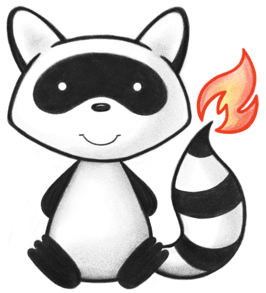
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.List; 034 035import org.hl7.fhir.exceptions.FHIRException; 036import org.hl7.fhir.instance.model.api.IAnyResource; 037import org.hl7.fhir.instance.model.api.IBaseReference; 038import org.hl7.fhir.instance.model.api.ICompositeType; 039import org.hl7.fhir.instance.model.api.IIdType; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.DatatypeDef; 044import ca.uhn.fhir.model.api.annotation.Description; 045 046/** 047 * A reference from one resource to another. 048 */ 049@DatatypeDef(name = "Reference") 050public class Reference extends BaseReference implements IBaseReference, ICompositeType { 051 052 /** 053 * A reference to a location at which the other resource is found. The reference 054 * may be a relative reference, in which case it is relative to the service base 055 * URL, or an absolute URL that resolves to the location where the resource is 056 * found. The reference may be version specific or not. If the reference is not 057 * to a FHIR RESTful server, then it should be assumed to be version specific. 058 * Internal fragment references (start with '#') refer to contained resources. 059 */ 060 @Child(name = "reference", type = { StringType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 061 @Description(shortDefinition = "Literal reference, Relative, internal or absolute URL", formalDefinition = "A reference to a location at which the other resource is found. The reference may be a relative reference, in which case it is relative to the service base URL, or an absolute URL that resolves to the location where the resource is found. The reference may be version specific or not. If the reference is not to a FHIR RESTful server, then it should be assumed to be version specific. Internal fragment references (start with '#') refer to contained resources.") 062 protected StringType reference; 063 064 /** 065 * The expected type of the target of the reference. If both Reference.type and 066 * Reference.reference are populated and Reference.reference is a FHIR URL, both 067 * SHALL be consistent. 068 * 069 * The type is the Canonical URL of Resource Definition that is the type this 070 * reference refers to. References are URLs that are relative to 071 * http://hl7.org/fhir/StructureDefinition/ e.g. "Patient" is a reference to 072 * http://hl7.org/fhir/StructureDefinition/Patient. Absolute URLs are only 073 * allowed for logical models (and can only be used in references in logical 074 * models, not resources). 075 */ 076 @Child(name = "type", type = { UriType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 077 @Description(shortDefinition = "Type the reference refers to (e.g. \"Patient\")", formalDefinition = "The expected type of the target of the reference. If both Reference.type and Reference.reference are populated and Reference.reference is a FHIR URL, both SHALL be consistent.\n\nThe type is the Canonical URL of Resource Definition that is the type this reference refers to. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition/ e.g. \"Patient\" is a reference to http://hl7.org/fhir/StructureDefinition/Patient. Absolute URLs are only allowed for logical models (and can only be used in references in logical models, not resources).") 078 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/resource-types") 079 protected UriType type; 080 081 /** 082 * An identifier for the target resource. This is used when there is no way to 083 * reference the other resource directly, either because the entity it 084 * represents is not available through a FHIR server, or because there is no way 085 * for the author of the resource to convert a known identifier to an actual 086 * location. There is no requirement that a Reference.identifier point to 087 * something that is actually exposed as a FHIR instance, but it SHALL point to 088 * a business concept that would be expected to be exposed as a FHIR instance, 089 * and that instance would need to be of a FHIR resource type allowed by the 090 * reference. 091 */ 092 @Child(name = "identifier", type = { 093 Identifier.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 094 @Description(shortDefinition = "Logical reference, when literal reference is not known", formalDefinition = "An identifier for the target resource. This is used when there is no way to reference the other resource directly, either because the entity it represents is not available through a FHIR server, or because there is no way for the author of the resource to convert a known identifier to an actual location. There is no requirement that a Reference.identifier point to something that is actually exposed as a FHIR instance, but it SHALL point to a business concept that would be expected to be exposed as a FHIR instance, and that instance would need to be of a FHIR resource type allowed by the reference.") 095 protected Identifier identifier; 096 097 /** 098 * Plain text narrative that identifies the resource in addition to the resource 099 * reference. 100 */ 101 @Child(name = "display", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 102 @Description(shortDefinition = "Text alternative for the resource", formalDefinition = "Plain text narrative that identifies the resource in addition to the resource reference.") 103 protected StringType display; 104 105 private static final long serialVersionUID = 784245805L; 106 107 /** 108 * Constructor 109 */ 110 public Reference() { 111 super(); 112 } 113 114 /** 115 * Constructor 116 * 117 * @param theReference The given reference string (e.g. "Patient/123" or 118 * "http://example.com/Patient/123") 119 */ 120 public Reference(String theReference) { 121 super(theReference); 122 } 123 124 /** 125 * Constructor 126 * 127 * @param theReference The given reference as an IdType (e.g. "Patient/123" or 128 * "http://example.com/Patient/123") 129 */ 130 public Reference(IIdType theReference) { 131 super(theReference); 132 } 133 134 /** 135 * Constructor 136 * 137 * @param theResource The resource represented by this reference 138 */ 139 public Reference(IAnyResource theResource) { 140 super(theResource); 141 } 142 143 /** 144 * @return {@link #reference} (A reference to a location at which the other 145 * resource is found. The reference may be a relative reference, in 146 * which case it is relative to the service base URL, or an absolute URL 147 * that resolves to the location where the resource is found. The 148 * reference may be version specific or not. If the reference is not to 149 * a FHIR RESTful server, then it should be assumed to be version 150 * specific. Internal fragment references (start with '#') refer to 151 * contained resources.). This is the underlying object with id, value 152 * and extensions. The accessor "getReference" gives direct access to 153 * the value 154 */ 155 public StringType getReferenceElement_() { 156 if (this.reference == null) 157 if (Configuration.errorOnAutoCreate()) 158 throw new Error("Attempt to auto-create Reference.reference"); 159 else if (Configuration.doAutoCreate()) 160 this.reference = new StringType(); // bb 161 return this.reference; 162 } 163 164 public boolean hasReferenceElement() { 165 return this.reference != null && !this.reference.isEmpty(); 166 } 167 168 public boolean hasReference() { 169 return this.reference != null && !this.reference.isEmpty(); 170 } 171 172 /** 173 * @param value {@link #reference} (A reference to a location at which the other 174 * resource is found. The reference may be a relative reference, in 175 * which case it is relative to the service base URL, or an 176 * absolute URL that resolves to the location where the resource is 177 * found. The reference may be version specific or not. If the 178 * reference is not to a FHIR RESTful server, then it should be 179 * assumed to be version specific. Internal fragment references 180 * (start with '#') refer to contained resources.). This is the 181 * underlying object with id, value and extensions. The accessor 182 * "getReference" gives direct access to the value 183 */ 184 public Reference setReferenceElement(StringType value) { 185 this.reference = value; 186 return this; 187 } 188 189 /** 190 * @return A reference to a location at which the other resource is found. The 191 * reference may be a relative reference, in which case it is relative 192 * to the service base URL, or an absolute URL that resolves to the 193 * location where the resource is found. The reference may be version 194 * specific or not. If the reference is not to a FHIR RESTful server, 195 * then it should be assumed to be version specific. Internal fragment 196 * references (start with '#') refer to contained resources. 197 */ 198 public String getReference() { 199 return this.reference == null ? null : this.reference.getValue(); 200 } 201 202 /** 203 * @param value A reference to a location at which the other resource is found. 204 * The reference may be a relative reference, in which case it is 205 * relative to the service base URL, or an absolute URL that 206 * resolves to the location where the resource is found. The 207 * reference may be version specific or not. If the reference is 208 * not to a FHIR RESTful server, then it should be assumed to be 209 * version specific. Internal fragment references (start with '#') 210 * refer to contained resources. 211 */ 212 public Reference setReference(String value) { 213 if (Utilities.noString(value)) 214 this.reference = null; 215 else { 216 if (this.reference == null) 217 this.reference = new StringType(); 218 this.reference.setValue(value); 219 } 220 return this; 221 } 222 223 /** 224 * @return {@link #type} (The expected type of the target of the reference. If 225 * both Reference.type and Reference.reference are populated and 226 * Reference.reference is a FHIR URL, both SHALL be consistent. 227 * 228 * The type is the Canonical URL of Resource Definition that is the type 229 * this reference refers to. References are URLs that are relative to 230 * http://hl7.org/fhir/StructureDefinition/ e.g. "Patient" is a 231 * reference to http://hl7.org/fhir/StructureDefinition/Patient. 232 * Absolute URLs are only allowed for logical models (and can only be 233 * used in references in logical models, not resources).). This is the 234 * underlying object with id, value and extensions. The accessor 235 * "getType" gives direct access to the value 236 */ 237 public UriType getTypeElement() { 238 if (this.type == null) 239 if (Configuration.errorOnAutoCreate()) 240 throw new Error("Attempt to auto-create Reference.type"); 241 else if (Configuration.doAutoCreate()) 242 this.type = new UriType(); // bb 243 return this.type; 244 } 245 246 public boolean hasTypeElement() { 247 return this.type != null && !this.type.isEmpty(); 248 } 249 250 public boolean hasType() { 251 return this.type != null && !this.type.isEmpty(); 252 } 253 254 /** 255 * @param value {@link #type} (The expected type of the target of the reference. 256 * If both Reference.type and Reference.reference are populated and 257 * Reference.reference is a FHIR URL, both SHALL be consistent. 258 * 259 * The type is the Canonical URL of Resource Definition that is the 260 * type this reference refers to. References are URLs that are 261 * relative to http://hl7.org/fhir/StructureDefinition/ e.g. 262 * "Patient" is a reference to 263 * http://hl7.org/fhir/StructureDefinition/Patient. Absolute URLs 264 * are only allowed for logical models (and can only be used in 265 * references in logical models, not resources).). This is the 266 * underlying object with id, value and extensions. The accessor 267 * "getType" gives direct access to the value 268 */ 269 public Reference setTypeElement(UriType value) { 270 this.type = value; 271 return this; 272 } 273 274 /** 275 * @return The expected type of the target of the reference. If both 276 * Reference.type and Reference.reference are populated and 277 * Reference.reference is a FHIR URL, both SHALL be consistent. 278 * 279 * The type is the Canonical URL of Resource Definition that is the type 280 * this reference refers to. References are URLs that are relative to 281 * http://hl7.org/fhir/StructureDefinition/ e.g. "Patient" is a 282 * reference to http://hl7.org/fhir/StructureDefinition/Patient. 283 * Absolute URLs are only allowed for logical models (and can only be 284 * used in references in logical models, not resources). 285 */ 286 public String getType() { 287 return this.type == null ? null : this.type.getValue(); 288 } 289 290 /** 291 * @param value The expected type of the target of the reference. If both 292 * Reference.type and Reference.reference are populated and 293 * Reference.reference is a FHIR URL, both SHALL be consistent. 294 * 295 * The type is the Canonical URL of Resource Definition that is the 296 * type this reference refers to. References are URLs that are 297 * relative to http://hl7.org/fhir/StructureDefinition/ e.g. 298 * "Patient" is a reference to 299 * http://hl7.org/fhir/StructureDefinition/Patient. Absolute URLs 300 * are only allowed for logical models (and can only be used in 301 * references in logical models, not resources). 302 */ 303 public Reference setType(String value) { 304 if (Utilities.noString(value)) 305 this.type = null; 306 else { 307 if (this.type == null) 308 this.type = new UriType(); 309 this.type.setValue(value); 310 } 311 return this; 312 } 313 314 /** 315 * @return {@link #identifier} (An identifier for the target resource. This is 316 * used when there is no way to reference the other resource directly, 317 * either because the entity it represents is not available through a 318 * FHIR server, or because there is no way for the author of the 319 * resource to convert a known identifier to an actual location. There 320 * is no requirement that a Reference.identifier point to something that 321 * is actually exposed as a FHIR instance, but it SHALL point to a 322 * business concept that would be expected to be exposed as a FHIR 323 * instance, and that instance would need to be of a FHIR resource type 324 * allowed by the reference.) 325 */ 326 public Identifier getIdentifier() { 327 if (this.identifier == null) 328 if (Configuration.errorOnAutoCreate()) 329 throw new Error("Attempt to auto-create Reference.identifier"); 330 else if (Configuration.doAutoCreate()) 331 this.identifier = new Identifier(); // cc 332 return this.identifier; 333 } 334 335 public boolean hasIdentifier() { 336 return this.identifier != null && !this.identifier.isEmpty(); 337 } 338 339 /** 340 * @param value {@link #identifier} (An identifier for the target resource. This 341 * is used when there is no way to reference the other resource 342 * directly, either because the entity it represents is not 343 * available through a FHIR server, or because there is no way for 344 * the author of the resource to convert a known identifier to an 345 * actual location. There is no requirement that a 346 * Reference.identifier point to something that is actually exposed 347 * as a FHIR instance, but it SHALL point to a business concept 348 * that would be expected to be exposed as a FHIR instance, and 349 * that instance would need to be of a FHIR resource type allowed 350 * by the reference.) 351 */ 352 public Reference setIdentifier(Identifier value) { 353 this.identifier = value; 354 return this; 355 } 356 357 /** 358 * @return {@link #display} (Plain text narrative that identifies the resource 359 * in addition to the resource reference.). This is the underlying 360 * object with id, value and extensions. The accessor "getDisplay" gives 361 * direct access to the value 362 */ 363 public StringType getDisplayElement() { 364 if (this.display == null) 365 if (Configuration.errorOnAutoCreate()) 366 throw new Error("Attempt to auto-create Reference.display"); 367 else if (Configuration.doAutoCreate()) 368 this.display = new StringType(); // bb 369 return this.display; 370 } 371 372 public boolean hasDisplayElement() { 373 return this.display != null && !this.display.isEmpty(); 374 } 375 376 public boolean hasDisplay() { 377 return this.display != null && !this.display.isEmpty(); 378 } 379 380 /** 381 * @param value {@link #display} (Plain text narrative that identifies the 382 * resource in addition to the resource reference.). This is the 383 * underlying object with id, value and extensions. The accessor 384 * "getDisplay" gives direct access to the value 385 */ 386 public Reference setDisplayElement(StringType value) { 387 this.display = value; 388 return this; 389 } 390 391 /** 392 * @return Plain text narrative that identifies the resource in addition to the 393 * resource reference. 394 */ 395 public String getDisplay() { 396 return this.display == null ? null : this.display.getValue(); 397 } 398 399 /** 400 * @param value Plain text narrative that identifies the resource in addition to 401 * the resource reference. 402 */ 403 public Reference setDisplay(String value) { 404 if (Utilities.noString(value)) 405 this.display = null; 406 else { 407 if (this.display == null) 408 this.display = new StringType(); 409 this.display.setValue(value); 410 } 411 return this; 412 } 413 414 /** 415 * Convenience setter which sets the reference to the complete 416 * {@link IIdType#getValue() value} of the given reference. 417 * 418 * @param theReference The reference, or <code>null</code> 419 * @return 420 * @return Returns a reference to this 421 */ 422 public Reference setReferenceElement(IIdType theReference) { 423 if (theReference != null) { 424 setReference(theReference.getValue()); 425 } else { 426 setReference(null); 427 } 428 return this; 429 } 430 431 protected void listChildren(List<Property> children) { 432 super.listChildren(children); 433 children.add(new Property("reference", "string", 434 "A reference to a location at which the other resource is found. The reference may be a relative reference, in which case it is relative to the service base URL, or an absolute URL that resolves to the location where the resource is found. The reference may be version specific or not. If the reference is not to a FHIR RESTful server, then it should be assumed to be version specific. Internal fragment references (start with '#') refer to contained resources.", 435 0, 1, reference)); 436 children.add(new Property("type", "uri", 437 "The expected type of the target of the reference. If both Reference.type and Reference.reference are populated and Reference.reference is a FHIR URL, both SHALL be consistent.\n\nThe type is the Canonical URL of Resource Definition that is the type this reference refers to. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition/ e.g. \"Patient\" is a reference to http://hl7.org/fhir/StructureDefinition/Patient. Absolute URLs are only allowed for logical models (and can only be used in references in logical models, not resources).", 438 0, 1, type)); 439 children.add(new Property("identifier", "Identifier", 440 "An identifier for the target resource. This is used when there is no way to reference the other resource directly, either because the entity it represents is not available through a FHIR server, or because there is no way for the author of the resource to convert a known identifier to an actual location. There is no requirement that a Reference.identifier point to something that is actually exposed as a FHIR instance, but it SHALL point to a business concept that would be expected to be exposed as a FHIR instance, and that instance would need to be of a FHIR resource type allowed by the reference.", 441 0, 1, identifier)); 442 children.add(new Property("display", "string", 443 "Plain text narrative that identifies the resource in addition to the resource reference.", 0, 1, display)); 444 } 445 446 @Override 447 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 448 switch (_hash) { 449 case -925155509: 450 /* reference */ return new Property("reference", "string", 451 "A reference to a location at which the other resource is found. The reference may be a relative reference, in which case it is relative to the service base URL, or an absolute URL that resolves to the location where the resource is found. The reference may be version specific or not. If the reference is not to a FHIR RESTful server, then it should be assumed to be version specific. Internal fragment references (start with '#') refer to contained resources.", 452 0, 1, reference); 453 case 3575610: 454 /* type */ return new Property("type", "uri", 455 "The expected type of the target of the reference. If both Reference.type and Reference.reference are populated and Reference.reference is a FHIR URL, both SHALL be consistent.\n\nThe type is the Canonical URL of Resource Definition that is the type this reference refers to. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition/ e.g. \"Patient\" is a reference to http://hl7.org/fhir/StructureDefinition/Patient. Absolute URLs are only allowed for logical models (and can only be used in references in logical models, not resources).", 456 0, 1, type); 457 case -1618432855: 458 /* identifier */ return new Property("identifier", "Identifier", 459 "An identifier for the target resource. This is used when there is no way to reference the other resource directly, either because the entity it represents is not available through a FHIR server, or because there is no way for the author of the resource to convert a known identifier to an actual location. There is no requirement that a Reference.identifier point to something that is actually exposed as a FHIR instance, but it SHALL point to a business concept that would be expected to be exposed as a FHIR instance, and that instance would need to be of a FHIR resource type allowed by the reference.", 460 0, 1, identifier); 461 case 1671764162: 462 /* display */ return new Property("display", "string", 463 "Plain text narrative that identifies the resource in addition to the resource reference.", 0, 1, display); 464 default: 465 return super.getNamedProperty(_hash, _name, _checkValid); 466 } 467 468 } 469 470 @Override 471 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 472 switch (hash) { 473 case -925155509: 474 /* reference */ return this.reference == null ? new Base[0] : new Base[] { this.reference }; // StringType 475 case 3575610: 476 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // UriType 477 case -1618432855: 478 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 479 case 1671764162: 480 /* display */ return this.display == null ? new Base[0] : new Base[] { this.display }; // StringType 481 default: 482 return super.getProperty(hash, name, checkValid); 483 } 484 485 } 486 487 @Override 488 public Base setProperty(int hash, String name, Base value) throws FHIRException { 489 switch (hash) { 490 case -925155509: // reference 491 this.reference = castToString(value); // StringType 492 return value; 493 case 3575610: // type 494 this.type = castToUri(value); // UriType 495 return value; 496 case -1618432855: // identifier 497 this.identifier = castToIdentifier(value); // Identifier 498 return value; 499 case 1671764162: // display 500 this.display = castToString(value); // StringType 501 return value; 502 default: 503 return super.setProperty(hash, name, value); 504 } 505 506 } 507 508 @Override 509 public Base setProperty(String name, Base value) throws FHIRException { 510 if (name.equals("reference")) { 511 this.reference = castToString(value); // StringType 512 } else if (name.equals("type")) { 513 this.type = castToUri(value); // UriType 514 } else if (name.equals("identifier")) { 515 this.identifier = castToIdentifier(value); // Identifier 516 } else if (name.equals("display")) { 517 this.display = castToString(value); // StringType 518 } else 519 return super.setProperty(name, value); 520 return value; 521 } 522 523 @Override 524 public void removeChild(String name, Base value) throws FHIRException { 525 if (name.equals("reference")) { 526 this.reference = null; 527 } else if (name.equals("type")) { 528 this.type = null; 529 } else if (name.equals("identifier")) { 530 this.identifier = null; 531 } else if (name.equals("display")) { 532 this.display = null; 533 } else 534 super.removeChild(name, value); 535 536 } 537 538 @Override 539 public Base makeProperty(int hash, String name) throws FHIRException { 540 switch (hash) { 541 case -925155509: 542 return getReferenceElement_(); 543 case 3575610: 544 return getTypeElement(); 545 case -1618432855: 546 return getIdentifier(); 547 case 1671764162: 548 return getDisplayElement(); 549 default: 550 return super.makeProperty(hash, name); 551 } 552 553 } 554 555 @Override 556 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 557 switch (hash) { 558 case -925155509: 559 /* reference */ return new String[] { "string" }; 560 case 3575610: 561 /* type */ return new String[] { "uri" }; 562 case -1618432855: 563 /* identifier */ return new String[] { "Identifier" }; 564 case 1671764162: 565 /* display */ return new String[] { "string" }; 566 default: 567 return super.getTypesForProperty(hash, name); 568 } 569 570 } 571 572 @Override 573 public Base addChild(String name) throws FHIRException { 574 if (name.equals("reference")) { 575 throw new FHIRException("Cannot call addChild on a singleton property Reference.reference"); 576 } else if (name.equals("type")) { 577 throw new FHIRException("Cannot call addChild on a singleton property Reference.type"); 578 } else if (name.equals("identifier")) { 579 this.identifier = new Identifier(); 580 return this.identifier; 581 } else if (name.equals("display")) { 582 throw new FHIRException("Cannot call addChild on a singleton property Reference.display"); 583 } else 584 return super.addChild(name); 585 } 586 587 public String fhirType() { 588 return "Reference"; 589 590 } 591 592 public Reference copy() { 593 Reference dst = new Reference(); 594 copyValues(dst); 595 return dst; 596 } 597 598 public void copyValues(Reference dst) { 599 super.copyValues(dst); 600 dst.reference = reference == null ? null : reference.copy(); 601 dst.type = type == null ? null : type.copy(); 602 dst.identifier = identifier == null ? null : identifier.copy(); 603 dst.display = display == null ? null : display.copy(); 604 } 605 606 protected Reference typedCopy() { 607 return copy(); 608 } 609 610 @Override 611 public boolean equalsDeep(Base other_) { 612 if (!super.equalsDeep(other_)) 613 return false; 614 if (!(other_ instanceof Reference)) 615 return false; 616 Reference o = (Reference) other_; 617 return compareDeep(reference, o.reference, true) && compareDeep(type, o.type, true) 618 && compareDeep(identifier, o.identifier, true) && compareDeep(display, o.display, true); 619 } 620 621 @Override 622 public boolean equalsShallow(Base other_) { 623 if (!super.equalsShallow(other_)) 624 return false; 625 if (!(other_ instanceof Reference)) 626 return false; 627 Reference o = (Reference) other_; 628 return compareValues(reference, o.reference, true) && compareValues(type, o.type, true) 629 && compareValues(display, o.display, true); 630 } 631 632 public boolean isEmpty() { 633 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(reference, type, identifier, display); 634 } 635 636}