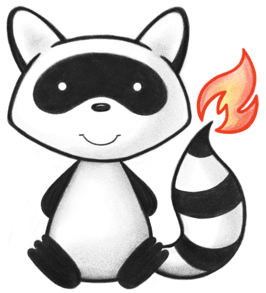
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047 048/** 049 * The ResearchDefinition resource describes the conditional state (population 050 * and any exposures being compared within the population) and outcome (if 051 * specified) that the knowledge (evidence, assertion, recommendation) is about. 052 */ 053@ResourceDef(name = "ResearchDefinition", profile = "http://hl7.org/fhir/StructureDefinition/ResearchDefinition") 054@ChildOrder(names = { "url", "identifier", "version", "name", "title", "shortTitle", "subtitle", "status", 055 "experimental", "subject[x]", "date", "publisher", "contact", "description", "comment", "useContext", 056 "jurisdiction", "purpose", "usage", "copyright", "approvalDate", "lastReviewDate", "effectivePeriod", "topic", 057 "author", "editor", "reviewer", "endorser", "relatedArtifact", "library", "population", "exposure", 058 "exposureAlternative", "outcome" }) 059public class ResearchDefinition extends MetadataResource { 060 061 /** 062 * A formal identifier that is used to identify this research definition when it 063 * is represented in other formats, or referenced in a specification, model, 064 * design or an instance. 065 */ 066 @Child(name = "identifier", type = { 067 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 068 @Description(shortDefinition = "Additional identifier for the research definition", formalDefinition = "A formal identifier that is used to identify this research definition when it is represented in other formats, or referenced in a specification, model, design or an instance.") 069 protected List<Identifier> identifier; 070 071 /** 072 * The short title provides an alternate title for use in informal descriptive 073 * contexts where the full, formal title is not necessary. 074 */ 075 @Child(name = "shortTitle", type = { 076 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 077 @Description(shortDefinition = "Title for use in informal contexts", formalDefinition = "The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary.") 078 protected StringType shortTitle; 079 080 /** 081 * An explanatory or alternate title for the ResearchDefinition giving 082 * additional information about its content. 083 */ 084 @Child(name = "subtitle", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 085 @Description(shortDefinition = "Subordinate title of the ResearchDefinition", formalDefinition = "An explanatory or alternate title for the ResearchDefinition giving additional information about its content.") 086 protected StringType subtitle; 087 088 /** 089 * The intended subjects for the ResearchDefinition. If this element is not 090 * provided, a Patient subject is assumed, but the subject of the 091 * ResearchDefinition can be anything. 092 */ 093 @Child(name = "subject", type = { CodeableConcept.class, 094 Group.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 095 @Description(shortDefinition = "E.g. Patient, Practitioner, RelatedPerson, Organization, Location, Device", formalDefinition = "The intended subjects for the ResearchDefinition. If this element is not provided, a Patient subject is assumed, but the subject of the ResearchDefinition can be anything.") 096 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/subject-type") 097 protected Type subject; 098 099 /** 100 * A human-readable string to clarify or explain concepts about the resource. 101 */ 102 @Child(name = "comment", type = { 103 StringType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 104 @Description(shortDefinition = "Used for footnotes or explanatory notes", formalDefinition = "A human-readable string to clarify or explain concepts about the resource.") 105 protected List<StringType> comment; 106 107 /** 108 * Explanation of why this research definition is needed and why it has been 109 * designed as it has. 110 */ 111 @Child(name = "purpose", type = { 112 MarkdownType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 113 @Description(shortDefinition = "Why this research definition is defined", formalDefinition = "Explanation of why this research definition is needed and why it has been designed as it has.") 114 protected MarkdownType purpose; 115 116 /** 117 * A detailed description, from a clinical perspective, of how the 118 * ResearchDefinition is used. 119 */ 120 @Child(name = "usage", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 121 @Description(shortDefinition = "Describes the clinical usage of the ResearchDefinition", formalDefinition = "A detailed description, from a clinical perspective, of how the ResearchDefinition is used.") 122 protected StringType usage; 123 124 /** 125 * A copyright statement relating to the research definition and/or its 126 * contents. Copyright statements are generally legal restrictions on the use 127 * and publishing of the research definition. 128 */ 129 @Child(name = "copyright", type = { 130 MarkdownType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 131 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the research definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the research definition.") 132 protected MarkdownType copyright; 133 134 /** 135 * The date on which the resource content was approved by the publisher. 136 * Approval happens once when the content is officially approved for usage. 137 */ 138 @Child(name = "approvalDate", type = { 139 DateType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 140 @Description(shortDefinition = "When the research definition was approved by publisher", formalDefinition = "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.") 141 protected DateType approvalDate; 142 143 /** 144 * The date on which the resource content was last reviewed. Review happens 145 * periodically after approval but does not change the original approval date. 146 */ 147 @Child(name = "lastReviewDate", type = { 148 DateType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 149 @Description(shortDefinition = "When the research definition was last reviewed", formalDefinition = "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.") 150 protected DateType lastReviewDate; 151 152 /** 153 * The period during which the research definition content was or is planned to 154 * be in active use. 155 */ 156 @Child(name = "effectivePeriod", type = { 157 Period.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 158 @Description(shortDefinition = "When the research definition is expected to be used", formalDefinition = "The period during which the research definition content was or is planned to be in active use.") 159 protected Period effectivePeriod; 160 161 /** 162 * Descriptive topics related to the content of the ResearchDefinition. Topics 163 * provide a high-level categorization grouping types of ResearchDefinitions 164 * that can be useful for filtering and searching. 165 */ 166 @Child(name = "topic", type = { 167 CodeableConcept.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 168 @Description(shortDefinition = "The category of the ResearchDefinition, such as Education, Treatment, Assessment, etc.", formalDefinition = "Descriptive topics related to the content of the ResearchDefinition. Topics provide a high-level categorization grouping types of ResearchDefinitions that can be useful for filtering and searching.") 169 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/definition-topic") 170 protected List<CodeableConcept> topic; 171 172 /** 173 * An individiual or organization primarily involved in the creation and 174 * maintenance of the content. 175 */ 176 @Child(name = "author", type = { 177 ContactDetail.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 178 @Description(shortDefinition = "Who authored the content", formalDefinition = "An individiual or organization primarily involved in the creation and maintenance of the content.") 179 protected List<ContactDetail> author; 180 181 /** 182 * An individual or organization primarily responsible for internal coherence of 183 * the content. 184 */ 185 @Child(name = "editor", type = { 186 ContactDetail.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 187 @Description(shortDefinition = "Who edited the content", formalDefinition = "An individual or organization primarily responsible for internal coherence of the content.") 188 protected List<ContactDetail> editor; 189 190 /** 191 * An individual or organization primarily responsible for review of some aspect 192 * of the content. 193 */ 194 @Child(name = "reviewer", type = { 195 ContactDetail.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 196 @Description(shortDefinition = "Who reviewed the content", formalDefinition = "An individual or organization primarily responsible for review of some aspect of the content.") 197 protected List<ContactDetail> reviewer; 198 199 /** 200 * An individual or organization responsible for officially endorsing the 201 * content for use in some setting. 202 */ 203 @Child(name = "endorser", type = { 204 ContactDetail.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 205 @Description(shortDefinition = "Who endorsed the content", formalDefinition = "An individual or organization responsible for officially endorsing the content for use in some setting.") 206 protected List<ContactDetail> endorser; 207 208 /** 209 * Related artifacts such as additional documentation, justification, or 210 * bibliographic references. 211 */ 212 @Child(name = "relatedArtifact", type = { 213 RelatedArtifact.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 214 @Description(shortDefinition = "Additional documentation, citations, etc.", formalDefinition = "Related artifacts such as additional documentation, justification, or bibliographic references.") 215 protected List<RelatedArtifact> relatedArtifact; 216 217 /** 218 * A reference to a Library resource containing the formal logic used by the 219 * ResearchDefinition. 220 */ 221 @Child(name = "library", type = { 222 CanonicalType.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 223 @Description(shortDefinition = "Logic used by the ResearchDefinition", formalDefinition = "A reference to a Library resource containing the formal logic used by the ResearchDefinition.") 224 protected List<CanonicalType> library; 225 226 /** 227 * A reference to a ResearchElementDefinition resource that defines the 228 * population for the research. 229 */ 230 @Child(name = "population", type = { 231 ResearchElementDefinition.class }, order = 18, min = 1, max = 1, modifier = false, summary = true) 232 @Description(shortDefinition = "What population?", formalDefinition = "A reference to a ResearchElementDefinition resource that defines the population for the research.") 233 protected Reference population; 234 235 /** 236 * The actual object that is the target of the reference (A reference to a 237 * ResearchElementDefinition resource that defines the population for the 238 * research.) 239 */ 240 protected ResearchElementDefinition populationTarget; 241 242 /** 243 * A reference to a ResearchElementDefinition resource that defines the exposure 244 * for the research. 245 */ 246 @Child(name = "exposure", type = { 247 ResearchElementDefinition.class }, order = 19, min = 0, max = 1, modifier = false, summary = true) 248 @Description(shortDefinition = "What exposure?", formalDefinition = "A reference to a ResearchElementDefinition resource that defines the exposure for the research.") 249 protected Reference exposure; 250 251 /** 252 * The actual object that is the target of the reference (A reference to a 253 * ResearchElementDefinition resource that defines the exposure for the 254 * research.) 255 */ 256 protected ResearchElementDefinition exposureTarget; 257 258 /** 259 * A reference to a ResearchElementDefinition resource that defines the 260 * exposureAlternative for the research. 261 */ 262 @Child(name = "exposureAlternative", type = { 263 ResearchElementDefinition.class }, order = 20, min = 0, max = 1, modifier = false, summary = true) 264 @Description(shortDefinition = "What alternative exposure state?", formalDefinition = "A reference to a ResearchElementDefinition resource that defines the exposureAlternative for the research.") 265 protected Reference exposureAlternative; 266 267 /** 268 * The actual object that is the target of the reference (A reference to a 269 * ResearchElementDefinition resource that defines the exposureAlternative for 270 * the research.) 271 */ 272 protected ResearchElementDefinition exposureAlternativeTarget; 273 274 /** 275 * A reference to a ResearchElementDefinition resomece that defines the outcome 276 * for the research. 277 */ 278 @Child(name = "outcome", type = { 279 ResearchElementDefinition.class }, order = 21, min = 0, max = 1, modifier = false, summary = true) 280 @Description(shortDefinition = "What outcome?", formalDefinition = "A reference to a ResearchElementDefinition resomece that defines the outcome for the research.") 281 protected Reference outcome; 282 283 /** 284 * The actual object that is the target of the reference (A reference to a 285 * ResearchElementDefinition resomece that defines the outcome for the 286 * research.) 287 */ 288 protected ResearchElementDefinition outcomeTarget; 289 290 private static final long serialVersionUID = -867649565L; 291 292 /** 293 * Constructor 294 */ 295 public ResearchDefinition() { 296 super(); 297 } 298 299 /** 300 * Constructor 301 */ 302 public ResearchDefinition(Enumeration<PublicationStatus> status, Reference population) { 303 super(); 304 this.status = status; 305 this.population = population; 306 } 307 308 /** 309 * @return {@link #url} (An absolute URI that is used to identify this research 310 * definition when it is referenced in a specification, model, design or 311 * an instance; also called its canonical identifier. This SHOULD be 312 * globally unique and SHOULD be a literal address at which at which an 313 * authoritative instance of this research definition is (or will be) 314 * published. This URL can be the target of a canonical reference. It 315 * SHALL remain the same when the research definition is stored on 316 * different servers.). This is the underlying object with id, value and 317 * extensions. The accessor "getUrl" gives direct access to the value 318 */ 319 public UriType getUrlElement() { 320 if (this.url == null) 321 if (Configuration.errorOnAutoCreate()) 322 throw new Error("Attempt to auto-create ResearchDefinition.url"); 323 else if (Configuration.doAutoCreate()) 324 this.url = new UriType(); // bb 325 return this.url; 326 } 327 328 public boolean hasUrlElement() { 329 return this.url != null && !this.url.isEmpty(); 330 } 331 332 public boolean hasUrl() { 333 return this.url != null && !this.url.isEmpty(); 334 } 335 336 /** 337 * @param value {@link #url} (An absolute URI that is used to identify this 338 * research definition when it is referenced in a specification, 339 * model, design or an instance; also called its canonical 340 * identifier. This SHOULD be globally unique and SHOULD be a 341 * literal address at which at which an authoritative instance of 342 * this research definition is (or will be) published. This URL can 343 * be the target of a canonical reference. It SHALL remain the same 344 * when the research definition is stored on different servers.). 345 * This is the underlying object with id, value and extensions. The 346 * accessor "getUrl" gives direct access to the value 347 */ 348 public ResearchDefinition setUrlElement(UriType value) { 349 this.url = value; 350 return this; 351 } 352 353 /** 354 * @return An absolute URI that is used to identify this research definition 355 * when it is referenced in a specification, model, design or an 356 * instance; also called its canonical identifier. This SHOULD be 357 * globally unique and SHOULD be a literal address at which at which an 358 * authoritative instance of this research definition is (or will be) 359 * published. This URL can be the target of a canonical reference. It 360 * SHALL remain the same when the research definition is stored on 361 * different servers. 362 */ 363 public String getUrl() { 364 return this.url == null ? null : this.url.getValue(); 365 } 366 367 /** 368 * @param value An absolute URI that is used to identify this research 369 * definition when it is referenced in a specification, model, 370 * design or an instance; also called its canonical identifier. 371 * This SHOULD be globally unique and SHOULD be a literal address 372 * at which at which an authoritative instance of this research 373 * definition is (or will be) published. This URL can be the target 374 * of a canonical reference. It SHALL remain the same when the 375 * research definition is stored on different servers. 376 */ 377 public ResearchDefinition setUrl(String value) { 378 if (Utilities.noString(value)) 379 this.url = null; 380 else { 381 if (this.url == null) 382 this.url = new UriType(); 383 this.url.setValue(value); 384 } 385 return this; 386 } 387 388 /** 389 * @return {@link #identifier} (A formal identifier that is used to identify 390 * this research definition when it is represented in other formats, or 391 * referenced in a specification, model, design or an instance.) 392 */ 393 public List<Identifier> getIdentifier() { 394 if (this.identifier == null) 395 this.identifier = new ArrayList<Identifier>(); 396 return this.identifier; 397 } 398 399 /** 400 * @return Returns a reference to <code>this</code> for easy method chaining 401 */ 402 public ResearchDefinition setIdentifier(List<Identifier> theIdentifier) { 403 this.identifier = theIdentifier; 404 return this; 405 } 406 407 public boolean hasIdentifier() { 408 if (this.identifier == null) 409 return false; 410 for (Identifier item : this.identifier) 411 if (!item.isEmpty()) 412 return true; 413 return false; 414 } 415 416 public Identifier addIdentifier() { // 3 417 Identifier t = new Identifier(); 418 if (this.identifier == null) 419 this.identifier = new ArrayList<Identifier>(); 420 this.identifier.add(t); 421 return t; 422 } 423 424 public ResearchDefinition addIdentifier(Identifier t) { // 3 425 if (t == null) 426 return this; 427 if (this.identifier == null) 428 this.identifier = new ArrayList<Identifier>(); 429 this.identifier.add(t); 430 return this; 431 } 432 433 /** 434 * @return The first repetition of repeating field {@link #identifier}, creating 435 * it if it does not already exist 436 */ 437 public Identifier getIdentifierFirstRep() { 438 if (getIdentifier().isEmpty()) { 439 addIdentifier(); 440 } 441 return getIdentifier().get(0); 442 } 443 444 /** 445 * @return {@link #version} (The identifier that is used to identify this 446 * version of the research definition when it is referenced in a 447 * specification, model, design or instance. This is an arbitrary value 448 * managed by the research definition author and is not expected to be 449 * globally unique. For example, it might be a timestamp (e.g. yyyymmdd) 450 * if a managed version is not available. There is also no expectation 451 * that versions can be placed in a lexicographical sequence. To provide 452 * a version consistent with the Decision Support Service specification, 453 * use the format Major.Minor.Revision (e.g. 1.0.0). For more 454 * information on versioning knowledge assets, refer to the Decision 455 * Support Service specification. Note that a version is required for 456 * non-experimental active artifacts.). This is the underlying object 457 * with id, value and extensions. The accessor "getVersion" gives direct 458 * access to the value 459 */ 460 public StringType getVersionElement() { 461 if (this.version == null) 462 if (Configuration.errorOnAutoCreate()) 463 throw new Error("Attempt to auto-create ResearchDefinition.version"); 464 else if (Configuration.doAutoCreate()) 465 this.version = new StringType(); // bb 466 return this.version; 467 } 468 469 public boolean hasVersionElement() { 470 return this.version != null && !this.version.isEmpty(); 471 } 472 473 public boolean hasVersion() { 474 return this.version != null && !this.version.isEmpty(); 475 } 476 477 /** 478 * @param value {@link #version} (The identifier that is used to identify this 479 * version of the research definition when it is referenced in a 480 * specification, model, design or instance. This is an arbitrary 481 * value managed by the research definition author and is not 482 * expected to be globally unique. For example, it might be a 483 * timestamp (e.g. yyyymmdd) if a managed version is not available. 484 * There is also no expectation that versions can be placed in a 485 * lexicographical sequence. To provide a version consistent with 486 * the Decision Support Service specification, use the format 487 * Major.Minor.Revision (e.g. 1.0.0). For more information on 488 * versioning knowledge assets, refer to the Decision Support 489 * Service specification. Note that a version is required for 490 * non-experimental active artifacts.). This is the underlying 491 * object with id, value and extensions. The accessor "getVersion" 492 * gives direct access to the value 493 */ 494 public ResearchDefinition setVersionElement(StringType value) { 495 this.version = value; 496 return this; 497 } 498 499 /** 500 * @return The identifier that is used to identify this version of the research 501 * definition when it is referenced in a specification, model, design or 502 * instance. This is an arbitrary value managed by the research 503 * definition author and is not expected to be globally unique. For 504 * example, it might be a timestamp (e.g. yyyymmdd) if a managed version 505 * is not available. There is also no expectation that versions can be 506 * placed in a lexicographical sequence. To provide a version consistent 507 * with the Decision Support Service specification, use the format 508 * Major.Minor.Revision (e.g. 1.0.0). For more information on versioning 509 * knowledge assets, refer to the Decision Support Service 510 * specification. Note that a version is required for non-experimental 511 * active artifacts. 512 */ 513 public String getVersion() { 514 return this.version == null ? null : this.version.getValue(); 515 } 516 517 /** 518 * @param value The identifier that is used to identify this version of the 519 * research definition when it is referenced in a specification, 520 * model, design or instance. This is an arbitrary value managed by 521 * the research definition author and is not expected to be 522 * globally unique. For example, it might be a timestamp (e.g. 523 * yyyymmdd) if a managed version is not available. There is also 524 * no expectation that versions can be placed in a lexicographical 525 * sequence. To provide a version consistent with the Decision 526 * Support Service specification, use the format 527 * Major.Minor.Revision (e.g. 1.0.0). For more information on 528 * versioning knowledge assets, refer to the Decision Support 529 * Service specification. Note that a version is required for 530 * non-experimental active artifacts. 531 */ 532 public ResearchDefinition setVersion(String value) { 533 if (Utilities.noString(value)) 534 this.version = null; 535 else { 536 if (this.version == null) 537 this.version = new StringType(); 538 this.version.setValue(value); 539 } 540 return this; 541 } 542 543 /** 544 * @return {@link #name} (A natural language name identifying the research 545 * definition. This name should be usable as an identifier for the 546 * module by machine processing applications such as code generation.). 547 * This is the underlying object with id, value and extensions. The 548 * accessor "getName" gives direct access to the value 549 */ 550 public StringType getNameElement() { 551 if (this.name == null) 552 if (Configuration.errorOnAutoCreate()) 553 throw new Error("Attempt to auto-create ResearchDefinition.name"); 554 else if (Configuration.doAutoCreate()) 555 this.name = new StringType(); // bb 556 return this.name; 557 } 558 559 public boolean hasNameElement() { 560 return this.name != null && !this.name.isEmpty(); 561 } 562 563 public boolean hasName() { 564 return this.name != null && !this.name.isEmpty(); 565 } 566 567 /** 568 * @param value {@link #name} (A natural language name identifying the research 569 * definition. This name should be usable as an identifier for the 570 * module by machine processing applications such as code 571 * generation.). This is the underlying object with id, value and 572 * extensions. The accessor "getName" gives direct access to the 573 * value 574 */ 575 public ResearchDefinition setNameElement(StringType value) { 576 this.name = value; 577 return this; 578 } 579 580 /** 581 * @return A natural language name identifying the research definition. This 582 * name should be usable as an identifier for the module by machine 583 * processing applications such as code generation. 584 */ 585 public String getName() { 586 return this.name == null ? null : this.name.getValue(); 587 } 588 589 /** 590 * @param value A natural language name identifying the research definition. 591 * This name should be usable as an identifier for the module by 592 * machine processing applications such as code generation. 593 */ 594 public ResearchDefinition setName(String value) { 595 if (Utilities.noString(value)) 596 this.name = null; 597 else { 598 if (this.name == null) 599 this.name = new StringType(); 600 this.name.setValue(value); 601 } 602 return this; 603 } 604 605 /** 606 * @return {@link #title} (A short, descriptive, user-friendly title for the 607 * research definition.). This is the underlying object with id, value 608 * and extensions. The accessor "getTitle" gives direct access to the 609 * value 610 */ 611 public StringType getTitleElement() { 612 if (this.title == null) 613 if (Configuration.errorOnAutoCreate()) 614 throw new Error("Attempt to auto-create ResearchDefinition.title"); 615 else if (Configuration.doAutoCreate()) 616 this.title = new StringType(); // bb 617 return this.title; 618 } 619 620 public boolean hasTitleElement() { 621 return this.title != null && !this.title.isEmpty(); 622 } 623 624 public boolean hasTitle() { 625 return this.title != null && !this.title.isEmpty(); 626 } 627 628 /** 629 * @param value {@link #title} (A short, descriptive, user-friendly title for 630 * the research definition.). This is the underlying object with 631 * id, value and extensions. The accessor "getTitle" gives direct 632 * access to the value 633 */ 634 public ResearchDefinition setTitleElement(StringType value) { 635 this.title = value; 636 return this; 637 } 638 639 /** 640 * @return A short, descriptive, user-friendly title for the research 641 * definition. 642 */ 643 public String getTitle() { 644 return this.title == null ? null : this.title.getValue(); 645 } 646 647 /** 648 * @param value A short, descriptive, user-friendly title for the research 649 * definition. 650 */ 651 public ResearchDefinition setTitle(String value) { 652 if (Utilities.noString(value)) 653 this.title = null; 654 else { 655 if (this.title == null) 656 this.title = new StringType(); 657 this.title.setValue(value); 658 } 659 return this; 660 } 661 662 /** 663 * @return {@link #shortTitle} (The short title provides an alternate title for 664 * use in informal descriptive contexts where the full, formal title is 665 * not necessary.). This is the underlying object with id, value and 666 * extensions. The accessor "getShortTitle" gives direct access to the 667 * value 668 */ 669 public StringType getShortTitleElement() { 670 if (this.shortTitle == null) 671 if (Configuration.errorOnAutoCreate()) 672 throw new Error("Attempt to auto-create ResearchDefinition.shortTitle"); 673 else if (Configuration.doAutoCreate()) 674 this.shortTitle = new StringType(); // bb 675 return this.shortTitle; 676 } 677 678 public boolean hasShortTitleElement() { 679 return this.shortTitle != null && !this.shortTitle.isEmpty(); 680 } 681 682 public boolean hasShortTitle() { 683 return this.shortTitle != null && !this.shortTitle.isEmpty(); 684 } 685 686 /** 687 * @param value {@link #shortTitle} (The short title provides an alternate title 688 * for use in informal descriptive contexts where the full, formal 689 * title is not necessary.). This is the underlying object with id, 690 * value and extensions. The accessor "getShortTitle" gives direct 691 * access to the value 692 */ 693 public ResearchDefinition setShortTitleElement(StringType value) { 694 this.shortTitle = value; 695 return this; 696 } 697 698 /** 699 * @return The short title provides an alternate title for use in informal 700 * descriptive contexts where the full, formal title is not necessary. 701 */ 702 public String getShortTitle() { 703 return this.shortTitle == null ? null : this.shortTitle.getValue(); 704 } 705 706 /** 707 * @param value The short title provides an alternate title for use in informal 708 * descriptive contexts where the full, formal title is not 709 * necessary. 710 */ 711 public ResearchDefinition setShortTitle(String value) { 712 if (Utilities.noString(value)) 713 this.shortTitle = null; 714 else { 715 if (this.shortTitle == null) 716 this.shortTitle = new StringType(); 717 this.shortTitle.setValue(value); 718 } 719 return this; 720 } 721 722 /** 723 * @return {@link #subtitle} (An explanatory or alternate title for the 724 * ResearchDefinition giving additional information about its content.). 725 * This is the underlying object with id, value and extensions. The 726 * accessor "getSubtitle" gives direct access to the value 727 */ 728 public StringType getSubtitleElement() { 729 if (this.subtitle == null) 730 if (Configuration.errorOnAutoCreate()) 731 throw new Error("Attempt to auto-create ResearchDefinition.subtitle"); 732 else if (Configuration.doAutoCreate()) 733 this.subtitle = new StringType(); // bb 734 return this.subtitle; 735 } 736 737 public boolean hasSubtitleElement() { 738 return this.subtitle != null && !this.subtitle.isEmpty(); 739 } 740 741 public boolean hasSubtitle() { 742 return this.subtitle != null && !this.subtitle.isEmpty(); 743 } 744 745 /** 746 * @param value {@link #subtitle} (An explanatory or alternate title for the 747 * ResearchDefinition giving additional information about its 748 * content.). This is the underlying object with id, value and 749 * extensions. The accessor "getSubtitle" gives direct access to 750 * the value 751 */ 752 public ResearchDefinition setSubtitleElement(StringType value) { 753 this.subtitle = value; 754 return this; 755 } 756 757 /** 758 * @return An explanatory or alternate title for the ResearchDefinition giving 759 * additional information about its content. 760 */ 761 public String getSubtitle() { 762 return this.subtitle == null ? null : this.subtitle.getValue(); 763 } 764 765 /** 766 * @param value An explanatory or alternate title for the ResearchDefinition 767 * giving additional information about its content. 768 */ 769 public ResearchDefinition setSubtitle(String value) { 770 if (Utilities.noString(value)) 771 this.subtitle = null; 772 else { 773 if (this.subtitle == null) 774 this.subtitle = new StringType(); 775 this.subtitle.setValue(value); 776 } 777 return this; 778 } 779 780 /** 781 * @return {@link #status} (The status of this research definition. Enables 782 * tracking the life-cycle of the content.). This is the underlying 783 * object with id, value and extensions. The accessor "getStatus" gives 784 * direct access to the value 785 */ 786 public Enumeration<PublicationStatus> getStatusElement() { 787 if (this.status == null) 788 if (Configuration.errorOnAutoCreate()) 789 throw new Error("Attempt to auto-create ResearchDefinition.status"); 790 else if (Configuration.doAutoCreate()) 791 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 792 return this.status; 793 } 794 795 public boolean hasStatusElement() { 796 return this.status != null && !this.status.isEmpty(); 797 } 798 799 public boolean hasStatus() { 800 return this.status != null && !this.status.isEmpty(); 801 } 802 803 /** 804 * @param value {@link #status} (The status of this research definition. Enables 805 * tracking the life-cycle of the content.). This is the underlying 806 * object with id, value and extensions. The accessor "getStatus" 807 * gives direct access to the value 808 */ 809 public ResearchDefinition setStatusElement(Enumeration<PublicationStatus> value) { 810 this.status = value; 811 return this; 812 } 813 814 /** 815 * @return The status of this research definition. Enables tracking the 816 * life-cycle of the content. 817 */ 818 public PublicationStatus getStatus() { 819 return this.status == null ? null : this.status.getValue(); 820 } 821 822 /** 823 * @param value The status of this research definition. Enables tracking the 824 * life-cycle of the content. 825 */ 826 public ResearchDefinition setStatus(PublicationStatus value) { 827 if (this.status == null) 828 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 829 this.status.setValue(value); 830 return this; 831 } 832 833 /** 834 * @return {@link #experimental} (A Boolean value to indicate that this research 835 * definition is authored for testing purposes (or 836 * education/evaluation/marketing) and is not intended to be used for 837 * genuine usage.). This is the underlying object with id, value and 838 * extensions. The accessor "getExperimental" gives direct access to the 839 * value 840 */ 841 public BooleanType getExperimentalElement() { 842 if (this.experimental == null) 843 if (Configuration.errorOnAutoCreate()) 844 throw new Error("Attempt to auto-create ResearchDefinition.experimental"); 845 else if (Configuration.doAutoCreate()) 846 this.experimental = new BooleanType(); // bb 847 return this.experimental; 848 } 849 850 public boolean hasExperimentalElement() { 851 return this.experimental != null && !this.experimental.isEmpty(); 852 } 853 854 public boolean hasExperimental() { 855 return this.experimental != null && !this.experimental.isEmpty(); 856 } 857 858 /** 859 * @param value {@link #experimental} (A Boolean value to indicate that this 860 * research definition is authored for testing purposes (or 861 * education/evaluation/marketing) and is not intended to be used 862 * for genuine usage.). This is the underlying object with id, 863 * value and extensions. The accessor "getExperimental" gives 864 * direct access to the value 865 */ 866 public ResearchDefinition setExperimentalElement(BooleanType value) { 867 this.experimental = value; 868 return this; 869 } 870 871 /** 872 * @return A Boolean value to indicate that this research definition is authored 873 * for testing purposes (or education/evaluation/marketing) and is not 874 * intended to be used for genuine usage. 875 */ 876 public boolean getExperimental() { 877 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 878 } 879 880 /** 881 * @param value A Boolean value to indicate that this research definition is 882 * authored for testing purposes (or 883 * education/evaluation/marketing) and is not intended to be used 884 * for genuine usage. 885 */ 886 public ResearchDefinition setExperimental(boolean value) { 887 if (this.experimental == null) 888 this.experimental = new BooleanType(); 889 this.experimental.setValue(value); 890 return this; 891 } 892 893 /** 894 * @return {@link #subject} (The intended subjects for the ResearchDefinition. 895 * If this element is not provided, a Patient subject is assumed, but 896 * the subject of the ResearchDefinition can be anything.) 897 */ 898 public Type getSubject() { 899 return this.subject; 900 } 901 902 /** 903 * @return {@link #subject} (The intended subjects for the ResearchDefinition. 904 * If this element is not provided, a Patient subject is assumed, but 905 * the subject of the ResearchDefinition can be anything.) 906 */ 907 public CodeableConcept getSubjectCodeableConcept() throws FHIRException { 908 if (this.subject == null) 909 this.subject = new CodeableConcept(); 910 if (!(this.subject instanceof CodeableConcept)) 911 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 912 + this.subject.getClass().getName() + " was encountered"); 913 return (CodeableConcept) this.subject; 914 } 915 916 public boolean hasSubjectCodeableConcept() { 917 return this.subject instanceof CodeableConcept; 918 } 919 920 /** 921 * @return {@link #subject} (The intended subjects for the ResearchDefinition. 922 * If this element is not provided, a Patient subject is assumed, but 923 * the subject of the ResearchDefinition can be anything.) 924 */ 925 public Reference getSubjectReference() throws FHIRException { 926 if (this.subject == null) 927 this.subject = new Reference(); 928 if (!(this.subject instanceof Reference)) 929 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.subject.getClass().getName() 930 + " was encountered"); 931 return (Reference) this.subject; 932 } 933 934 public boolean hasSubjectReference() { 935 return this.subject instanceof Reference; 936 } 937 938 public boolean hasSubject() { 939 return this.subject != null && !this.subject.isEmpty(); 940 } 941 942 /** 943 * @param value {@link #subject} (The intended subjects for the 944 * ResearchDefinition. If this element is not provided, a Patient 945 * subject is assumed, but the subject of the ResearchDefinition 946 * can be anything.) 947 */ 948 public ResearchDefinition setSubject(Type value) { 949 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 950 throw new Error("Not the right type for ResearchDefinition.subject[x]: " + value.fhirType()); 951 this.subject = value; 952 return this; 953 } 954 955 /** 956 * @return {@link #date} (The date (and optionally time) when the research 957 * definition was published. The date must change when the business 958 * version changes and it must change if the status code changes. In 959 * addition, it should change when the substantive content of the 960 * research definition changes.). This is the underlying object with id, 961 * value and extensions. The accessor "getDate" gives direct access to 962 * the value 963 */ 964 public DateTimeType getDateElement() { 965 if (this.date == null) 966 if (Configuration.errorOnAutoCreate()) 967 throw new Error("Attempt to auto-create ResearchDefinition.date"); 968 else if (Configuration.doAutoCreate()) 969 this.date = new DateTimeType(); // bb 970 return this.date; 971 } 972 973 public boolean hasDateElement() { 974 return this.date != null && !this.date.isEmpty(); 975 } 976 977 public boolean hasDate() { 978 return this.date != null && !this.date.isEmpty(); 979 } 980 981 /** 982 * @param value {@link #date} (The date (and optionally time) when the research 983 * definition was published. The date must change when the business 984 * version changes and it must change if the status code changes. 985 * In addition, it should change when the substantive content of 986 * the research definition changes.). This is the underlying object 987 * with id, value and extensions. The accessor "getDate" gives 988 * direct access to the value 989 */ 990 public ResearchDefinition setDateElement(DateTimeType value) { 991 this.date = value; 992 return this; 993 } 994 995 /** 996 * @return The date (and optionally time) when the research definition was 997 * published. The date must change when the business version changes and 998 * it must change if the status code changes. In addition, it should 999 * change when the substantive content of the research definition 1000 * changes. 1001 */ 1002 public Date getDate() { 1003 return this.date == null ? null : this.date.getValue(); 1004 } 1005 1006 /** 1007 * @param value The date (and optionally time) when the research definition was 1008 * published. The date must change when the business version 1009 * changes and it must change if the status code changes. In 1010 * addition, it should change when the substantive content of the 1011 * research definition changes. 1012 */ 1013 public ResearchDefinition setDate(Date value) { 1014 if (value == null) 1015 this.date = null; 1016 else { 1017 if (this.date == null) 1018 this.date = new DateTimeType(); 1019 this.date.setValue(value); 1020 } 1021 return this; 1022 } 1023 1024 /** 1025 * @return {@link #publisher} (The name of the organization or individual that 1026 * published the research definition.). This is the underlying object 1027 * with id, value and extensions. The accessor "getPublisher" gives 1028 * direct access to the value 1029 */ 1030 public StringType getPublisherElement() { 1031 if (this.publisher == null) 1032 if (Configuration.errorOnAutoCreate()) 1033 throw new Error("Attempt to auto-create ResearchDefinition.publisher"); 1034 else if (Configuration.doAutoCreate()) 1035 this.publisher = new StringType(); // bb 1036 return this.publisher; 1037 } 1038 1039 public boolean hasPublisherElement() { 1040 return this.publisher != null && !this.publisher.isEmpty(); 1041 } 1042 1043 public boolean hasPublisher() { 1044 return this.publisher != null && !this.publisher.isEmpty(); 1045 } 1046 1047 /** 1048 * @param value {@link #publisher} (The name of the organization or individual 1049 * that published the research definition.). This is the underlying 1050 * object with id, value and extensions. The accessor 1051 * "getPublisher" gives direct access to the value 1052 */ 1053 public ResearchDefinition setPublisherElement(StringType value) { 1054 this.publisher = value; 1055 return this; 1056 } 1057 1058 /** 1059 * @return The name of the organization or individual that published the 1060 * research definition. 1061 */ 1062 public String getPublisher() { 1063 return this.publisher == null ? null : this.publisher.getValue(); 1064 } 1065 1066 /** 1067 * @param value The name of the organization or individual that published the 1068 * research definition. 1069 */ 1070 public ResearchDefinition setPublisher(String value) { 1071 if (Utilities.noString(value)) 1072 this.publisher = null; 1073 else { 1074 if (this.publisher == null) 1075 this.publisher = new StringType(); 1076 this.publisher.setValue(value); 1077 } 1078 return this; 1079 } 1080 1081 /** 1082 * @return {@link #contact} (Contact details to assist a user in finding and 1083 * communicating with the publisher.) 1084 */ 1085 public List<ContactDetail> getContact() { 1086 if (this.contact == null) 1087 this.contact = new ArrayList<ContactDetail>(); 1088 return this.contact; 1089 } 1090 1091 /** 1092 * @return Returns a reference to <code>this</code> for easy method chaining 1093 */ 1094 public ResearchDefinition setContact(List<ContactDetail> theContact) { 1095 this.contact = theContact; 1096 return this; 1097 } 1098 1099 public boolean hasContact() { 1100 if (this.contact == null) 1101 return false; 1102 for (ContactDetail item : this.contact) 1103 if (!item.isEmpty()) 1104 return true; 1105 return false; 1106 } 1107 1108 public ContactDetail addContact() { // 3 1109 ContactDetail t = new ContactDetail(); 1110 if (this.contact == null) 1111 this.contact = new ArrayList<ContactDetail>(); 1112 this.contact.add(t); 1113 return t; 1114 } 1115 1116 public ResearchDefinition addContact(ContactDetail t) { // 3 1117 if (t == null) 1118 return this; 1119 if (this.contact == null) 1120 this.contact = new ArrayList<ContactDetail>(); 1121 this.contact.add(t); 1122 return this; 1123 } 1124 1125 /** 1126 * @return The first repetition of repeating field {@link #contact}, creating it 1127 * if it does not already exist 1128 */ 1129 public ContactDetail getContactFirstRep() { 1130 if (getContact().isEmpty()) { 1131 addContact(); 1132 } 1133 return getContact().get(0); 1134 } 1135 1136 /** 1137 * @return {@link #description} (A free text natural language description of the 1138 * research definition from a consumer's perspective.). This is the 1139 * underlying object with id, value and extensions. The accessor 1140 * "getDescription" gives direct access to the value 1141 */ 1142 public MarkdownType getDescriptionElement() { 1143 if (this.description == null) 1144 if (Configuration.errorOnAutoCreate()) 1145 throw new Error("Attempt to auto-create ResearchDefinition.description"); 1146 else if (Configuration.doAutoCreate()) 1147 this.description = new MarkdownType(); // bb 1148 return this.description; 1149 } 1150 1151 public boolean hasDescriptionElement() { 1152 return this.description != null && !this.description.isEmpty(); 1153 } 1154 1155 public boolean hasDescription() { 1156 return this.description != null && !this.description.isEmpty(); 1157 } 1158 1159 /** 1160 * @param value {@link #description} (A free text natural language description 1161 * of the research definition from a consumer's perspective.). This 1162 * is the underlying object with id, value and extensions. The 1163 * accessor "getDescription" gives direct access to the value 1164 */ 1165 public ResearchDefinition setDescriptionElement(MarkdownType value) { 1166 this.description = value; 1167 return this; 1168 } 1169 1170 /** 1171 * @return A free text natural language description of the research definition 1172 * from a consumer's perspective. 1173 */ 1174 public String getDescription() { 1175 return this.description == null ? null : this.description.getValue(); 1176 } 1177 1178 /** 1179 * @param value A free text natural language description of the research 1180 * definition from a consumer's perspective. 1181 */ 1182 public ResearchDefinition setDescription(String value) { 1183 if (value == null) 1184 this.description = null; 1185 else { 1186 if (this.description == null) 1187 this.description = new MarkdownType(); 1188 this.description.setValue(value); 1189 } 1190 return this; 1191 } 1192 1193 /** 1194 * @return {@link #comment} (A human-readable string to clarify or explain 1195 * concepts about the resource.) 1196 */ 1197 public List<StringType> getComment() { 1198 if (this.comment == null) 1199 this.comment = new ArrayList<StringType>(); 1200 return this.comment; 1201 } 1202 1203 /** 1204 * @return Returns a reference to <code>this</code> for easy method chaining 1205 */ 1206 public ResearchDefinition setComment(List<StringType> theComment) { 1207 this.comment = theComment; 1208 return this; 1209 } 1210 1211 public boolean hasComment() { 1212 if (this.comment == null) 1213 return false; 1214 for (StringType item : this.comment) 1215 if (!item.isEmpty()) 1216 return true; 1217 return false; 1218 } 1219 1220 /** 1221 * @return {@link #comment} (A human-readable string to clarify or explain 1222 * concepts about the resource.) 1223 */ 1224 public StringType addCommentElement() {// 2 1225 StringType t = new StringType(); 1226 if (this.comment == null) 1227 this.comment = new ArrayList<StringType>(); 1228 this.comment.add(t); 1229 return t; 1230 } 1231 1232 /** 1233 * @param value {@link #comment} (A human-readable string to clarify or explain 1234 * concepts about the resource.) 1235 */ 1236 public ResearchDefinition addComment(String value) { // 1 1237 StringType t = new StringType(); 1238 t.setValue(value); 1239 if (this.comment == null) 1240 this.comment = new ArrayList<StringType>(); 1241 this.comment.add(t); 1242 return this; 1243 } 1244 1245 /** 1246 * @param value {@link #comment} (A human-readable string to clarify or explain 1247 * concepts about the resource.) 1248 */ 1249 public boolean hasComment(String value) { 1250 if (this.comment == null) 1251 return false; 1252 for (StringType v : this.comment) 1253 if (v.getValue().equals(value)) // string 1254 return true; 1255 return false; 1256 } 1257 1258 /** 1259 * @return {@link #useContext} (The content was developed with a focus and 1260 * intent of supporting the contexts that are listed. These contexts may 1261 * be general categories (gender, age, ...) or may be references to 1262 * specific programs (insurance plans, studies, ...) and may be used to 1263 * assist with indexing and searching for appropriate research 1264 * definition instances.) 1265 */ 1266 public List<UsageContext> getUseContext() { 1267 if (this.useContext == null) 1268 this.useContext = new ArrayList<UsageContext>(); 1269 return this.useContext; 1270 } 1271 1272 /** 1273 * @return Returns a reference to <code>this</code> for easy method chaining 1274 */ 1275 public ResearchDefinition setUseContext(List<UsageContext> theUseContext) { 1276 this.useContext = theUseContext; 1277 return this; 1278 } 1279 1280 public boolean hasUseContext() { 1281 if (this.useContext == null) 1282 return false; 1283 for (UsageContext item : this.useContext) 1284 if (!item.isEmpty()) 1285 return true; 1286 return false; 1287 } 1288 1289 public UsageContext addUseContext() { // 3 1290 UsageContext t = new UsageContext(); 1291 if (this.useContext == null) 1292 this.useContext = new ArrayList<UsageContext>(); 1293 this.useContext.add(t); 1294 return t; 1295 } 1296 1297 public ResearchDefinition addUseContext(UsageContext t) { // 3 1298 if (t == null) 1299 return this; 1300 if (this.useContext == null) 1301 this.useContext = new ArrayList<UsageContext>(); 1302 this.useContext.add(t); 1303 return this; 1304 } 1305 1306 /** 1307 * @return The first repetition of repeating field {@link #useContext}, creating 1308 * it if it does not already exist 1309 */ 1310 public UsageContext getUseContextFirstRep() { 1311 if (getUseContext().isEmpty()) { 1312 addUseContext(); 1313 } 1314 return getUseContext().get(0); 1315 } 1316 1317 /** 1318 * @return {@link #jurisdiction} (A legal or geographic region in which the 1319 * research definition is intended to be used.) 1320 */ 1321 public List<CodeableConcept> getJurisdiction() { 1322 if (this.jurisdiction == null) 1323 this.jurisdiction = new ArrayList<CodeableConcept>(); 1324 return this.jurisdiction; 1325 } 1326 1327 /** 1328 * @return Returns a reference to <code>this</code> for easy method chaining 1329 */ 1330 public ResearchDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 1331 this.jurisdiction = theJurisdiction; 1332 return this; 1333 } 1334 1335 public boolean hasJurisdiction() { 1336 if (this.jurisdiction == null) 1337 return false; 1338 for (CodeableConcept item : this.jurisdiction) 1339 if (!item.isEmpty()) 1340 return true; 1341 return false; 1342 } 1343 1344 public CodeableConcept addJurisdiction() { // 3 1345 CodeableConcept t = new CodeableConcept(); 1346 if (this.jurisdiction == null) 1347 this.jurisdiction = new ArrayList<CodeableConcept>(); 1348 this.jurisdiction.add(t); 1349 return t; 1350 } 1351 1352 public ResearchDefinition addJurisdiction(CodeableConcept t) { // 3 1353 if (t == null) 1354 return this; 1355 if (this.jurisdiction == null) 1356 this.jurisdiction = new ArrayList<CodeableConcept>(); 1357 this.jurisdiction.add(t); 1358 return this; 1359 } 1360 1361 /** 1362 * @return The first repetition of repeating field {@link #jurisdiction}, 1363 * creating it if it does not already exist 1364 */ 1365 public CodeableConcept getJurisdictionFirstRep() { 1366 if (getJurisdiction().isEmpty()) { 1367 addJurisdiction(); 1368 } 1369 return getJurisdiction().get(0); 1370 } 1371 1372 /** 1373 * @return {@link #purpose} (Explanation of why this research definition is 1374 * needed and why it has been designed as it has.). This is the 1375 * underlying object with id, value and extensions. The accessor 1376 * "getPurpose" gives direct access to the value 1377 */ 1378 public MarkdownType getPurposeElement() { 1379 if (this.purpose == null) 1380 if (Configuration.errorOnAutoCreate()) 1381 throw new Error("Attempt to auto-create ResearchDefinition.purpose"); 1382 else if (Configuration.doAutoCreate()) 1383 this.purpose = new MarkdownType(); // bb 1384 return this.purpose; 1385 } 1386 1387 public boolean hasPurposeElement() { 1388 return this.purpose != null && !this.purpose.isEmpty(); 1389 } 1390 1391 public boolean hasPurpose() { 1392 return this.purpose != null && !this.purpose.isEmpty(); 1393 } 1394 1395 /** 1396 * @param value {@link #purpose} (Explanation of why this research definition is 1397 * needed and why it has been designed as it has.). This is the 1398 * underlying object with id, value and extensions. The accessor 1399 * "getPurpose" gives direct access to the value 1400 */ 1401 public ResearchDefinition setPurposeElement(MarkdownType value) { 1402 this.purpose = value; 1403 return this; 1404 } 1405 1406 /** 1407 * @return Explanation of why this research definition is needed and why it has 1408 * been designed as it has. 1409 */ 1410 public String getPurpose() { 1411 return this.purpose == null ? null : this.purpose.getValue(); 1412 } 1413 1414 /** 1415 * @param value Explanation of why this research definition is needed and why it 1416 * has been designed as it has. 1417 */ 1418 public ResearchDefinition setPurpose(String value) { 1419 if (value == null) 1420 this.purpose = null; 1421 else { 1422 if (this.purpose == null) 1423 this.purpose = new MarkdownType(); 1424 this.purpose.setValue(value); 1425 } 1426 return this; 1427 } 1428 1429 /** 1430 * @return {@link #usage} (A detailed description, from a clinical perspective, 1431 * of how the ResearchDefinition is used.). This is the underlying 1432 * object with id, value and extensions. The accessor "getUsage" gives 1433 * direct access to the value 1434 */ 1435 public StringType getUsageElement() { 1436 if (this.usage == null) 1437 if (Configuration.errorOnAutoCreate()) 1438 throw new Error("Attempt to auto-create ResearchDefinition.usage"); 1439 else if (Configuration.doAutoCreate()) 1440 this.usage = new StringType(); // bb 1441 return this.usage; 1442 } 1443 1444 public boolean hasUsageElement() { 1445 return this.usage != null && !this.usage.isEmpty(); 1446 } 1447 1448 public boolean hasUsage() { 1449 return this.usage != null && !this.usage.isEmpty(); 1450 } 1451 1452 /** 1453 * @param value {@link #usage} (A detailed description, from a clinical 1454 * perspective, of how the ResearchDefinition is used.). This is 1455 * the underlying object with id, value and extensions. The 1456 * accessor "getUsage" gives direct access to the value 1457 */ 1458 public ResearchDefinition setUsageElement(StringType value) { 1459 this.usage = value; 1460 return this; 1461 } 1462 1463 /** 1464 * @return A detailed description, from a clinical perspective, of how the 1465 * ResearchDefinition is used. 1466 */ 1467 public String getUsage() { 1468 return this.usage == null ? null : this.usage.getValue(); 1469 } 1470 1471 /** 1472 * @param value A detailed description, from a clinical perspective, of how the 1473 * ResearchDefinition is used. 1474 */ 1475 public ResearchDefinition setUsage(String value) { 1476 if (Utilities.noString(value)) 1477 this.usage = null; 1478 else { 1479 if (this.usage == null) 1480 this.usage = new StringType(); 1481 this.usage.setValue(value); 1482 } 1483 return this; 1484 } 1485 1486 /** 1487 * @return {@link #copyright} (A copyright statement relating to the research 1488 * definition and/or its contents. Copyright statements are generally 1489 * legal restrictions on the use and publishing of the research 1490 * definition.). This is the underlying object with id, value and 1491 * extensions. The accessor "getCopyright" gives direct access to the 1492 * value 1493 */ 1494 public MarkdownType getCopyrightElement() { 1495 if (this.copyright == null) 1496 if (Configuration.errorOnAutoCreate()) 1497 throw new Error("Attempt to auto-create ResearchDefinition.copyright"); 1498 else if (Configuration.doAutoCreate()) 1499 this.copyright = new MarkdownType(); // bb 1500 return this.copyright; 1501 } 1502 1503 public boolean hasCopyrightElement() { 1504 return this.copyright != null && !this.copyright.isEmpty(); 1505 } 1506 1507 public boolean hasCopyright() { 1508 return this.copyright != null && !this.copyright.isEmpty(); 1509 } 1510 1511 /** 1512 * @param value {@link #copyright} (A copyright statement relating to the 1513 * research definition and/or its contents. Copyright statements 1514 * are generally legal restrictions on the use and publishing of 1515 * the research definition.). This is the underlying object with 1516 * id, value and extensions. The accessor "getCopyright" gives 1517 * direct access to the value 1518 */ 1519 public ResearchDefinition setCopyrightElement(MarkdownType value) { 1520 this.copyright = value; 1521 return this; 1522 } 1523 1524 /** 1525 * @return A copyright statement relating to the research definition and/or its 1526 * contents. Copyright statements are generally legal restrictions on 1527 * the use and publishing of the research definition. 1528 */ 1529 public String getCopyright() { 1530 return this.copyright == null ? null : this.copyright.getValue(); 1531 } 1532 1533 /** 1534 * @param value A copyright statement relating to the research definition and/or 1535 * its contents. Copyright statements are generally legal 1536 * restrictions on the use and publishing of the research 1537 * definition. 1538 */ 1539 public ResearchDefinition setCopyright(String value) { 1540 if (value == null) 1541 this.copyright = null; 1542 else { 1543 if (this.copyright == null) 1544 this.copyright = new MarkdownType(); 1545 this.copyright.setValue(value); 1546 } 1547 return this; 1548 } 1549 1550 /** 1551 * @return {@link #approvalDate} (The date on which the resource content was 1552 * approved by the publisher. Approval happens once when the content is 1553 * officially approved for usage.). This is the underlying object with 1554 * id, value and extensions. The accessor "getApprovalDate" gives direct 1555 * access to the value 1556 */ 1557 public DateType getApprovalDateElement() { 1558 if (this.approvalDate == null) 1559 if (Configuration.errorOnAutoCreate()) 1560 throw new Error("Attempt to auto-create ResearchDefinition.approvalDate"); 1561 else if (Configuration.doAutoCreate()) 1562 this.approvalDate = new DateType(); // bb 1563 return this.approvalDate; 1564 } 1565 1566 public boolean hasApprovalDateElement() { 1567 return this.approvalDate != null && !this.approvalDate.isEmpty(); 1568 } 1569 1570 public boolean hasApprovalDate() { 1571 return this.approvalDate != null && !this.approvalDate.isEmpty(); 1572 } 1573 1574 /** 1575 * @param value {@link #approvalDate} (The date on which the resource content 1576 * was approved by the publisher. Approval happens once when the 1577 * content is officially approved for usage.). This is the 1578 * underlying object with id, value and extensions. The accessor 1579 * "getApprovalDate" gives direct access to the value 1580 */ 1581 public ResearchDefinition setApprovalDateElement(DateType value) { 1582 this.approvalDate = value; 1583 return this; 1584 } 1585 1586 /** 1587 * @return The date on which the resource content was approved by the publisher. 1588 * Approval happens once when the content is officially approved for 1589 * usage. 1590 */ 1591 public Date getApprovalDate() { 1592 return this.approvalDate == null ? null : this.approvalDate.getValue(); 1593 } 1594 1595 /** 1596 * @param value The date on which the resource content was approved by the 1597 * publisher. Approval happens once when the content is officially 1598 * approved for usage. 1599 */ 1600 public ResearchDefinition setApprovalDate(Date value) { 1601 if (value == null) 1602 this.approvalDate = null; 1603 else { 1604 if (this.approvalDate == null) 1605 this.approvalDate = new DateType(); 1606 this.approvalDate.setValue(value); 1607 } 1608 return this; 1609 } 1610 1611 /** 1612 * @return {@link #lastReviewDate} (The date on which the resource content was 1613 * last reviewed. Review happens periodically after approval but does 1614 * not change the original approval date.). This is the underlying 1615 * object with id, value and extensions. The accessor 1616 * "getLastReviewDate" gives direct access to the value 1617 */ 1618 public DateType getLastReviewDateElement() { 1619 if (this.lastReviewDate == null) 1620 if (Configuration.errorOnAutoCreate()) 1621 throw new Error("Attempt to auto-create ResearchDefinition.lastReviewDate"); 1622 else if (Configuration.doAutoCreate()) 1623 this.lastReviewDate = new DateType(); // bb 1624 return this.lastReviewDate; 1625 } 1626 1627 public boolean hasLastReviewDateElement() { 1628 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 1629 } 1630 1631 public boolean hasLastReviewDate() { 1632 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 1633 } 1634 1635 /** 1636 * @param value {@link #lastReviewDate} (The date on which the resource content 1637 * was last reviewed. Review happens periodically after approval 1638 * but does not change the original approval date.). This is the 1639 * underlying object with id, value and extensions. The accessor 1640 * "getLastReviewDate" gives direct access to the value 1641 */ 1642 public ResearchDefinition setLastReviewDateElement(DateType value) { 1643 this.lastReviewDate = value; 1644 return this; 1645 } 1646 1647 /** 1648 * @return The date on which the resource content was last reviewed. Review 1649 * happens periodically after approval but does not change the original 1650 * approval date. 1651 */ 1652 public Date getLastReviewDate() { 1653 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 1654 } 1655 1656 /** 1657 * @param value The date on which the resource content was last reviewed. Review 1658 * happens periodically after approval but does not change the 1659 * original approval date. 1660 */ 1661 public ResearchDefinition setLastReviewDate(Date value) { 1662 if (value == null) 1663 this.lastReviewDate = null; 1664 else { 1665 if (this.lastReviewDate == null) 1666 this.lastReviewDate = new DateType(); 1667 this.lastReviewDate.setValue(value); 1668 } 1669 return this; 1670 } 1671 1672 /** 1673 * @return {@link #effectivePeriod} (The period during which the research 1674 * definition content was or is planned to be in active use.) 1675 */ 1676 public Period getEffectivePeriod() { 1677 if (this.effectivePeriod == null) 1678 if (Configuration.errorOnAutoCreate()) 1679 throw new Error("Attempt to auto-create ResearchDefinition.effectivePeriod"); 1680 else if (Configuration.doAutoCreate()) 1681 this.effectivePeriod = new Period(); // cc 1682 return this.effectivePeriod; 1683 } 1684 1685 public boolean hasEffectivePeriod() { 1686 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 1687 } 1688 1689 /** 1690 * @param value {@link #effectivePeriod} (The period during which the research 1691 * definition content was or is planned to be in active use.) 1692 */ 1693 public ResearchDefinition setEffectivePeriod(Period value) { 1694 this.effectivePeriod = value; 1695 return this; 1696 } 1697 1698 /** 1699 * @return {@link #topic} (Descriptive topics related to the content of the 1700 * ResearchDefinition. Topics provide a high-level categorization 1701 * grouping types of ResearchDefinitions that can be useful for 1702 * filtering and searching.) 1703 */ 1704 public List<CodeableConcept> getTopic() { 1705 if (this.topic == null) 1706 this.topic = new ArrayList<CodeableConcept>(); 1707 return this.topic; 1708 } 1709 1710 /** 1711 * @return Returns a reference to <code>this</code> for easy method chaining 1712 */ 1713 public ResearchDefinition setTopic(List<CodeableConcept> theTopic) { 1714 this.topic = theTopic; 1715 return this; 1716 } 1717 1718 public boolean hasTopic() { 1719 if (this.topic == null) 1720 return false; 1721 for (CodeableConcept item : this.topic) 1722 if (!item.isEmpty()) 1723 return true; 1724 return false; 1725 } 1726 1727 public CodeableConcept addTopic() { // 3 1728 CodeableConcept t = new CodeableConcept(); 1729 if (this.topic == null) 1730 this.topic = new ArrayList<CodeableConcept>(); 1731 this.topic.add(t); 1732 return t; 1733 } 1734 1735 public ResearchDefinition addTopic(CodeableConcept t) { // 3 1736 if (t == null) 1737 return this; 1738 if (this.topic == null) 1739 this.topic = new ArrayList<CodeableConcept>(); 1740 this.topic.add(t); 1741 return this; 1742 } 1743 1744 /** 1745 * @return The first repetition of repeating field {@link #topic}, creating it 1746 * if it does not already exist 1747 */ 1748 public CodeableConcept getTopicFirstRep() { 1749 if (getTopic().isEmpty()) { 1750 addTopic(); 1751 } 1752 return getTopic().get(0); 1753 } 1754 1755 /** 1756 * @return {@link #author} (An individiual or organization primarily involved in 1757 * the creation and maintenance of the content.) 1758 */ 1759 public List<ContactDetail> getAuthor() { 1760 if (this.author == null) 1761 this.author = new ArrayList<ContactDetail>(); 1762 return this.author; 1763 } 1764 1765 /** 1766 * @return Returns a reference to <code>this</code> for easy method chaining 1767 */ 1768 public ResearchDefinition setAuthor(List<ContactDetail> theAuthor) { 1769 this.author = theAuthor; 1770 return this; 1771 } 1772 1773 public boolean hasAuthor() { 1774 if (this.author == null) 1775 return false; 1776 for (ContactDetail item : this.author) 1777 if (!item.isEmpty()) 1778 return true; 1779 return false; 1780 } 1781 1782 public ContactDetail addAuthor() { // 3 1783 ContactDetail t = new ContactDetail(); 1784 if (this.author == null) 1785 this.author = new ArrayList<ContactDetail>(); 1786 this.author.add(t); 1787 return t; 1788 } 1789 1790 public ResearchDefinition addAuthor(ContactDetail t) { // 3 1791 if (t == null) 1792 return this; 1793 if (this.author == null) 1794 this.author = new ArrayList<ContactDetail>(); 1795 this.author.add(t); 1796 return this; 1797 } 1798 1799 /** 1800 * @return The first repetition of repeating field {@link #author}, creating it 1801 * if it does not already exist 1802 */ 1803 public ContactDetail getAuthorFirstRep() { 1804 if (getAuthor().isEmpty()) { 1805 addAuthor(); 1806 } 1807 return getAuthor().get(0); 1808 } 1809 1810 /** 1811 * @return {@link #editor} (An individual or organization primarily responsible 1812 * for internal coherence of the content.) 1813 */ 1814 public List<ContactDetail> getEditor() { 1815 if (this.editor == null) 1816 this.editor = new ArrayList<ContactDetail>(); 1817 return this.editor; 1818 } 1819 1820 /** 1821 * @return Returns a reference to <code>this</code> for easy method chaining 1822 */ 1823 public ResearchDefinition setEditor(List<ContactDetail> theEditor) { 1824 this.editor = theEditor; 1825 return this; 1826 } 1827 1828 public boolean hasEditor() { 1829 if (this.editor == null) 1830 return false; 1831 for (ContactDetail item : this.editor) 1832 if (!item.isEmpty()) 1833 return true; 1834 return false; 1835 } 1836 1837 public ContactDetail addEditor() { // 3 1838 ContactDetail t = new ContactDetail(); 1839 if (this.editor == null) 1840 this.editor = new ArrayList<ContactDetail>(); 1841 this.editor.add(t); 1842 return t; 1843 } 1844 1845 public ResearchDefinition addEditor(ContactDetail t) { // 3 1846 if (t == null) 1847 return this; 1848 if (this.editor == null) 1849 this.editor = new ArrayList<ContactDetail>(); 1850 this.editor.add(t); 1851 return this; 1852 } 1853 1854 /** 1855 * @return The first repetition of repeating field {@link #editor}, creating it 1856 * if it does not already exist 1857 */ 1858 public ContactDetail getEditorFirstRep() { 1859 if (getEditor().isEmpty()) { 1860 addEditor(); 1861 } 1862 return getEditor().get(0); 1863 } 1864 1865 /** 1866 * @return {@link #reviewer} (An individual or organization primarily 1867 * responsible for review of some aspect of the content.) 1868 */ 1869 public List<ContactDetail> getReviewer() { 1870 if (this.reviewer == null) 1871 this.reviewer = new ArrayList<ContactDetail>(); 1872 return this.reviewer; 1873 } 1874 1875 /** 1876 * @return Returns a reference to <code>this</code> for easy method chaining 1877 */ 1878 public ResearchDefinition setReviewer(List<ContactDetail> theReviewer) { 1879 this.reviewer = theReviewer; 1880 return this; 1881 } 1882 1883 public boolean hasReviewer() { 1884 if (this.reviewer == null) 1885 return false; 1886 for (ContactDetail item : this.reviewer) 1887 if (!item.isEmpty()) 1888 return true; 1889 return false; 1890 } 1891 1892 public ContactDetail addReviewer() { // 3 1893 ContactDetail t = new ContactDetail(); 1894 if (this.reviewer == null) 1895 this.reviewer = new ArrayList<ContactDetail>(); 1896 this.reviewer.add(t); 1897 return t; 1898 } 1899 1900 public ResearchDefinition addReviewer(ContactDetail t) { // 3 1901 if (t == null) 1902 return this; 1903 if (this.reviewer == null) 1904 this.reviewer = new ArrayList<ContactDetail>(); 1905 this.reviewer.add(t); 1906 return this; 1907 } 1908 1909 /** 1910 * @return The first repetition of repeating field {@link #reviewer}, creating 1911 * it if it does not already exist 1912 */ 1913 public ContactDetail getReviewerFirstRep() { 1914 if (getReviewer().isEmpty()) { 1915 addReviewer(); 1916 } 1917 return getReviewer().get(0); 1918 } 1919 1920 /** 1921 * @return {@link #endorser} (An individual or organization responsible for 1922 * officially endorsing the content for use in some setting.) 1923 */ 1924 public List<ContactDetail> getEndorser() { 1925 if (this.endorser == null) 1926 this.endorser = new ArrayList<ContactDetail>(); 1927 return this.endorser; 1928 } 1929 1930 /** 1931 * @return Returns a reference to <code>this</code> for easy method chaining 1932 */ 1933 public ResearchDefinition setEndorser(List<ContactDetail> theEndorser) { 1934 this.endorser = theEndorser; 1935 return this; 1936 } 1937 1938 public boolean hasEndorser() { 1939 if (this.endorser == null) 1940 return false; 1941 for (ContactDetail item : this.endorser) 1942 if (!item.isEmpty()) 1943 return true; 1944 return false; 1945 } 1946 1947 public ContactDetail addEndorser() { // 3 1948 ContactDetail t = new ContactDetail(); 1949 if (this.endorser == null) 1950 this.endorser = new ArrayList<ContactDetail>(); 1951 this.endorser.add(t); 1952 return t; 1953 } 1954 1955 public ResearchDefinition addEndorser(ContactDetail t) { // 3 1956 if (t == null) 1957 return this; 1958 if (this.endorser == null) 1959 this.endorser = new ArrayList<ContactDetail>(); 1960 this.endorser.add(t); 1961 return this; 1962 } 1963 1964 /** 1965 * @return The first repetition of repeating field {@link #endorser}, creating 1966 * it if it does not already exist 1967 */ 1968 public ContactDetail getEndorserFirstRep() { 1969 if (getEndorser().isEmpty()) { 1970 addEndorser(); 1971 } 1972 return getEndorser().get(0); 1973 } 1974 1975 /** 1976 * @return {@link #relatedArtifact} (Related artifacts such as additional 1977 * documentation, justification, or bibliographic references.) 1978 */ 1979 public List<RelatedArtifact> getRelatedArtifact() { 1980 if (this.relatedArtifact == null) 1981 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 1982 return this.relatedArtifact; 1983 } 1984 1985 /** 1986 * @return Returns a reference to <code>this</code> for easy method chaining 1987 */ 1988 public ResearchDefinition setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 1989 this.relatedArtifact = theRelatedArtifact; 1990 return this; 1991 } 1992 1993 public boolean hasRelatedArtifact() { 1994 if (this.relatedArtifact == null) 1995 return false; 1996 for (RelatedArtifact item : this.relatedArtifact) 1997 if (!item.isEmpty()) 1998 return true; 1999 return false; 2000 } 2001 2002 public RelatedArtifact addRelatedArtifact() { // 3 2003 RelatedArtifact t = new RelatedArtifact(); 2004 if (this.relatedArtifact == null) 2005 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 2006 this.relatedArtifact.add(t); 2007 return t; 2008 } 2009 2010 public ResearchDefinition addRelatedArtifact(RelatedArtifact t) { // 3 2011 if (t == null) 2012 return this; 2013 if (this.relatedArtifact == null) 2014 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 2015 this.relatedArtifact.add(t); 2016 return this; 2017 } 2018 2019 /** 2020 * @return The first repetition of repeating field {@link #relatedArtifact}, 2021 * creating it if it does not already exist 2022 */ 2023 public RelatedArtifact getRelatedArtifactFirstRep() { 2024 if (getRelatedArtifact().isEmpty()) { 2025 addRelatedArtifact(); 2026 } 2027 return getRelatedArtifact().get(0); 2028 } 2029 2030 /** 2031 * @return {@link #library} (A reference to a Library resource containing the 2032 * formal logic used by the ResearchDefinition.) 2033 */ 2034 public List<CanonicalType> getLibrary() { 2035 if (this.library == null) 2036 this.library = new ArrayList<CanonicalType>(); 2037 return this.library; 2038 } 2039 2040 /** 2041 * @return Returns a reference to <code>this</code> for easy method chaining 2042 */ 2043 public ResearchDefinition setLibrary(List<CanonicalType> theLibrary) { 2044 this.library = theLibrary; 2045 return this; 2046 } 2047 2048 public boolean hasLibrary() { 2049 if (this.library == null) 2050 return false; 2051 for (CanonicalType item : this.library) 2052 if (!item.isEmpty()) 2053 return true; 2054 return false; 2055 } 2056 2057 /** 2058 * @return {@link #library} (A reference to a Library resource containing the 2059 * formal logic used by the ResearchDefinition.) 2060 */ 2061 public CanonicalType addLibraryElement() {// 2 2062 CanonicalType t = new CanonicalType(); 2063 if (this.library == null) 2064 this.library = new ArrayList<CanonicalType>(); 2065 this.library.add(t); 2066 return t; 2067 } 2068 2069 /** 2070 * @param value {@link #library} (A reference to a Library resource containing 2071 * the formal logic used by the ResearchDefinition.) 2072 */ 2073 public ResearchDefinition addLibrary(String value) { // 1 2074 CanonicalType t = new CanonicalType(); 2075 t.setValue(value); 2076 if (this.library == null) 2077 this.library = new ArrayList<CanonicalType>(); 2078 this.library.add(t); 2079 return this; 2080 } 2081 2082 /** 2083 * @param value {@link #library} (A reference to a Library resource containing 2084 * the formal logic used by the ResearchDefinition.) 2085 */ 2086 public boolean hasLibrary(String value) { 2087 if (this.library == null) 2088 return false; 2089 for (CanonicalType v : this.library) 2090 if (v.getValue().equals(value)) // canonical(Library) 2091 return true; 2092 return false; 2093 } 2094 2095 /** 2096 * @return {@link #population} (A reference to a ResearchElementDefinition 2097 * resource that defines the population for the research.) 2098 */ 2099 public Reference getPopulation() { 2100 if (this.population == null) 2101 if (Configuration.errorOnAutoCreate()) 2102 throw new Error("Attempt to auto-create ResearchDefinition.population"); 2103 else if (Configuration.doAutoCreate()) 2104 this.population = new Reference(); // cc 2105 return this.population; 2106 } 2107 2108 public boolean hasPopulation() { 2109 return this.population != null && !this.population.isEmpty(); 2110 } 2111 2112 /** 2113 * @param value {@link #population} (A reference to a ResearchElementDefinition 2114 * resource that defines the population for the research.) 2115 */ 2116 public ResearchDefinition setPopulation(Reference value) { 2117 this.population = value; 2118 return this; 2119 } 2120 2121 /** 2122 * @return {@link #population} The actual object that is the target of the 2123 * reference. The reference library doesn't populate this, but you can 2124 * use it to hold the resource if you resolve it. (A reference to a 2125 * ResearchElementDefinition resource that defines the population for 2126 * the research.) 2127 */ 2128 public ResearchElementDefinition getPopulationTarget() { 2129 if (this.populationTarget == null) 2130 if (Configuration.errorOnAutoCreate()) 2131 throw new Error("Attempt to auto-create ResearchDefinition.population"); 2132 else if (Configuration.doAutoCreate()) 2133 this.populationTarget = new ResearchElementDefinition(); // aa 2134 return this.populationTarget; 2135 } 2136 2137 /** 2138 * @param value {@link #population} The actual object that is the target of the 2139 * reference. The reference library doesn't use these, but you can 2140 * use it to hold the resource if you resolve it. (A reference to a 2141 * ResearchElementDefinition resource that defines the population 2142 * for the research.) 2143 */ 2144 public ResearchDefinition setPopulationTarget(ResearchElementDefinition value) { 2145 this.populationTarget = value; 2146 return this; 2147 } 2148 2149 /** 2150 * @return {@link #exposure} (A reference to a ResearchElementDefinition 2151 * resource that defines the exposure for the research.) 2152 */ 2153 public Reference getExposure() { 2154 if (this.exposure == null) 2155 if (Configuration.errorOnAutoCreate()) 2156 throw new Error("Attempt to auto-create ResearchDefinition.exposure"); 2157 else if (Configuration.doAutoCreate()) 2158 this.exposure = new Reference(); // cc 2159 return this.exposure; 2160 } 2161 2162 public boolean hasExposure() { 2163 return this.exposure != null && !this.exposure.isEmpty(); 2164 } 2165 2166 /** 2167 * @param value {@link #exposure} (A reference to a ResearchElementDefinition 2168 * resource that defines the exposure for the research.) 2169 */ 2170 public ResearchDefinition setExposure(Reference value) { 2171 this.exposure = value; 2172 return this; 2173 } 2174 2175 /** 2176 * @return {@link #exposure} The actual object that is the target of the 2177 * reference. The reference library doesn't populate this, but you can 2178 * use it to hold the resource if you resolve it. (A reference to a 2179 * ResearchElementDefinition resource that defines the exposure for the 2180 * research.) 2181 */ 2182 public ResearchElementDefinition getExposureTarget() { 2183 if (this.exposureTarget == null) 2184 if (Configuration.errorOnAutoCreate()) 2185 throw new Error("Attempt to auto-create ResearchDefinition.exposure"); 2186 else if (Configuration.doAutoCreate()) 2187 this.exposureTarget = new ResearchElementDefinition(); // aa 2188 return this.exposureTarget; 2189 } 2190 2191 /** 2192 * @param value {@link #exposure} The actual object that is the target of the 2193 * reference. The reference library doesn't use these, but you can 2194 * use it to hold the resource if you resolve it. (A reference to a 2195 * ResearchElementDefinition resource that defines the exposure for 2196 * the research.) 2197 */ 2198 public ResearchDefinition setExposureTarget(ResearchElementDefinition value) { 2199 this.exposureTarget = value; 2200 return this; 2201 } 2202 2203 /** 2204 * @return {@link #exposureAlternative} (A reference to a 2205 * ResearchElementDefinition resource that defines the 2206 * exposureAlternative for the research.) 2207 */ 2208 public Reference getExposureAlternative() { 2209 if (this.exposureAlternative == null) 2210 if (Configuration.errorOnAutoCreate()) 2211 throw new Error("Attempt to auto-create ResearchDefinition.exposureAlternative"); 2212 else if (Configuration.doAutoCreate()) 2213 this.exposureAlternative = new Reference(); // cc 2214 return this.exposureAlternative; 2215 } 2216 2217 public boolean hasExposureAlternative() { 2218 return this.exposureAlternative != null && !this.exposureAlternative.isEmpty(); 2219 } 2220 2221 /** 2222 * @param value {@link #exposureAlternative} (A reference to a 2223 * ResearchElementDefinition resource that defines the 2224 * exposureAlternative for the research.) 2225 */ 2226 public ResearchDefinition setExposureAlternative(Reference value) { 2227 this.exposureAlternative = value; 2228 return this; 2229 } 2230 2231 /** 2232 * @return {@link #exposureAlternative} The actual object that is the target of 2233 * the reference. The reference library doesn't populate this, but you 2234 * can use it to hold the resource if you resolve it. (A reference to a 2235 * ResearchElementDefinition resource that defines the 2236 * exposureAlternative for the research.) 2237 */ 2238 public ResearchElementDefinition getExposureAlternativeTarget() { 2239 if (this.exposureAlternativeTarget == null) 2240 if (Configuration.errorOnAutoCreate()) 2241 throw new Error("Attempt to auto-create ResearchDefinition.exposureAlternative"); 2242 else if (Configuration.doAutoCreate()) 2243 this.exposureAlternativeTarget = new ResearchElementDefinition(); // aa 2244 return this.exposureAlternativeTarget; 2245 } 2246 2247 /** 2248 * @param value {@link #exposureAlternative} The actual object that is the 2249 * target of the reference. The reference library doesn't use 2250 * these, but you can use it to hold the resource if you resolve 2251 * it. (A reference to a ResearchElementDefinition resource that 2252 * defines the exposureAlternative for the research.) 2253 */ 2254 public ResearchDefinition setExposureAlternativeTarget(ResearchElementDefinition value) { 2255 this.exposureAlternativeTarget = value; 2256 return this; 2257 } 2258 2259 /** 2260 * @return {@link #outcome} (A reference to a ResearchElementDefinition resomece 2261 * that defines the outcome for the research.) 2262 */ 2263 public Reference getOutcome() { 2264 if (this.outcome == null) 2265 if (Configuration.errorOnAutoCreate()) 2266 throw new Error("Attempt to auto-create ResearchDefinition.outcome"); 2267 else if (Configuration.doAutoCreate()) 2268 this.outcome = new Reference(); // cc 2269 return this.outcome; 2270 } 2271 2272 public boolean hasOutcome() { 2273 return this.outcome != null && !this.outcome.isEmpty(); 2274 } 2275 2276 /** 2277 * @param value {@link #outcome} (A reference to a ResearchElementDefinition 2278 * resomece that defines the outcome for the research.) 2279 */ 2280 public ResearchDefinition setOutcome(Reference value) { 2281 this.outcome = value; 2282 return this; 2283 } 2284 2285 /** 2286 * @return {@link #outcome} The actual object that is the target of the 2287 * reference. The reference library doesn't populate this, but you can 2288 * use it to hold the resource if you resolve it. (A reference to a 2289 * ResearchElementDefinition resomece that defines the outcome for the 2290 * research.) 2291 */ 2292 public ResearchElementDefinition getOutcomeTarget() { 2293 if (this.outcomeTarget == null) 2294 if (Configuration.errorOnAutoCreate()) 2295 throw new Error("Attempt to auto-create ResearchDefinition.outcome"); 2296 else if (Configuration.doAutoCreate()) 2297 this.outcomeTarget = new ResearchElementDefinition(); // aa 2298 return this.outcomeTarget; 2299 } 2300 2301 /** 2302 * @param value {@link #outcome} The actual object that is the target of the 2303 * reference. The reference library doesn't use these, but you can 2304 * use it to hold the resource if you resolve it. (A reference to a 2305 * ResearchElementDefinition resomece that defines the outcome for 2306 * the research.) 2307 */ 2308 public ResearchDefinition setOutcomeTarget(ResearchElementDefinition value) { 2309 this.outcomeTarget = value; 2310 return this; 2311 } 2312 2313 protected void listChildren(List<Property> children) { 2314 super.listChildren(children); 2315 children.add(new Property("url", "uri", 2316 "An absolute URI that is used to identify this research definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this research definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the research definition is stored on different servers.", 2317 0, 1, url)); 2318 children.add(new Property("identifier", "Identifier", 2319 "A formal identifier that is used to identify this research definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 2320 0, java.lang.Integer.MAX_VALUE, identifier)); 2321 children.add(new Property("version", "string", 2322 "The identifier that is used to identify this version of the research definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the research definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 2323 0, 1, version)); 2324 children.add(new Property("name", "string", 2325 "A natural language name identifying the research definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 2326 0, 1, name)); 2327 children.add(new Property("title", "string", 2328 "A short, descriptive, user-friendly title for the research definition.", 0, 1, title)); 2329 children.add(new Property("shortTitle", "string", 2330 "The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary.", 2331 0, 1, shortTitle)); 2332 children.add(new Property("subtitle", "string", 2333 "An explanatory or alternate title for the ResearchDefinition giving additional information about its content.", 2334 0, 1, subtitle)); 2335 children.add(new Property("status", "code", 2336 "The status of this research definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 2337 children.add(new Property("experimental", "boolean", 2338 "A Boolean value to indicate that this research definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 2339 0, 1, experimental)); 2340 children.add(new Property("subject[x]", "CodeableConcept|Reference(Group)", 2341 "The intended subjects for the ResearchDefinition. If this element is not provided, a Patient subject is assumed, but the subject of the ResearchDefinition can be anything.", 2342 0, 1, subject)); 2343 children.add(new Property("date", "dateTime", 2344 "The date (and optionally time) when the research definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the research definition changes.", 2345 0, 1, date)); 2346 children.add(new Property("publisher", "string", 2347 "The name of the organization or individual that published the research definition.", 0, 1, publisher)); 2348 children.add(new Property("contact", "ContactDetail", 2349 "Contact details to assist a user in finding and communicating with the publisher.", 0, 2350 java.lang.Integer.MAX_VALUE, contact)); 2351 children.add(new Property("description", "markdown", 2352 "A free text natural language description of the research definition from a consumer's perspective.", 0, 1, 2353 description)); 2354 children.add( 2355 new Property("comment", "string", "A human-readable string to clarify or explain concepts about the resource.", 2356 0, java.lang.Integer.MAX_VALUE, comment)); 2357 children.add(new Property("useContext", "UsageContext", 2358 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate research definition instances.", 2359 0, java.lang.Integer.MAX_VALUE, useContext)); 2360 children.add(new Property("jurisdiction", "CodeableConcept", 2361 "A legal or geographic region in which the research definition is intended to be used.", 0, 2362 java.lang.Integer.MAX_VALUE, jurisdiction)); 2363 children.add(new Property("purpose", "markdown", 2364 "Explanation of why this research definition is needed and why it has been designed as it has.", 0, 1, 2365 purpose)); 2366 children.add(new Property("usage", "string", 2367 "A detailed description, from a clinical perspective, of how the ResearchDefinition is used.", 0, 1, usage)); 2368 children.add(new Property("copyright", "markdown", 2369 "A copyright statement relating to the research definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the research definition.", 2370 0, 1, copyright)); 2371 children.add(new Property("approvalDate", "date", 2372 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 2373 0, 1, approvalDate)); 2374 children.add(new Property("lastReviewDate", "date", 2375 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 2376 0, 1, lastReviewDate)); 2377 children.add(new Property("effectivePeriod", "Period", 2378 "The period during which the research definition content was or is planned to be in active use.", 0, 1, 2379 effectivePeriod)); 2380 children.add(new Property("topic", "CodeableConcept", 2381 "Descriptive topics related to the content of the ResearchDefinition. Topics provide a high-level categorization grouping types of ResearchDefinitions that can be useful for filtering and searching.", 2382 0, java.lang.Integer.MAX_VALUE, topic)); 2383 children.add(new Property("author", "ContactDetail", 2384 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 2385 java.lang.Integer.MAX_VALUE, author)); 2386 children.add(new Property("editor", "ContactDetail", 2387 "An individual or organization primarily responsible for internal coherence of the content.", 0, 2388 java.lang.Integer.MAX_VALUE, editor)); 2389 children.add(new Property("reviewer", "ContactDetail", 2390 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 2391 java.lang.Integer.MAX_VALUE, reviewer)); 2392 children.add(new Property("endorser", "ContactDetail", 2393 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 2394 java.lang.Integer.MAX_VALUE, endorser)); 2395 children.add(new Property("relatedArtifact", "RelatedArtifact", 2396 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 2397 java.lang.Integer.MAX_VALUE, relatedArtifact)); 2398 children.add(new Property("library", "canonical(Library)", 2399 "A reference to a Library resource containing the formal logic used by the ResearchDefinition.", 0, 2400 java.lang.Integer.MAX_VALUE, library)); 2401 children.add(new Property("population", "Reference(ResearchElementDefinition)", 2402 "A reference to a ResearchElementDefinition resource that defines the population for the research.", 0, 1, 2403 population)); 2404 children.add(new Property("exposure", "Reference(ResearchElementDefinition)", 2405 "A reference to a ResearchElementDefinition resource that defines the exposure for the research.", 0, 1, 2406 exposure)); 2407 children.add(new Property("exposureAlternative", "Reference(ResearchElementDefinition)", 2408 "A reference to a ResearchElementDefinition resource that defines the exposureAlternative for the research.", 0, 2409 1, exposureAlternative)); 2410 children.add(new Property("outcome", "Reference(ResearchElementDefinition)", 2411 "A reference to a ResearchElementDefinition resomece that defines the outcome for the research.", 0, 1, 2412 outcome)); 2413 } 2414 2415 @Override 2416 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2417 switch (_hash) { 2418 case 116079: 2419 /* url */ return new Property("url", "uri", 2420 "An absolute URI that is used to identify this research definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this research definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the research definition is stored on different servers.", 2421 0, 1, url); 2422 case -1618432855: 2423 /* identifier */ return new Property("identifier", "Identifier", 2424 "A formal identifier that is used to identify this research definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 2425 0, java.lang.Integer.MAX_VALUE, identifier); 2426 case 351608024: 2427 /* version */ return new Property("version", "string", 2428 "The identifier that is used to identify this version of the research definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the research definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 2429 0, 1, version); 2430 case 3373707: 2431 /* name */ return new Property("name", "string", 2432 "A natural language name identifying the research definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 2433 0, 1, name); 2434 case 110371416: 2435 /* title */ return new Property("title", "string", 2436 "A short, descriptive, user-friendly title for the research definition.", 0, 1, title); 2437 case 1555503932: 2438 /* shortTitle */ return new Property("shortTitle", "string", 2439 "The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary.", 2440 0, 1, shortTitle); 2441 case -2060497896: 2442 /* subtitle */ return new Property("subtitle", "string", 2443 "An explanatory or alternate title for the ResearchDefinition giving additional information about its content.", 2444 0, 1, subtitle); 2445 case -892481550: 2446 /* status */ return new Property("status", "code", 2447 "The status of this research definition. Enables tracking the life-cycle of the content.", 0, 1, status); 2448 case -404562712: 2449 /* experimental */ return new Property("experimental", "boolean", 2450 "A Boolean value to indicate that this research definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 2451 0, 1, experimental); 2452 case -573640748: 2453 /* subject[x] */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 2454 "The intended subjects for the ResearchDefinition. If this element is not provided, a Patient subject is assumed, but the subject of the ResearchDefinition can be anything.", 2455 0, 1, subject); 2456 case -1867885268: 2457 /* subject */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 2458 "The intended subjects for the ResearchDefinition. If this element is not provided, a Patient subject is assumed, but the subject of the ResearchDefinition can be anything.", 2459 0, 1, subject); 2460 case -1257122603: 2461 /* subjectCodeableConcept */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 2462 "The intended subjects for the ResearchDefinition. If this element is not provided, a Patient subject is assumed, but the subject of the ResearchDefinition can be anything.", 2463 0, 1, subject); 2464 case 772938623: 2465 /* subjectReference */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 2466 "The intended subjects for the ResearchDefinition. If this element is not provided, a Patient subject is assumed, but the subject of the ResearchDefinition can be anything.", 2467 0, 1, subject); 2468 case 3076014: 2469 /* date */ return new Property("date", "dateTime", 2470 "The date (and optionally time) when the research definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the research definition changes.", 2471 0, 1, date); 2472 case 1447404028: 2473 /* publisher */ return new Property("publisher", "string", 2474 "The name of the organization or individual that published the research definition.", 0, 1, publisher); 2475 case 951526432: 2476 /* contact */ return new Property("contact", "ContactDetail", 2477 "Contact details to assist a user in finding and communicating with the publisher.", 0, 2478 java.lang.Integer.MAX_VALUE, contact); 2479 case -1724546052: 2480 /* description */ return new Property("description", "markdown", 2481 "A free text natural language description of the research definition from a consumer's perspective.", 0, 1, 2482 description); 2483 case 950398559: 2484 /* comment */ return new Property("comment", "string", 2485 "A human-readable string to clarify or explain concepts about the resource.", 0, java.lang.Integer.MAX_VALUE, 2486 comment); 2487 case -669707736: 2488 /* useContext */ return new Property("useContext", "UsageContext", 2489 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate research definition instances.", 2490 0, java.lang.Integer.MAX_VALUE, useContext); 2491 case -507075711: 2492 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 2493 "A legal or geographic region in which the research definition is intended to be used.", 0, 2494 java.lang.Integer.MAX_VALUE, jurisdiction); 2495 case -220463842: 2496 /* purpose */ return new Property("purpose", "markdown", 2497 "Explanation of why this research definition is needed and why it has been designed as it has.", 0, 1, 2498 purpose); 2499 case 111574433: 2500 /* usage */ return new Property("usage", "string", 2501 "A detailed description, from a clinical perspective, of how the ResearchDefinition is used.", 0, 1, usage); 2502 case 1522889671: 2503 /* copyright */ return new Property("copyright", "markdown", 2504 "A copyright statement relating to the research definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the research definition.", 2505 0, 1, copyright); 2506 case 223539345: 2507 /* approvalDate */ return new Property("approvalDate", "date", 2508 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 2509 0, 1, approvalDate); 2510 case -1687512484: 2511 /* lastReviewDate */ return new Property("lastReviewDate", "date", 2512 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 2513 0, 1, lastReviewDate); 2514 case -403934648: 2515 /* effectivePeriod */ return new Property("effectivePeriod", "Period", 2516 "The period during which the research definition content was or is planned to be in active use.", 0, 1, 2517 effectivePeriod); 2518 case 110546223: 2519 /* topic */ return new Property("topic", "CodeableConcept", 2520 "Descriptive topics related to the content of the ResearchDefinition. Topics provide a high-level categorization grouping types of ResearchDefinitions that can be useful for filtering and searching.", 2521 0, java.lang.Integer.MAX_VALUE, topic); 2522 case -1406328437: 2523 /* author */ return new Property("author", "ContactDetail", 2524 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 2525 java.lang.Integer.MAX_VALUE, author); 2526 case -1307827859: 2527 /* editor */ return new Property("editor", "ContactDetail", 2528 "An individual or organization primarily responsible for internal coherence of the content.", 0, 2529 java.lang.Integer.MAX_VALUE, editor); 2530 case -261190139: 2531 /* reviewer */ return new Property("reviewer", "ContactDetail", 2532 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 2533 java.lang.Integer.MAX_VALUE, reviewer); 2534 case 1740277666: 2535 /* endorser */ return new Property("endorser", "ContactDetail", 2536 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 2537 java.lang.Integer.MAX_VALUE, endorser); 2538 case 666807069: 2539 /* relatedArtifact */ return new Property("relatedArtifact", "RelatedArtifact", 2540 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 2541 java.lang.Integer.MAX_VALUE, relatedArtifact); 2542 case 166208699: 2543 /* library */ return new Property("library", "canonical(Library)", 2544 "A reference to a Library resource containing the formal logic used by the ResearchDefinition.", 0, 2545 java.lang.Integer.MAX_VALUE, library); 2546 case -2023558323: 2547 /* population */ return new Property("population", "Reference(ResearchElementDefinition)", 2548 "A reference to a ResearchElementDefinition resource that defines the population for the research.", 0, 1, 2549 population); 2550 case -1926005497: 2551 /* exposure */ return new Property("exposure", "Reference(ResearchElementDefinition)", 2552 "A reference to a ResearchElementDefinition resource that defines the exposure for the research.", 0, 1, 2553 exposure); 2554 case -1875462106: 2555 /* exposureAlternative */ return new Property("exposureAlternative", "Reference(ResearchElementDefinition)", 2556 "A reference to a ResearchElementDefinition resource that defines the exposureAlternative for the research.", 2557 0, 1, exposureAlternative); 2558 case -1106507950: 2559 /* outcome */ return new Property("outcome", "Reference(ResearchElementDefinition)", 2560 "A reference to a ResearchElementDefinition resomece that defines the outcome for the research.", 0, 1, 2561 outcome); 2562 default: 2563 return super.getNamedProperty(_hash, _name, _checkValid); 2564 } 2565 2566 } 2567 2568 @Override 2569 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2570 switch (hash) { 2571 case 116079: 2572 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 2573 case -1618432855: 2574 /* identifier */ return this.identifier == null ? new Base[0] 2575 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2576 case 351608024: 2577 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 2578 case 3373707: 2579 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 2580 case 110371416: 2581 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 2582 case 1555503932: 2583 /* shortTitle */ return this.shortTitle == null ? new Base[0] : new Base[] { this.shortTitle }; // StringType 2584 case -2060497896: 2585 /* subtitle */ return this.subtitle == null ? new Base[0] : new Base[] { this.subtitle }; // StringType 2586 case -892481550: 2587 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 2588 case -404562712: 2589 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 2590 case -1867885268: 2591 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Type 2592 case 3076014: 2593 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 2594 case 1447404028: 2595 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 2596 case 951526432: 2597 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 2598 case -1724546052: 2599 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 2600 case 950398559: 2601 /* comment */ return this.comment == null ? new Base[0] : this.comment.toArray(new Base[this.comment.size()]); // StringType 2602 case -669707736: 2603 /* useContext */ return this.useContext == null ? new Base[0] 2604 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 2605 case -507075711: 2606 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 2607 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 2608 case -220463842: 2609 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 2610 case 111574433: 2611 /* usage */ return this.usage == null ? new Base[0] : new Base[] { this.usage }; // StringType 2612 case 1522889671: 2613 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 2614 case 223539345: 2615 /* approvalDate */ return this.approvalDate == null ? new Base[0] : new Base[] { this.approvalDate }; // DateType 2616 case -1687512484: 2617 /* lastReviewDate */ return this.lastReviewDate == null ? new Base[0] : new Base[] { this.lastReviewDate }; // DateType 2618 case -403934648: 2619 /* effectivePeriod */ return this.effectivePeriod == null ? new Base[0] : new Base[] { this.effectivePeriod }; // Period 2620 case 110546223: 2621 /* topic */ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 2622 case -1406328437: 2623 /* author */ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 2624 case -1307827859: 2625 /* editor */ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 2626 case -261190139: 2627 /* reviewer */ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 2628 case 1740277666: 2629 /* endorser */ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 2630 case 666807069: 2631 /* relatedArtifact */ return this.relatedArtifact == null ? new Base[0] 2632 : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 2633 case 166208699: 2634 /* library */ return this.library == null ? new Base[0] : this.library.toArray(new Base[this.library.size()]); // CanonicalType 2635 case -2023558323: 2636 /* population */ return this.population == null ? new Base[0] : new Base[] { this.population }; // Reference 2637 case -1926005497: 2638 /* exposure */ return this.exposure == null ? new Base[0] : new Base[] { this.exposure }; // Reference 2639 case -1875462106: 2640 /* exposureAlternative */ return this.exposureAlternative == null ? new Base[0] 2641 : new Base[] { this.exposureAlternative }; // Reference 2642 case -1106507950: 2643 /* outcome */ return this.outcome == null ? new Base[0] : new Base[] { this.outcome }; // Reference 2644 default: 2645 return super.getProperty(hash, name, checkValid); 2646 } 2647 2648 } 2649 2650 @Override 2651 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2652 switch (hash) { 2653 case 116079: // url 2654 this.url = castToUri(value); // UriType 2655 return value; 2656 case -1618432855: // identifier 2657 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2658 return value; 2659 case 351608024: // version 2660 this.version = castToString(value); // StringType 2661 return value; 2662 case 3373707: // name 2663 this.name = castToString(value); // StringType 2664 return value; 2665 case 110371416: // title 2666 this.title = castToString(value); // StringType 2667 return value; 2668 case 1555503932: // shortTitle 2669 this.shortTitle = castToString(value); // StringType 2670 return value; 2671 case -2060497896: // subtitle 2672 this.subtitle = castToString(value); // StringType 2673 return value; 2674 case -892481550: // status 2675 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 2676 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2677 return value; 2678 case -404562712: // experimental 2679 this.experimental = castToBoolean(value); // BooleanType 2680 return value; 2681 case -1867885268: // subject 2682 this.subject = castToType(value); // Type 2683 return value; 2684 case 3076014: // date 2685 this.date = castToDateTime(value); // DateTimeType 2686 return value; 2687 case 1447404028: // publisher 2688 this.publisher = castToString(value); // StringType 2689 return value; 2690 case 951526432: // contact 2691 this.getContact().add(castToContactDetail(value)); // ContactDetail 2692 return value; 2693 case -1724546052: // description 2694 this.description = castToMarkdown(value); // MarkdownType 2695 return value; 2696 case 950398559: // comment 2697 this.getComment().add(castToString(value)); // StringType 2698 return value; 2699 case -669707736: // useContext 2700 this.getUseContext().add(castToUsageContext(value)); // UsageContext 2701 return value; 2702 case -507075711: // jurisdiction 2703 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 2704 return value; 2705 case -220463842: // purpose 2706 this.purpose = castToMarkdown(value); // MarkdownType 2707 return value; 2708 case 111574433: // usage 2709 this.usage = castToString(value); // StringType 2710 return value; 2711 case 1522889671: // copyright 2712 this.copyright = castToMarkdown(value); // MarkdownType 2713 return value; 2714 case 223539345: // approvalDate 2715 this.approvalDate = castToDate(value); // DateType 2716 return value; 2717 case -1687512484: // lastReviewDate 2718 this.lastReviewDate = castToDate(value); // DateType 2719 return value; 2720 case -403934648: // effectivePeriod 2721 this.effectivePeriod = castToPeriod(value); // Period 2722 return value; 2723 case 110546223: // topic 2724 this.getTopic().add(castToCodeableConcept(value)); // CodeableConcept 2725 return value; 2726 case -1406328437: // author 2727 this.getAuthor().add(castToContactDetail(value)); // ContactDetail 2728 return value; 2729 case -1307827859: // editor 2730 this.getEditor().add(castToContactDetail(value)); // ContactDetail 2731 return value; 2732 case -261190139: // reviewer 2733 this.getReviewer().add(castToContactDetail(value)); // ContactDetail 2734 return value; 2735 case 1740277666: // endorser 2736 this.getEndorser().add(castToContactDetail(value)); // ContactDetail 2737 return value; 2738 case 666807069: // relatedArtifact 2739 this.getRelatedArtifact().add(castToRelatedArtifact(value)); // RelatedArtifact 2740 return value; 2741 case 166208699: // library 2742 this.getLibrary().add(castToCanonical(value)); // CanonicalType 2743 return value; 2744 case -2023558323: // population 2745 this.population = castToReference(value); // Reference 2746 return value; 2747 case -1926005497: // exposure 2748 this.exposure = castToReference(value); // Reference 2749 return value; 2750 case -1875462106: // exposureAlternative 2751 this.exposureAlternative = castToReference(value); // Reference 2752 return value; 2753 case -1106507950: // outcome 2754 this.outcome = castToReference(value); // Reference 2755 return value; 2756 default: 2757 return super.setProperty(hash, name, value); 2758 } 2759 2760 } 2761 2762 @Override 2763 public Base setProperty(String name, Base value) throws FHIRException { 2764 if (name.equals("url")) { 2765 this.url = castToUri(value); // UriType 2766 } else if (name.equals("identifier")) { 2767 this.getIdentifier().add(castToIdentifier(value)); 2768 } else if (name.equals("version")) { 2769 this.version = castToString(value); // StringType 2770 } else if (name.equals("name")) { 2771 this.name = castToString(value); // StringType 2772 } else if (name.equals("title")) { 2773 this.title = castToString(value); // StringType 2774 } else if (name.equals("shortTitle")) { 2775 this.shortTitle = castToString(value); // StringType 2776 } else if (name.equals("subtitle")) { 2777 this.subtitle = castToString(value); // StringType 2778 } else if (name.equals("status")) { 2779 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 2780 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2781 } else if (name.equals("experimental")) { 2782 this.experimental = castToBoolean(value); // BooleanType 2783 } else if (name.equals("subject[x]")) { 2784 this.subject = castToType(value); // Type 2785 } else if (name.equals("date")) { 2786 this.date = castToDateTime(value); // DateTimeType 2787 } else if (name.equals("publisher")) { 2788 this.publisher = castToString(value); // StringType 2789 } else if (name.equals("contact")) { 2790 this.getContact().add(castToContactDetail(value)); 2791 } else if (name.equals("description")) { 2792 this.description = castToMarkdown(value); // MarkdownType 2793 } else if (name.equals("comment")) { 2794 this.getComment().add(castToString(value)); 2795 } else if (name.equals("useContext")) { 2796 this.getUseContext().add(castToUsageContext(value)); 2797 } else if (name.equals("jurisdiction")) { 2798 this.getJurisdiction().add(castToCodeableConcept(value)); 2799 } else if (name.equals("purpose")) { 2800 this.purpose = castToMarkdown(value); // MarkdownType 2801 } else if (name.equals("usage")) { 2802 this.usage = castToString(value); // StringType 2803 } else if (name.equals("copyright")) { 2804 this.copyright = castToMarkdown(value); // MarkdownType 2805 } else if (name.equals("approvalDate")) { 2806 this.approvalDate = castToDate(value); // DateType 2807 } else if (name.equals("lastReviewDate")) { 2808 this.lastReviewDate = castToDate(value); // DateType 2809 } else if (name.equals("effectivePeriod")) { 2810 this.effectivePeriod = castToPeriod(value); // Period 2811 } else if (name.equals("topic")) { 2812 this.getTopic().add(castToCodeableConcept(value)); 2813 } else if (name.equals("author")) { 2814 this.getAuthor().add(castToContactDetail(value)); 2815 } else if (name.equals("editor")) { 2816 this.getEditor().add(castToContactDetail(value)); 2817 } else if (name.equals("reviewer")) { 2818 this.getReviewer().add(castToContactDetail(value)); 2819 } else if (name.equals("endorser")) { 2820 this.getEndorser().add(castToContactDetail(value)); 2821 } else if (name.equals("relatedArtifact")) { 2822 this.getRelatedArtifact().add(castToRelatedArtifact(value)); 2823 } else if (name.equals("library")) { 2824 this.getLibrary().add(castToCanonical(value)); 2825 } else if (name.equals("population")) { 2826 this.population = castToReference(value); // Reference 2827 } else if (name.equals("exposure")) { 2828 this.exposure = castToReference(value); // Reference 2829 } else if (name.equals("exposureAlternative")) { 2830 this.exposureAlternative = castToReference(value); // Reference 2831 } else if (name.equals("outcome")) { 2832 this.outcome = castToReference(value); // Reference 2833 } else 2834 return super.setProperty(name, value); 2835 return value; 2836 } 2837 2838 @Override 2839 public void removeChild(String name, Base value) throws FHIRException { 2840 if (name.equals("url")) { 2841 this.url = null; 2842 } else if (name.equals("identifier")) { 2843 this.getIdentifier().remove(castToIdentifier(value)); 2844 } else if (name.equals("version")) { 2845 this.version = null; 2846 } else if (name.equals("name")) { 2847 this.name = null; 2848 } else if (name.equals("title")) { 2849 this.title = null; 2850 } else if (name.equals("shortTitle")) { 2851 this.shortTitle = null; 2852 } else if (name.equals("subtitle")) { 2853 this.subtitle = null; 2854 } else if (name.equals("status")) { 2855 this.status = null; 2856 } else if (name.equals("experimental")) { 2857 this.experimental = null; 2858 } else if (name.equals("subject[x]")) { 2859 this.subject = null; 2860 } else if (name.equals("date")) { 2861 this.date = null; 2862 } else if (name.equals("publisher")) { 2863 this.publisher = null; 2864 } else if (name.equals("contact")) { 2865 this.getContact().remove(castToContactDetail(value)); 2866 } else if (name.equals("description")) { 2867 this.description = null; 2868 } else if (name.equals("comment")) { 2869 this.getComment().remove(castToString(value)); 2870 } else if (name.equals("useContext")) { 2871 this.getUseContext().remove(castToUsageContext(value)); 2872 } else if (name.equals("jurisdiction")) { 2873 this.getJurisdiction().remove(castToCodeableConcept(value)); 2874 } else if (name.equals("purpose")) { 2875 this.purpose = null; 2876 } else if (name.equals("usage")) { 2877 this.usage = null; 2878 } else if (name.equals("copyright")) { 2879 this.copyright = null; 2880 } else if (name.equals("approvalDate")) { 2881 this.approvalDate = null; 2882 } else if (name.equals("lastReviewDate")) { 2883 this.lastReviewDate = null; 2884 } else if (name.equals("effectivePeriod")) { 2885 this.effectivePeriod = null; 2886 } else if (name.equals("topic")) { 2887 this.getTopic().remove(castToCodeableConcept(value)); 2888 } else if (name.equals("author")) { 2889 this.getAuthor().remove(castToContactDetail(value)); 2890 } else if (name.equals("editor")) { 2891 this.getEditor().remove(castToContactDetail(value)); 2892 } else if (name.equals("reviewer")) { 2893 this.getReviewer().remove(castToContactDetail(value)); 2894 } else if (name.equals("endorser")) { 2895 this.getEndorser().remove(castToContactDetail(value)); 2896 } else if (name.equals("relatedArtifact")) { 2897 this.getRelatedArtifact().remove(castToRelatedArtifact(value)); 2898 } else if (name.equals("library")) { 2899 this.getLibrary().remove(castToCanonical(value)); 2900 } else if (name.equals("population")) { 2901 this.population = null; 2902 } else if (name.equals("exposure")) { 2903 this.exposure = null; 2904 } else if (name.equals("exposureAlternative")) { 2905 this.exposureAlternative = null; 2906 } else if (name.equals("outcome")) { 2907 this.outcome = null; 2908 } else 2909 super.removeChild(name, value); 2910 2911 } 2912 2913 @Override 2914 public Base makeProperty(int hash, String name) throws FHIRException { 2915 switch (hash) { 2916 case 116079: 2917 return getUrlElement(); 2918 case -1618432855: 2919 return addIdentifier(); 2920 case 351608024: 2921 return getVersionElement(); 2922 case 3373707: 2923 return getNameElement(); 2924 case 110371416: 2925 return getTitleElement(); 2926 case 1555503932: 2927 return getShortTitleElement(); 2928 case -2060497896: 2929 return getSubtitleElement(); 2930 case -892481550: 2931 return getStatusElement(); 2932 case -404562712: 2933 return getExperimentalElement(); 2934 case -573640748: 2935 return getSubject(); 2936 case -1867885268: 2937 return getSubject(); 2938 case 3076014: 2939 return getDateElement(); 2940 case 1447404028: 2941 return getPublisherElement(); 2942 case 951526432: 2943 return addContact(); 2944 case -1724546052: 2945 return getDescriptionElement(); 2946 case 950398559: 2947 return addCommentElement(); 2948 case -669707736: 2949 return addUseContext(); 2950 case -507075711: 2951 return addJurisdiction(); 2952 case -220463842: 2953 return getPurposeElement(); 2954 case 111574433: 2955 return getUsageElement(); 2956 case 1522889671: 2957 return getCopyrightElement(); 2958 case 223539345: 2959 return getApprovalDateElement(); 2960 case -1687512484: 2961 return getLastReviewDateElement(); 2962 case -403934648: 2963 return getEffectivePeriod(); 2964 case 110546223: 2965 return addTopic(); 2966 case -1406328437: 2967 return addAuthor(); 2968 case -1307827859: 2969 return addEditor(); 2970 case -261190139: 2971 return addReviewer(); 2972 case 1740277666: 2973 return addEndorser(); 2974 case 666807069: 2975 return addRelatedArtifact(); 2976 case 166208699: 2977 return addLibraryElement(); 2978 case -2023558323: 2979 return getPopulation(); 2980 case -1926005497: 2981 return getExposure(); 2982 case -1875462106: 2983 return getExposureAlternative(); 2984 case -1106507950: 2985 return getOutcome(); 2986 default: 2987 return super.makeProperty(hash, name); 2988 } 2989 2990 } 2991 2992 @Override 2993 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2994 switch (hash) { 2995 case 116079: 2996 /* url */ return new String[] { "uri" }; 2997 case -1618432855: 2998 /* identifier */ return new String[] { "Identifier" }; 2999 case 351608024: 3000 /* version */ return new String[] { "string" }; 3001 case 3373707: 3002 /* name */ return new String[] { "string" }; 3003 case 110371416: 3004 /* title */ return new String[] { "string" }; 3005 case 1555503932: 3006 /* shortTitle */ return new String[] { "string" }; 3007 case -2060497896: 3008 /* subtitle */ return new String[] { "string" }; 3009 case -892481550: 3010 /* status */ return new String[] { "code" }; 3011 case -404562712: 3012 /* experimental */ return new String[] { "boolean" }; 3013 case -1867885268: 3014 /* subject */ return new String[] { "CodeableConcept", "Reference" }; 3015 case 3076014: 3016 /* date */ return new String[] { "dateTime" }; 3017 case 1447404028: 3018 /* publisher */ return new String[] { "string" }; 3019 case 951526432: 3020 /* contact */ return new String[] { "ContactDetail" }; 3021 case -1724546052: 3022 /* description */ return new String[] { "markdown" }; 3023 case 950398559: 3024 /* comment */ return new String[] { "string" }; 3025 case -669707736: 3026 /* useContext */ return new String[] { "UsageContext" }; 3027 case -507075711: 3028 /* jurisdiction */ return new String[] { "CodeableConcept" }; 3029 case -220463842: 3030 /* purpose */ return new String[] { "markdown" }; 3031 case 111574433: 3032 /* usage */ return new String[] { "string" }; 3033 case 1522889671: 3034 /* copyright */ return new String[] { "markdown" }; 3035 case 223539345: 3036 /* approvalDate */ return new String[] { "date" }; 3037 case -1687512484: 3038 /* lastReviewDate */ return new String[] { "date" }; 3039 case -403934648: 3040 /* effectivePeriod */ return new String[] { "Period" }; 3041 case 110546223: 3042 /* topic */ return new String[] { "CodeableConcept" }; 3043 case -1406328437: 3044 /* author */ return new String[] { "ContactDetail" }; 3045 case -1307827859: 3046 /* editor */ return new String[] { "ContactDetail" }; 3047 case -261190139: 3048 /* reviewer */ return new String[] { "ContactDetail" }; 3049 case 1740277666: 3050 /* endorser */ return new String[] { "ContactDetail" }; 3051 case 666807069: 3052 /* relatedArtifact */ return new String[] { "RelatedArtifact" }; 3053 case 166208699: 3054 /* library */ return new String[] { "canonical" }; 3055 case -2023558323: 3056 /* population */ return new String[] { "Reference" }; 3057 case -1926005497: 3058 /* exposure */ return new String[] { "Reference" }; 3059 case -1875462106: 3060 /* exposureAlternative */ return new String[] { "Reference" }; 3061 case -1106507950: 3062 /* outcome */ return new String[] { "Reference" }; 3063 default: 3064 return super.getTypesForProperty(hash, name); 3065 } 3066 3067 } 3068 3069 @Override 3070 public Base addChild(String name) throws FHIRException { 3071 if (name.equals("url")) { 3072 throw new FHIRException("Cannot call addChild on a singleton property ResearchDefinition.url"); 3073 } else if (name.equals("identifier")) { 3074 return addIdentifier(); 3075 } else if (name.equals("version")) { 3076 throw new FHIRException("Cannot call addChild on a singleton property ResearchDefinition.version"); 3077 } else if (name.equals("name")) { 3078 throw new FHIRException("Cannot call addChild on a singleton property ResearchDefinition.name"); 3079 } else if (name.equals("title")) { 3080 throw new FHIRException("Cannot call addChild on a singleton property ResearchDefinition.title"); 3081 } else if (name.equals("shortTitle")) { 3082 throw new FHIRException("Cannot call addChild on a singleton property ResearchDefinition.shortTitle"); 3083 } else if (name.equals("subtitle")) { 3084 throw new FHIRException("Cannot call addChild on a singleton property ResearchDefinition.subtitle"); 3085 } else if (name.equals("status")) { 3086 throw new FHIRException("Cannot call addChild on a singleton property ResearchDefinition.status"); 3087 } else if (name.equals("experimental")) { 3088 throw new FHIRException("Cannot call addChild on a singleton property ResearchDefinition.experimental"); 3089 } else if (name.equals("subjectCodeableConcept")) { 3090 this.subject = new CodeableConcept(); 3091 return this.subject; 3092 } else if (name.equals("subjectReference")) { 3093 this.subject = new Reference(); 3094 return this.subject; 3095 } else if (name.equals("date")) { 3096 throw new FHIRException("Cannot call addChild on a singleton property ResearchDefinition.date"); 3097 } else if (name.equals("publisher")) { 3098 throw new FHIRException("Cannot call addChild on a singleton property ResearchDefinition.publisher"); 3099 } else if (name.equals("contact")) { 3100 return addContact(); 3101 } else if (name.equals("description")) { 3102 throw new FHIRException("Cannot call addChild on a singleton property ResearchDefinition.description"); 3103 } else if (name.equals("comment")) { 3104 throw new FHIRException("Cannot call addChild on a singleton property ResearchDefinition.comment"); 3105 } else if (name.equals("useContext")) { 3106 return addUseContext(); 3107 } else if (name.equals("jurisdiction")) { 3108 return addJurisdiction(); 3109 } else if (name.equals("purpose")) { 3110 throw new FHIRException("Cannot call addChild on a singleton property ResearchDefinition.purpose"); 3111 } else if (name.equals("usage")) { 3112 throw new FHIRException("Cannot call addChild on a singleton property ResearchDefinition.usage"); 3113 } else if (name.equals("copyright")) { 3114 throw new FHIRException("Cannot call addChild on a singleton property ResearchDefinition.copyright"); 3115 } else if (name.equals("approvalDate")) { 3116 throw new FHIRException("Cannot call addChild on a singleton property ResearchDefinition.approvalDate"); 3117 } else if (name.equals("lastReviewDate")) { 3118 throw new FHIRException("Cannot call addChild on a singleton property ResearchDefinition.lastReviewDate"); 3119 } else if (name.equals("effectivePeriod")) { 3120 this.effectivePeriod = new Period(); 3121 return this.effectivePeriod; 3122 } else if (name.equals("topic")) { 3123 return addTopic(); 3124 } else if (name.equals("author")) { 3125 return addAuthor(); 3126 } else if (name.equals("editor")) { 3127 return addEditor(); 3128 } else if (name.equals("reviewer")) { 3129 return addReviewer(); 3130 } else if (name.equals("endorser")) { 3131 return addEndorser(); 3132 } else if (name.equals("relatedArtifact")) { 3133 return addRelatedArtifact(); 3134 } else if (name.equals("library")) { 3135 throw new FHIRException("Cannot call addChild on a singleton property ResearchDefinition.library"); 3136 } else if (name.equals("population")) { 3137 this.population = new Reference(); 3138 return this.population; 3139 } else if (name.equals("exposure")) { 3140 this.exposure = new Reference(); 3141 return this.exposure; 3142 } else if (name.equals("exposureAlternative")) { 3143 this.exposureAlternative = new Reference(); 3144 return this.exposureAlternative; 3145 } else if (name.equals("outcome")) { 3146 this.outcome = new Reference(); 3147 return this.outcome; 3148 } else 3149 return super.addChild(name); 3150 } 3151 3152 public String fhirType() { 3153 return "ResearchDefinition"; 3154 3155 } 3156 3157 public ResearchDefinition copy() { 3158 ResearchDefinition dst = new ResearchDefinition(); 3159 copyValues(dst); 3160 return dst; 3161 } 3162 3163 public void copyValues(ResearchDefinition dst) { 3164 super.copyValues(dst); 3165 dst.url = url == null ? null : url.copy(); 3166 if (identifier != null) { 3167 dst.identifier = new ArrayList<Identifier>(); 3168 for (Identifier i : identifier) 3169 dst.identifier.add(i.copy()); 3170 } 3171 ; 3172 dst.version = version == null ? null : version.copy(); 3173 dst.name = name == null ? null : name.copy(); 3174 dst.title = title == null ? null : title.copy(); 3175 dst.shortTitle = shortTitle == null ? null : shortTitle.copy(); 3176 dst.subtitle = subtitle == null ? null : subtitle.copy(); 3177 dst.status = status == null ? null : status.copy(); 3178 dst.experimental = experimental == null ? null : experimental.copy(); 3179 dst.subject = subject == null ? null : subject.copy(); 3180 dst.date = date == null ? null : date.copy(); 3181 dst.publisher = publisher == null ? null : publisher.copy(); 3182 if (contact != null) { 3183 dst.contact = new ArrayList<ContactDetail>(); 3184 for (ContactDetail i : contact) 3185 dst.contact.add(i.copy()); 3186 } 3187 ; 3188 dst.description = description == null ? null : description.copy(); 3189 if (comment != null) { 3190 dst.comment = new ArrayList<StringType>(); 3191 for (StringType i : comment) 3192 dst.comment.add(i.copy()); 3193 } 3194 ; 3195 if (useContext != null) { 3196 dst.useContext = new ArrayList<UsageContext>(); 3197 for (UsageContext i : useContext) 3198 dst.useContext.add(i.copy()); 3199 } 3200 ; 3201 if (jurisdiction != null) { 3202 dst.jurisdiction = new ArrayList<CodeableConcept>(); 3203 for (CodeableConcept i : jurisdiction) 3204 dst.jurisdiction.add(i.copy()); 3205 } 3206 ; 3207 dst.purpose = purpose == null ? null : purpose.copy(); 3208 dst.usage = usage == null ? null : usage.copy(); 3209 dst.copyright = copyright == null ? null : copyright.copy(); 3210 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 3211 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 3212 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 3213 if (topic != null) { 3214 dst.topic = new ArrayList<CodeableConcept>(); 3215 for (CodeableConcept i : topic) 3216 dst.topic.add(i.copy()); 3217 } 3218 ; 3219 if (author != null) { 3220 dst.author = new ArrayList<ContactDetail>(); 3221 for (ContactDetail i : author) 3222 dst.author.add(i.copy()); 3223 } 3224 ; 3225 if (editor != null) { 3226 dst.editor = new ArrayList<ContactDetail>(); 3227 for (ContactDetail i : editor) 3228 dst.editor.add(i.copy()); 3229 } 3230 ; 3231 if (reviewer != null) { 3232 dst.reviewer = new ArrayList<ContactDetail>(); 3233 for (ContactDetail i : reviewer) 3234 dst.reviewer.add(i.copy()); 3235 } 3236 ; 3237 if (endorser != null) { 3238 dst.endorser = new ArrayList<ContactDetail>(); 3239 for (ContactDetail i : endorser) 3240 dst.endorser.add(i.copy()); 3241 } 3242 ; 3243 if (relatedArtifact != null) { 3244 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 3245 for (RelatedArtifact i : relatedArtifact) 3246 dst.relatedArtifact.add(i.copy()); 3247 } 3248 ; 3249 if (library != null) { 3250 dst.library = new ArrayList<CanonicalType>(); 3251 for (CanonicalType i : library) 3252 dst.library.add(i.copy()); 3253 } 3254 ; 3255 dst.population = population == null ? null : population.copy(); 3256 dst.exposure = exposure == null ? null : exposure.copy(); 3257 dst.exposureAlternative = exposureAlternative == null ? null : exposureAlternative.copy(); 3258 dst.outcome = outcome == null ? null : outcome.copy(); 3259 } 3260 3261 protected ResearchDefinition typedCopy() { 3262 return copy(); 3263 } 3264 3265 @Override 3266 public boolean equalsDeep(Base other_) { 3267 if (!super.equalsDeep(other_)) 3268 return false; 3269 if (!(other_ instanceof ResearchDefinition)) 3270 return false; 3271 ResearchDefinition o = (ResearchDefinition) other_; 3272 return compareDeep(identifier, o.identifier, true) && compareDeep(shortTitle, o.shortTitle, true) 3273 && compareDeep(subtitle, o.subtitle, true) && compareDeep(subject, o.subject, true) 3274 && compareDeep(comment, o.comment, true) && compareDeep(purpose, o.purpose, true) 3275 && compareDeep(usage, o.usage, true) && compareDeep(copyright, o.copyright, true) 3276 && compareDeep(approvalDate, o.approvalDate, true) && compareDeep(lastReviewDate, o.lastReviewDate, true) 3277 && compareDeep(effectivePeriod, o.effectivePeriod, true) && compareDeep(topic, o.topic, true) 3278 && compareDeep(author, o.author, true) && compareDeep(editor, o.editor, true) 3279 && compareDeep(reviewer, o.reviewer, true) && compareDeep(endorser, o.endorser, true) 3280 && compareDeep(relatedArtifact, o.relatedArtifact, true) && compareDeep(library, o.library, true) 3281 && compareDeep(population, o.population, true) && compareDeep(exposure, o.exposure, true) 3282 && compareDeep(exposureAlternative, o.exposureAlternative, true) && compareDeep(outcome, o.outcome, true); 3283 } 3284 3285 @Override 3286 public boolean equalsShallow(Base other_) { 3287 if (!super.equalsShallow(other_)) 3288 return false; 3289 if (!(other_ instanceof ResearchDefinition)) 3290 return false; 3291 ResearchDefinition o = (ResearchDefinition) other_; 3292 return compareValues(shortTitle, o.shortTitle, true) && compareValues(subtitle, o.subtitle, true) 3293 && compareValues(comment, o.comment, true) && compareValues(purpose, o.purpose, true) 3294 && compareValues(usage, o.usage, true) && compareValues(copyright, o.copyright, true) 3295 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true); 3296 } 3297 3298 public boolean isEmpty() { 3299 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, shortTitle, subtitle, subject, comment, 3300 purpose, usage, copyright, approvalDate, lastReviewDate, effectivePeriod, topic, author, editor, reviewer, 3301 endorser, relatedArtifact, library, population, exposure, exposureAlternative, outcome); 3302 } 3303 3304 @Override 3305 public ResourceType getResourceType() { 3306 return ResourceType.ResearchDefinition; 3307 } 3308 3309 /** 3310 * Search parameter: <b>date</b> 3311 * <p> 3312 * Description: <b>The research definition publication date</b><br> 3313 * Type: <b>date</b><br> 3314 * Path: <b>ResearchDefinition.date</b><br> 3315 * </p> 3316 */ 3317 @SearchParamDefinition(name = "date", path = "ResearchDefinition.date", description = "The research definition publication date", type = "date") 3318 public static final String SP_DATE = "date"; 3319 /** 3320 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3321 * <p> 3322 * Description: <b>The research definition publication date</b><br> 3323 * Type: <b>date</b><br> 3324 * Path: <b>ResearchDefinition.date</b><br> 3325 * </p> 3326 */ 3327 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3328 SP_DATE); 3329 3330 /** 3331 * Search parameter: <b>identifier</b> 3332 * <p> 3333 * Description: <b>External identifier for the research definition</b><br> 3334 * Type: <b>token</b><br> 3335 * Path: <b>ResearchDefinition.identifier</b><br> 3336 * </p> 3337 */ 3338 @SearchParamDefinition(name = "identifier", path = "ResearchDefinition.identifier", description = "External identifier for the research definition", type = "token") 3339 public static final String SP_IDENTIFIER = "identifier"; 3340 /** 3341 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3342 * <p> 3343 * Description: <b>External identifier for the research definition</b><br> 3344 * Type: <b>token</b><br> 3345 * Path: <b>ResearchDefinition.identifier</b><br> 3346 * </p> 3347 */ 3348 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3349 SP_IDENTIFIER); 3350 3351 /** 3352 * Search parameter: <b>successor</b> 3353 * <p> 3354 * Description: <b>What resource is being referenced</b><br> 3355 * Type: <b>reference</b><br> 3356 * Path: <b>ResearchDefinition.relatedArtifact.resource</b><br> 3357 * </p> 3358 */ 3359 @SearchParamDefinition(name = "successor", path = "ResearchDefinition.relatedArtifact.where(type='successor').resource", description = "What resource is being referenced", type = "reference") 3360 public static final String SP_SUCCESSOR = "successor"; 3361 /** 3362 * <b>Fluent Client</b> search parameter constant for <b>successor</b> 3363 * <p> 3364 * Description: <b>What resource is being referenced</b><br> 3365 * Type: <b>reference</b><br> 3366 * Path: <b>ResearchDefinition.relatedArtifact.resource</b><br> 3367 * </p> 3368 */ 3369 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUCCESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3370 SP_SUCCESSOR); 3371 3372 /** 3373 * Constant for fluent queries to be used to add include statements. Specifies 3374 * the path value of "<b>ResearchDefinition:successor</b>". 3375 */ 3376 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUCCESSOR = new ca.uhn.fhir.model.api.Include( 3377 "ResearchDefinition:successor").toLocked(); 3378 3379 /** 3380 * Search parameter: <b>context-type-value</b> 3381 * <p> 3382 * Description: <b>A use context type and value assigned to the research 3383 * definition</b><br> 3384 * Type: <b>composite</b><br> 3385 * Path: <b></b><br> 3386 * </p> 3387 */ 3388 @SearchParamDefinition(name = "context-type-value", path = "ResearchDefinition.useContext", description = "A use context type and value assigned to the research definition", type = "composite", compositeOf = { 3389 "context-type", "context" }) 3390 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 3391 /** 3392 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 3393 * <p> 3394 * Description: <b>A use context type and value assigned to the research 3395 * definition</b><br> 3396 * Type: <b>composite</b><br> 3397 * Path: <b></b><br> 3398 * </p> 3399 */ 3400 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 3401 SP_CONTEXT_TYPE_VALUE); 3402 3403 /** 3404 * Search parameter: <b>jurisdiction</b> 3405 * <p> 3406 * Description: <b>Intended jurisdiction for the research definition</b><br> 3407 * Type: <b>token</b><br> 3408 * Path: <b>ResearchDefinition.jurisdiction</b><br> 3409 * </p> 3410 */ 3411 @SearchParamDefinition(name = "jurisdiction", path = "ResearchDefinition.jurisdiction", description = "Intended jurisdiction for the research definition", type = "token") 3412 public static final String SP_JURISDICTION = "jurisdiction"; 3413 /** 3414 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 3415 * <p> 3416 * Description: <b>Intended jurisdiction for the research definition</b><br> 3417 * Type: <b>token</b><br> 3418 * Path: <b>ResearchDefinition.jurisdiction</b><br> 3419 * </p> 3420 */ 3421 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3422 SP_JURISDICTION); 3423 3424 /** 3425 * Search parameter: <b>description</b> 3426 * <p> 3427 * Description: <b>The description of the research definition</b><br> 3428 * Type: <b>string</b><br> 3429 * Path: <b>ResearchDefinition.description</b><br> 3430 * </p> 3431 */ 3432 @SearchParamDefinition(name = "description", path = "ResearchDefinition.description", description = "The description of the research definition", type = "string") 3433 public static final String SP_DESCRIPTION = "description"; 3434 /** 3435 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3436 * <p> 3437 * Description: <b>The description of the research definition</b><br> 3438 * Type: <b>string</b><br> 3439 * Path: <b>ResearchDefinition.description</b><br> 3440 * </p> 3441 */ 3442 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 3443 SP_DESCRIPTION); 3444 3445 /** 3446 * Search parameter: <b>derived-from</b> 3447 * <p> 3448 * Description: <b>What resource is being referenced</b><br> 3449 * Type: <b>reference</b><br> 3450 * Path: <b>ResearchDefinition.relatedArtifact.resource</b><br> 3451 * </p> 3452 */ 3453 @SearchParamDefinition(name = "derived-from", path = "ResearchDefinition.relatedArtifact.where(type='derived-from').resource", description = "What resource is being referenced", type = "reference") 3454 public static final String SP_DERIVED_FROM = "derived-from"; 3455 /** 3456 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 3457 * <p> 3458 * Description: <b>What resource is being referenced</b><br> 3459 * Type: <b>reference</b><br> 3460 * Path: <b>ResearchDefinition.relatedArtifact.resource</b><br> 3461 * </p> 3462 */ 3463 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3464 SP_DERIVED_FROM); 3465 3466 /** 3467 * Constant for fluent queries to be used to add include statements. Specifies 3468 * the path value of "<b>ResearchDefinition:derived-from</b>". 3469 */ 3470 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include( 3471 "ResearchDefinition:derived-from").toLocked(); 3472 3473 /** 3474 * Search parameter: <b>context-type</b> 3475 * <p> 3476 * Description: <b>A type of use context assigned to the research 3477 * definition</b><br> 3478 * Type: <b>token</b><br> 3479 * Path: <b>ResearchDefinition.useContext.code</b><br> 3480 * </p> 3481 */ 3482 @SearchParamDefinition(name = "context-type", path = "ResearchDefinition.useContext.code", description = "A type of use context assigned to the research definition", type = "token") 3483 public static final String SP_CONTEXT_TYPE = "context-type"; 3484 /** 3485 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 3486 * <p> 3487 * Description: <b>A type of use context assigned to the research 3488 * definition</b><br> 3489 * Type: <b>token</b><br> 3490 * Path: <b>ResearchDefinition.useContext.code</b><br> 3491 * </p> 3492 */ 3493 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3494 SP_CONTEXT_TYPE); 3495 3496 /** 3497 * Search parameter: <b>predecessor</b> 3498 * <p> 3499 * Description: <b>What resource is being referenced</b><br> 3500 * Type: <b>reference</b><br> 3501 * Path: <b>ResearchDefinition.relatedArtifact.resource</b><br> 3502 * </p> 3503 */ 3504 @SearchParamDefinition(name = "predecessor", path = "ResearchDefinition.relatedArtifact.where(type='predecessor').resource", description = "What resource is being referenced", type = "reference") 3505 public static final String SP_PREDECESSOR = "predecessor"; 3506 /** 3507 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 3508 * <p> 3509 * Description: <b>What resource is being referenced</b><br> 3510 * Type: <b>reference</b><br> 3511 * Path: <b>ResearchDefinition.relatedArtifact.resource</b><br> 3512 * </p> 3513 */ 3514 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3515 SP_PREDECESSOR); 3516 3517 /** 3518 * Constant for fluent queries to be used to add include statements. Specifies 3519 * the path value of "<b>ResearchDefinition:predecessor</b>". 3520 */ 3521 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include( 3522 "ResearchDefinition:predecessor").toLocked(); 3523 3524 /** 3525 * Search parameter: <b>title</b> 3526 * <p> 3527 * Description: <b>The human-friendly name of the research definition</b><br> 3528 * Type: <b>string</b><br> 3529 * Path: <b>ResearchDefinition.title</b><br> 3530 * </p> 3531 */ 3532 @SearchParamDefinition(name = "title", path = "ResearchDefinition.title", description = "The human-friendly name of the research definition", type = "string") 3533 public static final String SP_TITLE = "title"; 3534 /** 3535 * <b>Fluent Client</b> search parameter constant for <b>title</b> 3536 * <p> 3537 * Description: <b>The human-friendly name of the research definition</b><br> 3538 * Type: <b>string</b><br> 3539 * Path: <b>ResearchDefinition.title</b><br> 3540 * </p> 3541 */ 3542 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 3543 SP_TITLE); 3544 3545 /** 3546 * Search parameter: <b>composed-of</b> 3547 * <p> 3548 * Description: <b>What resource is being referenced</b><br> 3549 * Type: <b>reference</b><br> 3550 * Path: <b>ResearchDefinition.relatedArtifact.resource</b><br> 3551 * </p> 3552 */ 3553 @SearchParamDefinition(name = "composed-of", path = "ResearchDefinition.relatedArtifact.where(type='composed-of').resource", description = "What resource is being referenced", type = "reference") 3554 public static final String SP_COMPOSED_OF = "composed-of"; 3555 /** 3556 * <b>Fluent Client</b> search parameter constant for <b>composed-of</b> 3557 * <p> 3558 * Description: <b>What resource is being referenced</b><br> 3559 * Type: <b>reference</b><br> 3560 * Path: <b>ResearchDefinition.relatedArtifact.resource</b><br> 3561 * </p> 3562 */ 3563 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPOSED_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3564 SP_COMPOSED_OF); 3565 3566 /** 3567 * Constant for fluent queries to be used to add include statements. Specifies 3568 * the path value of "<b>ResearchDefinition:composed-of</b>". 3569 */ 3570 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPOSED_OF = new ca.uhn.fhir.model.api.Include( 3571 "ResearchDefinition:composed-of").toLocked(); 3572 3573 /** 3574 * Search parameter: <b>version</b> 3575 * <p> 3576 * Description: <b>The business version of the research definition</b><br> 3577 * Type: <b>token</b><br> 3578 * Path: <b>ResearchDefinition.version</b><br> 3579 * </p> 3580 */ 3581 @SearchParamDefinition(name = "version", path = "ResearchDefinition.version", description = "The business version of the research definition", type = "token") 3582 public static final String SP_VERSION = "version"; 3583 /** 3584 * <b>Fluent Client</b> search parameter constant for <b>version</b> 3585 * <p> 3586 * Description: <b>The business version of the research definition</b><br> 3587 * Type: <b>token</b><br> 3588 * Path: <b>ResearchDefinition.version</b><br> 3589 * </p> 3590 */ 3591 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3592 SP_VERSION); 3593 3594 /** 3595 * Search parameter: <b>url</b> 3596 * <p> 3597 * Description: <b>The uri that identifies the research definition</b><br> 3598 * Type: <b>uri</b><br> 3599 * Path: <b>ResearchDefinition.url</b><br> 3600 * </p> 3601 */ 3602 @SearchParamDefinition(name = "url", path = "ResearchDefinition.url", description = "The uri that identifies the research definition", type = "uri") 3603 public static final String SP_URL = "url"; 3604 /** 3605 * <b>Fluent Client</b> search parameter constant for <b>url</b> 3606 * <p> 3607 * Description: <b>The uri that identifies the research definition</b><br> 3608 * Type: <b>uri</b><br> 3609 * Path: <b>ResearchDefinition.url</b><br> 3610 * </p> 3611 */ 3612 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 3613 3614 /** 3615 * Search parameter: <b>context-quantity</b> 3616 * <p> 3617 * Description: <b>A quantity- or range-valued use context assigned to the 3618 * research definition</b><br> 3619 * Type: <b>quantity</b><br> 3620 * Path: <b>ResearchDefinition.useContext.valueQuantity, 3621 * ResearchDefinition.useContext.valueRange</b><br> 3622 * </p> 3623 */ 3624 @SearchParamDefinition(name = "context-quantity", path = "(ResearchDefinition.useContext.value as Quantity) | (ResearchDefinition.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the research definition", type = "quantity") 3625 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 3626 /** 3627 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 3628 * <p> 3629 * Description: <b>A quantity- or range-valued use context assigned to the 3630 * research definition</b><br> 3631 * Type: <b>quantity</b><br> 3632 * Path: <b>ResearchDefinition.useContext.valueQuantity, 3633 * ResearchDefinition.useContext.valueRange</b><br> 3634 * </p> 3635 */ 3636 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 3637 SP_CONTEXT_QUANTITY); 3638 3639 /** 3640 * Search parameter: <b>effective</b> 3641 * <p> 3642 * Description: <b>The time during which the research definition is intended to 3643 * be in use</b><br> 3644 * Type: <b>date</b><br> 3645 * Path: <b>ResearchDefinition.effectivePeriod</b><br> 3646 * </p> 3647 */ 3648 @SearchParamDefinition(name = "effective", path = "ResearchDefinition.effectivePeriod", description = "The time during which the research definition is intended to be in use", type = "date") 3649 public static final String SP_EFFECTIVE = "effective"; 3650 /** 3651 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 3652 * <p> 3653 * Description: <b>The time during which the research definition is intended to 3654 * be in use</b><br> 3655 * Type: <b>date</b><br> 3656 * Path: <b>ResearchDefinition.effectivePeriod</b><br> 3657 * </p> 3658 */ 3659 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3660 SP_EFFECTIVE); 3661 3662 /** 3663 * Search parameter: <b>depends-on</b> 3664 * <p> 3665 * Description: <b>What resource is being referenced</b><br> 3666 * Type: <b>reference</b><br> 3667 * Path: <b>ResearchDefinition.relatedArtifact.resource, 3668 * ResearchDefinition.library</b><br> 3669 * </p> 3670 */ 3671 @SearchParamDefinition(name = "depends-on", path = "ResearchDefinition.relatedArtifact.where(type='depends-on').resource | ResearchDefinition.library", description = "What resource is being referenced", type = "reference") 3672 public static final String SP_DEPENDS_ON = "depends-on"; 3673 /** 3674 * <b>Fluent Client</b> search parameter constant for <b>depends-on</b> 3675 * <p> 3676 * Description: <b>What resource is being referenced</b><br> 3677 * Type: <b>reference</b><br> 3678 * Path: <b>ResearchDefinition.relatedArtifact.resource, 3679 * ResearchDefinition.library</b><br> 3680 * </p> 3681 */ 3682 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEPENDS_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3683 SP_DEPENDS_ON); 3684 3685 /** 3686 * Constant for fluent queries to be used to add include statements. Specifies 3687 * the path value of "<b>ResearchDefinition:depends-on</b>". 3688 */ 3689 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEPENDS_ON = new ca.uhn.fhir.model.api.Include( 3690 "ResearchDefinition:depends-on").toLocked(); 3691 3692 /** 3693 * Search parameter: <b>name</b> 3694 * <p> 3695 * Description: <b>Computationally friendly name of the research 3696 * definition</b><br> 3697 * Type: <b>string</b><br> 3698 * Path: <b>ResearchDefinition.name</b><br> 3699 * </p> 3700 */ 3701 @SearchParamDefinition(name = "name", path = "ResearchDefinition.name", description = "Computationally friendly name of the research definition", type = "string") 3702 public static final String SP_NAME = "name"; 3703 /** 3704 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3705 * <p> 3706 * Description: <b>Computationally friendly name of the research 3707 * definition</b><br> 3708 * Type: <b>string</b><br> 3709 * Path: <b>ResearchDefinition.name</b><br> 3710 * </p> 3711 */ 3712 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 3713 SP_NAME); 3714 3715 /** 3716 * Search parameter: <b>context</b> 3717 * <p> 3718 * Description: <b>A use context assigned to the research definition</b><br> 3719 * Type: <b>token</b><br> 3720 * Path: <b>ResearchDefinition.useContext.valueCodeableConcept</b><br> 3721 * </p> 3722 */ 3723 @SearchParamDefinition(name = "context", path = "(ResearchDefinition.useContext.value as CodeableConcept)", description = "A use context assigned to the research definition", type = "token") 3724 public static final String SP_CONTEXT = "context"; 3725 /** 3726 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3727 * <p> 3728 * Description: <b>A use context assigned to the research definition</b><br> 3729 * Type: <b>token</b><br> 3730 * Path: <b>ResearchDefinition.useContext.valueCodeableConcept</b><br> 3731 * </p> 3732 */ 3733 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3734 SP_CONTEXT); 3735 3736 /** 3737 * Search parameter: <b>publisher</b> 3738 * <p> 3739 * Description: <b>Name of the publisher of the research definition</b><br> 3740 * Type: <b>string</b><br> 3741 * Path: <b>ResearchDefinition.publisher</b><br> 3742 * </p> 3743 */ 3744 @SearchParamDefinition(name = "publisher", path = "ResearchDefinition.publisher", description = "Name of the publisher of the research definition", type = "string") 3745 public static final String SP_PUBLISHER = "publisher"; 3746 /** 3747 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 3748 * <p> 3749 * Description: <b>Name of the publisher of the research definition</b><br> 3750 * Type: <b>string</b><br> 3751 * Path: <b>ResearchDefinition.publisher</b><br> 3752 * </p> 3753 */ 3754 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 3755 SP_PUBLISHER); 3756 3757 /** 3758 * Search parameter: <b>topic</b> 3759 * <p> 3760 * Description: <b>Topics associated with the ResearchDefinition</b><br> 3761 * Type: <b>token</b><br> 3762 * Path: <b>ResearchDefinition.topic</b><br> 3763 * </p> 3764 */ 3765 @SearchParamDefinition(name = "topic", path = "ResearchDefinition.topic", description = "Topics associated with the ResearchDefinition", type = "token") 3766 public static final String SP_TOPIC = "topic"; 3767 /** 3768 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 3769 * <p> 3770 * Description: <b>Topics associated with the ResearchDefinition</b><br> 3771 * Type: <b>token</b><br> 3772 * Path: <b>ResearchDefinition.topic</b><br> 3773 * </p> 3774 */ 3775 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3776 SP_TOPIC); 3777 3778 /** 3779 * Search parameter: <b>context-type-quantity</b> 3780 * <p> 3781 * Description: <b>A use context type and quantity- or range-based value 3782 * assigned to the research definition</b><br> 3783 * Type: <b>composite</b><br> 3784 * Path: <b></b><br> 3785 * </p> 3786 */ 3787 @SearchParamDefinition(name = "context-type-quantity", path = "ResearchDefinition.useContext", description = "A use context type and quantity- or range-based value assigned to the research definition", type = "composite", compositeOf = { 3788 "context-type", "context-quantity" }) 3789 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 3790 /** 3791 * <b>Fluent Client</b> search parameter constant for 3792 * <b>context-type-quantity</b> 3793 * <p> 3794 * Description: <b>A use context type and quantity- or range-based value 3795 * assigned to the research definition</b><br> 3796 * Type: <b>composite</b><br> 3797 * Path: <b></b><br> 3798 * </p> 3799 */ 3800 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 3801 SP_CONTEXT_TYPE_QUANTITY); 3802 3803 /** 3804 * Search parameter: <b>status</b> 3805 * <p> 3806 * Description: <b>The current status of the research definition</b><br> 3807 * Type: <b>token</b><br> 3808 * Path: <b>ResearchDefinition.status</b><br> 3809 * </p> 3810 */ 3811 @SearchParamDefinition(name = "status", path = "ResearchDefinition.status", description = "The current status of the research definition", type = "token") 3812 public static final String SP_STATUS = "status"; 3813 /** 3814 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3815 * <p> 3816 * Description: <b>The current status of the research definition</b><br> 3817 * Type: <b>token</b><br> 3818 * Path: <b>ResearchDefinition.status</b><br> 3819 * </p> 3820 */ 3821 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3822 SP_STATUS); 3823 3824}