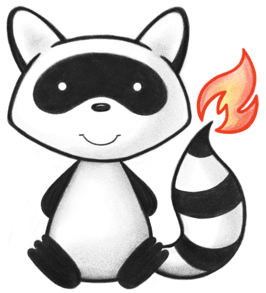
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032import java.util.ArrayList; 033import java.util.List; 034 035import org.hl7.fhir.utilities.MergedList.IMatcher; 036 037/** 038 * A child element or property defined by the FHIR specification This class is 039 * defined as a helper class when iterating the children of an element in a 040 * generic fashion 041 * 042 * At present, iteration is only based on the specification, but this may be 043 * changed to allow profile based expression at a later date 044 * 045 * note: there's no point in creating one of these classes outside this package 046 */ 047public class Property { 048 049 public static class PropertyMatcher implements IMatcher<Property> { 050 051 @Override 052 public boolean match(Property l, Property r) { 053 return l.getName().equals(r.getName()); 054 } 055 056 } 057 058 /** 059 * The name of the property as found in the FHIR specification 060 */ 061 private String name; 062 063 /** 064 * The type of the property as specified in the FHIR specification (e.g. 065 * type|type|Reference(Name|Name) 066 */ 067 private String typeCode; 068 069 /** 070 * The formal definition of the element given in the FHIR specification 071 */ 072 private String definition; 073 074 /** 075 * The minimum allowed cardinality - 0 or 1 when based on the specification 076 */ 077 private int minCardinality; 078 079 /** 080 * The maximum allowed cardinality - 1 or MAX_INT when based on the 081 * specification 082 */ 083 private int maxCardinality; 084 085 /** 086 * The actual elements that exist on this instance 087 */ 088 private List<Base> values = new ArrayList<Base>(); 089 090 /** 091 * For run time, if/once a property is hooked up to it's definition 092 */ 093 private StructureDefinition structure; 094 095 /** 096 * Internal constructor 097 */ 098 public Property(String name, String typeCode, String definition, int minCardinality, int maxCardinality, Base value) { 099 super(); 100 this.name = name; 101 this.typeCode = typeCode; 102 this.definition = definition; 103 this.minCardinality = minCardinality; 104 this.maxCardinality = maxCardinality; 105 if (value != null) 106 this.values.add(value); 107 } 108 109 /** 110 * Internal constructor 111 */ 112 public Property(String name, String typeCode, String definition, int minCardinality, int maxCardinality, 113 List<? extends Base> values) { 114 super(); 115 this.name = name; 116 this.typeCode = typeCode; 117 this.definition = definition; 118 this.minCardinality = minCardinality; 119 this.maxCardinality = maxCardinality; 120 if (values != null) 121 this.values.addAll(values); 122 } 123 124 /** 125 * @return The name of this property in the FHIR Specification 126 */ 127 public String getName() { 128 return name; 129 } 130 131 /** 132 * @return The stated type in the FHIR specification 133 */ 134 public String getTypeCode() { 135 return typeCode; 136 } 137 138 /** 139 * @return The definition of this element in the FHIR spec 140 */ 141 public String getDefinition() { 142 return definition; 143 } 144 145 /** 146 * @return the minimum cardinality for this element 147 */ 148 public int getMinCardinality() { 149 return minCardinality; 150 } 151 152 /** 153 * @return the maximum cardinality for this element 154 */ 155 public int getMaxCardinality() { 156 return maxCardinality; 157 } 158 159 /** 160 * @return the actual values - will only be 1 unless maximum cardinality == 161 * MAX_INT 162 */ 163 public List<Base> getValues() { 164 return values; 165 } 166 167 public boolean hasValues() { 168 for (Base e : getValues()) 169 if (e != null) 170 return true; 171 return false; 172 } 173 174 public StructureDefinition getStructure() { 175 return structure; 176 } 177 178 public void setStructure(StructureDefinition structure) { 179 this.structure = structure; 180 } 181 182 public boolean isList() { 183 return maxCardinality > 1; 184 } 185 186 public String toString() { 187 return name; 188 } 189 190}