128 } 129 } 130 131 public String getDefinition() { 132 switch (this) { 133 case ACTIVE: 134 return "The instance is currently in-force."; 135 case CANCELLED: 136 return "The instance is withdrawn, rescinded or reversed."; 137 case DRAFT: 138 return "A new instance the contents of which is not complete."; 139 case ENTEREDINERROR: 140 return "The instance was entered in error."; 141 case NULL: 142 return null; 143 default: 144 return "?"; 145 } 146 } 147 148 public String getDisplay() { 149 switch (this) { 150 case ACTIVE: 151 return "Active"; 152 case CANCELLED: 153 return "Cancelled"; 154 case DRAFT: 155 return "Draft"; 156 case ENTEREDINERROR: 157 return "Entered in Error"; 158 case NULL: 159 return null; 160 default: 161 return "?"; 162 } 163 } 164 } 165 166 public static class PaymentReconciliationStatusEnumFactory implements EnumFactory<PaymentReconciliationStatus> { 167 public PaymentReconciliationStatus fromCode(String codeString) throws IllegalArgumentException { 168 if (codeString == null || "".equals(codeString)) 169 if (codeString == null || "".equals(codeString)) 170 return null; 171 if ("active".equals(codeString)) 172 return PaymentReconciliationStatus.ACTIVE; 173 if ("cancelled".equals(codeString)) 174 return PaymentReconciliationStatus.CANCELLED; 175 if ("draft".equals(codeString)) 176 return PaymentReconciliationStatus.DRAFT; 177 if ("entered-in-error".equals(codeString)) 178 return PaymentReconciliationStatus.ENTEREDINERROR; 179 throw new IllegalArgumentException("Unknown PaymentReconciliationStatus code '" + codeString + "'"); 180 } 181 182 public Enumeration<PaymentReconciliationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 183 if (code == null) 184 return null; 185 if (code.isEmpty()) 186 return new Enumeration<PaymentReconciliationStatus>(this, PaymentReconciliationStatus.NULL, code); 187 String codeString = code.asStringValue(); 188 if (codeString == null || "".equals(codeString)) 189 return new Enumeration<PaymentReconciliationStatus>(this, PaymentReconciliationStatus.NULL, code); 190 if ("active".equals(codeString)) 191 return new Enumeration<PaymentReconciliationStatus>(this, PaymentReconciliationStatus.ACTIVE, code); 192 if ("cancelled".equals(codeString)) 193 return new Enumeration<PaymentReconciliationStatus>(this, PaymentReconciliationStatus.CANCELLED, code); 194 if ("draft".equals(codeString)) 195 return new Enumeration<PaymentReconciliationStatus>(this, PaymentReconciliationStatus.DRAFT, code); 196 if ("entered-in-error".equals(codeString)) 197 return new Enumeration<PaymentReconciliationStatus>(this, PaymentReconciliationStatus.ENTEREDINERROR, code); 198 throw new FHIRException("Unknown PaymentReconciliationStatus code '" + codeString + "'"); 199 } 200 201 public String toCode(PaymentReconciliationStatus code) { 202 if (code == PaymentReconciliationStatus.NULL) 203 return null; 204 if (code == PaymentReconciliationStatus.ACTIVE) 205 return "active"; 206 if (code == PaymentReconciliationStatus.CANCELLED) 207 return "cancelled"; 208 if (code == PaymentReconciliationStatus.DRAFT) 209 return "draft"; 210 if (code == PaymentReconciliationStatus.ENTEREDINERROR) 211 return "entered-in-error"; 212 return "?"; 213 } 214 215 public String toSystem(PaymentReconciliationStatus code) { 216 return code.getSystem(); 217 } 218 } 219 220 @Block() 221 public static class DetailsComponent extends BackboneElement implements IBaseBackboneElement { 222 /** 223 * Unique identifier for the current payment item for the referenced payable. 224 */ 225 @Child(name = "identifier", type = { 226 Identifier.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 227 @Description(shortDefinition = "Business identifier of the payment detail", formalDefinition = "Unique identifier for the current payment item for the referenced payable.") 228 protected Identifier identifier; 229 230 /** 231 * Unique identifier for the prior payment item for the referenced payable. 232 */ 233 @Child(name = "predecessor", type = { 234 Identifier.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 235 @Description(shortDefinition = "Business identifier of the prior payment detail", formalDefinition = "Unique identifier for the prior payment item for the referenced payable.") 236 protected Identifier predecessor; 237 238 /** 239 * Code to indicate the nature of the payment. 240 */ 241 @Child(name = "type", type = { 242 CodeableConcept.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 243 @Description(shortDefinition = "Category of payment", formalDefinition = "Code to indicate the nature of the payment.") 244 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/payment-type") 245 protected CodeableConcept type; 246 247 /** 248 * A resource, such as a Claim, the evaluation of which could lead to payment. 249 */ 250 @Child(name = "request", type = { Reference.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 251 @Description(shortDefinition = "Request giving rise to the payment", formalDefinition = "A resource, such as a Claim, the evaluation of which could lead to payment.") 252 protected Reference request; 253 254 /** 255 * The actual object that is the target of the reference (A resource, such as a 256 * Claim, the evaluation of which could lead to payment.) 257 */ 258 protected Resource requestTarget; 259 260 /** 261 * The party which submitted the claim or financial transaction. 262 */ 263 @Child(name = "submitter", type = { Practitioner.class, PractitionerRole.class, 264 Organization.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 265 @Description(shortDefinition = "Submitter of the request", formalDefinition = "The party which submitted the claim or financial transaction.") 266 protected Reference submitter; 267 268 /** 269 * The actual object that is the target of the reference (The party which 270 * submitted the claim or financial transaction.) 271 */ 272 protected Resource submitterTarget; 273 274 /** 275 * A resource, such as a ClaimResponse, which contains a commitment to payment. 276 */ 277 @Child(name = "response", type = { 278 Reference.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 279 @Description(shortDefinition = "Response committing to a payment", formalDefinition = "A resource, such as a ClaimResponse, which contains a commitment to payment.") 280 protected Reference response; 281 282 /** 283 * The actual object that is the target of the reference (A resource, such as a 284 * ClaimResponse, which contains a commitment to payment.) 285 */ 286 protected Resource responseTarget; 287 288 /** 289 * The date from the response resource containing a commitment to pay. 290 */ 291 @Child(name = "date", type = { DateType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 292 @Description(shortDefinition = "Date of commitment to pay", formalDefinition = "The date from the response resource containing a commitment to pay.") 293 protected DateType date; 294 295 /** 296 * A reference to the individual who is responsible for inquiries regarding the 297 * response and its payment. 298 */ 299 @Child(name = "responsible", type = { 300 PractitionerRole.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 301 @Description(shortDefinition = "Contact for the response", formalDefinition = "A reference to the individual who is responsible for inquiries regarding the response and its payment.") 302 protected Reference responsible; 303 304 /** 305 * The actual object that is the target of the reference (A reference to the 306 * individual who is responsible for inquiries regarding the response and its 307 * payment.) 308 */ 309 protected PractitionerRole responsibleTarget; 310 311 /** 312 * The party which is receiving the payment. 313 */ 314 @Child(name = "payee", type = { Practitioner.class, PractitionerRole.class, 315 Organization.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 316 @Description(shortDefinition = "Recipient of the payment", formalDefinition = "The party which is receiving the payment.") 317 protected Reference payee; 318 319 /** 320 * The actual object that is the target of the reference (The party which is 321 * receiving the payment.) 322 */ 323 protected Resource payeeTarget; 324 325 /** 326 * The monetary amount allocated from the total payment to the payable. 327 */ 328 @Child(name = "amount", type = { Money.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 329 @Description(shortDefinition = "Amount allocated to this payable", formalDefinition = "The monetary amount allocated from the total payment to the payable.") 330 protected Money amount; 331 332 private static final long serialVersionUID = -1361848619L; 333 334 /** 335 * Constructor 336 */ 337 public DetailsComponent() { 338 super(); 339 } 340 341 /** 342 * Constructor 343 */ 344 public DetailsComponent(CodeableConcept type) { 345 super(); 346 this.type = type; 347 } 348 349 /** 350 * @return {@link #identifier} (Unique identifier for the current payment item 351 * for the referenced payable.) 352 */ 353 public Identifier getIdentifier() { 354 if (this.identifier == null) 355 if (Configuration.errorOnAutoCreate()) 356 throw new Error("Attempt to auto-create DetailsComponent.identifier"); 357 else if (Configuration.doAutoCreate()) 358 this.identifier = new Identifier(); // cc 359 return this.identifier; 360 } 361 362 public boolean hasIdentifier() { 363 return this.identifier != null && !this.identifier.isEmpty(); 364 } 365 366 /** 367 * @param value {@link #identifier} (Unique identifier for the current payment 368 * item for the referenced payable.) 369 */ 370 public DetailsComponent setIdentifier(Identifier value) { 371 this.identifier = value; 372 return this; 373 } 374 375 /** 376 * @return {@link #predecessor} (Unique identifier for the prior payment item 377 * for the referenced payable.) 378 */ 379 public Identifier getPredecessor() { 380 if (this.predecessor == null) 381 if (Configuration.errorOnAutoCreate()) 382 throw new Error("Attempt to auto-create DetailsComponent.predecessor"); 383 else if (Configuration.doAutoCreate()) 384 this.predecessor = new Identifier(); // cc 385 return this.predecessor; 386 } 387 388 public boolean hasPredecessor() { 389 return this.predecessor != null && !this.predecessor.isEmpty(); 390 } 391 392 /** 393 * @param value {@link #predecessor} (Unique identifier for the prior payment 394 * item for the referenced payable.) 395 */ 396 public DetailsComponent setPredecessor(Identifier value) { 397 this.predecessor = value; 398 return this; 399 } 400 401 /** 402 * @return {@link #type} (Code to indicate the nature of the payment.) 403 */ 404 public CodeableConcept getType() { 405 if (this.type == null) 406 if (Configuration.errorOnAutoCreate()) 407 throw new Error("Attempt to auto-create DetailsComponent.type"); 408 else if (Configuration.doAutoCreate()) 409 this.type = new CodeableConcept(); // cc 410 return this.type; 411 } 412 413 public boolean hasType() { 414 return this.type != null && !this.type.isEmpty(); 415 } 416 417 /** 418 * @param value {@link #type} (Code to indicate the nature of the payment.) 419 */ 420 public DetailsComponent setType(CodeableConcept value) { 421 this.type = value; 422 return this; 423 } 424 425 /** 426 * @return {@link #request} (A resource, such as a Claim, the evaluation of 427 * which could lead to payment.) 428 */ 429 public Reference getRequest() { 430 if (this.request == null) 431 if (Configuration.errorOnAutoCreate()) 432 throw new Error("Attempt to auto-create DetailsComponent.request"); 433 else if (Configuration.doAutoCreate()) 434 this.request = new Reference(); // cc 435 return this.request; 436 } 437 438 public boolean hasRequest() { 439 return this.request != null && !this.request.isEmpty(); 440 } 441 442 /** 443 * @param value {@link #request} (A resource, such as a Claim, the evaluation of 444 * which could lead to payment.) 445 */ 446 public DetailsComponent setRequest(Reference value) { 447 this.request = value; 448 return this; 449 } 450 451 /** 452 * @return {@link #request} The actual object that is the target of the 453 * reference. The reference library doesn't populate this, but you can 454 * use it to hold the resource if you resolve it. (A resource, such as a 455 * Claim, the evaluation of which could lead to payment.) 456 */ 457 public Resource getRequestTarget() { 458 return this.requestTarget; 459 } 460 461 /** 462 * @param value {@link #request} The actual object that is the target of the 463 * reference. The reference library doesn't use these, but you can 464 * use it to hold the resource if you resolve it. (A resource, such 465 * as a Claim, the evaluation of which could lead to payment.) 466 */ 467 public DetailsComponent setRequestTarget(Resource value) { 468 this.requestTarget = value; 469 return this; 470 } 471 472 /** 473 * @return {@link #submitter} (The party which submitted the claim or financial 474 * transaction.) 475 */ 476 public Reference getSubmitter() { 477 if (this.submitter == null) 478 if (Configuration.errorOnAutoCreate()) 479 throw new Error("Attempt to auto-create DetailsComponent.submitter"); 480 else if (Configuration.doAutoCreate()) 481 this.submitter = new Reference(); // cc 482 return this.submitter; 483 } 484 485 public boolean hasSubmitter() { 486 return this.submitter != null && !this.submitter.isEmpty(); 487 } 488 489 /** 490 * @param value {@link #submitter} (The party which submitted the claim or 491 * financial transaction.) 492 */ 493 public DetailsComponent setSubmitter(Reference value) { 494 this.submitter = value; 495 return this; 496 } 497 498 /** 499 * @return {@link #submitter} The actual object that is the target of the 500 * reference. The reference library doesn't populate this, but you can 501 * use it to hold the resource if you resolve it. (The party which 502 * submitted the claim or financial transaction.) 503 */ 504 public Resource getSubmitterTarget() { 505 return this.submitterTarget; 506 } 507 508 /** 509 * @param value {@link #submitter} The actual object that is the target of the 510 * reference. The reference library doesn't use these, but you can 511 * use it to hold the resource if you resolve it. (The party which 512 * submitted the claim or financial transaction.) 513 */ 514 public DetailsComponent setSubmitterTarget(Resource value) { 515 this.submitterTarget = value; 516 return this; 517 } 518 519 /** 520 * @return {@link #response} (A resource, such as a ClaimResponse, which 521 * contains a commitment to payment.) 522 */ 523 public Reference getResponse() { 524 if (this.response == null) 525 if (Configuration.errorOnAutoCreate()) 526 throw new Error("Attempt to auto-create DetailsComponent.response"); 527 else if (Configuration.doAutoCreate()) 528 this.response = new Reference(); // cc 529 return this.response; 530 } 531 532 public boolean hasResponse() { 533 return this.response != null && !this.response.isEmpty(); 534 } 535 536 /** 537 * @param value {@link #response} (A resource, such as a ClaimResponse, which 538 * contains a commitment to payment.) 539 */ 540 public DetailsComponent setResponse(Reference value) { 541 this.response = value; 542 return this; 543 } 544 545 /** 546 * @return {@link #response} The actual object that is the target of the 547 * reference. The reference library doesn't populate this, but you can 548 * use it to hold the resource if you resolve it. (A resource, such as a 549 * ClaimResponse, which contains a commitment to payment.) 550 */ 551 public Resource getResponseTarget() { 552 return this.responseTarget; 553 } 554 555 /** 556 * @param value {@link #response} The actual object that is the target of the 557 * reference. The reference library doesn't use these, but you can 558 * use it to hold the resource if you resolve it. (A resource, such 559 * as a ClaimResponse, which contains a commitment to payment.) 560 */ 561 public DetailsComponent setResponseTarget(Resource value) { 562 this.responseTarget = value; 563 return this; 564 } 565 566 /** 567 * @return {@link #date} (The date from the response resource containing a 568 * commitment to pay.). This is the underlying object with id, value and 569 * extensions. The accessor "getDate" gives direct access to the value 570 */ 571 public DateType getDateElement() { 572 if (this.date == null) 573 if (Configuration.errorOnAutoCreate()) 574 throw new Error("Attempt to auto-create DetailsComponent.date"); 575 else if (Configuration.doAutoCreate()) 576 this.date = new DateType(); // bb 577 return this.date; 578 } 579 580 public boolean hasDateElement() { 581 return this.date != null && !this.date.isEmpty(); 582 } 583 584 public boolean hasDate() { 585 return this.date != null && !this.date.isEmpty(); 586 } 587 588 /** 589 * @param value {@link #date} (The date from the response resource containing a 590 * commitment to pay.). This is the underlying object with id, 591 * value and extensions. The accessor "getDate" gives direct access 592 * to the value 593 */ 594 public DetailsComponent setDateElement(DateType value) { 595 this.date = value; 596 return this; 597 } 598 599 /** 600 * @return The date from the response resource containing a commitment to pay. 601 */ 602 public Date getDate() { 603 return this.date == null ? null : this.date.getValue(); 604 } 605 606 /** 607 * @param value The date from the response resource containing a commitment to 608 * pay. 609 */ 610 public DetailsComponent setDate(Date value) { 611 if (value == null) 612 this.date = null; 613 else { 614 if (this.date == null) 615 this.date = new DateType(); 616 this.date.setValue(value); 617 } 618 return this; 619 } 620 621 /** 622 * @return {@link #responsible} (A reference to the individual who is 623 * responsible for inquiries regarding the response and its payment.) 624 */ 625 public Reference getResponsible() { 626 if (this.responsible == null) 627 if (Configuration.errorOnAutoCreate()) 628 throw new Error("Attempt to auto-create DetailsComponent.responsible"); 629 else if (Configuration.doAutoCreate()) 630 this.responsible = new Reference(); // cc 631 return this.responsible; 632 } 633 634 public boolean hasResponsible() { 635 return this.responsible != null && !this.responsible.isEmpty(); 636 } 637 638 /** 639 * @param value {@link #responsible} (A reference to the individual who is 640 * responsible for inquiries regarding the response and its 641 * payment.) 642 */ 643 public DetailsComponent setResponsible(Reference value) { 644 this.responsible = value; 645 return this; 646 } 647 648 /** 649 * @return {@link #responsible} The actual object that is the target of the 650 * reference. The reference library doesn't populate this, but you can 651 * use it to hold the resource if you resolve it. (A reference to the 652 * individual who is responsible for inquiries regarding the response 653 * and its payment.) 654 */ 655 public PractitionerRole getResponsibleTarget() { 656 if (this.responsibleTarget == null) 657 if (Configuration.errorOnAutoCreate()) 658 throw new Error("Attempt to auto-create DetailsComponent.responsible"); 659 else if (Configuration.doAutoCreate()) 660 this.responsibleTarget = new PractitionerRole(); // aa 661 return this.responsibleTarget; 662 } 663 664 /** 665 * @param value {@link #responsible} The actual object that is the target of the 666 * reference. The reference library doesn't use these, but you can 667 * use it to hold the resource if you resolve it. (A reference to 668 * the individual who is responsible for inquiries regarding the 669 * response and its payment.) 670 */ 671 public DetailsComponent setResponsibleTarget(PractitionerRole value) { 672 this.responsibleTarget = value; 673 return this; 674 } 675 676 /** 677 * @return {@link #payee} (The party which is receiving the payment.) 678 */ 679 public Reference getPayee() { 680 if (this.payee == null) 681 if (Configuration.errorOnAutoCreate()) 682 throw new Error("Attempt to auto-create DetailsComponent.payee"); 683 else if (Configuration.doAutoCreate()) 684 this.payee = new Reference(); // cc 685 return this.payee; 686 } 687 688 public boolean hasPayee() { 689 return this.payee != null && !this.payee.isEmpty(); 690 } 691 692 /** 693 * @param value {@link #payee} (The party which is receiving the payment.) 694 */ 695 public DetailsComponent setPayee(Reference value) { 696 this.payee = value; 697 return this; 698 } 699 700 /** 701 * @return {@link #payee} The actual object that is the target of the reference. 702 * The reference library doesn't populate this, but you can use it to 703 * hold the resource if you resolve it. (The party which is receiving 704 * the payment.) 705 */ 706 public Resource getPayeeTarget() { 707 return this.payeeTarget; 708 } 709 710 /** 711 * @param value {@link #payee} The actual object that is the target of the 712 * reference. The reference library doesn't use these, but you can 713 * use it to hold the resource if you resolve it. (The party which 714 * is receiving the payment.) 715 */ 716 public DetailsComponent setPayeeTarget(Resource value) { 717 this.payeeTarget = value; 718 return this; 719 } 720 721 /** 722 * @return {@link #amount} (The monetary amount allocated from the total payment 723 * to the payable.) 724 */ 725 public Money getAmount() { 726 if (this.amount == null) 727 if (Configuration.errorOnAutoCreate()) 728 throw new Error("Attempt to auto-create DetailsComponent.amount"); 729 else if (Configuration.doAutoCreate()) 730 this.amount = new Money(); // cc 731 return this.amount; 732 } 733 734 public boolean hasAmount() { 735 return this.amount != null && !this.amount.isEmpty(); 736 } 737 738 /** 739 * @param value {@link #amount} (The monetary amount allocated from the total 740 * payment to the payable.) 741 */ 742 public DetailsComponent setAmount(Money value) { 743 this.amount = value; 744 return this; 745 } 746 747 protected void listChildren(List<Property> children) { 748 super.listChildren(children); 749 children.add(new Property("identifier", "Identifier", 750 "Unique identifier for the current payment item for the referenced payable.", 0, 1, identifier)); 751 children.add(new Property("predecessor", "Identifier", 752 "Unique identifier for the prior payment item for the referenced payable.", 0, 1, predecessor)); 753 children.add(new Property("type", "CodeableConcept", "Code to indicate the nature of the payment.", 0, 1, type)); 754 children.add(new Property("request", "Reference(Any)", 755 "A resource, such as a Claim, the evaluation of which could lead to payment.", 0, 1, request)); 756 children.add(new Property("submitter", "Reference(Practitioner|PractitionerRole|Organization)", 757 "The party which submitted the claim or financial transaction.", 0, 1, submitter)); 758 children.add(new Property("response", "Reference(Any)", 759 "A resource, such as a ClaimResponse, which contains a commitment to payment.", 0, 1, response)); 760 children.add(new Property("date", "date", "The date from the response resource containing a commitment to pay.", 761 0, 1, date)); 762 children.add(new Property("responsible", "Reference(PractitionerRole)", 763 "A reference to the individual who is responsible for inquiries regarding the response and its payment.", 0, 764 1, responsible)); 765 children.add(new Property("payee", "Reference(Practitioner|PractitionerRole|Organization)", 766 "The party which is receiving the payment.", 0, 1, payee)); 767 children.add(new Property("amount", "Money", 768 "The monetary amount allocated from the total payment to the payable.", 0, 1, amount)); 769 } 770 771 @Override 772 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 773 switch (_hash) { 774 case -1618432855: 775 /* identifier */ return new Property("identifier", "Identifier", 776 "Unique identifier for the current payment item for the referenced payable.", 0, 1, identifier); 777 case -1925032183: 778 /* predecessor */ return new Property("predecessor", "Identifier", 779 "Unique identifier for the prior payment item for the referenced payable.", 0, 1, predecessor); 780 case 3575610: 781 /* type */ return new Property("type", "CodeableConcept", "Code to indicate the nature of the payment.", 0, 1, 782 type); 783 case 1095692943: 784 /* request */ return new Property("request", "Reference(Any)", 785 "A resource, such as a Claim, the evaluation of which could lead to payment.", 0, 1, request); 786 case 348678409: 787 /* submitter */ return new Property("submitter", "Reference(Practitioner|PractitionerRole|Organization)", 788 "The party which submitted the claim or financial transaction.", 0, 1, submitter); 789 case -340323263: 790 /* response */ return new Property("response", "Reference(Any)", 791 "A resource, such as a ClaimResponse, which contains a commitment to payment.", 0, 1, response); 792 case 3076014: 793 /* date */ return new Property("date", "date", 794 "The date from the response resource containing a commitment to pay.", 0, 1, date); 795 case 1847674614: 796 /* responsible */ return new Property("responsible", "Reference(PractitionerRole)", 797 "A reference to the individual who is responsible for inquiries regarding the response and its payment.", 0, 798 1, responsible); 799 case 106443592: 800 /* payee */ return new Property("payee", "Reference(Practitioner|PractitionerRole|Organization)", 801 "The party which is receiving the payment.", 0, 1, payee); 802 case -1413853096: 803 /* amount */ return new Property("amount", "Money", 804 "The monetary amount allocated from the total payment to the payable.", 0, 1, amount); 805 default: 806 return super.getNamedProperty(_hash, _name, _checkValid); 807 } 808 809 } 810 811 @Override 812 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 813 switch (hash) { 814 case -1618432855: 815 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 816 case -1925032183: 817 /* predecessor */ return this.predecessor == null ? new Base[0] : new Base[] { this.predecessor }; // Identifier 818 case 3575610: 819 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 820 case 1095692943: 821 /* request */ return this.request == null ? new Base[0] : new Base[] { this.request }; // Reference 822 case 348678409: 823 /* submitter */ return this.submitter == null ? new Base[0] : new Base[] { this.submitter }; // Reference 824 case -340323263: 825 /* response */ return this.response == null ? new Base[0] : new Base[] { this.response }; // Reference 826 case 3076014: 827 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateType 828 case 1847674614: 829 /* responsible */ return this.responsible == null ? new Base[0] : new Base[] { this.responsible }; // Reference 830 case 106443592: 831 /* payee */ return this.payee == null ? new Base[0] : new Base[] { this.payee }; // Reference 832 case -1413853096: 833 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // Money 834 default: 835 return super.getProperty(hash, name, checkValid); 836 } 837 838 } 839 840 @Override 841 public Base setProperty(int hash, String name, Base value) throws FHIRException { 842 switch (hash) { 843 case -1618432855: // identifier 844 this.identifier = castToIdentifier(value); // Identifier 845 return value; 846 case -1925032183: // predecessor 847 this.predecessor = castToIdentifier(value); // Identifier 848 return value; 849 case 3575610: // type 850 this.type = castToCodeableConcept(value); // CodeableConcept 851 return value; 852 case 1095692943: // request 853 this.request = castToReference(value); // Reference 854 return value; 855 case 348678409: // submitter 856 this.submitter = castToReference(value); // Reference 857 return value; 858 case -340323263: // response 859 this.response = castToReference(value); // Reference 860 return value; 861 case 3076014: // date 862 this.date = castToDate(value); // DateType 863 return value; 864 case 1847674614: // responsible 865 this.responsible = castToReference(value); // Reference 866 return value; 867 case 106443592: // payee 868 this.payee = castToReference(value); // Reference 869 return value; 870 case -1413853096: // amount 871 this.amount = castToMoney(value); // Money 872 return value; 873 default: 874 return super.setProperty(hash, name, value); 875 } 876 877 } 878 879 @Override 880 public Base setProperty(String name, Base value) throws FHIRException { 881 if (name.equals("identifier")) { 882 this.identifier = castToIdentifier(value); // Identifier 883 } else if (name.equals("predecessor")) { 884 this.predecessor = castToIdentifier(value); // Identifier 885 } else if (name.equals("type")) { 886 this.type = castToCodeableConcept(value); // CodeableConcept 887 } else if (name.equals("request")) { 888 this.request = castToReference(value); // Reference 889 } else if (name.equals("submitter")) { 890 this.submitter = castToReference(value); // Reference 891 } else if (name.equals("response")) { 892 this.response = castToReference(value); // Reference 893 } else if (name.equals("date")) { 894 this.date = castToDate(value); // DateType 895 } else if (name.equals("responsible")) { 896 this.responsible = castToReference(value); // Reference 897 } else if (name.equals("payee")) { 898 this.payee = castToReference(value); // Reference 899 } else if (name.equals("amount")) { 900 this.amount = castToMoney(value); // Money 901 } else 902 return super.setProperty(name, value); 903 return value; 904 } 905 906 @Override 907 public void removeChild(String name, Base value) throws FHIRException { 908 if (name.equals("identifier")) { 909 this.identifier = null; 910 } else if (name.equals("predecessor")) { 911 this.predecessor = null; 912 } else if (name.equals("type")) { 913 this.type = null; 914 } else if (name.equals("request")) { 915 this.request = null; 916 } else if (name.equals("submitter")) { 917 this.submitter = null; 918 } else if (name.equals("response")) { 919 this.response = null; 920 } else if (name.equals("date")) { 921 this.date = null; 922 } else if (name.equals("responsible")) { 923 this.responsible = null; 924 } else if (name.equals("payee")) { 925 this.payee = null; 926 } else if (name.equals("amount")) { 927 this.amount = null; 928 } else 929 super.removeChild(name, value); 930 931 } 932 933 @Override 934 public Base makeProperty(int hash, String name) throws FHIRException { 935 switch (hash) { 936 case -1618432855: 937 return getIdentifier(); 938 case -1925032183: 939 return getPredecessor(); 940 case 3575610: 941 return getType(); 942 case 1095692943: 943 return getRequest(); 944 case 348678409: 945 return getSubmitter(); 946 case -340323263: 947 return getResponse(); 948 case 3076014: 949 return getDateElement(); 950 case 1847674614: 951 return getResponsible(); 952 case 106443592: 953 return getPayee(); 954 case -1413853096: 955 return getAmount(); 956 default: 957 return super.makeProperty(hash, name); 958 } 959 960 } 961 962 @Override 963 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 964 switch (hash) { 965 case -1618432855: 966 /* identifier */ return new String[] { "Identifier" }; 967 case -1925032183: 968 /* predecessor */ return new String[] { "Identifier" }; 969 case 3575610: 970 /* type */ return new String[] { "CodeableConcept" }; 971 case 1095692943: 972 /* request */ return new String[] { "Reference" }; 973 case 348678409: 974 /* submitter */ return new String[] { "Reference" }; 975 case -340323263: 976 /* response */ return new String[] { "Reference" }; 977 case 3076014: 978 /* date */ return new String[] { "date" }; 979 case 1847674614: 980 /* responsible */ return new String[] { "Reference" }; 981 case 106443592: 982 /* payee */ return new String[] { "Reference" }; 983 case -1413853096: 984 /* amount */ return new String[] { "Money" }; 985 default: 986 return super.getTypesForProperty(hash, name); 987 } 988 989 } 990 991 @Override 992 public Base addChild(String name) throws FHIRException { 993 if (name.equals("identifier")) { 994 this.identifier = new Identifier(); 995 return this.identifier; 996 } else if (name.equals("predecessor")) { 997 this.predecessor = new Identifier(); 998 return this.predecessor; 999 } else if (name.equals("type")) { 1000 this.type = new CodeableConcept(); 1001 return this.type; 1002 } else if (name.equals("request")) { 1003 this.request = new Reference(); 1004 return this.request; 1005 } else if (name.equals("submitter")) { 1006 this.submitter = new Reference(); 1007 return this.submitter; 1008 } else if (name.equals("response")) { 1009 this.response = new Reference(); 1010 return this.response; 1011 } else if (name.equals("date")) { 1012 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.date"); 1013 } else if (name.equals("responsible")) { 1014 this.responsible = new Reference(); 1015 return this.responsible; 1016 } else if (name.equals("payee")) { 1017 this.payee = new Reference(); 1018 return this.payee; 1019 } else if (name.equals("amount")) { 1020 this.amount = new Money(); 1021 return this.amount; 1022 } else 1023 return super.addChild(name); 1024 } 1025 1026 public DetailsComponent copy() { 1027 DetailsComponent dst = new DetailsComponent(); 1028 copyValues(dst); 1029 return dst; 1030 } 1031 1032 public void copyValues(DetailsComponent dst) { 1033 super.copyValues(dst); 1034 dst.identifier = identifier == null ? null : identifier.copy(); 1035 dst.predecessor = predecessor == null ? null : predecessor.copy(); 1036 dst.type = type == null ? null : type.copy(); 1037 dst.request = request == null ? null : request.copy(); 1038 dst.submitter = submitter == null ? null : submitter.copy(); 1039 dst.response = response == null ? null : response.copy(); 1040 dst.date = date == null ? null : date.copy(); 1041 dst.responsible = responsible == null ? null : responsible.copy(); 1042 dst.payee = payee == null ? null : payee.copy(); 1043 dst.amount = amount == null ? null : amount.copy(); 1044 } 1045 1046 @Override 1047 public boolean equalsDeep(Base other_) { 1048 if (!super.equalsDeep(other_)) 1049 return false; 1050 if (!(other_ instanceof DetailsComponent)) 1051 return false; 1052 DetailsComponent o = (DetailsComponent) other_; 1053 return compareDeep(identifier, o.identifier, true) && compareDeep(predecessor, o.predecessor, true) 1054 && compareDeep(type, o.type, true) && compareDeep(request, o.request, true) 1055 && compareDeep(submitter, o.submitter, true) && compareDeep(response, o.response, true) 1056 && compareDeep(date, o.date, true) && compareDeep(responsible, o.responsible, true) 1057 && compareDeep(payee, o.payee, true) && compareDeep(amount, o.amount, true); 1058 } 1059 1060 @Override 1061 public boolean equalsShallow(Base other_) { 1062 if (!super.equalsShallow(other_)) 1063 return false; 1064 if (!(other_ instanceof DetailsComponent)) 1065 return false; 1066 DetailsComponent o = (DetailsComponent) other_; 1067 return compareValues(date, o.date, true); 1068 } 1069 1070 public boolean isEmpty() { 1071 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, predecessor, type, request, submitter, 1072 response, date, responsible, payee, amount); 1073 } 1074 1075 public String fhirType() { 1076 return "PaymentReconciliation.detail"; 1077 1078 } 1079 1080 } 1081 1082 @Block() 1083 public static class NotesComponent extends BackboneElement implements IBaseBackboneElement { 1084 /** 1085 * The business purpose of the note text. 1086 */ 1087 @Child(name = "type", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1088 @Description(shortDefinition = "display | print | printoper", formalDefinition = "The business purpose of the note text.") 1089 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/note-type") 1090 protected Enumeration<NoteType> type; 1091 1092 /** 1093 * The explanation or description associated with the processing. 1094 */ 1095 @Child(name = "text", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1096 @Description(shortDefinition = "Note explanatory text", formalDefinition = "The explanation or description associated with the processing.") 1097 protected StringType text; 1098 1099 private static final long serialVersionUID = 529250161L; 1100 1101 /** 1102 * Constructor 1103 */ 1104 public NotesComponent() { 1105 super(); 1106 } 1107 1108 /** 1109 * @return {@link #type} (The business purpose of the note text.). This is the 1110 * underlying object with id, value and extensions. The accessor 1111 * "getType" gives direct access to the value 1112 */ 1113 public Enumeration<NoteType> getTypeElement() { 1114 if (this.type == null) 1115 if (Configuration.errorOnAutoCreate()) 1116 throw new Error("Attempt to auto-create NotesComponent.type"); 1117 else if (Configuration.doAutoCreate()) 1118 this.type = new Enumeration<NoteType>(new NoteTypeEnumFactory()); // bb 1119 return this.type; 1120 } 1121 1122 public boolean hasTypeElement() { 1123 return this.type != null && !this.type.isEmpty(); 1124 } 1125 1126 public boolean hasType() { 1127 return this.type != null && !this.type.isEmpty(); 1128 } 1129 1130 /** 1131 * @param value {@link #type} (The business purpose of the note text.). This is 1132 * the underlying object with id, value and extensions. The 1133 * accessor "getType" gives direct access to the value 1134 */ 1135 public NotesComponent setTypeElement(Enumeration<NoteType> value) { 1136 this.type = value; 1137 return this; 1138 } 1139 1140 /** 1141 * @return The business purpose of the note text. 1142 */ 1143 public NoteType getType() { 1144 return this.type == null ? null : this.type.getValue(); 1145 } 1146 1147 /** 1148 * @param value The business purpose of the note text. 1149 */ 1150 public NotesComponent setType(NoteType value) { 1151 if (value == null) 1152 this.type = null; 1153 else { 1154 if (this.type == null) 1155 this.type = new Enumeration<NoteType>(new NoteTypeEnumFactory()); 1156 this.type.setValue(value); 1157 } 1158 return this; 1159 } 1160 1161 /** 1162 * @return {@link #text} (The explanation or description associated with the 1163 * processing.). This is the underlying object with id, value and 1164 * extensions. The accessor "getText" gives direct access to the value 1165 */ 1166 public StringType getTextElement() { 1167 if (this.text == null) 1168 if (Configuration.errorOnAutoCreate()) 1169 throw new Error("Attempt to auto-create NotesComponent.text"); 1170 else if (Configuration.doAutoCreate()) 1171 this.text = new StringType(); // bb 1172 return this.text; 1173 } 1174 1175 public boolean hasTextElement() { 1176 return this.text != null && !this.text.isEmpty(); 1177 } 1178 1179 public boolean hasText() { 1180 return this.text != null && !this.text.isEmpty(); 1181 } 1182 1183 /** 1184 * @param value {@link #text} (The explanation or description associated with 1185 * the processing.). This is the underlying object with id, value 1186 * and extensions. The accessor "getText" gives direct access to 1187 * the value 1188 */ 1189 public NotesComponent setTextElement(StringType value) { 1190 this.text = value; 1191 return this; 1192 } 1193 1194 /** 1195 * @return The explanation or description associated with the processing. 1196 */ 1197 public String getText() { 1198 return this.text == null ? null : this.text.getValue(); 1199 } 1200 1201 /** 1202 * @param value The explanation or description associated with the processing. 1203 */ 1204 public NotesComponent setText(String value) { 1205 if (Utilities.noString(value)) 1206 this.text = null; 1207 else { 1208 if (this.text == null) 1209 this.text = new StringType(); 1210 this.text.setValue(value); 1211 } 1212 return this; 1213 } 1214 1215 protected void listChildren(List<Property> children) { 1216 super.listChildren(children); 1217 children.add(new Property("type", "code", "The business purpose of the note text.", 0, 1, type)); 1218 children.add( 1219 new Property("text", "string", "The explanation or description associated with the processing.", 0, 1, text)); 1220 } 1221 1222 @Override 1223 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1224 switch (_hash) { 1225 case 3575610: 1226 /* type */ return new Property("type", "code", "The business purpose of the note text.", 0, 1, type); 1227 case 3556653: 1228 /* text */ return new Property("text", "string", 1229 "The explanation or description associated with the processing.", 0, 1, text); 1230 default: 1231 return super.getNamedProperty(_hash, _name, _checkValid); 1232 } 1233 1234 } 1235 1236 @Override 1237 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1238 switch (hash) { 1239 case 3575610: 1240 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<NoteType> 1241 case 3556653: 1242 /* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // StringType 1243 default: 1244 return super.getProperty(hash, name, checkValid); 1245 } 1246 1247 } 1248 1249 @Override 1250 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1251 switch (hash) { 1252 case 3575610: // type 1253 value = new NoteTypeEnumFactory().fromType(castToCode(value)); 1254 this.type = (Enumeration) value; // Enumeration<NoteType> 1255 return value; 1256 case 3556653: // text 1257 this.text = castToString(value); // StringType 1258 return value; 1259 default: 1260 return super.setProperty(hash, name, value); 1261 } 1262 1263 } 1264 1265 @Override 1266 public Base setProperty(String name, Base value) throws FHIRException { 1267 if (name.equals("type")) { 1268 value = new NoteTypeEnumFactory().fromType(castToCode(value)); 1269 this.type = (Enumeration) value; // Enumeration<NoteType> 1270 } else if (name.equals("text")) { 1271 this.text = castToString(value); // StringType 1272 } else 1273 return super.setProperty(name, value); 1274 return value; 1275 } 1276 1277 @Override 1278 public void removeChild(String name, Base value) throws FHIRException { 1279 if (name.equals("type")) { 1280 this.type = null; 1281 } else if (name.equals("text")) { 1282 this.text = null; 1283 } else 1284 super.removeChild(name, value); 1285 1286 } 1287 1288 @Override 1289 public Base makeProperty(int hash, String name) throws FHIRException { 1290 switch (hash) { 1291 case 3575610: 1292 return getTypeElement(); 1293 case 3556653: 1294 return getTextElement(); 1295 default: 1296 return super.makeProperty(hash, name); 1297 } 1298 1299 } 1300 1301 @Override 1302 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1303 switch (hash) { 1304 case 3575610: 1305 /* type */ return new String[] { "code" }; 1306 case 3556653: 1307 /* text */ return new String[] { "string" }; 1308 default: 1309 return super.getTypesForProperty(hash, name); 1310 } 1311 1312 } 1313 1314 @Override 1315 public Base addChild(String name) throws FHIRException { 1316 if (name.equals("type")) { 1317 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.type"); 1318 } else if (name.equals("text")) { 1319 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.text"); 1320 } else 1321 return super.addChild(name); 1322 } 1323 1324 public NotesComponent copy() { 1325 NotesComponent dst = new NotesComponent(); 1326 copyValues(dst); 1327 return dst; 1328 } 1329 1330 public void copyValues(NotesComponent dst) { 1331 super.copyValues(dst); 1332 dst.type = type == null ? null : type.copy(); 1333 dst.text = text == null ? null : text.copy(); 1334 } 1335 1336 @Override 1337 public boolean equalsDeep(Base other_) { 1338 if (!super.equalsDeep(other_)) 1339 return false; 1340 if (!(other_ instanceof NotesComponent)) 1341 return false; 1342 NotesComponent o = (NotesComponent) other_; 1343 return compareDeep(type, o.type, true) && compareDeep(text, o.text, true); 1344 } 1345 1346 @Override 1347 public boolean equalsShallow(Base other_) { 1348 if (!super.equalsShallow(other_)) 1349 return false; 1350 if (!(other_ instanceof NotesComponent)) 1351 return false; 1352 NotesComponent o = (NotesComponent) other_; 1353 return compareValues(type, o.type, true) && compareValues(text, o.text, true); 1354 } 1355 1356 public boolean isEmpty() { 1357 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, text); 1358 } 1359 1360 public String fhirType() { 1361 return "PaymentReconciliation.processNote"; 1362 1363 } 1364 1365 } 1366 1367 /** 1368 * A unique identifier assigned to this payment reconciliation. 1369 */ 1370 @Child(name = "identifier", type = { 1371 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1372 @Description(shortDefinition = "Business Identifier for a payment reconciliation", formalDefinition = "A unique identifier assigned to this payment reconciliation.") 1373 protected List<Identifier> identifier; 1374 1375 /** 1376 * The status of the resource instance. 1377 */ 1378 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 1379 @Description(shortDefinition = "active | cancelled | draft | entered-in-error", formalDefinition = "The status of the resource instance.") 1380 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/fm-status") 1381 protected Enumeration<PaymentReconciliationStatus> status; 1382 1383 /** 1384 * The period of time for which payments have been gathered into this bulk 1385 * payment for settlement. 1386 */ 1387 @Child(name = "period", type = { Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1388 @Description(shortDefinition = "Period covered", formalDefinition = "The period of time for which payments have been gathered into this bulk payment for settlement.") 1389 protected Period period; 1390 1391 /** 1392 * The date when the resource was created. 1393 */ 1394 @Child(name = "created", type = { DateTimeType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 1395 @Description(shortDefinition = "Creation date", formalDefinition = "The date when the resource was created.") 1396 protected DateTimeType created; 1397 1398 /** 1399 * The party who generated the payment. 1400 */ 1401 @Child(name = "paymentIssuer", type = { 1402 Organization.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1403 @Description(shortDefinition = "Party generating payment", formalDefinition = "The party who generated the payment.") 1404 protected Reference paymentIssuer; 1405 1406 /** 1407 * The actual object that is the target of the reference (The party who 1408 * generated the payment.) 1409 */ 1410 protected Organization paymentIssuerTarget; 1411 1412 /** 1413 * Original request resource reference. 1414 */ 1415 @Child(name = "request", type = { Task.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1416 @Description(shortDefinition = "Reference to requesting resource", formalDefinition = "Original request resource reference.") 1417 protected Reference request; 1418 1419 /** 1420 * The actual object that is the target of the reference (Original request 1421 * resource reference.) 1422 */ 1423 protected Task requestTarget; 1424 1425 /** 1426 * The practitioner who is responsible for the services rendered to the patient. 1427 */ 1428 @Child(name = "requestor", type = { Practitioner.class, PractitionerRole.class, 1429 Organization.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 1430 @Description(shortDefinition = "Responsible practitioner", formalDefinition = "The practitioner who is responsible for the services rendered to the patient.") 1431 protected Reference requestor; 1432 1433 /** 1434 * The actual object that is the target of the reference (The practitioner who 1435 * is responsible for the services rendered to the patient.) 1436 */ 1437 protected Resource requestorTarget; 1438 1439 /** 1440 * The outcome of a request for a reconciliation. 1441 */ 1442 @Child(name = "outcome", type = { CodeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 1443 @Description(shortDefinition = "queued | complete | error | partial", formalDefinition = "The outcome of a request for a reconciliation.") 1444 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/remittance-outcome") 1445 protected Enumeration<RemittanceOutcome> outcome; 1446 1447 /** 1448 * A human readable description of the status of the request for the 1449 * reconciliation. 1450 */ 1451 @Child(name = "disposition", type = { 1452 StringType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 1453 @Description(shortDefinition = "Disposition message", formalDefinition = "A human readable description of the status of the request for the reconciliation.") 1454 protected StringType disposition; 1455 1456 /** 1457 * The date of payment as indicated on the financial instrument. 1458 */ 1459 @Child(name = "paymentDate", type = { DateType.class }, order = 9, min = 1, max = 1, modifier = false, summary = true) 1460 @Description(shortDefinition = "When payment issued", formalDefinition = "The date of payment as indicated on the financial instrument.") 1461 protected DateType paymentDate; 1462 1463 /** 1464 * Total payment amount as indicated on the financial instrument. 1465 */ 1466 @Child(name = "paymentAmount", type = { Money.class }, order = 10, min = 1, max = 1, modifier = false, summary = true) 1467 @Description(shortDefinition = "Total amount of Payment", formalDefinition = "Total payment amount as indicated on the financial instrument.") 1468 protected Money paymentAmount; 1469 1470 /** 1471 * Issuer's unique identifier for the payment instrument. 1472 */ 1473 @Child(name = "paymentIdentifier", type = { 1474 Identifier.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 1475 @Description(shortDefinition = "Business identifier for the payment", formalDefinition = "Issuer's unique identifier for the payment instrument.") 1476 protected Identifier paymentIdentifier; 1477 1478 /** 1479 * Distribution of the payment amount for a previously acknowledged payable. 1480 */ 1481 @Child(name = "detail", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1482 @Description(shortDefinition = "Settlement particulars", formalDefinition = "Distribution of the payment amount for a previously acknowledged payable.") 1483 protected List<DetailsComponent> detail; 1484 1485 /** 1486 * A code for the form to be used for printing the content. 1487 */ 1488 @Child(name = "formCode", type = { 1489 CodeableConcept.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 1490 @Description(shortDefinition = "Printed form identifier", formalDefinition = "A code for the form to be used for printing the content.") 1491 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/forms") 1492 protected CodeableConcept formCode; 1493 1494 /** 1495 * A note that describes or explains the processing in a human readable form. 1496 */ 1497 @Child(name = "processNote", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1498 @Description(shortDefinition = "Note concerning processing", formalDefinition = "A note that describes or explains the processing in a human readable form.") 1499 protected List<NotesComponent> processNote; 1500 1501 private static final long serialVersionUID = -1620965037L; 1502 1503 /** 1504 * Constructor 1505 */ 1506 public PaymentReconciliation() { 1507 super(); 1508 } 1509 1510 /** 1511 * Constructor 1512 */ 1513 public PaymentReconciliation(Enumeration<PaymentReconciliationStatus> status, DateTimeType created, 1514 DateType paymentDate, Money paymentAmount) { 1515 super(); 1516 this.status = status; 1517 this.created = created; 1518 this.paymentDate = paymentDate; 1519 this.paymentAmount = paymentAmount; 1520 } 1521 1522 /** 1523 * @return {@link #identifier} (A unique identifier assigned to this payment 1524 * reconciliation.) 1525 */ 1526 public List<Identifier> getIdentifier() { 1527 if (this.identifier == null) 1528 this.identifier = new ArrayList<Identifier>(); 1529 return this.identifier; 1530 } 1531 1532 /** 1533 * @return Returns a reference to <code>this</code> for easy method chaining 1534 */ 1535 public PaymentReconciliation setIdentifier(List<Identifier> theIdentifier) { 1536 this.identifier = theIdentifier; 1537 return this; 1538 } 1539 1540 public boolean hasIdentifier() { 1541 if (this.identifier == null) 1542 return false; 1543 for (Identifier item : this.identifier) 1544 if (!item.isEmpty()) 1545 return true; 1546 return false; 1547 } 1548 1549 public Identifier addIdentifier() { // 3 1550 Identifier t = new Identifier(); 1551 if (this.identifier == null) 1552 this.identifier = new ArrayList<Identifier>(); 1553 this.identifier.add(t); 1554 return t; 1555 } 1556 1557 public PaymentReconciliation addIdentifier(Identifier t) { // 3 1558 if (t == null) 1559 return this; 1560 if (this.identifier == null) 1561 this.identifier = new ArrayList<Identifier>(); 1562 this.identifier.add(t); 1563 return this; 1564 } 1565 1566 /** 1567 * @return The first repetition of repeating field {@link #identifier}, creating 1568 * it if it does not already exist 1569 */ 1570 public Identifier getIdentifierFirstRep() { 1571 if (getIdentifier().isEmpty()) { 1572 addIdentifier(); 1573 } 1574 return getIdentifier().get(0); 1575 } 1576 1577 /** 1578 * @return {@link #status} (The status of the resource instance.). This is the 1579 * underlying object with id, value and extensions. The accessor 1580 * "getStatus" gives direct access to the value 1581 */ 1582 public Enumeration<PaymentReconciliationStatus> getStatusElement() { 1583 if (this.status == null) 1584 if (Configuration.errorOnAutoCreate()) 1585 throw new Error("Attempt to auto-create PaymentReconciliation.status"); 1586 else if (Configuration.doAutoCreate()) 1587 this.status = new Enumeration<PaymentReconciliationStatus>(new PaymentReconciliationStatusEnumFactory()); // bb 1588 return this.status; 1589 } 1590 1591 public boolean hasStatusElement() { 1592 return this.status != null && !this.status.isEmpty(); 1593 } 1594 1595 public boolean hasStatus() { 1596 return this.status != null && !this.status.isEmpty(); 1597 } 1598 1599 /** 1600 * @param value {@link #status} (The status of the resource instance.). This is 1601 * the underlying object with id, value and extensions. The 1602 * accessor "getStatus" gives direct access to the value 1603 */ 1604 public PaymentReconciliation setStatusElement(Enumeration<PaymentReconciliationStatus> value) { 1605 this.status = value; 1606 return this; 1607 } 1608 1609 /** 1610 * @return The status of the resource instance. 1611 */ 1612 public PaymentReconciliationStatus getStatus() { 1613 return this.status == null ? null : this.status.getValue(); 1614 } 1615 1616 /** 1617 * @param value The status of the resource instance. 1618 */ 1619 public PaymentReconciliation setStatus(PaymentReconciliationStatus value) { 1620 if (this.status == null) 1621 this.status = new Enumeration<PaymentReconciliationStatus>(new PaymentReconciliationStatusEnumFactory()); 1622 this.status.setValue(value); 1623 return this; 1624 } 1625 1626 /** 1627 * @return {@link #period} (The period of time for which payments have been 1628 * gathered into this bulk payment for settlement.) 1629 */ 1630 public Period getPeriod() { 1631 if (this.period == null) 1632 if (Configuration.errorOnAutoCreate()) 1633 throw new Error("Attempt to auto-create PaymentReconciliation.period"); 1634 else if (Configuration.doAutoCreate()) 1635 this.period = new Period(); // cc 1636 return this.period; 1637 } 1638 1639 public boolean hasPeriod() { 1640 return this.period != null && !this.period.isEmpty(); 1641 } 1642 1643 /** 1644 * @param value {@link #period} (The period of time for which payments have been 1645 * gathered into this bulk payment for settlement.) 1646 */ 1647 public PaymentReconciliation setPeriod(Period value) { 1648 this.period = value; 1649 return this; 1650 } 1651 1652 /** 1653 * @return {@link #created} (The date when the resource was created.). This is 1654 * the underlying object with id, value and extensions. The accessor 1655 * "getCreated" gives direct access to the value 1656 */ 1657 public DateTimeType getCreatedElement() { 1658 if (this.created == null) 1659 if (Configuration.errorOnAutoCreate()) 1660 throw new Error("Attempt to auto-create PaymentReconciliation.created"); 1661 else if (Configuration.doAutoCreate()) 1662 this.created = new DateTimeType(); // bb 1663 return this.created; 1664 } 1665 1666 public boolean hasCreatedElement() { 1667 return this.created != null && !this.created.isEmpty(); 1668 } 1669 1670 public boolean hasCreated() { 1671 return this.created != null && !this.created.isEmpty(); 1672 } 1673 1674 /** 1675 * @param value {@link #created} (The date when the resource was created.). This 1676 * is the underlying object with id, value and extensions. The 1677 * accessor "getCreated" gives direct access to the value 1678 */ 1679 public PaymentReconciliation setCreatedElement(DateTimeType value) { 1680 this.created = value; 1681 return this; 1682 } 1683 1684 /** 1685 * @return The date when the resource was created. 1686 */ 1687 public Date getCreated() { 1688 return this.created == null ? null : this.created.getValue(); 1689 } 1690 1691 /** 1692 * @param value The date when the resource was created. 1693 */ 1694 public PaymentReconciliation setCreated(Date value) { 1695 if (this.created == null) 1696 this.created = new DateTimeType(); 1697 this.created.setValue(value); 1698 return this; 1699 } 1700 1701 /** 1702 * @return {@link #paymentIssuer} (The party who generated the payment.) 1703 */ 1704 public Reference getPaymentIssuer() { 1705 if (this.paymentIssuer == null) 1706 if (Configuration.errorOnAutoCreate()) 1707 throw new Error("Attempt to auto-create PaymentReconciliation.paymentIssuer"); 1708 else if (Configuration.doAutoCreate()) 1709 this.paymentIssuer = new Reference(); // cc 1710 return this.paymentIssuer; 1711 } 1712 1713 public boolean hasPaymentIssuer() { 1714 return this.paymentIssuer != null && !this.paymentIssuer.isEmpty(); 1715 } 1716 1717 /** 1718 * @param value {@link #paymentIssuer} (The party who generated the payment.) 1719 */ 1720 public PaymentReconciliation setPaymentIssuer(Reference value) { 1721 this.paymentIssuer = value; 1722 return this; 1723 } 1724 1725 /** 1726 * @return {@link #paymentIssuer} The actual object that is the target of the 1727 * reference. The reference library doesn't populate this, but you can 1728 * use it to hold the resource if you resolve it. (The party who 1729 * generated the payment.) 1730 */ 1731 public Organization getPaymentIssuerTarget() { 1732 if (this.paymentIssuerTarget == null) 1733 if (Configuration.errorOnAutoCreate()) 1734 throw new Error("Attempt to auto-create PaymentReconciliation.paymentIssuer"); 1735 else if (Configuration.doAutoCreate()) 1736 this.paymentIssuerTarget = new Organization(); // aa 1737 return this.paymentIssuerTarget; 1738 } 1739 1740 /** 1741 * @param value {@link #paymentIssuer} The actual object that is the target of 1742 * the reference. The reference library doesn't use these, but you 1743 * can use it to hold the resource if you resolve it. (The party 1744 * who generated the payment.) 1745 */ 1746 public PaymentReconciliation setPaymentIssuerTarget(Organization value) { 1747 this.paymentIssuerTarget = value; 1748 return this; 1749 } 1750 1751 /** 1752 * @return {@link #request} (Original request resource reference.) 1753 */ 1754 public Reference getRequest() { 1755 if (this.request == null) 1756 if (Configuration.errorOnAutoCreate()) 1757 throw new Error("Attempt to auto-create PaymentReconciliation.request"); 1758 else if (Configuration.doAutoCreate()) 1759 this.request = new Reference(); // cc 1760 return this.request; 1761 } 1762 1763 public boolean hasRequest() { 1764 return this.request != null && !this.request.isEmpty(); 1765 } 1766 1767 /** 1768 * @param value {@link #request} (Original request resource reference.) 1769 */ 1770 public PaymentReconciliation setRequest(Reference value) { 1771 this.request = value; 1772 return this; 1773 } 1774 1775 /** 1776 * @return {@link #request} The actual object that is the target of the 1777 * reference. The reference library doesn't populate this, but you can 1778 * use it to hold the resource if you resolve it. (Original request 1779 * resource reference.) 1780 */ 1781 public Task getRequestTarget() { 1782 if (this.requestTarget == null) 1783 if (Configuration.errorOnAutoCreate()) 1784 throw new Error("Attempt to auto-create PaymentReconciliation.request"); 1785 else if (Configuration.doAutoCreate()) 1786 this.requestTarget = new Task(); // aa 1787 return this.requestTarget; 1788 } 1789 1790 /** 1791 * @param value {@link #request} The actual object that is the target of the 1792 * reference. The reference library doesn't use these, but you can 1793 * use it to hold the resource if you resolve it. (Original request 1794 * resource reference.) 1795 */ 1796 public PaymentReconciliation setRequestTarget(Task value) { 1797 this.requestTarget = value; 1798 return this; 1799 } 1800 1801 /** 1802 * @return {@link #requestor} (The practitioner who is responsible for the 1803 * services rendered to the patient.) 1804 */ 1805 public Reference getRequestor() { 1806 if (this.requestor == null) 1807 if (Configuration.errorOnAutoCreate()) 1808 throw new Error("Attempt to auto-create PaymentReconciliation.requestor"); 1809 else if (Configuration.doAutoCreate()) 1810 this.requestor = new Reference(); // cc 1811 return this.requestor; 1812 } 1813 1814 public boolean hasRequestor() { 1815 return this.requestor != null && !this.requestor.isEmpty(); 1816 } 1817 1818 /** 1819 * @param value {@link #requestor} (The practitioner who is responsible for the 1820 * services rendered to the patient.) 1821 */ 1822 public PaymentReconciliation setRequestor(Reference value) { 1823 this.requestor = value; 1824 return this; 1825 } 1826 1827 /** 1828 * @return {@link #requestor} The actual object that is the target of the 1829 * reference. The reference library doesn't populate this, but you can 1830 * use it to hold the resource if you resolve it. (The practitioner who 1831 * is responsible for the services rendered to the patient.) 1832 */ 1833 public Resource getRequestorTarget() { 1834 return this.requestorTarget; 1835 } 1836 1837 /** 1838 * @param value {@link #requestor} The actual object that is the target of the 1839 * reference. The reference library doesn't use these, but you can 1840 * use it to hold the resource if you resolve it. (The practitioner 1841 * who is responsible for the services rendered to the patient.) 1842 */ 1843 public PaymentReconciliation setRequestorTarget(Resource value) { 1844 this.requestorTarget = value; 1845 return this; 1846 } 1847 1848 /** 1849 * @return {@link #outcome} (The outcome of a request for a reconciliation.). 1850 * This is the underlying object with id, value and extensions. The 1851 * accessor "getOutcome" gives direct access to the value 1852 */ 1853 public Enumeration<RemittanceOutcome> getOutcomeElement() { 1854 if (this.outcome == null) 1855 if (Configuration.errorOnAutoCreate()) 1856 throw new Error("Attempt to auto-create PaymentReconciliation.outcome"); 1857 else if (Configuration.doAutoCreate()) 1858 this.outcome = new Enumeration<RemittanceOutcome>(new RemittanceOutcomeEnumFactory()); // bb 1859 return this.outcome; 1860 } 1861 1862 public boolean hasOutcomeElement() { 1863 return this.outcome != null && !this.outcome.isEmpty(); 1864 } 1865 1866 public boolean hasOutcome() { 1867 return this.outcome != null && !this.outcome.isEmpty(); 1868 } 1869 1870 /** 1871 * @param value {@link #outcome} (The outcome of a request for a 1872 * reconciliation.). This is the underlying object with id, value 1873 * and extensions. The accessor "getOutcome" gives direct access to 1874 * the value 1875 */ 1876 public PaymentReconciliation setOutcomeElement(Enumeration<RemittanceOutcome> value) { 1877 this.outcome = value; 1878 return this; 1879 } 1880 1881 /** 1882 * @return The outcome of a request for a reconciliation. 1883 */ 1884 public RemittanceOutcome getOutcome() { 1885 return this.outcome == null ? null : this.outcome.getValue(); 1886 } 1887 1888 /** 1889 * @param value The outcome of a request for a reconciliation. 1890 */ 1891 public PaymentReconciliation setOutcome(RemittanceOutcome value) { 1892 if (value == null) 1893 this.outcome = null; 1894 else { 1895 if (this.outcome == null) 1896 this.outcome = new Enumeration<RemittanceOutcome>(new RemittanceOutcomeEnumFactory()); 1897 this.outcome.setValue(value); 1898 } 1899 return this; 1900 } 1901 1902 /** 1903 * @return {@link #disposition} (A human readable description of the status of 1904 * the request for the reconciliation.). This is the underlying object 1905 * with id, value and extensions. The accessor "getDisposition" gives 1906 * direct access to the value 1907 */ 1908 public StringType getDispositionElement() { 1909 if (this.disposition == null) 1910 if (Configuration.errorOnAutoCreate()) 1911 throw new Error("Attempt to auto-create PaymentReconciliation.disposition"); 1912 else if (Configuration.doAutoCreate()) 1913 this.disposition = new StringType(); // bb 1914 return this.disposition; 1915 } 1916 1917 public boolean hasDispositionElement() { 1918 return this.disposition != null && !this.disposition.isEmpty(); 1919 } 1920 1921 public boolean hasDisposition() { 1922 return this.disposition != null && !this.disposition.isEmpty(); 1923 } 1924 1925 /** 1926 * @param value {@link #disposition} (A human readable description of the status 1927 * of the request for the reconciliation.). This is the underlying 1928 * object with id, value and extensions. The accessor 1929 * "getDisposition" gives direct access to the value 1930 */ 1931 public PaymentReconciliation setDispositionElement(StringType value) { 1932 this.disposition = value; 1933 return this; 1934 } 1935 1936 /** 1937 * @return A human readable description of the status of the request for the 1938 * reconciliation. 1939 */ 1940 public String getDisposition() { 1941 return this.disposition == null ? null : this.disposition.getValue(); 1942 } 1943 1944 /** 1945 * @param value A human readable description of the status of the request for 1946 * the reconciliation. 1947 */ 1948 public PaymentReconciliation setDisposition(String value) { 1949 if (Utilities.noString(value)) 1950 this.disposition = null; 1951 else { 1952 if (this.disposition == null) 1953 this.disposition = new StringType(); 1954 this.disposition.setValue(value); 1955 } 1956 return this; 1957 } 1958 1959 /** 1960 * @return {@link #paymentDate} (The date of payment as indicated on the 1961 * financial instrument.). This is the underlying object with id, value 1962 * and extensions. The accessor "getPaymentDate" gives direct access to 1963 * the value 1964 */ 1965 public DateType getPaymentDateElement() { 1966 if (this.paymentDate == null) 1967 if (Configuration.errorOnAutoCreate()) 1968 throw new Error("Attempt to auto-create PaymentReconciliation.paymentDate"); 1969 else if (Configuration.doAutoCreate()) 1970 this.paymentDate = new DateType(); // bb 1971 return this.paymentDate; 1972 } 1973 1974 public boolean hasPaymentDateElement() { 1975 return this.paymentDate != null && !this.paymentDate.isEmpty(); 1976 } 1977 1978 public boolean hasPaymentDate() { 1979 return this.paymentDate != null && !this.paymentDate.isEmpty(); 1980 } 1981 1982 /** 1983 * @param value {@link #paymentDate} (The date of payment as indicated on the 1984 * financial instrument.). This is the underlying object with id, 1985 * value and extensions. The accessor "getPaymentDate" gives direct 1986 * access to the value 1987 */ 1988 public PaymentReconciliation setPaymentDateElement(DateType value) { 1989 this.paymentDate = value; 1990 return this; 1991 } 1992 1993 /** 1994 * @return The date of payment as indicated on the financial instrument. 1995 */ 1996 public Date getPaymentDate() { 1997 return this.paymentDate == null ? null : this.paymentDate.getValue(); 1998 } 1999 2000 /** 2001 * @param value The date of payment as indicated on the financial instrument. 2002 */ 2003 public PaymentReconciliation setPaymentDate(Date value) { 2004 if (this.paymentDate == null) 2005 this.paymentDate = new DateType(); 2006 this.paymentDate.setValue(value); 2007 return this; 2008 } 2009 2010 /** 2011 * @return {@link #paymentAmount} (Total payment amount as indicated on the 2012 * financial instrument.) 2013 */ 2014 public Money getPaymentAmount() { 2015 if (this.paymentAmount == null) 2016 if (Configuration.errorOnAutoCreate()) 2017 throw new Error("Attempt to auto-create PaymentReconciliation.paymentAmount"); 2018 else if (Configuration.doAutoCreate()) 2019 this.paymentAmount = new Money(); // cc 2020 return this.paymentAmount; 2021 } 2022 2023 public boolean hasPaymentAmount() { 2024 return this.paymentAmount != null && !this.paymentAmount.isEmpty(); 2025 } 2026 2027 /** 2028 * @param value {@link #paymentAmount} (Total payment amount as indicated on the 2029 * financial instrument.) 2030 */ 2031 public PaymentReconciliation setPaymentAmount(Money value) { 2032 this.paymentAmount = value; 2033 return this; 2034 } 2035 2036 /** 2037 * @return {@link #paymentIdentifier} (Issuer's unique identifier for the 2038 * payment instrument.) 2039 */ 2040 public Identifier getPaymentIdentifier() { 2041 if (this.paymentIdentifier == null) 2042 if (Configuration.errorOnAutoCreate()) 2043 throw new Error("Attempt to auto-create PaymentReconciliation.paymentIdentifier"); 2044 else if (Configuration.doAutoCreate()) 2045 this.paymentIdentifier = new Identifier(); // cc 2046 return this.paymentIdentifier; 2047 } 2048 2049 public boolean hasPaymentIdentifier() { 2050 return this.paymentIdentifier != null && !this.paymentIdentifier.isEmpty(); 2051 } 2052 2053 /** 2054 * @param value {@link #paymentIdentifier} (Issuer's unique identifier for the 2055 * payment instrument.) 2056 */ 2057 public PaymentReconciliation setPaymentIdentifier(Identifier value) { 2058 this.paymentIdentifier = value; 2059 return this; 2060 } 2061 2062 /** 2063 * @return {@link #detail} (Distribution of the payment amount for a previously 2064 * acknowledged payable.) 2065 */ 2066 public List<DetailsComponent> getDetail() { 2067 if (this.detail == null) 2068 this.detail = new ArrayList<DetailsComponent>(); 2069 return this.detail; 2070 } 2071 2072 /** 2073 * @return Returns a reference to <code>this</code> for easy method chaining 2074 */ 2075 public PaymentReconciliation setDetail(List<DetailsComponent> theDetail) { 2076 this.detail = theDetail; 2077 return this; 2078 } 2079 2080 public boolean hasDetail() { 2081 if (this.detail == null) 2082 return false; 2083 for (DetailsComponent item : this.detail) 2084 if (!item.isEmpty()) 2085 return true; 2086 return false; 2087 } 2088 2089 public DetailsComponent addDetail() { // 3 2090 DetailsComponent t = new DetailsComponent(); 2091 if (this.detail == null) 2092 this.detail = new ArrayList<DetailsComponent>(); 2093 this.detail.add(t); 2094 return t; 2095 } 2096 2097 public PaymentReconciliation addDetail(DetailsComponent t) { // 3 2098 if (t == null) 2099 return this; 2100 if (this.detail == null) 2101 this.detail = new ArrayList<DetailsComponent>(); 2102 this.detail.add(t); 2103 return this; 2104 } 2105 2106 /** 2107 * @return The first repetition of repeating field {@link #detail}, creating it 2108 * if it does not already exist 2109 */ 2110 public DetailsComponent getDetailFirstRep() { 2111 if (getDetail().isEmpty()) { 2112 addDetail(); 2113 } 2114 return getDetail().get(0); 2115 } 2116 2117 /** 2118 * @return {@link #formCode} (A code for the form to be used for printing the 2119 * content.) 2120 */ 2121 public CodeableConcept getFormCode() { 2122 if (this.formCode == null) 2123 if (Configuration.errorOnAutoCreate()) 2124 throw new Error("Attempt to auto-create PaymentReconciliation.formCode"); 2125 else if (Configuration.doAutoCreate()) 2126 this.formCode = new CodeableConcept(); // cc 2127 return this.formCode; 2128 } 2129 2130 public boolean hasFormCode() { 2131 return this.formCode != null && !this.formCode.isEmpty(); 2132 } 2133 2134 /** 2135 * @param value {@link #formCode} (A code for the form to be used for printing 2136 * the content.) 2137 */ 2138 public PaymentReconciliation setFormCode(CodeableConcept value) { 2139 this.formCode = value; 2140 return this; 2141 } 2142 2143 /** 2144 * @return {@link #processNote} (A note that describes or explains the 2145 * processing in a human readable form.) 2146 */ 2147 public List<NotesComponent> getProcessNote() { 2148 if (this.processNote == null) 2149 this.processNote = new ArrayList<NotesComponent>(); 2150 return this.processNote; 2151 } 2152 2153 /** 2154 * @return Returns a reference to <code>this</code> for easy method chaining 2155 */ 2156 public PaymentReconciliation setProcessNote(List<NotesComponent> theProcessNote) { 2157 this.processNote = theProcessNote; 2158 return this; 2159 } 2160 2161 public boolean hasProcessNote() { 2162 if (this.processNote == null) 2163 return false; 2164 for (NotesComponent item : this.processNote) 2165 if (!item.isEmpty()) 2166 return true; 2167 return false; 2168 } 2169 2170 public NotesComponent addProcessNote() { // 3 2171 NotesComponent t = new NotesComponent(); 2172 if (this.processNote == null) 2173 this.processNote = new ArrayList<NotesComponent>(); 2174 this.processNote.add(t); 2175 return t; 2176 } 2177 2178 public PaymentReconciliation addProcessNote(NotesComponent t) { // 3 2179 if (t == null) 2180 return this; 2181 if (this.processNote == null) 2182 this.processNote = new ArrayList<NotesComponent>(); 2183 this.processNote.add(t); 2184 return this; 2185 } 2186 2187 /** 2188 * @return The first repetition of repeating field {@link #processNote}, 2189 * creating it if it does not already exist 2190 */ 2191 public NotesComponent getProcessNoteFirstRep() { 2192 if (getProcessNote().isEmpty()) { 2193 addProcessNote(); 2194 } 2195 return getProcessNote().get(0); 2196 } 2197 2198 protected void listChildren(List<Property> children) { 2199 super.listChildren(children); 2200 children.add(new Property("identifier", "Identifier", 2201 "A unique identifier assigned to this payment reconciliation.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2202 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 2203 children.add(new Property("period", "Period", 2204 "The period of time for which payments have been gathered into this bulk payment for settlement.", 0, 1, 2205 period)); 2206 children.add(new Property("created", "dateTime", "The date when the resource was created.", 0, 1, created)); 2207 children.add(new Property("paymentIssuer", "Reference(Organization)", "The party who generated the payment.", 0, 1, 2208 paymentIssuer)); 2209 children.add(new Property("request", "Reference(Task)", "Original request resource reference.", 0, 1, request)); 2210 children.add(new Property("requestor", "Reference(Practitioner|PractitionerRole|Organization)", 2211 "The practitioner who is responsible for the services rendered to the patient.", 0, 1, requestor)); 2212 children.add(new Property("outcome", "code", "The outcome of a request for a reconciliation.", 0, 1, outcome)); 2213 children.add(new Property("disposition", "string", 2214 "A human readable description of the status of the request for the reconciliation.", 0, 1, disposition)); 2215 children.add(new Property("paymentDate", "date", "The date of payment as indicated on the financial instrument.", 0, 2216 1, paymentDate)); 2217 children.add(new Property("paymentAmount", "Money", 2218 "Total payment amount as indicated on the financial instrument.", 0, 1, paymentAmount)); 2219 children.add(new Property("paymentIdentifier", "Identifier", 2220 "Issuer's unique identifier for the payment instrument.", 0, 1, paymentIdentifier)); 2221 children.add(new Property("detail", "", "Distribution of the payment amount for a previously acknowledged payable.", 2222 0, java.lang.Integer.MAX_VALUE, detail)); 2223 children.add(new Property("formCode", "CodeableConcept", "A code for the form to be used for printing the content.", 2224 0, 1, formCode)); 2225 children.add( 2226 new Property("processNote", "", "A note that describes or explains the processing in a human readable form.", 0, 2227 java.lang.Integer.MAX_VALUE, processNote)); 2228 } 2229 2230 @Override 2231 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2232 switch (_hash) { 2233 case -1618432855: 2234 /* identifier */ return new Property("identifier", "Identifier", 2235 "A unique identifier assigned to this payment reconciliation.", 0, java.lang.Integer.MAX_VALUE, identifier); 2236 case -892481550: 2237 /* status */ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 2238 case -991726143: 2239 /* period */ return new Property("period", "Period", 2240 "The period of time for which payments have been gathered into this bulk payment for settlement.", 0, 1, 2241 period); 2242 case 1028554472: 2243 /* created */ return new Property("created", "dateTime", "The date when the resource was created.", 0, 1, 2244 created); 2245 case 1144026207: 2246 /* paymentIssuer */ return new Property("paymentIssuer", "Reference(Organization)", 2247 "The party who generated the payment.", 0, 1, paymentIssuer); 2248 case 1095692943: 2249 /* request */ return new Property("request", "Reference(Task)", "Original request resource reference.", 0, 1, 2250 request); 2251 case 693934258: 2252 /* requestor */ return new Property("requestor", "Reference(Practitioner|PractitionerRole|Organization)", 2253 "The practitioner who is responsible for the services rendered to the patient.", 0, 1, requestor); 2254 case -1106507950: 2255 /* outcome */ return new Property("outcome", "code", "The outcome of a request for a reconciliation.", 0, 1, 2256 outcome); 2257 case 583380919: 2258 /* disposition */ return new Property("disposition", "string", 2259 "A human readable description of the status of the request for the reconciliation.", 0, 1, disposition); 2260 case -1540873516: 2261 /* paymentDate */ return new Property("paymentDate", "date", 2262 "The date of payment as indicated on the financial instrument.", 0, 1, paymentDate); 2263 case 909332990: 2264 /* paymentAmount */ return new Property("paymentAmount", "Money", 2265 "Total payment amount as indicated on the financial instrument.", 0, 1, paymentAmount); 2266 case 1555852111: 2267 /* paymentIdentifier */ return new Property("paymentIdentifier", "Identifier", 2268 "Issuer's unique identifier for the payment instrument.", 0, 1, paymentIdentifier); 2269 case -1335224239: 2270 /* detail */ return new Property("detail", "", 2271 "Distribution of the payment amount for a previously acknowledged payable.", 0, java.lang.Integer.MAX_VALUE, 2272 detail); 2273 case 473181393: 2274 /* formCode */ return new Property("formCode", "CodeableConcept", 2275 "A code for the form to be used for printing the content.", 0, 1, formCode); 2276 case 202339073: 2277 /* processNote */ return new Property("processNote", "", 2278 "A note that describes or explains the processing in a human readable form.", 0, java.lang.Integer.MAX_VALUE, 2279 processNote); 2280 default: 2281 return super.getNamedProperty(_hash, _name, _checkValid); 2282 } 2283 2284 } 2285 2286 @Override 2287 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2288 switch (hash) { 2289 case -1618432855: 2290 /* identifier */ return this.identifier == null ? new Base[0] 2291 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2292 case -892481550: 2293 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PaymentReconciliationStatus> 2294 case -991726143: 2295 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 2296 case 1028554472: 2297 /* created */ return this.created == null ? new Base[0] : new Base[] { this.created }; // DateTimeType 2298 case 1144026207: 2299 /* paymentIssuer */ return this.paymentIssuer == null ? new Base[0] : new Base[] { this.paymentIssuer }; // Reference 2300 case 1095692943: 2301 /* request */ return this.request == null ? new Base[0] : new Base[] { this.request }; // Reference 2302 case 693934258: 2303 /* requestor */ return this.requestor == null ? new Base[0] : new Base[] { this.requestor }; // Reference 2304 case -1106507950: 2305 /* outcome */ return this.outcome == null ? new Base[0] : new Base[] { this.outcome }; // Enumeration<RemittanceOutcome> 2306 case 583380919: 2307 /* disposition */ return this.disposition == null ? new Base[0] : new Base[] { this.disposition }; // StringType 2308 case -1540873516: 2309 /* paymentDate */ return this.paymentDate == null ? new Base[0] : new Base[] { this.paymentDate }; // DateType 2310 case 909332990: 2311 /* paymentAmount */ return this.paymentAmount == null ? new Base[0] : new Base[] { this.paymentAmount }; // Money 2312 case 1555852111: 2313 /* paymentIdentifier */ return this.paymentIdentifier == null ? new Base[0] 2314 : new Base[] { this.paymentIdentifier }; // Identifier 2315 case -1335224239: 2316 /* detail */ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // DetailsComponent 2317 case 473181393: 2318 /* formCode */ return this.formCode == null ? new Base[0] : new Base[] { this.formCode }; // CodeableConcept 2319 case 202339073: 2320 /* processNote */ return this.processNote == null ? new Base[0] 2321 : this.processNote.toArray(new Base[this.processNote.size()]); // NotesComponent 2322 default: 2323 return super.getProperty(hash, name, checkValid); 2324 } 2325 2326 } 2327 2328 @Override 2329 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2330 switch (hash) { 2331 case -1618432855: // identifier 2332 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2333 return value; 2334 case -892481550: // status 2335 value = new PaymentReconciliationStatusEnumFactory().fromType(castToCode(value)); 2336 this.status = (Enumeration) value; // Enumeration<PaymentReconciliationStatus> 2337 return value; 2338 case -991726143: // period 2339 this.period = castToPeriod(value); // Period 2340 return value; 2341 case 1028554472: // created 2342 this.created = castToDateTime(value); // DateTimeType 2343 return value; 2344 case 1144026207: // paymentIssuer 2345 this.paymentIssuer = castToReference(value); // Reference 2346 return value; 2347 case 1095692943: // request 2348 this.request = castToReference(value); // Reference 2349 return value; 2350 case 693934258: // requestor 2351 this.requestor = castToReference(value); // Reference 2352 return value; 2353 case -1106507950: // outcome 2354 value = new RemittanceOutcomeEnumFactory().fromType(castToCode(value)); 2355 this.outcome = (Enumeration) value; // Enumeration<RemittanceOutcome> 2356 return value; 2357 case 583380919: // disposition 2358 this.disposition = castToString(value); // StringType 2359 return value; 2360 case -1540873516: // paymentDate 2361 this.paymentDate = castToDate(value); // DateType 2362 return value; 2363 case 909332990: // paymentAmount 2364 this.paymentAmount = castToMoney(value); // Money 2365 return value; 2366 case 1555852111: // paymentIdentifier 2367 this.paymentIdentifier = castToIdentifier(value); // Identifier 2368 return value; 2369 case -1335224239: // detail 2370 this.getDetail().add((DetailsComponent) value); // DetailsComponent 2371 return value; 2372 case 473181393: // formCode 2373 this.formCode = castToCodeableConcept(value); // CodeableConcept 2374 return value; 2375 case 202339073: // processNote 2376 this.getProcessNote().add((NotesComponent) value); // NotesComponent 2377 return value; 2378 default: 2379 return super.setProperty(hash, name, value); 2380 } 2381 2382 } 2383 2384 @Override 2385 public Base setProperty(String name, Base value) throws FHIRException { 2386 if (name.equals("identifier")) { 2387 this.getIdentifier().add(castToIdentifier(value)); 2388 } else if (name.equals("status")) { 2389 value = new PaymentReconciliationStatusEnumFactory().fromType(castToCode(value)); 2390 this.status = (Enumeration) value; // Enumeration<PaymentReconciliationStatus> 2391 } else if (name.equals("period")) { 2392 this.period = castToPeriod(value); // Period 2393 } else if (name.equals("created")) { 2394 this.created = castToDateTime(value); // DateTimeType 2395 } else if (name.equals("paymentIssuer")) { 2396 this.paymentIssuer = castToReference(value); // Reference 2397 } else if (name.equals("request")) { 2398 this.request = castToReference(value); // Reference 2399 } else if (name.equals("requestor")) { 2400 this.requestor = castToReference(value); // Reference 2401 } else if (name.equals("outcome")) { 2402 value = new RemittanceOutcomeEnumFactory().fromType(castToCode(value)); 2403 this.outcome = (Enumeration) value; // Enumeration<RemittanceOutcome> 2404 } else if (name.equals("disposition")) { 2405 this.disposition = castToString(value); // StringType 2406 } else if (name.equals("paymentDate")) { 2407 this.paymentDate = castToDate(value); // DateType 2408 } else if (name.equals("paymentAmount")) { 2409 this.paymentAmount = castToMoney(value); // Money 2410 } else if (name.equals("paymentIdentifier")) { 2411 this.paymentIdentifier = castToIdentifier(value); // Identifier 2412 } else if (name.equals("detail")) { 2413 this.getDetail().add((DetailsComponent) value); 2414 } else if (name.equals("formCode")) { 2415 this.formCode = castToCodeableConcept(value); // CodeableConcept 2416 } else if (name.equals("processNote")) { 2417 this.getProcessNote().add((NotesComponent) value); 2418 } else 2419 return super.setProperty(name, value); 2420 return value; 2421 } 2422 2423 @Override 2424 public void removeChild(String name, Base value) throws FHIRException { 2425 if (name.equals("identifier")) { 2426 this.getIdentifier().remove(castToIdentifier(value)); 2427 } else if (name.equals("status")) { 2428 this.status = null; 2429 } else if (name.equals("period")) { 2430 this.period = null; 2431 } else if (name.equals("created")) { 2432 this.created = null; 2433 } else if (name.equals("paymentIssuer")) { 2434 this.paymentIssuer = null; 2435 } else if (name.equals("request")) { 2436 this.request = null; 2437 } else if (name.equals("requestor")) { 2438 this.requestor = null; 2439 } else if (name.equals("outcome")) { 2440 this.outcome = null; 2441 } else if (name.equals("disposition")) { 2442 this.disposition = null; 2443 } else if (name.equals("paymentDate")) { 2444 this.paymentDate = null; 2445 } else if (name.equals("paymentAmount")) { 2446 this.paymentAmount = null; 2447 } else if (name.equals("paymentIdentifier")) { 2448 this.paymentIdentifier = null; 2449 } else if (name.equals("detail")) { 2450 this.getDetail().remove((DetailsComponent) value); 2451 } else if (name.equals("formCode")) { 2452 this.formCode = null; 2453 } else if (name.equals("processNote")) { 2454 this.getProcessNote().remove((NotesComponent) value); 2455 } else 2456 super.removeChild(name, value); 2457 2458 } 2459 2460 @Override 2461 public Base makeProperty(int hash, String name) throws FHIRException { 2462 switch (hash) { 2463 case -1618432855: 2464 return addIdentifier(); 2465 case -892481550: 2466 return getStatusElement(); 2467 case -991726143: 2468 return getPeriod(); 2469 case 1028554472: 2470 return getCreatedElement(); 2471 case 1144026207: 2472 return getPaymentIssuer(); 2473 case 1095692943: 2474 return getRequest(); 2475 case 693934258: 2476 return getRequestor(); 2477 case -1106507950: 2478 return getOutcomeElement(); 2479 case 583380919: 2480 return getDispositionElement(); 2481 case -1540873516: 2482 return getPaymentDateElement(); 2483 case 909332990: 2484 return getPaymentAmount(); 2485 case 1555852111: 2486 return getPaymentIdentifier(); 2487 case -1335224239: 2488 return addDetail(); 2489 case 473181393: 2490 return getFormCode(); 2491 case 202339073: 2492 return addProcessNote(); 2493 default: 2494 return super.makeProperty(hash, name); 2495 } 2496 2497 } 2498 2499 @Override 2500 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2501 switch (hash) { 2502 case -1618432855: 2503 /* identifier */ return new String[] { "Identifier" }; 2504 case -892481550: 2505 /* status */ return new String[] { "code" }; 2506 case -991726143: 2507 /* period */ return new String[] { "Period" }; 2508 case 1028554472: 2509 /* created */ return new String[] { "dateTime" }; 2510 case 1144026207: 2511 /* paymentIssuer */ return new String[] { "Reference" }; 2512 case 1095692943: 2513 /* request */ return new String[] { "Reference" }; 2514 case 693934258: 2515 /* requestor */ return new String[] { "Reference" }; 2516 case -1106507950: 2517 /* outcome */ return new String[] { "code" }; 2518 case 583380919: 2519 /* disposition */ return new String[] { "string" }; 2520 case -1540873516: 2521 /* paymentDate */ return new String[] { "date" }; 2522 case 909332990: 2523 /* paymentAmount */ return new String[] { "Money" }; 2524 case 1555852111: 2525 /* paymentIdentifier */ return new String[] { "Identifier" }; 2526 case -1335224239: 2527 /* detail */ return new String[] {}; 2528 case 473181393: 2529 /* formCode */ return new String[] { "CodeableConcept" }; 2530 case 202339073: 2531 /* processNote */ return new String[] {}; 2532 default: 2533 return super.getTypesForProperty(hash, name); 2534 } 2535 2536 } 2537 2538 @Override 2539 public Base addChild(String name) throws FHIRException { 2540 if (name.equals("identifier")) { 2541 return addIdentifier(); 2542 } else if (name.equals("status")) { 2543 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.status"); 2544 } else if (name.equals("period")) { 2545 this.period = new Period(); 2546 return this.period; 2547 } else if (name.equals("created")) { 2548 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.created"); 2549 } else if (name.equals("paymentIssuer")) { 2550 this.paymentIssuer = new Reference(); 2551 return this.paymentIssuer; 2552 } else if (name.equals("request")) { 2553 this.request = new Reference(); 2554 return this.request; 2555 } else if (name.equals("requestor")) { 2556 this.requestor = new Reference(); 2557 return this.requestor; 2558 } else if (name.equals("outcome")) { 2559 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.outcome"); 2560 } else if (name.equals("disposition")) { 2561 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.disposition"); 2562 } else if (name.equals("paymentDate")) { 2563 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.paymentDate"); 2564 } else if (name.equals("paymentAmount")) { 2565 this.paymentAmount = new Money(); 2566 return this.paymentAmount; 2567 } else if (name.equals("paymentIdentifier")) { 2568 this.paymentIdentifier = new Identifier(); 2569 return this.paymentIdentifier; 2570 } else if (name.equals("detail")) { 2571 return addDetail(); 2572 } else if (name.equals("formCode")) { 2573 this.formCode = new CodeableConcept(); 2574 return this.formCode; 2575 } else if (name.equals("processNote")) { 2576 return addProcessNote(); 2577 } else 2578 return super.addChild(name); 2579 } 2580 2581 public String fhirType() { 2582 return "PaymentReconciliation"; 2583 2584 } 2585 2586 public PaymentReconciliation copy() { 2587 PaymentReconciliation dst = new PaymentReconciliation(); 2588 copyValues(dst); 2589 return dst; 2590 } 2591 2592 public void copyValues(PaymentReconciliation dst) { 2593 super.copyValues(dst); 2594 if (identifier != null) { 2595 dst.identifier = new ArrayList<Identifier>(); 2596 for (Identifier i : identifier) 2597 dst.identifier.add(i.copy()); 2598 } 2599 ; 2600 dst.status = status == null ? null : status.copy(); 2601 dst.period = period == null ? null : period.copy(); 2602 dst.created = created == null ? null : created.copy(); 2603 dst.paymentIssuer = paymentIssuer == null ? null : paymentIssuer.copy(); 2604 dst.request = request == null ? null : request.copy(); 2605 dst.requestor = requestor == null ? null : requestor.copy(); 2606 dst.outcome = outcome == null ? null : outcome.copy(); 2607 dst.disposition = disposition == null ? null : disposition.copy(); 2608 dst.paymentDate = paymentDate == null ? null : paymentDate.copy(); 2609 dst.paymentAmount = paymentAmount == null ? null : paymentAmount.copy(); 2610 dst.paymentIdentifier = paymentIdentifier == null ? null : paymentIdentifier.copy(); 2611 if (detail != null) { 2612 dst.detail = new ArrayList<DetailsComponent>(); 2613 for (DetailsComponent i : detail) 2614 dst.detail.add(i.copy()); 2615 } 2616 ; 2617 dst.formCode = formCode == null ? null : formCode.copy(); 2618 if (processNote != null) { 2619 dst.processNote = new ArrayList<NotesComponent>(); 2620 for (NotesComponent i : processNote) 2621 dst.processNote.add(i.copy()); 2622 } 2623 ; 2624 } 2625 2626 protected PaymentReconciliation typedCopy() { 2627 return copy(); 2628 } 2629 2630 @Override 2631 public boolean equalsDeep(Base other_) { 2632 if (!super.equalsDeep(other_)) 2633 return false; 2634 if (!(other_ instanceof PaymentReconciliation)) 2635 return false; 2636 PaymentReconciliation o = (PaymentReconciliation) other_; 2637 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 2638 && compareDeep(period, o.period, true) && compareDeep(created, o.created, true) 2639 && compareDeep(paymentIssuer, o.paymentIssuer, true) && compareDeep(request, o.request, true) 2640 && compareDeep(requestor, o.requestor, true) && compareDeep(outcome, o.outcome, true) 2641 && compareDeep(disposition, o.disposition, true) && compareDeep(paymentDate, o.paymentDate, true) 2642 && compareDeep(paymentAmount, o.paymentAmount, true) 2643 && compareDeep(paymentIdentifier, o.paymentIdentifier, true) && compareDeep(detail, o.detail, true) 2644 && compareDeep(formCode, o.formCode, true) && compareDeep(processNote, o.processNote, true); 2645 } 2646 2647 @Override 2648 public boolean equalsShallow(Base other_) { 2649 if (!super.equalsShallow(other_)) 2650 return false; 2651 if (!(other_ instanceof PaymentReconciliation)) 2652 return false; 2653 PaymentReconciliation o = (PaymentReconciliation) other_; 2654 return compareValues(status, o.status, true) && compareValues(created, o.created, true) 2655 && compareValues(outcome, o.outcome, true) && compareValues(disposition, o.disposition, true) 2656 && compareValues(paymentDate, o.paymentDate, true); 2657 } 2658 2659 public boolean isEmpty() { 2660 return super.isEmpty() 2661 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, period, created, paymentIssuer, request, requestor, 2662 outcome, disposition, paymentDate, paymentAmount, paymentIdentifier, detail, formCode, processNote); 2663 } 2664 2665 @Override 2666 public ResourceType getResourceType() { 2667 return ResourceType.PaymentReconciliation; 2668 } 2669 2670 /** 2671 * Search parameter: <b>identifier</b> 2672 * <p> 2673 * Description: <b>The business identifier of the ExplanationOfBenefit</b><br> 2674 * Type: <b>token</b><br> 2675 * Path: <b>PaymentReconciliation.identifier</b><br> 2676 * </p> 2677 */ 2678 @SearchParamDefinition(name = "identifier", path = "PaymentReconciliation.identifier", description = "The business identifier of the ExplanationOfBenefit", type = "token") 2679 public static final String SP_IDENTIFIER = "identifier"; 2680 /** 2681 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2682 * <p> 2683 * Description: <b>The business identifier of the ExplanationOfBenefit</b><br> 2684 * Type: <b>token</b><br> 2685 * Path: <b>PaymentReconciliation.identifier</b><br> 2686 * </p> 2687 */ 2688 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2689 SP_IDENTIFIER); 2690 2691 /** 2692 * Search parameter: <b>request</b> 2693 * <p> 2694 * Description: <b>The reference to the claim</b><br> 2695 * Type: <b>reference</b><br> 2696 * Path: <b>PaymentReconciliation.request</b><br> 2697 * </p> 2698 */ 2699 @SearchParamDefinition(name = "request", path = "PaymentReconciliation.request", description = "The reference to the claim", type = "reference", target = { 2700 Task.class }) 2701 public static final String SP_REQUEST = "request"; 2702 /** 2703 * <b>Fluent Client</b> search parameter constant for <b>request</b> 2704 * <p> 2705 * Description: <b>The reference to the claim</b><br> 2706 * Type: <b>reference</b><br> 2707 * Path: <b>PaymentReconciliation.request</b><br> 2708 * </p> 2709 */ 2710 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUEST = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2711 SP_REQUEST); 2712 2713 /** 2714 * Constant for fluent queries to be used to add include statements. Specifies 2715 * the path value of "<b>PaymentReconciliation:request</b>". 2716 */ 2717 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUEST = new ca.uhn.fhir.model.api.Include( 2718 "PaymentReconciliation:request").toLocked(); 2719 2720 /** 2721 * Search parameter: <b>disposition</b> 2722 * <p> 2723 * Description: <b>The contents of the disposition message</b><br> 2724 * Type: <b>string</b><br> 2725 * Path: <b>PaymentReconciliation.disposition</b><br> 2726 * </p> 2727 */ 2728 @SearchParamDefinition(name = "disposition", path = "PaymentReconciliation.disposition", description = "The contents of the disposition message", type = "string") 2729 public static final String SP_DISPOSITION = "disposition"; 2730 /** 2731 * <b>Fluent Client</b> search parameter constant for <b>disposition</b> 2732 * <p> 2733 * Description: <b>The contents of the disposition message</b><br> 2734 * Type: <b>string</b><br> 2735 * Path: <b>PaymentReconciliation.disposition</b><br> 2736 * </p> 2737 */ 2738 public static final ca.uhn.fhir.rest.gclient.StringClientParam DISPOSITION = new ca.uhn.fhir.rest.gclient.StringClientParam( 2739 SP_DISPOSITION); 2740 2741 /** 2742 * Search parameter: <b>created</b> 2743 * <p> 2744 * Description: <b>The creation date</b><br> 2745 * Type: <b>date</b><br> 2746 * Path: <b>PaymentReconciliation.created</b><br> 2747 * </p> 2748 */ 2749 @SearchParamDefinition(name = "created", path = "PaymentReconciliation.created", description = "The creation date", type = "date") 2750 public static final String SP_CREATED = "created"; 2751 /** 2752 * <b>Fluent Client</b> search parameter constant for <b>created</b> 2753 * <p> 2754 * Description: <b>The creation date</b><br> 2755 * Type: <b>date</b><br> 2756 * Path: <b>PaymentReconciliation.created</b><br> 2757 * </p> 2758 */ 2759 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam( 2760 SP_CREATED); 2761 2762 /** 2763 * Search parameter: <b>payment-issuer</b> 2764 * <p> 2765 * Description: <b>The organization which generated this resource</b><br> 2766 * Type: <b>reference</b><br> 2767 * Path: <b>PaymentReconciliation.paymentIssuer</b><br> 2768 * </p> 2769 */ 2770 @SearchParamDefinition(name = "payment-issuer", path = "PaymentReconciliation.paymentIssuer", description = "The organization which generated this resource", type = "reference", target = { 2771 Organization.class }) 2772 public static final String SP_PAYMENT_ISSUER = "payment-issuer"; 2773 /** 2774 * <b>Fluent Client</b> search parameter constant for <b>payment-issuer</b> 2775 * <p> 2776 * Description: <b>The organization which generated this resource</b><br> 2777 * Type: <b>reference</b><br> 2778 * Path: <b>PaymentReconciliation.paymentIssuer</b><br> 2779 * </p> 2780 */ 2781 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PAYMENT_ISSUER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2782 SP_PAYMENT_ISSUER); 2783 2784 /** 2785 * Constant for fluent queries to be used to add include statements. Specifies 2786 * the path value of "<b>PaymentReconciliation:payment-issuer</b>". 2787 */ 2788 public static final ca.uhn.fhir.model.api.Include INCLUDE_PAYMENT_ISSUER = new ca.uhn.fhir.model.api.Include( 2789 "PaymentReconciliation:payment-issuer").toLocked(); 2790 2791 /** 2792 * Search parameter: <b>outcome</b> 2793 * <p> 2794 * Description: <b>The processing outcome</b><br> 2795 * Type: <b>token</b><br> 2796 * Path: <b>PaymentReconciliation.outcome</b><br> 2797 * </p> 2798 */ 2799 @SearchParamDefinition(name = "outcome", path = "PaymentReconciliation.outcome", description = "The processing outcome", type = "token") 2800 public static final String SP_OUTCOME = "outcome"; 2801 /** 2802 * <b>Fluent Client</b> search parameter constant for <b>outcome</b> 2803 * <p> 2804 * Description: <b>The processing outcome</b><br> 2805 * Type: <b>token</b><br> 2806 * Path: <b>PaymentReconciliation.outcome</b><br> 2807 * </p> 2808 */ 2809 public static final ca.uhn.fhir.rest.gclient.TokenClientParam OUTCOME = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2810 SP_OUTCOME); 2811 2812 /** 2813 * Search parameter: <b>requestor</b> 2814 * <p> 2815 * Description: <b>The reference to the provider who submitted the claim</b><br> 2816 * Type: <b>reference</b><br> 2817 * Path: <b>PaymentReconciliation.requestor</b><br> 2818 * </p> 2819 */ 2820 @SearchParamDefinition(name = "requestor", path = "PaymentReconciliation.requestor", description = "The reference to the provider who submitted the claim", type = "reference", providesMembershipIn = { 2821 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Organization.class, 2822 Practitioner.class, PractitionerRole.class }) 2823 public static final String SP_REQUESTOR = "requestor"; 2824 /** 2825 * <b>Fluent Client</b> search parameter constant for <b>requestor</b> 2826 * <p> 2827 * Description: <b>The reference to the provider who submitted the claim</b><br> 2828 * Type: <b>reference</b><br> 2829 * Path: <b>PaymentReconciliation.requestor</b><br> 2830 * </p> 2831 */ 2832 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2833 SP_REQUESTOR); 2834 2835 /** 2836 * Constant for fluent queries to be used to add include statements. Specifies 2837 * the path value of "<b>PaymentReconciliation:requestor</b>". 2838 */ 2839 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTOR = new ca.uhn.fhir.model.api.Include( 2840 "PaymentReconciliation:requestor").toLocked(); 2841 2842 /** 2843 * Search parameter: <b>status</b> 2844 * <p> 2845 * Description: <b>The status of the payment reconciliation</b><br> 2846 * Type: <b>token</b><br> 2847 * Path: <b>PaymentReconciliation.status</b><br> 2848 * </p> 2849 */ 2850 @SearchParamDefinition(name = "status", path = "PaymentReconciliation.status", description = "The status of the payment reconciliation", type = "token") 2851 public static final String SP_STATUS = "status"; 2852 /** 2853 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2854 * <p> 2855 * Description: <b>The status of the payment reconciliation</b><br> 2856 * Type: <b>token</b><br> 2857 * Path: <b>PaymentReconciliation.status</b><br> 2858 * </p> 2859 */ 2860 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2861 SP_STATUS); 2862 2863}