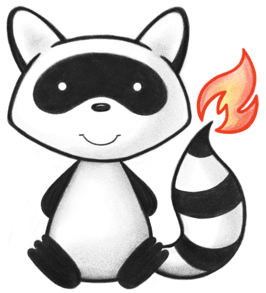
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.List; 034 035import org.hl7.fhir.exceptions.FHIRException; 036import org.hl7.fhir.instance.model.api.ICompositeType; 037 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.DatatypeDef; 040import ca.uhn.fhir.model.api.annotation.Description; 041 042/** 043 * A set of ordered Quantities defined by a low and high limit. 044 */ 045@DatatypeDef(name = "Range") 046public class Range extends Type implements ICompositeType { 047 048 /** 049 * The low limit. The boundary is inclusive. 050 */ 051 @Child(name = "low", type = { Quantity.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 052 @Description(shortDefinition = "Low limit", formalDefinition = "The low limit. The boundary is inclusive.") 053 protected Quantity low; 054 055 /** 056 * The high limit. The boundary is inclusive. 057 */ 058 @Child(name = "high", type = { Quantity.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 059 @Description(shortDefinition = "High limit", formalDefinition = "The high limit. The boundary is inclusive.") 060 protected Quantity high; 061 062 private static final long serialVersionUID = -474933350L; 063 064 /** 065 * Constructor 066 */ 067 public Range() { 068 super(); 069 } 070 071 /** 072 * @return {@link #low} (The low limit. The boundary is inclusive.) 073 */ 074 public Quantity getLow() { 075 if (this.low == null) 076 if (Configuration.errorOnAutoCreate()) 077 throw new Error("Attempt to auto-create Range.low"); 078 else if (Configuration.doAutoCreate()) 079 this.low = new Quantity(); // cc 080 return this.low; 081 } 082 083 public boolean hasLow() { 084 return this.low != null && !this.low.isEmpty(); 085 } 086 087 /** 088 * @param value {@link #low} (The low limit. The boundary is inclusive.) 089 */ 090 public Range setLow(Quantity value) { 091 this.low = value; 092 return this; 093 } 094 095 /** 096 * @return {@link #high} (The high limit. The boundary is inclusive.) 097 */ 098 public Quantity getHigh() { 099 if (this.high == null) 100 if (Configuration.errorOnAutoCreate()) 101 throw new Error("Attempt to auto-create Range.high"); 102 else if (Configuration.doAutoCreate()) 103 this.high = new Quantity(); // cc 104 return this.high; 105 } 106 107 public boolean hasHigh() { 108 return this.high != null && !this.high.isEmpty(); 109 } 110 111 /** 112 * @param value {@link #high} (The high limit. The boundary is inclusive.) 113 */ 114 public Range setHigh(Quantity value) { 115 this.high = value; 116 return this; 117 } 118 119 protected void listChildren(List<Property> children) { 120 super.listChildren(children); 121 children.add(new Property("low", "SimpleQuantity", "The low limit. The boundary is inclusive.", 0, 1, low)); 122 children.add(new Property("high", "SimpleQuantity", "The high limit. The boundary is inclusive.", 0, 1, high)); 123 } 124 125 @Override 126 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 127 switch (_hash) { 128 case 107348: 129 /* low */ return new Property("low", "SimpleQuantity", "The low limit. The boundary is inclusive.", 0, 1, low); 130 case 3202466: 131 /* high */ return new Property("high", "SimpleQuantity", "The high limit. The boundary is inclusive.", 0, 1, 132 high); 133 default: 134 return super.getNamedProperty(_hash, _name, _checkValid); 135 } 136 137 } 138 139 @Override 140 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 141 switch (hash) { 142 case 107348: 143 /* low */ return this.low == null ? new Base[0] : new Base[] { this.low }; // Quantity 144 case 3202466: 145 /* high */ return this.high == null ? new Base[0] : new Base[] { this.high }; // Quantity 146 default: 147 return super.getProperty(hash, name, checkValid); 148 } 149 150 } 151 152 @Override 153 public Base setProperty(int hash, String name, Base value) throws FHIRException { 154 switch (hash) { 155 case 107348: // low 156 this.low = castToQuantity(value); // Quantity 157 return value; 158 case 3202466: // high 159 this.high = castToQuantity(value); // Quantity 160 return value; 161 default: 162 return super.setProperty(hash, name, value); 163 } 164 165 } 166 167 @Override 168 public Base setProperty(String name, Base value) throws FHIRException { 169 if (name.equals("low")) { 170 this.low = castToQuantity(value); // Quantity 171 } else if (name.equals("high")) { 172 this.high = castToQuantity(value); // Quantity 173 } else 174 return super.setProperty(name, value); 175 return value; 176 } 177 178 @Override 179 public void removeChild(String name, Base value) throws FHIRException { 180 if (name.equals("low")) { 181 this.low = null; 182 } else if (name.equals("high")) { 183 this.high = null; 184 } else 185 super.removeChild(name, value); 186 187 } 188 189 @Override 190 public Base makeProperty(int hash, String name) throws FHIRException { 191 switch (hash) { 192 case 107348: 193 return getLow(); 194 case 3202466: 195 return getHigh(); 196 default: 197 return super.makeProperty(hash, name); 198 } 199 200 } 201 202 @Override 203 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 204 switch (hash) { 205 case 107348: 206 /* low */ return new String[] { "SimpleQuantity" }; 207 case 3202466: 208 /* high */ return new String[] { "SimpleQuantity" }; 209 default: 210 return super.getTypesForProperty(hash, name); 211 } 212 213 } 214 215 @Override 216 public Base addChild(String name) throws FHIRException { 217 if (name.equals("low")) { 218 this.low = new Quantity(); 219 return this.low; 220 } else if (name.equals("high")) { 221 this.high = new Quantity(); 222 return this.high; 223 } else 224 return super.addChild(name); 225 } 226 227 public String fhirType() { 228 return "Range"; 229 230 } 231 232 public Range copy() { 233 Range dst = new Range(); 234 copyValues(dst); 235 return dst; 236 } 237 238 public void copyValues(Range dst) { 239 super.copyValues(dst); 240 dst.low = low == null ? null : low.copy(); 241 dst.high = high == null ? null : high.copy(); 242 } 243 244 protected Range typedCopy() { 245 return copy(); 246 } 247 248 @Override 249 public boolean equalsDeep(Base other_) { 250 if (!super.equalsDeep(other_)) 251 return false; 252 if (!(other_ instanceof Range)) 253 return false; 254 Range o = (Range) other_; 255 return compareDeep(low, o.low, true) && compareDeep(high, o.high, true); 256 } 257 258 @Override 259 public boolean equalsShallow(Base other_) { 260 if (!super.equalsShallow(other_)) 261 return false; 262 if (!(other_ instanceof Range)) 263 return false; 264 Range o = (Range) other_; 265 return true; 266 } 267 268 public boolean isEmpty() { 269 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(low, high); 270 } 271 272}