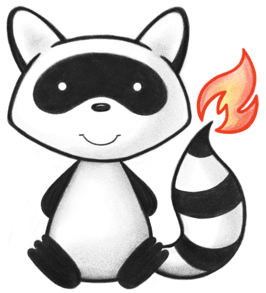
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.List; 034 035import org.hl7.fhir.exceptions.FHIRException; 036import org.hl7.fhir.instance.model.api.ICompositeType; 037 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.DatatypeDef; 040import ca.uhn.fhir.model.api.annotation.Description; 041 042/** 043 * A populatioof people with some set of grouping criteria. 044 */ 045@DatatypeDef(name = "Population") 046public class Population extends BackboneType implements ICompositeType { 047 048 /** 049 * The age of the specific population. 050 */ 051 @Child(name = "age", type = { Range.class, 052 CodeableConcept.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 053 @Description(shortDefinition = "The age of the specific population", formalDefinition = "The age of the specific population.") 054 protected Type age; 055 056 /** 057 * The gender of the specific population. 058 */ 059 @Child(name = "gender", type = { 060 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 061 @Description(shortDefinition = "The gender of the specific population", formalDefinition = "The gender of the specific population.") 062 protected CodeableConcept gender; 063 064 /** 065 * Race of the specific population. 066 */ 067 @Child(name = "race", type = { CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 068 @Description(shortDefinition = "Race of the specific population", formalDefinition = "Race of the specific population.") 069 protected CodeableConcept race; 070 071 /** 072 * The existing physiological conditions of the specific population to which 073 * this applies. 074 */ 075 @Child(name = "physiologicalCondition", type = { 076 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 077 @Description(shortDefinition = "The existing physiological conditions of the specific population to which this applies", formalDefinition = "The existing physiological conditions of the specific population to which this applies.") 078 protected CodeableConcept physiologicalCondition; 079 080 private static final long serialVersionUID = 495777760L; 081 082 /** 083 * Constructor 084 */ 085 public Population() { 086 super(); 087 } 088 089 /** 090 * @return {@link #age} (The age of the specific population.) 091 */ 092 public Type getAge() { 093 return this.age; 094 } 095 096 /** 097 * @return {@link #age} (The age of the specific population.) 098 */ 099 public Range getAgeRange() throws FHIRException { 100 if (this.age == null) 101 this.age = new Range(); 102 if (!(this.age instanceof Range)) 103 throw new FHIRException( 104 "Type mismatch: the type Range was expected, but " + this.age.getClass().getName() + " was encountered"); 105 return (Range) this.age; 106 } 107 108 public boolean hasAgeRange() { 109 return this.age instanceof Range; 110 } 111 112 /** 113 * @return {@link #age} (The age of the specific population.) 114 */ 115 public CodeableConcept getAgeCodeableConcept() throws FHIRException { 116 if (this.age == null) 117 this.age = new CodeableConcept(); 118 if (!(this.age instanceof CodeableConcept)) 119 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 120 + this.age.getClass().getName() + " was encountered"); 121 return (CodeableConcept) this.age; 122 } 123 124 public boolean hasAgeCodeableConcept() { 125 return this.age instanceof CodeableConcept; 126 } 127 128 public boolean hasAge() { 129 return this.age != null && !this.age.isEmpty(); 130 } 131 132 /** 133 * @param value {@link #age} (The age of the specific population.) 134 */ 135 public Population setAge(Type value) { 136 if (value != null && !(value instanceof Range || value instanceof CodeableConcept)) 137 throw new Error("Not the right type for Population.age[x]: " + value.fhirType()); 138 this.age = value; 139 return this; 140 } 141 142 /** 143 * @return {@link #gender} (The gender of the specific population.) 144 */ 145 public CodeableConcept getGender() { 146 if (this.gender == null) 147 if (Configuration.errorOnAutoCreate()) 148 throw new Error("Attempt to auto-create Population.gender"); 149 else if (Configuration.doAutoCreate()) 150 this.gender = new CodeableConcept(); // cc 151 return this.gender; 152 } 153 154 public boolean hasGender() { 155 return this.gender != null && !this.gender.isEmpty(); 156 } 157 158 /** 159 * @param value {@link #gender} (The gender of the specific population.) 160 */ 161 public Population setGender(CodeableConcept value) { 162 this.gender = value; 163 return this; 164 } 165 166 /** 167 * @return {@link #race} (Race of the specific population.) 168 */ 169 public CodeableConcept getRace() { 170 if (this.race == null) 171 if (Configuration.errorOnAutoCreate()) 172 throw new Error("Attempt to auto-create Population.race"); 173 else if (Configuration.doAutoCreate()) 174 this.race = new CodeableConcept(); // cc 175 return this.race; 176 } 177 178 public boolean hasRace() { 179 return this.race != null && !this.race.isEmpty(); 180 } 181 182 /** 183 * @param value {@link #race} (Race of the specific population.) 184 */ 185 public Population setRace(CodeableConcept value) { 186 this.race = value; 187 return this; 188 } 189 190 /** 191 * @return {@link #physiologicalCondition} (The existing physiological 192 * conditions of the specific population to which this applies.) 193 */ 194 public CodeableConcept getPhysiologicalCondition() { 195 if (this.physiologicalCondition == null) 196 if (Configuration.errorOnAutoCreate()) 197 throw new Error("Attempt to auto-create Population.physiologicalCondition"); 198 else if (Configuration.doAutoCreate()) 199 this.physiologicalCondition = new CodeableConcept(); // cc 200 return this.physiologicalCondition; 201 } 202 203 public boolean hasPhysiologicalCondition() { 204 return this.physiologicalCondition != null && !this.physiologicalCondition.isEmpty(); 205 } 206 207 /** 208 * @param value {@link #physiologicalCondition} (The existing physiological 209 * conditions of the specific population to which this applies.) 210 */ 211 public Population setPhysiologicalCondition(CodeableConcept value) { 212 this.physiologicalCondition = value; 213 return this; 214 } 215 216 protected void listChildren(List<Property> children) { 217 super.listChildren(children); 218 children.add(new Property("age[x]", "Range|CodeableConcept", "The age of the specific population.", 0, 1, age)); 219 children.add(new Property("gender", "CodeableConcept", "The gender of the specific population.", 0, 1, gender)); 220 children.add(new Property("race", "CodeableConcept", "Race of the specific population.", 0, 1, race)); 221 children.add(new Property("physiologicalCondition", "CodeableConcept", 222 "The existing physiological conditions of the specific population to which this applies.", 0, 1, 223 physiologicalCondition)); 224 } 225 226 @Override 227 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 228 switch (_hash) { 229 case -1419716831: 230 /* age[x] */ return new Property("age[x]", "Range|CodeableConcept", "The age of the specific population.", 0, 1, 231 age); 232 case 96511: 233 /* age */ return new Property("age[x]", "Range|CodeableConcept", "The age of the specific population.", 0, 1, 234 age); 235 case 1442748286: 236 /* ageRange */ return new Property("age[x]", "Range|CodeableConcept", "The age of the specific population.", 0, 1, 237 age); 238 case -1452658526: 239 /* ageCodeableConcept */ return new Property("age[x]", "Range|CodeableConcept", 240 "The age of the specific population.", 0, 1, age); 241 case -1249512767: 242 /* gender */ return new Property("gender", "CodeableConcept", "The gender of the specific population.", 0, 1, 243 gender); 244 case 3492561: 245 /* race */ return new Property("race", "CodeableConcept", "Race of the specific population.", 0, 1, race); 246 case -62715190: 247 /* physiologicalCondition */ return new Property("physiologicalCondition", "CodeableConcept", 248 "The existing physiological conditions of the specific population to which this applies.", 0, 1, 249 physiologicalCondition); 250 default: 251 return super.getNamedProperty(_hash, _name, _checkValid); 252 } 253 254 } 255 256 @Override 257 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 258 switch (hash) { 259 case 96511: 260 /* age */ return this.age == null ? new Base[0] : new Base[] { this.age }; // Type 261 case -1249512767: 262 /* gender */ return this.gender == null ? new Base[0] : new Base[] { this.gender }; // CodeableConcept 263 case 3492561: 264 /* race */ return this.race == null ? new Base[0] : new Base[] { this.race }; // CodeableConcept 265 case -62715190: 266 /* physiologicalCondition */ return this.physiologicalCondition == null ? new Base[0] 267 : new Base[] { this.physiologicalCondition }; // CodeableConcept 268 default: 269 return super.getProperty(hash, name, checkValid); 270 } 271 272 } 273 274 @Override 275 public Base setProperty(int hash, String name, Base value) throws FHIRException { 276 switch (hash) { 277 case 96511: // age 278 this.age = castToType(value); // Type 279 return value; 280 case -1249512767: // gender 281 this.gender = castToCodeableConcept(value); // CodeableConcept 282 return value; 283 case 3492561: // race 284 this.race = castToCodeableConcept(value); // CodeableConcept 285 return value; 286 case -62715190: // physiologicalCondition 287 this.physiologicalCondition = castToCodeableConcept(value); // CodeableConcept 288 return value; 289 default: 290 return super.setProperty(hash, name, value); 291 } 292 293 } 294 295 @Override 296 public Base setProperty(String name, Base value) throws FHIRException { 297 if (name.equals("age[x]")) { 298 this.age = castToType(value); // Type 299 } else if (name.equals("gender")) { 300 this.gender = castToCodeableConcept(value); // CodeableConcept 301 } else if (name.equals("race")) { 302 this.race = castToCodeableConcept(value); // CodeableConcept 303 } else if (name.equals("physiologicalCondition")) { 304 this.physiologicalCondition = castToCodeableConcept(value); // CodeableConcept 305 } else 306 return super.setProperty(name, value); 307 return value; 308 } 309 310 @Override 311 public void removeChild(String name, Base value) throws FHIRException { 312 if (name.equals("age[x]")) { 313 this.age = null; 314 } else if (name.equals("gender")) { 315 this.gender = null; 316 } else if (name.equals("race")) { 317 this.race = null; 318 } else if (name.equals("physiologicalCondition")) { 319 this.physiologicalCondition = null; 320 } else 321 super.removeChild(name, value); 322 323 } 324 325 @Override 326 public Base makeProperty(int hash, String name) throws FHIRException { 327 switch (hash) { 328 case -1419716831: 329 return getAge(); 330 case 96511: 331 return getAge(); 332 case -1249512767: 333 return getGender(); 334 case 3492561: 335 return getRace(); 336 case -62715190: 337 return getPhysiologicalCondition(); 338 default: 339 return super.makeProperty(hash, name); 340 } 341 342 } 343 344 @Override 345 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 346 switch (hash) { 347 case 96511: 348 /* age */ return new String[] { "Range", "CodeableConcept" }; 349 case -1249512767: 350 /* gender */ return new String[] { "CodeableConcept" }; 351 case 3492561: 352 /* race */ return new String[] { "CodeableConcept" }; 353 case -62715190: 354 /* physiologicalCondition */ return new String[] { "CodeableConcept" }; 355 default: 356 return super.getTypesForProperty(hash, name); 357 } 358 359 } 360 361 @Override 362 public Base addChild(String name) throws FHIRException { 363 if (name.equals("ageRange")) { 364 this.age = new Range(); 365 return this.age; 366 } else if (name.equals("ageCodeableConcept")) { 367 this.age = new CodeableConcept(); 368 return this.age; 369 } else if (name.equals("gender")) { 370 this.gender = new CodeableConcept(); 371 return this.gender; 372 } else if (name.equals("race")) { 373 this.race = new CodeableConcept(); 374 return this.race; 375 } else if (name.equals("physiologicalCondition")) { 376 this.physiologicalCondition = new CodeableConcept(); 377 return this.physiologicalCondition; 378 } else 379 return super.addChild(name); 380 } 381 382 public String fhirType() { 383 return "Population"; 384 385 } 386 387 public Population copy() { 388 Population dst = new Population(); 389 copyValues(dst); 390 return dst; 391 } 392 393 public void copyValues(Population dst) { 394 super.copyValues(dst); 395 dst.age = age == null ? null : age.copy(); 396 dst.gender = gender == null ? null : gender.copy(); 397 dst.race = race == null ? null : race.copy(); 398 dst.physiologicalCondition = physiologicalCondition == null ? null : physiologicalCondition.copy(); 399 } 400 401 protected Population typedCopy() { 402 return copy(); 403 } 404 405 @Override 406 public boolean equalsDeep(Base other_) { 407 if (!super.equalsDeep(other_)) 408 return false; 409 if (!(other_ instanceof Population)) 410 return false; 411 Population o = (Population) other_; 412 return compareDeep(age, o.age, true) && compareDeep(gender, o.gender, true) && compareDeep(race, o.race, true) 413 && compareDeep(physiologicalCondition, o.physiologicalCondition, true); 414 } 415 416 @Override 417 public boolean equalsShallow(Base other_) { 418 if (!super.equalsShallow(other_)) 419 return false; 420 if (!(other_ instanceof Population)) 421 return false; 422 Population o = (Population) other_; 423 return true; 424 } 425 426 public boolean isEmpty() { 427 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(age, gender, race, physiologicalCondition); 428 } 429 430}