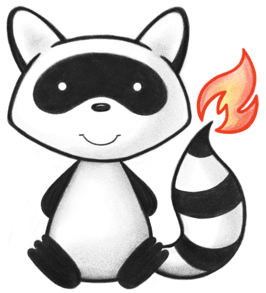
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * Provenance of a resource is a record that describes entities and processes 048 * involved in producing and delivering or otherwise influencing that resource. 049 * Provenance provides a critical foundation for assessing authenticity, 050 * enabling trust, and allowing reproducibility. Provenance assertions are a 051 * form of contextual metadata and can themselves become important records with 052 * their own provenance. Provenance statement indicates clinical significance in 053 * terms of confidence in authenticity, reliability, and trustworthiness, 054 * integrity, and stage in lifecycle (e.g. Document Completion - has the 055 * artifact been legally authenticated), all of which may impact security, 056 * privacy, and trust policies. 057 */ 058@ResourceDef(name = "Provenance", profile = "http://hl7.org/fhir/StructureDefinition/Provenance") 059public class Provenance extends DomainResource { 060 061 public enum ProvenanceEntityRole { 062 /** 063 * A transformation of an entity into another, an update of an entity resulting 064 * in a new one, or the construction of a new entity based on a pre-existing 065 * entity. 066 */ 067 DERIVATION, 068 /** 069 * A derivation for which the resulting entity is a revised version of some 070 * original. 071 */ 072 REVISION, 073 /** 074 * The repeat of (some or all of) an entity, such as text or image, by someone 075 * who might or might not be its original author. 076 */ 077 QUOTATION, 078 /** 079 * A primary source for a topic refers to something produced by some agent with 080 * direct experience and knowledge about the topic, at the time of the topic's 081 * study, without benefit from hindsight. 082 */ 083 SOURCE, 084 /** 085 * A derivation for which the entity is removed from accessibility usually 086 * through the use of the Delete operation. 087 */ 088 REMOVAL, 089 /** 090 * added to help the parsers with the generic types 091 */ 092 NULL; 093 094 public static ProvenanceEntityRole fromCode(String codeString) throws FHIRException { 095 if (codeString == null || "".equals(codeString)) 096 return null; 097 if ("derivation".equals(codeString)) 098 return DERIVATION; 099 if ("revision".equals(codeString)) 100 return REVISION; 101 if ("quotation".equals(codeString)) 102 return QUOTATION; 103 if ("source".equals(codeString)) 104 return SOURCE; 105 if ("removal".equals(codeString)) 106 return REMOVAL; 107 if (Configuration.isAcceptInvalidEnums()) 108 return null; 109 else 110 throw new FHIRException("Unknown ProvenanceEntityRole code '" + codeString + "'"); 111 } 112 113 public String toCode() { 114 switch (this) { 115 case DERIVATION: 116 return "derivation"; 117 case REVISION: 118 return "revision"; 119 case QUOTATION: 120 return "quotation"; 121 case SOURCE: 122 return "source"; 123 case REMOVAL: 124 return "removal"; 125 case NULL: 126 return null; 127 default: 128 return "?"; 129 } 130 } 131 132 public String getSystem() { 133 switch (this) { 134 case DERIVATION: 135 return "http://hl7.org/fhir/provenance-entity-role"; 136 case REVISION: 137 return "http://hl7.org/fhir/provenance-entity-role"; 138 case QUOTATION: 139 return "http://hl7.org/fhir/provenance-entity-role"; 140 case SOURCE: 141 return "http://hl7.org/fhir/provenance-entity-role"; 142 case REMOVAL: 143 return "http://hl7.org/fhir/provenance-entity-role"; 144 case NULL: 145 return null; 146 default: 147 return "?"; 148 } 149 } 150 151 public String getDefinition() { 152 switch (this) { 153 case DERIVATION: 154 return "A transformation of an entity into another, an update of an entity resulting in a new one, or the construction of a new entity based on a pre-existing entity."; 155 case REVISION: 156 return "A derivation for which the resulting entity is a revised version of some original."; 157 case QUOTATION: 158 return "The repeat of (some or all of) an entity, such as text or image, by someone who might or might not be its original author."; 159 case SOURCE: 160 return "A primary source for a topic refers to something produced by some agent with direct experience and knowledge about the topic, at the time of the topic's study, without benefit from hindsight."; 161 case REMOVAL: 162 return "A derivation for which the entity is removed from accessibility usually through the use of the Delete operation."; 163 case NULL: 164 return null; 165 default: 166 return "?"; 167 } 168 } 169 170 public String getDisplay() { 171 switch (this) { 172 case DERIVATION: 173 return "Derivation"; 174 case REVISION: 175 return "Revision"; 176 case QUOTATION: 177 return "Quotation"; 178 case SOURCE: 179 return "Source"; 180 case REMOVAL: 181 return "Removal"; 182 case NULL: 183 return null; 184 default: 185 return "?"; 186 } 187 } 188 } 189 190 public static class ProvenanceEntityRoleEnumFactory implements EnumFactory<ProvenanceEntityRole> { 191 public ProvenanceEntityRole fromCode(String codeString) throws IllegalArgumentException { 192 if (codeString == null || "".equals(codeString)) 193 if (codeString == null || "".equals(codeString)) 194 return null; 195 if ("derivation".equals(codeString)) 196 return ProvenanceEntityRole.DERIVATION; 197 if ("revision".equals(codeString)) 198 return ProvenanceEntityRole.REVISION; 199 if ("quotation".equals(codeString)) 200 return ProvenanceEntityRole.QUOTATION; 201 if ("source".equals(codeString)) 202 return ProvenanceEntityRole.SOURCE; 203 if ("removal".equals(codeString)) 204 return ProvenanceEntityRole.REMOVAL; 205 throw new IllegalArgumentException("Unknown ProvenanceEntityRole code '" + codeString + "'"); 206 } 207 208 public Enumeration<ProvenanceEntityRole> fromType(PrimitiveType<?> code) throws FHIRException { 209 if (code == null) 210 return null; 211 if (code.isEmpty()) 212 return new Enumeration<ProvenanceEntityRole>(this, ProvenanceEntityRole.NULL, code); 213 String codeString = code.asStringValue(); 214 if (codeString == null || "".equals(codeString)) 215 return new Enumeration<ProvenanceEntityRole>(this, ProvenanceEntityRole.NULL, code); 216 if ("derivation".equals(codeString)) 217 return new Enumeration<ProvenanceEntityRole>(this, ProvenanceEntityRole.DERIVATION, code); 218 if ("revision".equals(codeString)) 219 return new Enumeration<ProvenanceEntityRole>(this, ProvenanceEntityRole.REVISION, code); 220 if ("quotation".equals(codeString)) 221 return new Enumeration<ProvenanceEntityRole>(this, ProvenanceEntityRole.QUOTATION, code); 222 if ("source".equals(codeString)) 223 return new Enumeration<ProvenanceEntityRole>(this, ProvenanceEntityRole.SOURCE, code); 224 if ("removal".equals(codeString)) 225 return new Enumeration<ProvenanceEntityRole>(this, ProvenanceEntityRole.REMOVAL, code); 226 throw new FHIRException("Unknown ProvenanceEntityRole code '" + codeString + "'"); 227 } 228 229 public String toCode(ProvenanceEntityRole code) { 230 if (code == ProvenanceEntityRole.NULL) 231 return null; 232 if (code == ProvenanceEntityRole.DERIVATION) 233 return "derivation"; 234 if (code == ProvenanceEntityRole.REVISION) 235 return "revision"; 236 if (code == ProvenanceEntityRole.QUOTATION) 237 return "quotation"; 238 if (code == ProvenanceEntityRole.SOURCE) 239 return "source"; 240 if (code == ProvenanceEntityRole.REMOVAL) 241 return "removal"; 242 return "?"; 243 } 244 245 public String toSystem(ProvenanceEntityRole code) { 246 return code.getSystem(); 247 } 248 } 249 250 @Block() 251 public static class ProvenanceAgentComponent extends BackboneElement implements IBaseBackboneElement { 252 /** 253 * The participation the agent had with respect to the activity. 254 */ 255 @Child(name = "type", type = { 256 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 257 @Description(shortDefinition = "How the agent participated", formalDefinition = "The participation the agent had with respect to the activity.") 258 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/provenance-agent-type") 259 protected CodeableConcept type; 260 261 /** 262 * The function of the agent with respect to the activity. The security role 263 * enabling the agent with respect to the activity. 264 */ 265 @Child(name = "role", type = { 266 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 267 @Description(shortDefinition = "What the agents role was", formalDefinition = "The function of the agent with respect to the activity. The security role enabling the agent with respect to the activity.") 268 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/security-role-type") 269 protected List<CodeableConcept> role; 270 271 /** 272 * The individual, device or organization that participated in the event. 273 */ 274 @Child(name = "who", type = { Practitioner.class, PractitionerRole.class, RelatedPerson.class, Patient.class, 275 Device.class, Organization.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 276 @Description(shortDefinition = "Who participated", formalDefinition = "The individual, device or organization that participated in the event.") 277 protected Reference who; 278 279 /** 280 * The actual object that is the target of the reference (The individual, device 281 * or organization that participated in the event.) 282 */ 283 protected Resource whoTarget; 284 285 /** 286 * The individual, device, or organization for whom the change was made. 287 */ 288 @Child(name = "onBehalfOf", type = { Practitioner.class, PractitionerRole.class, RelatedPerson.class, Patient.class, 289 Device.class, Organization.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 290 @Description(shortDefinition = "Who the agent is representing", formalDefinition = "The individual, device, or organization for whom the change was made.") 291 protected Reference onBehalfOf; 292 293 /** 294 * The actual object that is the target of the reference (The individual, 295 * device, or organization for whom the change was made.) 296 */ 297 protected Resource onBehalfOfTarget; 298 299 private static final long serialVersionUID = -1363252586L; 300 301 /** 302 * Constructor 303 */ 304 public ProvenanceAgentComponent() { 305 super(); 306 } 307 308 /** 309 * Constructor 310 */ 311 public ProvenanceAgentComponent(Reference who) { 312 super(); 313 this.who = who; 314 } 315 316 /** 317 * @return {@link #type} (The participation the agent had with respect to the 318 * activity.) 319 */ 320 public CodeableConcept getType() { 321 if (this.type == null) 322 if (Configuration.errorOnAutoCreate()) 323 throw new Error("Attempt to auto-create ProvenanceAgentComponent.type"); 324 else if (Configuration.doAutoCreate()) 325 this.type = new CodeableConcept(); // cc 326 return this.type; 327 } 328 329 public boolean hasType() { 330 return this.type != null && !this.type.isEmpty(); 331 } 332 333 /** 334 * @param value {@link #type} (The participation the agent had with respect to 335 * the activity.) 336 */ 337 public ProvenanceAgentComponent setType(CodeableConcept value) { 338 this.type = value; 339 return this; 340 } 341 342 /** 343 * @return {@link #role} (The function of the agent with respect to the 344 * activity. The security role enabling the agent with respect to the 345 * activity.) 346 */ 347 public List<CodeableConcept> getRole() { 348 if (this.role == null) 349 this.role = new ArrayList<CodeableConcept>(); 350 return this.role; 351 } 352 353 /** 354 * @return Returns a reference to <code>this</code> for easy method chaining 355 */ 356 public ProvenanceAgentComponent setRole(List<CodeableConcept> theRole) { 357 this.role = theRole; 358 return this; 359 } 360 361 public boolean hasRole() { 362 if (this.role == null) 363 return false; 364 for (CodeableConcept item : this.role) 365 if (!item.isEmpty()) 366 return true; 367 return false; 368 } 369 370 public CodeableConcept addRole() { // 3 371 CodeableConcept t = new CodeableConcept(); 372 if (this.role == null) 373 this.role = new ArrayList<CodeableConcept>(); 374 this.role.add(t); 375 return t; 376 } 377 378 public ProvenanceAgentComponent addRole(CodeableConcept t) { // 3 379 if (t == null) 380 return this; 381 if (this.role == null) 382 this.role = new ArrayList<CodeableConcept>(); 383 this.role.add(t); 384 return this; 385 } 386 387 /** 388 * @return The first repetition of repeating field {@link #role}, creating it if 389 * it does not already exist 390 */ 391 public CodeableConcept getRoleFirstRep() { 392 if (getRole().isEmpty()) { 393 addRole(); 394 } 395 return getRole().get(0); 396 } 397 398 /** 399 * @return {@link #who} (The individual, device or organization that 400 * participated in the event.) 401 */ 402 public Reference getWho() { 403 if (this.who == null) 404 if (Configuration.errorOnAutoCreate()) 405 throw new Error("Attempt to auto-create ProvenanceAgentComponent.who"); 406 else if (Configuration.doAutoCreate()) 407 this.who = new Reference(); // cc 408 return this.who; 409 } 410 411 public boolean hasWho() { 412 return this.who != null && !this.who.isEmpty(); 413 } 414 415 /** 416 * @param value {@link #who} (The individual, device or organization that 417 * participated in the event.) 418 */ 419 public ProvenanceAgentComponent setWho(Reference value) { 420 this.who = value; 421 return this; 422 } 423 424 /** 425 * @return {@link #who} The actual object that is the target of the reference. 426 * The reference library doesn't populate this, but you can use it to 427 * hold the resource if you resolve it. (The individual, device or 428 * organization that participated in the event.) 429 */ 430 public Resource getWhoTarget() { 431 return this.whoTarget; 432 } 433 434 /** 435 * @param value {@link #who} The actual object that is the target of the 436 * reference. The reference library doesn't use these, but you can 437 * use it to hold the resource if you resolve it. (The individual, 438 * device or organization that participated in the event.) 439 */ 440 public ProvenanceAgentComponent setWhoTarget(Resource value) { 441 this.whoTarget = value; 442 return this; 443 } 444 445 /** 446 * @return {@link #onBehalfOf} (The individual, device, or organization for whom 447 * the change was made.) 448 */ 449 public Reference getOnBehalfOf() { 450 if (this.onBehalfOf == null) 451 if (Configuration.errorOnAutoCreate()) 452 throw new Error("Attempt to auto-create ProvenanceAgentComponent.onBehalfOf"); 453 else if (Configuration.doAutoCreate()) 454 this.onBehalfOf = new Reference(); // cc 455 return this.onBehalfOf; 456 } 457 458 public boolean hasOnBehalfOf() { 459 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 460 } 461 462 /** 463 * @param value {@link #onBehalfOf} (The individual, device, or organization for 464 * whom the change was made.) 465 */ 466 public ProvenanceAgentComponent setOnBehalfOf(Reference value) { 467 this.onBehalfOf = value; 468 return this; 469 } 470 471 /** 472 * @return {@link #onBehalfOf} The actual object that is the target of the 473 * reference. The reference library doesn't populate this, but you can 474 * use it to hold the resource if you resolve it. (The individual, 475 * device, or organization for whom the change was made.) 476 */ 477 public Resource getOnBehalfOfTarget() { 478 return this.onBehalfOfTarget; 479 } 480 481 /** 482 * @param value {@link #onBehalfOf} The actual object that is the target of the 483 * reference. The reference library doesn't use these, but you can 484 * use it to hold the resource if you resolve it. (The individual, 485 * device, or organization for whom the change was made.) 486 */ 487 public ProvenanceAgentComponent setOnBehalfOfTarget(Resource value) { 488 this.onBehalfOfTarget = value; 489 return this; 490 } 491 492 protected void listChildren(List<Property> children) { 493 super.listChildren(children); 494 children.add(new Property("type", "CodeableConcept", 495 "The participation the agent had with respect to the activity.", 0, 1, type)); 496 children.add(new Property("role", "CodeableConcept", 497 "The function of the agent with respect to the activity. The security role enabling the agent with respect to the activity.", 498 0, java.lang.Integer.MAX_VALUE, role)); 499 children 500 .add(new Property("who", "Reference(Practitioner|PractitionerRole|RelatedPerson|Patient|Device|Organization)", 501 "The individual, device or organization that participated in the event.", 0, 1, who)); 502 children.add(new Property("onBehalfOf", 503 "Reference(Practitioner|PractitionerRole|RelatedPerson|Patient|Device|Organization)", 504 "The individual, device, or organization for whom the change was made.", 0, 1, onBehalfOf)); 505 } 506 507 @Override 508 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 509 switch (_hash) { 510 case 3575610: 511 /* type */ return new Property("type", "CodeableConcept", 512 "The participation the agent had with respect to the activity.", 0, 1, type); 513 case 3506294: 514 /* role */ return new Property("role", "CodeableConcept", 515 "The function of the agent with respect to the activity. The security role enabling the agent with respect to the activity.", 516 0, java.lang.Integer.MAX_VALUE, role); 517 case 117694: 518 /* who */ return new Property("who", 519 "Reference(Practitioner|PractitionerRole|RelatedPerson|Patient|Device|Organization)", 520 "The individual, device or organization that participated in the event.", 0, 1, who); 521 case -14402964: 522 /* onBehalfOf */ return new Property("onBehalfOf", 523 "Reference(Practitioner|PractitionerRole|RelatedPerson|Patient|Device|Organization)", 524 "The individual, device, or organization for whom the change was made.", 0, 1, onBehalfOf); 525 default: 526 return super.getNamedProperty(_hash, _name, _checkValid); 527 } 528 529 } 530 531 @Override 532 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 533 switch (hash) { 534 case 3575610: 535 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 536 case 3506294: 537 /* role */ return this.role == null ? new Base[0] : this.role.toArray(new Base[this.role.size()]); // CodeableConcept 538 case 117694: 539 /* who */ return this.who == null ? new Base[0] : new Base[] { this.who }; // Reference 540 case -14402964: 541 /* onBehalfOf */ return this.onBehalfOf == null ? new Base[0] : new Base[] { this.onBehalfOf }; // Reference 542 default: 543 return super.getProperty(hash, name, checkValid); 544 } 545 546 } 547 548 @Override 549 public Base setProperty(int hash, String name, Base value) throws FHIRException { 550 switch (hash) { 551 case 3575610: // type 552 this.type = castToCodeableConcept(value); // CodeableConcept 553 return value; 554 case 3506294: // role 555 this.getRole().add(castToCodeableConcept(value)); // CodeableConcept 556 return value; 557 case 117694: // who 558 this.who = castToReference(value); // Reference 559 return value; 560 case -14402964: // onBehalfOf 561 this.onBehalfOf = castToReference(value); // Reference 562 return value; 563 default: 564 return super.setProperty(hash, name, value); 565 } 566 567 } 568 569 @Override 570 public Base setProperty(String name, Base value) throws FHIRException { 571 if (name.equals("type")) { 572 this.type = castToCodeableConcept(value); // CodeableConcept 573 } else if (name.equals("role")) { 574 this.getRole().add(castToCodeableConcept(value)); 575 } else if (name.equals("who")) { 576 this.who = castToReference(value); // Reference 577 } else if (name.equals("onBehalfOf")) { 578 this.onBehalfOf = castToReference(value); // Reference 579 } else 580 return super.setProperty(name, value); 581 return value; 582 } 583 584 @Override 585 public void removeChild(String name, Base value) throws FHIRException { 586 if (name.equals("type")) { 587 this.type = null; 588 } else if (name.equals("role")) { 589 this.getRole().remove(castToCodeableConcept(value)); 590 } else if (name.equals("who")) { 591 this.who = null; 592 } else if (name.equals("onBehalfOf")) { 593 this.onBehalfOf = null; 594 } else 595 super.removeChild(name, value); 596 597 } 598 599 @Override 600 public Base makeProperty(int hash, String name) throws FHIRException { 601 switch (hash) { 602 case 3575610: 603 return getType(); 604 case 3506294: 605 return addRole(); 606 case 117694: 607 return getWho(); 608 case -14402964: 609 return getOnBehalfOf(); 610 default: 611 return super.makeProperty(hash, name); 612 } 613 614 } 615 616 @Override 617 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 618 switch (hash) { 619 case 3575610: 620 /* type */ return new String[] { "CodeableConcept" }; 621 case 3506294: 622 /* role */ return new String[] { "CodeableConcept" }; 623 case 117694: 624 /* who */ return new String[] { "Reference" }; 625 case -14402964: 626 /* onBehalfOf */ return new String[] { "Reference" }; 627 default: 628 return super.getTypesForProperty(hash, name); 629 } 630 631 } 632 633 @Override 634 public Base addChild(String name) throws FHIRException { 635 if (name.equals("type")) { 636 this.type = new CodeableConcept(); 637 return this.type; 638 } else if (name.equals("role")) { 639 return addRole(); 640 } else if (name.equals("who")) { 641 this.who = new Reference(); 642 return this.who; 643 } else if (name.equals("onBehalfOf")) { 644 this.onBehalfOf = new Reference(); 645 return this.onBehalfOf; 646 } else 647 return super.addChild(name); 648 } 649 650 public ProvenanceAgentComponent copy() { 651 ProvenanceAgentComponent dst = new ProvenanceAgentComponent(); 652 copyValues(dst); 653 return dst; 654 } 655 656 public void copyValues(ProvenanceAgentComponent dst) { 657 super.copyValues(dst); 658 dst.type = type == null ? null : type.copy(); 659 if (role != null) { 660 dst.role = new ArrayList<CodeableConcept>(); 661 for (CodeableConcept i : role) 662 dst.role.add(i.copy()); 663 } 664 ; 665 dst.who = who == null ? null : who.copy(); 666 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 667 } 668 669 @Override 670 public boolean equalsDeep(Base other_) { 671 if (!super.equalsDeep(other_)) 672 return false; 673 if (!(other_ instanceof ProvenanceAgentComponent)) 674 return false; 675 ProvenanceAgentComponent o = (ProvenanceAgentComponent) other_; 676 return compareDeep(type, o.type, true) && compareDeep(role, o.role, true) && compareDeep(who, o.who, true) 677 && compareDeep(onBehalfOf, o.onBehalfOf, true); 678 } 679 680 @Override 681 public boolean equalsShallow(Base other_) { 682 if (!super.equalsShallow(other_)) 683 return false; 684 if (!(other_ instanceof ProvenanceAgentComponent)) 685 return false; 686 ProvenanceAgentComponent o = (ProvenanceAgentComponent) other_; 687 return true; 688 } 689 690 public boolean isEmpty() { 691 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, role, who, onBehalfOf); 692 } 693 694 public String fhirType() { 695 return "Provenance.agent"; 696 697 } 698 699 } 700 701 @Block() 702 public static class ProvenanceEntityComponent extends BackboneElement implements IBaseBackboneElement { 703 /** 704 * How the entity was used during the activity. 705 */ 706 @Child(name = "role", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 707 @Description(shortDefinition = "derivation | revision | quotation | source | removal", formalDefinition = "How the entity was used during the activity.") 708 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/provenance-entity-role") 709 protected Enumeration<ProvenanceEntityRole> role; 710 711 /** 712 * Identity of the Entity used. May be a logical or physical uri and maybe 713 * absolute or relative. 714 */ 715 @Child(name = "what", type = { Reference.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 716 @Description(shortDefinition = "Identity of entity", formalDefinition = "Identity of the Entity used. May be a logical or physical uri and maybe absolute or relative.") 717 protected Reference what; 718 719 /** 720 * The actual object that is the target of the reference (Identity of the Entity 721 * used. May be a logical or physical uri and maybe absolute or relative.) 722 */ 723 protected Resource whatTarget; 724 725 /** 726 * The entity is attributed to an agent to express the agent's responsibility 727 * for that entity, possibly along with other agents. This description can be 728 * understood as shorthand for saying that the agent was responsible for the 729 * activity which generated the entity. 730 */ 731 @Child(name = "agent", type = { 732 ProvenanceAgentComponent.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 733 @Description(shortDefinition = "Entity is attributed to this agent", formalDefinition = "The entity is attributed to an agent to express the agent's responsibility for that entity, possibly along with other agents. This description can be understood as shorthand for saying that the agent was responsible for the activity which generated the entity.") 734 protected List<ProvenanceAgentComponent> agent; 735 736 private static final long serialVersionUID = 144967401L; 737 738 /** 739 * Constructor 740 */ 741 public ProvenanceEntityComponent() { 742 super(); 743 } 744 745 /** 746 * Constructor 747 */ 748 public ProvenanceEntityComponent(Enumeration<ProvenanceEntityRole> role, Reference what) { 749 super(); 750 this.role = role; 751 this.what = what; 752 } 753 754 /** 755 * @return {@link #role} (How the entity was used during the activity.). This is 756 * the underlying object with id, value and extensions. The accessor 757 * "getRole" gives direct access to the value 758 */ 759 public Enumeration<ProvenanceEntityRole> getRoleElement() { 760 if (this.role == null) 761 if (Configuration.errorOnAutoCreate()) 762 throw new Error("Attempt to auto-create ProvenanceEntityComponent.role"); 763 else if (Configuration.doAutoCreate()) 764 this.role = new Enumeration<ProvenanceEntityRole>(new ProvenanceEntityRoleEnumFactory()); // bb 765 return this.role; 766 } 767 768 public boolean hasRoleElement() { 769 return this.role != null && !this.role.isEmpty(); 770 } 771 772 public boolean hasRole() { 773 return this.role != null && !this.role.isEmpty(); 774 } 775 776 /** 777 * @param value {@link #role} (How the entity was used during the activity.). 778 * This is the underlying object with id, value and extensions. The 779 * accessor "getRole" gives direct access to the value 780 */ 781 public ProvenanceEntityComponent setRoleElement(Enumeration<ProvenanceEntityRole> value) { 782 this.role = value; 783 return this; 784 } 785 786 /** 787 * @return How the entity was used during the activity. 788 */ 789 public ProvenanceEntityRole getRole() { 790 return this.role == null ? null : this.role.getValue(); 791 } 792 793 /** 794 * @param value How the entity was used during the activity. 795 */ 796 public ProvenanceEntityComponent setRole(ProvenanceEntityRole value) { 797 if (this.role == null) 798 this.role = new Enumeration<ProvenanceEntityRole>(new ProvenanceEntityRoleEnumFactory()); 799 this.role.setValue(value); 800 return this; 801 } 802 803 /** 804 * @return {@link #what} (Identity of the Entity used. May be a logical or 805 * physical uri and maybe absolute or relative.) 806 */ 807 public Reference getWhat() { 808 if (this.what == null) 809 if (Configuration.errorOnAutoCreate()) 810 throw new Error("Attempt to auto-create ProvenanceEntityComponent.what"); 811 else if (Configuration.doAutoCreate()) 812 this.what = new Reference(); // cc 813 return this.what; 814 } 815 816 public boolean hasWhat() { 817 return this.what != null && !this.what.isEmpty(); 818 } 819 820 /** 821 * @param value {@link #what} (Identity of the Entity used. May be a logical or 822 * physical uri and maybe absolute or relative.) 823 */ 824 public ProvenanceEntityComponent setWhat(Reference value) { 825 this.what = value; 826 return this; 827 } 828 829 /** 830 * @return {@link #what} The actual object that is the target of the reference. 831 * The reference library doesn't populate this, but you can use it to 832 * hold the resource if you resolve it. (Identity of the Entity used. 833 * May be a logical or physical uri and maybe absolute or relative.) 834 */ 835 public Resource getWhatTarget() { 836 return this.whatTarget; 837 } 838 839 /** 840 * @param value {@link #what} The actual object that is the target of the 841 * reference. The reference library doesn't use these, but you can 842 * use it to hold the resource if you resolve it. (Identity of the 843 * Entity used. May be a logical or physical uri and maybe absolute 844 * or relative.) 845 */ 846 public ProvenanceEntityComponent setWhatTarget(Resource value) { 847 this.whatTarget = value; 848 return this; 849 } 850 851 /** 852 * @return {@link #agent} (The entity is attributed to an agent to express the 853 * agent's responsibility for that entity, possibly along with other 854 * agents. This description can be understood as shorthand for saying 855 * that the agent was responsible for the activity which generated the 856 * entity.) 857 */ 858 public List<ProvenanceAgentComponent> getAgent() { 859 if (this.agent == null) 860 this.agent = new ArrayList<ProvenanceAgentComponent>(); 861 return this.agent; 862 } 863 864 /** 865 * @return Returns a reference to <code>this</code> for easy method chaining 866 */ 867 public ProvenanceEntityComponent setAgent(List<ProvenanceAgentComponent> theAgent) { 868 this.agent = theAgent; 869 return this; 870 } 871 872 public boolean hasAgent() { 873 if (this.agent == null) 874 return false; 875 for (ProvenanceAgentComponent item : this.agent) 876 if (!item.isEmpty()) 877 return true; 878 return false; 879 } 880 881 public ProvenanceAgentComponent addAgent() { // 3 882 ProvenanceAgentComponent t = new ProvenanceAgentComponent(); 883 if (this.agent == null) 884 this.agent = new ArrayList<ProvenanceAgentComponent>(); 885 this.agent.add(t); 886 return t; 887 } 888 889 public ProvenanceEntityComponent addAgent(ProvenanceAgentComponent t) { // 3 890 if (t == null) 891 return this; 892 if (this.agent == null) 893 this.agent = new ArrayList<ProvenanceAgentComponent>(); 894 this.agent.add(t); 895 return this; 896 } 897 898 /** 899 * @return The first repetition of repeating field {@link #agent}, creating it 900 * if it does not already exist 901 */ 902 public ProvenanceAgentComponent getAgentFirstRep() { 903 if (getAgent().isEmpty()) { 904 addAgent(); 905 } 906 return getAgent().get(0); 907 } 908 909 protected void listChildren(List<Property> children) { 910 super.listChildren(children); 911 children.add(new Property("role", "code", "How the entity was used during the activity.", 0, 1, role)); 912 children.add(new Property("what", "Reference(Any)", 913 "Identity of the Entity used. May be a logical or physical uri and maybe absolute or relative.", 0, 1, 914 what)); 915 children.add(new Property("agent", "@Provenance.agent", 916 "The entity is attributed to an agent to express the agent's responsibility for that entity, possibly along with other agents. This description can be understood as shorthand for saying that the agent was responsible for the activity which generated the entity.", 917 0, java.lang.Integer.MAX_VALUE, agent)); 918 } 919 920 @Override 921 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 922 switch (_hash) { 923 case 3506294: 924 /* role */ return new Property("role", "code", "How the entity was used during the activity.", 0, 1, role); 925 case 3648196: 926 /* what */ return new Property("what", "Reference(Any)", 927 "Identity of the Entity used. May be a logical or physical uri and maybe absolute or relative.", 0, 1, 928 what); 929 case 92750597: 930 /* agent */ return new Property("agent", "@Provenance.agent", 931 "The entity is attributed to an agent to express the agent's responsibility for that entity, possibly along with other agents. This description can be understood as shorthand for saying that the agent was responsible for the activity which generated the entity.", 932 0, java.lang.Integer.MAX_VALUE, agent); 933 default: 934 return super.getNamedProperty(_hash, _name, _checkValid); 935 } 936 937 } 938 939 @Override 940 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 941 switch (hash) { 942 case 3506294: 943 /* role */ return this.role == null ? new Base[0] : new Base[] { this.role }; // Enumeration<ProvenanceEntityRole> 944 case 3648196: 945 /* what */ return this.what == null ? new Base[0] : new Base[] { this.what }; // Reference 946 case 92750597: 947 /* agent */ return this.agent == null ? new Base[0] : this.agent.toArray(new Base[this.agent.size()]); // ProvenanceAgentComponent 948 default: 949 return super.getProperty(hash, name, checkValid); 950 } 951 952 } 953 954 @Override 955 public Base setProperty(int hash, String name, Base value) throws FHIRException { 956 switch (hash) { 957 case 3506294: // role 958 value = new ProvenanceEntityRoleEnumFactory().fromType(castToCode(value)); 959 this.role = (Enumeration) value; // Enumeration<ProvenanceEntityRole> 960 return value; 961 case 3648196: // what 962 this.what = castToReference(value); // Reference 963 return value; 964 case 92750597: // agent 965 this.getAgent().add((ProvenanceAgentComponent) value); // ProvenanceAgentComponent 966 return value; 967 default: 968 return super.setProperty(hash, name, value); 969 } 970 971 } 972 973 @Override 974 public Base setProperty(String name, Base value) throws FHIRException { 975 if (name.equals("role")) { 976 value = new ProvenanceEntityRoleEnumFactory().fromType(castToCode(value)); 977 this.role = (Enumeration) value; // Enumeration<ProvenanceEntityRole> 978 } else if (name.equals("what")) { 979 this.what = castToReference(value); // Reference 980 } else if (name.equals("agent")) { 981 this.getAgent().add((ProvenanceAgentComponent) value); 982 } else 983 return super.setProperty(name, value); 984 return value; 985 } 986 987 @Override 988 public void removeChild(String name, Base value) throws FHIRException { 989 if (name.equals("role")) { 990 this.role = null; 991 } else if (name.equals("what")) { 992 this.what = null; 993 } else if (name.equals("agent")) { 994 this.getAgent().remove((ProvenanceAgentComponent) value); 995 } else 996 super.removeChild(name, value); 997 998 } 999 1000 @Override 1001 public Base makeProperty(int hash, String name) throws FHIRException { 1002 switch (hash) { 1003 case 3506294: 1004 return getRoleElement(); 1005 case 3648196: 1006 return getWhat(); 1007 case 92750597: 1008 return addAgent(); 1009 default: 1010 return super.makeProperty(hash, name); 1011 } 1012 1013 } 1014 1015 @Override 1016 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1017 switch (hash) { 1018 case 3506294: 1019 /* role */ return new String[] { "code" }; 1020 case 3648196: 1021 /* what */ return new String[] { "Reference" }; 1022 case 92750597: 1023 /* agent */ return new String[] { "@Provenance.agent" }; 1024 default: 1025 return super.getTypesForProperty(hash, name); 1026 } 1027 1028 } 1029 1030 @Override 1031 public Base addChild(String name) throws FHIRException { 1032 if (name.equals("role")) { 1033 throw new FHIRException("Cannot call addChild on a singleton property Provenance.role"); 1034 } else if (name.equals("what")) { 1035 this.what = new Reference(); 1036 return this.what; 1037 } else if (name.equals("agent")) { 1038 return addAgent(); 1039 } else 1040 return super.addChild(name); 1041 } 1042 1043 public ProvenanceEntityComponent copy() { 1044 ProvenanceEntityComponent dst = new ProvenanceEntityComponent(); 1045 copyValues(dst); 1046 return dst; 1047 } 1048 1049 public void copyValues(ProvenanceEntityComponent dst) { 1050 super.copyValues(dst); 1051 dst.role = role == null ? null : role.copy(); 1052 dst.what = what == null ? null : what.copy(); 1053 if (agent != null) { 1054 dst.agent = new ArrayList<ProvenanceAgentComponent>(); 1055 for (ProvenanceAgentComponent i : agent) 1056 dst.agent.add(i.copy()); 1057 } 1058 ; 1059 } 1060 1061 @Override 1062 public boolean equalsDeep(Base other_) { 1063 if (!super.equalsDeep(other_)) 1064 return false; 1065 if (!(other_ instanceof ProvenanceEntityComponent)) 1066 return false; 1067 ProvenanceEntityComponent o = (ProvenanceEntityComponent) other_; 1068 return compareDeep(role, o.role, true) && compareDeep(what, o.what, true) && compareDeep(agent, o.agent, true); 1069 } 1070 1071 @Override 1072 public boolean equalsShallow(Base other_) { 1073 if (!super.equalsShallow(other_)) 1074 return false; 1075 if (!(other_ instanceof ProvenanceEntityComponent)) 1076 return false; 1077 ProvenanceEntityComponent o = (ProvenanceEntityComponent) other_; 1078 return compareValues(role, o.role, true); 1079 } 1080 1081 public boolean isEmpty() { 1082 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, what, agent); 1083 } 1084 1085 public String fhirType() { 1086 return "Provenance.entity"; 1087 1088 } 1089 1090 } 1091 1092 /** 1093 * The Reference(s) that were generated or updated by the activity described in 1094 * this resource. A provenance can point to more than one target if multiple 1095 * resources were created/updated by the same activity. 1096 */ 1097 @Child(name = "target", type = { 1098 Reference.class }, order = 0, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1099 @Description(shortDefinition = "Target Reference(s) (usually version specific)", formalDefinition = "The Reference(s) that were generated or updated by the activity described in this resource. A provenance can point to more than one target if multiple resources were created/updated by the same activity.") 1100 protected List<Reference> target; 1101 /** 1102 * The actual objects that are the target of the reference (The Reference(s) 1103 * that were generated or updated by the activity described in this resource. A 1104 * provenance can point to more than one target if multiple resources were 1105 * created/updated by the same activity.) 1106 */ 1107 protected List<Resource> targetTarget; 1108 1109 /** 1110 * The period during which the activity occurred. 1111 */ 1112 @Child(name = "occurred", type = { Period.class, 1113 DateTimeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1114 @Description(shortDefinition = "When the activity occurred", formalDefinition = "The period during which the activity occurred.") 1115 protected Type occurred; 1116 1117 /** 1118 * The instant of time at which the activity was recorded. 1119 */ 1120 @Child(name = "recorded", type = { InstantType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1121 @Description(shortDefinition = "When the activity was recorded / updated", formalDefinition = "The instant of time at which the activity was recorded.") 1122 protected InstantType recorded; 1123 1124 /** 1125 * Policy or plan the activity was defined by. Typically, a single activity may 1126 * have multiple applicable policy documents, such as patient consent, guarantor 1127 * funding, etc. 1128 */ 1129 @Child(name = "policy", type = { 1130 UriType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1131 @Description(shortDefinition = "Policy or plan the activity was defined by", formalDefinition = "Policy or plan the activity was defined by. Typically, a single activity may have multiple applicable policy documents, such as patient consent, guarantor funding, etc.") 1132 protected List<UriType> policy; 1133 1134 /** 1135 * Where the activity occurred, if relevant. 1136 */ 1137 @Child(name = "location", type = { Location.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1138 @Description(shortDefinition = "Where the activity occurred, if relevant", formalDefinition = "Where the activity occurred, if relevant.") 1139 protected Reference location; 1140 1141 /** 1142 * The actual object that is the target of the reference (Where the activity 1143 * occurred, if relevant.) 1144 */ 1145 protected Location locationTarget; 1146 1147 /** 1148 * The reason that the activity was taking place. 1149 */ 1150 @Child(name = "reason", type = { 1151 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1152 @Description(shortDefinition = "Reason the activity is occurring", formalDefinition = "The reason that the activity was taking place.") 1153 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-PurposeOfUse") 1154 protected List<CodeableConcept> reason; 1155 1156 /** 1157 * An activity is something that occurs over a period of time and acts upon or 1158 * with entities; it may include consuming, processing, transforming, modifying, 1159 * relocating, using, or generating entities. 1160 */ 1161 @Child(name = "activity", type = { 1162 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 1163 @Description(shortDefinition = "Activity that occurred", formalDefinition = "An activity is something that occurs over a period of time and acts upon or with entities; it may include consuming, processing, transforming, modifying, relocating, using, or generating entities.") 1164 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/provenance-activity-type") 1165 protected CodeableConcept activity; 1166 1167 /** 1168 * An actor taking a role in an activity for which it can be assigned some 1169 * degree of responsibility for the activity taking place. 1170 */ 1171 @Child(name = "agent", type = {}, order = 7, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1172 @Description(shortDefinition = "Actor involved", formalDefinition = "An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place.") 1173 protected List<ProvenanceAgentComponent> agent; 1174 1175 /** 1176 * An entity used in this activity. 1177 */ 1178 @Child(name = "entity", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1179 @Description(shortDefinition = "An entity used in this activity", formalDefinition = "An entity used in this activity.") 1180 protected List<ProvenanceEntityComponent> entity; 1181 1182 /** 1183 * A digital signature on the target Reference(s). The signer should match a 1184 * Provenance.agent. The purpose of the signature is indicated. 1185 */ 1186 @Child(name = "signature", type = { 1187 Signature.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1188 @Description(shortDefinition = "Signature on target", formalDefinition = "A digital signature on the target Reference(s). The signer should match a Provenance.agent. The purpose of the signature is indicated.") 1189 protected List<Signature> signature; 1190 1191 private static final long serialVersionUID = -1991881518L; 1192 1193 /** 1194 * Constructor 1195 */ 1196 public Provenance() { 1197 super(); 1198 } 1199 1200 /** 1201 * Constructor 1202 */ 1203 public Provenance(InstantType recorded) { 1204 super(); 1205 this.recorded = recorded; 1206 } 1207 1208 /** 1209 * @return {@link #target} (The Reference(s) that were generated or updated by 1210 * the activity described in this resource. A provenance can point to 1211 * more than one target if multiple resources were created/updated by 1212 * the same activity.) 1213 */ 1214 public List<Reference> getTarget() { 1215 if (this.target == null) 1216 this.target = new ArrayList<Reference>(); 1217 return this.target; 1218 } 1219 1220 /** 1221 * @return Returns a reference to <code>this</code> for easy method chaining 1222 */ 1223 public Provenance setTarget(List<Reference> theTarget) { 1224 this.target = theTarget; 1225 return this; 1226 } 1227 1228 public boolean hasTarget() { 1229 if (this.target == null) 1230 return false; 1231 for (Reference item : this.target) 1232 if (!item.isEmpty()) 1233 return true; 1234 return false; 1235 } 1236 1237 public Reference addTarget() { // 3 1238 Reference t = new Reference(); 1239 if (this.target == null) 1240 this.target = new ArrayList<Reference>(); 1241 this.target.add(t); 1242 return t; 1243 } 1244 1245 public Provenance addTarget(Reference t) { // 3 1246 if (t == null) 1247 return this; 1248 if (this.target == null) 1249 this.target = new ArrayList<Reference>(); 1250 this.target.add(t); 1251 return this; 1252 } 1253 1254 /** 1255 * @return The first repetition of repeating field {@link #target}, creating it 1256 * if it does not already exist 1257 */ 1258 public Reference getTargetFirstRep() { 1259 if (getTarget().isEmpty()) { 1260 addTarget(); 1261 } 1262 return getTarget().get(0); 1263 } 1264 1265 /** 1266 * @deprecated Use Reference#setResource(IBaseResource) instead 1267 */ 1268 @Deprecated 1269 public List<Resource> getTargetTarget() { 1270 if (this.targetTarget == null) 1271 this.targetTarget = new ArrayList<Resource>(); 1272 return this.targetTarget; 1273 } 1274 1275 /** 1276 * @return {@link #occurred} (The period during which the activity occurred.) 1277 */ 1278 public Type getOccurred() { 1279 return this.occurred; 1280 } 1281 1282 /** 1283 * @return {@link #occurred} (The period during which the activity occurred.) 1284 */ 1285 public Period getOccurredPeriod() throws FHIRException { 1286 if (this.occurred == null) 1287 this.occurred = new Period(); 1288 if (!(this.occurred instanceof Period)) 1289 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.occurred.getClass().getName() 1290 + " was encountered"); 1291 return (Period) this.occurred; 1292 } 1293 1294 public boolean hasOccurredPeriod() { 1295 return this != null && this.occurred instanceof Period; 1296 } 1297 1298 /** 1299 * @return {@link #occurred} (The period during which the activity occurred.) 1300 */ 1301 public DateTimeType getOccurredDateTimeType() throws FHIRException { 1302 if (this.occurred == null) 1303 this.occurred = new DateTimeType(); 1304 if (!(this.occurred instanceof DateTimeType)) 1305 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1306 + this.occurred.getClass().getName() + " was encountered"); 1307 return (DateTimeType) this.occurred; 1308 } 1309 1310 public boolean hasOccurredDateTimeType() { 1311 return this != null && this.occurred instanceof DateTimeType; 1312 } 1313 1314 public boolean hasOccurred() { 1315 return this.occurred != null && !this.occurred.isEmpty(); 1316 } 1317 1318 /** 1319 * @param value {@link #occurred} (The period during which the activity 1320 * occurred.) 1321 */ 1322 public Provenance setOccurred(Type value) { 1323 if (value != null && !(value instanceof Period || value instanceof DateTimeType)) 1324 throw new Error("Not the right type for Provenance.occurred[x]: " + value.fhirType()); 1325 this.occurred = value; 1326 return this; 1327 } 1328 1329 /** 1330 * @return {@link #recorded} (The instant of time at which the activity was 1331 * recorded.). This is the underlying object with id, value and 1332 * extensions. The accessor "getRecorded" gives direct access to the 1333 * value 1334 */ 1335 public InstantType getRecordedElement() { 1336 if (this.recorded == null) 1337 if (Configuration.errorOnAutoCreate()) 1338 throw new Error("Attempt to auto-create Provenance.recorded"); 1339 else if (Configuration.doAutoCreate()) 1340 this.recorded = new InstantType(); // bb 1341 return this.recorded; 1342 } 1343 1344 public boolean hasRecordedElement() { 1345 return this.recorded != null && !this.recorded.isEmpty(); 1346 } 1347 1348 public boolean hasRecorded() { 1349 return this.recorded != null && !this.recorded.isEmpty(); 1350 } 1351 1352 /** 1353 * @param value {@link #recorded} (The instant of time at which the activity was 1354 * recorded.). This is the underlying object with id, value and 1355 * extensions. The accessor "getRecorded" gives direct access to 1356 * the value 1357 */ 1358 public Provenance setRecordedElement(InstantType value) { 1359 this.recorded = value; 1360 return this; 1361 } 1362 1363 /** 1364 * @return The instant of time at which the activity was recorded. 1365 */ 1366 public Date getRecorded() { 1367 return this.recorded == null ? null : this.recorded.getValue(); 1368 } 1369 1370 /** 1371 * @param value The instant of time at which the activity was recorded. 1372 */ 1373 public Provenance setRecorded(Date value) { 1374 if (this.recorded == null) 1375 this.recorded = new InstantType(); 1376 this.recorded.setValue(value); 1377 return this; 1378 } 1379 1380 /** 1381 * @return {@link #policy} (Policy or plan the activity was defined by. 1382 * Typically, a single activity may have multiple applicable policy 1383 * documents, such as patient consent, guarantor funding, etc.) 1384 */ 1385 public List<UriType> getPolicy() { 1386 if (this.policy == null) 1387 this.policy = new ArrayList<UriType>(); 1388 return this.policy; 1389 } 1390 1391 /** 1392 * @return Returns a reference to <code>this</code> for easy method chaining 1393 */ 1394 public Provenance setPolicy(List<UriType> thePolicy) { 1395 this.policy = thePolicy; 1396 return this; 1397 } 1398 1399 public boolean hasPolicy() { 1400 if (this.policy == null) 1401 return false; 1402 for (UriType item : this.policy) 1403 if (!item.isEmpty()) 1404 return true; 1405 return false; 1406 } 1407 1408 /** 1409 * @return {@link #policy} (Policy or plan the activity was defined by. 1410 * Typically, a single activity may have multiple applicable policy 1411 * documents, such as patient consent, guarantor funding, etc.) 1412 */ 1413 public UriType addPolicyElement() {// 2 1414 UriType t = new UriType(); 1415 if (this.policy == null) 1416 this.policy = new ArrayList<UriType>(); 1417 this.policy.add(t); 1418 return t; 1419 } 1420 1421 /** 1422 * @param value {@link #policy} (Policy or plan the activity was defined by. 1423 * Typically, a single activity may have multiple applicable policy 1424 * documents, such as patient consent, guarantor funding, etc.) 1425 */ 1426 public Provenance addPolicy(String value) { // 1 1427 UriType t = new UriType(); 1428 t.setValue(value); 1429 if (this.policy == null) 1430 this.policy = new ArrayList<UriType>(); 1431 this.policy.add(t); 1432 return this; 1433 } 1434 1435 /** 1436 * @param value {@link #policy} (Policy or plan the activity was defined by. 1437 * Typically, a single activity may have multiple applicable policy 1438 * documents, such as patient consent, guarantor funding, etc.) 1439 */ 1440 public boolean hasPolicy(String value) { 1441 if (this.policy == null) 1442 return false; 1443 for (UriType v : this.policy) 1444 if (v.getValue().equals(value)) // uri 1445 return true; 1446 return false; 1447 } 1448 1449 /** 1450 * @return {@link #location} (Where the activity occurred, if relevant.) 1451 */ 1452 public Reference getLocation() { 1453 if (this.location == null) 1454 if (Configuration.errorOnAutoCreate()) 1455 throw new Error("Attempt to auto-create Provenance.location"); 1456 else if (Configuration.doAutoCreate()) 1457 this.location = new Reference(); // cc 1458 return this.location; 1459 } 1460 1461 public boolean hasLocation() { 1462 return this.location != null && !this.location.isEmpty(); 1463 } 1464 1465 /** 1466 * @param value {@link #location} (Where the activity occurred, if relevant.) 1467 */ 1468 public Provenance setLocation(Reference value) { 1469 this.location = value; 1470 return this; 1471 } 1472 1473 /** 1474 * @return {@link #location} The actual object that is the target of the 1475 * reference. The reference library doesn't populate this, but you can 1476 * use it to hold the resource if you resolve it. (Where the activity 1477 * occurred, if relevant.) 1478 */ 1479 public Location getLocationTarget() { 1480 if (this.locationTarget == null) 1481 if (Configuration.errorOnAutoCreate()) 1482 throw new Error("Attempt to auto-create Provenance.location"); 1483 else if (Configuration.doAutoCreate()) 1484 this.locationTarget = new Location(); // aa 1485 return this.locationTarget; 1486 } 1487 1488 /** 1489 * @param value {@link #location} The actual object that is the target of the 1490 * reference. The reference library doesn't use these, but you can 1491 * use it to hold the resource if you resolve it. (Where the 1492 * activity occurred, if relevant.) 1493 */ 1494 public Provenance setLocationTarget(Location value) { 1495 this.locationTarget = value; 1496 return this; 1497 } 1498 1499 /** 1500 * @return {@link #reason} (The reason that the activity was taking place.) 1501 */ 1502 public List<CodeableConcept> getReason() { 1503 if (this.reason == null) 1504 this.reason = new ArrayList<CodeableConcept>(); 1505 return this.reason; 1506 } 1507 1508 /** 1509 * @return Returns a reference to <code>this</code> for easy method chaining 1510 */ 1511 public Provenance setReason(List<CodeableConcept> theReason) { 1512 this.reason = theReason; 1513 return this; 1514 } 1515 1516 public boolean hasReason() { 1517 if (this.reason == null) 1518 return false; 1519 for (CodeableConcept item : this.reason) 1520 if (!item.isEmpty()) 1521 return true; 1522 return false; 1523 } 1524 1525 public CodeableConcept addReason() { // 3 1526 CodeableConcept t = new CodeableConcept(); 1527 if (this.reason == null) 1528 this.reason = new ArrayList<CodeableConcept>(); 1529 this.reason.add(t); 1530 return t; 1531 } 1532 1533 public Provenance addReason(CodeableConcept t) { // 3 1534 if (t == null) 1535 return this; 1536 if (this.reason == null) 1537 this.reason = new ArrayList<CodeableConcept>(); 1538 this.reason.add(t); 1539 return this; 1540 } 1541 1542 /** 1543 * @return The first repetition of repeating field {@link #reason}, creating it 1544 * if it does not already exist 1545 */ 1546 public CodeableConcept getReasonFirstRep() { 1547 if (getReason().isEmpty()) { 1548 addReason(); 1549 } 1550 return getReason().get(0); 1551 } 1552 1553 /** 1554 * @return {@link #activity} (An activity is something that occurs over a period 1555 * of time and acts upon or with entities; it may include consuming, 1556 * processing, transforming, modifying, relocating, using, or generating 1557 * entities.) 1558 */ 1559 public CodeableConcept getActivity() { 1560 if (this.activity == null) 1561 if (Configuration.errorOnAutoCreate()) 1562 throw new Error("Attempt to auto-create Provenance.activity"); 1563 else if (Configuration.doAutoCreate()) 1564 this.activity = new CodeableConcept(); // cc 1565 return this.activity; 1566 } 1567 1568 public boolean hasActivity() { 1569 return this.activity != null && !this.activity.isEmpty(); 1570 } 1571 1572 /** 1573 * @param value {@link #activity} (An activity is something that occurs over a 1574 * period of time and acts upon or with entities; it may include 1575 * consuming, processing, transforming, modifying, relocating, 1576 * using, or generating entities.) 1577 */ 1578 public Provenance setActivity(CodeableConcept value) { 1579 this.activity = value; 1580 return this; 1581 } 1582 1583 /** 1584 * @return {@link #agent} (An actor taking a role in an activity for which it 1585 * can be assigned some degree of responsibility for the activity taking 1586 * place.) 1587 */ 1588 public List<ProvenanceAgentComponent> getAgent() { 1589 if (this.agent == null) 1590 this.agent = new ArrayList<ProvenanceAgentComponent>(); 1591 return this.agent; 1592 } 1593 1594 /** 1595 * @return Returns a reference to <code>this</code> for easy method chaining 1596 */ 1597 public Provenance setAgent(List<ProvenanceAgentComponent> theAgent) { 1598 this.agent = theAgent; 1599 return this; 1600 } 1601 1602 public boolean hasAgent() { 1603 if (this.agent == null) 1604 return false; 1605 for (ProvenanceAgentComponent item : this.agent) 1606 if (!item.isEmpty()) 1607 return true; 1608 return false; 1609 } 1610 1611 public ProvenanceAgentComponent addAgent() { // 3 1612 ProvenanceAgentComponent t = new ProvenanceAgentComponent(); 1613 if (this.agent == null) 1614 this.agent = new ArrayList<ProvenanceAgentComponent>(); 1615 this.agent.add(t); 1616 return t; 1617 } 1618 1619 public Provenance addAgent(ProvenanceAgentComponent t) { // 3 1620 if (t == null) 1621 return this; 1622 if (this.agent == null) 1623 this.agent = new ArrayList<ProvenanceAgentComponent>(); 1624 this.agent.add(t); 1625 return this; 1626 } 1627 1628 /** 1629 * @return The first repetition of repeating field {@link #agent}, creating it 1630 * if it does not already exist 1631 */ 1632 public ProvenanceAgentComponent getAgentFirstRep() { 1633 if (getAgent().isEmpty()) { 1634 addAgent(); 1635 } 1636 return getAgent().get(0); 1637 } 1638 1639 /** 1640 * @return {@link #entity} (An entity used in this activity.) 1641 */ 1642 public List<ProvenanceEntityComponent> getEntity() { 1643 if (this.entity == null) 1644 this.entity = new ArrayList<ProvenanceEntityComponent>(); 1645 return this.entity; 1646 } 1647 1648 /** 1649 * @return Returns a reference to <code>this</code> for easy method chaining 1650 */ 1651 public Provenance setEntity(List<ProvenanceEntityComponent> theEntity) { 1652 this.entity = theEntity; 1653 return this; 1654 } 1655 1656 public boolean hasEntity() { 1657 if (this.entity == null) 1658 return false; 1659 for (ProvenanceEntityComponent item : this.entity) 1660 if (!item.isEmpty()) 1661 return true; 1662 return false; 1663 } 1664 1665 public ProvenanceEntityComponent addEntity() { // 3 1666 ProvenanceEntityComponent t = new ProvenanceEntityComponent(); 1667 if (this.entity == null) 1668 this.entity = new ArrayList<ProvenanceEntityComponent>(); 1669 this.entity.add(t); 1670 return t; 1671 } 1672 1673 public Provenance addEntity(ProvenanceEntityComponent t) { // 3 1674 if (t == null) 1675 return this; 1676 if (this.entity == null) 1677 this.entity = new ArrayList<ProvenanceEntityComponent>(); 1678 this.entity.add(t); 1679 return this; 1680 } 1681 1682 /** 1683 * @return The first repetition of repeating field {@link #entity}, creating it 1684 * if it does not already exist 1685 */ 1686 public ProvenanceEntityComponent getEntityFirstRep() { 1687 if (getEntity().isEmpty()) { 1688 addEntity(); 1689 } 1690 return getEntity().get(0); 1691 } 1692 1693 /** 1694 * @return {@link #signature} (A digital signature on the target Reference(s). 1695 * The signer should match a Provenance.agent. The purpose of the 1696 * signature is indicated.) 1697 */ 1698 public List<Signature> getSignature() { 1699 if (this.signature == null) 1700 this.signature = new ArrayList<Signature>(); 1701 return this.signature; 1702 } 1703 1704 /** 1705 * @return Returns a reference to <code>this</code> for easy method chaining 1706 */ 1707 public Provenance setSignature(List<Signature> theSignature) { 1708 this.signature = theSignature; 1709 return this; 1710 } 1711 1712 public boolean hasSignature() { 1713 if (this.signature == null) 1714 return false; 1715 for (Signature item : this.signature) 1716 if (!item.isEmpty()) 1717 return true; 1718 return false; 1719 } 1720 1721 public Signature addSignature() { // 3 1722 Signature t = new Signature(); 1723 if (this.signature == null) 1724 this.signature = new ArrayList<Signature>(); 1725 this.signature.add(t); 1726 return t; 1727 } 1728 1729 public Provenance addSignature(Signature t) { // 3 1730 if (t == null) 1731 return this; 1732 if (this.signature == null) 1733 this.signature = new ArrayList<Signature>(); 1734 this.signature.add(t); 1735 return this; 1736 } 1737 1738 /** 1739 * @return The first repetition of repeating field {@link #signature}, creating 1740 * it if it does not already exist 1741 */ 1742 public Signature getSignatureFirstRep() { 1743 if (getSignature().isEmpty()) { 1744 addSignature(); 1745 } 1746 return getSignature().get(0); 1747 } 1748 1749 protected void listChildren(List<Property> children) { 1750 super.listChildren(children); 1751 children.add(new Property("target", "Reference(Any)", 1752 "The Reference(s) that were generated or updated by the activity described in this resource. A provenance can point to more than one target if multiple resources were created/updated by the same activity.", 1753 0, java.lang.Integer.MAX_VALUE, target)); 1754 children.add(new Property("occurred[x]", "Period|dateTime", "The period during which the activity occurred.", 0, 1, 1755 occurred)); 1756 children.add( 1757 new Property("recorded", "instant", "The instant of time at which the activity was recorded.", 0, 1, recorded)); 1758 children.add(new Property("policy", "uri", 1759 "Policy or plan the activity was defined by. Typically, a single activity may have multiple applicable policy documents, such as patient consent, guarantor funding, etc.", 1760 0, java.lang.Integer.MAX_VALUE, policy)); 1761 children.add( 1762 new Property("location", "Reference(Location)", "Where the activity occurred, if relevant.", 0, 1, location)); 1763 children.add(new Property("reason", "CodeableConcept", "The reason that the activity was taking place.", 0, 1764 java.lang.Integer.MAX_VALUE, reason)); 1765 children.add(new Property("activity", "CodeableConcept", 1766 "An activity is something that occurs over a period of time and acts upon or with entities; it may include consuming, processing, transforming, modifying, relocating, using, or generating entities.", 1767 0, 1, activity)); 1768 children.add(new Property("agent", "", 1769 "An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place.", 1770 0, java.lang.Integer.MAX_VALUE, agent)); 1771 children 1772 .add(new Property("entity", "", "An entity used in this activity.", 0, java.lang.Integer.MAX_VALUE, entity)); 1773 children.add(new Property("signature", "Signature", 1774 "A digital signature on the target Reference(s). The signer should match a Provenance.agent. The purpose of the signature is indicated.", 1775 0, java.lang.Integer.MAX_VALUE, signature)); 1776 } 1777 1778 @Override 1779 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1780 switch (_hash) { 1781 case -880905839: 1782 /* target */ return new Property("target", "Reference(Any)", 1783 "The Reference(s) that were generated or updated by the activity described in this resource. A provenance can point to more than one target if multiple resources were created/updated by the same activity.", 1784 0, java.lang.Integer.MAX_VALUE, target); 1785 case 784181563: 1786 /* occurred[x] */ return new Property("occurred[x]", "Period|dateTime", 1787 "The period during which the activity occurred.", 0, 1, occurred); 1788 case 792816933: 1789 /* occurred */ return new Property("occurred[x]", "Period|dateTime", 1790 "The period during which the activity occurred.", 0, 1, occurred); 1791 case 894082886: 1792 /* occurredPeriod */ return new Property("occurred[x]", "Period|dateTime", 1793 "The period during which the activity occurred.", 0, 1, occurred); 1794 case 1579027424: 1795 /* occurredDateTime */ return new Property("occurred[x]", "Period|dateTime", 1796 "The period during which the activity occurred.", 0, 1, occurred); 1797 case -799233872: 1798 /* recorded */ return new Property("recorded", "instant", 1799 "The instant of time at which the activity was recorded.", 0, 1, recorded); 1800 case -982670030: 1801 /* policy */ return new Property("policy", "uri", 1802 "Policy or plan the activity was defined by. Typically, a single activity may have multiple applicable policy documents, such as patient consent, guarantor funding, etc.", 1803 0, java.lang.Integer.MAX_VALUE, policy); 1804 case 1901043637: 1805 /* location */ return new Property("location", "Reference(Location)", "Where the activity occurred, if relevant.", 1806 0, 1, location); 1807 case -934964668: 1808 /* reason */ return new Property("reason", "CodeableConcept", "The reason that the activity was taking place.", 0, 1809 java.lang.Integer.MAX_VALUE, reason); 1810 case -1655966961: 1811 /* activity */ return new Property("activity", "CodeableConcept", 1812 "An activity is something that occurs over a period of time and acts upon or with entities; it may include consuming, processing, transforming, modifying, relocating, using, or generating entities.", 1813 0, 1, activity); 1814 case 92750597: 1815 /* agent */ return new Property("agent", "", 1816 "An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place.", 1817 0, java.lang.Integer.MAX_VALUE, agent); 1818 case -1298275357: 1819 /* entity */ return new Property("entity", "", "An entity used in this activity.", 0, java.lang.Integer.MAX_VALUE, 1820 entity); 1821 case 1073584312: 1822 /* signature */ return new Property("signature", "Signature", 1823 "A digital signature on the target Reference(s). The signer should match a Provenance.agent. The purpose of the signature is indicated.", 1824 0, java.lang.Integer.MAX_VALUE, signature); 1825 default: 1826 return super.getNamedProperty(_hash, _name, _checkValid); 1827 } 1828 1829 } 1830 1831 @Override 1832 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1833 switch (hash) { 1834 case -880905839: 1835 /* target */ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // Reference 1836 case 792816933: 1837 /* occurred */ return this.occurred == null ? new Base[0] : new Base[] { this.occurred }; // Type 1838 case -799233872: 1839 /* recorded */ return this.recorded == null ? new Base[0] : new Base[] { this.recorded }; // InstantType 1840 case -982670030: 1841 /* policy */ return this.policy == null ? new Base[0] : this.policy.toArray(new Base[this.policy.size()]); // UriType 1842 case 1901043637: 1843 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // Reference 1844 case -934964668: 1845 /* reason */ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableConcept 1846 case -1655966961: 1847 /* activity */ return this.activity == null ? new Base[0] : new Base[] { this.activity }; // CodeableConcept 1848 case 92750597: 1849 /* agent */ return this.agent == null ? new Base[0] : this.agent.toArray(new Base[this.agent.size()]); // ProvenanceAgentComponent 1850 case -1298275357: 1851 /* entity */ return this.entity == null ? new Base[0] : this.entity.toArray(new Base[this.entity.size()]); // ProvenanceEntityComponent 1852 case 1073584312: 1853 /* signature */ return this.signature == null ? new Base[0] 1854 : this.signature.toArray(new Base[this.signature.size()]); // Signature 1855 default: 1856 return super.getProperty(hash, name, checkValid); 1857 } 1858 1859 } 1860 1861 @Override 1862 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1863 switch (hash) { 1864 case -880905839: // target 1865 this.getTarget().add(castToReference(value)); // Reference 1866 return value; 1867 case 792816933: // occurred 1868 this.occurred = castToType(value); // Type 1869 return value; 1870 case -799233872: // recorded 1871 this.recorded = castToInstant(value); // InstantType 1872 return value; 1873 case -982670030: // policy 1874 this.getPolicy().add(castToUri(value)); // UriType 1875 return value; 1876 case 1901043637: // location 1877 this.location = castToReference(value); // Reference 1878 return value; 1879 case -934964668: // reason 1880 this.getReason().add(castToCodeableConcept(value)); // CodeableConcept 1881 return value; 1882 case -1655966961: // activity 1883 this.activity = castToCodeableConcept(value); // CodeableConcept 1884 return value; 1885 case 92750597: // agent 1886 this.getAgent().add((ProvenanceAgentComponent) value); // ProvenanceAgentComponent 1887 return value; 1888 case -1298275357: // entity 1889 this.getEntity().add((ProvenanceEntityComponent) value); // ProvenanceEntityComponent 1890 return value; 1891 case 1073584312: // signature 1892 this.getSignature().add(castToSignature(value)); // Signature 1893 return value; 1894 default: 1895 return super.setProperty(hash, name, value); 1896 } 1897 1898 } 1899 1900 @Override 1901 public Base setProperty(String name, Base value) throws FHIRException { 1902 if (name.equals("target")) { 1903 this.getTarget().add(castToReference(value)); 1904 } else if (name.equals("occurred[x]")) { 1905 this.occurred = castToType(value); // Type 1906 } else if (name.equals("recorded")) { 1907 this.recorded = castToInstant(value); // InstantType 1908 } else if (name.equals("policy")) { 1909 this.getPolicy().add(castToUri(value)); 1910 } else if (name.equals("location")) { 1911 this.location = castToReference(value); // Reference 1912 } else if (name.equals("reason")) { 1913 this.getReason().add(castToCodeableConcept(value)); 1914 } else if (name.equals("activity")) { 1915 this.activity = castToCodeableConcept(value); // CodeableConcept 1916 } else if (name.equals("agent")) { 1917 this.getAgent().add((ProvenanceAgentComponent) value); 1918 } else if (name.equals("entity")) { 1919 this.getEntity().add((ProvenanceEntityComponent) value); 1920 } else if (name.equals("signature")) { 1921 this.getSignature().add(castToSignature(value)); 1922 } else 1923 return super.setProperty(name, value); 1924 return value; 1925 } 1926 1927 @Override 1928 public void removeChild(String name, Base value) throws FHIRException { 1929 if (name.equals("target")) { 1930 this.getTarget().remove(castToReference(value)); 1931 } else if (name.equals("occurred[x]")) { 1932 this.occurred = null; 1933 } else if (name.equals("recorded")) { 1934 this.recorded = null; 1935 } else if (name.equals("policy")) { 1936 this.getPolicy().remove(castToUri(value)); 1937 } else if (name.equals("location")) { 1938 this.location = null; 1939 } else if (name.equals("reason")) { 1940 this.getReason().remove(castToCodeableConcept(value)); 1941 } else if (name.equals("activity")) { 1942 this.activity = null; 1943 } else if (name.equals("agent")) { 1944 this.getAgent().remove((ProvenanceAgentComponent) value); 1945 } else if (name.equals("entity")) { 1946 this.getEntity().remove((ProvenanceEntityComponent) value); 1947 } else if (name.equals("signature")) { 1948 this.getSignature().remove(castToSignature(value)); 1949 } else 1950 super.removeChild(name, value); 1951 1952 } 1953 1954 @Override 1955 public Base makeProperty(int hash, String name) throws FHIRException { 1956 switch (hash) { 1957 case -880905839: 1958 return addTarget(); 1959 case 784181563: 1960 return getOccurred(); 1961 case 792816933: 1962 return getOccurred(); 1963 case -799233872: 1964 return getRecordedElement(); 1965 case -982670030: 1966 return addPolicyElement(); 1967 case 1901043637: 1968 return getLocation(); 1969 case -934964668: 1970 return addReason(); 1971 case -1655966961: 1972 return getActivity(); 1973 case 92750597: 1974 return addAgent(); 1975 case -1298275357: 1976 return addEntity(); 1977 case 1073584312: 1978 return addSignature(); 1979 default: 1980 return super.makeProperty(hash, name); 1981 } 1982 1983 } 1984 1985 @Override 1986 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1987 switch (hash) { 1988 case -880905839: 1989 /* target */ return new String[] { "Reference" }; 1990 case 792816933: 1991 /* occurred */ return new String[] { "Period", "dateTime" }; 1992 case -799233872: 1993 /* recorded */ return new String[] { "instant" }; 1994 case -982670030: 1995 /* policy */ return new String[] { "uri" }; 1996 case 1901043637: 1997 /* location */ return new String[] { "Reference" }; 1998 case -934964668: 1999 /* reason */ return new String[] { "CodeableConcept" }; 2000 case -1655966961: 2001 /* activity */ return new String[] { "CodeableConcept" }; 2002 case 92750597: 2003 /* agent */ return new String[] {}; 2004 case -1298275357: 2005 /* entity */ return new String[] {}; 2006 case 1073584312: 2007 /* signature */ return new String[] { "Signature" }; 2008 default: 2009 return super.getTypesForProperty(hash, name); 2010 } 2011 2012 } 2013 2014 @Override 2015 public Base addChild(String name) throws FHIRException { 2016 if (name.equals("target")) { 2017 return addTarget(); 2018 } else if (name.equals("occurredPeriod")) { 2019 this.occurred = new Period(); 2020 return this.occurred; 2021 } else if (name.equals("occurredDateTime")) { 2022 this.occurred = new DateTimeType(); 2023 return this.occurred; 2024 } else if (name.equals("recorded")) { 2025 throw new FHIRException("Cannot call addChild on a singleton property Provenance.recorded"); 2026 } else if (name.equals("policy")) { 2027 throw new FHIRException("Cannot call addChild on a singleton property Provenance.policy"); 2028 } else if (name.equals("location")) { 2029 this.location = new Reference(); 2030 return this.location; 2031 } else if (name.equals("reason")) { 2032 return addReason(); 2033 } else if (name.equals("activity")) { 2034 this.activity = new CodeableConcept(); 2035 return this.activity; 2036 } else if (name.equals("agent")) { 2037 return addAgent(); 2038 } else if (name.equals("entity")) { 2039 return addEntity(); 2040 } else if (name.equals("signature")) { 2041 return addSignature(); 2042 } else 2043 return super.addChild(name); 2044 } 2045 2046 public String fhirType() { 2047 return "Provenance"; 2048 2049 } 2050 2051 public Provenance copy() { 2052 Provenance dst = new Provenance(); 2053 copyValues(dst); 2054 return dst; 2055 } 2056 2057 public void copyValues(Provenance dst) { 2058 super.copyValues(dst); 2059 if (target != null) { 2060 dst.target = new ArrayList<Reference>(); 2061 for (Reference i : target) 2062 dst.target.add(i.copy()); 2063 } 2064 ; 2065 dst.occurred = occurred == null ? null : occurred.copy(); 2066 dst.recorded = recorded == null ? null : recorded.copy(); 2067 if (policy != null) { 2068 dst.policy = new ArrayList<UriType>(); 2069 for (UriType i : policy) 2070 dst.policy.add(i.copy()); 2071 } 2072 ; 2073 dst.location = location == null ? null : location.copy(); 2074 if (reason != null) { 2075 dst.reason = new ArrayList<CodeableConcept>(); 2076 for (CodeableConcept i : reason) 2077 dst.reason.add(i.copy()); 2078 } 2079 ; 2080 dst.activity = activity == null ? null : activity.copy(); 2081 if (agent != null) { 2082 dst.agent = new ArrayList<ProvenanceAgentComponent>(); 2083 for (ProvenanceAgentComponent i : agent) 2084 dst.agent.add(i.copy()); 2085 } 2086 ; 2087 if (entity != null) { 2088 dst.entity = new ArrayList<ProvenanceEntityComponent>(); 2089 for (ProvenanceEntityComponent i : entity) 2090 dst.entity.add(i.copy()); 2091 } 2092 ; 2093 if (signature != null) { 2094 dst.signature = new ArrayList<Signature>(); 2095 for (Signature i : signature) 2096 dst.signature.add(i.copy()); 2097 } 2098 ; 2099 } 2100 2101 protected Provenance typedCopy() { 2102 return copy(); 2103 } 2104 2105 @Override 2106 public boolean equalsDeep(Base other_) { 2107 if (!super.equalsDeep(other_)) 2108 return false; 2109 if (!(other_ instanceof Provenance)) 2110 return false; 2111 Provenance o = (Provenance) other_; 2112 return compareDeep(target, o.target, true) && compareDeep(occurred, o.occurred, true) 2113 && compareDeep(recorded, o.recorded, true) && compareDeep(policy, o.policy, true) 2114 && compareDeep(location, o.location, true) && compareDeep(reason, o.reason, true) 2115 && compareDeep(activity, o.activity, true) && compareDeep(agent, o.agent, true) 2116 && compareDeep(entity, o.entity, true) && compareDeep(signature, o.signature, true); 2117 } 2118 2119 @Override 2120 public boolean equalsShallow(Base other_) { 2121 if (!super.equalsShallow(other_)) 2122 return false; 2123 if (!(other_ instanceof Provenance)) 2124 return false; 2125 Provenance o = (Provenance) other_; 2126 return compareValues(recorded, o.recorded, true) && compareValues(policy, o.policy, true); 2127 } 2128 2129 public boolean isEmpty() { 2130 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(target, occurred, recorded, policy, location, reason, 2131 activity, agent, entity, signature); 2132 } 2133 2134 @Override 2135 public ResourceType getResourceType() { 2136 return ResourceType.Provenance; 2137 } 2138 2139 /** 2140 * Search parameter: <b>agent-type</b> 2141 * <p> 2142 * Description: <b>How the agent participated</b><br> 2143 * Type: <b>token</b><br> 2144 * Path: <b>Provenance.agent.type</b><br> 2145 * </p> 2146 */ 2147 @SearchParamDefinition(name = "agent-type", path = "Provenance.agent.type", description = "How the agent participated", type = "token") 2148 public static final String SP_AGENT_TYPE = "agent-type"; 2149 /** 2150 * <b>Fluent Client</b> search parameter constant for <b>agent-type</b> 2151 * <p> 2152 * Description: <b>How the agent participated</b><br> 2153 * Type: <b>token</b><br> 2154 * Path: <b>Provenance.agent.type</b><br> 2155 * </p> 2156 */ 2157 public static final ca.uhn.fhir.rest.gclient.TokenClientParam AGENT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2158 SP_AGENT_TYPE); 2159 2160 /** 2161 * Search parameter: <b>agent</b> 2162 * <p> 2163 * Description: <b>Who participated</b><br> 2164 * Type: <b>reference</b><br> 2165 * Path: <b>Provenance.agent.who</b><br> 2166 * </p> 2167 */ 2168 @SearchParamDefinition(name = "agent", path = "Provenance.agent.who", description = "Who participated", type = "reference", providesMembershipIn = { 2169 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 2170 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 2171 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, 2172 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 2173 public static final String SP_AGENT = "agent"; 2174 /** 2175 * <b>Fluent Client</b> search parameter constant for <b>agent</b> 2176 * <p> 2177 * Description: <b>Who participated</b><br> 2178 * Type: <b>reference</b><br> 2179 * Path: <b>Provenance.agent.who</b><br> 2180 * </p> 2181 */ 2182 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AGENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2183 SP_AGENT); 2184 2185 /** 2186 * Constant for fluent queries to be used to add include statements. Specifies 2187 * the path value of "<b>Provenance:agent</b>". 2188 */ 2189 public static final ca.uhn.fhir.model.api.Include INCLUDE_AGENT = new ca.uhn.fhir.model.api.Include( 2190 "Provenance:agent").toLocked(); 2191 2192 /** 2193 * Search parameter: <b>signature-type</b> 2194 * <p> 2195 * Description: <b>Indication of the reason the entity signed the 2196 * object(s)</b><br> 2197 * Type: <b>token</b><br> 2198 * Path: <b>Provenance.signature.type</b><br> 2199 * </p> 2200 */ 2201 @SearchParamDefinition(name = "signature-type", path = "Provenance.signature.type", description = "Indication of the reason the entity signed the object(s)", type = "token") 2202 public static final String SP_SIGNATURE_TYPE = "signature-type"; 2203 /** 2204 * <b>Fluent Client</b> search parameter constant for <b>signature-type</b> 2205 * <p> 2206 * Description: <b>Indication of the reason the entity signed the 2207 * object(s)</b><br> 2208 * Type: <b>token</b><br> 2209 * Path: <b>Provenance.signature.type</b><br> 2210 * </p> 2211 */ 2212 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SIGNATURE_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2213 SP_SIGNATURE_TYPE); 2214 2215 /** 2216 * Search parameter: <b>patient</b> 2217 * <p> 2218 * Description: <b>Target Reference(s) (usually version specific)</b><br> 2219 * Type: <b>reference</b><br> 2220 * Path: <b>Provenance.target</b><br> 2221 * </p> 2222 */ 2223 @SearchParamDefinition(name = "patient", path = "Provenance.target.where(resolve() is Patient)", description = "Target Reference(s) (usually version specific)", type = "reference", providesMembershipIn = { 2224 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 2225 public static final String SP_PATIENT = "patient"; 2226 /** 2227 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2228 * <p> 2229 * Description: <b>Target Reference(s) (usually version specific)</b><br> 2230 * Type: <b>reference</b><br> 2231 * Path: <b>Provenance.target</b><br> 2232 * </p> 2233 */ 2234 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2235 SP_PATIENT); 2236 2237 /** 2238 * Constant for fluent queries to be used to add include statements. Specifies 2239 * the path value of "<b>Provenance:patient</b>". 2240 */ 2241 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 2242 "Provenance:patient").toLocked(); 2243 2244 /** 2245 * Search parameter: <b>location</b> 2246 * <p> 2247 * Description: <b>Where the activity occurred, if relevant</b><br> 2248 * Type: <b>reference</b><br> 2249 * Path: <b>Provenance.location</b><br> 2250 * </p> 2251 */ 2252 @SearchParamDefinition(name = "location", path = "Provenance.location", description = "Where the activity occurred, if relevant", type = "reference", target = { 2253 Location.class }) 2254 public static final String SP_LOCATION = "location"; 2255 /** 2256 * <b>Fluent Client</b> search parameter constant for <b>location</b> 2257 * <p> 2258 * Description: <b>Where the activity occurred, if relevant</b><br> 2259 * Type: <b>reference</b><br> 2260 * Path: <b>Provenance.location</b><br> 2261 * </p> 2262 */ 2263 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2264 SP_LOCATION); 2265 2266 /** 2267 * Constant for fluent queries to be used to add include statements. Specifies 2268 * the path value of "<b>Provenance:location</b>". 2269 */ 2270 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include( 2271 "Provenance:location").toLocked(); 2272 2273 /** 2274 * Search parameter: <b>recorded</b> 2275 * <p> 2276 * Description: <b>When the activity was recorded / updated</b><br> 2277 * Type: <b>date</b><br> 2278 * Path: <b>Provenance.recorded</b><br> 2279 * </p> 2280 */ 2281 @SearchParamDefinition(name = "recorded", path = "Provenance.recorded", description = "When the activity was recorded / updated", type = "date") 2282 public static final String SP_RECORDED = "recorded"; 2283 /** 2284 * <b>Fluent Client</b> search parameter constant for <b>recorded</b> 2285 * <p> 2286 * Description: <b>When the activity was recorded / updated</b><br> 2287 * Type: <b>date</b><br> 2288 * Path: <b>Provenance.recorded</b><br> 2289 * </p> 2290 */ 2291 public static final ca.uhn.fhir.rest.gclient.DateClientParam RECORDED = new ca.uhn.fhir.rest.gclient.DateClientParam( 2292 SP_RECORDED); 2293 2294 /** 2295 * Search parameter: <b>agent-role</b> 2296 * <p> 2297 * Description: <b>What the agents role was</b><br> 2298 * Type: <b>token</b><br> 2299 * Path: <b>Provenance.agent.role</b><br> 2300 * </p> 2301 */ 2302 @SearchParamDefinition(name = "agent-role", path = "Provenance.agent.role", description = "What the agents role was", type = "token") 2303 public static final String SP_AGENT_ROLE = "agent-role"; 2304 /** 2305 * <b>Fluent Client</b> search parameter constant for <b>agent-role</b> 2306 * <p> 2307 * Description: <b>What the agents role was</b><br> 2308 * Type: <b>token</b><br> 2309 * Path: <b>Provenance.agent.role</b><br> 2310 * </p> 2311 */ 2312 public static final ca.uhn.fhir.rest.gclient.TokenClientParam AGENT_ROLE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2313 SP_AGENT_ROLE); 2314 2315 /** 2316 * Search parameter: <b>when</b> 2317 * <p> 2318 * Description: <b>When the activity occurred</b><br> 2319 * Type: <b>date</b><br> 2320 * Path: <b>Provenance.occurredDateTime</b><br> 2321 * </p> 2322 */ 2323 @SearchParamDefinition(name = "when", path = "(Provenance.occurred as dateTime)", description = "When the activity occurred", type = "date") 2324 public static final String SP_WHEN = "when"; 2325 /** 2326 * <b>Fluent Client</b> search parameter constant for <b>when</b> 2327 * <p> 2328 * Description: <b>When the activity occurred</b><br> 2329 * Type: <b>date</b><br> 2330 * Path: <b>Provenance.occurredDateTime</b><br> 2331 * </p> 2332 */ 2333 public static final ca.uhn.fhir.rest.gclient.DateClientParam WHEN = new ca.uhn.fhir.rest.gclient.DateClientParam( 2334 SP_WHEN); 2335 2336 /** 2337 * Search parameter: <b>entity</b> 2338 * <p> 2339 * Description: <b>Identity of entity</b><br> 2340 * Type: <b>reference</b><br> 2341 * Path: <b>Provenance.entity.what</b><br> 2342 * </p> 2343 */ 2344 @SearchParamDefinition(name = "entity", path = "Provenance.entity.what", description = "Identity of entity", type = "reference") 2345 public static final String SP_ENTITY = "entity"; 2346 /** 2347 * <b>Fluent Client</b> search parameter constant for <b>entity</b> 2348 * <p> 2349 * Description: <b>Identity of entity</b><br> 2350 * Type: <b>reference</b><br> 2351 * Path: <b>Provenance.entity.what</b><br> 2352 * </p> 2353 */ 2354 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2355 SP_ENTITY); 2356 2357 /** 2358 * Constant for fluent queries to be used to add include statements. Specifies 2359 * the path value of "<b>Provenance:entity</b>". 2360 */ 2361 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTITY = new ca.uhn.fhir.model.api.Include( 2362 "Provenance:entity").toLocked(); 2363 2364 /** 2365 * Search parameter: <b>target</b> 2366 * <p> 2367 * Description: <b>Target Reference(s) (usually version specific)</b><br> 2368 * Type: <b>reference</b><br> 2369 * Path: <b>Provenance.target</b><br> 2370 * </p> 2371 */ 2372 @SearchParamDefinition(name = "target", path = "Provenance.target", description = "Target Reference(s) (usually version specific)", type = "reference") 2373 public static final String SP_TARGET = "target"; 2374 /** 2375 * <b>Fluent Client</b> search parameter constant for <b>target</b> 2376 * <p> 2377 * Description: <b>Target Reference(s) (usually version specific)</b><br> 2378 * Type: <b>reference</b><br> 2379 * Path: <b>Provenance.target</b><br> 2380 * </p> 2381 */ 2382 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam TARGET = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2383 SP_TARGET); 2384 2385 /** 2386 * Constant for fluent queries to be used to add include statements. Specifies 2387 * the path value of "<b>Provenance:target</b>". 2388 */ 2389 public static final ca.uhn.fhir.model.api.Include INCLUDE_TARGET = new ca.uhn.fhir.model.api.Include( 2390 "Provenance:target").toLocked(); 2391 2392}