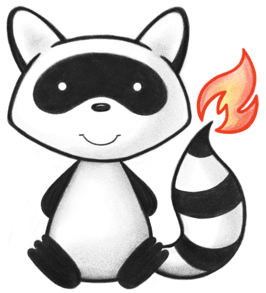
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.AdministrativeGender; 040import org.hl7.fhir.r4.model.Enumerations.AdministrativeGenderEnumFactory; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047 048/** 049 * Information about a person that is involved in the care for a patient, but 050 * who is not the target of healthcare, nor has a formal responsibility in the 051 * care process. 052 */ 053@ResourceDef(name = "RelatedPerson", profile = "http://hl7.org/fhir/StructureDefinition/RelatedPerson") 054public class RelatedPerson extends DomainResource { 055 056 @Block() 057 public static class RelatedPersonCommunicationComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * The ISO-639-1 alpha 2 code in lower case for the language, optionally 060 * followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper 061 * case; e.g. "en" for English, or "en-US" for American English versus "en-EN" 062 * for England English. 063 */ 064 @Child(name = "language", type = { 065 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 066 @Description(shortDefinition = "The language which can be used to communicate with the patient about his or her health", formalDefinition = "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-EN\" for England English.") 067 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/languages") 068 protected CodeableConcept language; 069 070 /** 071 * Indicates whether or not the patient prefers this language (over other 072 * languages he masters up a certain level). 073 */ 074 @Child(name = "preferred", type = { 075 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 076 @Description(shortDefinition = "Language preference indicator", formalDefinition = "Indicates whether or not the patient prefers this language (over other languages he masters up a certain level).") 077 protected BooleanType preferred; 078 079 private static final long serialVersionUID = 633792918L; 080 081 /** 082 * Constructor 083 */ 084 public RelatedPersonCommunicationComponent() { 085 super(); 086 } 087 088 /** 089 * Constructor 090 */ 091 public RelatedPersonCommunicationComponent(CodeableConcept language) { 092 super(); 093 this.language = language; 094 } 095 096 /** 097 * @return {@link #language} (The ISO-639-1 alpha 2 code in lower case for the 098 * language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 099 * code for the region in upper case; e.g. "en" for English, or "en-US" 100 * for American English versus "en-EN" for England English.) 101 */ 102 public CodeableConcept getLanguage() { 103 if (this.language == null) 104 if (Configuration.errorOnAutoCreate()) 105 throw new Error("Attempt to auto-create RelatedPersonCommunicationComponent.language"); 106 else if (Configuration.doAutoCreate()) 107 this.language = new CodeableConcept(); // cc 108 return this.language; 109 } 110 111 public boolean hasLanguage() { 112 return this.language != null && !this.language.isEmpty(); 113 } 114 115 /** 116 * @param value {@link #language} (The ISO-639-1 alpha 2 code in lower case for 117 * the language, optionally followed by a hyphen and the ISO-3166-1 118 * alpha 2 code for the region in upper case; e.g. "en" for 119 * English, or "en-US" for American English versus "en-EN" for 120 * England English.) 121 */ 122 public RelatedPersonCommunicationComponent setLanguage(CodeableConcept value) { 123 this.language = value; 124 return this; 125 } 126 127 /** 128 * @return {@link #preferred} (Indicates whether or not the patient prefers this 129 * language (over other languages he masters up a certain level).). This 130 * is the underlying object with id, value and extensions. The accessor 131 * "getPreferred" gives direct access to the value 132 */ 133 public BooleanType getPreferredElement() { 134 if (this.preferred == null) 135 if (Configuration.errorOnAutoCreate()) 136 throw new Error("Attempt to auto-create RelatedPersonCommunicationComponent.preferred"); 137 else if (Configuration.doAutoCreate()) 138 this.preferred = new BooleanType(); // bb 139 return this.preferred; 140 } 141 142 public boolean hasPreferredElement() { 143 return this.preferred != null && !this.preferred.isEmpty(); 144 } 145 146 public boolean hasPreferred() { 147 return this.preferred != null && !this.preferred.isEmpty(); 148 } 149 150 /** 151 * @param value {@link #preferred} (Indicates whether or not the patient prefers 152 * this language (over other languages he masters up a certain 153 * level).). This is the underlying object with id, value and 154 * extensions. The accessor "getPreferred" gives direct access to 155 * the value 156 */ 157 public RelatedPersonCommunicationComponent setPreferredElement(BooleanType value) { 158 this.preferred = value; 159 return this; 160 } 161 162 /** 163 * @return Indicates whether or not the patient prefers this language (over 164 * other languages he masters up a certain level). 165 */ 166 public boolean getPreferred() { 167 return this.preferred == null || this.preferred.isEmpty() ? false : this.preferred.getValue(); 168 } 169 170 /** 171 * @param value Indicates whether or not the patient prefers this language (over 172 * other languages he masters up a certain level). 173 */ 174 public RelatedPersonCommunicationComponent setPreferred(boolean value) { 175 if (this.preferred == null) 176 this.preferred = new BooleanType(); 177 this.preferred.setValue(value); 178 return this; 179 } 180 181 protected void listChildren(List<Property> children) { 182 super.listChildren(children); 183 children.add(new Property("language", "CodeableConcept", 184 "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-EN\" for England English.", 185 0, 1, language)); 186 children.add(new Property("preferred", "boolean", 187 "Indicates whether or not the patient prefers this language (over other languages he masters up a certain level).", 188 0, 1, preferred)); 189 } 190 191 @Override 192 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 193 switch (_hash) { 194 case -1613589672: 195 /* language */ return new Property("language", "CodeableConcept", 196 "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-EN\" for England English.", 197 0, 1, language); 198 case -1294005119: 199 /* preferred */ return new Property("preferred", "boolean", 200 "Indicates whether or not the patient prefers this language (over other languages he masters up a certain level).", 201 0, 1, preferred); 202 default: 203 return super.getNamedProperty(_hash, _name, _checkValid); 204 } 205 206 } 207 208 @Override 209 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 210 switch (hash) { 211 case -1613589672: 212 /* language */ return this.language == null ? new Base[0] : new Base[] { this.language }; // CodeableConcept 213 case -1294005119: 214 /* preferred */ return this.preferred == null ? new Base[0] : new Base[] { this.preferred }; // BooleanType 215 default: 216 return super.getProperty(hash, name, checkValid); 217 } 218 219 } 220 221 @Override 222 public Base setProperty(int hash, String name, Base value) throws FHIRException { 223 switch (hash) { 224 case -1613589672: // language 225 this.language = castToCodeableConcept(value); // CodeableConcept 226 return value; 227 case -1294005119: // preferred 228 this.preferred = castToBoolean(value); // BooleanType 229 return value; 230 default: 231 return super.setProperty(hash, name, value); 232 } 233 234 } 235 236 @Override 237 public Base setProperty(String name, Base value) throws FHIRException { 238 if (name.equals("language")) { 239 this.language = castToCodeableConcept(value); // CodeableConcept 240 } else if (name.equals("preferred")) { 241 this.preferred = castToBoolean(value); // BooleanType 242 } else 243 return super.setProperty(name, value); 244 return value; 245 } 246 247 @Override 248 public void removeChild(String name, Base value) throws FHIRException { 249 if (name.equals("language")) { 250 this.language = null; 251 } else if (name.equals("preferred")) { 252 this.preferred = null; 253 } else 254 super.removeChild(name, value); 255 256 } 257 258 @Override 259 public Base makeProperty(int hash, String name) throws FHIRException { 260 switch (hash) { 261 case -1613589672: 262 return getLanguage(); 263 case -1294005119: 264 return getPreferredElement(); 265 default: 266 return super.makeProperty(hash, name); 267 } 268 269 } 270 271 @Override 272 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 273 switch (hash) { 274 case -1613589672: 275 /* language */ return new String[] { "CodeableConcept" }; 276 case -1294005119: 277 /* preferred */ return new String[] { "boolean" }; 278 default: 279 return super.getTypesForProperty(hash, name); 280 } 281 282 } 283 284 @Override 285 public Base addChild(String name) throws FHIRException { 286 if (name.equals("language")) { 287 this.language = new CodeableConcept(); 288 return this.language; 289 } else if (name.equals("preferred")) { 290 throw new FHIRException("Cannot call addChild on a singleton property RelatedPerson.preferred"); 291 } else 292 return super.addChild(name); 293 } 294 295 public RelatedPersonCommunicationComponent copy() { 296 RelatedPersonCommunicationComponent dst = new RelatedPersonCommunicationComponent(); 297 copyValues(dst); 298 return dst; 299 } 300 301 public void copyValues(RelatedPersonCommunicationComponent dst) { 302 super.copyValues(dst); 303 dst.language = language == null ? null : language.copy(); 304 dst.preferred = preferred == null ? null : preferred.copy(); 305 } 306 307 @Override 308 public boolean equalsDeep(Base other_) { 309 if (!super.equalsDeep(other_)) 310 return false; 311 if (!(other_ instanceof RelatedPersonCommunicationComponent)) 312 return false; 313 RelatedPersonCommunicationComponent o = (RelatedPersonCommunicationComponent) other_; 314 return compareDeep(language, o.language, true) && compareDeep(preferred, o.preferred, true); 315 } 316 317 @Override 318 public boolean equalsShallow(Base other_) { 319 if (!super.equalsShallow(other_)) 320 return false; 321 if (!(other_ instanceof RelatedPersonCommunicationComponent)) 322 return false; 323 RelatedPersonCommunicationComponent o = (RelatedPersonCommunicationComponent) other_; 324 return compareValues(preferred, o.preferred, true); 325 } 326 327 public boolean isEmpty() { 328 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(language, preferred); 329 } 330 331 public String fhirType() { 332 return "RelatedPerson.communication"; 333 334 } 335 336 } 337 338 /** 339 * Identifier for a person within a particular scope. 340 */ 341 @Child(name = "identifier", type = { 342 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 343 @Description(shortDefinition = "A human identifier for this person", formalDefinition = "Identifier for a person within a particular scope.") 344 protected List<Identifier> identifier; 345 346 /** 347 * Whether this related person record is in active use. 348 */ 349 @Child(name = "active", type = { BooleanType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 350 @Description(shortDefinition = "Whether this related person's record is in active use", formalDefinition = "Whether this related person record is in active use.") 351 protected BooleanType active; 352 353 /** 354 * The patient this person is related to. 355 */ 356 @Child(name = "patient", type = { Patient.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 357 @Description(shortDefinition = "The patient this person is related to", formalDefinition = "The patient this person is related to.") 358 protected Reference patient; 359 360 /** 361 * The actual object that is the target of the reference (The patient this 362 * person is related to.) 363 */ 364 protected Patient patientTarget; 365 366 /** 367 * The nature of the relationship between a patient and the related person. 368 */ 369 @Child(name = "relationship", type = { 370 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 371 @Description(shortDefinition = "The nature of the relationship", formalDefinition = "The nature of the relationship between a patient and the related person.") 372 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/relatedperson-relationshiptype") 373 protected List<CodeableConcept> relationship; 374 375 /** 376 * A name associated with the person. 377 */ 378 @Child(name = "name", type = { 379 HumanName.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 380 @Description(shortDefinition = "A name associated with the person", formalDefinition = "A name associated with the person.") 381 protected List<HumanName> name; 382 383 /** 384 * A contact detail for the person, e.g. a telephone number or an email address. 385 */ 386 @Child(name = "telecom", type = { 387 ContactPoint.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 388 @Description(shortDefinition = "A contact detail for the person", formalDefinition = "A contact detail for the person, e.g. a telephone number or an email address.") 389 protected List<ContactPoint> telecom; 390 391 /** 392 * Administrative Gender - the gender that the person is considered to have for 393 * administration and record keeping purposes. 394 */ 395 @Child(name = "gender", type = { CodeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 396 @Description(shortDefinition = "male | female | other | unknown", formalDefinition = "Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes.") 397 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/administrative-gender") 398 protected Enumeration<AdministrativeGender> gender; 399 400 /** 401 * The date on which the related person was born. 402 */ 403 @Child(name = "birthDate", type = { DateType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 404 @Description(shortDefinition = "The date on which the related person was born", formalDefinition = "The date on which the related person was born.") 405 protected DateType birthDate; 406 407 /** 408 * Address where the related person can be contacted or visited. 409 */ 410 @Child(name = "address", type = { 411 Address.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 412 @Description(shortDefinition = "Address where the related person can be contacted or visited", formalDefinition = "Address where the related person can be contacted or visited.") 413 protected List<Address> address; 414 415 /** 416 * Image of the person. 417 */ 418 @Child(name = "photo", type = { 419 Attachment.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 420 @Description(shortDefinition = "Image of the person", formalDefinition = "Image of the person.") 421 protected List<Attachment> photo; 422 423 /** 424 * The period of time during which this relationship is or was active. If there 425 * are no dates defined, then the interval is unknown. 426 */ 427 @Child(name = "period", type = { Period.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 428 @Description(shortDefinition = "Period of time that this relationship is considered valid", formalDefinition = "The period of time during which this relationship is or was active. If there are no dates defined, then the interval is unknown.") 429 protected Period period; 430 431 /** 432 * A language which may be used to communicate with about the patient's health. 433 */ 434 @Child(name = "communication", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 435 @Description(shortDefinition = "A language which may be used to communicate with about the patient's health", formalDefinition = "A language which may be used to communicate with about the patient's health.") 436 protected List<RelatedPersonCommunicationComponent> communication; 437 438 private static final long serialVersionUID = -1396330390L; 439 440 /** 441 * Constructor 442 */ 443 public RelatedPerson() { 444 super(); 445 } 446 447 /** 448 * Constructor 449 */ 450 public RelatedPerson(Reference patient) { 451 super(); 452 this.patient = patient; 453 } 454 455 /** 456 * @return {@link #identifier} (Identifier for a person within a particular 457 * scope.) 458 */ 459 public List<Identifier> getIdentifier() { 460 if (this.identifier == null) 461 this.identifier = new ArrayList<Identifier>(); 462 return this.identifier; 463 } 464 465 /** 466 * @return Returns a reference to <code>this</code> for easy method chaining 467 */ 468 public RelatedPerson setIdentifier(List<Identifier> theIdentifier) { 469 this.identifier = theIdentifier; 470 return this; 471 } 472 473 public boolean hasIdentifier() { 474 if (this.identifier == null) 475 return false; 476 for (Identifier item : this.identifier) 477 if (!item.isEmpty()) 478 return true; 479 return false; 480 } 481 482 public Identifier addIdentifier() { // 3 483 Identifier t = new Identifier(); 484 if (this.identifier == null) 485 this.identifier = new ArrayList<Identifier>(); 486 this.identifier.add(t); 487 return t; 488 } 489 490 public RelatedPerson addIdentifier(Identifier t) { // 3 491 if (t == null) 492 return this; 493 if (this.identifier == null) 494 this.identifier = new ArrayList<Identifier>(); 495 this.identifier.add(t); 496 return this; 497 } 498 499 /** 500 * @return The first repetition of repeating field {@link #identifier}, creating 501 * it if it does not already exist 502 */ 503 public Identifier getIdentifierFirstRep() { 504 if (getIdentifier().isEmpty()) { 505 addIdentifier(); 506 } 507 return getIdentifier().get(0); 508 } 509 510 /** 511 * @return {@link #active} (Whether this related person record is in active 512 * use.). This is the underlying object with id, value and extensions. 513 * The accessor "getActive" gives direct access to the value 514 */ 515 public BooleanType getActiveElement() { 516 if (this.active == null) 517 if (Configuration.errorOnAutoCreate()) 518 throw new Error("Attempt to auto-create RelatedPerson.active"); 519 else if (Configuration.doAutoCreate()) 520 this.active = new BooleanType(); // bb 521 return this.active; 522 } 523 524 public boolean hasActiveElement() { 525 return this.active != null && !this.active.isEmpty(); 526 } 527 528 public boolean hasActive() { 529 return this.active != null && !this.active.isEmpty(); 530 } 531 532 /** 533 * @param value {@link #active} (Whether this related person record is in active 534 * use.). This is the underlying object with id, value and 535 * extensions. The accessor "getActive" gives direct access to the 536 * value 537 */ 538 public RelatedPerson setActiveElement(BooleanType value) { 539 this.active = value; 540 return this; 541 } 542 543 /** 544 * @return Whether this related person record is in active use. 545 */ 546 public boolean getActive() { 547 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 548 } 549 550 /** 551 * @param value Whether this related person record is in active use. 552 */ 553 public RelatedPerson setActive(boolean value) { 554 if (this.active == null) 555 this.active = new BooleanType(); 556 this.active.setValue(value); 557 return this; 558 } 559 560 /** 561 * @return {@link #patient} (The patient this person is related to.) 562 */ 563 public Reference getPatient() { 564 if (this.patient == null) 565 if (Configuration.errorOnAutoCreate()) 566 throw new Error("Attempt to auto-create RelatedPerson.patient"); 567 else if (Configuration.doAutoCreate()) 568 this.patient = new Reference(); // cc 569 return this.patient; 570 } 571 572 public boolean hasPatient() { 573 return this.patient != null && !this.patient.isEmpty(); 574 } 575 576 /** 577 * @param value {@link #patient} (The patient this person is related to.) 578 */ 579 public RelatedPerson setPatient(Reference value) { 580 this.patient = value; 581 return this; 582 } 583 584 /** 585 * @return {@link #patient} The actual object that is the target of the 586 * reference. The reference library doesn't populate this, but you can 587 * use it to hold the resource if you resolve it. (The patient this 588 * person is related to.) 589 */ 590 public Patient getPatientTarget() { 591 if (this.patientTarget == null) 592 if (Configuration.errorOnAutoCreate()) 593 throw new Error("Attempt to auto-create RelatedPerson.patient"); 594 else if (Configuration.doAutoCreate()) 595 this.patientTarget = new Patient(); // aa 596 return this.patientTarget; 597 } 598 599 /** 600 * @param value {@link #patient} The actual object that is the target of the 601 * reference. The reference library doesn't use these, but you can 602 * use it to hold the resource if you resolve it. (The patient this 603 * person is related to.) 604 */ 605 public RelatedPerson setPatientTarget(Patient value) { 606 this.patientTarget = value; 607 return this; 608 } 609 610 /** 611 * @return {@link #relationship} (The nature of the relationship between a 612 * patient and the related person.) 613 */ 614 public List<CodeableConcept> getRelationship() { 615 if (this.relationship == null) 616 this.relationship = new ArrayList<CodeableConcept>(); 617 return this.relationship; 618 } 619 620 /** 621 * @return Returns a reference to <code>this</code> for easy method chaining 622 */ 623 public RelatedPerson setRelationship(List<CodeableConcept> theRelationship) { 624 this.relationship = theRelationship; 625 return this; 626 } 627 628 public boolean hasRelationship() { 629 if (this.relationship == null) 630 return false; 631 for (CodeableConcept item : this.relationship) 632 if (!item.isEmpty()) 633 return true; 634 return false; 635 } 636 637 public CodeableConcept addRelationship() { // 3 638 CodeableConcept t = new CodeableConcept(); 639 if (this.relationship == null) 640 this.relationship = new ArrayList<CodeableConcept>(); 641 this.relationship.add(t); 642 return t; 643 } 644 645 public RelatedPerson addRelationship(CodeableConcept t) { // 3 646 if (t == null) 647 return this; 648 if (this.relationship == null) 649 this.relationship = new ArrayList<CodeableConcept>(); 650 this.relationship.add(t); 651 return this; 652 } 653 654 /** 655 * @return The first repetition of repeating field {@link #relationship}, 656 * creating it if it does not already exist 657 */ 658 public CodeableConcept getRelationshipFirstRep() { 659 if (getRelationship().isEmpty()) { 660 addRelationship(); 661 } 662 return getRelationship().get(0); 663 } 664 665 /** 666 * @return {@link #name} (A name associated with the person.) 667 */ 668 public List<HumanName> getName() { 669 if (this.name == null) 670 this.name = new ArrayList<HumanName>(); 671 return this.name; 672 } 673 674 /** 675 * @return Returns a reference to <code>this</code> for easy method chaining 676 */ 677 public RelatedPerson setName(List<HumanName> theName) { 678 this.name = theName; 679 return this; 680 } 681 682 public boolean hasName() { 683 if (this.name == null) 684 return false; 685 for (HumanName item : this.name) 686 if (!item.isEmpty()) 687 return true; 688 return false; 689 } 690 691 public HumanName addName() { // 3 692 HumanName t = new HumanName(); 693 if (this.name == null) 694 this.name = new ArrayList<HumanName>(); 695 this.name.add(t); 696 return t; 697 } 698 699 public RelatedPerson addName(HumanName t) { // 3 700 if (t == null) 701 return this; 702 if (this.name == null) 703 this.name = new ArrayList<HumanName>(); 704 this.name.add(t); 705 return this; 706 } 707 708 /** 709 * @return The first repetition of repeating field {@link #name}, creating it if 710 * it does not already exist 711 */ 712 public HumanName getNameFirstRep() { 713 if (getName().isEmpty()) { 714 addName(); 715 } 716 return getName().get(0); 717 } 718 719 /** 720 * @return {@link #telecom} (A contact detail for the person, e.g. a telephone 721 * number or an email address.) 722 */ 723 public List<ContactPoint> getTelecom() { 724 if (this.telecom == null) 725 this.telecom = new ArrayList<ContactPoint>(); 726 return this.telecom; 727 } 728 729 /** 730 * @return Returns a reference to <code>this</code> for easy method chaining 731 */ 732 public RelatedPerson setTelecom(List<ContactPoint> theTelecom) { 733 this.telecom = theTelecom; 734 return this; 735 } 736 737 public boolean hasTelecom() { 738 if (this.telecom == null) 739 return false; 740 for (ContactPoint item : this.telecom) 741 if (!item.isEmpty()) 742 return true; 743 return false; 744 } 745 746 public ContactPoint addTelecom() { // 3 747 ContactPoint t = new ContactPoint(); 748 if (this.telecom == null) 749 this.telecom = new ArrayList<ContactPoint>(); 750 this.telecom.add(t); 751 return t; 752 } 753 754 public RelatedPerson addTelecom(ContactPoint t) { // 3 755 if (t == null) 756 return this; 757 if (this.telecom == null) 758 this.telecom = new ArrayList<ContactPoint>(); 759 this.telecom.add(t); 760 return this; 761 } 762 763 /** 764 * @return The first repetition of repeating field {@link #telecom}, creating it 765 * if it does not already exist 766 */ 767 public ContactPoint getTelecomFirstRep() { 768 if (getTelecom().isEmpty()) { 769 addTelecom(); 770 } 771 return getTelecom().get(0); 772 } 773 774 /** 775 * @return {@link #gender} (Administrative Gender - the gender that the person 776 * is considered to have for administration and record keeping 777 * purposes.). This is the underlying object with id, value and 778 * extensions. The accessor "getGender" gives direct access to the value 779 */ 780 public Enumeration<AdministrativeGender> getGenderElement() { 781 if (this.gender == null) 782 if (Configuration.errorOnAutoCreate()) 783 throw new Error("Attempt to auto-create RelatedPerson.gender"); 784 else if (Configuration.doAutoCreate()) 785 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 786 return this.gender; 787 } 788 789 public boolean hasGenderElement() { 790 return this.gender != null && !this.gender.isEmpty(); 791 } 792 793 public boolean hasGender() { 794 return this.gender != null && !this.gender.isEmpty(); 795 } 796 797 /** 798 * @param value {@link #gender} (Administrative Gender - the gender that the 799 * person is considered to have for administration and record 800 * keeping purposes.). This is the underlying object with id, value 801 * and extensions. The accessor "getGender" gives direct access to 802 * the value 803 */ 804 public RelatedPerson setGenderElement(Enumeration<AdministrativeGender> value) { 805 this.gender = value; 806 return this; 807 } 808 809 /** 810 * @return Administrative Gender - the gender that the person is considered to 811 * have for administration and record keeping purposes. 812 */ 813 public AdministrativeGender getGender() { 814 return this.gender == null ? null : this.gender.getValue(); 815 } 816 817 /** 818 * @param value Administrative Gender - the gender that the person is considered 819 * to have for administration and record keeping purposes. 820 */ 821 public RelatedPerson setGender(AdministrativeGender value) { 822 if (value == null) 823 this.gender = null; 824 else { 825 if (this.gender == null) 826 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 827 this.gender.setValue(value); 828 } 829 return this; 830 } 831 832 /** 833 * @return {@link #birthDate} (The date on which the related person was born.). 834 * This is the underlying object with id, value and extensions. The 835 * accessor "getBirthDate" gives direct access to the value 836 */ 837 public DateType getBirthDateElement() { 838 if (this.birthDate == null) 839 if (Configuration.errorOnAutoCreate()) 840 throw new Error("Attempt to auto-create RelatedPerson.birthDate"); 841 else if (Configuration.doAutoCreate()) 842 this.birthDate = new DateType(); // bb 843 return this.birthDate; 844 } 845 846 public boolean hasBirthDateElement() { 847 return this.birthDate != null && !this.birthDate.isEmpty(); 848 } 849 850 public boolean hasBirthDate() { 851 return this.birthDate != null && !this.birthDate.isEmpty(); 852 } 853 854 /** 855 * @param value {@link #birthDate} (The date on which the related person was 856 * born.). This is the underlying object with id, value and 857 * extensions. The accessor "getBirthDate" gives direct access to 858 * the value 859 */ 860 public RelatedPerson setBirthDateElement(DateType value) { 861 this.birthDate = value; 862 return this; 863 } 864 865 /** 866 * @return The date on which the related person was born. 867 */ 868 public Date getBirthDate() { 869 return this.birthDate == null ? null : this.birthDate.getValue(); 870 } 871 872 /** 873 * @param value The date on which the related person was born. 874 */ 875 public RelatedPerson setBirthDate(Date value) { 876 if (value == null) 877 this.birthDate = null; 878 else { 879 if (this.birthDate == null) 880 this.birthDate = new DateType(); 881 this.birthDate.setValue(value); 882 } 883 return this; 884 } 885 886 /** 887 * @return {@link #address} (Address where the related person can be contacted 888 * or visited.) 889 */ 890 public List<Address> getAddress() { 891 if (this.address == null) 892 this.address = new ArrayList<Address>(); 893 return this.address; 894 } 895 896 /** 897 * @return Returns a reference to <code>this</code> for easy method chaining 898 */ 899 public RelatedPerson setAddress(List<Address> theAddress) { 900 this.address = theAddress; 901 return this; 902 } 903 904 public boolean hasAddress() { 905 if (this.address == null) 906 return false; 907 for (Address item : this.address) 908 if (!item.isEmpty()) 909 return true; 910 return false; 911 } 912 913 public Address addAddress() { // 3 914 Address t = new Address(); 915 if (this.address == null) 916 this.address = new ArrayList<Address>(); 917 this.address.add(t); 918 return t; 919 } 920 921 public RelatedPerson addAddress(Address t) { // 3 922 if (t == null) 923 return this; 924 if (this.address == null) 925 this.address = new ArrayList<Address>(); 926 this.address.add(t); 927 return this; 928 } 929 930 /** 931 * @return The first repetition of repeating field {@link #address}, creating it 932 * if it does not already exist 933 */ 934 public Address getAddressFirstRep() { 935 if (getAddress().isEmpty()) { 936 addAddress(); 937 } 938 return getAddress().get(0); 939 } 940 941 /** 942 * @return {@link #photo} (Image of the person.) 943 */ 944 public List<Attachment> getPhoto() { 945 if (this.photo == null) 946 this.photo = new ArrayList<Attachment>(); 947 return this.photo; 948 } 949 950 /** 951 * @return Returns a reference to <code>this</code> for easy method chaining 952 */ 953 public RelatedPerson setPhoto(List<Attachment> thePhoto) { 954 this.photo = thePhoto; 955 return this; 956 } 957 958 public boolean hasPhoto() { 959 if (this.photo == null) 960 return false; 961 for (Attachment item : this.photo) 962 if (!item.isEmpty()) 963 return true; 964 return false; 965 } 966 967 public Attachment addPhoto() { // 3 968 Attachment t = new Attachment(); 969 if (this.photo == null) 970 this.photo = new ArrayList<Attachment>(); 971 this.photo.add(t); 972 return t; 973 } 974 975 public RelatedPerson addPhoto(Attachment t) { // 3 976 if (t == null) 977 return this; 978 if (this.photo == null) 979 this.photo = new ArrayList<Attachment>(); 980 this.photo.add(t); 981 return this; 982 } 983 984 /** 985 * @return The first repetition of repeating field {@link #photo}, creating it 986 * if it does not already exist 987 */ 988 public Attachment getPhotoFirstRep() { 989 if (getPhoto().isEmpty()) { 990 addPhoto(); 991 } 992 return getPhoto().get(0); 993 } 994 995 /** 996 * @return {@link #period} (The period of time during which this relationship is 997 * or was active. If there are no dates defined, then the interval is 998 * unknown.) 999 */ 1000 public Period getPeriod() { 1001 if (this.period == null) 1002 if (Configuration.errorOnAutoCreate()) 1003 throw new Error("Attempt to auto-create RelatedPerson.period"); 1004 else if (Configuration.doAutoCreate()) 1005 this.period = new Period(); // cc 1006 return this.period; 1007 } 1008 1009 public boolean hasPeriod() { 1010 return this.period != null && !this.period.isEmpty(); 1011 } 1012 1013 /** 1014 * @param value {@link #period} (The period of time during which this 1015 * relationship is or was active. If there are no dates defined, 1016 * then the interval is unknown.) 1017 */ 1018 public RelatedPerson setPeriod(Period value) { 1019 this.period = value; 1020 return this; 1021 } 1022 1023 /** 1024 * @return {@link #communication} (A language which may be used to communicate 1025 * with about the patient's health.) 1026 */ 1027 public List<RelatedPersonCommunicationComponent> getCommunication() { 1028 if (this.communication == null) 1029 this.communication = new ArrayList<RelatedPersonCommunicationComponent>(); 1030 return this.communication; 1031 } 1032 1033 /** 1034 * @return Returns a reference to <code>this</code> for easy method chaining 1035 */ 1036 public RelatedPerson setCommunication(List<RelatedPersonCommunicationComponent> theCommunication) { 1037 this.communication = theCommunication; 1038 return this; 1039 } 1040 1041 public boolean hasCommunication() { 1042 if (this.communication == null) 1043 return false; 1044 for (RelatedPersonCommunicationComponent item : this.communication) 1045 if (!item.isEmpty()) 1046 return true; 1047 return false; 1048 } 1049 1050 public RelatedPersonCommunicationComponent addCommunication() { // 3 1051 RelatedPersonCommunicationComponent t = new RelatedPersonCommunicationComponent(); 1052 if (this.communication == null) 1053 this.communication = new ArrayList<RelatedPersonCommunicationComponent>(); 1054 this.communication.add(t); 1055 return t; 1056 } 1057 1058 public RelatedPerson addCommunication(RelatedPersonCommunicationComponent t) { // 3 1059 if (t == null) 1060 return this; 1061 if (this.communication == null) 1062 this.communication = new ArrayList<RelatedPersonCommunicationComponent>(); 1063 this.communication.add(t); 1064 return this; 1065 } 1066 1067 /** 1068 * @return The first repetition of repeating field {@link #communication}, 1069 * creating it if it does not already exist 1070 */ 1071 public RelatedPersonCommunicationComponent getCommunicationFirstRep() { 1072 if (getCommunication().isEmpty()) { 1073 addCommunication(); 1074 } 1075 return getCommunication().get(0); 1076 } 1077 1078 protected void listChildren(List<Property> children) { 1079 super.listChildren(children); 1080 children.add(new Property("identifier", "Identifier", "Identifier for a person within a particular scope.", 0, 1081 java.lang.Integer.MAX_VALUE, identifier)); 1082 children 1083 .add(new Property("active", "boolean", "Whether this related person record is in active use.", 0, 1, active)); 1084 children 1085 .add(new Property("patient", "Reference(Patient)", "The patient this person is related to.", 0, 1, patient)); 1086 children.add(new Property("relationship", "CodeableConcept", 1087 "The nature of the relationship between a patient and the related person.", 0, java.lang.Integer.MAX_VALUE, 1088 relationship)); 1089 children.add( 1090 new Property("name", "HumanName", "A name associated with the person.", 0, java.lang.Integer.MAX_VALUE, name)); 1091 children.add(new Property("telecom", "ContactPoint", 1092 "A contact detail for the person, e.g. a telephone number or an email address.", 0, java.lang.Integer.MAX_VALUE, 1093 telecom)); 1094 children.add(new Property("gender", "code", 1095 "Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes.", 1096 0, 1, gender)); 1097 children.add(new Property("birthDate", "date", "The date on which the related person was born.", 0, 1, birthDate)); 1098 children.add(new Property("address", "Address", "Address where the related person can be contacted or visited.", 0, 1099 java.lang.Integer.MAX_VALUE, address)); 1100 children.add(new Property("photo", "Attachment", "Image of the person.", 0, java.lang.Integer.MAX_VALUE, photo)); 1101 children.add(new Property("period", "Period", 1102 "The period of time during which this relationship is or was active. If there are no dates defined, then the interval is unknown.", 1103 0, 1, period)); 1104 children.add(new Property("communication", "", 1105 "A language which may be used to communicate with about the patient's health.", 0, java.lang.Integer.MAX_VALUE, 1106 communication)); 1107 } 1108 1109 @Override 1110 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1111 switch (_hash) { 1112 case -1618432855: 1113 /* identifier */ return new Property("identifier", "Identifier", 1114 "Identifier for a person within a particular scope.", 0, java.lang.Integer.MAX_VALUE, identifier); 1115 case -1422950650: 1116 /* active */ return new Property("active", "boolean", "Whether this related person record is in active use.", 0, 1117 1, active); 1118 case -791418107: 1119 /* patient */ return new Property("patient", "Reference(Patient)", "The patient this person is related to.", 0, 1, 1120 patient); 1121 case -261851592: 1122 /* relationship */ return new Property("relationship", "CodeableConcept", 1123 "The nature of the relationship between a patient and the related person.", 0, java.lang.Integer.MAX_VALUE, 1124 relationship); 1125 case 3373707: 1126 /* name */ return new Property("name", "HumanName", "A name associated with the person.", 0, 1127 java.lang.Integer.MAX_VALUE, name); 1128 case -1429363305: 1129 /* telecom */ return new Property("telecom", "ContactPoint", 1130 "A contact detail for the person, e.g. a telephone number or an email address.", 0, 1131 java.lang.Integer.MAX_VALUE, telecom); 1132 case -1249512767: 1133 /* gender */ return new Property("gender", "code", 1134 "Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes.", 1135 0, 1, gender); 1136 case -1210031859: 1137 /* birthDate */ return new Property("birthDate", "date", "The date on which the related person was born.", 0, 1, 1138 birthDate); 1139 case -1147692044: 1140 /* address */ return new Property("address", "Address", 1141 "Address where the related person can be contacted or visited.", 0, java.lang.Integer.MAX_VALUE, address); 1142 case 106642994: 1143 /* photo */ return new Property("photo", "Attachment", "Image of the person.", 0, java.lang.Integer.MAX_VALUE, 1144 photo); 1145 case -991726143: 1146 /* period */ return new Property("period", "Period", 1147 "The period of time during which this relationship is or was active. If there are no dates defined, then the interval is unknown.", 1148 0, 1, period); 1149 case -1035284522: 1150 /* communication */ return new Property("communication", "", 1151 "A language which may be used to communicate with about the patient's health.", 0, 1152 java.lang.Integer.MAX_VALUE, communication); 1153 default: 1154 return super.getNamedProperty(_hash, _name, _checkValid); 1155 } 1156 1157 } 1158 1159 @Override 1160 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1161 switch (hash) { 1162 case -1618432855: 1163 /* identifier */ return this.identifier == null ? new Base[0] 1164 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1165 case -1422950650: 1166 /* active */ return this.active == null ? new Base[0] : new Base[] { this.active }; // BooleanType 1167 case -791418107: 1168 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 1169 case -261851592: 1170 /* relationship */ return this.relationship == null ? new Base[0] 1171 : this.relationship.toArray(new Base[this.relationship.size()]); // CodeableConcept 1172 case 3373707: 1173 /* name */ return this.name == null ? new Base[0] : this.name.toArray(new Base[this.name.size()]); // HumanName 1174 case -1429363305: 1175 /* telecom */ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 1176 case -1249512767: 1177 /* gender */ return this.gender == null ? new Base[0] : new Base[] { this.gender }; // Enumeration<AdministrativeGender> 1178 case -1210031859: 1179 /* birthDate */ return this.birthDate == null ? new Base[0] : new Base[] { this.birthDate }; // DateType 1180 case -1147692044: 1181 /* address */ return this.address == null ? new Base[0] : this.address.toArray(new Base[this.address.size()]); // Address 1182 case 106642994: 1183 /* photo */ return this.photo == null ? new Base[0] : this.photo.toArray(new Base[this.photo.size()]); // Attachment 1184 case -991726143: 1185 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 1186 case -1035284522: 1187 /* communication */ return this.communication == null ? new Base[0] 1188 : this.communication.toArray(new Base[this.communication.size()]); // RelatedPersonCommunicationComponent 1189 default: 1190 return super.getProperty(hash, name, checkValid); 1191 } 1192 1193 } 1194 1195 @Override 1196 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1197 switch (hash) { 1198 case -1618432855: // identifier 1199 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1200 return value; 1201 case -1422950650: // active 1202 this.active = castToBoolean(value); // BooleanType 1203 return value; 1204 case -791418107: // patient 1205 this.patient = castToReference(value); // Reference 1206 return value; 1207 case -261851592: // relationship 1208 this.getRelationship().add(castToCodeableConcept(value)); // CodeableConcept 1209 return value; 1210 case 3373707: // name 1211 this.getName().add(castToHumanName(value)); // HumanName 1212 return value; 1213 case -1429363305: // telecom 1214 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 1215 return value; 1216 case -1249512767: // gender 1217 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 1218 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 1219 return value; 1220 case -1210031859: // birthDate 1221 this.birthDate = castToDate(value); // DateType 1222 return value; 1223 case -1147692044: // address 1224 this.getAddress().add(castToAddress(value)); // Address 1225 return value; 1226 case 106642994: // photo 1227 this.getPhoto().add(castToAttachment(value)); // Attachment 1228 return value; 1229 case -991726143: // period 1230 this.period = castToPeriod(value); // Period 1231 return value; 1232 case -1035284522: // communication 1233 this.getCommunication().add((RelatedPersonCommunicationComponent) value); // RelatedPersonCommunicationComponent 1234 return value; 1235 default: 1236 return super.setProperty(hash, name, value); 1237 } 1238 1239 } 1240 1241 @Override 1242 public Base setProperty(String name, Base value) throws FHIRException { 1243 if (name.equals("identifier")) { 1244 this.getIdentifier().add(castToIdentifier(value)); 1245 } else if (name.equals("active")) { 1246 this.active = castToBoolean(value); // BooleanType 1247 } else if (name.equals("patient")) { 1248 this.patient = castToReference(value); // Reference 1249 } else if (name.equals("relationship")) { 1250 this.getRelationship().add(castToCodeableConcept(value)); 1251 } else if (name.equals("name")) { 1252 this.getName().add(castToHumanName(value)); 1253 } else if (name.equals("telecom")) { 1254 this.getTelecom().add(castToContactPoint(value)); 1255 } else if (name.equals("gender")) { 1256 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 1257 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 1258 } else if (name.equals("birthDate")) { 1259 this.birthDate = castToDate(value); // DateType 1260 } else if (name.equals("address")) { 1261 this.getAddress().add(castToAddress(value)); 1262 } else if (name.equals("photo")) { 1263 this.getPhoto().add(castToAttachment(value)); 1264 } else if (name.equals("period")) { 1265 this.period = castToPeriod(value); // Period 1266 } else if (name.equals("communication")) { 1267 this.getCommunication().add((RelatedPersonCommunicationComponent) value); 1268 } else 1269 return super.setProperty(name, value); 1270 return value; 1271 } 1272 1273 @Override 1274 public void removeChild(String name, Base value) throws FHIRException { 1275 if (name.equals("identifier")) { 1276 this.getIdentifier().remove(castToIdentifier(value)); 1277 } else if (name.equals("active")) { 1278 this.active = null; 1279 } else if (name.equals("patient")) { 1280 this.patient = null; 1281 } else if (name.equals("relationship")) { 1282 this.getRelationship().remove(castToCodeableConcept(value)); 1283 } else if (name.equals("name")) { 1284 this.getName().remove(castToHumanName(value)); 1285 } else if (name.equals("telecom")) { 1286 this.getTelecom().remove(castToContactPoint(value)); 1287 } else if (name.equals("gender")) { 1288 this.gender = null; 1289 } else if (name.equals("birthDate")) { 1290 this.birthDate = null; 1291 } else if (name.equals("address")) { 1292 this.getAddress().remove(castToAddress(value)); 1293 } else if (name.equals("photo")) { 1294 this.getPhoto().remove(castToAttachment(value)); 1295 } else if (name.equals("period")) { 1296 this.period = null; 1297 } else if (name.equals("communication")) { 1298 this.getCommunication().remove((RelatedPersonCommunicationComponent) value); 1299 } else 1300 super.removeChild(name, value); 1301 1302 } 1303 1304 @Override 1305 public Base makeProperty(int hash, String name) throws FHIRException { 1306 switch (hash) { 1307 case -1618432855: 1308 return addIdentifier(); 1309 case -1422950650: 1310 return getActiveElement(); 1311 case -791418107: 1312 return getPatient(); 1313 case -261851592: 1314 return addRelationship(); 1315 case 3373707: 1316 return addName(); 1317 case -1429363305: 1318 return addTelecom(); 1319 case -1249512767: 1320 return getGenderElement(); 1321 case -1210031859: 1322 return getBirthDateElement(); 1323 case -1147692044: 1324 return addAddress(); 1325 case 106642994: 1326 return addPhoto(); 1327 case -991726143: 1328 return getPeriod(); 1329 case -1035284522: 1330 return addCommunication(); 1331 default: 1332 return super.makeProperty(hash, name); 1333 } 1334 1335 } 1336 1337 @Override 1338 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1339 switch (hash) { 1340 case -1618432855: 1341 /* identifier */ return new String[] { "Identifier" }; 1342 case -1422950650: 1343 /* active */ return new String[] { "boolean" }; 1344 case -791418107: 1345 /* patient */ return new String[] { "Reference" }; 1346 case -261851592: 1347 /* relationship */ return new String[] { "CodeableConcept" }; 1348 case 3373707: 1349 /* name */ return new String[] { "HumanName" }; 1350 case -1429363305: 1351 /* telecom */ return new String[] { "ContactPoint" }; 1352 case -1249512767: 1353 /* gender */ return new String[] { "code" }; 1354 case -1210031859: 1355 /* birthDate */ return new String[] { "date" }; 1356 case -1147692044: 1357 /* address */ return new String[] { "Address" }; 1358 case 106642994: 1359 /* photo */ return new String[] { "Attachment" }; 1360 case -991726143: 1361 /* period */ return new String[] { "Period" }; 1362 case -1035284522: 1363 /* communication */ return new String[] {}; 1364 default: 1365 return super.getTypesForProperty(hash, name); 1366 } 1367 1368 } 1369 1370 @Override 1371 public Base addChild(String name) throws FHIRException { 1372 if (name.equals("identifier")) { 1373 return addIdentifier(); 1374 } else if (name.equals("active")) { 1375 throw new FHIRException("Cannot call addChild on a singleton property RelatedPerson.active"); 1376 } else if (name.equals("patient")) { 1377 this.patient = new Reference(); 1378 return this.patient; 1379 } else if (name.equals("relationship")) { 1380 return addRelationship(); 1381 } else if (name.equals("name")) { 1382 return addName(); 1383 } else if (name.equals("telecom")) { 1384 return addTelecom(); 1385 } else if (name.equals("gender")) { 1386 throw new FHIRException("Cannot call addChild on a singleton property RelatedPerson.gender"); 1387 } else if (name.equals("birthDate")) { 1388 throw new FHIRException("Cannot call addChild on a singleton property RelatedPerson.birthDate"); 1389 } else if (name.equals("address")) { 1390 return addAddress(); 1391 } else if (name.equals("photo")) { 1392 return addPhoto(); 1393 } else if (name.equals("period")) { 1394 this.period = new Period(); 1395 return this.period; 1396 } else if (name.equals("communication")) { 1397 return addCommunication(); 1398 } else 1399 return super.addChild(name); 1400 } 1401 1402 public String fhirType() { 1403 return "RelatedPerson"; 1404 1405 } 1406 1407 public RelatedPerson copy() { 1408 RelatedPerson dst = new RelatedPerson(); 1409 copyValues(dst); 1410 return dst; 1411 } 1412 1413 public void copyValues(RelatedPerson dst) { 1414 super.copyValues(dst); 1415 if (identifier != null) { 1416 dst.identifier = new ArrayList<Identifier>(); 1417 for (Identifier i : identifier) 1418 dst.identifier.add(i.copy()); 1419 } 1420 ; 1421 dst.active = active == null ? null : active.copy(); 1422 dst.patient = patient == null ? null : patient.copy(); 1423 if (relationship != null) { 1424 dst.relationship = new ArrayList<CodeableConcept>(); 1425 for (CodeableConcept i : relationship) 1426 dst.relationship.add(i.copy()); 1427 } 1428 ; 1429 if (name != null) { 1430 dst.name = new ArrayList<HumanName>(); 1431 for (HumanName i : name) 1432 dst.name.add(i.copy()); 1433 } 1434 ; 1435 if (telecom != null) { 1436 dst.telecom = new ArrayList<ContactPoint>(); 1437 for (ContactPoint i : telecom) 1438 dst.telecom.add(i.copy()); 1439 } 1440 ; 1441 dst.gender = gender == null ? null : gender.copy(); 1442 dst.birthDate = birthDate == null ? null : birthDate.copy(); 1443 if (address != null) { 1444 dst.address = new ArrayList<Address>(); 1445 for (Address i : address) 1446 dst.address.add(i.copy()); 1447 } 1448 ; 1449 if (photo != null) { 1450 dst.photo = new ArrayList<Attachment>(); 1451 for (Attachment i : photo) 1452 dst.photo.add(i.copy()); 1453 } 1454 ; 1455 dst.period = period == null ? null : period.copy(); 1456 if (communication != null) { 1457 dst.communication = new ArrayList<RelatedPersonCommunicationComponent>(); 1458 for (RelatedPersonCommunicationComponent i : communication) 1459 dst.communication.add(i.copy()); 1460 } 1461 ; 1462 } 1463 1464 protected RelatedPerson typedCopy() { 1465 return copy(); 1466 } 1467 1468 @Override 1469 public boolean equalsDeep(Base other_) { 1470 if (!super.equalsDeep(other_)) 1471 return false; 1472 if (!(other_ instanceof RelatedPerson)) 1473 return false; 1474 RelatedPerson o = (RelatedPerson) other_; 1475 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) 1476 && compareDeep(patient, o.patient, true) && compareDeep(relationship, o.relationship, true) 1477 && compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true) 1478 && compareDeep(gender, o.gender, true) && compareDeep(birthDate, o.birthDate, true) 1479 && compareDeep(address, o.address, true) && compareDeep(photo, o.photo, true) 1480 && compareDeep(period, o.period, true) && compareDeep(communication, o.communication, true); 1481 } 1482 1483 @Override 1484 public boolean equalsShallow(Base other_) { 1485 if (!super.equalsShallow(other_)) 1486 return false; 1487 if (!(other_ instanceof RelatedPerson)) 1488 return false; 1489 RelatedPerson o = (RelatedPerson) other_; 1490 return compareValues(active, o.active, true) && compareValues(gender, o.gender, true) 1491 && compareValues(birthDate, o.birthDate, true); 1492 } 1493 1494 public boolean isEmpty() { 1495 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, patient, relationship, name, 1496 telecom, gender, birthDate, address, photo, period, communication); 1497 } 1498 1499 @Override 1500 public ResourceType getResourceType() { 1501 return ResourceType.RelatedPerson; 1502 } 1503 1504 /** 1505 * Search parameter: <b>identifier</b> 1506 * <p> 1507 * Description: <b>An Identifier of the RelatedPerson</b><br> 1508 * Type: <b>token</b><br> 1509 * Path: <b>RelatedPerson.identifier</b><br> 1510 * </p> 1511 */ 1512 @SearchParamDefinition(name = "identifier", path = "RelatedPerson.identifier", description = "An Identifier of the RelatedPerson", type = "token") 1513 public static final String SP_IDENTIFIER = "identifier"; 1514 /** 1515 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1516 * <p> 1517 * Description: <b>An Identifier of the RelatedPerson</b><br> 1518 * Type: <b>token</b><br> 1519 * Path: <b>RelatedPerson.identifier</b><br> 1520 * </p> 1521 */ 1522 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1523 SP_IDENTIFIER); 1524 1525 /** 1526 * Search parameter: <b>address</b> 1527 * <p> 1528 * Description: <b>A server defined search that may match any of the string 1529 * fields in the Address, including line, city, district, state, country, 1530 * postalCode, and/or text</b><br> 1531 * Type: <b>string</b><br> 1532 * Path: <b>RelatedPerson.address</b><br> 1533 * </p> 1534 */ 1535 @SearchParamDefinition(name = "address", path = "RelatedPerson.address", description = "A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text", type = "string") 1536 public static final String SP_ADDRESS = "address"; 1537 /** 1538 * <b>Fluent Client</b> search parameter constant for <b>address</b> 1539 * <p> 1540 * Description: <b>A server defined search that may match any of the string 1541 * fields in the Address, including line, city, district, state, country, 1542 * postalCode, and/or text</b><br> 1543 * Type: <b>string</b><br> 1544 * Path: <b>RelatedPerson.address</b><br> 1545 * </p> 1546 */ 1547 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS = new ca.uhn.fhir.rest.gclient.StringClientParam( 1548 SP_ADDRESS); 1549 1550 /** 1551 * Search parameter: <b>birthdate</b> 1552 * <p> 1553 * Description: <b>The Related Person's date of birth</b><br> 1554 * Type: <b>date</b><br> 1555 * Path: <b>RelatedPerson.birthDate</b><br> 1556 * </p> 1557 */ 1558 @SearchParamDefinition(name = "birthdate", path = "RelatedPerson.birthDate", description = "The Related Person's date of birth", type = "date") 1559 public static final String SP_BIRTHDATE = "birthdate"; 1560 /** 1561 * <b>Fluent Client</b> search parameter constant for <b>birthdate</b> 1562 * <p> 1563 * Description: <b>The Related Person's date of birth</b><br> 1564 * Type: <b>date</b><br> 1565 * Path: <b>RelatedPerson.birthDate</b><br> 1566 * </p> 1567 */ 1568 public static final ca.uhn.fhir.rest.gclient.DateClientParam BIRTHDATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 1569 SP_BIRTHDATE); 1570 1571 /** 1572 * Search parameter: <b>address-state</b> 1573 * <p> 1574 * Description: <b>A state specified in an address</b><br> 1575 * Type: <b>string</b><br> 1576 * Path: <b>RelatedPerson.address.state</b><br> 1577 * </p> 1578 */ 1579 @SearchParamDefinition(name = "address-state", path = "RelatedPerson.address.state", description = "A state specified in an address", type = "string") 1580 public static final String SP_ADDRESS_STATE = "address-state"; 1581 /** 1582 * <b>Fluent Client</b> search parameter constant for <b>address-state</b> 1583 * <p> 1584 * Description: <b>A state specified in an address</b><br> 1585 * Type: <b>string</b><br> 1586 * Path: <b>RelatedPerson.address.state</b><br> 1587 * </p> 1588 */ 1589 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_STATE = new ca.uhn.fhir.rest.gclient.StringClientParam( 1590 SP_ADDRESS_STATE); 1591 1592 /** 1593 * Search parameter: <b>gender</b> 1594 * <p> 1595 * Description: <b>Gender of the related person</b><br> 1596 * Type: <b>token</b><br> 1597 * Path: <b>RelatedPerson.gender</b><br> 1598 * </p> 1599 */ 1600 @SearchParamDefinition(name = "gender", path = "RelatedPerson.gender", description = "Gender of the related person", type = "token") 1601 public static final String SP_GENDER = "gender"; 1602 /** 1603 * <b>Fluent Client</b> search parameter constant for <b>gender</b> 1604 * <p> 1605 * Description: <b>Gender of the related person</b><br> 1606 * Type: <b>token</b><br> 1607 * Path: <b>RelatedPerson.gender</b><br> 1608 * </p> 1609 */ 1610 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GENDER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1611 SP_GENDER); 1612 1613 /** 1614 * Search parameter: <b>active</b> 1615 * <p> 1616 * Description: <b>Indicates if the related person record is active</b><br> 1617 * Type: <b>token</b><br> 1618 * Path: <b>RelatedPerson.active</b><br> 1619 * </p> 1620 */ 1621 @SearchParamDefinition(name = "active", path = "RelatedPerson.active", description = "Indicates if the related person record is active", type = "token") 1622 public static final String SP_ACTIVE = "active"; 1623 /** 1624 * <b>Fluent Client</b> search parameter constant for <b>active</b> 1625 * <p> 1626 * Description: <b>Indicates if the related person record is active</b><br> 1627 * Type: <b>token</b><br> 1628 * Path: <b>RelatedPerson.active</b><br> 1629 * </p> 1630 */ 1631 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1632 SP_ACTIVE); 1633 1634 /** 1635 * Search parameter: <b>address-postalcode</b> 1636 * <p> 1637 * Description: <b>A postal code specified in an address</b><br> 1638 * Type: <b>string</b><br> 1639 * Path: <b>RelatedPerson.address.postalCode</b><br> 1640 * </p> 1641 */ 1642 @SearchParamDefinition(name = "address-postalcode", path = "RelatedPerson.address.postalCode", description = "A postal code specified in an address", type = "string") 1643 public static final String SP_ADDRESS_POSTALCODE = "address-postalcode"; 1644 /** 1645 * <b>Fluent Client</b> search parameter constant for <b>address-postalcode</b> 1646 * <p> 1647 * Description: <b>A postal code specified in an address</b><br> 1648 * Type: <b>string</b><br> 1649 * Path: <b>RelatedPerson.address.postalCode</b><br> 1650 * </p> 1651 */ 1652 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_POSTALCODE = new ca.uhn.fhir.rest.gclient.StringClientParam( 1653 SP_ADDRESS_POSTALCODE); 1654 1655 /** 1656 * Search parameter: <b>address-country</b> 1657 * <p> 1658 * Description: <b>A country specified in an address</b><br> 1659 * Type: <b>string</b><br> 1660 * Path: <b>RelatedPerson.address.country</b><br> 1661 * </p> 1662 */ 1663 @SearchParamDefinition(name = "address-country", path = "RelatedPerson.address.country", description = "A country specified in an address", type = "string") 1664 public static final String SP_ADDRESS_COUNTRY = "address-country"; 1665 /** 1666 * <b>Fluent Client</b> search parameter constant for <b>address-country</b> 1667 * <p> 1668 * Description: <b>A country specified in an address</b><br> 1669 * Type: <b>string</b><br> 1670 * Path: <b>RelatedPerson.address.country</b><br> 1671 * </p> 1672 */ 1673 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_COUNTRY = new ca.uhn.fhir.rest.gclient.StringClientParam( 1674 SP_ADDRESS_COUNTRY); 1675 1676 /** 1677 * Search parameter: <b>phonetic</b> 1678 * <p> 1679 * Description: <b>A portion of name using some kind of phonetic matching 1680 * algorithm</b><br> 1681 * Type: <b>string</b><br> 1682 * Path: <b>RelatedPerson.name</b><br> 1683 * </p> 1684 */ 1685 @SearchParamDefinition(name = "phonetic", path = "RelatedPerson.name", description = "A portion of name using some kind of phonetic matching algorithm", type = "string") 1686 public static final String SP_PHONETIC = "phonetic"; 1687 /** 1688 * <b>Fluent Client</b> search parameter constant for <b>phonetic</b> 1689 * <p> 1690 * Description: <b>A portion of name using some kind of phonetic matching 1691 * algorithm</b><br> 1692 * Type: <b>string</b><br> 1693 * Path: <b>RelatedPerson.name</b><br> 1694 * </p> 1695 */ 1696 public static final ca.uhn.fhir.rest.gclient.StringClientParam PHONETIC = new ca.uhn.fhir.rest.gclient.StringClientParam( 1697 SP_PHONETIC); 1698 1699 /** 1700 * Search parameter: <b>phone</b> 1701 * <p> 1702 * Description: <b>A value in a phone contact</b><br> 1703 * Type: <b>token</b><br> 1704 * Path: <b>RelatedPerson.telecom(system=phone)</b><br> 1705 * </p> 1706 */ 1707 @SearchParamDefinition(name = "phone", path = "RelatedPerson.telecom.where(system='phone')", description = "A value in a phone contact", type = "token") 1708 public static final String SP_PHONE = "phone"; 1709 /** 1710 * <b>Fluent Client</b> search parameter constant for <b>phone</b> 1711 * <p> 1712 * Description: <b>A value in a phone contact</b><br> 1713 * Type: <b>token</b><br> 1714 * Path: <b>RelatedPerson.telecom(system=phone)</b><br> 1715 * </p> 1716 */ 1717 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PHONE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1718 SP_PHONE); 1719 1720 /** 1721 * Search parameter: <b>patient</b> 1722 * <p> 1723 * Description: <b>The patient this related person is related to</b><br> 1724 * Type: <b>reference</b><br> 1725 * Path: <b>RelatedPerson.patient</b><br> 1726 * </p> 1727 */ 1728 @SearchParamDefinition(name = "patient", path = "RelatedPerson.patient", description = "The patient this related person is related to", type = "reference", providesMembershipIn = { 1729 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 1730 public static final String SP_PATIENT = "patient"; 1731 /** 1732 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1733 * <p> 1734 * Description: <b>The patient this related person is related to</b><br> 1735 * Type: <b>reference</b><br> 1736 * Path: <b>RelatedPerson.patient</b><br> 1737 * </p> 1738 */ 1739 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1740 SP_PATIENT); 1741 1742 /** 1743 * Constant for fluent queries to be used to add include statements. Specifies 1744 * the path value of "<b>RelatedPerson:patient</b>". 1745 */ 1746 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 1747 "RelatedPerson:patient").toLocked(); 1748 1749 /** 1750 * Search parameter: <b>name</b> 1751 * <p> 1752 * Description: <b>A server defined search that may match any of the string 1753 * fields in the HumanName, including family, give, prefix, suffix, suffix, 1754 * and/or text</b><br> 1755 * Type: <b>string</b><br> 1756 * Path: <b>RelatedPerson.name</b><br> 1757 * </p> 1758 */ 1759 @SearchParamDefinition(name = "name", path = "RelatedPerson.name", description = "A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text", type = "string") 1760 public static final String SP_NAME = "name"; 1761 /** 1762 * <b>Fluent Client</b> search parameter constant for <b>name</b> 1763 * <p> 1764 * Description: <b>A server defined search that may match any of the string 1765 * fields in the HumanName, including family, give, prefix, suffix, suffix, 1766 * and/or text</b><br> 1767 * Type: <b>string</b><br> 1768 * Path: <b>RelatedPerson.name</b><br> 1769 * </p> 1770 */ 1771 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 1772 SP_NAME); 1773 1774 /** 1775 * Search parameter: <b>address-use</b> 1776 * <p> 1777 * Description: <b>A use code specified in an address</b><br> 1778 * Type: <b>token</b><br> 1779 * Path: <b>RelatedPerson.address.use</b><br> 1780 * </p> 1781 */ 1782 @SearchParamDefinition(name = "address-use", path = "RelatedPerson.address.use", description = "A use code specified in an address", type = "token") 1783 public static final String SP_ADDRESS_USE = "address-use"; 1784 /** 1785 * <b>Fluent Client</b> search parameter constant for <b>address-use</b> 1786 * <p> 1787 * Description: <b>A use code specified in an address</b><br> 1788 * Type: <b>token</b><br> 1789 * Path: <b>RelatedPerson.address.use</b><br> 1790 * </p> 1791 */ 1792 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADDRESS_USE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1793 SP_ADDRESS_USE); 1794 1795 /** 1796 * Search parameter: <b>telecom</b> 1797 * <p> 1798 * Description: <b>The value in any kind of contact</b><br> 1799 * Type: <b>token</b><br> 1800 * Path: <b>RelatedPerson.telecom</b><br> 1801 * </p> 1802 */ 1803 @SearchParamDefinition(name = "telecom", path = "RelatedPerson.telecom", description = "The value in any kind of contact", type = "token") 1804 public static final String SP_TELECOM = "telecom"; 1805 /** 1806 * <b>Fluent Client</b> search parameter constant for <b>telecom</b> 1807 * <p> 1808 * Description: <b>The value in any kind of contact</b><br> 1809 * Type: <b>token</b><br> 1810 * Path: <b>RelatedPerson.telecom</b><br> 1811 * </p> 1812 */ 1813 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TELECOM = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1814 SP_TELECOM); 1815 1816 /** 1817 * Search parameter: <b>address-city</b> 1818 * <p> 1819 * Description: <b>A city specified in an address</b><br> 1820 * Type: <b>string</b><br> 1821 * Path: <b>RelatedPerson.address.city</b><br> 1822 * </p> 1823 */ 1824 @SearchParamDefinition(name = "address-city", path = "RelatedPerson.address.city", description = "A city specified in an address", type = "string") 1825 public static final String SP_ADDRESS_CITY = "address-city"; 1826 /** 1827 * <b>Fluent Client</b> search parameter constant for <b>address-city</b> 1828 * <p> 1829 * Description: <b>A city specified in an address</b><br> 1830 * Type: <b>string</b><br> 1831 * Path: <b>RelatedPerson.address.city</b><br> 1832 * </p> 1833 */ 1834 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_CITY = new ca.uhn.fhir.rest.gclient.StringClientParam( 1835 SP_ADDRESS_CITY); 1836 1837 /** 1838 * Search parameter: <b>relationship</b> 1839 * <p> 1840 * Description: <b>The relationship between the patient and the 1841 * relatedperson</b><br> 1842 * Type: <b>token</b><br> 1843 * Path: <b>RelatedPerson.relationship</b><br> 1844 * </p> 1845 */ 1846 @SearchParamDefinition(name = "relationship", path = "RelatedPerson.relationship", description = "The relationship between the patient and the relatedperson", type = "token") 1847 public static final String SP_RELATIONSHIP = "relationship"; 1848 /** 1849 * <b>Fluent Client</b> search parameter constant for <b>relationship</b> 1850 * <p> 1851 * Description: <b>The relationship between the patient and the 1852 * relatedperson</b><br> 1853 * Type: <b>token</b><br> 1854 * Path: <b>RelatedPerson.relationship</b><br> 1855 * </p> 1856 */ 1857 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RELATIONSHIP = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1858 SP_RELATIONSHIP); 1859 1860 /** 1861 * Search parameter: <b>email</b> 1862 * <p> 1863 * Description: <b>A value in an email contact</b><br> 1864 * Type: <b>token</b><br> 1865 * Path: <b>RelatedPerson.telecom(system=email)</b><br> 1866 * </p> 1867 */ 1868 @SearchParamDefinition(name = "email", path = "RelatedPerson.telecom.where(system='email')", description = "A value in an email contact", type = "token") 1869 public static final String SP_EMAIL = "email"; 1870 /** 1871 * <b>Fluent Client</b> search parameter constant for <b>email</b> 1872 * <p> 1873 * Description: <b>A value in an email contact</b><br> 1874 * Type: <b>token</b><br> 1875 * Path: <b>RelatedPerson.telecom(system=email)</b><br> 1876 * </p> 1877 */ 1878 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EMAIL = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1879 SP_EMAIL); 1880 1881}