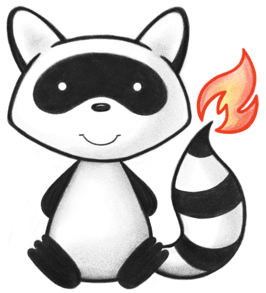
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.ICompositeType; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.DatatypeDef; 042import ca.uhn.fhir.model.api.annotation.Description; 043 044/** 045 * The marketing status describes the date when a medicinal product is actually 046 * put on the market or the date as of which it is no longer available. 047 */ 048@DatatypeDef(name = "ProdCharacteristic") 049public class ProdCharacteristic extends BackboneType implements ICompositeType { 050 051 /** 052 * Where applicable, the height can be specified using a numerical value and its 053 * unit of measurement The unit of measurement shall be specified in accordance 054 * with ISO 11240 and the resulting terminology The symbol and the symbol 055 * identifier shall be used. 056 */ 057 @Child(name = "height", type = { Quantity.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 058 @Description(shortDefinition = "Where applicable, the height can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used", formalDefinition = "Where applicable, the height can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.") 059 protected Quantity height; 060 061 /** 062 * Where applicable, the width can be specified using a numerical value and its 063 * unit of measurement The unit of measurement shall be specified in accordance 064 * with ISO 11240 and the resulting terminology The symbol and the symbol 065 * identifier shall be used. 066 */ 067 @Child(name = "width", type = { Quantity.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 068 @Description(shortDefinition = "Where applicable, the width can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used", formalDefinition = "Where applicable, the width can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.") 069 protected Quantity width; 070 071 /** 072 * Where applicable, the depth can be specified using a numerical value and its 073 * unit of measurement The unit of measurement shall be specified in accordance 074 * with ISO 11240 and the resulting terminology The symbol and the symbol 075 * identifier shall be used. 076 */ 077 @Child(name = "depth", type = { Quantity.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 078 @Description(shortDefinition = "Where applicable, the depth can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used", formalDefinition = "Where applicable, the depth can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.") 079 protected Quantity depth; 080 081 /** 082 * Where applicable, the weight can be specified using a numerical value and its 083 * unit of measurement The unit of measurement shall be specified in accordance 084 * with ISO 11240 and the resulting terminology The symbol and the symbol 085 * identifier shall be used. 086 */ 087 @Child(name = "weight", type = { Quantity.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 088 @Description(shortDefinition = "Where applicable, the weight can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used", formalDefinition = "Where applicable, the weight can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.") 089 protected Quantity weight; 090 091 /** 092 * Where applicable, the nominal volume can be specified using a numerical value 093 * and its unit of measurement The unit of measurement shall be specified in 094 * accordance with ISO 11240 and the resulting terminology The symbol and the 095 * symbol identifier shall be used. 096 */ 097 @Child(name = "nominalVolume", type = { 098 Quantity.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 099 @Description(shortDefinition = "Where applicable, the nominal volume can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used", formalDefinition = "Where applicable, the nominal volume can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.") 100 protected Quantity nominalVolume; 101 102 /** 103 * Where applicable, the external diameter can be specified using a numerical 104 * value and its unit of measurement The unit of measurement shall be specified 105 * in accordance with ISO 11240 and the resulting terminology The symbol and the 106 * symbol identifier shall be used. 107 */ 108 @Child(name = "externalDiameter", type = { 109 Quantity.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 110 @Description(shortDefinition = "Where applicable, the external diameter can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used", formalDefinition = "Where applicable, the external diameter can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.") 111 protected Quantity externalDiameter; 112 113 /** 114 * Where applicable, the shape can be specified An appropriate controlled 115 * vocabulary shall be used The term and the term identifier shall be used. 116 */ 117 @Child(name = "shape", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 118 @Description(shortDefinition = "Where applicable, the shape can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used", formalDefinition = "Where applicable, the shape can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used.") 119 protected StringType shape; 120 121 /** 122 * Where applicable, the color can be specified An appropriate controlled 123 * vocabulary shall be used The term and the term identifier shall be used. 124 */ 125 @Child(name = "color", type = { 126 StringType.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 127 @Description(shortDefinition = "Where applicable, the color can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used", formalDefinition = "Where applicable, the color can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used.") 128 protected List<StringType> color; 129 130 /** 131 * Where applicable, the imprint can be specified as text. 132 */ 133 @Child(name = "imprint", type = { 134 StringType.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 135 @Description(shortDefinition = "Where applicable, the imprint can be specified as text", formalDefinition = "Where applicable, the imprint can be specified as text.") 136 protected List<StringType> imprint; 137 138 /** 139 * Where applicable, the image can be provided The format of the image 140 * attachment shall be specified by regional implementations. 141 */ 142 @Child(name = "image", type = { 143 Attachment.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 144 @Description(shortDefinition = "Where applicable, the image can be provided The format of the image attachment shall be specified by regional implementations", formalDefinition = "Where applicable, the image can be provided The format of the image attachment shall be specified by regional implementations.") 145 protected List<Attachment> image; 146 147 /** 148 * Where applicable, the scoring can be specified An appropriate controlled 149 * vocabulary shall be used The term and the term identifier shall be used. 150 */ 151 @Child(name = "scoring", type = { 152 CodeableConcept.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 153 @Description(shortDefinition = "Where applicable, the scoring can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used", formalDefinition = "Where applicable, the scoring can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used.") 154 protected CodeableConcept scoring; 155 156 private static final long serialVersionUID = 1521671432L; 157 158 /** 159 * Constructor 160 */ 161 public ProdCharacteristic() { 162 super(); 163 } 164 165 /** 166 * @return {@link #height} (Where applicable, the height can be specified using 167 * a numerical value and its unit of measurement The unit of measurement 168 * shall be specified in accordance with ISO 11240 and the resulting 169 * terminology The symbol and the symbol identifier shall be used.) 170 */ 171 public Quantity getHeight() { 172 if (this.height == null) 173 if (Configuration.errorOnAutoCreate()) 174 throw new Error("Attempt to auto-create ProdCharacteristic.height"); 175 else if (Configuration.doAutoCreate()) 176 this.height = new Quantity(); // cc 177 return this.height; 178 } 179 180 public boolean hasHeight() { 181 return this.height != null && !this.height.isEmpty(); 182 } 183 184 /** 185 * @param value {@link #height} (Where applicable, the height can be specified 186 * using a numerical value and its unit of measurement The unit of 187 * measurement shall be specified in accordance with ISO 11240 and 188 * the resulting terminology The symbol and the symbol identifier 189 * shall be used.) 190 */ 191 public ProdCharacteristic setHeight(Quantity value) { 192 this.height = value; 193 return this; 194 } 195 196 /** 197 * @return {@link #width} (Where applicable, the width can be specified using a 198 * numerical value and its unit of measurement The unit of measurement 199 * shall be specified in accordance with ISO 11240 and the resulting 200 * terminology The symbol and the symbol identifier shall be used.) 201 */ 202 public Quantity getWidth() { 203 if (this.width == null) 204 if (Configuration.errorOnAutoCreate()) 205 throw new Error("Attempt to auto-create ProdCharacteristic.width"); 206 else if (Configuration.doAutoCreate()) 207 this.width = new Quantity(); // cc 208 return this.width; 209 } 210 211 public boolean hasWidth() { 212 return this.width != null && !this.width.isEmpty(); 213 } 214 215 /** 216 * @param value {@link #width} (Where applicable, the width can be specified 217 * using a numerical value and its unit of measurement The unit of 218 * measurement shall be specified in accordance with ISO 11240 and 219 * the resulting terminology The symbol and the symbol identifier 220 * shall be used.) 221 */ 222 public ProdCharacteristic setWidth(Quantity value) { 223 this.width = value; 224 return this; 225 } 226 227 /** 228 * @return {@link #depth} (Where applicable, the depth can be specified using a 229 * numerical value and its unit of measurement The unit of measurement 230 * shall be specified in accordance with ISO 11240 and the resulting 231 * terminology The symbol and the symbol identifier shall be used.) 232 */ 233 public Quantity getDepth() { 234 if (this.depth == null) 235 if (Configuration.errorOnAutoCreate()) 236 throw new Error("Attempt to auto-create ProdCharacteristic.depth"); 237 else if (Configuration.doAutoCreate()) 238 this.depth = new Quantity(); // cc 239 return this.depth; 240 } 241 242 public boolean hasDepth() { 243 return this.depth != null && !this.depth.isEmpty(); 244 } 245 246 /** 247 * @param value {@link #depth} (Where applicable, the depth can be specified 248 * using a numerical value and its unit of measurement The unit of 249 * measurement shall be specified in accordance with ISO 11240 and 250 * the resulting terminology The symbol and the symbol identifier 251 * shall be used.) 252 */ 253 public ProdCharacteristic setDepth(Quantity value) { 254 this.depth = value; 255 return this; 256 } 257 258 /** 259 * @return {@link #weight} (Where applicable, the weight can be specified using 260 * a numerical value and its unit of measurement The unit of measurement 261 * shall be specified in accordance with ISO 11240 and the resulting 262 * terminology The symbol and the symbol identifier shall be used.) 263 */ 264 public Quantity getWeight() { 265 if (this.weight == null) 266 if (Configuration.errorOnAutoCreate()) 267 throw new Error("Attempt to auto-create ProdCharacteristic.weight"); 268 else if (Configuration.doAutoCreate()) 269 this.weight = new Quantity(); // cc 270 return this.weight; 271 } 272 273 public boolean hasWeight() { 274 return this.weight != null && !this.weight.isEmpty(); 275 } 276 277 /** 278 * @param value {@link #weight} (Where applicable, the weight can be specified 279 * using a numerical value and its unit of measurement The unit of 280 * measurement shall be specified in accordance with ISO 11240 and 281 * the resulting terminology The symbol and the symbol identifier 282 * shall be used.) 283 */ 284 public ProdCharacteristic setWeight(Quantity value) { 285 this.weight = value; 286 return this; 287 } 288 289 /** 290 * @return {@link #nominalVolume} (Where applicable, the nominal volume can be 291 * specified using a numerical value and its unit of measurement The 292 * unit of measurement shall be specified in accordance with ISO 11240 293 * and the resulting terminology The symbol and the symbol identifier 294 * shall be used.) 295 */ 296 public Quantity getNominalVolume() { 297 if (this.nominalVolume == null) 298 if (Configuration.errorOnAutoCreate()) 299 throw new Error("Attempt to auto-create ProdCharacteristic.nominalVolume"); 300 else if (Configuration.doAutoCreate()) 301 this.nominalVolume = new Quantity(); // cc 302 return this.nominalVolume; 303 } 304 305 public boolean hasNominalVolume() { 306 return this.nominalVolume != null && !this.nominalVolume.isEmpty(); 307 } 308 309 /** 310 * @param value {@link #nominalVolume} (Where applicable, the nominal volume can 311 * be specified using a numerical value and its unit of measurement 312 * The unit of measurement shall be specified in accordance with 313 * ISO 11240 and the resulting terminology The symbol and the 314 * symbol identifier shall be used.) 315 */ 316 public ProdCharacteristic setNominalVolume(Quantity value) { 317 this.nominalVolume = value; 318 return this; 319 } 320 321 /** 322 * @return {@link #externalDiameter} (Where applicable, the external diameter 323 * can be specified using a numerical value and its unit of measurement 324 * The unit of measurement shall be specified in accordance with ISO 325 * 11240 and the resulting terminology The symbol and the symbol 326 * identifier shall be used.) 327 */ 328 public Quantity getExternalDiameter() { 329 if (this.externalDiameter == null) 330 if (Configuration.errorOnAutoCreate()) 331 throw new Error("Attempt to auto-create ProdCharacteristic.externalDiameter"); 332 else if (Configuration.doAutoCreate()) 333 this.externalDiameter = new Quantity(); // cc 334 return this.externalDiameter; 335 } 336 337 public boolean hasExternalDiameter() { 338 return this.externalDiameter != null && !this.externalDiameter.isEmpty(); 339 } 340 341 /** 342 * @param value {@link #externalDiameter} (Where applicable, the external 343 * diameter can be specified using a numerical value and its unit 344 * of measurement The unit of measurement shall be specified in 345 * accordance with ISO 11240 and the resulting terminology The 346 * symbol and the symbol identifier shall be used.) 347 */ 348 public ProdCharacteristic setExternalDiameter(Quantity value) { 349 this.externalDiameter = value; 350 return this; 351 } 352 353 /** 354 * @return {@link #shape} (Where applicable, the shape can be specified An 355 * appropriate controlled vocabulary shall be used The term and the term 356 * identifier shall be used.). This is the underlying object with id, 357 * value and extensions. The accessor "getShape" gives direct access to 358 * the value 359 */ 360 public StringType getShapeElement() { 361 if (this.shape == null) 362 if (Configuration.errorOnAutoCreate()) 363 throw new Error("Attempt to auto-create ProdCharacteristic.shape"); 364 else if (Configuration.doAutoCreate()) 365 this.shape = new StringType(); // bb 366 return this.shape; 367 } 368 369 public boolean hasShapeElement() { 370 return this.shape != null && !this.shape.isEmpty(); 371 } 372 373 public boolean hasShape() { 374 return this.shape != null && !this.shape.isEmpty(); 375 } 376 377 /** 378 * @param value {@link #shape} (Where applicable, the shape can be specified An 379 * appropriate controlled vocabulary shall be used The term and the 380 * term identifier shall be used.). This is the underlying object 381 * with id, value and extensions. The accessor "getShape" gives 382 * direct access to the value 383 */ 384 public ProdCharacteristic setShapeElement(StringType value) { 385 this.shape = value; 386 return this; 387 } 388 389 /** 390 * @return Where applicable, the shape can be specified An appropriate 391 * controlled vocabulary shall be used The term and the term identifier 392 * shall be used. 393 */ 394 public String getShape() { 395 return this.shape == null ? null : this.shape.getValue(); 396 } 397 398 /** 399 * @param value Where applicable, the shape can be specified An appropriate 400 * controlled vocabulary shall be used The term and the term 401 * identifier shall be used. 402 */ 403 public ProdCharacteristic setShape(String value) { 404 if (Utilities.noString(value)) 405 this.shape = null; 406 else { 407 if (this.shape == null) 408 this.shape = new StringType(); 409 this.shape.setValue(value); 410 } 411 return this; 412 } 413 414 /** 415 * @return {@link #color} (Where applicable, the color can be specified An 416 * appropriate controlled vocabulary shall be used The term and the term 417 * identifier shall be used.) 418 */ 419 public List<StringType> getColor() { 420 if (this.color == null) 421 this.color = new ArrayList<StringType>(); 422 return this.color; 423 } 424 425 /** 426 * @return Returns a reference to <code>this</code> for easy method chaining 427 */ 428 public ProdCharacteristic setColor(List<StringType> theColor) { 429 this.color = theColor; 430 return this; 431 } 432 433 public boolean hasColor() { 434 if (this.color == null) 435 return false; 436 for (StringType item : this.color) 437 if (!item.isEmpty()) 438 return true; 439 return false; 440 } 441 442 /** 443 * @return {@link #color} (Where applicable, the color can be specified An 444 * appropriate controlled vocabulary shall be used The term and the term 445 * identifier shall be used.) 446 */ 447 public StringType addColorElement() {// 2 448 StringType t = new StringType(); 449 if (this.color == null) 450 this.color = new ArrayList<StringType>(); 451 this.color.add(t); 452 return t; 453 } 454 455 /** 456 * @param value {@link #color} (Where applicable, the color can be specified An 457 * appropriate controlled vocabulary shall be used The term and the 458 * term identifier shall be used.) 459 */ 460 public ProdCharacteristic addColor(String value) { // 1 461 StringType t = new StringType(); 462 t.setValue(value); 463 if (this.color == null) 464 this.color = new ArrayList<StringType>(); 465 this.color.add(t); 466 return this; 467 } 468 469 /** 470 * @param value {@link #color} (Where applicable, the color can be specified An 471 * appropriate controlled vocabulary shall be used The term and the 472 * term identifier shall be used.) 473 */ 474 public boolean hasColor(String value) { 475 if (this.color == null) 476 return false; 477 for (StringType v : this.color) 478 if (v.getValue().equals(value)) // string 479 return true; 480 return false; 481 } 482 483 /** 484 * @return {@link #imprint} (Where applicable, the imprint can be specified as 485 * text.) 486 */ 487 public List<StringType> getImprint() { 488 if (this.imprint == null) 489 this.imprint = new ArrayList<StringType>(); 490 return this.imprint; 491 } 492 493 /** 494 * @return Returns a reference to <code>this</code> for easy method chaining 495 */ 496 public ProdCharacteristic setImprint(List<StringType> theImprint) { 497 this.imprint = theImprint; 498 return this; 499 } 500 501 public boolean hasImprint() { 502 if (this.imprint == null) 503 return false; 504 for (StringType item : this.imprint) 505 if (!item.isEmpty()) 506 return true; 507 return false; 508 } 509 510 /** 511 * @return {@link #imprint} (Where applicable, the imprint can be specified as 512 * text.) 513 */ 514 public StringType addImprintElement() {// 2 515 StringType t = new StringType(); 516 if (this.imprint == null) 517 this.imprint = new ArrayList<StringType>(); 518 this.imprint.add(t); 519 return t; 520 } 521 522 /** 523 * @param value {@link #imprint} (Where applicable, the imprint can be specified 524 * as text.) 525 */ 526 public ProdCharacteristic addImprint(String value) { // 1 527 StringType t = new StringType(); 528 t.setValue(value); 529 if (this.imprint == null) 530 this.imprint = new ArrayList<StringType>(); 531 this.imprint.add(t); 532 return this; 533 } 534 535 /** 536 * @param value {@link #imprint} (Where applicable, the imprint can be specified 537 * as text.) 538 */ 539 public boolean hasImprint(String value) { 540 if (this.imprint == null) 541 return false; 542 for (StringType v : this.imprint) 543 if (v.getValue().equals(value)) // string 544 return true; 545 return false; 546 } 547 548 /** 549 * @return {@link #image} (Where applicable, the image can be provided The 550 * format of the image attachment shall be specified by regional 551 * implementations.) 552 */ 553 public List<Attachment> getImage() { 554 if (this.image == null) 555 this.image = new ArrayList<Attachment>(); 556 return this.image; 557 } 558 559 /** 560 * @return Returns a reference to <code>this</code> for easy method chaining 561 */ 562 public ProdCharacteristic setImage(List<Attachment> theImage) { 563 this.image = theImage; 564 return this; 565 } 566 567 public boolean hasImage() { 568 if (this.image == null) 569 return false; 570 for (Attachment item : this.image) 571 if (!item.isEmpty()) 572 return true; 573 return false; 574 } 575 576 public Attachment addImage() { // 3 577 Attachment t = new Attachment(); 578 if (this.image == null) 579 this.image = new ArrayList<Attachment>(); 580 this.image.add(t); 581 return t; 582 } 583 584 public ProdCharacteristic addImage(Attachment t) { // 3 585 if (t == null) 586 return this; 587 if (this.image == null) 588 this.image = new ArrayList<Attachment>(); 589 this.image.add(t); 590 return this; 591 } 592 593 /** 594 * @return The first repetition of repeating field {@link #image}, creating it 595 * if it does not already exist 596 */ 597 public Attachment getImageFirstRep() { 598 if (getImage().isEmpty()) { 599 addImage(); 600 } 601 return getImage().get(0); 602 } 603 604 /** 605 * @return {@link #scoring} (Where applicable, the scoring can be specified An 606 * appropriate controlled vocabulary shall be used The term and the term 607 * identifier shall be used.) 608 */ 609 public CodeableConcept getScoring() { 610 if (this.scoring == null) 611 if (Configuration.errorOnAutoCreate()) 612 throw new Error("Attempt to auto-create ProdCharacteristic.scoring"); 613 else if (Configuration.doAutoCreate()) 614 this.scoring = new CodeableConcept(); // cc 615 return this.scoring; 616 } 617 618 public boolean hasScoring() { 619 return this.scoring != null && !this.scoring.isEmpty(); 620 } 621 622 /** 623 * @param value {@link #scoring} (Where applicable, the scoring can be specified 624 * An appropriate controlled vocabulary shall be used The term and 625 * the term identifier shall be used.) 626 */ 627 public ProdCharacteristic setScoring(CodeableConcept value) { 628 this.scoring = value; 629 return this; 630 } 631 632 protected void listChildren(List<Property> children) { 633 super.listChildren(children); 634 children.add(new Property("height", "Quantity", 635 "Where applicable, the height can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 636 0, 1, height)); 637 children.add(new Property("width", "Quantity", 638 "Where applicable, the width can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 639 0, 1, width)); 640 children.add(new Property("depth", "Quantity", 641 "Where applicable, the depth can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 642 0, 1, depth)); 643 children.add(new Property("weight", "Quantity", 644 "Where applicable, the weight can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 645 0, 1, weight)); 646 children.add(new Property("nominalVolume", "Quantity", 647 "Where applicable, the nominal volume can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 648 0, 1, nominalVolume)); 649 children.add(new Property("externalDiameter", "Quantity", 650 "Where applicable, the external diameter can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 651 0, 1, externalDiameter)); 652 children.add(new Property("shape", "string", 653 "Where applicable, the shape can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used.", 654 0, 1, shape)); 655 children.add(new Property("color", "string", 656 "Where applicable, the color can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used.", 657 0, java.lang.Integer.MAX_VALUE, color)); 658 children.add(new Property("imprint", "string", "Where applicable, the imprint can be specified as text.", 0, 659 java.lang.Integer.MAX_VALUE, imprint)); 660 children.add(new Property("image", "Attachment", 661 "Where applicable, the image can be provided The format of the image attachment shall be specified by regional implementations.", 662 0, java.lang.Integer.MAX_VALUE, image)); 663 children.add(new Property("scoring", "CodeableConcept", 664 "Where applicable, the scoring can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used.", 665 0, 1, scoring)); 666 } 667 668 @Override 669 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 670 switch (_hash) { 671 case -1221029593: 672 /* height */ return new Property("height", "Quantity", 673 "Where applicable, the height can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 674 0, 1, height); 675 case 113126854: 676 /* width */ return new Property("width", "Quantity", 677 "Where applicable, the width can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 678 0, 1, width); 679 case 95472323: 680 /* depth */ return new Property("depth", "Quantity", 681 "Where applicable, the depth can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 682 0, 1, depth); 683 case -791592328: 684 /* weight */ return new Property("weight", "Quantity", 685 "Where applicable, the weight can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 686 0, 1, weight); 687 case 1706919702: 688 /* nominalVolume */ return new Property("nominalVolume", "Quantity", 689 "Where applicable, the nominal volume can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 690 0, 1, nominalVolume); 691 case 161374584: 692 /* externalDiameter */ return new Property("externalDiameter", "Quantity", 693 "Where applicable, the external diameter can be specified using a numerical value and its unit of measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 694 0, 1, externalDiameter); 695 case 109399969: 696 /* shape */ return new Property("shape", "string", 697 "Where applicable, the shape can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used.", 698 0, 1, shape); 699 case 94842723: 700 /* color */ return new Property("color", "string", 701 "Where applicable, the color can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used.", 702 0, java.lang.Integer.MAX_VALUE, color); 703 case 1926118409: 704 /* imprint */ return new Property("imprint", "string", "Where applicable, the imprint can be specified as text.", 705 0, java.lang.Integer.MAX_VALUE, imprint); 706 case 100313435: 707 /* image */ return new Property("image", "Attachment", 708 "Where applicable, the image can be provided The format of the image attachment shall be specified by regional implementations.", 709 0, java.lang.Integer.MAX_VALUE, image); 710 case 1924005583: 711 /* scoring */ return new Property("scoring", "CodeableConcept", 712 "Where applicable, the scoring can be specified An appropriate controlled vocabulary shall be used The term and the term identifier shall be used.", 713 0, 1, scoring); 714 default: 715 return super.getNamedProperty(_hash, _name, _checkValid); 716 } 717 718 } 719 720 @Override 721 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 722 switch (hash) { 723 case -1221029593: 724 /* height */ return this.height == null ? new Base[0] : new Base[] { this.height }; // Quantity 725 case 113126854: 726 /* width */ return this.width == null ? new Base[0] : new Base[] { this.width }; // Quantity 727 case 95472323: 728 /* depth */ return this.depth == null ? new Base[0] : new Base[] { this.depth }; // Quantity 729 case -791592328: 730 /* weight */ return this.weight == null ? new Base[0] : new Base[] { this.weight }; // Quantity 731 case 1706919702: 732 /* nominalVolume */ return this.nominalVolume == null ? new Base[0] : new Base[] { this.nominalVolume }; // Quantity 733 case 161374584: 734 /* externalDiameter */ return this.externalDiameter == null ? new Base[0] : new Base[] { this.externalDiameter }; // Quantity 735 case 109399969: 736 /* shape */ return this.shape == null ? new Base[0] : new Base[] { this.shape }; // StringType 737 case 94842723: 738 /* color */ return this.color == null ? new Base[0] : this.color.toArray(new Base[this.color.size()]); // StringType 739 case 1926118409: 740 /* imprint */ return this.imprint == null ? new Base[0] : this.imprint.toArray(new Base[this.imprint.size()]); // StringType 741 case 100313435: 742 /* image */ return this.image == null ? new Base[0] : this.image.toArray(new Base[this.image.size()]); // Attachment 743 case 1924005583: 744 /* scoring */ return this.scoring == null ? new Base[0] : new Base[] { this.scoring }; // CodeableConcept 745 default: 746 return super.getProperty(hash, name, checkValid); 747 } 748 749 } 750 751 @Override 752 public Base setProperty(int hash, String name, Base value) throws FHIRException { 753 switch (hash) { 754 case -1221029593: // height 755 this.height = castToQuantity(value); // Quantity 756 return value; 757 case 113126854: // width 758 this.width = castToQuantity(value); // Quantity 759 return value; 760 case 95472323: // depth 761 this.depth = castToQuantity(value); // Quantity 762 return value; 763 case -791592328: // weight 764 this.weight = castToQuantity(value); // Quantity 765 return value; 766 case 1706919702: // nominalVolume 767 this.nominalVolume = castToQuantity(value); // Quantity 768 return value; 769 case 161374584: // externalDiameter 770 this.externalDiameter = castToQuantity(value); // Quantity 771 return value; 772 case 109399969: // shape 773 this.shape = castToString(value); // StringType 774 return value; 775 case 94842723: // color 776 this.getColor().add(castToString(value)); // StringType 777 return value; 778 case 1926118409: // imprint 779 this.getImprint().add(castToString(value)); // StringType 780 return value; 781 case 100313435: // image 782 this.getImage().add(castToAttachment(value)); // Attachment 783 return value; 784 case 1924005583: // scoring 785 this.scoring = castToCodeableConcept(value); // CodeableConcept 786 return value; 787 default: 788 return super.setProperty(hash, name, value); 789 } 790 791 } 792 793 @Override 794 public Base setProperty(String name, Base value) throws FHIRException { 795 if (name.equals("height")) { 796 this.height = castToQuantity(value); // Quantity 797 } else if (name.equals("width")) { 798 this.width = castToQuantity(value); // Quantity 799 } else if (name.equals("depth")) { 800 this.depth = castToQuantity(value); // Quantity 801 } else if (name.equals("weight")) { 802 this.weight = castToQuantity(value); // Quantity 803 } else if (name.equals("nominalVolume")) { 804 this.nominalVolume = castToQuantity(value); // Quantity 805 } else if (name.equals("externalDiameter")) { 806 this.externalDiameter = castToQuantity(value); // Quantity 807 } else if (name.equals("shape")) { 808 this.shape = castToString(value); // StringType 809 } else if (name.equals("color")) { 810 this.getColor().add(castToString(value)); 811 } else if (name.equals("imprint")) { 812 this.getImprint().add(castToString(value)); 813 } else if (name.equals("image")) { 814 this.getImage().add(castToAttachment(value)); 815 } else if (name.equals("scoring")) { 816 this.scoring = castToCodeableConcept(value); // CodeableConcept 817 } else 818 return super.setProperty(name, value); 819 return value; 820 } 821 822 @Override 823 public void removeChild(String name, Base value) throws FHIRException { 824 if (name.equals("height")) { 825 this.height = null; 826 } else if (name.equals("width")) { 827 this.width = null; 828 } else if (name.equals("depth")) { 829 this.depth = null; 830 } else if (name.equals("weight")) { 831 this.weight = null; 832 } else if (name.equals("nominalVolume")) { 833 this.nominalVolume = null; 834 } else if (name.equals("externalDiameter")) { 835 this.externalDiameter = null; 836 } else if (name.equals("shape")) { 837 this.shape = null; 838 } else if (name.equals("color")) { 839 this.getColor().remove(castToString(value)); 840 } else if (name.equals("imprint")) { 841 this.getImprint().remove(castToString(value)); 842 } else if (name.equals("image")) { 843 this.getImage().remove(castToAttachment(value)); 844 } else if (name.equals("scoring")) { 845 this.scoring = null; 846 } else 847 super.removeChild(name, value); 848 849 } 850 851 @Override 852 public Base makeProperty(int hash, String name) throws FHIRException { 853 switch (hash) { 854 case -1221029593: 855 return getHeight(); 856 case 113126854: 857 return getWidth(); 858 case 95472323: 859 return getDepth(); 860 case -791592328: 861 return getWeight(); 862 case 1706919702: 863 return getNominalVolume(); 864 case 161374584: 865 return getExternalDiameter(); 866 case 109399969: 867 return getShapeElement(); 868 case 94842723: 869 return addColorElement(); 870 case 1926118409: 871 return addImprintElement(); 872 case 100313435: 873 return addImage(); 874 case 1924005583: 875 return getScoring(); 876 default: 877 return super.makeProperty(hash, name); 878 } 879 880 } 881 882 @Override 883 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 884 switch (hash) { 885 case -1221029593: 886 /* height */ return new String[] { "Quantity" }; 887 case 113126854: 888 /* width */ return new String[] { "Quantity" }; 889 case 95472323: 890 /* depth */ return new String[] { "Quantity" }; 891 case -791592328: 892 /* weight */ return new String[] { "Quantity" }; 893 case 1706919702: 894 /* nominalVolume */ return new String[] { "Quantity" }; 895 case 161374584: 896 /* externalDiameter */ return new String[] { "Quantity" }; 897 case 109399969: 898 /* shape */ return new String[] { "string" }; 899 case 94842723: 900 /* color */ return new String[] { "string" }; 901 case 1926118409: 902 /* imprint */ return new String[] { "string" }; 903 case 100313435: 904 /* image */ return new String[] { "Attachment" }; 905 case 1924005583: 906 /* scoring */ return new String[] { "CodeableConcept" }; 907 default: 908 return super.getTypesForProperty(hash, name); 909 } 910 911 } 912 913 @Override 914 public Base addChild(String name) throws FHIRException { 915 if (name.equals("height")) { 916 this.height = new Quantity(); 917 return this.height; 918 } else if (name.equals("width")) { 919 this.width = new Quantity(); 920 return this.width; 921 } else if (name.equals("depth")) { 922 this.depth = new Quantity(); 923 return this.depth; 924 } else if (name.equals("weight")) { 925 this.weight = new Quantity(); 926 return this.weight; 927 } else if (name.equals("nominalVolume")) { 928 this.nominalVolume = new Quantity(); 929 return this.nominalVolume; 930 } else if (name.equals("externalDiameter")) { 931 this.externalDiameter = new Quantity(); 932 return this.externalDiameter; 933 } else if (name.equals("shape")) { 934 throw new FHIRException("Cannot call addChild on a singleton property ProdCharacteristic.shape"); 935 } else if (name.equals("color")) { 936 throw new FHIRException("Cannot call addChild on a singleton property ProdCharacteristic.color"); 937 } else if (name.equals("imprint")) { 938 throw new FHIRException("Cannot call addChild on a singleton property ProdCharacteristic.imprint"); 939 } else if (name.equals("image")) { 940 return addImage(); 941 } else if (name.equals("scoring")) { 942 this.scoring = new CodeableConcept(); 943 return this.scoring; 944 } else 945 return super.addChild(name); 946 } 947 948 public String fhirType() { 949 return "ProdCharacteristic"; 950 951 } 952 953 public ProdCharacteristic copy() { 954 ProdCharacteristic dst = new ProdCharacteristic(); 955 copyValues(dst); 956 return dst; 957 } 958 959 public void copyValues(ProdCharacteristic dst) { 960 super.copyValues(dst); 961 dst.height = height == null ? null : height.copy(); 962 dst.width = width == null ? null : width.copy(); 963 dst.depth = depth == null ? null : depth.copy(); 964 dst.weight = weight == null ? null : weight.copy(); 965 dst.nominalVolume = nominalVolume == null ? null : nominalVolume.copy(); 966 dst.externalDiameter = externalDiameter == null ? null : externalDiameter.copy(); 967 dst.shape = shape == null ? null : shape.copy(); 968 if (color != null) { 969 dst.color = new ArrayList<StringType>(); 970 for (StringType i : color) 971 dst.color.add(i.copy()); 972 } 973 ; 974 if (imprint != null) { 975 dst.imprint = new ArrayList<StringType>(); 976 for (StringType i : imprint) 977 dst.imprint.add(i.copy()); 978 } 979 ; 980 if (image != null) { 981 dst.image = new ArrayList<Attachment>(); 982 for (Attachment i : image) 983 dst.image.add(i.copy()); 984 } 985 ; 986 dst.scoring = scoring == null ? null : scoring.copy(); 987 } 988 989 protected ProdCharacteristic typedCopy() { 990 return copy(); 991 } 992 993 @Override 994 public boolean equalsDeep(Base other_) { 995 if (!super.equalsDeep(other_)) 996 return false; 997 if (!(other_ instanceof ProdCharacteristic)) 998 return false; 999 ProdCharacteristic o = (ProdCharacteristic) other_; 1000 return compareDeep(height, o.height, true) && compareDeep(width, o.width, true) && compareDeep(depth, o.depth, true) 1001 && compareDeep(weight, o.weight, true) && compareDeep(nominalVolume, o.nominalVolume, true) 1002 && compareDeep(externalDiameter, o.externalDiameter, true) && compareDeep(shape, o.shape, true) 1003 && compareDeep(color, o.color, true) && compareDeep(imprint, o.imprint, true) 1004 && compareDeep(image, o.image, true) && compareDeep(scoring, o.scoring, true); 1005 } 1006 1007 @Override 1008 public boolean equalsShallow(Base other_) { 1009 if (!super.equalsShallow(other_)) 1010 return false; 1011 if (!(other_ instanceof ProdCharacteristic)) 1012 return false; 1013 ProdCharacteristic o = (ProdCharacteristic) other_; 1014 return compareValues(shape, o.shape, true) && compareValues(color, o.color, true) 1015 && compareValues(imprint, o.imprint, true); 1016 } 1017 1018 public boolean isEmpty() { 1019 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(height, width, depth, weight, nominalVolume, 1020 externalDiameter, shape, color, imprint, image, scoring); 1021 } 1022 1023}