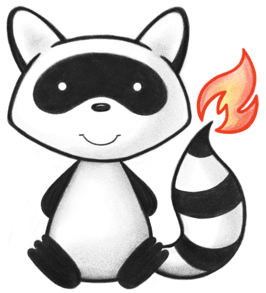
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049 050/** 051 * This resource allows for the definition of various types of plans as a 052 * sharable, consumable, and executable artifact. The resource is general enough 053 * to support the description of a broad range of clinical artifacts such as 054 * clinical decision support rules, order sets and protocols. 055 */ 056@ResourceDef(name = "PlanDefinition", profile = "http://hl7.org/fhir/StructureDefinition/PlanDefinition") 057@ChildOrder(names = { "url", "identifier", "version", "name", "title", "subtitle", "type", "status", "experimental", 058 "subject[x]", "date", "publisher", "contact", "description", "useContext", "jurisdiction", "purpose", "usage", 059 "copyright", "approvalDate", "lastReviewDate", "effectivePeriod", "topic", "author", "editor", "reviewer", 060 "endorser", "relatedArtifact", "library", "goal", "action" }) 061public class PlanDefinition extends MetadataResource { 062 063 public enum RequestPriority { 064 /** 065 * The request has normal priority. 066 */ 067 ROUTINE, 068 /** 069 * The request should be actioned promptly - higher priority than routine. 070 */ 071 URGENT, 072 /** 073 * The request should be actioned as soon as possible - higher priority than 074 * urgent. 075 */ 076 ASAP, 077 /** 078 * The request should be actioned immediately - highest possible priority. E.g. 079 * an emergency. 080 */ 081 STAT, 082 /** 083 * added to help the parsers with the generic types 084 */ 085 NULL; 086 087 public static RequestPriority fromCode(String codeString) throws FHIRException { 088 if (codeString == null || "".equals(codeString)) 089 return null; 090 if ("routine".equals(codeString)) 091 return ROUTINE; 092 if ("urgent".equals(codeString)) 093 return URGENT; 094 if ("asap".equals(codeString)) 095 return ASAP; 096 if ("stat".equals(codeString)) 097 return STAT; 098 if (Configuration.isAcceptInvalidEnums()) 099 return null; 100 else 101 throw new FHIRException("Unknown RequestPriority code '" + codeString + "'"); 102 } 103 104 public String toCode() { 105 switch (this) { 106 case ROUTINE: 107 return "routine"; 108 case URGENT: 109 return "urgent"; 110 case ASAP: 111 return "asap"; 112 case STAT: 113 return "stat"; 114 case NULL: 115 return null; 116 default: 117 return "?"; 118 } 119 } 120 121 public String getSystem() { 122 switch (this) { 123 case ROUTINE: 124 return "http://hl7.org/fhir/request-priority"; 125 case URGENT: 126 return "http://hl7.org/fhir/request-priority"; 127 case ASAP: 128 return "http://hl7.org/fhir/request-priority"; 129 case STAT: 130 return "http://hl7.org/fhir/request-priority"; 131 case NULL: 132 return null; 133 default: 134 return "?"; 135 } 136 } 137 138 public String getDefinition() { 139 switch (this) { 140 case ROUTINE: 141 return "The request has normal priority."; 142 case URGENT: 143 return "The request should be actioned promptly - higher priority than routine."; 144 case ASAP: 145 return "The request should be actioned as soon as possible - higher priority than urgent."; 146 case STAT: 147 return "The request should be actioned immediately - highest possible priority. E.g. an emergency."; 148 case NULL: 149 return null; 150 default: 151 return "?"; 152 } 153 } 154 155 public String getDisplay() { 156 switch (this) { 157 case ROUTINE: 158 return "Routine"; 159 case URGENT: 160 return "Urgent"; 161 case ASAP: 162 return "ASAP"; 163 case STAT: 164 return "STAT"; 165 case NULL: 166 return null; 167 default: 168 return "?"; 169 } 170 } 171 } 172 173 public static class RequestPriorityEnumFactory implements EnumFactory<RequestPriority> { 174 public RequestPriority fromCode(String codeString) throws IllegalArgumentException { 175 if (codeString == null || "".equals(codeString)) 176 if (codeString == null || "".equals(codeString)) 177 return null; 178 if ("routine".equals(codeString)) 179 return RequestPriority.ROUTINE; 180 if ("urgent".equals(codeString)) 181 return RequestPriority.URGENT; 182 if ("asap".equals(codeString)) 183 return RequestPriority.ASAP; 184 if ("stat".equals(codeString)) 185 return RequestPriority.STAT; 186 throw new IllegalArgumentException("Unknown RequestPriority code '" + codeString + "'"); 187 } 188 189 public Enumeration<RequestPriority> fromType(PrimitiveType<?> code) throws FHIRException { 190 if (code == null) 191 return null; 192 if (code.isEmpty()) 193 return new Enumeration<RequestPriority>(this, RequestPriority.NULL, code); 194 String codeString = code.asStringValue(); 195 if (codeString == null || "".equals(codeString)) 196 return new Enumeration<RequestPriority>(this, RequestPriority.NULL, code); 197 if ("routine".equals(codeString)) 198 return new Enumeration<RequestPriority>(this, RequestPriority.ROUTINE, code); 199 if ("urgent".equals(codeString)) 200 return new Enumeration<RequestPriority>(this, RequestPriority.URGENT, code); 201 if ("asap".equals(codeString)) 202 return new Enumeration<RequestPriority>(this, RequestPriority.ASAP, code); 203 if ("stat".equals(codeString)) 204 return new Enumeration<RequestPriority>(this, RequestPriority.STAT, code); 205 throw new FHIRException("Unknown RequestPriority code '" + codeString + "'"); 206 } 207 208 public String toCode(RequestPriority code) { 209 if (code == RequestPriority.NULL) 210 return null; 211 if (code == RequestPriority.ROUTINE) 212 return "routine"; 213 if (code == RequestPriority.URGENT) 214 return "urgent"; 215 if (code == RequestPriority.ASAP) 216 return "asap"; 217 if (code == RequestPriority.STAT) 218 return "stat"; 219 return "?"; 220 } 221 222 public String toSystem(RequestPriority code) { 223 return code.getSystem(); 224 } 225 } 226 227 public enum ActionConditionKind { 228 /** 229 * The condition describes whether or not a given action is applicable. 230 */ 231 APPLICABILITY, 232 /** 233 * The condition is a starting condition for the action. 234 */ 235 START, 236 /** 237 * The condition is a stop, or exit condition for the action. 238 */ 239 STOP, 240 /** 241 * added to help the parsers with the generic types 242 */ 243 NULL; 244 245 public static ActionConditionKind fromCode(String codeString) throws FHIRException { 246 if (codeString == null || "".equals(codeString)) 247 return null; 248 if ("applicability".equals(codeString)) 249 return APPLICABILITY; 250 if ("start".equals(codeString)) 251 return START; 252 if ("stop".equals(codeString)) 253 return STOP; 254 if (Configuration.isAcceptInvalidEnums()) 255 return null; 256 else 257 throw new FHIRException("Unknown ActionConditionKind code '" + codeString + "'"); 258 } 259 260 public String toCode() { 261 switch (this) { 262 case APPLICABILITY: 263 return "applicability"; 264 case START: 265 return "start"; 266 case STOP: 267 return "stop"; 268 case NULL: 269 return null; 270 default: 271 return "?"; 272 } 273 } 274 275 public String getSystem() { 276 switch (this) { 277 case APPLICABILITY: 278 return "http://hl7.org/fhir/action-condition-kind"; 279 case START: 280 return "http://hl7.org/fhir/action-condition-kind"; 281 case STOP: 282 return "http://hl7.org/fhir/action-condition-kind"; 283 case NULL: 284 return null; 285 default: 286 return "?"; 287 } 288 } 289 290 public String getDefinition() { 291 switch (this) { 292 case APPLICABILITY: 293 return "The condition describes whether or not a given action is applicable."; 294 case START: 295 return "The condition is a starting condition for the action."; 296 case STOP: 297 return "The condition is a stop, or exit condition for the action."; 298 case NULL: 299 return null; 300 default: 301 return "?"; 302 } 303 } 304 305 public String getDisplay() { 306 switch (this) { 307 case APPLICABILITY: 308 return "Applicability"; 309 case START: 310 return "Start"; 311 case STOP: 312 return "Stop"; 313 case NULL: 314 return null; 315 default: 316 return "?"; 317 } 318 } 319 } 320 321 public static class ActionConditionKindEnumFactory implements EnumFactory<ActionConditionKind> { 322 public ActionConditionKind fromCode(String codeString) throws IllegalArgumentException { 323 if (codeString == null || "".equals(codeString)) 324 if (codeString == null || "".equals(codeString)) 325 return null; 326 if ("applicability".equals(codeString)) 327 return ActionConditionKind.APPLICABILITY; 328 if ("start".equals(codeString)) 329 return ActionConditionKind.START; 330 if ("stop".equals(codeString)) 331 return ActionConditionKind.STOP; 332 throw new IllegalArgumentException("Unknown ActionConditionKind code '" + codeString + "'"); 333 } 334 335 public Enumeration<ActionConditionKind> fromType(PrimitiveType<?> code) throws FHIRException { 336 if (code == null) 337 return null; 338 if (code.isEmpty()) 339 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.NULL, code); 340 String codeString = code.asStringValue(); 341 if (codeString == null || "".equals(codeString)) 342 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.NULL, code); 343 if ("applicability".equals(codeString)) 344 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.APPLICABILITY, code); 345 if ("start".equals(codeString)) 346 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.START, code); 347 if ("stop".equals(codeString)) 348 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.STOP, code); 349 throw new FHIRException("Unknown ActionConditionKind code '" + codeString + "'"); 350 } 351 352 public String toCode(ActionConditionKind code) { 353 if (code == ActionConditionKind.NULL) 354 return null; 355 if (code == ActionConditionKind.APPLICABILITY) 356 return "applicability"; 357 if (code == ActionConditionKind.START) 358 return "start"; 359 if (code == ActionConditionKind.STOP) 360 return "stop"; 361 return "?"; 362 } 363 364 public String toSystem(ActionConditionKind code) { 365 return code.getSystem(); 366 } 367 } 368 369 public enum ActionRelationshipType { 370 /** 371 * The action must be performed before the start of the related action. 372 */ 373 BEFORESTART, 374 /** 375 * The action must be performed before the related action. 376 */ 377 BEFORE, 378 /** 379 * The action must be performed before the end of the related action. 380 */ 381 BEFOREEND, 382 /** 383 * The action must be performed concurrent with the start of the related action. 384 */ 385 CONCURRENTWITHSTART, 386 /** 387 * The action must be performed concurrent with the related action. 388 */ 389 CONCURRENT, 390 /** 391 * The action must be performed concurrent with the end of the related action. 392 */ 393 CONCURRENTWITHEND, 394 /** 395 * The action must be performed after the start of the related action. 396 */ 397 AFTERSTART, 398 /** 399 * The action must be performed after the related action. 400 */ 401 AFTER, 402 /** 403 * The action must be performed after the end of the related action. 404 */ 405 AFTEREND, 406 /** 407 * added to help the parsers with the generic types 408 */ 409 NULL; 410 411 public static ActionRelationshipType fromCode(String codeString) throws FHIRException { 412 if (codeString == null || "".equals(codeString)) 413 return null; 414 if ("before-start".equals(codeString)) 415 return BEFORESTART; 416 if ("before".equals(codeString)) 417 return BEFORE; 418 if ("before-end".equals(codeString)) 419 return BEFOREEND; 420 if ("concurrent-with-start".equals(codeString)) 421 return CONCURRENTWITHSTART; 422 if ("concurrent".equals(codeString)) 423 return CONCURRENT; 424 if ("concurrent-with-end".equals(codeString)) 425 return CONCURRENTWITHEND; 426 if ("after-start".equals(codeString)) 427 return AFTERSTART; 428 if ("after".equals(codeString)) 429 return AFTER; 430 if ("after-end".equals(codeString)) 431 return AFTEREND; 432 if (Configuration.isAcceptInvalidEnums()) 433 return null; 434 else 435 throw new FHIRException("Unknown ActionRelationshipType code '" + codeString + "'"); 436 } 437 438 public String toCode() { 439 switch (this) { 440 case BEFORESTART: 441 return "before-start"; 442 case BEFORE: 443 return "before"; 444 case BEFOREEND: 445 return "before-end"; 446 case CONCURRENTWITHSTART: 447 return "concurrent-with-start"; 448 case CONCURRENT: 449 return "concurrent"; 450 case CONCURRENTWITHEND: 451 return "concurrent-with-end"; 452 case AFTERSTART: 453 return "after-start"; 454 case AFTER: 455 return "after"; 456 case AFTEREND: 457 return "after-end"; 458 case NULL: 459 return null; 460 default: 461 return "?"; 462 } 463 } 464 465 public String getSystem() { 466 switch (this) { 467 case BEFORESTART: 468 return "http://hl7.org/fhir/action-relationship-type"; 469 case BEFORE: 470 return "http://hl7.org/fhir/action-relationship-type"; 471 case BEFOREEND: 472 return "http://hl7.org/fhir/action-relationship-type"; 473 case CONCURRENTWITHSTART: 474 return "http://hl7.org/fhir/action-relationship-type"; 475 case CONCURRENT: 476 return "http://hl7.org/fhir/action-relationship-type"; 477 case CONCURRENTWITHEND: 478 return "http://hl7.org/fhir/action-relationship-type"; 479 case AFTERSTART: 480 return "http://hl7.org/fhir/action-relationship-type"; 481 case AFTER: 482 return "http://hl7.org/fhir/action-relationship-type"; 483 case AFTEREND: 484 return "http://hl7.org/fhir/action-relationship-type"; 485 case NULL: 486 return null; 487 default: 488 return "?"; 489 } 490 } 491 492 public String getDefinition() { 493 switch (this) { 494 case BEFORESTART: 495 return "The action must be performed before the start of the related action."; 496 case BEFORE: 497 return "The action must be performed before the related action."; 498 case BEFOREEND: 499 return "The action must be performed before the end of the related action."; 500 case CONCURRENTWITHSTART: 501 return "The action must be performed concurrent with the start of the related action."; 502 case CONCURRENT: 503 return "The action must be performed concurrent with the related action."; 504 case CONCURRENTWITHEND: 505 return "The action must be performed concurrent with the end of the related action."; 506 case AFTERSTART: 507 return "The action must be performed after the start of the related action."; 508 case AFTER: 509 return "The action must be performed after the related action."; 510 case AFTEREND: 511 return "The action must be performed after the end of the related action."; 512 case NULL: 513 return null; 514 default: 515 return "?"; 516 } 517 } 518 519 public String getDisplay() { 520 switch (this) { 521 case BEFORESTART: 522 return "Before Start"; 523 case BEFORE: 524 return "Before"; 525 case BEFOREEND: 526 return "Before End"; 527 case CONCURRENTWITHSTART: 528 return "Concurrent With Start"; 529 case CONCURRENT: 530 return "Concurrent"; 531 case CONCURRENTWITHEND: 532 return "Concurrent With End"; 533 case AFTERSTART: 534 return "After Start"; 535 case AFTER: 536 return "After"; 537 case AFTEREND: 538 return "After End"; 539 case NULL: 540 return null; 541 default: 542 return "?"; 543 } 544 } 545 } 546 547 public static class ActionRelationshipTypeEnumFactory implements EnumFactory<ActionRelationshipType> { 548 public ActionRelationshipType fromCode(String codeString) throws IllegalArgumentException { 549 if (codeString == null || "".equals(codeString)) 550 if (codeString == null || "".equals(codeString)) 551 return null; 552 if ("before-start".equals(codeString)) 553 return ActionRelationshipType.BEFORESTART; 554 if ("before".equals(codeString)) 555 return ActionRelationshipType.BEFORE; 556 if ("before-end".equals(codeString)) 557 return ActionRelationshipType.BEFOREEND; 558 if ("concurrent-with-start".equals(codeString)) 559 return ActionRelationshipType.CONCURRENTWITHSTART; 560 if ("concurrent".equals(codeString)) 561 return ActionRelationshipType.CONCURRENT; 562 if ("concurrent-with-end".equals(codeString)) 563 return ActionRelationshipType.CONCURRENTWITHEND; 564 if ("after-start".equals(codeString)) 565 return ActionRelationshipType.AFTERSTART; 566 if ("after".equals(codeString)) 567 return ActionRelationshipType.AFTER; 568 if ("after-end".equals(codeString)) 569 return ActionRelationshipType.AFTEREND; 570 throw new IllegalArgumentException("Unknown ActionRelationshipType code '" + codeString + "'"); 571 } 572 573 public Enumeration<ActionRelationshipType> fromType(PrimitiveType<?> code) throws FHIRException { 574 if (code == null) 575 return null; 576 if (code.isEmpty()) 577 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.NULL, code); 578 String codeString = code.asStringValue(); 579 if (codeString == null || "".equals(codeString)) 580 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.NULL, code); 581 if ("before-start".equals(codeString)) 582 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.BEFORESTART, code); 583 if ("before".equals(codeString)) 584 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.BEFORE, code); 585 if ("before-end".equals(codeString)) 586 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.BEFOREEND, code); 587 if ("concurrent-with-start".equals(codeString)) 588 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.CONCURRENTWITHSTART, code); 589 if ("concurrent".equals(codeString)) 590 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.CONCURRENT, code); 591 if ("concurrent-with-end".equals(codeString)) 592 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.CONCURRENTWITHEND, code); 593 if ("after-start".equals(codeString)) 594 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.AFTERSTART, code); 595 if ("after".equals(codeString)) 596 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.AFTER, code); 597 if ("after-end".equals(codeString)) 598 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.AFTEREND, code); 599 throw new FHIRException("Unknown ActionRelationshipType code '" + codeString + "'"); 600 } 601 602 public String toCode(ActionRelationshipType code) { 603 if (code == ActionRelationshipType.NULL) 604 return null; 605 if (code == ActionRelationshipType.BEFORESTART) 606 return "before-start"; 607 if (code == ActionRelationshipType.BEFORE) 608 return "before"; 609 if (code == ActionRelationshipType.BEFOREEND) 610 return "before-end"; 611 if (code == ActionRelationshipType.CONCURRENTWITHSTART) 612 return "concurrent-with-start"; 613 if (code == ActionRelationshipType.CONCURRENT) 614 return "concurrent"; 615 if (code == ActionRelationshipType.CONCURRENTWITHEND) 616 return "concurrent-with-end"; 617 if (code == ActionRelationshipType.AFTERSTART) 618 return "after-start"; 619 if (code == ActionRelationshipType.AFTER) 620 return "after"; 621 if (code == ActionRelationshipType.AFTEREND) 622 return "after-end"; 623 return "?"; 624 } 625 626 public String toSystem(ActionRelationshipType code) { 627 return code.getSystem(); 628 } 629 } 630 631 public enum ActionParticipantType { 632 /** 633 * The participant is the patient under evaluation. 634 */ 635 PATIENT, 636 /** 637 * The participant is a practitioner involved in the patient's care. 638 */ 639 PRACTITIONER, 640 /** 641 * The participant is a person related to the patient. 642 */ 643 RELATEDPERSON, 644 /** 645 * The participant is a system or device used in the care of the patient. 646 */ 647 DEVICE, 648 /** 649 * added to help the parsers with the generic types 650 */ 651 NULL; 652 653 public static ActionParticipantType fromCode(String codeString) throws FHIRException { 654 if (codeString == null || "".equals(codeString)) 655 return null; 656 if ("patient".equals(codeString)) 657 return PATIENT; 658 if ("practitioner".equals(codeString)) 659 return PRACTITIONER; 660 if ("related-person".equals(codeString)) 661 return RELATEDPERSON; 662 if ("device".equals(codeString)) 663 return DEVICE; 664 if (Configuration.isAcceptInvalidEnums()) 665 return null; 666 else 667 throw new FHIRException("Unknown ActionParticipantType code '" + codeString + "'"); 668 } 669 670 public String toCode() { 671 switch (this) { 672 case PATIENT: 673 return "patient"; 674 case PRACTITIONER: 675 return "practitioner"; 676 case RELATEDPERSON: 677 return "related-person"; 678 case DEVICE: 679 return "device"; 680 case NULL: 681 return null; 682 default: 683 return "?"; 684 } 685 } 686 687 public String getSystem() { 688 switch (this) { 689 case PATIENT: 690 return "http://hl7.org/fhir/action-participant-type"; 691 case PRACTITIONER: 692 return "http://hl7.org/fhir/action-participant-type"; 693 case RELATEDPERSON: 694 return "http://hl7.org/fhir/action-participant-type"; 695 case DEVICE: 696 return "http://hl7.org/fhir/action-participant-type"; 697 case NULL: 698 return null; 699 default: 700 return "?"; 701 } 702 } 703 704 public String getDefinition() { 705 switch (this) { 706 case PATIENT: 707 return "The participant is the patient under evaluation."; 708 case PRACTITIONER: 709 return "The participant is a practitioner involved in the patient's care."; 710 case RELATEDPERSON: 711 return "The participant is a person related to the patient."; 712 case DEVICE: 713 return "The participant is a system or device used in the care of the patient."; 714 case NULL: 715 return null; 716 default: 717 return "?"; 718 } 719 } 720 721 public String getDisplay() { 722 switch (this) { 723 case PATIENT: 724 return "Patient"; 725 case PRACTITIONER: 726 return "Practitioner"; 727 case RELATEDPERSON: 728 return "Related Person"; 729 case DEVICE: 730 return "Device"; 731 case NULL: 732 return null; 733 default: 734 return "?"; 735 } 736 } 737 } 738 739 public static class ActionParticipantTypeEnumFactory implements EnumFactory<ActionParticipantType> { 740 public ActionParticipantType fromCode(String codeString) throws IllegalArgumentException { 741 if (codeString == null || "".equals(codeString)) 742 if (codeString == null || "".equals(codeString)) 743 return null; 744 if ("patient".equals(codeString)) 745 return ActionParticipantType.PATIENT; 746 if ("practitioner".equals(codeString)) 747 return ActionParticipantType.PRACTITIONER; 748 if ("related-person".equals(codeString)) 749 return ActionParticipantType.RELATEDPERSON; 750 if ("device".equals(codeString)) 751 return ActionParticipantType.DEVICE; 752 throw new IllegalArgumentException("Unknown ActionParticipantType code '" + codeString + "'"); 753 } 754 755 public Enumeration<ActionParticipantType> fromType(PrimitiveType<?> code) throws FHIRException { 756 if (code == null) 757 return null; 758 if (code.isEmpty()) 759 return new Enumeration<ActionParticipantType>(this, ActionParticipantType.NULL, code); 760 String codeString = code.asStringValue(); 761 if (codeString == null || "".equals(codeString)) 762 return new Enumeration<ActionParticipantType>(this, ActionParticipantType.NULL, code); 763 if ("patient".equals(codeString)) 764 return new Enumeration<ActionParticipantType>(this, ActionParticipantType.PATIENT, code); 765 if ("practitioner".equals(codeString)) 766 return new Enumeration<ActionParticipantType>(this, ActionParticipantType.PRACTITIONER, code); 767 if ("related-person".equals(codeString)) 768 return new Enumeration<ActionParticipantType>(this, ActionParticipantType.RELATEDPERSON, code); 769 if ("device".equals(codeString)) 770 return new Enumeration<ActionParticipantType>(this, ActionParticipantType.DEVICE, code); 771 throw new FHIRException("Unknown ActionParticipantType code '" + codeString + "'"); 772 } 773 774 public String toCode(ActionParticipantType code) { 775 if (code == ActionParticipantType.NULL) 776 return null; 777 if (code == ActionParticipantType.PATIENT) 778 return "patient"; 779 if (code == ActionParticipantType.PRACTITIONER) 780 return "practitioner"; 781 if (code == ActionParticipantType.RELATEDPERSON) 782 return "related-person"; 783 if (code == ActionParticipantType.DEVICE) 784 return "device"; 785 return "?"; 786 } 787 788 public String toSystem(ActionParticipantType code) { 789 return code.getSystem(); 790 } 791 } 792 793 public enum ActionGroupingBehavior { 794 /** 795 * Any group marked with this behavior should be displayed as a visual group to 796 * the end user. 797 */ 798 VISUALGROUP, 799 /** 800 * A group with this behavior logically groups its sub-elements, and may be 801 * shown as a visual group to the end user, but it is not required to do so. 802 */ 803 LOGICALGROUP, 804 /** 805 * A group of related alternative actions is a sentence group if the target 806 * referenced by the action is the same in all the actions and each action 807 * simply constitutes a different variation on how to specify the details for 808 * the target. For example, two actions that could be in a SentenceGroup are 809 * "aspirin, 500 mg, 2 times per day" and "aspirin, 300 mg, 3 times per day". In 810 * both cases, aspirin is the target referenced by the action, and the two 811 * actions represent different options for how aspirin might be ordered for the 812 * patient. Note that a SentenceGroup would almost always have an associated 813 * selection behavior of "AtMostOne", unless it's a required action, in which 814 * case, it would be "ExactlyOne". 815 */ 816 SENTENCEGROUP, 817 /** 818 * added to help the parsers with the generic types 819 */ 820 NULL; 821 822 public static ActionGroupingBehavior fromCode(String codeString) throws FHIRException { 823 if (codeString == null || "".equals(codeString)) 824 return null; 825 if ("visual-group".equals(codeString)) 826 return VISUALGROUP; 827 if ("logical-group".equals(codeString)) 828 return LOGICALGROUP; 829 if ("sentence-group".equals(codeString)) 830 return SENTENCEGROUP; 831 if (Configuration.isAcceptInvalidEnums()) 832 return null; 833 else 834 throw new FHIRException("Unknown ActionGroupingBehavior code '" + codeString + "'"); 835 } 836 837 public String toCode() { 838 switch (this) { 839 case VISUALGROUP: 840 return "visual-group"; 841 case LOGICALGROUP: 842 return "logical-group"; 843 case SENTENCEGROUP: 844 return "sentence-group"; 845 case NULL: 846 return null; 847 default: 848 return "?"; 849 } 850 } 851 852 public String getSystem() { 853 switch (this) { 854 case VISUALGROUP: 855 return "http://hl7.org/fhir/action-grouping-behavior"; 856 case LOGICALGROUP: 857 return "http://hl7.org/fhir/action-grouping-behavior"; 858 case SENTENCEGROUP: 859 return "http://hl7.org/fhir/action-grouping-behavior"; 860 case NULL: 861 return null; 862 default: 863 return "?"; 864 } 865 } 866 867 public String getDefinition() { 868 switch (this) { 869 case VISUALGROUP: 870 return "Any group marked with this behavior should be displayed as a visual group to the end user."; 871 case LOGICALGROUP: 872 return "A group with this behavior logically groups its sub-elements, and may be shown as a visual group to the end user, but it is not required to do so."; 873 case SENTENCEGROUP: 874 return "A group of related alternative actions is a sentence group if the target referenced by the action is the same in all the actions and each action simply constitutes a different variation on how to specify the details for the target. For example, two actions that could be in a SentenceGroup are \"aspirin, 500 mg, 2 times per day\" and \"aspirin, 300 mg, 3 times per day\". In both cases, aspirin is the target referenced by the action, and the two actions represent different options for how aspirin might be ordered for the patient. Note that a SentenceGroup would almost always have an associated selection behavior of \"AtMostOne\", unless it's a required action, in which case, it would be \"ExactlyOne\"."; 875 case NULL: 876 return null; 877 default: 878 return "?"; 879 } 880 } 881 882 public String getDisplay() { 883 switch (this) { 884 case VISUALGROUP: 885 return "Visual Group"; 886 case LOGICALGROUP: 887 return "Logical Group"; 888 case SENTENCEGROUP: 889 return "Sentence Group"; 890 case NULL: 891 return null; 892 default: 893 return "?"; 894 } 895 } 896 } 897 898 public static class ActionGroupingBehaviorEnumFactory implements EnumFactory<ActionGroupingBehavior> { 899 public ActionGroupingBehavior fromCode(String codeString) throws IllegalArgumentException { 900 if (codeString == null || "".equals(codeString)) 901 if (codeString == null || "".equals(codeString)) 902 return null; 903 if ("visual-group".equals(codeString)) 904 return ActionGroupingBehavior.VISUALGROUP; 905 if ("logical-group".equals(codeString)) 906 return ActionGroupingBehavior.LOGICALGROUP; 907 if ("sentence-group".equals(codeString)) 908 return ActionGroupingBehavior.SENTENCEGROUP; 909 throw new IllegalArgumentException("Unknown ActionGroupingBehavior code '" + codeString + "'"); 910 } 911 912 public Enumeration<ActionGroupingBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 913 if (code == null) 914 return null; 915 if (code.isEmpty()) 916 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.NULL, code); 917 String codeString = code.asStringValue(); 918 if (codeString == null || "".equals(codeString)) 919 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.NULL, code); 920 if ("visual-group".equals(codeString)) 921 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.VISUALGROUP, code); 922 if ("logical-group".equals(codeString)) 923 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.LOGICALGROUP, code); 924 if ("sentence-group".equals(codeString)) 925 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.SENTENCEGROUP, code); 926 throw new FHIRException("Unknown ActionGroupingBehavior code '" + codeString + "'"); 927 } 928 929 public String toCode(ActionGroupingBehavior code) { 930 if (code == ActionGroupingBehavior.NULL) 931 return null; 932 if (code == ActionGroupingBehavior.VISUALGROUP) 933 return "visual-group"; 934 if (code == ActionGroupingBehavior.LOGICALGROUP) 935 return "logical-group"; 936 if (code == ActionGroupingBehavior.SENTENCEGROUP) 937 return "sentence-group"; 938 return "?"; 939 } 940 941 public String toSystem(ActionGroupingBehavior code) { 942 return code.getSystem(); 943 } 944 } 945 946 public enum ActionSelectionBehavior { 947 /** 948 * Any number of the actions in the group may be chosen, from zero to all. 949 */ 950 ANY, 951 /** 952 * All the actions in the group must be selected as a single unit. 953 */ 954 ALL, 955 /** 956 * All the actions in the group are meant to be chosen as a single unit: either 957 * all must be selected by the end user, or none may be selected. 958 */ 959 ALLORNONE, 960 /** 961 * The end user must choose one and only one of the selectable actions in the 962 * group. The user SHALL NOT choose none of the actions in the group. 963 */ 964 EXACTLYONE, 965 /** 966 * The end user may choose zero or at most one of the actions in the group. 967 */ 968 ATMOSTONE, 969 /** 970 * The end user must choose a minimum of one, and as many additional as desired. 971 */ 972 ONEORMORE, 973 /** 974 * added to help the parsers with the generic types 975 */ 976 NULL; 977 978 public static ActionSelectionBehavior fromCode(String codeString) throws FHIRException { 979 if (codeString == null || "".equals(codeString)) 980 return null; 981 if ("any".equals(codeString)) 982 return ANY; 983 if ("all".equals(codeString)) 984 return ALL; 985 if ("all-or-none".equals(codeString)) 986 return ALLORNONE; 987 if ("exactly-one".equals(codeString)) 988 return EXACTLYONE; 989 if ("at-most-one".equals(codeString)) 990 return ATMOSTONE; 991 if ("one-or-more".equals(codeString)) 992 return ONEORMORE; 993 if (Configuration.isAcceptInvalidEnums()) 994 return null; 995 else 996 throw new FHIRException("Unknown ActionSelectionBehavior code '" + codeString + "'"); 997 } 998 999 public String toCode() { 1000 switch (this) { 1001 case ANY: 1002 return "any"; 1003 case ALL: 1004 return "all"; 1005 case ALLORNONE: 1006 return "all-or-none"; 1007 case EXACTLYONE: 1008 return "exactly-one"; 1009 case ATMOSTONE: 1010 return "at-most-one"; 1011 case ONEORMORE: 1012 return "one-or-more"; 1013 case NULL: 1014 return null; 1015 default: 1016 return "?"; 1017 } 1018 } 1019 1020 public String getSystem() { 1021 switch (this) { 1022 case ANY: 1023 return "http://hl7.org/fhir/action-selection-behavior"; 1024 case ALL: 1025 return "http://hl7.org/fhir/action-selection-behavior"; 1026 case ALLORNONE: 1027 return "http://hl7.org/fhir/action-selection-behavior"; 1028 case EXACTLYONE: 1029 return "http://hl7.org/fhir/action-selection-behavior"; 1030 case ATMOSTONE: 1031 return "http://hl7.org/fhir/action-selection-behavior"; 1032 case ONEORMORE: 1033 return "http://hl7.org/fhir/action-selection-behavior"; 1034 case NULL: 1035 return null; 1036 default: 1037 return "?"; 1038 } 1039 } 1040 1041 public String getDefinition() { 1042 switch (this) { 1043 case ANY: 1044 return "Any number of the actions in the group may be chosen, from zero to all."; 1045 case ALL: 1046 return "All the actions in the group must be selected as a single unit."; 1047 case ALLORNONE: 1048 return "All the actions in the group are meant to be chosen as a single unit: either all must be selected by the end user, or none may be selected."; 1049 case EXACTLYONE: 1050 return "The end user must choose one and only one of the selectable actions in the group. The user SHALL NOT choose none of the actions in the group."; 1051 case ATMOSTONE: 1052 return "The end user may choose zero or at most one of the actions in the group."; 1053 case ONEORMORE: 1054 return "The end user must choose a minimum of one, and as many additional as desired."; 1055 case NULL: 1056 return null; 1057 default: 1058 return "?"; 1059 } 1060 } 1061 1062 public String getDisplay() { 1063 switch (this) { 1064 case ANY: 1065 return "Any"; 1066 case ALL: 1067 return "All"; 1068 case ALLORNONE: 1069 return "All Or None"; 1070 case EXACTLYONE: 1071 return "Exactly One"; 1072 case ATMOSTONE: 1073 return "At Most One"; 1074 case ONEORMORE: 1075 return "One Or More"; 1076 case NULL: 1077 return null; 1078 default: 1079 return "?"; 1080 } 1081 } 1082 } 1083 1084 public static class ActionSelectionBehaviorEnumFactory implements EnumFactory<ActionSelectionBehavior> { 1085 public ActionSelectionBehavior fromCode(String codeString) throws IllegalArgumentException { 1086 if (codeString == null || "".equals(codeString)) 1087 if (codeString == null || "".equals(codeString)) 1088 return null; 1089 if ("any".equals(codeString)) 1090 return ActionSelectionBehavior.ANY; 1091 if ("all".equals(codeString)) 1092 return ActionSelectionBehavior.ALL; 1093 if ("all-or-none".equals(codeString)) 1094 return ActionSelectionBehavior.ALLORNONE; 1095 if ("exactly-one".equals(codeString)) 1096 return ActionSelectionBehavior.EXACTLYONE; 1097 if ("at-most-one".equals(codeString)) 1098 return ActionSelectionBehavior.ATMOSTONE; 1099 if ("one-or-more".equals(codeString)) 1100 return ActionSelectionBehavior.ONEORMORE; 1101 throw new IllegalArgumentException("Unknown ActionSelectionBehavior code '" + codeString + "'"); 1102 } 1103 1104 public Enumeration<ActionSelectionBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 1105 if (code == null) 1106 return null; 1107 if (code.isEmpty()) 1108 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.NULL, code); 1109 String codeString = code.asStringValue(); 1110 if (codeString == null || "".equals(codeString)) 1111 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.NULL, code); 1112 if ("any".equals(codeString)) 1113 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ANY, code); 1114 if ("all".equals(codeString)) 1115 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ALL, code); 1116 if ("all-or-none".equals(codeString)) 1117 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ALLORNONE, code); 1118 if ("exactly-one".equals(codeString)) 1119 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.EXACTLYONE, code); 1120 if ("at-most-one".equals(codeString)) 1121 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ATMOSTONE, code); 1122 if ("one-or-more".equals(codeString)) 1123 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ONEORMORE, code); 1124 throw new FHIRException("Unknown ActionSelectionBehavior code '" + codeString + "'"); 1125 } 1126 1127 public String toCode(ActionSelectionBehavior code) { 1128 if (code == ActionSelectionBehavior.NULL) 1129 return null; 1130 if (code == ActionSelectionBehavior.ANY) 1131 return "any"; 1132 if (code == ActionSelectionBehavior.ALL) 1133 return "all"; 1134 if (code == ActionSelectionBehavior.ALLORNONE) 1135 return "all-or-none"; 1136 if (code == ActionSelectionBehavior.EXACTLYONE) 1137 return "exactly-one"; 1138 if (code == ActionSelectionBehavior.ATMOSTONE) 1139 return "at-most-one"; 1140 if (code == ActionSelectionBehavior.ONEORMORE) 1141 return "one-or-more"; 1142 return "?"; 1143 } 1144 1145 public String toSystem(ActionSelectionBehavior code) { 1146 return code.getSystem(); 1147 } 1148 } 1149 1150 public enum ActionRequiredBehavior { 1151 /** 1152 * An action with this behavior must be included in the actions processed by the 1153 * end user; the end user SHALL NOT choose not to include this action. 1154 */ 1155 MUST, 1156 /** 1157 * An action with this behavior may be included in the set of actions processed 1158 * by the end user. 1159 */ 1160 COULD, 1161 /** 1162 * An action with this behavior must be included in the set of actions processed 1163 * by the end user, unless the end user provides documentation as to why the 1164 * action was not included. 1165 */ 1166 MUSTUNLESSDOCUMENTED, 1167 /** 1168 * added to help the parsers with the generic types 1169 */ 1170 NULL; 1171 1172 public static ActionRequiredBehavior fromCode(String codeString) throws FHIRException { 1173 if (codeString == null || "".equals(codeString)) 1174 return null; 1175 if ("must".equals(codeString)) 1176 return MUST; 1177 if ("could".equals(codeString)) 1178 return COULD; 1179 if ("must-unless-documented".equals(codeString)) 1180 return MUSTUNLESSDOCUMENTED; 1181 if (Configuration.isAcceptInvalidEnums()) 1182 return null; 1183 else 1184 throw new FHIRException("Unknown ActionRequiredBehavior code '" + codeString + "'"); 1185 } 1186 1187 public String toCode() { 1188 switch (this) { 1189 case MUST: 1190 return "must"; 1191 case COULD: 1192 return "could"; 1193 case MUSTUNLESSDOCUMENTED: 1194 return "must-unless-documented"; 1195 case NULL: 1196 return null; 1197 default: 1198 return "?"; 1199 } 1200 } 1201 1202 public String getSystem() { 1203 switch (this) { 1204 case MUST: 1205 return "http://hl7.org/fhir/action-required-behavior"; 1206 case COULD: 1207 return "http://hl7.org/fhir/action-required-behavior"; 1208 case MUSTUNLESSDOCUMENTED: 1209 return "http://hl7.org/fhir/action-required-behavior"; 1210 case NULL: 1211 return null; 1212 default: 1213 return "?"; 1214 } 1215 } 1216 1217 public String getDefinition() { 1218 switch (this) { 1219 case MUST: 1220 return "An action with this behavior must be included in the actions processed by the end user; the end user SHALL NOT choose not to include this action."; 1221 case COULD: 1222 return "An action with this behavior may be included in the set of actions processed by the end user."; 1223 case MUSTUNLESSDOCUMENTED: 1224 return "An action with this behavior must be included in the set of actions processed by the end user, unless the end user provides documentation as to why the action was not included."; 1225 case NULL: 1226 return null; 1227 default: 1228 return "?"; 1229 } 1230 } 1231 1232 public String getDisplay() { 1233 switch (this) { 1234 case MUST: 1235 return "Must"; 1236 case COULD: 1237 return "Could"; 1238 case MUSTUNLESSDOCUMENTED: 1239 return "Must Unless Documented"; 1240 case NULL: 1241 return null; 1242 default: 1243 return "?"; 1244 } 1245 } 1246 } 1247 1248 public static class ActionRequiredBehaviorEnumFactory implements EnumFactory<ActionRequiredBehavior> { 1249 public ActionRequiredBehavior fromCode(String codeString) throws IllegalArgumentException { 1250 if (codeString == null || "".equals(codeString)) 1251 if (codeString == null || "".equals(codeString)) 1252 return null; 1253 if ("must".equals(codeString)) 1254 return ActionRequiredBehavior.MUST; 1255 if ("could".equals(codeString)) 1256 return ActionRequiredBehavior.COULD; 1257 if ("must-unless-documented".equals(codeString)) 1258 return ActionRequiredBehavior.MUSTUNLESSDOCUMENTED; 1259 throw new IllegalArgumentException("Unknown ActionRequiredBehavior code '" + codeString + "'"); 1260 } 1261 1262 public Enumeration<ActionRequiredBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 1263 if (code == null) 1264 return null; 1265 if (code.isEmpty()) 1266 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.NULL, code); 1267 String codeString = code.asStringValue(); 1268 if (codeString == null || "".equals(codeString)) 1269 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.NULL, code); 1270 if ("must".equals(codeString)) 1271 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.MUST, code); 1272 if ("could".equals(codeString)) 1273 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.COULD, code); 1274 if ("must-unless-documented".equals(codeString)) 1275 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.MUSTUNLESSDOCUMENTED, code); 1276 throw new FHIRException("Unknown ActionRequiredBehavior code '" + codeString + "'"); 1277 } 1278 1279 public String toCode(ActionRequiredBehavior code) { 1280 if (code == ActionRequiredBehavior.NULL) 1281 return null; 1282 if (code == ActionRequiredBehavior.MUST) 1283 return "must"; 1284 if (code == ActionRequiredBehavior.COULD) 1285 return "could"; 1286 if (code == ActionRequiredBehavior.MUSTUNLESSDOCUMENTED) 1287 return "must-unless-documented"; 1288 return "?"; 1289 } 1290 1291 public String toSystem(ActionRequiredBehavior code) { 1292 return code.getSystem(); 1293 } 1294 } 1295 1296 public enum ActionPrecheckBehavior { 1297 /** 1298 * An action with this behavior is one of the most frequent action that is, or 1299 * should be, included by an end user, for the particular context in which the 1300 * action occurs. The system displaying the action to the end user should 1301 * consider "pre-checking" such an action as a convenience for the user. 1302 */ 1303 YES, 1304 /** 1305 * An action with this behavior is one of the less frequent actions included by 1306 * the end user, for the particular context in which the action occurs. The 1307 * system displaying the actions to the end user would typically not "pre-check" 1308 * such an action. 1309 */ 1310 NO, 1311 /** 1312 * added to help the parsers with the generic types 1313 */ 1314 NULL; 1315 1316 public static ActionPrecheckBehavior fromCode(String codeString) throws FHIRException { 1317 if (codeString == null || "".equals(codeString)) 1318 return null; 1319 if ("yes".equals(codeString)) 1320 return YES; 1321 if ("no".equals(codeString)) 1322 return NO; 1323 if (Configuration.isAcceptInvalidEnums()) 1324 return null; 1325 else 1326 throw new FHIRException("Unknown ActionPrecheckBehavior code '" + codeString + "'"); 1327 } 1328 1329 public String toCode() { 1330 switch (this) { 1331 case YES: 1332 return "yes"; 1333 case NO: 1334 return "no"; 1335 case NULL: 1336 return null; 1337 default: 1338 return "?"; 1339 } 1340 } 1341 1342 public String getSystem() { 1343 switch (this) { 1344 case YES: 1345 return "http://hl7.org/fhir/action-precheck-behavior"; 1346 case NO: 1347 return "http://hl7.org/fhir/action-precheck-behavior"; 1348 case NULL: 1349 return null; 1350 default: 1351 return "?"; 1352 } 1353 } 1354 1355 public String getDefinition() { 1356 switch (this) { 1357 case YES: 1358 return "An action with this behavior is one of the most frequent action that is, or should be, included by an end user, for the particular context in which the action occurs. The system displaying the action to the end user should consider \"pre-checking\" such an action as a convenience for the user."; 1359 case NO: 1360 return "An action with this behavior is one of the less frequent actions included by the end user, for the particular context in which the action occurs. The system displaying the actions to the end user would typically not \"pre-check\" such an action."; 1361 case NULL: 1362 return null; 1363 default: 1364 return "?"; 1365 } 1366 } 1367 1368 public String getDisplay() { 1369 switch (this) { 1370 case YES: 1371 return "Yes"; 1372 case NO: 1373 return "No"; 1374 case NULL: 1375 return null; 1376 default: 1377 return "?"; 1378 } 1379 } 1380 } 1381 1382 public static class ActionPrecheckBehaviorEnumFactory implements EnumFactory<ActionPrecheckBehavior> { 1383 public ActionPrecheckBehavior fromCode(String codeString) throws IllegalArgumentException { 1384 if (codeString == null || "".equals(codeString)) 1385 if (codeString == null || "".equals(codeString)) 1386 return null; 1387 if ("yes".equals(codeString)) 1388 return ActionPrecheckBehavior.YES; 1389 if ("no".equals(codeString)) 1390 return ActionPrecheckBehavior.NO; 1391 throw new IllegalArgumentException("Unknown ActionPrecheckBehavior code '" + codeString + "'"); 1392 } 1393 1394 public Enumeration<ActionPrecheckBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 1395 if (code == null) 1396 return null; 1397 if (code.isEmpty()) 1398 return new Enumeration<ActionPrecheckBehavior>(this, ActionPrecheckBehavior.NULL, code); 1399 String codeString = code.asStringValue(); 1400 if (codeString == null || "".equals(codeString)) 1401 return new Enumeration<ActionPrecheckBehavior>(this, ActionPrecheckBehavior.NULL, code); 1402 if ("yes".equals(codeString)) 1403 return new Enumeration<ActionPrecheckBehavior>(this, ActionPrecheckBehavior.YES, code); 1404 if ("no".equals(codeString)) 1405 return new Enumeration<ActionPrecheckBehavior>(this, ActionPrecheckBehavior.NO, code); 1406 throw new FHIRException("Unknown ActionPrecheckBehavior code '" + codeString + "'"); 1407 } 1408 1409 public String toCode(ActionPrecheckBehavior code) { 1410 if (code == ActionPrecheckBehavior.NULL) 1411 return null; 1412 if (code == ActionPrecheckBehavior.YES) 1413 return "yes"; 1414 if (code == ActionPrecheckBehavior.NO) 1415 return "no"; 1416 return "?"; 1417 } 1418 1419 public String toSystem(ActionPrecheckBehavior code) { 1420 return code.getSystem(); 1421 } 1422 } 1423 1424 public enum ActionCardinalityBehavior { 1425 /** 1426 * The action may only be selected one time. 1427 */ 1428 SINGLE, 1429 /** 1430 * The action may be selected multiple times. 1431 */ 1432 MULTIPLE, 1433 /** 1434 * added to help the parsers with the generic types 1435 */ 1436 NULL; 1437 1438 public static ActionCardinalityBehavior fromCode(String codeString) throws FHIRException { 1439 if (codeString == null || "".equals(codeString)) 1440 return null; 1441 if ("single".equals(codeString)) 1442 return SINGLE; 1443 if ("multiple".equals(codeString)) 1444 return MULTIPLE; 1445 if (Configuration.isAcceptInvalidEnums()) 1446 return null; 1447 else 1448 throw new FHIRException("Unknown ActionCardinalityBehavior code '" + codeString + "'"); 1449 } 1450 1451 public String toCode() { 1452 switch (this) { 1453 case SINGLE: 1454 return "single"; 1455 case MULTIPLE: 1456 return "multiple"; 1457 case NULL: 1458 return null; 1459 default: 1460 return "?"; 1461 } 1462 } 1463 1464 public String getSystem() { 1465 switch (this) { 1466 case SINGLE: 1467 return "http://hl7.org/fhir/action-cardinality-behavior"; 1468 case MULTIPLE: 1469 return "http://hl7.org/fhir/action-cardinality-behavior"; 1470 case NULL: 1471 return null; 1472 default: 1473 return "?"; 1474 } 1475 } 1476 1477 public String getDefinition() { 1478 switch (this) { 1479 case SINGLE: 1480 return "The action may only be selected one time."; 1481 case MULTIPLE: 1482 return "The action may be selected multiple times."; 1483 case NULL: 1484 return null; 1485 default: 1486 return "?"; 1487 } 1488 } 1489 1490 public String getDisplay() { 1491 switch (this) { 1492 case SINGLE: 1493 return "Single"; 1494 case MULTIPLE: 1495 return "Multiple"; 1496 case NULL: 1497 return null; 1498 default: 1499 return "?"; 1500 } 1501 } 1502 } 1503 1504 public static class ActionCardinalityBehaviorEnumFactory implements EnumFactory<ActionCardinalityBehavior> { 1505 public ActionCardinalityBehavior fromCode(String codeString) throws IllegalArgumentException { 1506 if (codeString == null || "".equals(codeString)) 1507 if (codeString == null || "".equals(codeString)) 1508 return null; 1509 if ("single".equals(codeString)) 1510 return ActionCardinalityBehavior.SINGLE; 1511 if ("multiple".equals(codeString)) 1512 return ActionCardinalityBehavior.MULTIPLE; 1513 throw new IllegalArgumentException("Unknown ActionCardinalityBehavior code '" + codeString + "'"); 1514 } 1515 1516 public Enumeration<ActionCardinalityBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 1517 if (code == null) 1518 return null; 1519 if (code.isEmpty()) 1520 return new Enumeration<ActionCardinalityBehavior>(this, ActionCardinalityBehavior.NULL, code); 1521 String codeString = code.asStringValue(); 1522 if (codeString == null || "".equals(codeString)) 1523 return new Enumeration<ActionCardinalityBehavior>(this, ActionCardinalityBehavior.NULL, code); 1524 if ("single".equals(codeString)) 1525 return new Enumeration<ActionCardinalityBehavior>(this, ActionCardinalityBehavior.SINGLE, code); 1526 if ("multiple".equals(codeString)) 1527 return new Enumeration<ActionCardinalityBehavior>(this, ActionCardinalityBehavior.MULTIPLE, code); 1528 throw new FHIRException("Unknown ActionCardinalityBehavior code '" + codeString + "'"); 1529 } 1530 1531 public String toCode(ActionCardinalityBehavior code) { 1532 if (code == ActionCardinalityBehavior.NULL) 1533 return null; 1534 if (code == ActionCardinalityBehavior.SINGLE) 1535 return "single"; 1536 if (code == ActionCardinalityBehavior.MULTIPLE) 1537 return "multiple"; 1538 return "?"; 1539 } 1540 1541 public String toSystem(ActionCardinalityBehavior code) { 1542 return code.getSystem(); 1543 } 1544 } 1545 1546 @Block() 1547 public static class PlanDefinitionGoalComponent extends BackboneElement implements IBaseBackboneElement { 1548 /** 1549 * Indicates a category the goal falls within. 1550 */ 1551 @Child(name = "category", type = { 1552 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1553 @Description(shortDefinition = "E.g. Treatment, dietary, behavioral", formalDefinition = "Indicates a category the goal falls within.") 1554 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/goal-category") 1555 protected CodeableConcept category; 1556 1557 /** 1558 * Human-readable and/or coded description of a specific desired objective of 1559 * care, such as "control blood pressure" or "negotiate an obstacle course" or 1560 * "dance with child at wedding". 1561 */ 1562 @Child(name = "description", type = { 1563 CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 1564 @Description(shortDefinition = "Code or text describing the goal", formalDefinition = "Human-readable and/or coded description of a specific desired objective of care, such as \"control blood pressure\" or \"negotiate an obstacle course\" or \"dance with child at wedding\".") 1565 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/clinical-findings") 1566 protected CodeableConcept description; 1567 1568 /** 1569 * Identifies the expected level of importance associated with 1570 * reaching/sustaining the defined goal. 1571 */ 1572 @Child(name = "priority", type = { 1573 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1574 @Description(shortDefinition = "high-priority | medium-priority | low-priority", formalDefinition = "Identifies the expected level of importance associated with reaching/sustaining the defined goal.") 1575 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/goal-priority") 1576 protected CodeableConcept priority; 1577 1578 /** 1579 * The event after which the goal should begin being pursued. 1580 */ 1581 @Child(name = "start", type = { 1582 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1583 @Description(shortDefinition = "When goal pursuit begins", formalDefinition = "The event after which the goal should begin being pursued.") 1584 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/goal-start-event") 1585 protected CodeableConcept start; 1586 1587 /** 1588 * Identifies problems, conditions, issues, or concerns the goal is intended to 1589 * address. 1590 */ 1591 @Child(name = "addresses", type = { 1592 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1593 @Description(shortDefinition = "What does the goal address", formalDefinition = "Identifies problems, conditions, issues, or concerns the goal is intended to address.") 1594 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/condition-code") 1595 protected List<CodeableConcept> addresses; 1596 1597 /** 1598 * Didactic or other informational resources associated with the goal that 1599 * provide further supporting information about the goal. Information resources 1600 * can include inline text commentary and links to web resources. 1601 */ 1602 @Child(name = "documentation", type = { 1603 RelatedArtifact.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1604 @Description(shortDefinition = "Supporting documentation for the goal", formalDefinition = "Didactic or other informational resources associated with the goal that provide further supporting information about the goal. Information resources can include inline text commentary and links to web resources.") 1605 protected List<RelatedArtifact> documentation; 1606 1607 /** 1608 * Indicates what should be done and within what timeframe. 1609 */ 1610 @Child(name = "target", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1611 @Description(shortDefinition = "Target outcome for the goal", formalDefinition = "Indicates what should be done and within what timeframe.") 1612 protected List<PlanDefinitionGoalTargetComponent> target; 1613 1614 private static final long serialVersionUID = -795308926L; 1615 1616 /** 1617 * Constructor 1618 */ 1619 public PlanDefinitionGoalComponent() { 1620 super(); 1621 } 1622 1623 /** 1624 * Constructor 1625 */ 1626 public PlanDefinitionGoalComponent(CodeableConcept description) { 1627 super(); 1628 this.description = description; 1629 } 1630 1631 /** 1632 * @return {@link #category} (Indicates a category the goal falls within.) 1633 */ 1634 public CodeableConcept getCategory() { 1635 if (this.category == null) 1636 if (Configuration.errorOnAutoCreate()) 1637 throw new Error("Attempt to auto-create PlanDefinitionGoalComponent.category"); 1638 else if (Configuration.doAutoCreate()) 1639 this.category = new CodeableConcept(); // cc 1640 return this.category; 1641 } 1642 1643 public boolean hasCategory() { 1644 return this.category != null && !this.category.isEmpty(); 1645 } 1646 1647 /** 1648 * @param value {@link #category} (Indicates a category the goal falls within.) 1649 */ 1650 public PlanDefinitionGoalComponent setCategory(CodeableConcept value) { 1651 this.category = value; 1652 return this; 1653 } 1654 1655 /** 1656 * @return {@link #description} (Human-readable and/or coded description of a 1657 * specific desired objective of care, such as "control blood pressure" 1658 * or "negotiate an obstacle course" or "dance with child at wedding".) 1659 */ 1660 public CodeableConcept getDescription() { 1661 if (this.description == null) 1662 if (Configuration.errorOnAutoCreate()) 1663 throw new Error("Attempt to auto-create PlanDefinitionGoalComponent.description"); 1664 else if (Configuration.doAutoCreate()) 1665 this.description = new CodeableConcept(); // cc 1666 return this.description; 1667 } 1668 1669 public boolean hasDescription() { 1670 return this.description != null && !this.description.isEmpty(); 1671 } 1672 1673 /** 1674 * @param value {@link #description} (Human-readable and/or coded description of 1675 * a specific desired objective of care, such as "control blood 1676 * pressure" or "negotiate an obstacle course" or "dance with child 1677 * at wedding".) 1678 */ 1679 public PlanDefinitionGoalComponent setDescription(CodeableConcept value) { 1680 this.description = value; 1681 return this; 1682 } 1683 1684 /** 1685 * @return {@link #priority} (Identifies the expected level of importance 1686 * associated with reaching/sustaining the defined goal.) 1687 */ 1688 public CodeableConcept getPriority() { 1689 if (this.priority == null) 1690 if (Configuration.errorOnAutoCreate()) 1691 throw new Error("Attempt to auto-create PlanDefinitionGoalComponent.priority"); 1692 else if (Configuration.doAutoCreate()) 1693 this.priority = new CodeableConcept(); // cc 1694 return this.priority; 1695 } 1696 1697 public boolean hasPriority() { 1698 return this.priority != null && !this.priority.isEmpty(); 1699 } 1700 1701 /** 1702 * @param value {@link #priority} (Identifies the expected level of importance 1703 * associated with reaching/sustaining the defined goal.) 1704 */ 1705 public PlanDefinitionGoalComponent setPriority(CodeableConcept value) { 1706 this.priority = value; 1707 return this; 1708 } 1709 1710 /** 1711 * @return {@link #start} (The event after which the goal should begin being 1712 * pursued.) 1713 */ 1714 public CodeableConcept getStart() { 1715 if (this.start == null) 1716 if (Configuration.errorOnAutoCreate()) 1717 throw new Error("Attempt to auto-create PlanDefinitionGoalComponent.start"); 1718 else if (Configuration.doAutoCreate()) 1719 this.start = new CodeableConcept(); // cc 1720 return this.start; 1721 } 1722 1723 public boolean hasStart() { 1724 return this.start != null && !this.start.isEmpty(); 1725 } 1726 1727 /** 1728 * @param value {@link #start} (The event after which the goal should begin 1729 * being pursued.) 1730 */ 1731 public PlanDefinitionGoalComponent setStart(CodeableConcept value) { 1732 this.start = value; 1733 return this; 1734 } 1735 1736 /** 1737 * @return {@link #addresses} (Identifies problems, conditions, issues, or 1738 * concerns the goal is intended to address.) 1739 */ 1740 public List<CodeableConcept> getAddresses() { 1741 if (this.addresses == null) 1742 this.addresses = new ArrayList<CodeableConcept>(); 1743 return this.addresses; 1744 } 1745 1746 /** 1747 * @return Returns a reference to <code>this</code> for easy method chaining 1748 */ 1749 public PlanDefinitionGoalComponent setAddresses(List<CodeableConcept> theAddresses) { 1750 this.addresses = theAddresses; 1751 return this; 1752 } 1753 1754 public boolean hasAddresses() { 1755 if (this.addresses == null) 1756 return false; 1757 for (CodeableConcept item : this.addresses) 1758 if (!item.isEmpty()) 1759 return true; 1760 return false; 1761 } 1762 1763 public CodeableConcept addAddresses() { // 3 1764 CodeableConcept t = new CodeableConcept(); 1765 if (this.addresses == null) 1766 this.addresses = new ArrayList<CodeableConcept>(); 1767 this.addresses.add(t); 1768 return t; 1769 } 1770 1771 public PlanDefinitionGoalComponent addAddresses(CodeableConcept t) { // 3 1772 if (t == null) 1773 return this; 1774 if (this.addresses == null) 1775 this.addresses = new ArrayList<CodeableConcept>(); 1776 this.addresses.add(t); 1777 return this; 1778 } 1779 1780 /** 1781 * @return The first repetition of repeating field {@link #addresses}, creating 1782 * it if it does not already exist 1783 */ 1784 public CodeableConcept getAddressesFirstRep() { 1785 if (getAddresses().isEmpty()) { 1786 addAddresses(); 1787 } 1788 return getAddresses().get(0); 1789 } 1790 1791 /** 1792 * @return {@link #documentation} (Didactic or other informational resources 1793 * associated with the goal that provide further supporting information 1794 * about the goal. Information resources can include inline text 1795 * commentary and links to web resources.) 1796 */ 1797 public List<RelatedArtifact> getDocumentation() { 1798 if (this.documentation == null) 1799 this.documentation = new ArrayList<RelatedArtifact>(); 1800 return this.documentation; 1801 } 1802 1803 /** 1804 * @return Returns a reference to <code>this</code> for easy method chaining 1805 */ 1806 public PlanDefinitionGoalComponent setDocumentation(List<RelatedArtifact> theDocumentation) { 1807 this.documentation = theDocumentation; 1808 return this; 1809 } 1810 1811 public boolean hasDocumentation() { 1812 if (this.documentation == null) 1813 return false; 1814 for (RelatedArtifact item : this.documentation) 1815 if (!item.isEmpty()) 1816 return true; 1817 return false; 1818 } 1819 1820 public RelatedArtifact addDocumentation() { // 3 1821 RelatedArtifact t = new RelatedArtifact(); 1822 if (this.documentation == null) 1823 this.documentation = new ArrayList<RelatedArtifact>(); 1824 this.documentation.add(t); 1825 return t; 1826 } 1827 1828 public PlanDefinitionGoalComponent addDocumentation(RelatedArtifact t) { // 3 1829 if (t == null) 1830 return this; 1831 if (this.documentation == null) 1832 this.documentation = new ArrayList<RelatedArtifact>(); 1833 this.documentation.add(t); 1834 return this; 1835 } 1836 1837 /** 1838 * @return The first repetition of repeating field {@link #documentation}, 1839 * creating it if it does not already exist 1840 */ 1841 public RelatedArtifact getDocumentationFirstRep() { 1842 if (getDocumentation().isEmpty()) { 1843 addDocumentation(); 1844 } 1845 return getDocumentation().get(0); 1846 } 1847 1848 /** 1849 * @return {@link #target} (Indicates what should be done and within what 1850 * timeframe.) 1851 */ 1852 public List<PlanDefinitionGoalTargetComponent> getTarget() { 1853 if (this.target == null) 1854 this.target = new ArrayList<PlanDefinitionGoalTargetComponent>(); 1855 return this.target; 1856 } 1857 1858 /** 1859 * @return Returns a reference to <code>this</code> for easy method chaining 1860 */ 1861 public PlanDefinitionGoalComponent setTarget(List<PlanDefinitionGoalTargetComponent> theTarget) { 1862 this.target = theTarget; 1863 return this; 1864 } 1865 1866 public boolean hasTarget() { 1867 if (this.target == null) 1868 return false; 1869 for (PlanDefinitionGoalTargetComponent item : this.target) 1870 if (!item.isEmpty()) 1871 return true; 1872 return false; 1873 } 1874 1875 public PlanDefinitionGoalTargetComponent addTarget() { // 3 1876 PlanDefinitionGoalTargetComponent t = new PlanDefinitionGoalTargetComponent(); 1877 if (this.target == null) 1878 this.target = new ArrayList<PlanDefinitionGoalTargetComponent>(); 1879 this.target.add(t); 1880 return t; 1881 } 1882 1883 public PlanDefinitionGoalComponent addTarget(PlanDefinitionGoalTargetComponent t) { // 3 1884 if (t == null) 1885 return this; 1886 if (this.target == null) 1887 this.target = new ArrayList<PlanDefinitionGoalTargetComponent>(); 1888 this.target.add(t); 1889 return this; 1890 } 1891 1892 /** 1893 * @return The first repetition of repeating field {@link #target}, creating it 1894 * if it does not already exist 1895 */ 1896 public PlanDefinitionGoalTargetComponent getTargetFirstRep() { 1897 if (getTarget().isEmpty()) { 1898 addTarget(); 1899 } 1900 return getTarget().get(0); 1901 } 1902 1903 protected void listChildren(List<Property> children) { 1904 super.listChildren(children); 1905 children.add( 1906 new Property("category", "CodeableConcept", "Indicates a category the goal falls within.", 0, 1, category)); 1907 children.add(new Property("description", "CodeableConcept", 1908 "Human-readable and/or coded description of a specific desired objective of care, such as \"control blood pressure\" or \"negotiate an obstacle course\" or \"dance with child at wedding\".", 1909 0, 1, description)); 1910 children.add(new Property("priority", "CodeableConcept", 1911 "Identifies the expected level of importance associated with reaching/sustaining the defined goal.", 0, 1, 1912 priority)); 1913 children.add(new Property("start", "CodeableConcept", 1914 "The event after which the goal should begin being pursued.", 0, 1, start)); 1915 children.add(new Property("addresses", "CodeableConcept", 1916 "Identifies problems, conditions, issues, or concerns the goal is intended to address.", 0, 1917 java.lang.Integer.MAX_VALUE, addresses)); 1918 children.add(new Property("documentation", "RelatedArtifact", 1919 "Didactic or other informational resources associated with the goal that provide further supporting information about the goal. Information resources can include inline text commentary and links to web resources.", 1920 0, java.lang.Integer.MAX_VALUE, documentation)); 1921 children.add(new Property("target", "", "Indicates what should be done and within what timeframe.", 0, 1922 java.lang.Integer.MAX_VALUE, target)); 1923 } 1924 1925 @Override 1926 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1927 switch (_hash) { 1928 case 50511102: 1929 /* category */ return new Property("category", "CodeableConcept", "Indicates a category the goal falls within.", 1930 0, 1, category); 1931 case -1724546052: 1932 /* description */ return new Property("description", "CodeableConcept", 1933 "Human-readable and/or coded description of a specific desired objective of care, such as \"control blood pressure\" or \"negotiate an obstacle course\" or \"dance with child at wedding\".", 1934 0, 1, description); 1935 case -1165461084: 1936 /* priority */ return new Property("priority", "CodeableConcept", 1937 "Identifies the expected level of importance associated with reaching/sustaining the defined goal.", 0, 1, 1938 priority); 1939 case 109757538: 1940 /* start */ return new Property("start", "CodeableConcept", 1941 "The event after which the goal should begin being pursued.", 0, 1, start); 1942 case 874544034: 1943 /* addresses */ return new Property("addresses", "CodeableConcept", 1944 "Identifies problems, conditions, issues, or concerns the goal is intended to address.", 0, 1945 java.lang.Integer.MAX_VALUE, addresses); 1946 case 1587405498: 1947 /* documentation */ return new Property("documentation", "RelatedArtifact", 1948 "Didactic or other informational resources associated with the goal that provide further supporting information about the goal. Information resources can include inline text commentary and links to web resources.", 1949 0, java.lang.Integer.MAX_VALUE, documentation); 1950 case -880905839: 1951 /* target */ return new Property("target", "", "Indicates what should be done and within what timeframe.", 0, 1952 java.lang.Integer.MAX_VALUE, target); 1953 default: 1954 return super.getNamedProperty(_hash, _name, _checkValid); 1955 } 1956 1957 } 1958 1959 @Override 1960 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1961 switch (hash) { 1962 case 50511102: 1963 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 1964 case -1724546052: 1965 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // CodeableConcept 1966 case -1165461084: 1967 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // CodeableConcept 1968 case 109757538: 1969 /* start */ return this.start == null ? new Base[0] : new Base[] { this.start }; // CodeableConcept 1970 case 874544034: 1971 /* addresses */ return this.addresses == null ? new Base[0] 1972 : this.addresses.toArray(new Base[this.addresses.size()]); // CodeableConcept 1973 case 1587405498: 1974 /* documentation */ return this.documentation == null ? new Base[0] 1975 : this.documentation.toArray(new Base[this.documentation.size()]); // RelatedArtifact 1976 case -880905839: 1977 /* target */ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // PlanDefinitionGoalTargetComponent 1978 default: 1979 return super.getProperty(hash, name, checkValid); 1980 } 1981 1982 } 1983 1984 @Override 1985 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1986 switch (hash) { 1987 case 50511102: // category 1988 this.category = castToCodeableConcept(value); // CodeableConcept 1989 return value; 1990 case -1724546052: // description 1991 this.description = castToCodeableConcept(value); // CodeableConcept 1992 return value; 1993 case -1165461084: // priority 1994 this.priority = castToCodeableConcept(value); // CodeableConcept 1995 return value; 1996 case 109757538: // start 1997 this.start = castToCodeableConcept(value); // CodeableConcept 1998 return value; 1999 case 874544034: // addresses 2000 this.getAddresses().add(castToCodeableConcept(value)); // CodeableConcept 2001 return value; 2002 case 1587405498: // documentation 2003 this.getDocumentation().add(castToRelatedArtifact(value)); // RelatedArtifact 2004 return value; 2005 case -880905839: // target 2006 this.getTarget().add((PlanDefinitionGoalTargetComponent) value); // PlanDefinitionGoalTargetComponent 2007 return value; 2008 default: 2009 return super.setProperty(hash, name, value); 2010 } 2011 2012 } 2013 2014 @Override 2015 public Base setProperty(String name, Base value) throws FHIRException { 2016 if (name.equals("category")) { 2017 this.category = castToCodeableConcept(value); // CodeableConcept 2018 } else if (name.equals("description")) { 2019 this.description = castToCodeableConcept(value); // CodeableConcept 2020 } else if (name.equals("priority")) { 2021 this.priority = castToCodeableConcept(value); // CodeableConcept 2022 } else if (name.equals("start")) { 2023 this.start = castToCodeableConcept(value); // CodeableConcept 2024 } else if (name.equals("addresses")) { 2025 this.getAddresses().add(castToCodeableConcept(value)); 2026 } else if (name.equals("documentation")) { 2027 this.getDocumentation().add(castToRelatedArtifact(value)); 2028 } else if (name.equals("target")) { 2029 this.getTarget().add((PlanDefinitionGoalTargetComponent) value); 2030 } else 2031 return super.setProperty(name, value); 2032 return value; 2033 } 2034 2035 @Override 2036 public void removeChild(String name, Base value) throws FHIRException { 2037 if (name.equals("category")) { 2038 this.category = null; 2039 } else if (name.equals("description")) { 2040 this.description = null; 2041 } else if (name.equals("priority")) { 2042 this.priority = null; 2043 } else if (name.equals("start")) { 2044 this.start = null; 2045 } else if (name.equals("addresses")) { 2046 this.getAddresses().remove(castToCodeableConcept(value)); 2047 } else if (name.equals("documentation")) { 2048 this.getDocumentation().remove(castToRelatedArtifact(value)); 2049 } else if (name.equals("target")) { 2050 this.getTarget().remove((PlanDefinitionGoalTargetComponent) value); 2051 } else 2052 super.removeChild(name, value); 2053 2054 } 2055 2056 @Override 2057 public Base makeProperty(int hash, String name) throws FHIRException { 2058 switch (hash) { 2059 case 50511102: 2060 return getCategory(); 2061 case -1724546052: 2062 return getDescription(); 2063 case -1165461084: 2064 return getPriority(); 2065 case 109757538: 2066 return getStart(); 2067 case 874544034: 2068 return addAddresses(); 2069 case 1587405498: 2070 return addDocumentation(); 2071 case -880905839: 2072 return addTarget(); 2073 default: 2074 return super.makeProperty(hash, name); 2075 } 2076 2077 } 2078 2079 @Override 2080 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2081 switch (hash) { 2082 case 50511102: 2083 /* category */ return new String[] { "CodeableConcept" }; 2084 case -1724546052: 2085 /* description */ return new String[] { "CodeableConcept" }; 2086 case -1165461084: 2087 /* priority */ return new String[] { "CodeableConcept" }; 2088 case 109757538: 2089 /* start */ return new String[] { "CodeableConcept" }; 2090 case 874544034: 2091 /* addresses */ return new String[] { "CodeableConcept" }; 2092 case 1587405498: 2093 /* documentation */ return new String[] { "RelatedArtifact" }; 2094 case -880905839: 2095 /* target */ return new String[] {}; 2096 default: 2097 return super.getTypesForProperty(hash, name); 2098 } 2099 2100 } 2101 2102 @Override 2103 public Base addChild(String name) throws FHIRException { 2104 if (name.equals("category")) { 2105 this.category = new CodeableConcept(); 2106 return this.category; 2107 } else if (name.equals("description")) { 2108 this.description = new CodeableConcept(); 2109 return this.description; 2110 } else if (name.equals("priority")) { 2111 this.priority = new CodeableConcept(); 2112 return this.priority; 2113 } else if (name.equals("start")) { 2114 this.start = new CodeableConcept(); 2115 return this.start; 2116 } else if (name.equals("addresses")) { 2117 return addAddresses(); 2118 } else if (name.equals("documentation")) { 2119 return addDocumentation(); 2120 } else if (name.equals("target")) { 2121 return addTarget(); 2122 } else 2123 return super.addChild(name); 2124 } 2125 2126 public PlanDefinitionGoalComponent copy() { 2127 PlanDefinitionGoalComponent dst = new PlanDefinitionGoalComponent(); 2128 copyValues(dst); 2129 return dst; 2130 } 2131 2132 public void copyValues(PlanDefinitionGoalComponent dst) { 2133 super.copyValues(dst); 2134 dst.category = category == null ? null : category.copy(); 2135 dst.description = description == null ? null : description.copy(); 2136 dst.priority = priority == null ? null : priority.copy(); 2137 dst.start = start == null ? null : start.copy(); 2138 if (addresses != null) { 2139 dst.addresses = new ArrayList<CodeableConcept>(); 2140 for (CodeableConcept i : addresses) 2141 dst.addresses.add(i.copy()); 2142 } 2143 ; 2144 if (documentation != null) { 2145 dst.documentation = new ArrayList<RelatedArtifact>(); 2146 for (RelatedArtifact i : documentation) 2147 dst.documentation.add(i.copy()); 2148 } 2149 ; 2150 if (target != null) { 2151 dst.target = new ArrayList<PlanDefinitionGoalTargetComponent>(); 2152 for (PlanDefinitionGoalTargetComponent i : target) 2153 dst.target.add(i.copy()); 2154 } 2155 ; 2156 } 2157 2158 @Override 2159 public boolean equalsDeep(Base other_) { 2160 if (!super.equalsDeep(other_)) 2161 return false; 2162 if (!(other_ instanceof PlanDefinitionGoalComponent)) 2163 return false; 2164 PlanDefinitionGoalComponent o = (PlanDefinitionGoalComponent) other_; 2165 return compareDeep(category, o.category, true) && compareDeep(description, o.description, true) 2166 && compareDeep(priority, o.priority, true) && compareDeep(start, o.start, true) 2167 && compareDeep(addresses, o.addresses, true) && compareDeep(documentation, o.documentation, true) 2168 && compareDeep(target, o.target, true); 2169 } 2170 2171 @Override 2172 public boolean equalsShallow(Base other_) { 2173 if (!super.equalsShallow(other_)) 2174 return false; 2175 if (!(other_ instanceof PlanDefinitionGoalComponent)) 2176 return false; 2177 PlanDefinitionGoalComponent o = (PlanDefinitionGoalComponent) other_; 2178 return true; 2179 } 2180 2181 public boolean isEmpty() { 2182 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, description, priority, start, addresses, 2183 documentation, target); 2184 } 2185 2186 public String fhirType() { 2187 return "PlanDefinition.goal"; 2188 2189 } 2190 2191 } 2192 2193 @Block() 2194 public static class PlanDefinitionGoalTargetComponent extends BackboneElement implements IBaseBackboneElement { 2195 /** 2196 * The parameter whose value is to be tracked, e.g. body weight, blood pressure, 2197 * or hemoglobin A1c level. 2198 */ 2199 @Child(name = "measure", type = { 2200 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2201 @Description(shortDefinition = "The parameter whose value is to be tracked", formalDefinition = "The parameter whose value is to be tracked, e.g. body weight, blood pressure, or hemoglobin A1c level.") 2202 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/observation-codes") 2203 protected CodeableConcept measure; 2204 2205 /** 2206 * The target value of the measure to be achieved to signify fulfillment of the 2207 * goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the 2208 * range can be specified. When a low value is missing, it indicates that the 2209 * goal is achieved at any value at or below the high value. Similarly, if the 2210 * high value is missing, it indicates that the goal is achieved at any value at 2211 * or above the low value. 2212 */ 2213 @Child(name = "detail", type = { Quantity.class, Range.class, 2214 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2215 @Description(shortDefinition = "The target value to be achieved", formalDefinition = "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.") 2216 protected Type detail; 2217 2218 /** 2219 * Indicates the timeframe after the start of the goal in which the goal should 2220 * be met. 2221 */ 2222 @Child(name = "due", type = { Duration.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2223 @Description(shortDefinition = "Reach goal within", formalDefinition = "Indicates the timeframe after the start of the goal in which the goal should be met.") 2224 protected Duration due; 2225 2226 private static final long serialVersionUID = -131874144L; 2227 2228 /** 2229 * Constructor 2230 */ 2231 public PlanDefinitionGoalTargetComponent() { 2232 super(); 2233 } 2234 2235 /** 2236 * @return {@link #measure} (The parameter whose value is to be tracked, e.g. 2237 * body weight, blood pressure, or hemoglobin A1c level.) 2238 */ 2239 public CodeableConcept getMeasure() { 2240 if (this.measure == null) 2241 if (Configuration.errorOnAutoCreate()) 2242 throw new Error("Attempt to auto-create PlanDefinitionGoalTargetComponent.measure"); 2243 else if (Configuration.doAutoCreate()) 2244 this.measure = new CodeableConcept(); // cc 2245 return this.measure; 2246 } 2247 2248 public boolean hasMeasure() { 2249 return this.measure != null && !this.measure.isEmpty(); 2250 } 2251 2252 /** 2253 * @param value {@link #measure} (The parameter whose value is to be tracked, 2254 * e.g. body weight, blood pressure, or hemoglobin A1c level.) 2255 */ 2256 public PlanDefinitionGoalTargetComponent setMeasure(CodeableConcept value) { 2257 this.measure = value; 2258 return this; 2259 } 2260 2261 /** 2262 * @return {@link #detail} (The target value of the measure to be achieved to 2263 * signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the 2264 * high or low or both values of the range can be specified. When a low 2265 * value is missing, it indicates that the goal is achieved at any value 2266 * at or below the high value. Similarly, if the high value is missing, 2267 * it indicates that the goal is achieved at any value at or above the 2268 * low value.) 2269 */ 2270 public Type getDetail() { 2271 return this.detail; 2272 } 2273 2274 /** 2275 * @return {@link #detail} (The target value of the measure to be achieved to 2276 * signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the 2277 * high or low or both values of the range can be specified. When a low 2278 * value is missing, it indicates that the goal is achieved at any value 2279 * at or below the high value. Similarly, if the high value is missing, 2280 * it indicates that the goal is achieved at any value at or above the 2281 * low value.) 2282 */ 2283 public Quantity getDetailQuantity() throws FHIRException { 2284 if (this.detail == null) 2285 this.detail = new Quantity(); 2286 if (!(this.detail instanceof Quantity)) 2287 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.detail.getClass().getName() 2288 + " was encountered"); 2289 return (Quantity) this.detail; 2290 } 2291 2292 public boolean hasDetailQuantity() { 2293 return this.detail instanceof Quantity; 2294 } 2295 2296 /** 2297 * @return {@link #detail} (The target value of the measure to be achieved to 2298 * signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the 2299 * high or low or both values of the range can be specified. When a low 2300 * value is missing, it indicates that the goal is achieved at any value 2301 * at or below the high value. Similarly, if the high value is missing, 2302 * it indicates that the goal is achieved at any value at or above the 2303 * low value.) 2304 */ 2305 public Range getDetailRange() throws FHIRException { 2306 if (this.detail == null) 2307 this.detail = new Range(); 2308 if (!(this.detail instanceof Range)) 2309 throw new FHIRException( 2310 "Type mismatch: the type Range was expected, but " + this.detail.getClass().getName() + " was encountered"); 2311 return (Range) this.detail; 2312 } 2313 2314 public boolean hasDetailRange() { 2315 return this.detail instanceof Range; 2316 } 2317 2318 /** 2319 * @return {@link #detail} (The target value of the measure to be achieved to 2320 * signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the 2321 * high or low or both values of the range can be specified. When a low 2322 * value is missing, it indicates that the goal is achieved at any value 2323 * at or below the high value. Similarly, if the high value is missing, 2324 * it indicates that the goal is achieved at any value at or above the 2325 * low value.) 2326 */ 2327 public CodeableConcept getDetailCodeableConcept() throws FHIRException { 2328 if (this.detail == null) 2329 this.detail = new CodeableConcept(); 2330 if (!(this.detail instanceof CodeableConcept)) 2331 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 2332 + this.detail.getClass().getName() + " was encountered"); 2333 return (CodeableConcept) this.detail; 2334 } 2335 2336 public boolean hasDetailCodeableConcept() { 2337 return this.detail instanceof CodeableConcept; 2338 } 2339 2340 public boolean hasDetail() { 2341 return this.detail != null && !this.detail.isEmpty(); 2342 } 2343 2344 /** 2345 * @param value {@link #detail} (The target value of the measure to be achieved 2346 * to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. 2347 * Either the high or low or both values of the range can be 2348 * specified. When a low value is missing, it indicates that the 2349 * goal is achieved at any value at or below the high value. 2350 * Similarly, if the high value is missing, it indicates that the 2351 * goal is achieved at any value at or above the low value.) 2352 */ 2353 public PlanDefinitionGoalTargetComponent setDetail(Type value) { 2354 if (value != null && !(value instanceof Quantity || value instanceof Range || value instanceof CodeableConcept)) 2355 throw new Error("Not the right type for PlanDefinition.goal.target.detail[x]: " + value.fhirType()); 2356 this.detail = value; 2357 return this; 2358 } 2359 2360 /** 2361 * @return {@link #due} (Indicates the timeframe after the start of the goal in 2362 * which the goal should be met.) 2363 */ 2364 public Duration getDue() { 2365 if (this.due == null) 2366 if (Configuration.errorOnAutoCreate()) 2367 throw new Error("Attempt to auto-create PlanDefinitionGoalTargetComponent.due"); 2368 else if (Configuration.doAutoCreate()) 2369 this.due = new Duration(); // cc 2370 return this.due; 2371 } 2372 2373 public boolean hasDue() { 2374 return this.due != null && !this.due.isEmpty(); 2375 } 2376 2377 /** 2378 * @param value {@link #due} (Indicates the timeframe after the start of the 2379 * goal in which the goal should be met.) 2380 */ 2381 public PlanDefinitionGoalTargetComponent setDue(Duration value) { 2382 this.due = value; 2383 return this; 2384 } 2385 2386 protected void listChildren(List<Property> children) { 2387 super.listChildren(children); 2388 children.add(new Property("measure", "CodeableConcept", 2389 "The parameter whose value is to be tracked, e.g. body weight, blood pressure, or hemoglobin A1c level.", 0, 2390 1, measure)); 2391 children.add(new Property("detail[x]", "Quantity|Range|CodeableConcept", 2392 "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.", 2393 0, 1, detail)); 2394 children.add(new Property("due", "Duration", 2395 "Indicates the timeframe after the start of the goal in which the goal should be met.", 0, 1, due)); 2396 } 2397 2398 @Override 2399 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2400 switch (_hash) { 2401 case 938321246: 2402 /* measure */ return new Property("measure", "CodeableConcept", 2403 "The parameter whose value is to be tracked, e.g. body weight, blood pressure, or hemoglobin A1c level.", 0, 2404 1, measure); 2405 case -1973084529: 2406 /* detail[x] */ return new Property("detail[x]", "Quantity|Range|CodeableConcept", 2407 "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.", 2408 0, 1, detail); 2409 case -1335224239: 2410 /* detail */ return new Property("detail[x]", "Quantity|Range|CodeableConcept", 2411 "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.", 2412 0, 1, detail); 2413 case -1313079300: 2414 /* detailQuantity */ return new Property("detail[x]", "Quantity|Range|CodeableConcept", 2415 "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.", 2416 0, 1, detail); 2417 case -2062632084: 2418 /* detailRange */ return new Property("detail[x]", "Quantity|Range|CodeableConcept", 2419 "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.", 2420 0, 1, detail); 2421 case -175586544: 2422 /* detailCodeableConcept */ return new Property("detail[x]", "Quantity|Range|CodeableConcept", 2423 "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.", 2424 0, 1, detail); 2425 case 99828: 2426 /* due */ return new Property("due", "Duration", 2427 "Indicates the timeframe after the start of the goal in which the goal should be met.", 0, 1, due); 2428 default: 2429 return super.getNamedProperty(_hash, _name, _checkValid); 2430 } 2431 2432 } 2433 2434 @Override 2435 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2436 switch (hash) { 2437 case 938321246: 2438 /* measure */ return this.measure == null ? new Base[0] : new Base[] { this.measure }; // CodeableConcept 2439 case -1335224239: 2440 /* detail */ return this.detail == null ? new Base[0] : new Base[] { this.detail }; // Type 2441 case 99828: 2442 /* due */ return this.due == null ? new Base[0] : new Base[] { this.due }; // Duration 2443 default: 2444 return super.getProperty(hash, name, checkValid); 2445 } 2446 2447 } 2448 2449 @Override 2450 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2451 switch (hash) { 2452 case 938321246: // measure 2453 this.measure = castToCodeableConcept(value); // CodeableConcept 2454 return value; 2455 case -1335224239: // detail 2456 this.detail = castToType(value); // Type 2457 return value; 2458 case 99828: // due 2459 this.due = castToDuration(value); // Duration 2460 return value; 2461 default: 2462 return super.setProperty(hash, name, value); 2463 } 2464 2465 } 2466 2467 @Override 2468 public Base setProperty(String name, Base value) throws FHIRException { 2469 if (name.equals("measure")) { 2470 this.measure = castToCodeableConcept(value); // CodeableConcept 2471 } else if (name.equals("detail[x]")) { 2472 this.detail = castToType(value); // Type 2473 } else if (name.equals("due")) { 2474 this.due = castToDuration(value); // Duration 2475 } else 2476 return super.setProperty(name, value); 2477 return value; 2478 } 2479 2480 @Override 2481 public void removeChild(String name, Base value) throws FHIRException { 2482 if (name.equals("measure")) { 2483 this.measure = null; 2484 } else if (name.equals("detail[x]")) { 2485 this.detail = null; 2486 } else if (name.equals("due")) { 2487 this.due = null; 2488 } else 2489 super.removeChild(name, value); 2490 2491 } 2492 2493 @Override 2494 public Base makeProperty(int hash, String name) throws FHIRException { 2495 switch (hash) { 2496 case 938321246: 2497 return getMeasure(); 2498 case -1973084529: 2499 return getDetail(); 2500 case -1335224239: 2501 return getDetail(); 2502 case 99828: 2503 return getDue(); 2504 default: 2505 return super.makeProperty(hash, name); 2506 } 2507 2508 } 2509 2510 @Override 2511 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2512 switch (hash) { 2513 case 938321246: 2514 /* measure */ return new String[] { "CodeableConcept" }; 2515 case -1335224239: 2516 /* detail */ return new String[] { "Quantity", "Range", "CodeableConcept" }; 2517 case 99828: 2518 /* due */ return new String[] { "Duration" }; 2519 default: 2520 return super.getTypesForProperty(hash, name); 2521 } 2522 2523 } 2524 2525 @Override 2526 public Base addChild(String name) throws FHIRException { 2527 if (name.equals("measure")) { 2528 this.measure = new CodeableConcept(); 2529 return this.measure; 2530 } else if (name.equals("detailQuantity")) { 2531 this.detail = new Quantity(); 2532 return this.detail; 2533 } else if (name.equals("detailRange")) { 2534 this.detail = new Range(); 2535 return this.detail; 2536 } else if (name.equals("detailCodeableConcept")) { 2537 this.detail = new CodeableConcept(); 2538 return this.detail; 2539 } else if (name.equals("due")) { 2540 this.due = new Duration(); 2541 return this.due; 2542 } else 2543 return super.addChild(name); 2544 } 2545 2546 public PlanDefinitionGoalTargetComponent copy() { 2547 PlanDefinitionGoalTargetComponent dst = new PlanDefinitionGoalTargetComponent(); 2548 copyValues(dst); 2549 return dst; 2550 } 2551 2552 public void copyValues(PlanDefinitionGoalTargetComponent dst) { 2553 super.copyValues(dst); 2554 dst.measure = measure == null ? null : measure.copy(); 2555 dst.detail = detail == null ? null : detail.copy(); 2556 dst.due = due == null ? null : due.copy(); 2557 } 2558 2559 @Override 2560 public boolean equalsDeep(Base other_) { 2561 if (!super.equalsDeep(other_)) 2562 return false; 2563 if (!(other_ instanceof PlanDefinitionGoalTargetComponent)) 2564 return false; 2565 PlanDefinitionGoalTargetComponent o = (PlanDefinitionGoalTargetComponent) other_; 2566 return compareDeep(measure, o.measure, true) && compareDeep(detail, o.detail, true) 2567 && compareDeep(due, o.due, true); 2568 } 2569 2570 @Override 2571 public boolean equalsShallow(Base other_) { 2572 if (!super.equalsShallow(other_)) 2573 return false; 2574 if (!(other_ instanceof PlanDefinitionGoalTargetComponent)) 2575 return false; 2576 PlanDefinitionGoalTargetComponent o = (PlanDefinitionGoalTargetComponent) other_; 2577 return true; 2578 } 2579 2580 public boolean isEmpty() { 2581 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(measure, detail, due); 2582 } 2583 2584 public String fhirType() { 2585 return "PlanDefinition.goal.target"; 2586 2587 } 2588 2589 } 2590 2591 @Block() 2592 public static class PlanDefinitionActionComponent extends BackboneElement implements IBaseBackboneElement { 2593 /** 2594 * A user-visible prefix for the action. 2595 */ 2596 @Child(name = "prefix", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2597 @Description(shortDefinition = "User-visible prefix for the action (e.g. 1. or A.)", formalDefinition = "A user-visible prefix for the action.") 2598 protected StringType prefix; 2599 2600 /** 2601 * The title of the action displayed to a user. 2602 */ 2603 @Child(name = "title", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2604 @Description(shortDefinition = "User-visible title", formalDefinition = "The title of the action displayed to a user.") 2605 protected StringType title; 2606 2607 /** 2608 * A brief description of the action used to provide a summary to display to the 2609 * user. 2610 */ 2611 @Child(name = "description", type = { 2612 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2613 @Description(shortDefinition = "Brief description of the action", formalDefinition = "A brief description of the action used to provide a summary to display to the user.") 2614 protected StringType description; 2615 2616 /** 2617 * A text equivalent of the action to be performed. This provides a 2618 * human-interpretable description of the action when the definition is consumed 2619 * by a system that might not be capable of interpreting it dynamically. 2620 */ 2621 @Child(name = "textEquivalent", type = { 2622 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2623 @Description(shortDefinition = "Static text equivalent of the action, used if the dynamic aspects cannot be interpreted by the receiving system", formalDefinition = "A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that might not be capable of interpreting it dynamically.") 2624 protected StringType textEquivalent; 2625 2626 /** 2627 * Indicates how quickly the action should be addressed with respect to other 2628 * actions. 2629 */ 2630 @Child(name = "priority", type = { CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 2631 @Description(shortDefinition = "routine | urgent | asap | stat", formalDefinition = "Indicates how quickly the action should be addressed with respect to other actions.") 2632 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-priority") 2633 protected Enumeration<RequestPriority> priority; 2634 2635 /** 2636 * A code that provides meaning for the action or action group. For example, a 2637 * section may have a LOINC code for the section of a documentation template. 2638 */ 2639 @Child(name = "code", type = { 2640 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2641 @Description(shortDefinition = "Code representing the meaning of the action or sub-actions", formalDefinition = "A code that provides meaning for the action or action group. For example, a section may have a LOINC code for the section of a documentation template.") 2642 protected List<CodeableConcept> code; 2643 2644 /** 2645 * A description of why this action is necessary or appropriate. 2646 */ 2647 @Child(name = "reason", type = { 2648 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2649 @Description(shortDefinition = "Why the action should be performed", formalDefinition = "A description of why this action is necessary or appropriate.") 2650 protected List<CodeableConcept> reason; 2651 2652 /** 2653 * Didactic or other informational resources associated with the action that can 2654 * be provided to the CDS recipient. Information resources can include inline 2655 * text commentary and links to web resources. 2656 */ 2657 @Child(name = "documentation", type = { 2658 RelatedArtifact.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2659 @Description(shortDefinition = "Supporting documentation for the intended performer of the action", formalDefinition = "Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources.") 2660 protected List<RelatedArtifact> documentation; 2661 2662 /** 2663 * Identifies goals that this action supports. The reference must be to a goal 2664 * element defined within this plan definition. 2665 */ 2666 @Child(name = "goalId", type = { 2667 IdType.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2668 @Description(shortDefinition = "What goals this action supports", formalDefinition = "Identifies goals that this action supports. The reference must be to a goal element defined within this plan definition.") 2669 protected List<IdType> goalId; 2670 2671 /** 2672 * A code or group definition that describes the intended subject of the action 2673 * and its children, if any. 2674 */ 2675 @Child(name = "subject", type = { CodeableConcept.class, 2676 Group.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 2677 @Description(shortDefinition = "Type of individual the action is focused on", formalDefinition = "A code or group definition that describes the intended subject of the action and its children, if any.") 2678 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/subject-type") 2679 protected Type subject; 2680 2681 /** 2682 * A description of when the action should be triggered. 2683 */ 2684 @Child(name = "trigger", type = { 2685 TriggerDefinition.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2686 @Description(shortDefinition = "When the action should be triggered", formalDefinition = "A description of when the action should be triggered.") 2687 protected List<TriggerDefinition> trigger; 2688 2689 /** 2690 * An expression that describes applicability criteria or start/stop conditions 2691 * for the action. 2692 */ 2693 @Child(name = "condition", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2694 @Description(shortDefinition = "Whether or not the action is applicable", formalDefinition = "An expression that describes applicability criteria or start/stop conditions for the action.") 2695 protected List<PlanDefinitionActionConditionComponent> condition; 2696 2697 /** 2698 * Defines input data requirements for the action. 2699 */ 2700 @Child(name = "input", type = { 2701 DataRequirement.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2702 @Description(shortDefinition = "Input data requirements", formalDefinition = "Defines input data requirements for the action.") 2703 protected List<DataRequirement> input; 2704 2705 /** 2706 * Defines the outputs of the action, if any. 2707 */ 2708 @Child(name = "output", type = { 2709 DataRequirement.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2710 @Description(shortDefinition = "Output data definition", formalDefinition = "Defines the outputs of the action, if any.") 2711 protected List<DataRequirement> output; 2712 2713 /** 2714 * A relationship to another action such as "before" or "30-60 minutes after 2715 * start of". 2716 */ 2717 @Child(name = "relatedAction", type = {}, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2718 @Description(shortDefinition = "Relationship to another action", formalDefinition = "A relationship to another action such as \"before\" or \"30-60 minutes after start of\".") 2719 protected List<PlanDefinitionActionRelatedActionComponent> relatedAction; 2720 2721 /** 2722 * An optional value describing when the action should be performed. 2723 */ 2724 @Child(name = "timing", type = { DateTimeType.class, Age.class, Period.class, Duration.class, Range.class, 2725 Timing.class }, order = 16, min = 0, max = 1, modifier = false, summary = false) 2726 @Description(shortDefinition = "When the action should take place", formalDefinition = "An optional value describing when the action should be performed.") 2727 protected Type timing; 2728 2729 /** 2730 * Indicates who should participate in performing the action described. 2731 */ 2732 @Child(name = "participant", type = {}, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2733 @Description(shortDefinition = "Who should participate in the action", formalDefinition = "Indicates who should participate in performing the action described.") 2734 protected List<PlanDefinitionActionParticipantComponent> participant; 2735 2736 /** 2737 * The type of action to perform (create, update, remove). 2738 */ 2739 @Child(name = "type", type = { 2740 CodeableConcept.class }, order = 18, min = 0, max = 1, modifier = false, summary = false) 2741 @Description(shortDefinition = "create | update | remove | fire-event", formalDefinition = "The type of action to perform (create, update, remove).") 2742 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-type") 2743 protected CodeableConcept type; 2744 2745 /** 2746 * Defines the grouping behavior for the action and its children. 2747 */ 2748 @Child(name = "groupingBehavior", type = { 2749 CodeType.class }, order = 19, min = 0, max = 1, modifier = false, summary = false) 2750 @Description(shortDefinition = "visual-group | logical-group | sentence-group", formalDefinition = "Defines the grouping behavior for the action and its children.") 2751 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-grouping-behavior") 2752 protected Enumeration<ActionGroupingBehavior> groupingBehavior; 2753 2754 /** 2755 * Defines the selection behavior for the action and its children. 2756 */ 2757 @Child(name = "selectionBehavior", type = { 2758 CodeType.class }, order = 20, min = 0, max = 1, modifier = false, summary = false) 2759 @Description(shortDefinition = "any | all | all-or-none | exactly-one | at-most-one | one-or-more", formalDefinition = "Defines the selection behavior for the action and its children.") 2760 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-selection-behavior") 2761 protected Enumeration<ActionSelectionBehavior> selectionBehavior; 2762 2763 /** 2764 * Defines the required behavior for the action. 2765 */ 2766 @Child(name = "requiredBehavior", type = { 2767 CodeType.class }, order = 21, min = 0, max = 1, modifier = false, summary = false) 2768 @Description(shortDefinition = "must | could | must-unless-documented", formalDefinition = "Defines the required behavior for the action.") 2769 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-required-behavior") 2770 protected Enumeration<ActionRequiredBehavior> requiredBehavior; 2771 2772 /** 2773 * Defines whether the action should usually be preselected. 2774 */ 2775 @Child(name = "precheckBehavior", type = { 2776 CodeType.class }, order = 22, min = 0, max = 1, modifier = false, summary = false) 2777 @Description(shortDefinition = "yes | no", formalDefinition = "Defines whether the action should usually be preselected.") 2778 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-precheck-behavior") 2779 protected Enumeration<ActionPrecheckBehavior> precheckBehavior; 2780 2781 /** 2782 * Defines whether the action can be selected multiple times. 2783 */ 2784 @Child(name = "cardinalityBehavior", type = { 2785 CodeType.class }, order = 23, min = 0, max = 1, modifier = false, summary = false) 2786 @Description(shortDefinition = "single | multiple", formalDefinition = "Defines whether the action can be selected multiple times.") 2787 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-cardinality-behavior") 2788 protected Enumeration<ActionCardinalityBehavior> cardinalityBehavior; 2789 2790 /** 2791 * A reference to an ActivityDefinition that describes the action to be taken in 2792 * detail, or a PlanDefinition that describes a series of actions to be taken. 2793 */ 2794 @Child(name = "definition", type = { CanonicalType.class, 2795 UriType.class }, order = 24, min = 0, max = 1, modifier = false, summary = false) 2796 @Description(shortDefinition = "Description of the activity to be performed", formalDefinition = "A reference to an ActivityDefinition that describes the action to be taken in detail, or a PlanDefinition that describes a series of actions to be taken.") 2797 protected Type definition; 2798 2799 /** 2800 * A reference to a StructureMap resource that defines a transform that can be 2801 * executed to produce the intent resource using the ActivityDefinition instance 2802 * as the input. 2803 */ 2804 @Child(name = "transform", type = { 2805 CanonicalType.class }, order = 25, min = 0, max = 1, modifier = false, summary = false) 2806 @Description(shortDefinition = "Transform to apply the template", formalDefinition = "A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.") 2807 protected CanonicalType transform; 2808 2809 /** 2810 * Customizations that should be applied to the statically defined resource. For 2811 * example, if the dosage of a medication must be computed based on the 2812 * patient's weight, a customization would be used to specify an expression that 2813 * calculated the weight, and the path on the resource that would contain the 2814 * result. 2815 */ 2816 @Child(name = "dynamicValue", type = {}, order = 26, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2817 @Description(shortDefinition = "Dynamic aspects of the definition", formalDefinition = "Customizations that should be applied to the statically defined resource. For example, if the dosage of a medication must be computed based on the patient's weight, a customization would be used to specify an expression that calculated the weight, and the path on the resource that would contain the result.") 2818 protected List<PlanDefinitionActionDynamicValueComponent> dynamicValue; 2819 2820 /** 2821 * Sub actions that are contained within the action. The behavior of this action 2822 * determines the functionality of the sub-actions. For example, a selection 2823 * behavior of at-most-one indicates that of the sub-actions, at most one may be 2824 * chosen as part of realizing the action definition. 2825 */ 2826 @Child(name = "action", type = { 2827 PlanDefinitionActionComponent.class }, order = 27, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2828 @Description(shortDefinition = "A sub-action", formalDefinition = "Sub actions that are contained within the action. The behavior of this action determines the functionality of the sub-actions. For example, a selection behavior of at-most-one indicates that of the sub-actions, at most one may be chosen as part of realizing the action definition.") 2829 protected List<PlanDefinitionActionComponent> action; 2830 2831 private static final long serialVersionUID = 158605540L; 2832 2833 /** 2834 * Constructor 2835 */ 2836 public PlanDefinitionActionComponent() { 2837 super(); 2838 } 2839 2840 /** 2841 * @return {@link #prefix} (A user-visible prefix for the action.). This is the 2842 * underlying object with id, value and extensions. The accessor 2843 * "getPrefix" gives direct access to the value 2844 */ 2845 public StringType getPrefixElement() { 2846 if (this.prefix == null) 2847 if (Configuration.errorOnAutoCreate()) 2848 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.prefix"); 2849 else if (Configuration.doAutoCreate()) 2850 this.prefix = new StringType(); // bb 2851 return this.prefix; 2852 } 2853 2854 public boolean hasPrefixElement() { 2855 return this.prefix != null && !this.prefix.isEmpty(); 2856 } 2857 2858 public boolean hasPrefix() { 2859 return this.prefix != null && !this.prefix.isEmpty(); 2860 } 2861 2862 /** 2863 * @param value {@link #prefix} (A user-visible prefix for the action.). This is 2864 * the underlying object with id, value and extensions. The 2865 * accessor "getPrefix" gives direct access to the value 2866 */ 2867 public PlanDefinitionActionComponent setPrefixElement(StringType value) { 2868 this.prefix = value; 2869 return this; 2870 } 2871 2872 /** 2873 * @return A user-visible prefix for the action. 2874 */ 2875 public String getPrefix() { 2876 return this.prefix == null ? null : this.prefix.getValue(); 2877 } 2878 2879 /** 2880 * @param value A user-visible prefix for the action. 2881 */ 2882 public PlanDefinitionActionComponent setPrefix(String value) { 2883 if (Utilities.noString(value)) 2884 this.prefix = null; 2885 else { 2886 if (this.prefix == null) 2887 this.prefix = new StringType(); 2888 this.prefix.setValue(value); 2889 } 2890 return this; 2891 } 2892 2893 /** 2894 * @return {@link #title} (The title of the action displayed to a user.). This 2895 * is the underlying object with id, value and extensions. The accessor 2896 * "getTitle" gives direct access to the value 2897 */ 2898 public StringType getTitleElement() { 2899 if (this.title == null) 2900 if (Configuration.errorOnAutoCreate()) 2901 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.title"); 2902 else if (Configuration.doAutoCreate()) 2903 this.title = new StringType(); // bb 2904 return this.title; 2905 } 2906 2907 public boolean hasTitleElement() { 2908 return this.title != null && !this.title.isEmpty(); 2909 } 2910 2911 public boolean hasTitle() { 2912 return this.title != null && !this.title.isEmpty(); 2913 } 2914 2915 /** 2916 * @param value {@link #title} (The title of the action displayed to a user.). 2917 * This is the underlying object with id, value and extensions. The 2918 * accessor "getTitle" gives direct access to the value 2919 */ 2920 public PlanDefinitionActionComponent setTitleElement(StringType value) { 2921 this.title = value; 2922 return this; 2923 } 2924 2925 /** 2926 * @return The title of the action displayed to a user. 2927 */ 2928 public String getTitle() { 2929 return this.title == null ? null : this.title.getValue(); 2930 } 2931 2932 /** 2933 * @param value The title of the action displayed to a user. 2934 */ 2935 public PlanDefinitionActionComponent setTitle(String value) { 2936 if (Utilities.noString(value)) 2937 this.title = null; 2938 else { 2939 if (this.title == null) 2940 this.title = new StringType(); 2941 this.title.setValue(value); 2942 } 2943 return this; 2944 } 2945 2946 /** 2947 * @return {@link #description} (A brief description of the action used to 2948 * provide a summary to display to the user.). This is the underlying 2949 * object with id, value and extensions. The accessor "getDescription" 2950 * gives direct access to the value 2951 */ 2952 public StringType getDescriptionElement() { 2953 if (this.description == null) 2954 if (Configuration.errorOnAutoCreate()) 2955 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.description"); 2956 else if (Configuration.doAutoCreate()) 2957 this.description = new StringType(); // bb 2958 return this.description; 2959 } 2960 2961 public boolean hasDescriptionElement() { 2962 return this.description != null && !this.description.isEmpty(); 2963 } 2964 2965 public boolean hasDescription() { 2966 return this.description != null && !this.description.isEmpty(); 2967 } 2968 2969 /** 2970 * @param value {@link #description} (A brief description of the action used to 2971 * provide a summary to display to the user.). This is the 2972 * underlying object with id, value and extensions. The accessor 2973 * "getDescription" gives direct access to the value 2974 */ 2975 public PlanDefinitionActionComponent setDescriptionElement(StringType value) { 2976 this.description = value; 2977 return this; 2978 } 2979 2980 /** 2981 * @return A brief description of the action used to provide a summary to 2982 * display to the user. 2983 */ 2984 public String getDescription() { 2985 return this.description == null ? null : this.description.getValue(); 2986 } 2987 2988 /** 2989 * @param value A brief description of the action used to provide a summary to 2990 * display to the user. 2991 */ 2992 public PlanDefinitionActionComponent setDescription(String value) { 2993 if (Utilities.noString(value)) 2994 this.description = null; 2995 else { 2996 if (this.description == null) 2997 this.description = new StringType(); 2998 this.description.setValue(value); 2999 } 3000 return this; 3001 } 3002 3003 /** 3004 * @return {@link #textEquivalent} (A text equivalent of the action to be 3005 * performed. This provides a human-interpretable description of the 3006 * action when the definition is consumed by a system that might not be 3007 * capable of interpreting it dynamically.). This is the underlying 3008 * object with id, value and extensions. The accessor 3009 * "getTextEquivalent" gives direct access to the value 3010 */ 3011 public StringType getTextEquivalentElement() { 3012 if (this.textEquivalent == null) 3013 if (Configuration.errorOnAutoCreate()) 3014 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.textEquivalent"); 3015 else if (Configuration.doAutoCreate()) 3016 this.textEquivalent = new StringType(); // bb 3017 return this.textEquivalent; 3018 } 3019 3020 public boolean hasTextEquivalentElement() { 3021 return this.textEquivalent != null && !this.textEquivalent.isEmpty(); 3022 } 3023 3024 public boolean hasTextEquivalent() { 3025 return this.textEquivalent != null && !this.textEquivalent.isEmpty(); 3026 } 3027 3028 /** 3029 * @param value {@link #textEquivalent} (A text equivalent of the action to be 3030 * performed. This provides a human-interpretable description of 3031 * the action when the definition is consumed by a system that 3032 * might not be capable of interpreting it dynamically.). This is 3033 * the underlying object with id, value and extensions. The 3034 * accessor "getTextEquivalent" gives direct access to the value 3035 */ 3036 public PlanDefinitionActionComponent setTextEquivalentElement(StringType value) { 3037 this.textEquivalent = value; 3038 return this; 3039 } 3040 3041 /** 3042 * @return A text equivalent of the action to be performed. This provides a 3043 * human-interpretable description of the action when the definition is 3044 * consumed by a system that might not be capable of interpreting it 3045 * dynamically. 3046 */ 3047 public String getTextEquivalent() { 3048 return this.textEquivalent == null ? null : this.textEquivalent.getValue(); 3049 } 3050 3051 /** 3052 * @param value A text equivalent of the action to be performed. This provides a 3053 * human-interpretable description of the action when the 3054 * definition is consumed by a system that might not be capable of 3055 * interpreting it dynamically. 3056 */ 3057 public PlanDefinitionActionComponent setTextEquivalent(String value) { 3058 if (Utilities.noString(value)) 3059 this.textEquivalent = null; 3060 else { 3061 if (this.textEquivalent == null) 3062 this.textEquivalent = new StringType(); 3063 this.textEquivalent.setValue(value); 3064 } 3065 return this; 3066 } 3067 3068 /** 3069 * @return {@link #priority} (Indicates how quickly the action should be 3070 * addressed with respect to other actions.). This is the underlying 3071 * object with id, value and extensions. The accessor "getPriority" 3072 * gives direct access to the value 3073 */ 3074 public Enumeration<RequestPriority> getPriorityElement() { 3075 if (this.priority == null) 3076 if (Configuration.errorOnAutoCreate()) 3077 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.priority"); 3078 else if (Configuration.doAutoCreate()) 3079 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 3080 return this.priority; 3081 } 3082 3083 public boolean hasPriorityElement() { 3084 return this.priority != null && !this.priority.isEmpty(); 3085 } 3086 3087 public boolean hasPriority() { 3088 return this.priority != null && !this.priority.isEmpty(); 3089 } 3090 3091 /** 3092 * @param value {@link #priority} (Indicates how quickly the action should be 3093 * addressed with respect to other actions.). This is the 3094 * underlying object with id, value and extensions. The accessor 3095 * "getPriority" gives direct access to the value 3096 */ 3097 public PlanDefinitionActionComponent setPriorityElement(Enumeration<RequestPriority> value) { 3098 this.priority = value; 3099 return this; 3100 } 3101 3102 /** 3103 * @return Indicates how quickly the action should be addressed with respect to 3104 * other actions. 3105 */ 3106 public RequestPriority getPriority() { 3107 return this.priority == null ? null : this.priority.getValue(); 3108 } 3109 3110 /** 3111 * @param value Indicates how quickly the action should be addressed with 3112 * respect to other actions. 3113 */ 3114 public PlanDefinitionActionComponent setPriority(RequestPriority value) { 3115 if (value == null) 3116 this.priority = null; 3117 else { 3118 if (this.priority == null) 3119 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 3120 this.priority.setValue(value); 3121 } 3122 return this; 3123 } 3124 3125 /** 3126 * @return {@link #code} (A code that provides meaning for the action or action 3127 * group. For example, a section may have a LOINC code for the section 3128 * of a documentation template.) 3129 */ 3130 public List<CodeableConcept> getCode() { 3131 if (this.code == null) 3132 this.code = new ArrayList<CodeableConcept>(); 3133 return this.code; 3134 } 3135 3136 /** 3137 * @return Returns a reference to <code>this</code> for easy method chaining 3138 */ 3139 public PlanDefinitionActionComponent setCode(List<CodeableConcept> theCode) { 3140 this.code = theCode; 3141 return this; 3142 } 3143 3144 public boolean hasCode() { 3145 if (this.code == null) 3146 return false; 3147 for (CodeableConcept item : this.code) 3148 if (!item.isEmpty()) 3149 return true; 3150 return false; 3151 } 3152 3153 public CodeableConcept addCode() { // 3 3154 CodeableConcept t = new CodeableConcept(); 3155 if (this.code == null) 3156 this.code = new ArrayList<CodeableConcept>(); 3157 this.code.add(t); 3158 return t; 3159 } 3160 3161 public PlanDefinitionActionComponent addCode(CodeableConcept t) { // 3 3162 if (t == null) 3163 return this; 3164 if (this.code == null) 3165 this.code = new ArrayList<CodeableConcept>(); 3166 this.code.add(t); 3167 return this; 3168 } 3169 3170 /** 3171 * @return The first repetition of repeating field {@link #code}, creating it if 3172 * it does not already exist 3173 */ 3174 public CodeableConcept getCodeFirstRep() { 3175 if (getCode().isEmpty()) { 3176 addCode(); 3177 } 3178 return getCode().get(0); 3179 } 3180 3181 /** 3182 * @return {@link #reason} (A description of why this action is necessary or 3183 * appropriate.) 3184 */ 3185 public List<CodeableConcept> getReason() { 3186 if (this.reason == null) 3187 this.reason = new ArrayList<CodeableConcept>(); 3188 return this.reason; 3189 } 3190 3191 /** 3192 * @return Returns a reference to <code>this</code> for easy method chaining 3193 */ 3194 public PlanDefinitionActionComponent setReason(List<CodeableConcept> theReason) { 3195 this.reason = theReason; 3196 return this; 3197 } 3198 3199 public boolean hasReason() { 3200 if (this.reason == null) 3201 return false; 3202 for (CodeableConcept item : this.reason) 3203 if (!item.isEmpty()) 3204 return true; 3205 return false; 3206 } 3207 3208 public CodeableConcept addReason() { // 3 3209 CodeableConcept t = new CodeableConcept(); 3210 if (this.reason == null) 3211 this.reason = new ArrayList<CodeableConcept>(); 3212 this.reason.add(t); 3213 return t; 3214 } 3215 3216 public PlanDefinitionActionComponent addReason(CodeableConcept t) { // 3 3217 if (t == null) 3218 return this; 3219 if (this.reason == null) 3220 this.reason = new ArrayList<CodeableConcept>(); 3221 this.reason.add(t); 3222 return this; 3223 } 3224 3225 /** 3226 * @return The first repetition of repeating field {@link #reason}, creating it 3227 * if it does not already exist 3228 */ 3229 public CodeableConcept getReasonFirstRep() { 3230 if (getReason().isEmpty()) { 3231 addReason(); 3232 } 3233 return getReason().get(0); 3234 } 3235 3236 /** 3237 * @return {@link #documentation} (Didactic or other informational resources 3238 * associated with the action that can be provided to the CDS recipient. 3239 * Information resources can include inline text commentary and links to 3240 * web resources.) 3241 */ 3242 public List<RelatedArtifact> getDocumentation() { 3243 if (this.documentation == null) 3244 this.documentation = new ArrayList<RelatedArtifact>(); 3245 return this.documentation; 3246 } 3247 3248 /** 3249 * @return Returns a reference to <code>this</code> for easy method chaining 3250 */ 3251 public PlanDefinitionActionComponent setDocumentation(List<RelatedArtifact> theDocumentation) { 3252 this.documentation = theDocumentation; 3253 return this; 3254 } 3255 3256 public boolean hasDocumentation() { 3257 if (this.documentation == null) 3258 return false; 3259 for (RelatedArtifact item : this.documentation) 3260 if (!item.isEmpty()) 3261 return true; 3262 return false; 3263 } 3264 3265 public RelatedArtifact addDocumentation() { // 3 3266 RelatedArtifact t = new RelatedArtifact(); 3267 if (this.documentation == null) 3268 this.documentation = new ArrayList<RelatedArtifact>(); 3269 this.documentation.add(t); 3270 return t; 3271 } 3272 3273 public PlanDefinitionActionComponent addDocumentation(RelatedArtifact t) { // 3 3274 if (t == null) 3275 return this; 3276 if (this.documentation == null) 3277 this.documentation = new ArrayList<RelatedArtifact>(); 3278 this.documentation.add(t); 3279 return this; 3280 } 3281 3282 /** 3283 * @return The first repetition of repeating field {@link #documentation}, 3284 * creating it if it does not already exist 3285 */ 3286 public RelatedArtifact getDocumentationFirstRep() { 3287 if (getDocumentation().isEmpty()) { 3288 addDocumentation(); 3289 } 3290 return getDocumentation().get(0); 3291 } 3292 3293 /** 3294 * @return {@link #goalId} (Identifies goals that this action supports. The 3295 * reference must be to a goal element defined within this plan 3296 * definition.) 3297 */ 3298 public List<IdType> getGoalId() { 3299 if (this.goalId == null) 3300 this.goalId = new ArrayList<IdType>(); 3301 return this.goalId; 3302 } 3303 3304 /** 3305 * @return Returns a reference to <code>this</code> for easy method chaining 3306 */ 3307 public PlanDefinitionActionComponent setGoalId(List<IdType> theGoalId) { 3308 this.goalId = theGoalId; 3309 return this; 3310 } 3311 3312 public boolean hasGoalId() { 3313 if (this.goalId == null) 3314 return false; 3315 for (IdType item : this.goalId) 3316 if (!item.isEmpty()) 3317 return true; 3318 return false; 3319 } 3320 3321 /** 3322 * @return {@link #goalId} (Identifies goals that this action supports. The 3323 * reference must be to a goal element defined within this plan 3324 * definition.) 3325 */ 3326 public IdType addGoalIdElement() {// 2 3327 IdType t = new IdType(); 3328 if (this.goalId == null) 3329 this.goalId = new ArrayList<IdType>(); 3330 this.goalId.add(t); 3331 return t; 3332 } 3333 3334 /** 3335 * @param value {@link #goalId} (Identifies goals that this action supports. The 3336 * reference must be to a goal element defined within this plan 3337 * definition.) 3338 */ 3339 public PlanDefinitionActionComponent addGoalId(String value) { // 1 3340 IdType t = new IdType(); 3341 t.setValue(value); 3342 if (this.goalId == null) 3343 this.goalId = new ArrayList<IdType>(); 3344 this.goalId.add(t); 3345 return this; 3346 } 3347 3348 /** 3349 * @param value {@link #goalId} (Identifies goals that this action supports. The 3350 * reference must be to a goal element defined within this plan 3351 * definition.) 3352 */ 3353 public boolean hasGoalId(String value) { 3354 if (this.goalId == null) 3355 return false; 3356 for (IdType v : this.goalId) 3357 if (v.getValue().equals(value)) // id 3358 return true; 3359 return false; 3360 } 3361 3362 /** 3363 * @return {@link #subject} (A code or group definition that describes the 3364 * intended subject of the action and its children, if any.) 3365 */ 3366 public Type getSubject() { 3367 return this.subject; 3368 } 3369 3370 /** 3371 * @return {@link #subject} (A code or group definition that describes the 3372 * intended subject of the action and its children, if any.) 3373 */ 3374 public CodeableConcept getSubjectCodeableConcept() throws FHIRException { 3375 if (this.subject == null) 3376 this.subject = new CodeableConcept(); 3377 if (!(this.subject instanceof CodeableConcept)) 3378 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 3379 + this.subject.getClass().getName() + " was encountered"); 3380 return (CodeableConcept) this.subject; 3381 } 3382 3383 public boolean hasSubjectCodeableConcept() { 3384 return this.subject instanceof CodeableConcept; 3385 } 3386 3387 /** 3388 * @return {@link #subject} (A code or group definition that describes the 3389 * intended subject of the action and its children, if any.) 3390 */ 3391 public Reference getSubjectReference() throws FHIRException { 3392 if (this.subject == null) 3393 this.subject = new Reference(); 3394 if (!(this.subject instanceof Reference)) 3395 throw new FHIRException("Type mismatch: the type Reference was expected, but " 3396 + this.subject.getClass().getName() + " was encountered"); 3397 return (Reference) this.subject; 3398 } 3399 3400 public boolean hasSubjectReference() { 3401 return this.subject instanceof Reference; 3402 } 3403 3404 public boolean hasSubject() { 3405 return this.subject != null && !this.subject.isEmpty(); 3406 } 3407 3408 /** 3409 * @param value {@link #subject} (A code or group definition that describes the 3410 * intended subject of the action and its children, if any.) 3411 */ 3412 public PlanDefinitionActionComponent setSubject(Type value) { 3413 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 3414 throw new Error("Not the right type for PlanDefinition.action.subject[x]: " + value.fhirType()); 3415 this.subject = value; 3416 return this; 3417 } 3418 3419 /** 3420 * @return {@link #trigger} (A description of when the action should be 3421 * triggered.) 3422 */ 3423 public List<TriggerDefinition> getTrigger() { 3424 if (this.trigger == null) 3425 this.trigger = new ArrayList<TriggerDefinition>(); 3426 return this.trigger; 3427 } 3428 3429 /** 3430 * @return Returns a reference to <code>this</code> for easy method chaining 3431 */ 3432 public PlanDefinitionActionComponent setTrigger(List<TriggerDefinition> theTrigger) { 3433 this.trigger = theTrigger; 3434 return this; 3435 } 3436 3437 public boolean hasTrigger() { 3438 if (this.trigger == null) 3439 return false; 3440 for (TriggerDefinition item : this.trigger) 3441 if (!item.isEmpty()) 3442 return true; 3443 return false; 3444 } 3445 3446 public TriggerDefinition addTrigger() { // 3 3447 TriggerDefinition t = new TriggerDefinition(); 3448 if (this.trigger == null) 3449 this.trigger = new ArrayList<TriggerDefinition>(); 3450 this.trigger.add(t); 3451 return t; 3452 } 3453 3454 public PlanDefinitionActionComponent addTrigger(TriggerDefinition t) { // 3 3455 if (t == null) 3456 return this; 3457 if (this.trigger == null) 3458 this.trigger = new ArrayList<TriggerDefinition>(); 3459 this.trigger.add(t); 3460 return this; 3461 } 3462 3463 /** 3464 * @return The first repetition of repeating field {@link #trigger}, creating it 3465 * if it does not already exist 3466 */ 3467 public TriggerDefinition getTriggerFirstRep() { 3468 if (getTrigger().isEmpty()) { 3469 addTrigger(); 3470 } 3471 return getTrigger().get(0); 3472 } 3473 3474 /** 3475 * @return {@link #condition} (An expression that describes applicability 3476 * criteria or start/stop conditions for the action.) 3477 */ 3478 public List<PlanDefinitionActionConditionComponent> getCondition() { 3479 if (this.condition == null) 3480 this.condition = new ArrayList<PlanDefinitionActionConditionComponent>(); 3481 return this.condition; 3482 } 3483 3484 /** 3485 * @return Returns a reference to <code>this</code> for easy method chaining 3486 */ 3487 public PlanDefinitionActionComponent setCondition(List<PlanDefinitionActionConditionComponent> theCondition) { 3488 this.condition = theCondition; 3489 return this; 3490 } 3491 3492 public boolean hasCondition() { 3493 if (this.condition == null) 3494 return false; 3495 for (PlanDefinitionActionConditionComponent item : this.condition) 3496 if (!item.isEmpty()) 3497 return true; 3498 return false; 3499 } 3500 3501 public PlanDefinitionActionConditionComponent addCondition() { // 3 3502 PlanDefinitionActionConditionComponent t = new PlanDefinitionActionConditionComponent(); 3503 if (this.condition == null) 3504 this.condition = new ArrayList<PlanDefinitionActionConditionComponent>(); 3505 this.condition.add(t); 3506 return t; 3507 } 3508 3509 public PlanDefinitionActionComponent addCondition(PlanDefinitionActionConditionComponent t) { // 3 3510 if (t == null) 3511 return this; 3512 if (this.condition == null) 3513 this.condition = new ArrayList<PlanDefinitionActionConditionComponent>(); 3514 this.condition.add(t); 3515 return this; 3516 } 3517 3518 /** 3519 * @return The first repetition of repeating field {@link #condition}, creating 3520 * it if it does not already exist 3521 */ 3522 public PlanDefinitionActionConditionComponent getConditionFirstRep() { 3523 if (getCondition().isEmpty()) { 3524 addCondition(); 3525 } 3526 return getCondition().get(0); 3527 } 3528 3529 /** 3530 * @return {@link #input} (Defines input data requirements for the action.) 3531 */ 3532 public List<DataRequirement> getInput() { 3533 if (this.input == null) 3534 this.input = new ArrayList<DataRequirement>(); 3535 return this.input; 3536 } 3537 3538 /** 3539 * @return Returns a reference to <code>this</code> for easy method chaining 3540 */ 3541 public PlanDefinitionActionComponent setInput(List<DataRequirement> theInput) { 3542 this.input = theInput; 3543 return this; 3544 } 3545 3546 public boolean hasInput() { 3547 if (this.input == null) 3548 return false; 3549 for (DataRequirement item : this.input) 3550 if (!item.isEmpty()) 3551 return true; 3552 return false; 3553 } 3554 3555 public DataRequirement addInput() { // 3 3556 DataRequirement t = new DataRequirement(); 3557 if (this.input == null) 3558 this.input = new ArrayList<DataRequirement>(); 3559 this.input.add(t); 3560 return t; 3561 } 3562 3563 public PlanDefinitionActionComponent addInput(DataRequirement t) { // 3 3564 if (t == null) 3565 return this; 3566 if (this.input == null) 3567 this.input = new ArrayList<DataRequirement>(); 3568 this.input.add(t); 3569 return this; 3570 } 3571 3572 /** 3573 * @return The first repetition of repeating field {@link #input}, creating it 3574 * if it does not already exist 3575 */ 3576 public DataRequirement getInputFirstRep() { 3577 if (getInput().isEmpty()) { 3578 addInput(); 3579 } 3580 return getInput().get(0); 3581 } 3582 3583 /** 3584 * @return {@link #output} (Defines the outputs of the action, if any.) 3585 */ 3586 public List<DataRequirement> getOutput() { 3587 if (this.output == null) 3588 this.output = new ArrayList<DataRequirement>(); 3589 return this.output; 3590 } 3591 3592 /** 3593 * @return Returns a reference to <code>this</code> for easy method chaining 3594 */ 3595 public PlanDefinitionActionComponent setOutput(List<DataRequirement> theOutput) { 3596 this.output = theOutput; 3597 return this; 3598 } 3599 3600 public boolean hasOutput() { 3601 if (this.output == null) 3602 return false; 3603 for (DataRequirement item : this.output) 3604 if (!item.isEmpty()) 3605 return true; 3606 return false; 3607 } 3608 3609 public DataRequirement addOutput() { // 3 3610 DataRequirement t = new DataRequirement(); 3611 if (this.output == null) 3612 this.output = new ArrayList<DataRequirement>(); 3613 this.output.add(t); 3614 return t; 3615 } 3616 3617 public PlanDefinitionActionComponent addOutput(DataRequirement t) { // 3 3618 if (t == null) 3619 return this; 3620 if (this.output == null) 3621 this.output = new ArrayList<DataRequirement>(); 3622 this.output.add(t); 3623 return this; 3624 } 3625 3626 /** 3627 * @return The first repetition of repeating field {@link #output}, creating it 3628 * if it does not already exist 3629 */ 3630 public DataRequirement getOutputFirstRep() { 3631 if (getOutput().isEmpty()) { 3632 addOutput(); 3633 } 3634 return getOutput().get(0); 3635 } 3636 3637 /** 3638 * @return {@link #relatedAction} (A relationship to another action such as 3639 * "before" or "30-60 minutes after start of".) 3640 */ 3641 public List<PlanDefinitionActionRelatedActionComponent> getRelatedAction() { 3642 if (this.relatedAction == null) 3643 this.relatedAction = new ArrayList<PlanDefinitionActionRelatedActionComponent>(); 3644 return this.relatedAction; 3645 } 3646 3647 /** 3648 * @return Returns a reference to <code>this</code> for easy method chaining 3649 */ 3650 public PlanDefinitionActionComponent setRelatedAction( 3651 List<PlanDefinitionActionRelatedActionComponent> theRelatedAction) { 3652 this.relatedAction = theRelatedAction; 3653 return this; 3654 } 3655 3656 public boolean hasRelatedAction() { 3657 if (this.relatedAction == null) 3658 return false; 3659 for (PlanDefinitionActionRelatedActionComponent item : this.relatedAction) 3660 if (!item.isEmpty()) 3661 return true; 3662 return false; 3663 } 3664 3665 public PlanDefinitionActionRelatedActionComponent addRelatedAction() { // 3 3666 PlanDefinitionActionRelatedActionComponent t = new PlanDefinitionActionRelatedActionComponent(); 3667 if (this.relatedAction == null) 3668 this.relatedAction = new ArrayList<PlanDefinitionActionRelatedActionComponent>(); 3669 this.relatedAction.add(t); 3670 return t; 3671 } 3672 3673 public PlanDefinitionActionComponent addRelatedAction(PlanDefinitionActionRelatedActionComponent t) { // 3 3674 if (t == null) 3675 return this; 3676 if (this.relatedAction == null) 3677 this.relatedAction = new ArrayList<PlanDefinitionActionRelatedActionComponent>(); 3678 this.relatedAction.add(t); 3679 return this; 3680 } 3681 3682 /** 3683 * @return The first repetition of repeating field {@link #relatedAction}, 3684 * creating it if it does not already exist 3685 */ 3686 public PlanDefinitionActionRelatedActionComponent getRelatedActionFirstRep() { 3687 if (getRelatedAction().isEmpty()) { 3688 addRelatedAction(); 3689 } 3690 return getRelatedAction().get(0); 3691 } 3692 3693 /** 3694 * @return {@link #timing} (An optional value describing when the action should 3695 * be performed.) 3696 */ 3697 public Type getTiming() { 3698 return this.timing; 3699 } 3700 3701 /** 3702 * @return {@link #timing} (An optional value describing when the action should 3703 * be performed.) 3704 */ 3705 public DateTimeType getTimingDateTimeType() throws FHIRException { 3706 if (this.timing == null) 3707 this.timing = new DateTimeType(); 3708 if (!(this.timing instanceof DateTimeType)) 3709 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 3710 + this.timing.getClass().getName() + " was encountered"); 3711 return (DateTimeType) this.timing; 3712 } 3713 3714 public boolean hasTimingDateTimeType() { 3715 return this.timing instanceof DateTimeType; 3716 } 3717 3718 /** 3719 * @return {@link #timing} (An optional value describing when the action should 3720 * be performed.) 3721 */ 3722 public Age getTimingAge() throws FHIRException { 3723 if (this.timing == null) 3724 this.timing = new Age(); 3725 if (!(this.timing instanceof Age)) 3726 throw new FHIRException( 3727 "Type mismatch: the type Age was expected, but " + this.timing.getClass().getName() + " was encountered"); 3728 return (Age) this.timing; 3729 } 3730 3731 public boolean hasTimingAge() { 3732 return this.timing instanceof Age; 3733 } 3734 3735 /** 3736 * @return {@link #timing} (An optional value describing when the action should 3737 * be performed.) 3738 */ 3739 public Period getTimingPeriod() throws FHIRException { 3740 if (this.timing == null) 3741 this.timing = new Period(); 3742 if (!(this.timing instanceof Period)) 3743 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.timing.getClass().getName() 3744 + " was encountered"); 3745 return (Period) this.timing; 3746 } 3747 3748 public boolean hasTimingPeriod() { 3749 return this.timing instanceof Period; 3750 } 3751 3752 /** 3753 * @return {@link #timing} (An optional value describing when the action should 3754 * be performed.) 3755 */ 3756 public Duration getTimingDuration() throws FHIRException { 3757 if (this.timing == null) 3758 this.timing = new Duration(); 3759 if (!(this.timing instanceof Duration)) 3760 throw new FHIRException("Type mismatch: the type Duration was expected, but " + this.timing.getClass().getName() 3761 + " was encountered"); 3762 return (Duration) this.timing; 3763 } 3764 3765 public boolean hasTimingDuration() { 3766 return this.timing instanceof Duration; 3767 } 3768 3769 /** 3770 * @return {@link #timing} (An optional value describing when the action should 3771 * be performed.) 3772 */ 3773 public Range getTimingRange() throws FHIRException { 3774 if (this.timing == null) 3775 this.timing = new Range(); 3776 if (!(this.timing instanceof Range)) 3777 throw new FHIRException( 3778 "Type mismatch: the type Range was expected, but " + this.timing.getClass().getName() + " was encountered"); 3779 return (Range) this.timing; 3780 } 3781 3782 public boolean hasTimingRange() { 3783 return this.timing instanceof Range; 3784 } 3785 3786 /** 3787 * @return {@link #timing} (An optional value describing when the action should 3788 * be performed.) 3789 */ 3790 public Timing getTimingTiming() throws FHIRException { 3791 if (this.timing == null) 3792 this.timing = new Timing(); 3793 if (!(this.timing instanceof Timing)) 3794 throw new FHIRException("Type mismatch: the type Timing was expected, but " + this.timing.getClass().getName() 3795 + " was encountered"); 3796 return (Timing) this.timing; 3797 } 3798 3799 public boolean hasTimingTiming() { 3800 return this.timing instanceof Timing; 3801 } 3802 3803 public boolean hasTiming() { 3804 return this.timing != null && !this.timing.isEmpty(); 3805 } 3806 3807 /** 3808 * @param value {@link #timing} (An optional value describing when the action 3809 * should be performed.) 3810 */ 3811 public PlanDefinitionActionComponent setTiming(Type value) { 3812 if (value != null && !(value instanceof DateTimeType || value instanceof Age || value instanceof Period 3813 || value instanceof Duration || value instanceof Range || value instanceof Timing)) 3814 throw new Error("Not the right type for PlanDefinition.action.timing[x]: " + value.fhirType()); 3815 this.timing = value; 3816 return this; 3817 } 3818 3819 /** 3820 * @return {@link #participant} (Indicates who should participate in performing 3821 * the action described.) 3822 */ 3823 public List<PlanDefinitionActionParticipantComponent> getParticipant() { 3824 if (this.participant == null) 3825 this.participant = new ArrayList<PlanDefinitionActionParticipantComponent>(); 3826 return this.participant; 3827 } 3828 3829 /** 3830 * @return Returns a reference to <code>this</code> for easy method chaining 3831 */ 3832 public PlanDefinitionActionComponent setParticipant(List<PlanDefinitionActionParticipantComponent> theParticipant) { 3833 this.participant = theParticipant; 3834 return this; 3835 } 3836 3837 public boolean hasParticipant() { 3838 if (this.participant == null) 3839 return false; 3840 for (PlanDefinitionActionParticipantComponent item : this.participant) 3841 if (!item.isEmpty()) 3842 return true; 3843 return false; 3844 } 3845 3846 public PlanDefinitionActionParticipantComponent addParticipant() { // 3 3847 PlanDefinitionActionParticipantComponent t = new PlanDefinitionActionParticipantComponent(); 3848 if (this.participant == null) 3849 this.participant = new ArrayList<PlanDefinitionActionParticipantComponent>(); 3850 this.participant.add(t); 3851 return t; 3852 } 3853 3854 public PlanDefinitionActionComponent addParticipant(PlanDefinitionActionParticipantComponent t) { // 3 3855 if (t == null) 3856 return this; 3857 if (this.participant == null) 3858 this.participant = new ArrayList<PlanDefinitionActionParticipantComponent>(); 3859 this.participant.add(t); 3860 return this; 3861 } 3862 3863 /** 3864 * @return The first repetition of repeating field {@link #participant}, 3865 * creating it if it does not already exist 3866 */ 3867 public PlanDefinitionActionParticipantComponent getParticipantFirstRep() { 3868 if (getParticipant().isEmpty()) { 3869 addParticipant(); 3870 } 3871 return getParticipant().get(0); 3872 } 3873 3874 /** 3875 * @return {@link #type} (The type of action to perform (create, update, 3876 * remove).) 3877 */ 3878 public CodeableConcept getType() { 3879 if (this.type == null) 3880 if (Configuration.errorOnAutoCreate()) 3881 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.type"); 3882 else if (Configuration.doAutoCreate()) 3883 this.type = new CodeableConcept(); // cc 3884 return this.type; 3885 } 3886 3887 public boolean hasType() { 3888 return this.type != null && !this.type.isEmpty(); 3889 } 3890 3891 /** 3892 * @param value {@link #type} (The type of action to perform (create, update, 3893 * remove).) 3894 */ 3895 public PlanDefinitionActionComponent setType(CodeableConcept value) { 3896 this.type = value; 3897 return this; 3898 } 3899 3900 /** 3901 * @return {@link #groupingBehavior} (Defines the grouping behavior for the 3902 * action and its children.). This is the underlying object with id, 3903 * value and extensions. The accessor "getGroupingBehavior" gives direct 3904 * access to the value 3905 */ 3906 public Enumeration<ActionGroupingBehavior> getGroupingBehaviorElement() { 3907 if (this.groupingBehavior == null) 3908 if (Configuration.errorOnAutoCreate()) 3909 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.groupingBehavior"); 3910 else if (Configuration.doAutoCreate()) 3911 this.groupingBehavior = new Enumeration<ActionGroupingBehavior>(new ActionGroupingBehaviorEnumFactory()); // bb 3912 return this.groupingBehavior; 3913 } 3914 3915 public boolean hasGroupingBehaviorElement() { 3916 return this.groupingBehavior != null && !this.groupingBehavior.isEmpty(); 3917 } 3918 3919 public boolean hasGroupingBehavior() { 3920 return this.groupingBehavior != null && !this.groupingBehavior.isEmpty(); 3921 } 3922 3923 /** 3924 * @param value {@link #groupingBehavior} (Defines the grouping behavior for the 3925 * action and its children.). This is the underlying object with 3926 * id, value and extensions. The accessor "getGroupingBehavior" 3927 * gives direct access to the value 3928 */ 3929 public PlanDefinitionActionComponent setGroupingBehaviorElement(Enumeration<ActionGroupingBehavior> value) { 3930 this.groupingBehavior = value; 3931 return this; 3932 } 3933 3934 /** 3935 * @return Defines the grouping behavior for the action and its children. 3936 */ 3937 public ActionGroupingBehavior getGroupingBehavior() { 3938 return this.groupingBehavior == null ? null : this.groupingBehavior.getValue(); 3939 } 3940 3941 /** 3942 * @param value Defines the grouping behavior for the action and its children. 3943 */ 3944 public PlanDefinitionActionComponent setGroupingBehavior(ActionGroupingBehavior value) { 3945 if (value == null) 3946 this.groupingBehavior = null; 3947 else { 3948 if (this.groupingBehavior == null) 3949 this.groupingBehavior = new Enumeration<ActionGroupingBehavior>(new ActionGroupingBehaviorEnumFactory()); 3950 this.groupingBehavior.setValue(value); 3951 } 3952 return this; 3953 } 3954 3955 /** 3956 * @return {@link #selectionBehavior} (Defines the selection behavior for the 3957 * action and its children.). This is the underlying object with id, 3958 * value and extensions. The accessor "getSelectionBehavior" gives 3959 * direct access to the value 3960 */ 3961 public Enumeration<ActionSelectionBehavior> getSelectionBehaviorElement() { 3962 if (this.selectionBehavior == null) 3963 if (Configuration.errorOnAutoCreate()) 3964 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.selectionBehavior"); 3965 else if (Configuration.doAutoCreate()) 3966 this.selectionBehavior = new Enumeration<ActionSelectionBehavior>(new ActionSelectionBehaviorEnumFactory()); // bb 3967 return this.selectionBehavior; 3968 } 3969 3970 public boolean hasSelectionBehaviorElement() { 3971 return this.selectionBehavior != null && !this.selectionBehavior.isEmpty(); 3972 } 3973 3974 public boolean hasSelectionBehavior() { 3975 return this.selectionBehavior != null && !this.selectionBehavior.isEmpty(); 3976 } 3977 3978 /** 3979 * @param value {@link #selectionBehavior} (Defines the selection behavior for 3980 * the action and its children.). This is the underlying object 3981 * with id, value and extensions. The accessor 3982 * "getSelectionBehavior" gives direct access to the value 3983 */ 3984 public PlanDefinitionActionComponent setSelectionBehaviorElement(Enumeration<ActionSelectionBehavior> value) { 3985 this.selectionBehavior = value; 3986 return this; 3987 } 3988 3989 /** 3990 * @return Defines the selection behavior for the action and its children. 3991 */ 3992 public ActionSelectionBehavior getSelectionBehavior() { 3993 return this.selectionBehavior == null ? null : this.selectionBehavior.getValue(); 3994 } 3995 3996 /** 3997 * @param value Defines the selection behavior for the action and its children. 3998 */ 3999 public PlanDefinitionActionComponent setSelectionBehavior(ActionSelectionBehavior value) { 4000 if (value == null) 4001 this.selectionBehavior = null; 4002 else { 4003 if (this.selectionBehavior == null) 4004 this.selectionBehavior = new Enumeration<ActionSelectionBehavior>(new ActionSelectionBehaviorEnumFactory()); 4005 this.selectionBehavior.setValue(value); 4006 } 4007 return this; 4008 } 4009 4010 /** 4011 * @return {@link #requiredBehavior} (Defines the required behavior for the 4012 * action.). This is the underlying object with id, value and 4013 * extensions. The accessor "getRequiredBehavior" gives direct access to 4014 * the value 4015 */ 4016 public Enumeration<ActionRequiredBehavior> getRequiredBehaviorElement() { 4017 if (this.requiredBehavior == null) 4018 if (Configuration.errorOnAutoCreate()) 4019 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.requiredBehavior"); 4020 else if (Configuration.doAutoCreate()) 4021 this.requiredBehavior = new Enumeration<ActionRequiredBehavior>(new ActionRequiredBehaviorEnumFactory()); // bb 4022 return this.requiredBehavior; 4023 } 4024 4025 public boolean hasRequiredBehaviorElement() { 4026 return this.requiredBehavior != null && !this.requiredBehavior.isEmpty(); 4027 } 4028 4029 public boolean hasRequiredBehavior() { 4030 return this.requiredBehavior != null && !this.requiredBehavior.isEmpty(); 4031 } 4032 4033 /** 4034 * @param value {@link #requiredBehavior} (Defines the required behavior for the 4035 * action.). This is the underlying object with id, value and 4036 * extensions. The accessor "getRequiredBehavior" gives direct 4037 * access to the value 4038 */ 4039 public PlanDefinitionActionComponent setRequiredBehaviorElement(Enumeration<ActionRequiredBehavior> value) { 4040 this.requiredBehavior = value; 4041 return this; 4042 } 4043 4044 /** 4045 * @return Defines the required behavior for the action. 4046 */ 4047 public ActionRequiredBehavior getRequiredBehavior() { 4048 return this.requiredBehavior == null ? null : this.requiredBehavior.getValue(); 4049 } 4050 4051 /** 4052 * @param value Defines the required behavior for the action. 4053 */ 4054 public PlanDefinitionActionComponent setRequiredBehavior(ActionRequiredBehavior value) { 4055 if (value == null) 4056 this.requiredBehavior = null; 4057 else { 4058 if (this.requiredBehavior == null) 4059 this.requiredBehavior = new Enumeration<ActionRequiredBehavior>(new ActionRequiredBehaviorEnumFactory()); 4060 this.requiredBehavior.setValue(value); 4061 } 4062 return this; 4063 } 4064 4065 /** 4066 * @return {@link #precheckBehavior} (Defines whether the action should usually 4067 * be preselected.). This is the underlying object with id, value and 4068 * extensions. The accessor "getPrecheckBehavior" gives direct access to 4069 * the value 4070 */ 4071 public Enumeration<ActionPrecheckBehavior> getPrecheckBehaviorElement() { 4072 if (this.precheckBehavior == null) 4073 if (Configuration.errorOnAutoCreate()) 4074 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.precheckBehavior"); 4075 else if (Configuration.doAutoCreate()) 4076 this.precheckBehavior = new Enumeration<ActionPrecheckBehavior>(new ActionPrecheckBehaviorEnumFactory()); // bb 4077 return this.precheckBehavior; 4078 } 4079 4080 public boolean hasPrecheckBehaviorElement() { 4081 return this.precheckBehavior != null && !this.precheckBehavior.isEmpty(); 4082 } 4083 4084 public boolean hasPrecheckBehavior() { 4085 return this.precheckBehavior != null && !this.precheckBehavior.isEmpty(); 4086 } 4087 4088 /** 4089 * @param value {@link #precheckBehavior} (Defines whether the action should 4090 * usually be preselected.). This is the underlying object with id, 4091 * value and extensions. The accessor "getPrecheckBehavior" gives 4092 * direct access to the value 4093 */ 4094 public PlanDefinitionActionComponent setPrecheckBehaviorElement(Enumeration<ActionPrecheckBehavior> value) { 4095 this.precheckBehavior = value; 4096 return this; 4097 } 4098 4099 /** 4100 * @return Defines whether the action should usually be preselected. 4101 */ 4102 public ActionPrecheckBehavior getPrecheckBehavior() { 4103 return this.precheckBehavior == null ? null : this.precheckBehavior.getValue(); 4104 } 4105 4106 /** 4107 * @param value Defines whether the action should usually be preselected. 4108 */ 4109 public PlanDefinitionActionComponent setPrecheckBehavior(ActionPrecheckBehavior value) { 4110 if (value == null) 4111 this.precheckBehavior = null; 4112 else { 4113 if (this.precheckBehavior == null) 4114 this.precheckBehavior = new Enumeration<ActionPrecheckBehavior>(new ActionPrecheckBehaviorEnumFactory()); 4115 this.precheckBehavior.setValue(value); 4116 } 4117 return this; 4118 } 4119 4120 /** 4121 * @return {@link #cardinalityBehavior} (Defines whether the action can be 4122 * selected multiple times.). This is the underlying object with id, 4123 * value and extensions. The accessor "getCardinalityBehavior" gives 4124 * direct access to the value 4125 */ 4126 public Enumeration<ActionCardinalityBehavior> getCardinalityBehaviorElement() { 4127 if (this.cardinalityBehavior == null) 4128 if (Configuration.errorOnAutoCreate()) 4129 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.cardinalityBehavior"); 4130 else if (Configuration.doAutoCreate()) 4131 this.cardinalityBehavior = new Enumeration<ActionCardinalityBehavior>( 4132 new ActionCardinalityBehaviorEnumFactory()); // bb 4133 return this.cardinalityBehavior; 4134 } 4135 4136 public boolean hasCardinalityBehaviorElement() { 4137 return this.cardinalityBehavior != null && !this.cardinalityBehavior.isEmpty(); 4138 } 4139 4140 public boolean hasCardinalityBehavior() { 4141 return this.cardinalityBehavior != null && !this.cardinalityBehavior.isEmpty(); 4142 } 4143 4144 /** 4145 * @param value {@link #cardinalityBehavior} (Defines whether the action can be 4146 * selected multiple times.). This is the underlying object with 4147 * id, value and extensions. The accessor "getCardinalityBehavior" 4148 * gives direct access to the value 4149 */ 4150 public PlanDefinitionActionComponent setCardinalityBehaviorElement(Enumeration<ActionCardinalityBehavior> value) { 4151 this.cardinalityBehavior = value; 4152 return this; 4153 } 4154 4155 /** 4156 * @return Defines whether the action can be selected multiple times. 4157 */ 4158 public ActionCardinalityBehavior getCardinalityBehavior() { 4159 return this.cardinalityBehavior == null ? null : this.cardinalityBehavior.getValue(); 4160 } 4161 4162 /** 4163 * @param value Defines whether the action can be selected multiple times. 4164 */ 4165 public PlanDefinitionActionComponent setCardinalityBehavior(ActionCardinalityBehavior value) { 4166 if (value == null) 4167 this.cardinalityBehavior = null; 4168 else { 4169 if (this.cardinalityBehavior == null) 4170 this.cardinalityBehavior = new Enumeration<ActionCardinalityBehavior>( 4171 new ActionCardinalityBehaviorEnumFactory()); 4172 this.cardinalityBehavior.setValue(value); 4173 } 4174 return this; 4175 } 4176 4177 /** 4178 * @return {@link #definition} (A reference to an ActivityDefinition that 4179 * describes the action to be taken in detail, or a PlanDefinition that 4180 * describes a series of actions to be taken.) 4181 */ 4182 public Type getDefinition() { 4183 return this.definition; 4184 } 4185 4186 /** 4187 * @return {@link #definition} (A reference to an ActivityDefinition that 4188 * describes the action to be taken in detail, or a PlanDefinition that 4189 * describes a series of actions to be taken.) 4190 */ 4191 public CanonicalType getDefinitionCanonicalType() throws FHIRException { 4192 if (this.definition == null) 4193 this.definition = new CanonicalType(); 4194 if (!(this.definition instanceof CanonicalType)) 4195 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but " 4196 + this.definition.getClass().getName() + " was encountered"); 4197 return (CanonicalType) this.definition; 4198 } 4199 4200 public boolean hasDefinitionCanonicalType() { 4201 return this.definition instanceof CanonicalType; 4202 } 4203 4204 /** 4205 * @return {@link #definition} (A reference to an ActivityDefinition that 4206 * describes the action to be taken in detail, or a PlanDefinition that 4207 * describes a series of actions to be taken.) 4208 */ 4209 public UriType getDefinitionUriType() throws FHIRException { 4210 if (this.definition == null) 4211 this.definition = new UriType(); 4212 if (!(this.definition instanceof UriType)) 4213 throw new FHIRException("Type mismatch: the type UriType was expected, but " 4214 + this.definition.getClass().getName() + " was encountered"); 4215 return (UriType) this.definition; 4216 } 4217 4218 public boolean hasDefinitionUriType() { 4219 return this.definition instanceof UriType; 4220 } 4221 4222 public boolean hasDefinition() { 4223 return this.definition != null && !this.definition.isEmpty(); 4224 } 4225 4226 /** 4227 * @param value {@link #definition} (A reference to an ActivityDefinition that 4228 * describes the action to be taken in detail, or a PlanDefinition 4229 * that describes a series of actions to be taken.) 4230 */ 4231 public PlanDefinitionActionComponent setDefinition(Type value) { 4232 if (value != null && !(value instanceof CanonicalType || value instanceof UriType)) 4233 throw new Error("Not the right type for PlanDefinition.action.definition[x]: " + value.fhirType()); 4234 this.definition = value; 4235 return this; 4236 } 4237 4238 /** 4239 * @return {@link #transform} (A reference to a StructureMap resource that 4240 * defines a transform that can be executed to produce the intent 4241 * resource using the ActivityDefinition instance as the input.). This 4242 * is the underlying object with id, value and extensions. The accessor 4243 * "getTransform" gives direct access to the value 4244 */ 4245 public CanonicalType getTransformElement() { 4246 if (this.transform == null) 4247 if (Configuration.errorOnAutoCreate()) 4248 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.transform"); 4249 else if (Configuration.doAutoCreate()) 4250 this.transform = new CanonicalType(); // bb 4251 return this.transform; 4252 } 4253 4254 public boolean hasTransformElement() { 4255 return this.transform != null && !this.transform.isEmpty(); 4256 } 4257 4258 public boolean hasTransform() { 4259 return this.transform != null && !this.transform.isEmpty(); 4260 } 4261 4262 /** 4263 * @param value {@link #transform} (A reference to a StructureMap resource that 4264 * defines a transform that can be executed to produce the intent 4265 * resource using the ActivityDefinition instance as the input.). 4266 * This is the underlying object with id, value and extensions. The 4267 * accessor "getTransform" gives direct access to the value 4268 */ 4269 public PlanDefinitionActionComponent setTransformElement(CanonicalType value) { 4270 this.transform = value; 4271 return this; 4272 } 4273 4274 /** 4275 * @return A reference to a StructureMap resource that defines a transform that 4276 * can be executed to produce the intent resource using the 4277 * ActivityDefinition instance as the input. 4278 */ 4279 public String getTransform() { 4280 return this.transform == null ? null : this.transform.getValue(); 4281 } 4282 4283 /** 4284 * @param value A reference to a StructureMap resource that defines a transform 4285 * that can be executed to produce the intent resource using the 4286 * ActivityDefinition instance as the input. 4287 */ 4288 public PlanDefinitionActionComponent setTransform(String value) { 4289 if (Utilities.noString(value)) 4290 this.transform = null; 4291 else { 4292 if (this.transform == null) 4293 this.transform = new CanonicalType(); 4294 this.transform.setValue(value); 4295 } 4296 return this; 4297 } 4298 4299 /** 4300 * @return {@link #dynamicValue} (Customizations that should be applied to the 4301 * statically defined resource. For example, if the dosage of a 4302 * medication must be computed based on the patient's weight, a 4303 * customization would be used to specify an expression that calculated 4304 * the weight, and the path on the resource that would contain the 4305 * result.) 4306 */ 4307 public List<PlanDefinitionActionDynamicValueComponent> getDynamicValue() { 4308 if (this.dynamicValue == null) 4309 this.dynamicValue = new ArrayList<PlanDefinitionActionDynamicValueComponent>(); 4310 return this.dynamicValue; 4311 } 4312 4313 /** 4314 * @return Returns a reference to <code>this</code> for easy method chaining 4315 */ 4316 public PlanDefinitionActionComponent setDynamicValue( 4317 List<PlanDefinitionActionDynamicValueComponent> theDynamicValue) { 4318 this.dynamicValue = theDynamicValue; 4319 return this; 4320 } 4321 4322 public boolean hasDynamicValue() { 4323 if (this.dynamicValue == null) 4324 return false; 4325 for (PlanDefinitionActionDynamicValueComponent item : this.dynamicValue) 4326 if (!item.isEmpty()) 4327 return true; 4328 return false; 4329 } 4330 4331 public PlanDefinitionActionDynamicValueComponent addDynamicValue() { // 3 4332 PlanDefinitionActionDynamicValueComponent t = new PlanDefinitionActionDynamicValueComponent(); 4333 if (this.dynamicValue == null) 4334 this.dynamicValue = new ArrayList<PlanDefinitionActionDynamicValueComponent>(); 4335 this.dynamicValue.add(t); 4336 return t; 4337 } 4338 4339 public PlanDefinitionActionComponent addDynamicValue(PlanDefinitionActionDynamicValueComponent t) { // 3 4340 if (t == null) 4341 return this; 4342 if (this.dynamicValue == null) 4343 this.dynamicValue = new ArrayList<PlanDefinitionActionDynamicValueComponent>(); 4344 this.dynamicValue.add(t); 4345 return this; 4346 } 4347 4348 /** 4349 * @return The first repetition of repeating field {@link #dynamicValue}, 4350 * creating it if it does not already exist 4351 */ 4352 public PlanDefinitionActionDynamicValueComponent getDynamicValueFirstRep() { 4353 if (getDynamicValue().isEmpty()) { 4354 addDynamicValue(); 4355 } 4356 return getDynamicValue().get(0); 4357 } 4358 4359 /** 4360 * @return {@link #action} (Sub actions that are contained within the action. 4361 * The behavior of this action determines the functionality of the 4362 * sub-actions. For example, a selection behavior of at-most-one 4363 * indicates that of the sub-actions, at most one may be chosen as part 4364 * of realizing the action definition.) 4365 */ 4366 public List<PlanDefinitionActionComponent> getAction() { 4367 if (this.action == null) 4368 this.action = new ArrayList<PlanDefinitionActionComponent>(); 4369 return this.action; 4370 } 4371 4372 /** 4373 * @return Returns a reference to <code>this</code> for easy method chaining 4374 */ 4375 public PlanDefinitionActionComponent setAction(List<PlanDefinitionActionComponent> theAction) { 4376 this.action = theAction; 4377 return this; 4378 } 4379 4380 public boolean hasAction() { 4381 if (this.action == null) 4382 return false; 4383 for (PlanDefinitionActionComponent item : this.action) 4384 if (!item.isEmpty()) 4385 return true; 4386 return false; 4387 } 4388 4389 public PlanDefinitionActionComponent addAction() { // 3 4390 PlanDefinitionActionComponent t = new PlanDefinitionActionComponent(); 4391 if (this.action == null) 4392 this.action = new ArrayList<PlanDefinitionActionComponent>(); 4393 this.action.add(t); 4394 return t; 4395 } 4396 4397 public PlanDefinitionActionComponent addAction(PlanDefinitionActionComponent t) { // 3 4398 if (t == null) 4399 return this; 4400 if (this.action == null) 4401 this.action = new ArrayList<PlanDefinitionActionComponent>(); 4402 this.action.add(t); 4403 return this; 4404 } 4405 4406 /** 4407 * @return The first repetition of repeating field {@link #action}, creating it 4408 * if it does not already exist 4409 */ 4410 public PlanDefinitionActionComponent getActionFirstRep() { 4411 if (getAction().isEmpty()) { 4412 addAction(); 4413 } 4414 return getAction().get(0); 4415 } 4416 4417 protected void listChildren(List<Property> children) { 4418 super.listChildren(children); 4419 children.add(new Property("prefix", "string", "A user-visible prefix for the action.", 0, 1, prefix)); 4420 children.add(new Property("title", "string", "The title of the action displayed to a user.", 0, 1, title)); 4421 children.add(new Property("description", "string", 4422 "A brief description of the action used to provide a summary to display to the user.", 0, 1, description)); 4423 children.add(new Property("textEquivalent", "string", 4424 "A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that might not be capable of interpreting it dynamically.", 4425 0, 1, textEquivalent)); 4426 children.add(new Property("priority", "code", 4427 "Indicates how quickly the action should be addressed with respect to other actions.", 0, 1, priority)); 4428 children.add(new Property("code", "CodeableConcept", 4429 "A code that provides meaning for the action or action group. For example, a section may have a LOINC code for the section of a documentation template.", 4430 0, java.lang.Integer.MAX_VALUE, code)); 4431 children.add(new Property("reason", "CodeableConcept", 4432 "A description of why this action is necessary or appropriate.", 0, java.lang.Integer.MAX_VALUE, reason)); 4433 children.add(new Property("documentation", "RelatedArtifact", 4434 "Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources.", 4435 0, java.lang.Integer.MAX_VALUE, documentation)); 4436 children.add(new Property("goalId", "id", 4437 "Identifies goals that this action supports. The reference must be to a goal element defined within this plan definition.", 4438 0, java.lang.Integer.MAX_VALUE, goalId)); 4439 children.add(new Property("subject[x]", "CodeableConcept|Reference(Group)", 4440 "A code or group definition that describes the intended subject of the action and its children, if any.", 0, 4441 1, subject)); 4442 children.add(new Property("trigger", "TriggerDefinition", "A description of when the action should be triggered.", 4443 0, java.lang.Integer.MAX_VALUE, trigger)); 4444 children.add(new Property("condition", "", 4445 "An expression that describes applicability criteria or start/stop conditions for the action.", 0, 4446 java.lang.Integer.MAX_VALUE, condition)); 4447 children.add(new Property("input", "DataRequirement", "Defines input data requirements for the action.", 0, 4448 java.lang.Integer.MAX_VALUE, input)); 4449 children.add(new Property("output", "DataRequirement", "Defines the outputs of the action, if any.", 0, 4450 java.lang.Integer.MAX_VALUE, output)); 4451 children.add(new Property("relatedAction", "", 4452 "A relationship to another action such as \"before\" or \"30-60 minutes after start of\".", 0, 4453 java.lang.Integer.MAX_VALUE, relatedAction)); 4454 children.add(new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 4455 "An optional value describing when the action should be performed.", 0, 1, timing)); 4456 children 4457 .add(new Property("participant", "", "Indicates who should participate in performing the action described.", 4458 0, java.lang.Integer.MAX_VALUE, participant)); 4459 children.add(new Property("type", "CodeableConcept", "The type of action to perform (create, update, remove).", 0, 4460 1, type)); 4461 children.add(new Property("groupingBehavior", "code", 4462 "Defines the grouping behavior for the action and its children.", 0, 1, groupingBehavior)); 4463 children.add(new Property("selectionBehavior", "code", 4464 "Defines the selection behavior for the action and its children.", 0, 1, selectionBehavior)); 4465 children.add(new Property("requiredBehavior", "code", "Defines the required behavior for the action.", 0, 1, 4466 requiredBehavior)); 4467 children.add(new Property("precheckBehavior", "code", "Defines whether the action should usually be preselected.", 4468 0, 1, precheckBehavior)); 4469 children.add(new Property("cardinalityBehavior", "code", 4470 "Defines whether the action can be selected multiple times.", 0, 1, cardinalityBehavior)); 4471 children.add(new Property("definition[x]", "canonical(ActivityDefinition|PlanDefinition|Questionnaire)|uri", 4472 "A reference to an ActivityDefinition that describes the action to be taken in detail, or a PlanDefinition that describes a series of actions to be taken.", 4473 0, 1, definition)); 4474 children.add(new Property("transform", "canonical(StructureMap)", 4475 "A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.", 4476 0, 1, transform)); 4477 children.add(new Property("dynamicValue", "", 4478 "Customizations that should be applied to the statically defined resource. For example, if the dosage of a medication must be computed based on the patient's weight, a customization would be used to specify an expression that calculated the weight, and the path on the resource that would contain the result.", 4479 0, java.lang.Integer.MAX_VALUE, dynamicValue)); 4480 children.add(new Property("action", "@PlanDefinition.action", 4481 "Sub actions that are contained within the action. The behavior of this action determines the functionality of the sub-actions. For example, a selection behavior of at-most-one indicates that of the sub-actions, at most one may be chosen as part of realizing the action definition.", 4482 0, java.lang.Integer.MAX_VALUE, action)); 4483 } 4484 4485 @Override 4486 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4487 switch (_hash) { 4488 case -980110702: 4489 /* prefix */ return new Property("prefix", "string", "A user-visible prefix for the action.", 0, 1, prefix); 4490 case 110371416: 4491 /* title */ return new Property("title", "string", "The title of the action displayed to a user.", 0, 1, title); 4492 case -1724546052: 4493 /* description */ return new Property("description", "string", 4494 "A brief description of the action used to provide a summary to display to the user.", 0, 1, description); 4495 case -900391049: 4496 /* textEquivalent */ return new Property("textEquivalent", "string", 4497 "A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that might not be capable of interpreting it dynamically.", 4498 0, 1, textEquivalent); 4499 case -1165461084: 4500 /* priority */ return new Property("priority", "code", 4501 "Indicates how quickly the action should be addressed with respect to other actions.", 0, 1, priority); 4502 case 3059181: 4503 /* code */ return new Property("code", "CodeableConcept", 4504 "A code that provides meaning for the action or action group. For example, a section may have a LOINC code for the section of a documentation template.", 4505 0, java.lang.Integer.MAX_VALUE, code); 4506 case -934964668: 4507 /* reason */ return new Property("reason", "CodeableConcept", 4508 "A description of why this action is necessary or appropriate.", 0, java.lang.Integer.MAX_VALUE, reason); 4509 case 1587405498: 4510 /* documentation */ return new Property("documentation", "RelatedArtifact", 4511 "Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources.", 4512 0, java.lang.Integer.MAX_VALUE, documentation); 4513 case -1240658034: 4514 /* goalId */ return new Property("goalId", "id", 4515 "Identifies goals that this action supports. The reference must be to a goal element defined within this plan definition.", 4516 0, java.lang.Integer.MAX_VALUE, goalId); 4517 case -573640748: 4518 /* subject[x] */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 4519 "A code or group definition that describes the intended subject of the action and its children, if any.", 0, 4520 1, subject); 4521 case -1867885268: 4522 /* subject */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 4523 "A code or group definition that describes the intended subject of the action and its children, if any.", 0, 4524 1, subject); 4525 case -1257122603: 4526 /* subjectCodeableConcept */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 4527 "A code or group definition that describes the intended subject of the action and its children, if any.", 0, 4528 1, subject); 4529 case 772938623: 4530 /* subjectReference */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 4531 "A code or group definition that describes the intended subject of the action and its children, if any.", 0, 4532 1, subject); 4533 case -1059891784: 4534 /* trigger */ return new Property("trigger", "TriggerDefinition", 4535 "A description of when the action should be triggered.", 0, java.lang.Integer.MAX_VALUE, trigger); 4536 case -861311717: 4537 /* condition */ return new Property("condition", "", 4538 "An expression that describes applicability criteria or start/stop conditions for the action.", 0, 4539 java.lang.Integer.MAX_VALUE, condition); 4540 case 100358090: 4541 /* input */ return new Property("input", "DataRequirement", "Defines input data requirements for the action.", 4542 0, java.lang.Integer.MAX_VALUE, input); 4543 case -1005512447: 4544 /* output */ return new Property("output", "DataRequirement", "Defines the outputs of the action, if any.", 0, 4545 java.lang.Integer.MAX_VALUE, output); 4546 case -384107967: 4547 /* relatedAction */ return new Property("relatedAction", "", 4548 "A relationship to another action such as \"before\" or \"30-60 minutes after start of\".", 0, 4549 java.lang.Integer.MAX_VALUE, relatedAction); 4550 case 164632566: 4551 /* timing[x] */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 4552 "An optional value describing when the action should be performed.", 0, 1, timing); 4553 case -873664438: 4554 /* timing */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 4555 "An optional value describing when the action should be performed.", 0, 1, timing); 4556 case -1837458939: 4557 /* timingDateTime */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 4558 "An optional value describing when the action should be performed.", 0, 1, timing); 4559 case 164607061: 4560 /* timingAge */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 4561 "An optional value describing when the action should be performed.", 0, 1, timing); 4562 case -615615829: 4563 /* timingPeriod */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 4564 "An optional value describing when the action should be performed.", 0, 1, timing); 4565 case -1327253506: 4566 /* timingDuration */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 4567 "An optional value describing when the action should be performed.", 0, 1, timing); 4568 case -710871277: 4569 /* timingRange */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 4570 "An optional value describing when the action should be performed.", 0, 1, timing); 4571 case -497554124: 4572 /* timingTiming */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 4573 "An optional value describing when the action should be performed.", 0, 1, timing); 4574 case 767422259: 4575 /* participant */ return new Property("participant", "", 4576 "Indicates who should participate in performing the action described.", 0, java.lang.Integer.MAX_VALUE, 4577 participant); 4578 case 3575610: 4579 /* type */ return new Property("type", "CodeableConcept", 4580 "The type of action to perform (create, update, remove).", 0, 1, type); 4581 case 586678389: 4582 /* groupingBehavior */ return new Property("groupingBehavior", "code", 4583 "Defines the grouping behavior for the action and its children.", 0, 1, groupingBehavior); 4584 case 168639486: 4585 /* selectionBehavior */ return new Property("selectionBehavior", "code", 4586 "Defines the selection behavior for the action and its children.", 0, 1, selectionBehavior); 4587 case -1163906287: 4588 /* requiredBehavior */ return new Property("requiredBehavior", "code", 4589 "Defines the required behavior for the action.", 0, 1, requiredBehavior); 4590 case -1174249033: 4591 /* precheckBehavior */ return new Property("precheckBehavior", "code", 4592 "Defines whether the action should usually be preselected.", 0, 1, precheckBehavior); 4593 case -922577408: 4594 /* cardinalityBehavior */ return new Property("cardinalityBehavior", "code", 4595 "Defines whether the action can be selected multiple times.", 0, 1, cardinalityBehavior); 4596 case -1139422643: 4597 /* definition[x] */ return new Property("definition[x]", 4598 "canonical(ActivityDefinition|PlanDefinition|Questionnaire)|uri", 4599 "A reference to an ActivityDefinition that describes the action to be taken in detail, or a PlanDefinition that describes a series of actions to be taken.", 4600 0, 1, definition); 4601 case -1014418093: 4602 /* definition */ return new Property("definition[x]", 4603 "canonical(ActivityDefinition|PlanDefinition|Questionnaire)|uri", 4604 "A reference to an ActivityDefinition that describes the action to be taken in detail, or a PlanDefinition that describes a series of actions to be taken.", 4605 0, 1, definition); 4606 case 933485793: 4607 /* definitionCanonical */ return new Property("definition[x]", 4608 "canonical(ActivityDefinition|PlanDefinition|Questionnaire)|uri", 4609 "A reference to an ActivityDefinition that describes the action to be taken in detail, or a PlanDefinition that describes a series of actions to be taken.", 4610 0, 1, definition); 4611 case -1139428583: 4612 /* definitionUri */ return new Property("definition[x]", 4613 "canonical(ActivityDefinition|PlanDefinition|Questionnaire)|uri", 4614 "A reference to an ActivityDefinition that describes the action to be taken in detail, or a PlanDefinition that describes a series of actions to be taken.", 4615 0, 1, definition); 4616 case 1052666732: 4617 /* transform */ return new Property("transform", "canonical(StructureMap)", 4618 "A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.", 4619 0, 1, transform); 4620 case 572625010: 4621 /* dynamicValue */ return new Property("dynamicValue", "", 4622 "Customizations that should be applied to the statically defined resource. For example, if the dosage of a medication must be computed based on the patient's weight, a customization would be used to specify an expression that calculated the weight, and the path on the resource that would contain the result.", 4623 0, java.lang.Integer.MAX_VALUE, dynamicValue); 4624 case -1422950858: 4625 /* action */ return new Property("action", "@PlanDefinition.action", 4626 "Sub actions that are contained within the action. The behavior of this action determines the functionality of the sub-actions. For example, a selection behavior of at-most-one indicates that of the sub-actions, at most one may be chosen as part of realizing the action definition.", 4627 0, java.lang.Integer.MAX_VALUE, action); 4628 default: 4629 return super.getNamedProperty(_hash, _name, _checkValid); 4630 } 4631 4632 } 4633 4634 @Override 4635 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4636 switch (hash) { 4637 case -980110702: 4638 /* prefix */ return this.prefix == null ? new Base[0] : new Base[] { this.prefix }; // StringType 4639 case 110371416: 4640 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 4641 case -1724546052: 4642 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 4643 case -900391049: 4644 /* textEquivalent */ return this.textEquivalent == null ? new Base[0] : new Base[] { this.textEquivalent }; // StringType 4645 case -1165461084: 4646 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // Enumeration<RequestPriority> 4647 case 3059181: 4648 /* code */ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 4649 case -934964668: 4650 /* reason */ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableConcept 4651 case 1587405498: 4652 /* documentation */ return this.documentation == null ? new Base[0] 4653 : this.documentation.toArray(new Base[this.documentation.size()]); // RelatedArtifact 4654 case -1240658034: 4655 /* goalId */ return this.goalId == null ? new Base[0] : this.goalId.toArray(new Base[this.goalId.size()]); // IdType 4656 case -1867885268: 4657 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Type 4658 case -1059891784: 4659 /* trigger */ return this.trigger == null ? new Base[0] : this.trigger.toArray(new Base[this.trigger.size()]); // TriggerDefinition 4660 case -861311717: 4661 /* condition */ return this.condition == null ? new Base[0] 4662 : this.condition.toArray(new Base[this.condition.size()]); // PlanDefinitionActionConditionComponent 4663 case 100358090: 4664 /* input */ return this.input == null ? new Base[0] : this.input.toArray(new Base[this.input.size()]); // DataRequirement 4665 case -1005512447: 4666 /* output */ return this.output == null ? new Base[0] : this.output.toArray(new Base[this.output.size()]); // DataRequirement 4667 case -384107967: 4668 /* relatedAction */ return this.relatedAction == null ? new Base[0] 4669 : this.relatedAction.toArray(new Base[this.relatedAction.size()]); // PlanDefinitionActionRelatedActionComponent 4670 case -873664438: 4671 /* timing */ return this.timing == null ? new Base[0] : new Base[] { this.timing }; // Type 4672 case 767422259: 4673 /* participant */ return this.participant == null ? new Base[0] 4674 : this.participant.toArray(new Base[this.participant.size()]); // PlanDefinitionActionParticipantComponent 4675 case 3575610: 4676 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 4677 case 586678389: 4678 /* groupingBehavior */ return this.groupingBehavior == null ? new Base[0] 4679 : new Base[] { this.groupingBehavior }; // Enumeration<ActionGroupingBehavior> 4680 case 168639486: 4681 /* selectionBehavior */ return this.selectionBehavior == null ? new Base[0] 4682 : new Base[] { this.selectionBehavior }; // Enumeration<ActionSelectionBehavior> 4683 case -1163906287: 4684 /* requiredBehavior */ return this.requiredBehavior == null ? new Base[0] 4685 : new Base[] { this.requiredBehavior }; // Enumeration<ActionRequiredBehavior> 4686 case -1174249033: 4687 /* precheckBehavior */ return this.precheckBehavior == null ? new Base[0] 4688 : new Base[] { this.precheckBehavior }; // Enumeration<ActionPrecheckBehavior> 4689 case -922577408: 4690 /* cardinalityBehavior */ return this.cardinalityBehavior == null ? new Base[0] 4691 : new Base[] { this.cardinalityBehavior }; // Enumeration<ActionCardinalityBehavior> 4692 case -1014418093: 4693 /* definition */ return this.definition == null ? new Base[0] : new Base[] { this.definition }; // Type 4694 case 1052666732: 4695 /* transform */ return this.transform == null ? new Base[0] : new Base[] { this.transform }; // CanonicalType 4696 case 572625010: 4697 /* dynamicValue */ return this.dynamicValue == null ? new Base[0] 4698 : this.dynamicValue.toArray(new Base[this.dynamicValue.size()]); // PlanDefinitionActionDynamicValueComponent 4699 case -1422950858: 4700 /* action */ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // PlanDefinitionActionComponent 4701 default: 4702 return super.getProperty(hash, name, checkValid); 4703 } 4704 4705 } 4706 4707 @Override 4708 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4709 switch (hash) { 4710 case -980110702: // prefix 4711 this.prefix = castToString(value); // StringType 4712 return value; 4713 case 110371416: // title 4714 this.title = castToString(value); // StringType 4715 return value; 4716 case -1724546052: // description 4717 this.description = castToString(value); // StringType 4718 return value; 4719 case -900391049: // textEquivalent 4720 this.textEquivalent = castToString(value); // StringType 4721 return value; 4722 case -1165461084: // priority 4723 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 4724 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 4725 return value; 4726 case 3059181: // code 4727 this.getCode().add(castToCodeableConcept(value)); // CodeableConcept 4728 return value; 4729 case -934964668: // reason 4730 this.getReason().add(castToCodeableConcept(value)); // CodeableConcept 4731 return value; 4732 case 1587405498: // documentation 4733 this.getDocumentation().add(castToRelatedArtifact(value)); // RelatedArtifact 4734 return value; 4735 case -1240658034: // goalId 4736 this.getGoalId().add(castToId(value)); // IdType 4737 return value; 4738 case -1867885268: // subject 4739 this.subject = castToType(value); // Type 4740 return value; 4741 case -1059891784: // trigger 4742 this.getTrigger().add(castToTriggerDefinition(value)); // TriggerDefinition 4743 return value; 4744 case -861311717: // condition 4745 this.getCondition().add((PlanDefinitionActionConditionComponent) value); // PlanDefinitionActionConditionComponent 4746 return value; 4747 case 100358090: // input 4748 this.getInput().add(castToDataRequirement(value)); // DataRequirement 4749 return value; 4750 case -1005512447: // output 4751 this.getOutput().add(castToDataRequirement(value)); // DataRequirement 4752 return value; 4753 case -384107967: // relatedAction 4754 this.getRelatedAction().add((PlanDefinitionActionRelatedActionComponent) value); // PlanDefinitionActionRelatedActionComponent 4755 return value; 4756 case -873664438: // timing 4757 this.timing = castToType(value); // Type 4758 return value; 4759 case 767422259: // participant 4760 this.getParticipant().add((PlanDefinitionActionParticipantComponent) value); // PlanDefinitionActionParticipantComponent 4761 return value; 4762 case 3575610: // type 4763 this.type = castToCodeableConcept(value); // CodeableConcept 4764 return value; 4765 case 586678389: // groupingBehavior 4766 value = new ActionGroupingBehaviorEnumFactory().fromType(castToCode(value)); 4767 this.groupingBehavior = (Enumeration) value; // Enumeration<ActionGroupingBehavior> 4768 return value; 4769 case 168639486: // selectionBehavior 4770 value = new ActionSelectionBehaviorEnumFactory().fromType(castToCode(value)); 4771 this.selectionBehavior = (Enumeration) value; // Enumeration<ActionSelectionBehavior> 4772 return value; 4773 case -1163906287: // requiredBehavior 4774 value = new ActionRequiredBehaviorEnumFactory().fromType(castToCode(value)); 4775 this.requiredBehavior = (Enumeration) value; // Enumeration<ActionRequiredBehavior> 4776 return value; 4777 case -1174249033: // precheckBehavior 4778 value = new ActionPrecheckBehaviorEnumFactory().fromType(castToCode(value)); 4779 this.precheckBehavior = (Enumeration) value; // Enumeration<ActionPrecheckBehavior> 4780 return value; 4781 case -922577408: // cardinalityBehavior 4782 value = new ActionCardinalityBehaviorEnumFactory().fromType(castToCode(value)); 4783 this.cardinalityBehavior = (Enumeration) value; // Enumeration<ActionCardinalityBehavior> 4784 return value; 4785 case -1014418093: // definition 4786 this.definition = castToType(value); // Type 4787 return value; 4788 case 1052666732: // transform 4789 this.transform = castToCanonical(value); // CanonicalType 4790 return value; 4791 case 572625010: // dynamicValue 4792 this.getDynamicValue().add((PlanDefinitionActionDynamicValueComponent) value); // PlanDefinitionActionDynamicValueComponent 4793 return value; 4794 case -1422950858: // action 4795 this.getAction().add((PlanDefinitionActionComponent) value); // PlanDefinitionActionComponent 4796 return value; 4797 default: 4798 return super.setProperty(hash, name, value); 4799 } 4800 4801 } 4802 4803 @Override 4804 public Base setProperty(String name, Base value) throws FHIRException { 4805 if (name.equals("prefix")) { 4806 this.prefix = castToString(value); // StringType 4807 } else if (name.equals("title")) { 4808 this.title = castToString(value); // StringType 4809 } else if (name.equals("description")) { 4810 this.description = castToString(value); // StringType 4811 } else if (name.equals("textEquivalent")) { 4812 this.textEquivalent = castToString(value); // StringType 4813 } else if (name.equals("priority")) { 4814 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 4815 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 4816 } else if (name.equals("code")) { 4817 this.getCode().add(castToCodeableConcept(value)); 4818 } else if (name.equals("reason")) { 4819 this.getReason().add(castToCodeableConcept(value)); 4820 } else if (name.equals("documentation")) { 4821 this.getDocumentation().add(castToRelatedArtifact(value)); 4822 } else if (name.equals("goalId")) { 4823 this.getGoalId().add(castToId(value)); 4824 } else if (name.equals("subject[x]")) { 4825 this.subject = castToType(value); // Type 4826 } else if (name.equals("trigger")) { 4827 this.getTrigger().add(castToTriggerDefinition(value)); 4828 } else if (name.equals("condition")) { 4829 this.getCondition().add((PlanDefinitionActionConditionComponent) value); 4830 } else if (name.equals("input")) { 4831 this.getInput().add(castToDataRequirement(value)); 4832 } else if (name.equals("output")) { 4833 this.getOutput().add(castToDataRequirement(value)); 4834 } else if (name.equals("relatedAction")) { 4835 this.getRelatedAction().add((PlanDefinitionActionRelatedActionComponent) value); 4836 } else if (name.equals("timing[x]")) { 4837 this.timing = castToType(value); // Type 4838 } else if (name.equals("participant")) { 4839 this.getParticipant().add((PlanDefinitionActionParticipantComponent) value); 4840 } else if (name.equals("type")) { 4841 this.type = castToCodeableConcept(value); // CodeableConcept 4842 } else if (name.equals("groupingBehavior")) { 4843 value = new ActionGroupingBehaviorEnumFactory().fromType(castToCode(value)); 4844 this.groupingBehavior = (Enumeration) value; // Enumeration<ActionGroupingBehavior> 4845 } else if (name.equals("selectionBehavior")) { 4846 value = new ActionSelectionBehaviorEnumFactory().fromType(castToCode(value)); 4847 this.selectionBehavior = (Enumeration) value; // Enumeration<ActionSelectionBehavior> 4848 } else if (name.equals("requiredBehavior")) { 4849 value = new ActionRequiredBehaviorEnumFactory().fromType(castToCode(value)); 4850 this.requiredBehavior = (Enumeration) value; // Enumeration<ActionRequiredBehavior> 4851 } else if (name.equals("precheckBehavior")) { 4852 value = new ActionPrecheckBehaviorEnumFactory().fromType(castToCode(value)); 4853 this.precheckBehavior = (Enumeration) value; // Enumeration<ActionPrecheckBehavior> 4854 } else if (name.equals("cardinalityBehavior")) { 4855 value = new ActionCardinalityBehaviorEnumFactory().fromType(castToCode(value)); 4856 this.cardinalityBehavior = (Enumeration) value; // Enumeration<ActionCardinalityBehavior> 4857 } else if (name.equals("definition[x]")) { 4858 this.definition = castToType(value); // Type 4859 } else if (name.equals("transform")) { 4860 this.transform = castToCanonical(value); // CanonicalType 4861 } else if (name.equals("dynamicValue")) { 4862 this.getDynamicValue().add((PlanDefinitionActionDynamicValueComponent) value); 4863 } else if (name.equals("action")) { 4864 this.getAction().add((PlanDefinitionActionComponent) value); 4865 } else 4866 return super.setProperty(name, value); 4867 return value; 4868 } 4869 4870 @Override 4871 public void removeChild(String name, Base value) throws FHIRException { 4872 if (name.equals("prefix")) { 4873 this.prefix = null; 4874 } else if (name.equals("title")) { 4875 this.title = null; 4876 } else if (name.equals("description")) { 4877 this.description = null; 4878 } else if (name.equals("textEquivalent")) { 4879 this.textEquivalent = null; 4880 } else if (name.equals("priority")) { 4881 this.priority = null; 4882 } else if (name.equals("code")) { 4883 this.getCode().remove(castToCodeableConcept(value)); 4884 } else if (name.equals("reason")) { 4885 this.getReason().remove(castToCodeableConcept(value)); 4886 } else if (name.equals("documentation")) { 4887 this.getDocumentation().remove(castToRelatedArtifact(value)); 4888 } else if (name.equals("goalId")) { 4889 this.getGoalId().remove(castToId(value)); 4890 } else if (name.equals("subject[x]")) { 4891 this.subject = null; 4892 } else if (name.equals("trigger")) { 4893 this.getTrigger().remove(castToTriggerDefinition(value)); 4894 } else if (name.equals("condition")) { 4895 this.getCondition().remove((PlanDefinitionActionConditionComponent) value); 4896 } else if (name.equals("input")) { 4897 this.getInput().remove(castToDataRequirement(value)); 4898 } else if (name.equals("output")) { 4899 this.getOutput().remove(castToDataRequirement(value)); 4900 } else if (name.equals("relatedAction")) { 4901 this.getRelatedAction().remove((PlanDefinitionActionRelatedActionComponent) value); 4902 } else if (name.equals("timing[x]")) { 4903 this.timing = null; 4904 } else if (name.equals("participant")) { 4905 this.getParticipant().remove((PlanDefinitionActionParticipantComponent) value); 4906 } else if (name.equals("type")) { 4907 this.type = null; 4908 } else if (name.equals("groupingBehavior")) { 4909 this.groupingBehavior = null; 4910 } else if (name.equals("selectionBehavior")) { 4911 this.selectionBehavior = null; 4912 } else if (name.equals("requiredBehavior")) { 4913 this.requiredBehavior = null; 4914 } else if (name.equals("precheckBehavior")) { 4915 this.precheckBehavior = null; 4916 } else if (name.equals("cardinalityBehavior")) { 4917 this.cardinalityBehavior = null; 4918 } else if (name.equals("definition[x]")) { 4919 this.definition = null; 4920 } else if (name.equals("transform")) { 4921 this.transform = null; 4922 } else if (name.equals("dynamicValue")) { 4923 this.getDynamicValue().remove((PlanDefinitionActionDynamicValueComponent) value); 4924 } else if (name.equals("action")) { 4925 this.getAction().remove((PlanDefinitionActionComponent) value); 4926 } else 4927 super.removeChild(name, value); 4928 4929 } 4930 4931 @Override 4932 public Base makeProperty(int hash, String name) throws FHIRException { 4933 switch (hash) { 4934 case -980110702: 4935 return getPrefixElement(); 4936 case 110371416: 4937 return getTitleElement(); 4938 case -1724546052: 4939 return getDescriptionElement(); 4940 case -900391049: 4941 return getTextEquivalentElement(); 4942 case -1165461084: 4943 return getPriorityElement(); 4944 case 3059181: 4945 return addCode(); 4946 case -934964668: 4947 return addReason(); 4948 case 1587405498: 4949 return addDocumentation(); 4950 case -1240658034: 4951 return addGoalIdElement(); 4952 case -573640748: 4953 return getSubject(); 4954 case -1867885268: 4955 return getSubject(); 4956 case -1059891784: 4957 return addTrigger(); 4958 case -861311717: 4959 return addCondition(); 4960 case 100358090: 4961 return addInput(); 4962 case -1005512447: 4963 return addOutput(); 4964 case -384107967: 4965 return addRelatedAction(); 4966 case 164632566: 4967 return getTiming(); 4968 case -873664438: 4969 return getTiming(); 4970 case 767422259: 4971 return addParticipant(); 4972 case 3575610: 4973 return getType(); 4974 case 586678389: 4975 return getGroupingBehaviorElement(); 4976 case 168639486: 4977 return getSelectionBehaviorElement(); 4978 case -1163906287: 4979 return getRequiredBehaviorElement(); 4980 case -1174249033: 4981 return getPrecheckBehaviorElement(); 4982 case -922577408: 4983 return getCardinalityBehaviorElement(); 4984 case -1139422643: 4985 return getDefinition(); 4986 case -1014418093: 4987 return getDefinition(); 4988 case 1052666732: 4989 return getTransformElement(); 4990 case 572625010: 4991 return addDynamicValue(); 4992 case -1422950858: 4993 return addAction(); 4994 default: 4995 return super.makeProperty(hash, name); 4996 } 4997 4998 } 4999 5000 @Override 5001 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5002 switch (hash) { 5003 case -980110702: 5004 /* prefix */ return new String[] { "string" }; 5005 case 110371416: 5006 /* title */ return new String[] { "string" }; 5007 case -1724546052: 5008 /* description */ return new String[] { "string" }; 5009 case -900391049: 5010 /* textEquivalent */ return new String[] { "string" }; 5011 case -1165461084: 5012 /* priority */ return new String[] { "code" }; 5013 case 3059181: 5014 /* code */ return new String[] { "CodeableConcept" }; 5015 case -934964668: 5016 /* reason */ return new String[] { "CodeableConcept" }; 5017 case 1587405498: 5018 /* documentation */ return new String[] { "RelatedArtifact" }; 5019 case -1240658034: 5020 /* goalId */ return new String[] { "id" }; 5021 case -1867885268: 5022 /* subject */ return new String[] { "CodeableConcept", "Reference" }; 5023 case -1059891784: 5024 /* trigger */ return new String[] { "TriggerDefinition" }; 5025 case -861311717: 5026 /* condition */ return new String[] {}; 5027 case 100358090: 5028 /* input */ return new String[] { "DataRequirement" }; 5029 case -1005512447: 5030 /* output */ return new String[] { "DataRequirement" }; 5031 case -384107967: 5032 /* relatedAction */ return new String[] {}; 5033 case -873664438: 5034 /* timing */ return new String[] { "dateTime", "Age", "Period", "Duration", "Range", "Timing" }; 5035 case 767422259: 5036 /* participant */ return new String[] {}; 5037 case 3575610: 5038 /* type */ return new String[] { "CodeableConcept" }; 5039 case 586678389: 5040 /* groupingBehavior */ return new String[] { "code" }; 5041 case 168639486: 5042 /* selectionBehavior */ return new String[] { "code" }; 5043 case -1163906287: 5044 /* requiredBehavior */ return new String[] { "code" }; 5045 case -1174249033: 5046 /* precheckBehavior */ return new String[] { "code" }; 5047 case -922577408: 5048 /* cardinalityBehavior */ return new String[] { "code" }; 5049 case -1014418093: 5050 /* definition */ return new String[] { "canonical", "uri" }; 5051 case 1052666732: 5052 /* transform */ return new String[] { "canonical" }; 5053 case 572625010: 5054 /* dynamicValue */ return new String[] {}; 5055 case -1422950858: 5056 /* action */ return new String[] { "@PlanDefinition.action" }; 5057 default: 5058 return super.getTypesForProperty(hash, name); 5059 } 5060 5061 } 5062 5063 @Override 5064 public Base addChild(String name) throws FHIRException { 5065 if (name.equals("prefix")) { 5066 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.prefix"); 5067 } else if (name.equals("title")) { 5068 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.title"); 5069 } else if (name.equals("description")) { 5070 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.description"); 5071 } else if (name.equals("textEquivalent")) { 5072 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.textEquivalent"); 5073 } else if (name.equals("priority")) { 5074 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.priority"); 5075 } else if (name.equals("code")) { 5076 return addCode(); 5077 } else if (name.equals("reason")) { 5078 return addReason(); 5079 } else if (name.equals("documentation")) { 5080 return addDocumentation(); 5081 } else if (name.equals("goalId")) { 5082 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.goalId"); 5083 } else if (name.equals("subjectCodeableConcept")) { 5084 this.subject = new CodeableConcept(); 5085 return this.subject; 5086 } else if (name.equals("subjectReference")) { 5087 this.subject = new Reference(); 5088 return this.subject; 5089 } else if (name.equals("trigger")) { 5090 return addTrigger(); 5091 } else if (name.equals("condition")) { 5092 return addCondition(); 5093 } else if (name.equals("input")) { 5094 return addInput(); 5095 } else if (name.equals("output")) { 5096 return addOutput(); 5097 } else if (name.equals("relatedAction")) { 5098 return addRelatedAction(); 5099 } else if (name.equals("timingDateTime")) { 5100 this.timing = new DateTimeType(); 5101 return this.timing; 5102 } else if (name.equals("timingAge")) { 5103 this.timing = new Age(); 5104 return this.timing; 5105 } else if (name.equals("timingPeriod")) { 5106 this.timing = new Period(); 5107 return this.timing; 5108 } else if (name.equals("timingDuration")) { 5109 this.timing = new Duration(); 5110 return this.timing; 5111 } else if (name.equals("timingRange")) { 5112 this.timing = new Range(); 5113 return this.timing; 5114 } else if (name.equals("timingTiming")) { 5115 this.timing = new Timing(); 5116 return this.timing; 5117 } else if (name.equals("participant")) { 5118 return addParticipant(); 5119 } else if (name.equals("type")) { 5120 this.type = new CodeableConcept(); 5121 return this.type; 5122 } else if (name.equals("groupingBehavior")) { 5123 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.groupingBehavior"); 5124 } else if (name.equals("selectionBehavior")) { 5125 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.selectionBehavior"); 5126 } else if (name.equals("requiredBehavior")) { 5127 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.requiredBehavior"); 5128 } else if (name.equals("precheckBehavior")) { 5129 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.precheckBehavior"); 5130 } else if (name.equals("cardinalityBehavior")) { 5131 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.cardinalityBehavior"); 5132 } else if (name.equals("definitionCanonical")) { 5133 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.definition[x]"); 5134 } else if (name.equals("definitionUri")) { 5135 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.definition[x]"); 5136 } else if (name.equals("transform")) { 5137 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.transform"); 5138 } else if (name.equals("dynamicValue")) { 5139 return addDynamicValue(); 5140 } else if (name.equals("action")) { 5141 return addAction(); 5142 } else 5143 return super.addChild(name); 5144 } 5145 5146 public PlanDefinitionActionComponent copy() { 5147 PlanDefinitionActionComponent dst = new PlanDefinitionActionComponent(); 5148 copyValues(dst); 5149 return dst; 5150 } 5151 5152 public void copyValues(PlanDefinitionActionComponent dst) { 5153 super.copyValues(dst); 5154 dst.prefix = prefix == null ? null : prefix.copy(); 5155 dst.title = title == null ? null : title.copy(); 5156 dst.description = description == null ? null : description.copy(); 5157 dst.textEquivalent = textEquivalent == null ? null : textEquivalent.copy(); 5158 dst.priority = priority == null ? null : priority.copy(); 5159 if (code != null) { 5160 dst.code = new ArrayList<CodeableConcept>(); 5161 for (CodeableConcept i : code) 5162 dst.code.add(i.copy()); 5163 } 5164 ; 5165 if (reason != null) { 5166 dst.reason = new ArrayList<CodeableConcept>(); 5167 for (CodeableConcept i : reason) 5168 dst.reason.add(i.copy()); 5169 } 5170 ; 5171 if (documentation != null) { 5172 dst.documentation = new ArrayList<RelatedArtifact>(); 5173 for (RelatedArtifact i : documentation) 5174 dst.documentation.add(i.copy()); 5175 } 5176 ; 5177 if (goalId != null) { 5178 dst.goalId = new ArrayList<IdType>(); 5179 for (IdType i : goalId) 5180 dst.goalId.add(i.copy()); 5181 } 5182 ; 5183 dst.subject = subject == null ? null : subject.copy(); 5184 if (trigger != null) { 5185 dst.trigger = new ArrayList<TriggerDefinition>(); 5186 for (TriggerDefinition i : trigger) 5187 dst.trigger.add(i.copy()); 5188 } 5189 ; 5190 if (condition != null) { 5191 dst.condition = new ArrayList<PlanDefinitionActionConditionComponent>(); 5192 for (PlanDefinitionActionConditionComponent i : condition) 5193 dst.condition.add(i.copy()); 5194 } 5195 ; 5196 if (input != null) { 5197 dst.input = new ArrayList<DataRequirement>(); 5198 for (DataRequirement i : input) 5199 dst.input.add(i.copy()); 5200 } 5201 ; 5202 if (output != null) { 5203 dst.output = new ArrayList<DataRequirement>(); 5204 for (DataRequirement i : output) 5205 dst.output.add(i.copy()); 5206 } 5207 ; 5208 if (relatedAction != null) { 5209 dst.relatedAction = new ArrayList<PlanDefinitionActionRelatedActionComponent>(); 5210 for (PlanDefinitionActionRelatedActionComponent i : relatedAction) 5211 dst.relatedAction.add(i.copy()); 5212 } 5213 ; 5214 dst.timing = timing == null ? null : timing.copy(); 5215 if (participant != null) { 5216 dst.participant = new ArrayList<PlanDefinitionActionParticipantComponent>(); 5217 for (PlanDefinitionActionParticipantComponent i : participant) 5218 dst.participant.add(i.copy()); 5219 } 5220 ; 5221 dst.type = type == null ? null : type.copy(); 5222 dst.groupingBehavior = groupingBehavior == null ? null : groupingBehavior.copy(); 5223 dst.selectionBehavior = selectionBehavior == null ? null : selectionBehavior.copy(); 5224 dst.requiredBehavior = requiredBehavior == null ? null : requiredBehavior.copy(); 5225 dst.precheckBehavior = precheckBehavior == null ? null : precheckBehavior.copy(); 5226 dst.cardinalityBehavior = cardinalityBehavior == null ? null : cardinalityBehavior.copy(); 5227 dst.definition = definition == null ? null : definition.copy(); 5228 dst.transform = transform == null ? null : transform.copy(); 5229 if (dynamicValue != null) { 5230 dst.dynamicValue = new ArrayList<PlanDefinitionActionDynamicValueComponent>(); 5231 for (PlanDefinitionActionDynamicValueComponent i : dynamicValue) 5232 dst.dynamicValue.add(i.copy()); 5233 } 5234 ; 5235 if (action != null) { 5236 dst.action = new ArrayList<PlanDefinitionActionComponent>(); 5237 for (PlanDefinitionActionComponent i : action) 5238 dst.action.add(i.copy()); 5239 } 5240 ; 5241 } 5242 5243 @Override 5244 public boolean equalsDeep(Base other_) { 5245 if (!super.equalsDeep(other_)) 5246 return false; 5247 if (!(other_ instanceof PlanDefinitionActionComponent)) 5248 return false; 5249 PlanDefinitionActionComponent o = (PlanDefinitionActionComponent) other_; 5250 return compareDeep(prefix, o.prefix, true) && compareDeep(title, o.title, true) 5251 && compareDeep(description, o.description, true) && compareDeep(textEquivalent, o.textEquivalent, true) 5252 && compareDeep(priority, o.priority, true) && compareDeep(code, o.code, true) 5253 && compareDeep(reason, o.reason, true) && compareDeep(documentation, o.documentation, true) 5254 && compareDeep(goalId, o.goalId, true) && compareDeep(subject, o.subject, true) 5255 && compareDeep(trigger, o.trigger, true) && compareDeep(condition, o.condition, true) 5256 && compareDeep(input, o.input, true) && compareDeep(output, o.output, true) 5257 && compareDeep(relatedAction, o.relatedAction, true) && compareDeep(timing, o.timing, true) 5258 && compareDeep(participant, o.participant, true) && compareDeep(type, o.type, true) 5259 && compareDeep(groupingBehavior, o.groupingBehavior, true) 5260 && compareDeep(selectionBehavior, o.selectionBehavior, true) 5261 && compareDeep(requiredBehavior, o.requiredBehavior, true) 5262 && compareDeep(precheckBehavior, o.precheckBehavior, true) 5263 && compareDeep(cardinalityBehavior, o.cardinalityBehavior, true) 5264 && compareDeep(definition, o.definition, true) && compareDeep(transform, o.transform, true) 5265 && compareDeep(dynamicValue, o.dynamicValue, true) && compareDeep(action, o.action, true); 5266 } 5267 5268 @Override 5269 public boolean equalsShallow(Base other_) { 5270 if (!super.equalsShallow(other_)) 5271 return false; 5272 if (!(other_ instanceof PlanDefinitionActionComponent)) 5273 return false; 5274 PlanDefinitionActionComponent o = (PlanDefinitionActionComponent) other_; 5275 return compareValues(prefix, o.prefix, true) && compareValues(title, o.title, true) 5276 && compareValues(description, o.description, true) && compareValues(textEquivalent, o.textEquivalent, true) 5277 && compareValues(priority, o.priority, true) && compareValues(goalId, o.goalId, true) 5278 && compareValues(groupingBehavior, o.groupingBehavior, true) 5279 && compareValues(selectionBehavior, o.selectionBehavior, true) 5280 && compareValues(requiredBehavior, o.requiredBehavior, true) 5281 && compareValues(precheckBehavior, o.precheckBehavior, true) 5282 && compareValues(cardinalityBehavior, o.cardinalityBehavior, true); 5283 } 5284 5285 public boolean isEmpty() { 5286 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(prefix, title, description, textEquivalent, 5287 priority, code, reason, documentation, goalId, subject, trigger, condition, input, output, relatedAction, 5288 timing, participant, type, groupingBehavior, selectionBehavior, requiredBehavior, precheckBehavior, 5289 cardinalityBehavior, definition, transform, dynamicValue, action); 5290 } 5291 5292 public String fhirType() { 5293 return "PlanDefinition.action"; 5294 5295 } 5296 5297 } 5298 5299 @Block() 5300 public static class PlanDefinitionActionConditionComponent extends BackboneElement implements IBaseBackboneElement { 5301 /** 5302 * The kind of condition. 5303 */ 5304 @Child(name = "kind", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 5305 @Description(shortDefinition = "applicability | start | stop", formalDefinition = "The kind of condition.") 5306 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-condition-kind") 5307 protected Enumeration<ActionConditionKind> kind; 5308 5309 /** 5310 * An expression that returns true or false, indicating whether the condition is 5311 * satisfied. 5312 */ 5313 @Child(name = "expression", type = { 5314 Expression.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 5315 @Description(shortDefinition = "Boolean-valued expression", formalDefinition = "An expression that returns true or false, indicating whether the condition is satisfied.") 5316 protected Expression expression; 5317 5318 private static final long serialVersionUID = -455150438L; 5319 5320 /** 5321 * Constructor 5322 */ 5323 public PlanDefinitionActionConditionComponent() { 5324 super(); 5325 } 5326 5327 /** 5328 * Constructor 5329 */ 5330 public PlanDefinitionActionConditionComponent(Enumeration<ActionConditionKind> kind) { 5331 super(); 5332 this.kind = kind; 5333 } 5334 5335 /** 5336 * @return {@link #kind} (The kind of condition.). This is the underlying object 5337 * with id, value and extensions. The accessor "getKind" gives direct 5338 * access to the value 5339 */ 5340 public Enumeration<ActionConditionKind> getKindElement() { 5341 if (this.kind == null) 5342 if (Configuration.errorOnAutoCreate()) 5343 throw new Error("Attempt to auto-create PlanDefinitionActionConditionComponent.kind"); 5344 else if (Configuration.doAutoCreate()) 5345 this.kind = new Enumeration<ActionConditionKind>(new ActionConditionKindEnumFactory()); // bb 5346 return this.kind; 5347 } 5348 5349 public boolean hasKindElement() { 5350 return this.kind != null && !this.kind.isEmpty(); 5351 } 5352 5353 public boolean hasKind() { 5354 return this.kind != null && !this.kind.isEmpty(); 5355 } 5356 5357 /** 5358 * @param value {@link #kind} (The kind of condition.). This is the underlying 5359 * object with id, value and extensions. The accessor "getKind" 5360 * gives direct access to the value 5361 */ 5362 public PlanDefinitionActionConditionComponent setKindElement(Enumeration<ActionConditionKind> value) { 5363 this.kind = value; 5364 return this; 5365 } 5366 5367 /** 5368 * @return The kind of condition. 5369 */ 5370 public ActionConditionKind getKind() { 5371 return this.kind == null ? null : this.kind.getValue(); 5372 } 5373 5374 /** 5375 * @param value The kind of condition. 5376 */ 5377 public PlanDefinitionActionConditionComponent setKind(ActionConditionKind value) { 5378 if (this.kind == null) 5379 this.kind = new Enumeration<ActionConditionKind>(new ActionConditionKindEnumFactory()); 5380 this.kind.setValue(value); 5381 return this; 5382 } 5383 5384 /** 5385 * @return {@link #expression} (An expression that returns true or false, 5386 * indicating whether the condition is satisfied.) 5387 */ 5388 public Expression getExpression() { 5389 if (this.expression == null) 5390 if (Configuration.errorOnAutoCreate()) 5391 throw new Error("Attempt to auto-create PlanDefinitionActionConditionComponent.expression"); 5392 else if (Configuration.doAutoCreate()) 5393 this.expression = new Expression(); // cc 5394 return this.expression; 5395 } 5396 5397 public boolean hasExpression() { 5398 return this.expression != null && !this.expression.isEmpty(); 5399 } 5400 5401 /** 5402 * @param value {@link #expression} (An expression that returns true or false, 5403 * indicating whether the condition is satisfied.) 5404 */ 5405 public PlanDefinitionActionConditionComponent setExpression(Expression value) { 5406 this.expression = value; 5407 return this; 5408 } 5409 5410 protected void listChildren(List<Property> children) { 5411 super.listChildren(children); 5412 children.add(new Property("kind", "code", "The kind of condition.", 0, 1, kind)); 5413 children.add(new Property("expression", "Expression", 5414 "An expression that returns true or false, indicating whether the condition is satisfied.", 0, 1, 5415 expression)); 5416 } 5417 5418 @Override 5419 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5420 switch (_hash) { 5421 case 3292052: 5422 /* kind */ return new Property("kind", "code", "The kind of condition.", 0, 1, kind); 5423 case -1795452264: 5424 /* expression */ return new Property("expression", "Expression", 5425 "An expression that returns true or false, indicating whether the condition is satisfied.", 0, 1, 5426 expression); 5427 default: 5428 return super.getNamedProperty(_hash, _name, _checkValid); 5429 } 5430 5431 } 5432 5433 @Override 5434 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5435 switch (hash) { 5436 case 3292052: 5437 /* kind */ return this.kind == null ? new Base[0] : new Base[] { this.kind }; // Enumeration<ActionConditionKind> 5438 case -1795452264: 5439 /* expression */ return this.expression == null ? new Base[0] : new Base[] { this.expression }; // Expression 5440 default: 5441 return super.getProperty(hash, name, checkValid); 5442 } 5443 5444 } 5445 5446 @Override 5447 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5448 switch (hash) { 5449 case 3292052: // kind 5450 value = new ActionConditionKindEnumFactory().fromType(castToCode(value)); 5451 this.kind = (Enumeration) value; // Enumeration<ActionConditionKind> 5452 return value; 5453 case -1795452264: // expression 5454 this.expression = castToExpression(value); // Expression 5455 return value; 5456 default: 5457 return super.setProperty(hash, name, value); 5458 } 5459 5460 } 5461 5462 @Override 5463 public Base setProperty(String name, Base value) throws FHIRException { 5464 if (name.equals("kind")) { 5465 value = new ActionConditionKindEnumFactory().fromType(castToCode(value)); 5466 this.kind = (Enumeration) value; // Enumeration<ActionConditionKind> 5467 } else if (name.equals("expression")) { 5468 this.expression = castToExpression(value); // Expression 5469 } else 5470 return super.setProperty(name, value); 5471 return value; 5472 } 5473 5474 @Override 5475 public void removeChild(String name, Base value) throws FHIRException { 5476 if (name.equals("kind")) { 5477 this.kind = null; 5478 } else if (name.equals("expression")) { 5479 this.expression = null; 5480 } else 5481 super.removeChild(name, value); 5482 5483 } 5484 5485 @Override 5486 public Base makeProperty(int hash, String name) throws FHIRException { 5487 switch (hash) { 5488 case 3292052: 5489 return getKindElement(); 5490 case -1795452264: 5491 return getExpression(); 5492 default: 5493 return super.makeProperty(hash, name); 5494 } 5495 5496 } 5497 5498 @Override 5499 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5500 switch (hash) { 5501 case 3292052: 5502 /* kind */ return new String[] { "code" }; 5503 case -1795452264: 5504 /* expression */ return new String[] { "Expression" }; 5505 default: 5506 return super.getTypesForProperty(hash, name); 5507 } 5508 5509 } 5510 5511 @Override 5512 public Base addChild(String name) throws FHIRException { 5513 if (name.equals("kind")) { 5514 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.kind"); 5515 } else if (name.equals("expression")) { 5516 this.expression = new Expression(); 5517 return this.expression; 5518 } else 5519 return super.addChild(name); 5520 } 5521 5522 public PlanDefinitionActionConditionComponent copy() { 5523 PlanDefinitionActionConditionComponent dst = new PlanDefinitionActionConditionComponent(); 5524 copyValues(dst); 5525 return dst; 5526 } 5527 5528 public void copyValues(PlanDefinitionActionConditionComponent dst) { 5529 super.copyValues(dst); 5530 dst.kind = kind == null ? null : kind.copy(); 5531 dst.expression = expression == null ? null : expression.copy(); 5532 } 5533 5534 @Override 5535 public boolean equalsDeep(Base other_) { 5536 if (!super.equalsDeep(other_)) 5537 return false; 5538 if (!(other_ instanceof PlanDefinitionActionConditionComponent)) 5539 return false; 5540 PlanDefinitionActionConditionComponent o = (PlanDefinitionActionConditionComponent) other_; 5541 return compareDeep(kind, o.kind, true) && compareDeep(expression, o.expression, true); 5542 } 5543 5544 @Override 5545 public boolean equalsShallow(Base other_) { 5546 if (!super.equalsShallow(other_)) 5547 return false; 5548 if (!(other_ instanceof PlanDefinitionActionConditionComponent)) 5549 return false; 5550 PlanDefinitionActionConditionComponent o = (PlanDefinitionActionConditionComponent) other_; 5551 return compareValues(kind, o.kind, true); 5552 } 5553 5554 public boolean isEmpty() { 5555 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(kind, expression); 5556 } 5557 5558 public String fhirType() { 5559 return "PlanDefinition.action.condition"; 5560 5561 } 5562 5563 } 5564 5565 @Block() 5566 public static class PlanDefinitionActionRelatedActionComponent extends BackboneElement 5567 implements IBaseBackboneElement { 5568 /** 5569 * The element id of the related action. 5570 */ 5571 @Child(name = "actionId", type = { IdType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 5572 @Description(shortDefinition = "What action is this related to", formalDefinition = "The element id of the related action.") 5573 protected IdType actionId; 5574 5575 /** 5576 * The relationship of this action to the related action. 5577 */ 5578 @Child(name = "relationship", type = { 5579 CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 5580 @Description(shortDefinition = "before-start | before | before-end | concurrent-with-start | concurrent | concurrent-with-end | after-start | after | after-end", formalDefinition = "The relationship of this action to the related action.") 5581 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-relationship-type") 5582 protected Enumeration<ActionRelationshipType> relationship; 5583 5584 /** 5585 * A duration or range of durations to apply to the relationship. For example, 5586 * 30-60 minutes before. 5587 */ 5588 @Child(name = "offset", type = { Duration.class, 5589 Range.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 5590 @Description(shortDefinition = "Time offset for the relationship", formalDefinition = "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.") 5591 protected Type offset; 5592 5593 private static final long serialVersionUID = 1063306770L; 5594 5595 /** 5596 * Constructor 5597 */ 5598 public PlanDefinitionActionRelatedActionComponent() { 5599 super(); 5600 } 5601 5602 /** 5603 * Constructor 5604 */ 5605 public PlanDefinitionActionRelatedActionComponent(IdType actionId, 5606 Enumeration<ActionRelationshipType> relationship) { 5607 super(); 5608 this.actionId = actionId; 5609 this.relationship = relationship; 5610 } 5611 5612 /** 5613 * @return {@link #actionId} (The element id of the related action.). This is 5614 * the underlying object with id, value and extensions. The accessor 5615 * "getActionId" gives direct access to the value 5616 */ 5617 public IdType getActionIdElement() { 5618 if (this.actionId == null) 5619 if (Configuration.errorOnAutoCreate()) 5620 throw new Error("Attempt to auto-create PlanDefinitionActionRelatedActionComponent.actionId"); 5621 else if (Configuration.doAutoCreate()) 5622 this.actionId = new IdType(); // bb 5623 return this.actionId; 5624 } 5625 5626 public boolean hasActionIdElement() { 5627 return this.actionId != null && !this.actionId.isEmpty(); 5628 } 5629 5630 public boolean hasActionId() { 5631 return this.actionId != null && !this.actionId.isEmpty(); 5632 } 5633 5634 /** 5635 * @param value {@link #actionId} (The element id of the related action.). This 5636 * is the underlying object with id, value and extensions. The 5637 * accessor "getActionId" gives direct access to the value 5638 */ 5639 public PlanDefinitionActionRelatedActionComponent setActionIdElement(IdType value) { 5640 this.actionId = value; 5641 return this; 5642 } 5643 5644 /** 5645 * @return The element id of the related action. 5646 */ 5647 public String getActionId() { 5648 return this.actionId == null ? null : this.actionId.getValue(); 5649 } 5650 5651 /** 5652 * @param value The element id of the related action. 5653 */ 5654 public PlanDefinitionActionRelatedActionComponent setActionId(String value) { 5655 if (this.actionId == null) 5656 this.actionId = new IdType(); 5657 this.actionId.setValue(value); 5658 return this; 5659 } 5660 5661 /** 5662 * @return {@link #relationship} (The relationship of this action to the related 5663 * action.). This is the underlying object with id, value and 5664 * extensions. The accessor "getRelationship" gives direct access to the 5665 * value 5666 */ 5667 public Enumeration<ActionRelationshipType> getRelationshipElement() { 5668 if (this.relationship == null) 5669 if (Configuration.errorOnAutoCreate()) 5670 throw new Error("Attempt to auto-create PlanDefinitionActionRelatedActionComponent.relationship"); 5671 else if (Configuration.doAutoCreate()) 5672 this.relationship = new Enumeration<ActionRelationshipType>(new ActionRelationshipTypeEnumFactory()); // bb 5673 return this.relationship; 5674 } 5675 5676 public boolean hasRelationshipElement() { 5677 return this.relationship != null && !this.relationship.isEmpty(); 5678 } 5679 5680 public boolean hasRelationship() { 5681 return this.relationship != null && !this.relationship.isEmpty(); 5682 } 5683 5684 /** 5685 * @param value {@link #relationship} (The relationship of this action to the 5686 * related action.). This is the underlying object with id, value 5687 * and extensions. The accessor "getRelationship" gives direct 5688 * access to the value 5689 */ 5690 public PlanDefinitionActionRelatedActionComponent setRelationshipElement( 5691 Enumeration<ActionRelationshipType> value) { 5692 this.relationship = value; 5693 return this; 5694 } 5695 5696 /** 5697 * @return The relationship of this action to the related action. 5698 */ 5699 public ActionRelationshipType getRelationship() { 5700 return this.relationship == null ? null : this.relationship.getValue(); 5701 } 5702 5703 /** 5704 * @param value The relationship of this action to the related action. 5705 */ 5706 public PlanDefinitionActionRelatedActionComponent setRelationship(ActionRelationshipType value) { 5707 if (this.relationship == null) 5708 this.relationship = new Enumeration<ActionRelationshipType>(new ActionRelationshipTypeEnumFactory()); 5709 this.relationship.setValue(value); 5710 return this; 5711 } 5712 5713 /** 5714 * @return {@link #offset} (A duration or range of durations to apply to the 5715 * relationship. For example, 30-60 minutes before.) 5716 */ 5717 public Type getOffset() { 5718 return this.offset; 5719 } 5720 5721 /** 5722 * @return {@link #offset} (A duration or range of durations to apply to the 5723 * relationship. For example, 30-60 minutes before.) 5724 */ 5725 public Duration getOffsetDuration() throws FHIRException { 5726 if (this.offset == null) 5727 this.offset = new Duration(); 5728 if (!(this.offset instanceof Duration)) 5729 throw new FHIRException("Type mismatch: the type Duration was expected, but " + this.offset.getClass().getName() 5730 + " was encountered"); 5731 return (Duration) this.offset; 5732 } 5733 5734 public boolean hasOffsetDuration() { 5735 return this.offset instanceof Duration; 5736 } 5737 5738 /** 5739 * @return {@link #offset} (A duration or range of durations to apply to the 5740 * relationship. For example, 30-60 minutes before.) 5741 */ 5742 public Range getOffsetRange() throws FHIRException { 5743 if (this.offset == null) 5744 this.offset = new Range(); 5745 if (!(this.offset instanceof Range)) 5746 throw new FHIRException( 5747 "Type mismatch: the type Range was expected, but " + this.offset.getClass().getName() + " was encountered"); 5748 return (Range) this.offset; 5749 } 5750 5751 public boolean hasOffsetRange() { 5752 return this.offset instanceof Range; 5753 } 5754 5755 public boolean hasOffset() { 5756 return this.offset != null && !this.offset.isEmpty(); 5757 } 5758 5759 /** 5760 * @param value {@link #offset} (A duration or range of durations to apply to 5761 * the relationship. For example, 30-60 minutes before.) 5762 */ 5763 public PlanDefinitionActionRelatedActionComponent setOffset(Type value) { 5764 if (value != null && !(value instanceof Duration || value instanceof Range)) 5765 throw new Error("Not the right type for PlanDefinition.action.relatedAction.offset[x]: " + value.fhirType()); 5766 this.offset = value; 5767 return this; 5768 } 5769 5770 protected void listChildren(List<Property> children) { 5771 super.listChildren(children); 5772 children.add(new Property("actionId", "id", "The element id of the related action.", 0, 1, actionId)); 5773 children.add(new Property("relationship", "code", "The relationship of this action to the related action.", 0, 1, 5774 relationship)); 5775 children.add(new Property("offset[x]", "Duration|Range", 5776 "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, 5777 offset)); 5778 } 5779 5780 @Override 5781 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5782 switch (_hash) { 5783 case -1656172047: 5784 /* actionId */ return new Property("actionId", "id", "The element id of the related action.", 0, 1, actionId); 5785 case -261851592: 5786 /* relationship */ return new Property("relationship", "code", 5787 "The relationship of this action to the related action.", 0, 1, relationship); 5788 case -1960684787: 5789 /* offset[x] */ return new Property("offset[x]", "Duration|Range", 5790 "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, 5791 offset); 5792 case -1019779949: 5793 /* offset */ return new Property("offset[x]", "Duration|Range", 5794 "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, 5795 offset); 5796 case 134075207: 5797 /* offsetDuration */ return new Property("offset[x]", "Duration|Range", 5798 "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, 5799 offset); 5800 case 1263585386: 5801 /* offsetRange */ return new Property("offset[x]", "Duration|Range", 5802 "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, 5803 offset); 5804 default: 5805 return super.getNamedProperty(_hash, _name, _checkValid); 5806 } 5807 5808 } 5809 5810 @Override 5811 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5812 switch (hash) { 5813 case -1656172047: 5814 /* actionId */ return this.actionId == null ? new Base[0] : new Base[] { this.actionId }; // IdType 5815 case -261851592: 5816 /* relationship */ return this.relationship == null ? new Base[0] : new Base[] { this.relationship }; // Enumeration<ActionRelationshipType> 5817 case -1019779949: 5818 /* offset */ return this.offset == null ? new Base[0] : new Base[] { this.offset }; // Type 5819 default: 5820 return super.getProperty(hash, name, checkValid); 5821 } 5822 5823 } 5824 5825 @Override 5826 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5827 switch (hash) { 5828 case -1656172047: // actionId 5829 this.actionId = castToId(value); // IdType 5830 return value; 5831 case -261851592: // relationship 5832 value = new ActionRelationshipTypeEnumFactory().fromType(castToCode(value)); 5833 this.relationship = (Enumeration) value; // Enumeration<ActionRelationshipType> 5834 return value; 5835 case -1019779949: // offset 5836 this.offset = castToType(value); // Type 5837 return value; 5838 default: 5839 return super.setProperty(hash, name, value); 5840 } 5841 5842 } 5843 5844 @Override 5845 public Base setProperty(String name, Base value) throws FHIRException { 5846 if (name.equals("actionId")) { 5847 this.actionId = castToId(value); // IdType 5848 } else if (name.equals("relationship")) { 5849 value = new ActionRelationshipTypeEnumFactory().fromType(castToCode(value)); 5850 this.relationship = (Enumeration) value; // Enumeration<ActionRelationshipType> 5851 } else if (name.equals("offset[x]")) { 5852 this.offset = castToType(value); // Type 5853 } else 5854 return super.setProperty(name, value); 5855 return value; 5856 } 5857 5858 @Override 5859 public void removeChild(String name, Base value) throws FHIRException { 5860 if (name.equals("actionId")) { 5861 this.actionId = null; 5862 } else if (name.equals("relationship")) { 5863 this.relationship = null; 5864 } else if (name.equals("offset[x]")) { 5865 this.offset = null; 5866 } else 5867 super.removeChild(name, value); 5868 5869 } 5870 5871 @Override 5872 public Base makeProperty(int hash, String name) throws FHIRException { 5873 switch (hash) { 5874 case -1656172047: 5875 return getActionIdElement(); 5876 case -261851592: 5877 return getRelationshipElement(); 5878 case -1960684787: 5879 return getOffset(); 5880 case -1019779949: 5881 return getOffset(); 5882 default: 5883 return super.makeProperty(hash, name); 5884 } 5885 5886 } 5887 5888 @Override 5889 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5890 switch (hash) { 5891 case -1656172047: 5892 /* actionId */ return new String[] { "id" }; 5893 case -261851592: 5894 /* relationship */ return new String[] { "code" }; 5895 case -1019779949: 5896 /* offset */ return new String[] { "Duration", "Range" }; 5897 default: 5898 return super.getTypesForProperty(hash, name); 5899 } 5900 5901 } 5902 5903 @Override 5904 public Base addChild(String name) throws FHIRException { 5905 if (name.equals("actionId")) { 5906 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.actionId"); 5907 } else if (name.equals("relationship")) { 5908 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.relationship"); 5909 } else if (name.equals("offsetDuration")) { 5910 this.offset = new Duration(); 5911 return this.offset; 5912 } else if (name.equals("offsetRange")) { 5913 this.offset = new Range(); 5914 return this.offset; 5915 } else 5916 return super.addChild(name); 5917 } 5918 5919 public PlanDefinitionActionRelatedActionComponent copy() { 5920 PlanDefinitionActionRelatedActionComponent dst = new PlanDefinitionActionRelatedActionComponent(); 5921 copyValues(dst); 5922 return dst; 5923 } 5924 5925 public void copyValues(PlanDefinitionActionRelatedActionComponent dst) { 5926 super.copyValues(dst); 5927 dst.actionId = actionId == null ? null : actionId.copy(); 5928 dst.relationship = relationship == null ? null : relationship.copy(); 5929 dst.offset = offset == null ? null : offset.copy(); 5930 } 5931 5932 @Override 5933 public boolean equalsDeep(Base other_) { 5934 if (!super.equalsDeep(other_)) 5935 return false; 5936 if (!(other_ instanceof PlanDefinitionActionRelatedActionComponent)) 5937 return false; 5938 PlanDefinitionActionRelatedActionComponent o = (PlanDefinitionActionRelatedActionComponent) other_; 5939 return compareDeep(actionId, o.actionId, true) && compareDeep(relationship, o.relationship, true) 5940 && compareDeep(offset, o.offset, true); 5941 } 5942 5943 @Override 5944 public boolean equalsShallow(Base other_) { 5945 if (!super.equalsShallow(other_)) 5946 return false; 5947 if (!(other_ instanceof PlanDefinitionActionRelatedActionComponent)) 5948 return false; 5949 PlanDefinitionActionRelatedActionComponent o = (PlanDefinitionActionRelatedActionComponent) other_; 5950 return compareValues(actionId, o.actionId, true) && compareValues(relationship, o.relationship, true); 5951 } 5952 5953 public boolean isEmpty() { 5954 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(actionId, relationship, offset); 5955 } 5956 5957 public String fhirType() { 5958 return "PlanDefinition.action.relatedAction"; 5959 5960 } 5961 5962 } 5963 5964 @Block() 5965 public static class PlanDefinitionActionParticipantComponent extends BackboneElement implements IBaseBackboneElement { 5966 /** 5967 * The type of participant in the action. 5968 */ 5969 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 5970 @Description(shortDefinition = "patient | practitioner | related-person | device", formalDefinition = "The type of participant in the action.") 5971 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-participant-type") 5972 protected Enumeration<ActionParticipantType> type; 5973 5974 /** 5975 * The role the participant should play in performing the described action. 5976 */ 5977 @Child(name = "role", type = { 5978 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 5979 @Description(shortDefinition = "E.g. Nurse, Surgeon, Parent", formalDefinition = "The role the participant should play in performing the described action.") 5980 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-participant-role") 5981 protected CodeableConcept role; 5982 5983 private static final long serialVersionUID = -1152013659L; 5984 5985 /** 5986 * Constructor 5987 */ 5988 public PlanDefinitionActionParticipantComponent() { 5989 super(); 5990 } 5991 5992 /** 5993 * Constructor 5994 */ 5995 public PlanDefinitionActionParticipantComponent(Enumeration<ActionParticipantType> type) { 5996 super(); 5997 this.type = type; 5998 } 5999 6000 /** 6001 * @return {@link #type} (The type of participant in the action.). This is the 6002 * underlying object with id, value and extensions. The accessor 6003 * "getType" gives direct access to the value 6004 */ 6005 public Enumeration<ActionParticipantType> getTypeElement() { 6006 if (this.type == null) 6007 if (Configuration.errorOnAutoCreate()) 6008 throw new Error("Attempt to auto-create PlanDefinitionActionParticipantComponent.type"); 6009 else if (Configuration.doAutoCreate()) 6010 this.type = new Enumeration<ActionParticipantType>(new ActionParticipantTypeEnumFactory()); // bb 6011 return this.type; 6012 } 6013 6014 public boolean hasTypeElement() { 6015 return this.type != null && !this.type.isEmpty(); 6016 } 6017 6018 public boolean hasType() { 6019 return this.type != null && !this.type.isEmpty(); 6020 } 6021 6022 /** 6023 * @param value {@link #type} (The type of participant in the action.). This is 6024 * the underlying object with id, value and extensions. The 6025 * accessor "getType" gives direct access to the value 6026 */ 6027 public PlanDefinitionActionParticipantComponent setTypeElement(Enumeration<ActionParticipantType> value) { 6028 this.type = value; 6029 return this; 6030 } 6031 6032 /** 6033 * @return The type of participant in the action. 6034 */ 6035 public ActionParticipantType getType() { 6036 return this.type == null ? null : this.type.getValue(); 6037 } 6038 6039 /** 6040 * @param value The type of participant in the action. 6041 */ 6042 public PlanDefinitionActionParticipantComponent setType(ActionParticipantType value) { 6043 if (this.type == null) 6044 this.type = new Enumeration<ActionParticipantType>(new ActionParticipantTypeEnumFactory()); 6045 this.type.setValue(value); 6046 return this; 6047 } 6048 6049 /** 6050 * @return {@link #role} (The role the participant should play in performing the 6051 * described action.) 6052 */ 6053 public CodeableConcept getRole() { 6054 if (this.role == null) 6055 if (Configuration.errorOnAutoCreate()) 6056 throw new Error("Attempt to auto-create PlanDefinitionActionParticipantComponent.role"); 6057 else if (Configuration.doAutoCreate()) 6058 this.role = new CodeableConcept(); // cc 6059 return this.role; 6060 } 6061 6062 public boolean hasRole() { 6063 return this.role != null && !this.role.isEmpty(); 6064 } 6065 6066 /** 6067 * @param value {@link #role} (The role the participant should play in 6068 * performing the described action.) 6069 */ 6070 public PlanDefinitionActionParticipantComponent setRole(CodeableConcept value) { 6071 this.role = value; 6072 return this; 6073 } 6074 6075 protected void listChildren(List<Property> children) { 6076 super.listChildren(children); 6077 children.add(new Property("type", "code", "The type of participant in the action.", 0, 1, type)); 6078 children.add(new Property("role", "CodeableConcept", 6079 "The role the participant should play in performing the described action.", 0, 1, role)); 6080 } 6081 6082 @Override 6083 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6084 switch (_hash) { 6085 case 3575610: 6086 /* type */ return new Property("type", "code", "The type of participant in the action.", 0, 1, type); 6087 case 3506294: 6088 /* role */ return new Property("role", "CodeableConcept", 6089 "The role the participant should play in performing the described action.", 0, 1, role); 6090 default: 6091 return super.getNamedProperty(_hash, _name, _checkValid); 6092 } 6093 6094 } 6095 6096 @Override 6097 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6098 switch (hash) { 6099 case 3575610: 6100 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<ActionParticipantType> 6101 case 3506294: 6102 /* role */ return this.role == null ? new Base[0] : new Base[] { this.role }; // CodeableConcept 6103 default: 6104 return super.getProperty(hash, name, checkValid); 6105 } 6106 6107 } 6108 6109 @Override 6110 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6111 switch (hash) { 6112 case 3575610: // type 6113 value = new ActionParticipantTypeEnumFactory().fromType(castToCode(value)); 6114 this.type = (Enumeration) value; // Enumeration<ActionParticipantType> 6115 return value; 6116 case 3506294: // role 6117 this.role = castToCodeableConcept(value); // CodeableConcept 6118 return value; 6119 default: 6120 return super.setProperty(hash, name, value); 6121 } 6122 6123 } 6124 6125 @Override 6126 public Base setProperty(String name, Base value) throws FHIRException { 6127 if (name.equals("type")) { 6128 value = new ActionParticipantTypeEnumFactory().fromType(castToCode(value)); 6129 this.type = (Enumeration) value; // Enumeration<ActionParticipantType> 6130 } else if (name.equals("role")) { 6131 this.role = castToCodeableConcept(value); // CodeableConcept 6132 } else 6133 return super.setProperty(name, value); 6134 return value; 6135 } 6136 6137 @Override 6138 public void removeChild(String name, Base value) throws FHIRException { 6139 if (name.equals("type")) { 6140 this.type = null; 6141 } else if (name.equals("role")) { 6142 this.role = null; 6143 } else 6144 super.removeChild(name, value); 6145 6146 } 6147 6148 @Override 6149 public Base makeProperty(int hash, String name) throws FHIRException { 6150 switch (hash) { 6151 case 3575610: 6152 return getTypeElement(); 6153 case 3506294: 6154 return getRole(); 6155 default: 6156 return super.makeProperty(hash, name); 6157 } 6158 6159 } 6160 6161 @Override 6162 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6163 switch (hash) { 6164 case 3575610: 6165 /* type */ return new String[] { "code" }; 6166 case 3506294: 6167 /* role */ return new String[] { "CodeableConcept" }; 6168 default: 6169 return super.getTypesForProperty(hash, name); 6170 } 6171 6172 } 6173 6174 @Override 6175 public Base addChild(String name) throws FHIRException { 6176 if (name.equals("type")) { 6177 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.type"); 6178 } else if (name.equals("role")) { 6179 this.role = new CodeableConcept(); 6180 return this.role; 6181 } else 6182 return super.addChild(name); 6183 } 6184 6185 public PlanDefinitionActionParticipantComponent copy() { 6186 PlanDefinitionActionParticipantComponent dst = new PlanDefinitionActionParticipantComponent(); 6187 copyValues(dst); 6188 return dst; 6189 } 6190 6191 public void copyValues(PlanDefinitionActionParticipantComponent dst) { 6192 super.copyValues(dst); 6193 dst.type = type == null ? null : type.copy(); 6194 dst.role = role == null ? null : role.copy(); 6195 } 6196 6197 @Override 6198 public boolean equalsDeep(Base other_) { 6199 if (!super.equalsDeep(other_)) 6200 return false; 6201 if (!(other_ instanceof PlanDefinitionActionParticipantComponent)) 6202 return false; 6203 PlanDefinitionActionParticipantComponent o = (PlanDefinitionActionParticipantComponent) other_; 6204 return compareDeep(type, o.type, true) && compareDeep(role, o.role, true); 6205 } 6206 6207 @Override 6208 public boolean equalsShallow(Base other_) { 6209 if (!super.equalsShallow(other_)) 6210 return false; 6211 if (!(other_ instanceof PlanDefinitionActionParticipantComponent)) 6212 return false; 6213 PlanDefinitionActionParticipantComponent o = (PlanDefinitionActionParticipantComponent) other_; 6214 return compareValues(type, o.type, true); 6215 } 6216 6217 public boolean isEmpty() { 6218 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, role); 6219 } 6220 6221 public String fhirType() { 6222 return "PlanDefinition.action.participant"; 6223 6224 } 6225 6226 } 6227 6228 @Block() 6229 public static class PlanDefinitionActionDynamicValueComponent extends BackboneElement 6230 implements IBaseBackboneElement { 6231 /** 6232 * The path to the element to be customized. This is the path on the resource 6233 * that will hold the result of the calculation defined by the expression. The 6234 * specified path SHALL be a FHIRPath resolveable on the specified target type 6235 * of the ActivityDefinition, and SHALL consist only of identifiers, constant 6236 * indexers, and a restricted subset of functions. The path is allowed to 6237 * contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to 6238 * traverse multiple-cardinality sub-elements (see the [Simple FHIRPath 6239 * Profile](fhirpath.html#simple) for full details). 6240 */ 6241 @Child(name = "path", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 6242 @Description(shortDefinition = "The path to the element to be set dynamically", formalDefinition = "The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolveable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).") 6243 protected StringType path; 6244 6245 /** 6246 * An expression specifying the value of the customized element. 6247 */ 6248 @Child(name = "expression", type = { 6249 Expression.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 6250 @Description(shortDefinition = "An expression that provides the dynamic value for the customization", formalDefinition = "An expression specifying the value of the customized element.") 6251 protected Expression expression; 6252 6253 private static final long serialVersionUID = 1064529082L; 6254 6255 /** 6256 * Constructor 6257 */ 6258 public PlanDefinitionActionDynamicValueComponent() { 6259 super(); 6260 } 6261 6262 /** 6263 * @return {@link #path} (The path to the element to be customized. This is the 6264 * path on the resource that will hold the result of the calculation 6265 * defined by the expression. The specified path SHALL be a FHIRPath 6266 * resolveable on the specified target type of the ActivityDefinition, 6267 * and SHALL consist only of identifiers, constant indexers, and a 6268 * restricted subset of functions. The path is allowed to contain 6269 * qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to 6270 * traverse multiple-cardinality sub-elements (see the [Simple FHIRPath 6271 * Profile](fhirpath.html#simple) for full details).). This is the 6272 * underlying object with id, value and extensions. The accessor 6273 * "getPath" gives direct access to the value 6274 */ 6275 public StringType getPathElement() { 6276 if (this.path == null) 6277 if (Configuration.errorOnAutoCreate()) 6278 throw new Error("Attempt to auto-create PlanDefinitionActionDynamicValueComponent.path"); 6279 else if (Configuration.doAutoCreate()) 6280 this.path = new StringType(); // bb 6281 return this.path; 6282 } 6283 6284 public boolean hasPathElement() { 6285 return this.path != null && !this.path.isEmpty(); 6286 } 6287 6288 public boolean hasPath() { 6289 return this.path != null && !this.path.isEmpty(); 6290 } 6291 6292 /** 6293 * @param value {@link #path} (The path to the element to be customized. This is 6294 * the path on the resource that will hold the result of the 6295 * calculation defined by the expression. The specified path SHALL 6296 * be a FHIRPath resolveable on the specified target type of the 6297 * ActivityDefinition, and SHALL consist only of identifiers, 6298 * constant indexers, and a restricted subset of functions. The 6299 * path is allowed to contain qualifiers (.) to traverse 6300 * sub-elements, as well as indexers ([x]) to traverse 6301 * multiple-cardinality sub-elements (see the [Simple FHIRPath 6302 * Profile](fhirpath.html#simple) for full details).). This is the 6303 * underlying object with id, value and extensions. The accessor 6304 * "getPath" gives direct access to the value 6305 */ 6306 public PlanDefinitionActionDynamicValueComponent setPathElement(StringType value) { 6307 this.path = value; 6308 return this; 6309 } 6310 6311 /** 6312 * @return The path to the element to be customized. This is the path on the 6313 * resource that will hold the result of the calculation defined by the 6314 * expression. The specified path SHALL be a FHIRPath resolveable on the 6315 * specified target type of the ActivityDefinition, and SHALL consist 6316 * only of identifiers, constant indexers, and a restricted subset of 6317 * functions. The path is allowed to contain qualifiers (.) to traverse 6318 * sub-elements, as well as indexers ([x]) to traverse 6319 * multiple-cardinality sub-elements (see the [Simple FHIRPath 6320 * Profile](fhirpath.html#simple) for full details). 6321 */ 6322 public String getPath() { 6323 return this.path == null ? null : this.path.getValue(); 6324 } 6325 6326 /** 6327 * @param value The path to the element to be customized. This is the path on 6328 * the resource that will hold the result of the calculation 6329 * defined by the expression. The specified path SHALL be a 6330 * FHIRPath resolveable on the specified target type of the 6331 * ActivityDefinition, and SHALL consist only of identifiers, 6332 * constant indexers, and a restricted subset of functions. The 6333 * path is allowed to contain qualifiers (.) to traverse 6334 * sub-elements, as well as indexers ([x]) to traverse 6335 * multiple-cardinality sub-elements (see the [Simple FHIRPath 6336 * Profile](fhirpath.html#simple) for full details). 6337 */ 6338 public PlanDefinitionActionDynamicValueComponent setPath(String value) { 6339 if (Utilities.noString(value)) 6340 this.path = null; 6341 else { 6342 if (this.path == null) 6343 this.path = new StringType(); 6344 this.path.setValue(value); 6345 } 6346 return this; 6347 } 6348 6349 /** 6350 * @return {@link #expression} (An expression specifying the value of the 6351 * customized element.) 6352 */ 6353 public Expression getExpression() { 6354 if (this.expression == null) 6355 if (Configuration.errorOnAutoCreate()) 6356 throw new Error("Attempt to auto-create PlanDefinitionActionDynamicValueComponent.expression"); 6357 else if (Configuration.doAutoCreate()) 6358 this.expression = new Expression(); // cc 6359 return this.expression; 6360 } 6361 6362 public boolean hasExpression() { 6363 return this.expression != null && !this.expression.isEmpty(); 6364 } 6365 6366 /** 6367 * @param value {@link #expression} (An expression specifying the value of the 6368 * customized element.) 6369 */ 6370 public PlanDefinitionActionDynamicValueComponent setExpression(Expression value) { 6371 this.expression = value; 6372 return this; 6373 } 6374 6375 protected void listChildren(List<Property> children) { 6376 super.listChildren(children); 6377 children.add(new Property("path", "string", 6378 "The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolveable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).", 6379 0, 1, path)); 6380 children.add(new Property("expression", "Expression", 6381 "An expression specifying the value of the customized element.", 0, 1, expression)); 6382 } 6383 6384 @Override 6385 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6386 switch (_hash) { 6387 case 3433509: 6388 /* path */ return new Property("path", "string", 6389 "The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolveable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).", 6390 0, 1, path); 6391 case -1795452264: 6392 /* expression */ return new Property("expression", "Expression", 6393 "An expression specifying the value of the customized element.", 0, 1, expression); 6394 default: 6395 return super.getNamedProperty(_hash, _name, _checkValid); 6396 } 6397 6398 } 6399 6400 @Override 6401 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6402 switch (hash) { 6403 case 3433509: 6404 /* path */ return this.path == null ? new Base[0] : new Base[] { this.path }; // StringType 6405 case -1795452264: 6406 /* expression */ return this.expression == null ? new Base[0] : new Base[] { this.expression }; // Expression 6407 default: 6408 return super.getProperty(hash, name, checkValid); 6409 } 6410 6411 } 6412 6413 @Override 6414 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6415 switch (hash) { 6416 case 3433509: // path 6417 this.path = castToString(value); // StringType 6418 return value; 6419 case -1795452264: // expression 6420 this.expression = castToExpression(value); // Expression 6421 return value; 6422 default: 6423 return super.setProperty(hash, name, value); 6424 } 6425 6426 } 6427 6428 @Override 6429 public Base setProperty(String name, Base value) throws FHIRException { 6430 if (name.equals("path")) { 6431 this.path = castToString(value); // StringType 6432 } else if (name.equals("expression")) { 6433 this.expression = castToExpression(value); // Expression 6434 } else 6435 return super.setProperty(name, value); 6436 return value; 6437 } 6438 6439 @Override 6440 public void removeChild(String name, Base value) throws FHIRException { 6441 if (name.equals("path")) { 6442 this.path = null; 6443 } else if (name.equals("expression")) { 6444 this.expression = null; 6445 } else 6446 super.removeChild(name, value); 6447 6448 } 6449 6450 @Override 6451 public Base makeProperty(int hash, String name) throws FHIRException { 6452 switch (hash) { 6453 case 3433509: 6454 return getPathElement(); 6455 case -1795452264: 6456 return getExpression(); 6457 default: 6458 return super.makeProperty(hash, name); 6459 } 6460 6461 } 6462 6463 @Override 6464 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6465 switch (hash) { 6466 case 3433509: 6467 /* path */ return new String[] { "string" }; 6468 case -1795452264: 6469 /* expression */ return new String[] { "Expression" }; 6470 default: 6471 return super.getTypesForProperty(hash, name); 6472 } 6473 6474 } 6475 6476 @Override 6477 public Base addChild(String name) throws FHIRException { 6478 if (name.equals("path")) { 6479 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.path"); 6480 } else if (name.equals("expression")) { 6481 this.expression = new Expression(); 6482 return this.expression; 6483 } else 6484 return super.addChild(name); 6485 } 6486 6487 public PlanDefinitionActionDynamicValueComponent copy() { 6488 PlanDefinitionActionDynamicValueComponent dst = new PlanDefinitionActionDynamicValueComponent(); 6489 copyValues(dst); 6490 return dst; 6491 } 6492 6493 public void copyValues(PlanDefinitionActionDynamicValueComponent dst) { 6494 super.copyValues(dst); 6495 dst.path = path == null ? null : path.copy(); 6496 dst.expression = expression == null ? null : expression.copy(); 6497 } 6498 6499 @Override 6500 public boolean equalsDeep(Base other_) { 6501 if (!super.equalsDeep(other_)) 6502 return false; 6503 if (!(other_ instanceof PlanDefinitionActionDynamicValueComponent)) 6504 return false; 6505 PlanDefinitionActionDynamicValueComponent o = (PlanDefinitionActionDynamicValueComponent) other_; 6506 return compareDeep(path, o.path, true) && compareDeep(expression, o.expression, true); 6507 } 6508 6509 @Override 6510 public boolean equalsShallow(Base other_) { 6511 if (!super.equalsShallow(other_)) 6512 return false; 6513 if (!(other_ instanceof PlanDefinitionActionDynamicValueComponent)) 6514 return false; 6515 PlanDefinitionActionDynamicValueComponent o = (PlanDefinitionActionDynamicValueComponent) other_; 6516 return compareValues(path, o.path, true); 6517 } 6518 6519 public boolean isEmpty() { 6520 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(path, expression); 6521 } 6522 6523 public String fhirType() { 6524 return "PlanDefinition.action.dynamicValue"; 6525 6526 } 6527 6528 } 6529 6530 /** 6531 * A formal identifier that is used to identify this plan definition when it is 6532 * represented in other formats, or referenced in a specification, model, design 6533 * or an instance. 6534 */ 6535 @Child(name = "identifier", type = { 6536 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 6537 @Description(shortDefinition = "Additional identifier for the plan definition", formalDefinition = "A formal identifier that is used to identify this plan definition when it is represented in other formats, or referenced in a specification, model, design or an instance.") 6538 protected List<Identifier> identifier; 6539 6540 /** 6541 * An explanatory or alternate title for the plan definition giving additional 6542 * information about its content. 6543 */ 6544 @Child(name = "subtitle", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 6545 @Description(shortDefinition = "Subordinate title of the plan definition", formalDefinition = "An explanatory or alternate title for the plan definition giving additional information about its content.") 6546 protected StringType subtitle; 6547 6548 /** 6549 * A high-level category for the plan definition that distinguishes the kinds of 6550 * systems that would be interested in the plan definition. 6551 */ 6552 @Child(name = "type", type = { CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 6553 @Description(shortDefinition = "order-set | clinical-protocol | eca-rule | workflow-definition", formalDefinition = "A high-level category for the plan definition that distinguishes the kinds of systems that would be interested in the plan definition.") 6554 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/plan-definition-type") 6555 protected CodeableConcept type; 6556 6557 /** 6558 * A code or group definition that describes the intended subject of the plan 6559 * definition. 6560 */ 6561 @Child(name = "subject", type = { CodeableConcept.class, 6562 Group.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 6563 @Description(shortDefinition = "Type of individual the plan definition is focused on", formalDefinition = "A code or group definition that describes the intended subject of the plan definition.") 6564 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/subject-type") 6565 protected Type subject; 6566 6567 /** 6568 * Explanation of why this plan definition is needed and why it has been 6569 * designed as it has. 6570 */ 6571 @Child(name = "purpose", type = { 6572 MarkdownType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 6573 @Description(shortDefinition = "Why this plan definition is defined", formalDefinition = "Explanation of why this plan definition is needed and why it has been designed as it has.") 6574 protected MarkdownType purpose; 6575 6576 /** 6577 * A detailed description of how the plan definition is used from a clinical 6578 * perspective. 6579 */ 6580 @Child(name = "usage", type = { StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 6581 @Description(shortDefinition = "Describes the clinical usage of the plan", formalDefinition = "A detailed description of how the plan definition is used from a clinical perspective.") 6582 protected StringType usage; 6583 6584 /** 6585 * A copyright statement relating to the plan definition and/or its contents. 6586 * Copyright statements are generally legal restrictions on the use and 6587 * publishing of the plan definition. 6588 */ 6589 @Child(name = "copyright", type = { 6590 MarkdownType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 6591 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the plan definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the plan definition.") 6592 protected MarkdownType copyright; 6593 6594 /** 6595 * The date on which the resource content was approved by the publisher. 6596 * Approval happens once when the content is officially approved for usage. 6597 */ 6598 @Child(name = "approvalDate", type = { 6599 DateType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 6600 @Description(shortDefinition = "When the plan definition was approved by publisher", formalDefinition = "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.") 6601 protected DateType approvalDate; 6602 6603 /** 6604 * The date on which the resource content was last reviewed. Review happens 6605 * periodically after approval but does not change the original approval date. 6606 */ 6607 @Child(name = "lastReviewDate", type = { 6608 DateType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 6609 @Description(shortDefinition = "When the plan definition was last reviewed", formalDefinition = "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.") 6610 protected DateType lastReviewDate; 6611 6612 /** 6613 * The period during which the plan definition content was or is planned to be 6614 * in active use. 6615 */ 6616 @Child(name = "effectivePeriod", type = { 6617 Period.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 6618 @Description(shortDefinition = "When the plan definition is expected to be used", formalDefinition = "The period during which the plan definition content was or is planned to be in active use.") 6619 protected Period effectivePeriod; 6620 6621 /** 6622 * Descriptive topics related to the content of the plan definition. Topics 6623 * provide a high-level categorization of the definition that can be useful for 6624 * filtering and searching. 6625 */ 6626 @Child(name = "topic", type = { 6627 CodeableConcept.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6628 @Description(shortDefinition = "E.g. Education, Treatment, Assessment", formalDefinition = "Descriptive topics related to the content of the plan definition. Topics provide a high-level categorization of the definition that can be useful for filtering and searching.") 6629 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/definition-topic") 6630 protected List<CodeableConcept> topic; 6631 6632 /** 6633 * An individiual or organization primarily involved in the creation and 6634 * maintenance of the content. 6635 */ 6636 @Child(name = "author", type = { 6637 ContactDetail.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6638 @Description(shortDefinition = "Who authored the content", formalDefinition = "An individiual or organization primarily involved in the creation and maintenance of the content.") 6639 protected List<ContactDetail> author; 6640 6641 /** 6642 * An individual or organization primarily responsible for internal coherence of 6643 * the content. 6644 */ 6645 @Child(name = "editor", type = { 6646 ContactDetail.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6647 @Description(shortDefinition = "Who edited the content", formalDefinition = "An individual or organization primarily responsible for internal coherence of the content.") 6648 protected List<ContactDetail> editor; 6649 6650 /** 6651 * An individual or organization primarily responsible for review of some aspect 6652 * of the content. 6653 */ 6654 @Child(name = "reviewer", type = { 6655 ContactDetail.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6656 @Description(shortDefinition = "Who reviewed the content", formalDefinition = "An individual or organization primarily responsible for review of some aspect of the content.") 6657 protected List<ContactDetail> reviewer; 6658 6659 /** 6660 * An individual or organization responsible for officially endorsing the 6661 * content for use in some setting. 6662 */ 6663 @Child(name = "endorser", type = { 6664 ContactDetail.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6665 @Description(shortDefinition = "Who endorsed the content", formalDefinition = "An individual or organization responsible for officially endorsing the content for use in some setting.") 6666 protected List<ContactDetail> endorser; 6667 6668 /** 6669 * Related artifacts such as additional documentation, justification, or 6670 * bibliographic references. 6671 */ 6672 @Child(name = "relatedArtifact", type = { 6673 RelatedArtifact.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6674 @Description(shortDefinition = "Additional documentation, citations", formalDefinition = "Related artifacts such as additional documentation, justification, or bibliographic references.") 6675 protected List<RelatedArtifact> relatedArtifact; 6676 6677 /** 6678 * A reference to a Library resource containing any formal logic used by the 6679 * plan definition. 6680 */ 6681 @Child(name = "library", type = { 6682 CanonicalType.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6683 @Description(shortDefinition = "Logic used by the plan definition", formalDefinition = "A reference to a Library resource containing any formal logic used by the plan definition.") 6684 protected List<CanonicalType> library; 6685 6686 /** 6687 * Goals that describe what the activities within the plan are intended to 6688 * achieve. For example, weight loss, restoring an activity of daily living, 6689 * obtaining herd immunity via immunization, meeting a process improvement 6690 * objective, etc. 6691 */ 6692 @Child(name = "goal", type = {}, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6693 @Description(shortDefinition = "What the plan is trying to accomplish", formalDefinition = "Goals that describe what the activities within the plan are intended to achieve. For example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc.") 6694 protected List<PlanDefinitionGoalComponent> goal; 6695 6696 /** 6697 * An action or group of actions to be taken as part of the plan. 6698 */ 6699 @Child(name = "action", type = {}, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6700 @Description(shortDefinition = "Action defined by the plan", formalDefinition = "An action or group of actions to be taken as part of the plan.") 6701 protected List<PlanDefinitionActionComponent> action; 6702 6703 private static final long serialVersionUID = -1725695645L; 6704 6705 /** 6706 * Constructor 6707 */ 6708 public PlanDefinition() { 6709 super(); 6710 } 6711 6712 /** 6713 * Constructor 6714 */ 6715 public PlanDefinition(Enumeration<PublicationStatus> status) { 6716 super(); 6717 this.status = status; 6718 } 6719 6720 /** 6721 * @return {@link #url} (An absolute URI that is used to identify this plan 6722 * definition when it is referenced in a specification, model, design or 6723 * an instance; also called its canonical identifier. This SHOULD be 6724 * globally unique and SHOULD be a literal address at which at which an 6725 * authoritative instance of this plan definition is (or will be) 6726 * published. This URL can be the target of a canonical reference. It 6727 * SHALL remain the same when the plan definition is stored on different 6728 * servers.). This is the underlying object with id, value and 6729 * extensions. The accessor "getUrl" gives direct access to the value 6730 */ 6731 public UriType getUrlElement() { 6732 if (this.url == null) 6733 if (Configuration.errorOnAutoCreate()) 6734 throw new Error("Attempt to auto-create PlanDefinition.url"); 6735 else if (Configuration.doAutoCreate()) 6736 this.url = new UriType(); // bb 6737 return this.url; 6738 } 6739 6740 public boolean hasUrlElement() { 6741 return this.url != null && !this.url.isEmpty(); 6742 } 6743 6744 public boolean hasUrl() { 6745 return this.url != null && !this.url.isEmpty(); 6746 } 6747 6748 /** 6749 * @param value {@link #url} (An absolute URI that is used to identify this plan 6750 * definition when it is referenced in a specification, model, 6751 * design or an instance; also called its canonical identifier. 6752 * This SHOULD be globally unique and SHOULD be a literal address 6753 * at which at which an authoritative instance of this plan 6754 * definition is (or will be) published. This URL can be the target 6755 * of a canonical reference. It SHALL remain the same when the plan 6756 * definition is stored on different servers.). This is the 6757 * underlying object with id, value and extensions. The accessor 6758 * "getUrl" gives direct access to the value 6759 */ 6760 public PlanDefinition setUrlElement(UriType value) { 6761 this.url = value; 6762 return this; 6763 } 6764 6765 /** 6766 * @return An absolute URI that is used to identify this plan definition when it 6767 * is referenced in a specification, model, design or an instance; also 6768 * called its canonical identifier. This SHOULD be globally unique and 6769 * SHOULD be a literal address at which at which an authoritative 6770 * instance of this plan definition is (or will be) published. This URL 6771 * can be the target of a canonical reference. It SHALL remain the same 6772 * when the plan definition is stored on different servers. 6773 */ 6774 public String getUrl() { 6775 return this.url == null ? null : this.url.getValue(); 6776 } 6777 6778 /** 6779 * @param value An absolute URI that is used to identify this plan definition 6780 * when it is referenced in a specification, model, design or an 6781 * instance; also called its canonical identifier. This SHOULD be 6782 * globally unique and SHOULD be a literal address at which at 6783 * which an authoritative instance of this plan definition is (or 6784 * will be) published. This URL can be the target of a canonical 6785 * reference. It SHALL remain the same when the plan definition is 6786 * stored on different servers. 6787 */ 6788 public PlanDefinition setUrl(String value) { 6789 if (Utilities.noString(value)) 6790 this.url = null; 6791 else { 6792 if (this.url == null) 6793 this.url = new UriType(); 6794 this.url.setValue(value); 6795 } 6796 return this; 6797 } 6798 6799 /** 6800 * @return {@link #identifier} (A formal identifier that is used to identify 6801 * this plan definition when it is represented in other formats, or 6802 * referenced in a specification, model, design or an instance.) 6803 */ 6804 public List<Identifier> getIdentifier() { 6805 if (this.identifier == null) 6806 this.identifier = new ArrayList<Identifier>(); 6807 return this.identifier; 6808 } 6809 6810 /** 6811 * @return Returns a reference to <code>this</code> for easy method chaining 6812 */ 6813 public PlanDefinition setIdentifier(List<Identifier> theIdentifier) { 6814 this.identifier = theIdentifier; 6815 return this; 6816 } 6817 6818 public boolean hasIdentifier() { 6819 if (this.identifier == null) 6820 return false; 6821 for (Identifier item : this.identifier) 6822 if (!item.isEmpty()) 6823 return true; 6824 return false; 6825 } 6826 6827 public Identifier addIdentifier() { // 3 6828 Identifier t = new Identifier(); 6829 if (this.identifier == null) 6830 this.identifier = new ArrayList<Identifier>(); 6831 this.identifier.add(t); 6832 return t; 6833 } 6834 6835 public PlanDefinition addIdentifier(Identifier t) { // 3 6836 if (t == null) 6837 return this; 6838 if (this.identifier == null) 6839 this.identifier = new ArrayList<Identifier>(); 6840 this.identifier.add(t); 6841 return this; 6842 } 6843 6844 /** 6845 * @return The first repetition of repeating field {@link #identifier}, creating 6846 * it if it does not already exist 6847 */ 6848 public Identifier getIdentifierFirstRep() { 6849 if (getIdentifier().isEmpty()) { 6850 addIdentifier(); 6851 } 6852 return getIdentifier().get(0); 6853 } 6854 6855 /** 6856 * @return {@link #version} (The identifier that is used to identify this 6857 * version of the plan definition when it is referenced in a 6858 * specification, model, design or instance. This is an arbitrary value 6859 * managed by the plan definition author and is not expected to be 6860 * globally unique. For example, it might be a timestamp (e.g. yyyymmdd) 6861 * if a managed version is not available. There is also no expectation 6862 * that versions can be placed in a lexicographical sequence. To provide 6863 * a version consistent with the Decision Support Service specification, 6864 * use the format Major.Minor.Revision (e.g. 1.0.0). For more 6865 * information on versioning knowledge assets, refer to the Decision 6866 * Support Service specification. Note that a version is required for 6867 * non-experimental active artifacts.). This is the underlying object 6868 * with id, value and extensions. The accessor "getVersion" gives direct 6869 * access to the value 6870 */ 6871 public StringType getVersionElement() { 6872 if (this.version == null) 6873 if (Configuration.errorOnAutoCreate()) 6874 throw new Error("Attempt to auto-create PlanDefinition.version"); 6875 else if (Configuration.doAutoCreate()) 6876 this.version = new StringType(); // bb 6877 return this.version; 6878 } 6879 6880 public boolean hasVersionElement() { 6881 return this.version != null && !this.version.isEmpty(); 6882 } 6883 6884 public boolean hasVersion() { 6885 return this.version != null && !this.version.isEmpty(); 6886 } 6887 6888 /** 6889 * @param value {@link #version} (The identifier that is used to identify this 6890 * version of the plan definition when it is referenced in a 6891 * specification, model, design or instance. This is an arbitrary 6892 * value managed by the plan definition author and is not expected 6893 * to be globally unique. For example, it might be a timestamp 6894 * (e.g. yyyymmdd) if a managed version is not available. There is 6895 * also no expectation that versions can be placed in a 6896 * lexicographical sequence. To provide a version consistent with 6897 * the Decision Support Service specification, use the format 6898 * Major.Minor.Revision (e.g. 1.0.0). For more information on 6899 * versioning knowledge assets, refer to the Decision Support 6900 * Service specification. Note that a version is required for 6901 * non-experimental active artifacts.). This is the underlying 6902 * object with id, value and extensions. The accessor "getVersion" 6903 * gives direct access to the value 6904 */ 6905 public PlanDefinition setVersionElement(StringType value) { 6906 this.version = value; 6907 return this; 6908 } 6909 6910 /** 6911 * @return The identifier that is used to identify this version of the plan 6912 * definition when it is referenced in a specification, model, design or 6913 * instance. This is an arbitrary value managed by the plan definition 6914 * author and is not expected to be globally unique. For example, it 6915 * might be a timestamp (e.g. yyyymmdd) if a managed version is not 6916 * available. There is also no expectation that versions can be placed 6917 * in a lexicographical sequence. To provide a version consistent with 6918 * the Decision Support Service specification, use the format 6919 * Major.Minor.Revision (e.g. 1.0.0). For more information on versioning 6920 * knowledge assets, refer to the Decision Support Service 6921 * specification. Note that a version is required for non-experimental 6922 * active artifacts. 6923 */ 6924 public String getVersion() { 6925 return this.version == null ? null : this.version.getValue(); 6926 } 6927 6928 /** 6929 * @param value The identifier that is used to identify this version of the plan 6930 * definition when it is referenced in a specification, model, 6931 * design or instance. This is an arbitrary value managed by the 6932 * plan definition author and is not expected to be globally 6933 * unique. For example, it might be a timestamp (e.g. yyyymmdd) if 6934 * a managed version is not available. There is also no expectation 6935 * that versions can be placed in a lexicographical sequence. To 6936 * provide a version consistent with the Decision Support Service 6937 * specification, use the format Major.Minor.Revision (e.g. 1.0.0). 6938 * For more information on versioning knowledge assets, refer to 6939 * the Decision Support Service specification. Note that a version 6940 * is required for non-experimental active artifacts. 6941 */ 6942 public PlanDefinition setVersion(String value) { 6943 if (Utilities.noString(value)) 6944 this.version = null; 6945 else { 6946 if (this.version == null) 6947 this.version = new StringType(); 6948 this.version.setValue(value); 6949 } 6950 return this; 6951 } 6952 6953 /** 6954 * @return {@link #name} (A natural language name identifying the plan 6955 * definition. This name should be usable as an identifier for the 6956 * module by machine processing applications such as code generation.). 6957 * This is the underlying object with id, value and extensions. The 6958 * accessor "getName" gives direct access to the value 6959 */ 6960 public StringType getNameElement() { 6961 if (this.name == null) 6962 if (Configuration.errorOnAutoCreate()) 6963 throw new Error("Attempt to auto-create PlanDefinition.name"); 6964 else if (Configuration.doAutoCreate()) 6965 this.name = new StringType(); // bb 6966 return this.name; 6967 } 6968 6969 public boolean hasNameElement() { 6970 return this.name != null && !this.name.isEmpty(); 6971 } 6972 6973 public boolean hasName() { 6974 return this.name != null && !this.name.isEmpty(); 6975 } 6976 6977 /** 6978 * @param value {@link #name} (A natural language name identifying the plan 6979 * definition. This name should be usable as an identifier for the 6980 * module by machine processing applications such as code 6981 * generation.). This is the underlying object with id, value and 6982 * extensions. The accessor "getName" gives direct access to the 6983 * value 6984 */ 6985 public PlanDefinition setNameElement(StringType value) { 6986 this.name = value; 6987 return this; 6988 } 6989 6990 /** 6991 * @return A natural language name identifying the plan definition. This name 6992 * should be usable as an identifier for the module by machine 6993 * processing applications such as code generation. 6994 */ 6995 public String getName() { 6996 return this.name == null ? null : this.name.getValue(); 6997 } 6998 6999 /** 7000 * @param value A natural language name identifying the plan definition. This 7001 * name should be usable as an identifier for the module by machine 7002 * processing applications such as code generation. 7003 */ 7004 public PlanDefinition setName(String value) { 7005 if (Utilities.noString(value)) 7006 this.name = null; 7007 else { 7008 if (this.name == null) 7009 this.name = new StringType(); 7010 this.name.setValue(value); 7011 } 7012 return this; 7013 } 7014 7015 /** 7016 * @return {@link #title} (A short, descriptive, user-friendly title for the 7017 * plan definition.). This is the underlying object with id, value and 7018 * extensions. The accessor "getTitle" gives direct access to the value 7019 */ 7020 public StringType getTitleElement() { 7021 if (this.title == null) 7022 if (Configuration.errorOnAutoCreate()) 7023 throw new Error("Attempt to auto-create PlanDefinition.title"); 7024 else if (Configuration.doAutoCreate()) 7025 this.title = new StringType(); // bb 7026 return this.title; 7027 } 7028 7029 public boolean hasTitleElement() { 7030 return this.title != null && !this.title.isEmpty(); 7031 } 7032 7033 public boolean hasTitle() { 7034 return this.title != null && !this.title.isEmpty(); 7035 } 7036 7037 /** 7038 * @param value {@link #title} (A short, descriptive, user-friendly title for 7039 * the plan definition.). This is the underlying object with id, 7040 * value and extensions. The accessor "getTitle" gives direct 7041 * access to the value 7042 */ 7043 public PlanDefinition setTitleElement(StringType value) { 7044 this.title = value; 7045 return this; 7046 } 7047 7048 /** 7049 * @return A short, descriptive, user-friendly title for the plan definition. 7050 */ 7051 public String getTitle() { 7052 return this.title == null ? null : this.title.getValue(); 7053 } 7054 7055 /** 7056 * @param value A short, descriptive, user-friendly title for the plan 7057 * definition. 7058 */ 7059 public PlanDefinition setTitle(String value) { 7060 if (Utilities.noString(value)) 7061 this.title = null; 7062 else { 7063 if (this.title == null) 7064 this.title = new StringType(); 7065 this.title.setValue(value); 7066 } 7067 return this; 7068 } 7069 7070 /** 7071 * @return {@link #subtitle} (An explanatory or alternate title for the plan 7072 * definition giving additional information about its content.). This is 7073 * the underlying object with id, value and extensions. The accessor 7074 * "getSubtitle" gives direct access to the value 7075 */ 7076 public StringType getSubtitleElement() { 7077 if (this.subtitle == null) 7078 if (Configuration.errorOnAutoCreate()) 7079 throw new Error("Attempt to auto-create PlanDefinition.subtitle"); 7080 else if (Configuration.doAutoCreate()) 7081 this.subtitle = new StringType(); // bb 7082 return this.subtitle; 7083 } 7084 7085 public boolean hasSubtitleElement() { 7086 return this.subtitle != null && !this.subtitle.isEmpty(); 7087 } 7088 7089 public boolean hasSubtitle() { 7090 return this.subtitle != null && !this.subtitle.isEmpty(); 7091 } 7092 7093 /** 7094 * @param value {@link #subtitle} (An explanatory or alternate title for the 7095 * plan definition giving additional information about its 7096 * content.). This is the underlying object with id, value and 7097 * extensions. The accessor "getSubtitle" gives direct access to 7098 * the value 7099 */ 7100 public PlanDefinition setSubtitleElement(StringType value) { 7101 this.subtitle = value; 7102 return this; 7103 } 7104 7105 /** 7106 * @return An explanatory or alternate title for the plan definition giving 7107 * additional information about its content. 7108 */ 7109 public String getSubtitle() { 7110 return this.subtitle == null ? null : this.subtitle.getValue(); 7111 } 7112 7113 /** 7114 * @param value An explanatory or alternate title for the plan definition giving 7115 * additional information about its content. 7116 */ 7117 public PlanDefinition setSubtitle(String value) { 7118 if (Utilities.noString(value)) 7119 this.subtitle = null; 7120 else { 7121 if (this.subtitle == null) 7122 this.subtitle = new StringType(); 7123 this.subtitle.setValue(value); 7124 } 7125 return this; 7126 } 7127 7128 /** 7129 * @return {@link #type} (A high-level category for the plan definition that 7130 * distinguishes the kinds of systems that would be interested in the 7131 * plan definition.) 7132 */ 7133 public CodeableConcept getType() { 7134 if (this.type == null) 7135 if (Configuration.errorOnAutoCreate()) 7136 throw new Error("Attempt to auto-create PlanDefinition.type"); 7137 else if (Configuration.doAutoCreate()) 7138 this.type = new CodeableConcept(); // cc 7139 return this.type; 7140 } 7141 7142 public boolean hasType() { 7143 return this.type != null && !this.type.isEmpty(); 7144 } 7145 7146 /** 7147 * @param value {@link #type} (A high-level category for the plan definition 7148 * that distinguishes the kinds of systems that would be interested 7149 * in the plan definition.) 7150 */ 7151 public PlanDefinition setType(CodeableConcept value) { 7152 this.type = value; 7153 return this; 7154 } 7155 7156 /** 7157 * @return {@link #status} (The status of this plan definition. Enables tracking 7158 * the life-cycle of the content.). This is the underlying object with 7159 * id, value and extensions. The accessor "getStatus" gives direct 7160 * access to the value 7161 */ 7162 public Enumeration<PublicationStatus> getStatusElement() { 7163 if (this.status == null) 7164 if (Configuration.errorOnAutoCreate()) 7165 throw new Error("Attempt to auto-create PlanDefinition.status"); 7166 else if (Configuration.doAutoCreate()) 7167 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 7168 return this.status; 7169 } 7170 7171 public boolean hasStatusElement() { 7172 return this.status != null && !this.status.isEmpty(); 7173 } 7174 7175 public boolean hasStatus() { 7176 return this.status != null && !this.status.isEmpty(); 7177 } 7178 7179 /** 7180 * @param value {@link #status} (The status of this plan definition. Enables 7181 * tracking the life-cycle of the content.). This is the underlying 7182 * object with id, value and extensions. The accessor "getStatus" 7183 * gives direct access to the value 7184 */ 7185 public PlanDefinition setStatusElement(Enumeration<PublicationStatus> value) { 7186 this.status = value; 7187 return this; 7188 } 7189 7190 /** 7191 * @return The status of this plan definition. Enables tracking the life-cycle 7192 * of the content. 7193 */ 7194 public PublicationStatus getStatus() { 7195 return this.status == null ? null : this.status.getValue(); 7196 } 7197 7198 /** 7199 * @param value The status of this plan definition. Enables tracking the 7200 * life-cycle of the content. 7201 */ 7202 public PlanDefinition setStatus(PublicationStatus value) { 7203 if (this.status == null) 7204 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 7205 this.status.setValue(value); 7206 return this; 7207 } 7208 7209 /** 7210 * @return {@link #experimental} (A Boolean value to indicate that this plan 7211 * definition is authored for testing purposes (or 7212 * education/evaluation/marketing) and is not intended to be used for 7213 * genuine usage.). This is the underlying object with id, value and 7214 * extensions. The accessor "getExperimental" gives direct access to the 7215 * value 7216 */ 7217 public BooleanType getExperimentalElement() { 7218 if (this.experimental == null) 7219 if (Configuration.errorOnAutoCreate()) 7220 throw new Error("Attempt to auto-create PlanDefinition.experimental"); 7221 else if (Configuration.doAutoCreate()) 7222 this.experimental = new BooleanType(); // bb 7223 return this.experimental; 7224 } 7225 7226 public boolean hasExperimentalElement() { 7227 return this.experimental != null && !this.experimental.isEmpty(); 7228 } 7229 7230 public boolean hasExperimental() { 7231 return this.experimental != null && !this.experimental.isEmpty(); 7232 } 7233 7234 /** 7235 * @param value {@link #experimental} (A Boolean value to indicate that this 7236 * plan definition is authored for testing purposes (or 7237 * education/evaluation/marketing) and is not intended to be used 7238 * for genuine usage.). This is the underlying object with id, 7239 * value and extensions. The accessor "getExperimental" gives 7240 * direct access to the value 7241 */ 7242 public PlanDefinition setExperimentalElement(BooleanType value) { 7243 this.experimental = value; 7244 return this; 7245 } 7246 7247 /** 7248 * @return A Boolean value to indicate that this plan definition is authored for 7249 * testing purposes (or education/evaluation/marketing) and is not 7250 * intended to be used for genuine usage. 7251 */ 7252 public boolean getExperimental() { 7253 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 7254 } 7255 7256 /** 7257 * @param value A Boolean value to indicate that this plan definition is 7258 * authored for testing purposes (or 7259 * education/evaluation/marketing) and is not intended to be used 7260 * for genuine usage. 7261 */ 7262 public PlanDefinition setExperimental(boolean value) { 7263 if (this.experimental == null) 7264 this.experimental = new BooleanType(); 7265 this.experimental.setValue(value); 7266 return this; 7267 } 7268 7269 /** 7270 * @return {@link #subject} (A code or group definition that describes the 7271 * intended subject of the plan definition.) 7272 */ 7273 public Type getSubject() { 7274 return this.subject; 7275 } 7276 7277 /** 7278 * @return {@link #subject} (A code or group definition that describes the 7279 * intended subject of the plan definition.) 7280 */ 7281 public CodeableConcept getSubjectCodeableConcept() throws FHIRException { 7282 if (this.subject == null) 7283 this.subject = new CodeableConcept(); 7284 if (!(this.subject instanceof CodeableConcept)) 7285 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 7286 + this.subject.getClass().getName() + " was encountered"); 7287 return (CodeableConcept) this.subject; 7288 } 7289 7290 public boolean hasSubjectCodeableConcept() { 7291 return this.subject instanceof CodeableConcept; 7292 } 7293 7294 /** 7295 * @return {@link #subject} (A code or group definition that describes the 7296 * intended subject of the plan definition.) 7297 */ 7298 public Reference getSubjectReference() throws FHIRException { 7299 if (this.subject == null) 7300 this.subject = new Reference(); 7301 if (!(this.subject instanceof Reference)) 7302 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.subject.getClass().getName() 7303 + " was encountered"); 7304 return (Reference) this.subject; 7305 } 7306 7307 public boolean hasSubjectReference() { 7308 return this.subject instanceof Reference; 7309 } 7310 7311 public boolean hasSubject() { 7312 return this.subject != null && !this.subject.isEmpty(); 7313 } 7314 7315 /** 7316 * @param value {@link #subject} (A code or group definition that describes the 7317 * intended subject of the plan definition.) 7318 */ 7319 public PlanDefinition setSubject(Type value) { 7320 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 7321 throw new Error("Not the right type for PlanDefinition.subject[x]: " + value.fhirType()); 7322 this.subject = value; 7323 return this; 7324 } 7325 7326 /** 7327 * @return {@link #date} (The date (and optionally time) when the plan 7328 * definition was published. The date must change when the business 7329 * version changes and it must change if the status code changes. In 7330 * addition, it should change when the substantive content of the plan 7331 * definition changes.). This is the underlying object with id, value 7332 * and extensions. The accessor "getDate" gives direct access to the 7333 * value 7334 */ 7335 public DateTimeType getDateElement() { 7336 if (this.date == null) 7337 if (Configuration.errorOnAutoCreate()) 7338 throw new Error("Attempt to auto-create PlanDefinition.date"); 7339 else if (Configuration.doAutoCreate()) 7340 this.date = new DateTimeType(); // bb 7341 return this.date; 7342 } 7343 7344 public boolean hasDateElement() { 7345 return this.date != null && !this.date.isEmpty(); 7346 } 7347 7348 public boolean hasDate() { 7349 return this.date != null && !this.date.isEmpty(); 7350 } 7351 7352 /** 7353 * @param value {@link #date} (The date (and optionally time) when the plan 7354 * definition was published. The date must change when the business 7355 * version changes and it must change if the status code changes. 7356 * In addition, it should change when the substantive content of 7357 * the plan definition changes.). This is the underlying object 7358 * with id, value and extensions. The accessor "getDate" gives 7359 * direct access to the value 7360 */ 7361 public PlanDefinition setDateElement(DateTimeType value) { 7362 this.date = value; 7363 return this; 7364 } 7365 7366 /** 7367 * @return The date (and optionally time) when the plan definition was 7368 * published. The date must change when the business version changes and 7369 * it must change if the status code changes. In addition, it should 7370 * change when the substantive content of the plan definition changes. 7371 */ 7372 public Date getDate() { 7373 return this.date == null ? null : this.date.getValue(); 7374 } 7375 7376 /** 7377 * @param value The date (and optionally time) when the plan definition was 7378 * published. The date must change when the business version 7379 * changes and it must change if the status code changes. In 7380 * addition, it should change when the substantive content of the 7381 * plan definition changes. 7382 */ 7383 public PlanDefinition setDate(Date value) { 7384 if (value == null) 7385 this.date = null; 7386 else { 7387 if (this.date == null) 7388 this.date = new DateTimeType(); 7389 this.date.setValue(value); 7390 } 7391 return this; 7392 } 7393 7394 /** 7395 * @return {@link #publisher} (The name of the organization or individual that 7396 * published the plan definition.). This is the underlying object with 7397 * id, value and extensions. The accessor "getPublisher" gives direct 7398 * access to the value 7399 */ 7400 public StringType getPublisherElement() { 7401 if (this.publisher == null) 7402 if (Configuration.errorOnAutoCreate()) 7403 throw new Error("Attempt to auto-create PlanDefinition.publisher"); 7404 else if (Configuration.doAutoCreate()) 7405 this.publisher = new StringType(); // bb 7406 return this.publisher; 7407 } 7408 7409 public boolean hasPublisherElement() { 7410 return this.publisher != null && !this.publisher.isEmpty(); 7411 } 7412 7413 public boolean hasPublisher() { 7414 return this.publisher != null && !this.publisher.isEmpty(); 7415 } 7416 7417 /** 7418 * @param value {@link #publisher} (The name of the organization or individual 7419 * that published the plan definition.). This is the underlying 7420 * object with id, value and extensions. The accessor 7421 * "getPublisher" gives direct access to the value 7422 */ 7423 public PlanDefinition setPublisherElement(StringType value) { 7424 this.publisher = value; 7425 return this; 7426 } 7427 7428 /** 7429 * @return The name of the organization or individual that published the plan 7430 * definition. 7431 */ 7432 public String getPublisher() { 7433 return this.publisher == null ? null : this.publisher.getValue(); 7434 } 7435 7436 /** 7437 * @param value The name of the organization or individual that published the 7438 * plan definition. 7439 */ 7440 public PlanDefinition setPublisher(String value) { 7441 if (Utilities.noString(value)) 7442 this.publisher = null; 7443 else { 7444 if (this.publisher == null) 7445 this.publisher = new StringType(); 7446 this.publisher.setValue(value); 7447 } 7448 return this; 7449 } 7450 7451 /** 7452 * @return {@link #contact} (Contact details to assist a user in finding and 7453 * communicating with the publisher.) 7454 */ 7455 public List<ContactDetail> getContact() { 7456 if (this.contact == null) 7457 this.contact = new ArrayList<ContactDetail>(); 7458 return this.contact; 7459 } 7460 7461 /** 7462 * @return Returns a reference to <code>this</code> for easy method chaining 7463 */ 7464 public PlanDefinition setContact(List<ContactDetail> theContact) { 7465 this.contact = theContact; 7466 return this; 7467 } 7468 7469 public boolean hasContact() { 7470 if (this.contact == null) 7471 return false; 7472 for (ContactDetail item : this.contact) 7473 if (!item.isEmpty()) 7474 return true; 7475 return false; 7476 } 7477 7478 public ContactDetail addContact() { // 3 7479 ContactDetail t = new ContactDetail(); 7480 if (this.contact == null) 7481 this.contact = new ArrayList<ContactDetail>(); 7482 this.contact.add(t); 7483 return t; 7484 } 7485 7486 public PlanDefinition addContact(ContactDetail t) { // 3 7487 if (t == null) 7488 return this; 7489 if (this.contact == null) 7490 this.contact = new ArrayList<ContactDetail>(); 7491 this.contact.add(t); 7492 return this; 7493 } 7494 7495 /** 7496 * @return The first repetition of repeating field {@link #contact}, creating it 7497 * if it does not already exist 7498 */ 7499 public ContactDetail getContactFirstRep() { 7500 if (getContact().isEmpty()) { 7501 addContact(); 7502 } 7503 return getContact().get(0); 7504 } 7505 7506 /** 7507 * @return {@link #description} (A free text natural language description of the 7508 * plan definition from a consumer's perspective.). This is the 7509 * underlying object with id, value and extensions. The accessor 7510 * "getDescription" gives direct access to the value 7511 */ 7512 public MarkdownType getDescriptionElement() { 7513 if (this.description == null) 7514 if (Configuration.errorOnAutoCreate()) 7515 throw new Error("Attempt to auto-create PlanDefinition.description"); 7516 else if (Configuration.doAutoCreate()) 7517 this.description = new MarkdownType(); // bb 7518 return this.description; 7519 } 7520 7521 public boolean hasDescriptionElement() { 7522 return this.description != null && !this.description.isEmpty(); 7523 } 7524 7525 public boolean hasDescription() { 7526 return this.description != null && !this.description.isEmpty(); 7527 } 7528 7529 /** 7530 * @param value {@link #description} (A free text natural language description 7531 * of the plan definition from a consumer's perspective.). This is 7532 * the underlying object with id, value and extensions. The 7533 * accessor "getDescription" gives direct access to the value 7534 */ 7535 public PlanDefinition setDescriptionElement(MarkdownType value) { 7536 this.description = value; 7537 return this; 7538 } 7539 7540 /** 7541 * @return A free text natural language description of the plan definition from 7542 * a consumer's perspective. 7543 */ 7544 public String getDescription() { 7545 return this.description == null ? null : this.description.getValue(); 7546 } 7547 7548 /** 7549 * @param value A free text natural language description of the plan definition 7550 * from a consumer's perspective. 7551 */ 7552 public PlanDefinition setDescription(String value) { 7553 if (value == null) 7554 this.description = null; 7555 else { 7556 if (this.description == null) 7557 this.description = new MarkdownType(); 7558 this.description.setValue(value); 7559 } 7560 return this; 7561 } 7562 7563 /** 7564 * @return {@link #useContext} (The content was developed with a focus and 7565 * intent of supporting the contexts that are listed. These contexts may 7566 * be general categories (gender, age, ...) or may be references to 7567 * specific programs (insurance plans, studies, ...) and may be used to 7568 * assist with indexing and searching for appropriate plan definition 7569 * instances.) 7570 */ 7571 public List<UsageContext> getUseContext() { 7572 if (this.useContext == null) 7573 this.useContext = new ArrayList<UsageContext>(); 7574 return this.useContext; 7575 } 7576 7577 /** 7578 * @return Returns a reference to <code>this</code> for easy method chaining 7579 */ 7580 public PlanDefinition setUseContext(List<UsageContext> theUseContext) { 7581 this.useContext = theUseContext; 7582 return this; 7583 } 7584 7585 public boolean hasUseContext() { 7586 if (this.useContext == null) 7587 return false; 7588 for (UsageContext item : this.useContext) 7589 if (!item.isEmpty()) 7590 return true; 7591 return false; 7592 } 7593 7594 public UsageContext addUseContext() { // 3 7595 UsageContext t = new UsageContext(); 7596 if (this.useContext == null) 7597 this.useContext = new ArrayList<UsageContext>(); 7598 this.useContext.add(t); 7599 return t; 7600 } 7601 7602 public PlanDefinition addUseContext(UsageContext t) { // 3 7603 if (t == null) 7604 return this; 7605 if (this.useContext == null) 7606 this.useContext = new ArrayList<UsageContext>(); 7607 this.useContext.add(t); 7608 return this; 7609 } 7610 7611 /** 7612 * @return The first repetition of repeating field {@link #useContext}, creating 7613 * it if it does not already exist 7614 */ 7615 public UsageContext getUseContextFirstRep() { 7616 if (getUseContext().isEmpty()) { 7617 addUseContext(); 7618 } 7619 return getUseContext().get(0); 7620 } 7621 7622 /** 7623 * @return {@link #jurisdiction} (A legal or geographic region in which the plan 7624 * definition is intended to be used.) 7625 */ 7626 public List<CodeableConcept> getJurisdiction() { 7627 if (this.jurisdiction == null) 7628 this.jurisdiction = new ArrayList<CodeableConcept>(); 7629 return this.jurisdiction; 7630 } 7631 7632 /** 7633 * @return Returns a reference to <code>this</code> for easy method chaining 7634 */ 7635 public PlanDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 7636 this.jurisdiction = theJurisdiction; 7637 return this; 7638 } 7639 7640 public boolean hasJurisdiction() { 7641 if (this.jurisdiction == null) 7642 return false; 7643 for (CodeableConcept item : this.jurisdiction) 7644 if (!item.isEmpty()) 7645 return true; 7646 return false; 7647 } 7648 7649 public CodeableConcept addJurisdiction() { // 3 7650 CodeableConcept t = new CodeableConcept(); 7651 if (this.jurisdiction == null) 7652 this.jurisdiction = new ArrayList<CodeableConcept>(); 7653 this.jurisdiction.add(t); 7654 return t; 7655 } 7656 7657 public PlanDefinition addJurisdiction(CodeableConcept t) { // 3 7658 if (t == null) 7659 return this; 7660 if (this.jurisdiction == null) 7661 this.jurisdiction = new ArrayList<CodeableConcept>(); 7662 this.jurisdiction.add(t); 7663 return this; 7664 } 7665 7666 /** 7667 * @return The first repetition of repeating field {@link #jurisdiction}, 7668 * creating it if it does not already exist 7669 */ 7670 public CodeableConcept getJurisdictionFirstRep() { 7671 if (getJurisdiction().isEmpty()) { 7672 addJurisdiction(); 7673 } 7674 return getJurisdiction().get(0); 7675 } 7676 7677 /** 7678 * @return {@link #purpose} (Explanation of why this plan definition is needed 7679 * and why it has been designed as it has.). This is the underlying 7680 * object with id, value and extensions. The accessor "getPurpose" gives 7681 * direct access to the value 7682 */ 7683 public MarkdownType getPurposeElement() { 7684 if (this.purpose == null) 7685 if (Configuration.errorOnAutoCreate()) 7686 throw new Error("Attempt to auto-create PlanDefinition.purpose"); 7687 else if (Configuration.doAutoCreate()) 7688 this.purpose = new MarkdownType(); // bb 7689 return this.purpose; 7690 } 7691 7692 public boolean hasPurposeElement() { 7693 return this.purpose != null && !this.purpose.isEmpty(); 7694 } 7695 7696 public boolean hasPurpose() { 7697 return this.purpose != null && !this.purpose.isEmpty(); 7698 } 7699 7700 /** 7701 * @param value {@link #purpose} (Explanation of why this plan definition is 7702 * needed and why it has been designed as it has.). This is the 7703 * underlying object with id, value and extensions. The accessor 7704 * "getPurpose" gives direct access to the value 7705 */ 7706 public PlanDefinition setPurposeElement(MarkdownType value) { 7707 this.purpose = value; 7708 return this; 7709 } 7710 7711 /** 7712 * @return Explanation of why this plan definition is needed and why it has been 7713 * designed as it has. 7714 */ 7715 public String getPurpose() { 7716 return this.purpose == null ? null : this.purpose.getValue(); 7717 } 7718 7719 /** 7720 * @param value Explanation of why this plan definition is needed and why it has 7721 * been designed as it has. 7722 */ 7723 public PlanDefinition setPurpose(String value) { 7724 if (value == null) 7725 this.purpose = null; 7726 else { 7727 if (this.purpose == null) 7728 this.purpose = new MarkdownType(); 7729 this.purpose.setValue(value); 7730 } 7731 return this; 7732 } 7733 7734 /** 7735 * @return {@link #usage} (A detailed description of how the plan definition is 7736 * used from a clinical perspective.). This is the underlying object 7737 * with id, value and extensions. The accessor "getUsage" gives direct 7738 * access to the value 7739 */ 7740 public StringType getUsageElement() { 7741 if (this.usage == null) 7742 if (Configuration.errorOnAutoCreate()) 7743 throw new Error("Attempt to auto-create PlanDefinition.usage"); 7744 else if (Configuration.doAutoCreate()) 7745 this.usage = new StringType(); // bb 7746 return this.usage; 7747 } 7748 7749 public boolean hasUsageElement() { 7750 return this.usage != null && !this.usage.isEmpty(); 7751 } 7752 7753 public boolean hasUsage() { 7754 return this.usage != null && !this.usage.isEmpty(); 7755 } 7756 7757 /** 7758 * @param value {@link #usage} (A detailed description of how the plan 7759 * definition is used from a clinical perspective.). This is the 7760 * underlying object with id, value and extensions. The accessor 7761 * "getUsage" gives direct access to the value 7762 */ 7763 public PlanDefinition setUsageElement(StringType value) { 7764 this.usage = value; 7765 return this; 7766 } 7767 7768 /** 7769 * @return A detailed description of how the plan definition is used from a 7770 * clinical perspective. 7771 */ 7772 public String getUsage() { 7773 return this.usage == null ? null : this.usage.getValue(); 7774 } 7775 7776 /** 7777 * @param value A detailed description of how the plan definition is used from a 7778 * clinical perspective. 7779 */ 7780 public PlanDefinition setUsage(String value) { 7781 if (Utilities.noString(value)) 7782 this.usage = null; 7783 else { 7784 if (this.usage == null) 7785 this.usage = new StringType(); 7786 this.usage.setValue(value); 7787 } 7788 return this; 7789 } 7790 7791 /** 7792 * @return {@link #copyright} (A copyright statement relating to the plan 7793 * definition and/or its contents. Copyright statements are generally 7794 * legal restrictions on the use and publishing of the plan 7795 * definition.). This is the underlying object with id, value and 7796 * extensions. The accessor "getCopyright" gives direct access to the 7797 * value 7798 */ 7799 public MarkdownType getCopyrightElement() { 7800 if (this.copyright == null) 7801 if (Configuration.errorOnAutoCreate()) 7802 throw new Error("Attempt to auto-create PlanDefinition.copyright"); 7803 else if (Configuration.doAutoCreate()) 7804 this.copyright = new MarkdownType(); // bb 7805 return this.copyright; 7806 } 7807 7808 public boolean hasCopyrightElement() { 7809 return this.copyright != null && !this.copyright.isEmpty(); 7810 } 7811 7812 public boolean hasCopyright() { 7813 return this.copyright != null && !this.copyright.isEmpty(); 7814 } 7815 7816 /** 7817 * @param value {@link #copyright} (A copyright statement relating to the plan 7818 * definition and/or its contents. Copyright statements are 7819 * generally legal restrictions on the use and publishing of the 7820 * plan definition.). This is the underlying object with id, value 7821 * and extensions. The accessor "getCopyright" gives direct access 7822 * to the value 7823 */ 7824 public PlanDefinition setCopyrightElement(MarkdownType value) { 7825 this.copyright = value; 7826 return this; 7827 } 7828 7829 /** 7830 * @return A copyright statement relating to the plan definition and/or its 7831 * contents. Copyright statements are generally legal restrictions on 7832 * the use and publishing of the plan definition. 7833 */ 7834 public String getCopyright() { 7835 return this.copyright == null ? null : this.copyright.getValue(); 7836 } 7837 7838 /** 7839 * @param value A copyright statement relating to the plan definition and/or its 7840 * contents. Copyright statements are generally legal restrictions 7841 * on the use and publishing of the plan definition. 7842 */ 7843 public PlanDefinition setCopyright(String value) { 7844 if (value == null) 7845 this.copyright = null; 7846 else { 7847 if (this.copyright == null) 7848 this.copyright = new MarkdownType(); 7849 this.copyright.setValue(value); 7850 } 7851 return this; 7852 } 7853 7854 /** 7855 * @return {@link #approvalDate} (The date on which the resource content was 7856 * approved by the publisher. Approval happens once when the content is 7857 * officially approved for usage.). This is the underlying object with 7858 * id, value and extensions. The accessor "getApprovalDate" gives direct 7859 * access to the value 7860 */ 7861 public DateType getApprovalDateElement() { 7862 if (this.approvalDate == null) 7863 if (Configuration.errorOnAutoCreate()) 7864 throw new Error("Attempt to auto-create PlanDefinition.approvalDate"); 7865 else if (Configuration.doAutoCreate()) 7866 this.approvalDate = new DateType(); // bb 7867 return this.approvalDate; 7868 } 7869 7870 public boolean hasApprovalDateElement() { 7871 return this.approvalDate != null && !this.approvalDate.isEmpty(); 7872 } 7873 7874 public boolean hasApprovalDate() { 7875 return this.approvalDate != null && !this.approvalDate.isEmpty(); 7876 } 7877 7878 /** 7879 * @param value {@link #approvalDate} (The date on which the resource content 7880 * was approved by the publisher. Approval happens once when the 7881 * content is officially approved for usage.). This is the 7882 * underlying object with id, value and extensions. The accessor 7883 * "getApprovalDate" gives direct access to the value 7884 */ 7885 public PlanDefinition setApprovalDateElement(DateType value) { 7886 this.approvalDate = value; 7887 return this; 7888 } 7889 7890 /** 7891 * @return The date on which the resource content was approved by the publisher. 7892 * Approval happens once when the content is officially approved for 7893 * usage. 7894 */ 7895 public Date getApprovalDate() { 7896 return this.approvalDate == null ? null : this.approvalDate.getValue(); 7897 } 7898 7899 /** 7900 * @param value The date on which the resource content was approved by the 7901 * publisher. Approval happens once when the content is officially 7902 * approved for usage. 7903 */ 7904 public PlanDefinition setApprovalDate(Date value) { 7905 if (value == null) 7906 this.approvalDate = null; 7907 else { 7908 if (this.approvalDate == null) 7909 this.approvalDate = new DateType(); 7910 this.approvalDate.setValue(value); 7911 } 7912 return this; 7913 } 7914 7915 /** 7916 * @return {@link #lastReviewDate} (The date on which the resource content was 7917 * last reviewed. Review happens periodically after approval but does 7918 * not change the original approval date.). This is the underlying 7919 * object with id, value and extensions. The accessor 7920 * "getLastReviewDate" gives direct access to the value 7921 */ 7922 public DateType getLastReviewDateElement() { 7923 if (this.lastReviewDate == null) 7924 if (Configuration.errorOnAutoCreate()) 7925 throw new Error("Attempt to auto-create PlanDefinition.lastReviewDate"); 7926 else if (Configuration.doAutoCreate()) 7927 this.lastReviewDate = new DateType(); // bb 7928 return this.lastReviewDate; 7929 } 7930 7931 public boolean hasLastReviewDateElement() { 7932 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 7933 } 7934 7935 public boolean hasLastReviewDate() { 7936 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 7937 } 7938 7939 /** 7940 * @param value {@link #lastReviewDate} (The date on which the resource content 7941 * was last reviewed. Review happens periodically after approval 7942 * but does not change the original approval date.). This is the 7943 * underlying object with id, value and extensions. The accessor 7944 * "getLastReviewDate" gives direct access to the value 7945 */ 7946 public PlanDefinition setLastReviewDateElement(DateType value) { 7947 this.lastReviewDate = value; 7948 return this; 7949 } 7950 7951 /** 7952 * @return The date on which the resource content was last reviewed. Review 7953 * happens periodically after approval but does not change the original 7954 * approval date. 7955 */ 7956 public Date getLastReviewDate() { 7957 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 7958 } 7959 7960 /** 7961 * @param value The date on which the resource content was last reviewed. Review 7962 * happens periodically after approval but does not change the 7963 * original approval date. 7964 */ 7965 public PlanDefinition setLastReviewDate(Date value) { 7966 if (value == null) 7967 this.lastReviewDate = null; 7968 else { 7969 if (this.lastReviewDate == null) 7970 this.lastReviewDate = new DateType(); 7971 this.lastReviewDate.setValue(value); 7972 } 7973 return this; 7974 } 7975 7976 /** 7977 * @return {@link #effectivePeriod} (The period during which the plan definition 7978 * content was or is planned to be in active use.) 7979 */ 7980 public Period getEffectivePeriod() { 7981 if (this.effectivePeriod == null) 7982 if (Configuration.errorOnAutoCreate()) 7983 throw new Error("Attempt to auto-create PlanDefinition.effectivePeriod"); 7984 else if (Configuration.doAutoCreate()) 7985 this.effectivePeriod = new Period(); // cc 7986 return this.effectivePeriod; 7987 } 7988 7989 public boolean hasEffectivePeriod() { 7990 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 7991 } 7992 7993 /** 7994 * @param value {@link #effectivePeriod} (The period during which the plan 7995 * definition content was or is planned to be in active use.) 7996 */ 7997 public PlanDefinition setEffectivePeriod(Period value) { 7998 this.effectivePeriod = value; 7999 return this; 8000 } 8001 8002 /** 8003 * @return {@link #topic} (Descriptive topics related to the content of the plan 8004 * definition. Topics provide a high-level categorization of the 8005 * definition that can be useful for filtering and searching.) 8006 */ 8007 public List<CodeableConcept> getTopic() { 8008 if (this.topic == null) 8009 this.topic = new ArrayList<CodeableConcept>(); 8010 return this.topic; 8011 } 8012 8013 /** 8014 * @return Returns a reference to <code>this</code> for easy method chaining 8015 */ 8016 public PlanDefinition setTopic(List<CodeableConcept> theTopic) { 8017 this.topic = theTopic; 8018 return this; 8019 } 8020 8021 public boolean hasTopic() { 8022 if (this.topic == null) 8023 return false; 8024 for (CodeableConcept item : this.topic) 8025 if (!item.isEmpty()) 8026 return true; 8027 return false; 8028 } 8029 8030 public CodeableConcept addTopic() { // 3 8031 CodeableConcept t = new CodeableConcept(); 8032 if (this.topic == null) 8033 this.topic = new ArrayList<CodeableConcept>(); 8034 this.topic.add(t); 8035 return t; 8036 } 8037 8038 public PlanDefinition addTopic(CodeableConcept t) { // 3 8039 if (t == null) 8040 return this; 8041 if (this.topic == null) 8042 this.topic = new ArrayList<CodeableConcept>(); 8043 this.topic.add(t); 8044 return this; 8045 } 8046 8047 /** 8048 * @return The first repetition of repeating field {@link #topic}, creating it 8049 * if it does not already exist 8050 */ 8051 public CodeableConcept getTopicFirstRep() { 8052 if (getTopic().isEmpty()) { 8053 addTopic(); 8054 } 8055 return getTopic().get(0); 8056 } 8057 8058 /** 8059 * @return {@link #author} (An individiual or organization primarily involved in 8060 * the creation and maintenance of the content.) 8061 */ 8062 public List<ContactDetail> getAuthor() { 8063 if (this.author == null) 8064 this.author = new ArrayList<ContactDetail>(); 8065 return this.author; 8066 } 8067 8068 /** 8069 * @return Returns a reference to <code>this</code> for easy method chaining 8070 */ 8071 public PlanDefinition setAuthor(List<ContactDetail> theAuthor) { 8072 this.author = theAuthor; 8073 return this; 8074 } 8075 8076 public boolean hasAuthor() { 8077 if (this.author == null) 8078 return false; 8079 for (ContactDetail item : this.author) 8080 if (!item.isEmpty()) 8081 return true; 8082 return false; 8083 } 8084 8085 public ContactDetail addAuthor() { // 3 8086 ContactDetail t = new ContactDetail(); 8087 if (this.author == null) 8088 this.author = new ArrayList<ContactDetail>(); 8089 this.author.add(t); 8090 return t; 8091 } 8092 8093 public PlanDefinition addAuthor(ContactDetail t) { // 3 8094 if (t == null) 8095 return this; 8096 if (this.author == null) 8097 this.author = new ArrayList<ContactDetail>(); 8098 this.author.add(t); 8099 return this; 8100 } 8101 8102 /** 8103 * @return The first repetition of repeating field {@link #author}, creating it 8104 * if it does not already exist 8105 */ 8106 public ContactDetail getAuthorFirstRep() { 8107 if (getAuthor().isEmpty()) { 8108 addAuthor(); 8109 } 8110 return getAuthor().get(0); 8111 } 8112 8113 /** 8114 * @return {@link #editor} (An individual or organization primarily responsible 8115 * for internal coherence of the content.) 8116 */ 8117 public List<ContactDetail> getEditor() { 8118 if (this.editor == null) 8119 this.editor = new ArrayList<ContactDetail>(); 8120 return this.editor; 8121 } 8122 8123 /** 8124 * @return Returns a reference to <code>this</code> for easy method chaining 8125 */ 8126 public PlanDefinition setEditor(List<ContactDetail> theEditor) { 8127 this.editor = theEditor; 8128 return this; 8129 } 8130 8131 public boolean hasEditor() { 8132 if (this.editor == null) 8133 return false; 8134 for (ContactDetail item : this.editor) 8135 if (!item.isEmpty()) 8136 return true; 8137 return false; 8138 } 8139 8140 public ContactDetail addEditor() { // 3 8141 ContactDetail t = new ContactDetail(); 8142 if (this.editor == null) 8143 this.editor = new ArrayList<ContactDetail>(); 8144 this.editor.add(t); 8145 return t; 8146 } 8147 8148 public PlanDefinition addEditor(ContactDetail t) { // 3 8149 if (t == null) 8150 return this; 8151 if (this.editor == null) 8152 this.editor = new ArrayList<ContactDetail>(); 8153 this.editor.add(t); 8154 return this; 8155 } 8156 8157 /** 8158 * @return The first repetition of repeating field {@link #editor}, creating it 8159 * if it does not already exist 8160 */ 8161 public ContactDetail getEditorFirstRep() { 8162 if (getEditor().isEmpty()) { 8163 addEditor(); 8164 } 8165 return getEditor().get(0); 8166 } 8167 8168 /** 8169 * @return {@link #reviewer} (An individual or organization primarily 8170 * responsible for review of some aspect of the content.) 8171 */ 8172 public List<ContactDetail> getReviewer() { 8173 if (this.reviewer == null) 8174 this.reviewer = new ArrayList<ContactDetail>(); 8175 return this.reviewer; 8176 } 8177 8178 /** 8179 * @return Returns a reference to <code>this</code> for easy method chaining 8180 */ 8181 public PlanDefinition setReviewer(List<ContactDetail> theReviewer) { 8182 this.reviewer = theReviewer; 8183 return this; 8184 } 8185 8186 public boolean hasReviewer() { 8187 if (this.reviewer == null) 8188 return false; 8189 for (ContactDetail item : this.reviewer) 8190 if (!item.isEmpty()) 8191 return true; 8192 return false; 8193 } 8194 8195 public ContactDetail addReviewer() { // 3 8196 ContactDetail t = new ContactDetail(); 8197 if (this.reviewer == null) 8198 this.reviewer = new ArrayList<ContactDetail>(); 8199 this.reviewer.add(t); 8200 return t; 8201 } 8202 8203 public PlanDefinition addReviewer(ContactDetail t) { // 3 8204 if (t == null) 8205 return this; 8206 if (this.reviewer == null) 8207 this.reviewer = new ArrayList<ContactDetail>(); 8208 this.reviewer.add(t); 8209 return this; 8210 } 8211 8212 /** 8213 * @return The first repetition of repeating field {@link #reviewer}, creating 8214 * it if it does not already exist 8215 */ 8216 public ContactDetail getReviewerFirstRep() { 8217 if (getReviewer().isEmpty()) { 8218 addReviewer(); 8219 } 8220 return getReviewer().get(0); 8221 } 8222 8223 /** 8224 * @return {@link #endorser} (An individual or organization responsible for 8225 * officially endorsing the content for use in some setting.) 8226 */ 8227 public List<ContactDetail> getEndorser() { 8228 if (this.endorser == null) 8229 this.endorser = new ArrayList<ContactDetail>(); 8230 return this.endorser; 8231 } 8232 8233 /** 8234 * @return Returns a reference to <code>this</code> for easy method chaining 8235 */ 8236 public PlanDefinition setEndorser(List<ContactDetail> theEndorser) { 8237 this.endorser = theEndorser; 8238 return this; 8239 } 8240 8241 public boolean hasEndorser() { 8242 if (this.endorser == null) 8243 return false; 8244 for (ContactDetail item : this.endorser) 8245 if (!item.isEmpty()) 8246 return true; 8247 return false; 8248 } 8249 8250 public ContactDetail addEndorser() { // 3 8251 ContactDetail t = new ContactDetail(); 8252 if (this.endorser == null) 8253 this.endorser = new ArrayList<ContactDetail>(); 8254 this.endorser.add(t); 8255 return t; 8256 } 8257 8258 public PlanDefinition addEndorser(ContactDetail t) { // 3 8259 if (t == null) 8260 return this; 8261 if (this.endorser == null) 8262 this.endorser = new ArrayList<ContactDetail>(); 8263 this.endorser.add(t); 8264 return this; 8265 } 8266 8267 /** 8268 * @return The first repetition of repeating field {@link #endorser}, creating 8269 * it if it does not already exist 8270 */ 8271 public ContactDetail getEndorserFirstRep() { 8272 if (getEndorser().isEmpty()) { 8273 addEndorser(); 8274 } 8275 return getEndorser().get(0); 8276 } 8277 8278 /** 8279 * @return {@link #relatedArtifact} (Related artifacts such as additional 8280 * documentation, justification, or bibliographic references.) 8281 */ 8282 public List<RelatedArtifact> getRelatedArtifact() { 8283 if (this.relatedArtifact == null) 8284 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 8285 return this.relatedArtifact; 8286 } 8287 8288 /** 8289 * @return Returns a reference to <code>this</code> for easy method chaining 8290 */ 8291 public PlanDefinition setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 8292 this.relatedArtifact = theRelatedArtifact; 8293 return this; 8294 } 8295 8296 public boolean hasRelatedArtifact() { 8297 if (this.relatedArtifact == null) 8298 return false; 8299 for (RelatedArtifact item : this.relatedArtifact) 8300 if (!item.isEmpty()) 8301 return true; 8302 return false; 8303 } 8304 8305 public RelatedArtifact addRelatedArtifact() { // 3 8306 RelatedArtifact t = new RelatedArtifact(); 8307 if (this.relatedArtifact == null) 8308 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 8309 this.relatedArtifact.add(t); 8310 return t; 8311 } 8312 8313 public PlanDefinition addRelatedArtifact(RelatedArtifact t) { // 3 8314 if (t == null) 8315 return this; 8316 if (this.relatedArtifact == null) 8317 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 8318 this.relatedArtifact.add(t); 8319 return this; 8320 } 8321 8322 /** 8323 * @return The first repetition of repeating field {@link #relatedArtifact}, 8324 * creating it if it does not already exist 8325 */ 8326 public RelatedArtifact getRelatedArtifactFirstRep() { 8327 if (getRelatedArtifact().isEmpty()) { 8328 addRelatedArtifact(); 8329 } 8330 return getRelatedArtifact().get(0); 8331 } 8332 8333 /** 8334 * @return {@link #library} (A reference to a Library resource containing any 8335 * formal logic used by the plan definition.) 8336 */ 8337 public List<CanonicalType> getLibrary() { 8338 if (this.library == null) 8339 this.library = new ArrayList<CanonicalType>(); 8340 return this.library; 8341 } 8342 8343 /** 8344 * @return Returns a reference to <code>this</code> for easy method chaining 8345 */ 8346 public PlanDefinition setLibrary(List<CanonicalType> theLibrary) { 8347 this.library = theLibrary; 8348 return this; 8349 } 8350 8351 public boolean hasLibrary() { 8352 if (this.library == null) 8353 return false; 8354 for (CanonicalType item : this.library) 8355 if (!item.isEmpty()) 8356 return true; 8357 return false; 8358 } 8359 8360 /** 8361 * @return {@link #library} (A reference to a Library resource containing any 8362 * formal logic used by the plan definition.) 8363 */ 8364 public CanonicalType addLibraryElement() {// 2 8365 CanonicalType t = new CanonicalType(); 8366 if (this.library == null) 8367 this.library = new ArrayList<CanonicalType>(); 8368 this.library.add(t); 8369 return t; 8370 } 8371 8372 /** 8373 * @param value {@link #library} (A reference to a Library resource containing 8374 * any formal logic used by the plan definition.) 8375 */ 8376 public PlanDefinition addLibrary(String value) { // 1 8377 CanonicalType t = new CanonicalType(); 8378 t.setValue(value); 8379 if (this.library == null) 8380 this.library = new ArrayList<CanonicalType>(); 8381 this.library.add(t); 8382 return this; 8383 } 8384 8385 /** 8386 * @param value {@link #library} (A reference to a Library resource containing 8387 * any formal logic used by the plan definition.) 8388 */ 8389 public boolean hasLibrary(String value) { 8390 if (this.library == null) 8391 return false; 8392 for (CanonicalType v : this.library) 8393 if (v.getValue().equals(value)) // canonical(Library) 8394 return true; 8395 return false; 8396 } 8397 8398 /** 8399 * @return {@link #goal} (Goals that describe what the activities within the 8400 * plan are intended to achieve. For example, weight loss, restoring an 8401 * activity of daily living, obtaining herd immunity via immunization, 8402 * meeting a process improvement objective, etc.) 8403 */ 8404 public List<PlanDefinitionGoalComponent> getGoal() { 8405 if (this.goal == null) 8406 this.goal = new ArrayList<PlanDefinitionGoalComponent>(); 8407 return this.goal; 8408 } 8409 8410 /** 8411 * @return Returns a reference to <code>this</code> for easy method chaining 8412 */ 8413 public PlanDefinition setGoal(List<PlanDefinitionGoalComponent> theGoal) { 8414 this.goal = theGoal; 8415 return this; 8416 } 8417 8418 public boolean hasGoal() { 8419 if (this.goal == null) 8420 return false; 8421 for (PlanDefinitionGoalComponent item : this.goal) 8422 if (!item.isEmpty()) 8423 return true; 8424 return false; 8425 } 8426 8427 public PlanDefinitionGoalComponent addGoal() { // 3 8428 PlanDefinitionGoalComponent t = new PlanDefinitionGoalComponent(); 8429 if (this.goal == null) 8430 this.goal = new ArrayList<PlanDefinitionGoalComponent>(); 8431 this.goal.add(t); 8432 return t; 8433 } 8434 8435 public PlanDefinition addGoal(PlanDefinitionGoalComponent t) { // 3 8436 if (t == null) 8437 return this; 8438 if (this.goal == null) 8439 this.goal = new ArrayList<PlanDefinitionGoalComponent>(); 8440 this.goal.add(t); 8441 return this; 8442 } 8443 8444 /** 8445 * @return The first repetition of repeating field {@link #goal}, creating it if 8446 * it does not already exist 8447 */ 8448 public PlanDefinitionGoalComponent getGoalFirstRep() { 8449 if (getGoal().isEmpty()) { 8450 addGoal(); 8451 } 8452 return getGoal().get(0); 8453 } 8454 8455 /** 8456 * @return {@link #action} (An action or group of actions to be taken as part of 8457 * the plan.) 8458 */ 8459 public List<PlanDefinitionActionComponent> getAction() { 8460 if (this.action == null) 8461 this.action = new ArrayList<PlanDefinitionActionComponent>(); 8462 return this.action; 8463 } 8464 8465 /** 8466 * @return Returns a reference to <code>this</code> for easy method chaining 8467 */ 8468 public PlanDefinition setAction(List<PlanDefinitionActionComponent> theAction) { 8469 this.action = theAction; 8470 return this; 8471 } 8472 8473 public boolean hasAction() { 8474 if (this.action == null) 8475 return false; 8476 for (PlanDefinitionActionComponent item : this.action) 8477 if (!item.isEmpty()) 8478 return true; 8479 return false; 8480 } 8481 8482 public PlanDefinitionActionComponent addAction() { // 3 8483 PlanDefinitionActionComponent t = new PlanDefinitionActionComponent(); 8484 if (this.action == null) 8485 this.action = new ArrayList<PlanDefinitionActionComponent>(); 8486 this.action.add(t); 8487 return t; 8488 } 8489 8490 public PlanDefinition addAction(PlanDefinitionActionComponent t) { // 3 8491 if (t == null) 8492 return this; 8493 if (this.action == null) 8494 this.action = new ArrayList<PlanDefinitionActionComponent>(); 8495 this.action.add(t); 8496 return this; 8497 } 8498 8499 /** 8500 * @return The first repetition of repeating field {@link #action}, creating it 8501 * if it does not already exist 8502 */ 8503 public PlanDefinitionActionComponent getActionFirstRep() { 8504 if (getAction().isEmpty()) { 8505 addAction(); 8506 } 8507 return getAction().get(0); 8508 } 8509 8510 protected void listChildren(List<Property> children) { 8511 super.listChildren(children); 8512 children.add(new Property("url", "uri", 8513 "An absolute URI that is used to identify this plan definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this plan definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the plan definition is stored on different servers.", 8514 0, 1, url)); 8515 children.add(new Property("identifier", "Identifier", 8516 "A formal identifier that is used to identify this plan definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 8517 0, java.lang.Integer.MAX_VALUE, identifier)); 8518 children.add(new Property("version", "string", 8519 "The identifier that is used to identify this version of the plan definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the plan definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 8520 0, 1, version)); 8521 children.add(new Property("name", "string", 8522 "A natural language name identifying the plan definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 8523 0, 1, name)); 8524 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the plan definition.", 8525 0, 1, title)); 8526 children.add(new Property("subtitle", "string", 8527 "An explanatory or alternate title for the plan definition giving additional information about its content.", 0, 8528 1, subtitle)); 8529 children.add(new Property("type", "CodeableConcept", 8530 "A high-level category for the plan definition that distinguishes the kinds of systems that would be interested in the plan definition.", 8531 0, 1, type)); 8532 children.add(new Property("status", "code", 8533 "The status of this plan definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 8534 children.add(new Property("experimental", "boolean", 8535 "A Boolean value to indicate that this plan definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 8536 0, 1, experimental)); 8537 children.add(new Property("subject[x]", "CodeableConcept|Reference(Group)", 8538 "A code or group definition that describes the intended subject of the plan definition.", 0, 1, subject)); 8539 children.add(new Property("date", "dateTime", 8540 "The date (and optionally time) when the plan definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the plan definition changes.", 8541 0, 1, date)); 8542 children.add(new Property("publisher", "string", 8543 "The name of the organization or individual that published the plan definition.", 0, 1, publisher)); 8544 children.add(new Property("contact", "ContactDetail", 8545 "Contact details to assist a user in finding and communicating with the publisher.", 0, 8546 java.lang.Integer.MAX_VALUE, contact)); 8547 children.add(new Property("description", "markdown", 8548 "A free text natural language description of the plan definition from a consumer's perspective.", 0, 1, 8549 description)); 8550 children.add(new Property("useContext", "UsageContext", 8551 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate plan definition instances.", 8552 0, java.lang.Integer.MAX_VALUE, useContext)); 8553 children.add(new Property("jurisdiction", "CodeableConcept", 8554 "A legal or geographic region in which the plan definition is intended to be used.", 0, 8555 java.lang.Integer.MAX_VALUE, jurisdiction)); 8556 children.add(new Property("purpose", "markdown", 8557 "Explanation of why this plan definition is needed and why it has been designed as it has.", 0, 1, purpose)); 8558 children.add(new Property("usage", "string", 8559 "A detailed description of how the plan definition is used from a clinical perspective.", 0, 1, usage)); 8560 children.add(new Property("copyright", "markdown", 8561 "A copyright statement relating to the plan definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the plan definition.", 8562 0, 1, copyright)); 8563 children.add(new Property("approvalDate", "date", 8564 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 8565 0, 1, approvalDate)); 8566 children.add(new Property("lastReviewDate", "date", 8567 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 8568 0, 1, lastReviewDate)); 8569 children.add(new Property("effectivePeriod", "Period", 8570 "The period during which the plan definition content was or is planned to be in active use.", 0, 1, 8571 effectivePeriod)); 8572 children.add(new Property("topic", "CodeableConcept", 8573 "Descriptive topics related to the content of the plan definition. Topics provide a high-level categorization of the definition that can be useful for filtering and searching.", 8574 0, java.lang.Integer.MAX_VALUE, topic)); 8575 children.add(new Property("author", "ContactDetail", 8576 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 8577 java.lang.Integer.MAX_VALUE, author)); 8578 children.add(new Property("editor", "ContactDetail", 8579 "An individual or organization primarily responsible for internal coherence of the content.", 0, 8580 java.lang.Integer.MAX_VALUE, editor)); 8581 children.add(new Property("reviewer", "ContactDetail", 8582 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 8583 java.lang.Integer.MAX_VALUE, reviewer)); 8584 children.add(new Property("endorser", "ContactDetail", 8585 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 8586 java.lang.Integer.MAX_VALUE, endorser)); 8587 children.add(new Property("relatedArtifact", "RelatedArtifact", 8588 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 8589 java.lang.Integer.MAX_VALUE, relatedArtifact)); 8590 children.add(new Property("library", "canonical(Library)", 8591 "A reference to a Library resource containing any formal logic used by the plan definition.", 0, 8592 java.lang.Integer.MAX_VALUE, library)); 8593 children.add(new Property("goal", "", 8594 "Goals that describe what the activities within the plan are intended to achieve. For example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc.", 8595 0, java.lang.Integer.MAX_VALUE, goal)); 8596 children.add(new Property("action", "", "An action or group of actions to be taken as part of the plan.", 0, 8597 java.lang.Integer.MAX_VALUE, action)); 8598 } 8599 8600 @Override 8601 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8602 switch (_hash) { 8603 case 116079: 8604 /* url */ return new Property("url", "uri", 8605 "An absolute URI that is used to identify this plan definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this plan definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the plan definition is stored on different servers.", 8606 0, 1, url); 8607 case -1618432855: 8608 /* identifier */ return new Property("identifier", "Identifier", 8609 "A formal identifier that is used to identify this plan definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 8610 0, java.lang.Integer.MAX_VALUE, identifier); 8611 case 351608024: 8612 /* version */ return new Property("version", "string", 8613 "The identifier that is used to identify this version of the plan definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the plan definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 8614 0, 1, version); 8615 case 3373707: 8616 /* name */ return new Property("name", "string", 8617 "A natural language name identifying the plan definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 8618 0, 1, name); 8619 case 110371416: 8620 /* title */ return new Property("title", "string", 8621 "A short, descriptive, user-friendly title for the plan definition.", 0, 1, title); 8622 case -2060497896: 8623 /* subtitle */ return new Property("subtitle", "string", 8624 "An explanatory or alternate title for the plan definition giving additional information about its content.", 8625 0, 1, subtitle); 8626 case 3575610: 8627 /* type */ return new Property("type", "CodeableConcept", 8628 "A high-level category for the plan definition that distinguishes the kinds of systems that would be interested in the plan definition.", 8629 0, 1, type); 8630 case -892481550: 8631 /* status */ return new Property("status", "code", 8632 "The status of this plan definition. Enables tracking the life-cycle of the content.", 0, 1, status); 8633 case -404562712: 8634 /* experimental */ return new Property("experimental", "boolean", 8635 "A Boolean value to indicate that this plan definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 8636 0, 1, experimental); 8637 case -573640748: 8638 /* subject[x] */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 8639 "A code or group definition that describes the intended subject of the plan definition.", 0, 1, subject); 8640 case -1867885268: 8641 /* subject */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 8642 "A code or group definition that describes the intended subject of the plan definition.", 0, 1, subject); 8643 case -1257122603: 8644 /* subjectCodeableConcept */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 8645 "A code or group definition that describes the intended subject of the plan definition.", 0, 1, subject); 8646 case 772938623: 8647 /* subjectReference */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 8648 "A code or group definition that describes the intended subject of the plan definition.", 0, 1, subject); 8649 case 3076014: 8650 /* date */ return new Property("date", "dateTime", 8651 "The date (and optionally time) when the plan definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the plan definition changes.", 8652 0, 1, date); 8653 case 1447404028: 8654 /* publisher */ return new Property("publisher", "string", 8655 "The name of the organization or individual that published the plan definition.", 0, 1, publisher); 8656 case 951526432: 8657 /* contact */ return new Property("contact", "ContactDetail", 8658 "Contact details to assist a user in finding and communicating with the publisher.", 0, 8659 java.lang.Integer.MAX_VALUE, contact); 8660 case -1724546052: 8661 /* description */ return new Property("description", "markdown", 8662 "A free text natural language description of the plan definition from a consumer's perspective.", 0, 1, 8663 description); 8664 case -669707736: 8665 /* useContext */ return new Property("useContext", "UsageContext", 8666 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate plan definition instances.", 8667 0, java.lang.Integer.MAX_VALUE, useContext); 8668 case -507075711: 8669 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 8670 "A legal or geographic region in which the plan definition is intended to be used.", 0, 8671 java.lang.Integer.MAX_VALUE, jurisdiction); 8672 case -220463842: 8673 /* purpose */ return new Property("purpose", "markdown", 8674 "Explanation of why this plan definition is needed and why it has been designed as it has.", 0, 1, purpose); 8675 case 111574433: 8676 /* usage */ return new Property("usage", "string", 8677 "A detailed description of how the plan definition is used from a clinical perspective.", 0, 1, usage); 8678 case 1522889671: 8679 /* copyright */ return new Property("copyright", "markdown", 8680 "A copyright statement relating to the plan definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the plan definition.", 8681 0, 1, copyright); 8682 case 223539345: 8683 /* approvalDate */ return new Property("approvalDate", "date", 8684 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 8685 0, 1, approvalDate); 8686 case -1687512484: 8687 /* lastReviewDate */ return new Property("lastReviewDate", "date", 8688 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 8689 0, 1, lastReviewDate); 8690 case -403934648: 8691 /* effectivePeriod */ return new Property("effectivePeriod", "Period", 8692 "The period during which the plan definition content was or is planned to be in active use.", 0, 1, 8693 effectivePeriod); 8694 case 110546223: 8695 /* topic */ return new Property("topic", "CodeableConcept", 8696 "Descriptive topics related to the content of the plan definition. Topics provide a high-level categorization of the definition that can be useful for filtering and searching.", 8697 0, java.lang.Integer.MAX_VALUE, topic); 8698 case -1406328437: 8699 /* author */ return new Property("author", "ContactDetail", 8700 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 8701 java.lang.Integer.MAX_VALUE, author); 8702 case -1307827859: 8703 /* editor */ return new Property("editor", "ContactDetail", 8704 "An individual or organization primarily responsible for internal coherence of the content.", 0, 8705 java.lang.Integer.MAX_VALUE, editor); 8706 case -261190139: 8707 /* reviewer */ return new Property("reviewer", "ContactDetail", 8708 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 8709 java.lang.Integer.MAX_VALUE, reviewer); 8710 case 1740277666: 8711 /* endorser */ return new Property("endorser", "ContactDetail", 8712 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 8713 java.lang.Integer.MAX_VALUE, endorser); 8714 case 666807069: 8715 /* relatedArtifact */ return new Property("relatedArtifact", "RelatedArtifact", 8716 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 8717 java.lang.Integer.MAX_VALUE, relatedArtifact); 8718 case 166208699: 8719 /* library */ return new Property("library", "canonical(Library)", 8720 "A reference to a Library resource containing any formal logic used by the plan definition.", 0, 8721 java.lang.Integer.MAX_VALUE, library); 8722 case 3178259: 8723 /* goal */ return new Property("goal", "", 8724 "Goals that describe what the activities within the plan are intended to achieve. For example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc.", 8725 0, java.lang.Integer.MAX_VALUE, goal); 8726 case -1422950858: 8727 /* action */ return new Property("action", "", "An action or group of actions to be taken as part of the plan.", 8728 0, java.lang.Integer.MAX_VALUE, action); 8729 default: 8730 return super.getNamedProperty(_hash, _name, _checkValid); 8731 } 8732 8733 } 8734 8735 @Override 8736 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8737 switch (hash) { 8738 case 116079: 8739 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 8740 case -1618432855: 8741 /* identifier */ return this.identifier == null ? new Base[0] 8742 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 8743 case 351608024: 8744 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 8745 case 3373707: 8746 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 8747 case 110371416: 8748 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 8749 case -2060497896: 8750 /* subtitle */ return this.subtitle == null ? new Base[0] : new Base[] { this.subtitle }; // StringType 8751 case 3575610: 8752 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 8753 case -892481550: 8754 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 8755 case -404562712: 8756 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 8757 case -1867885268: 8758 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Type 8759 case 3076014: 8760 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 8761 case 1447404028: 8762 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 8763 case 951526432: 8764 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 8765 case -1724546052: 8766 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 8767 case -669707736: 8768 /* useContext */ return this.useContext == null ? new Base[0] 8769 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 8770 case -507075711: 8771 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 8772 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 8773 case -220463842: 8774 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 8775 case 111574433: 8776 /* usage */ return this.usage == null ? new Base[0] : new Base[] { this.usage }; // StringType 8777 case 1522889671: 8778 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 8779 case 223539345: 8780 /* approvalDate */ return this.approvalDate == null ? new Base[0] : new Base[] { this.approvalDate }; // DateType 8781 case -1687512484: 8782 /* lastReviewDate */ return this.lastReviewDate == null ? new Base[0] : new Base[] { this.lastReviewDate }; // DateType 8783 case -403934648: 8784 /* effectivePeriod */ return this.effectivePeriod == null ? new Base[0] : new Base[] { this.effectivePeriod }; // Period 8785 case 110546223: 8786 /* topic */ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 8787 case -1406328437: 8788 /* author */ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 8789 case -1307827859: 8790 /* editor */ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 8791 case -261190139: 8792 /* reviewer */ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 8793 case 1740277666: 8794 /* endorser */ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 8795 case 666807069: 8796 /* relatedArtifact */ return this.relatedArtifact == null ? new Base[0] 8797 : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 8798 case 166208699: 8799 /* library */ return this.library == null ? new Base[0] : this.library.toArray(new Base[this.library.size()]); // CanonicalType 8800 case 3178259: 8801 /* goal */ return this.goal == null ? new Base[0] : this.goal.toArray(new Base[this.goal.size()]); // PlanDefinitionGoalComponent 8802 case -1422950858: 8803 /* action */ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // PlanDefinitionActionComponent 8804 default: 8805 return super.getProperty(hash, name, checkValid); 8806 } 8807 8808 } 8809 8810 @Override 8811 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8812 switch (hash) { 8813 case 116079: // url 8814 this.url = castToUri(value); // UriType 8815 return value; 8816 case -1618432855: // identifier 8817 this.getIdentifier().add(castToIdentifier(value)); // Identifier 8818 return value; 8819 case 351608024: // version 8820 this.version = castToString(value); // StringType 8821 return value; 8822 case 3373707: // name 8823 this.name = castToString(value); // StringType 8824 return value; 8825 case 110371416: // title 8826 this.title = castToString(value); // StringType 8827 return value; 8828 case -2060497896: // subtitle 8829 this.subtitle = castToString(value); // StringType 8830 return value; 8831 case 3575610: // type 8832 this.type = castToCodeableConcept(value); // CodeableConcept 8833 return value; 8834 case -892481550: // status 8835 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 8836 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 8837 return value; 8838 case -404562712: // experimental 8839 this.experimental = castToBoolean(value); // BooleanType 8840 return value; 8841 case -1867885268: // subject 8842 this.subject = castToType(value); // Type 8843 return value; 8844 case 3076014: // date 8845 this.date = castToDateTime(value); // DateTimeType 8846 return value; 8847 case 1447404028: // publisher 8848 this.publisher = castToString(value); // StringType 8849 return value; 8850 case 951526432: // contact 8851 this.getContact().add(castToContactDetail(value)); // ContactDetail 8852 return value; 8853 case -1724546052: // description 8854 this.description = castToMarkdown(value); // MarkdownType 8855 return value; 8856 case -669707736: // useContext 8857 this.getUseContext().add(castToUsageContext(value)); // UsageContext 8858 return value; 8859 case -507075711: // jurisdiction 8860 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 8861 return value; 8862 case -220463842: // purpose 8863 this.purpose = castToMarkdown(value); // MarkdownType 8864 return value; 8865 case 111574433: // usage 8866 this.usage = castToString(value); // StringType 8867 return value; 8868 case 1522889671: // copyright 8869 this.copyright = castToMarkdown(value); // MarkdownType 8870 return value; 8871 case 223539345: // approvalDate 8872 this.approvalDate = castToDate(value); // DateType 8873 return value; 8874 case -1687512484: // lastReviewDate 8875 this.lastReviewDate = castToDate(value); // DateType 8876 return value; 8877 case -403934648: // effectivePeriod 8878 this.effectivePeriod = castToPeriod(value); // Period 8879 return value; 8880 case 110546223: // topic 8881 this.getTopic().add(castToCodeableConcept(value)); // CodeableConcept 8882 return value; 8883 case -1406328437: // author 8884 this.getAuthor().add(castToContactDetail(value)); // ContactDetail 8885 return value; 8886 case -1307827859: // editor 8887 this.getEditor().add(castToContactDetail(value)); // ContactDetail 8888 return value; 8889 case -261190139: // reviewer 8890 this.getReviewer().add(castToContactDetail(value)); // ContactDetail 8891 return value; 8892 case 1740277666: // endorser 8893 this.getEndorser().add(castToContactDetail(value)); // ContactDetail 8894 return value; 8895 case 666807069: // relatedArtifact 8896 this.getRelatedArtifact().add(castToRelatedArtifact(value)); // RelatedArtifact 8897 return value; 8898 case 166208699: // library 8899 this.getLibrary().add(castToCanonical(value)); // CanonicalType 8900 return value; 8901 case 3178259: // goal 8902 this.getGoal().add((PlanDefinitionGoalComponent) value); // PlanDefinitionGoalComponent 8903 return value; 8904 case -1422950858: // action 8905 this.getAction().add((PlanDefinitionActionComponent) value); // PlanDefinitionActionComponent 8906 return value; 8907 default: 8908 return super.setProperty(hash, name, value); 8909 } 8910 8911 } 8912 8913 @Override 8914 public Base setProperty(String name, Base value) throws FHIRException { 8915 if (name.equals("url")) { 8916 this.url = castToUri(value); // UriType 8917 } else if (name.equals("identifier")) { 8918 this.getIdentifier().add(castToIdentifier(value)); 8919 } else if (name.equals("version")) { 8920 this.version = castToString(value); // StringType 8921 } else if (name.equals("name")) { 8922 this.name = castToString(value); // StringType 8923 } else if (name.equals("title")) { 8924 this.title = castToString(value); // StringType 8925 } else if (name.equals("subtitle")) { 8926 this.subtitle = castToString(value); // StringType 8927 } else if (name.equals("type")) { 8928 this.type = castToCodeableConcept(value); // CodeableConcept 8929 } else if (name.equals("status")) { 8930 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 8931 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 8932 } else if (name.equals("experimental")) { 8933 this.experimental = castToBoolean(value); // BooleanType 8934 } else if (name.equals("subject[x]")) { 8935 this.subject = castToType(value); // Type 8936 } else if (name.equals("date")) { 8937 this.date = castToDateTime(value); // DateTimeType 8938 } else if (name.equals("publisher")) { 8939 this.publisher = castToString(value); // StringType 8940 } else if (name.equals("contact")) { 8941 this.getContact().add(castToContactDetail(value)); 8942 } else if (name.equals("description")) { 8943 this.description = castToMarkdown(value); // MarkdownType 8944 } else if (name.equals("useContext")) { 8945 this.getUseContext().add(castToUsageContext(value)); 8946 } else if (name.equals("jurisdiction")) { 8947 this.getJurisdiction().add(castToCodeableConcept(value)); 8948 } else if (name.equals("purpose")) { 8949 this.purpose = castToMarkdown(value); // MarkdownType 8950 } else if (name.equals("usage")) { 8951 this.usage = castToString(value); // StringType 8952 } else if (name.equals("copyright")) { 8953 this.copyright = castToMarkdown(value); // MarkdownType 8954 } else if (name.equals("approvalDate")) { 8955 this.approvalDate = castToDate(value); // DateType 8956 } else if (name.equals("lastReviewDate")) { 8957 this.lastReviewDate = castToDate(value); // DateType 8958 } else if (name.equals("effectivePeriod")) { 8959 this.effectivePeriod = castToPeriod(value); // Period 8960 } else if (name.equals("topic")) { 8961 this.getTopic().add(castToCodeableConcept(value)); 8962 } else if (name.equals("author")) { 8963 this.getAuthor().add(castToContactDetail(value)); 8964 } else if (name.equals("editor")) { 8965 this.getEditor().add(castToContactDetail(value)); 8966 } else if (name.equals("reviewer")) { 8967 this.getReviewer().add(castToContactDetail(value)); 8968 } else if (name.equals("endorser")) { 8969 this.getEndorser().add(castToContactDetail(value)); 8970 } else if (name.equals("relatedArtifact")) { 8971 this.getRelatedArtifact().add(castToRelatedArtifact(value)); 8972 } else if (name.equals("library")) { 8973 this.getLibrary().add(castToCanonical(value)); 8974 } else if (name.equals("goal")) { 8975 this.getGoal().add((PlanDefinitionGoalComponent) value); 8976 } else if (name.equals("action")) { 8977 this.getAction().add((PlanDefinitionActionComponent) value); 8978 } else 8979 return super.setProperty(name, value); 8980 return value; 8981 } 8982 8983 @Override 8984 public void removeChild(String name, Base value) throws FHIRException { 8985 if (name.equals("url")) { 8986 this.url = null; 8987 } else if (name.equals("identifier")) { 8988 this.getIdentifier().remove(castToIdentifier(value)); 8989 } else if (name.equals("version")) { 8990 this.version = null; 8991 } else if (name.equals("name")) { 8992 this.name = null; 8993 } else if (name.equals("title")) { 8994 this.title = null; 8995 } else if (name.equals("subtitle")) { 8996 this.subtitle = null; 8997 } else if (name.equals("type")) { 8998 this.type = null; 8999 } else if (name.equals("status")) { 9000 this.status = null; 9001 } else if (name.equals("experimental")) { 9002 this.experimental = null; 9003 } else if (name.equals("subject[x]")) { 9004 this.subject = null; 9005 } else if (name.equals("date")) { 9006 this.date = null; 9007 } else if (name.equals("publisher")) { 9008 this.publisher = null; 9009 } else if (name.equals("contact")) { 9010 this.getContact().remove(castToContactDetail(value)); 9011 } else if (name.equals("description")) { 9012 this.description = null; 9013 } else if (name.equals("useContext")) { 9014 this.getUseContext().remove(castToUsageContext(value)); 9015 } else if (name.equals("jurisdiction")) { 9016 this.getJurisdiction().remove(castToCodeableConcept(value)); 9017 } else if (name.equals("purpose")) { 9018 this.purpose = null; 9019 } else if (name.equals("usage")) { 9020 this.usage = null; 9021 } else if (name.equals("copyright")) { 9022 this.copyright = null; 9023 } else if (name.equals("approvalDate")) { 9024 this.approvalDate = null; 9025 } else if (name.equals("lastReviewDate")) { 9026 this.lastReviewDate = null; 9027 } else if (name.equals("effectivePeriod")) { 9028 this.effectivePeriod = null; 9029 } else if (name.equals("topic")) { 9030 this.getTopic().remove(castToCodeableConcept(value)); 9031 } else if (name.equals("author")) { 9032 this.getAuthor().remove(castToContactDetail(value)); 9033 } else if (name.equals("editor")) { 9034 this.getEditor().remove(castToContactDetail(value)); 9035 } else if (name.equals("reviewer")) { 9036 this.getReviewer().remove(castToContactDetail(value)); 9037 } else if (name.equals("endorser")) { 9038 this.getEndorser().remove(castToContactDetail(value)); 9039 } else if (name.equals("relatedArtifact")) { 9040 this.getRelatedArtifact().remove(castToRelatedArtifact(value)); 9041 } else if (name.equals("library")) { 9042 this.getLibrary().remove(castToCanonical(value)); 9043 } else if (name.equals("goal")) { 9044 this.getGoal().remove((PlanDefinitionGoalComponent) value); 9045 } else if (name.equals("action")) { 9046 this.getAction().remove((PlanDefinitionActionComponent) value); 9047 } else 9048 super.removeChild(name, value); 9049 9050 } 9051 9052 @Override 9053 public Base makeProperty(int hash, String name) throws FHIRException { 9054 switch (hash) { 9055 case 116079: 9056 return getUrlElement(); 9057 case -1618432855: 9058 return addIdentifier(); 9059 case 351608024: 9060 return getVersionElement(); 9061 case 3373707: 9062 return getNameElement(); 9063 case 110371416: 9064 return getTitleElement(); 9065 case -2060497896: 9066 return getSubtitleElement(); 9067 case 3575610: 9068 return getType(); 9069 case -892481550: 9070 return getStatusElement(); 9071 case -404562712: 9072 return getExperimentalElement(); 9073 case -573640748: 9074 return getSubject(); 9075 case -1867885268: 9076 return getSubject(); 9077 case 3076014: 9078 return getDateElement(); 9079 case 1447404028: 9080 return getPublisherElement(); 9081 case 951526432: 9082 return addContact(); 9083 case -1724546052: 9084 return getDescriptionElement(); 9085 case -669707736: 9086 return addUseContext(); 9087 case -507075711: 9088 return addJurisdiction(); 9089 case -220463842: 9090 return getPurposeElement(); 9091 case 111574433: 9092 return getUsageElement(); 9093 case 1522889671: 9094 return getCopyrightElement(); 9095 case 223539345: 9096 return getApprovalDateElement(); 9097 case -1687512484: 9098 return getLastReviewDateElement(); 9099 case -403934648: 9100 return getEffectivePeriod(); 9101 case 110546223: 9102 return addTopic(); 9103 case -1406328437: 9104 return addAuthor(); 9105 case -1307827859: 9106 return addEditor(); 9107 case -261190139: 9108 return addReviewer(); 9109 case 1740277666: 9110 return addEndorser(); 9111 case 666807069: 9112 return addRelatedArtifact(); 9113 case 166208699: 9114 return addLibraryElement(); 9115 case 3178259: 9116 return addGoal(); 9117 case -1422950858: 9118 return addAction(); 9119 default: 9120 return super.makeProperty(hash, name); 9121 } 9122 9123 } 9124 9125 @Override 9126 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 9127 switch (hash) { 9128 case 116079: 9129 /* url */ return new String[] { "uri" }; 9130 case -1618432855: 9131 /* identifier */ return new String[] { "Identifier" }; 9132 case 351608024: 9133 /* version */ return new String[] { "string" }; 9134 case 3373707: 9135 /* name */ return new String[] { "string" }; 9136 case 110371416: 9137 /* title */ return new String[] { "string" }; 9138 case -2060497896: 9139 /* subtitle */ return new String[] { "string" }; 9140 case 3575610: 9141 /* type */ return new String[] { "CodeableConcept" }; 9142 case -892481550: 9143 /* status */ return new String[] { "code" }; 9144 case -404562712: 9145 /* experimental */ return new String[] { "boolean" }; 9146 case -1867885268: 9147 /* subject */ return new String[] { "CodeableConcept", "Reference" }; 9148 case 3076014: 9149 /* date */ return new String[] { "dateTime" }; 9150 case 1447404028: 9151 /* publisher */ return new String[] { "string" }; 9152 case 951526432: 9153 /* contact */ return new String[] { "ContactDetail" }; 9154 case -1724546052: 9155 /* description */ return new String[] { "markdown" }; 9156 case -669707736: 9157 /* useContext */ return new String[] { "UsageContext" }; 9158 case -507075711: 9159 /* jurisdiction */ return new String[] { "CodeableConcept" }; 9160 case -220463842: 9161 /* purpose */ return new String[] { "markdown" }; 9162 case 111574433: 9163 /* usage */ return new String[] { "string" }; 9164 case 1522889671: 9165 /* copyright */ return new String[] { "markdown" }; 9166 case 223539345: 9167 /* approvalDate */ return new String[] { "date" }; 9168 case -1687512484: 9169 /* lastReviewDate */ return new String[] { "date" }; 9170 case -403934648: 9171 /* effectivePeriod */ return new String[] { "Period" }; 9172 case 110546223: 9173 /* topic */ return new String[] { "CodeableConcept" }; 9174 case -1406328437: 9175 /* author */ return new String[] { "ContactDetail" }; 9176 case -1307827859: 9177 /* editor */ return new String[] { "ContactDetail" }; 9178 case -261190139: 9179 /* reviewer */ return new String[] { "ContactDetail" }; 9180 case 1740277666: 9181 /* endorser */ return new String[] { "ContactDetail" }; 9182 case 666807069: 9183 /* relatedArtifact */ return new String[] { "RelatedArtifact" }; 9184 case 166208699: 9185 /* library */ return new String[] { "canonical" }; 9186 case 3178259: 9187 /* goal */ return new String[] {}; 9188 case -1422950858: 9189 /* action */ return new String[] {}; 9190 default: 9191 return super.getTypesForProperty(hash, name); 9192 } 9193 9194 } 9195 9196 @Override 9197 public Base addChild(String name) throws FHIRException { 9198 if (name.equals("url")) { 9199 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.url"); 9200 } else if (name.equals("identifier")) { 9201 return addIdentifier(); 9202 } else if (name.equals("version")) { 9203 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.version"); 9204 } else if (name.equals("name")) { 9205 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.name"); 9206 } else if (name.equals("title")) { 9207 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.title"); 9208 } else if (name.equals("subtitle")) { 9209 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.subtitle"); 9210 } else if (name.equals("type")) { 9211 this.type = new CodeableConcept(); 9212 return this.type; 9213 } else if (name.equals("status")) { 9214 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.status"); 9215 } else if (name.equals("experimental")) { 9216 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.experimental"); 9217 } else if (name.equals("subjectCodeableConcept")) { 9218 this.subject = new CodeableConcept(); 9219 return this.subject; 9220 } else if (name.equals("subjectReference")) { 9221 this.subject = new Reference(); 9222 return this.subject; 9223 } else if (name.equals("date")) { 9224 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.date"); 9225 } else if (name.equals("publisher")) { 9226 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.publisher"); 9227 } else if (name.equals("contact")) { 9228 return addContact(); 9229 } else if (name.equals("description")) { 9230 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.description"); 9231 } else if (name.equals("useContext")) { 9232 return addUseContext(); 9233 } else if (name.equals("jurisdiction")) { 9234 return addJurisdiction(); 9235 } else if (name.equals("purpose")) { 9236 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.purpose"); 9237 } else if (name.equals("usage")) { 9238 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.usage"); 9239 } else if (name.equals("copyright")) { 9240 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.copyright"); 9241 } else if (name.equals("approvalDate")) { 9242 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.approvalDate"); 9243 } else if (name.equals("lastReviewDate")) { 9244 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.lastReviewDate"); 9245 } else if (name.equals("effectivePeriod")) { 9246 this.effectivePeriod = new Period(); 9247 return this.effectivePeriod; 9248 } else if (name.equals("topic")) { 9249 return addTopic(); 9250 } else if (name.equals("author")) { 9251 return addAuthor(); 9252 } else if (name.equals("editor")) { 9253 return addEditor(); 9254 } else if (name.equals("reviewer")) { 9255 return addReviewer(); 9256 } else if (name.equals("endorser")) { 9257 return addEndorser(); 9258 } else if (name.equals("relatedArtifact")) { 9259 return addRelatedArtifact(); 9260 } else if (name.equals("library")) { 9261 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.library"); 9262 } else if (name.equals("goal")) { 9263 return addGoal(); 9264 } else if (name.equals("action")) { 9265 return addAction(); 9266 } else 9267 return super.addChild(name); 9268 } 9269 9270 public String fhirType() { 9271 return "PlanDefinition"; 9272 9273 } 9274 9275 public PlanDefinition copy() { 9276 PlanDefinition dst = new PlanDefinition(); 9277 copyValues(dst); 9278 return dst; 9279 } 9280 9281 public void copyValues(PlanDefinition dst) { 9282 super.copyValues(dst); 9283 dst.url = url == null ? null : url.copy(); 9284 if (identifier != null) { 9285 dst.identifier = new ArrayList<Identifier>(); 9286 for (Identifier i : identifier) 9287 dst.identifier.add(i.copy()); 9288 } 9289 ; 9290 dst.version = version == null ? null : version.copy(); 9291 dst.name = name == null ? null : name.copy(); 9292 dst.title = title == null ? null : title.copy(); 9293 dst.subtitle = subtitle == null ? null : subtitle.copy(); 9294 dst.type = type == null ? null : type.copy(); 9295 dst.status = status == null ? null : status.copy(); 9296 dst.experimental = experimental == null ? null : experimental.copy(); 9297 dst.subject = subject == null ? null : subject.copy(); 9298 dst.date = date == null ? null : date.copy(); 9299 dst.publisher = publisher == null ? null : publisher.copy(); 9300 if (contact != null) { 9301 dst.contact = new ArrayList<ContactDetail>(); 9302 for (ContactDetail i : contact) 9303 dst.contact.add(i.copy()); 9304 } 9305 ; 9306 dst.description = description == null ? null : description.copy(); 9307 if (useContext != null) { 9308 dst.useContext = new ArrayList<UsageContext>(); 9309 for (UsageContext i : useContext) 9310 dst.useContext.add(i.copy()); 9311 } 9312 ; 9313 if (jurisdiction != null) { 9314 dst.jurisdiction = new ArrayList<CodeableConcept>(); 9315 for (CodeableConcept i : jurisdiction) 9316 dst.jurisdiction.add(i.copy()); 9317 } 9318 ; 9319 dst.purpose = purpose == null ? null : purpose.copy(); 9320 dst.usage = usage == null ? null : usage.copy(); 9321 dst.copyright = copyright == null ? null : copyright.copy(); 9322 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 9323 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 9324 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 9325 if (topic != null) { 9326 dst.topic = new ArrayList<CodeableConcept>(); 9327 for (CodeableConcept i : topic) 9328 dst.topic.add(i.copy()); 9329 } 9330 ; 9331 if (author != null) { 9332 dst.author = new ArrayList<ContactDetail>(); 9333 for (ContactDetail i : author) 9334 dst.author.add(i.copy()); 9335 } 9336 ; 9337 if (editor != null) { 9338 dst.editor = new ArrayList<ContactDetail>(); 9339 for (ContactDetail i : editor) 9340 dst.editor.add(i.copy()); 9341 } 9342 ; 9343 if (reviewer != null) { 9344 dst.reviewer = new ArrayList<ContactDetail>(); 9345 for (ContactDetail i : reviewer) 9346 dst.reviewer.add(i.copy()); 9347 } 9348 ; 9349 if (endorser != null) { 9350 dst.endorser = new ArrayList<ContactDetail>(); 9351 for (ContactDetail i : endorser) 9352 dst.endorser.add(i.copy()); 9353 } 9354 ; 9355 if (relatedArtifact != null) { 9356 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 9357 for (RelatedArtifact i : relatedArtifact) 9358 dst.relatedArtifact.add(i.copy()); 9359 } 9360 ; 9361 if (library != null) { 9362 dst.library = new ArrayList<CanonicalType>(); 9363 for (CanonicalType i : library) 9364 dst.library.add(i.copy()); 9365 } 9366 ; 9367 if (goal != null) { 9368 dst.goal = new ArrayList<PlanDefinitionGoalComponent>(); 9369 for (PlanDefinitionGoalComponent i : goal) 9370 dst.goal.add(i.copy()); 9371 } 9372 ; 9373 if (action != null) { 9374 dst.action = new ArrayList<PlanDefinitionActionComponent>(); 9375 for (PlanDefinitionActionComponent i : action) 9376 dst.action.add(i.copy()); 9377 } 9378 ; 9379 } 9380 9381 protected PlanDefinition typedCopy() { 9382 return copy(); 9383 } 9384 9385 @Override 9386 public boolean equalsDeep(Base other_) { 9387 if (!super.equalsDeep(other_)) 9388 return false; 9389 if (!(other_ instanceof PlanDefinition)) 9390 return false; 9391 PlanDefinition o = (PlanDefinition) other_; 9392 return compareDeep(identifier, o.identifier, true) && compareDeep(subtitle, o.subtitle, true) 9393 && compareDeep(type, o.type, true) && compareDeep(subject, o.subject, true) 9394 && compareDeep(purpose, o.purpose, true) && compareDeep(usage, o.usage, true) 9395 && compareDeep(copyright, o.copyright, true) && compareDeep(approvalDate, o.approvalDate, true) 9396 && compareDeep(lastReviewDate, o.lastReviewDate, true) && compareDeep(effectivePeriod, o.effectivePeriod, true) 9397 && compareDeep(topic, o.topic, true) && compareDeep(author, o.author, true) 9398 && compareDeep(editor, o.editor, true) && compareDeep(reviewer, o.reviewer, true) 9399 && compareDeep(endorser, o.endorser, true) && compareDeep(relatedArtifact, o.relatedArtifact, true) 9400 && compareDeep(library, o.library, true) && compareDeep(goal, o.goal, true) 9401 && compareDeep(action, o.action, true); 9402 } 9403 9404 @Override 9405 public boolean equalsShallow(Base other_) { 9406 if (!super.equalsShallow(other_)) 9407 return false; 9408 if (!(other_ instanceof PlanDefinition)) 9409 return false; 9410 PlanDefinition o = (PlanDefinition) other_; 9411 return compareValues(subtitle, o.subtitle, true) && compareValues(purpose, o.purpose, true) 9412 && compareValues(usage, o.usage, true) && compareValues(copyright, o.copyright, true) 9413 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true); 9414 } 9415 9416 public boolean isEmpty() { 9417 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, subtitle, type, subject, purpose, usage, 9418 copyright, approvalDate, lastReviewDate, effectivePeriod, topic, author, editor, reviewer, endorser, 9419 relatedArtifact, library, goal, action); 9420 } 9421 9422 @Override 9423 public ResourceType getResourceType() { 9424 return ResourceType.PlanDefinition; 9425 } 9426 9427 /** 9428 * Search parameter: <b>date</b> 9429 * <p> 9430 * Description: <b>The plan definition publication date</b><br> 9431 * Type: <b>date</b><br> 9432 * Path: <b>PlanDefinition.date</b><br> 9433 * </p> 9434 */ 9435 @SearchParamDefinition(name = "date", path = "PlanDefinition.date", description = "The plan definition publication date", type = "date") 9436 public static final String SP_DATE = "date"; 9437 /** 9438 * <b>Fluent Client</b> search parameter constant for <b>date</b> 9439 * <p> 9440 * Description: <b>The plan definition publication date</b><br> 9441 * Type: <b>date</b><br> 9442 * Path: <b>PlanDefinition.date</b><br> 9443 * </p> 9444 */ 9445 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 9446 SP_DATE); 9447 9448 /** 9449 * Search parameter: <b>identifier</b> 9450 * <p> 9451 * Description: <b>External identifier for the plan definition</b><br> 9452 * Type: <b>token</b><br> 9453 * Path: <b>PlanDefinition.identifier</b><br> 9454 * </p> 9455 */ 9456 @SearchParamDefinition(name = "identifier", path = "PlanDefinition.identifier", description = "External identifier for the plan definition", type = "token") 9457 public static final String SP_IDENTIFIER = "identifier"; 9458 /** 9459 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 9460 * <p> 9461 * Description: <b>External identifier for the plan definition</b><br> 9462 * Type: <b>token</b><br> 9463 * Path: <b>PlanDefinition.identifier</b><br> 9464 * </p> 9465 */ 9466 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 9467 SP_IDENTIFIER); 9468 9469 /** 9470 * Search parameter: <b>successor</b> 9471 * <p> 9472 * Description: <b>What resource is being referenced</b><br> 9473 * Type: <b>reference</b><br> 9474 * Path: <b>PlanDefinition.relatedArtifact.resource</b><br> 9475 * </p> 9476 */ 9477 @SearchParamDefinition(name = "successor", path = "PlanDefinition.relatedArtifact.where(type='successor').resource", description = "What resource is being referenced", type = "reference") 9478 public static final String SP_SUCCESSOR = "successor"; 9479 /** 9480 * <b>Fluent Client</b> search parameter constant for <b>successor</b> 9481 * <p> 9482 * Description: <b>What resource is being referenced</b><br> 9483 * Type: <b>reference</b><br> 9484 * Path: <b>PlanDefinition.relatedArtifact.resource</b><br> 9485 * </p> 9486 */ 9487 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUCCESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 9488 SP_SUCCESSOR); 9489 9490 /** 9491 * Constant for fluent queries to be used to add include statements. Specifies 9492 * the path value of "<b>PlanDefinition:successor</b>". 9493 */ 9494 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUCCESSOR = new ca.uhn.fhir.model.api.Include( 9495 "PlanDefinition:successor").toLocked(); 9496 9497 /** 9498 * Search parameter: <b>context-type-value</b> 9499 * <p> 9500 * Description: <b>A use context type and value assigned to the plan 9501 * definition</b><br> 9502 * Type: <b>composite</b><br> 9503 * Path: <b></b><br> 9504 * </p> 9505 */ 9506 @SearchParamDefinition(name = "context-type-value", path = "PlanDefinition.useContext", description = "A use context type and value assigned to the plan definition", type = "composite", compositeOf = { 9507 "context-type", "context" }) 9508 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 9509 /** 9510 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 9511 * <p> 9512 * Description: <b>A use context type and value assigned to the plan 9513 * definition</b><br> 9514 * Type: <b>composite</b><br> 9515 * Path: <b></b><br> 9516 * </p> 9517 */ 9518 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 9519 SP_CONTEXT_TYPE_VALUE); 9520 9521 /** 9522 * Search parameter: <b>jurisdiction</b> 9523 * <p> 9524 * Description: <b>Intended jurisdiction for the plan definition</b><br> 9525 * Type: <b>token</b><br> 9526 * Path: <b>PlanDefinition.jurisdiction</b><br> 9527 * </p> 9528 */ 9529 @SearchParamDefinition(name = "jurisdiction", path = "PlanDefinition.jurisdiction", description = "Intended jurisdiction for the plan definition", type = "token") 9530 public static final String SP_JURISDICTION = "jurisdiction"; 9531 /** 9532 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 9533 * <p> 9534 * Description: <b>Intended jurisdiction for the plan definition</b><br> 9535 * Type: <b>token</b><br> 9536 * Path: <b>PlanDefinition.jurisdiction</b><br> 9537 * </p> 9538 */ 9539 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 9540 SP_JURISDICTION); 9541 9542 /** 9543 * Search parameter: <b>description</b> 9544 * <p> 9545 * Description: <b>The description of the plan definition</b><br> 9546 * Type: <b>string</b><br> 9547 * Path: <b>PlanDefinition.description</b><br> 9548 * </p> 9549 */ 9550 @SearchParamDefinition(name = "description", path = "PlanDefinition.description", description = "The description of the plan definition", type = "string") 9551 public static final String SP_DESCRIPTION = "description"; 9552 /** 9553 * <b>Fluent Client</b> search parameter constant for <b>description</b> 9554 * <p> 9555 * Description: <b>The description of the plan definition</b><br> 9556 * Type: <b>string</b><br> 9557 * Path: <b>PlanDefinition.description</b><br> 9558 * </p> 9559 */ 9560 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 9561 SP_DESCRIPTION); 9562 9563 /** 9564 * Search parameter: <b>derived-from</b> 9565 * <p> 9566 * Description: <b>What resource is being referenced</b><br> 9567 * Type: <b>reference</b><br> 9568 * Path: <b>PlanDefinition.relatedArtifact.resource</b><br> 9569 * </p> 9570 */ 9571 @SearchParamDefinition(name = "derived-from", path = "PlanDefinition.relatedArtifact.where(type='derived-from').resource", description = "What resource is being referenced", type = "reference") 9572 public static final String SP_DERIVED_FROM = "derived-from"; 9573 /** 9574 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 9575 * <p> 9576 * Description: <b>What resource is being referenced</b><br> 9577 * Type: <b>reference</b><br> 9578 * Path: <b>PlanDefinition.relatedArtifact.resource</b><br> 9579 * </p> 9580 */ 9581 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 9582 SP_DERIVED_FROM); 9583 9584 /** 9585 * Constant for fluent queries to be used to add include statements. Specifies 9586 * the path value of "<b>PlanDefinition:derived-from</b>". 9587 */ 9588 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include( 9589 "PlanDefinition:derived-from").toLocked(); 9590 9591 /** 9592 * Search parameter: <b>context-type</b> 9593 * <p> 9594 * Description: <b>A type of use context assigned to the plan definition</b><br> 9595 * Type: <b>token</b><br> 9596 * Path: <b>PlanDefinition.useContext.code</b><br> 9597 * </p> 9598 */ 9599 @SearchParamDefinition(name = "context-type", path = "PlanDefinition.useContext.code", description = "A type of use context assigned to the plan definition", type = "token") 9600 public static final String SP_CONTEXT_TYPE = "context-type"; 9601 /** 9602 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 9603 * <p> 9604 * Description: <b>A type of use context assigned to the plan definition</b><br> 9605 * Type: <b>token</b><br> 9606 * Path: <b>PlanDefinition.useContext.code</b><br> 9607 * </p> 9608 */ 9609 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 9610 SP_CONTEXT_TYPE); 9611 9612 /** 9613 * Search parameter: <b>predecessor</b> 9614 * <p> 9615 * Description: <b>What resource is being referenced</b><br> 9616 * Type: <b>reference</b><br> 9617 * Path: <b>PlanDefinition.relatedArtifact.resource</b><br> 9618 * </p> 9619 */ 9620 @SearchParamDefinition(name = "predecessor", path = "PlanDefinition.relatedArtifact.where(type='predecessor').resource", description = "What resource is being referenced", type = "reference") 9621 public static final String SP_PREDECESSOR = "predecessor"; 9622 /** 9623 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 9624 * <p> 9625 * Description: <b>What resource is being referenced</b><br> 9626 * Type: <b>reference</b><br> 9627 * Path: <b>PlanDefinition.relatedArtifact.resource</b><br> 9628 * </p> 9629 */ 9630 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 9631 SP_PREDECESSOR); 9632 9633 /** 9634 * Constant for fluent queries to be used to add include statements. Specifies 9635 * the path value of "<b>PlanDefinition:predecessor</b>". 9636 */ 9637 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include( 9638 "PlanDefinition:predecessor").toLocked(); 9639 9640 /** 9641 * Search parameter: <b>title</b> 9642 * <p> 9643 * Description: <b>The human-friendly name of the plan definition</b><br> 9644 * Type: <b>string</b><br> 9645 * Path: <b>PlanDefinition.title</b><br> 9646 * </p> 9647 */ 9648 @SearchParamDefinition(name = "title", path = "PlanDefinition.title", description = "The human-friendly name of the plan definition", type = "string") 9649 public static final String SP_TITLE = "title"; 9650 /** 9651 * <b>Fluent Client</b> search parameter constant for <b>title</b> 9652 * <p> 9653 * Description: <b>The human-friendly name of the plan definition</b><br> 9654 * Type: <b>string</b><br> 9655 * Path: <b>PlanDefinition.title</b><br> 9656 * </p> 9657 */ 9658 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 9659 SP_TITLE); 9660 9661 /** 9662 * Search parameter: <b>composed-of</b> 9663 * <p> 9664 * Description: <b>What resource is being referenced</b><br> 9665 * Type: <b>reference</b><br> 9666 * Path: <b>PlanDefinition.relatedArtifact.resource</b><br> 9667 * </p> 9668 */ 9669 @SearchParamDefinition(name = "composed-of", path = "PlanDefinition.relatedArtifact.where(type='composed-of').resource", description = "What resource is being referenced", type = "reference") 9670 public static final String SP_COMPOSED_OF = "composed-of"; 9671 /** 9672 * <b>Fluent Client</b> search parameter constant for <b>composed-of</b> 9673 * <p> 9674 * Description: <b>What resource is being referenced</b><br> 9675 * Type: <b>reference</b><br> 9676 * Path: <b>PlanDefinition.relatedArtifact.resource</b><br> 9677 * </p> 9678 */ 9679 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPOSED_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 9680 SP_COMPOSED_OF); 9681 9682 /** 9683 * Constant for fluent queries to be used to add include statements. Specifies 9684 * the path value of "<b>PlanDefinition:composed-of</b>". 9685 */ 9686 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPOSED_OF = new ca.uhn.fhir.model.api.Include( 9687 "PlanDefinition:composed-of").toLocked(); 9688 9689 /** 9690 * Search parameter: <b>type</b> 9691 * <p> 9692 * Description: <b>The type of artifact the plan (e.g. order-set, eca-rule, 9693 * protocol)</b><br> 9694 * Type: <b>token</b><br> 9695 * Path: <b>PlanDefinition.type</b><br> 9696 * </p> 9697 */ 9698 @SearchParamDefinition(name = "type", path = "PlanDefinition.type", description = "The type of artifact the plan (e.g. order-set, eca-rule, protocol)", type = "token") 9699 public static final String SP_TYPE = "type"; 9700 /** 9701 * <b>Fluent Client</b> search parameter constant for <b>type</b> 9702 * <p> 9703 * Description: <b>The type of artifact the plan (e.g. order-set, eca-rule, 9704 * protocol)</b><br> 9705 * Type: <b>token</b><br> 9706 * Path: <b>PlanDefinition.type</b><br> 9707 * </p> 9708 */ 9709 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 9710 SP_TYPE); 9711 9712 /** 9713 * Search parameter: <b>version</b> 9714 * <p> 9715 * Description: <b>The business version of the plan definition</b><br> 9716 * Type: <b>token</b><br> 9717 * Path: <b>PlanDefinition.version</b><br> 9718 * </p> 9719 */ 9720 @SearchParamDefinition(name = "version", path = "PlanDefinition.version", description = "The business version of the plan definition", type = "token") 9721 public static final String SP_VERSION = "version"; 9722 /** 9723 * <b>Fluent Client</b> search parameter constant for <b>version</b> 9724 * <p> 9725 * Description: <b>The business version of the plan definition</b><br> 9726 * Type: <b>token</b><br> 9727 * Path: <b>PlanDefinition.version</b><br> 9728 * </p> 9729 */ 9730 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 9731 SP_VERSION); 9732 9733 /** 9734 * Search parameter: <b>url</b> 9735 * <p> 9736 * Description: <b>The uri that identifies the plan definition</b><br> 9737 * Type: <b>uri</b><br> 9738 * Path: <b>PlanDefinition.url</b><br> 9739 * </p> 9740 */ 9741 @SearchParamDefinition(name = "url", path = "PlanDefinition.url", description = "The uri that identifies the plan definition", type = "uri") 9742 public static final String SP_URL = "url"; 9743 /** 9744 * <b>Fluent Client</b> search parameter constant for <b>url</b> 9745 * <p> 9746 * Description: <b>The uri that identifies the plan definition</b><br> 9747 * Type: <b>uri</b><br> 9748 * Path: <b>PlanDefinition.url</b><br> 9749 * </p> 9750 */ 9751 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 9752 9753 /** 9754 * Search parameter: <b>context-quantity</b> 9755 * <p> 9756 * Description: <b>A quantity- or range-valued use context assigned to the plan 9757 * definition</b><br> 9758 * Type: <b>quantity</b><br> 9759 * Path: <b>PlanDefinition.useContext.valueQuantity, 9760 * PlanDefinition.useContext.valueRange</b><br> 9761 * </p> 9762 */ 9763 @SearchParamDefinition(name = "context-quantity", path = "(PlanDefinition.useContext.value as Quantity) | (PlanDefinition.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the plan definition", type = "quantity") 9764 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 9765 /** 9766 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 9767 * <p> 9768 * Description: <b>A quantity- or range-valued use context assigned to the plan 9769 * definition</b><br> 9770 * Type: <b>quantity</b><br> 9771 * Path: <b>PlanDefinition.useContext.valueQuantity, 9772 * PlanDefinition.useContext.valueRange</b><br> 9773 * </p> 9774 */ 9775 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 9776 SP_CONTEXT_QUANTITY); 9777 9778 /** 9779 * Search parameter: <b>effective</b> 9780 * <p> 9781 * Description: <b>The time during which the plan definition is intended to be 9782 * in use</b><br> 9783 * Type: <b>date</b><br> 9784 * Path: <b>PlanDefinition.effectivePeriod</b><br> 9785 * </p> 9786 */ 9787 @SearchParamDefinition(name = "effective", path = "PlanDefinition.effectivePeriod", description = "The time during which the plan definition is intended to be in use", type = "date") 9788 public static final String SP_EFFECTIVE = "effective"; 9789 /** 9790 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 9791 * <p> 9792 * Description: <b>The time during which the plan definition is intended to be 9793 * in use</b><br> 9794 * Type: <b>date</b><br> 9795 * Path: <b>PlanDefinition.effectivePeriod</b><br> 9796 * </p> 9797 */ 9798 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam( 9799 SP_EFFECTIVE); 9800 9801 /** 9802 * Search parameter: <b>depends-on</b> 9803 * <p> 9804 * Description: <b>What resource is being referenced</b><br> 9805 * Type: <b>reference</b><br> 9806 * Path: <b>PlanDefinition.relatedArtifact.resource, 9807 * PlanDefinition.library</b><br> 9808 * </p> 9809 */ 9810 @SearchParamDefinition(name = "depends-on", path = "PlanDefinition.relatedArtifact.where(type='depends-on').resource | PlanDefinition.library", description = "What resource is being referenced", type = "reference") 9811 public static final String SP_DEPENDS_ON = "depends-on"; 9812 /** 9813 * <b>Fluent Client</b> search parameter constant for <b>depends-on</b> 9814 * <p> 9815 * Description: <b>What resource is being referenced</b><br> 9816 * Type: <b>reference</b><br> 9817 * Path: <b>PlanDefinition.relatedArtifact.resource, 9818 * PlanDefinition.library</b><br> 9819 * </p> 9820 */ 9821 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEPENDS_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 9822 SP_DEPENDS_ON); 9823 9824 /** 9825 * Constant for fluent queries to be used to add include statements. Specifies 9826 * the path value of "<b>PlanDefinition:depends-on</b>". 9827 */ 9828 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEPENDS_ON = new ca.uhn.fhir.model.api.Include( 9829 "PlanDefinition:depends-on").toLocked(); 9830 9831 /** 9832 * Search parameter: <b>name</b> 9833 * <p> 9834 * Description: <b>Computationally friendly name of the plan definition</b><br> 9835 * Type: <b>string</b><br> 9836 * Path: <b>PlanDefinition.name</b><br> 9837 * </p> 9838 */ 9839 @SearchParamDefinition(name = "name", path = "PlanDefinition.name", description = "Computationally friendly name of the plan definition", type = "string") 9840 public static final String SP_NAME = "name"; 9841 /** 9842 * <b>Fluent Client</b> search parameter constant for <b>name</b> 9843 * <p> 9844 * Description: <b>Computationally friendly name of the plan definition</b><br> 9845 * Type: <b>string</b><br> 9846 * Path: <b>PlanDefinition.name</b><br> 9847 * </p> 9848 */ 9849 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 9850 SP_NAME); 9851 9852 /** 9853 * Search parameter: <b>context</b> 9854 * <p> 9855 * Description: <b>A use context assigned to the plan definition</b><br> 9856 * Type: <b>token</b><br> 9857 * Path: <b>PlanDefinition.useContext.valueCodeableConcept</b><br> 9858 * </p> 9859 */ 9860 @SearchParamDefinition(name = "context", path = "(PlanDefinition.useContext.value as CodeableConcept)", description = "A use context assigned to the plan definition", type = "token") 9861 public static final String SP_CONTEXT = "context"; 9862 /** 9863 * <b>Fluent Client</b> search parameter constant for <b>context</b> 9864 * <p> 9865 * Description: <b>A use context assigned to the plan definition</b><br> 9866 * Type: <b>token</b><br> 9867 * Path: <b>PlanDefinition.useContext.valueCodeableConcept</b><br> 9868 * </p> 9869 */ 9870 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 9871 SP_CONTEXT); 9872 9873 /** 9874 * Search parameter: <b>publisher</b> 9875 * <p> 9876 * Description: <b>Name of the publisher of the plan definition</b><br> 9877 * Type: <b>string</b><br> 9878 * Path: <b>PlanDefinition.publisher</b><br> 9879 * </p> 9880 */ 9881 @SearchParamDefinition(name = "publisher", path = "PlanDefinition.publisher", description = "Name of the publisher of the plan definition", type = "string") 9882 public static final String SP_PUBLISHER = "publisher"; 9883 /** 9884 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 9885 * <p> 9886 * Description: <b>Name of the publisher of the plan definition</b><br> 9887 * Type: <b>string</b><br> 9888 * Path: <b>PlanDefinition.publisher</b><br> 9889 * </p> 9890 */ 9891 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 9892 SP_PUBLISHER); 9893 9894 /** 9895 * Search parameter: <b>topic</b> 9896 * <p> 9897 * Description: <b>Topics associated with the module</b><br> 9898 * Type: <b>token</b><br> 9899 * Path: <b>PlanDefinition.topic</b><br> 9900 * </p> 9901 */ 9902 @SearchParamDefinition(name = "topic", path = "PlanDefinition.topic", description = "Topics associated with the module", type = "token") 9903 public static final String SP_TOPIC = "topic"; 9904 /** 9905 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 9906 * <p> 9907 * Description: <b>Topics associated with the module</b><br> 9908 * Type: <b>token</b><br> 9909 * Path: <b>PlanDefinition.topic</b><br> 9910 * </p> 9911 */ 9912 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam( 9913 SP_TOPIC); 9914 9915 /** 9916 * Search parameter: <b>definition</b> 9917 * <p> 9918 * Description: <b>Activity or plan definitions used by plan definition</b><br> 9919 * Type: <b>reference</b><br> 9920 * Path: <b>PlanDefinition.action.definition[x]</b><br> 9921 * </p> 9922 */ 9923 @SearchParamDefinition(name = "definition", path = "PlanDefinition.action.definition", description = "Activity or plan definitions used by plan definition", type = "reference", target = { 9924 ActivityDefinition.class, PlanDefinition.class, Questionnaire.class }) 9925 public static final String SP_DEFINITION = "definition"; 9926 /** 9927 * <b>Fluent Client</b> search parameter constant for <b>definition</b> 9928 * <p> 9929 * Description: <b>Activity or plan definitions used by plan definition</b><br> 9930 * Type: <b>reference</b><br> 9931 * Path: <b>PlanDefinition.action.definition[x]</b><br> 9932 * </p> 9933 */ 9934 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEFINITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 9935 SP_DEFINITION); 9936 9937 /** 9938 * Constant for fluent queries to be used to add include statements. Specifies 9939 * the path value of "<b>PlanDefinition:definition</b>". 9940 */ 9941 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEFINITION = new ca.uhn.fhir.model.api.Include( 9942 "PlanDefinition:definition").toLocked(); 9943 9944 /** 9945 * Search parameter: <b>context-type-quantity</b> 9946 * <p> 9947 * Description: <b>A use context type and quantity- or range-based value 9948 * assigned to the plan definition</b><br> 9949 * Type: <b>composite</b><br> 9950 * Path: <b></b><br> 9951 * </p> 9952 */ 9953 @SearchParamDefinition(name = "context-type-quantity", path = "PlanDefinition.useContext", description = "A use context type and quantity- or range-based value assigned to the plan definition", type = "composite", compositeOf = { 9954 "context-type", "context-quantity" }) 9955 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 9956 /** 9957 * <b>Fluent Client</b> search parameter constant for 9958 * <b>context-type-quantity</b> 9959 * <p> 9960 * Description: <b>A use context type and quantity- or range-based value 9961 * assigned to the plan definition</b><br> 9962 * Type: <b>composite</b><br> 9963 * Path: <b></b><br> 9964 * </p> 9965 */ 9966 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 9967 SP_CONTEXT_TYPE_QUANTITY); 9968 9969 /** 9970 * Search parameter: <b>status</b> 9971 * <p> 9972 * Description: <b>The current status of the plan definition</b><br> 9973 * Type: <b>token</b><br> 9974 * Path: <b>PlanDefinition.status</b><br> 9975 * </p> 9976 */ 9977 @SearchParamDefinition(name = "status", path = "PlanDefinition.status", description = "The current status of the plan definition", type = "token") 9978 public static final String SP_STATUS = "status"; 9979 /** 9980 * <b>Fluent Client</b> search parameter constant for <b>status</b> 9981 * <p> 9982 * Description: <b>The current status of the plan definition</b><br> 9983 * Type: <b>token</b><br> 9984 * Path: <b>PlanDefinition.status</b><br> 9985 * </p> 9986 */ 9987 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 9988 SP_STATUS); 9989 9990}