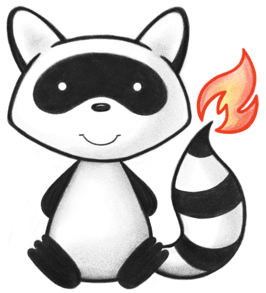
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.List; 034 035import org.hl7.fhir.exceptions.FHIRException; 036import org.hl7.fhir.instance.model.api.IAnyResource; 037import org.hl7.fhir.utilities.Utilities; 038 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.Description; 041 042/** 043 * This is the base resource type for everything. 044 */ 045public abstract class Resource extends BaseResource implements IAnyResource { 046 047 /** 048 * The logical id of the resource, as used in the URL for the resource. Once 049 * assigned, this value never changes. 050 */ 051 @Child(name = "id", type = { IdType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 052 @Description(shortDefinition = "Logical id of this artifact", formalDefinition = "The logical id of the resource, as used in the URL for the resource. Once assigned, this value never changes.") 053 protected IdType id; 054 055 /** 056 * The metadata about the resource. This is content that is maintained by the 057 * infrastructure. Changes to the content might not always be associated with 058 * version changes to the resource. 059 */ 060 @Child(name = "meta", type = { Meta.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 061 @Description(shortDefinition = "Metadata about the resource", formalDefinition = "The metadata about the resource. This is content that is maintained by the infrastructure. Changes to the content might not always be associated with version changes to the resource.") 062 protected Meta meta; 063 064 /** 065 * A reference to a set of rules that were followed when the resource was 066 * constructed, and which must be understood when processing the content. Often, 067 * this is a reference to an implementation guide that defines the special rules 068 * along with other profiles etc. 069 */ 070 @Child(name = "implicitRules", type = { UriType.class }, order = 2, min = 0, max = 1, modifier = true, summary = true) 071 @Description(shortDefinition = "A set of rules under which this content was created", formalDefinition = "A reference to a set of rules that were followed when the resource was constructed, and which must be understood when processing the content. Often, this is a reference to an implementation guide that defines the special rules along with other profiles etc.") 072 protected UriType implicitRules; 073 074 /** 075 * The base language in which the resource is written. 076 */ 077 @Child(name = "language", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 078 @Description(shortDefinition = "Language of the resource content", formalDefinition = "The base language in which the resource is written.") 079 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/languages") 080 protected CodeType language; 081 082 private static final long serialVersionUID = -559462759L; 083 084 /** 085 * Constructor 086 */ 087 public Resource() { 088 super(); 089 } 090 091 /** 092 * @return {@link #id} (The logical id of the resource, as used in the URL for 093 * the resource. Once assigned, this value never changes.). This is the 094 * underlying object with id, value and extensions. The accessor "getId" 095 * gives direct access to the value 096 */ 097 public IdType getIdElement() { 098 if (this.id == null) 099 if (Configuration.errorOnAutoCreate()) 100 throw new Error("Attempt to auto-create Resource.id"); 101 else if (Configuration.doAutoCreate()) 102 this.id = new IdType(); // bb 103 return this.id; 104 } 105 106 public boolean hasIdElement() { 107 return this.id != null && !this.id.isEmpty(); 108 } 109 110 public boolean hasId() { 111 return this.id != null && !this.id.isEmpty(); 112 } 113 114 /** 115 * @param value {@link #id} (The logical id of the resource, as used in the URL 116 * for the resource. Once assigned, this value never changes.). 117 * This is the underlying object with id, value and extensions. The 118 * accessor "getId" gives direct access to the value 119 */ 120 public Resource setIdElement(IdType value) { 121 this.id = value; 122 return this; 123 } 124 125 /** 126 * @return The most complete id value of the resource, containing all available 127 * context and history. Once assigned this value never changes. NOTE: 128 * this value is NOT limited to just the logical id property of a 129 * resource id. 130 * @see IdType 131 * @see IdType#getValue() 132 */ 133 public String getId() { 134 return this.id == null ? null : this.id.getValue(); 135 } 136 137 /** 138 * @param value The id value of the resource. Once assigned, this value never 139 * changes. 140 * 141 * @see IdType 142 * @see IdType#setValue(String) 143 */ 144 public Resource setId(String value) { 145 if (Utilities.noString(value)) 146 this.id = null; 147 else { 148 if (this.id == null) 149 this.id = new IdType(); 150 this.id.setValue(value); 151 } 152 return this; 153 } 154 155 /** 156 * @return the logical ID part of this resource's id 157 * @see IdType#getIdPart() 158 */ 159 public String getIdPart() { 160 return getIdElement().getIdPart(); 161 } 162 163 /** 164 * @return {@link #meta} (The metadata about the resource. This is content that 165 * is maintained by the infrastructure. Changes to the content might not 166 * always be associated with version changes to the resource.) 167 */ 168 public Meta getMeta() { 169 if (this.meta == null) 170 if (Configuration.errorOnAutoCreate()) 171 throw new Error("Attempt to auto-create Resource.meta"); 172 else if (Configuration.doAutoCreate()) 173 this.meta = new Meta(); // cc 174 return this.meta; 175 } 176 177 public boolean hasMeta() { 178 return this.meta != null && !this.meta.isEmpty(); 179 } 180 181 /** 182 * @param value {@link #meta} (The metadata about the resource. This is content 183 * that is maintained by the infrastructure. Changes to the content 184 * might not always be associated with version changes to the 185 * resource.) 186 */ 187 public Resource setMeta(Meta value) { 188 this.meta = value; 189 return this; 190 } 191 192 /** 193 * @return {@link #implicitRules} (A reference to a set of rules that were 194 * followed when the resource was constructed, and which must be 195 * understood when processing the content. Often, this is a reference to 196 * an implementation guide that defines the special rules along with 197 * other profiles etc.). This is the underlying object with id, value 198 * and extensions. The accessor "getImplicitRules" gives direct access 199 * to the value 200 */ 201 public UriType getImplicitRulesElement() { 202 if (this.implicitRules == null) 203 if (Configuration.errorOnAutoCreate()) 204 throw new Error("Attempt to auto-create Resource.implicitRules"); 205 else if (Configuration.doAutoCreate()) 206 this.implicitRules = new UriType(); // bb 207 return this.implicitRules; 208 } 209 210 public boolean hasImplicitRulesElement() { 211 return this.implicitRules != null && !this.implicitRules.isEmpty(); 212 } 213 214 public boolean hasImplicitRules() { 215 return this.implicitRules != null && !this.implicitRules.isEmpty(); 216 } 217 218 /** 219 * @param value {@link #implicitRules} (A reference to a set of rules that were 220 * followed when the resource was constructed, and which must be 221 * understood when processing the content. Often, this is a 222 * reference to an implementation guide that defines the special 223 * rules along with other profiles etc.). This is the underlying 224 * object with id, value and extensions. The accessor 225 * "getImplicitRules" gives direct access to the value 226 */ 227 public Resource setImplicitRulesElement(UriType value) { 228 this.implicitRules = value; 229 return this; 230 } 231 232 /** 233 * @return A reference to a set of rules that were followed when the resource 234 * was constructed, and which must be understood when processing the 235 * content. Often, this is a reference to an implementation guide that 236 * defines the special rules along with other profiles etc. 237 */ 238 public String getImplicitRules() { 239 return this.implicitRules == null ? null : this.implicitRules.getValue(); 240 } 241 242 /** 243 * @param value A reference to a set of rules that were followed when the 244 * resource was constructed, and which must be understood when 245 * processing the content. Often, this is a reference to an 246 * implementation guide that defines the special rules along with 247 * other profiles etc. 248 */ 249 public Resource setImplicitRules(String value) { 250 if (Utilities.noString(value)) 251 this.implicitRules = null; 252 else { 253 if (this.implicitRules == null) 254 this.implicitRules = new UriType(); 255 this.implicitRules.setValue(value); 256 } 257 return this; 258 } 259 260 /** 261 * @return {@link #language} (The base language in which the resource is 262 * written.). This is the underlying object with id, value and 263 * extensions. The accessor "getLanguage" gives direct access to the 264 * value 265 */ 266 public CodeType getLanguageElement() { 267 if (this.language == null) 268 if (Configuration.errorOnAutoCreate()) 269 throw new Error("Attempt to auto-create Resource.language"); 270 else if (Configuration.doAutoCreate()) 271 this.language = new CodeType(); // bb 272 return this.language; 273 } 274 275 public boolean hasLanguageElement() { 276 return this.language != null && !this.language.isEmpty(); 277 } 278 279 public boolean hasLanguage() { 280 return this.language != null && !this.language.isEmpty(); 281 } 282 283 /** 284 * @param value {@link #language} (The base language in which the resource is 285 * written.). This is the underlying object with id, value and 286 * extensions. The accessor "getLanguage" gives direct access to 287 * the value 288 */ 289 public Resource setLanguageElement(CodeType value) { 290 this.language = value; 291 return this; 292 } 293 294 /** 295 * @return The base language in which the resource is written. 296 */ 297 public String getLanguage() { 298 return this.language == null ? null : this.language.getValue(); 299 } 300 301 /** 302 * @param value The base language in which the resource is written. 303 */ 304 public Resource setLanguage(String value) { 305 if (Utilities.noString(value)) 306 this.language = null; 307 else { 308 if (this.language == null) 309 this.language = new CodeType(); 310 this.language.setValue(value); 311 } 312 return this; 313 } 314 315 protected void listChildren(List<Property> children) { 316 children.add(new Property("id", "id", 317 "The logical id of the resource, as used in the URL for the resource. Once assigned, this value never changes.", 318 0, 1, id)); 319 children.add(new Property("meta", "Meta", 320 "The metadata about the resource. This is content that is maintained by the infrastructure. Changes to the content might not always be associated with version changes to the resource.", 321 0, 1, meta)); 322 children.add(new Property("implicitRules", "uri", 323 "A reference to a set of rules that were followed when the resource was constructed, and which must be understood when processing the content. Often, this is a reference to an implementation guide that defines the special rules along with other profiles etc.", 324 0, 1, implicitRules)); 325 children 326 .add(new Property("language", "code", "The base language in which the resource is written.", 0, 1, language)); 327 } 328 329 @Override 330 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 331 switch (_hash) { 332 case 3355: 333 /* id */ return new Property("id", "id", 334 "The logical id of the resource, as used in the URL for the resource. Once assigned, this value never changes.", 335 0, 1, id); 336 case 3347973: 337 /* meta */ return new Property("meta", "Meta", 338 "The metadata about the resource. This is content that is maintained by the infrastructure. Changes to the content might not always be associated with version changes to the resource.", 339 0, 1, meta); 340 case -961826286: 341 /* implicitRules */ return new Property("implicitRules", "uri", 342 "A reference to a set of rules that were followed when the resource was constructed, and which must be understood when processing the content. Often, this is a reference to an implementation guide that defines the special rules along with other profiles etc.", 343 0, 1, implicitRules); 344 case -1613589672: 345 /* language */ return new Property("language", "code", "The base language in which the resource is written.", 0, 346 1, language); 347 default: 348 return super.getNamedProperty(_hash, _name, _checkValid); 349 } 350 351 } 352 353 @Override 354 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 355 switch (hash) { 356 case 3355: 357 /* id */ return this.id == null ? new Base[0] : new Base[] { this.id }; // IdType 358 case 3347973: 359 /* meta */ return this.meta == null ? new Base[0] : new Base[] { this.meta }; // Meta 360 case -961826286: 361 /* implicitRules */ return this.implicitRules == null ? new Base[0] : new Base[] { this.implicitRules }; // UriType 362 case -1613589672: 363 /* language */ return this.language == null ? new Base[0] : new Base[] { this.language }; // CodeType 364 default: 365 return super.getProperty(hash, name, checkValid); 366 } 367 368 } 369 370 @Override 371 public Base setProperty(int hash, String name, Base value) throws FHIRException { 372 switch (hash) { 373 case 3355: // id 374 this.id = castToId(value); // IdType 375 return value; 376 case 3347973: // meta 377 this.meta = castToMeta(value); // Meta 378 return value; 379 case -961826286: // implicitRules 380 this.implicitRules = castToUri(value); // UriType 381 return value; 382 case -1613589672: // language 383 this.language = castToCode(value); // CodeType 384 return value; 385 default: 386 return super.setProperty(hash, name, value); 387 } 388 389 } 390 391 @Override 392 public Base setProperty(String name, Base value) throws FHIRException { 393 if (name.equals("id")) { 394 this.id = castToId(value); // IdType 395 } else if (name.equals("meta")) { 396 this.meta = castToMeta(value); // Meta 397 } else if (name.equals("implicitRules")) { 398 this.implicitRules = castToUri(value); // UriType 399 } else if (name.equals("language")) { 400 this.language = castToCode(value); // CodeType 401 } else 402 return super.setProperty(name, value); 403 return value; 404 } 405 406 @Override 407 public Base makeProperty(int hash, String name) throws FHIRException { 408 switch (hash) { 409 case 3355: 410 return getIdElement(); 411 case 3347973: 412 return getMeta(); 413 case -961826286: 414 return getImplicitRulesElement(); 415 case -1613589672: 416 return getLanguageElement(); 417 default: 418 return super.makeProperty(hash, name); 419 } 420 421 } 422 423 @Override 424 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 425 switch (hash) { 426 case 3355: 427 /* id */ return new String[] { "id" }; 428 case 3347973: 429 /* meta */ return new String[] { "Meta" }; 430 case -961826286: 431 /* implicitRules */ return new String[] { "uri" }; 432 case -1613589672: 433 /* language */ return new String[] { "code" }; 434 default: 435 return super.getTypesForProperty(hash, name); 436 } 437 438 } 439 440 @Override 441 public Base addChild(String name) throws FHIRException { 442 if (name.equals("id")) { 443 throw new FHIRException("Cannot call addChild on a singleton property Resource.id"); 444 } else if (name.equals("meta")) { 445 this.meta = new Meta(); 446 return this.meta; 447 } else if (name.equals("implicitRules")) { 448 throw new FHIRException("Cannot call addChild on a singleton property Resource.implicitRules"); 449 } else if (name.equals("language")) { 450 throw new FHIRException("Cannot call addChild on a singleton property Resource.language"); 451 } else 452 return super.addChild(name); 453 } 454 455 public String fhirType() { 456 return "Resource"; 457 458 } 459 460 public abstract Resource copy(); 461 462 public void copyValues(Resource dst) { 463 dst.id = id == null ? null : id.copy(); 464 dst.meta = meta == null ? null : meta.copy(); 465 dst.implicitRules = implicitRules == null ? null : implicitRules.copy(); 466 dst.language = language == null ? null : language.copy(); 467 } 468 469 @Override 470 public boolean equalsDeep(Base other_) { 471 if (!super.equalsDeep(other_)) 472 return false; 473 if (!(other_ instanceof Resource)) 474 return false; 475 Resource o = (Resource) other_; 476 return compareDeep(id, o.id, true) && compareDeep(meta, o.meta, true) 477 && compareDeep(implicitRules, o.implicitRules, true) && compareDeep(language, o.language, true); 478 } 479 480 @Override 481 public boolean equalsShallow(Base other_) { 482 if (!super.equalsShallow(other_)) 483 return false; 484 if (!(other_ instanceof Resource)) 485 return false; 486 Resource o = (Resource) other_; 487 return compareValues(id, o.id, true) && compareValues(implicitRules, o.implicitRules, true) 488 && compareValues(language, o.language, true); 489 } 490 491 public boolean isEmpty() { 492 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(id, meta, implicitRules, language); 493 } 494 495 @Override 496 public String getIdBase() { 497 return getId(); 498 } 499 500 @Override 501 public void setIdBase(String value) { 502 setId(value); 503 } 504 505 public abstract ResourceType getResourceType(); 506// added from java-adornments.txt: 507 508 public String getLanguage(String defValue) { 509 return hasLanguage() ? getLanguage() : defValue; 510 } 511 512// end addition 513 514}