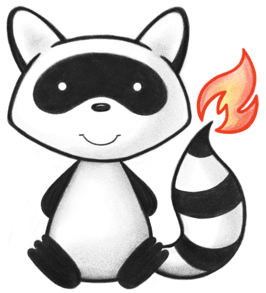
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * A structured set of questions and their answers. The questions are ordered 049 * and grouped into coherent subsets, corresponding to the structure of the 050 * grouping of the questionnaire being responded to. 051 */ 052@ResourceDef(name = "QuestionnaireResponse", profile = "http://hl7.org/fhir/StructureDefinition/QuestionnaireResponse") 053public class QuestionnaireResponse extends DomainResource { 054 055 public enum QuestionnaireResponseStatus { 056 /** 057 * This QuestionnaireResponse has been partially filled out with answers but 058 * changes or additions are still expected to be made to it. 059 */ 060 INPROGRESS, 061 /** 062 * This QuestionnaireResponse has been filled out with answers and the current 063 * content is regarded as definitive. 064 */ 065 COMPLETED, 066 /** 067 * This QuestionnaireResponse has been filled out with answers, then marked as 068 * complete, yet changes or additions have been made to it afterwards. 069 */ 070 AMENDED, 071 /** 072 * This QuestionnaireResponse was entered in error and voided. 073 */ 074 ENTEREDINERROR, 075 /** 076 * This QuestionnaireResponse has been partially filled out with answers but has 077 * been abandoned. It is unknown whether changes or additions are expected to be 078 * made to it. 079 */ 080 STOPPED, 081 /** 082 * added to help the parsers with the generic types 083 */ 084 NULL; 085 086 public static QuestionnaireResponseStatus fromCode(String codeString) throws FHIRException { 087 if (codeString == null || "".equals(codeString)) 088 return null; 089 if ("in-progress".equals(codeString)) 090 return INPROGRESS; 091 if ("completed".equals(codeString)) 092 return COMPLETED; 093 if ("amended".equals(codeString)) 094 return AMENDED; 095 if ("entered-in-error".equals(codeString)) 096 return ENTEREDINERROR; 097 if ("stopped".equals(codeString)) 098 return STOPPED; 099 if (Configuration.isAcceptInvalidEnums()) 100 return null; 101 else 102 throw new FHIRException("Unknown QuestionnaireResponseStatus code '" + codeString + "'"); 103 } 104 105 public String toCode() { 106 switch (this) { 107 case INPROGRESS: 108 return "in-progress"; 109 case COMPLETED: 110 return "completed"; 111 case AMENDED: 112 return "amended"; 113 case ENTEREDINERROR: 114 return "entered-in-error"; 115 case STOPPED: 116 return "stopped"; 117 case NULL: 118 return null; 119 default: 120 return "?"; 121 } 122 } 123 124 public String getSystem() { 125 switch (this) { 126 case INPROGRESS: 127 return "http://hl7.org/fhir/questionnaire-answers-status"; 128 case COMPLETED: 129 return "http://hl7.org/fhir/questionnaire-answers-status"; 130 case AMENDED: 131 return "http://hl7.org/fhir/questionnaire-answers-status"; 132 case ENTEREDINERROR: 133 return "http://hl7.org/fhir/questionnaire-answers-status"; 134 case STOPPED: 135 return "http://hl7.org/fhir/questionnaire-answers-status"; 136 case NULL: 137 return null; 138 default: 139 return "?"; 140 } 141 } 142 143 public String getDefinition() { 144 switch (this) { 145 case INPROGRESS: 146 return "This QuestionnaireResponse has been partially filled out with answers but changes or additions are still expected to be made to it."; 147 case COMPLETED: 148 return "This QuestionnaireResponse has been filled out with answers and the current content is regarded as definitive."; 149 case AMENDED: 150 return "This QuestionnaireResponse has been filled out with answers, then marked as complete, yet changes or additions have been made to it afterwards."; 151 case ENTEREDINERROR: 152 return "This QuestionnaireResponse was entered in error and voided."; 153 case STOPPED: 154 return "This QuestionnaireResponse has been partially filled out with answers but has been abandoned. It is unknown whether changes or additions are expected to be made to it."; 155 case NULL: 156 return null; 157 default: 158 return "?"; 159 } 160 } 161 162 public String getDisplay() { 163 switch (this) { 164 case INPROGRESS: 165 return "In Progress"; 166 case COMPLETED: 167 return "Completed"; 168 case AMENDED: 169 return "Amended"; 170 case ENTEREDINERROR: 171 return "Entered in Error"; 172 case STOPPED: 173 return "Stopped"; 174 case NULL: 175 return null; 176 default: 177 return "?"; 178 } 179 } 180 } 181 182 public static class QuestionnaireResponseStatusEnumFactory implements EnumFactory<QuestionnaireResponseStatus> { 183 public QuestionnaireResponseStatus fromCode(String codeString) throws IllegalArgumentException { 184 if (codeString == null || "".equals(codeString)) 185 if (codeString == null || "".equals(codeString)) 186 return null; 187 if ("in-progress".equals(codeString)) 188 return QuestionnaireResponseStatus.INPROGRESS; 189 if ("completed".equals(codeString)) 190 return QuestionnaireResponseStatus.COMPLETED; 191 if ("amended".equals(codeString)) 192 return QuestionnaireResponseStatus.AMENDED; 193 if ("entered-in-error".equals(codeString)) 194 return QuestionnaireResponseStatus.ENTEREDINERROR; 195 if ("stopped".equals(codeString)) 196 return QuestionnaireResponseStatus.STOPPED; 197 throw new IllegalArgumentException("Unknown QuestionnaireResponseStatus code '" + codeString + "'"); 198 } 199 200 public Enumeration<QuestionnaireResponseStatus> fromType(PrimitiveType<?> code) throws FHIRException { 201 if (code == null) 202 return null; 203 if (code.isEmpty()) 204 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.NULL, code); 205 String codeString = code.asStringValue(); 206 if (codeString == null || "".equals(codeString)) 207 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.NULL, code); 208 if ("in-progress".equals(codeString)) 209 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.INPROGRESS, code); 210 if ("completed".equals(codeString)) 211 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.COMPLETED, code); 212 if ("amended".equals(codeString)) 213 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.AMENDED, code); 214 if ("entered-in-error".equals(codeString)) 215 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.ENTEREDINERROR, code); 216 if ("stopped".equals(codeString)) 217 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.STOPPED, code); 218 throw new FHIRException("Unknown QuestionnaireResponseStatus code '" + codeString + "'"); 219 } 220 221 public String toCode(QuestionnaireResponseStatus code) { 222 if (code == QuestionnaireResponseStatus.NULL) 223 return null; 224 if (code == QuestionnaireResponseStatus.INPROGRESS) 225 return "in-progress"; 226 if (code == QuestionnaireResponseStatus.COMPLETED) 227 return "completed"; 228 if (code == QuestionnaireResponseStatus.AMENDED) 229 return "amended"; 230 if (code == QuestionnaireResponseStatus.ENTEREDINERROR) 231 return "entered-in-error"; 232 if (code == QuestionnaireResponseStatus.STOPPED) 233 return "stopped"; 234 return "?"; 235 } 236 237 public String toSystem(QuestionnaireResponseStatus code) { 238 return code.getSystem(); 239 } 240 } 241 242 @Block() 243 public static class QuestionnaireResponseItemComponent extends BackboneElement implements IBaseBackboneElement { 244 /** 245 * The item from the Questionnaire that corresponds to this item in the 246 * QuestionnaireResponse resource. 247 */ 248 @Child(name = "linkId", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 249 @Description(shortDefinition = "Pointer to specific item from Questionnaire", formalDefinition = "The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource.") 250 protected StringType linkId; 251 252 /** 253 * A reference to an [[[ElementDefinition]]] that provides the details for the 254 * item. 255 */ 256 @Child(name = "definition", type = { 257 UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 258 @Description(shortDefinition = "ElementDefinition - details for the item", formalDefinition = "A reference to an [[[ElementDefinition]]] that provides the details for the item.") 259 protected UriType definition; 260 261 /** 262 * Text that is displayed above the contents of the group or as the text of the 263 * question being answered. 264 */ 265 @Child(name = "text", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 266 @Description(shortDefinition = "Name for group or question text", formalDefinition = "Text that is displayed above the contents of the group or as the text of the question being answered.") 267 protected StringType text; 268 269 /** 270 * The respondent's answer(s) to the question. 271 */ 272 @Child(name = "answer", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 273 @Description(shortDefinition = "The response(s) to the question", formalDefinition = "The respondent's answer(s) to the question.") 274 protected List<QuestionnaireResponseItemAnswerComponent> answer; 275 276 /** 277 * Questions or sub-groups nested beneath a question or group. 278 */ 279 @Child(name = "item", type = { 280 QuestionnaireResponseItemComponent.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 281 @Description(shortDefinition = "Nested questionnaire response items", formalDefinition = "Questions or sub-groups nested beneath a question or group.") 282 protected List<QuestionnaireResponseItemComponent> item; 283 284 private static final long serialVersionUID = -1395483402L; 285 286 /** 287 * Constructor 288 */ 289 public QuestionnaireResponseItemComponent() { 290 super(); 291 } 292 293 /** 294 * Constructor 295 */ 296 public QuestionnaireResponseItemComponent(StringType linkId) { 297 super(); 298 this.linkId = linkId; 299 } 300 301 /** 302 * @return {@link #linkId} (The item from the Questionnaire that corresponds to 303 * this item in the QuestionnaireResponse resource.). This is the 304 * underlying object with id, value and extensions. The accessor 305 * "getLinkId" gives direct access to the value 306 */ 307 public StringType getLinkIdElement() { 308 if (this.linkId == null) 309 if (Configuration.errorOnAutoCreate()) 310 throw new Error("Attempt to auto-create QuestionnaireResponseItemComponent.linkId"); 311 else if (Configuration.doAutoCreate()) 312 this.linkId = new StringType(); // bb 313 return this.linkId; 314 } 315 316 public boolean hasLinkIdElement() { 317 return this.linkId != null && !this.linkId.isEmpty(); 318 } 319 320 public boolean hasLinkId() { 321 return this.linkId != null && !this.linkId.isEmpty(); 322 } 323 324 /** 325 * @param value {@link #linkId} (The item from the Questionnaire that 326 * corresponds to this item in the QuestionnaireResponse 327 * resource.). This is the underlying object with id, value and 328 * extensions. The accessor "getLinkId" gives direct access to the 329 * value 330 */ 331 public QuestionnaireResponseItemComponent setLinkIdElement(StringType value) { 332 this.linkId = value; 333 return this; 334 } 335 336 /** 337 * @return The item from the Questionnaire that corresponds to this item in the 338 * QuestionnaireResponse resource. 339 */ 340 public String getLinkId() { 341 return this.linkId == null ? null : this.linkId.getValue(); 342 } 343 344 /** 345 * @param value The item from the Questionnaire that corresponds to this item in 346 * the QuestionnaireResponse resource. 347 */ 348 public QuestionnaireResponseItemComponent setLinkId(String value) { 349 if (this.linkId == null) 350 this.linkId = new StringType(); 351 this.linkId.setValue(value); 352 return this; 353 } 354 355 /** 356 * @return {@link #definition} (A reference to an [[[ElementDefinition]]] that 357 * provides the details for the item.). This is the underlying object 358 * with id, value and extensions. The accessor "getDefinition" gives 359 * direct access to the value 360 */ 361 public UriType getDefinitionElement() { 362 if (this.definition == null) 363 if (Configuration.errorOnAutoCreate()) 364 throw new Error("Attempt to auto-create QuestionnaireResponseItemComponent.definition"); 365 else if (Configuration.doAutoCreate()) 366 this.definition = new UriType(); // bb 367 return this.definition; 368 } 369 370 public boolean hasDefinitionElement() { 371 return this.definition != null && !this.definition.isEmpty(); 372 } 373 374 public boolean hasDefinition() { 375 return this.definition != null && !this.definition.isEmpty(); 376 } 377 378 /** 379 * @param value {@link #definition} (A reference to an [[[ElementDefinition]]] 380 * that provides the details for the item.). This is the underlying 381 * object with id, value and extensions. The accessor 382 * "getDefinition" gives direct access to the value 383 */ 384 public QuestionnaireResponseItemComponent setDefinitionElement(UriType value) { 385 this.definition = value; 386 return this; 387 } 388 389 /** 390 * @return A reference to an [[[ElementDefinition]]] that provides the details 391 * for the item. 392 */ 393 public String getDefinition() { 394 return this.definition == null ? null : this.definition.getValue(); 395 } 396 397 /** 398 * @param value A reference to an [[[ElementDefinition]]] that provides the 399 * details for the item. 400 */ 401 public QuestionnaireResponseItemComponent setDefinition(String value) { 402 if (Utilities.noString(value)) 403 this.definition = null; 404 else { 405 if (this.definition == null) 406 this.definition = new UriType(); 407 this.definition.setValue(value); 408 } 409 return this; 410 } 411 412 /** 413 * @return {@link #text} (Text that is displayed above the contents of the group 414 * or as the text of the question being answered.). This is the 415 * underlying object with id, value and extensions. The accessor 416 * "getText" gives direct access to the value 417 */ 418 public StringType getTextElement() { 419 if (this.text == null) 420 if (Configuration.errorOnAutoCreate()) 421 throw new Error("Attempt to auto-create QuestionnaireResponseItemComponent.text"); 422 else if (Configuration.doAutoCreate()) 423 this.text = new StringType(); // bb 424 return this.text; 425 } 426 427 public boolean hasTextElement() { 428 return this.text != null && !this.text.isEmpty(); 429 } 430 431 public boolean hasText() { 432 return this.text != null && !this.text.isEmpty(); 433 } 434 435 /** 436 * @param value {@link #text} (Text that is displayed above the contents of the 437 * group or as the text of the question being answered.). This is 438 * the underlying object with id, value and extensions. The 439 * accessor "getText" gives direct access to the value 440 */ 441 public QuestionnaireResponseItemComponent setTextElement(StringType value) { 442 this.text = value; 443 return this; 444 } 445 446 /** 447 * @return Text that is displayed above the contents of the group or as the text 448 * of the question being answered. 449 */ 450 public String getText() { 451 return this.text == null ? null : this.text.getValue(); 452 } 453 454 /** 455 * @param value Text that is displayed above the contents of the group or as the 456 * text of the question being answered. 457 */ 458 public QuestionnaireResponseItemComponent setText(String value) { 459 if (Utilities.noString(value)) 460 this.text = null; 461 else { 462 if (this.text == null) 463 this.text = new StringType(); 464 this.text.setValue(value); 465 } 466 return this; 467 } 468 469 /** 470 * @return {@link #answer} (The respondent's answer(s) to the question.) 471 */ 472 public List<QuestionnaireResponseItemAnswerComponent> getAnswer() { 473 if (this.answer == null) 474 this.answer = new ArrayList<QuestionnaireResponseItemAnswerComponent>(); 475 return this.answer; 476 } 477 478 /** 479 * @return Returns a reference to <code>this</code> for easy method chaining 480 */ 481 public QuestionnaireResponseItemComponent setAnswer(List<QuestionnaireResponseItemAnswerComponent> theAnswer) { 482 this.answer = theAnswer; 483 return this; 484 } 485 486 public boolean hasAnswer() { 487 if (this.answer == null) 488 return false; 489 for (QuestionnaireResponseItemAnswerComponent item : this.answer) 490 if (!item.isEmpty()) 491 return true; 492 return false; 493 } 494 495 public QuestionnaireResponseItemAnswerComponent addAnswer() { // 3 496 QuestionnaireResponseItemAnswerComponent t = new QuestionnaireResponseItemAnswerComponent(); 497 if (this.answer == null) 498 this.answer = new ArrayList<QuestionnaireResponseItemAnswerComponent>(); 499 this.answer.add(t); 500 return t; 501 } 502 503 public QuestionnaireResponseItemComponent addAnswer(QuestionnaireResponseItemAnswerComponent t) { // 3 504 if (t == null) 505 return this; 506 if (this.answer == null) 507 this.answer = new ArrayList<QuestionnaireResponseItemAnswerComponent>(); 508 this.answer.add(t); 509 return this; 510 } 511 512 /** 513 * @return The first repetition of repeating field {@link #answer}, creating it 514 * if it does not already exist 515 */ 516 public QuestionnaireResponseItemAnswerComponent getAnswerFirstRep() { 517 if (getAnswer().isEmpty()) { 518 addAnswer(); 519 } 520 return getAnswer().get(0); 521 } 522 523 /** 524 * @return {@link #item} (Questions or sub-groups nested beneath a question or 525 * group.) 526 */ 527 public List<QuestionnaireResponseItemComponent> getItem() { 528 if (this.item == null) 529 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 530 return this.item; 531 } 532 533 /** 534 * @return Returns a reference to <code>this</code> for easy method chaining 535 */ 536 public QuestionnaireResponseItemComponent setItem(List<QuestionnaireResponseItemComponent> theItem) { 537 this.item = theItem; 538 return this; 539 } 540 541 public boolean hasItem() { 542 if (this.item == null) 543 return false; 544 for (QuestionnaireResponseItemComponent item : this.item) 545 if (!item.isEmpty()) 546 return true; 547 return false; 548 } 549 550 public QuestionnaireResponseItemComponent addItem() { // 3 551 QuestionnaireResponseItemComponent t = new QuestionnaireResponseItemComponent(); 552 if (this.item == null) 553 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 554 this.item.add(t); 555 return t; 556 } 557 558 public QuestionnaireResponseItemComponent addItem(QuestionnaireResponseItemComponent t) { // 3 559 if (t == null) 560 return this; 561 if (this.item == null) 562 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 563 this.item.add(t); 564 return this; 565 } 566 567 /** 568 * @return The first repetition of repeating field {@link #item}, creating it if 569 * it does not already exist 570 */ 571 public QuestionnaireResponseItemComponent getItemFirstRep() { 572 if (getItem().isEmpty()) { 573 addItem(); 574 } 575 return getItem().get(0); 576 } 577 578 protected void listChildren(List<Property> children) { 579 super.listChildren(children); 580 children.add(new Property("linkId", "string", 581 "The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource.", 0, 1, 582 linkId)); 583 children.add(new Property("definition", "uri", 584 "A reference to an [[[ElementDefinition]]] that provides the details for the item.", 0, 1, definition)); 585 children.add(new Property("text", "string", 586 "Text that is displayed above the contents of the group or as the text of the question being answered.", 0, 1, 587 text)); 588 children.add(new Property("answer", "", "The respondent's answer(s) to the question.", 0, 589 java.lang.Integer.MAX_VALUE, answer)); 590 children.add(new Property("item", "@QuestionnaireResponse.item", 591 "Questions or sub-groups nested beneath a question or group.", 0, java.lang.Integer.MAX_VALUE, item)); 592 } 593 594 @Override 595 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 596 switch (_hash) { 597 case -1102667083: 598 /* linkId */ return new Property("linkId", "string", 599 "The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource.", 0, 600 1, linkId); 601 case -1014418093: 602 /* definition */ return new Property("definition", "uri", 603 "A reference to an [[[ElementDefinition]]] that provides the details for the item.", 0, 1, definition); 604 case 3556653: 605 /* text */ return new Property("text", "string", 606 "Text that is displayed above the contents of the group or as the text of the question being answered.", 0, 607 1, text); 608 case -1412808770: 609 /* answer */ return new Property("answer", "", "The respondent's answer(s) to the question.", 0, 610 java.lang.Integer.MAX_VALUE, answer); 611 case 3242771: 612 /* item */ return new Property("item", "@QuestionnaireResponse.item", 613 "Questions or sub-groups nested beneath a question or group.", 0, java.lang.Integer.MAX_VALUE, item); 614 default: 615 return super.getNamedProperty(_hash, _name, _checkValid); 616 } 617 618 } 619 620 @Override 621 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 622 switch (hash) { 623 case -1102667083: 624 /* linkId */ return this.linkId == null ? new Base[0] : new Base[] { this.linkId }; // StringType 625 case -1014418093: 626 /* definition */ return this.definition == null ? new Base[0] : new Base[] { this.definition }; // UriType 627 case 3556653: 628 /* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // StringType 629 case -1412808770: 630 /* answer */ return this.answer == null ? new Base[0] : this.answer.toArray(new Base[this.answer.size()]); // QuestionnaireResponseItemAnswerComponent 631 case 3242771: 632 /* item */ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // QuestionnaireResponseItemComponent 633 default: 634 return super.getProperty(hash, name, checkValid); 635 } 636 637 } 638 639 @Override 640 public Base setProperty(int hash, String name, Base value) throws FHIRException { 641 switch (hash) { 642 case -1102667083: // linkId 643 this.linkId = castToString(value); // StringType 644 return value; 645 case -1014418093: // definition 646 this.definition = castToUri(value); // UriType 647 return value; 648 case 3556653: // text 649 this.text = castToString(value); // StringType 650 return value; 651 case -1412808770: // answer 652 this.getAnswer().add((QuestionnaireResponseItemAnswerComponent) value); // QuestionnaireResponseItemAnswerComponent 653 return value; 654 case 3242771: // item 655 this.getItem().add((QuestionnaireResponseItemComponent) value); // QuestionnaireResponseItemComponent 656 return value; 657 default: 658 return super.setProperty(hash, name, value); 659 } 660 661 } 662 663 @Override 664 public Base setProperty(String name, Base value) throws FHIRException { 665 if (name.equals("linkId")) { 666 this.linkId = castToString(value); // StringType 667 } else if (name.equals("definition")) { 668 this.definition = castToUri(value); // UriType 669 } else if (name.equals("text")) { 670 this.text = castToString(value); // StringType 671 } else if (name.equals("answer")) { 672 this.getAnswer().add((QuestionnaireResponseItemAnswerComponent) value); 673 } else if (name.equals("item")) { 674 this.getItem().add((QuestionnaireResponseItemComponent) value); 675 } else 676 return super.setProperty(name, value); 677 return value; 678 } 679 680 @Override 681 public void removeChild(String name, Base value) throws FHIRException { 682 if (name.equals("linkId")) { 683 this.linkId = null; 684 } else if (name.equals("definition")) { 685 this.definition = null; 686 } else if (name.equals("text")) { 687 this.text = null; 688 } else if (name.equals("answer")) { 689 this.getAnswer().remove((QuestionnaireResponseItemAnswerComponent) value); 690 } else if (name.equals("item")) { 691 this.getItem().remove((QuestionnaireResponseItemComponent) value); 692 } else 693 super.removeChild(name, value); 694 695 } 696 697 @Override 698 public Base makeProperty(int hash, String name) throws FHIRException { 699 switch (hash) { 700 case -1102667083: 701 return getLinkIdElement(); 702 case -1014418093: 703 return getDefinitionElement(); 704 case 3556653: 705 return getTextElement(); 706 case -1412808770: 707 return addAnswer(); 708 case 3242771: 709 return addItem(); 710 default: 711 return super.makeProperty(hash, name); 712 } 713 714 } 715 716 @Override 717 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 718 switch (hash) { 719 case -1102667083: 720 /* linkId */ return new String[] { "string" }; 721 case -1014418093: 722 /* definition */ return new String[] { "uri" }; 723 case 3556653: 724 /* text */ return new String[] { "string" }; 725 case -1412808770: 726 /* answer */ return new String[] {}; 727 case 3242771: 728 /* item */ return new String[] { "@QuestionnaireResponse.item" }; 729 default: 730 return super.getTypesForProperty(hash, name); 731 } 732 733 } 734 735 @Override 736 public Base addChild(String name) throws FHIRException { 737 if (name.equals("linkId")) { 738 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.linkId"); 739 } else if (name.equals("definition")) { 740 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.definition"); 741 } else if (name.equals("text")) { 742 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.text"); 743 } else if (name.equals("answer")) { 744 return addAnswer(); 745 } else if (name.equals("item")) { 746 return addItem(); 747 } else 748 return super.addChild(name); 749 } 750 751 public QuestionnaireResponseItemComponent copy() { 752 QuestionnaireResponseItemComponent dst = new QuestionnaireResponseItemComponent(); 753 copyValues(dst); 754 return dst; 755 } 756 757 public void copyValues(QuestionnaireResponseItemComponent dst) { 758 super.copyValues(dst); 759 dst.linkId = linkId == null ? null : linkId.copy(); 760 dst.definition = definition == null ? null : definition.copy(); 761 dst.text = text == null ? null : text.copy(); 762 if (answer != null) { 763 dst.answer = new ArrayList<QuestionnaireResponseItemAnswerComponent>(); 764 for (QuestionnaireResponseItemAnswerComponent i : answer) 765 dst.answer.add(i.copy()); 766 } 767 ; 768 if (item != null) { 769 dst.item = new ArrayList<QuestionnaireResponseItemComponent>(); 770 for (QuestionnaireResponseItemComponent i : item) 771 dst.item.add(i.copy()); 772 } 773 ; 774 } 775 776 @Override 777 public boolean equalsDeep(Base other_) { 778 if (!super.equalsDeep(other_)) 779 return false; 780 if (!(other_ instanceof QuestionnaireResponseItemComponent)) 781 return false; 782 QuestionnaireResponseItemComponent o = (QuestionnaireResponseItemComponent) other_; 783 return compareDeep(linkId, o.linkId, true) && compareDeep(definition, o.definition, true) 784 && compareDeep(text, o.text, true) && compareDeep(answer, o.answer, true) && compareDeep(item, o.item, true); 785 } 786 787 @Override 788 public boolean equalsShallow(Base other_) { 789 if (!super.equalsShallow(other_)) 790 return false; 791 if (!(other_ instanceof QuestionnaireResponseItemComponent)) 792 return false; 793 QuestionnaireResponseItemComponent o = (QuestionnaireResponseItemComponent) other_; 794 return compareValues(linkId, o.linkId, true) && compareValues(definition, o.definition, true) 795 && compareValues(text, o.text, true); 796 } 797 798 public boolean isEmpty() { 799 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(linkId, definition, text, answer, item); 800 } 801 802 public String fhirType() { 803 return "QuestionnaireResponse.item"; 804 805 } 806 807 } 808 809 @Block() 810 public static class QuestionnaireResponseItemAnswerComponent extends BackboneElement implements IBaseBackboneElement { 811 /** 812 * The answer (or one of the answers) provided by the respondent to the 813 * question. 814 */ 815 @Child(name = "value", type = { BooleanType.class, DecimalType.class, IntegerType.class, DateType.class, 816 DateTimeType.class, TimeType.class, StringType.class, UriType.class, Attachment.class, Coding.class, 817 Quantity.class, Reference.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 818 @Description(shortDefinition = "Single-valued answer to the question", formalDefinition = "The answer (or one of the answers) provided by the respondent to the question.") 819 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/questionnaire-answers") 820 protected Type value; 821 822 /** 823 * Nested groups and/or questions found within this particular answer. 824 */ 825 @Child(name = "item", type = { 826 QuestionnaireResponseItemComponent.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 827 @Description(shortDefinition = "Nested groups and questions", formalDefinition = "Nested groups and/or questions found within this particular answer.") 828 protected List<QuestionnaireResponseItemComponent> item; 829 830 private static final long serialVersionUID = 2052422636L; 831 832 /** 833 * Constructor 834 */ 835 public QuestionnaireResponseItemAnswerComponent() { 836 super(); 837 } 838 839 /** 840 * @return {@link #value} (The answer (or one of the answers) provided by the 841 * respondent to the question.) 842 */ 843 public Type getValue() { 844 return this.value; 845 } 846 847 /** 848 * @return {@link #value} (The answer (or one of the answers) provided by the 849 * respondent to the question.) 850 */ 851 public BooleanType getValueBooleanType() throws FHIRException { 852 if (this.value == null) 853 this.value = new BooleanType(); 854 if (!(this.value instanceof BooleanType)) 855 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 856 + this.value.getClass().getName() + " was encountered"); 857 return (BooleanType) this.value; 858 } 859 860 public boolean hasValueBooleanType() { 861 return this.value instanceof BooleanType; 862 } 863 864 /** 865 * @return {@link #value} (The answer (or one of the answers) provided by the 866 * respondent to the question.) 867 */ 868 public DecimalType getValueDecimalType() throws FHIRException { 869 if (this.value == null) 870 this.value = new DecimalType(); 871 if (!(this.value instanceof DecimalType)) 872 throw new FHIRException("Type mismatch: the type DecimalType was expected, but " 873 + this.value.getClass().getName() + " was encountered"); 874 return (DecimalType) this.value; 875 } 876 877 public boolean hasValueDecimalType() { 878 return this.value instanceof DecimalType; 879 } 880 881 /** 882 * @return {@link #value} (The answer (or one of the answers) provided by the 883 * respondent to the question.) 884 */ 885 public IntegerType getValueIntegerType() throws FHIRException { 886 if (this.value == null) 887 this.value = new IntegerType(); 888 if (!(this.value instanceof IntegerType)) 889 throw new FHIRException("Type mismatch: the type IntegerType was expected, but " 890 + this.value.getClass().getName() + " was encountered"); 891 return (IntegerType) this.value; 892 } 893 894 public boolean hasValueIntegerType() { 895 return this.value instanceof IntegerType; 896 } 897 898 /** 899 * @return {@link #value} (The answer (or one of the answers) provided by the 900 * respondent to the question.) 901 */ 902 public DateType getValueDateType() throws FHIRException { 903 if (this.value == null) 904 this.value = new DateType(); 905 if (!(this.value instanceof DateType)) 906 throw new FHIRException("Type mismatch: the type DateType was expected, but " + this.value.getClass().getName() 907 + " was encountered"); 908 return (DateType) this.value; 909 } 910 911 public boolean hasValueDateType() { 912 return this.value instanceof DateType; 913 } 914 915 /** 916 * @return {@link #value} (The answer (or one of the answers) provided by the 917 * respondent to the question.) 918 */ 919 public DateTimeType getValueDateTimeType() throws FHIRException { 920 if (this.value == null) 921 this.value = new DateTimeType(); 922 if (!(this.value instanceof DateTimeType)) 923 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 924 + this.value.getClass().getName() + " was encountered"); 925 return (DateTimeType) this.value; 926 } 927 928 public boolean hasValueDateTimeType() { 929 return this.value instanceof DateTimeType; 930 } 931 932 /** 933 * @return {@link #value} (The answer (or one of the answers) provided by the 934 * respondent to the question.) 935 */ 936 public TimeType getValueTimeType() throws FHIRException { 937 if (this.value == null) 938 this.value = new TimeType(); 939 if (!(this.value instanceof TimeType)) 940 throw new FHIRException("Type mismatch: the type TimeType was expected, but " + this.value.getClass().getName() 941 + " was encountered"); 942 return (TimeType) this.value; 943 } 944 945 public boolean hasValueTimeType() { 946 return this.value instanceof TimeType; 947 } 948 949 /** 950 * @return {@link #value} (The answer (or one of the answers) provided by the 951 * respondent to the question.) 952 */ 953 public StringType getValueStringType() throws FHIRException { 954 if (this.value == null) 955 this.value = new StringType(); 956 if (!(this.value instanceof StringType)) 957 throw new FHIRException("Type mismatch: the type StringType was expected, but " 958 + this.value.getClass().getName() + " was encountered"); 959 return (StringType) this.value; 960 } 961 962 public boolean hasValueStringType() { 963 return this.value instanceof StringType; 964 } 965 966 /** 967 * @return {@link #value} (The answer (or one of the answers) provided by the 968 * respondent to the question.) 969 */ 970 public UriType getValueUriType() throws FHIRException { 971 if (this.value == null) 972 this.value = new UriType(); 973 if (!(this.value instanceof UriType)) 974 throw new FHIRException("Type mismatch: the type UriType was expected, but " + this.value.getClass().getName() 975 + " was encountered"); 976 return (UriType) this.value; 977 } 978 979 public boolean hasValueUriType() { 980 return this.value instanceof UriType; 981 } 982 983 /** 984 * @return {@link #value} (The answer (or one of the answers) provided by the 985 * respondent to the question.) 986 */ 987 public Attachment getValueAttachment() throws FHIRException { 988 if (this.value == null) 989 this.value = new Attachment(); 990 if (!(this.value instanceof Attachment)) 991 throw new FHIRException("Type mismatch: the type Attachment was expected, but " 992 + this.value.getClass().getName() + " was encountered"); 993 return (Attachment) this.value; 994 } 995 996 public boolean hasValueAttachment() { 997 return this.value instanceof Attachment; 998 } 999 1000 /** 1001 * @return {@link #value} (The answer (or one of the answers) provided by the 1002 * respondent to the question.) 1003 */ 1004 public Coding getValueCoding() throws FHIRException { 1005 if (this.value == null) 1006 this.value = new Coding(); 1007 if (!(this.value instanceof Coding)) 1008 throw new FHIRException( 1009 "Type mismatch: the type Coding was expected, but " + this.value.getClass().getName() + " was encountered"); 1010 return (Coding) this.value; 1011 } 1012 1013 public boolean hasValueCoding() { 1014 return this.value instanceof Coding; 1015 } 1016 1017 /** 1018 * @return {@link #value} (The answer (or one of the answers) provided by the 1019 * respondent to the question.) 1020 */ 1021 public Quantity getValueQuantity() throws FHIRException { 1022 if (this.value == null) 1023 this.value = new Quantity(); 1024 if (!(this.value instanceof Quantity)) 1025 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.value.getClass().getName() 1026 + " was encountered"); 1027 return (Quantity) this.value; 1028 } 1029 1030 public boolean hasValueQuantity() { 1031 return this.value instanceof Quantity; 1032 } 1033 1034 /** 1035 * @return {@link #value} (The answer (or one of the answers) provided by the 1036 * respondent to the question.) 1037 */ 1038 public Reference getValueReference() throws FHIRException { 1039 if (this.value == null) 1040 this.value = new Reference(); 1041 if (!(this.value instanceof Reference)) 1042 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.value.getClass().getName() 1043 + " was encountered"); 1044 return (Reference) this.value; 1045 } 1046 1047 public boolean hasValueReference() { 1048 return this.value instanceof Reference; 1049 } 1050 1051 public boolean hasValue() { 1052 return this.value != null && !this.value.isEmpty(); 1053 } 1054 1055 /** 1056 * @param value {@link #value} (The answer (or one of the answers) provided by 1057 * the respondent to the question.) 1058 */ 1059 public QuestionnaireResponseItemAnswerComponent setValue(Type value) { 1060 if (value != null 1061 && !(value instanceof BooleanType || value instanceof DecimalType || value instanceof IntegerType 1062 || value instanceof DateType || value instanceof DateTimeType || value instanceof TimeType 1063 || value instanceof StringType || value instanceof UriType || value instanceof Attachment 1064 || value instanceof Coding || value instanceof Quantity || value instanceof Reference)) 1065 throw new Error("Not the right type for QuestionnaireResponse.item.answer.value[x]: " + value.fhirType()); 1066 this.value = value; 1067 return this; 1068 } 1069 1070 /** 1071 * @return {@link #item} (Nested groups and/or questions found within this 1072 * particular answer.) 1073 */ 1074 public List<QuestionnaireResponseItemComponent> getItem() { 1075 if (this.item == null) 1076 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 1077 return this.item; 1078 } 1079 1080 /** 1081 * @return Returns a reference to <code>this</code> for easy method chaining 1082 */ 1083 public QuestionnaireResponseItemAnswerComponent setItem(List<QuestionnaireResponseItemComponent> theItem) { 1084 this.item = theItem; 1085 return this; 1086 } 1087 1088 public boolean hasItem() { 1089 if (this.item == null) 1090 return false; 1091 for (QuestionnaireResponseItemComponent item : this.item) 1092 if (!item.isEmpty()) 1093 return true; 1094 return false; 1095 } 1096 1097 public QuestionnaireResponseItemComponent addItem() { // 3 1098 QuestionnaireResponseItemComponent t = new QuestionnaireResponseItemComponent(); 1099 if (this.item == null) 1100 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 1101 this.item.add(t); 1102 return t; 1103 } 1104 1105 public QuestionnaireResponseItemAnswerComponent addItem(QuestionnaireResponseItemComponent t) { // 3 1106 if (t == null) 1107 return this; 1108 if (this.item == null) 1109 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 1110 this.item.add(t); 1111 return this; 1112 } 1113 1114 /** 1115 * @return The first repetition of repeating field {@link #item}, creating it if 1116 * it does not already exist 1117 */ 1118 public QuestionnaireResponseItemComponent getItemFirstRep() { 1119 if (getItem().isEmpty()) { 1120 addItem(); 1121 } 1122 return getItem().get(0); 1123 } 1124 1125 protected void listChildren(List<Property> children) { 1126 super.listChildren(children); 1127 children.add(new Property("value[x]", 1128 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1129 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value)); 1130 children.add(new Property("item", "@QuestionnaireResponse.item", 1131 "Nested groups and/or questions found within this particular answer.", 0, java.lang.Integer.MAX_VALUE, item)); 1132 } 1133 1134 @Override 1135 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1136 switch (_hash) { 1137 case -1410166417: 1138 /* value[x] */ return new Property("value[x]", 1139 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1140 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1141 case 111972721: 1142 /* value */ return new Property("value[x]", 1143 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1144 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1145 case 733421943: 1146 /* valueBoolean */ return new Property("value[x]", 1147 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1148 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1149 case -2083993440: 1150 /* valueDecimal */ return new Property("value[x]", 1151 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1152 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1153 case -1668204915: 1154 /* valueInteger */ return new Property("value[x]", 1155 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1156 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1157 case -766192449: 1158 /* valueDate */ return new Property("value[x]", 1159 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1160 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1161 case 1047929900: 1162 /* valueDateTime */ return new Property("value[x]", 1163 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1164 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1165 case -765708322: 1166 /* valueTime */ return new Property("value[x]", 1167 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1168 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1169 case -1424603934: 1170 /* valueString */ return new Property("value[x]", 1171 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1172 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1173 case -1410172357: 1174 /* valueUri */ return new Property("value[x]", 1175 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1176 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1177 case -475566732: 1178 /* valueAttachment */ return new Property("value[x]", 1179 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1180 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1181 case -1887705029: 1182 /* valueCoding */ return new Property("value[x]", 1183 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1184 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1185 case -2029823716: 1186 /* valueQuantity */ return new Property("value[x]", 1187 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1188 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1189 case 1755241690: 1190 /* valueReference */ return new Property("value[x]", 1191 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1192 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1193 case 3242771: 1194 /* item */ return new Property("item", "@QuestionnaireResponse.item", 1195 "Nested groups and/or questions found within this particular answer.", 0, java.lang.Integer.MAX_VALUE, 1196 item); 1197 default: 1198 return super.getNamedProperty(_hash, _name, _checkValid); 1199 } 1200 1201 } 1202 1203 @Override 1204 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1205 switch (hash) { 1206 case 111972721: 1207 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 1208 case 3242771: 1209 /* item */ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // QuestionnaireResponseItemComponent 1210 default: 1211 return super.getProperty(hash, name, checkValid); 1212 } 1213 1214 } 1215 1216 @Override 1217 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1218 switch (hash) { 1219 case 111972721: // value 1220 this.value = castToType(value); // Type 1221 return value; 1222 case 3242771: // item 1223 this.getItem().add((QuestionnaireResponseItemComponent) value); // QuestionnaireResponseItemComponent 1224 return value; 1225 default: 1226 return super.setProperty(hash, name, value); 1227 } 1228 1229 } 1230 1231 @Override 1232 public Base setProperty(String name, Base value) throws FHIRException { 1233 if (name.equals("value[x]")) { 1234 this.value = castToType(value); // Type 1235 } else if (name.equals("item")) { 1236 this.getItem().add((QuestionnaireResponseItemComponent) value); 1237 } else 1238 return super.setProperty(name, value); 1239 return value; 1240 } 1241 1242 @Override 1243 public void removeChild(String name, Base value) throws FHIRException { 1244 if (name.equals("value[x]")) { 1245 this.value = null; 1246 } else if (name.equals("item")) { 1247 this.getItem().remove((QuestionnaireResponseItemComponent) value); 1248 } else 1249 super.removeChild(name, value); 1250 1251 } 1252 1253 @Override 1254 public Base makeProperty(int hash, String name) throws FHIRException { 1255 switch (hash) { 1256 case -1410166417: 1257 return getValue(); 1258 case 111972721: 1259 return getValue(); 1260 case 3242771: 1261 return addItem(); 1262 default: 1263 return super.makeProperty(hash, name); 1264 } 1265 1266 } 1267 1268 @Override 1269 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1270 switch (hash) { 1271 case 111972721: 1272 /* value */ return new String[] { "boolean", "decimal", "integer", "date", "dateTime", "time", "string", "uri", 1273 "Attachment", "Coding", "Quantity", "Reference" }; 1274 case 3242771: 1275 /* item */ return new String[] { "@QuestionnaireResponse.item" }; 1276 default: 1277 return super.getTypesForProperty(hash, name); 1278 } 1279 1280 } 1281 1282 @Override 1283 public Base addChild(String name) throws FHIRException { 1284 if (name.equals("valueBoolean")) { 1285 this.value = new BooleanType(); 1286 return this.value; 1287 } else if (name.equals("valueDecimal")) { 1288 this.value = new DecimalType(); 1289 return this.value; 1290 } else if (name.equals("valueInteger")) { 1291 this.value = new IntegerType(); 1292 return this.value; 1293 } else if (name.equals("valueDate")) { 1294 this.value = new DateType(); 1295 return this.value; 1296 } else if (name.equals("valueDateTime")) { 1297 this.value = new DateTimeType(); 1298 return this.value; 1299 } else if (name.equals("valueTime")) { 1300 this.value = new TimeType(); 1301 return this.value; 1302 } else if (name.equals("valueString")) { 1303 this.value = new StringType(); 1304 return this.value; 1305 } else if (name.equals("valueUri")) { 1306 this.value = new UriType(); 1307 return this.value; 1308 } else if (name.equals("valueAttachment")) { 1309 this.value = new Attachment(); 1310 return this.value; 1311 } else if (name.equals("valueCoding")) { 1312 this.value = new Coding(); 1313 return this.value; 1314 } else if (name.equals("valueQuantity")) { 1315 this.value = new Quantity(); 1316 return this.value; 1317 } else if (name.equals("valueReference")) { 1318 this.value = new Reference(); 1319 return this.value; 1320 } else if (name.equals("item")) { 1321 return addItem(); 1322 } else 1323 return super.addChild(name); 1324 } 1325 1326 public QuestionnaireResponseItemAnswerComponent copy() { 1327 QuestionnaireResponseItemAnswerComponent dst = new QuestionnaireResponseItemAnswerComponent(); 1328 copyValues(dst); 1329 return dst; 1330 } 1331 1332 public void copyValues(QuestionnaireResponseItemAnswerComponent dst) { 1333 super.copyValues(dst); 1334 dst.value = value == null ? null : value.copy(); 1335 if (item != null) { 1336 dst.item = new ArrayList<QuestionnaireResponseItemComponent>(); 1337 for (QuestionnaireResponseItemComponent i : item) 1338 dst.item.add(i.copy()); 1339 } 1340 ; 1341 } 1342 1343 @Override 1344 public boolean equalsDeep(Base other_) { 1345 if (!super.equalsDeep(other_)) 1346 return false; 1347 if (!(other_ instanceof QuestionnaireResponseItemAnswerComponent)) 1348 return false; 1349 QuestionnaireResponseItemAnswerComponent o = (QuestionnaireResponseItemAnswerComponent) other_; 1350 return compareDeep(value, o.value, true) && compareDeep(item, o.item, true); 1351 } 1352 1353 @Override 1354 public boolean equalsShallow(Base other_) { 1355 if (!super.equalsShallow(other_)) 1356 return false; 1357 if (!(other_ instanceof QuestionnaireResponseItemAnswerComponent)) 1358 return false; 1359 QuestionnaireResponseItemAnswerComponent o = (QuestionnaireResponseItemAnswerComponent) other_; 1360 return true; 1361 } 1362 1363 public boolean isEmpty() { 1364 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value, item); 1365 } 1366 1367 public String fhirType() { 1368 return "QuestionnaireResponse.item.answer"; 1369 1370 } 1371 1372 } 1373 1374 /** 1375 * A business identifier assigned to a particular completed (or partially 1376 * completed) questionnaire. 1377 */ 1378 @Child(name = "identifier", type = { 1379 Identifier.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 1380 @Description(shortDefinition = "Unique id for this set of answers", formalDefinition = "A business identifier assigned to a particular completed (or partially completed) questionnaire.") 1381 protected Identifier identifier; 1382 1383 /** 1384 * The order, proposal or plan that is fulfilled in whole or in part by this 1385 * QuestionnaireResponse. For example, a ServiceRequest seeking an intake 1386 * assessment or a decision support recommendation to assess for post-partum 1387 * depression. 1388 */ 1389 @Child(name = "basedOn", type = { CarePlan.class, 1390 ServiceRequest.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1391 @Description(shortDefinition = "Request fulfilled by this QuestionnaireResponse", formalDefinition = "The order, proposal or plan that is fulfilled in whole or in part by this QuestionnaireResponse. For example, a ServiceRequest seeking an intake assessment or a decision support recommendation to assess for post-partum depression.") 1392 protected List<Reference> basedOn; 1393 /** 1394 * The actual objects that are the target of the reference (The order, proposal 1395 * or plan that is fulfilled in whole or in part by this QuestionnaireResponse. 1396 * For example, a ServiceRequest seeking an intake assessment or a decision 1397 * support recommendation to assess for post-partum depression.) 1398 */ 1399 protected List<Resource> basedOnTarget; 1400 1401 /** 1402 * A procedure or observation that this questionnaire was performed as part of 1403 * the execution of. For example, the surgery a checklist was executed as part 1404 * of. 1405 */ 1406 @Child(name = "partOf", type = { Observation.class, 1407 Procedure.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1408 @Description(shortDefinition = "Part of this action", formalDefinition = "A procedure or observation that this questionnaire was performed as part of the execution of. For example, the surgery a checklist was executed as part of.") 1409 protected List<Reference> partOf; 1410 /** 1411 * The actual objects that are the target of the reference (A procedure or 1412 * observation that this questionnaire was performed as part of the execution 1413 * of. For example, the surgery a checklist was executed as part of.) 1414 */ 1415 protected List<Resource> partOfTarget; 1416 1417 /** 1418 * The Questionnaire that defines and organizes the questions for which answers 1419 * are being provided. 1420 */ 1421 @Child(name = "questionnaire", type = { 1422 CanonicalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1423 @Description(shortDefinition = "Form being answered", formalDefinition = "The Questionnaire that defines and organizes the questions for which answers are being provided.") 1424 protected CanonicalType questionnaire; 1425 1426 /** 1427 * The position of the questionnaire response within its overall lifecycle. 1428 */ 1429 @Child(name = "status", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = true, summary = true) 1430 @Description(shortDefinition = "in-progress | completed | amended | entered-in-error | stopped", formalDefinition = "The position of the questionnaire response within its overall lifecycle.") 1431 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/questionnaire-answers-status") 1432 protected Enumeration<QuestionnaireResponseStatus> status; 1433 1434 /** 1435 * The subject of the questionnaire response. This could be a patient, 1436 * organization, practitioner, device, etc. This is who/what the answers apply 1437 * to, but is not necessarily the source of information. 1438 */ 1439 @Child(name = "subject", type = { Reference.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1440 @Description(shortDefinition = "The subject of the questions", formalDefinition = "The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information.") 1441 protected Reference subject; 1442 1443 /** 1444 * The actual object that is the target of the reference (The subject of the 1445 * questionnaire response. This could be a patient, organization, practitioner, 1446 * device, etc. This is who/what the answers apply to, but is not necessarily 1447 * the source of information.) 1448 */ 1449 protected Resource subjectTarget; 1450 1451 /** 1452 * The Encounter during which this questionnaire response was created or to 1453 * which the creation of this record is tightly associated. 1454 */ 1455 @Child(name = "encounter", type = { Encounter.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1456 @Description(shortDefinition = "Encounter created as part of", formalDefinition = "The Encounter during which this questionnaire response was created or to which the creation of this record is tightly associated.") 1457 protected Reference encounter; 1458 1459 /** 1460 * The actual object that is the target of the reference (The Encounter during 1461 * which this questionnaire response was created or to which the creation of 1462 * this record is tightly associated.) 1463 */ 1464 protected Encounter encounterTarget; 1465 1466 /** 1467 * The date and/or time that this set of answers were last changed. 1468 */ 1469 @Child(name = "authored", type = { 1470 DateTimeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1471 @Description(shortDefinition = "Date the answers were gathered", formalDefinition = "The date and/or time that this set of answers were last changed.") 1472 protected DateTimeType authored; 1473 1474 /** 1475 * Person who received the answers to the questions in the QuestionnaireResponse 1476 * and recorded them in the system. 1477 */ 1478 @Child(name = "author", type = { Device.class, Practitioner.class, PractitionerRole.class, Patient.class, 1479 RelatedPerson.class, Organization.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1480 @Description(shortDefinition = "Person who received and recorded the answers", formalDefinition = "Person who received the answers to the questions in the QuestionnaireResponse and recorded them in the system.") 1481 protected Reference author; 1482 1483 /** 1484 * The actual object that is the target of the reference (Person who received 1485 * the answers to the questions in the QuestionnaireResponse and recorded them 1486 * in the system.) 1487 */ 1488 protected Resource authorTarget; 1489 1490 /** 1491 * The person who answered the questions about the subject. 1492 */ 1493 @Child(name = "source", type = { Patient.class, Practitioner.class, PractitionerRole.class, 1494 RelatedPerson.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 1495 @Description(shortDefinition = "The person who answered the questions", formalDefinition = "The person who answered the questions about the subject.") 1496 protected Reference source; 1497 1498 /** 1499 * The actual object that is the target of the reference (The person who 1500 * answered the questions about the subject.) 1501 */ 1502 protected Resource sourceTarget; 1503 1504 /** 1505 * A group or question item from the original questionnaire for which answers 1506 * are provided. 1507 */ 1508 @Child(name = "item", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1509 @Description(shortDefinition = "Groups and questions", formalDefinition = "A group or question item from the original questionnaire for which answers are provided.") 1510 protected List<QuestionnaireResponseItemComponent> item; 1511 1512 private static final long serialVersionUID = -259908687L; 1513 1514 /** 1515 * Constructor 1516 */ 1517 public QuestionnaireResponse() { 1518 super(); 1519 } 1520 1521 /** 1522 * Constructor 1523 */ 1524 public QuestionnaireResponse(Enumeration<QuestionnaireResponseStatus> status) { 1525 super(); 1526 this.status = status; 1527 } 1528 1529 /** 1530 * @return {@link #identifier} (A business identifier assigned to a particular 1531 * completed (or partially completed) questionnaire.) 1532 */ 1533 public Identifier getIdentifier() { 1534 if (this.identifier == null) 1535 if (Configuration.errorOnAutoCreate()) 1536 throw new Error("Attempt to auto-create QuestionnaireResponse.identifier"); 1537 else if (Configuration.doAutoCreate()) 1538 this.identifier = new Identifier(); // cc 1539 return this.identifier; 1540 } 1541 1542 public boolean hasIdentifier() { 1543 return this.identifier != null && !this.identifier.isEmpty(); 1544 } 1545 1546 /** 1547 * @param value {@link #identifier} (A business identifier assigned to a 1548 * particular completed (or partially completed) questionnaire.) 1549 */ 1550 public QuestionnaireResponse setIdentifier(Identifier value) { 1551 this.identifier = value; 1552 return this; 1553 } 1554 1555 /** 1556 * @return {@link #basedOn} (The order, proposal or plan that is fulfilled in 1557 * whole or in part by this QuestionnaireResponse. For example, a 1558 * ServiceRequest seeking an intake assessment or a decision support 1559 * recommendation to assess for post-partum depression.) 1560 */ 1561 public List<Reference> getBasedOn() { 1562 if (this.basedOn == null) 1563 this.basedOn = new ArrayList<Reference>(); 1564 return this.basedOn; 1565 } 1566 1567 /** 1568 * @return Returns a reference to <code>this</code> for easy method chaining 1569 */ 1570 public QuestionnaireResponse setBasedOn(List<Reference> theBasedOn) { 1571 this.basedOn = theBasedOn; 1572 return this; 1573 } 1574 1575 public boolean hasBasedOn() { 1576 if (this.basedOn == null) 1577 return false; 1578 for (Reference item : this.basedOn) 1579 if (!item.isEmpty()) 1580 return true; 1581 return false; 1582 } 1583 1584 public Reference addBasedOn() { // 3 1585 Reference t = new Reference(); 1586 if (this.basedOn == null) 1587 this.basedOn = new ArrayList<Reference>(); 1588 this.basedOn.add(t); 1589 return t; 1590 } 1591 1592 public QuestionnaireResponse addBasedOn(Reference t) { // 3 1593 if (t == null) 1594 return this; 1595 if (this.basedOn == null) 1596 this.basedOn = new ArrayList<Reference>(); 1597 this.basedOn.add(t); 1598 return this; 1599 } 1600 1601 /** 1602 * @return The first repetition of repeating field {@link #basedOn}, creating it 1603 * if it does not already exist 1604 */ 1605 public Reference getBasedOnFirstRep() { 1606 if (getBasedOn().isEmpty()) { 1607 addBasedOn(); 1608 } 1609 return getBasedOn().get(0); 1610 } 1611 1612 /** 1613 * @return {@link #partOf} (A procedure or observation that this questionnaire 1614 * was performed as part of the execution of. For example, the surgery a 1615 * checklist was executed as part of.) 1616 */ 1617 public List<Reference> getPartOf() { 1618 if (this.partOf == null) 1619 this.partOf = new ArrayList<Reference>(); 1620 return this.partOf; 1621 } 1622 1623 /** 1624 * @return Returns a reference to <code>this</code> for easy method chaining 1625 */ 1626 public QuestionnaireResponse setPartOf(List<Reference> thePartOf) { 1627 this.partOf = thePartOf; 1628 return this; 1629 } 1630 1631 public boolean hasPartOf() { 1632 if (this.partOf == null) 1633 return false; 1634 for (Reference item : this.partOf) 1635 if (!item.isEmpty()) 1636 return true; 1637 return false; 1638 } 1639 1640 public Reference addPartOf() { // 3 1641 Reference t = new Reference(); 1642 if (this.partOf == null) 1643 this.partOf = new ArrayList<Reference>(); 1644 this.partOf.add(t); 1645 return t; 1646 } 1647 1648 public QuestionnaireResponse addPartOf(Reference t) { // 3 1649 if (t == null) 1650 return this; 1651 if (this.partOf == null) 1652 this.partOf = new ArrayList<Reference>(); 1653 this.partOf.add(t); 1654 return this; 1655 } 1656 1657 /** 1658 * @return The first repetition of repeating field {@link #partOf}, creating it 1659 * if it does not already exist 1660 */ 1661 public Reference getPartOfFirstRep() { 1662 if (getPartOf().isEmpty()) { 1663 addPartOf(); 1664 } 1665 return getPartOf().get(0); 1666 } 1667 1668 /** 1669 * @return {@link #questionnaire} (The Questionnaire that defines and organizes 1670 * the questions for which answers are being provided.). This is the 1671 * underlying object with id, value and extensions. The accessor 1672 * "getQuestionnaire" gives direct access to the value 1673 */ 1674 public CanonicalType getQuestionnaireElement() { 1675 if (this.questionnaire == null) 1676 if (Configuration.errorOnAutoCreate()) 1677 throw new Error("Attempt to auto-create QuestionnaireResponse.questionnaire"); 1678 else if (Configuration.doAutoCreate()) 1679 this.questionnaire = new CanonicalType(); // bb 1680 return this.questionnaire; 1681 } 1682 1683 public boolean hasQuestionnaireElement() { 1684 return this.questionnaire != null && !this.questionnaire.isEmpty(); 1685 } 1686 1687 public boolean hasQuestionnaire() { 1688 return this.questionnaire != null && !this.questionnaire.isEmpty(); 1689 } 1690 1691 /** 1692 * @param value {@link #questionnaire} (The Questionnaire that defines and 1693 * organizes the questions for which answers are being provided.). 1694 * This is the underlying object with id, value and extensions. The 1695 * accessor "getQuestionnaire" gives direct access to the value 1696 */ 1697 public QuestionnaireResponse setQuestionnaireElement(CanonicalType value) { 1698 this.questionnaire = value; 1699 return this; 1700 } 1701 1702 /** 1703 * @return The Questionnaire that defines and organizes the questions for which 1704 * answers are being provided. 1705 */ 1706 public String getQuestionnaire() { 1707 return this.questionnaire == null ? null : this.questionnaire.getValue(); 1708 } 1709 1710 /** 1711 * @param value The Questionnaire that defines and organizes the questions for 1712 * which answers are being provided. 1713 */ 1714 public QuestionnaireResponse setQuestionnaire(String value) { 1715 if (Utilities.noString(value)) 1716 this.questionnaire = null; 1717 else { 1718 if (this.questionnaire == null) 1719 this.questionnaire = new CanonicalType(); 1720 this.questionnaire.setValue(value); 1721 } 1722 return this; 1723 } 1724 1725 /** 1726 * @return {@link #status} (The position of the questionnaire response within 1727 * its overall lifecycle.). This is the underlying object with id, value 1728 * and extensions. The accessor "getStatus" gives direct access to the 1729 * value 1730 */ 1731 public Enumeration<QuestionnaireResponseStatus> getStatusElement() { 1732 if (this.status == null) 1733 if (Configuration.errorOnAutoCreate()) 1734 throw new Error("Attempt to auto-create QuestionnaireResponse.status"); 1735 else if (Configuration.doAutoCreate()) 1736 this.status = new Enumeration<QuestionnaireResponseStatus>(new QuestionnaireResponseStatusEnumFactory()); // bb 1737 return this.status; 1738 } 1739 1740 public boolean hasStatusElement() { 1741 return this.status != null && !this.status.isEmpty(); 1742 } 1743 1744 public boolean hasStatus() { 1745 return this.status != null && !this.status.isEmpty(); 1746 } 1747 1748 /** 1749 * @param value {@link #status} (The position of the questionnaire response 1750 * within its overall lifecycle.). This is the underlying object 1751 * with id, value and extensions. The accessor "getStatus" gives 1752 * direct access to the value 1753 */ 1754 public QuestionnaireResponse setStatusElement(Enumeration<QuestionnaireResponseStatus> value) { 1755 this.status = value; 1756 return this; 1757 } 1758 1759 /** 1760 * @return The position of the questionnaire response within its overall 1761 * lifecycle. 1762 */ 1763 public QuestionnaireResponseStatus getStatus() { 1764 return this.status == null ? null : this.status.getValue(); 1765 } 1766 1767 /** 1768 * @param value The position of the questionnaire response within its overall 1769 * lifecycle. 1770 */ 1771 public QuestionnaireResponse setStatus(QuestionnaireResponseStatus value) { 1772 if (this.status == null) 1773 this.status = new Enumeration<QuestionnaireResponseStatus>(new QuestionnaireResponseStatusEnumFactory()); 1774 this.status.setValue(value); 1775 return this; 1776 } 1777 1778 /** 1779 * @return {@link #subject} (The subject of the questionnaire response. This 1780 * could be a patient, organization, practitioner, device, etc. This is 1781 * who/what the answers apply to, but is not necessarily the source of 1782 * information.) 1783 */ 1784 public Reference getSubject() { 1785 if (this.subject == null) 1786 if (Configuration.errorOnAutoCreate()) 1787 throw new Error("Attempt to auto-create QuestionnaireResponse.subject"); 1788 else if (Configuration.doAutoCreate()) 1789 this.subject = new Reference(); // cc 1790 return this.subject; 1791 } 1792 1793 public boolean hasSubject() { 1794 return this.subject != null && !this.subject.isEmpty(); 1795 } 1796 1797 /** 1798 * @param value {@link #subject} (The subject of the questionnaire response. 1799 * This could be a patient, organization, practitioner, device, 1800 * etc. This is who/what the answers apply to, but is not 1801 * necessarily the source of information.) 1802 */ 1803 public QuestionnaireResponse setSubject(Reference value) { 1804 this.subject = value; 1805 return this; 1806 } 1807 1808 /** 1809 * @return {@link #subject} The actual object that is the target of the 1810 * reference. The reference library doesn't populate this, but you can 1811 * use it to hold the resource if you resolve it. (The subject of the 1812 * questionnaire response. This could be a patient, organization, 1813 * practitioner, device, etc. This is who/what the answers apply to, but 1814 * is not necessarily the source of information.) 1815 */ 1816 public Resource getSubjectTarget() { 1817 return this.subjectTarget; 1818 } 1819 1820 /** 1821 * @param value {@link #subject} The actual object that is the target of the 1822 * reference. The reference library doesn't use these, but you can 1823 * use it to hold the resource if you resolve it. (The subject of 1824 * the questionnaire response. This could be a patient, 1825 * organization, practitioner, device, etc. This is who/what the 1826 * answers apply to, but is not necessarily the source of 1827 * information.) 1828 */ 1829 public QuestionnaireResponse setSubjectTarget(Resource value) { 1830 this.subjectTarget = value; 1831 return this; 1832 } 1833 1834 /** 1835 * @return {@link #encounter} (The Encounter during which this questionnaire 1836 * response was created or to which the creation of this record is 1837 * tightly associated.) 1838 */ 1839 public Reference getEncounter() { 1840 if (this.encounter == null) 1841 if (Configuration.errorOnAutoCreate()) 1842 throw new Error("Attempt to auto-create QuestionnaireResponse.encounter"); 1843 else if (Configuration.doAutoCreate()) 1844 this.encounter = new Reference(); // cc 1845 return this.encounter; 1846 } 1847 1848 public boolean hasEncounter() { 1849 return this.encounter != null && !this.encounter.isEmpty(); 1850 } 1851 1852 /** 1853 * @param value {@link #encounter} (The Encounter during which this 1854 * questionnaire response was created or to which the creation of 1855 * this record is tightly associated.) 1856 */ 1857 public QuestionnaireResponse setEncounter(Reference value) { 1858 this.encounter = value; 1859 return this; 1860 } 1861 1862 /** 1863 * @return {@link #encounter} The actual object that is the target of the 1864 * reference. The reference library doesn't populate this, but you can 1865 * use it to hold the resource if you resolve it. (The Encounter during 1866 * which this questionnaire response was created or to which the 1867 * creation of this record is tightly associated.) 1868 */ 1869 public Encounter getEncounterTarget() { 1870 if (this.encounterTarget == null) 1871 if (Configuration.errorOnAutoCreate()) 1872 throw new Error("Attempt to auto-create QuestionnaireResponse.encounter"); 1873 else if (Configuration.doAutoCreate()) 1874 this.encounterTarget = new Encounter(); // aa 1875 return this.encounterTarget; 1876 } 1877 1878 /** 1879 * @param value {@link #encounter} The actual object that is the target of the 1880 * reference. The reference library doesn't use these, but you can 1881 * use it to hold the resource if you resolve it. (The Encounter 1882 * during which this questionnaire response was created or to which 1883 * the creation of this record is tightly associated.) 1884 */ 1885 public QuestionnaireResponse setEncounterTarget(Encounter value) { 1886 this.encounterTarget = value; 1887 return this; 1888 } 1889 1890 /** 1891 * @return {@link #authored} (The date and/or time that this set of answers were 1892 * last changed.). This is the underlying object with id, value and 1893 * extensions. The accessor "getAuthored" gives direct access to the 1894 * value 1895 */ 1896 public DateTimeType getAuthoredElement() { 1897 if (this.authored == null) 1898 if (Configuration.errorOnAutoCreate()) 1899 throw new Error("Attempt to auto-create QuestionnaireResponse.authored"); 1900 else if (Configuration.doAutoCreate()) 1901 this.authored = new DateTimeType(); // bb 1902 return this.authored; 1903 } 1904 1905 public boolean hasAuthoredElement() { 1906 return this.authored != null && !this.authored.isEmpty(); 1907 } 1908 1909 public boolean hasAuthored() { 1910 return this.authored != null && !this.authored.isEmpty(); 1911 } 1912 1913 /** 1914 * @param value {@link #authored} (The date and/or time that this set of answers 1915 * were last changed.). This is the underlying object with id, 1916 * value and extensions. The accessor "getAuthored" gives direct 1917 * access to the value 1918 */ 1919 public QuestionnaireResponse setAuthoredElement(DateTimeType value) { 1920 this.authored = value; 1921 return this; 1922 } 1923 1924 /** 1925 * @return The date and/or time that this set of answers were last changed. 1926 */ 1927 public Date getAuthored() { 1928 return this.authored == null ? null : this.authored.getValue(); 1929 } 1930 1931 /** 1932 * @param value The date and/or time that this set of answers were last changed. 1933 */ 1934 public QuestionnaireResponse setAuthored(Date value) { 1935 if (value == null) 1936 this.authored = null; 1937 else { 1938 if (this.authored == null) 1939 this.authored = new DateTimeType(); 1940 this.authored.setValue(value); 1941 } 1942 return this; 1943 } 1944 1945 /** 1946 * @return {@link #author} (Person who received the answers to the questions in 1947 * the QuestionnaireResponse and recorded them in the system.) 1948 */ 1949 public Reference getAuthor() { 1950 if (this.author == null) 1951 if (Configuration.errorOnAutoCreate()) 1952 throw new Error("Attempt to auto-create QuestionnaireResponse.author"); 1953 else if (Configuration.doAutoCreate()) 1954 this.author = new Reference(); // cc 1955 return this.author; 1956 } 1957 1958 public boolean hasAuthor() { 1959 return this.author != null && !this.author.isEmpty(); 1960 } 1961 1962 /** 1963 * @param value {@link #author} (Person who received the answers to the 1964 * questions in the QuestionnaireResponse and recorded them in the 1965 * system.) 1966 */ 1967 public QuestionnaireResponse setAuthor(Reference value) { 1968 this.author = value; 1969 return this; 1970 } 1971 1972 /** 1973 * @return {@link #author} The actual object that is the target of the 1974 * reference. The reference library doesn't populate this, but you can 1975 * use it to hold the resource if you resolve it. (Person who received 1976 * the answers to the questions in the QuestionnaireResponse and 1977 * recorded them in the system.) 1978 */ 1979 public Resource getAuthorTarget() { 1980 return this.authorTarget; 1981 } 1982 1983 /** 1984 * @param value {@link #author} The actual object that is the target of the 1985 * reference. The reference library doesn't use these, but you can 1986 * use it to hold the resource if you resolve it. (Person who 1987 * received the answers to the questions in the 1988 * QuestionnaireResponse and recorded them in the system.) 1989 */ 1990 public QuestionnaireResponse setAuthorTarget(Resource value) { 1991 this.authorTarget = value; 1992 return this; 1993 } 1994 1995 /** 1996 * @return {@link #source} (The person who answered the questions about the 1997 * subject.) 1998 */ 1999 public Reference getSource() { 2000 if (this.source == null) 2001 if (Configuration.errorOnAutoCreate()) 2002 throw new Error("Attempt to auto-create QuestionnaireResponse.source"); 2003 else if (Configuration.doAutoCreate()) 2004 this.source = new Reference(); // cc 2005 return this.source; 2006 } 2007 2008 public boolean hasSource() { 2009 return this.source != null && !this.source.isEmpty(); 2010 } 2011 2012 /** 2013 * @param value {@link #source} (The person who answered the questions about the 2014 * subject.) 2015 */ 2016 public QuestionnaireResponse setSource(Reference value) { 2017 this.source = value; 2018 return this; 2019 } 2020 2021 /** 2022 * @return {@link #source} The actual object that is the target of the 2023 * reference. The reference library doesn't populate this, but you can 2024 * use it to hold the resource if you resolve it. (The person who 2025 * answered the questions about the subject.) 2026 */ 2027 public Resource getSourceTarget() { 2028 return this.sourceTarget; 2029 } 2030 2031 /** 2032 * @param value {@link #source} The actual object that is the target of the 2033 * reference. The reference library doesn't use these, but you can 2034 * use it to hold the resource if you resolve it. (The person who 2035 * answered the questions about the subject.) 2036 */ 2037 public QuestionnaireResponse setSourceTarget(Resource value) { 2038 this.sourceTarget = value; 2039 return this; 2040 } 2041 2042 /** 2043 * @return {@link #item} (A group or question item from the original 2044 * questionnaire for which answers are provided.) 2045 */ 2046 public List<QuestionnaireResponseItemComponent> getItem() { 2047 if (this.item == null) 2048 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 2049 return this.item; 2050 } 2051 2052 /** 2053 * @return Returns a reference to <code>this</code> for easy method chaining 2054 */ 2055 public QuestionnaireResponse setItem(List<QuestionnaireResponseItemComponent> theItem) { 2056 this.item = theItem; 2057 return this; 2058 } 2059 2060 public boolean hasItem() { 2061 if (this.item == null) 2062 return false; 2063 for (QuestionnaireResponseItemComponent item : this.item) 2064 if (!item.isEmpty()) 2065 return true; 2066 return false; 2067 } 2068 2069 public QuestionnaireResponseItemComponent addItem() { // 3 2070 QuestionnaireResponseItemComponent t = new QuestionnaireResponseItemComponent(); 2071 if (this.item == null) 2072 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 2073 this.item.add(t); 2074 return t; 2075 } 2076 2077 public QuestionnaireResponse addItem(QuestionnaireResponseItemComponent t) { // 3 2078 if (t == null) 2079 return this; 2080 if (this.item == null) 2081 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 2082 this.item.add(t); 2083 return this; 2084 } 2085 2086 /** 2087 * @return The first repetition of repeating field {@link #item}, creating it if 2088 * it does not already exist 2089 */ 2090 public QuestionnaireResponseItemComponent getItemFirstRep() { 2091 if (getItem().isEmpty()) { 2092 addItem(); 2093 } 2094 return getItem().get(0); 2095 } 2096 2097 protected void listChildren(List<Property> children) { 2098 super.listChildren(children); 2099 children.add(new Property("identifier", "Identifier", 2100 "A business identifier assigned to a particular completed (or partially completed) questionnaire.", 0, 1, 2101 identifier)); 2102 children.add(new Property("basedOn", "Reference(CarePlan|ServiceRequest)", 2103 "The order, proposal or plan that is fulfilled in whole or in part by this QuestionnaireResponse. For example, a ServiceRequest seeking an intake assessment or a decision support recommendation to assess for post-partum depression.", 2104 0, java.lang.Integer.MAX_VALUE, basedOn)); 2105 children.add(new Property("partOf", "Reference(Observation|Procedure)", 2106 "A procedure or observation that this questionnaire was performed as part of the execution of. For example, the surgery a checklist was executed as part of.", 2107 0, java.lang.Integer.MAX_VALUE, partOf)); 2108 children.add(new Property("questionnaire", "canonical(Questionnaire)", 2109 "The Questionnaire that defines and organizes the questions for which answers are being provided.", 0, 1, 2110 questionnaire)); 2111 children.add(new Property("status", "code", 2112 "The position of the questionnaire response within its overall lifecycle.", 0, 1, status)); 2113 children.add(new Property("subject", "Reference(Any)", 2114 "The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information.", 2115 0, 1, subject)); 2116 children.add(new Property("encounter", "Reference(Encounter)", 2117 "The Encounter during which this questionnaire response was created or to which the creation of this record is tightly associated.", 2118 0, 1, encounter)); 2119 children.add(new Property("authored", "dateTime", 2120 "The date and/or time that this set of answers were last changed.", 0, 1, authored)); 2121 children.add(new Property("author", 2122 "Reference(Device|Practitioner|PractitionerRole|Patient|RelatedPerson|Organization)", 2123 "Person who received the answers to the questions in the QuestionnaireResponse and recorded them in the system.", 2124 0, 1, author)); 2125 children.add(new Property("source", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson)", 2126 "The person who answered the questions about the subject.", 0, 1, source)); 2127 children.add(new Property("item", "", 2128 "A group or question item from the original questionnaire for which answers are provided.", 0, 2129 java.lang.Integer.MAX_VALUE, item)); 2130 } 2131 2132 @Override 2133 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2134 switch (_hash) { 2135 case -1618432855: 2136 /* identifier */ return new Property("identifier", "Identifier", 2137 "A business identifier assigned to a particular completed (or partially completed) questionnaire.", 0, 1, 2138 identifier); 2139 case -332612366: 2140 /* basedOn */ return new Property("basedOn", "Reference(CarePlan|ServiceRequest)", 2141 "The order, proposal or plan that is fulfilled in whole or in part by this QuestionnaireResponse. For example, a ServiceRequest seeking an intake assessment or a decision support recommendation to assess for post-partum depression.", 2142 0, java.lang.Integer.MAX_VALUE, basedOn); 2143 case -995410646: 2144 /* partOf */ return new Property("partOf", "Reference(Observation|Procedure)", 2145 "A procedure or observation that this questionnaire was performed as part of the execution of. For example, the surgery a checklist was executed as part of.", 2146 0, java.lang.Integer.MAX_VALUE, partOf); 2147 case -1017049693: 2148 /* questionnaire */ return new Property("questionnaire", "canonical(Questionnaire)", 2149 "The Questionnaire that defines and organizes the questions for which answers are being provided.", 0, 1, 2150 questionnaire); 2151 case -892481550: 2152 /* status */ return new Property("status", "code", 2153 "The position of the questionnaire response within its overall lifecycle.", 0, 1, status); 2154 case -1867885268: 2155 /* subject */ return new Property("subject", "Reference(Any)", 2156 "The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information.", 2157 0, 1, subject); 2158 case 1524132147: 2159 /* encounter */ return new Property("encounter", "Reference(Encounter)", 2160 "The Encounter during which this questionnaire response was created or to which the creation of this record is tightly associated.", 2161 0, 1, encounter); 2162 case 1433073514: 2163 /* authored */ return new Property("authored", "dateTime", 2164 "The date and/or time that this set of answers were last changed.", 0, 1, authored); 2165 case -1406328437: 2166 /* author */ return new Property("author", 2167 "Reference(Device|Practitioner|PractitionerRole|Patient|RelatedPerson|Organization)", 2168 "Person who received the answers to the questions in the QuestionnaireResponse and recorded them in the system.", 2169 0, 1, author); 2170 case -896505829: 2171 /* source */ return new Property("source", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson)", 2172 "The person who answered the questions about the subject.", 0, 1, source); 2173 case 3242771: 2174 /* item */ return new Property("item", "", 2175 "A group or question item from the original questionnaire for which answers are provided.", 0, 2176 java.lang.Integer.MAX_VALUE, item); 2177 default: 2178 return super.getNamedProperty(_hash, _name, _checkValid); 2179 } 2180 2181 } 2182 2183 @Override 2184 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2185 switch (hash) { 2186 case -1618432855: 2187 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 2188 case -332612366: 2189 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 2190 case -995410646: 2191 /* partOf */ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 2192 case -1017049693: 2193 /* questionnaire */ return this.questionnaire == null ? new Base[0] : new Base[] { this.questionnaire }; // CanonicalType 2194 case -892481550: 2195 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<QuestionnaireResponseStatus> 2196 case -1867885268: 2197 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 2198 case 1524132147: 2199 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 2200 case 1433073514: 2201 /* authored */ return this.authored == null ? new Base[0] : new Base[] { this.authored }; // DateTimeType 2202 case -1406328437: 2203 /* author */ return this.author == null ? new Base[0] : new Base[] { this.author }; // Reference 2204 case -896505829: 2205 /* source */ return this.source == null ? new Base[0] : new Base[] { this.source }; // Reference 2206 case 3242771: 2207 /* item */ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // QuestionnaireResponseItemComponent 2208 default: 2209 return super.getProperty(hash, name, checkValid); 2210 } 2211 2212 } 2213 2214 @Override 2215 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2216 switch (hash) { 2217 case -1618432855: // identifier 2218 this.identifier = castToIdentifier(value); // Identifier 2219 return value; 2220 case -332612366: // basedOn 2221 this.getBasedOn().add(castToReference(value)); // Reference 2222 return value; 2223 case -995410646: // partOf 2224 this.getPartOf().add(castToReference(value)); // Reference 2225 return value; 2226 case -1017049693: // questionnaire 2227 this.questionnaire = castToCanonical(value); // CanonicalType 2228 return value; 2229 case -892481550: // status 2230 value = new QuestionnaireResponseStatusEnumFactory().fromType(castToCode(value)); 2231 this.status = (Enumeration) value; // Enumeration<QuestionnaireResponseStatus> 2232 return value; 2233 case -1867885268: // subject 2234 this.subject = castToReference(value); // Reference 2235 return value; 2236 case 1524132147: // encounter 2237 this.encounter = castToReference(value); // Reference 2238 return value; 2239 case 1433073514: // authored 2240 this.authored = castToDateTime(value); // DateTimeType 2241 return value; 2242 case -1406328437: // author 2243 this.author = castToReference(value); // Reference 2244 return value; 2245 case -896505829: // source 2246 this.source = castToReference(value); // Reference 2247 return value; 2248 case 3242771: // item 2249 this.getItem().add((QuestionnaireResponseItemComponent) value); // QuestionnaireResponseItemComponent 2250 return value; 2251 default: 2252 return super.setProperty(hash, name, value); 2253 } 2254 2255 } 2256 2257 @Override 2258 public Base setProperty(String name, Base value) throws FHIRException { 2259 if (name.equals("identifier")) { 2260 this.identifier = castToIdentifier(value); // Identifier 2261 } else if (name.equals("basedOn")) { 2262 this.getBasedOn().add(castToReference(value)); 2263 } else if (name.equals("partOf")) { 2264 this.getPartOf().add(castToReference(value)); 2265 } else if (name.equals("questionnaire")) { 2266 this.questionnaire = castToCanonical(value); // CanonicalType 2267 } else if (name.equals("status")) { 2268 value = new QuestionnaireResponseStatusEnumFactory().fromType(castToCode(value)); 2269 this.status = (Enumeration) value; // Enumeration<QuestionnaireResponseStatus> 2270 } else if (name.equals("subject")) { 2271 this.subject = castToReference(value); // Reference 2272 } else if (name.equals("encounter")) { 2273 this.encounter = castToReference(value); // Reference 2274 } else if (name.equals("authored")) { 2275 this.authored = castToDateTime(value); // DateTimeType 2276 } else if (name.equals("author")) { 2277 this.author = castToReference(value); // Reference 2278 } else if (name.equals("source")) { 2279 this.source = castToReference(value); // Reference 2280 } else if (name.equals("item")) { 2281 this.getItem().add((QuestionnaireResponseItemComponent) value); 2282 } else 2283 return super.setProperty(name, value); 2284 return value; 2285 } 2286 2287 @Override 2288 public void removeChild(String name, Base value) throws FHIRException { 2289 if (name.equals("identifier")) { 2290 this.identifier = null; 2291 } else if (name.equals("basedOn")) { 2292 this.getBasedOn().remove(castToReference(value)); 2293 } else if (name.equals("partOf")) { 2294 this.getPartOf().remove(castToReference(value)); 2295 } else if (name.equals("questionnaire")) { 2296 this.questionnaire = null; 2297 } else if (name.equals("status")) { 2298 this.status = null; 2299 } else if (name.equals("subject")) { 2300 this.subject = null; 2301 } else if (name.equals("encounter")) { 2302 this.encounter = null; 2303 } else if (name.equals("authored")) { 2304 this.authored = null; 2305 } else if (name.equals("author")) { 2306 this.author = null; 2307 } else if (name.equals("source")) { 2308 this.source = null; 2309 } else if (name.equals("item")) { 2310 this.getItem().remove((QuestionnaireResponseItemComponent) value); 2311 } else 2312 super.removeChild(name, value); 2313 2314 } 2315 2316 @Override 2317 public Base makeProperty(int hash, String name) throws FHIRException { 2318 switch (hash) { 2319 case -1618432855: 2320 return getIdentifier(); 2321 case -332612366: 2322 return addBasedOn(); 2323 case -995410646: 2324 return addPartOf(); 2325 case -1017049693: 2326 return getQuestionnaireElement(); 2327 case -892481550: 2328 return getStatusElement(); 2329 case -1867885268: 2330 return getSubject(); 2331 case 1524132147: 2332 return getEncounter(); 2333 case 1433073514: 2334 return getAuthoredElement(); 2335 case -1406328437: 2336 return getAuthor(); 2337 case -896505829: 2338 return getSource(); 2339 case 3242771: 2340 return addItem(); 2341 default: 2342 return super.makeProperty(hash, name); 2343 } 2344 2345 } 2346 2347 @Override 2348 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2349 switch (hash) { 2350 case -1618432855: 2351 /* identifier */ return new String[] { "Identifier" }; 2352 case -332612366: 2353 /* basedOn */ return new String[] { "Reference" }; 2354 case -995410646: 2355 /* partOf */ return new String[] { "Reference" }; 2356 case -1017049693: 2357 /* questionnaire */ return new String[] { "canonical" }; 2358 case -892481550: 2359 /* status */ return new String[] { "code" }; 2360 case -1867885268: 2361 /* subject */ return new String[] { "Reference" }; 2362 case 1524132147: 2363 /* encounter */ return new String[] { "Reference" }; 2364 case 1433073514: 2365 /* authored */ return new String[] { "dateTime" }; 2366 case -1406328437: 2367 /* author */ return new String[] { "Reference" }; 2368 case -896505829: 2369 /* source */ return new String[] { "Reference" }; 2370 case 3242771: 2371 /* item */ return new String[] {}; 2372 default: 2373 return super.getTypesForProperty(hash, name); 2374 } 2375 2376 } 2377 2378 @Override 2379 public Base addChild(String name) throws FHIRException { 2380 if (name.equals("identifier")) { 2381 this.identifier = new Identifier(); 2382 return this.identifier; 2383 } else if (name.equals("basedOn")) { 2384 return addBasedOn(); 2385 } else if (name.equals("partOf")) { 2386 return addPartOf(); 2387 } else if (name.equals("questionnaire")) { 2388 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.questionnaire"); 2389 } else if (name.equals("status")) { 2390 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.status"); 2391 } else if (name.equals("subject")) { 2392 this.subject = new Reference(); 2393 return this.subject; 2394 } else if (name.equals("encounter")) { 2395 this.encounter = new Reference(); 2396 return this.encounter; 2397 } else if (name.equals("authored")) { 2398 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.authored"); 2399 } else if (name.equals("author")) { 2400 this.author = new Reference(); 2401 return this.author; 2402 } else if (name.equals("source")) { 2403 this.source = new Reference(); 2404 return this.source; 2405 } else if (name.equals("item")) { 2406 return addItem(); 2407 } else 2408 return super.addChild(name); 2409 } 2410 2411 public String fhirType() { 2412 return "QuestionnaireResponse"; 2413 2414 } 2415 2416 public QuestionnaireResponse copy() { 2417 QuestionnaireResponse dst = new QuestionnaireResponse(); 2418 copyValues(dst); 2419 return dst; 2420 } 2421 2422 public void copyValues(QuestionnaireResponse dst) { 2423 super.copyValues(dst); 2424 dst.identifier = identifier == null ? null : identifier.copy(); 2425 if (basedOn != null) { 2426 dst.basedOn = new ArrayList<Reference>(); 2427 for (Reference i : basedOn) 2428 dst.basedOn.add(i.copy()); 2429 } 2430 ; 2431 if (partOf != null) { 2432 dst.partOf = new ArrayList<Reference>(); 2433 for (Reference i : partOf) 2434 dst.partOf.add(i.copy()); 2435 } 2436 ; 2437 dst.questionnaire = questionnaire == null ? null : questionnaire.copy(); 2438 dst.status = status == null ? null : status.copy(); 2439 dst.subject = subject == null ? null : subject.copy(); 2440 dst.encounter = encounter == null ? null : encounter.copy(); 2441 dst.authored = authored == null ? null : authored.copy(); 2442 dst.author = author == null ? null : author.copy(); 2443 dst.source = source == null ? null : source.copy(); 2444 if (item != null) { 2445 dst.item = new ArrayList<QuestionnaireResponseItemComponent>(); 2446 for (QuestionnaireResponseItemComponent i : item) 2447 dst.item.add(i.copy()); 2448 } 2449 ; 2450 } 2451 2452 protected QuestionnaireResponse typedCopy() { 2453 return copy(); 2454 } 2455 2456 @Override 2457 public boolean equalsDeep(Base other_) { 2458 if (!super.equalsDeep(other_)) 2459 return false; 2460 if (!(other_ instanceof QuestionnaireResponse)) 2461 return false; 2462 QuestionnaireResponse o = (QuestionnaireResponse) other_; 2463 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) 2464 && compareDeep(partOf, o.partOf, true) && compareDeep(questionnaire, o.questionnaire, true) 2465 && compareDeep(status, o.status, true) && compareDeep(subject, o.subject, true) 2466 && compareDeep(encounter, o.encounter, true) && compareDeep(authored, o.authored, true) 2467 && compareDeep(author, o.author, true) && compareDeep(source, o.source, true) 2468 && compareDeep(item, o.item, true); 2469 } 2470 2471 @Override 2472 public boolean equalsShallow(Base other_) { 2473 if (!super.equalsShallow(other_)) 2474 return false; 2475 if (!(other_ instanceof QuestionnaireResponse)) 2476 return false; 2477 QuestionnaireResponse o = (QuestionnaireResponse) other_; 2478 return compareValues(status, o.status, true) && compareValues(authored, o.authored, true); 2479 } 2480 2481 public boolean isEmpty() { 2482 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, partOf, questionnaire, status, 2483 subject, encounter, authored, author, source, item); 2484 } 2485 2486 @Override 2487 public ResourceType getResourceType() { 2488 return ResourceType.QuestionnaireResponse; 2489 } 2490 2491 /** 2492 * Search parameter: <b>authored</b> 2493 * <p> 2494 * Description: <b>When the questionnaire response was last changed</b><br> 2495 * Type: <b>date</b><br> 2496 * Path: <b>QuestionnaireResponse.authored</b><br> 2497 * </p> 2498 */ 2499 @SearchParamDefinition(name = "authored", path = "QuestionnaireResponse.authored", description = "When the questionnaire response was last changed", type = "date") 2500 public static final String SP_AUTHORED = "authored"; 2501 /** 2502 * <b>Fluent Client</b> search parameter constant for <b>authored</b> 2503 * <p> 2504 * Description: <b>When the questionnaire response was last changed</b><br> 2505 * Type: <b>date</b><br> 2506 * Path: <b>QuestionnaireResponse.authored</b><br> 2507 * </p> 2508 */ 2509 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORED = new ca.uhn.fhir.rest.gclient.DateClientParam( 2510 SP_AUTHORED); 2511 2512 /** 2513 * Search parameter: <b>identifier</b> 2514 * <p> 2515 * Description: <b>The unique identifier for the questionnaire response</b><br> 2516 * Type: <b>token</b><br> 2517 * Path: <b>QuestionnaireResponse.identifier</b><br> 2518 * </p> 2519 */ 2520 @SearchParamDefinition(name = "identifier", path = "QuestionnaireResponse.identifier", description = "The unique identifier for the questionnaire response", type = "token") 2521 public static final String SP_IDENTIFIER = "identifier"; 2522 /** 2523 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2524 * <p> 2525 * Description: <b>The unique identifier for the questionnaire response</b><br> 2526 * Type: <b>token</b><br> 2527 * Path: <b>QuestionnaireResponse.identifier</b><br> 2528 * </p> 2529 */ 2530 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2531 SP_IDENTIFIER); 2532 2533 /** 2534 * Search parameter: <b>questionnaire</b> 2535 * <p> 2536 * Description: <b>The questionnaire the answers are provided for</b><br> 2537 * Type: <b>reference</b><br> 2538 * Path: <b>QuestionnaireResponse.questionnaire</b><br> 2539 * </p> 2540 */ 2541 @SearchParamDefinition(name = "questionnaire", path = "QuestionnaireResponse.questionnaire", description = "The questionnaire the answers are provided for", type = "reference", target = { 2542 Questionnaire.class }) 2543 public static final String SP_QUESTIONNAIRE = "questionnaire"; 2544 /** 2545 * <b>Fluent Client</b> search parameter constant for <b>questionnaire</b> 2546 * <p> 2547 * Description: <b>The questionnaire the answers are provided for</b><br> 2548 * Type: <b>reference</b><br> 2549 * Path: <b>QuestionnaireResponse.questionnaire</b><br> 2550 * </p> 2551 */ 2552 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam QUESTIONNAIRE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2553 SP_QUESTIONNAIRE); 2554 2555 /** 2556 * Constant for fluent queries to be used to add include statements. Specifies 2557 * the path value of "<b>QuestionnaireResponse:questionnaire</b>". 2558 */ 2559 public static final ca.uhn.fhir.model.api.Include INCLUDE_QUESTIONNAIRE = new ca.uhn.fhir.model.api.Include( 2560 "QuestionnaireResponse:questionnaire").toLocked(); 2561 2562 /** 2563 * Search parameter: <b>based-on</b> 2564 * <p> 2565 * Description: <b>Plan/proposal/order fulfilled by this questionnaire 2566 * response</b><br> 2567 * Type: <b>reference</b><br> 2568 * Path: <b>QuestionnaireResponse.basedOn</b><br> 2569 * </p> 2570 */ 2571 @SearchParamDefinition(name = "based-on", path = "QuestionnaireResponse.basedOn", description = "Plan/proposal/order fulfilled by this questionnaire response", type = "reference", target = { 2572 CarePlan.class, ServiceRequest.class }) 2573 public static final String SP_BASED_ON = "based-on"; 2574 /** 2575 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 2576 * <p> 2577 * Description: <b>Plan/proposal/order fulfilled by this questionnaire 2578 * response</b><br> 2579 * Type: <b>reference</b><br> 2580 * Path: <b>QuestionnaireResponse.basedOn</b><br> 2581 * </p> 2582 */ 2583 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2584 SP_BASED_ON); 2585 2586 /** 2587 * Constant for fluent queries to be used to add include statements. Specifies 2588 * the path value of "<b>QuestionnaireResponse:based-on</b>". 2589 */ 2590 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include( 2591 "QuestionnaireResponse:based-on").toLocked(); 2592 2593 /** 2594 * Search parameter: <b>subject</b> 2595 * <p> 2596 * Description: <b>The subject of the questionnaire response</b><br> 2597 * Type: <b>reference</b><br> 2598 * Path: <b>QuestionnaireResponse.subject</b><br> 2599 * </p> 2600 */ 2601 @SearchParamDefinition(name = "subject", path = "QuestionnaireResponse.subject", description = "The subject of the questionnaire response", type = "reference", providesMembershipIn = { 2602 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }) 2603 public static final String SP_SUBJECT = "subject"; 2604 /** 2605 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2606 * <p> 2607 * Description: <b>The subject of the questionnaire response</b><br> 2608 * Type: <b>reference</b><br> 2609 * Path: <b>QuestionnaireResponse.subject</b><br> 2610 * </p> 2611 */ 2612 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2613 SP_SUBJECT); 2614 2615 /** 2616 * Constant for fluent queries to be used to add include statements. Specifies 2617 * the path value of "<b>QuestionnaireResponse:subject</b>". 2618 */ 2619 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 2620 "QuestionnaireResponse:subject").toLocked(); 2621 2622 /** 2623 * Search parameter: <b>author</b> 2624 * <p> 2625 * Description: <b>The author of the questionnaire response</b><br> 2626 * Type: <b>reference</b><br> 2627 * Path: <b>QuestionnaireResponse.author</b><br> 2628 * </p> 2629 */ 2630 @SearchParamDefinition(name = "author", path = "QuestionnaireResponse.author", description = "The author of the questionnaire response", type = "reference", providesMembershipIn = { 2631 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 2632 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 2633 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 2634 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, 2635 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 2636 public static final String SP_AUTHOR = "author"; 2637 /** 2638 * <b>Fluent Client</b> search parameter constant for <b>author</b> 2639 * <p> 2640 * Description: <b>The author of the questionnaire response</b><br> 2641 * Type: <b>reference</b><br> 2642 * Path: <b>QuestionnaireResponse.author</b><br> 2643 * </p> 2644 */ 2645 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2646 SP_AUTHOR); 2647 2648 /** 2649 * Constant for fluent queries to be used to add include statements. Specifies 2650 * the path value of "<b>QuestionnaireResponse:author</b>". 2651 */ 2652 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include( 2653 "QuestionnaireResponse:author").toLocked(); 2654 2655 /** 2656 * Search parameter: <b>patient</b> 2657 * <p> 2658 * Description: <b>The patient that is the subject of the questionnaire 2659 * response</b><br> 2660 * Type: <b>reference</b><br> 2661 * Path: <b>QuestionnaireResponse.subject</b><br> 2662 * </p> 2663 */ 2664 @SearchParamDefinition(name = "patient", path = "QuestionnaireResponse.subject.where(resolve() is Patient)", description = "The patient that is the subject of the questionnaire response", type = "reference", target = { 2665 Patient.class }) 2666 public static final String SP_PATIENT = "patient"; 2667 /** 2668 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2669 * <p> 2670 * Description: <b>The patient that is the subject of the questionnaire 2671 * response</b><br> 2672 * Type: <b>reference</b><br> 2673 * Path: <b>QuestionnaireResponse.subject</b><br> 2674 * </p> 2675 */ 2676 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2677 SP_PATIENT); 2678 2679 /** 2680 * Constant for fluent queries to be used to add include statements. Specifies 2681 * the path value of "<b>QuestionnaireResponse:patient</b>". 2682 */ 2683 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 2684 "QuestionnaireResponse:patient").toLocked(); 2685 2686 /** 2687 * Search parameter: <b>part-of</b> 2688 * <p> 2689 * Description: <b>Procedure or observation this questionnaire response was 2690 * performed as a part of</b><br> 2691 * Type: <b>reference</b><br> 2692 * Path: <b>QuestionnaireResponse.partOf</b><br> 2693 * </p> 2694 */ 2695 @SearchParamDefinition(name = "part-of", path = "QuestionnaireResponse.partOf", description = "Procedure or observation this questionnaire response was performed as a part of", type = "reference", target = { 2696 Observation.class, Procedure.class }) 2697 public static final String SP_PART_OF = "part-of"; 2698 /** 2699 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 2700 * <p> 2701 * Description: <b>Procedure or observation this questionnaire response was 2702 * performed as a part of</b><br> 2703 * Type: <b>reference</b><br> 2704 * Path: <b>QuestionnaireResponse.partOf</b><br> 2705 * </p> 2706 */ 2707 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2708 SP_PART_OF); 2709 2710 /** 2711 * Constant for fluent queries to be used to add include statements. Specifies 2712 * the path value of "<b>QuestionnaireResponse:part-of</b>". 2713 */ 2714 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include( 2715 "QuestionnaireResponse:part-of").toLocked(); 2716 2717 /** 2718 * Search parameter: <b>encounter</b> 2719 * <p> 2720 * Description: <b>Encounter associated with the questionnaire response</b><br> 2721 * Type: <b>reference</b><br> 2722 * Path: <b>QuestionnaireResponse.encounter</b><br> 2723 * </p> 2724 */ 2725 @SearchParamDefinition(name = "encounter", path = "QuestionnaireResponse.encounter", description = "Encounter associated with the questionnaire response", type = "reference", providesMembershipIn = { 2726 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 2727 public static final String SP_ENCOUNTER = "encounter"; 2728 /** 2729 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2730 * <p> 2731 * Description: <b>Encounter associated with the questionnaire response</b><br> 2732 * Type: <b>reference</b><br> 2733 * Path: <b>QuestionnaireResponse.encounter</b><br> 2734 * </p> 2735 */ 2736 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2737 SP_ENCOUNTER); 2738 2739 /** 2740 * Constant for fluent queries to be used to add include statements. Specifies 2741 * the path value of "<b>QuestionnaireResponse:encounter</b>". 2742 */ 2743 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 2744 "QuestionnaireResponse:encounter").toLocked(); 2745 2746 /** 2747 * Search parameter: <b>source</b> 2748 * <p> 2749 * Description: <b>The individual providing the information reflected in the 2750 * questionnaire respose</b><br> 2751 * Type: <b>reference</b><br> 2752 * Path: <b>QuestionnaireResponse.source</b><br> 2753 * </p> 2754 */ 2755 @SearchParamDefinition(name = "source", path = "QuestionnaireResponse.source", description = "The individual providing the information reflected in the questionnaire respose", type = "reference", providesMembershipIn = { 2756 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 2757 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Patient.class, 2758 Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 2759 public static final String SP_SOURCE = "source"; 2760 /** 2761 * <b>Fluent Client</b> search parameter constant for <b>source</b> 2762 * <p> 2763 * Description: <b>The individual providing the information reflected in the 2764 * questionnaire respose</b><br> 2765 * Type: <b>reference</b><br> 2766 * Path: <b>QuestionnaireResponse.source</b><br> 2767 * </p> 2768 */ 2769 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2770 SP_SOURCE); 2771 2772 /** 2773 * Constant for fluent queries to be used to add include statements. Specifies 2774 * the path value of "<b>QuestionnaireResponse:source</b>". 2775 */ 2776 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include( 2777 "QuestionnaireResponse:source").toLocked(); 2778 2779 /** 2780 * Search parameter: <b>status</b> 2781 * <p> 2782 * Description: <b>The status of the questionnaire response</b><br> 2783 * Type: <b>token</b><br> 2784 * Path: <b>QuestionnaireResponse.status</b><br> 2785 * </p> 2786 */ 2787 @SearchParamDefinition(name = "status", path = "QuestionnaireResponse.status", description = "The status of the questionnaire response", type = "token") 2788 public static final String SP_STATUS = "status"; 2789 /** 2790 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2791 * <p> 2792 * Description: <b>The status of the questionnaire response</b><br> 2793 * Type: <b>token</b><br> 2794 * Path: <b>QuestionnaireResponse.status</b><br> 2795 * </p> 2796 */ 2797 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2798 SP_STATUS); 2799 2800}