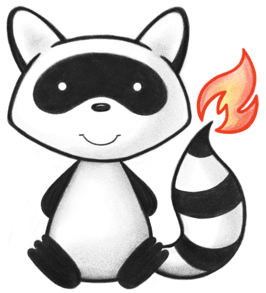
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.List; 034 035import org.hl7.fhir.exceptions.FHIRException; 036import org.hl7.fhir.instance.model.api.ICompositeType; 037 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.DatatypeDef; 040import ca.uhn.fhir.model.api.annotation.Description; 041 042/** 043 * A relationship of two Quantity values - expressed as a numerator and a 044 * denominator. 045 */ 046@DatatypeDef(name = "Ratio") 047public class Ratio extends Type implements ICompositeType { 048 049 /** 050 * The value of the numerator. 051 */ 052 @Child(name = "numerator", type = { Quantity.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 053 @Description(shortDefinition = "Numerator value", formalDefinition = "The value of the numerator.") 054 protected Quantity numerator; 055 056 /** 057 * The value of the denominator. 058 */ 059 @Child(name = "denominator", type = { Quantity.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 060 @Description(shortDefinition = "Denominator value", formalDefinition = "The value of the denominator.") 061 protected Quantity denominator; 062 063 private static final long serialVersionUID = 479922563L; 064 065 /** 066 * Constructor 067 */ 068 public Ratio() { 069 super(); 070 } 071 072 /** 073 * @return {@link #numerator} (The value of the numerator.) 074 */ 075 public Quantity getNumerator() { 076 if (this.numerator == null) 077 if (Configuration.errorOnAutoCreate()) 078 throw new Error("Attempt to auto-create Ratio.numerator"); 079 else if (Configuration.doAutoCreate()) 080 this.numerator = new Quantity(); // cc 081 return this.numerator; 082 } 083 084 public boolean hasNumerator() { 085 return this.numerator != null && !this.numerator.isEmpty(); 086 } 087 088 /** 089 * @param value {@link #numerator} (The value of the numerator.) 090 */ 091 public Ratio setNumerator(Quantity value) { 092 this.numerator = value; 093 return this; 094 } 095 096 /** 097 * @return {@link #denominator} (The value of the denominator.) 098 */ 099 public Quantity getDenominator() { 100 if (this.denominator == null) 101 if (Configuration.errorOnAutoCreate()) 102 throw new Error("Attempt to auto-create Ratio.denominator"); 103 else if (Configuration.doAutoCreate()) 104 this.denominator = new Quantity(); // cc 105 return this.denominator; 106 } 107 108 public boolean hasDenominator() { 109 return this.denominator != null && !this.denominator.isEmpty(); 110 } 111 112 /** 113 * @param value {@link #denominator} (The value of the denominator.) 114 */ 115 public Ratio setDenominator(Quantity value) { 116 this.denominator = value; 117 return this; 118 } 119 120 protected void listChildren(List<Property> children) { 121 super.listChildren(children); 122 children.add(new Property("numerator", "Quantity", "The value of the numerator.", 0, 1, numerator)); 123 children.add(new Property("denominator", "Quantity", "The value of the denominator.", 0, 1, denominator)); 124 } 125 126 @Override 127 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 128 switch (_hash) { 129 case 1747334793: 130 /* numerator */ return new Property("numerator", "Quantity", "The value of the numerator.", 0, 1, numerator); 131 case -1983274394: 132 /* denominator */ return new Property("denominator", "Quantity", "The value of the denominator.", 0, 1, 133 denominator); 134 default: 135 return super.getNamedProperty(_hash, _name, _checkValid); 136 } 137 138 } 139 140 @Override 141 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 142 switch (hash) { 143 case 1747334793: 144 /* numerator */ return this.numerator == null ? new Base[0] : new Base[] { this.numerator }; // Quantity 145 case -1983274394: 146 /* denominator */ return this.denominator == null ? new Base[0] : new Base[] { this.denominator }; // Quantity 147 default: 148 return super.getProperty(hash, name, checkValid); 149 } 150 151 } 152 153 @Override 154 public Base setProperty(int hash, String name, Base value) throws FHIRException { 155 switch (hash) { 156 case 1747334793: // numerator 157 this.numerator = castToQuantity(value); // Quantity 158 return value; 159 case -1983274394: // denominator 160 this.denominator = castToQuantity(value); // Quantity 161 return value; 162 default: 163 return super.setProperty(hash, name, value); 164 } 165 166 } 167 168 @Override 169 public Base setProperty(String name, Base value) throws FHIRException { 170 if (name.equals("numerator")) { 171 this.numerator = castToQuantity(value); // Quantity 172 } else if (name.equals("denominator")) { 173 this.denominator = castToQuantity(value); // Quantity 174 } else 175 return super.setProperty(name, value); 176 return value; 177 } 178 179 @Override 180 public void removeChild(String name, Base value) throws FHIRException { 181 if (name.equals("numerator")) { 182 this.numerator = null; 183 } else if (name.equals("denominator")) { 184 this.denominator = null; 185 } else 186 super.removeChild(name, value); 187 188 } 189 190 @Override 191 public Base makeProperty(int hash, String name) throws FHIRException { 192 switch (hash) { 193 case 1747334793: 194 return getNumerator(); 195 case -1983274394: 196 return getDenominator(); 197 default: 198 return super.makeProperty(hash, name); 199 } 200 201 } 202 203 @Override 204 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 205 switch (hash) { 206 case 1747334793: 207 /* numerator */ return new String[] { "Quantity" }; 208 case -1983274394: 209 /* denominator */ return new String[] { "Quantity" }; 210 default: 211 return super.getTypesForProperty(hash, name); 212 } 213 214 } 215 216 @Override 217 public Base addChild(String name) throws FHIRException { 218 if (name.equals("numerator")) { 219 this.numerator = new Quantity(); 220 return this.numerator; 221 } else if (name.equals("denominator")) { 222 this.denominator = new Quantity(); 223 return this.denominator; 224 } else 225 return super.addChild(name); 226 } 227 228 public String fhirType() { 229 return "Ratio"; 230 231 } 232 233 public Ratio copy() { 234 Ratio dst = new Ratio(); 235 copyValues(dst); 236 return dst; 237 } 238 239 public void copyValues(Ratio dst) { 240 super.copyValues(dst); 241 dst.numerator = numerator == null ? null : numerator.copy(); 242 dst.denominator = denominator == null ? null : denominator.copy(); 243 } 244 245 protected Ratio typedCopy() { 246 return copy(); 247 } 248 249 @Override 250 public boolean equalsDeep(Base other_) { 251 if (!super.equalsDeep(other_)) 252 return false; 253 if (!(other_ instanceof Ratio)) 254 return false; 255 Ratio o = (Ratio) other_; 256 return compareDeep(numerator, o.numerator, true) && compareDeep(denominator, o.denominator, true); 257 } 258 259 @Override 260 public boolean equalsShallow(Base other_) { 261 if (!super.equalsShallow(other_)) 262 return false; 263 if (!(other_ instanceof Ratio)) 264 return false; 265 Ratio o = (Ratio) other_; 266 return true; 267 } 268 269 public boolean isEmpty() { 270 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(numerator, denominator); 271 } 272 273}