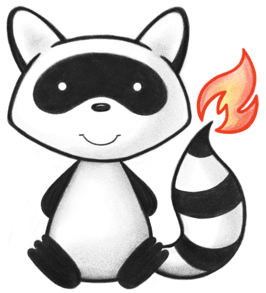
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049 050/** 051 * The ResearchElementDefinition resource describes a "PICO" element that 052 * knowledge (evidence, assertion, recommendation) is about. 053 */ 054@ResourceDef(name = "ResearchElementDefinition", profile = "http://hl7.org/fhir/StructureDefinition/ResearchElementDefinition") 055@ChildOrder(names = { "url", "identifier", "version", "name", "title", "shortTitle", "subtitle", "status", 056 "experimental", "subject[x]", "date", "publisher", "contact", "description", "comment", "useContext", 057 "jurisdiction", "purpose", "usage", "copyright", "approvalDate", "lastReviewDate", "effectivePeriod", "topic", 058 "author", "editor", "reviewer", "endorser", "relatedArtifact", "library", "type", "variableType", 059 "characteristic" }) 060public class ResearchElementDefinition extends MetadataResource { 061 062 public enum ResearchElementType { 063 /** 064 * The element defines the population that forms the basis for research. 065 */ 066 POPULATION, 067 /** 068 * The element defines an exposure within the population that is being 069 * researched. 070 */ 071 EXPOSURE, 072 /** 073 * The element defines an outcome within the population that is being 074 * researched. 075 */ 076 OUTCOME, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 082 public static ResearchElementType fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("population".equals(codeString)) 086 return POPULATION; 087 if ("exposure".equals(codeString)) 088 return EXPOSURE; 089 if ("outcome".equals(codeString)) 090 return OUTCOME; 091 if (Configuration.isAcceptInvalidEnums()) 092 return null; 093 else 094 throw new FHIRException("Unknown ResearchElementType code '" + codeString + "'"); 095 } 096 097 public String toCode() { 098 switch (this) { 099 case POPULATION: 100 return "population"; 101 case EXPOSURE: 102 return "exposure"; 103 case OUTCOME: 104 return "outcome"; 105 case NULL: 106 return null; 107 default: 108 return "?"; 109 } 110 } 111 112 public String getSystem() { 113 switch (this) { 114 case POPULATION: 115 return "http://hl7.org/fhir/research-element-type"; 116 case EXPOSURE: 117 return "http://hl7.org/fhir/research-element-type"; 118 case OUTCOME: 119 return "http://hl7.org/fhir/research-element-type"; 120 case NULL: 121 return null; 122 default: 123 return "?"; 124 } 125 } 126 127 public String getDefinition() { 128 switch (this) { 129 case POPULATION: 130 return "The element defines the population that forms the basis for research."; 131 case EXPOSURE: 132 return "The element defines an exposure within the population that is being researched."; 133 case OUTCOME: 134 return "The element defines an outcome within the population that is being researched."; 135 case NULL: 136 return null; 137 default: 138 return "?"; 139 } 140 } 141 142 public String getDisplay() { 143 switch (this) { 144 case POPULATION: 145 return "Population"; 146 case EXPOSURE: 147 return "Exposure"; 148 case OUTCOME: 149 return "Outcome"; 150 case NULL: 151 return null; 152 default: 153 return "?"; 154 } 155 } 156 } 157 158 public static class ResearchElementTypeEnumFactory implements EnumFactory<ResearchElementType> { 159 public ResearchElementType fromCode(String codeString) throws IllegalArgumentException { 160 if (codeString == null || "".equals(codeString)) 161 if (codeString == null || "".equals(codeString)) 162 return null; 163 if ("population".equals(codeString)) 164 return ResearchElementType.POPULATION; 165 if ("exposure".equals(codeString)) 166 return ResearchElementType.EXPOSURE; 167 if ("outcome".equals(codeString)) 168 return ResearchElementType.OUTCOME; 169 throw new IllegalArgumentException("Unknown ResearchElementType code '" + codeString + "'"); 170 } 171 172 public Enumeration<ResearchElementType> fromType(PrimitiveType<?> code) throws FHIRException { 173 if (code == null) 174 return null; 175 if (code.isEmpty()) 176 return new Enumeration<ResearchElementType>(this, ResearchElementType.NULL, code); 177 String codeString = code.asStringValue(); 178 if (codeString == null || "".equals(codeString)) 179 return new Enumeration<ResearchElementType>(this, ResearchElementType.NULL, code); 180 if ("population".equals(codeString)) 181 return new Enumeration<ResearchElementType>(this, ResearchElementType.POPULATION, code); 182 if ("exposure".equals(codeString)) 183 return new Enumeration<ResearchElementType>(this, ResearchElementType.EXPOSURE, code); 184 if ("outcome".equals(codeString)) 185 return new Enumeration<ResearchElementType>(this, ResearchElementType.OUTCOME, code); 186 throw new FHIRException("Unknown ResearchElementType code '" + codeString + "'"); 187 } 188 189 public String toCode(ResearchElementType code) { 190 if (code == ResearchElementType.NULL) 191 return null; 192 if (code == ResearchElementType.POPULATION) 193 return "population"; 194 if (code == ResearchElementType.EXPOSURE) 195 return "exposure"; 196 if (code == ResearchElementType.OUTCOME) 197 return "outcome"; 198 return "?"; 199 } 200 201 public String toSystem(ResearchElementType code) { 202 return code.getSystem(); 203 } 204 } 205 206 public enum VariableType { 207 /** 208 * The variable is dichotomous, such as present or absent. 209 */ 210 DICHOTOMOUS, 211 /** 212 * The variable is a continuous result such as a quantity. 213 */ 214 CONTINUOUS, 215 /** 216 * The variable is described narratively rather than quantitatively. 217 */ 218 DESCRIPTIVE, 219 /** 220 * added to help the parsers with the generic types 221 */ 222 NULL; 223 224 public static VariableType fromCode(String codeString) throws FHIRException { 225 if (codeString == null || "".equals(codeString)) 226 return null; 227 if ("dichotomous".equals(codeString)) 228 return DICHOTOMOUS; 229 if ("continuous".equals(codeString)) 230 return CONTINUOUS; 231 if ("descriptive".equals(codeString)) 232 return DESCRIPTIVE; 233 if (Configuration.isAcceptInvalidEnums()) 234 return null; 235 else 236 throw new FHIRException("Unknown VariableType code '" + codeString + "'"); 237 } 238 239 public String toCode() { 240 switch (this) { 241 case DICHOTOMOUS: 242 return "dichotomous"; 243 case CONTINUOUS: 244 return "continuous"; 245 case DESCRIPTIVE: 246 return "descriptive"; 247 case NULL: 248 return null; 249 default: 250 return "?"; 251 } 252 } 253 254 public String getSystem() { 255 switch (this) { 256 case DICHOTOMOUS: 257 return "http://hl7.org/fhir/variable-type"; 258 case CONTINUOUS: 259 return "http://hl7.org/fhir/variable-type"; 260 case DESCRIPTIVE: 261 return "http://hl7.org/fhir/variable-type"; 262 case NULL: 263 return null; 264 default: 265 return "?"; 266 } 267 } 268 269 public String getDefinition() { 270 switch (this) { 271 case DICHOTOMOUS: 272 return "The variable is dichotomous, such as present or absent."; 273 case CONTINUOUS: 274 return "The variable is a continuous result such as a quantity."; 275 case DESCRIPTIVE: 276 return "The variable is described narratively rather than quantitatively."; 277 case NULL: 278 return null; 279 default: 280 return "?"; 281 } 282 } 283 284 public String getDisplay() { 285 switch (this) { 286 case DICHOTOMOUS: 287 return "Dichotomous"; 288 case CONTINUOUS: 289 return "Continuous"; 290 case DESCRIPTIVE: 291 return "Descriptive"; 292 case NULL: 293 return null; 294 default: 295 return "?"; 296 } 297 } 298 } 299 300 public static class VariableTypeEnumFactory implements EnumFactory<VariableType> { 301 public VariableType fromCode(String codeString) throws IllegalArgumentException { 302 if (codeString == null || "".equals(codeString)) 303 if (codeString == null || "".equals(codeString)) 304 return null; 305 if ("dichotomous".equals(codeString)) 306 return VariableType.DICHOTOMOUS; 307 if ("continuous".equals(codeString)) 308 return VariableType.CONTINUOUS; 309 if ("descriptive".equals(codeString)) 310 return VariableType.DESCRIPTIVE; 311 throw new IllegalArgumentException("Unknown VariableType code '" + codeString + "'"); 312 } 313 314 public Enumeration<VariableType> fromType(PrimitiveType<?> code) throws FHIRException { 315 if (code == null) 316 return null; 317 if (code.isEmpty()) 318 return new Enumeration<VariableType>(this, VariableType.NULL, code); 319 String codeString = code.asStringValue(); 320 if (codeString == null || "".equals(codeString)) 321 return new Enumeration<VariableType>(this, VariableType.NULL, code); 322 if ("dichotomous".equals(codeString)) 323 return new Enumeration<VariableType>(this, VariableType.DICHOTOMOUS, code); 324 if ("continuous".equals(codeString)) 325 return new Enumeration<VariableType>(this, VariableType.CONTINUOUS, code); 326 if ("descriptive".equals(codeString)) 327 return new Enumeration<VariableType>(this, VariableType.DESCRIPTIVE, code); 328 throw new FHIRException("Unknown VariableType code '" + codeString + "'"); 329 } 330 331 public String toCode(VariableType code) { 332 if (code == VariableType.NULL) 333 return null; 334 if (code == VariableType.DICHOTOMOUS) 335 return "dichotomous"; 336 if (code == VariableType.CONTINUOUS) 337 return "continuous"; 338 if (code == VariableType.DESCRIPTIVE) 339 return "descriptive"; 340 return "?"; 341 } 342 343 public String toSystem(VariableType code) { 344 return code.getSystem(); 345 } 346 } 347 348 public enum GroupMeasure { 349 /** 350 * Aggregated using Mean of participant values. 351 */ 352 MEAN, 353 /** 354 * Aggregated using Median of participant values. 355 */ 356 MEDIAN, 357 /** 358 * Aggregated using Mean of study mean values. 359 */ 360 MEANOFMEAN, 361 /** 362 * Aggregated using Mean of study median values. 363 */ 364 MEANOFMEDIAN, 365 /** 366 * Aggregated using Median of study mean values. 367 */ 368 MEDIANOFMEAN, 369 /** 370 * Aggregated using Median of study median values. 371 */ 372 MEDIANOFMEDIAN, 373 /** 374 * added to help the parsers with the generic types 375 */ 376 NULL; 377 378 public static GroupMeasure fromCode(String codeString) throws FHIRException { 379 if (codeString == null || "".equals(codeString)) 380 return null; 381 if ("mean".equals(codeString)) 382 return MEAN; 383 if ("median".equals(codeString)) 384 return MEDIAN; 385 if ("mean-of-mean".equals(codeString)) 386 return MEANOFMEAN; 387 if ("mean-of-median".equals(codeString)) 388 return MEANOFMEDIAN; 389 if ("median-of-mean".equals(codeString)) 390 return MEDIANOFMEAN; 391 if ("median-of-median".equals(codeString)) 392 return MEDIANOFMEDIAN; 393 if (Configuration.isAcceptInvalidEnums()) 394 return null; 395 else 396 throw new FHIRException("Unknown GroupMeasure code '" + codeString + "'"); 397 } 398 399 public String toCode() { 400 switch (this) { 401 case MEAN: 402 return "mean"; 403 case MEDIAN: 404 return "median"; 405 case MEANOFMEAN: 406 return "mean-of-mean"; 407 case MEANOFMEDIAN: 408 return "mean-of-median"; 409 case MEDIANOFMEAN: 410 return "median-of-mean"; 411 case MEDIANOFMEDIAN: 412 return "median-of-median"; 413 case NULL: 414 return null; 415 default: 416 return "?"; 417 } 418 } 419 420 public String getSystem() { 421 switch (this) { 422 case MEAN: 423 return "http://hl7.org/fhir/group-measure"; 424 case MEDIAN: 425 return "http://hl7.org/fhir/group-measure"; 426 case MEANOFMEAN: 427 return "http://hl7.org/fhir/group-measure"; 428 case MEANOFMEDIAN: 429 return "http://hl7.org/fhir/group-measure"; 430 case MEDIANOFMEAN: 431 return "http://hl7.org/fhir/group-measure"; 432 case MEDIANOFMEDIAN: 433 return "http://hl7.org/fhir/group-measure"; 434 case NULL: 435 return null; 436 default: 437 return "?"; 438 } 439 } 440 441 public String getDefinition() { 442 switch (this) { 443 case MEAN: 444 return "Aggregated using Mean of participant values."; 445 case MEDIAN: 446 return "Aggregated using Median of participant values."; 447 case MEANOFMEAN: 448 return "Aggregated using Mean of study mean values."; 449 case MEANOFMEDIAN: 450 return "Aggregated using Mean of study median values."; 451 case MEDIANOFMEAN: 452 return "Aggregated using Median of study mean values."; 453 case MEDIANOFMEDIAN: 454 return "Aggregated using Median of study median values."; 455 case NULL: 456 return null; 457 default: 458 return "?"; 459 } 460 } 461 462 public String getDisplay() { 463 switch (this) { 464 case MEAN: 465 return "Mean"; 466 case MEDIAN: 467 return "Median"; 468 case MEANOFMEAN: 469 return "Mean of Study Means"; 470 case MEANOFMEDIAN: 471 return "Mean of Study Medins"; 472 case MEDIANOFMEAN: 473 return "Median of Study Means"; 474 case MEDIANOFMEDIAN: 475 return "Median of Study Medians"; 476 case NULL: 477 return null; 478 default: 479 return "?"; 480 } 481 } 482 } 483 484 public static class GroupMeasureEnumFactory implements EnumFactory<GroupMeasure> { 485 public GroupMeasure fromCode(String codeString) throws IllegalArgumentException { 486 if (codeString == null || "".equals(codeString)) 487 if (codeString == null || "".equals(codeString)) 488 return null; 489 if ("mean".equals(codeString)) 490 return GroupMeasure.MEAN; 491 if ("median".equals(codeString)) 492 return GroupMeasure.MEDIAN; 493 if ("mean-of-mean".equals(codeString)) 494 return GroupMeasure.MEANOFMEAN; 495 if ("mean-of-median".equals(codeString)) 496 return GroupMeasure.MEANOFMEDIAN; 497 if ("median-of-mean".equals(codeString)) 498 return GroupMeasure.MEDIANOFMEAN; 499 if ("median-of-median".equals(codeString)) 500 return GroupMeasure.MEDIANOFMEDIAN; 501 throw new IllegalArgumentException("Unknown GroupMeasure code '" + codeString + "'"); 502 } 503 504 public Enumeration<GroupMeasure> fromType(PrimitiveType<?> code) throws FHIRException { 505 if (code == null) 506 return null; 507 if (code.isEmpty()) 508 return new Enumeration<GroupMeasure>(this, GroupMeasure.NULL, code); 509 String codeString = code.asStringValue(); 510 if (codeString == null || "".equals(codeString)) 511 return new Enumeration<GroupMeasure>(this, GroupMeasure.NULL, code); 512 if ("mean".equals(codeString)) 513 return new Enumeration<GroupMeasure>(this, GroupMeasure.MEAN, code); 514 if ("median".equals(codeString)) 515 return new Enumeration<GroupMeasure>(this, GroupMeasure.MEDIAN, code); 516 if ("mean-of-mean".equals(codeString)) 517 return new Enumeration<GroupMeasure>(this, GroupMeasure.MEANOFMEAN, code); 518 if ("mean-of-median".equals(codeString)) 519 return new Enumeration<GroupMeasure>(this, GroupMeasure.MEANOFMEDIAN, code); 520 if ("median-of-mean".equals(codeString)) 521 return new Enumeration<GroupMeasure>(this, GroupMeasure.MEDIANOFMEAN, code); 522 if ("median-of-median".equals(codeString)) 523 return new Enumeration<GroupMeasure>(this, GroupMeasure.MEDIANOFMEDIAN, code); 524 throw new FHIRException("Unknown GroupMeasure code '" + codeString + "'"); 525 } 526 527 public String toCode(GroupMeasure code) { 528 if (code == GroupMeasure.NULL) 529 return null; 530 if (code == GroupMeasure.MEAN) 531 return "mean"; 532 if (code == GroupMeasure.MEDIAN) 533 return "median"; 534 if (code == GroupMeasure.MEANOFMEAN) 535 return "mean-of-mean"; 536 if (code == GroupMeasure.MEANOFMEDIAN) 537 return "mean-of-median"; 538 if (code == GroupMeasure.MEDIANOFMEAN) 539 return "median-of-mean"; 540 if (code == GroupMeasure.MEDIANOFMEDIAN) 541 return "median-of-median"; 542 return "?"; 543 } 544 545 public String toSystem(GroupMeasure code) { 546 return code.getSystem(); 547 } 548 } 549 550 @Block() 551 public static class ResearchElementDefinitionCharacteristicComponent extends BackboneElement 552 implements IBaseBackboneElement { 553 /** 554 * Define members of the research element using Codes (such as condition, 555 * medication, or observation), Expressions ( using an expression language such 556 * as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in 557 * the last year). 558 */ 559 @Child(name = "definition", type = { CodeableConcept.class, CanonicalType.class, Expression.class, 560 DataRequirement.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 561 @Description(shortDefinition = "What code or expression defines members?", formalDefinition = "Define members of the research element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).") 562 protected Type definition; 563 564 /** 565 * Use UsageContext to define the members of the population, such as Age Ranges, 566 * Genders, Settings. 567 */ 568 @Child(name = "usageContext", type = { 569 UsageContext.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 570 @Description(shortDefinition = "What code/value pairs define members?", formalDefinition = "Use UsageContext to define the members of the population, such as Age Ranges, Genders, Settings.") 571 protected List<UsageContext> usageContext; 572 573 /** 574 * When true, members with this characteristic are excluded from the element. 575 */ 576 @Child(name = "exclude", type = { 577 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 578 @Description(shortDefinition = "Whether the characteristic includes or excludes members", formalDefinition = "When true, members with this characteristic are excluded from the element.") 579 protected BooleanType exclude; 580 581 /** 582 * Specifies the UCUM unit for the outcome. 583 */ 584 @Child(name = "unitOfMeasure", type = { 585 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 586 @Description(shortDefinition = "What unit is the outcome described in?", formalDefinition = "Specifies the UCUM unit for the outcome.") 587 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ucum-units") 588 protected CodeableConcept unitOfMeasure; 589 590 /** 591 * A narrative description of the time period the study covers. 592 */ 593 @Child(name = "studyEffectiveDescription", type = { 594 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 595 @Description(shortDefinition = "What time period does the study cover", formalDefinition = "A narrative description of the time period the study covers.") 596 protected StringType studyEffectiveDescription; 597 598 /** 599 * Indicates what effective period the study covers. 600 */ 601 @Child(name = "studyEffective", type = { DateTimeType.class, Period.class, Duration.class, 602 Timing.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 603 @Description(shortDefinition = "What time period does the study cover", formalDefinition = "Indicates what effective period the study covers.") 604 protected Type studyEffective; 605 606 /** 607 * Indicates duration from the study initiation. 608 */ 609 @Child(name = "studyEffectiveTimeFromStart", type = { 610 Duration.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 611 @Description(shortDefinition = "Observation time from study start", formalDefinition = "Indicates duration from the study initiation.") 612 protected Duration studyEffectiveTimeFromStart; 613 614 /** 615 * Indicates how elements are aggregated within the study effective period. 616 */ 617 @Child(name = "studyEffectiveGroupMeasure", type = { 618 CodeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 619 @Description(shortDefinition = "mean | median | mean-of-mean | mean-of-median | median-of-mean | median-of-median", formalDefinition = "Indicates how elements are aggregated within the study effective period.") 620 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/group-measure") 621 protected Enumeration<GroupMeasure> studyEffectiveGroupMeasure; 622 623 /** 624 * A narrative description of the time period the study covers. 625 */ 626 @Child(name = "participantEffectiveDescription", type = { 627 StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 628 @Description(shortDefinition = "What time period do participants cover", formalDefinition = "A narrative description of the time period the study covers.") 629 protected StringType participantEffectiveDescription; 630 631 /** 632 * Indicates what effective period the study covers. 633 */ 634 @Child(name = "participantEffective", type = { DateTimeType.class, Period.class, Duration.class, 635 Timing.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 636 @Description(shortDefinition = "What time period do participants cover", formalDefinition = "Indicates what effective period the study covers.") 637 protected Type participantEffective; 638 639 /** 640 * Indicates duration from the participant's study entry. 641 */ 642 @Child(name = "participantEffectiveTimeFromStart", type = { 643 Duration.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 644 @Description(shortDefinition = "Observation time from study start", formalDefinition = "Indicates duration from the participant's study entry.") 645 protected Duration participantEffectiveTimeFromStart; 646 647 /** 648 * Indicates how elements are aggregated within the study effective period. 649 */ 650 @Child(name = "participantEffectiveGroupMeasure", type = { 651 CodeType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 652 @Description(shortDefinition = "mean | median | mean-of-mean | mean-of-median | median-of-mean | median-of-median", formalDefinition = "Indicates how elements are aggregated within the study effective period.") 653 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/group-measure") 654 protected Enumeration<GroupMeasure> participantEffectiveGroupMeasure; 655 656 private static final long serialVersionUID = -1102952665L; 657 658 /** 659 * Constructor 660 */ 661 public ResearchElementDefinitionCharacteristicComponent() { 662 super(); 663 } 664 665 /** 666 * Constructor 667 */ 668 public ResearchElementDefinitionCharacteristicComponent(Type definition) { 669 super(); 670 this.definition = definition; 671 } 672 673 /** 674 * @return {@link #definition} (Define members of the research element using 675 * Codes (such as condition, medication, or observation), Expressions ( 676 * using an expression language such as FHIRPath or CQL) or 677 * DataRequirements (such as Diabetes diagnosis onset in the last 678 * year).) 679 */ 680 public Type getDefinition() { 681 return this.definition; 682 } 683 684 /** 685 * @return {@link #definition} (Define members of the research element using 686 * Codes (such as condition, medication, or observation), Expressions ( 687 * using an expression language such as FHIRPath or CQL) or 688 * DataRequirements (such as Diabetes diagnosis onset in the last 689 * year).) 690 */ 691 public CodeableConcept getDefinitionCodeableConcept() throws FHIRException { 692 if (this.definition == null) 693 this.definition = new CodeableConcept(); 694 if (!(this.definition instanceof CodeableConcept)) 695 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 696 + this.definition.getClass().getName() + " was encountered"); 697 return (CodeableConcept) this.definition; 698 } 699 700 public boolean hasDefinitionCodeableConcept() { 701 return this != null && this.definition instanceof CodeableConcept; 702 } 703 704 /** 705 * @return {@link #definition} (Define members of the research element using 706 * Codes (such as condition, medication, or observation), Expressions ( 707 * using an expression language such as FHIRPath or CQL) or 708 * DataRequirements (such as Diabetes diagnosis onset in the last 709 * year).) 710 */ 711 public CanonicalType getDefinitionCanonicalType() throws FHIRException { 712 if (this.definition == null) 713 this.definition = new CanonicalType(); 714 if (!(this.definition instanceof CanonicalType)) 715 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but " 716 + this.definition.getClass().getName() + " was encountered"); 717 return (CanonicalType) this.definition; 718 } 719 720 public boolean hasDefinitionCanonicalType() { 721 return this != null && this.definition instanceof CanonicalType; 722 } 723 724 /** 725 * @return {@link #definition} (Define members of the research element using 726 * Codes (such as condition, medication, or observation), Expressions ( 727 * using an expression language such as FHIRPath or CQL) or 728 * DataRequirements (such as Diabetes diagnosis onset in the last 729 * year).) 730 */ 731 public Expression getDefinitionExpression() throws FHIRException { 732 if (this.definition == null) 733 this.definition = new Expression(); 734 if (!(this.definition instanceof Expression)) 735 throw new FHIRException("Type mismatch: the type Expression was expected, but " 736 + this.definition.getClass().getName() + " was encountered"); 737 return (Expression) this.definition; 738 } 739 740 public boolean hasDefinitionExpression() { 741 return this != null && this.definition instanceof Expression; 742 } 743 744 /** 745 * @return {@link #definition} (Define members of the research element using 746 * Codes (such as condition, medication, or observation), Expressions ( 747 * using an expression language such as FHIRPath or CQL) or 748 * DataRequirements (such as Diabetes diagnosis onset in the last 749 * year).) 750 */ 751 public DataRequirement getDefinitionDataRequirement() throws FHIRException { 752 if (this.definition == null) 753 this.definition = new DataRequirement(); 754 if (!(this.definition instanceof DataRequirement)) 755 throw new FHIRException("Type mismatch: the type DataRequirement was expected, but " 756 + this.definition.getClass().getName() + " was encountered"); 757 return (DataRequirement) this.definition; 758 } 759 760 public boolean hasDefinitionDataRequirement() { 761 return this != null && this.definition instanceof DataRequirement; 762 } 763 764 public boolean hasDefinition() { 765 return this.definition != null && !this.definition.isEmpty(); 766 } 767 768 /** 769 * @param value {@link #definition} (Define members of the research element 770 * using Codes (such as condition, medication, or observation), 771 * Expressions ( using an expression language such as FHIRPath or 772 * CQL) or DataRequirements (such as Diabetes diagnosis onset in 773 * the last year).) 774 */ 775 public ResearchElementDefinitionCharacteristicComponent setDefinition(Type value) { 776 if (value != null && !(value instanceof CodeableConcept || value instanceof CanonicalType 777 || value instanceof Expression || value instanceof DataRequirement)) 778 throw new Error( 779 "Not the right type for ResearchElementDefinition.characteristic.definition[x]: " + value.fhirType()); 780 this.definition = value; 781 return this; 782 } 783 784 /** 785 * @return {@link #usageContext} (Use UsageContext to define the members of the 786 * population, such as Age Ranges, Genders, Settings.) 787 */ 788 public List<UsageContext> getUsageContext() { 789 if (this.usageContext == null) 790 this.usageContext = new ArrayList<UsageContext>(); 791 return this.usageContext; 792 } 793 794 /** 795 * @return Returns a reference to <code>this</code> for easy method chaining 796 */ 797 public ResearchElementDefinitionCharacteristicComponent setUsageContext(List<UsageContext> theUsageContext) { 798 this.usageContext = theUsageContext; 799 return this; 800 } 801 802 public boolean hasUsageContext() { 803 if (this.usageContext == null) 804 return false; 805 for (UsageContext item : this.usageContext) 806 if (!item.isEmpty()) 807 return true; 808 return false; 809 } 810 811 public UsageContext addUsageContext() { // 3 812 UsageContext t = new UsageContext(); 813 if (this.usageContext == null) 814 this.usageContext = new ArrayList<UsageContext>(); 815 this.usageContext.add(t); 816 return t; 817 } 818 819 public ResearchElementDefinitionCharacteristicComponent addUsageContext(UsageContext t) { // 3 820 if (t == null) 821 return this; 822 if (this.usageContext == null) 823 this.usageContext = new ArrayList<UsageContext>(); 824 this.usageContext.add(t); 825 return this; 826 } 827 828 /** 829 * @return The first repetition of repeating field {@link #usageContext}, 830 * creating it if it does not already exist 831 */ 832 public UsageContext getUsageContextFirstRep() { 833 if (getUsageContext().isEmpty()) { 834 addUsageContext(); 835 } 836 return getUsageContext().get(0); 837 } 838 839 /** 840 * @return {@link #exclude} (When true, members with this characteristic are 841 * excluded from the element.). This is the underlying object with id, 842 * value and extensions. The accessor "getExclude" gives direct access 843 * to the value 844 */ 845 public BooleanType getExcludeElement() { 846 if (this.exclude == null) 847 if (Configuration.errorOnAutoCreate()) 848 throw new Error("Attempt to auto-create ResearchElementDefinitionCharacteristicComponent.exclude"); 849 else if (Configuration.doAutoCreate()) 850 this.exclude = new BooleanType(); // bb 851 return this.exclude; 852 } 853 854 public boolean hasExcludeElement() { 855 return this.exclude != null && !this.exclude.isEmpty(); 856 } 857 858 public boolean hasExclude() { 859 return this.exclude != null && !this.exclude.isEmpty(); 860 } 861 862 /** 863 * @param value {@link #exclude} (When true, members with this characteristic 864 * are excluded from the element.). This is the underlying object 865 * with id, value and extensions. The accessor "getExclude" gives 866 * direct access to the value 867 */ 868 public ResearchElementDefinitionCharacteristicComponent setExcludeElement(BooleanType value) { 869 this.exclude = value; 870 return this; 871 } 872 873 /** 874 * @return When true, members with this characteristic are excluded from the 875 * element. 876 */ 877 public boolean getExclude() { 878 return this.exclude == null || this.exclude.isEmpty() ? false : this.exclude.getValue(); 879 } 880 881 /** 882 * @param value When true, members with this characteristic are excluded from 883 * the element. 884 */ 885 public ResearchElementDefinitionCharacteristicComponent setExclude(boolean value) { 886 if (this.exclude == null) 887 this.exclude = new BooleanType(); 888 this.exclude.setValue(value); 889 return this; 890 } 891 892 /** 893 * @return {@link #unitOfMeasure} (Specifies the UCUM unit for the outcome.) 894 */ 895 public CodeableConcept getUnitOfMeasure() { 896 if (this.unitOfMeasure == null) 897 if (Configuration.errorOnAutoCreate()) 898 throw new Error("Attempt to auto-create ResearchElementDefinitionCharacteristicComponent.unitOfMeasure"); 899 else if (Configuration.doAutoCreate()) 900 this.unitOfMeasure = new CodeableConcept(); // cc 901 return this.unitOfMeasure; 902 } 903 904 public boolean hasUnitOfMeasure() { 905 return this.unitOfMeasure != null && !this.unitOfMeasure.isEmpty(); 906 } 907 908 /** 909 * @param value {@link #unitOfMeasure} (Specifies the UCUM unit for the 910 * outcome.) 911 */ 912 public ResearchElementDefinitionCharacteristicComponent setUnitOfMeasure(CodeableConcept value) { 913 this.unitOfMeasure = value; 914 return this; 915 } 916 917 /** 918 * @return {@link #studyEffectiveDescription} (A narrative description of the 919 * time period the study covers.). This is the underlying object with 920 * id, value and extensions. The accessor "getStudyEffectiveDescription" 921 * gives direct access to the value 922 */ 923 public StringType getStudyEffectiveDescriptionElement() { 924 if (this.studyEffectiveDescription == null) 925 if (Configuration.errorOnAutoCreate()) 926 throw new Error( 927 "Attempt to auto-create ResearchElementDefinitionCharacteristicComponent.studyEffectiveDescription"); 928 else if (Configuration.doAutoCreate()) 929 this.studyEffectiveDescription = new StringType(); // bb 930 return this.studyEffectiveDescription; 931 } 932 933 public boolean hasStudyEffectiveDescriptionElement() { 934 return this.studyEffectiveDescription != null && !this.studyEffectiveDescription.isEmpty(); 935 } 936 937 public boolean hasStudyEffectiveDescription() { 938 return this.studyEffectiveDescription != null && !this.studyEffectiveDescription.isEmpty(); 939 } 940 941 /** 942 * @param value {@link #studyEffectiveDescription} (A narrative description of 943 * the time period the study covers.). This is the underlying 944 * object with id, value and extensions. The accessor 945 * "getStudyEffectiveDescription" gives direct access to the value 946 */ 947 public ResearchElementDefinitionCharacteristicComponent setStudyEffectiveDescriptionElement(StringType value) { 948 this.studyEffectiveDescription = value; 949 return this; 950 } 951 952 /** 953 * @return A narrative description of the time period the study covers. 954 */ 955 public String getStudyEffectiveDescription() { 956 return this.studyEffectiveDescription == null ? null : this.studyEffectiveDescription.getValue(); 957 } 958 959 /** 960 * @param value A narrative description of the time period the study covers. 961 */ 962 public ResearchElementDefinitionCharacteristicComponent setStudyEffectiveDescription(String value) { 963 if (Utilities.noString(value)) 964 this.studyEffectiveDescription = null; 965 else { 966 if (this.studyEffectiveDescription == null) 967 this.studyEffectiveDescription = new StringType(); 968 this.studyEffectiveDescription.setValue(value); 969 } 970 return this; 971 } 972 973 /** 974 * @return {@link #studyEffective} (Indicates what effective period the study 975 * covers.) 976 */ 977 public Type getStudyEffective() { 978 return this.studyEffective; 979 } 980 981 /** 982 * @return {@link #studyEffective} (Indicates what effective period the study 983 * covers.) 984 */ 985 public DateTimeType getStudyEffectiveDateTimeType() throws FHIRException { 986 if (this.studyEffective == null) 987 this.studyEffective = new DateTimeType(); 988 if (!(this.studyEffective instanceof DateTimeType)) 989 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 990 + this.studyEffective.getClass().getName() + " was encountered"); 991 return (DateTimeType) this.studyEffective; 992 } 993 994 public boolean hasStudyEffectiveDateTimeType() { 995 return this != null && this.studyEffective instanceof DateTimeType; 996 } 997 998 /** 999 * @return {@link #studyEffective} (Indicates what effective period the study 1000 * covers.) 1001 */ 1002 public Period getStudyEffectivePeriod() throws FHIRException { 1003 if (this.studyEffective == null) 1004 this.studyEffective = new Period(); 1005 if (!(this.studyEffective instanceof Period)) 1006 throw new FHIRException("Type mismatch: the type Period was expected, but " 1007 + this.studyEffective.getClass().getName() + " was encountered"); 1008 return (Period) this.studyEffective; 1009 } 1010 1011 public boolean hasStudyEffectivePeriod() { 1012 return this != null && this.studyEffective instanceof Period; 1013 } 1014 1015 /** 1016 * @return {@link #studyEffective} (Indicates what effective period the study 1017 * covers.) 1018 */ 1019 public Duration getStudyEffectiveDuration() throws FHIRException { 1020 if (this.studyEffective == null) 1021 this.studyEffective = new Duration(); 1022 if (!(this.studyEffective instanceof Duration)) 1023 throw new FHIRException("Type mismatch: the type Duration was expected, but " 1024 + this.studyEffective.getClass().getName() + " was encountered"); 1025 return (Duration) this.studyEffective; 1026 } 1027 1028 public boolean hasStudyEffectiveDuration() { 1029 return this != null && this.studyEffective instanceof Duration; 1030 } 1031 1032 /** 1033 * @return {@link #studyEffective} (Indicates what effective period the study 1034 * covers.) 1035 */ 1036 public Timing getStudyEffectiveTiming() throws FHIRException { 1037 if (this.studyEffective == null) 1038 this.studyEffective = new Timing(); 1039 if (!(this.studyEffective instanceof Timing)) 1040 throw new FHIRException("Type mismatch: the type Timing was expected, but " 1041 + this.studyEffective.getClass().getName() + " was encountered"); 1042 return (Timing) this.studyEffective; 1043 } 1044 1045 public boolean hasStudyEffectiveTiming() { 1046 return this != null && this.studyEffective instanceof Timing; 1047 } 1048 1049 public boolean hasStudyEffective() { 1050 return this.studyEffective != null && !this.studyEffective.isEmpty(); 1051 } 1052 1053 /** 1054 * @param value {@link #studyEffective} (Indicates what effective period the 1055 * study covers.) 1056 */ 1057 public ResearchElementDefinitionCharacteristicComponent setStudyEffective(Type value) { 1058 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Duration 1059 || value instanceof Timing)) 1060 throw new Error( 1061 "Not the right type for ResearchElementDefinition.characteristic.studyEffective[x]: " + value.fhirType()); 1062 this.studyEffective = value; 1063 return this; 1064 } 1065 1066 /** 1067 * @return {@link #studyEffectiveTimeFromStart} (Indicates duration from the 1068 * study initiation.) 1069 */ 1070 public Duration getStudyEffectiveTimeFromStart() { 1071 if (this.studyEffectiveTimeFromStart == null) 1072 if (Configuration.errorOnAutoCreate()) 1073 throw new Error( 1074 "Attempt to auto-create ResearchElementDefinitionCharacteristicComponent.studyEffectiveTimeFromStart"); 1075 else if (Configuration.doAutoCreate()) 1076 this.studyEffectiveTimeFromStart = new Duration(); // cc 1077 return this.studyEffectiveTimeFromStart; 1078 } 1079 1080 public boolean hasStudyEffectiveTimeFromStart() { 1081 return this.studyEffectiveTimeFromStart != null && !this.studyEffectiveTimeFromStart.isEmpty(); 1082 } 1083 1084 /** 1085 * @param value {@link #studyEffectiveTimeFromStart} (Indicates duration from 1086 * the study initiation.) 1087 */ 1088 public ResearchElementDefinitionCharacteristicComponent setStudyEffectiveTimeFromStart(Duration value) { 1089 this.studyEffectiveTimeFromStart = value; 1090 return this; 1091 } 1092 1093 /** 1094 * @return {@link #studyEffectiveGroupMeasure} (Indicates how elements are 1095 * aggregated within the study effective period.). This is the 1096 * underlying object with id, value and extensions. The accessor 1097 * "getStudyEffectiveGroupMeasure" gives direct access to the value 1098 */ 1099 public Enumeration<GroupMeasure> getStudyEffectiveGroupMeasureElement() { 1100 if (this.studyEffectiveGroupMeasure == null) 1101 if (Configuration.errorOnAutoCreate()) 1102 throw new Error( 1103 "Attempt to auto-create ResearchElementDefinitionCharacteristicComponent.studyEffectiveGroupMeasure"); 1104 else if (Configuration.doAutoCreate()) 1105 this.studyEffectiveGroupMeasure = new Enumeration<GroupMeasure>(new GroupMeasureEnumFactory()); // bb 1106 return this.studyEffectiveGroupMeasure; 1107 } 1108 1109 public boolean hasStudyEffectiveGroupMeasureElement() { 1110 return this.studyEffectiveGroupMeasure != null && !this.studyEffectiveGroupMeasure.isEmpty(); 1111 } 1112 1113 public boolean hasStudyEffectiveGroupMeasure() { 1114 return this.studyEffectiveGroupMeasure != null && !this.studyEffectiveGroupMeasure.isEmpty(); 1115 } 1116 1117 /** 1118 * @param value {@link #studyEffectiveGroupMeasure} (Indicates how elements are 1119 * aggregated within the study effective period.). This is the 1120 * underlying object with id, value and extensions. The accessor 1121 * "getStudyEffectiveGroupMeasure" gives direct access to the value 1122 */ 1123 public ResearchElementDefinitionCharacteristicComponent setStudyEffectiveGroupMeasureElement( 1124 Enumeration<GroupMeasure> value) { 1125 this.studyEffectiveGroupMeasure = value; 1126 return this; 1127 } 1128 1129 /** 1130 * @return Indicates how elements are aggregated within the study effective 1131 * period. 1132 */ 1133 public GroupMeasure getStudyEffectiveGroupMeasure() { 1134 return this.studyEffectiveGroupMeasure == null ? null : this.studyEffectiveGroupMeasure.getValue(); 1135 } 1136 1137 /** 1138 * @param value Indicates how elements are aggregated within the study effective 1139 * period. 1140 */ 1141 public ResearchElementDefinitionCharacteristicComponent setStudyEffectiveGroupMeasure(GroupMeasure value) { 1142 if (value == null) 1143 this.studyEffectiveGroupMeasure = null; 1144 else { 1145 if (this.studyEffectiveGroupMeasure == null) 1146 this.studyEffectiveGroupMeasure = new Enumeration<GroupMeasure>(new GroupMeasureEnumFactory()); 1147 this.studyEffectiveGroupMeasure.setValue(value); 1148 } 1149 return this; 1150 } 1151 1152 /** 1153 * @return {@link #participantEffectiveDescription} (A narrative description of 1154 * the time period the study covers.). This is the underlying object 1155 * with id, value and extensions. The accessor 1156 * "getParticipantEffectiveDescription" gives direct access to the value 1157 */ 1158 public StringType getParticipantEffectiveDescriptionElement() { 1159 if (this.participantEffectiveDescription == null) 1160 if (Configuration.errorOnAutoCreate()) 1161 throw new Error( 1162 "Attempt to auto-create ResearchElementDefinitionCharacteristicComponent.participantEffectiveDescription"); 1163 else if (Configuration.doAutoCreate()) 1164 this.participantEffectiveDescription = new StringType(); // bb 1165 return this.participantEffectiveDescription; 1166 } 1167 1168 public boolean hasParticipantEffectiveDescriptionElement() { 1169 return this.participantEffectiveDescription != null && !this.participantEffectiveDescription.isEmpty(); 1170 } 1171 1172 public boolean hasParticipantEffectiveDescription() { 1173 return this.participantEffectiveDescription != null && !this.participantEffectiveDescription.isEmpty(); 1174 } 1175 1176 /** 1177 * @param value {@link #participantEffectiveDescription} (A narrative 1178 * description of the time period the study covers.). This is the 1179 * underlying object with id, value and extensions. The accessor 1180 * "getParticipantEffectiveDescription" gives direct access to the 1181 * value 1182 */ 1183 public ResearchElementDefinitionCharacteristicComponent setParticipantEffectiveDescriptionElement( 1184 StringType value) { 1185 this.participantEffectiveDescription = value; 1186 return this; 1187 } 1188 1189 /** 1190 * @return A narrative description of the time period the study covers. 1191 */ 1192 public String getParticipantEffectiveDescription() { 1193 return this.participantEffectiveDescription == null ? null : this.participantEffectiveDescription.getValue(); 1194 } 1195 1196 /** 1197 * @param value A narrative description of the time period the study covers. 1198 */ 1199 public ResearchElementDefinitionCharacteristicComponent setParticipantEffectiveDescription(String value) { 1200 if (Utilities.noString(value)) 1201 this.participantEffectiveDescription = null; 1202 else { 1203 if (this.participantEffectiveDescription == null) 1204 this.participantEffectiveDescription = new StringType(); 1205 this.participantEffectiveDescription.setValue(value); 1206 } 1207 return this; 1208 } 1209 1210 /** 1211 * @return {@link #participantEffective} (Indicates what effective period the 1212 * study covers.) 1213 */ 1214 public Type getParticipantEffective() { 1215 return this.participantEffective; 1216 } 1217 1218 /** 1219 * @return {@link #participantEffective} (Indicates what effective period the 1220 * study covers.) 1221 */ 1222 public DateTimeType getParticipantEffectiveDateTimeType() throws FHIRException { 1223 if (this.participantEffective == null) 1224 this.participantEffective = new DateTimeType(); 1225 if (!(this.participantEffective instanceof DateTimeType)) 1226 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1227 + this.participantEffective.getClass().getName() + " was encountered"); 1228 return (DateTimeType) this.participantEffective; 1229 } 1230 1231 public boolean hasParticipantEffectiveDateTimeType() { 1232 return this != null && this.participantEffective instanceof DateTimeType; 1233 } 1234 1235 /** 1236 * @return {@link #participantEffective} (Indicates what effective period the 1237 * study covers.) 1238 */ 1239 public Period getParticipantEffectivePeriod() throws FHIRException { 1240 if (this.participantEffective == null) 1241 this.participantEffective = new Period(); 1242 if (!(this.participantEffective instanceof Period)) 1243 throw new FHIRException("Type mismatch: the type Period was expected, but " 1244 + this.participantEffective.getClass().getName() + " was encountered"); 1245 return (Period) this.participantEffective; 1246 } 1247 1248 public boolean hasParticipantEffectivePeriod() { 1249 return this != null && this.participantEffective instanceof Period; 1250 } 1251 1252 /** 1253 * @return {@link #participantEffective} (Indicates what effective period the 1254 * study covers.) 1255 */ 1256 public Duration getParticipantEffectiveDuration() throws FHIRException { 1257 if (this.participantEffective == null) 1258 this.participantEffective = new Duration(); 1259 if (!(this.participantEffective instanceof Duration)) 1260 throw new FHIRException("Type mismatch: the type Duration was expected, but " 1261 + this.participantEffective.getClass().getName() + " was encountered"); 1262 return (Duration) this.participantEffective; 1263 } 1264 1265 public boolean hasParticipantEffectiveDuration() { 1266 return this != null && this.participantEffective instanceof Duration; 1267 } 1268 1269 /** 1270 * @return {@link #participantEffective} (Indicates what effective period the 1271 * study covers.) 1272 */ 1273 public Timing getParticipantEffectiveTiming() throws FHIRException { 1274 if (this.participantEffective == null) 1275 this.participantEffective = new Timing(); 1276 if (!(this.participantEffective instanceof Timing)) 1277 throw new FHIRException("Type mismatch: the type Timing was expected, but " 1278 + this.participantEffective.getClass().getName() + " was encountered"); 1279 return (Timing) this.participantEffective; 1280 } 1281 1282 public boolean hasParticipantEffectiveTiming() { 1283 return this != null && this.participantEffective instanceof Timing; 1284 } 1285 1286 public boolean hasParticipantEffective() { 1287 return this.participantEffective != null && !this.participantEffective.isEmpty(); 1288 } 1289 1290 /** 1291 * @param value {@link #participantEffective} (Indicates what effective period 1292 * the study covers.) 1293 */ 1294 public ResearchElementDefinitionCharacteristicComponent setParticipantEffective(Type value) { 1295 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Duration 1296 || value instanceof Timing)) 1297 throw new Error("Not the right type for ResearchElementDefinition.characteristic.participantEffective[x]: " 1298 + value.fhirType()); 1299 this.participantEffective = value; 1300 return this; 1301 } 1302 1303 /** 1304 * @return {@link #participantEffectiveTimeFromStart} (Indicates duration from 1305 * the participant's study entry.) 1306 */ 1307 public Duration getParticipantEffectiveTimeFromStart() { 1308 if (this.participantEffectiveTimeFromStart == null) 1309 if (Configuration.errorOnAutoCreate()) 1310 throw new Error( 1311 "Attempt to auto-create ResearchElementDefinitionCharacteristicComponent.participantEffectiveTimeFromStart"); 1312 else if (Configuration.doAutoCreate()) 1313 this.participantEffectiveTimeFromStart = new Duration(); // cc 1314 return this.participantEffectiveTimeFromStart; 1315 } 1316 1317 public boolean hasParticipantEffectiveTimeFromStart() { 1318 return this.participantEffectiveTimeFromStart != null && !this.participantEffectiveTimeFromStart.isEmpty(); 1319 } 1320 1321 /** 1322 * @param value {@link #participantEffectiveTimeFromStart} (Indicates duration 1323 * from the participant's study entry.) 1324 */ 1325 public ResearchElementDefinitionCharacteristicComponent setParticipantEffectiveTimeFromStart(Duration value) { 1326 this.participantEffectiveTimeFromStart = value; 1327 return this; 1328 } 1329 1330 /** 1331 * @return {@link #participantEffectiveGroupMeasure} (Indicates how elements are 1332 * aggregated within the study effective period.). This is the 1333 * underlying object with id, value and extensions. The accessor 1334 * "getParticipantEffectiveGroupMeasure" gives direct access to the 1335 * value 1336 */ 1337 public Enumeration<GroupMeasure> getParticipantEffectiveGroupMeasureElement() { 1338 if (this.participantEffectiveGroupMeasure == null) 1339 if (Configuration.errorOnAutoCreate()) 1340 throw new Error( 1341 "Attempt to auto-create ResearchElementDefinitionCharacteristicComponent.participantEffectiveGroupMeasure"); 1342 else if (Configuration.doAutoCreate()) 1343 this.participantEffectiveGroupMeasure = new Enumeration<GroupMeasure>(new GroupMeasureEnumFactory()); // bb 1344 return this.participantEffectiveGroupMeasure; 1345 } 1346 1347 public boolean hasParticipantEffectiveGroupMeasureElement() { 1348 return this.participantEffectiveGroupMeasure != null && !this.participantEffectiveGroupMeasure.isEmpty(); 1349 } 1350 1351 public boolean hasParticipantEffectiveGroupMeasure() { 1352 return this.participantEffectiveGroupMeasure != null && !this.participantEffectiveGroupMeasure.isEmpty(); 1353 } 1354 1355 /** 1356 * @param value {@link #participantEffectiveGroupMeasure} (Indicates how 1357 * elements are aggregated within the study effective period.). 1358 * This is the underlying object with id, value and extensions. The 1359 * accessor "getParticipantEffectiveGroupMeasure" gives direct 1360 * access to the value 1361 */ 1362 public ResearchElementDefinitionCharacteristicComponent setParticipantEffectiveGroupMeasureElement( 1363 Enumeration<GroupMeasure> value) { 1364 this.participantEffectiveGroupMeasure = value; 1365 return this; 1366 } 1367 1368 /** 1369 * @return Indicates how elements are aggregated within the study effective 1370 * period. 1371 */ 1372 public GroupMeasure getParticipantEffectiveGroupMeasure() { 1373 return this.participantEffectiveGroupMeasure == null ? null : this.participantEffectiveGroupMeasure.getValue(); 1374 } 1375 1376 /** 1377 * @param value Indicates how elements are aggregated within the study effective 1378 * period. 1379 */ 1380 public ResearchElementDefinitionCharacteristicComponent setParticipantEffectiveGroupMeasure(GroupMeasure value) { 1381 if (value == null) 1382 this.participantEffectiveGroupMeasure = null; 1383 else { 1384 if (this.participantEffectiveGroupMeasure == null) 1385 this.participantEffectiveGroupMeasure = new Enumeration<GroupMeasure>(new GroupMeasureEnumFactory()); 1386 this.participantEffectiveGroupMeasure.setValue(value); 1387 } 1388 return this; 1389 } 1390 1391 protected void listChildren(List<Property> children) { 1392 super.listChildren(children); 1393 children.add(new Property("definition[x]", "CodeableConcept|canonical(ValueSet)|Expression|DataRequirement", 1394 "Define members of the research element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1395 0, 1, definition)); 1396 children.add(new Property("usageContext", "UsageContext", 1397 "Use UsageContext to define the members of the population, such as Age Ranges, Genders, Settings.", 0, 1398 java.lang.Integer.MAX_VALUE, usageContext)); 1399 children.add(new Property("exclude", "boolean", 1400 "When true, members with this characteristic are excluded from the element.", 0, 1, exclude)); 1401 children.add(new Property("unitOfMeasure", "CodeableConcept", "Specifies the UCUM unit for the outcome.", 0, 1, 1402 unitOfMeasure)); 1403 children.add(new Property("studyEffectiveDescription", "string", 1404 "A narrative description of the time period the study covers.", 0, 1, studyEffectiveDescription)); 1405 children.add(new Property("studyEffective[x]", "dateTime|Period|Duration|Timing", 1406 "Indicates what effective period the study covers.", 0, 1, studyEffective)); 1407 children.add(new Property("studyEffectiveTimeFromStart", "Duration", 1408 "Indicates duration from the study initiation.", 0, 1, studyEffectiveTimeFromStart)); 1409 children.add(new Property("studyEffectiveGroupMeasure", "code", 1410 "Indicates how elements are aggregated within the study effective period.", 0, 1, 1411 studyEffectiveGroupMeasure)); 1412 children.add(new Property("participantEffectiveDescription", "string", 1413 "A narrative description of the time period the study covers.", 0, 1, participantEffectiveDescription)); 1414 children.add(new Property("participantEffective[x]", "dateTime|Period|Duration|Timing", 1415 "Indicates what effective period the study covers.", 0, 1, participantEffective)); 1416 children.add(new Property("participantEffectiveTimeFromStart", "Duration", 1417 "Indicates duration from the participant's study entry.", 0, 1, participantEffectiveTimeFromStart)); 1418 children.add(new Property("participantEffectiveGroupMeasure", "code", 1419 "Indicates how elements are aggregated within the study effective period.", 0, 1, 1420 participantEffectiveGroupMeasure)); 1421 } 1422 1423 @Override 1424 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1425 switch (_hash) { 1426 case -1139422643: 1427 /* definition[x] */ return new Property("definition[x]", 1428 "CodeableConcept|canonical(ValueSet)|Expression|DataRequirement", 1429 "Define members of the research element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1430 0, 1, definition); 1431 case -1014418093: 1432 /* definition */ return new Property("definition[x]", 1433 "CodeableConcept|canonical(ValueSet)|Expression|DataRequirement", 1434 "Define members of the research element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1435 0, 1, definition); 1436 case -1446002226: 1437 /* definitionCodeableConcept */ return new Property("definition[x]", 1438 "CodeableConcept|canonical(ValueSet)|Expression|DataRequirement", 1439 "Define members of the research element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1440 0, 1, definition); 1441 case 933485793: 1442 /* definitionCanonical */ return new Property("definition[x]", 1443 "CodeableConcept|canonical(ValueSet)|Expression|DataRequirement", 1444 "Define members of the research element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1445 0, 1, definition); 1446 case 1463703627: 1447 /* definitionExpression */ return new Property("definition[x]", 1448 "CodeableConcept|canonical(ValueSet)|Expression|DataRequirement", 1449 "Define members of the research element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1450 0, 1, definition); 1451 case -660350874: 1452 /* definitionDataRequirement */ return new Property("definition[x]", 1453 "CodeableConcept|canonical(ValueSet)|Expression|DataRequirement", 1454 "Define members of the research element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1455 0, 1, definition); 1456 case 907012302: 1457 /* usageContext */ return new Property("usageContext", "UsageContext", 1458 "Use UsageContext to define the members of the population, such as Age Ranges, Genders, Settings.", 0, 1459 java.lang.Integer.MAX_VALUE, usageContext); 1460 case -1321148966: 1461 /* exclude */ return new Property("exclude", "boolean", 1462 "When true, members with this characteristic are excluded from the element.", 0, 1, exclude); 1463 case -750257565: 1464 /* unitOfMeasure */ return new Property("unitOfMeasure", "CodeableConcept", 1465 "Specifies the UCUM unit for the outcome.", 0, 1, unitOfMeasure); 1466 case 237553470: 1467 /* studyEffectiveDescription */ return new Property("studyEffectiveDescription", "string", 1468 "A narrative description of the time period the study covers.", 0, 1, studyEffectiveDescription); 1469 case -1832549918: 1470 /* studyEffective[x] */ return new Property("studyEffective[x]", "dateTime|Period|Duration|Timing", 1471 "Indicates what effective period the study covers.", 0, 1, studyEffective); 1472 case -836391458: 1473 /* studyEffective */ return new Property("studyEffective[x]", "dateTime|Period|Duration|Timing", 1474 "Indicates what effective period the study covers.", 0, 1, studyEffective); 1475 case 439780249: 1476 /* studyEffectiveDateTime */ return new Property("studyEffective[x]", "dateTime|Period|Duration|Timing", 1477 "Indicates what effective period the study covers.", 0, 1, studyEffective); 1478 case -497045185: 1479 /* studyEffectivePeriod */ return new Property("studyEffective[x]", "dateTime|Period|Duration|Timing", 1480 "Indicates what effective period the study covers.", 0, 1, studyEffective); 1481 case 949985682: 1482 /* studyEffectiveDuration */ return new Property("studyEffective[x]", "dateTime|Period|Duration|Timing", 1483 "Indicates what effective period the study covers.", 0, 1, studyEffective); 1484 case -378983480: 1485 /* studyEffectiveTiming */ return new Property("studyEffective[x]", "dateTime|Period|Duration|Timing", 1486 "Indicates what effective period the study covers.", 0, 1, studyEffective); 1487 case -2107828915: 1488 /* studyEffectiveTimeFromStart */ return new Property("studyEffectiveTimeFromStart", "Duration", 1489 "Indicates duration from the study initiation.", 0, 1, studyEffectiveTimeFromStart); 1490 case 1284435677: 1491 /* studyEffectiveGroupMeasure */ return new Property("studyEffectiveGroupMeasure", "code", 1492 "Indicates how elements are aggregated within the study effective period.", 0, 1, 1493 studyEffectiveGroupMeasure); 1494 case 1333186472: 1495 /* participantEffectiveDescription */ return new Property("participantEffectiveDescription", "string", 1496 "A narrative description of the time period the study covers.", 0, 1, participantEffectiveDescription); 1497 case 1777308748: 1498 /* participantEffective[x] */ return new Property("participantEffective[x]", "dateTime|Period|Duration|Timing", 1499 "Indicates what effective period the study covers.", 0, 1, participantEffective); 1500 case 1376306100: 1501 /* participantEffective */ return new Property("participantEffective[x]", "dateTime|Period|Duration|Timing", 1502 "Indicates what effective period the study covers.", 0, 1, participantEffective); 1503 case -1721146513: 1504 /* participantEffectiveDateTime */ return new Property("participantEffective[x]", 1505 "dateTime|Period|Duration|Timing", "Indicates what effective period the study covers.", 0, 1, 1506 participantEffective); 1507 case -883650923: 1508 /* participantEffectivePeriod */ return new Property("participantEffective[x]", 1509 "dateTime|Period|Duration|Timing", "Indicates what effective period the study covers.", 0, 1, 1510 participantEffective); 1511 case -1210941080: 1512 /* participantEffectiveDuration */ return new Property("participantEffective[x]", 1513 "dateTime|Period|Duration|Timing", "Indicates what effective period the study covers.", 0, 1, 1514 participantEffective); 1515 case -765589218: 1516 /* participantEffectiveTiming */ return new Property("participantEffective[x]", 1517 "dateTime|Period|Duration|Timing", "Indicates what effective period the study covers.", 0, 1, 1518 participantEffective); 1519 case -1471501513: 1520 /* participantEffectiveTimeFromStart */ return new Property("participantEffectiveTimeFromStart", "Duration", 1521 "Indicates duration from the participant's study entry.", 0, 1, participantEffectiveTimeFromStart); 1522 case 889320371: 1523 /* participantEffectiveGroupMeasure */ return new Property("participantEffectiveGroupMeasure", "code", 1524 "Indicates how elements are aggregated within the study effective period.", 0, 1, 1525 participantEffectiveGroupMeasure); 1526 default: 1527 return super.getNamedProperty(_hash, _name, _checkValid); 1528 } 1529 1530 } 1531 1532 @Override 1533 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1534 switch (hash) { 1535 case -1014418093: 1536 /* definition */ return this.definition == null ? new Base[0] : new Base[] { this.definition }; // Type 1537 case 907012302: 1538 /* usageContext */ return this.usageContext == null ? new Base[0] 1539 : this.usageContext.toArray(new Base[this.usageContext.size()]); // UsageContext 1540 case -1321148966: 1541 /* exclude */ return this.exclude == null ? new Base[0] : new Base[] { this.exclude }; // BooleanType 1542 case -750257565: 1543 /* unitOfMeasure */ return this.unitOfMeasure == null ? new Base[0] : new Base[] { this.unitOfMeasure }; // CodeableConcept 1544 case 237553470: 1545 /* studyEffectiveDescription */ return this.studyEffectiveDescription == null ? new Base[0] 1546 : new Base[] { this.studyEffectiveDescription }; // StringType 1547 case -836391458: 1548 /* studyEffective */ return this.studyEffective == null ? new Base[0] : new Base[] { this.studyEffective }; // Type 1549 case -2107828915: 1550 /* studyEffectiveTimeFromStart */ return this.studyEffectiveTimeFromStart == null ? new Base[0] 1551 : new Base[] { this.studyEffectiveTimeFromStart }; // Duration 1552 case 1284435677: 1553 /* studyEffectiveGroupMeasure */ return this.studyEffectiveGroupMeasure == null ? new Base[0] 1554 : new Base[] { this.studyEffectiveGroupMeasure }; // Enumeration<GroupMeasure> 1555 case 1333186472: 1556 /* participantEffectiveDescription */ return this.participantEffectiveDescription == null ? new Base[0] 1557 : new Base[] { this.participantEffectiveDescription }; // StringType 1558 case 1376306100: 1559 /* participantEffective */ return this.participantEffective == null ? new Base[0] 1560 : new Base[] { this.participantEffective }; // Type 1561 case -1471501513: 1562 /* participantEffectiveTimeFromStart */ return this.participantEffectiveTimeFromStart == null ? new Base[0] 1563 : new Base[] { this.participantEffectiveTimeFromStart }; // Duration 1564 case 889320371: 1565 /* participantEffectiveGroupMeasure */ return this.participantEffectiveGroupMeasure == null ? new Base[0] 1566 : new Base[] { this.participantEffectiveGroupMeasure }; // Enumeration<GroupMeasure> 1567 default: 1568 return super.getProperty(hash, name, checkValid); 1569 } 1570 1571 } 1572 1573 @Override 1574 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1575 switch (hash) { 1576 case -1014418093: // definition 1577 this.definition = castToType(value); // Type 1578 return value; 1579 case 907012302: // usageContext 1580 this.getUsageContext().add(castToUsageContext(value)); // UsageContext 1581 return value; 1582 case -1321148966: // exclude 1583 this.exclude = castToBoolean(value); // BooleanType 1584 return value; 1585 case -750257565: // unitOfMeasure 1586 this.unitOfMeasure = castToCodeableConcept(value); // CodeableConcept 1587 return value; 1588 case 237553470: // studyEffectiveDescription 1589 this.studyEffectiveDescription = castToString(value); // StringType 1590 return value; 1591 case -836391458: // studyEffective 1592 this.studyEffective = castToType(value); // Type 1593 return value; 1594 case -2107828915: // studyEffectiveTimeFromStart 1595 this.studyEffectiveTimeFromStart = castToDuration(value); // Duration 1596 return value; 1597 case 1284435677: // studyEffectiveGroupMeasure 1598 value = new GroupMeasureEnumFactory().fromType(castToCode(value)); 1599 this.studyEffectiveGroupMeasure = (Enumeration) value; // Enumeration<GroupMeasure> 1600 return value; 1601 case 1333186472: // participantEffectiveDescription 1602 this.participantEffectiveDescription = castToString(value); // StringType 1603 return value; 1604 case 1376306100: // participantEffective 1605 this.participantEffective = castToType(value); // Type 1606 return value; 1607 case -1471501513: // participantEffectiveTimeFromStart 1608 this.participantEffectiveTimeFromStart = castToDuration(value); // Duration 1609 return value; 1610 case 889320371: // participantEffectiveGroupMeasure 1611 value = new GroupMeasureEnumFactory().fromType(castToCode(value)); 1612 this.participantEffectiveGroupMeasure = (Enumeration) value; // Enumeration<GroupMeasure> 1613 return value; 1614 default: 1615 return super.setProperty(hash, name, value); 1616 } 1617 1618 } 1619 1620 @Override 1621 public Base setProperty(String name, Base value) throws FHIRException { 1622 if (name.equals("definition[x]")) { 1623 this.definition = castToType(value); // Type 1624 } else if (name.equals("usageContext")) { 1625 this.getUsageContext().add(castToUsageContext(value)); 1626 } else if (name.equals("exclude")) { 1627 this.exclude = castToBoolean(value); // BooleanType 1628 } else if (name.equals("unitOfMeasure")) { 1629 this.unitOfMeasure = castToCodeableConcept(value); // CodeableConcept 1630 } else if (name.equals("studyEffectiveDescription")) { 1631 this.studyEffectiveDescription = castToString(value); // StringType 1632 } else if (name.equals("studyEffective[x]")) { 1633 this.studyEffective = castToType(value); // Type 1634 } else if (name.equals("studyEffectiveTimeFromStart")) { 1635 this.studyEffectiveTimeFromStart = castToDuration(value); // Duration 1636 } else if (name.equals("studyEffectiveGroupMeasure")) { 1637 value = new GroupMeasureEnumFactory().fromType(castToCode(value)); 1638 this.studyEffectiveGroupMeasure = (Enumeration) value; // Enumeration<GroupMeasure> 1639 } else if (name.equals("participantEffectiveDescription")) { 1640 this.participantEffectiveDescription = castToString(value); // StringType 1641 } else if (name.equals("participantEffective[x]")) { 1642 this.participantEffective = castToType(value); // Type 1643 } else if (name.equals("participantEffectiveTimeFromStart")) { 1644 this.participantEffectiveTimeFromStart = castToDuration(value); // Duration 1645 } else if (name.equals("participantEffectiveGroupMeasure")) { 1646 value = new GroupMeasureEnumFactory().fromType(castToCode(value)); 1647 this.participantEffectiveGroupMeasure = (Enumeration) value; // Enumeration<GroupMeasure> 1648 } else 1649 return super.setProperty(name, value); 1650 return value; 1651 } 1652 1653 @Override 1654 public void removeChild(String name, Base value) throws FHIRException { 1655 if (name.equals("definition[x]")) { 1656 this.definition = null; 1657 } else if (name.equals("usageContext")) { 1658 this.getUsageContext().remove(castToUsageContext(value)); 1659 } else if (name.equals("exclude")) { 1660 this.exclude = null; 1661 } else if (name.equals("unitOfMeasure")) { 1662 this.unitOfMeasure = null; 1663 } else if (name.equals("studyEffectiveDescription")) { 1664 this.studyEffectiveDescription = null; 1665 } else if (name.equals("studyEffective[x]")) { 1666 this.studyEffective = null; 1667 } else if (name.equals("studyEffectiveTimeFromStart")) { 1668 this.studyEffectiveTimeFromStart = null; 1669 } else if (name.equals("studyEffectiveGroupMeasure")) { 1670 this.studyEffectiveGroupMeasure = null; 1671 } else if (name.equals("participantEffectiveDescription")) { 1672 this.participantEffectiveDescription = null; 1673 } else if (name.equals("participantEffective[x]")) { 1674 this.participantEffective = null; 1675 } else if (name.equals("participantEffectiveTimeFromStart")) { 1676 this.participantEffectiveTimeFromStart = null; 1677 } else if (name.equals("participantEffectiveGroupMeasure")) { 1678 this.participantEffectiveGroupMeasure = null; 1679 } else 1680 super.removeChild(name, value); 1681 1682 } 1683 1684 @Override 1685 public Base makeProperty(int hash, String name) throws FHIRException { 1686 switch (hash) { 1687 case -1139422643: 1688 return getDefinition(); 1689 case -1014418093: 1690 return getDefinition(); 1691 case 907012302: 1692 return addUsageContext(); 1693 case -1321148966: 1694 return getExcludeElement(); 1695 case -750257565: 1696 return getUnitOfMeasure(); 1697 case 237553470: 1698 return getStudyEffectiveDescriptionElement(); 1699 case -1832549918: 1700 return getStudyEffective(); 1701 case -836391458: 1702 return getStudyEffective(); 1703 case -2107828915: 1704 return getStudyEffectiveTimeFromStart(); 1705 case 1284435677: 1706 return getStudyEffectiveGroupMeasureElement(); 1707 case 1333186472: 1708 return getParticipantEffectiveDescriptionElement(); 1709 case 1777308748: 1710 return getParticipantEffective(); 1711 case 1376306100: 1712 return getParticipantEffective(); 1713 case -1471501513: 1714 return getParticipantEffectiveTimeFromStart(); 1715 case 889320371: 1716 return getParticipantEffectiveGroupMeasureElement(); 1717 default: 1718 return super.makeProperty(hash, name); 1719 } 1720 1721 } 1722 1723 @Override 1724 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1725 switch (hash) { 1726 case -1014418093: 1727 /* definition */ return new String[] { "CodeableConcept", "canonical", "Expression", "DataRequirement" }; 1728 case 907012302: 1729 /* usageContext */ return new String[] { "UsageContext" }; 1730 case -1321148966: 1731 /* exclude */ return new String[] { "boolean" }; 1732 case -750257565: 1733 /* unitOfMeasure */ return new String[] { "CodeableConcept" }; 1734 case 237553470: 1735 /* studyEffectiveDescription */ return new String[] { "string" }; 1736 case -836391458: 1737 /* studyEffective */ return new String[] { "dateTime", "Period", "Duration", "Timing" }; 1738 case -2107828915: 1739 /* studyEffectiveTimeFromStart */ return new String[] { "Duration" }; 1740 case 1284435677: 1741 /* studyEffectiveGroupMeasure */ return new String[] { "code" }; 1742 case 1333186472: 1743 /* participantEffectiveDescription */ return new String[] { "string" }; 1744 case 1376306100: 1745 /* participantEffective */ return new String[] { "dateTime", "Period", "Duration", "Timing" }; 1746 case -1471501513: 1747 /* participantEffectiveTimeFromStart */ return new String[] { "Duration" }; 1748 case 889320371: 1749 /* participantEffectiveGroupMeasure */ return new String[] { "code" }; 1750 default: 1751 return super.getTypesForProperty(hash, name); 1752 } 1753 1754 } 1755 1756 @Override 1757 public Base addChild(String name) throws FHIRException { 1758 if (name.equals("definitionCodeableConcept")) { 1759 this.definition = new CodeableConcept(); 1760 return this.definition; 1761 } else if (name.equals("definitionCanonical")) { 1762 this.definition = new CanonicalType(); 1763 return this.definition; 1764 } else if (name.equals("definitionExpression")) { 1765 this.definition = new Expression(); 1766 return this.definition; 1767 } else if (name.equals("definitionDataRequirement")) { 1768 this.definition = new DataRequirement(); 1769 return this.definition; 1770 } else if (name.equals("usageContext")) { 1771 return addUsageContext(); 1772 } else if (name.equals("exclude")) { 1773 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.exclude"); 1774 } else if (name.equals("unitOfMeasure")) { 1775 this.unitOfMeasure = new CodeableConcept(); 1776 return this.unitOfMeasure; 1777 } else if (name.equals("studyEffectiveDescription")) { 1778 throw new FHIRException( 1779 "Cannot call addChild on a singleton property ResearchElementDefinition.studyEffectiveDescription"); 1780 } else if (name.equals("studyEffectiveDateTime")) { 1781 this.studyEffective = new DateTimeType(); 1782 return this.studyEffective; 1783 } else if (name.equals("studyEffectivePeriod")) { 1784 this.studyEffective = new Period(); 1785 return this.studyEffective; 1786 } else if (name.equals("studyEffectiveDuration")) { 1787 this.studyEffective = new Duration(); 1788 return this.studyEffective; 1789 } else if (name.equals("studyEffectiveTiming")) { 1790 this.studyEffective = new Timing(); 1791 return this.studyEffective; 1792 } else if (name.equals("studyEffectiveTimeFromStart")) { 1793 this.studyEffectiveTimeFromStart = new Duration(); 1794 return this.studyEffectiveTimeFromStart; 1795 } else if (name.equals("studyEffectiveGroupMeasure")) { 1796 throw new FHIRException( 1797 "Cannot call addChild on a singleton property ResearchElementDefinition.studyEffectiveGroupMeasure"); 1798 } else if (name.equals("participantEffectiveDescription")) { 1799 throw new FHIRException( 1800 "Cannot call addChild on a singleton property ResearchElementDefinition.participantEffectiveDescription"); 1801 } else if (name.equals("participantEffectiveDateTime")) { 1802 this.participantEffective = new DateTimeType(); 1803 return this.participantEffective; 1804 } else if (name.equals("participantEffectivePeriod")) { 1805 this.participantEffective = new Period(); 1806 return this.participantEffective; 1807 } else if (name.equals("participantEffectiveDuration")) { 1808 this.participantEffective = new Duration(); 1809 return this.participantEffective; 1810 } else if (name.equals("participantEffectiveTiming")) { 1811 this.participantEffective = new Timing(); 1812 return this.participantEffective; 1813 } else if (name.equals("participantEffectiveTimeFromStart")) { 1814 this.participantEffectiveTimeFromStart = new Duration(); 1815 return this.participantEffectiveTimeFromStart; 1816 } else if (name.equals("participantEffectiveGroupMeasure")) { 1817 throw new FHIRException( 1818 "Cannot call addChild on a singleton property ResearchElementDefinition.participantEffectiveGroupMeasure"); 1819 } else 1820 return super.addChild(name); 1821 } 1822 1823 public ResearchElementDefinitionCharacteristicComponent copy() { 1824 ResearchElementDefinitionCharacteristicComponent dst = new ResearchElementDefinitionCharacteristicComponent(); 1825 copyValues(dst); 1826 return dst; 1827 } 1828 1829 public void copyValues(ResearchElementDefinitionCharacteristicComponent dst) { 1830 super.copyValues(dst); 1831 dst.definition = definition == null ? null : definition.copy(); 1832 if (usageContext != null) { 1833 dst.usageContext = new ArrayList<UsageContext>(); 1834 for (UsageContext i : usageContext) 1835 dst.usageContext.add(i.copy()); 1836 } 1837 ; 1838 dst.exclude = exclude == null ? null : exclude.copy(); 1839 dst.unitOfMeasure = unitOfMeasure == null ? null : unitOfMeasure.copy(); 1840 dst.studyEffectiveDescription = studyEffectiveDescription == null ? null : studyEffectiveDescription.copy(); 1841 dst.studyEffective = studyEffective == null ? null : studyEffective.copy(); 1842 dst.studyEffectiveTimeFromStart = studyEffectiveTimeFromStart == null ? null : studyEffectiveTimeFromStart.copy(); 1843 dst.studyEffectiveGroupMeasure = studyEffectiveGroupMeasure == null ? null : studyEffectiveGroupMeasure.copy(); 1844 dst.participantEffectiveDescription = participantEffectiveDescription == null ? null 1845 : participantEffectiveDescription.copy(); 1846 dst.participantEffective = participantEffective == null ? null : participantEffective.copy(); 1847 dst.participantEffectiveTimeFromStart = participantEffectiveTimeFromStart == null ? null 1848 : participantEffectiveTimeFromStart.copy(); 1849 dst.participantEffectiveGroupMeasure = participantEffectiveGroupMeasure == null ? null 1850 : participantEffectiveGroupMeasure.copy(); 1851 } 1852 1853 @Override 1854 public boolean equalsDeep(Base other_) { 1855 if (!super.equalsDeep(other_)) 1856 return false; 1857 if (!(other_ instanceof ResearchElementDefinitionCharacteristicComponent)) 1858 return false; 1859 ResearchElementDefinitionCharacteristicComponent o = (ResearchElementDefinitionCharacteristicComponent) other_; 1860 return compareDeep(definition, o.definition, true) && compareDeep(usageContext, o.usageContext, true) 1861 && compareDeep(exclude, o.exclude, true) && compareDeep(unitOfMeasure, o.unitOfMeasure, true) 1862 && compareDeep(studyEffectiveDescription, o.studyEffectiveDescription, true) 1863 && compareDeep(studyEffective, o.studyEffective, true) 1864 && compareDeep(studyEffectiveTimeFromStart, o.studyEffectiveTimeFromStart, true) 1865 && compareDeep(studyEffectiveGroupMeasure, o.studyEffectiveGroupMeasure, true) 1866 && compareDeep(participantEffectiveDescription, o.participantEffectiveDescription, true) 1867 && compareDeep(participantEffective, o.participantEffective, true) 1868 && compareDeep(participantEffectiveTimeFromStart, o.participantEffectiveTimeFromStart, true) 1869 && compareDeep(participantEffectiveGroupMeasure, o.participantEffectiveGroupMeasure, true); 1870 } 1871 1872 @Override 1873 public boolean equalsShallow(Base other_) { 1874 if (!super.equalsShallow(other_)) 1875 return false; 1876 if (!(other_ instanceof ResearchElementDefinitionCharacteristicComponent)) 1877 return false; 1878 ResearchElementDefinitionCharacteristicComponent o = (ResearchElementDefinitionCharacteristicComponent) other_; 1879 return compareValues(exclude, o.exclude, true) 1880 && compareValues(studyEffectiveDescription, o.studyEffectiveDescription, true) 1881 && compareValues(studyEffectiveGroupMeasure, o.studyEffectiveGroupMeasure, true) 1882 && compareValues(participantEffectiveDescription, o.participantEffectiveDescription, true) 1883 && compareValues(participantEffectiveGroupMeasure, o.participantEffectiveGroupMeasure, true); 1884 } 1885 1886 public boolean isEmpty() { 1887 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(definition, usageContext, exclude, unitOfMeasure, 1888 studyEffectiveDescription, studyEffective, studyEffectiveTimeFromStart, studyEffectiveGroupMeasure, 1889 participantEffectiveDescription, participantEffective, participantEffectiveTimeFromStart, 1890 participantEffectiveGroupMeasure); 1891 } 1892 1893 public String fhirType() { 1894 return "ResearchElementDefinition.characteristic"; 1895 1896 } 1897 1898 } 1899 1900 /** 1901 * A formal identifier that is used to identify this research element definition 1902 * when it is represented in other formats, or referenced in a specification, 1903 * model, design or an instance. 1904 */ 1905 @Child(name = "identifier", type = { 1906 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1907 @Description(shortDefinition = "Additional identifier for the research element definition", formalDefinition = "A formal identifier that is used to identify this research element definition when it is represented in other formats, or referenced in a specification, model, design or an instance.") 1908 protected List<Identifier> identifier; 1909 1910 /** 1911 * The short title provides an alternate title for use in informal descriptive 1912 * contexts where the full, formal title is not necessary. 1913 */ 1914 @Child(name = "shortTitle", type = { 1915 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1916 @Description(shortDefinition = "Title for use in informal contexts", formalDefinition = "The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary.") 1917 protected StringType shortTitle; 1918 1919 /** 1920 * An explanatory or alternate title for the ResearchElementDefinition giving 1921 * additional information about its content. 1922 */ 1923 @Child(name = "subtitle", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1924 @Description(shortDefinition = "Subordinate title of the ResearchElementDefinition", formalDefinition = "An explanatory or alternate title for the ResearchElementDefinition giving additional information about its content.") 1925 protected StringType subtitle; 1926 1927 /** 1928 * The intended subjects for the ResearchElementDefinition. If this element is 1929 * not provided, a Patient subject is assumed, but the subject of the 1930 * ResearchElementDefinition can be anything. 1931 */ 1932 @Child(name = "subject", type = { CodeableConcept.class, 1933 Group.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1934 @Description(shortDefinition = "E.g. Patient, Practitioner, RelatedPerson, Organization, Location, Device", formalDefinition = "The intended subjects for the ResearchElementDefinition. If this element is not provided, a Patient subject is assumed, but the subject of the ResearchElementDefinition can be anything.") 1935 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/subject-type") 1936 protected Type subject; 1937 1938 /** 1939 * A human-readable string to clarify or explain concepts about the resource. 1940 */ 1941 @Child(name = "comment", type = { 1942 StringType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1943 @Description(shortDefinition = "Used for footnotes or explanatory notes", formalDefinition = "A human-readable string to clarify or explain concepts about the resource.") 1944 protected List<StringType> comment; 1945 1946 /** 1947 * Explanation of why this research element definition is needed and why it has 1948 * been designed as it has. 1949 */ 1950 @Child(name = "purpose", type = { 1951 MarkdownType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1952 @Description(shortDefinition = "Why this research element definition is defined", formalDefinition = "Explanation of why this research element definition is needed and why it has been designed as it has.") 1953 protected MarkdownType purpose; 1954 1955 /** 1956 * A detailed description, from a clinical perspective, of how the 1957 * ResearchElementDefinition is used. 1958 */ 1959 @Child(name = "usage", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 1960 @Description(shortDefinition = "Describes the clinical usage of the ResearchElementDefinition", formalDefinition = "A detailed description, from a clinical perspective, of how the ResearchElementDefinition is used.") 1961 protected StringType usage; 1962 1963 /** 1964 * A copyright statement relating to the research element definition and/or its 1965 * contents. Copyright statements are generally legal restrictions on the use 1966 * and publishing of the research element definition. 1967 */ 1968 @Child(name = "copyright", type = { 1969 MarkdownType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 1970 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the research element definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the research element definition.") 1971 protected MarkdownType copyright; 1972 1973 /** 1974 * The date on which the resource content was approved by the publisher. 1975 * Approval happens once when the content is officially approved for usage. 1976 */ 1977 @Child(name = "approvalDate", type = { 1978 DateType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 1979 @Description(shortDefinition = "When the research element definition was approved by publisher", formalDefinition = "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.") 1980 protected DateType approvalDate; 1981 1982 /** 1983 * The date on which the resource content was last reviewed. Review happens 1984 * periodically after approval but does not change the original approval date. 1985 */ 1986 @Child(name = "lastReviewDate", type = { 1987 DateType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1988 @Description(shortDefinition = "When the research element definition was last reviewed", formalDefinition = "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.") 1989 protected DateType lastReviewDate; 1990 1991 /** 1992 * The period during which the research element definition content was or is 1993 * planned to be in active use. 1994 */ 1995 @Child(name = "effectivePeriod", type = { 1996 Period.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 1997 @Description(shortDefinition = "When the research element definition is expected to be used", formalDefinition = "The period during which the research element definition content was or is planned to be in active use.") 1998 protected Period effectivePeriod; 1999 2000 /** 2001 * Descriptive topics related to the content of the ResearchElementDefinition. 2002 * Topics provide a high-level categorization grouping types of 2003 * ResearchElementDefinitions that can be useful for filtering and searching. 2004 */ 2005 @Child(name = "topic", type = { 2006 CodeableConcept.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2007 @Description(shortDefinition = "The category of the ResearchElementDefinition, such as Education, Treatment, Assessment, etc.", formalDefinition = "Descriptive topics related to the content of the ResearchElementDefinition. Topics provide a high-level categorization grouping types of ResearchElementDefinitions that can be useful for filtering and searching.") 2008 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/definition-topic") 2009 protected List<CodeableConcept> topic; 2010 2011 /** 2012 * An individiual or organization primarily involved in the creation and 2013 * maintenance of the content. 2014 */ 2015 @Child(name = "author", type = { 2016 ContactDetail.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2017 @Description(shortDefinition = "Who authored the content", formalDefinition = "An individiual or organization primarily involved in the creation and maintenance of the content.") 2018 protected List<ContactDetail> author; 2019 2020 /** 2021 * An individual or organization primarily responsible for internal coherence of 2022 * the content. 2023 */ 2024 @Child(name = "editor", type = { 2025 ContactDetail.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2026 @Description(shortDefinition = "Who edited the content", formalDefinition = "An individual or organization primarily responsible for internal coherence of the content.") 2027 protected List<ContactDetail> editor; 2028 2029 /** 2030 * An individual or organization primarily responsible for review of some aspect 2031 * of the content. 2032 */ 2033 @Child(name = "reviewer", type = { 2034 ContactDetail.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2035 @Description(shortDefinition = "Who reviewed the content", formalDefinition = "An individual or organization primarily responsible for review of some aspect of the content.") 2036 protected List<ContactDetail> reviewer; 2037 2038 /** 2039 * An individual or organization responsible for officially endorsing the 2040 * content for use in some setting. 2041 */ 2042 @Child(name = "endorser", type = { 2043 ContactDetail.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2044 @Description(shortDefinition = "Who endorsed the content", formalDefinition = "An individual or organization responsible for officially endorsing the content for use in some setting.") 2045 protected List<ContactDetail> endorser; 2046 2047 /** 2048 * Related artifacts such as additional documentation, justification, or 2049 * bibliographic references. 2050 */ 2051 @Child(name = "relatedArtifact", type = { 2052 RelatedArtifact.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2053 @Description(shortDefinition = "Additional documentation, citations, etc.", formalDefinition = "Related artifacts such as additional documentation, justification, or bibliographic references.") 2054 protected List<RelatedArtifact> relatedArtifact; 2055 2056 /** 2057 * A reference to a Library resource containing the formal logic used by the 2058 * ResearchElementDefinition. 2059 */ 2060 @Child(name = "library", type = { 2061 CanonicalType.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2062 @Description(shortDefinition = "Logic used by the ResearchElementDefinition", formalDefinition = "A reference to a Library resource containing the formal logic used by the ResearchElementDefinition.") 2063 protected List<CanonicalType> library; 2064 2065 /** 2066 * The type of research element, a population, an exposure, or an outcome. 2067 */ 2068 @Child(name = "type", type = { CodeType.class }, order = 18, min = 1, max = 1, modifier = false, summary = true) 2069 @Description(shortDefinition = "population | exposure | outcome", formalDefinition = "The type of research element, a population, an exposure, or an outcome.") 2070 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/research-element-type") 2071 protected Enumeration<ResearchElementType> type; 2072 2073 /** 2074 * The type of the outcome (e.g. Dichotomous, Continuous, or Descriptive). 2075 */ 2076 @Child(name = "variableType", type = { 2077 CodeType.class }, order = 19, min = 0, max = 1, modifier = false, summary = false) 2078 @Description(shortDefinition = "dichotomous | continuous | descriptive", formalDefinition = "The type of the outcome (e.g. Dichotomous, Continuous, or Descriptive).") 2079 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/variable-type") 2080 protected Enumeration<VariableType> variableType; 2081 2082 /** 2083 * A characteristic that defines the members of the research element. Multiple 2084 * characteristics are applied with "and" semantics. 2085 */ 2086 @Child(name = "characteristic", type = {}, order = 20, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2087 @Description(shortDefinition = "What defines the members of the research element", formalDefinition = "A characteristic that defines the members of the research element. Multiple characteristics are applied with \"and\" semantics.") 2088 protected List<ResearchElementDefinitionCharacteristicComponent> characteristic; 2089 2090 private static final long serialVersionUID = 1483216033L; 2091 2092 /** 2093 * Constructor 2094 */ 2095 public ResearchElementDefinition() { 2096 super(); 2097 } 2098 2099 /** 2100 * Constructor 2101 */ 2102 public ResearchElementDefinition(Enumeration<PublicationStatus> status, Enumeration<ResearchElementType> type) { 2103 super(); 2104 this.status = status; 2105 this.type = type; 2106 } 2107 2108 /** 2109 * @return {@link #url} (An absolute URI that is used to identify this research 2110 * element definition when it is referenced in a specification, model, 2111 * design or an instance; also called its canonical identifier. This 2112 * SHOULD be globally unique and SHOULD be a literal address at which at 2113 * which an authoritative instance of this research element definition 2114 * is (or will be) published. This URL can be the target of a canonical 2115 * reference. It SHALL remain the same when the research element 2116 * definition is stored on different servers.). This is the underlying 2117 * object with id, value and extensions. The accessor "getUrl" gives 2118 * direct access to the value 2119 */ 2120 public UriType getUrlElement() { 2121 if (this.url == null) 2122 if (Configuration.errorOnAutoCreate()) 2123 throw new Error("Attempt to auto-create ResearchElementDefinition.url"); 2124 else if (Configuration.doAutoCreate()) 2125 this.url = new UriType(); // bb 2126 return this.url; 2127 } 2128 2129 public boolean hasUrlElement() { 2130 return this.url != null && !this.url.isEmpty(); 2131 } 2132 2133 public boolean hasUrl() { 2134 return this.url != null && !this.url.isEmpty(); 2135 } 2136 2137 /** 2138 * @param value {@link #url} (An absolute URI that is used to identify this 2139 * research element definition when it is referenced in a 2140 * specification, model, design or an instance; also called its 2141 * canonical identifier. This SHOULD be globally unique and SHOULD 2142 * be a literal address at which at which an authoritative instance 2143 * of this research element definition is (or will be) published. 2144 * This URL can be the target of a canonical reference. It SHALL 2145 * remain the same when the research element definition is stored 2146 * on different servers.). This is the underlying object with id, 2147 * value and extensions. The accessor "getUrl" gives direct access 2148 * to the value 2149 */ 2150 public ResearchElementDefinition setUrlElement(UriType value) { 2151 this.url = value; 2152 return this; 2153 } 2154 2155 /** 2156 * @return An absolute URI that is used to identify this research element 2157 * definition when it is referenced in a specification, model, design or 2158 * an instance; also called its canonical identifier. This SHOULD be 2159 * globally unique and SHOULD be a literal address at which at which an 2160 * authoritative instance of this research element definition is (or 2161 * will be) published. This URL can be the target of a canonical 2162 * reference. It SHALL remain the same when the research element 2163 * definition is stored on different servers. 2164 */ 2165 public String getUrl() { 2166 return this.url == null ? null : this.url.getValue(); 2167 } 2168 2169 /** 2170 * @param value An absolute URI that is used to identify this research element 2171 * definition when it is referenced in a specification, model, 2172 * design or an instance; also called its canonical identifier. 2173 * This SHOULD be globally unique and SHOULD be a literal address 2174 * at which at which an authoritative instance of this research 2175 * element definition is (or will be) published. This URL can be 2176 * the target of a canonical reference. It SHALL remain the same 2177 * when the research element definition is stored on different 2178 * servers. 2179 */ 2180 public ResearchElementDefinition setUrl(String value) { 2181 if (Utilities.noString(value)) 2182 this.url = null; 2183 else { 2184 if (this.url == null) 2185 this.url = new UriType(); 2186 this.url.setValue(value); 2187 } 2188 return this; 2189 } 2190 2191 /** 2192 * @return {@link #identifier} (A formal identifier that is used to identify 2193 * this research element definition when it is represented in other 2194 * formats, or referenced in a specification, model, design or an 2195 * instance.) 2196 */ 2197 public List<Identifier> getIdentifier() { 2198 if (this.identifier == null) 2199 this.identifier = new ArrayList<Identifier>(); 2200 return this.identifier; 2201 } 2202 2203 /** 2204 * @return Returns a reference to <code>this</code> for easy method chaining 2205 */ 2206 public ResearchElementDefinition setIdentifier(List<Identifier> theIdentifier) { 2207 this.identifier = theIdentifier; 2208 return this; 2209 } 2210 2211 public boolean hasIdentifier() { 2212 if (this.identifier == null) 2213 return false; 2214 for (Identifier item : this.identifier) 2215 if (!item.isEmpty()) 2216 return true; 2217 return false; 2218 } 2219 2220 public Identifier addIdentifier() { // 3 2221 Identifier t = new Identifier(); 2222 if (this.identifier == null) 2223 this.identifier = new ArrayList<Identifier>(); 2224 this.identifier.add(t); 2225 return t; 2226 } 2227 2228 public ResearchElementDefinition addIdentifier(Identifier t) { // 3 2229 if (t == null) 2230 return this; 2231 if (this.identifier == null) 2232 this.identifier = new ArrayList<Identifier>(); 2233 this.identifier.add(t); 2234 return this; 2235 } 2236 2237 /** 2238 * @return The first repetition of repeating field {@link #identifier}, creating 2239 * it if it does not already exist 2240 */ 2241 public Identifier getIdentifierFirstRep() { 2242 if (getIdentifier().isEmpty()) { 2243 addIdentifier(); 2244 } 2245 return getIdentifier().get(0); 2246 } 2247 2248 /** 2249 * @return {@link #version} (The identifier that is used to identify this 2250 * version of the research element definition when it is referenced in a 2251 * specification, model, design or instance. This is an arbitrary value 2252 * managed by the research element definition author and is not expected 2253 * to be globally unique. For example, it might be a timestamp (e.g. 2254 * yyyymmdd) if a managed version is not available. There is also no 2255 * expectation that versions can be placed in a lexicographical 2256 * sequence. To provide a version consistent with the Decision Support 2257 * Service specification, use the format Major.Minor.Revision (e.g. 2258 * 1.0.0). For more information on versioning knowledge assets, refer to 2259 * the Decision Support Service specification. Note that a version is 2260 * required for non-experimental active artifacts.). This is the 2261 * underlying object with id, value and extensions. The accessor 2262 * "getVersion" gives direct access to the value 2263 */ 2264 public StringType getVersionElement() { 2265 if (this.version == null) 2266 if (Configuration.errorOnAutoCreate()) 2267 throw new Error("Attempt to auto-create ResearchElementDefinition.version"); 2268 else if (Configuration.doAutoCreate()) 2269 this.version = new StringType(); // bb 2270 return this.version; 2271 } 2272 2273 public boolean hasVersionElement() { 2274 return this.version != null && !this.version.isEmpty(); 2275 } 2276 2277 public boolean hasVersion() { 2278 return this.version != null && !this.version.isEmpty(); 2279 } 2280 2281 /** 2282 * @param value {@link #version} (The identifier that is used to identify this 2283 * version of the research element definition when it is referenced 2284 * in a specification, model, design or instance. This is an 2285 * arbitrary value managed by the research element definition 2286 * author and is not expected to be globally unique. For example, 2287 * it might be a timestamp (e.g. yyyymmdd) if a managed version is 2288 * not available. There is also no expectation that versions can be 2289 * placed in a lexicographical sequence. To provide a version 2290 * consistent with the Decision Support Service specification, use 2291 * the format Major.Minor.Revision (e.g. 1.0.0). For more 2292 * information on versioning knowledge assets, refer to the 2293 * Decision Support Service specification. Note that a version is 2294 * required for non-experimental active artifacts.). This is the 2295 * underlying object with id, value and extensions. The accessor 2296 * "getVersion" gives direct access to the value 2297 */ 2298 public ResearchElementDefinition setVersionElement(StringType value) { 2299 this.version = value; 2300 return this; 2301 } 2302 2303 /** 2304 * @return The identifier that is used to identify this version of the research 2305 * element definition when it is referenced in a specification, model, 2306 * design or instance. This is an arbitrary value managed by the 2307 * research element definition author and is not expected to be globally 2308 * unique. For example, it might be a timestamp (e.g. yyyymmdd) if a 2309 * managed version is not available. There is also no expectation that 2310 * versions can be placed in a lexicographical sequence. To provide a 2311 * version consistent with the Decision Support Service specification, 2312 * use the format Major.Minor.Revision (e.g. 1.0.0). For more 2313 * information on versioning knowledge assets, refer to the Decision 2314 * Support Service specification. Note that a version is required for 2315 * non-experimental active artifacts. 2316 */ 2317 public String getVersion() { 2318 return this.version == null ? null : this.version.getValue(); 2319 } 2320 2321 /** 2322 * @param value The identifier that is used to identify this version of the 2323 * research element definition when it is referenced in a 2324 * specification, model, design or instance. This is an arbitrary 2325 * value managed by the research element definition author and is 2326 * not expected to be globally unique. For example, it might be a 2327 * timestamp (e.g. yyyymmdd) if a managed version is not available. 2328 * There is also no expectation that versions can be placed in a 2329 * lexicographical sequence. To provide a version consistent with 2330 * the Decision Support Service specification, use the format 2331 * Major.Minor.Revision (e.g. 1.0.0). For more information on 2332 * versioning knowledge assets, refer to the Decision Support 2333 * Service specification. Note that a version is required for 2334 * non-experimental active artifacts. 2335 */ 2336 public ResearchElementDefinition setVersion(String value) { 2337 if (Utilities.noString(value)) 2338 this.version = null; 2339 else { 2340 if (this.version == null) 2341 this.version = new StringType(); 2342 this.version.setValue(value); 2343 } 2344 return this; 2345 } 2346 2347 /** 2348 * @return {@link #name} (A natural language name identifying the research 2349 * element definition. This name should be usable as an identifier for 2350 * the module by machine processing applications such as code 2351 * generation.). This is the underlying object with id, value and 2352 * extensions. The accessor "getName" gives direct access to the value 2353 */ 2354 public StringType getNameElement() { 2355 if (this.name == null) 2356 if (Configuration.errorOnAutoCreate()) 2357 throw new Error("Attempt to auto-create ResearchElementDefinition.name"); 2358 else if (Configuration.doAutoCreate()) 2359 this.name = new StringType(); // bb 2360 return this.name; 2361 } 2362 2363 public boolean hasNameElement() { 2364 return this.name != null && !this.name.isEmpty(); 2365 } 2366 2367 public boolean hasName() { 2368 return this.name != null && !this.name.isEmpty(); 2369 } 2370 2371 /** 2372 * @param value {@link #name} (A natural language name identifying the research 2373 * element definition. This name should be usable as an identifier 2374 * for the module by machine processing applications such as code 2375 * generation.). This is the underlying object with id, value and 2376 * extensions. The accessor "getName" gives direct access to the 2377 * value 2378 */ 2379 public ResearchElementDefinition setNameElement(StringType value) { 2380 this.name = value; 2381 return this; 2382 } 2383 2384 /** 2385 * @return A natural language name identifying the research element definition. 2386 * This name should be usable as an identifier for the module by machine 2387 * processing applications such as code generation. 2388 */ 2389 public String getName() { 2390 return this.name == null ? null : this.name.getValue(); 2391 } 2392 2393 /** 2394 * @param value A natural language name identifying the research element 2395 * definition. This name should be usable as an identifier for the 2396 * module by machine processing applications such as code 2397 * generation. 2398 */ 2399 public ResearchElementDefinition setName(String value) { 2400 if (Utilities.noString(value)) 2401 this.name = null; 2402 else { 2403 if (this.name == null) 2404 this.name = new StringType(); 2405 this.name.setValue(value); 2406 } 2407 return this; 2408 } 2409 2410 /** 2411 * @return {@link #title} (A short, descriptive, user-friendly title for the 2412 * research element definition.). This is the underlying object with id, 2413 * value and extensions. The accessor "getTitle" gives direct access to 2414 * the value 2415 */ 2416 public StringType getTitleElement() { 2417 if (this.title == null) 2418 if (Configuration.errorOnAutoCreate()) 2419 throw new Error("Attempt to auto-create ResearchElementDefinition.title"); 2420 else if (Configuration.doAutoCreate()) 2421 this.title = new StringType(); // bb 2422 return this.title; 2423 } 2424 2425 public boolean hasTitleElement() { 2426 return this.title != null && !this.title.isEmpty(); 2427 } 2428 2429 public boolean hasTitle() { 2430 return this.title != null && !this.title.isEmpty(); 2431 } 2432 2433 /** 2434 * @param value {@link #title} (A short, descriptive, user-friendly title for 2435 * the research element definition.). This is the underlying object 2436 * with id, value and extensions. The accessor "getTitle" gives 2437 * direct access to the value 2438 */ 2439 public ResearchElementDefinition setTitleElement(StringType value) { 2440 this.title = value; 2441 return this; 2442 } 2443 2444 /** 2445 * @return A short, descriptive, user-friendly title for the research element 2446 * definition. 2447 */ 2448 public String getTitle() { 2449 return this.title == null ? null : this.title.getValue(); 2450 } 2451 2452 /** 2453 * @param value A short, descriptive, user-friendly title for the research 2454 * element definition. 2455 */ 2456 public ResearchElementDefinition setTitle(String value) { 2457 if (Utilities.noString(value)) 2458 this.title = null; 2459 else { 2460 if (this.title == null) 2461 this.title = new StringType(); 2462 this.title.setValue(value); 2463 } 2464 return this; 2465 } 2466 2467 /** 2468 * @return {@link #shortTitle} (The short title provides an alternate title for 2469 * use in informal descriptive contexts where the full, formal title is 2470 * not necessary.). This is the underlying object with id, value and 2471 * extensions. The accessor "getShortTitle" gives direct access to the 2472 * value 2473 */ 2474 public StringType getShortTitleElement() { 2475 if (this.shortTitle == null) 2476 if (Configuration.errorOnAutoCreate()) 2477 throw new Error("Attempt to auto-create ResearchElementDefinition.shortTitle"); 2478 else if (Configuration.doAutoCreate()) 2479 this.shortTitle = new StringType(); // bb 2480 return this.shortTitle; 2481 } 2482 2483 public boolean hasShortTitleElement() { 2484 return this.shortTitle != null && !this.shortTitle.isEmpty(); 2485 } 2486 2487 public boolean hasShortTitle() { 2488 return this.shortTitle != null && !this.shortTitle.isEmpty(); 2489 } 2490 2491 /** 2492 * @param value {@link #shortTitle} (The short title provides an alternate title 2493 * for use in informal descriptive contexts where the full, formal 2494 * title is not necessary.). This is the underlying object with id, 2495 * value and extensions. The accessor "getShortTitle" gives direct 2496 * access to the value 2497 */ 2498 public ResearchElementDefinition setShortTitleElement(StringType value) { 2499 this.shortTitle = value; 2500 return this; 2501 } 2502 2503 /** 2504 * @return The short title provides an alternate title for use in informal 2505 * descriptive contexts where the full, formal title is not necessary. 2506 */ 2507 public String getShortTitle() { 2508 return this.shortTitle == null ? null : this.shortTitle.getValue(); 2509 } 2510 2511 /** 2512 * @param value The short title provides an alternate title for use in informal 2513 * descriptive contexts where the full, formal title is not 2514 * necessary. 2515 */ 2516 public ResearchElementDefinition setShortTitle(String value) { 2517 if (Utilities.noString(value)) 2518 this.shortTitle = null; 2519 else { 2520 if (this.shortTitle == null) 2521 this.shortTitle = new StringType(); 2522 this.shortTitle.setValue(value); 2523 } 2524 return this; 2525 } 2526 2527 /** 2528 * @return {@link #subtitle} (An explanatory or alternate title for the 2529 * ResearchElementDefinition giving additional information about its 2530 * content.). This is the underlying object with id, value and 2531 * extensions. The accessor "getSubtitle" gives direct access to the 2532 * value 2533 */ 2534 public StringType getSubtitleElement() { 2535 if (this.subtitle == null) 2536 if (Configuration.errorOnAutoCreate()) 2537 throw new Error("Attempt to auto-create ResearchElementDefinition.subtitle"); 2538 else if (Configuration.doAutoCreate()) 2539 this.subtitle = new StringType(); // bb 2540 return this.subtitle; 2541 } 2542 2543 public boolean hasSubtitleElement() { 2544 return this.subtitle != null && !this.subtitle.isEmpty(); 2545 } 2546 2547 public boolean hasSubtitle() { 2548 return this.subtitle != null && !this.subtitle.isEmpty(); 2549 } 2550 2551 /** 2552 * @param value {@link #subtitle} (An explanatory or alternate title for the 2553 * ResearchElementDefinition giving additional information about 2554 * its content.). This is the underlying object with id, value and 2555 * extensions. The accessor "getSubtitle" gives direct access to 2556 * the value 2557 */ 2558 public ResearchElementDefinition setSubtitleElement(StringType value) { 2559 this.subtitle = value; 2560 return this; 2561 } 2562 2563 /** 2564 * @return An explanatory or alternate title for the ResearchElementDefinition 2565 * giving additional information about its content. 2566 */ 2567 public String getSubtitle() { 2568 return this.subtitle == null ? null : this.subtitle.getValue(); 2569 } 2570 2571 /** 2572 * @param value An explanatory or alternate title for the 2573 * ResearchElementDefinition giving additional information about 2574 * its content. 2575 */ 2576 public ResearchElementDefinition setSubtitle(String value) { 2577 if (Utilities.noString(value)) 2578 this.subtitle = null; 2579 else { 2580 if (this.subtitle == null) 2581 this.subtitle = new StringType(); 2582 this.subtitle.setValue(value); 2583 } 2584 return this; 2585 } 2586 2587 /** 2588 * @return {@link #status} (The status of this research element definition. 2589 * Enables tracking the life-cycle of the content.). This is the 2590 * underlying object with id, value and extensions. The accessor 2591 * "getStatus" gives direct access to the value 2592 */ 2593 public Enumeration<PublicationStatus> getStatusElement() { 2594 if (this.status == null) 2595 if (Configuration.errorOnAutoCreate()) 2596 throw new Error("Attempt to auto-create ResearchElementDefinition.status"); 2597 else if (Configuration.doAutoCreate()) 2598 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2599 return this.status; 2600 } 2601 2602 public boolean hasStatusElement() { 2603 return this.status != null && !this.status.isEmpty(); 2604 } 2605 2606 public boolean hasStatus() { 2607 return this.status != null && !this.status.isEmpty(); 2608 } 2609 2610 /** 2611 * @param value {@link #status} (The status of this research element definition. 2612 * Enables tracking the life-cycle of the content.). This is the 2613 * underlying object with id, value and extensions. The accessor 2614 * "getStatus" gives direct access to the value 2615 */ 2616 public ResearchElementDefinition setStatusElement(Enumeration<PublicationStatus> value) { 2617 this.status = value; 2618 return this; 2619 } 2620 2621 /** 2622 * @return The status of this research element definition. Enables tracking the 2623 * life-cycle of the content. 2624 */ 2625 public PublicationStatus getStatus() { 2626 return this.status == null ? null : this.status.getValue(); 2627 } 2628 2629 /** 2630 * @param value The status of this research element definition. Enables tracking 2631 * the life-cycle of the content. 2632 */ 2633 public ResearchElementDefinition setStatus(PublicationStatus value) { 2634 if (this.status == null) 2635 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2636 this.status.setValue(value); 2637 return this; 2638 } 2639 2640 /** 2641 * @return {@link #experimental} (A Boolean value to indicate that this research 2642 * element definition is authored for testing purposes (or 2643 * education/evaluation/marketing) and is not intended to be used for 2644 * genuine usage.). This is the underlying object with id, value and 2645 * extensions. The accessor "getExperimental" gives direct access to the 2646 * value 2647 */ 2648 public BooleanType getExperimentalElement() { 2649 if (this.experimental == null) 2650 if (Configuration.errorOnAutoCreate()) 2651 throw new Error("Attempt to auto-create ResearchElementDefinition.experimental"); 2652 else if (Configuration.doAutoCreate()) 2653 this.experimental = new BooleanType(); // bb 2654 return this.experimental; 2655 } 2656 2657 public boolean hasExperimentalElement() { 2658 return this.experimental != null && !this.experimental.isEmpty(); 2659 } 2660 2661 public boolean hasExperimental() { 2662 return this.experimental != null && !this.experimental.isEmpty(); 2663 } 2664 2665 /** 2666 * @param value {@link #experimental} (A Boolean value to indicate that this 2667 * research element definition is authored for testing purposes (or 2668 * education/evaluation/marketing) and is not intended to be used 2669 * for genuine usage.). This is the underlying object with id, 2670 * value and extensions. The accessor "getExperimental" gives 2671 * direct access to the value 2672 */ 2673 public ResearchElementDefinition setExperimentalElement(BooleanType value) { 2674 this.experimental = value; 2675 return this; 2676 } 2677 2678 /** 2679 * @return A Boolean value to indicate that this research element definition is 2680 * authored for testing purposes (or education/evaluation/marketing) and 2681 * is not intended to be used for genuine usage. 2682 */ 2683 public boolean getExperimental() { 2684 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 2685 } 2686 2687 /** 2688 * @param value A Boolean value to indicate that this research element 2689 * definition is authored for testing purposes (or 2690 * education/evaluation/marketing) and is not intended to be used 2691 * for genuine usage. 2692 */ 2693 public ResearchElementDefinition setExperimental(boolean value) { 2694 if (this.experimental == null) 2695 this.experimental = new BooleanType(); 2696 this.experimental.setValue(value); 2697 return this; 2698 } 2699 2700 /** 2701 * @return {@link #subject} (The intended subjects for the 2702 * ResearchElementDefinition. If this element is not provided, a Patient 2703 * subject is assumed, but the subject of the ResearchElementDefinition 2704 * can be anything.) 2705 */ 2706 public Type getSubject() { 2707 return this.subject; 2708 } 2709 2710 /** 2711 * @return {@link #subject} (The intended subjects for the 2712 * ResearchElementDefinition. If this element is not provided, a Patient 2713 * subject is assumed, but the subject of the ResearchElementDefinition 2714 * can be anything.) 2715 */ 2716 public CodeableConcept getSubjectCodeableConcept() throws FHIRException { 2717 if (this.subject == null) 2718 this.subject = new CodeableConcept(); 2719 if (!(this.subject instanceof CodeableConcept)) 2720 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 2721 + this.subject.getClass().getName() + " was encountered"); 2722 return (CodeableConcept) this.subject; 2723 } 2724 2725 public boolean hasSubjectCodeableConcept() { 2726 return this != null && this.subject instanceof CodeableConcept; 2727 } 2728 2729 /** 2730 * @return {@link #subject} (The intended subjects for the 2731 * ResearchElementDefinition. If this element is not provided, a Patient 2732 * subject is assumed, but the subject of the ResearchElementDefinition 2733 * can be anything.) 2734 */ 2735 public Reference getSubjectReference() throws FHIRException { 2736 if (this.subject == null) 2737 this.subject = new Reference(); 2738 if (!(this.subject instanceof Reference)) 2739 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.subject.getClass().getName() 2740 + " was encountered"); 2741 return (Reference) this.subject; 2742 } 2743 2744 public boolean hasSubjectReference() { 2745 return this != null && this.subject instanceof Reference; 2746 } 2747 2748 public boolean hasSubject() { 2749 return this.subject != null && !this.subject.isEmpty(); 2750 } 2751 2752 /** 2753 * @param value {@link #subject} (The intended subjects for the 2754 * ResearchElementDefinition. If this element is not provided, a 2755 * Patient subject is assumed, but the subject of the 2756 * ResearchElementDefinition can be anything.) 2757 */ 2758 public ResearchElementDefinition setSubject(Type value) { 2759 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2760 throw new Error("Not the right type for ResearchElementDefinition.subject[x]: " + value.fhirType()); 2761 this.subject = value; 2762 return this; 2763 } 2764 2765 /** 2766 * @return {@link #date} (The date (and optionally time) when the research 2767 * element definition was published. The date must change when the 2768 * business version changes and it must change if the status code 2769 * changes. In addition, it should change when the substantive content 2770 * of the research element definition changes.). This is the underlying 2771 * object with id, value and extensions. The accessor "getDate" gives 2772 * direct access to the value 2773 */ 2774 public DateTimeType getDateElement() { 2775 if (this.date == null) 2776 if (Configuration.errorOnAutoCreate()) 2777 throw new Error("Attempt to auto-create ResearchElementDefinition.date"); 2778 else if (Configuration.doAutoCreate()) 2779 this.date = new DateTimeType(); // bb 2780 return this.date; 2781 } 2782 2783 public boolean hasDateElement() { 2784 return this.date != null && !this.date.isEmpty(); 2785 } 2786 2787 public boolean hasDate() { 2788 return this.date != null && !this.date.isEmpty(); 2789 } 2790 2791 /** 2792 * @param value {@link #date} (The date (and optionally time) when the research 2793 * element definition was published. The date must change when the 2794 * business version changes and it must change if the status code 2795 * changes. In addition, it should change when the substantive 2796 * content of the research element definition changes.). This is 2797 * the underlying object with id, value and extensions. The 2798 * accessor "getDate" gives direct access to the value 2799 */ 2800 public ResearchElementDefinition setDateElement(DateTimeType value) { 2801 this.date = value; 2802 return this; 2803 } 2804 2805 /** 2806 * @return The date (and optionally time) when the research element definition 2807 * was published. The date must change when the business version changes 2808 * and it must change if the status code changes. In addition, it should 2809 * change when the substantive content of the research element 2810 * definition changes. 2811 */ 2812 public Date getDate() { 2813 return this.date == null ? null : this.date.getValue(); 2814 } 2815 2816 /** 2817 * @param value The date (and optionally time) when the research element 2818 * definition was published. The date must change when the business 2819 * version changes and it must change if the status code changes. 2820 * In addition, it should change when the substantive content of 2821 * the research element definition changes. 2822 */ 2823 public ResearchElementDefinition setDate(Date value) { 2824 if (value == null) 2825 this.date = null; 2826 else { 2827 if (this.date == null) 2828 this.date = new DateTimeType(); 2829 this.date.setValue(value); 2830 } 2831 return this; 2832 } 2833 2834 /** 2835 * @return {@link #publisher} (The name of the organization or individual that 2836 * published the research element definition.). This is the underlying 2837 * object with id, value and extensions. The accessor "getPublisher" 2838 * gives direct access to the value 2839 */ 2840 public StringType getPublisherElement() { 2841 if (this.publisher == null) 2842 if (Configuration.errorOnAutoCreate()) 2843 throw new Error("Attempt to auto-create ResearchElementDefinition.publisher"); 2844 else if (Configuration.doAutoCreate()) 2845 this.publisher = new StringType(); // bb 2846 return this.publisher; 2847 } 2848 2849 public boolean hasPublisherElement() { 2850 return this.publisher != null && !this.publisher.isEmpty(); 2851 } 2852 2853 public boolean hasPublisher() { 2854 return this.publisher != null && !this.publisher.isEmpty(); 2855 } 2856 2857 /** 2858 * @param value {@link #publisher} (The name of the organization or individual 2859 * that published the research element definition.). This is the 2860 * underlying object with id, value and extensions. The accessor 2861 * "getPublisher" gives direct access to the value 2862 */ 2863 public ResearchElementDefinition setPublisherElement(StringType value) { 2864 this.publisher = value; 2865 return this; 2866 } 2867 2868 /** 2869 * @return The name of the organization or individual that published the 2870 * research element definition. 2871 */ 2872 public String getPublisher() { 2873 return this.publisher == null ? null : this.publisher.getValue(); 2874 } 2875 2876 /** 2877 * @param value The name of the organization or individual that published the 2878 * research element definition. 2879 */ 2880 public ResearchElementDefinition setPublisher(String value) { 2881 if (Utilities.noString(value)) 2882 this.publisher = null; 2883 else { 2884 if (this.publisher == null) 2885 this.publisher = new StringType(); 2886 this.publisher.setValue(value); 2887 } 2888 return this; 2889 } 2890 2891 /** 2892 * @return {@link #contact} (Contact details to assist a user in finding and 2893 * communicating with the publisher.) 2894 */ 2895 public List<ContactDetail> getContact() { 2896 if (this.contact == null) 2897 this.contact = new ArrayList<ContactDetail>(); 2898 return this.contact; 2899 } 2900 2901 /** 2902 * @return Returns a reference to <code>this</code> for easy method chaining 2903 */ 2904 public ResearchElementDefinition setContact(List<ContactDetail> theContact) { 2905 this.contact = theContact; 2906 return this; 2907 } 2908 2909 public boolean hasContact() { 2910 if (this.contact == null) 2911 return false; 2912 for (ContactDetail item : this.contact) 2913 if (!item.isEmpty()) 2914 return true; 2915 return false; 2916 } 2917 2918 public ContactDetail addContact() { // 3 2919 ContactDetail t = new ContactDetail(); 2920 if (this.contact == null) 2921 this.contact = new ArrayList<ContactDetail>(); 2922 this.contact.add(t); 2923 return t; 2924 } 2925 2926 public ResearchElementDefinition addContact(ContactDetail t) { // 3 2927 if (t == null) 2928 return this; 2929 if (this.contact == null) 2930 this.contact = new ArrayList<ContactDetail>(); 2931 this.contact.add(t); 2932 return this; 2933 } 2934 2935 /** 2936 * @return The first repetition of repeating field {@link #contact}, creating it 2937 * if it does not already exist 2938 */ 2939 public ContactDetail getContactFirstRep() { 2940 if (getContact().isEmpty()) { 2941 addContact(); 2942 } 2943 return getContact().get(0); 2944 } 2945 2946 /** 2947 * @return {@link #description} (A free text natural language description of the 2948 * research element definition from a consumer's perspective.). This is 2949 * the underlying object with id, value and extensions. The accessor 2950 * "getDescription" gives direct access to the value 2951 */ 2952 public MarkdownType getDescriptionElement() { 2953 if (this.description == null) 2954 if (Configuration.errorOnAutoCreate()) 2955 throw new Error("Attempt to auto-create ResearchElementDefinition.description"); 2956 else if (Configuration.doAutoCreate()) 2957 this.description = new MarkdownType(); // bb 2958 return this.description; 2959 } 2960 2961 public boolean hasDescriptionElement() { 2962 return this.description != null && !this.description.isEmpty(); 2963 } 2964 2965 public boolean hasDescription() { 2966 return this.description != null && !this.description.isEmpty(); 2967 } 2968 2969 /** 2970 * @param value {@link #description} (A free text natural language description 2971 * of the research element definition from a consumer's 2972 * perspective.). This is the underlying object with id, value and 2973 * extensions. The accessor "getDescription" gives direct access to 2974 * the value 2975 */ 2976 public ResearchElementDefinition setDescriptionElement(MarkdownType value) { 2977 this.description = value; 2978 return this; 2979 } 2980 2981 /** 2982 * @return A free text natural language description of the research element 2983 * definition from a consumer's perspective. 2984 */ 2985 public String getDescription() { 2986 return this.description == null ? null : this.description.getValue(); 2987 } 2988 2989 /** 2990 * @param value A free text natural language description of the research element 2991 * definition from a consumer's perspective. 2992 */ 2993 public ResearchElementDefinition setDescription(String value) { 2994 if (value == null) 2995 this.description = null; 2996 else { 2997 if (this.description == null) 2998 this.description = new MarkdownType(); 2999 this.description.setValue(value); 3000 } 3001 return this; 3002 } 3003 3004 /** 3005 * @return {@link #comment} (A human-readable string to clarify or explain 3006 * concepts about the resource.) 3007 */ 3008 public List<StringType> getComment() { 3009 if (this.comment == null) 3010 this.comment = new ArrayList<StringType>(); 3011 return this.comment; 3012 } 3013 3014 /** 3015 * @return Returns a reference to <code>this</code> for easy method chaining 3016 */ 3017 public ResearchElementDefinition setComment(List<StringType> theComment) { 3018 this.comment = theComment; 3019 return this; 3020 } 3021 3022 public boolean hasComment() { 3023 if (this.comment == null) 3024 return false; 3025 for (StringType item : this.comment) 3026 if (!item.isEmpty()) 3027 return true; 3028 return false; 3029 } 3030 3031 /** 3032 * @return {@link #comment} (A human-readable string to clarify or explain 3033 * concepts about the resource.) 3034 */ 3035 public StringType addCommentElement() {// 2 3036 StringType t = new StringType(); 3037 if (this.comment == null) 3038 this.comment = new ArrayList<StringType>(); 3039 this.comment.add(t); 3040 return t; 3041 } 3042 3043 /** 3044 * @param value {@link #comment} (A human-readable string to clarify or explain 3045 * concepts about the resource.) 3046 */ 3047 public ResearchElementDefinition addComment(String value) { // 1 3048 StringType t = new StringType(); 3049 t.setValue(value); 3050 if (this.comment == null) 3051 this.comment = new ArrayList<StringType>(); 3052 this.comment.add(t); 3053 return this; 3054 } 3055 3056 /** 3057 * @param value {@link #comment} (A human-readable string to clarify or explain 3058 * concepts about the resource.) 3059 */ 3060 public boolean hasComment(String value) { 3061 if (this.comment == null) 3062 return false; 3063 for (StringType v : this.comment) 3064 if (v.getValue().equals(value)) // string 3065 return true; 3066 return false; 3067 } 3068 3069 /** 3070 * @return {@link #useContext} (The content was developed with a focus and 3071 * intent of supporting the contexts that are listed. These contexts may 3072 * be general categories (gender, age, ...) or may be references to 3073 * specific programs (insurance plans, studies, ...) and may be used to 3074 * assist with indexing and searching for appropriate research element 3075 * definition instances.) 3076 */ 3077 public List<UsageContext> getUseContext() { 3078 if (this.useContext == null) 3079 this.useContext = new ArrayList<UsageContext>(); 3080 return this.useContext; 3081 } 3082 3083 /** 3084 * @return Returns a reference to <code>this</code> for easy method chaining 3085 */ 3086 public ResearchElementDefinition setUseContext(List<UsageContext> theUseContext) { 3087 this.useContext = theUseContext; 3088 return this; 3089 } 3090 3091 public boolean hasUseContext() { 3092 if (this.useContext == null) 3093 return false; 3094 for (UsageContext item : this.useContext) 3095 if (!item.isEmpty()) 3096 return true; 3097 return false; 3098 } 3099 3100 public UsageContext addUseContext() { // 3 3101 UsageContext t = new UsageContext(); 3102 if (this.useContext == null) 3103 this.useContext = new ArrayList<UsageContext>(); 3104 this.useContext.add(t); 3105 return t; 3106 } 3107 3108 public ResearchElementDefinition addUseContext(UsageContext t) { // 3 3109 if (t == null) 3110 return this; 3111 if (this.useContext == null) 3112 this.useContext = new ArrayList<UsageContext>(); 3113 this.useContext.add(t); 3114 return this; 3115 } 3116 3117 /** 3118 * @return The first repetition of repeating field {@link #useContext}, creating 3119 * it if it does not already exist 3120 */ 3121 public UsageContext getUseContextFirstRep() { 3122 if (getUseContext().isEmpty()) { 3123 addUseContext(); 3124 } 3125 return getUseContext().get(0); 3126 } 3127 3128 /** 3129 * @return {@link #jurisdiction} (A legal or geographic region in which the 3130 * research element definition is intended to be used.) 3131 */ 3132 public List<CodeableConcept> getJurisdiction() { 3133 if (this.jurisdiction == null) 3134 this.jurisdiction = new ArrayList<CodeableConcept>(); 3135 return this.jurisdiction; 3136 } 3137 3138 /** 3139 * @return Returns a reference to <code>this</code> for easy method chaining 3140 */ 3141 public ResearchElementDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 3142 this.jurisdiction = theJurisdiction; 3143 return this; 3144 } 3145 3146 public boolean hasJurisdiction() { 3147 if (this.jurisdiction == null) 3148 return false; 3149 for (CodeableConcept item : this.jurisdiction) 3150 if (!item.isEmpty()) 3151 return true; 3152 return false; 3153 } 3154 3155 public CodeableConcept addJurisdiction() { // 3 3156 CodeableConcept t = new CodeableConcept(); 3157 if (this.jurisdiction == null) 3158 this.jurisdiction = new ArrayList<CodeableConcept>(); 3159 this.jurisdiction.add(t); 3160 return t; 3161 } 3162 3163 public ResearchElementDefinition addJurisdiction(CodeableConcept t) { // 3 3164 if (t == null) 3165 return this; 3166 if (this.jurisdiction == null) 3167 this.jurisdiction = new ArrayList<CodeableConcept>(); 3168 this.jurisdiction.add(t); 3169 return this; 3170 } 3171 3172 /** 3173 * @return The first repetition of repeating field {@link #jurisdiction}, 3174 * creating it if it does not already exist 3175 */ 3176 public CodeableConcept getJurisdictionFirstRep() { 3177 if (getJurisdiction().isEmpty()) { 3178 addJurisdiction(); 3179 } 3180 return getJurisdiction().get(0); 3181 } 3182 3183 /** 3184 * @return {@link #purpose} (Explanation of why this research element definition 3185 * is needed and why it has been designed as it has.). This is the 3186 * underlying object with id, value and extensions. The accessor 3187 * "getPurpose" gives direct access to the value 3188 */ 3189 public MarkdownType getPurposeElement() { 3190 if (this.purpose == null) 3191 if (Configuration.errorOnAutoCreate()) 3192 throw new Error("Attempt to auto-create ResearchElementDefinition.purpose"); 3193 else if (Configuration.doAutoCreate()) 3194 this.purpose = new MarkdownType(); // bb 3195 return this.purpose; 3196 } 3197 3198 public boolean hasPurposeElement() { 3199 return this.purpose != null && !this.purpose.isEmpty(); 3200 } 3201 3202 public boolean hasPurpose() { 3203 return this.purpose != null && !this.purpose.isEmpty(); 3204 } 3205 3206 /** 3207 * @param value {@link #purpose} (Explanation of why this research element 3208 * definition is needed and why it has been designed as it has.). 3209 * This is the underlying object with id, value and extensions. The 3210 * accessor "getPurpose" gives direct access to the value 3211 */ 3212 public ResearchElementDefinition setPurposeElement(MarkdownType value) { 3213 this.purpose = value; 3214 return this; 3215 } 3216 3217 /** 3218 * @return Explanation of why this research element definition is needed and why 3219 * it has been designed as it has. 3220 */ 3221 public String getPurpose() { 3222 return this.purpose == null ? null : this.purpose.getValue(); 3223 } 3224 3225 /** 3226 * @param value Explanation of why this research element definition is needed 3227 * and why it has been designed as it has. 3228 */ 3229 public ResearchElementDefinition setPurpose(String value) { 3230 if (value == null) 3231 this.purpose = null; 3232 else { 3233 if (this.purpose == null) 3234 this.purpose = new MarkdownType(); 3235 this.purpose.setValue(value); 3236 } 3237 return this; 3238 } 3239 3240 /** 3241 * @return {@link #usage} (A detailed description, from a clinical perspective, 3242 * of how the ResearchElementDefinition is used.). This is the 3243 * underlying object with id, value and extensions. The accessor 3244 * "getUsage" gives direct access to the value 3245 */ 3246 public StringType getUsageElement() { 3247 if (this.usage == null) 3248 if (Configuration.errorOnAutoCreate()) 3249 throw new Error("Attempt to auto-create ResearchElementDefinition.usage"); 3250 else if (Configuration.doAutoCreate()) 3251 this.usage = new StringType(); // bb 3252 return this.usage; 3253 } 3254 3255 public boolean hasUsageElement() { 3256 return this.usage != null && !this.usage.isEmpty(); 3257 } 3258 3259 public boolean hasUsage() { 3260 return this.usage != null && !this.usage.isEmpty(); 3261 } 3262 3263 /** 3264 * @param value {@link #usage} (A detailed description, from a clinical 3265 * perspective, of how the ResearchElementDefinition is used.). 3266 * This is the underlying object with id, value and extensions. The 3267 * accessor "getUsage" gives direct access to the value 3268 */ 3269 public ResearchElementDefinition setUsageElement(StringType value) { 3270 this.usage = value; 3271 return this; 3272 } 3273 3274 /** 3275 * @return A detailed description, from a clinical perspective, of how the 3276 * ResearchElementDefinition is used. 3277 */ 3278 public String getUsage() { 3279 return this.usage == null ? null : this.usage.getValue(); 3280 } 3281 3282 /** 3283 * @param value A detailed description, from a clinical perspective, of how the 3284 * ResearchElementDefinition is used. 3285 */ 3286 public ResearchElementDefinition setUsage(String value) { 3287 if (Utilities.noString(value)) 3288 this.usage = null; 3289 else { 3290 if (this.usage == null) 3291 this.usage = new StringType(); 3292 this.usage.setValue(value); 3293 } 3294 return this; 3295 } 3296 3297 /** 3298 * @return {@link #copyright} (A copyright statement relating to the research 3299 * element definition and/or its contents. Copyright statements are 3300 * generally legal restrictions on the use and publishing of the 3301 * research element definition.). This is the underlying object with id, 3302 * value and extensions. The accessor "getCopyright" gives direct access 3303 * to the value 3304 */ 3305 public MarkdownType getCopyrightElement() { 3306 if (this.copyright == null) 3307 if (Configuration.errorOnAutoCreate()) 3308 throw new Error("Attempt to auto-create ResearchElementDefinition.copyright"); 3309 else if (Configuration.doAutoCreate()) 3310 this.copyright = new MarkdownType(); // bb 3311 return this.copyright; 3312 } 3313 3314 public boolean hasCopyrightElement() { 3315 return this.copyright != null && !this.copyright.isEmpty(); 3316 } 3317 3318 public boolean hasCopyright() { 3319 return this.copyright != null && !this.copyright.isEmpty(); 3320 } 3321 3322 /** 3323 * @param value {@link #copyright} (A copyright statement relating to the 3324 * research element definition and/or its contents. Copyright 3325 * statements are generally legal restrictions on the use and 3326 * publishing of the research element definition.). This is the 3327 * underlying object with id, value and extensions. The accessor 3328 * "getCopyright" gives direct access to the value 3329 */ 3330 public ResearchElementDefinition setCopyrightElement(MarkdownType value) { 3331 this.copyright = value; 3332 return this; 3333 } 3334 3335 /** 3336 * @return A copyright statement relating to the research element definition 3337 * and/or its contents. Copyright statements are generally legal 3338 * restrictions on the use and publishing of the research element 3339 * definition. 3340 */ 3341 public String getCopyright() { 3342 return this.copyright == null ? null : this.copyright.getValue(); 3343 } 3344 3345 /** 3346 * @param value A copyright statement relating to the research element 3347 * definition and/or its contents. Copyright statements are 3348 * generally legal restrictions on the use and publishing of the 3349 * research element definition. 3350 */ 3351 public ResearchElementDefinition setCopyright(String value) { 3352 if (value == null) 3353 this.copyright = null; 3354 else { 3355 if (this.copyright == null) 3356 this.copyright = new MarkdownType(); 3357 this.copyright.setValue(value); 3358 } 3359 return this; 3360 } 3361 3362 /** 3363 * @return {@link #approvalDate} (The date on which the resource content was 3364 * approved by the publisher. Approval happens once when the content is 3365 * officially approved for usage.). This is the underlying object with 3366 * id, value and extensions. The accessor "getApprovalDate" gives direct 3367 * access to the value 3368 */ 3369 public DateType getApprovalDateElement() { 3370 if (this.approvalDate == null) 3371 if (Configuration.errorOnAutoCreate()) 3372 throw new Error("Attempt to auto-create ResearchElementDefinition.approvalDate"); 3373 else if (Configuration.doAutoCreate()) 3374 this.approvalDate = new DateType(); // bb 3375 return this.approvalDate; 3376 } 3377 3378 public boolean hasApprovalDateElement() { 3379 return this.approvalDate != null && !this.approvalDate.isEmpty(); 3380 } 3381 3382 public boolean hasApprovalDate() { 3383 return this.approvalDate != null && !this.approvalDate.isEmpty(); 3384 } 3385 3386 /** 3387 * @param value {@link #approvalDate} (The date on which the resource content 3388 * was approved by the publisher. Approval happens once when the 3389 * content is officially approved for usage.). This is the 3390 * underlying object with id, value and extensions. The accessor 3391 * "getApprovalDate" gives direct access to the value 3392 */ 3393 public ResearchElementDefinition setApprovalDateElement(DateType value) { 3394 this.approvalDate = value; 3395 return this; 3396 } 3397 3398 /** 3399 * @return The date on which the resource content was approved by the publisher. 3400 * Approval happens once when the content is officially approved for 3401 * usage. 3402 */ 3403 public Date getApprovalDate() { 3404 return this.approvalDate == null ? null : this.approvalDate.getValue(); 3405 } 3406 3407 /** 3408 * @param value The date on which the resource content was approved by the 3409 * publisher. Approval happens once when the content is officially 3410 * approved for usage. 3411 */ 3412 public ResearchElementDefinition setApprovalDate(Date value) { 3413 if (value == null) 3414 this.approvalDate = null; 3415 else { 3416 if (this.approvalDate == null) 3417 this.approvalDate = new DateType(); 3418 this.approvalDate.setValue(value); 3419 } 3420 return this; 3421 } 3422 3423 /** 3424 * @return {@link #lastReviewDate} (The date on which the resource content was 3425 * last reviewed. Review happens periodically after approval but does 3426 * not change the original approval date.). This is the underlying 3427 * object with id, value and extensions. The accessor 3428 * "getLastReviewDate" gives direct access to the value 3429 */ 3430 public DateType getLastReviewDateElement() { 3431 if (this.lastReviewDate == null) 3432 if (Configuration.errorOnAutoCreate()) 3433 throw new Error("Attempt to auto-create ResearchElementDefinition.lastReviewDate"); 3434 else if (Configuration.doAutoCreate()) 3435 this.lastReviewDate = new DateType(); // bb 3436 return this.lastReviewDate; 3437 } 3438 3439 public boolean hasLastReviewDateElement() { 3440 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 3441 } 3442 3443 public boolean hasLastReviewDate() { 3444 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 3445 } 3446 3447 /** 3448 * @param value {@link #lastReviewDate} (The date on which the resource content 3449 * was last reviewed. Review happens periodically after approval 3450 * but does not change the original approval date.). This is the 3451 * underlying object with id, value and extensions. The accessor 3452 * "getLastReviewDate" gives direct access to the value 3453 */ 3454 public ResearchElementDefinition setLastReviewDateElement(DateType value) { 3455 this.lastReviewDate = value; 3456 return this; 3457 } 3458 3459 /** 3460 * @return The date on which the resource content was last reviewed. Review 3461 * happens periodically after approval but does not change the original 3462 * approval date. 3463 */ 3464 public Date getLastReviewDate() { 3465 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 3466 } 3467 3468 /** 3469 * @param value The date on which the resource content was last reviewed. Review 3470 * happens periodically after approval but does not change the 3471 * original approval date. 3472 */ 3473 public ResearchElementDefinition setLastReviewDate(Date value) { 3474 if (value == null) 3475 this.lastReviewDate = null; 3476 else { 3477 if (this.lastReviewDate == null) 3478 this.lastReviewDate = new DateType(); 3479 this.lastReviewDate.setValue(value); 3480 } 3481 return this; 3482 } 3483 3484 /** 3485 * @return {@link #effectivePeriod} (The period during which the research 3486 * element definition content was or is planned to be in active use.) 3487 */ 3488 public Period getEffectivePeriod() { 3489 if (this.effectivePeriod == null) 3490 if (Configuration.errorOnAutoCreate()) 3491 throw new Error("Attempt to auto-create ResearchElementDefinition.effectivePeriod"); 3492 else if (Configuration.doAutoCreate()) 3493 this.effectivePeriod = new Period(); // cc 3494 return this.effectivePeriod; 3495 } 3496 3497 public boolean hasEffectivePeriod() { 3498 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 3499 } 3500 3501 /** 3502 * @param value {@link #effectivePeriod} (The period during which the research 3503 * element definition content was or is planned to be in active 3504 * use.) 3505 */ 3506 public ResearchElementDefinition setEffectivePeriod(Period value) { 3507 this.effectivePeriod = value; 3508 return this; 3509 } 3510 3511 /** 3512 * @return {@link #topic} (Descriptive topics related to the content of the 3513 * ResearchElementDefinition. Topics provide a high-level categorization 3514 * grouping types of ResearchElementDefinitions that can be useful for 3515 * filtering and searching.) 3516 */ 3517 public List<CodeableConcept> getTopic() { 3518 if (this.topic == null) 3519 this.topic = new ArrayList<CodeableConcept>(); 3520 return this.topic; 3521 } 3522 3523 /** 3524 * @return Returns a reference to <code>this</code> for easy method chaining 3525 */ 3526 public ResearchElementDefinition setTopic(List<CodeableConcept> theTopic) { 3527 this.topic = theTopic; 3528 return this; 3529 } 3530 3531 public boolean hasTopic() { 3532 if (this.topic == null) 3533 return false; 3534 for (CodeableConcept item : this.topic) 3535 if (!item.isEmpty()) 3536 return true; 3537 return false; 3538 } 3539 3540 public CodeableConcept addTopic() { // 3 3541 CodeableConcept t = new CodeableConcept(); 3542 if (this.topic == null) 3543 this.topic = new ArrayList<CodeableConcept>(); 3544 this.topic.add(t); 3545 return t; 3546 } 3547 3548 public ResearchElementDefinition addTopic(CodeableConcept t) { // 3 3549 if (t == null) 3550 return this; 3551 if (this.topic == null) 3552 this.topic = new ArrayList<CodeableConcept>(); 3553 this.topic.add(t); 3554 return this; 3555 } 3556 3557 /** 3558 * @return The first repetition of repeating field {@link #topic}, creating it 3559 * if it does not already exist 3560 */ 3561 public CodeableConcept getTopicFirstRep() { 3562 if (getTopic().isEmpty()) { 3563 addTopic(); 3564 } 3565 return getTopic().get(0); 3566 } 3567 3568 /** 3569 * @return {@link #author} (An individiual or organization primarily involved in 3570 * the creation and maintenance of the content.) 3571 */ 3572 public List<ContactDetail> getAuthor() { 3573 if (this.author == null) 3574 this.author = new ArrayList<ContactDetail>(); 3575 return this.author; 3576 } 3577 3578 /** 3579 * @return Returns a reference to <code>this</code> for easy method chaining 3580 */ 3581 public ResearchElementDefinition setAuthor(List<ContactDetail> theAuthor) { 3582 this.author = theAuthor; 3583 return this; 3584 } 3585 3586 public boolean hasAuthor() { 3587 if (this.author == null) 3588 return false; 3589 for (ContactDetail item : this.author) 3590 if (!item.isEmpty()) 3591 return true; 3592 return false; 3593 } 3594 3595 public ContactDetail addAuthor() { // 3 3596 ContactDetail t = new ContactDetail(); 3597 if (this.author == null) 3598 this.author = new ArrayList<ContactDetail>(); 3599 this.author.add(t); 3600 return t; 3601 } 3602 3603 public ResearchElementDefinition addAuthor(ContactDetail t) { // 3 3604 if (t == null) 3605 return this; 3606 if (this.author == null) 3607 this.author = new ArrayList<ContactDetail>(); 3608 this.author.add(t); 3609 return this; 3610 } 3611 3612 /** 3613 * @return The first repetition of repeating field {@link #author}, creating it 3614 * if it does not already exist 3615 */ 3616 public ContactDetail getAuthorFirstRep() { 3617 if (getAuthor().isEmpty()) { 3618 addAuthor(); 3619 } 3620 return getAuthor().get(0); 3621 } 3622 3623 /** 3624 * @return {@link #editor} (An individual or organization primarily responsible 3625 * for internal coherence of the content.) 3626 */ 3627 public List<ContactDetail> getEditor() { 3628 if (this.editor == null) 3629 this.editor = new ArrayList<ContactDetail>(); 3630 return this.editor; 3631 } 3632 3633 /** 3634 * @return Returns a reference to <code>this</code> for easy method chaining 3635 */ 3636 public ResearchElementDefinition setEditor(List<ContactDetail> theEditor) { 3637 this.editor = theEditor; 3638 return this; 3639 } 3640 3641 public boolean hasEditor() { 3642 if (this.editor == null) 3643 return false; 3644 for (ContactDetail item : this.editor) 3645 if (!item.isEmpty()) 3646 return true; 3647 return false; 3648 } 3649 3650 public ContactDetail addEditor() { // 3 3651 ContactDetail t = new ContactDetail(); 3652 if (this.editor == null) 3653 this.editor = new ArrayList<ContactDetail>(); 3654 this.editor.add(t); 3655 return t; 3656 } 3657 3658 public ResearchElementDefinition addEditor(ContactDetail t) { // 3 3659 if (t == null) 3660 return this; 3661 if (this.editor == null) 3662 this.editor = new ArrayList<ContactDetail>(); 3663 this.editor.add(t); 3664 return this; 3665 } 3666 3667 /** 3668 * @return The first repetition of repeating field {@link #editor}, creating it 3669 * if it does not already exist 3670 */ 3671 public ContactDetail getEditorFirstRep() { 3672 if (getEditor().isEmpty()) { 3673 addEditor(); 3674 } 3675 return getEditor().get(0); 3676 } 3677 3678 /** 3679 * @return {@link #reviewer} (An individual or organization primarily 3680 * responsible for review of some aspect of the content.) 3681 */ 3682 public List<ContactDetail> getReviewer() { 3683 if (this.reviewer == null) 3684 this.reviewer = new ArrayList<ContactDetail>(); 3685 return this.reviewer; 3686 } 3687 3688 /** 3689 * @return Returns a reference to <code>this</code> for easy method chaining 3690 */ 3691 public ResearchElementDefinition setReviewer(List<ContactDetail> theReviewer) { 3692 this.reviewer = theReviewer; 3693 return this; 3694 } 3695 3696 public boolean hasReviewer() { 3697 if (this.reviewer == null) 3698 return false; 3699 for (ContactDetail item : this.reviewer) 3700 if (!item.isEmpty()) 3701 return true; 3702 return false; 3703 } 3704 3705 public ContactDetail addReviewer() { // 3 3706 ContactDetail t = new ContactDetail(); 3707 if (this.reviewer == null) 3708 this.reviewer = new ArrayList<ContactDetail>(); 3709 this.reviewer.add(t); 3710 return t; 3711 } 3712 3713 public ResearchElementDefinition addReviewer(ContactDetail t) { // 3 3714 if (t == null) 3715 return this; 3716 if (this.reviewer == null) 3717 this.reviewer = new ArrayList<ContactDetail>(); 3718 this.reviewer.add(t); 3719 return this; 3720 } 3721 3722 /** 3723 * @return The first repetition of repeating field {@link #reviewer}, creating 3724 * it if it does not already exist 3725 */ 3726 public ContactDetail getReviewerFirstRep() { 3727 if (getReviewer().isEmpty()) { 3728 addReviewer(); 3729 } 3730 return getReviewer().get(0); 3731 } 3732 3733 /** 3734 * @return {@link #endorser} (An individual or organization responsible for 3735 * officially endorsing the content for use in some setting.) 3736 */ 3737 public List<ContactDetail> getEndorser() { 3738 if (this.endorser == null) 3739 this.endorser = new ArrayList<ContactDetail>(); 3740 return this.endorser; 3741 } 3742 3743 /** 3744 * @return Returns a reference to <code>this</code> for easy method chaining 3745 */ 3746 public ResearchElementDefinition setEndorser(List<ContactDetail> theEndorser) { 3747 this.endorser = theEndorser; 3748 return this; 3749 } 3750 3751 public boolean hasEndorser() { 3752 if (this.endorser == null) 3753 return false; 3754 for (ContactDetail item : this.endorser) 3755 if (!item.isEmpty()) 3756 return true; 3757 return false; 3758 } 3759 3760 public ContactDetail addEndorser() { // 3 3761 ContactDetail t = new ContactDetail(); 3762 if (this.endorser == null) 3763 this.endorser = new ArrayList<ContactDetail>(); 3764 this.endorser.add(t); 3765 return t; 3766 } 3767 3768 public ResearchElementDefinition addEndorser(ContactDetail t) { // 3 3769 if (t == null) 3770 return this; 3771 if (this.endorser == null) 3772 this.endorser = new ArrayList<ContactDetail>(); 3773 this.endorser.add(t); 3774 return this; 3775 } 3776 3777 /** 3778 * @return The first repetition of repeating field {@link #endorser}, creating 3779 * it if it does not already exist 3780 */ 3781 public ContactDetail getEndorserFirstRep() { 3782 if (getEndorser().isEmpty()) { 3783 addEndorser(); 3784 } 3785 return getEndorser().get(0); 3786 } 3787 3788 /** 3789 * @return {@link #relatedArtifact} (Related artifacts such as additional 3790 * documentation, justification, or bibliographic references.) 3791 */ 3792 public List<RelatedArtifact> getRelatedArtifact() { 3793 if (this.relatedArtifact == null) 3794 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3795 return this.relatedArtifact; 3796 } 3797 3798 /** 3799 * @return Returns a reference to <code>this</code> for easy method chaining 3800 */ 3801 public ResearchElementDefinition setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 3802 this.relatedArtifact = theRelatedArtifact; 3803 return this; 3804 } 3805 3806 public boolean hasRelatedArtifact() { 3807 if (this.relatedArtifact == null) 3808 return false; 3809 for (RelatedArtifact item : this.relatedArtifact) 3810 if (!item.isEmpty()) 3811 return true; 3812 return false; 3813 } 3814 3815 public RelatedArtifact addRelatedArtifact() { // 3 3816 RelatedArtifact t = new RelatedArtifact(); 3817 if (this.relatedArtifact == null) 3818 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3819 this.relatedArtifact.add(t); 3820 return t; 3821 } 3822 3823 public ResearchElementDefinition addRelatedArtifact(RelatedArtifact t) { // 3 3824 if (t == null) 3825 return this; 3826 if (this.relatedArtifact == null) 3827 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3828 this.relatedArtifact.add(t); 3829 return this; 3830 } 3831 3832 /** 3833 * @return The first repetition of repeating field {@link #relatedArtifact}, 3834 * creating it if it does not already exist 3835 */ 3836 public RelatedArtifact getRelatedArtifactFirstRep() { 3837 if (getRelatedArtifact().isEmpty()) { 3838 addRelatedArtifact(); 3839 } 3840 return getRelatedArtifact().get(0); 3841 } 3842 3843 /** 3844 * @return {@link #library} (A reference to a Library resource containing the 3845 * formal logic used by the ResearchElementDefinition.) 3846 */ 3847 public List<CanonicalType> getLibrary() { 3848 if (this.library == null) 3849 this.library = new ArrayList<CanonicalType>(); 3850 return this.library; 3851 } 3852 3853 /** 3854 * @return Returns a reference to <code>this</code> for easy method chaining 3855 */ 3856 public ResearchElementDefinition setLibrary(List<CanonicalType> theLibrary) { 3857 this.library = theLibrary; 3858 return this; 3859 } 3860 3861 public boolean hasLibrary() { 3862 if (this.library == null) 3863 return false; 3864 for (CanonicalType item : this.library) 3865 if (!item.isEmpty()) 3866 return true; 3867 return false; 3868 } 3869 3870 /** 3871 * @return {@link #library} (A reference to a Library resource containing the 3872 * formal logic used by the ResearchElementDefinition.) 3873 */ 3874 public CanonicalType addLibraryElement() {// 2 3875 CanonicalType t = new CanonicalType(); 3876 if (this.library == null) 3877 this.library = new ArrayList<CanonicalType>(); 3878 this.library.add(t); 3879 return t; 3880 } 3881 3882 /** 3883 * @param value {@link #library} (A reference to a Library resource containing 3884 * the formal logic used by the ResearchElementDefinition.) 3885 */ 3886 public ResearchElementDefinition addLibrary(String value) { // 1 3887 CanonicalType t = new CanonicalType(); 3888 t.setValue(value); 3889 if (this.library == null) 3890 this.library = new ArrayList<CanonicalType>(); 3891 this.library.add(t); 3892 return this; 3893 } 3894 3895 /** 3896 * @param value {@link #library} (A reference to a Library resource containing 3897 * the formal logic used by the ResearchElementDefinition.) 3898 */ 3899 public boolean hasLibrary(String value) { 3900 if (this.library == null) 3901 return false; 3902 for (CanonicalType v : this.library) 3903 if (v.getValue().equals(value)) // canonical(Library) 3904 return true; 3905 return false; 3906 } 3907 3908 /** 3909 * @return {@link #type} (The type of research element, a population, an 3910 * exposure, or an outcome.). This is the underlying object with id, 3911 * value and extensions. The accessor "getType" gives direct access to 3912 * the value 3913 */ 3914 public Enumeration<ResearchElementType> getTypeElement() { 3915 if (this.type == null) 3916 if (Configuration.errorOnAutoCreate()) 3917 throw new Error("Attempt to auto-create ResearchElementDefinition.type"); 3918 else if (Configuration.doAutoCreate()) 3919 this.type = new Enumeration<ResearchElementType>(new ResearchElementTypeEnumFactory()); // bb 3920 return this.type; 3921 } 3922 3923 public boolean hasTypeElement() { 3924 return this.type != null && !this.type.isEmpty(); 3925 } 3926 3927 public boolean hasType() { 3928 return this.type != null && !this.type.isEmpty(); 3929 } 3930 3931 /** 3932 * @param value {@link #type} (The type of research element, a population, an 3933 * exposure, or an outcome.). This is the underlying object with 3934 * id, value and extensions. The accessor "getType" gives direct 3935 * access to the value 3936 */ 3937 public ResearchElementDefinition setTypeElement(Enumeration<ResearchElementType> value) { 3938 this.type = value; 3939 return this; 3940 } 3941 3942 /** 3943 * @return The type of research element, a population, an exposure, or an 3944 * outcome. 3945 */ 3946 public ResearchElementType getType() { 3947 return this.type == null ? null : this.type.getValue(); 3948 } 3949 3950 /** 3951 * @param value The type of research element, a population, an exposure, or an 3952 * outcome. 3953 */ 3954 public ResearchElementDefinition setType(ResearchElementType value) { 3955 if (this.type == null) 3956 this.type = new Enumeration<ResearchElementType>(new ResearchElementTypeEnumFactory()); 3957 this.type.setValue(value); 3958 return this; 3959 } 3960 3961 /** 3962 * @return {@link #variableType} (The type of the outcome (e.g. Dichotomous, 3963 * Continuous, or Descriptive).). This is the underlying object with id, 3964 * value and extensions. The accessor "getVariableType" gives direct 3965 * access to the value 3966 */ 3967 public Enumeration<VariableType> getVariableTypeElement() { 3968 if (this.variableType == null) 3969 if (Configuration.errorOnAutoCreate()) 3970 throw new Error("Attempt to auto-create ResearchElementDefinition.variableType"); 3971 else if (Configuration.doAutoCreate()) 3972 this.variableType = new Enumeration<VariableType>(new VariableTypeEnumFactory()); // bb 3973 return this.variableType; 3974 } 3975 3976 public boolean hasVariableTypeElement() { 3977 return this.variableType != null && !this.variableType.isEmpty(); 3978 } 3979 3980 public boolean hasVariableType() { 3981 return this.variableType != null && !this.variableType.isEmpty(); 3982 } 3983 3984 /** 3985 * @param value {@link #variableType} (The type of the outcome (e.g. 3986 * Dichotomous, Continuous, or Descriptive).). This is the 3987 * underlying object with id, value and extensions. The accessor 3988 * "getVariableType" gives direct access to the value 3989 */ 3990 public ResearchElementDefinition setVariableTypeElement(Enumeration<VariableType> value) { 3991 this.variableType = value; 3992 return this; 3993 } 3994 3995 /** 3996 * @return The type of the outcome (e.g. Dichotomous, Continuous, or 3997 * Descriptive). 3998 */ 3999 public VariableType getVariableType() { 4000 return this.variableType == null ? null : this.variableType.getValue(); 4001 } 4002 4003 /** 4004 * @param value The type of the outcome (e.g. Dichotomous, Continuous, or 4005 * Descriptive). 4006 */ 4007 public ResearchElementDefinition setVariableType(VariableType value) { 4008 if (value == null) 4009 this.variableType = null; 4010 else { 4011 if (this.variableType == null) 4012 this.variableType = new Enumeration<VariableType>(new VariableTypeEnumFactory()); 4013 this.variableType.setValue(value); 4014 } 4015 return this; 4016 } 4017 4018 /** 4019 * @return {@link #characteristic} (A characteristic that defines the members of 4020 * the research element. Multiple characteristics are applied with "and" 4021 * semantics.) 4022 */ 4023 public List<ResearchElementDefinitionCharacteristicComponent> getCharacteristic() { 4024 if (this.characteristic == null) 4025 this.characteristic = new ArrayList<ResearchElementDefinitionCharacteristicComponent>(); 4026 return this.characteristic; 4027 } 4028 4029 /** 4030 * @return Returns a reference to <code>this</code> for easy method chaining 4031 */ 4032 public ResearchElementDefinition setCharacteristic( 4033 List<ResearchElementDefinitionCharacteristicComponent> theCharacteristic) { 4034 this.characteristic = theCharacteristic; 4035 return this; 4036 } 4037 4038 public boolean hasCharacteristic() { 4039 if (this.characteristic == null) 4040 return false; 4041 for (ResearchElementDefinitionCharacteristicComponent item : this.characteristic) 4042 if (!item.isEmpty()) 4043 return true; 4044 return false; 4045 } 4046 4047 public ResearchElementDefinitionCharacteristicComponent addCharacteristic() { // 3 4048 ResearchElementDefinitionCharacteristicComponent t = new ResearchElementDefinitionCharacteristicComponent(); 4049 if (this.characteristic == null) 4050 this.characteristic = new ArrayList<ResearchElementDefinitionCharacteristicComponent>(); 4051 this.characteristic.add(t); 4052 return t; 4053 } 4054 4055 public ResearchElementDefinition addCharacteristic(ResearchElementDefinitionCharacteristicComponent t) { // 3 4056 if (t == null) 4057 return this; 4058 if (this.characteristic == null) 4059 this.characteristic = new ArrayList<ResearchElementDefinitionCharacteristicComponent>(); 4060 this.characteristic.add(t); 4061 return this; 4062 } 4063 4064 /** 4065 * @return The first repetition of repeating field {@link #characteristic}, 4066 * creating it if it does not already exist 4067 */ 4068 public ResearchElementDefinitionCharacteristicComponent getCharacteristicFirstRep() { 4069 if (getCharacteristic().isEmpty()) { 4070 addCharacteristic(); 4071 } 4072 return getCharacteristic().get(0); 4073 } 4074 4075 protected void listChildren(List<Property> children) { 4076 super.listChildren(children); 4077 children.add(new Property("url", "uri", 4078 "An absolute URI that is used to identify this research element definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this research element definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the research element definition is stored on different servers.", 4079 0, 1, url)); 4080 children.add(new Property("identifier", "Identifier", 4081 "A formal identifier that is used to identify this research element definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 4082 0, java.lang.Integer.MAX_VALUE, identifier)); 4083 children.add(new Property("version", "string", 4084 "The identifier that is used to identify this version of the research element definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the research element definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 4085 0, 1, version)); 4086 children.add(new Property("name", "string", 4087 "A natural language name identifying the research element definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 4088 0, 1, name)); 4089 children.add(new Property("title", "string", 4090 "A short, descriptive, user-friendly title for the research element definition.", 0, 1, title)); 4091 children.add(new Property("shortTitle", "string", 4092 "The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary.", 4093 0, 1, shortTitle)); 4094 children.add(new Property("subtitle", "string", 4095 "An explanatory or alternate title for the ResearchElementDefinition giving additional information about its content.", 4096 0, 1, subtitle)); 4097 children.add(new Property("status", "code", 4098 "The status of this research element definition. Enables tracking the life-cycle of the content.", 0, 1, 4099 status)); 4100 children.add(new Property("experimental", "boolean", 4101 "A Boolean value to indicate that this research element definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 4102 0, 1, experimental)); 4103 children.add(new Property("subject[x]", "CodeableConcept|Reference(Group)", 4104 "The intended subjects for the ResearchElementDefinition. If this element is not provided, a Patient subject is assumed, but the subject of the ResearchElementDefinition can be anything.", 4105 0, 1, subject)); 4106 children.add(new Property("date", "dateTime", 4107 "The date (and optionally time) when the research element definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the research element definition changes.", 4108 0, 1, date)); 4109 children.add(new Property("publisher", "string", 4110 "The name of the organization or individual that published the research element definition.", 0, 1, publisher)); 4111 children.add(new Property("contact", "ContactDetail", 4112 "Contact details to assist a user in finding and communicating with the publisher.", 0, 4113 java.lang.Integer.MAX_VALUE, contact)); 4114 children.add(new Property("description", "markdown", 4115 "A free text natural language description of the research element definition from a consumer's perspective.", 0, 4116 1, description)); 4117 children.add( 4118 new Property("comment", "string", "A human-readable string to clarify or explain concepts about the resource.", 4119 0, java.lang.Integer.MAX_VALUE, comment)); 4120 children.add(new Property("useContext", "UsageContext", 4121 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate research element definition instances.", 4122 0, java.lang.Integer.MAX_VALUE, useContext)); 4123 children.add(new Property("jurisdiction", "CodeableConcept", 4124 "A legal or geographic region in which the research element definition is intended to be used.", 0, 4125 java.lang.Integer.MAX_VALUE, jurisdiction)); 4126 children.add(new Property("purpose", "markdown", 4127 "Explanation of why this research element definition is needed and why it has been designed as it has.", 0, 1, 4128 purpose)); 4129 children.add(new Property("usage", "string", 4130 "A detailed description, from a clinical perspective, of how the ResearchElementDefinition is used.", 0, 1, 4131 usage)); 4132 children.add(new Property("copyright", "markdown", 4133 "A copyright statement relating to the research element definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the research element definition.", 4134 0, 1, copyright)); 4135 children.add(new Property("approvalDate", "date", 4136 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 4137 0, 1, approvalDate)); 4138 children.add(new Property("lastReviewDate", "date", 4139 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 4140 0, 1, lastReviewDate)); 4141 children.add(new Property("effectivePeriod", "Period", 4142 "The period during which the research element definition content was or is planned to be in active use.", 0, 1, 4143 effectivePeriod)); 4144 children.add(new Property("topic", "CodeableConcept", 4145 "Descriptive topics related to the content of the ResearchElementDefinition. Topics provide a high-level categorization grouping types of ResearchElementDefinitions that can be useful for filtering and searching.", 4146 0, java.lang.Integer.MAX_VALUE, topic)); 4147 children.add(new Property("author", "ContactDetail", 4148 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 4149 java.lang.Integer.MAX_VALUE, author)); 4150 children.add(new Property("editor", "ContactDetail", 4151 "An individual or organization primarily responsible for internal coherence of the content.", 0, 4152 java.lang.Integer.MAX_VALUE, editor)); 4153 children.add(new Property("reviewer", "ContactDetail", 4154 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 4155 java.lang.Integer.MAX_VALUE, reviewer)); 4156 children.add(new Property("endorser", "ContactDetail", 4157 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 4158 java.lang.Integer.MAX_VALUE, endorser)); 4159 children.add(new Property("relatedArtifact", "RelatedArtifact", 4160 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 4161 java.lang.Integer.MAX_VALUE, relatedArtifact)); 4162 children.add(new Property("library", "canonical(Library)", 4163 "A reference to a Library resource containing the formal logic used by the ResearchElementDefinition.", 0, 4164 java.lang.Integer.MAX_VALUE, library)); 4165 children.add(new Property("type", "code", "The type of research element, a population, an exposure, or an outcome.", 4166 0, 1, type)); 4167 children.add(new Property("variableType", "code", 4168 "The type of the outcome (e.g. Dichotomous, Continuous, or Descriptive).", 0, 1, variableType)); 4169 children.add(new Property("characteristic", "", 4170 "A characteristic that defines the members of the research element. Multiple characteristics are applied with \"and\" semantics.", 4171 0, java.lang.Integer.MAX_VALUE, characteristic)); 4172 } 4173 4174 @Override 4175 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4176 switch (_hash) { 4177 case 116079: 4178 /* url */ return new Property("url", "uri", 4179 "An absolute URI that is used to identify this research element definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this research element definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the research element definition is stored on different servers.", 4180 0, 1, url); 4181 case -1618432855: 4182 /* identifier */ return new Property("identifier", "Identifier", 4183 "A formal identifier that is used to identify this research element definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 4184 0, java.lang.Integer.MAX_VALUE, identifier); 4185 case 351608024: 4186 /* version */ return new Property("version", "string", 4187 "The identifier that is used to identify this version of the research element definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the research element definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 4188 0, 1, version); 4189 case 3373707: 4190 /* name */ return new Property("name", "string", 4191 "A natural language name identifying the research element definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 4192 0, 1, name); 4193 case 110371416: 4194 /* title */ return new Property("title", "string", 4195 "A short, descriptive, user-friendly title for the research element definition.", 0, 1, title); 4196 case 1555503932: 4197 /* shortTitle */ return new Property("shortTitle", "string", 4198 "The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary.", 4199 0, 1, shortTitle); 4200 case -2060497896: 4201 /* subtitle */ return new Property("subtitle", "string", 4202 "An explanatory or alternate title for the ResearchElementDefinition giving additional information about its content.", 4203 0, 1, subtitle); 4204 case -892481550: 4205 /* status */ return new Property("status", "code", 4206 "The status of this research element definition. Enables tracking the life-cycle of the content.", 0, 1, 4207 status); 4208 case -404562712: 4209 /* experimental */ return new Property("experimental", "boolean", 4210 "A Boolean value to indicate that this research element definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 4211 0, 1, experimental); 4212 case -573640748: 4213 /* subject[x] */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 4214 "The intended subjects for the ResearchElementDefinition. If this element is not provided, a Patient subject is assumed, but the subject of the ResearchElementDefinition can be anything.", 4215 0, 1, subject); 4216 case -1867885268: 4217 /* subject */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 4218 "The intended subjects for the ResearchElementDefinition. If this element is not provided, a Patient subject is assumed, but the subject of the ResearchElementDefinition can be anything.", 4219 0, 1, subject); 4220 case -1257122603: 4221 /* subjectCodeableConcept */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 4222 "The intended subjects for the ResearchElementDefinition. If this element is not provided, a Patient subject is assumed, but the subject of the ResearchElementDefinition can be anything.", 4223 0, 1, subject); 4224 case 772938623: 4225 /* subjectReference */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 4226 "The intended subjects for the ResearchElementDefinition. If this element is not provided, a Patient subject is assumed, but the subject of the ResearchElementDefinition can be anything.", 4227 0, 1, subject); 4228 case 3076014: 4229 /* date */ return new Property("date", "dateTime", 4230 "The date (and optionally time) when the research element definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the research element definition changes.", 4231 0, 1, date); 4232 case 1447404028: 4233 /* publisher */ return new Property("publisher", "string", 4234 "The name of the organization or individual that published the research element definition.", 0, 1, 4235 publisher); 4236 case 951526432: 4237 /* contact */ return new Property("contact", "ContactDetail", 4238 "Contact details to assist a user in finding and communicating with the publisher.", 0, 4239 java.lang.Integer.MAX_VALUE, contact); 4240 case -1724546052: 4241 /* description */ return new Property("description", "markdown", 4242 "A free text natural language description of the research element definition from a consumer's perspective.", 4243 0, 1, description); 4244 case 950398559: 4245 /* comment */ return new Property("comment", "string", 4246 "A human-readable string to clarify or explain concepts about the resource.", 0, java.lang.Integer.MAX_VALUE, 4247 comment); 4248 case -669707736: 4249 /* useContext */ return new Property("useContext", "UsageContext", 4250 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate research element definition instances.", 4251 0, java.lang.Integer.MAX_VALUE, useContext); 4252 case -507075711: 4253 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 4254 "A legal or geographic region in which the research element definition is intended to be used.", 0, 4255 java.lang.Integer.MAX_VALUE, jurisdiction); 4256 case -220463842: 4257 /* purpose */ return new Property("purpose", "markdown", 4258 "Explanation of why this research element definition is needed and why it has been designed as it has.", 0, 1, 4259 purpose); 4260 case 111574433: 4261 /* usage */ return new Property("usage", "string", 4262 "A detailed description, from a clinical perspective, of how the ResearchElementDefinition is used.", 0, 1, 4263 usage); 4264 case 1522889671: 4265 /* copyright */ return new Property("copyright", "markdown", 4266 "A copyright statement relating to the research element definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the research element definition.", 4267 0, 1, copyright); 4268 case 223539345: 4269 /* approvalDate */ return new Property("approvalDate", "date", 4270 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 4271 0, 1, approvalDate); 4272 case -1687512484: 4273 /* lastReviewDate */ return new Property("lastReviewDate", "date", 4274 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 4275 0, 1, lastReviewDate); 4276 case -403934648: 4277 /* effectivePeriod */ return new Property("effectivePeriod", "Period", 4278 "The period during which the research element definition content was or is planned to be in active use.", 0, 4279 1, effectivePeriod); 4280 case 110546223: 4281 /* topic */ return new Property("topic", "CodeableConcept", 4282 "Descriptive topics related to the content of the ResearchElementDefinition. Topics provide a high-level categorization grouping types of ResearchElementDefinitions that can be useful for filtering and searching.", 4283 0, java.lang.Integer.MAX_VALUE, topic); 4284 case -1406328437: 4285 /* author */ return new Property("author", "ContactDetail", 4286 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 4287 java.lang.Integer.MAX_VALUE, author); 4288 case -1307827859: 4289 /* editor */ return new Property("editor", "ContactDetail", 4290 "An individual or organization primarily responsible for internal coherence of the content.", 0, 4291 java.lang.Integer.MAX_VALUE, editor); 4292 case -261190139: 4293 /* reviewer */ return new Property("reviewer", "ContactDetail", 4294 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 4295 java.lang.Integer.MAX_VALUE, reviewer); 4296 case 1740277666: 4297 /* endorser */ return new Property("endorser", "ContactDetail", 4298 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 4299 java.lang.Integer.MAX_VALUE, endorser); 4300 case 666807069: 4301 /* relatedArtifact */ return new Property("relatedArtifact", "RelatedArtifact", 4302 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 4303 java.lang.Integer.MAX_VALUE, relatedArtifact); 4304 case 166208699: 4305 /* library */ return new Property("library", "canonical(Library)", 4306 "A reference to a Library resource containing the formal logic used by the ResearchElementDefinition.", 0, 4307 java.lang.Integer.MAX_VALUE, library); 4308 case 3575610: 4309 /* type */ return new Property("type", "code", 4310 "The type of research element, a population, an exposure, or an outcome.", 0, 1, type); 4311 case -372820010: 4312 /* variableType */ return new Property("variableType", "code", 4313 "The type of the outcome (e.g. Dichotomous, Continuous, or Descriptive).", 0, 1, variableType); 4314 case 366313883: 4315 /* characteristic */ return new Property("characteristic", "", 4316 "A characteristic that defines the members of the research element. Multiple characteristics are applied with \"and\" semantics.", 4317 0, java.lang.Integer.MAX_VALUE, characteristic); 4318 default: 4319 return super.getNamedProperty(_hash, _name, _checkValid); 4320 } 4321 4322 } 4323 4324 @Override 4325 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4326 switch (hash) { 4327 case 116079: 4328 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 4329 case -1618432855: 4330 /* identifier */ return this.identifier == null ? new Base[0] 4331 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4332 case 351608024: 4333 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 4334 case 3373707: 4335 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 4336 case 110371416: 4337 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 4338 case 1555503932: 4339 /* shortTitle */ return this.shortTitle == null ? new Base[0] : new Base[] { this.shortTitle }; // StringType 4340 case -2060497896: 4341 /* subtitle */ return this.subtitle == null ? new Base[0] : new Base[] { this.subtitle }; // StringType 4342 case -892481550: 4343 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 4344 case -404562712: 4345 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 4346 case -1867885268: 4347 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Type 4348 case 3076014: 4349 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 4350 case 1447404028: 4351 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 4352 case 951526432: 4353 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 4354 case -1724546052: 4355 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 4356 case 950398559: 4357 /* comment */ return this.comment == null ? new Base[0] : this.comment.toArray(new Base[this.comment.size()]); // StringType 4358 case -669707736: 4359 /* useContext */ return this.useContext == null ? new Base[0] 4360 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 4361 case -507075711: 4362 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 4363 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 4364 case -220463842: 4365 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 4366 case 111574433: 4367 /* usage */ return this.usage == null ? new Base[0] : new Base[] { this.usage }; // StringType 4368 case 1522889671: 4369 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 4370 case 223539345: 4371 /* approvalDate */ return this.approvalDate == null ? new Base[0] : new Base[] { this.approvalDate }; // DateType 4372 case -1687512484: 4373 /* lastReviewDate */ return this.lastReviewDate == null ? new Base[0] : new Base[] { this.lastReviewDate }; // DateType 4374 case -403934648: 4375 /* effectivePeriod */ return this.effectivePeriod == null ? new Base[0] : new Base[] { this.effectivePeriod }; // Period 4376 case 110546223: 4377 /* topic */ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 4378 case -1406328437: 4379 /* author */ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 4380 case -1307827859: 4381 /* editor */ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 4382 case -261190139: 4383 /* reviewer */ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 4384 case 1740277666: 4385 /* endorser */ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 4386 case 666807069: 4387 /* relatedArtifact */ return this.relatedArtifact == null ? new Base[0] 4388 : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 4389 case 166208699: 4390 /* library */ return this.library == null ? new Base[0] : this.library.toArray(new Base[this.library.size()]); // CanonicalType 4391 case 3575610: 4392 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<ResearchElementType> 4393 case -372820010: 4394 /* variableType */ return this.variableType == null ? new Base[0] : new Base[] { this.variableType }; // Enumeration<VariableType> 4395 case 366313883: 4396 /* characteristic */ return this.characteristic == null ? new Base[0] 4397 : this.characteristic.toArray(new Base[this.characteristic.size()]); // ResearchElementDefinitionCharacteristicComponent 4398 default: 4399 return super.getProperty(hash, name, checkValid); 4400 } 4401 4402 } 4403 4404 @Override 4405 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4406 switch (hash) { 4407 case 116079: // url 4408 this.url = castToUri(value); // UriType 4409 return value; 4410 case -1618432855: // identifier 4411 this.getIdentifier().add(castToIdentifier(value)); // Identifier 4412 return value; 4413 case 351608024: // version 4414 this.version = castToString(value); // StringType 4415 return value; 4416 case 3373707: // name 4417 this.name = castToString(value); // StringType 4418 return value; 4419 case 110371416: // title 4420 this.title = castToString(value); // StringType 4421 return value; 4422 case 1555503932: // shortTitle 4423 this.shortTitle = castToString(value); // StringType 4424 return value; 4425 case -2060497896: // subtitle 4426 this.subtitle = castToString(value); // StringType 4427 return value; 4428 case -892481550: // status 4429 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 4430 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4431 return value; 4432 case -404562712: // experimental 4433 this.experimental = castToBoolean(value); // BooleanType 4434 return value; 4435 case -1867885268: // subject 4436 this.subject = castToType(value); // Type 4437 return value; 4438 case 3076014: // date 4439 this.date = castToDateTime(value); // DateTimeType 4440 return value; 4441 case 1447404028: // publisher 4442 this.publisher = castToString(value); // StringType 4443 return value; 4444 case 951526432: // contact 4445 this.getContact().add(castToContactDetail(value)); // ContactDetail 4446 return value; 4447 case -1724546052: // description 4448 this.description = castToMarkdown(value); // MarkdownType 4449 return value; 4450 case 950398559: // comment 4451 this.getComment().add(castToString(value)); // StringType 4452 return value; 4453 case -669707736: // useContext 4454 this.getUseContext().add(castToUsageContext(value)); // UsageContext 4455 return value; 4456 case -507075711: // jurisdiction 4457 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 4458 return value; 4459 case -220463842: // purpose 4460 this.purpose = castToMarkdown(value); // MarkdownType 4461 return value; 4462 case 111574433: // usage 4463 this.usage = castToString(value); // StringType 4464 return value; 4465 case 1522889671: // copyright 4466 this.copyright = castToMarkdown(value); // MarkdownType 4467 return value; 4468 case 223539345: // approvalDate 4469 this.approvalDate = castToDate(value); // DateType 4470 return value; 4471 case -1687512484: // lastReviewDate 4472 this.lastReviewDate = castToDate(value); // DateType 4473 return value; 4474 case -403934648: // effectivePeriod 4475 this.effectivePeriod = castToPeriod(value); // Period 4476 return value; 4477 case 110546223: // topic 4478 this.getTopic().add(castToCodeableConcept(value)); // CodeableConcept 4479 return value; 4480 case -1406328437: // author 4481 this.getAuthor().add(castToContactDetail(value)); // ContactDetail 4482 return value; 4483 case -1307827859: // editor 4484 this.getEditor().add(castToContactDetail(value)); // ContactDetail 4485 return value; 4486 case -261190139: // reviewer 4487 this.getReviewer().add(castToContactDetail(value)); // ContactDetail 4488 return value; 4489 case 1740277666: // endorser 4490 this.getEndorser().add(castToContactDetail(value)); // ContactDetail 4491 return value; 4492 case 666807069: // relatedArtifact 4493 this.getRelatedArtifact().add(castToRelatedArtifact(value)); // RelatedArtifact 4494 return value; 4495 case 166208699: // library 4496 this.getLibrary().add(castToCanonical(value)); // CanonicalType 4497 return value; 4498 case 3575610: // type 4499 value = new ResearchElementTypeEnumFactory().fromType(castToCode(value)); 4500 this.type = (Enumeration) value; // Enumeration<ResearchElementType> 4501 return value; 4502 case -372820010: // variableType 4503 value = new VariableTypeEnumFactory().fromType(castToCode(value)); 4504 this.variableType = (Enumeration) value; // Enumeration<VariableType> 4505 return value; 4506 case 366313883: // characteristic 4507 this.getCharacteristic().add((ResearchElementDefinitionCharacteristicComponent) value); // ResearchElementDefinitionCharacteristicComponent 4508 return value; 4509 default: 4510 return super.setProperty(hash, name, value); 4511 } 4512 4513 } 4514 4515 @Override 4516 public Base setProperty(String name, Base value) throws FHIRException { 4517 if (name.equals("url")) { 4518 this.url = castToUri(value); // UriType 4519 } else if (name.equals("identifier")) { 4520 this.getIdentifier().add(castToIdentifier(value)); 4521 } else if (name.equals("version")) { 4522 this.version = castToString(value); // StringType 4523 } else if (name.equals("name")) { 4524 this.name = castToString(value); // StringType 4525 } else if (name.equals("title")) { 4526 this.title = castToString(value); // StringType 4527 } else if (name.equals("shortTitle")) { 4528 this.shortTitle = castToString(value); // StringType 4529 } else if (name.equals("subtitle")) { 4530 this.subtitle = castToString(value); // StringType 4531 } else if (name.equals("status")) { 4532 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 4533 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4534 } else if (name.equals("experimental")) { 4535 this.experimental = castToBoolean(value); // BooleanType 4536 } else if (name.equals("subject[x]")) { 4537 this.subject = castToType(value); // Type 4538 } else if (name.equals("date")) { 4539 this.date = castToDateTime(value); // DateTimeType 4540 } else if (name.equals("publisher")) { 4541 this.publisher = castToString(value); // StringType 4542 } else if (name.equals("contact")) { 4543 this.getContact().add(castToContactDetail(value)); 4544 } else if (name.equals("description")) { 4545 this.description = castToMarkdown(value); // MarkdownType 4546 } else if (name.equals("comment")) { 4547 this.getComment().add(castToString(value)); 4548 } else if (name.equals("useContext")) { 4549 this.getUseContext().add(castToUsageContext(value)); 4550 } else if (name.equals("jurisdiction")) { 4551 this.getJurisdiction().add(castToCodeableConcept(value)); 4552 } else if (name.equals("purpose")) { 4553 this.purpose = castToMarkdown(value); // MarkdownType 4554 } else if (name.equals("usage")) { 4555 this.usage = castToString(value); // StringType 4556 } else if (name.equals("copyright")) { 4557 this.copyright = castToMarkdown(value); // MarkdownType 4558 } else if (name.equals("approvalDate")) { 4559 this.approvalDate = castToDate(value); // DateType 4560 } else if (name.equals("lastReviewDate")) { 4561 this.lastReviewDate = castToDate(value); // DateType 4562 } else if (name.equals("effectivePeriod")) { 4563 this.effectivePeriod = castToPeriod(value); // Period 4564 } else if (name.equals("topic")) { 4565 this.getTopic().add(castToCodeableConcept(value)); 4566 } else if (name.equals("author")) { 4567 this.getAuthor().add(castToContactDetail(value)); 4568 } else if (name.equals("editor")) { 4569 this.getEditor().add(castToContactDetail(value)); 4570 } else if (name.equals("reviewer")) { 4571 this.getReviewer().add(castToContactDetail(value)); 4572 } else if (name.equals("endorser")) { 4573 this.getEndorser().add(castToContactDetail(value)); 4574 } else if (name.equals("relatedArtifact")) { 4575 this.getRelatedArtifact().add(castToRelatedArtifact(value)); 4576 } else if (name.equals("library")) { 4577 this.getLibrary().add(castToCanonical(value)); 4578 } else if (name.equals("type")) { 4579 value = new ResearchElementTypeEnumFactory().fromType(castToCode(value)); 4580 this.type = (Enumeration) value; // Enumeration<ResearchElementType> 4581 } else if (name.equals("variableType")) { 4582 value = new VariableTypeEnumFactory().fromType(castToCode(value)); 4583 this.variableType = (Enumeration) value; // Enumeration<VariableType> 4584 } else if (name.equals("characteristic")) { 4585 this.getCharacteristic().add((ResearchElementDefinitionCharacteristicComponent) value); 4586 } else 4587 return super.setProperty(name, value); 4588 return value; 4589 } 4590 4591 @Override 4592 public void removeChild(String name, Base value) throws FHIRException { 4593 if (name.equals("url")) { 4594 this.url = null; 4595 } else if (name.equals("identifier")) { 4596 this.getIdentifier().remove(castToIdentifier(value)); 4597 } else if (name.equals("version")) { 4598 this.version = null; 4599 } else if (name.equals("name")) { 4600 this.name = null; 4601 } else if (name.equals("title")) { 4602 this.title = null; 4603 } else if (name.equals("shortTitle")) { 4604 this.shortTitle = null; 4605 } else if (name.equals("subtitle")) { 4606 this.subtitle = null; 4607 } else if (name.equals("status")) { 4608 this.status = null; 4609 } else if (name.equals("experimental")) { 4610 this.experimental = null; 4611 } else if (name.equals("subject[x]")) { 4612 this.subject = null; 4613 } else if (name.equals("date")) { 4614 this.date = null; 4615 } else if (name.equals("publisher")) { 4616 this.publisher = null; 4617 } else if (name.equals("contact")) { 4618 this.getContact().remove(castToContactDetail(value)); 4619 } else if (name.equals("description")) { 4620 this.description = null; 4621 } else if (name.equals("comment")) { 4622 this.getComment().remove(castToString(value)); 4623 } else if (name.equals("useContext")) { 4624 this.getUseContext().remove(castToUsageContext(value)); 4625 } else if (name.equals("jurisdiction")) { 4626 this.getJurisdiction().remove(castToCodeableConcept(value)); 4627 } else if (name.equals("purpose")) { 4628 this.purpose = null; 4629 } else if (name.equals("usage")) { 4630 this.usage = null; 4631 } else if (name.equals("copyright")) { 4632 this.copyright = null; 4633 } else if (name.equals("approvalDate")) { 4634 this.approvalDate = null; 4635 } else if (name.equals("lastReviewDate")) { 4636 this.lastReviewDate = null; 4637 } else if (name.equals("effectivePeriod")) { 4638 this.effectivePeriod = null; 4639 } else if (name.equals("topic")) { 4640 this.getTopic().remove(castToCodeableConcept(value)); 4641 } else if (name.equals("author")) { 4642 this.getAuthor().remove(castToContactDetail(value)); 4643 } else if (name.equals("editor")) { 4644 this.getEditor().remove(castToContactDetail(value)); 4645 } else if (name.equals("reviewer")) { 4646 this.getReviewer().remove(castToContactDetail(value)); 4647 } else if (name.equals("endorser")) { 4648 this.getEndorser().remove(castToContactDetail(value)); 4649 } else if (name.equals("relatedArtifact")) { 4650 this.getRelatedArtifact().remove(castToRelatedArtifact(value)); 4651 } else if (name.equals("library")) { 4652 this.getLibrary().remove(castToCanonical(value)); 4653 } else if (name.equals("type")) { 4654 this.type = null; 4655 } else if (name.equals("variableType")) { 4656 this.variableType = null; 4657 } else if (name.equals("characteristic")) { 4658 this.getCharacteristic().remove((ResearchElementDefinitionCharacteristicComponent) value); 4659 } else 4660 super.removeChild(name, value); 4661 4662 } 4663 4664 @Override 4665 public Base makeProperty(int hash, String name) throws FHIRException { 4666 switch (hash) { 4667 case 116079: 4668 return getUrlElement(); 4669 case -1618432855: 4670 return addIdentifier(); 4671 case 351608024: 4672 return getVersionElement(); 4673 case 3373707: 4674 return getNameElement(); 4675 case 110371416: 4676 return getTitleElement(); 4677 case 1555503932: 4678 return getShortTitleElement(); 4679 case -2060497896: 4680 return getSubtitleElement(); 4681 case -892481550: 4682 return getStatusElement(); 4683 case -404562712: 4684 return getExperimentalElement(); 4685 case -573640748: 4686 return getSubject(); 4687 case -1867885268: 4688 return getSubject(); 4689 case 3076014: 4690 return getDateElement(); 4691 case 1447404028: 4692 return getPublisherElement(); 4693 case 951526432: 4694 return addContact(); 4695 case -1724546052: 4696 return getDescriptionElement(); 4697 case 950398559: 4698 return addCommentElement(); 4699 case -669707736: 4700 return addUseContext(); 4701 case -507075711: 4702 return addJurisdiction(); 4703 case -220463842: 4704 return getPurposeElement(); 4705 case 111574433: 4706 return getUsageElement(); 4707 case 1522889671: 4708 return getCopyrightElement(); 4709 case 223539345: 4710 return getApprovalDateElement(); 4711 case -1687512484: 4712 return getLastReviewDateElement(); 4713 case -403934648: 4714 return getEffectivePeriod(); 4715 case 110546223: 4716 return addTopic(); 4717 case -1406328437: 4718 return addAuthor(); 4719 case -1307827859: 4720 return addEditor(); 4721 case -261190139: 4722 return addReviewer(); 4723 case 1740277666: 4724 return addEndorser(); 4725 case 666807069: 4726 return addRelatedArtifact(); 4727 case 166208699: 4728 return addLibraryElement(); 4729 case 3575610: 4730 return getTypeElement(); 4731 case -372820010: 4732 return getVariableTypeElement(); 4733 case 366313883: 4734 return addCharacteristic(); 4735 default: 4736 return super.makeProperty(hash, name); 4737 } 4738 4739 } 4740 4741 @Override 4742 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4743 switch (hash) { 4744 case 116079: 4745 /* url */ return new String[] { "uri" }; 4746 case -1618432855: 4747 /* identifier */ return new String[] { "Identifier" }; 4748 case 351608024: 4749 /* version */ return new String[] { "string" }; 4750 case 3373707: 4751 /* name */ return new String[] { "string" }; 4752 case 110371416: 4753 /* title */ return new String[] { "string" }; 4754 case 1555503932: 4755 /* shortTitle */ return new String[] { "string" }; 4756 case -2060497896: 4757 /* subtitle */ return new String[] { "string" }; 4758 case -892481550: 4759 /* status */ return new String[] { "code" }; 4760 case -404562712: 4761 /* experimental */ return new String[] { "boolean" }; 4762 case -1867885268: 4763 /* subject */ return new String[] { "CodeableConcept", "Reference" }; 4764 case 3076014: 4765 /* date */ return new String[] { "dateTime" }; 4766 case 1447404028: 4767 /* publisher */ return new String[] { "string" }; 4768 case 951526432: 4769 /* contact */ return new String[] { "ContactDetail" }; 4770 case -1724546052: 4771 /* description */ return new String[] { "markdown" }; 4772 case 950398559: 4773 /* comment */ return new String[] { "string" }; 4774 case -669707736: 4775 /* useContext */ return new String[] { "UsageContext" }; 4776 case -507075711: 4777 /* jurisdiction */ return new String[] { "CodeableConcept" }; 4778 case -220463842: 4779 /* purpose */ return new String[] { "markdown" }; 4780 case 111574433: 4781 /* usage */ return new String[] { "string" }; 4782 case 1522889671: 4783 /* copyright */ return new String[] { "markdown" }; 4784 case 223539345: 4785 /* approvalDate */ return new String[] { "date" }; 4786 case -1687512484: 4787 /* lastReviewDate */ return new String[] { "date" }; 4788 case -403934648: 4789 /* effectivePeriod */ return new String[] { "Period" }; 4790 case 110546223: 4791 /* topic */ return new String[] { "CodeableConcept" }; 4792 case -1406328437: 4793 /* author */ return new String[] { "ContactDetail" }; 4794 case -1307827859: 4795 /* editor */ return new String[] { "ContactDetail" }; 4796 case -261190139: 4797 /* reviewer */ return new String[] { "ContactDetail" }; 4798 case 1740277666: 4799 /* endorser */ return new String[] { "ContactDetail" }; 4800 case 666807069: 4801 /* relatedArtifact */ return new String[] { "RelatedArtifact" }; 4802 case 166208699: 4803 /* library */ return new String[] { "canonical" }; 4804 case 3575610: 4805 /* type */ return new String[] { "code" }; 4806 case -372820010: 4807 /* variableType */ return new String[] { "code" }; 4808 case 366313883: 4809 /* characteristic */ return new String[] {}; 4810 default: 4811 return super.getTypesForProperty(hash, name); 4812 } 4813 4814 } 4815 4816 @Override 4817 public Base addChild(String name) throws FHIRException { 4818 if (name.equals("url")) { 4819 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.url"); 4820 } else if (name.equals("identifier")) { 4821 return addIdentifier(); 4822 } else if (name.equals("version")) { 4823 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.version"); 4824 } else if (name.equals("name")) { 4825 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.name"); 4826 } else if (name.equals("title")) { 4827 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.title"); 4828 } else if (name.equals("shortTitle")) { 4829 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.shortTitle"); 4830 } else if (name.equals("subtitle")) { 4831 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.subtitle"); 4832 } else if (name.equals("status")) { 4833 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.status"); 4834 } else if (name.equals("experimental")) { 4835 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.experimental"); 4836 } else if (name.equals("subjectCodeableConcept")) { 4837 this.subject = new CodeableConcept(); 4838 return this.subject; 4839 } else if (name.equals("subjectReference")) { 4840 this.subject = new Reference(); 4841 return this.subject; 4842 } else if (name.equals("date")) { 4843 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.date"); 4844 } else if (name.equals("publisher")) { 4845 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.publisher"); 4846 } else if (name.equals("contact")) { 4847 return addContact(); 4848 } else if (name.equals("description")) { 4849 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.description"); 4850 } else if (name.equals("comment")) { 4851 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.comment"); 4852 } else if (name.equals("useContext")) { 4853 return addUseContext(); 4854 } else if (name.equals("jurisdiction")) { 4855 return addJurisdiction(); 4856 } else if (name.equals("purpose")) { 4857 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.purpose"); 4858 } else if (name.equals("usage")) { 4859 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.usage"); 4860 } else if (name.equals("copyright")) { 4861 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.copyright"); 4862 } else if (name.equals("approvalDate")) { 4863 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.approvalDate"); 4864 } else if (name.equals("lastReviewDate")) { 4865 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.lastReviewDate"); 4866 } else if (name.equals("effectivePeriod")) { 4867 this.effectivePeriod = new Period(); 4868 return this.effectivePeriod; 4869 } else if (name.equals("topic")) { 4870 return addTopic(); 4871 } else if (name.equals("author")) { 4872 return addAuthor(); 4873 } else if (name.equals("editor")) { 4874 return addEditor(); 4875 } else if (name.equals("reviewer")) { 4876 return addReviewer(); 4877 } else if (name.equals("endorser")) { 4878 return addEndorser(); 4879 } else if (name.equals("relatedArtifact")) { 4880 return addRelatedArtifact(); 4881 } else if (name.equals("library")) { 4882 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.library"); 4883 } else if (name.equals("type")) { 4884 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.type"); 4885 } else if (name.equals("variableType")) { 4886 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.variableType"); 4887 } else if (name.equals("characteristic")) { 4888 return addCharacteristic(); 4889 } else 4890 return super.addChild(name); 4891 } 4892 4893 public String fhirType() { 4894 return "ResearchElementDefinition"; 4895 4896 } 4897 4898 public ResearchElementDefinition copy() { 4899 ResearchElementDefinition dst = new ResearchElementDefinition(); 4900 copyValues(dst); 4901 return dst; 4902 } 4903 4904 public void copyValues(ResearchElementDefinition dst) { 4905 super.copyValues(dst); 4906 dst.url = url == null ? null : url.copy(); 4907 if (identifier != null) { 4908 dst.identifier = new ArrayList<Identifier>(); 4909 for (Identifier i : identifier) 4910 dst.identifier.add(i.copy()); 4911 } 4912 ; 4913 dst.version = version == null ? null : version.copy(); 4914 dst.name = name == null ? null : name.copy(); 4915 dst.title = title == null ? null : title.copy(); 4916 dst.shortTitle = shortTitle == null ? null : shortTitle.copy(); 4917 dst.subtitle = subtitle == null ? null : subtitle.copy(); 4918 dst.status = status == null ? null : status.copy(); 4919 dst.experimental = experimental == null ? null : experimental.copy(); 4920 dst.subject = subject == null ? null : subject.copy(); 4921 dst.date = date == null ? null : date.copy(); 4922 dst.publisher = publisher == null ? null : publisher.copy(); 4923 if (contact != null) { 4924 dst.contact = new ArrayList<ContactDetail>(); 4925 for (ContactDetail i : contact) 4926 dst.contact.add(i.copy()); 4927 } 4928 ; 4929 dst.description = description == null ? null : description.copy(); 4930 if (comment != null) { 4931 dst.comment = new ArrayList<StringType>(); 4932 for (StringType i : comment) 4933 dst.comment.add(i.copy()); 4934 } 4935 ; 4936 if (useContext != null) { 4937 dst.useContext = new ArrayList<UsageContext>(); 4938 for (UsageContext i : useContext) 4939 dst.useContext.add(i.copy()); 4940 } 4941 ; 4942 if (jurisdiction != null) { 4943 dst.jurisdiction = new ArrayList<CodeableConcept>(); 4944 for (CodeableConcept i : jurisdiction) 4945 dst.jurisdiction.add(i.copy()); 4946 } 4947 ; 4948 dst.purpose = purpose == null ? null : purpose.copy(); 4949 dst.usage = usage == null ? null : usage.copy(); 4950 dst.copyright = copyright == null ? null : copyright.copy(); 4951 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 4952 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 4953 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 4954 if (topic != null) { 4955 dst.topic = new ArrayList<CodeableConcept>(); 4956 for (CodeableConcept i : topic) 4957 dst.topic.add(i.copy()); 4958 } 4959 ; 4960 if (author != null) { 4961 dst.author = new ArrayList<ContactDetail>(); 4962 for (ContactDetail i : author) 4963 dst.author.add(i.copy()); 4964 } 4965 ; 4966 if (editor != null) { 4967 dst.editor = new ArrayList<ContactDetail>(); 4968 for (ContactDetail i : editor) 4969 dst.editor.add(i.copy()); 4970 } 4971 ; 4972 if (reviewer != null) { 4973 dst.reviewer = new ArrayList<ContactDetail>(); 4974 for (ContactDetail i : reviewer) 4975 dst.reviewer.add(i.copy()); 4976 } 4977 ; 4978 if (endorser != null) { 4979 dst.endorser = new ArrayList<ContactDetail>(); 4980 for (ContactDetail i : endorser) 4981 dst.endorser.add(i.copy()); 4982 } 4983 ; 4984 if (relatedArtifact != null) { 4985 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 4986 for (RelatedArtifact i : relatedArtifact) 4987 dst.relatedArtifact.add(i.copy()); 4988 } 4989 ; 4990 if (library != null) { 4991 dst.library = new ArrayList<CanonicalType>(); 4992 for (CanonicalType i : library) 4993 dst.library.add(i.copy()); 4994 } 4995 ; 4996 dst.type = type == null ? null : type.copy(); 4997 dst.variableType = variableType == null ? null : variableType.copy(); 4998 if (characteristic != null) { 4999 dst.characteristic = new ArrayList<ResearchElementDefinitionCharacteristicComponent>(); 5000 for (ResearchElementDefinitionCharacteristicComponent i : characteristic) 5001 dst.characteristic.add(i.copy()); 5002 } 5003 ; 5004 } 5005 5006 protected ResearchElementDefinition typedCopy() { 5007 return copy(); 5008 } 5009 5010 @Override 5011 public boolean equalsDeep(Base other_) { 5012 if (!super.equalsDeep(other_)) 5013 return false; 5014 if (!(other_ instanceof ResearchElementDefinition)) 5015 return false; 5016 ResearchElementDefinition o = (ResearchElementDefinition) other_; 5017 return compareDeep(identifier, o.identifier, true) && compareDeep(shortTitle, o.shortTitle, true) 5018 && compareDeep(subtitle, o.subtitle, true) && compareDeep(subject, o.subject, true) 5019 && compareDeep(comment, o.comment, true) && compareDeep(purpose, o.purpose, true) 5020 && compareDeep(usage, o.usage, true) && compareDeep(copyright, o.copyright, true) 5021 && compareDeep(approvalDate, o.approvalDate, true) && compareDeep(lastReviewDate, o.lastReviewDate, true) 5022 && compareDeep(effectivePeriod, o.effectivePeriod, true) && compareDeep(topic, o.topic, true) 5023 && compareDeep(author, o.author, true) && compareDeep(editor, o.editor, true) 5024 && compareDeep(reviewer, o.reviewer, true) && compareDeep(endorser, o.endorser, true) 5025 && compareDeep(relatedArtifact, o.relatedArtifact, true) && compareDeep(library, o.library, true) 5026 && compareDeep(type, o.type, true) && compareDeep(variableType, o.variableType, true) 5027 && compareDeep(characteristic, o.characteristic, true); 5028 } 5029 5030 @Override 5031 public boolean equalsShallow(Base other_) { 5032 if (!super.equalsShallow(other_)) 5033 return false; 5034 if (!(other_ instanceof ResearchElementDefinition)) 5035 return false; 5036 ResearchElementDefinition o = (ResearchElementDefinition) other_; 5037 return compareValues(shortTitle, o.shortTitle, true) && compareValues(subtitle, o.subtitle, true) 5038 && compareValues(comment, o.comment, true) && compareValues(purpose, o.purpose, true) 5039 && compareValues(usage, o.usage, true) && compareValues(copyright, o.copyright, true) 5040 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true) 5041 && compareValues(type, o.type, true) && compareValues(variableType, o.variableType, true); 5042 } 5043 5044 public boolean isEmpty() { 5045 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, shortTitle, subtitle, subject, comment, 5046 purpose, usage, copyright, approvalDate, lastReviewDate, effectivePeriod, topic, author, editor, reviewer, 5047 endorser, relatedArtifact, library, type, variableType, characteristic); 5048 } 5049 5050 @Override 5051 public ResourceType getResourceType() { 5052 return ResourceType.ResearchElementDefinition; 5053 } 5054 5055 /** 5056 * Search parameter: <b>date</b> 5057 * <p> 5058 * Description: <b>The research element definition publication date</b><br> 5059 * Type: <b>date</b><br> 5060 * Path: <b>ResearchElementDefinition.date</b><br> 5061 * </p> 5062 */ 5063 @SearchParamDefinition(name = "date", path = "ResearchElementDefinition.date", description = "The research element definition publication date", type = "date") 5064 public static final String SP_DATE = "date"; 5065 /** 5066 * <b>Fluent Client</b> search parameter constant for <b>date</b> 5067 * <p> 5068 * Description: <b>The research element definition publication date</b><br> 5069 * Type: <b>date</b><br> 5070 * Path: <b>ResearchElementDefinition.date</b><br> 5071 * </p> 5072 */ 5073 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 5074 SP_DATE); 5075 5076 /** 5077 * Search parameter: <b>identifier</b> 5078 * <p> 5079 * Description: <b>External identifier for the research element 5080 * definition</b><br> 5081 * Type: <b>token</b><br> 5082 * Path: <b>ResearchElementDefinition.identifier</b><br> 5083 * </p> 5084 */ 5085 @SearchParamDefinition(name = "identifier", path = "ResearchElementDefinition.identifier", description = "External identifier for the research element definition", type = "token") 5086 public static final String SP_IDENTIFIER = "identifier"; 5087 /** 5088 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 5089 * <p> 5090 * Description: <b>External identifier for the research element 5091 * definition</b><br> 5092 * Type: <b>token</b><br> 5093 * Path: <b>ResearchElementDefinition.identifier</b><br> 5094 * </p> 5095 */ 5096 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5097 SP_IDENTIFIER); 5098 5099 /** 5100 * Search parameter: <b>successor</b> 5101 * <p> 5102 * Description: <b>What resource is being referenced</b><br> 5103 * Type: <b>reference</b><br> 5104 * Path: <b>ResearchElementDefinition.relatedArtifact.resource</b><br> 5105 * </p> 5106 */ 5107 @SearchParamDefinition(name = "successor", path = "ResearchElementDefinition.relatedArtifact.where(type='successor').resource", description = "What resource is being referenced", type = "reference") 5108 public static final String SP_SUCCESSOR = "successor"; 5109 /** 5110 * <b>Fluent Client</b> search parameter constant for <b>successor</b> 5111 * <p> 5112 * Description: <b>What resource is being referenced</b><br> 5113 * Type: <b>reference</b><br> 5114 * Path: <b>ResearchElementDefinition.relatedArtifact.resource</b><br> 5115 * </p> 5116 */ 5117 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUCCESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5118 SP_SUCCESSOR); 5119 5120 /** 5121 * Constant for fluent queries to be used to add include statements. Specifies 5122 * the path value of "<b>ResearchElementDefinition:successor</b>". 5123 */ 5124 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUCCESSOR = new ca.uhn.fhir.model.api.Include( 5125 "ResearchElementDefinition:successor").toLocked(); 5126 5127 /** 5128 * Search parameter: <b>context-type-value</b> 5129 * <p> 5130 * Description: <b>A use context type and value assigned to the research element 5131 * definition</b><br> 5132 * Type: <b>composite</b><br> 5133 * Path: <b></b><br> 5134 * </p> 5135 */ 5136 @SearchParamDefinition(name = "context-type-value", path = "ResearchElementDefinition.useContext", description = "A use context type and value assigned to the research element definition", type = "composite", compositeOf = { 5137 "context-type", "context" }) 5138 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 5139 /** 5140 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 5141 * <p> 5142 * Description: <b>A use context type and value assigned to the research element 5143 * definition</b><br> 5144 * Type: <b>composite</b><br> 5145 * Path: <b></b><br> 5146 * </p> 5147 */ 5148 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 5149 SP_CONTEXT_TYPE_VALUE); 5150 5151 /** 5152 * Search parameter: <b>jurisdiction</b> 5153 * <p> 5154 * Description: <b>Intended jurisdiction for the research element 5155 * definition</b><br> 5156 * Type: <b>token</b><br> 5157 * Path: <b>ResearchElementDefinition.jurisdiction</b><br> 5158 * </p> 5159 */ 5160 @SearchParamDefinition(name = "jurisdiction", path = "ResearchElementDefinition.jurisdiction", description = "Intended jurisdiction for the research element definition", type = "token") 5161 public static final String SP_JURISDICTION = "jurisdiction"; 5162 /** 5163 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 5164 * <p> 5165 * Description: <b>Intended jurisdiction for the research element 5166 * definition</b><br> 5167 * Type: <b>token</b><br> 5168 * Path: <b>ResearchElementDefinition.jurisdiction</b><br> 5169 * </p> 5170 */ 5171 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5172 SP_JURISDICTION); 5173 5174 /** 5175 * Search parameter: <b>description</b> 5176 * <p> 5177 * Description: <b>The description of the research element definition</b><br> 5178 * Type: <b>string</b><br> 5179 * Path: <b>ResearchElementDefinition.description</b><br> 5180 * </p> 5181 */ 5182 @SearchParamDefinition(name = "description", path = "ResearchElementDefinition.description", description = "The description of the research element definition", type = "string") 5183 public static final String SP_DESCRIPTION = "description"; 5184 /** 5185 * <b>Fluent Client</b> search parameter constant for <b>description</b> 5186 * <p> 5187 * Description: <b>The description of the research element definition</b><br> 5188 * Type: <b>string</b><br> 5189 * Path: <b>ResearchElementDefinition.description</b><br> 5190 * </p> 5191 */ 5192 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 5193 SP_DESCRIPTION); 5194 5195 /** 5196 * Search parameter: <b>derived-from</b> 5197 * <p> 5198 * Description: <b>What resource is being referenced</b><br> 5199 * Type: <b>reference</b><br> 5200 * Path: <b>ResearchElementDefinition.relatedArtifact.resource</b><br> 5201 * </p> 5202 */ 5203 @SearchParamDefinition(name = "derived-from", path = "ResearchElementDefinition.relatedArtifact.where(type='derived-from').resource", description = "What resource is being referenced", type = "reference") 5204 public static final String SP_DERIVED_FROM = "derived-from"; 5205 /** 5206 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 5207 * <p> 5208 * Description: <b>What resource is being referenced</b><br> 5209 * Type: <b>reference</b><br> 5210 * Path: <b>ResearchElementDefinition.relatedArtifact.resource</b><br> 5211 * </p> 5212 */ 5213 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5214 SP_DERIVED_FROM); 5215 5216 /** 5217 * Constant for fluent queries to be used to add include statements. Specifies 5218 * the path value of "<b>ResearchElementDefinition:derived-from</b>". 5219 */ 5220 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include( 5221 "ResearchElementDefinition:derived-from").toLocked(); 5222 5223 /** 5224 * Search parameter: <b>context-type</b> 5225 * <p> 5226 * Description: <b>A type of use context assigned to the research element 5227 * definition</b><br> 5228 * Type: <b>token</b><br> 5229 * Path: <b>ResearchElementDefinition.useContext.code</b><br> 5230 * </p> 5231 */ 5232 @SearchParamDefinition(name = "context-type", path = "ResearchElementDefinition.useContext.code", description = "A type of use context assigned to the research element definition", type = "token") 5233 public static final String SP_CONTEXT_TYPE = "context-type"; 5234 /** 5235 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 5236 * <p> 5237 * Description: <b>A type of use context assigned to the research element 5238 * definition</b><br> 5239 * Type: <b>token</b><br> 5240 * Path: <b>ResearchElementDefinition.useContext.code</b><br> 5241 * </p> 5242 */ 5243 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5244 SP_CONTEXT_TYPE); 5245 5246 /** 5247 * Search parameter: <b>predecessor</b> 5248 * <p> 5249 * Description: <b>What resource is being referenced</b><br> 5250 * Type: <b>reference</b><br> 5251 * Path: <b>ResearchElementDefinition.relatedArtifact.resource</b><br> 5252 * </p> 5253 */ 5254 @SearchParamDefinition(name = "predecessor", path = "ResearchElementDefinition.relatedArtifact.where(type='predecessor').resource", description = "What resource is being referenced", type = "reference") 5255 public static final String SP_PREDECESSOR = "predecessor"; 5256 /** 5257 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 5258 * <p> 5259 * Description: <b>What resource is being referenced</b><br> 5260 * Type: <b>reference</b><br> 5261 * Path: <b>ResearchElementDefinition.relatedArtifact.resource</b><br> 5262 * </p> 5263 */ 5264 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5265 SP_PREDECESSOR); 5266 5267 /** 5268 * Constant for fluent queries to be used to add include statements. Specifies 5269 * the path value of "<b>ResearchElementDefinition:predecessor</b>". 5270 */ 5271 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include( 5272 "ResearchElementDefinition:predecessor").toLocked(); 5273 5274 /** 5275 * Search parameter: <b>title</b> 5276 * <p> 5277 * Description: <b>The human-friendly name of the research element 5278 * definition</b><br> 5279 * Type: <b>string</b><br> 5280 * Path: <b>ResearchElementDefinition.title</b><br> 5281 * </p> 5282 */ 5283 @SearchParamDefinition(name = "title", path = "ResearchElementDefinition.title", description = "The human-friendly name of the research element definition", type = "string") 5284 public static final String SP_TITLE = "title"; 5285 /** 5286 * <b>Fluent Client</b> search parameter constant for <b>title</b> 5287 * <p> 5288 * Description: <b>The human-friendly name of the research element 5289 * definition</b><br> 5290 * Type: <b>string</b><br> 5291 * Path: <b>ResearchElementDefinition.title</b><br> 5292 * </p> 5293 */ 5294 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 5295 SP_TITLE); 5296 5297 /** 5298 * Search parameter: <b>composed-of</b> 5299 * <p> 5300 * Description: <b>What resource is being referenced</b><br> 5301 * Type: <b>reference</b><br> 5302 * Path: <b>ResearchElementDefinition.relatedArtifact.resource</b><br> 5303 * </p> 5304 */ 5305 @SearchParamDefinition(name = "composed-of", path = "ResearchElementDefinition.relatedArtifact.where(type='composed-of').resource", description = "What resource is being referenced", type = "reference") 5306 public static final String SP_COMPOSED_OF = "composed-of"; 5307 /** 5308 * <b>Fluent Client</b> search parameter constant for <b>composed-of</b> 5309 * <p> 5310 * Description: <b>What resource is being referenced</b><br> 5311 * Type: <b>reference</b><br> 5312 * Path: <b>ResearchElementDefinition.relatedArtifact.resource</b><br> 5313 * </p> 5314 */ 5315 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPOSED_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5316 SP_COMPOSED_OF); 5317 5318 /** 5319 * Constant for fluent queries to be used to add include statements. Specifies 5320 * the path value of "<b>ResearchElementDefinition:composed-of</b>". 5321 */ 5322 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPOSED_OF = new ca.uhn.fhir.model.api.Include( 5323 "ResearchElementDefinition:composed-of").toLocked(); 5324 5325 /** 5326 * Search parameter: <b>version</b> 5327 * <p> 5328 * Description: <b>The business version of the research element 5329 * definition</b><br> 5330 * Type: <b>token</b><br> 5331 * Path: <b>ResearchElementDefinition.version</b><br> 5332 * </p> 5333 */ 5334 @SearchParamDefinition(name = "version", path = "ResearchElementDefinition.version", description = "The business version of the research element definition", type = "token") 5335 public static final String SP_VERSION = "version"; 5336 /** 5337 * <b>Fluent Client</b> search parameter constant for <b>version</b> 5338 * <p> 5339 * Description: <b>The business version of the research element 5340 * definition</b><br> 5341 * Type: <b>token</b><br> 5342 * Path: <b>ResearchElementDefinition.version</b><br> 5343 * </p> 5344 */ 5345 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5346 SP_VERSION); 5347 5348 /** 5349 * Search parameter: <b>url</b> 5350 * <p> 5351 * Description: <b>The uri that identifies the research element 5352 * definition</b><br> 5353 * Type: <b>uri</b><br> 5354 * Path: <b>ResearchElementDefinition.url</b><br> 5355 * </p> 5356 */ 5357 @SearchParamDefinition(name = "url", path = "ResearchElementDefinition.url", description = "The uri that identifies the research element definition", type = "uri") 5358 public static final String SP_URL = "url"; 5359 /** 5360 * <b>Fluent Client</b> search parameter constant for <b>url</b> 5361 * <p> 5362 * Description: <b>The uri that identifies the research element 5363 * definition</b><br> 5364 * Type: <b>uri</b><br> 5365 * Path: <b>ResearchElementDefinition.url</b><br> 5366 * </p> 5367 */ 5368 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 5369 5370 /** 5371 * Search parameter: <b>context-quantity</b> 5372 * <p> 5373 * Description: <b>A quantity- or range-valued use context assigned to the 5374 * research element definition</b><br> 5375 * Type: <b>quantity</b><br> 5376 * Path: <b>ResearchElementDefinition.useContext.valueQuantity, 5377 * ResearchElementDefinition.useContext.valueRange</b><br> 5378 * </p> 5379 */ 5380 @SearchParamDefinition(name = "context-quantity", path = "(ResearchElementDefinition.useContext.value as Quantity) | (ResearchElementDefinition.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the research element definition", type = "quantity") 5381 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 5382 /** 5383 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 5384 * <p> 5385 * Description: <b>A quantity- or range-valued use context assigned to the 5386 * research element definition</b><br> 5387 * Type: <b>quantity</b><br> 5388 * Path: <b>ResearchElementDefinition.useContext.valueQuantity, 5389 * ResearchElementDefinition.useContext.valueRange</b><br> 5390 * </p> 5391 */ 5392 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 5393 SP_CONTEXT_QUANTITY); 5394 5395 /** 5396 * Search parameter: <b>effective</b> 5397 * <p> 5398 * Description: <b>The time during which the research element definition is 5399 * intended to be in use</b><br> 5400 * Type: <b>date</b><br> 5401 * Path: <b>ResearchElementDefinition.effectivePeriod</b><br> 5402 * </p> 5403 */ 5404 @SearchParamDefinition(name = "effective", path = "ResearchElementDefinition.effectivePeriod", description = "The time during which the research element definition is intended to be in use", type = "date") 5405 public static final String SP_EFFECTIVE = "effective"; 5406 /** 5407 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 5408 * <p> 5409 * Description: <b>The time during which the research element definition is 5410 * intended to be in use</b><br> 5411 * Type: <b>date</b><br> 5412 * Path: <b>ResearchElementDefinition.effectivePeriod</b><br> 5413 * </p> 5414 */ 5415 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam( 5416 SP_EFFECTIVE); 5417 5418 /** 5419 * Search parameter: <b>depends-on</b> 5420 * <p> 5421 * Description: <b>What resource is being referenced</b><br> 5422 * Type: <b>reference</b><br> 5423 * Path: <b>ResearchElementDefinition.relatedArtifact.resource, 5424 * ResearchElementDefinition.library</b><br> 5425 * </p> 5426 */ 5427 @SearchParamDefinition(name = "depends-on", path = "ResearchElementDefinition.relatedArtifact.where(type='depends-on').resource | ResearchElementDefinition.library", description = "What resource is being referenced", type = "reference") 5428 public static final String SP_DEPENDS_ON = "depends-on"; 5429 /** 5430 * <b>Fluent Client</b> search parameter constant for <b>depends-on</b> 5431 * <p> 5432 * Description: <b>What resource is being referenced</b><br> 5433 * Type: <b>reference</b><br> 5434 * Path: <b>ResearchElementDefinition.relatedArtifact.resource, 5435 * ResearchElementDefinition.library</b><br> 5436 * </p> 5437 */ 5438 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEPENDS_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5439 SP_DEPENDS_ON); 5440 5441 /** 5442 * Constant for fluent queries to be used to add include statements. Specifies 5443 * the path value of "<b>ResearchElementDefinition:depends-on</b>". 5444 */ 5445 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEPENDS_ON = new ca.uhn.fhir.model.api.Include( 5446 "ResearchElementDefinition:depends-on").toLocked(); 5447 5448 /** 5449 * Search parameter: <b>name</b> 5450 * <p> 5451 * Description: <b>Computationally friendly name of the research element 5452 * definition</b><br> 5453 * Type: <b>string</b><br> 5454 * Path: <b>ResearchElementDefinition.name</b><br> 5455 * </p> 5456 */ 5457 @SearchParamDefinition(name = "name", path = "ResearchElementDefinition.name", description = "Computationally friendly name of the research element definition", type = "string") 5458 public static final String SP_NAME = "name"; 5459 /** 5460 * <b>Fluent Client</b> search parameter constant for <b>name</b> 5461 * <p> 5462 * Description: <b>Computationally friendly name of the research element 5463 * definition</b><br> 5464 * Type: <b>string</b><br> 5465 * Path: <b>ResearchElementDefinition.name</b><br> 5466 * </p> 5467 */ 5468 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 5469 SP_NAME); 5470 5471 /** 5472 * Search parameter: <b>context</b> 5473 * <p> 5474 * Description: <b>A use context assigned to the research element 5475 * definition</b><br> 5476 * Type: <b>token</b><br> 5477 * Path: <b>ResearchElementDefinition.useContext.valueCodeableConcept</b><br> 5478 * </p> 5479 */ 5480 @SearchParamDefinition(name = "context", path = "(ResearchElementDefinition.useContext.value as CodeableConcept)", description = "A use context assigned to the research element definition", type = "token") 5481 public static final String SP_CONTEXT = "context"; 5482 /** 5483 * <b>Fluent Client</b> search parameter constant for <b>context</b> 5484 * <p> 5485 * Description: <b>A use context assigned to the research element 5486 * definition</b><br> 5487 * Type: <b>token</b><br> 5488 * Path: <b>ResearchElementDefinition.useContext.valueCodeableConcept</b><br> 5489 * </p> 5490 */ 5491 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5492 SP_CONTEXT); 5493 5494 /** 5495 * Search parameter: <b>publisher</b> 5496 * <p> 5497 * Description: <b>Name of the publisher of the research element 5498 * definition</b><br> 5499 * Type: <b>string</b><br> 5500 * Path: <b>ResearchElementDefinition.publisher</b><br> 5501 * </p> 5502 */ 5503 @SearchParamDefinition(name = "publisher", path = "ResearchElementDefinition.publisher", description = "Name of the publisher of the research element definition", type = "string") 5504 public static final String SP_PUBLISHER = "publisher"; 5505 /** 5506 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 5507 * <p> 5508 * Description: <b>Name of the publisher of the research element 5509 * definition</b><br> 5510 * Type: <b>string</b><br> 5511 * Path: <b>ResearchElementDefinition.publisher</b><br> 5512 * </p> 5513 */ 5514 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 5515 SP_PUBLISHER); 5516 5517 /** 5518 * Search parameter: <b>topic</b> 5519 * <p> 5520 * Description: <b>Topics associated with the ResearchElementDefinition</b><br> 5521 * Type: <b>token</b><br> 5522 * Path: <b>ResearchElementDefinition.topic</b><br> 5523 * </p> 5524 */ 5525 @SearchParamDefinition(name = "topic", path = "ResearchElementDefinition.topic", description = "Topics associated with the ResearchElementDefinition", type = "token") 5526 public static final String SP_TOPIC = "topic"; 5527 /** 5528 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 5529 * <p> 5530 * Description: <b>Topics associated with the ResearchElementDefinition</b><br> 5531 * Type: <b>token</b><br> 5532 * Path: <b>ResearchElementDefinition.topic</b><br> 5533 * </p> 5534 */ 5535 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5536 SP_TOPIC); 5537 5538 /** 5539 * Search parameter: <b>context-type-quantity</b> 5540 * <p> 5541 * Description: <b>A use context type and quantity- or range-based value 5542 * assigned to the research element definition</b><br> 5543 * Type: <b>composite</b><br> 5544 * Path: <b></b><br> 5545 * </p> 5546 */ 5547 @SearchParamDefinition(name = "context-type-quantity", path = "ResearchElementDefinition.useContext", description = "A use context type and quantity- or range-based value assigned to the research element definition", type = "composite", compositeOf = { 5548 "context-type", "context-quantity" }) 5549 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 5550 /** 5551 * <b>Fluent Client</b> search parameter constant for 5552 * <b>context-type-quantity</b> 5553 * <p> 5554 * Description: <b>A use context type and quantity- or range-based value 5555 * assigned to the research element definition</b><br> 5556 * Type: <b>composite</b><br> 5557 * Path: <b></b><br> 5558 * </p> 5559 */ 5560 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 5561 SP_CONTEXT_TYPE_QUANTITY); 5562 5563 /** 5564 * Search parameter: <b>status</b> 5565 * <p> 5566 * Description: <b>The current status of the research element definition</b><br> 5567 * Type: <b>token</b><br> 5568 * Path: <b>ResearchElementDefinition.status</b><br> 5569 * </p> 5570 */ 5571 @SearchParamDefinition(name = "status", path = "ResearchElementDefinition.status", description = "The current status of the research element definition", type = "token") 5572 public static final String SP_STATUS = "status"; 5573 /** 5574 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5575 * <p> 5576 * Description: <b>The current status of the research element definition</b><br> 5577 * Type: <b>token</b><br> 5578 * Path: <b>ResearchElementDefinition.status</b><br> 5579 * </p> 5580 */ 5581 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5582 SP_STATUS); 5583 5584}