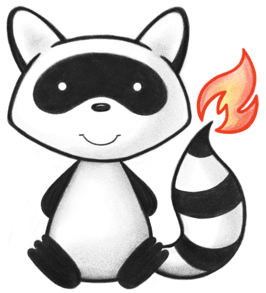
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * A group of related requests that can be used to capture intended activities 049 * that have inter-dependencies such as "give this medication after that one". 050 */ 051@ResourceDef(name = "RequestGroup", profile = "http://hl7.org/fhir/StructureDefinition/RequestGroup") 052public class RequestGroup extends DomainResource { 053 054 public enum RequestStatus { 055 /** 056 * The request has been created but is not yet complete or ready for action. 057 */ 058 DRAFT, 059 /** 060 * The request is in force and ready to be acted upon. 061 */ 062 ACTIVE, 063 /** 064 * The request (and any implicit authorization to act) has been temporarily 065 * withdrawn but is expected to resume in the future. 066 */ 067 ONHOLD, 068 /** 069 * The request (and any implicit authorization to act) has been terminated prior 070 * to the known full completion of the intended actions. No further activity 071 * should occur. 072 */ 073 REVOKED, 074 /** 075 * The activity described by the request has been fully performed. No further 076 * activity will occur. 077 */ 078 COMPLETED, 079 /** 080 * This request should never have existed and should be considered 'void'. (It 081 * is possible that real-world decisions were based on it. If real-world 082 * activity has occurred, the status should be "revoked" rather than 083 * "entered-in-error".). 084 */ 085 ENTEREDINERROR, 086 /** 087 * The authoring/source system does not know which of the status values 088 * currently applies for this request. Note: This concept is not to be used for 089 * "other" - one of the listed statuses is presumed to apply, but the 090 * authoring/source system does not know which. 091 */ 092 UNKNOWN, 093 /** 094 * added to help the parsers with the generic types 095 */ 096 NULL; 097 098 public static RequestStatus fromCode(String codeString) throws FHIRException { 099 if (codeString == null || "".equals(codeString)) 100 return null; 101 if ("draft".equals(codeString)) 102 return DRAFT; 103 if ("active".equals(codeString)) 104 return ACTIVE; 105 if ("on-hold".equals(codeString)) 106 return ONHOLD; 107 if ("revoked".equals(codeString)) 108 return REVOKED; 109 if ("completed".equals(codeString)) 110 return COMPLETED; 111 if ("entered-in-error".equals(codeString)) 112 return ENTEREDINERROR; 113 if ("unknown".equals(codeString)) 114 return UNKNOWN; 115 if (Configuration.isAcceptInvalidEnums()) 116 return null; 117 else 118 throw new FHIRException("Unknown RequestStatus code '" + codeString + "'"); 119 } 120 121 public String toCode() { 122 switch (this) { 123 case DRAFT: 124 return "draft"; 125 case ACTIVE: 126 return "active"; 127 case ONHOLD: 128 return "on-hold"; 129 case REVOKED: 130 return "revoked"; 131 case COMPLETED: 132 return "completed"; 133 case ENTEREDINERROR: 134 return "entered-in-error"; 135 case UNKNOWN: 136 return "unknown"; 137 case NULL: 138 return null; 139 default: 140 return "?"; 141 } 142 } 143 144 public String getSystem() { 145 switch (this) { 146 case DRAFT: 147 return "http://hl7.org/fhir/request-status"; 148 case ACTIVE: 149 return "http://hl7.org/fhir/request-status"; 150 case ONHOLD: 151 return "http://hl7.org/fhir/request-status"; 152 case REVOKED: 153 return "http://hl7.org/fhir/request-status"; 154 case COMPLETED: 155 return "http://hl7.org/fhir/request-status"; 156 case ENTEREDINERROR: 157 return "http://hl7.org/fhir/request-status"; 158 case UNKNOWN: 159 return "http://hl7.org/fhir/request-status"; 160 case NULL: 161 return null; 162 default: 163 return "?"; 164 } 165 } 166 167 public String getDefinition() { 168 switch (this) { 169 case DRAFT: 170 return "The request has been created but is not yet complete or ready for action."; 171 case ACTIVE: 172 return "The request is in force and ready to be acted upon."; 173 case ONHOLD: 174 return "The request (and any implicit authorization to act) has been temporarily withdrawn but is expected to resume in the future."; 175 case REVOKED: 176 return "The request (and any implicit authorization to act) has been terminated prior to the known full completion of the intended actions. No further activity should occur."; 177 case COMPLETED: 178 return "The activity described by the request has been fully performed. No further activity will occur."; 179 case ENTEREDINERROR: 180 return "This request should never have existed and should be considered 'void'. (It is possible that real-world decisions were based on it. If real-world activity has occurred, the status should be \"revoked\" rather than \"entered-in-error\".)."; 181 case UNKNOWN: 182 return "The authoring/source system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 183 case NULL: 184 return null; 185 default: 186 return "?"; 187 } 188 } 189 190 public String getDisplay() { 191 switch (this) { 192 case DRAFT: 193 return "Draft"; 194 case ACTIVE: 195 return "Active"; 196 case ONHOLD: 197 return "On Hold"; 198 case REVOKED: 199 return "Revoked"; 200 case COMPLETED: 201 return "Completed"; 202 case ENTEREDINERROR: 203 return "Entered in Error"; 204 case UNKNOWN: 205 return "Unknown"; 206 case NULL: 207 return null; 208 default: 209 return "?"; 210 } 211 } 212 } 213 214 public static class RequestStatusEnumFactory implements EnumFactory<RequestStatus> { 215 public RequestStatus fromCode(String codeString) throws IllegalArgumentException { 216 if (codeString == null || "".equals(codeString)) 217 if (codeString == null || "".equals(codeString)) 218 return null; 219 if ("draft".equals(codeString)) 220 return RequestStatus.DRAFT; 221 if ("active".equals(codeString)) 222 return RequestStatus.ACTIVE; 223 if ("on-hold".equals(codeString)) 224 return RequestStatus.ONHOLD; 225 if ("revoked".equals(codeString)) 226 return RequestStatus.REVOKED; 227 if ("completed".equals(codeString)) 228 return RequestStatus.COMPLETED; 229 if ("entered-in-error".equals(codeString)) 230 return RequestStatus.ENTEREDINERROR; 231 if ("unknown".equals(codeString)) 232 return RequestStatus.UNKNOWN; 233 throw new IllegalArgumentException("Unknown RequestStatus code '" + codeString + "'"); 234 } 235 236 public Enumeration<RequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 237 if (code == null) 238 return null; 239 if (code.isEmpty()) 240 return new Enumeration<RequestStatus>(this, RequestStatus.NULL, code); 241 String codeString = code.asStringValue(); 242 if (codeString == null || "".equals(codeString)) 243 return new Enumeration<RequestStatus>(this, RequestStatus.NULL, code); 244 if ("draft".equals(codeString)) 245 return new Enumeration<RequestStatus>(this, RequestStatus.DRAFT, code); 246 if ("active".equals(codeString)) 247 return new Enumeration<RequestStatus>(this, RequestStatus.ACTIVE, code); 248 if ("on-hold".equals(codeString)) 249 return new Enumeration<RequestStatus>(this, RequestStatus.ONHOLD, code); 250 if ("revoked".equals(codeString)) 251 return new Enumeration<RequestStatus>(this, RequestStatus.REVOKED, code); 252 if ("completed".equals(codeString)) 253 return new Enumeration<RequestStatus>(this, RequestStatus.COMPLETED, code); 254 if ("entered-in-error".equals(codeString)) 255 return new Enumeration<RequestStatus>(this, RequestStatus.ENTEREDINERROR, code); 256 if ("unknown".equals(codeString)) 257 return new Enumeration<RequestStatus>(this, RequestStatus.UNKNOWN, code); 258 throw new FHIRException("Unknown RequestStatus code '" + codeString + "'"); 259 } 260 261 public String toCode(RequestStatus code) { 262 if (code == RequestStatus.NULL) 263 return null; 264 if (code == RequestStatus.DRAFT) 265 return "draft"; 266 if (code == RequestStatus.ACTIVE) 267 return "active"; 268 if (code == RequestStatus.ONHOLD) 269 return "on-hold"; 270 if (code == RequestStatus.REVOKED) 271 return "revoked"; 272 if (code == RequestStatus.COMPLETED) 273 return "completed"; 274 if (code == RequestStatus.ENTEREDINERROR) 275 return "entered-in-error"; 276 if (code == RequestStatus.UNKNOWN) 277 return "unknown"; 278 return "?"; 279 } 280 281 public String toSystem(RequestStatus code) { 282 return code.getSystem(); 283 } 284 } 285 286 public enum RequestIntent { 287 /** 288 * The request is a suggestion made by someone/something that does not have an 289 * intention to ensure it occurs and without providing an authorization to act. 290 */ 291 PROPOSAL, 292 /** 293 * The request represents an intention to ensure something occurs without 294 * providing an authorization for others to act. 295 */ 296 PLAN, 297 /** 298 * The request represents a legally binding instruction authored by a Patient or 299 * RelatedPerson. 300 */ 301 DIRECTIVE, 302 /** 303 * The request represents a request/demand and authorization for action by a 304 * Practitioner. 305 */ 306 ORDER, 307 /** 308 * The request represents an original authorization for action. 309 */ 310 ORIGINALORDER, 311 /** 312 * The request represents an automatically generated supplemental authorization 313 * for action based on a parent authorization together with initial results of 314 * the action taken against that parent authorization. 315 */ 316 REFLEXORDER, 317 /** 318 * The request represents the view of an authorization instantiated by a 319 * fulfilling system representing the details of the fulfiller's intention to 320 * act upon a submitted order. 321 */ 322 FILLERORDER, 323 /** 324 * An order created in fulfillment of a broader order that represents the 325 * authorization for a single activity occurrence. E.g. The administration of a 326 * single dose of a drug. 327 */ 328 INSTANCEORDER, 329 /** 330 * The request represents a component or option for a RequestGroup that 331 * establishes timing, conditionality and/or other constraints among a set of 332 * requests. Refer to [[[RequestGroup]]] for additional information on how this 333 * status is used. 334 */ 335 OPTION, 336 /** 337 * added to help the parsers with the generic types 338 */ 339 NULL; 340 341 public static RequestIntent fromCode(String codeString) throws FHIRException { 342 if (codeString == null || "".equals(codeString)) 343 return null; 344 if ("proposal".equals(codeString)) 345 return PROPOSAL; 346 if ("plan".equals(codeString)) 347 return PLAN; 348 if ("directive".equals(codeString)) 349 return DIRECTIVE; 350 if ("order".equals(codeString)) 351 return ORDER; 352 if ("original-order".equals(codeString)) 353 return ORIGINALORDER; 354 if ("reflex-order".equals(codeString)) 355 return REFLEXORDER; 356 if ("filler-order".equals(codeString)) 357 return FILLERORDER; 358 if ("instance-order".equals(codeString)) 359 return INSTANCEORDER; 360 if ("option".equals(codeString)) 361 return OPTION; 362 if (Configuration.isAcceptInvalidEnums()) 363 return null; 364 else 365 throw new FHIRException("Unknown RequestIntent code '" + codeString + "'"); 366 } 367 368 public String toCode() { 369 switch (this) { 370 case PROPOSAL: 371 return "proposal"; 372 case PLAN: 373 return "plan"; 374 case DIRECTIVE: 375 return "directive"; 376 case ORDER: 377 return "order"; 378 case ORIGINALORDER: 379 return "original-order"; 380 case REFLEXORDER: 381 return "reflex-order"; 382 case FILLERORDER: 383 return "filler-order"; 384 case INSTANCEORDER: 385 return "instance-order"; 386 case OPTION: 387 return "option"; 388 case NULL: 389 return null; 390 default: 391 return "?"; 392 } 393 } 394 395 public String getSystem() { 396 switch (this) { 397 case PROPOSAL: 398 return "http://hl7.org/fhir/request-intent"; 399 case PLAN: 400 return "http://hl7.org/fhir/request-intent"; 401 case DIRECTIVE: 402 return "http://hl7.org/fhir/request-intent"; 403 case ORDER: 404 return "http://hl7.org/fhir/request-intent"; 405 case ORIGINALORDER: 406 return "http://hl7.org/fhir/request-intent"; 407 case REFLEXORDER: 408 return "http://hl7.org/fhir/request-intent"; 409 case FILLERORDER: 410 return "http://hl7.org/fhir/request-intent"; 411 case INSTANCEORDER: 412 return "http://hl7.org/fhir/request-intent"; 413 case OPTION: 414 return "http://hl7.org/fhir/request-intent"; 415 case NULL: 416 return null; 417 default: 418 return "?"; 419 } 420 } 421 422 public String getDefinition() { 423 switch (this) { 424 case PROPOSAL: 425 return "The request is a suggestion made by someone/something that does not have an intention to ensure it occurs and without providing an authorization to act."; 426 case PLAN: 427 return "The request represents an intention to ensure something occurs without providing an authorization for others to act."; 428 case DIRECTIVE: 429 return "The request represents a legally binding instruction authored by a Patient or RelatedPerson."; 430 case ORDER: 431 return "The request represents a request/demand and authorization for action by a Practitioner."; 432 case ORIGINALORDER: 433 return "The request represents an original authorization for action."; 434 case REFLEXORDER: 435 return "The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization."; 436 case FILLERORDER: 437 return "The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order."; 438 case INSTANCEORDER: 439 return "An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug."; 440 case OPTION: 441 return "The request represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests. Refer to [[[RequestGroup]]] for additional information on how this status is used."; 442 case NULL: 443 return null; 444 default: 445 return "?"; 446 } 447 } 448 449 public String getDisplay() { 450 switch (this) { 451 case PROPOSAL: 452 return "Proposal"; 453 case PLAN: 454 return "Plan"; 455 case DIRECTIVE: 456 return "Directive"; 457 case ORDER: 458 return "Order"; 459 case ORIGINALORDER: 460 return "Original Order"; 461 case REFLEXORDER: 462 return "Reflex Order"; 463 case FILLERORDER: 464 return "Filler Order"; 465 case INSTANCEORDER: 466 return "Instance Order"; 467 case OPTION: 468 return "Option"; 469 case NULL: 470 return null; 471 default: 472 return "?"; 473 } 474 } 475 } 476 477 public static class RequestIntentEnumFactory implements EnumFactory<RequestIntent> { 478 public RequestIntent fromCode(String codeString) throws IllegalArgumentException { 479 if (codeString == null || "".equals(codeString)) 480 if (codeString == null || "".equals(codeString)) 481 return null; 482 if ("proposal".equals(codeString)) 483 return RequestIntent.PROPOSAL; 484 if ("plan".equals(codeString)) 485 return RequestIntent.PLAN; 486 if ("directive".equals(codeString)) 487 return RequestIntent.DIRECTIVE; 488 if ("order".equals(codeString)) 489 return RequestIntent.ORDER; 490 if ("original-order".equals(codeString)) 491 return RequestIntent.ORIGINALORDER; 492 if ("reflex-order".equals(codeString)) 493 return RequestIntent.REFLEXORDER; 494 if ("filler-order".equals(codeString)) 495 return RequestIntent.FILLERORDER; 496 if ("instance-order".equals(codeString)) 497 return RequestIntent.INSTANCEORDER; 498 if ("option".equals(codeString)) 499 return RequestIntent.OPTION; 500 throw new IllegalArgumentException("Unknown RequestIntent code '" + codeString + "'"); 501 } 502 503 public Enumeration<RequestIntent> fromType(PrimitiveType<?> code) throws FHIRException { 504 if (code == null) 505 return null; 506 if (code.isEmpty()) 507 return new Enumeration<RequestIntent>(this, RequestIntent.NULL, code); 508 String codeString = code.asStringValue(); 509 if (codeString == null || "".equals(codeString)) 510 return new Enumeration<RequestIntent>(this, RequestIntent.NULL, code); 511 if ("proposal".equals(codeString)) 512 return new Enumeration<RequestIntent>(this, RequestIntent.PROPOSAL, code); 513 if ("plan".equals(codeString)) 514 return new Enumeration<RequestIntent>(this, RequestIntent.PLAN, code); 515 if ("directive".equals(codeString)) 516 return new Enumeration<RequestIntent>(this, RequestIntent.DIRECTIVE, code); 517 if ("order".equals(codeString)) 518 return new Enumeration<RequestIntent>(this, RequestIntent.ORDER, code); 519 if ("original-order".equals(codeString)) 520 return new Enumeration<RequestIntent>(this, RequestIntent.ORIGINALORDER, code); 521 if ("reflex-order".equals(codeString)) 522 return new Enumeration<RequestIntent>(this, RequestIntent.REFLEXORDER, code); 523 if ("filler-order".equals(codeString)) 524 return new Enumeration<RequestIntent>(this, RequestIntent.FILLERORDER, code); 525 if ("instance-order".equals(codeString)) 526 return new Enumeration<RequestIntent>(this, RequestIntent.INSTANCEORDER, code); 527 if ("option".equals(codeString)) 528 return new Enumeration<RequestIntent>(this, RequestIntent.OPTION, code); 529 throw new FHIRException("Unknown RequestIntent code '" + codeString + "'"); 530 } 531 532 public String toCode(RequestIntent code) { 533 if (code == RequestIntent.NULL) 534 return null; 535 if (code == RequestIntent.PROPOSAL) 536 return "proposal"; 537 if (code == RequestIntent.PLAN) 538 return "plan"; 539 if (code == RequestIntent.DIRECTIVE) 540 return "directive"; 541 if (code == RequestIntent.ORDER) 542 return "order"; 543 if (code == RequestIntent.ORIGINALORDER) 544 return "original-order"; 545 if (code == RequestIntent.REFLEXORDER) 546 return "reflex-order"; 547 if (code == RequestIntent.FILLERORDER) 548 return "filler-order"; 549 if (code == RequestIntent.INSTANCEORDER) 550 return "instance-order"; 551 if (code == RequestIntent.OPTION) 552 return "option"; 553 return "?"; 554 } 555 556 public String toSystem(RequestIntent code) { 557 return code.getSystem(); 558 } 559 } 560 561 public enum RequestPriority { 562 /** 563 * The request has normal priority. 564 */ 565 ROUTINE, 566 /** 567 * The request should be actioned promptly - higher priority than routine. 568 */ 569 URGENT, 570 /** 571 * The request should be actioned as soon as possible - higher priority than 572 * urgent. 573 */ 574 ASAP, 575 /** 576 * The request should be actioned immediately - highest possible priority. E.g. 577 * an emergency. 578 */ 579 STAT, 580 /** 581 * added to help the parsers with the generic types 582 */ 583 NULL; 584 585 public static RequestPriority fromCode(String codeString) throws FHIRException { 586 if (codeString == null || "".equals(codeString)) 587 return null; 588 if ("routine".equals(codeString)) 589 return ROUTINE; 590 if ("urgent".equals(codeString)) 591 return URGENT; 592 if ("asap".equals(codeString)) 593 return ASAP; 594 if ("stat".equals(codeString)) 595 return STAT; 596 if (Configuration.isAcceptInvalidEnums()) 597 return null; 598 else 599 throw new FHIRException("Unknown RequestPriority code '" + codeString + "'"); 600 } 601 602 public String toCode() { 603 switch (this) { 604 case ROUTINE: 605 return "routine"; 606 case URGENT: 607 return "urgent"; 608 case ASAP: 609 return "asap"; 610 case STAT: 611 return "stat"; 612 case NULL: 613 return null; 614 default: 615 return "?"; 616 } 617 } 618 619 public String getSystem() { 620 switch (this) { 621 case ROUTINE: 622 return "http://hl7.org/fhir/request-priority"; 623 case URGENT: 624 return "http://hl7.org/fhir/request-priority"; 625 case ASAP: 626 return "http://hl7.org/fhir/request-priority"; 627 case STAT: 628 return "http://hl7.org/fhir/request-priority"; 629 case NULL: 630 return null; 631 default: 632 return "?"; 633 } 634 } 635 636 public String getDefinition() { 637 switch (this) { 638 case ROUTINE: 639 return "The request has normal priority."; 640 case URGENT: 641 return "The request should be actioned promptly - higher priority than routine."; 642 case ASAP: 643 return "The request should be actioned as soon as possible - higher priority than urgent."; 644 case STAT: 645 return "The request should be actioned immediately - highest possible priority. E.g. an emergency."; 646 case NULL: 647 return null; 648 default: 649 return "?"; 650 } 651 } 652 653 public String getDisplay() { 654 switch (this) { 655 case ROUTINE: 656 return "Routine"; 657 case URGENT: 658 return "Urgent"; 659 case ASAP: 660 return "ASAP"; 661 case STAT: 662 return "STAT"; 663 case NULL: 664 return null; 665 default: 666 return "?"; 667 } 668 } 669 } 670 671 public static class RequestPriorityEnumFactory implements EnumFactory<RequestPriority> { 672 public RequestPriority fromCode(String codeString) throws IllegalArgumentException { 673 if (codeString == null || "".equals(codeString)) 674 if (codeString == null || "".equals(codeString)) 675 return null; 676 if ("routine".equals(codeString)) 677 return RequestPriority.ROUTINE; 678 if ("urgent".equals(codeString)) 679 return RequestPriority.URGENT; 680 if ("asap".equals(codeString)) 681 return RequestPriority.ASAP; 682 if ("stat".equals(codeString)) 683 return RequestPriority.STAT; 684 throw new IllegalArgumentException("Unknown RequestPriority code '" + codeString + "'"); 685 } 686 687 public Enumeration<RequestPriority> fromType(PrimitiveType<?> code) throws FHIRException { 688 if (code == null) 689 return null; 690 if (code.isEmpty()) 691 return new Enumeration<RequestPriority>(this, RequestPriority.NULL, code); 692 String codeString = code.asStringValue(); 693 if (codeString == null || "".equals(codeString)) 694 return new Enumeration<RequestPriority>(this, RequestPriority.NULL, code); 695 if ("routine".equals(codeString)) 696 return new Enumeration<RequestPriority>(this, RequestPriority.ROUTINE, code); 697 if ("urgent".equals(codeString)) 698 return new Enumeration<RequestPriority>(this, RequestPriority.URGENT, code); 699 if ("asap".equals(codeString)) 700 return new Enumeration<RequestPriority>(this, RequestPriority.ASAP, code); 701 if ("stat".equals(codeString)) 702 return new Enumeration<RequestPriority>(this, RequestPriority.STAT, code); 703 throw new FHIRException("Unknown RequestPriority code '" + codeString + "'"); 704 } 705 706 public String toCode(RequestPriority code) { 707 if (code == RequestPriority.NULL) 708 return null; 709 if (code == RequestPriority.ROUTINE) 710 return "routine"; 711 if (code == RequestPriority.URGENT) 712 return "urgent"; 713 if (code == RequestPriority.ASAP) 714 return "asap"; 715 if (code == RequestPriority.STAT) 716 return "stat"; 717 return "?"; 718 } 719 720 public String toSystem(RequestPriority code) { 721 return code.getSystem(); 722 } 723 } 724 725 public enum ActionConditionKind { 726 /** 727 * The condition describes whether or not a given action is applicable. 728 */ 729 APPLICABILITY, 730 /** 731 * The condition is a starting condition for the action. 732 */ 733 START, 734 /** 735 * The condition is a stop, or exit condition for the action. 736 */ 737 STOP, 738 /** 739 * added to help the parsers with the generic types 740 */ 741 NULL; 742 743 public static ActionConditionKind fromCode(String codeString) throws FHIRException { 744 if (codeString == null || "".equals(codeString)) 745 return null; 746 if ("applicability".equals(codeString)) 747 return APPLICABILITY; 748 if ("start".equals(codeString)) 749 return START; 750 if ("stop".equals(codeString)) 751 return STOP; 752 if (Configuration.isAcceptInvalidEnums()) 753 return null; 754 else 755 throw new FHIRException("Unknown ActionConditionKind code '" + codeString + "'"); 756 } 757 758 public String toCode() { 759 switch (this) { 760 case APPLICABILITY: 761 return "applicability"; 762 case START: 763 return "start"; 764 case STOP: 765 return "stop"; 766 case NULL: 767 return null; 768 default: 769 return "?"; 770 } 771 } 772 773 public String getSystem() { 774 switch (this) { 775 case APPLICABILITY: 776 return "http://hl7.org/fhir/action-condition-kind"; 777 case START: 778 return "http://hl7.org/fhir/action-condition-kind"; 779 case STOP: 780 return "http://hl7.org/fhir/action-condition-kind"; 781 case NULL: 782 return null; 783 default: 784 return "?"; 785 } 786 } 787 788 public String getDefinition() { 789 switch (this) { 790 case APPLICABILITY: 791 return "The condition describes whether or not a given action is applicable."; 792 case START: 793 return "The condition is a starting condition for the action."; 794 case STOP: 795 return "The condition is a stop, or exit condition for the action."; 796 case NULL: 797 return null; 798 default: 799 return "?"; 800 } 801 } 802 803 public String getDisplay() { 804 switch (this) { 805 case APPLICABILITY: 806 return "Applicability"; 807 case START: 808 return "Start"; 809 case STOP: 810 return "Stop"; 811 case NULL: 812 return null; 813 default: 814 return "?"; 815 } 816 } 817 } 818 819 public static class ActionConditionKindEnumFactory implements EnumFactory<ActionConditionKind> { 820 public ActionConditionKind fromCode(String codeString) throws IllegalArgumentException { 821 if (codeString == null || "".equals(codeString)) 822 if (codeString == null || "".equals(codeString)) 823 return null; 824 if ("applicability".equals(codeString)) 825 return ActionConditionKind.APPLICABILITY; 826 if ("start".equals(codeString)) 827 return ActionConditionKind.START; 828 if ("stop".equals(codeString)) 829 return ActionConditionKind.STOP; 830 throw new IllegalArgumentException("Unknown ActionConditionKind code '" + codeString + "'"); 831 } 832 833 public Enumeration<ActionConditionKind> fromType(PrimitiveType<?> code) throws FHIRException { 834 if (code == null) 835 return null; 836 if (code.isEmpty()) 837 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.NULL, code); 838 String codeString = code.asStringValue(); 839 if (codeString == null || "".equals(codeString)) 840 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.NULL, code); 841 if ("applicability".equals(codeString)) 842 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.APPLICABILITY, code); 843 if ("start".equals(codeString)) 844 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.START, code); 845 if ("stop".equals(codeString)) 846 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.STOP, code); 847 throw new FHIRException("Unknown ActionConditionKind code '" + codeString + "'"); 848 } 849 850 public String toCode(ActionConditionKind code) { 851 if (code == ActionConditionKind.NULL) 852 return null; 853 if (code == ActionConditionKind.APPLICABILITY) 854 return "applicability"; 855 if (code == ActionConditionKind.START) 856 return "start"; 857 if (code == ActionConditionKind.STOP) 858 return "stop"; 859 return "?"; 860 } 861 862 public String toSystem(ActionConditionKind code) { 863 return code.getSystem(); 864 } 865 } 866 867 public enum ActionRelationshipType { 868 /** 869 * The action must be performed before the start of the related action. 870 */ 871 BEFORESTART, 872 /** 873 * The action must be performed before the related action. 874 */ 875 BEFORE, 876 /** 877 * The action must be performed before the end of the related action. 878 */ 879 BEFOREEND, 880 /** 881 * The action must be performed concurrent with the start of the related action. 882 */ 883 CONCURRENTWITHSTART, 884 /** 885 * The action must be performed concurrent with the related action. 886 */ 887 CONCURRENT, 888 /** 889 * The action must be performed concurrent with the end of the related action. 890 */ 891 CONCURRENTWITHEND, 892 /** 893 * The action must be performed after the start of the related action. 894 */ 895 AFTERSTART, 896 /** 897 * The action must be performed after the related action. 898 */ 899 AFTER, 900 /** 901 * The action must be performed after the end of the related action. 902 */ 903 AFTEREND, 904 /** 905 * added to help the parsers with the generic types 906 */ 907 NULL; 908 909 public static ActionRelationshipType fromCode(String codeString) throws FHIRException { 910 if (codeString == null || "".equals(codeString)) 911 return null; 912 if ("before-start".equals(codeString)) 913 return BEFORESTART; 914 if ("before".equals(codeString)) 915 return BEFORE; 916 if ("before-end".equals(codeString)) 917 return BEFOREEND; 918 if ("concurrent-with-start".equals(codeString)) 919 return CONCURRENTWITHSTART; 920 if ("concurrent".equals(codeString)) 921 return CONCURRENT; 922 if ("concurrent-with-end".equals(codeString)) 923 return CONCURRENTWITHEND; 924 if ("after-start".equals(codeString)) 925 return AFTERSTART; 926 if ("after".equals(codeString)) 927 return AFTER; 928 if ("after-end".equals(codeString)) 929 return AFTEREND; 930 if (Configuration.isAcceptInvalidEnums()) 931 return null; 932 else 933 throw new FHIRException("Unknown ActionRelationshipType code '" + codeString + "'"); 934 } 935 936 public String toCode() { 937 switch (this) { 938 case BEFORESTART: 939 return "before-start"; 940 case BEFORE: 941 return "before"; 942 case BEFOREEND: 943 return "before-end"; 944 case CONCURRENTWITHSTART: 945 return "concurrent-with-start"; 946 case CONCURRENT: 947 return "concurrent"; 948 case CONCURRENTWITHEND: 949 return "concurrent-with-end"; 950 case AFTERSTART: 951 return "after-start"; 952 case AFTER: 953 return "after"; 954 case AFTEREND: 955 return "after-end"; 956 case NULL: 957 return null; 958 default: 959 return "?"; 960 } 961 } 962 963 public String getSystem() { 964 switch (this) { 965 case BEFORESTART: 966 return "http://hl7.org/fhir/action-relationship-type"; 967 case BEFORE: 968 return "http://hl7.org/fhir/action-relationship-type"; 969 case BEFOREEND: 970 return "http://hl7.org/fhir/action-relationship-type"; 971 case CONCURRENTWITHSTART: 972 return "http://hl7.org/fhir/action-relationship-type"; 973 case CONCURRENT: 974 return "http://hl7.org/fhir/action-relationship-type"; 975 case CONCURRENTWITHEND: 976 return "http://hl7.org/fhir/action-relationship-type"; 977 case AFTERSTART: 978 return "http://hl7.org/fhir/action-relationship-type"; 979 case AFTER: 980 return "http://hl7.org/fhir/action-relationship-type"; 981 case AFTEREND: 982 return "http://hl7.org/fhir/action-relationship-type"; 983 case NULL: 984 return null; 985 default: 986 return "?"; 987 } 988 } 989 990 public String getDefinition() { 991 switch (this) { 992 case BEFORESTART: 993 return "The action must be performed before the start of the related action."; 994 case BEFORE: 995 return "The action must be performed before the related action."; 996 case BEFOREEND: 997 return "The action must be performed before the end of the related action."; 998 case CONCURRENTWITHSTART: 999 return "The action must be performed concurrent with the start of the related action."; 1000 case CONCURRENT: 1001 return "The action must be performed concurrent with the related action."; 1002 case CONCURRENTWITHEND: 1003 return "The action must be performed concurrent with the end of the related action."; 1004 case AFTERSTART: 1005 return "The action must be performed after the start of the related action."; 1006 case AFTER: 1007 return "The action must be performed after the related action."; 1008 case AFTEREND: 1009 return "The action must be performed after the end of the related action."; 1010 case NULL: 1011 return null; 1012 default: 1013 return "?"; 1014 } 1015 } 1016 1017 public String getDisplay() { 1018 switch (this) { 1019 case BEFORESTART: 1020 return "Before Start"; 1021 case BEFORE: 1022 return "Before"; 1023 case BEFOREEND: 1024 return "Before End"; 1025 case CONCURRENTWITHSTART: 1026 return "Concurrent With Start"; 1027 case CONCURRENT: 1028 return "Concurrent"; 1029 case CONCURRENTWITHEND: 1030 return "Concurrent With End"; 1031 case AFTERSTART: 1032 return "After Start"; 1033 case AFTER: 1034 return "After"; 1035 case AFTEREND: 1036 return "After End"; 1037 case NULL: 1038 return null; 1039 default: 1040 return "?"; 1041 } 1042 } 1043 } 1044 1045 public static class ActionRelationshipTypeEnumFactory implements EnumFactory<ActionRelationshipType> { 1046 public ActionRelationshipType fromCode(String codeString) throws IllegalArgumentException { 1047 if (codeString == null || "".equals(codeString)) 1048 if (codeString == null || "".equals(codeString)) 1049 return null; 1050 if ("before-start".equals(codeString)) 1051 return ActionRelationshipType.BEFORESTART; 1052 if ("before".equals(codeString)) 1053 return ActionRelationshipType.BEFORE; 1054 if ("before-end".equals(codeString)) 1055 return ActionRelationshipType.BEFOREEND; 1056 if ("concurrent-with-start".equals(codeString)) 1057 return ActionRelationshipType.CONCURRENTWITHSTART; 1058 if ("concurrent".equals(codeString)) 1059 return ActionRelationshipType.CONCURRENT; 1060 if ("concurrent-with-end".equals(codeString)) 1061 return ActionRelationshipType.CONCURRENTWITHEND; 1062 if ("after-start".equals(codeString)) 1063 return ActionRelationshipType.AFTERSTART; 1064 if ("after".equals(codeString)) 1065 return ActionRelationshipType.AFTER; 1066 if ("after-end".equals(codeString)) 1067 return ActionRelationshipType.AFTEREND; 1068 throw new IllegalArgumentException("Unknown ActionRelationshipType code '" + codeString + "'"); 1069 } 1070 1071 public Enumeration<ActionRelationshipType> fromType(PrimitiveType<?> code) throws FHIRException { 1072 if (code == null) 1073 return null; 1074 if (code.isEmpty()) 1075 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.NULL, code); 1076 String codeString = code.asStringValue(); 1077 if (codeString == null || "".equals(codeString)) 1078 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.NULL, code); 1079 if ("before-start".equals(codeString)) 1080 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.BEFORESTART, code); 1081 if ("before".equals(codeString)) 1082 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.BEFORE, code); 1083 if ("before-end".equals(codeString)) 1084 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.BEFOREEND, code); 1085 if ("concurrent-with-start".equals(codeString)) 1086 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.CONCURRENTWITHSTART, code); 1087 if ("concurrent".equals(codeString)) 1088 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.CONCURRENT, code); 1089 if ("concurrent-with-end".equals(codeString)) 1090 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.CONCURRENTWITHEND, code); 1091 if ("after-start".equals(codeString)) 1092 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.AFTERSTART, code); 1093 if ("after".equals(codeString)) 1094 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.AFTER, code); 1095 if ("after-end".equals(codeString)) 1096 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.AFTEREND, code); 1097 throw new FHIRException("Unknown ActionRelationshipType code '" + codeString + "'"); 1098 } 1099 1100 public String toCode(ActionRelationshipType code) { 1101 if (code == ActionRelationshipType.NULL) 1102 return null; 1103 if (code == ActionRelationshipType.BEFORESTART) 1104 return "before-start"; 1105 if (code == ActionRelationshipType.BEFORE) 1106 return "before"; 1107 if (code == ActionRelationshipType.BEFOREEND) 1108 return "before-end"; 1109 if (code == ActionRelationshipType.CONCURRENTWITHSTART) 1110 return "concurrent-with-start"; 1111 if (code == ActionRelationshipType.CONCURRENT) 1112 return "concurrent"; 1113 if (code == ActionRelationshipType.CONCURRENTWITHEND) 1114 return "concurrent-with-end"; 1115 if (code == ActionRelationshipType.AFTERSTART) 1116 return "after-start"; 1117 if (code == ActionRelationshipType.AFTER) 1118 return "after"; 1119 if (code == ActionRelationshipType.AFTEREND) 1120 return "after-end"; 1121 return "?"; 1122 } 1123 1124 public String toSystem(ActionRelationshipType code) { 1125 return code.getSystem(); 1126 } 1127 } 1128 1129 public enum ActionGroupingBehavior { 1130 /** 1131 * Any group marked with this behavior should be displayed as a visual group to 1132 * the end user. 1133 */ 1134 VISUALGROUP, 1135 /** 1136 * A group with this behavior logically groups its sub-elements, and may be 1137 * shown as a visual group to the end user, but it is not required to do so. 1138 */ 1139 LOGICALGROUP, 1140 /** 1141 * A group of related alternative actions is a sentence group if the target 1142 * referenced by the action is the same in all the actions and each action 1143 * simply constitutes a different variation on how to specify the details for 1144 * the target. For example, two actions that could be in a SentenceGroup are 1145 * "aspirin, 500 mg, 2 times per day" and "aspirin, 300 mg, 3 times per day". In 1146 * both cases, aspirin is the target referenced by the action, and the two 1147 * actions represent different options for how aspirin might be ordered for the 1148 * patient. Note that a SentenceGroup would almost always have an associated 1149 * selection behavior of "AtMostOne", unless it's a required action, in which 1150 * case, it would be "ExactlyOne". 1151 */ 1152 SENTENCEGROUP, 1153 /** 1154 * added to help the parsers with the generic types 1155 */ 1156 NULL; 1157 1158 public static ActionGroupingBehavior fromCode(String codeString) throws FHIRException { 1159 if (codeString == null || "".equals(codeString)) 1160 return null; 1161 if ("visual-group".equals(codeString)) 1162 return VISUALGROUP; 1163 if ("logical-group".equals(codeString)) 1164 return LOGICALGROUP; 1165 if ("sentence-group".equals(codeString)) 1166 return SENTENCEGROUP; 1167 if (Configuration.isAcceptInvalidEnums()) 1168 return null; 1169 else 1170 throw new FHIRException("Unknown ActionGroupingBehavior code '" + codeString + "'"); 1171 } 1172 1173 public String toCode() { 1174 switch (this) { 1175 case VISUALGROUP: 1176 return "visual-group"; 1177 case LOGICALGROUP: 1178 return "logical-group"; 1179 case SENTENCEGROUP: 1180 return "sentence-group"; 1181 case NULL: 1182 return null; 1183 default: 1184 return "?"; 1185 } 1186 } 1187 1188 public String getSystem() { 1189 switch (this) { 1190 case VISUALGROUP: 1191 return "http://hl7.org/fhir/action-grouping-behavior"; 1192 case LOGICALGROUP: 1193 return "http://hl7.org/fhir/action-grouping-behavior"; 1194 case SENTENCEGROUP: 1195 return "http://hl7.org/fhir/action-grouping-behavior"; 1196 case NULL: 1197 return null; 1198 default: 1199 return "?"; 1200 } 1201 } 1202 1203 public String getDefinition() { 1204 switch (this) { 1205 case VISUALGROUP: 1206 return "Any group marked with this behavior should be displayed as a visual group to the end user."; 1207 case LOGICALGROUP: 1208 return "A group with this behavior logically groups its sub-elements, and may be shown as a visual group to the end user, but it is not required to do so."; 1209 case SENTENCEGROUP: 1210 return "A group of related alternative actions is a sentence group if the target referenced by the action is the same in all the actions and each action simply constitutes a different variation on how to specify the details for the target. For example, two actions that could be in a SentenceGroup are \"aspirin, 500 mg, 2 times per day\" and \"aspirin, 300 mg, 3 times per day\". In both cases, aspirin is the target referenced by the action, and the two actions represent different options for how aspirin might be ordered for the patient. Note that a SentenceGroup would almost always have an associated selection behavior of \"AtMostOne\", unless it's a required action, in which case, it would be \"ExactlyOne\"."; 1211 case NULL: 1212 return null; 1213 default: 1214 return "?"; 1215 } 1216 } 1217 1218 public String getDisplay() { 1219 switch (this) { 1220 case VISUALGROUP: 1221 return "Visual Group"; 1222 case LOGICALGROUP: 1223 return "Logical Group"; 1224 case SENTENCEGROUP: 1225 return "Sentence Group"; 1226 case NULL: 1227 return null; 1228 default: 1229 return "?"; 1230 } 1231 } 1232 } 1233 1234 public static class ActionGroupingBehaviorEnumFactory implements EnumFactory<ActionGroupingBehavior> { 1235 public ActionGroupingBehavior fromCode(String codeString) throws IllegalArgumentException { 1236 if (codeString == null || "".equals(codeString)) 1237 if (codeString == null || "".equals(codeString)) 1238 return null; 1239 if ("visual-group".equals(codeString)) 1240 return ActionGroupingBehavior.VISUALGROUP; 1241 if ("logical-group".equals(codeString)) 1242 return ActionGroupingBehavior.LOGICALGROUP; 1243 if ("sentence-group".equals(codeString)) 1244 return ActionGroupingBehavior.SENTENCEGROUP; 1245 throw new IllegalArgumentException("Unknown ActionGroupingBehavior code '" + codeString + "'"); 1246 } 1247 1248 public Enumeration<ActionGroupingBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 1249 if (code == null) 1250 return null; 1251 if (code.isEmpty()) 1252 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.NULL, code); 1253 String codeString = code.asStringValue(); 1254 if (codeString == null || "".equals(codeString)) 1255 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.NULL, code); 1256 if ("visual-group".equals(codeString)) 1257 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.VISUALGROUP, code); 1258 if ("logical-group".equals(codeString)) 1259 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.LOGICALGROUP, code); 1260 if ("sentence-group".equals(codeString)) 1261 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.SENTENCEGROUP, code); 1262 throw new FHIRException("Unknown ActionGroupingBehavior code '" + codeString + "'"); 1263 } 1264 1265 public String toCode(ActionGroupingBehavior code) { 1266 if (code == ActionGroupingBehavior.NULL) 1267 return null; 1268 if (code == ActionGroupingBehavior.VISUALGROUP) 1269 return "visual-group"; 1270 if (code == ActionGroupingBehavior.LOGICALGROUP) 1271 return "logical-group"; 1272 if (code == ActionGroupingBehavior.SENTENCEGROUP) 1273 return "sentence-group"; 1274 return "?"; 1275 } 1276 1277 public String toSystem(ActionGroupingBehavior code) { 1278 return code.getSystem(); 1279 } 1280 } 1281 1282 public enum ActionSelectionBehavior { 1283 /** 1284 * Any number of the actions in the group may be chosen, from zero to all. 1285 */ 1286 ANY, 1287 /** 1288 * All the actions in the group must be selected as a single unit. 1289 */ 1290 ALL, 1291 /** 1292 * All the actions in the group are meant to be chosen as a single unit: either 1293 * all must be selected by the end user, or none may be selected. 1294 */ 1295 ALLORNONE, 1296 /** 1297 * The end user must choose one and only one of the selectable actions in the 1298 * group. The user SHALL NOT choose none of the actions in the group. 1299 */ 1300 EXACTLYONE, 1301 /** 1302 * The end user may choose zero or at most one of the actions in the group. 1303 */ 1304 ATMOSTONE, 1305 /** 1306 * The end user must choose a minimum of one, and as many additional as desired. 1307 */ 1308 ONEORMORE, 1309 /** 1310 * added to help the parsers with the generic types 1311 */ 1312 NULL; 1313 1314 public static ActionSelectionBehavior fromCode(String codeString) throws FHIRException { 1315 if (codeString == null || "".equals(codeString)) 1316 return null; 1317 if ("any".equals(codeString)) 1318 return ANY; 1319 if ("all".equals(codeString)) 1320 return ALL; 1321 if ("all-or-none".equals(codeString)) 1322 return ALLORNONE; 1323 if ("exactly-one".equals(codeString)) 1324 return EXACTLYONE; 1325 if ("at-most-one".equals(codeString)) 1326 return ATMOSTONE; 1327 if ("one-or-more".equals(codeString)) 1328 return ONEORMORE; 1329 if (Configuration.isAcceptInvalidEnums()) 1330 return null; 1331 else 1332 throw new FHIRException("Unknown ActionSelectionBehavior code '" + codeString + "'"); 1333 } 1334 1335 public String toCode() { 1336 switch (this) { 1337 case ANY: 1338 return "any"; 1339 case ALL: 1340 return "all"; 1341 case ALLORNONE: 1342 return "all-or-none"; 1343 case EXACTLYONE: 1344 return "exactly-one"; 1345 case ATMOSTONE: 1346 return "at-most-one"; 1347 case ONEORMORE: 1348 return "one-or-more"; 1349 case NULL: 1350 return null; 1351 default: 1352 return "?"; 1353 } 1354 } 1355 1356 public String getSystem() { 1357 switch (this) { 1358 case ANY: 1359 return "http://hl7.org/fhir/action-selection-behavior"; 1360 case ALL: 1361 return "http://hl7.org/fhir/action-selection-behavior"; 1362 case ALLORNONE: 1363 return "http://hl7.org/fhir/action-selection-behavior"; 1364 case EXACTLYONE: 1365 return "http://hl7.org/fhir/action-selection-behavior"; 1366 case ATMOSTONE: 1367 return "http://hl7.org/fhir/action-selection-behavior"; 1368 case ONEORMORE: 1369 return "http://hl7.org/fhir/action-selection-behavior"; 1370 case NULL: 1371 return null; 1372 default: 1373 return "?"; 1374 } 1375 } 1376 1377 public String getDefinition() { 1378 switch (this) { 1379 case ANY: 1380 return "Any number of the actions in the group may be chosen, from zero to all."; 1381 case ALL: 1382 return "All the actions in the group must be selected as a single unit."; 1383 case ALLORNONE: 1384 return "All the actions in the group are meant to be chosen as a single unit: either all must be selected by the end user, or none may be selected."; 1385 case EXACTLYONE: 1386 return "The end user must choose one and only one of the selectable actions in the group. The user SHALL NOT choose none of the actions in the group."; 1387 case ATMOSTONE: 1388 return "The end user may choose zero or at most one of the actions in the group."; 1389 case ONEORMORE: 1390 return "The end user must choose a minimum of one, and as many additional as desired."; 1391 case NULL: 1392 return null; 1393 default: 1394 return "?"; 1395 } 1396 } 1397 1398 public String getDisplay() { 1399 switch (this) { 1400 case ANY: 1401 return "Any"; 1402 case ALL: 1403 return "All"; 1404 case ALLORNONE: 1405 return "All Or None"; 1406 case EXACTLYONE: 1407 return "Exactly One"; 1408 case ATMOSTONE: 1409 return "At Most One"; 1410 case ONEORMORE: 1411 return "One Or More"; 1412 case NULL: 1413 return null; 1414 default: 1415 return "?"; 1416 } 1417 } 1418 } 1419 1420 public static class ActionSelectionBehaviorEnumFactory implements EnumFactory<ActionSelectionBehavior> { 1421 public ActionSelectionBehavior fromCode(String codeString) throws IllegalArgumentException { 1422 if (codeString == null || "".equals(codeString)) 1423 if (codeString == null || "".equals(codeString)) 1424 return null; 1425 if ("any".equals(codeString)) 1426 return ActionSelectionBehavior.ANY; 1427 if ("all".equals(codeString)) 1428 return ActionSelectionBehavior.ALL; 1429 if ("all-or-none".equals(codeString)) 1430 return ActionSelectionBehavior.ALLORNONE; 1431 if ("exactly-one".equals(codeString)) 1432 return ActionSelectionBehavior.EXACTLYONE; 1433 if ("at-most-one".equals(codeString)) 1434 return ActionSelectionBehavior.ATMOSTONE; 1435 if ("one-or-more".equals(codeString)) 1436 return ActionSelectionBehavior.ONEORMORE; 1437 throw new IllegalArgumentException("Unknown ActionSelectionBehavior code '" + codeString + "'"); 1438 } 1439 1440 public Enumeration<ActionSelectionBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 1441 if (code == null) 1442 return null; 1443 if (code.isEmpty()) 1444 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.NULL, code); 1445 String codeString = code.asStringValue(); 1446 if (codeString == null || "".equals(codeString)) 1447 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.NULL, code); 1448 if ("any".equals(codeString)) 1449 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ANY, code); 1450 if ("all".equals(codeString)) 1451 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ALL, code); 1452 if ("all-or-none".equals(codeString)) 1453 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ALLORNONE, code); 1454 if ("exactly-one".equals(codeString)) 1455 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.EXACTLYONE, code); 1456 if ("at-most-one".equals(codeString)) 1457 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ATMOSTONE, code); 1458 if ("one-or-more".equals(codeString)) 1459 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ONEORMORE, code); 1460 throw new FHIRException("Unknown ActionSelectionBehavior code '" + codeString + "'"); 1461 } 1462 1463 public String toCode(ActionSelectionBehavior code) { 1464 if (code == ActionSelectionBehavior.NULL) 1465 return null; 1466 if (code == ActionSelectionBehavior.ANY) 1467 return "any"; 1468 if (code == ActionSelectionBehavior.ALL) 1469 return "all"; 1470 if (code == ActionSelectionBehavior.ALLORNONE) 1471 return "all-or-none"; 1472 if (code == ActionSelectionBehavior.EXACTLYONE) 1473 return "exactly-one"; 1474 if (code == ActionSelectionBehavior.ATMOSTONE) 1475 return "at-most-one"; 1476 if (code == ActionSelectionBehavior.ONEORMORE) 1477 return "one-or-more"; 1478 return "?"; 1479 } 1480 1481 public String toSystem(ActionSelectionBehavior code) { 1482 return code.getSystem(); 1483 } 1484 } 1485 1486 public enum ActionRequiredBehavior { 1487 /** 1488 * An action with this behavior must be included in the actions processed by the 1489 * end user; the end user SHALL NOT choose not to include this action. 1490 */ 1491 MUST, 1492 /** 1493 * An action with this behavior may be included in the set of actions processed 1494 * by the end user. 1495 */ 1496 COULD, 1497 /** 1498 * An action with this behavior must be included in the set of actions processed 1499 * by the end user, unless the end user provides documentation as to why the 1500 * action was not included. 1501 */ 1502 MUSTUNLESSDOCUMENTED, 1503 /** 1504 * added to help the parsers with the generic types 1505 */ 1506 NULL; 1507 1508 public static ActionRequiredBehavior fromCode(String codeString) throws FHIRException { 1509 if (codeString == null || "".equals(codeString)) 1510 return null; 1511 if ("must".equals(codeString)) 1512 return MUST; 1513 if ("could".equals(codeString)) 1514 return COULD; 1515 if ("must-unless-documented".equals(codeString)) 1516 return MUSTUNLESSDOCUMENTED; 1517 if (Configuration.isAcceptInvalidEnums()) 1518 return null; 1519 else 1520 throw new FHIRException("Unknown ActionRequiredBehavior code '" + codeString + "'"); 1521 } 1522 1523 public String toCode() { 1524 switch (this) { 1525 case MUST: 1526 return "must"; 1527 case COULD: 1528 return "could"; 1529 case MUSTUNLESSDOCUMENTED: 1530 return "must-unless-documented"; 1531 case NULL: 1532 return null; 1533 default: 1534 return "?"; 1535 } 1536 } 1537 1538 public String getSystem() { 1539 switch (this) { 1540 case MUST: 1541 return "http://hl7.org/fhir/action-required-behavior"; 1542 case COULD: 1543 return "http://hl7.org/fhir/action-required-behavior"; 1544 case MUSTUNLESSDOCUMENTED: 1545 return "http://hl7.org/fhir/action-required-behavior"; 1546 case NULL: 1547 return null; 1548 default: 1549 return "?"; 1550 } 1551 } 1552 1553 public String getDefinition() { 1554 switch (this) { 1555 case MUST: 1556 return "An action with this behavior must be included in the actions processed by the end user; the end user SHALL NOT choose not to include this action."; 1557 case COULD: 1558 return "An action with this behavior may be included in the set of actions processed by the end user."; 1559 case MUSTUNLESSDOCUMENTED: 1560 return "An action with this behavior must be included in the set of actions processed by the end user, unless the end user provides documentation as to why the action was not included."; 1561 case NULL: 1562 return null; 1563 default: 1564 return "?"; 1565 } 1566 } 1567 1568 public String getDisplay() { 1569 switch (this) { 1570 case MUST: 1571 return "Must"; 1572 case COULD: 1573 return "Could"; 1574 case MUSTUNLESSDOCUMENTED: 1575 return "Must Unless Documented"; 1576 case NULL: 1577 return null; 1578 default: 1579 return "?"; 1580 } 1581 } 1582 } 1583 1584 public static class ActionRequiredBehaviorEnumFactory implements EnumFactory<ActionRequiredBehavior> { 1585 public ActionRequiredBehavior fromCode(String codeString) throws IllegalArgumentException { 1586 if (codeString == null || "".equals(codeString)) 1587 if (codeString == null || "".equals(codeString)) 1588 return null; 1589 if ("must".equals(codeString)) 1590 return ActionRequiredBehavior.MUST; 1591 if ("could".equals(codeString)) 1592 return ActionRequiredBehavior.COULD; 1593 if ("must-unless-documented".equals(codeString)) 1594 return ActionRequiredBehavior.MUSTUNLESSDOCUMENTED; 1595 throw new IllegalArgumentException("Unknown ActionRequiredBehavior code '" + codeString + "'"); 1596 } 1597 1598 public Enumeration<ActionRequiredBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 1599 if (code == null) 1600 return null; 1601 if (code.isEmpty()) 1602 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.NULL, code); 1603 String codeString = code.asStringValue(); 1604 if (codeString == null || "".equals(codeString)) 1605 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.NULL, code); 1606 if ("must".equals(codeString)) 1607 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.MUST, code); 1608 if ("could".equals(codeString)) 1609 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.COULD, code); 1610 if ("must-unless-documented".equals(codeString)) 1611 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.MUSTUNLESSDOCUMENTED, code); 1612 throw new FHIRException("Unknown ActionRequiredBehavior code '" + codeString + "'"); 1613 } 1614 1615 public String toCode(ActionRequiredBehavior code) { 1616 if (code == ActionRequiredBehavior.NULL) 1617 return null; 1618 if (code == ActionRequiredBehavior.MUST) 1619 return "must"; 1620 if (code == ActionRequiredBehavior.COULD) 1621 return "could"; 1622 if (code == ActionRequiredBehavior.MUSTUNLESSDOCUMENTED) 1623 return "must-unless-documented"; 1624 return "?"; 1625 } 1626 1627 public String toSystem(ActionRequiredBehavior code) { 1628 return code.getSystem(); 1629 } 1630 } 1631 1632 public enum ActionPrecheckBehavior { 1633 /** 1634 * An action with this behavior is one of the most frequent action that is, or 1635 * should be, included by an end user, for the particular context in which the 1636 * action occurs. The system displaying the action to the end user should 1637 * consider "pre-checking" such an action as a convenience for the user. 1638 */ 1639 YES, 1640 /** 1641 * An action with this behavior is one of the less frequent actions included by 1642 * the end user, for the particular context in which the action occurs. The 1643 * system displaying the actions to the end user would typically not "pre-check" 1644 * such an action. 1645 */ 1646 NO, 1647 /** 1648 * added to help the parsers with the generic types 1649 */ 1650 NULL; 1651 1652 public static ActionPrecheckBehavior fromCode(String codeString) throws FHIRException { 1653 if (codeString == null || "".equals(codeString)) 1654 return null; 1655 if ("yes".equals(codeString)) 1656 return YES; 1657 if ("no".equals(codeString)) 1658 return NO; 1659 if (Configuration.isAcceptInvalidEnums()) 1660 return null; 1661 else 1662 throw new FHIRException("Unknown ActionPrecheckBehavior code '" + codeString + "'"); 1663 } 1664 1665 public String toCode() { 1666 switch (this) { 1667 case YES: 1668 return "yes"; 1669 case NO: 1670 return "no"; 1671 case NULL: 1672 return null; 1673 default: 1674 return "?"; 1675 } 1676 } 1677 1678 public String getSystem() { 1679 switch (this) { 1680 case YES: 1681 return "http://hl7.org/fhir/action-precheck-behavior"; 1682 case NO: 1683 return "http://hl7.org/fhir/action-precheck-behavior"; 1684 case NULL: 1685 return null; 1686 default: 1687 return "?"; 1688 } 1689 } 1690 1691 public String getDefinition() { 1692 switch (this) { 1693 case YES: 1694 return "An action with this behavior is one of the most frequent action that is, or should be, included by an end user, for the particular context in which the action occurs. The system displaying the action to the end user should consider \"pre-checking\" such an action as a convenience for the user."; 1695 case NO: 1696 return "An action with this behavior is one of the less frequent actions included by the end user, for the particular context in which the action occurs. The system displaying the actions to the end user would typically not \"pre-check\" such an action."; 1697 case NULL: 1698 return null; 1699 default: 1700 return "?"; 1701 } 1702 } 1703 1704 public String getDisplay() { 1705 switch (this) { 1706 case YES: 1707 return "Yes"; 1708 case NO: 1709 return "No"; 1710 case NULL: 1711 return null; 1712 default: 1713 return "?"; 1714 } 1715 } 1716 } 1717 1718 public static class ActionPrecheckBehaviorEnumFactory implements EnumFactory<ActionPrecheckBehavior> { 1719 public ActionPrecheckBehavior fromCode(String codeString) throws IllegalArgumentException { 1720 if (codeString == null || "".equals(codeString)) 1721 if (codeString == null || "".equals(codeString)) 1722 return null; 1723 if ("yes".equals(codeString)) 1724 return ActionPrecheckBehavior.YES; 1725 if ("no".equals(codeString)) 1726 return ActionPrecheckBehavior.NO; 1727 throw new IllegalArgumentException("Unknown ActionPrecheckBehavior code '" + codeString + "'"); 1728 } 1729 1730 public Enumeration<ActionPrecheckBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 1731 if (code == null) 1732 return null; 1733 if (code.isEmpty()) 1734 return new Enumeration<ActionPrecheckBehavior>(this, ActionPrecheckBehavior.NULL, code); 1735 String codeString = code.asStringValue(); 1736 if (codeString == null || "".equals(codeString)) 1737 return new Enumeration<ActionPrecheckBehavior>(this, ActionPrecheckBehavior.NULL, code); 1738 if ("yes".equals(codeString)) 1739 return new Enumeration<ActionPrecheckBehavior>(this, ActionPrecheckBehavior.YES, code); 1740 if ("no".equals(codeString)) 1741 return new Enumeration<ActionPrecheckBehavior>(this, ActionPrecheckBehavior.NO, code); 1742 throw new FHIRException("Unknown ActionPrecheckBehavior code '" + codeString + "'"); 1743 } 1744 1745 public String toCode(ActionPrecheckBehavior code) { 1746 if (code == ActionPrecheckBehavior.NULL) 1747 return null; 1748 if (code == ActionPrecheckBehavior.YES) 1749 return "yes"; 1750 if (code == ActionPrecheckBehavior.NO) 1751 return "no"; 1752 return "?"; 1753 } 1754 1755 public String toSystem(ActionPrecheckBehavior code) { 1756 return code.getSystem(); 1757 } 1758 } 1759 1760 public enum ActionCardinalityBehavior { 1761 /** 1762 * The action may only be selected one time. 1763 */ 1764 SINGLE, 1765 /** 1766 * The action may be selected multiple times. 1767 */ 1768 MULTIPLE, 1769 /** 1770 * added to help the parsers with the generic types 1771 */ 1772 NULL; 1773 1774 public static ActionCardinalityBehavior fromCode(String codeString) throws FHIRException { 1775 if (codeString == null || "".equals(codeString)) 1776 return null; 1777 if ("single".equals(codeString)) 1778 return SINGLE; 1779 if ("multiple".equals(codeString)) 1780 return MULTIPLE; 1781 if (Configuration.isAcceptInvalidEnums()) 1782 return null; 1783 else 1784 throw new FHIRException("Unknown ActionCardinalityBehavior code '" + codeString + "'"); 1785 } 1786 1787 public String toCode() { 1788 switch (this) { 1789 case SINGLE: 1790 return "single"; 1791 case MULTIPLE: 1792 return "multiple"; 1793 case NULL: 1794 return null; 1795 default: 1796 return "?"; 1797 } 1798 } 1799 1800 public String getSystem() { 1801 switch (this) { 1802 case SINGLE: 1803 return "http://hl7.org/fhir/action-cardinality-behavior"; 1804 case MULTIPLE: 1805 return "http://hl7.org/fhir/action-cardinality-behavior"; 1806 case NULL: 1807 return null; 1808 default: 1809 return "?"; 1810 } 1811 } 1812 1813 public String getDefinition() { 1814 switch (this) { 1815 case SINGLE: 1816 return "The action may only be selected one time."; 1817 case MULTIPLE: 1818 return "The action may be selected multiple times."; 1819 case NULL: 1820 return null; 1821 default: 1822 return "?"; 1823 } 1824 } 1825 1826 public String getDisplay() { 1827 switch (this) { 1828 case SINGLE: 1829 return "Single"; 1830 case MULTIPLE: 1831 return "Multiple"; 1832 case NULL: 1833 return null; 1834 default: 1835 return "?"; 1836 } 1837 } 1838 } 1839 1840 public static class ActionCardinalityBehaviorEnumFactory implements EnumFactory<ActionCardinalityBehavior> { 1841 public ActionCardinalityBehavior fromCode(String codeString) throws IllegalArgumentException { 1842 if (codeString == null || "".equals(codeString)) 1843 if (codeString == null || "".equals(codeString)) 1844 return null; 1845 if ("single".equals(codeString)) 1846 return ActionCardinalityBehavior.SINGLE; 1847 if ("multiple".equals(codeString)) 1848 return ActionCardinalityBehavior.MULTIPLE; 1849 throw new IllegalArgumentException("Unknown ActionCardinalityBehavior code '" + codeString + "'"); 1850 } 1851 1852 public Enumeration<ActionCardinalityBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 1853 if (code == null) 1854 return null; 1855 if (code.isEmpty()) 1856 return new Enumeration<ActionCardinalityBehavior>(this, ActionCardinalityBehavior.NULL, code); 1857 String codeString = code.asStringValue(); 1858 if (codeString == null || "".equals(codeString)) 1859 return new Enumeration<ActionCardinalityBehavior>(this, ActionCardinalityBehavior.NULL, code); 1860 if ("single".equals(codeString)) 1861 return new Enumeration<ActionCardinalityBehavior>(this, ActionCardinalityBehavior.SINGLE, code); 1862 if ("multiple".equals(codeString)) 1863 return new Enumeration<ActionCardinalityBehavior>(this, ActionCardinalityBehavior.MULTIPLE, code); 1864 throw new FHIRException("Unknown ActionCardinalityBehavior code '" + codeString + "'"); 1865 } 1866 1867 public String toCode(ActionCardinalityBehavior code) { 1868 if (code == ActionCardinalityBehavior.NULL) 1869 return null; 1870 if (code == ActionCardinalityBehavior.SINGLE) 1871 return "single"; 1872 if (code == ActionCardinalityBehavior.MULTIPLE) 1873 return "multiple"; 1874 return "?"; 1875 } 1876 1877 public String toSystem(ActionCardinalityBehavior code) { 1878 return code.getSystem(); 1879 } 1880 } 1881 1882 @Block() 1883 public static class RequestGroupActionComponent extends BackboneElement implements IBaseBackboneElement { 1884 /** 1885 * A user-visible prefix for the action. 1886 */ 1887 @Child(name = "prefix", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1888 @Description(shortDefinition = "User-visible prefix for the action (e.g. 1. or A.)", formalDefinition = "A user-visible prefix for the action.") 1889 protected StringType prefix; 1890 1891 /** 1892 * The title of the action displayed to a user. 1893 */ 1894 @Child(name = "title", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1895 @Description(shortDefinition = "User-visible title", formalDefinition = "The title of the action displayed to a user.") 1896 protected StringType title; 1897 1898 /** 1899 * A short description of the action used to provide a summary to display to the 1900 * user. 1901 */ 1902 @Child(name = "description", type = { 1903 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1904 @Description(shortDefinition = "Short description of the action", formalDefinition = "A short description of the action used to provide a summary to display to the user.") 1905 protected StringType description; 1906 1907 /** 1908 * A text equivalent of the action to be performed. This provides a 1909 * human-interpretable description of the action when the definition is consumed 1910 * by a system that might not be capable of interpreting it dynamically. 1911 */ 1912 @Child(name = "textEquivalent", type = { 1913 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1914 @Description(shortDefinition = "Static text equivalent of the action, used if the dynamic aspects cannot be interpreted by the receiving system", formalDefinition = "A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that might not be capable of interpreting it dynamically.") 1915 protected StringType textEquivalent; 1916 1917 /** 1918 * Indicates how quickly the action should be addressed with respect to other 1919 * actions. 1920 */ 1921 @Child(name = "priority", type = { CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1922 @Description(shortDefinition = "routine | urgent | asap | stat", formalDefinition = "Indicates how quickly the action should be addressed with respect to other actions.") 1923 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-priority") 1924 protected Enumeration<RequestPriority> priority; 1925 1926 /** 1927 * A code that provides meaning for the action or action group. For example, a 1928 * section may have a LOINC code for a section of a documentation template. 1929 */ 1930 @Child(name = "code", type = { 1931 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1932 @Description(shortDefinition = "Code representing the meaning of the action or sub-actions", formalDefinition = "A code that provides meaning for the action or action group. For example, a section may have a LOINC code for a section of a documentation template.") 1933 protected List<CodeableConcept> code; 1934 1935 /** 1936 * Didactic or other informational resources associated with the action that can 1937 * be provided to the CDS recipient. Information resources can include inline 1938 * text commentary and links to web resources. 1939 */ 1940 @Child(name = "documentation", type = { 1941 RelatedArtifact.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1942 @Description(shortDefinition = "Supporting documentation for the intended performer of the action", formalDefinition = "Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources.") 1943 protected List<RelatedArtifact> documentation; 1944 1945 /** 1946 * An expression that describes applicability criteria, or start/stop conditions 1947 * for the action. 1948 */ 1949 @Child(name = "condition", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1950 @Description(shortDefinition = "Whether or not the action is applicable", formalDefinition = "An expression that describes applicability criteria, or start/stop conditions for the action.") 1951 protected List<RequestGroupActionConditionComponent> condition; 1952 1953 /** 1954 * A relationship to another action such as "before" or "30-60 minutes after 1955 * start of". 1956 */ 1957 @Child(name = "relatedAction", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1958 @Description(shortDefinition = "Relationship to another action", formalDefinition = "A relationship to another action such as \"before\" or \"30-60 minutes after start of\".") 1959 protected List<RequestGroupActionRelatedActionComponent> relatedAction; 1960 1961 /** 1962 * An optional value describing when the action should be performed. 1963 */ 1964 @Child(name = "timing", type = { DateTimeType.class, Age.class, Period.class, Duration.class, Range.class, 1965 Timing.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 1966 @Description(shortDefinition = "When the action should take place", formalDefinition = "An optional value describing when the action should be performed.") 1967 protected Type timing; 1968 1969 /** 1970 * The participant that should perform or be responsible for this action. 1971 */ 1972 @Child(name = "participant", type = { Patient.class, Practitioner.class, PractitionerRole.class, 1973 RelatedPerson.class, 1974 Device.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1975 @Description(shortDefinition = "Who should perform the action", formalDefinition = "The participant that should perform or be responsible for this action.") 1976 protected List<Reference> participant; 1977 /** 1978 * The actual objects that are the target of the reference (The participant that 1979 * should perform or be responsible for this action.) 1980 */ 1981 protected List<Resource> participantTarget; 1982 1983 /** 1984 * The type of action to perform (create, update, remove). 1985 */ 1986 @Child(name = "type", type = { 1987 CodeableConcept.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 1988 @Description(shortDefinition = "create | update | remove | fire-event", formalDefinition = "The type of action to perform (create, update, remove).") 1989 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-type") 1990 protected CodeableConcept type; 1991 1992 /** 1993 * Defines the grouping behavior for the action and its children. 1994 */ 1995 @Child(name = "groupingBehavior", type = { 1996 CodeType.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 1997 @Description(shortDefinition = "visual-group | logical-group | sentence-group", formalDefinition = "Defines the grouping behavior for the action and its children.") 1998 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-grouping-behavior") 1999 protected Enumeration<ActionGroupingBehavior> groupingBehavior; 2000 2001 /** 2002 * Defines the selection behavior for the action and its children. 2003 */ 2004 @Child(name = "selectionBehavior", type = { 2005 CodeType.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 2006 @Description(shortDefinition = "any | all | all-or-none | exactly-one | at-most-one | one-or-more", formalDefinition = "Defines the selection behavior for the action and its children.") 2007 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-selection-behavior") 2008 protected Enumeration<ActionSelectionBehavior> selectionBehavior; 2009 2010 /** 2011 * Defines expectations around whether an action is required. 2012 */ 2013 @Child(name = "requiredBehavior", type = { 2014 CodeType.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 2015 @Description(shortDefinition = "must | could | must-unless-documented", formalDefinition = "Defines expectations around whether an action is required.") 2016 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-required-behavior") 2017 protected Enumeration<ActionRequiredBehavior> requiredBehavior; 2018 2019 /** 2020 * Defines whether the action should usually be preselected. 2021 */ 2022 @Child(name = "precheckBehavior", type = { 2023 CodeType.class }, order = 16, min = 0, max = 1, modifier = false, summary = false) 2024 @Description(shortDefinition = "yes | no", formalDefinition = "Defines whether the action should usually be preselected.") 2025 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-precheck-behavior") 2026 protected Enumeration<ActionPrecheckBehavior> precheckBehavior; 2027 2028 /** 2029 * Defines whether the action can be selected multiple times. 2030 */ 2031 @Child(name = "cardinalityBehavior", type = { 2032 CodeType.class }, order = 17, min = 0, max = 1, modifier = false, summary = false) 2033 @Description(shortDefinition = "single | multiple", formalDefinition = "Defines whether the action can be selected multiple times.") 2034 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-cardinality-behavior") 2035 protected Enumeration<ActionCardinalityBehavior> cardinalityBehavior; 2036 2037 /** 2038 * The resource that is the target of the action (e.g. CommunicationRequest). 2039 */ 2040 @Child(name = "resource", type = { 2041 Reference.class }, order = 18, min = 0, max = 1, modifier = false, summary = false) 2042 @Description(shortDefinition = "The target of the action", formalDefinition = "The resource that is the target of the action (e.g. CommunicationRequest).") 2043 protected Reference resource; 2044 2045 /** 2046 * The actual object that is the target of the reference (The resource that is 2047 * the target of the action (e.g. CommunicationRequest).) 2048 */ 2049 protected Resource resourceTarget; 2050 2051 /** 2052 * Sub actions. 2053 */ 2054 @Child(name = "action", type = { 2055 RequestGroupActionComponent.class }, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2056 @Description(shortDefinition = "Sub action", formalDefinition = "Sub actions.") 2057 protected List<RequestGroupActionComponent> action; 2058 2059 private static final long serialVersionUID = 296752321L; 2060 2061 /** 2062 * Constructor 2063 */ 2064 public RequestGroupActionComponent() { 2065 super(); 2066 } 2067 2068 /** 2069 * @return {@link #prefix} (A user-visible prefix for the action.). This is the 2070 * underlying object with id, value and extensions. The accessor 2071 * "getPrefix" gives direct access to the value 2072 */ 2073 public StringType getPrefixElement() { 2074 if (this.prefix == null) 2075 if (Configuration.errorOnAutoCreate()) 2076 throw new Error("Attempt to auto-create RequestGroupActionComponent.prefix"); 2077 else if (Configuration.doAutoCreate()) 2078 this.prefix = new StringType(); // bb 2079 return this.prefix; 2080 } 2081 2082 public boolean hasPrefixElement() { 2083 return this.prefix != null && !this.prefix.isEmpty(); 2084 } 2085 2086 public boolean hasPrefix() { 2087 return this.prefix != null && !this.prefix.isEmpty(); 2088 } 2089 2090 /** 2091 * @param value {@link #prefix} (A user-visible prefix for the action.). This is 2092 * the underlying object with id, value and extensions. The 2093 * accessor "getPrefix" gives direct access to the value 2094 */ 2095 public RequestGroupActionComponent setPrefixElement(StringType value) { 2096 this.prefix = value; 2097 return this; 2098 } 2099 2100 /** 2101 * @return A user-visible prefix for the action. 2102 */ 2103 public String getPrefix() { 2104 return this.prefix == null ? null : this.prefix.getValue(); 2105 } 2106 2107 /** 2108 * @param value A user-visible prefix for the action. 2109 */ 2110 public RequestGroupActionComponent setPrefix(String value) { 2111 if (Utilities.noString(value)) 2112 this.prefix = null; 2113 else { 2114 if (this.prefix == null) 2115 this.prefix = new StringType(); 2116 this.prefix.setValue(value); 2117 } 2118 return this; 2119 } 2120 2121 /** 2122 * @return {@link #title} (The title of the action displayed to a user.). This 2123 * is the underlying object with id, value and extensions. The accessor 2124 * "getTitle" gives direct access to the value 2125 */ 2126 public StringType getTitleElement() { 2127 if (this.title == null) 2128 if (Configuration.errorOnAutoCreate()) 2129 throw new Error("Attempt to auto-create RequestGroupActionComponent.title"); 2130 else if (Configuration.doAutoCreate()) 2131 this.title = new StringType(); // bb 2132 return this.title; 2133 } 2134 2135 public boolean hasTitleElement() { 2136 return this.title != null && !this.title.isEmpty(); 2137 } 2138 2139 public boolean hasTitle() { 2140 return this.title != null && !this.title.isEmpty(); 2141 } 2142 2143 /** 2144 * @param value {@link #title} (The title of the action displayed to a user.). 2145 * This is the underlying object with id, value and extensions. The 2146 * accessor "getTitle" gives direct access to the value 2147 */ 2148 public RequestGroupActionComponent setTitleElement(StringType value) { 2149 this.title = value; 2150 return this; 2151 } 2152 2153 /** 2154 * @return The title of the action displayed to a user. 2155 */ 2156 public String getTitle() { 2157 return this.title == null ? null : this.title.getValue(); 2158 } 2159 2160 /** 2161 * @param value The title of the action displayed to a user. 2162 */ 2163 public RequestGroupActionComponent setTitle(String value) { 2164 if (Utilities.noString(value)) 2165 this.title = null; 2166 else { 2167 if (this.title == null) 2168 this.title = new StringType(); 2169 this.title.setValue(value); 2170 } 2171 return this; 2172 } 2173 2174 /** 2175 * @return {@link #description} (A short description of the action used to 2176 * provide a summary to display to the user.). This is the underlying 2177 * object with id, value and extensions. The accessor "getDescription" 2178 * gives direct access to the value 2179 */ 2180 public StringType getDescriptionElement() { 2181 if (this.description == null) 2182 if (Configuration.errorOnAutoCreate()) 2183 throw new Error("Attempt to auto-create RequestGroupActionComponent.description"); 2184 else if (Configuration.doAutoCreate()) 2185 this.description = new StringType(); // bb 2186 return this.description; 2187 } 2188 2189 public boolean hasDescriptionElement() { 2190 return this.description != null && !this.description.isEmpty(); 2191 } 2192 2193 public boolean hasDescription() { 2194 return this.description != null && !this.description.isEmpty(); 2195 } 2196 2197 /** 2198 * @param value {@link #description} (A short description of the action used to 2199 * provide a summary to display to the user.). This is the 2200 * underlying object with id, value and extensions. The accessor 2201 * "getDescription" gives direct access to the value 2202 */ 2203 public RequestGroupActionComponent setDescriptionElement(StringType value) { 2204 this.description = value; 2205 return this; 2206 } 2207 2208 /** 2209 * @return A short description of the action used to provide a summary to 2210 * display to the user. 2211 */ 2212 public String getDescription() { 2213 return this.description == null ? null : this.description.getValue(); 2214 } 2215 2216 /** 2217 * @param value A short description of the action used to provide a summary to 2218 * display to the user. 2219 */ 2220 public RequestGroupActionComponent setDescription(String value) { 2221 if (Utilities.noString(value)) 2222 this.description = null; 2223 else { 2224 if (this.description == null) 2225 this.description = new StringType(); 2226 this.description.setValue(value); 2227 } 2228 return this; 2229 } 2230 2231 /** 2232 * @return {@link #textEquivalent} (A text equivalent of the action to be 2233 * performed. This provides a human-interpretable description of the 2234 * action when the definition is consumed by a system that might not be 2235 * capable of interpreting it dynamically.). This is the underlying 2236 * object with id, value and extensions. The accessor 2237 * "getTextEquivalent" gives direct access to the value 2238 */ 2239 public StringType getTextEquivalentElement() { 2240 if (this.textEquivalent == null) 2241 if (Configuration.errorOnAutoCreate()) 2242 throw new Error("Attempt to auto-create RequestGroupActionComponent.textEquivalent"); 2243 else if (Configuration.doAutoCreate()) 2244 this.textEquivalent = new StringType(); // bb 2245 return this.textEquivalent; 2246 } 2247 2248 public boolean hasTextEquivalentElement() { 2249 return this.textEquivalent != null && !this.textEquivalent.isEmpty(); 2250 } 2251 2252 public boolean hasTextEquivalent() { 2253 return this.textEquivalent != null && !this.textEquivalent.isEmpty(); 2254 } 2255 2256 /** 2257 * @param value {@link #textEquivalent} (A text equivalent of the action to be 2258 * performed. This provides a human-interpretable description of 2259 * the action when the definition is consumed by a system that 2260 * might not be capable of interpreting it dynamically.). This is 2261 * the underlying object with id, value and extensions. The 2262 * accessor "getTextEquivalent" gives direct access to the value 2263 */ 2264 public RequestGroupActionComponent setTextEquivalentElement(StringType value) { 2265 this.textEquivalent = value; 2266 return this; 2267 } 2268 2269 /** 2270 * @return A text equivalent of the action to be performed. This provides a 2271 * human-interpretable description of the action when the definition is 2272 * consumed by a system that might not be capable of interpreting it 2273 * dynamically. 2274 */ 2275 public String getTextEquivalent() { 2276 return this.textEquivalent == null ? null : this.textEquivalent.getValue(); 2277 } 2278 2279 /** 2280 * @param value A text equivalent of the action to be performed. This provides a 2281 * human-interpretable description of the action when the 2282 * definition is consumed by a system that might not be capable of 2283 * interpreting it dynamically. 2284 */ 2285 public RequestGroupActionComponent setTextEquivalent(String value) { 2286 if (Utilities.noString(value)) 2287 this.textEquivalent = null; 2288 else { 2289 if (this.textEquivalent == null) 2290 this.textEquivalent = new StringType(); 2291 this.textEquivalent.setValue(value); 2292 } 2293 return this; 2294 } 2295 2296 /** 2297 * @return {@link #priority} (Indicates how quickly the action should be 2298 * addressed with respect to other actions.). This is the underlying 2299 * object with id, value and extensions. The accessor "getPriority" 2300 * gives direct access to the value 2301 */ 2302 public Enumeration<RequestPriority> getPriorityElement() { 2303 if (this.priority == null) 2304 if (Configuration.errorOnAutoCreate()) 2305 throw new Error("Attempt to auto-create RequestGroupActionComponent.priority"); 2306 else if (Configuration.doAutoCreate()) 2307 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 2308 return this.priority; 2309 } 2310 2311 public boolean hasPriorityElement() { 2312 return this.priority != null && !this.priority.isEmpty(); 2313 } 2314 2315 public boolean hasPriority() { 2316 return this.priority != null && !this.priority.isEmpty(); 2317 } 2318 2319 /** 2320 * @param value {@link #priority} (Indicates how quickly the action should be 2321 * addressed with respect to other actions.). This is the 2322 * underlying object with id, value and extensions. The accessor 2323 * "getPriority" gives direct access to the value 2324 */ 2325 public RequestGroupActionComponent setPriorityElement(Enumeration<RequestPriority> value) { 2326 this.priority = value; 2327 return this; 2328 } 2329 2330 /** 2331 * @return Indicates how quickly the action should be addressed with respect to 2332 * other actions. 2333 */ 2334 public RequestPriority getPriority() { 2335 return this.priority == null ? null : this.priority.getValue(); 2336 } 2337 2338 /** 2339 * @param value Indicates how quickly the action should be addressed with 2340 * respect to other actions. 2341 */ 2342 public RequestGroupActionComponent setPriority(RequestPriority value) { 2343 if (value == null) 2344 this.priority = null; 2345 else { 2346 if (this.priority == null) 2347 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 2348 this.priority.setValue(value); 2349 } 2350 return this; 2351 } 2352 2353 /** 2354 * @return {@link #code} (A code that provides meaning for the action or action 2355 * group. For example, a section may have a LOINC code for a section of 2356 * a documentation template.) 2357 */ 2358 public List<CodeableConcept> getCode() { 2359 if (this.code == null) 2360 this.code = new ArrayList<CodeableConcept>(); 2361 return this.code; 2362 } 2363 2364 /** 2365 * @return Returns a reference to <code>this</code> for easy method chaining 2366 */ 2367 public RequestGroupActionComponent setCode(List<CodeableConcept> theCode) { 2368 this.code = theCode; 2369 return this; 2370 } 2371 2372 public boolean hasCode() { 2373 if (this.code == null) 2374 return false; 2375 for (CodeableConcept item : this.code) 2376 if (!item.isEmpty()) 2377 return true; 2378 return false; 2379 } 2380 2381 public CodeableConcept addCode() { // 3 2382 CodeableConcept t = new CodeableConcept(); 2383 if (this.code == null) 2384 this.code = new ArrayList<CodeableConcept>(); 2385 this.code.add(t); 2386 return t; 2387 } 2388 2389 public RequestGroupActionComponent addCode(CodeableConcept t) { // 3 2390 if (t == null) 2391 return this; 2392 if (this.code == null) 2393 this.code = new ArrayList<CodeableConcept>(); 2394 this.code.add(t); 2395 return this; 2396 } 2397 2398 /** 2399 * @return The first repetition of repeating field {@link #code}, creating it if 2400 * it does not already exist 2401 */ 2402 public CodeableConcept getCodeFirstRep() { 2403 if (getCode().isEmpty()) { 2404 addCode(); 2405 } 2406 return getCode().get(0); 2407 } 2408 2409 /** 2410 * @return {@link #documentation} (Didactic or other informational resources 2411 * associated with the action that can be provided to the CDS recipient. 2412 * Information resources can include inline text commentary and links to 2413 * web resources.) 2414 */ 2415 public List<RelatedArtifact> getDocumentation() { 2416 if (this.documentation == null) 2417 this.documentation = new ArrayList<RelatedArtifact>(); 2418 return this.documentation; 2419 } 2420 2421 /** 2422 * @return Returns a reference to <code>this</code> for easy method chaining 2423 */ 2424 public RequestGroupActionComponent setDocumentation(List<RelatedArtifact> theDocumentation) { 2425 this.documentation = theDocumentation; 2426 return this; 2427 } 2428 2429 public boolean hasDocumentation() { 2430 if (this.documentation == null) 2431 return false; 2432 for (RelatedArtifact item : this.documentation) 2433 if (!item.isEmpty()) 2434 return true; 2435 return false; 2436 } 2437 2438 public RelatedArtifact addDocumentation() { // 3 2439 RelatedArtifact t = new RelatedArtifact(); 2440 if (this.documentation == null) 2441 this.documentation = new ArrayList<RelatedArtifact>(); 2442 this.documentation.add(t); 2443 return t; 2444 } 2445 2446 public RequestGroupActionComponent addDocumentation(RelatedArtifact t) { // 3 2447 if (t == null) 2448 return this; 2449 if (this.documentation == null) 2450 this.documentation = new ArrayList<RelatedArtifact>(); 2451 this.documentation.add(t); 2452 return this; 2453 } 2454 2455 /** 2456 * @return The first repetition of repeating field {@link #documentation}, 2457 * creating it if it does not already exist 2458 */ 2459 public RelatedArtifact getDocumentationFirstRep() { 2460 if (getDocumentation().isEmpty()) { 2461 addDocumentation(); 2462 } 2463 return getDocumentation().get(0); 2464 } 2465 2466 /** 2467 * @return {@link #condition} (An expression that describes applicability 2468 * criteria, or start/stop conditions for the action.) 2469 */ 2470 public List<RequestGroupActionConditionComponent> getCondition() { 2471 if (this.condition == null) 2472 this.condition = new ArrayList<RequestGroupActionConditionComponent>(); 2473 return this.condition; 2474 } 2475 2476 /** 2477 * @return Returns a reference to <code>this</code> for easy method chaining 2478 */ 2479 public RequestGroupActionComponent setCondition(List<RequestGroupActionConditionComponent> theCondition) { 2480 this.condition = theCondition; 2481 return this; 2482 } 2483 2484 public boolean hasCondition() { 2485 if (this.condition == null) 2486 return false; 2487 for (RequestGroupActionConditionComponent item : this.condition) 2488 if (!item.isEmpty()) 2489 return true; 2490 return false; 2491 } 2492 2493 public RequestGroupActionConditionComponent addCondition() { // 3 2494 RequestGroupActionConditionComponent t = new RequestGroupActionConditionComponent(); 2495 if (this.condition == null) 2496 this.condition = new ArrayList<RequestGroupActionConditionComponent>(); 2497 this.condition.add(t); 2498 return t; 2499 } 2500 2501 public RequestGroupActionComponent addCondition(RequestGroupActionConditionComponent t) { // 3 2502 if (t == null) 2503 return this; 2504 if (this.condition == null) 2505 this.condition = new ArrayList<RequestGroupActionConditionComponent>(); 2506 this.condition.add(t); 2507 return this; 2508 } 2509 2510 /** 2511 * @return The first repetition of repeating field {@link #condition}, creating 2512 * it if it does not already exist 2513 */ 2514 public RequestGroupActionConditionComponent getConditionFirstRep() { 2515 if (getCondition().isEmpty()) { 2516 addCondition(); 2517 } 2518 return getCondition().get(0); 2519 } 2520 2521 /** 2522 * @return {@link #relatedAction} (A relationship to another action such as 2523 * "before" or "30-60 minutes after start of".) 2524 */ 2525 public List<RequestGroupActionRelatedActionComponent> getRelatedAction() { 2526 if (this.relatedAction == null) 2527 this.relatedAction = new ArrayList<RequestGroupActionRelatedActionComponent>(); 2528 return this.relatedAction; 2529 } 2530 2531 /** 2532 * @return Returns a reference to <code>this</code> for easy method chaining 2533 */ 2534 public RequestGroupActionComponent setRelatedAction( 2535 List<RequestGroupActionRelatedActionComponent> theRelatedAction) { 2536 this.relatedAction = theRelatedAction; 2537 return this; 2538 } 2539 2540 public boolean hasRelatedAction() { 2541 if (this.relatedAction == null) 2542 return false; 2543 for (RequestGroupActionRelatedActionComponent item : this.relatedAction) 2544 if (!item.isEmpty()) 2545 return true; 2546 return false; 2547 } 2548 2549 public RequestGroupActionRelatedActionComponent addRelatedAction() { // 3 2550 RequestGroupActionRelatedActionComponent t = new RequestGroupActionRelatedActionComponent(); 2551 if (this.relatedAction == null) 2552 this.relatedAction = new ArrayList<RequestGroupActionRelatedActionComponent>(); 2553 this.relatedAction.add(t); 2554 return t; 2555 } 2556 2557 public RequestGroupActionComponent addRelatedAction(RequestGroupActionRelatedActionComponent t) { // 3 2558 if (t == null) 2559 return this; 2560 if (this.relatedAction == null) 2561 this.relatedAction = new ArrayList<RequestGroupActionRelatedActionComponent>(); 2562 this.relatedAction.add(t); 2563 return this; 2564 } 2565 2566 /** 2567 * @return The first repetition of repeating field {@link #relatedAction}, 2568 * creating it if it does not already exist 2569 */ 2570 public RequestGroupActionRelatedActionComponent getRelatedActionFirstRep() { 2571 if (getRelatedAction().isEmpty()) { 2572 addRelatedAction(); 2573 } 2574 return getRelatedAction().get(0); 2575 } 2576 2577 /** 2578 * @return {@link #timing} (An optional value describing when the action should 2579 * be performed.) 2580 */ 2581 public Type getTiming() { 2582 return this.timing; 2583 } 2584 2585 /** 2586 * @return {@link #timing} (An optional value describing when the action should 2587 * be performed.) 2588 */ 2589 public DateTimeType getTimingDateTimeType() throws FHIRException { 2590 if (this.timing == null) 2591 this.timing = new DateTimeType(); 2592 if (!(this.timing instanceof DateTimeType)) 2593 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 2594 + this.timing.getClass().getName() + " was encountered"); 2595 return (DateTimeType) this.timing; 2596 } 2597 2598 public boolean hasTimingDateTimeType() { 2599 return this != null && this.timing instanceof DateTimeType; 2600 } 2601 2602 /** 2603 * @return {@link #timing} (An optional value describing when the action should 2604 * be performed.) 2605 */ 2606 public Age getTimingAge() throws FHIRException { 2607 if (this.timing == null) 2608 this.timing = new Age(); 2609 if (!(this.timing instanceof Age)) 2610 throw new FHIRException( 2611 "Type mismatch: the type Age was expected, but " + this.timing.getClass().getName() + " was encountered"); 2612 return (Age) this.timing; 2613 } 2614 2615 public boolean hasTimingAge() { 2616 return this != null && this.timing instanceof Age; 2617 } 2618 2619 /** 2620 * @return {@link #timing} (An optional value describing when the action should 2621 * be performed.) 2622 */ 2623 public Period getTimingPeriod() throws FHIRException { 2624 if (this.timing == null) 2625 this.timing = new Period(); 2626 if (!(this.timing instanceof Period)) 2627 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.timing.getClass().getName() 2628 + " was encountered"); 2629 return (Period) this.timing; 2630 } 2631 2632 public boolean hasTimingPeriod() { 2633 return this != null && this.timing instanceof Period; 2634 } 2635 2636 /** 2637 * @return {@link #timing} (An optional value describing when the action should 2638 * be performed.) 2639 */ 2640 public Duration getTimingDuration() throws FHIRException { 2641 if (this.timing == null) 2642 this.timing = new Duration(); 2643 if (!(this.timing instanceof Duration)) 2644 throw new FHIRException("Type mismatch: the type Duration was expected, but " + this.timing.getClass().getName() 2645 + " was encountered"); 2646 return (Duration) this.timing; 2647 } 2648 2649 public boolean hasTimingDuration() { 2650 return this != null && this.timing instanceof Duration; 2651 } 2652 2653 /** 2654 * @return {@link #timing} (An optional value describing when the action should 2655 * be performed.) 2656 */ 2657 public Range getTimingRange() throws FHIRException { 2658 if (this.timing == null) 2659 this.timing = new Range(); 2660 if (!(this.timing instanceof Range)) 2661 throw new FHIRException( 2662 "Type mismatch: the type Range was expected, but " + this.timing.getClass().getName() + " was encountered"); 2663 return (Range) this.timing; 2664 } 2665 2666 public boolean hasTimingRange() { 2667 return this != null && this.timing instanceof Range; 2668 } 2669 2670 /** 2671 * @return {@link #timing} (An optional value describing when the action should 2672 * be performed.) 2673 */ 2674 public Timing getTimingTiming() throws FHIRException { 2675 if (this.timing == null) 2676 this.timing = new Timing(); 2677 if (!(this.timing instanceof Timing)) 2678 throw new FHIRException("Type mismatch: the type Timing was expected, but " + this.timing.getClass().getName() 2679 + " was encountered"); 2680 return (Timing) this.timing; 2681 } 2682 2683 public boolean hasTimingTiming() { 2684 return this != null && this.timing instanceof Timing; 2685 } 2686 2687 public boolean hasTiming() { 2688 return this.timing != null && !this.timing.isEmpty(); 2689 } 2690 2691 /** 2692 * @param value {@link #timing} (An optional value describing when the action 2693 * should be performed.) 2694 */ 2695 public RequestGroupActionComponent setTiming(Type value) { 2696 if (value != null && !(value instanceof DateTimeType || value instanceof Age || value instanceof Period 2697 || value instanceof Duration || value instanceof Range || value instanceof Timing)) 2698 throw new Error("Not the right type for RequestGroup.action.timing[x]: " + value.fhirType()); 2699 this.timing = value; 2700 return this; 2701 } 2702 2703 /** 2704 * @return {@link #participant} (The participant that should perform or be 2705 * responsible for this action.) 2706 */ 2707 public List<Reference> getParticipant() { 2708 if (this.participant == null) 2709 this.participant = new ArrayList<Reference>(); 2710 return this.participant; 2711 } 2712 2713 /** 2714 * @return Returns a reference to <code>this</code> for easy method chaining 2715 */ 2716 public RequestGroupActionComponent setParticipant(List<Reference> theParticipant) { 2717 this.participant = theParticipant; 2718 return this; 2719 } 2720 2721 public boolean hasParticipant() { 2722 if (this.participant == null) 2723 return false; 2724 for (Reference item : this.participant) 2725 if (!item.isEmpty()) 2726 return true; 2727 return false; 2728 } 2729 2730 public Reference addParticipant() { // 3 2731 Reference t = new Reference(); 2732 if (this.participant == null) 2733 this.participant = new ArrayList<Reference>(); 2734 this.participant.add(t); 2735 return t; 2736 } 2737 2738 public RequestGroupActionComponent addParticipant(Reference t) { // 3 2739 if (t == null) 2740 return this; 2741 if (this.participant == null) 2742 this.participant = new ArrayList<Reference>(); 2743 this.participant.add(t); 2744 return this; 2745 } 2746 2747 /** 2748 * @return The first repetition of repeating field {@link #participant}, 2749 * creating it if it does not already exist 2750 */ 2751 public Reference getParticipantFirstRep() { 2752 if (getParticipant().isEmpty()) { 2753 addParticipant(); 2754 } 2755 return getParticipant().get(0); 2756 } 2757 2758 /** 2759 * @deprecated Use Reference#setResource(IBaseResource) instead 2760 */ 2761 @Deprecated 2762 public List<Resource> getParticipantTarget() { 2763 if (this.participantTarget == null) 2764 this.participantTarget = new ArrayList<Resource>(); 2765 return this.participantTarget; 2766 } 2767 2768 /** 2769 * @return {@link #type} (The type of action to perform (create, update, 2770 * remove).) 2771 */ 2772 public CodeableConcept getType() { 2773 if (this.type == null) 2774 if (Configuration.errorOnAutoCreate()) 2775 throw new Error("Attempt to auto-create RequestGroupActionComponent.type"); 2776 else if (Configuration.doAutoCreate()) 2777 this.type = new CodeableConcept(); // cc 2778 return this.type; 2779 } 2780 2781 public boolean hasType() { 2782 return this.type != null && !this.type.isEmpty(); 2783 } 2784 2785 /** 2786 * @param value {@link #type} (The type of action to perform (create, update, 2787 * remove).) 2788 */ 2789 public RequestGroupActionComponent setType(CodeableConcept value) { 2790 this.type = value; 2791 return this; 2792 } 2793 2794 /** 2795 * @return {@link #groupingBehavior} (Defines the grouping behavior for the 2796 * action and its children.). This is the underlying object with id, 2797 * value and extensions. The accessor "getGroupingBehavior" gives direct 2798 * access to the value 2799 */ 2800 public Enumeration<ActionGroupingBehavior> getGroupingBehaviorElement() { 2801 if (this.groupingBehavior == null) 2802 if (Configuration.errorOnAutoCreate()) 2803 throw new Error("Attempt to auto-create RequestGroupActionComponent.groupingBehavior"); 2804 else if (Configuration.doAutoCreate()) 2805 this.groupingBehavior = new Enumeration<ActionGroupingBehavior>(new ActionGroupingBehaviorEnumFactory()); // bb 2806 return this.groupingBehavior; 2807 } 2808 2809 public boolean hasGroupingBehaviorElement() { 2810 return this.groupingBehavior != null && !this.groupingBehavior.isEmpty(); 2811 } 2812 2813 public boolean hasGroupingBehavior() { 2814 return this.groupingBehavior != null && !this.groupingBehavior.isEmpty(); 2815 } 2816 2817 /** 2818 * @param value {@link #groupingBehavior} (Defines the grouping behavior for the 2819 * action and its children.). This is the underlying object with 2820 * id, value and extensions. The accessor "getGroupingBehavior" 2821 * gives direct access to the value 2822 */ 2823 public RequestGroupActionComponent setGroupingBehaviorElement(Enumeration<ActionGroupingBehavior> value) { 2824 this.groupingBehavior = value; 2825 return this; 2826 } 2827 2828 /** 2829 * @return Defines the grouping behavior for the action and its children. 2830 */ 2831 public ActionGroupingBehavior getGroupingBehavior() { 2832 return this.groupingBehavior == null ? null : this.groupingBehavior.getValue(); 2833 } 2834 2835 /** 2836 * @param value Defines the grouping behavior for the action and its children. 2837 */ 2838 public RequestGroupActionComponent setGroupingBehavior(ActionGroupingBehavior value) { 2839 if (value == null) 2840 this.groupingBehavior = null; 2841 else { 2842 if (this.groupingBehavior == null) 2843 this.groupingBehavior = new Enumeration<ActionGroupingBehavior>(new ActionGroupingBehaviorEnumFactory()); 2844 this.groupingBehavior.setValue(value); 2845 } 2846 return this; 2847 } 2848 2849 /** 2850 * @return {@link #selectionBehavior} (Defines the selection behavior for the 2851 * action and its children.). This is the underlying object with id, 2852 * value and extensions. The accessor "getSelectionBehavior" gives 2853 * direct access to the value 2854 */ 2855 public Enumeration<ActionSelectionBehavior> getSelectionBehaviorElement() { 2856 if (this.selectionBehavior == null) 2857 if (Configuration.errorOnAutoCreate()) 2858 throw new Error("Attempt to auto-create RequestGroupActionComponent.selectionBehavior"); 2859 else if (Configuration.doAutoCreate()) 2860 this.selectionBehavior = new Enumeration<ActionSelectionBehavior>(new ActionSelectionBehaviorEnumFactory()); // bb 2861 return this.selectionBehavior; 2862 } 2863 2864 public boolean hasSelectionBehaviorElement() { 2865 return this.selectionBehavior != null && !this.selectionBehavior.isEmpty(); 2866 } 2867 2868 public boolean hasSelectionBehavior() { 2869 return this.selectionBehavior != null && !this.selectionBehavior.isEmpty(); 2870 } 2871 2872 /** 2873 * @param value {@link #selectionBehavior} (Defines the selection behavior for 2874 * the action and its children.). This is the underlying object 2875 * with id, value and extensions. The accessor 2876 * "getSelectionBehavior" gives direct access to the value 2877 */ 2878 public RequestGroupActionComponent setSelectionBehaviorElement(Enumeration<ActionSelectionBehavior> value) { 2879 this.selectionBehavior = value; 2880 return this; 2881 } 2882 2883 /** 2884 * @return Defines the selection behavior for the action and its children. 2885 */ 2886 public ActionSelectionBehavior getSelectionBehavior() { 2887 return this.selectionBehavior == null ? null : this.selectionBehavior.getValue(); 2888 } 2889 2890 /** 2891 * @param value Defines the selection behavior for the action and its children. 2892 */ 2893 public RequestGroupActionComponent setSelectionBehavior(ActionSelectionBehavior value) { 2894 if (value == null) 2895 this.selectionBehavior = null; 2896 else { 2897 if (this.selectionBehavior == null) 2898 this.selectionBehavior = new Enumeration<ActionSelectionBehavior>(new ActionSelectionBehaviorEnumFactory()); 2899 this.selectionBehavior.setValue(value); 2900 } 2901 return this; 2902 } 2903 2904 /** 2905 * @return {@link #requiredBehavior} (Defines expectations around whether an 2906 * action is required.). This is the underlying object with id, value 2907 * and extensions. The accessor "getRequiredBehavior" gives direct 2908 * access to the value 2909 */ 2910 public Enumeration<ActionRequiredBehavior> getRequiredBehaviorElement() { 2911 if (this.requiredBehavior == null) 2912 if (Configuration.errorOnAutoCreate()) 2913 throw new Error("Attempt to auto-create RequestGroupActionComponent.requiredBehavior"); 2914 else if (Configuration.doAutoCreate()) 2915 this.requiredBehavior = new Enumeration<ActionRequiredBehavior>(new ActionRequiredBehaviorEnumFactory()); // bb 2916 return this.requiredBehavior; 2917 } 2918 2919 public boolean hasRequiredBehaviorElement() { 2920 return this.requiredBehavior != null && !this.requiredBehavior.isEmpty(); 2921 } 2922 2923 public boolean hasRequiredBehavior() { 2924 return this.requiredBehavior != null && !this.requiredBehavior.isEmpty(); 2925 } 2926 2927 /** 2928 * @param value {@link #requiredBehavior} (Defines expectations around whether 2929 * an action is required.). This is the underlying object with id, 2930 * value and extensions. The accessor "getRequiredBehavior" gives 2931 * direct access to the value 2932 */ 2933 public RequestGroupActionComponent setRequiredBehaviorElement(Enumeration<ActionRequiredBehavior> value) { 2934 this.requiredBehavior = value; 2935 return this; 2936 } 2937 2938 /** 2939 * @return Defines expectations around whether an action is required. 2940 */ 2941 public ActionRequiredBehavior getRequiredBehavior() { 2942 return this.requiredBehavior == null ? null : this.requiredBehavior.getValue(); 2943 } 2944 2945 /** 2946 * @param value Defines expectations around whether an action is required. 2947 */ 2948 public RequestGroupActionComponent setRequiredBehavior(ActionRequiredBehavior value) { 2949 if (value == null) 2950 this.requiredBehavior = null; 2951 else { 2952 if (this.requiredBehavior == null) 2953 this.requiredBehavior = new Enumeration<ActionRequiredBehavior>(new ActionRequiredBehaviorEnumFactory()); 2954 this.requiredBehavior.setValue(value); 2955 } 2956 return this; 2957 } 2958 2959 /** 2960 * @return {@link #precheckBehavior} (Defines whether the action should usually 2961 * be preselected.). This is the underlying object with id, value and 2962 * extensions. The accessor "getPrecheckBehavior" gives direct access to 2963 * the value 2964 */ 2965 public Enumeration<ActionPrecheckBehavior> getPrecheckBehaviorElement() { 2966 if (this.precheckBehavior == null) 2967 if (Configuration.errorOnAutoCreate()) 2968 throw new Error("Attempt to auto-create RequestGroupActionComponent.precheckBehavior"); 2969 else if (Configuration.doAutoCreate()) 2970 this.precheckBehavior = new Enumeration<ActionPrecheckBehavior>(new ActionPrecheckBehaviorEnumFactory()); // bb 2971 return this.precheckBehavior; 2972 } 2973 2974 public boolean hasPrecheckBehaviorElement() { 2975 return this.precheckBehavior != null && !this.precheckBehavior.isEmpty(); 2976 } 2977 2978 public boolean hasPrecheckBehavior() { 2979 return this.precheckBehavior != null && !this.precheckBehavior.isEmpty(); 2980 } 2981 2982 /** 2983 * @param value {@link #precheckBehavior} (Defines whether the action should 2984 * usually be preselected.). This is the underlying object with id, 2985 * value and extensions. The accessor "getPrecheckBehavior" gives 2986 * direct access to the value 2987 */ 2988 public RequestGroupActionComponent setPrecheckBehaviorElement(Enumeration<ActionPrecheckBehavior> value) { 2989 this.precheckBehavior = value; 2990 return this; 2991 } 2992 2993 /** 2994 * @return Defines whether the action should usually be preselected. 2995 */ 2996 public ActionPrecheckBehavior getPrecheckBehavior() { 2997 return this.precheckBehavior == null ? null : this.precheckBehavior.getValue(); 2998 } 2999 3000 /** 3001 * @param value Defines whether the action should usually be preselected. 3002 */ 3003 public RequestGroupActionComponent setPrecheckBehavior(ActionPrecheckBehavior value) { 3004 if (value == null) 3005 this.precheckBehavior = null; 3006 else { 3007 if (this.precheckBehavior == null) 3008 this.precheckBehavior = new Enumeration<ActionPrecheckBehavior>(new ActionPrecheckBehaviorEnumFactory()); 3009 this.precheckBehavior.setValue(value); 3010 } 3011 return this; 3012 } 3013 3014 /** 3015 * @return {@link #cardinalityBehavior} (Defines whether the action can be 3016 * selected multiple times.). This is the underlying object with id, 3017 * value and extensions. The accessor "getCardinalityBehavior" gives 3018 * direct access to the value 3019 */ 3020 public Enumeration<ActionCardinalityBehavior> getCardinalityBehaviorElement() { 3021 if (this.cardinalityBehavior == null) 3022 if (Configuration.errorOnAutoCreate()) 3023 throw new Error("Attempt to auto-create RequestGroupActionComponent.cardinalityBehavior"); 3024 else if (Configuration.doAutoCreate()) 3025 this.cardinalityBehavior = new Enumeration<ActionCardinalityBehavior>( 3026 new ActionCardinalityBehaviorEnumFactory()); // bb 3027 return this.cardinalityBehavior; 3028 } 3029 3030 public boolean hasCardinalityBehaviorElement() { 3031 return this.cardinalityBehavior != null && !this.cardinalityBehavior.isEmpty(); 3032 } 3033 3034 public boolean hasCardinalityBehavior() { 3035 return this.cardinalityBehavior != null && !this.cardinalityBehavior.isEmpty(); 3036 } 3037 3038 /** 3039 * @param value {@link #cardinalityBehavior} (Defines whether the action can be 3040 * selected multiple times.). This is the underlying object with 3041 * id, value and extensions. The accessor "getCardinalityBehavior" 3042 * gives direct access to the value 3043 */ 3044 public RequestGroupActionComponent setCardinalityBehaviorElement(Enumeration<ActionCardinalityBehavior> value) { 3045 this.cardinalityBehavior = value; 3046 return this; 3047 } 3048 3049 /** 3050 * @return Defines whether the action can be selected multiple times. 3051 */ 3052 public ActionCardinalityBehavior getCardinalityBehavior() { 3053 return this.cardinalityBehavior == null ? null : this.cardinalityBehavior.getValue(); 3054 } 3055 3056 /** 3057 * @param value Defines whether the action can be selected multiple times. 3058 */ 3059 public RequestGroupActionComponent setCardinalityBehavior(ActionCardinalityBehavior value) { 3060 if (value == null) 3061 this.cardinalityBehavior = null; 3062 else { 3063 if (this.cardinalityBehavior == null) 3064 this.cardinalityBehavior = new Enumeration<ActionCardinalityBehavior>( 3065 new ActionCardinalityBehaviorEnumFactory()); 3066 this.cardinalityBehavior.setValue(value); 3067 } 3068 return this; 3069 } 3070 3071 /** 3072 * @return {@link #resource} (The resource that is the target of the action 3073 * (e.g. CommunicationRequest).) 3074 */ 3075 public Reference getResource() { 3076 if (this.resource == null) 3077 if (Configuration.errorOnAutoCreate()) 3078 throw new Error("Attempt to auto-create RequestGroupActionComponent.resource"); 3079 else if (Configuration.doAutoCreate()) 3080 this.resource = new Reference(); // cc 3081 return this.resource; 3082 } 3083 3084 public boolean hasResource() { 3085 return this.resource != null && !this.resource.isEmpty(); 3086 } 3087 3088 /** 3089 * @param value {@link #resource} (The resource that is the target of the action 3090 * (e.g. CommunicationRequest).) 3091 */ 3092 public RequestGroupActionComponent setResource(Reference value) { 3093 this.resource = value; 3094 return this; 3095 } 3096 3097 /** 3098 * @return {@link #resource} The actual object that is the target of the 3099 * reference. The reference library doesn't populate this, but you can 3100 * use it to hold the resource if you resolve it. (The resource that is 3101 * the target of the action (e.g. CommunicationRequest).) 3102 */ 3103 public Resource getResourceTarget() { 3104 return this.resourceTarget; 3105 } 3106 3107 /** 3108 * @param value {@link #resource} The actual object that is the target of the 3109 * reference. The reference library doesn't use these, but you can 3110 * use it to hold the resource if you resolve it. (The resource 3111 * that is the target of the action (e.g. CommunicationRequest).) 3112 */ 3113 public RequestGroupActionComponent setResourceTarget(Resource value) { 3114 this.resourceTarget = value; 3115 return this; 3116 } 3117 3118 /** 3119 * @return {@link #action} (Sub actions.) 3120 */ 3121 public List<RequestGroupActionComponent> getAction() { 3122 if (this.action == null) 3123 this.action = new ArrayList<RequestGroupActionComponent>(); 3124 return this.action; 3125 } 3126 3127 /** 3128 * @return Returns a reference to <code>this</code> for easy method chaining 3129 */ 3130 public RequestGroupActionComponent setAction(List<RequestGroupActionComponent> theAction) { 3131 this.action = theAction; 3132 return this; 3133 } 3134 3135 public boolean hasAction() { 3136 if (this.action == null) 3137 return false; 3138 for (RequestGroupActionComponent item : this.action) 3139 if (!item.isEmpty()) 3140 return true; 3141 return false; 3142 } 3143 3144 public RequestGroupActionComponent addAction() { // 3 3145 RequestGroupActionComponent t = new RequestGroupActionComponent(); 3146 if (this.action == null) 3147 this.action = new ArrayList<RequestGroupActionComponent>(); 3148 this.action.add(t); 3149 return t; 3150 } 3151 3152 public RequestGroupActionComponent addAction(RequestGroupActionComponent t) { // 3 3153 if (t == null) 3154 return this; 3155 if (this.action == null) 3156 this.action = new ArrayList<RequestGroupActionComponent>(); 3157 this.action.add(t); 3158 return this; 3159 } 3160 3161 /** 3162 * @return The first repetition of repeating field {@link #action}, creating it 3163 * if it does not already exist 3164 */ 3165 public RequestGroupActionComponent getActionFirstRep() { 3166 if (getAction().isEmpty()) { 3167 addAction(); 3168 } 3169 return getAction().get(0); 3170 } 3171 3172 protected void listChildren(List<Property> children) { 3173 super.listChildren(children); 3174 children.add(new Property("prefix", "string", "A user-visible prefix for the action.", 0, 1, prefix)); 3175 children.add(new Property("title", "string", "The title of the action displayed to a user.", 0, 1, title)); 3176 children.add(new Property("description", "string", 3177 "A short description of the action used to provide a summary to display to the user.", 0, 1, description)); 3178 children.add(new Property("textEquivalent", "string", 3179 "A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that might not be capable of interpreting it dynamically.", 3180 0, 1, textEquivalent)); 3181 children.add(new Property("priority", "code", 3182 "Indicates how quickly the action should be addressed with respect to other actions.", 0, 1, priority)); 3183 children.add(new Property("code", "CodeableConcept", 3184 "A code that provides meaning for the action or action group. For example, a section may have a LOINC code for a section of a documentation template.", 3185 0, java.lang.Integer.MAX_VALUE, code)); 3186 children.add(new Property("documentation", "RelatedArtifact", 3187 "Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources.", 3188 0, java.lang.Integer.MAX_VALUE, documentation)); 3189 children.add(new Property("condition", "", 3190 "An expression that describes applicability criteria, or start/stop conditions for the action.", 0, 3191 java.lang.Integer.MAX_VALUE, condition)); 3192 children.add(new Property("relatedAction", "", 3193 "A relationship to another action such as \"before\" or \"30-60 minutes after start of\".", 0, 3194 java.lang.Integer.MAX_VALUE, relatedAction)); 3195 children.add(new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 3196 "An optional value describing when the action should be performed.", 0, 1, timing)); 3197 children.add(new Property("participant", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Device)", 3198 "The participant that should perform or be responsible for this action.", 0, java.lang.Integer.MAX_VALUE, 3199 participant)); 3200 children.add(new Property("type", "CodeableConcept", "The type of action to perform (create, update, remove).", 0, 3201 1, type)); 3202 children.add(new Property("groupingBehavior", "code", 3203 "Defines the grouping behavior for the action and its children.", 0, 1, groupingBehavior)); 3204 children.add(new Property("selectionBehavior", "code", 3205 "Defines the selection behavior for the action and its children.", 0, 1, selectionBehavior)); 3206 children.add(new Property("requiredBehavior", "code", 3207 "Defines expectations around whether an action is required.", 0, 1, requiredBehavior)); 3208 children.add(new Property("precheckBehavior", "code", "Defines whether the action should usually be preselected.", 3209 0, 1, precheckBehavior)); 3210 children.add(new Property("cardinalityBehavior", "code", 3211 "Defines whether the action can be selected multiple times.", 0, 1, cardinalityBehavior)); 3212 children.add(new Property("resource", "Reference(Any)", 3213 "The resource that is the target of the action (e.g. CommunicationRequest).", 0, 1, resource)); 3214 children 3215 .add(new Property("action", "@RequestGroup.action", "Sub actions.", 0, java.lang.Integer.MAX_VALUE, action)); 3216 } 3217 3218 @Override 3219 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3220 switch (_hash) { 3221 case -980110702: 3222 /* prefix */ return new Property("prefix", "string", "A user-visible prefix for the action.", 0, 1, prefix); 3223 case 110371416: 3224 /* title */ return new Property("title", "string", "The title of the action displayed to a user.", 0, 1, title); 3225 case -1724546052: 3226 /* description */ return new Property("description", "string", 3227 "A short description of the action used to provide a summary to display to the user.", 0, 1, description); 3228 case -900391049: 3229 /* textEquivalent */ return new Property("textEquivalent", "string", 3230 "A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that might not be capable of interpreting it dynamically.", 3231 0, 1, textEquivalent); 3232 case -1165461084: 3233 /* priority */ return new Property("priority", "code", 3234 "Indicates how quickly the action should be addressed with respect to other actions.", 0, 1, priority); 3235 case 3059181: 3236 /* code */ return new Property("code", "CodeableConcept", 3237 "A code that provides meaning for the action or action group. For example, a section may have a LOINC code for a section of a documentation template.", 3238 0, java.lang.Integer.MAX_VALUE, code); 3239 case 1587405498: 3240 /* documentation */ return new Property("documentation", "RelatedArtifact", 3241 "Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources.", 3242 0, java.lang.Integer.MAX_VALUE, documentation); 3243 case -861311717: 3244 /* condition */ return new Property("condition", "", 3245 "An expression that describes applicability criteria, or start/stop conditions for the action.", 0, 3246 java.lang.Integer.MAX_VALUE, condition); 3247 case -384107967: 3248 /* relatedAction */ return new Property("relatedAction", "", 3249 "A relationship to another action such as \"before\" or \"30-60 minutes after start of\".", 0, 3250 java.lang.Integer.MAX_VALUE, relatedAction); 3251 case 164632566: 3252 /* timing[x] */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 3253 "An optional value describing when the action should be performed.", 0, 1, timing); 3254 case -873664438: 3255 /* timing */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 3256 "An optional value describing when the action should be performed.", 0, 1, timing); 3257 case -1837458939: 3258 /* timingDateTime */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 3259 "An optional value describing when the action should be performed.", 0, 1, timing); 3260 case 164607061: 3261 /* timingAge */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 3262 "An optional value describing when the action should be performed.", 0, 1, timing); 3263 case -615615829: 3264 /* timingPeriod */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 3265 "An optional value describing when the action should be performed.", 0, 1, timing); 3266 case -1327253506: 3267 /* timingDuration */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 3268 "An optional value describing when the action should be performed.", 0, 1, timing); 3269 case -710871277: 3270 /* timingRange */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 3271 "An optional value describing when the action should be performed.", 0, 1, timing); 3272 case -497554124: 3273 /* timingTiming */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 3274 "An optional value describing when the action should be performed.", 0, 1, timing); 3275 case 767422259: 3276 /* participant */ return new Property("participant", 3277 "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Device)", 3278 "The participant that should perform or be responsible for this action.", 0, java.lang.Integer.MAX_VALUE, 3279 participant); 3280 case 3575610: 3281 /* type */ return new Property("type", "CodeableConcept", 3282 "The type of action to perform (create, update, remove).", 0, 1, type); 3283 case 586678389: 3284 /* groupingBehavior */ return new Property("groupingBehavior", "code", 3285 "Defines the grouping behavior for the action and its children.", 0, 1, groupingBehavior); 3286 case 168639486: 3287 /* selectionBehavior */ return new Property("selectionBehavior", "code", 3288 "Defines the selection behavior for the action and its children.", 0, 1, selectionBehavior); 3289 case -1163906287: 3290 /* requiredBehavior */ return new Property("requiredBehavior", "code", 3291 "Defines expectations around whether an action is required.", 0, 1, requiredBehavior); 3292 case -1174249033: 3293 /* precheckBehavior */ return new Property("precheckBehavior", "code", 3294 "Defines whether the action should usually be preselected.", 0, 1, precheckBehavior); 3295 case -922577408: 3296 /* cardinalityBehavior */ return new Property("cardinalityBehavior", "code", 3297 "Defines whether the action can be selected multiple times.", 0, 1, cardinalityBehavior); 3298 case -341064690: 3299 /* resource */ return new Property("resource", "Reference(Any)", 3300 "The resource that is the target of the action (e.g. CommunicationRequest).", 0, 1, resource); 3301 case -1422950858: 3302 /* action */ return new Property("action", "@RequestGroup.action", "Sub actions.", 0, 3303 java.lang.Integer.MAX_VALUE, action); 3304 default: 3305 return super.getNamedProperty(_hash, _name, _checkValid); 3306 } 3307 3308 } 3309 3310 @Override 3311 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3312 switch (hash) { 3313 case -980110702: 3314 /* prefix */ return this.prefix == null ? new Base[0] : new Base[] { this.prefix }; // StringType 3315 case 110371416: 3316 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 3317 case -1724546052: 3318 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 3319 case -900391049: 3320 /* textEquivalent */ return this.textEquivalent == null ? new Base[0] : new Base[] { this.textEquivalent }; // StringType 3321 case -1165461084: 3322 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // Enumeration<RequestPriority> 3323 case 3059181: 3324 /* code */ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 3325 case 1587405498: 3326 /* documentation */ return this.documentation == null ? new Base[0] 3327 : this.documentation.toArray(new Base[this.documentation.size()]); // RelatedArtifact 3328 case -861311717: 3329 /* condition */ return this.condition == null ? new Base[0] 3330 : this.condition.toArray(new Base[this.condition.size()]); // RequestGroupActionConditionComponent 3331 case -384107967: 3332 /* relatedAction */ return this.relatedAction == null ? new Base[0] 3333 : this.relatedAction.toArray(new Base[this.relatedAction.size()]); // RequestGroupActionRelatedActionComponent 3334 case -873664438: 3335 /* timing */ return this.timing == null ? new Base[0] : new Base[] { this.timing }; // Type 3336 case 767422259: 3337 /* participant */ return this.participant == null ? new Base[0] 3338 : this.participant.toArray(new Base[this.participant.size()]); // Reference 3339 case 3575610: 3340 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 3341 case 586678389: 3342 /* groupingBehavior */ return this.groupingBehavior == null ? new Base[0] 3343 : new Base[] { this.groupingBehavior }; // Enumeration<ActionGroupingBehavior> 3344 case 168639486: 3345 /* selectionBehavior */ return this.selectionBehavior == null ? new Base[0] 3346 : new Base[] { this.selectionBehavior }; // Enumeration<ActionSelectionBehavior> 3347 case -1163906287: 3348 /* requiredBehavior */ return this.requiredBehavior == null ? new Base[0] 3349 : new Base[] { this.requiredBehavior }; // Enumeration<ActionRequiredBehavior> 3350 case -1174249033: 3351 /* precheckBehavior */ return this.precheckBehavior == null ? new Base[0] 3352 : new Base[] { this.precheckBehavior }; // Enumeration<ActionPrecheckBehavior> 3353 case -922577408: 3354 /* cardinalityBehavior */ return this.cardinalityBehavior == null ? new Base[0] 3355 : new Base[] { this.cardinalityBehavior }; // Enumeration<ActionCardinalityBehavior> 3356 case -341064690: 3357 /* resource */ return this.resource == null ? new Base[0] : new Base[] { this.resource }; // Reference 3358 case -1422950858: 3359 /* action */ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // RequestGroupActionComponent 3360 default: 3361 return super.getProperty(hash, name, checkValid); 3362 } 3363 3364 } 3365 3366 @Override 3367 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3368 switch (hash) { 3369 case -980110702: // prefix 3370 this.prefix = castToString(value); // StringType 3371 return value; 3372 case 110371416: // title 3373 this.title = castToString(value); // StringType 3374 return value; 3375 case -1724546052: // description 3376 this.description = castToString(value); // StringType 3377 return value; 3378 case -900391049: // textEquivalent 3379 this.textEquivalent = castToString(value); // StringType 3380 return value; 3381 case -1165461084: // priority 3382 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 3383 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 3384 return value; 3385 case 3059181: // code 3386 this.getCode().add(castToCodeableConcept(value)); // CodeableConcept 3387 return value; 3388 case 1587405498: // documentation 3389 this.getDocumentation().add(castToRelatedArtifact(value)); // RelatedArtifact 3390 return value; 3391 case -861311717: // condition 3392 this.getCondition().add((RequestGroupActionConditionComponent) value); // RequestGroupActionConditionComponent 3393 return value; 3394 case -384107967: // relatedAction 3395 this.getRelatedAction().add((RequestGroupActionRelatedActionComponent) value); // RequestGroupActionRelatedActionComponent 3396 return value; 3397 case -873664438: // timing 3398 this.timing = castToType(value); // Type 3399 return value; 3400 case 767422259: // participant 3401 this.getParticipant().add(castToReference(value)); // Reference 3402 return value; 3403 case 3575610: // type 3404 this.type = castToCodeableConcept(value); // CodeableConcept 3405 return value; 3406 case 586678389: // groupingBehavior 3407 value = new ActionGroupingBehaviorEnumFactory().fromType(castToCode(value)); 3408 this.groupingBehavior = (Enumeration) value; // Enumeration<ActionGroupingBehavior> 3409 return value; 3410 case 168639486: // selectionBehavior 3411 value = new ActionSelectionBehaviorEnumFactory().fromType(castToCode(value)); 3412 this.selectionBehavior = (Enumeration) value; // Enumeration<ActionSelectionBehavior> 3413 return value; 3414 case -1163906287: // requiredBehavior 3415 value = new ActionRequiredBehaviorEnumFactory().fromType(castToCode(value)); 3416 this.requiredBehavior = (Enumeration) value; // Enumeration<ActionRequiredBehavior> 3417 return value; 3418 case -1174249033: // precheckBehavior 3419 value = new ActionPrecheckBehaviorEnumFactory().fromType(castToCode(value)); 3420 this.precheckBehavior = (Enumeration) value; // Enumeration<ActionPrecheckBehavior> 3421 return value; 3422 case -922577408: // cardinalityBehavior 3423 value = new ActionCardinalityBehaviorEnumFactory().fromType(castToCode(value)); 3424 this.cardinalityBehavior = (Enumeration) value; // Enumeration<ActionCardinalityBehavior> 3425 return value; 3426 case -341064690: // resource 3427 this.resource = castToReference(value); // Reference 3428 return value; 3429 case -1422950858: // action 3430 this.getAction().add((RequestGroupActionComponent) value); // RequestGroupActionComponent 3431 return value; 3432 default: 3433 return super.setProperty(hash, name, value); 3434 } 3435 3436 } 3437 3438 @Override 3439 public Base setProperty(String name, Base value) throws FHIRException { 3440 if (name.equals("prefix")) { 3441 this.prefix = castToString(value); // StringType 3442 } else if (name.equals("title")) { 3443 this.title = castToString(value); // StringType 3444 } else if (name.equals("description")) { 3445 this.description = castToString(value); // StringType 3446 } else if (name.equals("textEquivalent")) { 3447 this.textEquivalent = castToString(value); // StringType 3448 } else if (name.equals("priority")) { 3449 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 3450 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 3451 } else if (name.equals("code")) { 3452 this.getCode().add(castToCodeableConcept(value)); 3453 } else if (name.equals("documentation")) { 3454 this.getDocumentation().add(castToRelatedArtifact(value)); 3455 } else if (name.equals("condition")) { 3456 this.getCondition().add((RequestGroupActionConditionComponent) value); 3457 } else if (name.equals("relatedAction")) { 3458 this.getRelatedAction().add((RequestGroupActionRelatedActionComponent) value); 3459 } else if (name.equals("timing[x]")) { 3460 this.timing = castToType(value); // Type 3461 } else if (name.equals("participant")) { 3462 this.getParticipant().add(castToReference(value)); 3463 } else if (name.equals("type")) { 3464 this.type = castToCodeableConcept(value); // CodeableConcept 3465 } else if (name.equals("groupingBehavior")) { 3466 value = new ActionGroupingBehaviorEnumFactory().fromType(castToCode(value)); 3467 this.groupingBehavior = (Enumeration) value; // Enumeration<ActionGroupingBehavior> 3468 } else if (name.equals("selectionBehavior")) { 3469 value = new ActionSelectionBehaviorEnumFactory().fromType(castToCode(value)); 3470 this.selectionBehavior = (Enumeration) value; // Enumeration<ActionSelectionBehavior> 3471 } else if (name.equals("requiredBehavior")) { 3472 value = new ActionRequiredBehaviorEnumFactory().fromType(castToCode(value)); 3473 this.requiredBehavior = (Enumeration) value; // Enumeration<ActionRequiredBehavior> 3474 } else if (name.equals("precheckBehavior")) { 3475 value = new ActionPrecheckBehaviorEnumFactory().fromType(castToCode(value)); 3476 this.precheckBehavior = (Enumeration) value; // Enumeration<ActionPrecheckBehavior> 3477 } else if (name.equals("cardinalityBehavior")) { 3478 value = new ActionCardinalityBehaviorEnumFactory().fromType(castToCode(value)); 3479 this.cardinalityBehavior = (Enumeration) value; // Enumeration<ActionCardinalityBehavior> 3480 } else if (name.equals("resource")) { 3481 this.resource = castToReference(value); // Reference 3482 } else if (name.equals("action")) { 3483 this.getAction().add((RequestGroupActionComponent) value); 3484 } else 3485 return super.setProperty(name, value); 3486 return value; 3487 } 3488 3489 @Override 3490 public void removeChild(String name, Base value) throws FHIRException { 3491 if (name.equals("prefix")) { 3492 this.prefix = null; 3493 } else if (name.equals("title")) { 3494 this.title = null; 3495 } else if (name.equals("description")) { 3496 this.description = null; 3497 } else if (name.equals("textEquivalent")) { 3498 this.textEquivalent = null; 3499 } else if (name.equals("priority")) { 3500 this.priority = null; 3501 } else if (name.equals("code")) { 3502 this.getCode().remove(castToCodeableConcept(value)); 3503 } else if (name.equals("documentation")) { 3504 this.getDocumentation().remove(castToRelatedArtifact(value)); 3505 } else if (name.equals("condition")) { 3506 this.getCondition().remove((RequestGroupActionConditionComponent) value); 3507 } else if (name.equals("relatedAction")) { 3508 this.getRelatedAction().remove((RequestGroupActionRelatedActionComponent) value); 3509 } else if (name.equals("timing[x]")) { 3510 this.timing = null; 3511 } else if (name.equals("participant")) { 3512 this.getParticipant().remove(castToReference(value)); 3513 } else if (name.equals("type")) { 3514 this.type = null; 3515 } else if (name.equals("groupingBehavior")) { 3516 this.groupingBehavior = null; 3517 } else if (name.equals("selectionBehavior")) { 3518 this.selectionBehavior = null; 3519 } else if (name.equals("requiredBehavior")) { 3520 this.requiredBehavior = null; 3521 } else if (name.equals("precheckBehavior")) { 3522 this.precheckBehavior = null; 3523 } else if (name.equals("cardinalityBehavior")) { 3524 this.cardinalityBehavior = null; 3525 } else if (name.equals("resource")) { 3526 this.resource = null; 3527 } else if (name.equals("action")) { 3528 this.getAction().remove((RequestGroupActionComponent) value); 3529 } else 3530 super.removeChild(name, value); 3531 3532 } 3533 3534 @Override 3535 public Base makeProperty(int hash, String name) throws FHIRException { 3536 switch (hash) { 3537 case -980110702: 3538 return getPrefixElement(); 3539 case 110371416: 3540 return getTitleElement(); 3541 case -1724546052: 3542 return getDescriptionElement(); 3543 case -900391049: 3544 return getTextEquivalentElement(); 3545 case -1165461084: 3546 return getPriorityElement(); 3547 case 3059181: 3548 return addCode(); 3549 case 1587405498: 3550 return addDocumentation(); 3551 case -861311717: 3552 return addCondition(); 3553 case -384107967: 3554 return addRelatedAction(); 3555 case 164632566: 3556 return getTiming(); 3557 case -873664438: 3558 return getTiming(); 3559 case 767422259: 3560 return addParticipant(); 3561 case 3575610: 3562 return getType(); 3563 case 586678389: 3564 return getGroupingBehaviorElement(); 3565 case 168639486: 3566 return getSelectionBehaviorElement(); 3567 case -1163906287: 3568 return getRequiredBehaviorElement(); 3569 case -1174249033: 3570 return getPrecheckBehaviorElement(); 3571 case -922577408: 3572 return getCardinalityBehaviorElement(); 3573 case -341064690: 3574 return getResource(); 3575 case -1422950858: 3576 return addAction(); 3577 default: 3578 return super.makeProperty(hash, name); 3579 } 3580 3581 } 3582 3583 @Override 3584 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3585 switch (hash) { 3586 case -980110702: 3587 /* prefix */ return new String[] { "string" }; 3588 case 110371416: 3589 /* title */ return new String[] { "string" }; 3590 case -1724546052: 3591 /* description */ return new String[] { "string" }; 3592 case -900391049: 3593 /* textEquivalent */ return new String[] { "string" }; 3594 case -1165461084: 3595 /* priority */ return new String[] { "code" }; 3596 case 3059181: 3597 /* code */ return new String[] { "CodeableConcept" }; 3598 case 1587405498: 3599 /* documentation */ return new String[] { "RelatedArtifact" }; 3600 case -861311717: 3601 /* condition */ return new String[] {}; 3602 case -384107967: 3603 /* relatedAction */ return new String[] {}; 3604 case -873664438: 3605 /* timing */ return new String[] { "dateTime", "Age", "Period", "Duration", "Range", "Timing" }; 3606 case 767422259: 3607 /* participant */ return new String[] { "Reference" }; 3608 case 3575610: 3609 /* type */ return new String[] { "CodeableConcept" }; 3610 case 586678389: 3611 /* groupingBehavior */ return new String[] { "code" }; 3612 case 168639486: 3613 /* selectionBehavior */ return new String[] { "code" }; 3614 case -1163906287: 3615 /* requiredBehavior */ return new String[] { "code" }; 3616 case -1174249033: 3617 /* precheckBehavior */ return new String[] { "code" }; 3618 case -922577408: 3619 /* cardinalityBehavior */ return new String[] { "code" }; 3620 case -341064690: 3621 /* resource */ return new String[] { "Reference" }; 3622 case -1422950858: 3623 /* action */ return new String[] { "@RequestGroup.action" }; 3624 default: 3625 return super.getTypesForProperty(hash, name); 3626 } 3627 3628 } 3629 3630 @Override 3631 public Base addChild(String name) throws FHIRException { 3632 if (name.equals("prefix")) { 3633 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.prefix"); 3634 } else if (name.equals("title")) { 3635 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.title"); 3636 } else if (name.equals("description")) { 3637 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.description"); 3638 } else if (name.equals("textEquivalent")) { 3639 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.textEquivalent"); 3640 } else if (name.equals("priority")) { 3641 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.priority"); 3642 } else if (name.equals("code")) { 3643 return addCode(); 3644 } else if (name.equals("documentation")) { 3645 return addDocumentation(); 3646 } else if (name.equals("condition")) { 3647 return addCondition(); 3648 } else if (name.equals("relatedAction")) { 3649 return addRelatedAction(); 3650 } else if (name.equals("timingDateTime")) { 3651 this.timing = new DateTimeType(); 3652 return this.timing; 3653 } else if (name.equals("timingAge")) { 3654 this.timing = new Age(); 3655 return this.timing; 3656 } else if (name.equals("timingPeriod")) { 3657 this.timing = new Period(); 3658 return this.timing; 3659 } else if (name.equals("timingDuration")) { 3660 this.timing = new Duration(); 3661 return this.timing; 3662 } else if (name.equals("timingRange")) { 3663 this.timing = new Range(); 3664 return this.timing; 3665 } else if (name.equals("timingTiming")) { 3666 this.timing = new Timing(); 3667 return this.timing; 3668 } else if (name.equals("participant")) { 3669 return addParticipant(); 3670 } else if (name.equals("type")) { 3671 this.type = new CodeableConcept(); 3672 return this.type; 3673 } else if (name.equals("groupingBehavior")) { 3674 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.groupingBehavior"); 3675 } else if (name.equals("selectionBehavior")) { 3676 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.selectionBehavior"); 3677 } else if (name.equals("requiredBehavior")) { 3678 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.requiredBehavior"); 3679 } else if (name.equals("precheckBehavior")) { 3680 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.precheckBehavior"); 3681 } else if (name.equals("cardinalityBehavior")) { 3682 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.cardinalityBehavior"); 3683 } else if (name.equals("resource")) { 3684 this.resource = new Reference(); 3685 return this.resource; 3686 } else if (name.equals("action")) { 3687 return addAction(); 3688 } else 3689 return super.addChild(name); 3690 } 3691 3692 public RequestGroupActionComponent copy() { 3693 RequestGroupActionComponent dst = new RequestGroupActionComponent(); 3694 copyValues(dst); 3695 return dst; 3696 } 3697 3698 public void copyValues(RequestGroupActionComponent dst) { 3699 super.copyValues(dst); 3700 dst.prefix = prefix == null ? null : prefix.copy(); 3701 dst.title = title == null ? null : title.copy(); 3702 dst.description = description == null ? null : description.copy(); 3703 dst.textEquivalent = textEquivalent == null ? null : textEquivalent.copy(); 3704 dst.priority = priority == null ? null : priority.copy(); 3705 if (code != null) { 3706 dst.code = new ArrayList<CodeableConcept>(); 3707 for (CodeableConcept i : code) 3708 dst.code.add(i.copy()); 3709 } 3710 ; 3711 if (documentation != null) { 3712 dst.documentation = new ArrayList<RelatedArtifact>(); 3713 for (RelatedArtifact i : documentation) 3714 dst.documentation.add(i.copy()); 3715 } 3716 ; 3717 if (condition != null) { 3718 dst.condition = new ArrayList<RequestGroupActionConditionComponent>(); 3719 for (RequestGroupActionConditionComponent i : condition) 3720 dst.condition.add(i.copy()); 3721 } 3722 ; 3723 if (relatedAction != null) { 3724 dst.relatedAction = new ArrayList<RequestGroupActionRelatedActionComponent>(); 3725 for (RequestGroupActionRelatedActionComponent i : relatedAction) 3726 dst.relatedAction.add(i.copy()); 3727 } 3728 ; 3729 dst.timing = timing == null ? null : timing.copy(); 3730 if (participant != null) { 3731 dst.participant = new ArrayList<Reference>(); 3732 for (Reference i : participant) 3733 dst.participant.add(i.copy()); 3734 } 3735 ; 3736 dst.type = type == null ? null : type.copy(); 3737 dst.groupingBehavior = groupingBehavior == null ? null : groupingBehavior.copy(); 3738 dst.selectionBehavior = selectionBehavior == null ? null : selectionBehavior.copy(); 3739 dst.requiredBehavior = requiredBehavior == null ? null : requiredBehavior.copy(); 3740 dst.precheckBehavior = precheckBehavior == null ? null : precheckBehavior.copy(); 3741 dst.cardinalityBehavior = cardinalityBehavior == null ? null : cardinalityBehavior.copy(); 3742 dst.resource = resource == null ? null : resource.copy(); 3743 if (action != null) { 3744 dst.action = new ArrayList<RequestGroupActionComponent>(); 3745 for (RequestGroupActionComponent i : action) 3746 dst.action.add(i.copy()); 3747 } 3748 ; 3749 } 3750 3751 @Override 3752 public boolean equalsDeep(Base other_) { 3753 if (!super.equalsDeep(other_)) 3754 return false; 3755 if (!(other_ instanceof RequestGroupActionComponent)) 3756 return false; 3757 RequestGroupActionComponent o = (RequestGroupActionComponent) other_; 3758 return compareDeep(prefix, o.prefix, true) && compareDeep(title, o.title, true) 3759 && compareDeep(description, o.description, true) && compareDeep(textEquivalent, o.textEquivalent, true) 3760 && compareDeep(priority, o.priority, true) && compareDeep(code, o.code, true) 3761 && compareDeep(documentation, o.documentation, true) && compareDeep(condition, o.condition, true) 3762 && compareDeep(relatedAction, o.relatedAction, true) && compareDeep(timing, o.timing, true) 3763 && compareDeep(participant, o.participant, true) && compareDeep(type, o.type, true) 3764 && compareDeep(groupingBehavior, o.groupingBehavior, true) 3765 && compareDeep(selectionBehavior, o.selectionBehavior, true) 3766 && compareDeep(requiredBehavior, o.requiredBehavior, true) 3767 && compareDeep(precheckBehavior, o.precheckBehavior, true) 3768 && compareDeep(cardinalityBehavior, o.cardinalityBehavior, true) && compareDeep(resource, o.resource, true) 3769 && compareDeep(action, o.action, true); 3770 } 3771 3772 @Override 3773 public boolean equalsShallow(Base other_) { 3774 if (!super.equalsShallow(other_)) 3775 return false; 3776 if (!(other_ instanceof RequestGroupActionComponent)) 3777 return false; 3778 RequestGroupActionComponent o = (RequestGroupActionComponent) other_; 3779 return compareValues(prefix, o.prefix, true) && compareValues(title, o.title, true) 3780 && compareValues(description, o.description, true) && compareValues(textEquivalent, o.textEquivalent, true) 3781 && compareValues(priority, o.priority, true) && compareValues(groupingBehavior, o.groupingBehavior, true) 3782 && compareValues(selectionBehavior, o.selectionBehavior, true) 3783 && compareValues(requiredBehavior, o.requiredBehavior, true) 3784 && compareValues(precheckBehavior, o.precheckBehavior, true) 3785 && compareValues(cardinalityBehavior, o.cardinalityBehavior, true); 3786 } 3787 3788 public boolean isEmpty() { 3789 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(prefix, title, description, textEquivalent, 3790 priority, code, documentation, condition, relatedAction, timing, participant, type, groupingBehavior, 3791 selectionBehavior, requiredBehavior, precheckBehavior, cardinalityBehavior, resource, action); 3792 } 3793 3794 public String fhirType() { 3795 return "RequestGroup.action"; 3796 3797 } 3798 3799 } 3800 3801 @Block() 3802 public static class RequestGroupActionConditionComponent extends BackboneElement implements IBaseBackboneElement { 3803 /** 3804 * The kind of condition. 3805 */ 3806 @Child(name = "kind", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 3807 @Description(shortDefinition = "applicability | start | stop", formalDefinition = "The kind of condition.") 3808 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-condition-kind") 3809 protected Enumeration<ActionConditionKind> kind; 3810 3811 /** 3812 * An expression that returns true or false, indicating whether or not the 3813 * condition is satisfied. 3814 */ 3815 @Child(name = "expression", type = { 3816 Expression.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 3817 @Description(shortDefinition = "Boolean-valued expression", formalDefinition = "An expression that returns true or false, indicating whether or not the condition is satisfied.") 3818 protected Expression expression; 3819 3820 private static final long serialVersionUID = -455150438L; 3821 3822 /** 3823 * Constructor 3824 */ 3825 public RequestGroupActionConditionComponent() { 3826 super(); 3827 } 3828 3829 /** 3830 * Constructor 3831 */ 3832 public RequestGroupActionConditionComponent(Enumeration<ActionConditionKind> kind) { 3833 super(); 3834 this.kind = kind; 3835 } 3836 3837 /** 3838 * @return {@link #kind} (The kind of condition.). This is the underlying object 3839 * with id, value and extensions. The accessor "getKind" gives direct 3840 * access to the value 3841 */ 3842 public Enumeration<ActionConditionKind> getKindElement() { 3843 if (this.kind == null) 3844 if (Configuration.errorOnAutoCreate()) 3845 throw new Error("Attempt to auto-create RequestGroupActionConditionComponent.kind"); 3846 else if (Configuration.doAutoCreate()) 3847 this.kind = new Enumeration<ActionConditionKind>(new ActionConditionKindEnumFactory()); // bb 3848 return this.kind; 3849 } 3850 3851 public boolean hasKindElement() { 3852 return this.kind != null && !this.kind.isEmpty(); 3853 } 3854 3855 public boolean hasKind() { 3856 return this.kind != null && !this.kind.isEmpty(); 3857 } 3858 3859 /** 3860 * @param value {@link #kind} (The kind of condition.). This is the underlying 3861 * object with id, value and extensions. The accessor "getKind" 3862 * gives direct access to the value 3863 */ 3864 public RequestGroupActionConditionComponent setKindElement(Enumeration<ActionConditionKind> value) { 3865 this.kind = value; 3866 return this; 3867 } 3868 3869 /** 3870 * @return The kind of condition. 3871 */ 3872 public ActionConditionKind getKind() { 3873 return this.kind == null ? null : this.kind.getValue(); 3874 } 3875 3876 /** 3877 * @param value The kind of condition. 3878 */ 3879 public RequestGroupActionConditionComponent setKind(ActionConditionKind value) { 3880 if (this.kind == null) 3881 this.kind = new Enumeration<ActionConditionKind>(new ActionConditionKindEnumFactory()); 3882 this.kind.setValue(value); 3883 return this; 3884 } 3885 3886 /** 3887 * @return {@link #expression} (An expression that returns true or false, 3888 * indicating whether or not the condition is satisfied.) 3889 */ 3890 public Expression getExpression() { 3891 if (this.expression == null) 3892 if (Configuration.errorOnAutoCreate()) 3893 throw new Error("Attempt to auto-create RequestGroupActionConditionComponent.expression"); 3894 else if (Configuration.doAutoCreate()) 3895 this.expression = new Expression(); // cc 3896 return this.expression; 3897 } 3898 3899 public boolean hasExpression() { 3900 return this.expression != null && !this.expression.isEmpty(); 3901 } 3902 3903 /** 3904 * @param value {@link #expression} (An expression that returns true or false, 3905 * indicating whether or not the condition is satisfied.) 3906 */ 3907 public RequestGroupActionConditionComponent setExpression(Expression value) { 3908 this.expression = value; 3909 return this; 3910 } 3911 3912 protected void listChildren(List<Property> children) { 3913 super.listChildren(children); 3914 children.add(new Property("kind", "code", "The kind of condition.", 0, 1, kind)); 3915 children.add(new Property("expression", "Expression", 3916 "An expression that returns true or false, indicating whether or not the condition is satisfied.", 0, 1, 3917 expression)); 3918 } 3919 3920 @Override 3921 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3922 switch (_hash) { 3923 case 3292052: 3924 /* kind */ return new Property("kind", "code", "The kind of condition.", 0, 1, kind); 3925 case -1795452264: 3926 /* expression */ return new Property("expression", "Expression", 3927 "An expression that returns true or false, indicating whether or not the condition is satisfied.", 0, 1, 3928 expression); 3929 default: 3930 return super.getNamedProperty(_hash, _name, _checkValid); 3931 } 3932 3933 } 3934 3935 @Override 3936 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3937 switch (hash) { 3938 case 3292052: 3939 /* kind */ return this.kind == null ? new Base[0] : new Base[] { this.kind }; // Enumeration<ActionConditionKind> 3940 case -1795452264: 3941 /* expression */ return this.expression == null ? new Base[0] : new Base[] { this.expression }; // Expression 3942 default: 3943 return super.getProperty(hash, name, checkValid); 3944 } 3945 3946 } 3947 3948 @Override 3949 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3950 switch (hash) { 3951 case 3292052: // kind 3952 value = new ActionConditionKindEnumFactory().fromType(castToCode(value)); 3953 this.kind = (Enumeration) value; // Enumeration<ActionConditionKind> 3954 return value; 3955 case -1795452264: // expression 3956 this.expression = castToExpression(value); // Expression 3957 return value; 3958 default: 3959 return super.setProperty(hash, name, value); 3960 } 3961 3962 } 3963 3964 @Override 3965 public Base setProperty(String name, Base value) throws FHIRException { 3966 if (name.equals("kind")) { 3967 value = new ActionConditionKindEnumFactory().fromType(castToCode(value)); 3968 this.kind = (Enumeration) value; // Enumeration<ActionConditionKind> 3969 } else if (name.equals("expression")) { 3970 this.expression = castToExpression(value); // Expression 3971 } else 3972 return super.setProperty(name, value); 3973 return value; 3974 } 3975 3976 @Override 3977 public void removeChild(String name, Base value) throws FHIRException { 3978 if (name.equals("kind")) { 3979 this.kind = null; 3980 } else if (name.equals("expression")) { 3981 this.expression = null; 3982 } else 3983 super.removeChild(name, value); 3984 3985 } 3986 3987 @Override 3988 public Base makeProperty(int hash, String name) throws FHIRException { 3989 switch (hash) { 3990 case 3292052: 3991 return getKindElement(); 3992 case -1795452264: 3993 return getExpression(); 3994 default: 3995 return super.makeProperty(hash, name); 3996 } 3997 3998 } 3999 4000 @Override 4001 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4002 switch (hash) { 4003 case 3292052: 4004 /* kind */ return new String[] { "code" }; 4005 case -1795452264: 4006 /* expression */ return new String[] { "Expression" }; 4007 default: 4008 return super.getTypesForProperty(hash, name); 4009 } 4010 4011 } 4012 4013 @Override 4014 public Base addChild(String name) throws FHIRException { 4015 if (name.equals("kind")) { 4016 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.kind"); 4017 } else if (name.equals("expression")) { 4018 this.expression = new Expression(); 4019 return this.expression; 4020 } else 4021 return super.addChild(name); 4022 } 4023 4024 public RequestGroupActionConditionComponent copy() { 4025 RequestGroupActionConditionComponent dst = new RequestGroupActionConditionComponent(); 4026 copyValues(dst); 4027 return dst; 4028 } 4029 4030 public void copyValues(RequestGroupActionConditionComponent dst) { 4031 super.copyValues(dst); 4032 dst.kind = kind == null ? null : kind.copy(); 4033 dst.expression = expression == null ? null : expression.copy(); 4034 } 4035 4036 @Override 4037 public boolean equalsDeep(Base other_) { 4038 if (!super.equalsDeep(other_)) 4039 return false; 4040 if (!(other_ instanceof RequestGroupActionConditionComponent)) 4041 return false; 4042 RequestGroupActionConditionComponent o = (RequestGroupActionConditionComponent) other_; 4043 return compareDeep(kind, o.kind, true) && compareDeep(expression, o.expression, true); 4044 } 4045 4046 @Override 4047 public boolean equalsShallow(Base other_) { 4048 if (!super.equalsShallow(other_)) 4049 return false; 4050 if (!(other_ instanceof RequestGroupActionConditionComponent)) 4051 return false; 4052 RequestGroupActionConditionComponent o = (RequestGroupActionConditionComponent) other_; 4053 return compareValues(kind, o.kind, true); 4054 } 4055 4056 public boolean isEmpty() { 4057 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(kind, expression); 4058 } 4059 4060 public String fhirType() { 4061 return "RequestGroup.action.condition"; 4062 4063 } 4064 4065 } 4066 4067 @Block() 4068 public static class RequestGroupActionRelatedActionComponent extends BackboneElement implements IBaseBackboneElement { 4069 /** 4070 * The element id of the action this is related to. 4071 */ 4072 @Child(name = "actionId", type = { IdType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 4073 @Description(shortDefinition = "What action this is related to", formalDefinition = "The element id of the action this is related to.") 4074 protected IdType actionId; 4075 4076 /** 4077 * The relationship of this action to the related action. 4078 */ 4079 @Child(name = "relationship", type = { 4080 CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 4081 @Description(shortDefinition = "before-start | before | before-end | concurrent-with-start | concurrent | concurrent-with-end | after-start | after | after-end", formalDefinition = "The relationship of this action to the related action.") 4082 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-relationship-type") 4083 protected Enumeration<ActionRelationshipType> relationship; 4084 4085 /** 4086 * A duration or range of durations to apply to the relationship. For example, 4087 * 30-60 minutes before. 4088 */ 4089 @Child(name = "offset", type = { Duration.class, 4090 Range.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 4091 @Description(shortDefinition = "Time offset for the relationship", formalDefinition = "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.") 4092 protected Type offset; 4093 4094 private static final long serialVersionUID = 1063306770L; 4095 4096 /** 4097 * Constructor 4098 */ 4099 public RequestGroupActionRelatedActionComponent() { 4100 super(); 4101 } 4102 4103 /** 4104 * Constructor 4105 */ 4106 public RequestGroupActionRelatedActionComponent(IdType actionId, Enumeration<ActionRelationshipType> relationship) { 4107 super(); 4108 this.actionId = actionId; 4109 this.relationship = relationship; 4110 } 4111 4112 /** 4113 * @return {@link #actionId} (The element id of the action this is related to.). 4114 * This is the underlying object with id, value and extensions. The 4115 * accessor "getActionId" gives direct access to the value 4116 */ 4117 public IdType getActionIdElement() { 4118 if (this.actionId == null) 4119 if (Configuration.errorOnAutoCreate()) 4120 throw new Error("Attempt to auto-create RequestGroupActionRelatedActionComponent.actionId"); 4121 else if (Configuration.doAutoCreate()) 4122 this.actionId = new IdType(); // bb 4123 return this.actionId; 4124 } 4125 4126 public boolean hasActionIdElement() { 4127 return this.actionId != null && !this.actionId.isEmpty(); 4128 } 4129 4130 public boolean hasActionId() { 4131 return this.actionId != null && !this.actionId.isEmpty(); 4132 } 4133 4134 /** 4135 * @param value {@link #actionId} (The element id of the action this is related 4136 * to.). This is the underlying object with id, value and 4137 * extensions. The accessor "getActionId" gives direct access to 4138 * the value 4139 */ 4140 public RequestGroupActionRelatedActionComponent setActionIdElement(IdType value) { 4141 this.actionId = value; 4142 return this; 4143 } 4144 4145 /** 4146 * @return The element id of the action this is related to. 4147 */ 4148 public String getActionId() { 4149 return this.actionId == null ? null : this.actionId.getValue(); 4150 } 4151 4152 /** 4153 * @param value The element id of the action this is related to. 4154 */ 4155 public RequestGroupActionRelatedActionComponent setActionId(String value) { 4156 if (this.actionId == null) 4157 this.actionId = new IdType(); 4158 this.actionId.setValue(value); 4159 return this; 4160 } 4161 4162 /** 4163 * @return {@link #relationship} (The relationship of this action to the related 4164 * action.). This is the underlying object with id, value and 4165 * extensions. The accessor "getRelationship" gives direct access to the 4166 * value 4167 */ 4168 public Enumeration<ActionRelationshipType> getRelationshipElement() { 4169 if (this.relationship == null) 4170 if (Configuration.errorOnAutoCreate()) 4171 throw new Error("Attempt to auto-create RequestGroupActionRelatedActionComponent.relationship"); 4172 else if (Configuration.doAutoCreate()) 4173 this.relationship = new Enumeration<ActionRelationshipType>(new ActionRelationshipTypeEnumFactory()); // bb 4174 return this.relationship; 4175 } 4176 4177 public boolean hasRelationshipElement() { 4178 return this.relationship != null && !this.relationship.isEmpty(); 4179 } 4180 4181 public boolean hasRelationship() { 4182 return this.relationship != null && !this.relationship.isEmpty(); 4183 } 4184 4185 /** 4186 * @param value {@link #relationship} (The relationship of this action to the 4187 * related action.). This is the underlying object with id, value 4188 * and extensions. The accessor "getRelationship" gives direct 4189 * access to the value 4190 */ 4191 public RequestGroupActionRelatedActionComponent setRelationshipElement(Enumeration<ActionRelationshipType> value) { 4192 this.relationship = value; 4193 return this; 4194 } 4195 4196 /** 4197 * @return The relationship of this action to the related action. 4198 */ 4199 public ActionRelationshipType getRelationship() { 4200 return this.relationship == null ? null : this.relationship.getValue(); 4201 } 4202 4203 /** 4204 * @param value The relationship of this action to the related action. 4205 */ 4206 public RequestGroupActionRelatedActionComponent setRelationship(ActionRelationshipType value) { 4207 if (this.relationship == null) 4208 this.relationship = new Enumeration<ActionRelationshipType>(new ActionRelationshipTypeEnumFactory()); 4209 this.relationship.setValue(value); 4210 return this; 4211 } 4212 4213 /** 4214 * @return {@link #offset} (A duration or range of durations to apply to the 4215 * relationship. For example, 30-60 minutes before.) 4216 */ 4217 public Type getOffset() { 4218 return this.offset; 4219 } 4220 4221 /** 4222 * @return {@link #offset} (A duration or range of durations to apply to the 4223 * relationship. For example, 30-60 minutes before.) 4224 */ 4225 public Duration getOffsetDuration() throws FHIRException { 4226 if (this.offset == null) 4227 this.offset = new Duration(); 4228 if (!(this.offset instanceof Duration)) 4229 throw new FHIRException("Type mismatch: the type Duration was expected, but " + this.offset.getClass().getName() 4230 + " was encountered"); 4231 return (Duration) this.offset; 4232 } 4233 4234 public boolean hasOffsetDuration() { 4235 return this != null && this.offset instanceof Duration; 4236 } 4237 4238 /** 4239 * @return {@link #offset} (A duration or range of durations to apply to the 4240 * relationship. For example, 30-60 minutes before.) 4241 */ 4242 public Range getOffsetRange() throws FHIRException { 4243 if (this.offset == null) 4244 this.offset = new Range(); 4245 if (!(this.offset instanceof Range)) 4246 throw new FHIRException( 4247 "Type mismatch: the type Range was expected, but " + this.offset.getClass().getName() + " was encountered"); 4248 return (Range) this.offset; 4249 } 4250 4251 public boolean hasOffsetRange() { 4252 return this != null && this.offset instanceof Range; 4253 } 4254 4255 public boolean hasOffset() { 4256 return this.offset != null && !this.offset.isEmpty(); 4257 } 4258 4259 /** 4260 * @param value {@link #offset} (A duration or range of durations to apply to 4261 * the relationship. For example, 30-60 minutes before.) 4262 */ 4263 public RequestGroupActionRelatedActionComponent setOffset(Type value) { 4264 if (value != null && !(value instanceof Duration || value instanceof Range)) 4265 throw new Error("Not the right type for RequestGroup.action.relatedAction.offset[x]: " + value.fhirType()); 4266 this.offset = value; 4267 return this; 4268 } 4269 4270 protected void listChildren(List<Property> children) { 4271 super.listChildren(children); 4272 children.add(new Property("actionId", "id", "The element id of the action this is related to.", 0, 1, actionId)); 4273 children.add(new Property("relationship", "code", "The relationship of this action to the related action.", 0, 1, 4274 relationship)); 4275 children.add(new Property("offset[x]", "Duration|Range", 4276 "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, 4277 offset)); 4278 } 4279 4280 @Override 4281 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4282 switch (_hash) { 4283 case -1656172047: 4284 /* actionId */ return new Property("actionId", "id", "The element id of the action this is related to.", 0, 1, 4285 actionId); 4286 case -261851592: 4287 /* relationship */ return new Property("relationship", "code", 4288 "The relationship of this action to the related action.", 0, 1, relationship); 4289 case -1960684787: 4290 /* offset[x] */ return new Property("offset[x]", "Duration|Range", 4291 "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, 4292 offset); 4293 case -1019779949: 4294 /* offset */ return new Property("offset[x]", "Duration|Range", 4295 "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, 4296 offset); 4297 case 134075207: 4298 /* offsetDuration */ return new Property("offset[x]", "Duration|Range", 4299 "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, 4300 offset); 4301 case 1263585386: 4302 /* offsetRange */ return new Property("offset[x]", "Duration|Range", 4303 "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, 4304 offset); 4305 default: 4306 return super.getNamedProperty(_hash, _name, _checkValid); 4307 } 4308 4309 } 4310 4311 @Override 4312 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4313 switch (hash) { 4314 case -1656172047: 4315 /* actionId */ return this.actionId == null ? new Base[0] : new Base[] { this.actionId }; // IdType 4316 case -261851592: 4317 /* relationship */ return this.relationship == null ? new Base[0] : new Base[] { this.relationship }; // Enumeration<ActionRelationshipType> 4318 case -1019779949: 4319 /* offset */ return this.offset == null ? new Base[0] : new Base[] { this.offset }; // Type 4320 default: 4321 return super.getProperty(hash, name, checkValid); 4322 } 4323 4324 } 4325 4326 @Override 4327 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4328 switch (hash) { 4329 case -1656172047: // actionId 4330 this.actionId = castToId(value); // IdType 4331 return value; 4332 case -261851592: // relationship 4333 value = new ActionRelationshipTypeEnumFactory().fromType(castToCode(value)); 4334 this.relationship = (Enumeration) value; // Enumeration<ActionRelationshipType> 4335 return value; 4336 case -1019779949: // offset 4337 this.offset = castToType(value); // Type 4338 return value; 4339 default: 4340 return super.setProperty(hash, name, value); 4341 } 4342 4343 } 4344 4345 @Override 4346 public Base setProperty(String name, Base value) throws FHIRException { 4347 if (name.equals("actionId")) { 4348 this.actionId = castToId(value); // IdType 4349 } else if (name.equals("relationship")) { 4350 value = new ActionRelationshipTypeEnumFactory().fromType(castToCode(value)); 4351 this.relationship = (Enumeration) value; // Enumeration<ActionRelationshipType> 4352 } else if (name.equals("offset[x]")) { 4353 this.offset = castToType(value); // Type 4354 } else 4355 return super.setProperty(name, value); 4356 return value; 4357 } 4358 4359 @Override 4360 public void removeChild(String name, Base value) throws FHIRException { 4361 if (name.equals("actionId")) { 4362 this.actionId = null; 4363 } else if (name.equals("relationship")) { 4364 this.relationship = null; 4365 } else if (name.equals("offset[x]")) { 4366 this.offset = null; 4367 } else 4368 super.removeChild(name, value); 4369 4370 } 4371 4372 @Override 4373 public Base makeProperty(int hash, String name) throws FHIRException { 4374 switch (hash) { 4375 case -1656172047: 4376 return getActionIdElement(); 4377 case -261851592: 4378 return getRelationshipElement(); 4379 case -1960684787: 4380 return getOffset(); 4381 case -1019779949: 4382 return getOffset(); 4383 default: 4384 return super.makeProperty(hash, name); 4385 } 4386 4387 } 4388 4389 @Override 4390 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4391 switch (hash) { 4392 case -1656172047: 4393 /* actionId */ return new String[] { "id" }; 4394 case -261851592: 4395 /* relationship */ return new String[] { "code" }; 4396 case -1019779949: 4397 /* offset */ return new String[] { "Duration", "Range" }; 4398 default: 4399 return super.getTypesForProperty(hash, name); 4400 } 4401 4402 } 4403 4404 @Override 4405 public Base addChild(String name) throws FHIRException { 4406 if (name.equals("actionId")) { 4407 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.actionId"); 4408 } else if (name.equals("relationship")) { 4409 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.relationship"); 4410 } else if (name.equals("offsetDuration")) { 4411 this.offset = new Duration(); 4412 return this.offset; 4413 } else if (name.equals("offsetRange")) { 4414 this.offset = new Range(); 4415 return this.offset; 4416 } else 4417 return super.addChild(name); 4418 } 4419 4420 public RequestGroupActionRelatedActionComponent copy() { 4421 RequestGroupActionRelatedActionComponent dst = new RequestGroupActionRelatedActionComponent(); 4422 copyValues(dst); 4423 return dst; 4424 } 4425 4426 public void copyValues(RequestGroupActionRelatedActionComponent dst) { 4427 super.copyValues(dst); 4428 dst.actionId = actionId == null ? null : actionId.copy(); 4429 dst.relationship = relationship == null ? null : relationship.copy(); 4430 dst.offset = offset == null ? null : offset.copy(); 4431 } 4432 4433 @Override 4434 public boolean equalsDeep(Base other_) { 4435 if (!super.equalsDeep(other_)) 4436 return false; 4437 if (!(other_ instanceof RequestGroupActionRelatedActionComponent)) 4438 return false; 4439 RequestGroupActionRelatedActionComponent o = (RequestGroupActionRelatedActionComponent) other_; 4440 return compareDeep(actionId, o.actionId, true) && compareDeep(relationship, o.relationship, true) 4441 && compareDeep(offset, o.offset, true); 4442 } 4443 4444 @Override 4445 public boolean equalsShallow(Base other_) { 4446 if (!super.equalsShallow(other_)) 4447 return false; 4448 if (!(other_ instanceof RequestGroupActionRelatedActionComponent)) 4449 return false; 4450 RequestGroupActionRelatedActionComponent o = (RequestGroupActionRelatedActionComponent) other_; 4451 return compareValues(actionId, o.actionId, true) && compareValues(relationship, o.relationship, true); 4452 } 4453 4454 public boolean isEmpty() { 4455 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(actionId, relationship, offset); 4456 } 4457 4458 public String fhirType() { 4459 return "RequestGroup.action.relatedAction"; 4460 4461 } 4462 4463 } 4464 4465 /** 4466 * Allows a service to provide a unique, business identifier for the request. 4467 */ 4468 @Child(name = "identifier", type = { 4469 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4470 @Description(shortDefinition = "Business identifier", formalDefinition = "Allows a service to provide a unique, business identifier for the request.") 4471 protected List<Identifier> identifier; 4472 4473 /** 4474 * A canonical URL referencing a FHIR-defined protocol, guideline, orderset or 4475 * other definition that is adhered to in whole or in part by this request. 4476 */ 4477 @Child(name = "instantiatesCanonical", type = { 4478 CanonicalType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4479 @Description(shortDefinition = "Instantiates FHIR protocol or definition", formalDefinition = "A canonical URL referencing a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.") 4480 protected List<CanonicalType> instantiatesCanonical; 4481 4482 /** 4483 * A URL referencing an externally defined protocol, guideline, orderset or 4484 * other definition that is adhered to in whole or in part by this request. 4485 */ 4486 @Child(name = "instantiatesUri", type = { 4487 UriType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4488 @Description(shortDefinition = "Instantiates external protocol or definition", formalDefinition = "A URL referencing an externally defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.") 4489 protected List<UriType> instantiatesUri; 4490 4491 /** 4492 * A plan, proposal or order that is fulfilled in whole or in part by this 4493 * request. 4494 */ 4495 @Child(name = "basedOn", type = { 4496 Reference.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4497 @Description(shortDefinition = "Fulfills plan, proposal, or order", formalDefinition = "A plan, proposal or order that is fulfilled in whole or in part by this request.") 4498 protected List<Reference> basedOn; 4499 /** 4500 * The actual objects that are the target of the reference (A plan, proposal or 4501 * order that is fulfilled in whole or in part by this request.) 4502 */ 4503 protected List<Resource> basedOnTarget; 4504 4505 /** 4506 * Completed or terminated request(s) whose function is taken by this new 4507 * request. 4508 */ 4509 @Child(name = "replaces", type = { 4510 Reference.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4511 @Description(shortDefinition = "Request(s) replaced by this request", formalDefinition = "Completed or terminated request(s) whose function is taken by this new request.") 4512 protected List<Reference> replaces; 4513 /** 4514 * The actual objects that are the target of the reference (Completed or 4515 * terminated request(s) whose function is taken by this new request.) 4516 */ 4517 protected List<Resource> replacesTarget; 4518 4519 /** 4520 * A shared identifier common to all requests that were authorized more or less 4521 * simultaneously by a single author, representing the identifier of the 4522 * requisition, prescription or similar form. 4523 */ 4524 @Child(name = "groupIdentifier", type = { 4525 Identifier.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 4526 @Description(shortDefinition = "Composite request this is part of", formalDefinition = "A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.") 4527 protected Identifier groupIdentifier; 4528 4529 /** 4530 * The current state of the request. For request groups, the status reflects the 4531 * status of all the requests in the group. 4532 */ 4533 @Child(name = "status", type = { CodeType.class }, order = 6, min = 1, max = 1, modifier = true, summary = true) 4534 @Description(shortDefinition = "draft | active | on-hold | revoked | completed | entered-in-error | unknown", formalDefinition = "The current state of the request. For request groups, the status reflects the status of all the requests in the group.") 4535 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-status") 4536 protected Enumeration<RequestStatus> status; 4537 4538 /** 4539 * Indicates the level of authority/intentionality associated with the request 4540 * and where the request fits into the workflow chain. 4541 */ 4542 @Child(name = "intent", type = { CodeType.class }, order = 7, min = 1, max = 1, modifier = true, summary = true) 4543 @Description(shortDefinition = "proposal | plan | directive | order | original-order | reflex-order | filler-order | instance-order | option", formalDefinition = "Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain.") 4544 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-intent") 4545 protected Enumeration<RequestIntent> intent; 4546 4547 /** 4548 * Indicates how quickly the request should be addressed with respect to other 4549 * requests. 4550 */ 4551 @Child(name = "priority", type = { CodeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 4552 @Description(shortDefinition = "routine | urgent | asap | stat", formalDefinition = "Indicates how quickly the request should be addressed with respect to other requests.") 4553 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-priority") 4554 protected Enumeration<RequestPriority> priority; 4555 4556 /** 4557 * A code that identifies what the overall request group is. 4558 */ 4559 @Child(name = "code", type = { CodeableConcept.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 4560 @Description(shortDefinition = "What's being requested/ordered", formalDefinition = "A code that identifies what the overall request group is.") 4561 protected CodeableConcept code; 4562 4563 /** 4564 * The subject for which the request group was created. 4565 */ 4566 @Child(name = "subject", type = { Patient.class, 4567 Group.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 4568 @Description(shortDefinition = "Who the request group is about", formalDefinition = "The subject for which the request group was created.") 4569 protected Reference subject; 4570 4571 /** 4572 * The actual object that is the target of the reference (The subject for which 4573 * the request group was created.) 4574 */ 4575 protected Resource subjectTarget; 4576 4577 /** 4578 * Describes the context of the request group, if any. 4579 */ 4580 @Child(name = "encounter", type = { 4581 Encounter.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 4582 @Description(shortDefinition = "Created as part of", formalDefinition = "Describes the context of the request group, if any.") 4583 protected Reference encounter; 4584 4585 /** 4586 * The actual object that is the target of the reference (Describes the context 4587 * of the request group, if any.) 4588 */ 4589 protected Encounter encounterTarget; 4590 4591 /** 4592 * Indicates when the request group was created. 4593 */ 4594 @Child(name = "authoredOn", type = { 4595 DateTimeType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 4596 @Description(shortDefinition = "When the request group was authored", formalDefinition = "Indicates when the request group was created.") 4597 protected DateTimeType authoredOn; 4598 4599 /** 4600 * Provides a reference to the author of the request group. 4601 */ 4602 @Child(name = "author", type = { Device.class, Practitioner.class, 4603 PractitionerRole.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 4604 @Description(shortDefinition = "Device or practitioner that authored the request group", formalDefinition = "Provides a reference to the author of the request group.") 4605 protected Reference author; 4606 4607 /** 4608 * The actual object that is the target of the reference (Provides a reference 4609 * to the author of the request group.) 4610 */ 4611 protected Resource authorTarget; 4612 4613 /** 4614 * Describes the reason for the request group in coded or textual form. 4615 */ 4616 @Child(name = "reasonCode", type = { 4617 CodeableConcept.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4618 @Description(shortDefinition = "Why the request group is needed", formalDefinition = "Describes the reason for the request group in coded or textual form.") 4619 protected List<CodeableConcept> reasonCode; 4620 4621 /** 4622 * Indicates another resource whose existence justifies this request group. 4623 */ 4624 @Child(name = "reasonReference", type = { Condition.class, Observation.class, DiagnosticReport.class, 4625 DocumentReference.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4626 @Description(shortDefinition = "Why the request group is needed", formalDefinition = "Indicates another resource whose existence justifies this request group.") 4627 protected List<Reference> reasonReference; 4628 /** 4629 * The actual objects that are the target of the reference (Indicates another 4630 * resource whose existence justifies this request group.) 4631 */ 4632 protected List<Resource> reasonReferenceTarget; 4633 4634 /** 4635 * Provides a mechanism to communicate additional information about the 4636 * response. 4637 */ 4638 @Child(name = "note", type = { 4639 Annotation.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4640 @Description(shortDefinition = "Additional notes about the response", formalDefinition = "Provides a mechanism to communicate additional information about the response.") 4641 protected List<Annotation> note; 4642 4643 /** 4644 * The actions, if any, produced by the evaluation of the artifact. 4645 */ 4646 @Child(name = "action", type = {}, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4647 @Description(shortDefinition = "Proposed actions, if any", formalDefinition = "The actions, if any, produced by the evaluation of the artifact.") 4648 protected List<RequestGroupActionComponent> action; 4649 4650 private static final long serialVersionUID = -2053492070L; 4651 4652 /** 4653 * Constructor 4654 */ 4655 public RequestGroup() { 4656 super(); 4657 } 4658 4659 /** 4660 * Constructor 4661 */ 4662 public RequestGroup(Enumeration<RequestStatus> status, Enumeration<RequestIntent> intent) { 4663 super(); 4664 this.status = status; 4665 this.intent = intent; 4666 } 4667 4668 /** 4669 * @return {@link #identifier} (Allows a service to provide a unique, business 4670 * identifier for the request.) 4671 */ 4672 public List<Identifier> getIdentifier() { 4673 if (this.identifier == null) 4674 this.identifier = new ArrayList<Identifier>(); 4675 return this.identifier; 4676 } 4677 4678 /** 4679 * @return Returns a reference to <code>this</code> for easy method chaining 4680 */ 4681 public RequestGroup setIdentifier(List<Identifier> theIdentifier) { 4682 this.identifier = theIdentifier; 4683 return this; 4684 } 4685 4686 public boolean hasIdentifier() { 4687 if (this.identifier == null) 4688 return false; 4689 for (Identifier item : this.identifier) 4690 if (!item.isEmpty()) 4691 return true; 4692 return false; 4693 } 4694 4695 public Identifier addIdentifier() { // 3 4696 Identifier t = new Identifier(); 4697 if (this.identifier == null) 4698 this.identifier = new ArrayList<Identifier>(); 4699 this.identifier.add(t); 4700 return t; 4701 } 4702 4703 public RequestGroup addIdentifier(Identifier t) { // 3 4704 if (t == null) 4705 return this; 4706 if (this.identifier == null) 4707 this.identifier = new ArrayList<Identifier>(); 4708 this.identifier.add(t); 4709 return this; 4710 } 4711 4712 /** 4713 * @return The first repetition of repeating field {@link #identifier}, creating 4714 * it if it does not already exist 4715 */ 4716 public Identifier getIdentifierFirstRep() { 4717 if (getIdentifier().isEmpty()) { 4718 addIdentifier(); 4719 } 4720 return getIdentifier().get(0); 4721 } 4722 4723 /** 4724 * @return {@link #instantiatesCanonical} (A canonical URL referencing a 4725 * FHIR-defined protocol, guideline, orderset or other definition that 4726 * is adhered to in whole or in part by this request.) 4727 */ 4728 public List<CanonicalType> getInstantiatesCanonical() { 4729 if (this.instantiatesCanonical == null) 4730 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 4731 return this.instantiatesCanonical; 4732 } 4733 4734 /** 4735 * @return Returns a reference to <code>this</code> for easy method chaining 4736 */ 4737 public RequestGroup setInstantiatesCanonical(List<CanonicalType> theInstantiatesCanonical) { 4738 this.instantiatesCanonical = theInstantiatesCanonical; 4739 return this; 4740 } 4741 4742 public boolean hasInstantiatesCanonical() { 4743 if (this.instantiatesCanonical == null) 4744 return false; 4745 for (CanonicalType item : this.instantiatesCanonical) 4746 if (!item.isEmpty()) 4747 return true; 4748 return false; 4749 } 4750 4751 /** 4752 * @return {@link #instantiatesCanonical} (A canonical URL referencing a 4753 * FHIR-defined protocol, guideline, orderset or other definition that 4754 * is adhered to in whole or in part by this request.) 4755 */ 4756 public CanonicalType addInstantiatesCanonicalElement() {// 2 4757 CanonicalType t = new CanonicalType(); 4758 if (this.instantiatesCanonical == null) 4759 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 4760 this.instantiatesCanonical.add(t); 4761 return t; 4762 } 4763 4764 /** 4765 * @param value {@link #instantiatesCanonical} (A canonical URL referencing a 4766 * FHIR-defined protocol, guideline, orderset or other definition 4767 * that is adhered to in whole or in part by this request.) 4768 */ 4769 public RequestGroup addInstantiatesCanonical(String value) { // 1 4770 CanonicalType t = new CanonicalType(); 4771 t.setValue(value); 4772 if (this.instantiatesCanonical == null) 4773 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 4774 this.instantiatesCanonical.add(t); 4775 return this; 4776 } 4777 4778 /** 4779 * @param value {@link #instantiatesCanonical} (A canonical URL referencing a 4780 * FHIR-defined protocol, guideline, orderset or other definition 4781 * that is adhered to in whole or in part by this request.) 4782 */ 4783 public boolean hasInstantiatesCanonical(String value) { 4784 if (this.instantiatesCanonical == null) 4785 return false; 4786 for (CanonicalType v : this.instantiatesCanonical) 4787 if (v.getValue().equals(value)) // canonical 4788 return true; 4789 return false; 4790 } 4791 4792 /** 4793 * @return {@link #instantiatesUri} (A URL referencing an externally defined 4794 * protocol, guideline, orderset or other definition that is adhered to 4795 * in whole or in part by this request.) 4796 */ 4797 public List<UriType> getInstantiatesUri() { 4798 if (this.instantiatesUri == null) 4799 this.instantiatesUri = new ArrayList<UriType>(); 4800 return this.instantiatesUri; 4801 } 4802 4803 /** 4804 * @return Returns a reference to <code>this</code> for easy method chaining 4805 */ 4806 public RequestGroup setInstantiatesUri(List<UriType> theInstantiatesUri) { 4807 this.instantiatesUri = theInstantiatesUri; 4808 return this; 4809 } 4810 4811 public boolean hasInstantiatesUri() { 4812 if (this.instantiatesUri == null) 4813 return false; 4814 for (UriType item : this.instantiatesUri) 4815 if (!item.isEmpty()) 4816 return true; 4817 return false; 4818 } 4819 4820 /** 4821 * @return {@link #instantiatesUri} (A URL referencing an externally defined 4822 * protocol, guideline, orderset or other definition that is adhered to 4823 * in whole or in part by this request.) 4824 */ 4825 public UriType addInstantiatesUriElement() {// 2 4826 UriType t = new UriType(); 4827 if (this.instantiatesUri == null) 4828 this.instantiatesUri = new ArrayList<UriType>(); 4829 this.instantiatesUri.add(t); 4830 return t; 4831 } 4832 4833 /** 4834 * @param value {@link #instantiatesUri} (A URL referencing an externally 4835 * defined protocol, guideline, orderset or other definition that 4836 * is adhered to in whole or in part by this request.) 4837 */ 4838 public RequestGroup addInstantiatesUri(String value) { // 1 4839 UriType t = new UriType(); 4840 t.setValue(value); 4841 if (this.instantiatesUri == null) 4842 this.instantiatesUri = new ArrayList<UriType>(); 4843 this.instantiatesUri.add(t); 4844 return this; 4845 } 4846 4847 /** 4848 * @param value {@link #instantiatesUri} (A URL referencing an externally 4849 * defined protocol, guideline, orderset or other definition that 4850 * is adhered to in whole or in part by this request.) 4851 */ 4852 public boolean hasInstantiatesUri(String value) { 4853 if (this.instantiatesUri == null) 4854 return false; 4855 for (UriType v : this.instantiatesUri) 4856 if (v.getValue().equals(value)) // uri 4857 return true; 4858 return false; 4859 } 4860 4861 /** 4862 * @return {@link #basedOn} (A plan, proposal or order that is fulfilled in 4863 * whole or in part by this request.) 4864 */ 4865 public List<Reference> getBasedOn() { 4866 if (this.basedOn == null) 4867 this.basedOn = new ArrayList<Reference>(); 4868 return this.basedOn; 4869 } 4870 4871 /** 4872 * @return Returns a reference to <code>this</code> for easy method chaining 4873 */ 4874 public RequestGroup setBasedOn(List<Reference> theBasedOn) { 4875 this.basedOn = theBasedOn; 4876 return this; 4877 } 4878 4879 public boolean hasBasedOn() { 4880 if (this.basedOn == null) 4881 return false; 4882 for (Reference item : this.basedOn) 4883 if (!item.isEmpty()) 4884 return true; 4885 return false; 4886 } 4887 4888 public Reference addBasedOn() { // 3 4889 Reference t = new Reference(); 4890 if (this.basedOn == null) 4891 this.basedOn = new ArrayList<Reference>(); 4892 this.basedOn.add(t); 4893 return t; 4894 } 4895 4896 public RequestGroup addBasedOn(Reference t) { // 3 4897 if (t == null) 4898 return this; 4899 if (this.basedOn == null) 4900 this.basedOn = new ArrayList<Reference>(); 4901 this.basedOn.add(t); 4902 return this; 4903 } 4904 4905 /** 4906 * @return The first repetition of repeating field {@link #basedOn}, creating it 4907 * if it does not already exist 4908 */ 4909 public Reference getBasedOnFirstRep() { 4910 if (getBasedOn().isEmpty()) { 4911 addBasedOn(); 4912 } 4913 return getBasedOn().get(0); 4914 } 4915 4916 /** 4917 * @deprecated Use Reference#setResource(IBaseResource) instead 4918 */ 4919 @Deprecated 4920 public List<Resource> getBasedOnTarget() { 4921 if (this.basedOnTarget == null) 4922 this.basedOnTarget = new ArrayList<Resource>(); 4923 return this.basedOnTarget; 4924 } 4925 4926 /** 4927 * @return {@link #replaces} (Completed or terminated request(s) whose function 4928 * is taken by this new request.) 4929 */ 4930 public List<Reference> getReplaces() { 4931 if (this.replaces == null) 4932 this.replaces = new ArrayList<Reference>(); 4933 return this.replaces; 4934 } 4935 4936 /** 4937 * @return Returns a reference to <code>this</code> for easy method chaining 4938 */ 4939 public RequestGroup setReplaces(List<Reference> theReplaces) { 4940 this.replaces = theReplaces; 4941 return this; 4942 } 4943 4944 public boolean hasReplaces() { 4945 if (this.replaces == null) 4946 return false; 4947 for (Reference item : this.replaces) 4948 if (!item.isEmpty()) 4949 return true; 4950 return false; 4951 } 4952 4953 public Reference addReplaces() { // 3 4954 Reference t = new Reference(); 4955 if (this.replaces == null) 4956 this.replaces = new ArrayList<Reference>(); 4957 this.replaces.add(t); 4958 return t; 4959 } 4960 4961 public RequestGroup addReplaces(Reference t) { // 3 4962 if (t == null) 4963 return this; 4964 if (this.replaces == null) 4965 this.replaces = new ArrayList<Reference>(); 4966 this.replaces.add(t); 4967 return this; 4968 } 4969 4970 /** 4971 * @return The first repetition of repeating field {@link #replaces}, creating 4972 * it if it does not already exist 4973 */ 4974 public Reference getReplacesFirstRep() { 4975 if (getReplaces().isEmpty()) { 4976 addReplaces(); 4977 } 4978 return getReplaces().get(0); 4979 } 4980 4981 /** 4982 * @deprecated Use Reference#setResource(IBaseResource) instead 4983 */ 4984 @Deprecated 4985 public List<Resource> getReplacesTarget() { 4986 if (this.replacesTarget == null) 4987 this.replacesTarget = new ArrayList<Resource>(); 4988 return this.replacesTarget; 4989 } 4990 4991 /** 4992 * @return {@link #groupIdentifier} (A shared identifier common to all requests 4993 * that were authorized more or less simultaneously by a single author, 4994 * representing the identifier of the requisition, prescription or 4995 * similar form.) 4996 */ 4997 public Identifier getGroupIdentifier() { 4998 if (this.groupIdentifier == null) 4999 if (Configuration.errorOnAutoCreate()) 5000 throw new Error("Attempt to auto-create RequestGroup.groupIdentifier"); 5001 else if (Configuration.doAutoCreate()) 5002 this.groupIdentifier = new Identifier(); // cc 5003 return this.groupIdentifier; 5004 } 5005 5006 public boolean hasGroupIdentifier() { 5007 return this.groupIdentifier != null && !this.groupIdentifier.isEmpty(); 5008 } 5009 5010 /** 5011 * @param value {@link #groupIdentifier} (A shared identifier common to all 5012 * requests that were authorized more or less simultaneously by a 5013 * single author, representing the identifier of the requisition, 5014 * prescription or similar form.) 5015 */ 5016 public RequestGroup setGroupIdentifier(Identifier value) { 5017 this.groupIdentifier = value; 5018 return this; 5019 } 5020 5021 /** 5022 * @return {@link #status} (The current state of the request. For request 5023 * groups, the status reflects the status of all the requests in the 5024 * group.). This is the underlying object with id, value and extensions. 5025 * The accessor "getStatus" gives direct access to the value 5026 */ 5027 public Enumeration<RequestStatus> getStatusElement() { 5028 if (this.status == null) 5029 if (Configuration.errorOnAutoCreate()) 5030 throw new Error("Attempt to auto-create RequestGroup.status"); 5031 else if (Configuration.doAutoCreate()) 5032 this.status = new Enumeration<RequestStatus>(new RequestStatusEnumFactory()); // bb 5033 return this.status; 5034 } 5035 5036 public boolean hasStatusElement() { 5037 return this.status != null && !this.status.isEmpty(); 5038 } 5039 5040 public boolean hasStatus() { 5041 return this.status != null && !this.status.isEmpty(); 5042 } 5043 5044 /** 5045 * @param value {@link #status} (The current state of the request. For request 5046 * groups, the status reflects the status of all the requests in 5047 * the group.). This is the underlying object with id, value and 5048 * extensions. The accessor "getStatus" gives direct access to the 5049 * value 5050 */ 5051 public RequestGroup setStatusElement(Enumeration<RequestStatus> value) { 5052 this.status = value; 5053 return this; 5054 } 5055 5056 /** 5057 * @return The current state of the request. For request groups, the status 5058 * reflects the status of all the requests in the group. 5059 */ 5060 public RequestStatus getStatus() { 5061 return this.status == null ? null : this.status.getValue(); 5062 } 5063 5064 /** 5065 * @param value The current state of the request. For request groups, the status 5066 * reflects the status of all the requests in the group. 5067 */ 5068 public RequestGroup setStatus(RequestStatus value) { 5069 if (this.status == null) 5070 this.status = new Enumeration<RequestStatus>(new RequestStatusEnumFactory()); 5071 this.status.setValue(value); 5072 return this; 5073 } 5074 5075 /** 5076 * @return {@link #intent} (Indicates the level of authority/intentionality 5077 * associated with the request and where the request fits into the 5078 * workflow chain.). This is the underlying object with id, value and 5079 * extensions. The accessor "getIntent" gives direct access to the value 5080 */ 5081 public Enumeration<RequestIntent> getIntentElement() { 5082 if (this.intent == null) 5083 if (Configuration.errorOnAutoCreate()) 5084 throw new Error("Attempt to auto-create RequestGroup.intent"); 5085 else if (Configuration.doAutoCreate()) 5086 this.intent = new Enumeration<RequestIntent>(new RequestIntentEnumFactory()); // bb 5087 return this.intent; 5088 } 5089 5090 public boolean hasIntentElement() { 5091 return this.intent != null && !this.intent.isEmpty(); 5092 } 5093 5094 public boolean hasIntent() { 5095 return this.intent != null && !this.intent.isEmpty(); 5096 } 5097 5098 /** 5099 * @param value {@link #intent} (Indicates the level of authority/intentionality 5100 * associated with the request and where the request fits into the 5101 * workflow chain.). This is the underlying object with id, value 5102 * and extensions. The accessor "getIntent" gives direct access to 5103 * the value 5104 */ 5105 public RequestGroup setIntentElement(Enumeration<RequestIntent> value) { 5106 this.intent = value; 5107 return this; 5108 } 5109 5110 /** 5111 * @return Indicates the level of authority/intentionality associated with the 5112 * request and where the request fits into the workflow chain. 5113 */ 5114 public RequestIntent getIntent() { 5115 return this.intent == null ? null : this.intent.getValue(); 5116 } 5117 5118 /** 5119 * @param value Indicates the level of authority/intentionality associated with 5120 * the request and where the request fits into the workflow chain. 5121 */ 5122 public RequestGroup setIntent(RequestIntent value) { 5123 if (this.intent == null) 5124 this.intent = new Enumeration<RequestIntent>(new RequestIntentEnumFactory()); 5125 this.intent.setValue(value); 5126 return this; 5127 } 5128 5129 /** 5130 * @return {@link #priority} (Indicates how quickly the request should be 5131 * addressed with respect to other requests.). This is the underlying 5132 * object with id, value and extensions. The accessor "getPriority" 5133 * gives direct access to the value 5134 */ 5135 public Enumeration<RequestPriority> getPriorityElement() { 5136 if (this.priority == null) 5137 if (Configuration.errorOnAutoCreate()) 5138 throw new Error("Attempt to auto-create RequestGroup.priority"); 5139 else if (Configuration.doAutoCreate()) 5140 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 5141 return this.priority; 5142 } 5143 5144 public boolean hasPriorityElement() { 5145 return this.priority != null && !this.priority.isEmpty(); 5146 } 5147 5148 public boolean hasPriority() { 5149 return this.priority != null && !this.priority.isEmpty(); 5150 } 5151 5152 /** 5153 * @param value {@link #priority} (Indicates how quickly the request should be 5154 * addressed with respect to other requests.). This is the 5155 * underlying object with id, value and extensions. The accessor 5156 * "getPriority" gives direct access to the value 5157 */ 5158 public RequestGroup setPriorityElement(Enumeration<RequestPriority> value) { 5159 this.priority = value; 5160 return this; 5161 } 5162 5163 /** 5164 * @return Indicates how quickly the request should be addressed with respect to 5165 * other requests. 5166 */ 5167 public RequestPriority getPriority() { 5168 return this.priority == null ? null : this.priority.getValue(); 5169 } 5170 5171 /** 5172 * @param value Indicates how quickly the request should be addressed with 5173 * respect to other requests. 5174 */ 5175 public RequestGroup setPriority(RequestPriority value) { 5176 if (value == null) 5177 this.priority = null; 5178 else { 5179 if (this.priority == null) 5180 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 5181 this.priority.setValue(value); 5182 } 5183 return this; 5184 } 5185 5186 /** 5187 * @return {@link #code} (A code that identifies what the overall request group 5188 * is.) 5189 */ 5190 public CodeableConcept getCode() { 5191 if (this.code == null) 5192 if (Configuration.errorOnAutoCreate()) 5193 throw new Error("Attempt to auto-create RequestGroup.code"); 5194 else if (Configuration.doAutoCreate()) 5195 this.code = new CodeableConcept(); // cc 5196 return this.code; 5197 } 5198 5199 public boolean hasCode() { 5200 return this.code != null && !this.code.isEmpty(); 5201 } 5202 5203 /** 5204 * @param value {@link #code} (A code that identifies what the overall request 5205 * group is.) 5206 */ 5207 public RequestGroup setCode(CodeableConcept value) { 5208 this.code = value; 5209 return this; 5210 } 5211 5212 /** 5213 * @return {@link #subject} (The subject for which the request group was 5214 * created.) 5215 */ 5216 public Reference getSubject() { 5217 if (this.subject == null) 5218 if (Configuration.errorOnAutoCreate()) 5219 throw new Error("Attempt to auto-create RequestGroup.subject"); 5220 else if (Configuration.doAutoCreate()) 5221 this.subject = new Reference(); // cc 5222 return this.subject; 5223 } 5224 5225 public boolean hasSubject() { 5226 return this.subject != null && !this.subject.isEmpty(); 5227 } 5228 5229 /** 5230 * @param value {@link #subject} (The subject for which the request group was 5231 * created.) 5232 */ 5233 public RequestGroup setSubject(Reference value) { 5234 this.subject = value; 5235 return this; 5236 } 5237 5238 /** 5239 * @return {@link #subject} The actual object that is the target of the 5240 * reference. The reference library doesn't populate this, but you can 5241 * use it to hold the resource if you resolve it. (The subject for which 5242 * the request group was created.) 5243 */ 5244 public Resource getSubjectTarget() { 5245 return this.subjectTarget; 5246 } 5247 5248 /** 5249 * @param value {@link #subject} The actual object that is the target of the 5250 * reference. The reference library doesn't use these, but you can 5251 * use it to hold the resource if you resolve it. (The subject for 5252 * which the request group was created.) 5253 */ 5254 public RequestGroup setSubjectTarget(Resource value) { 5255 this.subjectTarget = value; 5256 return this; 5257 } 5258 5259 /** 5260 * @return {@link #encounter} (Describes the context of the request group, if 5261 * any.) 5262 */ 5263 public Reference getEncounter() { 5264 if (this.encounter == null) 5265 if (Configuration.errorOnAutoCreate()) 5266 throw new Error("Attempt to auto-create RequestGroup.encounter"); 5267 else if (Configuration.doAutoCreate()) 5268 this.encounter = new Reference(); // cc 5269 return this.encounter; 5270 } 5271 5272 public boolean hasEncounter() { 5273 return this.encounter != null && !this.encounter.isEmpty(); 5274 } 5275 5276 /** 5277 * @param value {@link #encounter} (Describes the context of the request group, 5278 * if any.) 5279 */ 5280 public RequestGroup setEncounter(Reference value) { 5281 this.encounter = value; 5282 return this; 5283 } 5284 5285 /** 5286 * @return {@link #encounter} The actual object that is the target of the 5287 * reference. The reference library doesn't populate this, but you can 5288 * use it to hold the resource if you resolve it. (Describes the context 5289 * of the request group, if any.) 5290 */ 5291 public Encounter getEncounterTarget() { 5292 if (this.encounterTarget == null) 5293 if (Configuration.errorOnAutoCreate()) 5294 throw new Error("Attempt to auto-create RequestGroup.encounter"); 5295 else if (Configuration.doAutoCreate()) 5296 this.encounterTarget = new Encounter(); // aa 5297 return this.encounterTarget; 5298 } 5299 5300 /** 5301 * @param value {@link #encounter} The actual object that is the target of the 5302 * reference. The reference library doesn't use these, but you can 5303 * use it to hold the resource if you resolve it. (Describes the 5304 * context of the request group, if any.) 5305 */ 5306 public RequestGroup setEncounterTarget(Encounter value) { 5307 this.encounterTarget = value; 5308 return this; 5309 } 5310 5311 /** 5312 * @return {@link #authoredOn} (Indicates when the request group was created.). 5313 * This is the underlying object with id, value and extensions. The 5314 * accessor "getAuthoredOn" gives direct access to the value 5315 */ 5316 public DateTimeType getAuthoredOnElement() { 5317 if (this.authoredOn == null) 5318 if (Configuration.errorOnAutoCreate()) 5319 throw new Error("Attempt to auto-create RequestGroup.authoredOn"); 5320 else if (Configuration.doAutoCreate()) 5321 this.authoredOn = new DateTimeType(); // bb 5322 return this.authoredOn; 5323 } 5324 5325 public boolean hasAuthoredOnElement() { 5326 return this.authoredOn != null && !this.authoredOn.isEmpty(); 5327 } 5328 5329 public boolean hasAuthoredOn() { 5330 return this.authoredOn != null && !this.authoredOn.isEmpty(); 5331 } 5332 5333 /** 5334 * @param value {@link #authoredOn} (Indicates when the request group was 5335 * created.). This is the underlying object with id, value and 5336 * extensions. The accessor "getAuthoredOn" gives direct access to 5337 * the value 5338 */ 5339 public RequestGroup setAuthoredOnElement(DateTimeType value) { 5340 this.authoredOn = value; 5341 return this; 5342 } 5343 5344 /** 5345 * @return Indicates when the request group was created. 5346 */ 5347 public Date getAuthoredOn() { 5348 return this.authoredOn == null ? null : this.authoredOn.getValue(); 5349 } 5350 5351 /** 5352 * @param value Indicates when the request group was created. 5353 */ 5354 public RequestGroup setAuthoredOn(Date value) { 5355 if (value == null) 5356 this.authoredOn = null; 5357 else { 5358 if (this.authoredOn == null) 5359 this.authoredOn = new DateTimeType(); 5360 this.authoredOn.setValue(value); 5361 } 5362 return this; 5363 } 5364 5365 /** 5366 * @return {@link #author} (Provides a reference to the author of the request 5367 * group.) 5368 */ 5369 public Reference getAuthor() { 5370 if (this.author == null) 5371 if (Configuration.errorOnAutoCreate()) 5372 throw new Error("Attempt to auto-create RequestGroup.author"); 5373 else if (Configuration.doAutoCreate()) 5374 this.author = new Reference(); // cc 5375 return this.author; 5376 } 5377 5378 public boolean hasAuthor() { 5379 return this.author != null && !this.author.isEmpty(); 5380 } 5381 5382 /** 5383 * @param value {@link #author} (Provides a reference to the author of the 5384 * request group.) 5385 */ 5386 public RequestGroup setAuthor(Reference value) { 5387 this.author = value; 5388 return this; 5389 } 5390 5391 /** 5392 * @return {@link #author} The actual object that is the target of the 5393 * reference. The reference library doesn't populate this, but you can 5394 * use it to hold the resource if you resolve it. (Provides a reference 5395 * to the author of the request group.) 5396 */ 5397 public Resource getAuthorTarget() { 5398 return this.authorTarget; 5399 } 5400 5401 /** 5402 * @param value {@link #author} The actual object that is the target of the 5403 * reference. The reference library doesn't use these, but you can 5404 * use it to hold the resource if you resolve it. (Provides a 5405 * reference to the author of the request group.) 5406 */ 5407 public RequestGroup setAuthorTarget(Resource value) { 5408 this.authorTarget = value; 5409 return this; 5410 } 5411 5412 /** 5413 * @return {@link #reasonCode} (Describes the reason for the request group in 5414 * coded or textual form.) 5415 */ 5416 public List<CodeableConcept> getReasonCode() { 5417 if (this.reasonCode == null) 5418 this.reasonCode = new ArrayList<CodeableConcept>(); 5419 return this.reasonCode; 5420 } 5421 5422 /** 5423 * @return Returns a reference to <code>this</code> for easy method chaining 5424 */ 5425 public RequestGroup setReasonCode(List<CodeableConcept> theReasonCode) { 5426 this.reasonCode = theReasonCode; 5427 return this; 5428 } 5429 5430 public boolean hasReasonCode() { 5431 if (this.reasonCode == null) 5432 return false; 5433 for (CodeableConcept item : this.reasonCode) 5434 if (!item.isEmpty()) 5435 return true; 5436 return false; 5437 } 5438 5439 public CodeableConcept addReasonCode() { // 3 5440 CodeableConcept t = new CodeableConcept(); 5441 if (this.reasonCode == null) 5442 this.reasonCode = new ArrayList<CodeableConcept>(); 5443 this.reasonCode.add(t); 5444 return t; 5445 } 5446 5447 public RequestGroup addReasonCode(CodeableConcept t) { // 3 5448 if (t == null) 5449 return this; 5450 if (this.reasonCode == null) 5451 this.reasonCode = new ArrayList<CodeableConcept>(); 5452 this.reasonCode.add(t); 5453 return this; 5454 } 5455 5456 /** 5457 * @return The first repetition of repeating field {@link #reasonCode}, creating 5458 * it if it does not already exist 5459 */ 5460 public CodeableConcept getReasonCodeFirstRep() { 5461 if (getReasonCode().isEmpty()) { 5462 addReasonCode(); 5463 } 5464 return getReasonCode().get(0); 5465 } 5466 5467 /** 5468 * @return {@link #reasonReference} (Indicates another resource whose existence 5469 * justifies this request group.) 5470 */ 5471 public List<Reference> getReasonReference() { 5472 if (this.reasonReference == null) 5473 this.reasonReference = new ArrayList<Reference>(); 5474 return this.reasonReference; 5475 } 5476 5477 /** 5478 * @return Returns a reference to <code>this</code> for easy method chaining 5479 */ 5480 public RequestGroup setReasonReference(List<Reference> theReasonReference) { 5481 this.reasonReference = theReasonReference; 5482 return this; 5483 } 5484 5485 public boolean hasReasonReference() { 5486 if (this.reasonReference == null) 5487 return false; 5488 for (Reference item : this.reasonReference) 5489 if (!item.isEmpty()) 5490 return true; 5491 return false; 5492 } 5493 5494 public Reference addReasonReference() { // 3 5495 Reference t = new Reference(); 5496 if (this.reasonReference == null) 5497 this.reasonReference = new ArrayList<Reference>(); 5498 this.reasonReference.add(t); 5499 return t; 5500 } 5501 5502 public RequestGroup addReasonReference(Reference t) { // 3 5503 if (t == null) 5504 return this; 5505 if (this.reasonReference == null) 5506 this.reasonReference = new ArrayList<Reference>(); 5507 this.reasonReference.add(t); 5508 return this; 5509 } 5510 5511 /** 5512 * @return The first repetition of repeating field {@link #reasonReference}, 5513 * creating it if it does not already exist 5514 */ 5515 public Reference getReasonReferenceFirstRep() { 5516 if (getReasonReference().isEmpty()) { 5517 addReasonReference(); 5518 } 5519 return getReasonReference().get(0); 5520 } 5521 5522 /** 5523 * @deprecated Use Reference#setResource(IBaseResource) instead 5524 */ 5525 @Deprecated 5526 public List<Resource> getReasonReferenceTarget() { 5527 if (this.reasonReferenceTarget == null) 5528 this.reasonReferenceTarget = new ArrayList<Resource>(); 5529 return this.reasonReferenceTarget; 5530 } 5531 5532 /** 5533 * @return {@link #note} (Provides a mechanism to communicate additional 5534 * information about the response.) 5535 */ 5536 public List<Annotation> getNote() { 5537 if (this.note == null) 5538 this.note = new ArrayList<Annotation>(); 5539 return this.note; 5540 } 5541 5542 /** 5543 * @return Returns a reference to <code>this</code> for easy method chaining 5544 */ 5545 public RequestGroup setNote(List<Annotation> theNote) { 5546 this.note = theNote; 5547 return this; 5548 } 5549 5550 public boolean hasNote() { 5551 if (this.note == null) 5552 return false; 5553 for (Annotation item : this.note) 5554 if (!item.isEmpty()) 5555 return true; 5556 return false; 5557 } 5558 5559 public Annotation addNote() { // 3 5560 Annotation t = new Annotation(); 5561 if (this.note == null) 5562 this.note = new ArrayList<Annotation>(); 5563 this.note.add(t); 5564 return t; 5565 } 5566 5567 public RequestGroup addNote(Annotation t) { // 3 5568 if (t == null) 5569 return this; 5570 if (this.note == null) 5571 this.note = new ArrayList<Annotation>(); 5572 this.note.add(t); 5573 return this; 5574 } 5575 5576 /** 5577 * @return The first repetition of repeating field {@link #note}, creating it if 5578 * it does not already exist 5579 */ 5580 public Annotation getNoteFirstRep() { 5581 if (getNote().isEmpty()) { 5582 addNote(); 5583 } 5584 return getNote().get(0); 5585 } 5586 5587 /** 5588 * @return {@link #action} (The actions, if any, produced by the evaluation of 5589 * the artifact.) 5590 */ 5591 public List<RequestGroupActionComponent> getAction() { 5592 if (this.action == null) 5593 this.action = new ArrayList<RequestGroupActionComponent>(); 5594 return this.action; 5595 } 5596 5597 /** 5598 * @return Returns a reference to <code>this</code> for easy method chaining 5599 */ 5600 public RequestGroup setAction(List<RequestGroupActionComponent> theAction) { 5601 this.action = theAction; 5602 return this; 5603 } 5604 5605 public boolean hasAction() { 5606 if (this.action == null) 5607 return false; 5608 for (RequestGroupActionComponent item : this.action) 5609 if (!item.isEmpty()) 5610 return true; 5611 return false; 5612 } 5613 5614 public RequestGroupActionComponent addAction() { // 3 5615 RequestGroupActionComponent t = new RequestGroupActionComponent(); 5616 if (this.action == null) 5617 this.action = new ArrayList<RequestGroupActionComponent>(); 5618 this.action.add(t); 5619 return t; 5620 } 5621 5622 public RequestGroup addAction(RequestGroupActionComponent t) { // 3 5623 if (t == null) 5624 return this; 5625 if (this.action == null) 5626 this.action = new ArrayList<RequestGroupActionComponent>(); 5627 this.action.add(t); 5628 return this; 5629 } 5630 5631 /** 5632 * @return The first repetition of repeating field {@link #action}, creating it 5633 * if it does not already exist 5634 */ 5635 public RequestGroupActionComponent getActionFirstRep() { 5636 if (getAction().isEmpty()) { 5637 addAction(); 5638 } 5639 return getAction().get(0); 5640 } 5641 5642 protected void listChildren(List<Property> children) { 5643 super.listChildren(children); 5644 children.add(new Property("identifier", "Identifier", 5645 "Allows a service to provide a unique, business identifier for the request.", 0, java.lang.Integer.MAX_VALUE, 5646 identifier)); 5647 children.add(new Property("instantiatesCanonical", "canonical", 5648 "A canonical URL referencing a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.", 5649 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical)); 5650 children.add(new Property("instantiatesUri", "uri", 5651 "A URL referencing an externally defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.", 5652 0, java.lang.Integer.MAX_VALUE, instantiatesUri)); 5653 children.add(new Property("basedOn", "Reference(Any)", 5654 "A plan, proposal or order that is fulfilled in whole or in part by this request.", 0, 5655 java.lang.Integer.MAX_VALUE, basedOn)); 5656 children.add(new Property("replaces", "Reference(Any)", 5657 "Completed or terminated request(s) whose function is taken by this new request.", 0, 5658 java.lang.Integer.MAX_VALUE, replaces)); 5659 children.add(new Property("groupIdentifier", "Identifier", 5660 "A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.", 5661 0, 1, groupIdentifier)); 5662 children.add(new Property("status", "code", 5663 "The current state of the request. For request groups, the status reflects the status of all the requests in the group.", 5664 0, 1, status)); 5665 children.add(new Property("intent", "code", 5666 "Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain.", 5667 0, 1, intent)); 5668 children.add(new Property("priority", "code", 5669 "Indicates how quickly the request should be addressed with respect to other requests.", 0, 1, priority)); 5670 children.add(new Property("code", "CodeableConcept", "A code that identifies what the overall request group is.", 0, 5671 1, code)); 5672 children.add(new Property("subject", "Reference(Patient|Group)", 5673 "The subject for which the request group was created.", 0, 1, subject)); 5674 children.add(new Property("encounter", "Reference(Encounter)", 5675 "Describes the context of the request group, if any.", 0, 1, encounter)); 5676 children 5677 .add(new Property("authoredOn", "dateTime", "Indicates when the request group was created.", 0, 1, authoredOn)); 5678 children.add(new Property("author", "Reference(Device|Practitioner|PractitionerRole)", 5679 "Provides a reference to the author of the request group.", 0, 1, author)); 5680 children.add(new Property("reasonCode", "CodeableConcept", 5681 "Describes the reason for the request group in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, 5682 reasonCode)); 5683 children.add(new Property("reasonReference", "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 5684 "Indicates another resource whose existence justifies this request group.", 0, java.lang.Integer.MAX_VALUE, 5685 reasonReference)); 5686 children.add(new Property("note", "Annotation", 5687 "Provides a mechanism to communicate additional information about the response.", 0, 5688 java.lang.Integer.MAX_VALUE, note)); 5689 children.add(new Property("action", "", "The actions, if any, produced by the evaluation of the artifact.", 0, 5690 java.lang.Integer.MAX_VALUE, action)); 5691 } 5692 5693 @Override 5694 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5695 switch (_hash) { 5696 case -1618432855: 5697 /* identifier */ return new Property("identifier", "Identifier", 5698 "Allows a service to provide a unique, business identifier for the request.", 0, java.lang.Integer.MAX_VALUE, 5699 identifier); 5700 case 8911915: 5701 /* instantiatesCanonical */ return new Property("instantiatesCanonical", "canonical", 5702 "A canonical URL referencing a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.", 5703 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical); 5704 case -1926393373: 5705 /* instantiatesUri */ return new Property("instantiatesUri", "uri", 5706 "A URL referencing an externally defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.", 5707 0, java.lang.Integer.MAX_VALUE, instantiatesUri); 5708 case -332612366: 5709 /* basedOn */ return new Property("basedOn", "Reference(Any)", 5710 "A plan, proposal or order that is fulfilled in whole or in part by this request.", 0, 5711 java.lang.Integer.MAX_VALUE, basedOn); 5712 case -430332865: 5713 /* replaces */ return new Property("replaces", "Reference(Any)", 5714 "Completed or terminated request(s) whose function is taken by this new request.", 0, 5715 java.lang.Integer.MAX_VALUE, replaces); 5716 case -445338488: 5717 /* groupIdentifier */ return new Property("groupIdentifier", "Identifier", 5718 "A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.", 5719 0, 1, groupIdentifier); 5720 case -892481550: 5721 /* status */ return new Property("status", "code", 5722 "The current state of the request. For request groups, the status reflects the status of all the requests in the group.", 5723 0, 1, status); 5724 case -1183762788: 5725 /* intent */ return new Property("intent", "code", 5726 "Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain.", 5727 0, 1, intent); 5728 case -1165461084: 5729 /* priority */ return new Property("priority", "code", 5730 "Indicates how quickly the request should be addressed with respect to other requests.", 0, 1, priority); 5731 case 3059181: 5732 /* code */ return new Property("code", "CodeableConcept", 5733 "A code that identifies what the overall request group is.", 0, 1, code); 5734 case -1867885268: 5735 /* subject */ return new Property("subject", "Reference(Patient|Group)", 5736 "The subject for which the request group was created.", 0, 1, subject); 5737 case 1524132147: 5738 /* encounter */ return new Property("encounter", "Reference(Encounter)", 5739 "Describes the context of the request group, if any.", 0, 1, encounter); 5740 case -1500852503: 5741 /* authoredOn */ return new Property("authoredOn", "dateTime", "Indicates when the request group was created.", 0, 5742 1, authoredOn); 5743 case -1406328437: 5744 /* author */ return new Property("author", "Reference(Device|Practitioner|PractitionerRole)", 5745 "Provides a reference to the author of the request group.", 0, 1, author); 5746 case 722137681: 5747 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 5748 "Describes the reason for the request group in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, 5749 reasonCode); 5750 case -1146218137: 5751 /* reasonReference */ return new Property("reasonReference", 5752 "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 5753 "Indicates another resource whose existence justifies this request group.", 0, java.lang.Integer.MAX_VALUE, 5754 reasonReference); 5755 case 3387378: 5756 /* note */ return new Property("note", "Annotation", 5757 "Provides a mechanism to communicate additional information about the response.", 0, 5758 java.lang.Integer.MAX_VALUE, note); 5759 case -1422950858: 5760 /* action */ return new Property("action", "", "The actions, if any, produced by the evaluation of the artifact.", 5761 0, java.lang.Integer.MAX_VALUE, action); 5762 default: 5763 return super.getNamedProperty(_hash, _name, _checkValid); 5764 } 5765 5766 } 5767 5768 @Override 5769 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5770 switch (hash) { 5771 case -1618432855: 5772 /* identifier */ return this.identifier == null ? new Base[0] 5773 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 5774 case 8911915: 5775 /* instantiatesCanonical */ return this.instantiatesCanonical == null ? new Base[0] 5776 : this.instantiatesCanonical.toArray(new Base[this.instantiatesCanonical.size()]); // CanonicalType 5777 case -1926393373: 5778 /* instantiatesUri */ return this.instantiatesUri == null ? new Base[0] 5779 : this.instantiatesUri.toArray(new Base[this.instantiatesUri.size()]); // UriType 5780 case -332612366: 5781 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 5782 case -430332865: 5783 /* replaces */ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // Reference 5784 case -445338488: 5785 /* groupIdentifier */ return this.groupIdentifier == null ? new Base[0] : new Base[] { this.groupIdentifier }; // Identifier 5786 case -892481550: 5787 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<RequestStatus> 5788 case -1183762788: 5789 /* intent */ return this.intent == null ? new Base[0] : new Base[] { this.intent }; // Enumeration<RequestIntent> 5790 case -1165461084: 5791 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // Enumeration<RequestPriority> 5792 case 3059181: 5793 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 5794 case -1867885268: 5795 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 5796 case 1524132147: 5797 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 5798 case -1500852503: 5799 /* authoredOn */ return this.authoredOn == null ? new Base[0] : new Base[] { this.authoredOn }; // DateTimeType 5800 case -1406328437: 5801 /* author */ return this.author == null ? new Base[0] : new Base[] { this.author }; // Reference 5802 case 722137681: 5803 /* reasonCode */ return this.reasonCode == null ? new Base[0] 5804 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 5805 case -1146218137: 5806 /* reasonReference */ return this.reasonReference == null ? new Base[0] 5807 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 5808 case 3387378: 5809 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 5810 case -1422950858: 5811 /* action */ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // RequestGroupActionComponent 5812 default: 5813 return super.getProperty(hash, name, checkValid); 5814 } 5815 5816 } 5817 5818 @Override 5819 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5820 switch (hash) { 5821 case -1618432855: // identifier 5822 this.getIdentifier().add(castToIdentifier(value)); // Identifier 5823 return value; 5824 case 8911915: // instantiatesCanonical 5825 this.getInstantiatesCanonical().add(castToCanonical(value)); // CanonicalType 5826 return value; 5827 case -1926393373: // instantiatesUri 5828 this.getInstantiatesUri().add(castToUri(value)); // UriType 5829 return value; 5830 case -332612366: // basedOn 5831 this.getBasedOn().add(castToReference(value)); // Reference 5832 return value; 5833 case -430332865: // replaces 5834 this.getReplaces().add(castToReference(value)); // Reference 5835 return value; 5836 case -445338488: // groupIdentifier 5837 this.groupIdentifier = castToIdentifier(value); // Identifier 5838 return value; 5839 case -892481550: // status 5840 value = new RequestStatusEnumFactory().fromType(castToCode(value)); 5841 this.status = (Enumeration) value; // Enumeration<RequestStatus> 5842 return value; 5843 case -1183762788: // intent 5844 value = new RequestIntentEnumFactory().fromType(castToCode(value)); 5845 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 5846 return value; 5847 case -1165461084: // priority 5848 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 5849 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 5850 return value; 5851 case 3059181: // code 5852 this.code = castToCodeableConcept(value); // CodeableConcept 5853 return value; 5854 case -1867885268: // subject 5855 this.subject = castToReference(value); // Reference 5856 return value; 5857 case 1524132147: // encounter 5858 this.encounter = castToReference(value); // Reference 5859 return value; 5860 case -1500852503: // authoredOn 5861 this.authoredOn = castToDateTime(value); // DateTimeType 5862 return value; 5863 case -1406328437: // author 5864 this.author = castToReference(value); // Reference 5865 return value; 5866 case 722137681: // reasonCode 5867 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 5868 return value; 5869 case -1146218137: // reasonReference 5870 this.getReasonReference().add(castToReference(value)); // Reference 5871 return value; 5872 case 3387378: // note 5873 this.getNote().add(castToAnnotation(value)); // Annotation 5874 return value; 5875 case -1422950858: // action 5876 this.getAction().add((RequestGroupActionComponent) value); // RequestGroupActionComponent 5877 return value; 5878 default: 5879 return super.setProperty(hash, name, value); 5880 } 5881 5882 } 5883 5884 @Override 5885 public Base setProperty(String name, Base value) throws FHIRException { 5886 if (name.equals("identifier")) { 5887 this.getIdentifier().add(castToIdentifier(value)); 5888 } else if (name.equals("instantiatesCanonical")) { 5889 this.getInstantiatesCanonical().add(castToCanonical(value)); 5890 } else if (name.equals("instantiatesUri")) { 5891 this.getInstantiatesUri().add(castToUri(value)); 5892 } else if (name.equals("basedOn")) { 5893 this.getBasedOn().add(castToReference(value)); 5894 } else if (name.equals("replaces")) { 5895 this.getReplaces().add(castToReference(value)); 5896 } else if (name.equals("groupIdentifier")) { 5897 this.groupIdentifier = castToIdentifier(value); // Identifier 5898 } else if (name.equals("status")) { 5899 value = new RequestStatusEnumFactory().fromType(castToCode(value)); 5900 this.status = (Enumeration) value; // Enumeration<RequestStatus> 5901 } else if (name.equals("intent")) { 5902 value = new RequestIntentEnumFactory().fromType(castToCode(value)); 5903 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 5904 } else if (name.equals("priority")) { 5905 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 5906 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 5907 } else if (name.equals("code")) { 5908 this.code = castToCodeableConcept(value); // CodeableConcept 5909 } else if (name.equals("subject")) { 5910 this.subject = castToReference(value); // Reference 5911 } else if (name.equals("encounter")) { 5912 this.encounter = castToReference(value); // Reference 5913 } else if (name.equals("authoredOn")) { 5914 this.authoredOn = castToDateTime(value); // DateTimeType 5915 } else if (name.equals("author")) { 5916 this.author = castToReference(value); // Reference 5917 } else if (name.equals("reasonCode")) { 5918 this.getReasonCode().add(castToCodeableConcept(value)); 5919 } else if (name.equals("reasonReference")) { 5920 this.getReasonReference().add(castToReference(value)); 5921 } else if (name.equals("note")) { 5922 this.getNote().add(castToAnnotation(value)); 5923 } else if (name.equals("action")) { 5924 this.getAction().add((RequestGroupActionComponent) value); 5925 } else 5926 return super.setProperty(name, value); 5927 return value; 5928 } 5929 5930 @Override 5931 public void removeChild(String name, Base value) throws FHIRException { 5932 if (name.equals("identifier")) { 5933 this.getIdentifier().remove(castToIdentifier(value)); 5934 } else if (name.equals("instantiatesCanonical")) { 5935 this.getInstantiatesCanonical().remove(castToCanonical(value)); 5936 } else if (name.equals("instantiatesUri")) { 5937 this.getInstantiatesUri().remove(castToUri(value)); 5938 } else if (name.equals("basedOn")) { 5939 this.getBasedOn().remove(castToReference(value)); 5940 } else if (name.equals("replaces")) { 5941 this.getReplaces().remove(castToReference(value)); 5942 } else if (name.equals("groupIdentifier")) { 5943 this.groupIdentifier = null; 5944 } else if (name.equals("status")) { 5945 this.status = null; 5946 } else if (name.equals("intent")) { 5947 this.intent = null; 5948 } else if (name.equals("priority")) { 5949 this.priority = null; 5950 } else if (name.equals("code")) { 5951 this.code = null; 5952 } else if (name.equals("subject")) { 5953 this.subject = null; 5954 } else if (name.equals("encounter")) { 5955 this.encounter = null; 5956 } else if (name.equals("authoredOn")) { 5957 this.authoredOn = null; 5958 } else if (name.equals("author")) { 5959 this.author = null; 5960 } else if (name.equals("reasonCode")) { 5961 this.getReasonCode().remove(castToCodeableConcept(value)); 5962 } else if (name.equals("reasonReference")) { 5963 this.getReasonReference().remove(castToReference(value)); 5964 } else if (name.equals("note")) { 5965 this.getNote().remove(castToAnnotation(value)); 5966 } else if (name.equals("action")) { 5967 this.getAction().remove((RequestGroupActionComponent) value); 5968 } else 5969 super.removeChild(name, value); 5970 5971 } 5972 5973 @Override 5974 public Base makeProperty(int hash, String name) throws FHIRException { 5975 switch (hash) { 5976 case -1618432855: 5977 return addIdentifier(); 5978 case 8911915: 5979 return addInstantiatesCanonicalElement(); 5980 case -1926393373: 5981 return addInstantiatesUriElement(); 5982 case -332612366: 5983 return addBasedOn(); 5984 case -430332865: 5985 return addReplaces(); 5986 case -445338488: 5987 return getGroupIdentifier(); 5988 case -892481550: 5989 return getStatusElement(); 5990 case -1183762788: 5991 return getIntentElement(); 5992 case -1165461084: 5993 return getPriorityElement(); 5994 case 3059181: 5995 return getCode(); 5996 case -1867885268: 5997 return getSubject(); 5998 case 1524132147: 5999 return getEncounter(); 6000 case -1500852503: 6001 return getAuthoredOnElement(); 6002 case -1406328437: 6003 return getAuthor(); 6004 case 722137681: 6005 return addReasonCode(); 6006 case -1146218137: 6007 return addReasonReference(); 6008 case 3387378: 6009 return addNote(); 6010 case -1422950858: 6011 return addAction(); 6012 default: 6013 return super.makeProperty(hash, name); 6014 } 6015 6016 } 6017 6018 @Override 6019 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6020 switch (hash) { 6021 case -1618432855: 6022 /* identifier */ return new String[] { "Identifier" }; 6023 case 8911915: 6024 /* instantiatesCanonical */ return new String[] { "canonical" }; 6025 case -1926393373: 6026 /* instantiatesUri */ return new String[] { "uri" }; 6027 case -332612366: 6028 /* basedOn */ return new String[] { "Reference" }; 6029 case -430332865: 6030 /* replaces */ return new String[] { "Reference" }; 6031 case -445338488: 6032 /* groupIdentifier */ return new String[] { "Identifier" }; 6033 case -892481550: 6034 /* status */ return new String[] { "code" }; 6035 case -1183762788: 6036 /* intent */ return new String[] { "code" }; 6037 case -1165461084: 6038 /* priority */ return new String[] { "code" }; 6039 case 3059181: 6040 /* code */ return new String[] { "CodeableConcept" }; 6041 case -1867885268: 6042 /* subject */ return new String[] { "Reference" }; 6043 case 1524132147: 6044 /* encounter */ return new String[] { "Reference" }; 6045 case -1500852503: 6046 /* authoredOn */ return new String[] { "dateTime" }; 6047 case -1406328437: 6048 /* author */ return new String[] { "Reference" }; 6049 case 722137681: 6050 /* reasonCode */ return new String[] { "CodeableConcept" }; 6051 case -1146218137: 6052 /* reasonReference */ return new String[] { "Reference" }; 6053 case 3387378: 6054 /* note */ return new String[] { "Annotation" }; 6055 case -1422950858: 6056 /* action */ return new String[] {}; 6057 default: 6058 return super.getTypesForProperty(hash, name); 6059 } 6060 6061 } 6062 6063 @Override 6064 public Base addChild(String name) throws FHIRException { 6065 if (name.equals("identifier")) { 6066 return addIdentifier(); 6067 } else if (name.equals("instantiatesCanonical")) { 6068 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.instantiatesCanonical"); 6069 } else if (name.equals("instantiatesUri")) { 6070 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.instantiatesUri"); 6071 } else if (name.equals("basedOn")) { 6072 return addBasedOn(); 6073 } else if (name.equals("replaces")) { 6074 return addReplaces(); 6075 } else if (name.equals("groupIdentifier")) { 6076 this.groupIdentifier = new Identifier(); 6077 return this.groupIdentifier; 6078 } else if (name.equals("status")) { 6079 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.status"); 6080 } else if (name.equals("intent")) { 6081 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.intent"); 6082 } else if (name.equals("priority")) { 6083 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.priority"); 6084 } else if (name.equals("code")) { 6085 this.code = new CodeableConcept(); 6086 return this.code; 6087 } else if (name.equals("subject")) { 6088 this.subject = new Reference(); 6089 return this.subject; 6090 } else if (name.equals("encounter")) { 6091 this.encounter = new Reference(); 6092 return this.encounter; 6093 } else if (name.equals("authoredOn")) { 6094 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.authoredOn"); 6095 } else if (name.equals("author")) { 6096 this.author = new Reference(); 6097 return this.author; 6098 } else if (name.equals("reasonCode")) { 6099 return addReasonCode(); 6100 } else if (name.equals("reasonReference")) { 6101 return addReasonReference(); 6102 } else if (name.equals("note")) { 6103 return addNote(); 6104 } else if (name.equals("action")) { 6105 return addAction(); 6106 } else 6107 return super.addChild(name); 6108 } 6109 6110 public String fhirType() { 6111 return "RequestGroup"; 6112 6113 } 6114 6115 public RequestGroup copy() { 6116 RequestGroup dst = new RequestGroup(); 6117 copyValues(dst); 6118 return dst; 6119 } 6120 6121 public void copyValues(RequestGroup dst) { 6122 super.copyValues(dst); 6123 if (identifier != null) { 6124 dst.identifier = new ArrayList<Identifier>(); 6125 for (Identifier i : identifier) 6126 dst.identifier.add(i.copy()); 6127 } 6128 ; 6129 if (instantiatesCanonical != null) { 6130 dst.instantiatesCanonical = new ArrayList<CanonicalType>(); 6131 for (CanonicalType i : instantiatesCanonical) 6132 dst.instantiatesCanonical.add(i.copy()); 6133 } 6134 ; 6135 if (instantiatesUri != null) { 6136 dst.instantiatesUri = new ArrayList<UriType>(); 6137 for (UriType i : instantiatesUri) 6138 dst.instantiatesUri.add(i.copy()); 6139 } 6140 ; 6141 if (basedOn != null) { 6142 dst.basedOn = new ArrayList<Reference>(); 6143 for (Reference i : basedOn) 6144 dst.basedOn.add(i.copy()); 6145 } 6146 ; 6147 if (replaces != null) { 6148 dst.replaces = new ArrayList<Reference>(); 6149 for (Reference i : replaces) 6150 dst.replaces.add(i.copy()); 6151 } 6152 ; 6153 dst.groupIdentifier = groupIdentifier == null ? null : groupIdentifier.copy(); 6154 dst.status = status == null ? null : status.copy(); 6155 dst.intent = intent == null ? null : intent.copy(); 6156 dst.priority = priority == null ? null : priority.copy(); 6157 dst.code = code == null ? null : code.copy(); 6158 dst.subject = subject == null ? null : subject.copy(); 6159 dst.encounter = encounter == null ? null : encounter.copy(); 6160 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 6161 dst.author = author == null ? null : author.copy(); 6162 if (reasonCode != null) { 6163 dst.reasonCode = new ArrayList<CodeableConcept>(); 6164 for (CodeableConcept i : reasonCode) 6165 dst.reasonCode.add(i.copy()); 6166 } 6167 ; 6168 if (reasonReference != null) { 6169 dst.reasonReference = new ArrayList<Reference>(); 6170 for (Reference i : reasonReference) 6171 dst.reasonReference.add(i.copy()); 6172 } 6173 ; 6174 if (note != null) { 6175 dst.note = new ArrayList<Annotation>(); 6176 for (Annotation i : note) 6177 dst.note.add(i.copy()); 6178 } 6179 ; 6180 if (action != null) { 6181 dst.action = new ArrayList<RequestGroupActionComponent>(); 6182 for (RequestGroupActionComponent i : action) 6183 dst.action.add(i.copy()); 6184 } 6185 ; 6186 } 6187 6188 protected RequestGroup typedCopy() { 6189 return copy(); 6190 } 6191 6192 @Override 6193 public boolean equalsDeep(Base other_) { 6194 if (!super.equalsDeep(other_)) 6195 return false; 6196 if (!(other_ instanceof RequestGroup)) 6197 return false; 6198 RequestGroup o = (RequestGroup) other_; 6199 return compareDeep(identifier, o.identifier, true) 6200 && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 6201 && compareDeep(instantiatesUri, o.instantiatesUri, true) && compareDeep(basedOn, o.basedOn, true) 6202 && compareDeep(replaces, o.replaces, true) && compareDeep(groupIdentifier, o.groupIdentifier, true) 6203 && compareDeep(status, o.status, true) && compareDeep(intent, o.intent, true) 6204 && compareDeep(priority, o.priority, true) && compareDeep(code, o.code, true) 6205 && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) 6206 && compareDeep(authoredOn, o.authoredOn, true) && compareDeep(author, o.author, true) 6207 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 6208 && compareDeep(note, o.note, true) && compareDeep(action, o.action, true); 6209 } 6210 6211 @Override 6212 public boolean equalsShallow(Base other_) { 6213 if (!super.equalsShallow(other_)) 6214 return false; 6215 if (!(other_ instanceof RequestGroup)) 6216 return false; 6217 RequestGroup o = (RequestGroup) other_; 6218 return compareValues(instantiatesCanonical, o.instantiatesCanonical, true) 6219 && compareValues(instantiatesUri, o.instantiatesUri, true) && compareValues(status, o.status, true) 6220 && compareValues(intent, o.intent, true) && compareValues(priority, o.priority, true) 6221 && compareValues(authoredOn, o.authoredOn, true); 6222 } 6223 6224 public boolean isEmpty() { 6225 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, instantiatesCanonical, instantiatesUri, 6226 basedOn, replaces, groupIdentifier, status, intent, priority, code, subject, encounter, authoredOn, author, 6227 reasonCode, reasonReference, note, action); 6228 } 6229 6230 @Override 6231 public ResourceType getResourceType() { 6232 return ResourceType.RequestGroup; 6233 } 6234 6235 /** 6236 * Search parameter: <b>authored</b> 6237 * <p> 6238 * Description: <b>The date the request group was authored</b><br> 6239 * Type: <b>date</b><br> 6240 * Path: <b>RequestGroup.authoredOn</b><br> 6241 * </p> 6242 */ 6243 @SearchParamDefinition(name = "authored", path = "RequestGroup.authoredOn", description = "The date the request group was authored", type = "date") 6244 public static final String SP_AUTHORED = "authored"; 6245 /** 6246 * <b>Fluent Client</b> search parameter constant for <b>authored</b> 6247 * <p> 6248 * Description: <b>The date the request group was authored</b><br> 6249 * Type: <b>date</b><br> 6250 * Path: <b>RequestGroup.authoredOn</b><br> 6251 * </p> 6252 */ 6253 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORED = new ca.uhn.fhir.rest.gclient.DateClientParam( 6254 SP_AUTHORED); 6255 6256 /** 6257 * Search parameter: <b>identifier</b> 6258 * <p> 6259 * Description: <b>External identifiers for the request group</b><br> 6260 * Type: <b>token</b><br> 6261 * Path: <b>RequestGroup.identifier</b><br> 6262 * </p> 6263 */ 6264 @SearchParamDefinition(name = "identifier", path = "RequestGroup.identifier", description = "External identifiers for the request group", type = "token") 6265 public static final String SP_IDENTIFIER = "identifier"; 6266 /** 6267 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 6268 * <p> 6269 * Description: <b>External identifiers for the request group</b><br> 6270 * Type: <b>token</b><br> 6271 * Path: <b>RequestGroup.identifier</b><br> 6272 * </p> 6273 */ 6274 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6275 SP_IDENTIFIER); 6276 6277 /** 6278 * Search parameter: <b>code</b> 6279 * <p> 6280 * Description: <b>The code of the request group</b><br> 6281 * Type: <b>token</b><br> 6282 * Path: <b>RequestGroup.code</b><br> 6283 * </p> 6284 */ 6285 @SearchParamDefinition(name = "code", path = "RequestGroup.code", description = "The code of the request group", type = "token") 6286 public static final String SP_CODE = "code"; 6287 /** 6288 * <b>Fluent Client</b> search parameter constant for <b>code</b> 6289 * <p> 6290 * Description: <b>The code of the request group</b><br> 6291 * Type: <b>token</b><br> 6292 * Path: <b>RequestGroup.code</b><br> 6293 * </p> 6294 */ 6295 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6296 SP_CODE); 6297 6298 /** 6299 * Search parameter: <b>subject</b> 6300 * <p> 6301 * Description: <b>The subject that the request group is about</b><br> 6302 * Type: <b>reference</b><br> 6303 * Path: <b>RequestGroup.subject</b><br> 6304 * </p> 6305 */ 6306 @SearchParamDefinition(name = "subject", path = "RequestGroup.subject", description = "The subject that the request group is about", type = "reference", providesMembershipIn = { 6307 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Group.class, Patient.class }) 6308 public static final String SP_SUBJECT = "subject"; 6309 /** 6310 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 6311 * <p> 6312 * Description: <b>The subject that the request group is about</b><br> 6313 * Type: <b>reference</b><br> 6314 * Path: <b>RequestGroup.subject</b><br> 6315 * </p> 6316 */ 6317 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6318 SP_SUBJECT); 6319 6320 /** 6321 * Constant for fluent queries to be used to add include statements. Specifies 6322 * the path value of "<b>RequestGroup:subject</b>". 6323 */ 6324 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 6325 "RequestGroup:subject").toLocked(); 6326 6327 /** 6328 * Search parameter: <b>author</b> 6329 * <p> 6330 * Description: <b>The author of the request group</b><br> 6331 * Type: <b>reference</b><br> 6332 * Path: <b>RequestGroup.author</b><br> 6333 * </p> 6334 */ 6335 @SearchParamDefinition(name = "author", path = "RequestGroup.author", description = "The author of the request group", type = "reference", providesMembershipIn = { 6336 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 6337 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Device.class, 6338 Practitioner.class, PractitionerRole.class }) 6339 public static final String SP_AUTHOR = "author"; 6340 /** 6341 * <b>Fluent Client</b> search parameter constant for <b>author</b> 6342 * <p> 6343 * Description: <b>The author of the request group</b><br> 6344 * Type: <b>reference</b><br> 6345 * Path: <b>RequestGroup.author</b><br> 6346 * </p> 6347 */ 6348 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6349 SP_AUTHOR); 6350 6351 /** 6352 * Constant for fluent queries to be used to add include statements. Specifies 6353 * the path value of "<b>RequestGroup:author</b>". 6354 */ 6355 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include( 6356 "RequestGroup:author").toLocked(); 6357 6358 /** 6359 * Search parameter: <b>instantiates-canonical</b> 6360 * <p> 6361 * Description: <b>The FHIR-based definition from which the request group is 6362 * realized</b><br> 6363 * Type: <b>reference</b><br> 6364 * Path: <b>RequestGroup.instantiatesCanonical</b><br> 6365 * </p> 6366 */ 6367 @SearchParamDefinition(name = "instantiates-canonical", path = "RequestGroup.instantiatesCanonical", description = "The FHIR-based definition from which the request group is realized", type = "reference") 6368 public static final String SP_INSTANTIATES_CANONICAL = "instantiates-canonical"; 6369 /** 6370 * <b>Fluent Client</b> search parameter constant for 6371 * <b>instantiates-canonical</b> 6372 * <p> 6373 * Description: <b>The FHIR-based definition from which the request group is 6374 * realized</b><br> 6375 * Type: <b>reference</b><br> 6376 * Path: <b>RequestGroup.instantiatesCanonical</b><br> 6377 * </p> 6378 */ 6379 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSTANTIATES_CANONICAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6380 SP_INSTANTIATES_CANONICAL); 6381 6382 /** 6383 * Constant for fluent queries to be used to add include statements. Specifies 6384 * the path value of "<b>RequestGroup:instantiates-canonical</b>". 6385 */ 6386 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSTANTIATES_CANONICAL = new ca.uhn.fhir.model.api.Include( 6387 "RequestGroup:instantiates-canonical").toLocked(); 6388 6389 /** 6390 * Search parameter: <b>encounter</b> 6391 * <p> 6392 * Description: <b>The encounter the request group applies to</b><br> 6393 * Type: <b>reference</b><br> 6394 * Path: <b>RequestGroup.encounter</b><br> 6395 * </p> 6396 */ 6397 @SearchParamDefinition(name = "encounter", path = "RequestGroup.encounter", description = "The encounter the request group applies to", type = "reference", providesMembershipIn = { 6398 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 6399 public static final String SP_ENCOUNTER = "encounter"; 6400 /** 6401 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 6402 * <p> 6403 * Description: <b>The encounter the request group applies to</b><br> 6404 * Type: <b>reference</b><br> 6405 * Path: <b>RequestGroup.encounter</b><br> 6406 * </p> 6407 */ 6408 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6409 SP_ENCOUNTER); 6410 6411 /** 6412 * Constant for fluent queries to be used to add include statements. Specifies 6413 * the path value of "<b>RequestGroup:encounter</b>". 6414 */ 6415 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 6416 "RequestGroup:encounter").toLocked(); 6417 6418 /** 6419 * Search parameter: <b>priority</b> 6420 * <p> 6421 * Description: <b>The priority of the request group</b><br> 6422 * Type: <b>token</b><br> 6423 * Path: <b>RequestGroup.priority</b><br> 6424 * </p> 6425 */ 6426 @SearchParamDefinition(name = "priority", path = "RequestGroup.priority", description = "The priority of the request group", type = "token") 6427 public static final String SP_PRIORITY = "priority"; 6428 /** 6429 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 6430 * <p> 6431 * Description: <b>The priority of the request group</b><br> 6432 * Type: <b>token</b><br> 6433 * Path: <b>RequestGroup.priority</b><br> 6434 * </p> 6435 */ 6436 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6437 SP_PRIORITY); 6438 6439 /** 6440 * Search parameter: <b>intent</b> 6441 * <p> 6442 * Description: <b>The intent of the request group</b><br> 6443 * Type: <b>token</b><br> 6444 * Path: <b>RequestGroup.intent</b><br> 6445 * </p> 6446 */ 6447 @SearchParamDefinition(name = "intent", path = "RequestGroup.intent", description = "The intent of the request group", type = "token") 6448 public static final String SP_INTENT = "intent"; 6449 /** 6450 * <b>Fluent Client</b> search parameter constant for <b>intent</b> 6451 * <p> 6452 * Description: <b>The intent of the request group</b><br> 6453 * Type: <b>token</b><br> 6454 * Path: <b>RequestGroup.intent</b><br> 6455 * </p> 6456 */ 6457 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6458 SP_INTENT); 6459 6460 /** 6461 * Search parameter: <b>participant</b> 6462 * <p> 6463 * Description: <b>The participant in the requests in the group</b><br> 6464 * Type: <b>reference</b><br> 6465 * Path: <b>RequestGroup.action.participant</b><br> 6466 * </p> 6467 */ 6468 @SearchParamDefinition(name = "participant", path = "RequestGroup.action.participant", description = "The participant in the requests in the group", type = "reference", providesMembershipIn = { 6469 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 6470 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 6471 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, Patient.class, 6472 Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 6473 public static final String SP_PARTICIPANT = "participant"; 6474 /** 6475 * <b>Fluent Client</b> search parameter constant for <b>participant</b> 6476 * <p> 6477 * Description: <b>The participant in the requests in the group</b><br> 6478 * Type: <b>reference</b><br> 6479 * Path: <b>RequestGroup.action.participant</b><br> 6480 * </p> 6481 */ 6482 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTICIPANT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6483 SP_PARTICIPANT); 6484 6485 /** 6486 * Constant for fluent queries to be used to add include statements. Specifies 6487 * the path value of "<b>RequestGroup:participant</b>". 6488 */ 6489 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTICIPANT = new ca.uhn.fhir.model.api.Include( 6490 "RequestGroup:participant").toLocked(); 6491 6492 /** 6493 * Search parameter: <b>group-identifier</b> 6494 * <p> 6495 * Description: <b>The group identifier for the request group</b><br> 6496 * Type: <b>token</b><br> 6497 * Path: <b>RequestGroup.groupIdentifier</b><br> 6498 * </p> 6499 */ 6500 @SearchParamDefinition(name = "group-identifier", path = "RequestGroup.groupIdentifier", description = "The group identifier for the request group", type = "token") 6501 public static final String SP_GROUP_IDENTIFIER = "group-identifier"; 6502 /** 6503 * <b>Fluent Client</b> search parameter constant for <b>group-identifier</b> 6504 * <p> 6505 * Description: <b>The group identifier for the request group</b><br> 6506 * Type: <b>token</b><br> 6507 * Path: <b>RequestGroup.groupIdentifier</b><br> 6508 * </p> 6509 */ 6510 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GROUP_IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6511 SP_GROUP_IDENTIFIER); 6512 6513 /** 6514 * Search parameter: <b>patient</b> 6515 * <p> 6516 * Description: <b>The identity of a patient to search for request 6517 * groups</b><br> 6518 * Type: <b>reference</b><br> 6519 * Path: <b>RequestGroup.subject</b><br> 6520 * </p> 6521 */ 6522 @SearchParamDefinition(name = "patient", path = "RequestGroup.subject.where(resolve() is Patient)", description = "The identity of a patient to search for request groups", type = "reference", target = { 6523 Patient.class }) 6524 public static final String SP_PATIENT = "patient"; 6525 /** 6526 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 6527 * <p> 6528 * Description: <b>The identity of a patient to search for request 6529 * groups</b><br> 6530 * Type: <b>reference</b><br> 6531 * Path: <b>RequestGroup.subject</b><br> 6532 * </p> 6533 */ 6534 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6535 SP_PATIENT); 6536 6537 /** 6538 * Constant for fluent queries to be used to add include statements. Specifies 6539 * the path value of "<b>RequestGroup:patient</b>". 6540 */ 6541 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 6542 "RequestGroup:patient").toLocked(); 6543 6544 /** 6545 * Search parameter: <b>instantiates-uri</b> 6546 * <p> 6547 * Description: <b>The external definition from which the request group is 6548 * realized</b><br> 6549 * Type: <b>uri</b><br> 6550 * Path: <b>RequestGroup.instantiatesUri</b><br> 6551 * </p> 6552 */ 6553 @SearchParamDefinition(name = "instantiates-uri", path = "RequestGroup.instantiatesUri", description = "The external definition from which the request group is realized", type = "uri") 6554 public static final String SP_INSTANTIATES_URI = "instantiates-uri"; 6555 /** 6556 * <b>Fluent Client</b> search parameter constant for <b>instantiates-uri</b> 6557 * <p> 6558 * Description: <b>The external definition from which the request group is 6559 * realized</b><br> 6560 * Type: <b>uri</b><br> 6561 * Path: <b>RequestGroup.instantiatesUri</b><br> 6562 * </p> 6563 */ 6564 public static final ca.uhn.fhir.rest.gclient.UriClientParam INSTANTIATES_URI = new ca.uhn.fhir.rest.gclient.UriClientParam( 6565 SP_INSTANTIATES_URI); 6566 6567 /** 6568 * Search parameter: <b>status</b> 6569 * <p> 6570 * Description: <b>The status of the request group</b><br> 6571 * Type: <b>token</b><br> 6572 * Path: <b>RequestGroup.status</b><br> 6573 * </p> 6574 */ 6575 @SearchParamDefinition(name = "status", path = "RequestGroup.status", description = "The status of the request group", type = "token") 6576 public static final String SP_STATUS = "status"; 6577 /** 6578 * <b>Fluent Client</b> search parameter constant for <b>status</b> 6579 * <p> 6580 * Description: <b>The status of the request group</b><br> 6581 * Type: <b>token</b><br> 6582 * Path: <b>RequestGroup.status</b><br> 6583 * </p> 6584 */ 6585 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6586 SP_STATUS); 6587 6588}