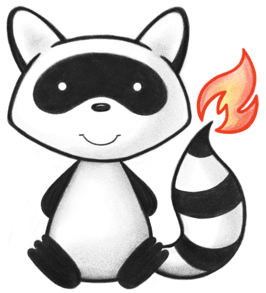
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.List; 034 035import org.hl7.fhir.exceptions.FHIRException; 036import org.hl7.fhir.instance.model.api.ICompositeType; 037import org.hl7.fhir.utilities.Utilities; 038 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.DatatypeDef; 041import ca.uhn.fhir.model.api.annotation.Description; 042 043/** 044 * Related artifacts such as additional documentation, justification, or 045 * bibliographic references. 046 */ 047@DatatypeDef(name = "RelatedArtifact") 048public class RelatedArtifact extends Type implements ICompositeType { 049 050 public enum RelatedArtifactType { 051 /** 052 * Additional documentation for the knowledge resource. This would include 053 * additional instructions on usage as well as additional information on 054 * clinical context or appropriateness. 055 */ 056 DOCUMENTATION, 057 /** 058 * A summary of the justification for the knowledge resource including 059 * supporting evidence, relevant guidelines, or other clinically important 060 * information. This information is intended to provide a way to make the 061 * justification for the knowledge resource available to the consumer of 062 * interventions or results produced by the knowledge resource. 063 */ 064 JUSTIFICATION, 065 /** 066 * Bibliographic citation for papers, references, or other relevant material for 067 * the knowledge resource. This is intended to allow for citation of related 068 * material, but that was not necessarily specifically prepared in connection 069 * with this knowledge resource. 070 */ 071 CITATION, 072 /** 073 * The previous version of the knowledge resource. 074 */ 075 PREDECESSOR, 076 /** 077 * The next version of the knowledge resource. 078 */ 079 SUCCESSOR, 080 /** 081 * The knowledge resource is derived from the related artifact. This is intended 082 * to capture the relationship in which a particular knowledge resource is based 083 * on the content of another artifact, but is modified to capture either a 084 * different set of overall requirements, or a more specific set of requirements 085 * such as those involved in a particular institution or clinical setting. 086 */ 087 DERIVEDFROM, 088 /** 089 * The knowledge resource depends on the given related artifact. 090 */ 091 DEPENDSON, 092 /** 093 * The knowledge resource is composed of the given related artifact. 094 */ 095 COMPOSEDOF, 096 /** 097 * added to help the parsers with the generic types 098 */ 099 NULL; 100 101 public static RelatedArtifactType fromCode(String codeString) throws FHIRException { 102 if (codeString == null || "".equals(codeString)) 103 return null; 104 if ("documentation".equals(codeString)) 105 return DOCUMENTATION; 106 if ("justification".equals(codeString)) 107 return JUSTIFICATION; 108 if ("citation".equals(codeString)) 109 return CITATION; 110 if ("predecessor".equals(codeString)) 111 return PREDECESSOR; 112 if ("successor".equals(codeString)) 113 return SUCCESSOR; 114 if ("derived-from".equals(codeString)) 115 return DERIVEDFROM; 116 if ("depends-on".equals(codeString)) 117 return DEPENDSON; 118 if ("composed-of".equals(codeString)) 119 return COMPOSEDOF; 120 if (Configuration.isAcceptInvalidEnums()) 121 return null; 122 else 123 throw new FHIRException("Unknown RelatedArtifactType code '" + codeString + "'"); 124 } 125 126 public String toCode() { 127 switch (this) { 128 case DOCUMENTATION: 129 return "documentation"; 130 case JUSTIFICATION: 131 return "justification"; 132 case CITATION: 133 return "citation"; 134 case PREDECESSOR: 135 return "predecessor"; 136 case SUCCESSOR: 137 return "successor"; 138 case DERIVEDFROM: 139 return "derived-from"; 140 case DEPENDSON: 141 return "depends-on"; 142 case COMPOSEDOF: 143 return "composed-of"; 144 case NULL: 145 return null; 146 default: 147 return "?"; 148 } 149 } 150 151 public String getSystem() { 152 switch (this) { 153 case DOCUMENTATION: 154 return "http://hl7.org/fhir/related-artifact-type"; 155 case JUSTIFICATION: 156 return "http://hl7.org/fhir/related-artifact-type"; 157 case CITATION: 158 return "http://hl7.org/fhir/related-artifact-type"; 159 case PREDECESSOR: 160 return "http://hl7.org/fhir/related-artifact-type"; 161 case SUCCESSOR: 162 return "http://hl7.org/fhir/related-artifact-type"; 163 case DERIVEDFROM: 164 return "http://hl7.org/fhir/related-artifact-type"; 165 case DEPENDSON: 166 return "http://hl7.org/fhir/related-artifact-type"; 167 case COMPOSEDOF: 168 return "http://hl7.org/fhir/related-artifact-type"; 169 case NULL: 170 return null; 171 default: 172 return "?"; 173 } 174 } 175 176 public String getDefinition() { 177 switch (this) { 178 case DOCUMENTATION: 179 return "Additional documentation for the knowledge resource. This would include additional instructions on usage as well as additional information on clinical context or appropriateness."; 180 case JUSTIFICATION: 181 return "A summary of the justification for the knowledge resource including supporting evidence, relevant guidelines, or other clinically important information. This information is intended to provide a way to make the justification for the knowledge resource available to the consumer of interventions or results produced by the knowledge resource."; 182 case CITATION: 183 return "Bibliographic citation for papers, references, or other relevant material for the knowledge resource. This is intended to allow for citation of related material, but that was not necessarily specifically prepared in connection with this knowledge resource."; 184 case PREDECESSOR: 185 return "The previous version of the knowledge resource."; 186 case SUCCESSOR: 187 return "The next version of the knowledge resource."; 188 case DERIVEDFROM: 189 return "The knowledge resource is derived from the related artifact. This is intended to capture the relationship in which a particular knowledge resource is based on the content of another artifact, but is modified to capture either a different set of overall requirements, or a more specific set of requirements such as those involved in a particular institution or clinical setting."; 190 case DEPENDSON: 191 return "The knowledge resource depends on the given related artifact."; 192 case COMPOSEDOF: 193 return "The knowledge resource is composed of the given related artifact."; 194 case NULL: 195 return null; 196 default: 197 return "?"; 198 } 199 } 200 201 public String getDisplay() { 202 switch (this) { 203 case DOCUMENTATION: 204 return "Documentation"; 205 case JUSTIFICATION: 206 return "Justification"; 207 case CITATION: 208 return "Citation"; 209 case PREDECESSOR: 210 return "Predecessor"; 211 case SUCCESSOR: 212 return "Successor"; 213 case DERIVEDFROM: 214 return "Derived From"; 215 case DEPENDSON: 216 return "Depends On"; 217 case COMPOSEDOF: 218 return "Composed Of"; 219 case NULL: 220 return null; 221 default: 222 return "?"; 223 } 224 } 225 } 226 227 public static class RelatedArtifactTypeEnumFactory implements EnumFactory<RelatedArtifactType> { 228 public RelatedArtifactType fromCode(String codeString) throws IllegalArgumentException { 229 if (codeString == null || "".equals(codeString)) 230 if (codeString == null || "".equals(codeString)) 231 return null; 232 if ("documentation".equals(codeString)) 233 return RelatedArtifactType.DOCUMENTATION; 234 if ("justification".equals(codeString)) 235 return RelatedArtifactType.JUSTIFICATION; 236 if ("citation".equals(codeString)) 237 return RelatedArtifactType.CITATION; 238 if ("predecessor".equals(codeString)) 239 return RelatedArtifactType.PREDECESSOR; 240 if ("successor".equals(codeString)) 241 return RelatedArtifactType.SUCCESSOR; 242 if ("derived-from".equals(codeString)) 243 return RelatedArtifactType.DERIVEDFROM; 244 if ("depends-on".equals(codeString)) 245 return RelatedArtifactType.DEPENDSON; 246 if ("composed-of".equals(codeString)) 247 return RelatedArtifactType.COMPOSEDOF; 248 throw new IllegalArgumentException("Unknown RelatedArtifactType code '" + codeString + "'"); 249 } 250 251 public Enumeration<RelatedArtifactType> fromType(PrimitiveType<?> code) throws FHIRException { 252 if (code == null) 253 return null; 254 if (code.isEmpty()) 255 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.NULL, code); 256 String codeString = code.asStringValue(); 257 if (codeString == null || "".equals(codeString)) 258 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.NULL, code); 259 if ("documentation".equals(codeString)) 260 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.DOCUMENTATION, code); 261 if ("justification".equals(codeString)) 262 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.JUSTIFICATION, code); 263 if ("citation".equals(codeString)) 264 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.CITATION, code); 265 if ("predecessor".equals(codeString)) 266 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.PREDECESSOR, code); 267 if ("successor".equals(codeString)) 268 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.SUCCESSOR, code); 269 if ("derived-from".equals(codeString)) 270 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.DERIVEDFROM, code); 271 if ("depends-on".equals(codeString)) 272 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.DEPENDSON, code); 273 if ("composed-of".equals(codeString)) 274 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.COMPOSEDOF, code); 275 throw new FHIRException("Unknown RelatedArtifactType code '" + codeString + "'"); 276 } 277 278 public String toCode(RelatedArtifactType code) { 279 if (code == RelatedArtifactType.NULL) 280 return null; 281 if (code == RelatedArtifactType.DOCUMENTATION) 282 return "documentation"; 283 if (code == RelatedArtifactType.JUSTIFICATION) 284 return "justification"; 285 if (code == RelatedArtifactType.CITATION) 286 return "citation"; 287 if (code == RelatedArtifactType.PREDECESSOR) 288 return "predecessor"; 289 if (code == RelatedArtifactType.SUCCESSOR) 290 return "successor"; 291 if (code == RelatedArtifactType.DERIVEDFROM) 292 return "derived-from"; 293 if (code == RelatedArtifactType.DEPENDSON) 294 return "depends-on"; 295 if (code == RelatedArtifactType.COMPOSEDOF) 296 return "composed-of"; 297 return "?"; 298 } 299 300 public String toSystem(RelatedArtifactType code) { 301 return code.getSystem(); 302 } 303 } 304 305 /** 306 * The type of relationship to the related artifact. 307 */ 308 @Child(name = "type", type = { CodeType.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 309 @Description(shortDefinition = "documentation | justification | citation | predecessor | successor | derived-from | depends-on | composed-of", formalDefinition = "The type of relationship to the related artifact.") 310 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/related-artifact-type") 311 protected Enumeration<RelatedArtifactType> type; 312 313 /** 314 * A short label that can be used to reference the citation from elsewhere in 315 * the containing artifact, such as a footnote index. 316 */ 317 @Child(name = "label", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 318 @Description(shortDefinition = "Short label", formalDefinition = "A short label that can be used to reference the citation from elsewhere in the containing artifact, such as a footnote index.") 319 protected StringType label; 320 321 /** 322 * A brief description of the document or knowledge resource being referenced, 323 * suitable for display to a consumer. 324 */ 325 @Child(name = "display", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 326 @Description(shortDefinition = "Brief description of the related artifact", formalDefinition = "A brief description of the document or knowledge resource being referenced, suitable for display to a consumer.") 327 protected StringType display; 328 329 /** 330 * A bibliographic citation for the related artifact. This text SHOULD be 331 * formatted according to an accepted citation format. 332 */ 333 @Child(name = "citation", type = { 334 MarkdownType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 335 @Description(shortDefinition = "Bibliographic citation for the artifact", formalDefinition = "A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format.") 336 protected MarkdownType citation; 337 338 /** 339 * A url for the artifact that can be followed to access the actual content. 340 */ 341 @Child(name = "url", type = { UrlType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 342 @Description(shortDefinition = "Where the artifact can be accessed", formalDefinition = "A url for the artifact that can be followed to access the actual content.") 343 protected UrlType url; 344 345 /** 346 * The document being referenced, represented as an attachment. This is 347 * exclusive with the resource element. 348 */ 349 @Child(name = "document", type = { Attachment.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 350 @Description(shortDefinition = "What document is being referenced", formalDefinition = "The document being referenced, represented as an attachment. This is exclusive with the resource element.") 351 protected Attachment document; 352 353 /** 354 * The related resource, such as a library, value set, profile, or other 355 * knowledge resource. 356 */ 357 @Child(name = "resource", type = { 358 CanonicalType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 359 @Description(shortDefinition = "What resource is being referenced", formalDefinition = "The related resource, such as a library, value set, profile, or other knowledge resource.") 360 protected CanonicalType resource; 361 362 private static final long serialVersionUID = -695743528L; 363 364 /** 365 * Constructor 366 */ 367 public RelatedArtifact() { 368 super(); 369 } 370 371 /** 372 * Constructor 373 */ 374 public RelatedArtifact(Enumeration<RelatedArtifactType> type) { 375 super(); 376 this.type = type; 377 } 378 379 /** 380 * @return {@link #type} (The type of relationship to the related artifact.). 381 * This is the underlying object with id, value and extensions. The 382 * accessor "getType" gives direct access to the value 383 */ 384 public Enumeration<RelatedArtifactType> getTypeElement() { 385 if (this.type == null) 386 if (Configuration.errorOnAutoCreate()) 387 throw new Error("Attempt to auto-create RelatedArtifact.type"); 388 else if (Configuration.doAutoCreate()) 389 this.type = new Enumeration<RelatedArtifactType>(new RelatedArtifactTypeEnumFactory()); // bb 390 return this.type; 391 } 392 393 public boolean hasTypeElement() { 394 return this.type != null && !this.type.isEmpty(); 395 } 396 397 public boolean hasType() { 398 return this.type != null && !this.type.isEmpty(); 399 } 400 401 /** 402 * @param value {@link #type} (The type of relationship to the related 403 * artifact.). This is the underlying object with id, value and 404 * extensions. The accessor "getType" gives direct access to the 405 * value 406 */ 407 public RelatedArtifact setTypeElement(Enumeration<RelatedArtifactType> value) { 408 this.type = value; 409 return this; 410 } 411 412 /** 413 * @return The type of relationship to the related artifact. 414 */ 415 public RelatedArtifactType getType() { 416 return this.type == null ? null : this.type.getValue(); 417 } 418 419 /** 420 * @param value The type of relationship to the related artifact. 421 */ 422 public RelatedArtifact setType(RelatedArtifactType value) { 423 if (this.type == null) 424 this.type = new Enumeration<RelatedArtifactType>(new RelatedArtifactTypeEnumFactory()); 425 this.type.setValue(value); 426 return this; 427 } 428 429 /** 430 * @return {@link #label} (A short label that can be used to reference the 431 * citation from elsewhere in the containing artifact, such as a 432 * footnote index.). This is the underlying object with id, value and 433 * extensions. The accessor "getLabel" gives direct access to the value 434 */ 435 public StringType getLabelElement() { 436 if (this.label == null) 437 if (Configuration.errorOnAutoCreate()) 438 throw new Error("Attempt to auto-create RelatedArtifact.label"); 439 else if (Configuration.doAutoCreate()) 440 this.label = new StringType(); // bb 441 return this.label; 442 } 443 444 public boolean hasLabelElement() { 445 return this.label != null && !this.label.isEmpty(); 446 } 447 448 public boolean hasLabel() { 449 return this.label != null && !this.label.isEmpty(); 450 } 451 452 /** 453 * @param value {@link #label} (A short label that can be used to reference the 454 * citation from elsewhere in the containing artifact, such as a 455 * footnote index.). This is the underlying object with id, value 456 * and extensions. The accessor "getLabel" gives direct access to 457 * the value 458 */ 459 public RelatedArtifact setLabelElement(StringType value) { 460 this.label = value; 461 return this; 462 } 463 464 /** 465 * @return A short label that can be used to reference the citation from 466 * elsewhere in the containing artifact, such as a footnote index. 467 */ 468 public String getLabel() { 469 return this.label == null ? null : this.label.getValue(); 470 } 471 472 /** 473 * @param value A short label that can be used to reference the citation from 474 * elsewhere in the containing artifact, such as a footnote index. 475 */ 476 public RelatedArtifact setLabel(String value) { 477 if (Utilities.noString(value)) 478 this.label = null; 479 else { 480 if (this.label == null) 481 this.label = new StringType(); 482 this.label.setValue(value); 483 } 484 return this; 485 } 486 487 /** 488 * @return {@link #display} (A brief description of the document or knowledge 489 * resource being referenced, suitable for display to a consumer.). This 490 * is the underlying object with id, value and extensions. The accessor 491 * "getDisplay" gives direct access to the value 492 */ 493 public StringType getDisplayElement() { 494 if (this.display == null) 495 if (Configuration.errorOnAutoCreate()) 496 throw new Error("Attempt to auto-create RelatedArtifact.display"); 497 else if (Configuration.doAutoCreate()) 498 this.display = new StringType(); // bb 499 return this.display; 500 } 501 502 public boolean hasDisplayElement() { 503 return this.display != null && !this.display.isEmpty(); 504 } 505 506 public boolean hasDisplay() { 507 return this.display != null && !this.display.isEmpty(); 508 } 509 510 /** 511 * @param value {@link #display} (A brief description of the document or 512 * knowledge resource being referenced, suitable for display to a 513 * consumer.). This is the underlying object with id, value and 514 * extensions. The accessor "getDisplay" gives direct access to the 515 * value 516 */ 517 public RelatedArtifact setDisplayElement(StringType value) { 518 this.display = value; 519 return this; 520 } 521 522 /** 523 * @return A brief description of the document or knowledge resource being 524 * referenced, suitable for display to a consumer. 525 */ 526 public String getDisplay() { 527 return this.display == null ? null : this.display.getValue(); 528 } 529 530 /** 531 * @param value A brief description of the document or knowledge resource being 532 * referenced, suitable for display to a consumer. 533 */ 534 public RelatedArtifact setDisplay(String value) { 535 if (Utilities.noString(value)) 536 this.display = null; 537 else { 538 if (this.display == null) 539 this.display = new StringType(); 540 this.display.setValue(value); 541 } 542 return this; 543 } 544 545 /** 546 * @return {@link #citation} (A bibliographic citation for the related artifact. 547 * This text SHOULD be formatted according to an accepted citation 548 * format.). This is the underlying object with id, value and 549 * extensions. The accessor "getCitation" gives direct access to the 550 * value 551 */ 552 public MarkdownType getCitationElement() { 553 if (this.citation == null) 554 if (Configuration.errorOnAutoCreate()) 555 throw new Error("Attempt to auto-create RelatedArtifact.citation"); 556 else if (Configuration.doAutoCreate()) 557 this.citation = new MarkdownType(); // bb 558 return this.citation; 559 } 560 561 public boolean hasCitationElement() { 562 return this.citation != null && !this.citation.isEmpty(); 563 } 564 565 public boolean hasCitation() { 566 return this.citation != null && !this.citation.isEmpty(); 567 } 568 569 /** 570 * @param value {@link #citation} (A bibliographic citation for the related 571 * artifact. This text SHOULD be formatted according to an accepted 572 * citation format.). This is the underlying object with id, value 573 * and extensions. The accessor "getCitation" gives direct access 574 * to the value 575 */ 576 public RelatedArtifact setCitationElement(MarkdownType value) { 577 this.citation = value; 578 return this; 579 } 580 581 /** 582 * @return A bibliographic citation for the related artifact. This text SHOULD 583 * be formatted according to an accepted citation format. 584 */ 585 public String getCitation() { 586 return this.citation == null ? null : this.citation.getValue(); 587 } 588 589 /** 590 * @param value A bibliographic citation for the related artifact. This text 591 * SHOULD be formatted according to an accepted citation format. 592 */ 593 public RelatedArtifact setCitation(String value) { 594 if (value == null) 595 this.citation = null; 596 else { 597 if (this.citation == null) 598 this.citation = new MarkdownType(); 599 this.citation.setValue(value); 600 } 601 return this; 602 } 603 604 /** 605 * @return {@link #url} (A url for the artifact that can be followed to access 606 * the actual content.). This is the underlying object with id, value 607 * and extensions. The accessor "getUrl" gives direct access to the 608 * value 609 */ 610 public UrlType getUrlElement() { 611 if (this.url == null) 612 if (Configuration.errorOnAutoCreate()) 613 throw new Error("Attempt to auto-create RelatedArtifact.url"); 614 else if (Configuration.doAutoCreate()) 615 this.url = new UrlType(); // bb 616 return this.url; 617 } 618 619 public boolean hasUrlElement() { 620 return this.url != null && !this.url.isEmpty(); 621 } 622 623 public boolean hasUrl() { 624 return this.url != null && !this.url.isEmpty(); 625 } 626 627 /** 628 * @param value {@link #url} (A url for the artifact that can be followed to 629 * access the actual content.). This is the underlying object with 630 * id, value and extensions. The accessor "getUrl" gives direct 631 * access to the value 632 */ 633 public RelatedArtifact setUrlElement(UrlType value) { 634 this.url = value; 635 return this; 636 } 637 638 /** 639 * @return A url for the artifact that can be followed to access the actual 640 * content. 641 */ 642 public String getUrl() { 643 return this.url == null ? null : this.url.getValue(); 644 } 645 646 /** 647 * @param value A url for the artifact that can be followed to access the actual 648 * content. 649 */ 650 public RelatedArtifact setUrl(String value) { 651 if (Utilities.noString(value)) 652 this.url = null; 653 else { 654 if (this.url == null) 655 this.url = new UrlType(); 656 this.url.setValue(value); 657 } 658 return this; 659 } 660 661 /** 662 * @return {@link #document} (The document being referenced, represented as an 663 * attachment. This is exclusive with the resource element.) 664 */ 665 public Attachment getDocument() { 666 if (this.document == null) 667 if (Configuration.errorOnAutoCreate()) 668 throw new Error("Attempt to auto-create RelatedArtifact.document"); 669 else if (Configuration.doAutoCreate()) 670 this.document = new Attachment(); // cc 671 return this.document; 672 } 673 674 public boolean hasDocument() { 675 return this.document != null && !this.document.isEmpty(); 676 } 677 678 /** 679 * @param value {@link #document} (The document being referenced, represented as 680 * an attachment. This is exclusive with the resource element.) 681 */ 682 public RelatedArtifact setDocument(Attachment value) { 683 this.document = value; 684 return this; 685 } 686 687 /** 688 * @return {@link #resource} (The related resource, such as a library, value 689 * set, profile, or other knowledge resource.). This is the underlying 690 * object with id, value and extensions. The accessor "getResource" 691 * gives direct access to the value 692 */ 693 public CanonicalType getResourceElement() { 694 if (this.resource == null) 695 if (Configuration.errorOnAutoCreate()) 696 throw new Error("Attempt to auto-create RelatedArtifact.resource"); 697 else if (Configuration.doAutoCreate()) 698 this.resource = new CanonicalType(); // bb 699 return this.resource; 700 } 701 702 public boolean hasResourceElement() { 703 return this.resource != null && !this.resource.isEmpty(); 704 } 705 706 public boolean hasResource() { 707 return this.resource != null && !this.resource.isEmpty(); 708 } 709 710 /** 711 * @param value {@link #resource} (The related resource, such as a library, 712 * value set, profile, or other knowledge resource.). This is the 713 * underlying object with id, value and extensions. The accessor 714 * "getResource" gives direct access to the value 715 */ 716 public RelatedArtifact setResourceElement(CanonicalType value) { 717 this.resource = value; 718 return this; 719 } 720 721 /** 722 * @return The related resource, such as a library, value set, profile, or other 723 * knowledge resource. 724 */ 725 public String getResource() { 726 return this.resource == null ? null : this.resource.getValue(); 727 } 728 729 /** 730 * @param value The related resource, such as a library, value set, profile, or 731 * other knowledge resource. 732 */ 733 public RelatedArtifact setResource(String value) { 734 if (Utilities.noString(value)) 735 this.resource = null; 736 else { 737 if (this.resource == null) 738 this.resource = new CanonicalType(); 739 this.resource.setValue(value); 740 } 741 return this; 742 } 743 744 protected void listChildren(List<Property> children) { 745 super.listChildren(children); 746 children.add(new Property("type", "code", "The type of relationship to the related artifact.", 0, 1, type)); 747 children.add(new Property("label", "string", 748 "A short label that can be used to reference the citation from elsewhere in the containing artifact, such as a footnote index.", 749 0, 1, label)); 750 children.add(new Property("display", "string", 751 "A brief description of the document or knowledge resource being referenced, suitable for display to a consumer.", 752 0, 1, display)); 753 children.add(new Property("citation", "markdown", 754 "A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format.", 755 0, 1, citation)); 756 children.add(new Property("url", "url", "A url for the artifact that can be followed to access the actual content.", 757 0, 1, url)); 758 children.add(new Property("document", "Attachment", 759 "The document being referenced, represented as an attachment. This is exclusive with the resource element.", 0, 760 1, document)); 761 children.add(new Property("resource", "canonical(Any)", 762 "The related resource, such as a library, value set, profile, or other knowledge resource.", 0, 1, resource)); 763 } 764 765 @Override 766 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 767 switch (_hash) { 768 case 3575610: 769 /* type */ return new Property("type", "code", "The type of relationship to the related artifact.", 0, 1, type); 770 case 102727412: 771 /* label */ return new Property("label", "string", 772 "A short label that can be used to reference the citation from elsewhere in the containing artifact, such as a footnote index.", 773 0, 1, label); 774 case 1671764162: 775 /* display */ return new Property("display", "string", 776 "A brief description of the document or knowledge resource being referenced, suitable for display to a consumer.", 777 0, 1, display); 778 case -1442706713: 779 /* citation */ return new Property("citation", "markdown", 780 "A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format.", 781 0, 1, citation); 782 case 116079: 783 /* url */ return new Property("url", "url", 784 "A url for the artifact that can be followed to access the actual content.", 0, 1, url); 785 case 861720859: 786 /* document */ return new Property("document", "Attachment", 787 "The document being referenced, represented as an attachment. This is exclusive with the resource element.", 788 0, 1, document); 789 case -341064690: 790 /* resource */ return new Property("resource", "canonical(Any)", 791 "The related resource, such as a library, value set, profile, or other knowledge resource.", 0, 1, resource); 792 default: 793 return super.getNamedProperty(_hash, _name, _checkValid); 794 } 795 796 } 797 798 @Override 799 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 800 switch (hash) { 801 case 3575610: 802 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<RelatedArtifactType> 803 case 102727412: 804 /* label */ return this.label == null ? new Base[0] : new Base[] { this.label }; // StringType 805 case 1671764162: 806 /* display */ return this.display == null ? new Base[0] : new Base[] { this.display }; // StringType 807 case -1442706713: 808 /* citation */ return this.citation == null ? new Base[0] : new Base[] { this.citation }; // MarkdownType 809 case 116079: 810 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UrlType 811 case 861720859: 812 /* document */ return this.document == null ? new Base[0] : new Base[] { this.document }; // Attachment 813 case -341064690: 814 /* resource */ return this.resource == null ? new Base[0] : new Base[] { this.resource }; // CanonicalType 815 default: 816 return super.getProperty(hash, name, checkValid); 817 } 818 819 } 820 821 @Override 822 public Base setProperty(int hash, String name, Base value) throws FHIRException { 823 switch (hash) { 824 case 3575610: // type 825 value = new RelatedArtifactTypeEnumFactory().fromType(castToCode(value)); 826 this.type = (Enumeration) value; // Enumeration<RelatedArtifactType> 827 return value; 828 case 102727412: // label 829 this.label = castToString(value); // StringType 830 return value; 831 case 1671764162: // display 832 this.display = castToString(value); // StringType 833 return value; 834 case -1442706713: // citation 835 this.citation = castToMarkdown(value); // MarkdownType 836 return value; 837 case 116079: // url 838 this.url = castToUrl(value); // UrlType 839 return value; 840 case 861720859: // document 841 this.document = castToAttachment(value); // Attachment 842 return value; 843 case -341064690: // resource 844 this.resource = castToCanonical(value); // CanonicalType 845 return value; 846 default: 847 return super.setProperty(hash, name, value); 848 } 849 850 } 851 852 @Override 853 public Base setProperty(String name, Base value) throws FHIRException { 854 if (name.equals("type")) { 855 value = new RelatedArtifactTypeEnumFactory().fromType(castToCode(value)); 856 this.type = (Enumeration) value; // Enumeration<RelatedArtifactType> 857 } else if (name.equals("label")) { 858 this.label = castToString(value); // StringType 859 } else if (name.equals("display")) { 860 this.display = castToString(value); // StringType 861 } else if (name.equals("citation")) { 862 this.citation = castToMarkdown(value); // MarkdownType 863 } else if (name.equals("url")) { 864 this.url = castToUrl(value); // UrlType 865 } else if (name.equals("document")) { 866 this.document = castToAttachment(value); // Attachment 867 } else if (name.equals("resource")) { 868 this.resource = castToCanonical(value); // CanonicalType 869 } else 870 return super.setProperty(name, value); 871 return value; 872 } 873 874 @Override 875 public void removeChild(String name, Base value) throws FHIRException { 876 if (name.equals("type")) { 877 this.type = null; 878 } else if (name.equals("label")) { 879 this.label = null; 880 } else if (name.equals("display")) { 881 this.display = null; 882 } else if (name.equals("citation")) { 883 this.citation = null; 884 } else if (name.equals("url")) { 885 this.url = null; 886 } else if (name.equals("document")) { 887 this.document = null; 888 } else if (name.equals("resource")) { 889 this.resource = null; 890 } else 891 super.removeChild(name, value); 892 893 } 894 895 @Override 896 public Base makeProperty(int hash, String name) throws FHIRException { 897 switch (hash) { 898 case 3575610: 899 return getTypeElement(); 900 case 102727412: 901 return getLabelElement(); 902 case 1671764162: 903 return getDisplayElement(); 904 case -1442706713: 905 return getCitationElement(); 906 case 116079: 907 return getUrlElement(); 908 case 861720859: 909 return getDocument(); 910 case -341064690: 911 return getResourceElement(); 912 default: 913 return super.makeProperty(hash, name); 914 } 915 916 } 917 918 @Override 919 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 920 switch (hash) { 921 case 3575610: 922 /* type */ return new String[] { "code" }; 923 case 102727412: 924 /* label */ return new String[] { "string" }; 925 case 1671764162: 926 /* display */ return new String[] { "string" }; 927 case -1442706713: 928 /* citation */ return new String[] { "markdown" }; 929 case 116079: 930 /* url */ return new String[] { "url" }; 931 case 861720859: 932 /* document */ return new String[] { "Attachment" }; 933 case -341064690: 934 /* resource */ return new String[] { "canonical" }; 935 default: 936 return super.getTypesForProperty(hash, name); 937 } 938 939 } 940 941 @Override 942 public Base addChild(String name) throws FHIRException { 943 if (name.equals("type")) { 944 throw new FHIRException("Cannot call addChild on a singleton property RelatedArtifact.type"); 945 } else if (name.equals("label")) { 946 throw new FHIRException("Cannot call addChild on a singleton property RelatedArtifact.label"); 947 } else if (name.equals("display")) { 948 throw new FHIRException("Cannot call addChild on a singleton property RelatedArtifact.display"); 949 } else if (name.equals("citation")) { 950 throw new FHIRException("Cannot call addChild on a singleton property RelatedArtifact.citation"); 951 } else if (name.equals("url")) { 952 throw new FHIRException("Cannot call addChild on a singleton property RelatedArtifact.url"); 953 } else if (name.equals("document")) { 954 this.document = new Attachment(); 955 return this.document; 956 } else if (name.equals("resource")) { 957 throw new FHIRException("Cannot call addChild on a singleton property RelatedArtifact.resource"); 958 } else 959 return super.addChild(name); 960 } 961 962 public String fhirType() { 963 return "RelatedArtifact"; 964 965 } 966 967 public RelatedArtifact copy() { 968 RelatedArtifact dst = new RelatedArtifact(); 969 copyValues(dst); 970 return dst; 971 } 972 973 public void copyValues(RelatedArtifact dst) { 974 super.copyValues(dst); 975 dst.type = type == null ? null : type.copy(); 976 dst.label = label == null ? null : label.copy(); 977 dst.display = display == null ? null : display.copy(); 978 dst.citation = citation == null ? null : citation.copy(); 979 dst.url = url == null ? null : url.copy(); 980 dst.document = document == null ? null : document.copy(); 981 dst.resource = resource == null ? null : resource.copy(); 982 } 983 984 protected RelatedArtifact typedCopy() { 985 return copy(); 986 } 987 988 @Override 989 public boolean equalsDeep(Base other_) { 990 if (!super.equalsDeep(other_)) 991 return false; 992 if (!(other_ instanceof RelatedArtifact)) 993 return false; 994 RelatedArtifact o = (RelatedArtifact) other_; 995 return compareDeep(type, o.type, true) && compareDeep(label, o.label, true) && compareDeep(display, o.display, true) 996 && compareDeep(citation, o.citation, true) && compareDeep(url, o.url, true) 997 && compareDeep(document, o.document, true) && compareDeep(resource, o.resource, true); 998 } 999 1000 @Override 1001 public boolean equalsShallow(Base other_) { 1002 if (!super.equalsShallow(other_)) 1003 return false; 1004 if (!(other_ instanceof RelatedArtifact)) 1005 return false; 1006 RelatedArtifact o = (RelatedArtifact) other_; 1007 return compareValues(type, o.type, true) && compareValues(label, o.label, true) 1008 && compareValues(display, o.display, true) && compareValues(citation, o.citation, true) 1009 && compareValues(url, o.url, true); 1010 } 1011 1012 public boolean isEmpty() { 1013 return super.isEmpty() 1014 && ca.uhn.fhir.util.ElementUtil.isEmpty(type, label, display, citation, url, document, resource); 1015 } 1016 1017}