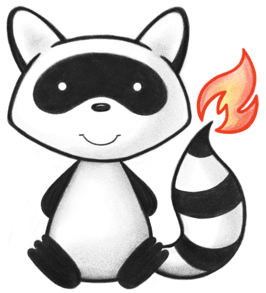
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 042import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.ResourceDef; 050import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 051 052/** 053 * The RiskEvidenceSynthesis resource describes the likelihood of an outcome in 054 * a population plus exposure state where the risk estimate is derived from a 055 * combination of research studies. 056 */ 057@ResourceDef(name = "RiskEvidenceSynthesis", profile = "http://hl7.org/fhir/StructureDefinition/RiskEvidenceSynthesis") 058@ChildOrder(names = { "url", "identifier", "version", "name", "title", "status", "date", "publisher", "contact", 059 "description", "note", "useContext", "jurisdiction", "copyright", "approvalDate", "lastReviewDate", 060 "effectivePeriod", "topic", "author", "editor", "reviewer", "endorser", "relatedArtifact", "synthesisType", 061 "studyType", "population", "exposure", "outcome", "sampleSize", "riskEstimate", "certainty" }) 062public class RiskEvidenceSynthesis extends MetadataResource { 063 064 @Block() 065 public static class RiskEvidenceSynthesisSampleSizeComponent extends BackboneElement implements IBaseBackboneElement { 066 /** 067 * Human-readable summary of sample size. 068 */ 069 @Child(name = "description", type = { 070 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 071 @Description(shortDefinition = "Description of sample size", formalDefinition = "Human-readable summary of sample size.") 072 protected StringType description; 073 074 /** 075 * Number of studies included in this evidence synthesis. 076 */ 077 @Child(name = "numberOfStudies", type = { 078 IntegerType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 079 @Description(shortDefinition = "How many studies?", formalDefinition = "Number of studies included in this evidence synthesis.") 080 protected IntegerType numberOfStudies; 081 082 /** 083 * Number of participants included in this evidence synthesis. 084 */ 085 @Child(name = "numberOfParticipants", type = { 086 IntegerType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 087 @Description(shortDefinition = "How many participants?", formalDefinition = "Number of participants included in this evidence synthesis.") 088 protected IntegerType numberOfParticipants; 089 090 private static final long serialVersionUID = -1116074476L; 091 092 /** 093 * Constructor 094 */ 095 public RiskEvidenceSynthesisSampleSizeComponent() { 096 super(); 097 } 098 099 /** 100 * @return {@link #description} (Human-readable summary of sample size.). This 101 * is the underlying object with id, value and extensions. The accessor 102 * "getDescription" gives direct access to the value 103 */ 104 public StringType getDescriptionElement() { 105 if (this.description == null) 106 if (Configuration.errorOnAutoCreate()) 107 throw new Error("Attempt to auto-create RiskEvidenceSynthesisSampleSizeComponent.description"); 108 else if (Configuration.doAutoCreate()) 109 this.description = new StringType(); // bb 110 return this.description; 111 } 112 113 public boolean hasDescriptionElement() { 114 return this.description != null && !this.description.isEmpty(); 115 } 116 117 public boolean hasDescription() { 118 return this.description != null && !this.description.isEmpty(); 119 } 120 121 /** 122 * @param value {@link #description} (Human-readable summary of sample size.). 123 * This is the underlying object with id, value and extensions. The 124 * accessor "getDescription" gives direct access to the value 125 */ 126 public RiskEvidenceSynthesisSampleSizeComponent setDescriptionElement(StringType value) { 127 this.description = value; 128 return this; 129 } 130 131 /** 132 * @return Human-readable summary of sample size. 133 */ 134 public String getDescription() { 135 return this.description == null ? null : this.description.getValue(); 136 } 137 138 /** 139 * @param value Human-readable summary of sample size. 140 */ 141 public RiskEvidenceSynthesisSampleSizeComponent setDescription(String value) { 142 if (Utilities.noString(value)) 143 this.description = null; 144 else { 145 if (this.description == null) 146 this.description = new StringType(); 147 this.description.setValue(value); 148 } 149 return this; 150 } 151 152 /** 153 * @return {@link #numberOfStudies} (Number of studies included in this evidence 154 * synthesis.). This is the underlying object with id, value and 155 * extensions. The accessor "getNumberOfStudies" gives direct access to 156 * the value 157 */ 158 public IntegerType getNumberOfStudiesElement() { 159 if (this.numberOfStudies == null) 160 if (Configuration.errorOnAutoCreate()) 161 throw new Error("Attempt to auto-create RiskEvidenceSynthesisSampleSizeComponent.numberOfStudies"); 162 else if (Configuration.doAutoCreate()) 163 this.numberOfStudies = new IntegerType(); // bb 164 return this.numberOfStudies; 165 } 166 167 public boolean hasNumberOfStudiesElement() { 168 return this.numberOfStudies != null && !this.numberOfStudies.isEmpty(); 169 } 170 171 public boolean hasNumberOfStudies() { 172 return this.numberOfStudies != null && !this.numberOfStudies.isEmpty(); 173 } 174 175 /** 176 * @param value {@link #numberOfStudies} (Number of studies included in this 177 * evidence synthesis.). This is the underlying object with id, 178 * value and extensions. The accessor "getNumberOfStudies" gives 179 * direct access to the value 180 */ 181 public RiskEvidenceSynthesisSampleSizeComponent setNumberOfStudiesElement(IntegerType value) { 182 this.numberOfStudies = value; 183 return this; 184 } 185 186 /** 187 * @return Number of studies included in this evidence synthesis. 188 */ 189 public int getNumberOfStudies() { 190 return this.numberOfStudies == null || this.numberOfStudies.isEmpty() ? 0 : this.numberOfStudies.getValue(); 191 } 192 193 /** 194 * @param value Number of studies included in this evidence synthesis. 195 */ 196 public RiskEvidenceSynthesisSampleSizeComponent setNumberOfStudies(int value) { 197 if (this.numberOfStudies == null) 198 this.numberOfStudies = new IntegerType(); 199 this.numberOfStudies.setValue(value); 200 return this; 201 } 202 203 /** 204 * @return {@link #numberOfParticipants} (Number of participants included in 205 * this evidence synthesis.). This is the underlying object with id, 206 * value and extensions. The accessor "getNumberOfParticipants" gives 207 * direct access to the value 208 */ 209 public IntegerType getNumberOfParticipantsElement() { 210 if (this.numberOfParticipants == null) 211 if (Configuration.errorOnAutoCreate()) 212 throw new Error("Attempt to auto-create RiskEvidenceSynthesisSampleSizeComponent.numberOfParticipants"); 213 else if (Configuration.doAutoCreate()) 214 this.numberOfParticipants = new IntegerType(); // bb 215 return this.numberOfParticipants; 216 } 217 218 public boolean hasNumberOfParticipantsElement() { 219 return this.numberOfParticipants != null && !this.numberOfParticipants.isEmpty(); 220 } 221 222 public boolean hasNumberOfParticipants() { 223 return this.numberOfParticipants != null && !this.numberOfParticipants.isEmpty(); 224 } 225 226 /** 227 * @param value {@link #numberOfParticipants} (Number of participants included 228 * in this evidence synthesis.). This is the underlying object with 229 * id, value and extensions. The accessor "getNumberOfParticipants" 230 * gives direct access to the value 231 */ 232 public RiskEvidenceSynthesisSampleSizeComponent setNumberOfParticipantsElement(IntegerType value) { 233 this.numberOfParticipants = value; 234 return this; 235 } 236 237 /** 238 * @return Number of participants included in this evidence synthesis. 239 */ 240 public int getNumberOfParticipants() { 241 return this.numberOfParticipants == null || this.numberOfParticipants.isEmpty() ? 0 242 : this.numberOfParticipants.getValue(); 243 } 244 245 /** 246 * @param value Number of participants included in this evidence synthesis. 247 */ 248 public RiskEvidenceSynthesisSampleSizeComponent setNumberOfParticipants(int value) { 249 if (this.numberOfParticipants == null) 250 this.numberOfParticipants = new IntegerType(); 251 this.numberOfParticipants.setValue(value); 252 return this; 253 } 254 255 protected void listChildren(List<Property> children) { 256 super.listChildren(children); 257 children.add(new Property("description", "string", "Human-readable summary of sample size.", 0, 1, description)); 258 children.add(new Property("numberOfStudies", "integer", "Number of studies included in this evidence synthesis.", 259 0, 1, numberOfStudies)); 260 children.add(new Property("numberOfParticipants", "integer", 261 "Number of participants included in this evidence synthesis.", 0, 1, numberOfParticipants)); 262 } 263 264 @Override 265 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 266 switch (_hash) { 267 case -1724546052: 268 /* description */ return new Property("description", "string", "Human-readable summary of sample size.", 0, 1, 269 description); 270 case -177467129: 271 /* numberOfStudies */ return new Property("numberOfStudies", "integer", 272 "Number of studies included in this evidence synthesis.", 0, 1, numberOfStudies); 273 case 1799357120: 274 /* numberOfParticipants */ return new Property("numberOfParticipants", "integer", 275 "Number of participants included in this evidence synthesis.", 0, 1, numberOfParticipants); 276 default: 277 return super.getNamedProperty(_hash, _name, _checkValid); 278 } 279 280 } 281 282 @Override 283 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 284 switch (hash) { 285 case -1724546052: 286 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 287 case -177467129: 288 /* numberOfStudies */ return this.numberOfStudies == null ? new Base[0] : new Base[] { this.numberOfStudies }; // IntegerType 289 case 1799357120: 290 /* numberOfParticipants */ return this.numberOfParticipants == null ? new Base[0] 291 : new Base[] { this.numberOfParticipants }; // IntegerType 292 default: 293 return super.getProperty(hash, name, checkValid); 294 } 295 296 } 297 298 @Override 299 public Base setProperty(int hash, String name, Base value) throws FHIRException { 300 switch (hash) { 301 case -1724546052: // description 302 this.description = castToString(value); // StringType 303 return value; 304 case -177467129: // numberOfStudies 305 this.numberOfStudies = castToInteger(value); // IntegerType 306 return value; 307 case 1799357120: // numberOfParticipants 308 this.numberOfParticipants = castToInteger(value); // IntegerType 309 return value; 310 default: 311 return super.setProperty(hash, name, value); 312 } 313 314 } 315 316 @Override 317 public Base setProperty(String name, Base value) throws FHIRException { 318 if (name.equals("description")) { 319 this.description = castToString(value); // StringType 320 } else if (name.equals("numberOfStudies")) { 321 this.numberOfStudies = castToInteger(value); // IntegerType 322 } else if (name.equals("numberOfParticipants")) { 323 this.numberOfParticipants = castToInteger(value); // IntegerType 324 } else 325 return super.setProperty(name, value); 326 return value; 327 } 328 329 @Override 330 public void removeChild(String name, Base value) throws FHIRException { 331 if (name.equals("description")) { 332 this.description = null; 333 } else if (name.equals("numberOfStudies")) { 334 this.numberOfStudies = null; 335 } else if (name.equals("numberOfParticipants")) { 336 this.numberOfParticipants = null; 337 } else 338 super.removeChild(name, value); 339 340 } 341 342 @Override 343 public Base makeProperty(int hash, String name) throws FHIRException { 344 switch (hash) { 345 case -1724546052: 346 return getDescriptionElement(); 347 case -177467129: 348 return getNumberOfStudiesElement(); 349 case 1799357120: 350 return getNumberOfParticipantsElement(); 351 default: 352 return super.makeProperty(hash, name); 353 } 354 355 } 356 357 @Override 358 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 359 switch (hash) { 360 case -1724546052: 361 /* description */ return new String[] { "string" }; 362 case -177467129: 363 /* numberOfStudies */ return new String[] { "integer" }; 364 case 1799357120: 365 /* numberOfParticipants */ return new String[] { "integer" }; 366 default: 367 return super.getTypesForProperty(hash, name); 368 } 369 370 } 371 372 @Override 373 public Base addChild(String name) throws FHIRException { 374 if (name.equals("description")) { 375 throw new FHIRException("Cannot call addChild on a singleton property RiskEvidenceSynthesis.description"); 376 } else if (name.equals("numberOfStudies")) { 377 throw new FHIRException("Cannot call addChild on a singleton property RiskEvidenceSynthesis.numberOfStudies"); 378 } else if (name.equals("numberOfParticipants")) { 379 throw new FHIRException("Cannot call addChild on a singleton property RiskEvidenceSynthesis.numberOfParticipants"); 380 } else 381 return super.addChild(name); 382 } 383 384 public RiskEvidenceSynthesisSampleSizeComponent copy() { 385 RiskEvidenceSynthesisSampleSizeComponent dst = new RiskEvidenceSynthesisSampleSizeComponent(); 386 copyValues(dst); 387 return dst; 388 } 389 390 public void copyValues(RiskEvidenceSynthesisSampleSizeComponent dst) { 391 super.copyValues(dst); 392 dst.description = description == null ? null : description.copy(); 393 dst.numberOfStudies = numberOfStudies == null ? null : numberOfStudies.copy(); 394 dst.numberOfParticipants = numberOfParticipants == null ? null : numberOfParticipants.copy(); 395 } 396 397 @Override 398 public boolean equalsDeep(Base other_) { 399 if (!super.equalsDeep(other_)) 400 return false; 401 if (!(other_ instanceof RiskEvidenceSynthesisSampleSizeComponent)) 402 return false; 403 RiskEvidenceSynthesisSampleSizeComponent o = (RiskEvidenceSynthesisSampleSizeComponent) other_; 404 return compareDeep(description, o.description, true) && compareDeep(numberOfStudies, o.numberOfStudies, true) 405 && compareDeep(numberOfParticipants, o.numberOfParticipants, true); 406 } 407 408 @Override 409 public boolean equalsShallow(Base other_) { 410 if (!super.equalsShallow(other_)) 411 return false; 412 if (!(other_ instanceof RiskEvidenceSynthesisSampleSizeComponent)) 413 return false; 414 RiskEvidenceSynthesisSampleSizeComponent o = (RiskEvidenceSynthesisSampleSizeComponent) other_; 415 return compareValues(description, o.description, true) && compareValues(numberOfStudies, o.numberOfStudies, true) 416 && compareValues(numberOfParticipants, o.numberOfParticipants, true); 417 } 418 419 public boolean isEmpty() { 420 return super.isEmpty() 421 && ca.uhn.fhir.util.ElementUtil.isEmpty(description, numberOfStudies, numberOfParticipants); 422 } 423 424 public String fhirType() { 425 return "RiskEvidenceSynthesis.sampleSize"; 426 427 } 428 429 } 430 431 @Block() 432 public static class RiskEvidenceSynthesisRiskEstimateComponent extends BackboneElement 433 implements IBaseBackboneElement { 434 /** 435 * Human-readable summary of risk estimate. 436 */ 437 @Child(name = "description", type = { 438 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 439 @Description(shortDefinition = "Description of risk estimate", formalDefinition = "Human-readable summary of risk estimate.") 440 protected StringType description; 441 442 /** 443 * Examples include proportion and mean. 444 */ 445 @Child(name = "type", type = { 446 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 447 @Description(shortDefinition = "Type of risk estimate", formalDefinition = "Examples include proportion and mean.") 448 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/risk-estimate-type") 449 protected CodeableConcept type; 450 451 /** 452 * The point estimate of the risk estimate. 453 */ 454 @Child(name = "value", type = { DecimalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 455 @Description(shortDefinition = "Point estimate", formalDefinition = "The point estimate of the risk estimate.") 456 protected DecimalType value; 457 458 /** 459 * Specifies the UCUM unit for the outcome. 460 */ 461 @Child(name = "unitOfMeasure", type = { 462 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 463 @Description(shortDefinition = "What unit is the outcome described in?", formalDefinition = "Specifies the UCUM unit for the outcome.") 464 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ucum-units") 465 protected CodeableConcept unitOfMeasure; 466 467 /** 468 * The sample size for the group that was measured for this risk estimate. 469 */ 470 @Child(name = "denominatorCount", type = { 471 IntegerType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 472 @Description(shortDefinition = "Sample size for group measured", formalDefinition = "The sample size for the group that was measured for this risk estimate.") 473 protected IntegerType denominatorCount; 474 475 /** 476 * The number of group members with the outcome of interest. 477 */ 478 @Child(name = "numeratorCount", type = { 479 IntegerType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 480 @Description(shortDefinition = "Number with the outcome", formalDefinition = "The number of group members with the outcome of interest.") 481 protected IntegerType numeratorCount; 482 483 /** 484 * A description of the precision of the estimate for the effect. 485 */ 486 @Child(name = "precisionEstimate", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 487 @Description(shortDefinition = "How precise the estimate is", formalDefinition = "A description of the precision of the estimate for the effect.") 488 protected List<RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent> precisionEstimate; 489 490 private static final long serialVersionUID = -15987415L; 491 492 /** 493 * Constructor 494 */ 495 public RiskEvidenceSynthesisRiskEstimateComponent() { 496 super(); 497 } 498 499 /** 500 * @return {@link #description} (Human-readable summary of risk estimate.). This 501 * is the underlying object with id, value and extensions. The accessor 502 * "getDescription" gives direct access to the value 503 */ 504 public StringType getDescriptionElement() { 505 if (this.description == null) 506 if (Configuration.errorOnAutoCreate()) 507 throw new Error("Attempt to auto-create RiskEvidenceSynthesisRiskEstimateComponent.description"); 508 else if (Configuration.doAutoCreate()) 509 this.description = new StringType(); // bb 510 return this.description; 511 } 512 513 public boolean hasDescriptionElement() { 514 return this.description != null && !this.description.isEmpty(); 515 } 516 517 public boolean hasDescription() { 518 return this.description != null && !this.description.isEmpty(); 519 } 520 521 /** 522 * @param value {@link #description} (Human-readable summary of risk estimate.). 523 * This is the underlying object with id, value and extensions. The 524 * accessor "getDescription" gives direct access to the value 525 */ 526 public RiskEvidenceSynthesisRiskEstimateComponent setDescriptionElement(StringType value) { 527 this.description = value; 528 return this; 529 } 530 531 /** 532 * @return Human-readable summary of risk estimate. 533 */ 534 public String getDescription() { 535 return this.description == null ? null : this.description.getValue(); 536 } 537 538 /** 539 * @param value Human-readable summary of risk estimate. 540 */ 541 public RiskEvidenceSynthesisRiskEstimateComponent setDescription(String value) { 542 if (Utilities.noString(value)) 543 this.description = null; 544 else { 545 if (this.description == null) 546 this.description = new StringType(); 547 this.description.setValue(value); 548 } 549 return this; 550 } 551 552 /** 553 * @return {@link #type} (Examples include proportion and mean.) 554 */ 555 public CodeableConcept getType() { 556 if (this.type == null) 557 if (Configuration.errorOnAutoCreate()) 558 throw new Error("Attempt to auto-create RiskEvidenceSynthesisRiskEstimateComponent.type"); 559 else if (Configuration.doAutoCreate()) 560 this.type = new CodeableConcept(); // cc 561 return this.type; 562 } 563 564 public boolean hasType() { 565 return this.type != null && !this.type.isEmpty(); 566 } 567 568 /** 569 * @param value {@link #type} (Examples include proportion and mean.) 570 */ 571 public RiskEvidenceSynthesisRiskEstimateComponent setType(CodeableConcept value) { 572 this.type = value; 573 return this; 574 } 575 576 /** 577 * @return {@link #value} (The point estimate of the risk estimate.). This is 578 * the underlying object with id, value and extensions. The accessor 579 * "getValue" gives direct access to the value 580 */ 581 public DecimalType getValueElement() { 582 if (this.value == null) 583 if (Configuration.errorOnAutoCreate()) 584 throw new Error("Attempt to auto-create RiskEvidenceSynthesisRiskEstimateComponent.value"); 585 else if (Configuration.doAutoCreate()) 586 this.value = new DecimalType(); // bb 587 return this.value; 588 } 589 590 public boolean hasValueElement() { 591 return this.value != null && !this.value.isEmpty(); 592 } 593 594 public boolean hasValue() { 595 return this.value != null && !this.value.isEmpty(); 596 } 597 598 /** 599 * @param value {@link #value} (The point estimate of the risk estimate.). This 600 * is the underlying object with id, value and extensions. The 601 * accessor "getValue" gives direct access to the value 602 */ 603 public RiskEvidenceSynthesisRiskEstimateComponent setValueElement(DecimalType value) { 604 this.value = value; 605 return this; 606 } 607 608 /** 609 * @return The point estimate of the risk estimate. 610 */ 611 public BigDecimal getValue() { 612 return this.value == null ? null : this.value.getValue(); 613 } 614 615 /** 616 * @param value The point estimate of the risk estimate. 617 */ 618 public RiskEvidenceSynthesisRiskEstimateComponent setValue(BigDecimal value) { 619 if (value == null) 620 this.value = null; 621 else { 622 if (this.value == null) 623 this.value = new DecimalType(); 624 this.value.setValue(value); 625 } 626 return this; 627 } 628 629 /** 630 * @param value The point estimate of the risk estimate. 631 */ 632 public RiskEvidenceSynthesisRiskEstimateComponent setValue(long value) { 633 this.value = new DecimalType(); 634 this.value.setValue(value); 635 return this; 636 } 637 638 /** 639 * @param value The point estimate of the risk estimate. 640 */ 641 public RiskEvidenceSynthesisRiskEstimateComponent setValue(double value) { 642 this.value = new DecimalType(); 643 this.value.setValue(value); 644 return this; 645 } 646 647 /** 648 * @return {@link #unitOfMeasure} (Specifies the UCUM unit for the outcome.) 649 */ 650 public CodeableConcept getUnitOfMeasure() { 651 if (this.unitOfMeasure == null) 652 if (Configuration.errorOnAutoCreate()) 653 throw new Error("Attempt to auto-create RiskEvidenceSynthesisRiskEstimateComponent.unitOfMeasure"); 654 else if (Configuration.doAutoCreate()) 655 this.unitOfMeasure = new CodeableConcept(); // cc 656 return this.unitOfMeasure; 657 } 658 659 public boolean hasUnitOfMeasure() { 660 return this.unitOfMeasure != null && !this.unitOfMeasure.isEmpty(); 661 } 662 663 /** 664 * @param value {@link #unitOfMeasure} (Specifies the UCUM unit for the 665 * outcome.) 666 */ 667 public RiskEvidenceSynthesisRiskEstimateComponent setUnitOfMeasure(CodeableConcept value) { 668 this.unitOfMeasure = value; 669 return this; 670 } 671 672 /** 673 * @return {@link #denominatorCount} (The sample size for the group that was 674 * measured for this risk estimate.). This is the underlying object with 675 * id, value and extensions. The accessor "getDenominatorCount" gives 676 * direct access to the value 677 */ 678 public IntegerType getDenominatorCountElement() { 679 if (this.denominatorCount == null) 680 if (Configuration.errorOnAutoCreate()) 681 throw new Error("Attempt to auto-create RiskEvidenceSynthesisRiskEstimateComponent.denominatorCount"); 682 else if (Configuration.doAutoCreate()) 683 this.denominatorCount = new IntegerType(); // bb 684 return this.denominatorCount; 685 } 686 687 public boolean hasDenominatorCountElement() { 688 return this.denominatorCount != null && !this.denominatorCount.isEmpty(); 689 } 690 691 public boolean hasDenominatorCount() { 692 return this.denominatorCount != null && !this.denominatorCount.isEmpty(); 693 } 694 695 /** 696 * @param value {@link #denominatorCount} (The sample size for the group that 697 * was measured for this risk estimate.). This is the underlying 698 * object with id, value and extensions. The accessor 699 * "getDenominatorCount" gives direct access to the value 700 */ 701 public RiskEvidenceSynthesisRiskEstimateComponent setDenominatorCountElement(IntegerType value) { 702 this.denominatorCount = value; 703 return this; 704 } 705 706 /** 707 * @return The sample size for the group that was measured for this risk 708 * estimate. 709 */ 710 public int getDenominatorCount() { 711 return this.denominatorCount == null || this.denominatorCount.isEmpty() ? 0 : this.denominatorCount.getValue(); 712 } 713 714 /** 715 * @param value The sample size for the group that was measured for this risk 716 * estimate. 717 */ 718 public RiskEvidenceSynthesisRiskEstimateComponent setDenominatorCount(int value) { 719 if (this.denominatorCount == null) 720 this.denominatorCount = new IntegerType(); 721 this.denominatorCount.setValue(value); 722 return this; 723 } 724 725 /** 726 * @return {@link #numeratorCount} (The number of group members with the outcome 727 * of interest.). This is the underlying object with id, value and 728 * extensions. The accessor "getNumeratorCount" gives direct access to 729 * the value 730 */ 731 public IntegerType getNumeratorCountElement() { 732 if (this.numeratorCount == null) 733 if (Configuration.errorOnAutoCreate()) 734 throw new Error("Attempt to auto-create RiskEvidenceSynthesisRiskEstimateComponent.numeratorCount"); 735 else if (Configuration.doAutoCreate()) 736 this.numeratorCount = new IntegerType(); // bb 737 return this.numeratorCount; 738 } 739 740 public boolean hasNumeratorCountElement() { 741 return this.numeratorCount != null && !this.numeratorCount.isEmpty(); 742 } 743 744 public boolean hasNumeratorCount() { 745 return this.numeratorCount != null && !this.numeratorCount.isEmpty(); 746 } 747 748 /** 749 * @param value {@link #numeratorCount} (The number of group members with the 750 * outcome of interest.). This is the underlying object with id, 751 * value and extensions. The accessor "getNumeratorCount" gives 752 * direct access to the value 753 */ 754 public RiskEvidenceSynthesisRiskEstimateComponent setNumeratorCountElement(IntegerType value) { 755 this.numeratorCount = value; 756 return this; 757 } 758 759 /** 760 * @return The number of group members with the outcome of interest. 761 */ 762 public int getNumeratorCount() { 763 return this.numeratorCount == null || this.numeratorCount.isEmpty() ? 0 : this.numeratorCount.getValue(); 764 } 765 766 /** 767 * @param value The number of group members with the outcome of interest. 768 */ 769 public RiskEvidenceSynthesisRiskEstimateComponent setNumeratorCount(int value) { 770 if (this.numeratorCount == null) 771 this.numeratorCount = new IntegerType(); 772 this.numeratorCount.setValue(value); 773 return this; 774 } 775 776 /** 777 * @return {@link #precisionEstimate} (A description of the precision of the 778 * estimate for the effect.) 779 */ 780 public List<RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent> getPrecisionEstimate() { 781 if (this.precisionEstimate == null) 782 this.precisionEstimate = new ArrayList<RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent>(); 783 return this.precisionEstimate; 784 } 785 786 /** 787 * @return Returns a reference to <code>this</code> for easy method chaining 788 */ 789 public RiskEvidenceSynthesisRiskEstimateComponent setPrecisionEstimate( 790 List<RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent> thePrecisionEstimate) { 791 this.precisionEstimate = thePrecisionEstimate; 792 return this; 793 } 794 795 public boolean hasPrecisionEstimate() { 796 if (this.precisionEstimate == null) 797 return false; 798 for (RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent item : this.precisionEstimate) 799 if (!item.isEmpty()) 800 return true; 801 return false; 802 } 803 804 public RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent addPrecisionEstimate() { // 3 805 RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent t = new RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent(); 806 if (this.precisionEstimate == null) 807 this.precisionEstimate = new ArrayList<RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent>(); 808 this.precisionEstimate.add(t); 809 return t; 810 } 811 812 public RiskEvidenceSynthesisRiskEstimateComponent addPrecisionEstimate( 813 RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent t) { // 3 814 if (t == null) 815 return this; 816 if (this.precisionEstimate == null) 817 this.precisionEstimate = new ArrayList<RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent>(); 818 this.precisionEstimate.add(t); 819 return this; 820 } 821 822 /** 823 * @return The first repetition of repeating field {@link #precisionEstimate}, 824 * creating it if it does not already exist 825 */ 826 public RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent getPrecisionEstimateFirstRep() { 827 if (getPrecisionEstimate().isEmpty()) { 828 addPrecisionEstimate(); 829 } 830 return getPrecisionEstimate().get(0); 831 } 832 833 protected void listChildren(List<Property> children) { 834 super.listChildren(children); 835 children 836 .add(new Property("description", "string", "Human-readable summary of risk estimate.", 0, 1, description)); 837 children.add(new Property("type", "CodeableConcept", "Examples include proportion and mean.", 0, 1, type)); 838 children.add(new Property("value", "decimal", "The point estimate of the risk estimate.", 0, 1, value)); 839 children.add(new Property("unitOfMeasure", "CodeableConcept", "Specifies the UCUM unit for the outcome.", 0, 1, 840 unitOfMeasure)); 841 children.add(new Property("denominatorCount", "integer", 842 "The sample size for the group that was measured for this risk estimate.", 0, 1, denominatorCount)); 843 children.add(new Property("numeratorCount", "integer", 844 "The number of group members with the outcome of interest.", 0, 1, numeratorCount)); 845 children 846 .add(new Property("precisionEstimate", "", "A description of the precision of the estimate for the effect.", 847 0, java.lang.Integer.MAX_VALUE, precisionEstimate)); 848 } 849 850 @Override 851 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 852 switch (_hash) { 853 case -1724546052: 854 /* description */ return new Property("description", "string", "Human-readable summary of risk estimate.", 0, 1, 855 description); 856 case 3575610: 857 /* type */ return new Property("type", "CodeableConcept", "Examples include proportion and mean.", 0, 1, type); 858 case 111972721: 859 /* value */ return new Property("value", "decimal", "The point estimate of the risk estimate.", 0, 1, value); 860 case -750257565: 861 /* unitOfMeasure */ return new Property("unitOfMeasure", "CodeableConcept", 862 "Specifies the UCUM unit for the outcome.", 0, 1, unitOfMeasure); 863 case 1323191881: 864 /* denominatorCount */ return new Property("denominatorCount", "integer", 865 "The sample size for the group that was measured for this risk estimate.", 0, 1, denominatorCount); 866 case -755509242: 867 /* numeratorCount */ return new Property("numeratorCount", "integer", 868 "The number of group members with the outcome of interest.", 0, 1, numeratorCount); 869 case 339632070: 870 /* precisionEstimate */ return new Property("precisionEstimate", "", 871 "A description of the precision of the estimate for the effect.", 0, java.lang.Integer.MAX_VALUE, 872 precisionEstimate); 873 default: 874 return super.getNamedProperty(_hash, _name, _checkValid); 875 } 876 877 } 878 879 @Override 880 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 881 switch (hash) { 882 case -1724546052: 883 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 884 case 3575610: 885 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 886 case 111972721: 887 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // DecimalType 888 case -750257565: 889 /* unitOfMeasure */ return this.unitOfMeasure == null ? new Base[0] : new Base[] { this.unitOfMeasure }; // CodeableConcept 890 case 1323191881: 891 /* denominatorCount */ return this.denominatorCount == null ? new Base[0] 892 : new Base[] { this.denominatorCount }; // IntegerType 893 case -755509242: 894 /* numeratorCount */ return this.numeratorCount == null ? new Base[0] : new Base[] { this.numeratorCount }; // IntegerType 895 case 339632070: 896 /* precisionEstimate */ return this.precisionEstimate == null ? new Base[0] 897 : this.precisionEstimate.toArray(new Base[this.precisionEstimate.size()]); // RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent 898 default: 899 return super.getProperty(hash, name, checkValid); 900 } 901 902 } 903 904 @Override 905 public Base setProperty(int hash, String name, Base value) throws FHIRException { 906 switch (hash) { 907 case -1724546052: // description 908 this.description = castToString(value); // StringType 909 return value; 910 case 3575610: // type 911 this.type = castToCodeableConcept(value); // CodeableConcept 912 return value; 913 case 111972721: // value 914 this.value = castToDecimal(value); // DecimalType 915 return value; 916 case -750257565: // unitOfMeasure 917 this.unitOfMeasure = castToCodeableConcept(value); // CodeableConcept 918 return value; 919 case 1323191881: // denominatorCount 920 this.denominatorCount = castToInteger(value); // IntegerType 921 return value; 922 case -755509242: // numeratorCount 923 this.numeratorCount = castToInteger(value); // IntegerType 924 return value; 925 case 339632070: // precisionEstimate 926 this.getPrecisionEstimate().add((RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent) value); // RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent 927 return value; 928 default: 929 return super.setProperty(hash, name, value); 930 } 931 932 } 933 934 @Override 935 public Base setProperty(String name, Base value) throws FHIRException { 936 if (name.equals("description")) { 937 this.description = castToString(value); // StringType 938 } else if (name.equals("type")) { 939 this.type = castToCodeableConcept(value); // CodeableConcept 940 } else if (name.equals("value")) { 941 this.value = castToDecimal(value); // DecimalType 942 } else if (name.equals("unitOfMeasure")) { 943 this.unitOfMeasure = castToCodeableConcept(value); // CodeableConcept 944 } else if (name.equals("denominatorCount")) { 945 this.denominatorCount = castToInteger(value); // IntegerType 946 } else if (name.equals("numeratorCount")) { 947 this.numeratorCount = castToInteger(value); // IntegerType 948 } else if (name.equals("precisionEstimate")) { 949 this.getPrecisionEstimate().add((RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent) value); 950 } else 951 return super.setProperty(name, value); 952 return value; 953 } 954 955 @Override 956 public void removeChild(String name, Base value) throws FHIRException { 957 if (name.equals("description")) { 958 this.description = null; 959 } else if (name.equals("type")) { 960 this.type = null; 961 } else if (name.equals("value")) { 962 this.value = null; 963 } else if (name.equals("unitOfMeasure")) { 964 this.unitOfMeasure = null; 965 } else if (name.equals("denominatorCount")) { 966 this.denominatorCount = null; 967 } else if (name.equals("numeratorCount")) { 968 this.numeratorCount = null; 969 } else if (name.equals("precisionEstimate")) { 970 this.getPrecisionEstimate().remove((RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent) value); 971 } else 972 super.removeChild(name, value); 973 974 } 975 976 @Override 977 public Base makeProperty(int hash, String name) throws FHIRException { 978 switch (hash) { 979 case -1724546052: 980 return getDescriptionElement(); 981 case 3575610: 982 return getType(); 983 case 111972721: 984 return getValueElement(); 985 case -750257565: 986 return getUnitOfMeasure(); 987 case 1323191881: 988 return getDenominatorCountElement(); 989 case -755509242: 990 return getNumeratorCountElement(); 991 case 339632070: 992 return addPrecisionEstimate(); 993 default: 994 return super.makeProperty(hash, name); 995 } 996 997 } 998 999 @Override 1000 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1001 switch (hash) { 1002 case -1724546052: 1003 /* description */ return new String[] { "string" }; 1004 case 3575610: 1005 /* type */ return new String[] { "CodeableConcept" }; 1006 case 111972721: 1007 /* value */ return new String[] { "decimal" }; 1008 case -750257565: 1009 /* unitOfMeasure */ return new String[] { "CodeableConcept" }; 1010 case 1323191881: 1011 /* denominatorCount */ return new String[] { "integer" }; 1012 case -755509242: 1013 /* numeratorCount */ return new String[] { "integer" }; 1014 case 339632070: 1015 /* precisionEstimate */ return new String[] {}; 1016 default: 1017 return super.getTypesForProperty(hash, name); 1018 } 1019 1020 } 1021 1022 @Override 1023 public Base addChild(String name) throws FHIRException { 1024 if (name.equals("description")) { 1025 throw new FHIRException("Cannot call addChild on a singleton property RiskEvidenceSynthesis.description"); 1026 } else if (name.equals("type")) { 1027 this.type = new CodeableConcept(); 1028 return this.type; 1029 } else if (name.equals("value")) { 1030 throw new FHIRException("Cannot call addChild on a singleton property RiskEvidenceSynthesis.value"); 1031 } else if (name.equals("unitOfMeasure")) { 1032 this.unitOfMeasure = new CodeableConcept(); 1033 return this.unitOfMeasure; 1034 } else if (name.equals("denominatorCount")) { 1035 throw new FHIRException("Cannot call addChild on a singleton property RiskEvidenceSynthesis.denominatorCount"); 1036 } else if (name.equals("numeratorCount")) { 1037 throw new FHIRException("Cannot call addChild on a singleton property RiskEvidenceSynthesis.numeratorCount"); 1038 } else if (name.equals("precisionEstimate")) { 1039 return addPrecisionEstimate(); 1040 } else 1041 return super.addChild(name); 1042 } 1043 1044 public RiskEvidenceSynthesisRiskEstimateComponent copy() { 1045 RiskEvidenceSynthesisRiskEstimateComponent dst = new RiskEvidenceSynthesisRiskEstimateComponent(); 1046 copyValues(dst); 1047 return dst; 1048 } 1049 1050 public void copyValues(RiskEvidenceSynthesisRiskEstimateComponent dst) { 1051 super.copyValues(dst); 1052 dst.description = description == null ? null : description.copy(); 1053 dst.type = type == null ? null : type.copy(); 1054 dst.value = value == null ? null : value.copy(); 1055 dst.unitOfMeasure = unitOfMeasure == null ? null : unitOfMeasure.copy(); 1056 dst.denominatorCount = denominatorCount == null ? null : denominatorCount.copy(); 1057 dst.numeratorCount = numeratorCount == null ? null : numeratorCount.copy(); 1058 if (precisionEstimate != null) { 1059 dst.precisionEstimate = new ArrayList<RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent>(); 1060 for (RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent i : precisionEstimate) 1061 dst.precisionEstimate.add(i.copy()); 1062 } 1063 ; 1064 } 1065 1066 @Override 1067 public boolean equalsDeep(Base other_) { 1068 if (!super.equalsDeep(other_)) 1069 return false; 1070 if (!(other_ instanceof RiskEvidenceSynthesisRiskEstimateComponent)) 1071 return false; 1072 RiskEvidenceSynthesisRiskEstimateComponent o = (RiskEvidenceSynthesisRiskEstimateComponent) other_; 1073 return compareDeep(description, o.description, true) && compareDeep(type, o.type, true) 1074 && compareDeep(value, o.value, true) && compareDeep(unitOfMeasure, o.unitOfMeasure, true) 1075 && compareDeep(denominatorCount, o.denominatorCount, true) 1076 && compareDeep(numeratorCount, o.numeratorCount, true) 1077 && compareDeep(precisionEstimate, o.precisionEstimate, true); 1078 } 1079 1080 @Override 1081 public boolean equalsShallow(Base other_) { 1082 if (!super.equalsShallow(other_)) 1083 return false; 1084 if (!(other_ instanceof RiskEvidenceSynthesisRiskEstimateComponent)) 1085 return false; 1086 RiskEvidenceSynthesisRiskEstimateComponent o = (RiskEvidenceSynthesisRiskEstimateComponent) other_; 1087 return compareValues(description, o.description, true) && compareValues(value, o.value, true) 1088 && compareValues(denominatorCount, o.denominatorCount, true) 1089 && compareValues(numeratorCount, o.numeratorCount, true); 1090 } 1091 1092 public boolean isEmpty() { 1093 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, type, value, unitOfMeasure, 1094 denominatorCount, numeratorCount, precisionEstimate); 1095 } 1096 1097 public String fhirType() { 1098 return "RiskEvidenceSynthesis.riskEstimate"; 1099 1100 } 1101 1102 } 1103 1104 @Block() 1105 public static class RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent extends BackboneElement 1106 implements IBaseBackboneElement { 1107 /** 1108 * Examples include confidence interval and interquartile range. 1109 */ 1110 @Child(name = "type", type = { 1111 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1112 @Description(shortDefinition = "Type of precision estimate", formalDefinition = "Examples include confidence interval and interquartile range.") 1113 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/precision-estimate-type") 1114 protected CodeableConcept type; 1115 1116 /** 1117 * Use 95 for a 95% confidence interval. 1118 */ 1119 @Child(name = "level", type = { DecimalType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1120 @Description(shortDefinition = "Level of confidence interval", formalDefinition = "Use 95 for a 95% confidence interval.") 1121 protected DecimalType level; 1122 1123 /** 1124 * Lower bound of confidence interval. 1125 */ 1126 @Child(name = "from", type = { DecimalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1127 @Description(shortDefinition = "Lower bound", formalDefinition = "Lower bound of confidence interval.") 1128 protected DecimalType from; 1129 1130 /** 1131 * Upper bound of confidence interval. 1132 */ 1133 @Child(name = "to", type = { DecimalType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1134 @Description(shortDefinition = "Upper bound", formalDefinition = "Upper bound of confidence interval.") 1135 protected DecimalType to; 1136 1137 private static final long serialVersionUID = -110178057L; 1138 1139 /** 1140 * Constructor 1141 */ 1142 public RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent() { 1143 super(); 1144 } 1145 1146 /** 1147 * @return {@link #type} (Examples include confidence interval and interquartile 1148 * range.) 1149 */ 1150 public CodeableConcept getType() { 1151 if (this.type == null) 1152 if (Configuration.errorOnAutoCreate()) 1153 throw new Error("Attempt to auto-create RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent.type"); 1154 else if (Configuration.doAutoCreate()) 1155 this.type = new CodeableConcept(); // cc 1156 return this.type; 1157 } 1158 1159 public boolean hasType() { 1160 return this.type != null && !this.type.isEmpty(); 1161 } 1162 1163 /** 1164 * @param value {@link #type} (Examples include confidence interval and 1165 * interquartile range.) 1166 */ 1167 public RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent setType(CodeableConcept value) { 1168 this.type = value; 1169 return this; 1170 } 1171 1172 /** 1173 * @return {@link #level} (Use 95 for a 95% confidence interval.). This is the 1174 * underlying object with id, value and extensions. The accessor 1175 * "getLevel" gives direct access to the value 1176 */ 1177 public DecimalType getLevelElement() { 1178 if (this.level == null) 1179 if (Configuration.errorOnAutoCreate()) 1180 throw new Error("Attempt to auto-create RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent.level"); 1181 else if (Configuration.doAutoCreate()) 1182 this.level = new DecimalType(); // bb 1183 return this.level; 1184 } 1185 1186 public boolean hasLevelElement() { 1187 return this.level != null && !this.level.isEmpty(); 1188 } 1189 1190 public boolean hasLevel() { 1191 return this.level != null && !this.level.isEmpty(); 1192 } 1193 1194 /** 1195 * @param value {@link #level} (Use 95 for a 95% confidence interval.). This is 1196 * the underlying object with id, value and extensions. The 1197 * accessor "getLevel" gives direct access to the value 1198 */ 1199 public RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent setLevelElement(DecimalType value) { 1200 this.level = value; 1201 return this; 1202 } 1203 1204 /** 1205 * @return Use 95 for a 95% confidence interval. 1206 */ 1207 public BigDecimal getLevel() { 1208 return this.level == null ? null : this.level.getValue(); 1209 } 1210 1211 /** 1212 * @param value Use 95 for a 95% confidence interval. 1213 */ 1214 public RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent setLevel(BigDecimal value) { 1215 if (value == null) 1216 this.level = null; 1217 else { 1218 if (this.level == null) 1219 this.level = new DecimalType(); 1220 this.level.setValue(value); 1221 } 1222 return this; 1223 } 1224 1225 /** 1226 * @param value Use 95 for a 95% confidence interval. 1227 */ 1228 public RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent setLevel(long value) { 1229 this.level = new DecimalType(); 1230 this.level.setValue(value); 1231 return this; 1232 } 1233 1234 /** 1235 * @param value Use 95 for a 95% confidence interval. 1236 */ 1237 public RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent setLevel(double value) { 1238 this.level = new DecimalType(); 1239 this.level.setValue(value); 1240 return this; 1241 } 1242 1243 /** 1244 * @return {@link #from} (Lower bound of confidence interval.). This is the 1245 * underlying object with id, value and extensions. The accessor 1246 * "getFrom" gives direct access to the value 1247 */ 1248 public DecimalType getFromElement() { 1249 if (this.from == null) 1250 if (Configuration.errorOnAutoCreate()) 1251 throw new Error("Attempt to auto-create RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent.from"); 1252 else if (Configuration.doAutoCreate()) 1253 this.from = new DecimalType(); // bb 1254 return this.from; 1255 } 1256 1257 public boolean hasFromElement() { 1258 return this.from != null && !this.from.isEmpty(); 1259 } 1260 1261 public boolean hasFrom() { 1262 return this.from != null && !this.from.isEmpty(); 1263 } 1264 1265 /** 1266 * @param value {@link #from} (Lower bound of confidence interval.). This is the 1267 * underlying object with id, value and extensions. The accessor 1268 * "getFrom" gives direct access to the value 1269 */ 1270 public RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent setFromElement(DecimalType value) { 1271 this.from = value; 1272 return this; 1273 } 1274 1275 /** 1276 * @return Lower bound of confidence interval. 1277 */ 1278 public BigDecimal getFrom() { 1279 return this.from == null ? null : this.from.getValue(); 1280 } 1281 1282 /** 1283 * @param value Lower bound of confidence interval. 1284 */ 1285 public RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent setFrom(BigDecimal value) { 1286 if (value == null) 1287 this.from = null; 1288 else { 1289 if (this.from == null) 1290 this.from = new DecimalType(); 1291 this.from.setValue(value); 1292 } 1293 return this; 1294 } 1295 1296 /** 1297 * @param value Lower bound of confidence interval. 1298 */ 1299 public RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent setFrom(long value) { 1300 this.from = new DecimalType(); 1301 this.from.setValue(value); 1302 return this; 1303 } 1304 1305 /** 1306 * @param value Lower bound of confidence interval. 1307 */ 1308 public RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent setFrom(double value) { 1309 this.from = new DecimalType(); 1310 this.from.setValue(value); 1311 return this; 1312 } 1313 1314 /** 1315 * @return {@link #to} (Upper bound of confidence interval.). This is the 1316 * underlying object with id, value and extensions. The accessor "getTo" 1317 * gives direct access to the value 1318 */ 1319 public DecimalType getToElement() { 1320 if (this.to == null) 1321 if (Configuration.errorOnAutoCreate()) 1322 throw new Error("Attempt to auto-create RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent.to"); 1323 else if (Configuration.doAutoCreate()) 1324 this.to = new DecimalType(); // bb 1325 return this.to; 1326 } 1327 1328 public boolean hasToElement() { 1329 return this.to != null && !this.to.isEmpty(); 1330 } 1331 1332 public boolean hasTo() { 1333 return this.to != null && !this.to.isEmpty(); 1334 } 1335 1336 /** 1337 * @param value {@link #to} (Upper bound of confidence interval.). This is the 1338 * underlying object with id, value and extensions. The accessor 1339 * "getTo" gives direct access to the value 1340 */ 1341 public RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent setToElement(DecimalType value) { 1342 this.to = value; 1343 return this; 1344 } 1345 1346 /** 1347 * @return Upper bound of confidence interval. 1348 */ 1349 public BigDecimal getTo() { 1350 return this.to == null ? null : this.to.getValue(); 1351 } 1352 1353 /** 1354 * @param value Upper bound of confidence interval. 1355 */ 1356 public RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent setTo(BigDecimal value) { 1357 if (value == null) 1358 this.to = null; 1359 else { 1360 if (this.to == null) 1361 this.to = new DecimalType(); 1362 this.to.setValue(value); 1363 } 1364 return this; 1365 } 1366 1367 /** 1368 * @param value Upper bound of confidence interval. 1369 */ 1370 public RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent setTo(long value) { 1371 this.to = new DecimalType(); 1372 this.to.setValue(value); 1373 return this; 1374 } 1375 1376 /** 1377 * @param value Upper bound of confidence interval. 1378 */ 1379 public RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent setTo(double value) { 1380 this.to = new DecimalType(); 1381 this.to.setValue(value); 1382 return this; 1383 } 1384 1385 protected void listChildren(List<Property> children) { 1386 super.listChildren(children); 1387 children.add(new Property("type", "CodeableConcept", 1388 "Examples include confidence interval and interquartile range.", 0, 1, type)); 1389 children.add(new Property("level", "decimal", "Use 95 for a 95% confidence interval.", 0, 1, level)); 1390 children.add(new Property("from", "decimal", "Lower bound of confidence interval.", 0, 1, from)); 1391 children.add(new Property("to", "decimal", "Upper bound of confidence interval.", 0, 1, to)); 1392 } 1393 1394 @Override 1395 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1396 switch (_hash) { 1397 case 3575610: 1398 /* type */ return new Property("type", "CodeableConcept", 1399 "Examples include confidence interval and interquartile range.", 0, 1, type); 1400 case 102865796: 1401 /* level */ return new Property("level", "decimal", "Use 95 for a 95% confidence interval.", 0, 1, level); 1402 case 3151786: 1403 /* from */ return new Property("from", "decimal", "Lower bound of confidence interval.", 0, 1, from); 1404 case 3707: 1405 /* to */ return new Property("to", "decimal", "Upper bound of confidence interval.", 0, 1, to); 1406 default: 1407 return super.getNamedProperty(_hash, _name, _checkValid); 1408 } 1409 1410 } 1411 1412 @Override 1413 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1414 switch (hash) { 1415 case 3575610: 1416 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1417 case 102865796: 1418 /* level */ return this.level == null ? new Base[0] : new Base[] { this.level }; // DecimalType 1419 case 3151786: 1420 /* from */ return this.from == null ? new Base[0] : new Base[] { this.from }; // DecimalType 1421 case 3707: 1422 /* to */ return this.to == null ? new Base[0] : new Base[] { this.to }; // DecimalType 1423 default: 1424 return super.getProperty(hash, name, checkValid); 1425 } 1426 1427 } 1428 1429 @Override 1430 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1431 switch (hash) { 1432 case 3575610: // type 1433 this.type = castToCodeableConcept(value); // CodeableConcept 1434 return value; 1435 case 102865796: // level 1436 this.level = castToDecimal(value); // DecimalType 1437 return value; 1438 case 3151786: // from 1439 this.from = castToDecimal(value); // DecimalType 1440 return value; 1441 case 3707: // to 1442 this.to = castToDecimal(value); // DecimalType 1443 return value; 1444 default: 1445 return super.setProperty(hash, name, value); 1446 } 1447 1448 } 1449 1450 @Override 1451 public Base setProperty(String name, Base value) throws FHIRException { 1452 if (name.equals("type")) { 1453 this.type = castToCodeableConcept(value); // CodeableConcept 1454 } else if (name.equals("level")) { 1455 this.level = castToDecimal(value); // DecimalType 1456 } else if (name.equals("from")) { 1457 this.from = castToDecimal(value); // DecimalType 1458 } else if (name.equals("to")) { 1459 this.to = castToDecimal(value); // DecimalType 1460 } else 1461 return super.setProperty(name, value); 1462 return value; 1463 } 1464 1465 @Override 1466 public void removeChild(String name, Base value) throws FHIRException { 1467 if (name.equals("type")) { 1468 this.type = null; 1469 } else if (name.equals("level")) { 1470 this.level = null; 1471 } else if (name.equals("from")) { 1472 this.from = null; 1473 } else if (name.equals("to")) { 1474 this.to = null; 1475 } else 1476 super.removeChild(name, value); 1477 1478 } 1479 1480 @Override 1481 public Base makeProperty(int hash, String name) throws FHIRException { 1482 switch (hash) { 1483 case 3575610: 1484 return getType(); 1485 case 102865796: 1486 return getLevelElement(); 1487 case 3151786: 1488 return getFromElement(); 1489 case 3707: 1490 return getToElement(); 1491 default: 1492 return super.makeProperty(hash, name); 1493 } 1494 1495 } 1496 1497 @Override 1498 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1499 switch (hash) { 1500 case 3575610: 1501 /* type */ return new String[] { "CodeableConcept" }; 1502 case 102865796: 1503 /* level */ return new String[] { "decimal" }; 1504 case 3151786: 1505 /* from */ return new String[] { "decimal" }; 1506 case 3707: 1507 /* to */ return new String[] { "decimal" }; 1508 default: 1509 return super.getTypesForProperty(hash, name); 1510 } 1511 1512 } 1513 1514 @Override 1515 public Base addChild(String name) throws FHIRException { 1516 if (name.equals("type")) { 1517 this.type = new CodeableConcept(); 1518 return this.type; 1519 } else if (name.equals("level")) { 1520 throw new FHIRException("Cannot call addChild on a singleton property RiskEvidenceSynthesis.level"); 1521 } else if (name.equals("from")) { 1522 throw new FHIRException("Cannot call addChild on a singleton property RiskEvidenceSynthesis.from"); 1523 } else if (name.equals("to")) { 1524 throw new FHIRException("Cannot call addChild on a singleton property RiskEvidenceSynthesis.to"); 1525 } else 1526 return super.addChild(name); 1527 } 1528 1529 public RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent copy() { 1530 RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent dst = new RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent(); 1531 copyValues(dst); 1532 return dst; 1533 } 1534 1535 public void copyValues(RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent dst) { 1536 super.copyValues(dst); 1537 dst.type = type == null ? null : type.copy(); 1538 dst.level = level == null ? null : level.copy(); 1539 dst.from = from == null ? null : from.copy(); 1540 dst.to = to == null ? null : to.copy(); 1541 } 1542 1543 @Override 1544 public boolean equalsDeep(Base other_) { 1545 if (!super.equalsDeep(other_)) 1546 return false; 1547 if (!(other_ instanceof RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent)) 1548 return false; 1549 RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent o = (RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent) other_; 1550 return compareDeep(type, o.type, true) && compareDeep(level, o.level, true) && compareDeep(from, o.from, true) 1551 && compareDeep(to, o.to, true); 1552 } 1553 1554 @Override 1555 public boolean equalsShallow(Base other_) { 1556 if (!super.equalsShallow(other_)) 1557 return false; 1558 if (!(other_ instanceof RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent)) 1559 return false; 1560 RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent o = (RiskEvidenceSynthesisRiskEstimatePrecisionEstimateComponent) other_; 1561 return compareValues(level, o.level, true) && compareValues(from, o.from, true) && compareValues(to, o.to, true); 1562 } 1563 1564 public boolean isEmpty() { 1565 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, level, from, to); 1566 } 1567 1568 public String fhirType() { 1569 return "RiskEvidenceSynthesis.riskEstimate.precisionEstimate"; 1570 1571 } 1572 1573 } 1574 1575 @Block() 1576 public static class RiskEvidenceSynthesisCertaintyComponent extends BackboneElement implements IBaseBackboneElement { 1577 /** 1578 * A rating of the certainty of the effect estimate. 1579 */ 1580 @Child(name = "rating", type = { 1581 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1582 @Description(shortDefinition = "Certainty rating", formalDefinition = "A rating of the certainty of the effect estimate.") 1583 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/evidence-quality") 1584 protected List<CodeableConcept> rating; 1585 1586 /** 1587 * A human-readable string to clarify or explain concepts about the resource. 1588 */ 1589 @Child(name = "note", type = { 1590 Annotation.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1591 @Description(shortDefinition = "Used for footnotes or explanatory notes", formalDefinition = "A human-readable string to clarify or explain concepts about the resource.") 1592 protected List<Annotation> note; 1593 1594 /** 1595 * A description of a component of the overall certainty. 1596 */ 1597 @Child(name = "certaintySubcomponent", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1598 @Description(shortDefinition = "A component that contributes to the overall certainty", formalDefinition = "A description of a component of the overall certainty.") 1599 protected List<RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent> certaintySubcomponent; 1600 1601 private static final long serialVersionUID = 663360871L; 1602 1603 /** 1604 * Constructor 1605 */ 1606 public RiskEvidenceSynthesisCertaintyComponent() { 1607 super(); 1608 } 1609 1610 /** 1611 * @return {@link #rating} (A rating of the certainty of the effect estimate.) 1612 */ 1613 public List<CodeableConcept> getRating() { 1614 if (this.rating == null) 1615 this.rating = new ArrayList<CodeableConcept>(); 1616 return this.rating; 1617 } 1618 1619 /** 1620 * @return Returns a reference to <code>this</code> for easy method chaining 1621 */ 1622 public RiskEvidenceSynthesisCertaintyComponent setRating(List<CodeableConcept> theRating) { 1623 this.rating = theRating; 1624 return this; 1625 } 1626 1627 public boolean hasRating() { 1628 if (this.rating == null) 1629 return false; 1630 for (CodeableConcept item : this.rating) 1631 if (!item.isEmpty()) 1632 return true; 1633 return false; 1634 } 1635 1636 public CodeableConcept addRating() { // 3 1637 CodeableConcept t = new CodeableConcept(); 1638 if (this.rating == null) 1639 this.rating = new ArrayList<CodeableConcept>(); 1640 this.rating.add(t); 1641 return t; 1642 } 1643 1644 public RiskEvidenceSynthesisCertaintyComponent addRating(CodeableConcept t) { // 3 1645 if (t == null) 1646 return this; 1647 if (this.rating == null) 1648 this.rating = new ArrayList<CodeableConcept>(); 1649 this.rating.add(t); 1650 return this; 1651 } 1652 1653 /** 1654 * @return The first repetition of repeating field {@link #rating}, creating it 1655 * if it does not already exist 1656 */ 1657 public CodeableConcept getRatingFirstRep() { 1658 if (getRating().isEmpty()) { 1659 addRating(); 1660 } 1661 return getRating().get(0); 1662 } 1663 1664 /** 1665 * @return {@link #note} (A human-readable string to clarify or explain concepts 1666 * about the resource.) 1667 */ 1668 public List<Annotation> getNote() { 1669 if (this.note == null) 1670 this.note = new ArrayList<Annotation>(); 1671 return this.note; 1672 } 1673 1674 /** 1675 * @return Returns a reference to <code>this</code> for easy method chaining 1676 */ 1677 public RiskEvidenceSynthesisCertaintyComponent setNote(List<Annotation> theNote) { 1678 this.note = theNote; 1679 return this; 1680 } 1681 1682 public boolean hasNote() { 1683 if (this.note == null) 1684 return false; 1685 for (Annotation item : this.note) 1686 if (!item.isEmpty()) 1687 return true; 1688 return false; 1689 } 1690 1691 public Annotation addNote() { // 3 1692 Annotation t = new Annotation(); 1693 if (this.note == null) 1694 this.note = new ArrayList<Annotation>(); 1695 this.note.add(t); 1696 return t; 1697 } 1698 1699 public RiskEvidenceSynthesisCertaintyComponent addNote(Annotation t) { // 3 1700 if (t == null) 1701 return this; 1702 if (this.note == null) 1703 this.note = new ArrayList<Annotation>(); 1704 this.note.add(t); 1705 return this; 1706 } 1707 1708 /** 1709 * @return The first repetition of repeating field {@link #note}, creating it if 1710 * it does not already exist 1711 */ 1712 public Annotation getNoteFirstRep() { 1713 if (getNote().isEmpty()) { 1714 addNote(); 1715 } 1716 return getNote().get(0); 1717 } 1718 1719 /** 1720 * @return {@link #certaintySubcomponent} (A description of a component of the 1721 * overall certainty.) 1722 */ 1723 public List<RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent> getCertaintySubcomponent() { 1724 if (this.certaintySubcomponent == null) 1725 this.certaintySubcomponent = new ArrayList<RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent>(); 1726 return this.certaintySubcomponent; 1727 } 1728 1729 /** 1730 * @return Returns a reference to <code>this</code> for easy method chaining 1731 */ 1732 public RiskEvidenceSynthesisCertaintyComponent setCertaintySubcomponent( 1733 List<RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent> theCertaintySubcomponent) { 1734 this.certaintySubcomponent = theCertaintySubcomponent; 1735 return this; 1736 } 1737 1738 public boolean hasCertaintySubcomponent() { 1739 if (this.certaintySubcomponent == null) 1740 return false; 1741 for (RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent item : this.certaintySubcomponent) 1742 if (!item.isEmpty()) 1743 return true; 1744 return false; 1745 } 1746 1747 public RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent addCertaintySubcomponent() { // 3 1748 RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent t = new RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent(); 1749 if (this.certaintySubcomponent == null) 1750 this.certaintySubcomponent = new ArrayList<RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent>(); 1751 this.certaintySubcomponent.add(t); 1752 return t; 1753 } 1754 1755 public RiskEvidenceSynthesisCertaintyComponent addCertaintySubcomponent( 1756 RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent t) { // 3 1757 if (t == null) 1758 return this; 1759 if (this.certaintySubcomponent == null) 1760 this.certaintySubcomponent = new ArrayList<RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent>(); 1761 this.certaintySubcomponent.add(t); 1762 return this; 1763 } 1764 1765 /** 1766 * @return The first repetition of repeating field 1767 * {@link #certaintySubcomponent}, creating it if it does not already 1768 * exist 1769 */ 1770 public RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent getCertaintySubcomponentFirstRep() { 1771 if (getCertaintySubcomponent().isEmpty()) { 1772 addCertaintySubcomponent(); 1773 } 1774 return getCertaintySubcomponent().get(0); 1775 } 1776 1777 protected void listChildren(List<Property> children) { 1778 super.listChildren(children); 1779 children.add(new Property("rating", "CodeableConcept", "A rating of the certainty of the effect estimate.", 0, 1780 java.lang.Integer.MAX_VALUE, rating)); 1781 children.add(new Property("note", "Annotation", 1782 "A human-readable string to clarify or explain concepts about the resource.", 0, java.lang.Integer.MAX_VALUE, 1783 note)); 1784 children.add(new Property("certaintySubcomponent", "", "A description of a component of the overall certainty.", 1785 0, java.lang.Integer.MAX_VALUE, certaintySubcomponent)); 1786 } 1787 1788 @Override 1789 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1790 switch (_hash) { 1791 case -938102371: 1792 /* rating */ return new Property("rating", "CodeableConcept", 1793 "A rating of the certainty of the effect estimate.", 0, java.lang.Integer.MAX_VALUE, rating); 1794 case 3387378: 1795 /* note */ return new Property("note", "Annotation", 1796 "A human-readable string to clarify or explain concepts about the resource.", 0, 1797 java.lang.Integer.MAX_VALUE, note); 1798 case 1806398212: 1799 /* certaintySubcomponent */ return new Property("certaintySubcomponent", "", 1800 "A description of a component of the overall certainty.", 0, java.lang.Integer.MAX_VALUE, 1801 certaintySubcomponent); 1802 default: 1803 return super.getNamedProperty(_hash, _name, _checkValid); 1804 } 1805 1806 } 1807 1808 @Override 1809 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1810 switch (hash) { 1811 case -938102371: 1812 /* rating */ return this.rating == null ? new Base[0] : this.rating.toArray(new Base[this.rating.size()]); // CodeableConcept 1813 case 3387378: 1814 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1815 case 1806398212: 1816 /* certaintySubcomponent */ return this.certaintySubcomponent == null ? new Base[0] 1817 : this.certaintySubcomponent.toArray(new Base[this.certaintySubcomponent.size()]); // RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent 1818 default: 1819 return super.getProperty(hash, name, checkValid); 1820 } 1821 1822 } 1823 1824 @Override 1825 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1826 switch (hash) { 1827 case -938102371: // rating 1828 this.getRating().add(castToCodeableConcept(value)); // CodeableConcept 1829 return value; 1830 case 3387378: // note 1831 this.getNote().add(castToAnnotation(value)); // Annotation 1832 return value; 1833 case 1806398212: // certaintySubcomponent 1834 this.getCertaintySubcomponent().add((RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent) value); // RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent 1835 return value; 1836 default: 1837 return super.setProperty(hash, name, value); 1838 } 1839 1840 } 1841 1842 @Override 1843 public Base setProperty(String name, Base value) throws FHIRException { 1844 if (name.equals("rating")) { 1845 this.getRating().add(castToCodeableConcept(value)); 1846 } else if (name.equals("note")) { 1847 this.getNote().add(castToAnnotation(value)); 1848 } else if (name.equals("certaintySubcomponent")) { 1849 this.getCertaintySubcomponent().add((RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent) value); 1850 } else 1851 return super.setProperty(name, value); 1852 return value; 1853 } 1854 1855 @Override 1856 public void removeChild(String name, Base value) throws FHIRException { 1857 if (name.equals("rating")) { 1858 this.getRating().remove(castToCodeableConcept(value)); 1859 } else if (name.equals("note")) { 1860 this.getNote().remove(castToAnnotation(value)); 1861 } else if (name.equals("certaintySubcomponent")) { 1862 this.getCertaintySubcomponent().remove((RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent) value); 1863 } else 1864 super.removeChild(name, value); 1865 1866 } 1867 1868 @Override 1869 public Base makeProperty(int hash, String name) throws FHIRException { 1870 switch (hash) { 1871 case -938102371: 1872 return addRating(); 1873 case 3387378: 1874 return addNote(); 1875 case 1806398212: 1876 return addCertaintySubcomponent(); 1877 default: 1878 return super.makeProperty(hash, name); 1879 } 1880 1881 } 1882 1883 @Override 1884 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1885 switch (hash) { 1886 case -938102371: 1887 /* rating */ return new String[] { "CodeableConcept" }; 1888 case 3387378: 1889 /* note */ return new String[] { "Annotation" }; 1890 case 1806398212: 1891 /* certaintySubcomponent */ return new String[] {}; 1892 default: 1893 return super.getTypesForProperty(hash, name); 1894 } 1895 1896 } 1897 1898 @Override 1899 public Base addChild(String name) throws FHIRException { 1900 if (name.equals("rating")) { 1901 return addRating(); 1902 } else if (name.equals("note")) { 1903 return addNote(); 1904 } else if (name.equals("certaintySubcomponent")) { 1905 return addCertaintySubcomponent(); 1906 } else 1907 return super.addChild(name); 1908 } 1909 1910 public RiskEvidenceSynthesisCertaintyComponent copy() { 1911 RiskEvidenceSynthesisCertaintyComponent dst = new RiskEvidenceSynthesisCertaintyComponent(); 1912 copyValues(dst); 1913 return dst; 1914 } 1915 1916 public void copyValues(RiskEvidenceSynthesisCertaintyComponent dst) { 1917 super.copyValues(dst); 1918 if (rating != null) { 1919 dst.rating = new ArrayList<CodeableConcept>(); 1920 for (CodeableConcept i : rating) 1921 dst.rating.add(i.copy()); 1922 } 1923 ; 1924 if (note != null) { 1925 dst.note = new ArrayList<Annotation>(); 1926 for (Annotation i : note) 1927 dst.note.add(i.copy()); 1928 } 1929 ; 1930 if (certaintySubcomponent != null) { 1931 dst.certaintySubcomponent = new ArrayList<RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent>(); 1932 for (RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent i : certaintySubcomponent) 1933 dst.certaintySubcomponent.add(i.copy()); 1934 } 1935 ; 1936 } 1937 1938 @Override 1939 public boolean equalsDeep(Base other_) { 1940 if (!super.equalsDeep(other_)) 1941 return false; 1942 if (!(other_ instanceof RiskEvidenceSynthesisCertaintyComponent)) 1943 return false; 1944 RiskEvidenceSynthesisCertaintyComponent o = (RiskEvidenceSynthesisCertaintyComponent) other_; 1945 return compareDeep(rating, o.rating, true) && compareDeep(note, o.note, true) 1946 && compareDeep(certaintySubcomponent, o.certaintySubcomponent, true); 1947 } 1948 1949 @Override 1950 public boolean equalsShallow(Base other_) { 1951 if (!super.equalsShallow(other_)) 1952 return false; 1953 if (!(other_ instanceof RiskEvidenceSynthesisCertaintyComponent)) 1954 return false; 1955 RiskEvidenceSynthesisCertaintyComponent o = (RiskEvidenceSynthesisCertaintyComponent) other_; 1956 return true; 1957 } 1958 1959 public boolean isEmpty() { 1960 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(rating, note, certaintySubcomponent); 1961 } 1962 1963 public String fhirType() { 1964 return "RiskEvidenceSynthesis.certainty"; 1965 1966 } 1967 1968 } 1969 1970 @Block() 1971 public static class RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent extends BackboneElement 1972 implements IBaseBackboneElement { 1973 /** 1974 * Type of subcomponent of certainty rating. 1975 */ 1976 @Child(name = "type", type = { 1977 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1978 @Description(shortDefinition = "Type of subcomponent of certainty rating", formalDefinition = "Type of subcomponent of certainty rating.") 1979 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/certainty-subcomponent-type") 1980 protected CodeableConcept type; 1981 1982 /** 1983 * A rating of a subcomponent of rating certainty. 1984 */ 1985 @Child(name = "rating", type = { 1986 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1987 @Description(shortDefinition = "Subcomponent certainty rating", formalDefinition = "A rating of a subcomponent of rating certainty.") 1988 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/certainty-subcomponent-rating") 1989 protected List<CodeableConcept> rating; 1990 1991 /** 1992 * A human-readable string to clarify or explain concepts about the resource. 1993 */ 1994 @Child(name = "note", type = { 1995 Annotation.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1996 @Description(shortDefinition = "Used for footnotes or explanatory notes", formalDefinition = "A human-readable string to clarify or explain concepts about the resource.") 1997 protected List<Annotation> note; 1998 1999 private static final long serialVersionUID = -411994816L; 2000 2001 /** 2002 * Constructor 2003 */ 2004 public RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent() { 2005 super(); 2006 } 2007 2008 /** 2009 * @return {@link #type} (Type of subcomponent of certainty rating.) 2010 */ 2011 public CodeableConcept getType() { 2012 if (this.type == null) 2013 if (Configuration.errorOnAutoCreate()) 2014 throw new Error("Attempt to auto-create RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent.type"); 2015 else if (Configuration.doAutoCreate()) 2016 this.type = new CodeableConcept(); // cc 2017 return this.type; 2018 } 2019 2020 public boolean hasType() { 2021 return this.type != null && !this.type.isEmpty(); 2022 } 2023 2024 /** 2025 * @param value {@link #type} (Type of subcomponent of certainty rating.) 2026 */ 2027 public RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent setType(CodeableConcept value) { 2028 this.type = value; 2029 return this; 2030 } 2031 2032 /** 2033 * @return {@link #rating} (A rating of a subcomponent of rating certainty.) 2034 */ 2035 public List<CodeableConcept> getRating() { 2036 if (this.rating == null) 2037 this.rating = new ArrayList<CodeableConcept>(); 2038 return this.rating; 2039 } 2040 2041 /** 2042 * @return Returns a reference to <code>this</code> for easy method chaining 2043 */ 2044 public RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent setRating(List<CodeableConcept> theRating) { 2045 this.rating = theRating; 2046 return this; 2047 } 2048 2049 public boolean hasRating() { 2050 if (this.rating == null) 2051 return false; 2052 for (CodeableConcept item : this.rating) 2053 if (!item.isEmpty()) 2054 return true; 2055 return false; 2056 } 2057 2058 public CodeableConcept addRating() { // 3 2059 CodeableConcept t = new CodeableConcept(); 2060 if (this.rating == null) 2061 this.rating = new ArrayList<CodeableConcept>(); 2062 this.rating.add(t); 2063 return t; 2064 } 2065 2066 public RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent addRating(CodeableConcept t) { // 3 2067 if (t == null) 2068 return this; 2069 if (this.rating == null) 2070 this.rating = new ArrayList<CodeableConcept>(); 2071 this.rating.add(t); 2072 return this; 2073 } 2074 2075 /** 2076 * @return The first repetition of repeating field {@link #rating}, creating it 2077 * if it does not already exist 2078 */ 2079 public CodeableConcept getRatingFirstRep() { 2080 if (getRating().isEmpty()) { 2081 addRating(); 2082 } 2083 return getRating().get(0); 2084 } 2085 2086 /** 2087 * @return {@link #note} (A human-readable string to clarify or explain concepts 2088 * about the resource.) 2089 */ 2090 public List<Annotation> getNote() { 2091 if (this.note == null) 2092 this.note = new ArrayList<Annotation>(); 2093 return this.note; 2094 } 2095 2096 /** 2097 * @return Returns a reference to <code>this</code> for easy method chaining 2098 */ 2099 public RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent setNote(List<Annotation> theNote) { 2100 this.note = theNote; 2101 return this; 2102 } 2103 2104 public boolean hasNote() { 2105 if (this.note == null) 2106 return false; 2107 for (Annotation item : this.note) 2108 if (!item.isEmpty()) 2109 return true; 2110 return false; 2111 } 2112 2113 public Annotation addNote() { // 3 2114 Annotation t = new Annotation(); 2115 if (this.note == null) 2116 this.note = new ArrayList<Annotation>(); 2117 this.note.add(t); 2118 return t; 2119 } 2120 2121 public RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent addNote(Annotation t) { // 3 2122 if (t == null) 2123 return this; 2124 if (this.note == null) 2125 this.note = new ArrayList<Annotation>(); 2126 this.note.add(t); 2127 return this; 2128 } 2129 2130 /** 2131 * @return The first repetition of repeating field {@link #note}, creating it if 2132 * it does not already exist 2133 */ 2134 public Annotation getNoteFirstRep() { 2135 if (getNote().isEmpty()) { 2136 addNote(); 2137 } 2138 return getNote().get(0); 2139 } 2140 2141 protected void listChildren(List<Property> children) { 2142 super.listChildren(children); 2143 children.add(new Property("type", "CodeableConcept", "Type of subcomponent of certainty rating.", 0, 1, type)); 2144 children.add(new Property("rating", "CodeableConcept", "A rating of a subcomponent of rating certainty.", 0, 2145 java.lang.Integer.MAX_VALUE, rating)); 2146 children.add(new Property("note", "Annotation", 2147 "A human-readable string to clarify or explain concepts about the resource.", 0, java.lang.Integer.MAX_VALUE, 2148 note)); 2149 } 2150 2151 @Override 2152 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2153 switch (_hash) { 2154 case 3575610: 2155 /* type */ return new Property("type", "CodeableConcept", "Type of subcomponent of certainty rating.", 0, 1, 2156 type); 2157 case -938102371: 2158 /* rating */ return new Property("rating", "CodeableConcept", "A rating of a subcomponent of rating certainty.", 2159 0, java.lang.Integer.MAX_VALUE, rating); 2160 case 3387378: 2161 /* note */ return new Property("note", "Annotation", 2162 "A human-readable string to clarify or explain concepts about the resource.", 0, 2163 java.lang.Integer.MAX_VALUE, note); 2164 default: 2165 return super.getNamedProperty(_hash, _name, _checkValid); 2166 } 2167 2168 } 2169 2170 @Override 2171 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2172 switch (hash) { 2173 case 3575610: 2174 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2175 case -938102371: 2176 /* rating */ return this.rating == null ? new Base[0] : this.rating.toArray(new Base[this.rating.size()]); // CodeableConcept 2177 case 3387378: 2178 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2179 default: 2180 return super.getProperty(hash, name, checkValid); 2181 } 2182 2183 } 2184 2185 @Override 2186 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2187 switch (hash) { 2188 case 3575610: // type 2189 this.type = castToCodeableConcept(value); // CodeableConcept 2190 return value; 2191 case -938102371: // rating 2192 this.getRating().add(castToCodeableConcept(value)); // CodeableConcept 2193 return value; 2194 case 3387378: // note 2195 this.getNote().add(castToAnnotation(value)); // Annotation 2196 return value; 2197 default: 2198 return super.setProperty(hash, name, value); 2199 } 2200 2201 } 2202 2203 @Override 2204 public Base setProperty(String name, Base value) throws FHIRException { 2205 if (name.equals("type")) { 2206 this.type = castToCodeableConcept(value); // CodeableConcept 2207 } else if (name.equals("rating")) { 2208 this.getRating().add(castToCodeableConcept(value)); 2209 } else if (name.equals("note")) { 2210 this.getNote().add(castToAnnotation(value)); 2211 } else 2212 return super.setProperty(name, value); 2213 return value; 2214 } 2215 2216 @Override 2217 public void removeChild(String name, Base value) throws FHIRException { 2218 if (name.equals("type")) { 2219 this.type = null; 2220 } else if (name.equals("rating")) { 2221 this.getRating().remove(castToCodeableConcept(value)); 2222 } else if (name.equals("note")) { 2223 this.getNote().remove(castToAnnotation(value)); 2224 } else 2225 super.removeChild(name, value); 2226 2227 } 2228 2229 @Override 2230 public Base makeProperty(int hash, String name) throws FHIRException { 2231 switch (hash) { 2232 case 3575610: 2233 return getType(); 2234 case -938102371: 2235 return addRating(); 2236 case 3387378: 2237 return addNote(); 2238 default: 2239 return super.makeProperty(hash, name); 2240 } 2241 2242 } 2243 2244 @Override 2245 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2246 switch (hash) { 2247 case 3575610: 2248 /* type */ return new String[] { "CodeableConcept" }; 2249 case -938102371: 2250 /* rating */ return new String[] { "CodeableConcept" }; 2251 case 3387378: 2252 /* note */ return new String[] { "Annotation" }; 2253 default: 2254 return super.getTypesForProperty(hash, name); 2255 } 2256 2257 } 2258 2259 @Override 2260 public Base addChild(String name) throws FHIRException { 2261 if (name.equals("type")) { 2262 this.type = new CodeableConcept(); 2263 return this.type; 2264 } else if (name.equals("rating")) { 2265 return addRating(); 2266 } else if (name.equals("note")) { 2267 return addNote(); 2268 } else 2269 return super.addChild(name); 2270 } 2271 2272 public RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent copy() { 2273 RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent dst = new RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent(); 2274 copyValues(dst); 2275 return dst; 2276 } 2277 2278 public void copyValues(RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent dst) { 2279 super.copyValues(dst); 2280 dst.type = type == null ? null : type.copy(); 2281 if (rating != null) { 2282 dst.rating = new ArrayList<CodeableConcept>(); 2283 for (CodeableConcept i : rating) 2284 dst.rating.add(i.copy()); 2285 } 2286 ; 2287 if (note != null) { 2288 dst.note = new ArrayList<Annotation>(); 2289 for (Annotation i : note) 2290 dst.note.add(i.copy()); 2291 } 2292 ; 2293 } 2294 2295 @Override 2296 public boolean equalsDeep(Base other_) { 2297 if (!super.equalsDeep(other_)) 2298 return false; 2299 if (!(other_ instanceof RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent)) 2300 return false; 2301 RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent o = (RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent) other_; 2302 return compareDeep(type, o.type, true) && compareDeep(rating, o.rating, true) && compareDeep(note, o.note, true); 2303 } 2304 2305 @Override 2306 public boolean equalsShallow(Base other_) { 2307 if (!super.equalsShallow(other_)) 2308 return false; 2309 if (!(other_ instanceof RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent)) 2310 return false; 2311 RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent o = (RiskEvidenceSynthesisCertaintyCertaintySubcomponentComponent) other_; 2312 return true; 2313 } 2314 2315 public boolean isEmpty() { 2316 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, rating, note); 2317 } 2318 2319 public String fhirType() { 2320 return "RiskEvidenceSynthesis.certainty.certaintySubcomponent"; 2321 2322 } 2323 2324 } 2325 2326 /** 2327 * A formal identifier that is used to identify this risk evidence synthesis 2328 * when it is represented in other formats, or referenced in a specification, 2329 * model, design or an instance. 2330 */ 2331 @Child(name = "identifier", type = { 2332 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2333 @Description(shortDefinition = "Additional identifier for the risk evidence synthesis", formalDefinition = "A formal identifier that is used to identify this risk evidence synthesis when it is represented in other formats, or referenced in a specification, model, design or an instance.") 2334 protected List<Identifier> identifier; 2335 2336 /** 2337 * A human-readable string to clarify or explain concepts about the resource. 2338 */ 2339 @Child(name = "note", type = { 2340 Annotation.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2341 @Description(shortDefinition = "Used for footnotes or explanatory notes", formalDefinition = "A human-readable string to clarify or explain concepts about the resource.") 2342 protected List<Annotation> note; 2343 2344 /** 2345 * A copyright statement relating to the risk evidence synthesis and/or its 2346 * contents. Copyright statements are generally legal restrictions on the use 2347 * and publishing of the risk evidence synthesis. 2348 */ 2349 @Child(name = "copyright", type = { 2350 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2351 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the risk evidence synthesis and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the risk evidence synthesis.") 2352 protected MarkdownType copyright; 2353 2354 /** 2355 * The date on which the resource content was approved by the publisher. 2356 * Approval happens once when the content is officially approved for usage. 2357 */ 2358 @Child(name = "approvalDate", type = { 2359 DateType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2360 @Description(shortDefinition = "When the risk evidence synthesis was approved by publisher", formalDefinition = "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.") 2361 protected DateType approvalDate; 2362 2363 /** 2364 * The date on which the resource content was last reviewed. Review happens 2365 * periodically after approval but does not change the original approval date. 2366 */ 2367 @Child(name = "lastReviewDate", type = { 2368 DateType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2369 @Description(shortDefinition = "When the risk evidence synthesis was last reviewed", formalDefinition = "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.") 2370 protected DateType lastReviewDate; 2371 2372 /** 2373 * The period during which the risk evidence synthesis content was or is planned 2374 * to be in active use. 2375 */ 2376 @Child(name = "effectivePeriod", type = { 2377 Period.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 2378 @Description(shortDefinition = "When the risk evidence synthesis is expected to be used", formalDefinition = "The period during which the risk evidence synthesis content was or is planned to be in active use.") 2379 protected Period effectivePeriod; 2380 2381 /** 2382 * Descriptive topics related to the content of the RiskEvidenceSynthesis. 2383 * Topics provide a high-level categorization grouping types of 2384 * EffectEvidenceSynthesiss that can be useful for filtering and searching. 2385 */ 2386 @Child(name = "topic", type = { 2387 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2388 @Description(shortDefinition = "The category of the EffectEvidenceSynthesis, such as Education, Treatment, Assessment, etc.", formalDefinition = "Descriptive topics related to the content of the RiskEvidenceSynthesis. Topics provide a high-level categorization grouping types of EffectEvidenceSynthesiss that can be useful for filtering and searching.") 2389 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/definition-topic") 2390 protected List<CodeableConcept> topic; 2391 2392 /** 2393 * An individiual or organization primarily involved in the creation and 2394 * maintenance of the content. 2395 */ 2396 @Child(name = "author", type = { 2397 ContactDetail.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2398 @Description(shortDefinition = "Who authored the content", formalDefinition = "An individiual or organization primarily involved in the creation and maintenance of the content.") 2399 protected List<ContactDetail> author; 2400 2401 /** 2402 * An individual or organization primarily responsible for internal coherence of 2403 * the content. 2404 */ 2405 @Child(name = "editor", type = { 2406 ContactDetail.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2407 @Description(shortDefinition = "Who edited the content", formalDefinition = "An individual or organization primarily responsible for internal coherence of the content.") 2408 protected List<ContactDetail> editor; 2409 2410 /** 2411 * An individual or organization primarily responsible for review of some aspect 2412 * of the content. 2413 */ 2414 @Child(name = "reviewer", type = { 2415 ContactDetail.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2416 @Description(shortDefinition = "Who reviewed the content", formalDefinition = "An individual or organization primarily responsible for review of some aspect of the content.") 2417 protected List<ContactDetail> reviewer; 2418 2419 /** 2420 * An individual or organization responsible for officially endorsing the 2421 * content for use in some setting. 2422 */ 2423 @Child(name = "endorser", type = { 2424 ContactDetail.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2425 @Description(shortDefinition = "Who endorsed the content", formalDefinition = "An individual or organization responsible for officially endorsing the content for use in some setting.") 2426 protected List<ContactDetail> endorser; 2427 2428 /** 2429 * Related artifacts such as additional documentation, justification, or 2430 * bibliographic references. 2431 */ 2432 @Child(name = "relatedArtifact", type = { 2433 RelatedArtifact.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2434 @Description(shortDefinition = "Additional documentation, citations, etc.", formalDefinition = "Related artifacts such as additional documentation, justification, or bibliographic references.") 2435 protected List<RelatedArtifact> relatedArtifact; 2436 2437 /** 2438 * Type of synthesis eg meta-analysis. 2439 */ 2440 @Child(name = "synthesisType", type = { 2441 CodeableConcept.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 2442 @Description(shortDefinition = "Type of synthesis", formalDefinition = "Type of synthesis eg meta-analysis.") 2443 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/synthesis-type") 2444 protected CodeableConcept synthesisType; 2445 2446 /** 2447 * Type of study eg randomized trial. 2448 */ 2449 @Child(name = "studyType", type = { 2450 CodeableConcept.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 2451 @Description(shortDefinition = "Type of study", formalDefinition = "Type of study eg randomized trial.") 2452 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/study-type") 2453 protected CodeableConcept studyType; 2454 2455 /** 2456 * A reference to a EvidenceVariable resource that defines the population for 2457 * the research. 2458 */ 2459 @Child(name = "population", type = { 2460 EvidenceVariable.class }, order = 14, min = 1, max = 1, modifier = false, summary = true) 2461 @Description(shortDefinition = "What population?", formalDefinition = "A reference to a EvidenceVariable resource that defines the population for the research.") 2462 protected Reference population; 2463 2464 /** 2465 * The actual object that is the target of the reference (A reference to a 2466 * EvidenceVariable resource that defines the population for the research.) 2467 */ 2468 protected EvidenceVariable populationTarget; 2469 2470 /** 2471 * A reference to a EvidenceVariable resource that defines the exposure for the 2472 * research. 2473 */ 2474 @Child(name = "exposure", type = { 2475 EvidenceVariable.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 2476 @Description(shortDefinition = "What exposure?", formalDefinition = "A reference to a EvidenceVariable resource that defines the exposure for the research.") 2477 protected Reference exposure; 2478 2479 /** 2480 * The actual object that is the target of the reference (A reference to a 2481 * EvidenceVariable resource that defines the exposure for the research.) 2482 */ 2483 protected EvidenceVariable exposureTarget; 2484 2485 /** 2486 * A reference to a EvidenceVariable resomece that defines the outcome for the 2487 * research. 2488 */ 2489 @Child(name = "outcome", type = { 2490 EvidenceVariable.class }, order = 16, min = 1, max = 1, modifier = false, summary = true) 2491 @Description(shortDefinition = "What outcome?", formalDefinition = "A reference to a EvidenceVariable resomece that defines the outcome for the research.") 2492 protected Reference outcome; 2493 2494 /** 2495 * The actual object that is the target of the reference (A reference to a 2496 * EvidenceVariable resomece that defines the outcome for the research.) 2497 */ 2498 protected EvidenceVariable outcomeTarget; 2499 2500 /** 2501 * A description of the size of the sample involved in the synthesis. 2502 */ 2503 @Child(name = "sampleSize", type = {}, order = 17, min = 0, max = 1, modifier = false, summary = false) 2504 @Description(shortDefinition = "What sample size was involved?", formalDefinition = "A description of the size of the sample involved in the synthesis.") 2505 protected RiskEvidenceSynthesisSampleSizeComponent sampleSize; 2506 2507 /** 2508 * The estimated risk of the outcome. 2509 */ 2510 @Child(name = "riskEstimate", type = {}, order = 18, min = 0, max = 1, modifier = false, summary = true) 2511 @Description(shortDefinition = "What was the estimated risk", formalDefinition = "The estimated risk of the outcome.") 2512 protected RiskEvidenceSynthesisRiskEstimateComponent riskEstimate; 2513 2514 /** 2515 * A description of the certainty of the risk estimate. 2516 */ 2517 @Child(name = "certainty", type = {}, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2518 @Description(shortDefinition = "How certain is the risk", formalDefinition = "A description of the certainty of the risk estimate.") 2519 protected List<RiskEvidenceSynthesisCertaintyComponent> certainty; 2520 2521 private static final long serialVersionUID = 706492815L; 2522 2523 /** 2524 * Constructor 2525 */ 2526 public RiskEvidenceSynthesis() { 2527 super(); 2528 } 2529 2530 /** 2531 * Constructor 2532 */ 2533 public RiskEvidenceSynthesis(Enumeration<PublicationStatus> status, Reference population, Reference outcome) { 2534 super(); 2535 this.status = status; 2536 this.population = population; 2537 this.outcome = outcome; 2538 } 2539 2540 /** 2541 * @return {@link #url} (An absolute URI that is used to identify this risk 2542 * evidence synthesis when it is referenced in a specification, model, 2543 * design or an instance; also called its canonical identifier. This 2544 * SHOULD be globally unique and SHOULD be a literal address at which at 2545 * which an authoritative instance of this risk evidence synthesis is 2546 * (or will be) published. This URL can be the target of a canonical 2547 * reference. It SHALL remain the same when the risk evidence synthesis 2548 * is stored on different servers.). This is the underlying object with 2549 * id, value and extensions. The accessor "getUrl" gives direct access 2550 * to the value 2551 */ 2552 public UriType getUrlElement() { 2553 if (this.url == null) 2554 if (Configuration.errorOnAutoCreate()) 2555 throw new Error("Attempt to auto-create RiskEvidenceSynthesis.url"); 2556 else if (Configuration.doAutoCreate()) 2557 this.url = new UriType(); // bb 2558 return this.url; 2559 } 2560 2561 public boolean hasUrlElement() { 2562 return this.url != null && !this.url.isEmpty(); 2563 } 2564 2565 public boolean hasUrl() { 2566 return this.url != null && !this.url.isEmpty(); 2567 } 2568 2569 /** 2570 * @param value {@link #url} (An absolute URI that is used to identify this risk 2571 * evidence synthesis when it is referenced in a specification, 2572 * model, design or an instance; also called its canonical 2573 * identifier. This SHOULD be globally unique and SHOULD be a 2574 * literal address at which at which an authoritative instance of 2575 * this risk evidence synthesis is (or will be) published. This URL 2576 * can be the target of a canonical reference. It SHALL remain the 2577 * same when the risk evidence synthesis is stored on different 2578 * servers.). This is the underlying object with id, value and 2579 * extensions. The accessor "getUrl" gives direct access to the 2580 * value 2581 */ 2582 public RiskEvidenceSynthesis setUrlElement(UriType value) { 2583 this.url = value; 2584 return this; 2585 } 2586 2587 /** 2588 * @return An absolute URI that is used to identify this risk evidence synthesis 2589 * when it is referenced in a specification, model, design or an 2590 * instance; also called its canonical identifier. This SHOULD be 2591 * globally unique and SHOULD be a literal address at which at which an 2592 * authoritative instance of this risk evidence synthesis is (or will 2593 * be) published. This URL can be the target of a canonical reference. 2594 * It SHALL remain the same when the risk evidence synthesis is stored 2595 * on different servers. 2596 */ 2597 public String getUrl() { 2598 return this.url == null ? null : this.url.getValue(); 2599 } 2600 2601 /** 2602 * @param value An absolute URI that is used to identify this risk evidence 2603 * synthesis when it is referenced in a specification, model, 2604 * design or an instance; also called its canonical identifier. 2605 * This SHOULD be globally unique and SHOULD be a literal address 2606 * at which at which an authoritative instance of this risk 2607 * evidence synthesis is (or will be) published. This URL can be 2608 * the target of a canonical reference. It SHALL remain the same 2609 * when the risk evidence synthesis is stored on different servers. 2610 */ 2611 public RiskEvidenceSynthesis setUrl(String value) { 2612 if (Utilities.noString(value)) 2613 this.url = null; 2614 else { 2615 if (this.url == null) 2616 this.url = new UriType(); 2617 this.url.setValue(value); 2618 } 2619 return this; 2620 } 2621 2622 /** 2623 * @return {@link #identifier} (A formal identifier that is used to identify 2624 * this risk evidence synthesis when it is represented in other formats, 2625 * or referenced in a specification, model, design or an instance.) 2626 */ 2627 public List<Identifier> getIdentifier() { 2628 if (this.identifier == null) 2629 this.identifier = new ArrayList<Identifier>(); 2630 return this.identifier; 2631 } 2632 2633 /** 2634 * @return Returns a reference to <code>this</code> for easy method chaining 2635 */ 2636 public RiskEvidenceSynthesis setIdentifier(List<Identifier> theIdentifier) { 2637 this.identifier = theIdentifier; 2638 return this; 2639 } 2640 2641 public boolean hasIdentifier() { 2642 if (this.identifier == null) 2643 return false; 2644 for (Identifier item : this.identifier) 2645 if (!item.isEmpty()) 2646 return true; 2647 return false; 2648 } 2649 2650 public Identifier addIdentifier() { // 3 2651 Identifier t = new Identifier(); 2652 if (this.identifier == null) 2653 this.identifier = new ArrayList<Identifier>(); 2654 this.identifier.add(t); 2655 return t; 2656 } 2657 2658 public RiskEvidenceSynthesis addIdentifier(Identifier t) { // 3 2659 if (t == null) 2660 return this; 2661 if (this.identifier == null) 2662 this.identifier = new ArrayList<Identifier>(); 2663 this.identifier.add(t); 2664 return this; 2665 } 2666 2667 /** 2668 * @return The first repetition of repeating field {@link #identifier}, creating 2669 * it if it does not already exist 2670 */ 2671 public Identifier getIdentifierFirstRep() { 2672 if (getIdentifier().isEmpty()) { 2673 addIdentifier(); 2674 } 2675 return getIdentifier().get(0); 2676 } 2677 2678 /** 2679 * @return {@link #version} (The identifier that is used to identify this 2680 * version of the risk evidence synthesis when it is referenced in a 2681 * specification, model, design or instance. This is an arbitrary value 2682 * managed by the risk evidence synthesis author and is not expected to 2683 * be globally unique. For example, it might be a timestamp (e.g. 2684 * yyyymmdd) if a managed version is not available. There is also no 2685 * expectation that versions can be placed in a lexicographical 2686 * sequence.). This is the underlying object with id, value and 2687 * extensions. The accessor "getVersion" gives direct access to the 2688 * value 2689 */ 2690 public StringType getVersionElement() { 2691 if (this.version == null) 2692 if (Configuration.errorOnAutoCreate()) 2693 throw new Error("Attempt to auto-create RiskEvidenceSynthesis.version"); 2694 else if (Configuration.doAutoCreate()) 2695 this.version = new StringType(); // bb 2696 return this.version; 2697 } 2698 2699 public boolean hasVersionElement() { 2700 return this.version != null && !this.version.isEmpty(); 2701 } 2702 2703 public boolean hasVersion() { 2704 return this.version != null && !this.version.isEmpty(); 2705 } 2706 2707 /** 2708 * @param value {@link #version} (The identifier that is used to identify this 2709 * version of the risk evidence synthesis when it is referenced in 2710 * a specification, model, design or instance. This is an arbitrary 2711 * value managed by the risk evidence synthesis author and is not 2712 * expected to be globally unique. For example, it might be a 2713 * timestamp (e.g. yyyymmdd) if a managed version is not available. 2714 * There is also no expectation that versions can be placed in a 2715 * lexicographical sequence.). This is the underlying object with 2716 * id, value and extensions. The accessor "getVersion" gives direct 2717 * access to the value 2718 */ 2719 public RiskEvidenceSynthesis setVersionElement(StringType value) { 2720 this.version = value; 2721 return this; 2722 } 2723 2724 /** 2725 * @return The identifier that is used to identify this version of the risk 2726 * evidence synthesis when it is referenced in a specification, model, 2727 * design or instance. This is an arbitrary value managed by the risk 2728 * evidence synthesis author and is not expected to be globally unique. 2729 * For example, it might be a timestamp (e.g. yyyymmdd) if a managed 2730 * version is not available. There is also no expectation that versions 2731 * can be placed in a lexicographical sequence. 2732 */ 2733 public String getVersion() { 2734 return this.version == null ? null : this.version.getValue(); 2735 } 2736 2737 /** 2738 * @param value The identifier that is used to identify this version of the risk 2739 * evidence synthesis when it is referenced in a specification, 2740 * model, design or instance. This is an arbitrary value managed by 2741 * the risk evidence synthesis author and is not expected to be 2742 * globally unique. For example, it might be a timestamp (e.g. 2743 * yyyymmdd) if a managed version is not available. There is also 2744 * no expectation that versions can be placed in a lexicographical 2745 * sequence. 2746 */ 2747 public RiskEvidenceSynthesis setVersion(String value) { 2748 if (Utilities.noString(value)) 2749 this.version = null; 2750 else { 2751 if (this.version == null) 2752 this.version = new StringType(); 2753 this.version.setValue(value); 2754 } 2755 return this; 2756 } 2757 2758 /** 2759 * @return {@link #name} (A natural language name identifying the risk evidence 2760 * synthesis. This name should be usable as an identifier for the module 2761 * by machine processing applications such as code generation.). This is 2762 * the underlying object with id, value and extensions. The accessor 2763 * "getName" gives direct access to the value 2764 */ 2765 public StringType getNameElement() { 2766 if (this.name == null) 2767 if (Configuration.errorOnAutoCreate()) 2768 throw new Error("Attempt to auto-create RiskEvidenceSynthesis.name"); 2769 else if (Configuration.doAutoCreate()) 2770 this.name = new StringType(); // bb 2771 return this.name; 2772 } 2773 2774 public boolean hasNameElement() { 2775 return this.name != null && !this.name.isEmpty(); 2776 } 2777 2778 public boolean hasName() { 2779 return this.name != null && !this.name.isEmpty(); 2780 } 2781 2782 /** 2783 * @param value {@link #name} (A natural language name identifying the risk 2784 * evidence synthesis. This name should be usable as an identifier 2785 * for the module by machine processing applications such as code 2786 * generation.). This is the underlying object with id, value and 2787 * extensions. The accessor "getName" gives direct access to the 2788 * value 2789 */ 2790 public RiskEvidenceSynthesis setNameElement(StringType value) { 2791 this.name = value; 2792 return this; 2793 } 2794 2795 /** 2796 * @return A natural language name identifying the risk evidence synthesis. This 2797 * name should be usable as an identifier for the module by machine 2798 * processing applications such as code generation. 2799 */ 2800 public String getName() { 2801 return this.name == null ? null : this.name.getValue(); 2802 } 2803 2804 /** 2805 * @param value A natural language name identifying the risk evidence synthesis. 2806 * This name should be usable as an identifier for the module by 2807 * machine processing applications such as code generation. 2808 */ 2809 public RiskEvidenceSynthesis setName(String value) { 2810 if (Utilities.noString(value)) 2811 this.name = null; 2812 else { 2813 if (this.name == null) 2814 this.name = new StringType(); 2815 this.name.setValue(value); 2816 } 2817 return this; 2818 } 2819 2820 /** 2821 * @return {@link #title} (A short, descriptive, user-friendly title for the 2822 * risk evidence synthesis.). This is the underlying object with id, 2823 * value and extensions. The accessor "getTitle" gives direct access to 2824 * the value 2825 */ 2826 public StringType getTitleElement() { 2827 if (this.title == null) 2828 if (Configuration.errorOnAutoCreate()) 2829 throw new Error("Attempt to auto-create RiskEvidenceSynthesis.title"); 2830 else if (Configuration.doAutoCreate()) 2831 this.title = new StringType(); // bb 2832 return this.title; 2833 } 2834 2835 public boolean hasTitleElement() { 2836 return this.title != null && !this.title.isEmpty(); 2837 } 2838 2839 public boolean hasTitle() { 2840 return this.title != null && !this.title.isEmpty(); 2841 } 2842 2843 /** 2844 * @param value {@link #title} (A short, descriptive, user-friendly title for 2845 * the risk evidence synthesis.). This is the underlying object 2846 * with id, value and extensions. The accessor "getTitle" gives 2847 * direct access to the value 2848 */ 2849 public RiskEvidenceSynthesis setTitleElement(StringType value) { 2850 this.title = value; 2851 return this; 2852 } 2853 2854 /** 2855 * @return A short, descriptive, user-friendly title for the risk evidence 2856 * synthesis. 2857 */ 2858 public String getTitle() { 2859 return this.title == null ? null : this.title.getValue(); 2860 } 2861 2862 /** 2863 * @param value A short, descriptive, user-friendly title for the risk evidence 2864 * synthesis. 2865 */ 2866 public RiskEvidenceSynthesis setTitle(String value) { 2867 if (Utilities.noString(value)) 2868 this.title = null; 2869 else { 2870 if (this.title == null) 2871 this.title = new StringType(); 2872 this.title.setValue(value); 2873 } 2874 return this; 2875 } 2876 2877 /** 2878 * @return {@link #status} (The status of this risk evidence synthesis. Enables 2879 * tracking the life-cycle of the content.). This is the underlying 2880 * object with id, value and extensions. The accessor "getStatus" gives 2881 * direct access to the value 2882 */ 2883 public Enumeration<PublicationStatus> getStatusElement() { 2884 if (this.status == null) 2885 if (Configuration.errorOnAutoCreate()) 2886 throw new Error("Attempt to auto-create RiskEvidenceSynthesis.status"); 2887 else if (Configuration.doAutoCreate()) 2888 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2889 return this.status; 2890 } 2891 2892 public boolean hasStatusElement() { 2893 return this.status != null && !this.status.isEmpty(); 2894 } 2895 2896 public boolean hasStatus() { 2897 return this.status != null && !this.status.isEmpty(); 2898 } 2899 2900 /** 2901 * @param value {@link #status} (The status of this risk evidence synthesis. 2902 * Enables tracking the life-cycle of the content.). This is the 2903 * underlying object with id, value and extensions. The accessor 2904 * "getStatus" gives direct access to the value 2905 */ 2906 public RiskEvidenceSynthesis setStatusElement(Enumeration<PublicationStatus> value) { 2907 this.status = value; 2908 return this; 2909 } 2910 2911 /** 2912 * @return The status of this risk evidence synthesis. Enables tracking the 2913 * life-cycle of the content. 2914 */ 2915 public PublicationStatus getStatus() { 2916 return this.status == null ? null : this.status.getValue(); 2917 } 2918 2919 /** 2920 * @param value The status of this risk evidence synthesis. Enables tracking the 2921 * life-cycle of the content. 2922 */ 2923 public RiskEvidenceSynthesis setStatus(PublicationStatus value) { 2924 if (this.status == null) 2925 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2926 this.status.setValue(value); 2927 return this; 2928 } 2929 2930 /** 2931 * @return {@link #date} (The date (and optionally time) when the risk evidence 2932 * synthesis was published. The date must change when the business 2933 * version changes and it must change if the status code changes. In 2934 * addition, it should change when the substantive content of the risk 2935 * evidence synthesis changes.). This is the underlying object with id, 2936 * value and extensions. The accessor "getDate" gives direct access to 2937 * the value 2938 */ 2939 public DateTimeType getDateElement() { 2940 if (this.date == null) 2941 if (Configuration.errorOnAutoCreate()) 2942 throw new Error("Attempt to auto-create RiskEvidenceSynthesis.date"); 2943 else if (Configuration.doAutoCreate()) 2944 this.date = new DateTimeType(); // bb 2945 return this.date; 2946 } 2947 2948 public boolean hasDateElement() { 2949 return this.date != null && !this.date.isEmpty(); 2950 } 2951 2952 public boolean hasDate() { 2953 return this.date != null && !this.date.isEmpty(); 2954 } 2955 2956 /** 2957 * @param value {@link #date} (The date (and optionally time) when the risk 2958 * evidence synthesis was published. The date must change when the 2959 * business version changes and it must change if the status code 2960 * changes. In addition, it should change when the substantive 2961 * content of the risk evidence synthesis changes.). This is the 2962 * underlying object with id, value and extensions. The accessor 2963 * "getDate" gives direct access to the value 2964 */ 2965 public RiskEvidenceSynthesis setDateElement(DateTimeType value) { 2966 this.date = value; 2967 return this; 2968 } 2969 2970 /** 2971 * @return The date (and optionally time) when the risk evidence synthesis was 2972 * published. The date must change when the business version changes and 2973 * it must change if the status code changes. In addition, it should 2974 * change when the substantive content of the risk evidence synthesis 2975 * changes. 2976 */ 2977 public Date getDate() { 2978 return this.date == null ? null : this.date.getValue(); 2979 } 2980 2981 /** 2982 * @param value The date (and optionally time) when the risk evidence synthesis 2983 * was published. The date must change when the business version 2984 * changes and it must change if the status code changes. In 2985 * addition, it should change when the substantive content of the 2986 * risk evidence synthesis changes. 2987 */ 2988 public RiskEvidenceSynthesis setDate(Date value) { 2989 if (value == null) 2990 this.date = null; 2991 else { 2992 if (this.date == null) 2993 this.date = new DateTimeType(); 2994 this.date.setValue(value); 2995 } 2996 return this; 2997 } 2998 2999 /** 3000 * @return {@link #publisher} (The name of the organization or individual that 3001 * published the risk evidence synthesis.). This is the underlying 3002 * object with id, value and extensions. The accessor "getPublisher" 3003 * gives direct access to the value 3004 */ 3005 public StringType getPublisherElement() { 3006 if (this.publisher == null) 3007 if (Configuration.errorOnAutoCreate()) 3008 throw new Error("Attempt to auto-create RiskEvidenceSynthesis.publisher"); 3009 else if (Configuration.doAutoCreate()) 3010 this.publisher = new StringType(); // bb 3011 return this.publisher; 3012 } 3013 3014 public boolean hasPublisherElement() { 3015 return this.publisher != null && !this.publisher.isEmpty(); 3016 } 3017 3018 public boolean hasPublisher() { 3019 return this.publisher != null && !this.publisher.isEmpty(); 3020 } 3021 3022 /** 3023 * @param value {@link #publisher} (The name of the organization or individual 3024 * that published the risk evidence synthesis.). This is the 3025 * underlying object with id, value and extensions. The accessor 3026 * "getPublisher" gives direct access to the value 3027 */ 3028 public RiskEvidenceSynthesis setPublisherElement(StringType value) { 3029 this.publisher = value; 3030 return this; 3031 } 3032 3033 /** 3034 * @return The name of the organization or individual that published the risk 3035 * evidence synthesis. 3036 */ 3037 public String getPublisher() { 3038 return this.publisher == null ? null : this.publisher.getValue(); 3039 } 3040 3041 /** 3042 * @param value The name of the organization or individual that published the 3043 * risk evidence synthesis. 3044 */ 3045 public RiskEvidenceSynthesis setPublisher(String value) { 3046 if (Utilities.noString(value)) 3047 this.publisher = null; 3048 else { 3049 if (this.publisher == null) 3050 this.publisher = new StringType(); 3051 this.publisher.setValue(value); 3052 } 3053 return this; 3054 } 3055 3056 /** 3057 * @return {@link #contact} (Contact details to assist a user in finding and 3058 * communicating with the publisher.) 3059 */ 3060 public List<ContactDetail> getContact() { 3061 if (this.contact == null) 3062 this.contact = new ArrayList<ContactDetail>(); 3063 return this.contact; 3064 } 3065 3066 /** 3067 * @return Returns a reference to <code>this</code> for easy method chaining 3068 */ 3069 public RiskEvidenceSynthesis setContact(List<ContactDetail> theContact) { 3070 this.contact = theContact; 3071 return this; 3072 } 3073 3074 public boolean hasContact() { 3075 if (this.contact == null) 3076 return false; 3077 for (ContactDetail item : this.contact) 3078 if (!item.isEmpty()) 3079 return true; 3080 return false; 3081 } 3082 3083 public ContactDetail addContact() { // 3 3084 ContactDetail t = new ContactDetail(); 3085 if (this.contact == null) 3086 this.contact = new ArrayList<ContactDetail>(); 3087 this.contact.add(t); 3088 return t; 3089 } 3090 3091 public RiskEvidenceSynthesis addContact(ContactDetail t) { // 3 3092 if (t == null) 3093 return this; 3094 if (this.contact == null) 3095 this.contact = new ArrayList<ContactDetail>(); 3096 this.contact.add(t); 3097 return this; 3098 } 3099 3100 /** 3101 * @return The first repetition of repeating field {@link #contact}, creating it 3102 * if it does not already exist 3103 */ 3104 public ContactDetail getContactFirstRep() { 3105 if (getContact().isEmpty()) { 3106 addContact(); 3107 } 3108 return getContact().get(0); 3109 } 3110 3111 /** 3112 * @return {@link #description} (A free text natural language description of the 3113 * risk evidence synthesis from a consumer's perspective.). This is the 3114 * underlying object with id, value and extensions. The accessor 3115 * "getDescription" gives direct access to the value 3116 */ 3117 public MarkdownType getDescriptionElement() { 3118 if (this.description == null) 3119 if (Configuration.errorOnAutoCreate()) 3120 throw new Error("Attempt to auto-create RiskEvidenceSynthesis.description"); 3121 else if (Configuration.doAutoCreate()) 3122 this.description = new MarkdownType(); // bb 3123 return this.description; 3124 } 3125 3126 public boolean hasDescriptionElement() { 3127 return this.description != null && !this.description.isEmpty(); 3128 } 3129 3130 public boolean hasDescription() { 3131 return this.description != null && !this.description.isEmpty(); 3132 } 3133 3134 /** 3135 * @param value {@link #description} (A free text natural language description 3136 * of the risk evidence synthesis from a consumer's perspective.). 3137 * This is the underlying object with id, value and extensions. The 3138 * accessor "getDescription" gives direct access to the value 3139 */ 3140 public RiskEvidenceSynthesis setDescriptionElement(MarkdownType value) { 3141 this.description = value; 3142 return this; 3143 } 3144 3145 /** 3146 * @return A free text natural language description of the risk evidence 3147 * synthesis from a consumer's perspective. 3148 */ 3149 public String getDescription() { 3150 return this.description == null ? null : this.description.getValue(); 3151 } 3152 3153 /** 3154 * @param value A free text natural language description of the risk evidence 3155 * synthesis from a consumer's perspective. 3156 */ 3157 public RiskEvidenceSynthesis setDescription(String value) { 3158 if (value == null) 3159 this.description = null; 3160 else { 3161 if (this.description == null) 3162 this.description = new MarkdownType(); 3163 this.description.setValue(value); 3164 } 3165 return this; 3166 } 3167 3168 /** 3169 * @return {@link #note} (A human-readable string to clarify or explain concepts 3170 * about the resource.) 3171 */ 3172 public List<Annotation> getNote() { 3173 if (this.note == null) 3174 this.note = new ArrayList<Annotation>(); 3175 return this.note; 3176 } 3177 3178 /** 3179 * @return Returns a reference to <code>this</code> for easy method chaining 3180 */ 3181 public RiskEvidenceSynthesis setNote(List<Annotation> theNote) { 3182 this.note = theNote; 3183 return this; 3184 } 3185 3186 public boolean hasNote() { 3187 if (this.note == null) 3188 return false; 3189 for (Annotation item : this.note) 3190 if (!item.isEmpty()) 3191 return true; 3192 return false; 3193 } 3194 3195 public Annotation addNote() { // 3 3196 Annotation t = new Annotation(); 3197 if (this.note == null) 3198 this.note = new ArrayList<Annotation>(); 3199 this.note.add(t); 3200 return t; 3201 } 3202 3203 public RiskEvidenceSynthesis addNote(Annotation t) { // 3 3204 if (t == null) 3205 return this; 3206 if (this.note == null) 3207 this.note = new ArrayList<Annotation>(); 3208 this.note.add(t); 3209 return this; 3210 } 3211 3212 /** 3213 * @return The first repetition of repeating field {@link #note}, creating it if 3214 * it does not already exist 3215 */ 3216 public Annotation getNoteFirstRep() { 3217 if (getNote().isEmpty()) { 3218 addNote(); 3219 } 3220 return getNote().get(0); 3221 } 3222 3223 /** 3224 * @return {@link #useContext} (The content was developed with a focus and 3225 * intent of supporting the contexts that are listed. These contexts may 3226 * be general categories (gender, age, ...) or may be references to 3227 * specific programs (insurance plans, studies, ...) and may be used to 3228 * assist with indexing and searching for appropriate risk evidence 3229 * synthesis instances.) 3230 */ 3231 public List<UsageContext> getUseContext() { 3232 if (this.useContext == null) 3233 this.useContext = new ArrayList<UsageContext>(); 3234 return this.useContext; 3235 } 3236 3237 /** 3238 * @return Returns a reference to <code>this</code> for easy method chaining 3239 */ 3240 public RiskEvidenceSynthesis setUseContext(List<UsageContext> theUseContext) { 3241 this.useContext = theUseContext; 3242 return this; 3243 } 3244 3245 public boolean hasUseContext() { 3246 if (this.useContext == null) 3247 return false; 3248 for (UsageContext item : this.useContext) 3249 if (!item.isEmpty()) 3250 return true; 3251 return false; 3252 } 3253 3254 public UsageContext addUseContext() { // 3 3255 UsageContext t = new UsageContext(); 3256 if (this.useContext == null) 3257 this.useContext = new ArrayList<UsageContext>(); 3258 this.useContext.add(t); 3259 return t; 3260 } 3261 3262 public RiskEvidenceSynthesis addUseContext(UsageContext t) { // 3 3263 if (t == null) 3264 return this; 3265 if (this.useContext == null) 3266 this.useContext = new ArrayList<UsageContext>(); 3267 this.useContext.add(t); 3268 return this; 3269 } 3270 3271 /** 3272 * @return The first repetition of repeating field {@link #useContext}, creating 3273 * it if it does not already exist 3274 */ 3275 public UsageContext getUseContextFirstRep() { 3276 if (getUseContext().isEmpty()) { 3277 addUseContext(); 3278 } 3279 return getUseContext().get(0); 3280 } 3281 3282 /** 3283 * @return {@link #jurisdiction} (A legal or geographic region in which the risk 3284 * evidence synthesis is intended to be used.) 3285 */ 3286 public List<CodeableConcept> getJurisdiction() { 3287 if (this.jurisdiction == null) 3288 this.jurisdiction = new ArrayList<CodeableConcept>(); 3289 return this.jurisdiction; 3290 } 3291 3292 /** 3293 * @return Returns a reference to <code>this</code> for easy method chaining 3294 */ 3295 public RiskEvidenceSynthesis setJurisdiction(List<CodeableConcept> theJurisdiction) { 3296 this.jurisdiction = theJurisdiction; 3297 return this; 3298 } 3299 3300 public boolean hasJurisdiction() { 3301 if (this.jurisdiction == null) 3302 return false; 3303 for (CodeableConcept item : this.jurisdiction) 3304 if (!item.isEmpty()) 3305 return true; 3306 return false; 3307 } 3308 3309 public CodeableConcept addJurisdiction() { // 3 3310 CodeableConcept t = new CodeableConcept(); 3311 if (this.jurisdiction == null) 3312 this.jurisdiction = new ArrayList<CodeableConcept>(); 3313 this.jurisdiction.add(t); 3314 return t; 3315 } 3316 3317 public RiskEvidenceSynthesis addJurisdiction(CodeableConcept t) { // 3 3318 if (t == null) 3319 return this; 3320 if (this.jurisdiction == null) 3321 this.jurisdiction = new ArrayList<CodeableConcept>(); 3322 this.jurisdiction.add(t); 3323 return this; 3324 } 3325 3326 /** 3327 * @return The first repetition of repeating field {@link #jurisdiction}, 3328 * creating it if it does not already exist 3329 */ 3330 public CodeableConcept getJurisdictionFirstRep() { 3331 if (getJurisdiction().isEmpty()) { 3332 addJurisdiction(); 3333 } 3334 return getJurisdiction().get(0); 3335 } 3336 3337 /** 3338 * @return {@link #copyright} (A copyright statement relating to the risk 3339 * evidence synthesis and/or its contents. Copyright statements are 3340 * generally legal restrictions on the use and publishing of the risk 3341 * evidence synthesis.). This is the underlying object with id, value 3342 * and extensions. The accessor "getCopyright" gives direct access to 3343 * the value 3344 */ 3345 public MarkdownType getCopyrightElement() { 3346 if (this.copyright == null) 3347 if (Configuration.errorOnAutoCreate()) 3348 throw new Error("Attempt to auto-create RiskEvidenceSynthesis.copyright"); 3349 else if (Configuration.doAutoCreate()) 3350 this.copyright = new MarkdownType(); // bb 3351 return this.copyright; 3352 } 3353 3354 public boolean hasCopyrightElement() { 3355 return this.copyright != null && !this.copyright.isEmpty(); 3356 } 3357 3358 public boolean hasCopyright() { 3359 return this.copyright != null && !this.copyright.isEmpty(); 3360 } 3361 3362 /** 3363 * @param value {@link #copyright} (A copyright statement relating to the risk 3364 * evidence synthesis and/or its contents. Copyright statements are 3365 * generally legal restrictions on the use and publishing of the 3366 * risk evidence synthesis.). This is the underlying object with 3367 * id, value and extensions. The accessor "getCopyright" gives 3368 * direct access to the value 3369 */ 3370 public RiskEvidenceSynthesis setCopyrightElement(MarkdownType value) { 3371 this.copyright = value; 3372 return this; 3373 } 3374 3375 /** 3376 * @return A copyright statement relating to the risk evidence synthesis and/or 3377 * its contents. Copyright statements are generally legal restrictions 3378 * on the use and publishing of the risk evidence synthesis. 3379 */ 3380 public String getCopyright() { 3381 return this.copyright == null ? null : this.copyright.getValue(); 3382 } 3383 3384 /** 3385 * @param value A copyright statement relating to the risk evidence synthesis 3386 * and/or its contents. Copyright statements are generally legal 3387 * restrictions on the use and publishing of the risk evidence 3388 * synthesis. 3389 */ 3390 public RiskEvidenceSynthesis setCopyright(String value) { 3391 if (value == null) 3392 this.copyright = null; 3393 else { 3394 if (this.copyright == null) 3395 this.copyright = new MarkdownType(); 3396 this.copyright.setValue(value); 3397 } 3398 return this; 3399 } 3400 3401 /** 3402 * @return {@link #approvalDate} (The date on which the resource content was 3403 * approved by the publisher. Approval happens once when the content is 3404 * officially approved for usage.). This is the underlying object with 3405 * id, value and extensions. The accessor "getApprovalDate" gives direct 3406 * access to the value 3407 */ 3408 public DateType getApprovalDateElement() { 3409 if (this.approvalDate == null) 3410 if (Configuration.errorOnAutoCreate()) 3411 throw new Error("Attempt to auto-create RiskEvidenceSynthesis.approvalDate"); 3412 else if (Configuration.doAutoCreate()) 3413 this.approvalDate = new DateType(); // bb 3414 return this.approvalDate; 3415 } 3416 3417 public boolean hasApprovalDateElement() { 3418 return this.approvalDate != null && !this.approvalDate.isEmpty(); 3419 } 3420 3421 public boolean hasApprovalDate() { 3422 return this.approvalDate != null && !this.approvalDate.isEmpty(); 3423 } 3424 3425 /** 3426 * @param value {@link #approvalDate} (The date on which the resource content 3427 * was approved by the publisher. Approval happens once when the 3428 * content is officially approved for usage.). This is the 3429 * underlying object with id, value and extensions. The accessor 3430 * "getApprovalDate" gives direct access to the value 3431 */ 3432 public RiskEvidenceSynthesis setApprovalDateElement(DateType value) { 3433 this.approvalDate = value; 3434 return this; 3435 } 3436 3437 /** 3438 * @return The date on which the resource content was approved by the publisher. 3439 * Approval happens once when the content is officially approved for 3440 * usage. 3441 */ 3442 public Date getApprovalDate() { 3443 return this.approvalDate == null ? null : this.approvalDate.getValue(); 3444 } 3445 3446 /** 3447 * @param value The date on which the resource content was approved by the 3448 * publisher. Approval happens once when the content is officially 3449 * approved for usage. 3450 */ 3451 public RiskEvidenceSynthesis setApprovalDate(Date value) { 3452 if (value == null) 3453 this.approvalDate = null; 3454 else { 3455 if (this.approvalDate == null) 3456 this.approvalDate = new DateType(); 3457 this.approvalDate.setValue(value); 3458 } 3459 return this; 3460 } 3461 3462 /** 3463 * @return {@link #lastReviewDate} (The date on which the resource content was 3464 * last reviewed. Review happens periodically after approval but does 3465 * not change the original approval date.). This is the underlying 3466 * object with id, value and extensions. The accessor 3467 * "getLastReviewDate" gives direct access to the value 3468 */ 3469 public DateType getLastReviewDateElement() { 3470 if (this.lastReviewDate == null) 3471 if (Configuration.errorOnAutoCreate()) 3472 throw new Error("Attempt to auto-create RiskEvidenceSynthesis.lastReviewDate"); 3473 else if (Configuration.doAutoCreate()) 3474 this.lastReviewDate = new DateType(); // bb 3475 return this.lastReviewDate; 3476 } 3477 3478 public boolean hasLastReviewDateElement() { 3479 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 3480 } 3481 3482 public boolean hasLastReviewDate() { 3483 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 3484 } 3485 3486 /** 3487 * @param value {@link #lastReviewDate} (The date on which the resource content 3488 * was last reviewed. Review happens periodically after approval 3489 * but does not change the original approval date.). This is the 3490 * underlying object with id, value and extensions. The accessor 3491 * "getLastReviewDate" gives direct access to the value 3492 */ 3493 public RiskEvidenceSynthesis setLastReviewDateElement(DateType value) { 3494 this.lastReviewDate = value; 3495 return this; 3496 } 3497 3498 /** 3499 * @return The date on which the resource content was last reviewed. Review 3500 * happens periodically after approval but does not change the original 3501 * approval date. 3502 */ 3503 public Date getLastReviewDate() { 3504 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 3505 } 3506 3507 /** 3508 * @param value The date on which the resource content was last reviewed. Review 3509 * happens periodically after approval but does not change the 3510 * original approval date. 3511 */ 3512 public RiskEvidenceSynthesis setLastReviewDate(Date value) { 3513 if (value == null) 3514 this.lastReviewDate = null; 3515 else { 3516 if (this.lastReviewDate == null) 3517 this.lastReviewDate = new DateType(); 3518 this.lastReviewDate.setValue(value); 3519 } 3520 return this; 3521 } 3522 3523 /** 3524 * @return {@link #effectivePeriod} (The period during which the risk evidence 3525 * synthesis content was or is planned to be in active use.) 3526 */ 3527 public Period getEffectivePeriod() { 3528 if (this.effectivePeriod == null) 3529 if (Configuration.errorOnAutoCreate()) 3530 throw new Error("Attempt to auto-create RiskEvidenceSynthesis.effectivePeriod"); 3531 else if (Configuration.doAutoCreate()) 3532 this.effectivePeriod = new Period(); // cc 3533 return this.effectivePeriod; 3534 } 3535 3536 public boolean hasEffectivePeriod() { 3537 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 3538 } 3539 3540 /** 3541 * @param value {@link #effectivePeriod} (The period during which the risk 3542 * evidence synthesis content was or is planned to be in active 3543 * use.) 3544 */ 3545 public RiskEvidenceSynthesis setEffectivePeriod(Period value) { 3546 this.effectivePeriod = value; 3547 return this; 3548 } 3549 3550 /** 3551 * @return {@link #topic} (Descriptive topics related to the content of the 3552 * RiskEvidenceSynthesis. Topics provide a high-level categorization 3553 * grouping types of EffectEvidenceSynthesiss that can be useful for 3554 * filtering and searching.) 3555 */ 3556 public List<CodeableConcept> getTopic() { 3557 if (this.topic == null) 3558 this.topic = new ArrayList<CodeableConcept>(); 3559 return this.topic; 3560 } 3561 3562 /** 3563 * @return Returns a reference to <code>this</code> for easy method chaining 3564 */ 3565 public RiskEvidenceSynthesis setTopic(List<CodeableConcept> theTopic) { 3566 this.topic = theTopic; 3567 return this; 3568 } 3569 3570 public boolean hasTopic() { 3571 if (this.topic == null) 3572 return false; 3573 for (CodeableConcept item : this.topic) 3574 if (!item.isEmpty()) 3575 return true; 3576 return false; 3577 } 3578 3579 public CodeableConcept addTopic() { // 3 3580 CodeableConcept t = new CodeableConcept(); 3581 if (this.topic == null) 3582 this.topic = new ArrayList<CodeableConcept>(); 3583 this.topic.add(t); 3584 return t; 3585 } 3586 3587 public RiskEvidenceSynthesis addTopic(CodeableConcept t) { // 3 3588 if (t == null) 3589 return this; 3590 if (this.topic == null) 3591 this.topic = new ArrayList<CodeableConcept>(); 3592 this.topic.add(t); 3593 return this; 3594 } 3595 3596 /** 3597 * @return The first repetition of repeating field {@link #topic}, creating it 3598 * if it does not already exist 3599 */ 3600 public CodeableConcept getTopicFirstRep() { 3601 if (getTopic().isEmpty()) { 3602 addTopic(); 3603 } 3604 return getTopic().get(0); 3605 } 3606 3607 /** 3608 * @return {@link #author} (An individiual or organization primarily involved in 3609 * the creation and maintenance of the content.) 3610 */ 3611 public List<ContactDetail> getAuthor() { 3612 if (this.author == null) 3613 this.author = new ArrayList<ContactDetail>(); 3614 return this.author; 3615 } 3616 3617 /** 3618 * @return Returns a reference to <code>this</code> for easy method chaining 3619 */ 3620 public RiskEvidenceSynthesis setAuthor(List<ContactDetail> theAuthor) { 3621 this.author = theAuthor; 3622 return this; 3623 } 3624 3625 public boolean hasAuthor() { 3626 if (this.author == null) 3627 return false; 3628 for (ContactDetail item : this.author) 3629 if (!item.isEmpty()) 3630 return true; 3631 return false; 3632 } 3633 3634 public ContactDetail addAuthor() { // 3 3635 ContactDetail t = new ContactDetail(); 3636 if (this.author == null) 3637 this.author = new ArrayList<ContactDetail>(); 3638 this.author.add(t); 3639 return t; 3640 } 3641 3642 public RiskEvidenceSynthesis addAuthor(ContactDetail t) { // 3 3643 if (t == null) 3644 return this; 3645 if (this.author == null) 3646 this.author = new ArrayList<ContactDetail>(); 3647 this.author.add(t); 3648 return this; 3649 } 3650 3651 /** 3652 * @return The first repetition of repeating field {@link #author}, creating it 3653 * if it does not already exist 3654 */ 3655 public ContactDetail getAuthorFirstRep() { 3656 if (getAuthor().isEmpty()) { 3657 addAuthor(); 3658 } 3659 return getAuthor().get(0); 3660 } 3661 3662 /** 3663 * @return {@link #editor} (An individual or organization primarily responsible 3664 * for internal coherence of the content.) 3665 */ 3666 public List<ContactDetail> getEditor() { 3667 if (this.editor == null) 3668 this.editor = new ArrayList<ContactDetail>(); 3669 return this.editor; 3670 } 3671 3672 /** 3673 * @return Returns a reference to <code>this</code> for easy method chaining 3674 */ 3675 public RiskEvidenceSynthesis setEditor(List<ContactDetail> theEditor) { 3676 this.editor = theEditor; 3677 return this; 3678 } 3679 3680 public boolean hasEditor() { 3681 if (this.editor == null) 3682 return false; 3683 for (ContactDetail item : this.editor) 3684 if (!item.isEmpty()) 3685 return true; 3686 return false; 3687 } 3688 3689 public ContactDetail addEditor() { // 3 3690 ContactDetail t = new ContactDetail(); 3691 if (this.editor == null) 3692 this.editor = new ArrayList<ContactDetail>(); 3693 this.editor.add(t); 3694 return t; 3695 } 3696 3697 public RiskEvidenceSynthesis addEditor(ContactDetail t) { // 3 3698 if (t == null) 3699 return this; 3700 if (this.editor == null) 3701 this.editor = new ArrayList<ContactDetail>(); 3702 this.editor.add(t); 3703 return this; 3704 } 3705 3706 /** 3707 * @return The first repetition of repeating field {@link #editor}, creating it 3708 * if it does not already exist 3709 */ 3710 public ContactDetail getEditorFirstRep() { 3711 if (getEditor().isEmpty()) { 3712 addEditor(); 3713 } 3714 return getEditor().get(0); 3715 } 3716 3717 /** 3718 * @return {@link #reviewer} (An individual or organization primarily 3719 * responsible for review of some aspect of the content.) 3720 */ 3721 public List<ContactDetail> getReviewer() { 3722 if (this.reviewer == null) 3723 this.reviewer = new ArrayList<ContactDetail>(); 3724 return this.reviewer; 3725 } 3726 3727 /** 3728 * @return Returns a reference to <code>this</code> for easy method chaining 3729 */ 3730 public RiskEvidenceSynthesis setReviewer(List<ContactDetail> theReviewer) { 3731 this.reviewer = theReviewer; 3732 return this; 3733 } 3734 3735 public boolean hasReviewer() { 3736 if (this.reviewer == null) 3737 return false; 3738 for (ContactDetail item : this.reviewer) 3739 if (!item.isEmpty()) 3740 return true; 3741 return false; 3742 } 3743 3744 public ContactDetail addReviewer() { // 3 3745 ContactDetail t = new ContactDetail(); 3746 if (this.reviewer == null) 3747 this.reviewer = new ArrayList<ContactDetail>(); 3748 this.reviewer.add(t); 3749 return t; 3750 } 3751 3752 public RiskEvidenceSynthesis addReviewer(ContactDetail t) { // 3 3753 if (t == null) 3754 return this; 3755 if (this.reviewer == null) 3756 this.reviewer = new ArrayList<ContactDetail>(); 3757 this.reviewer.add(t); 3758 return this; 3759 } 3760 3761 /** 3762 * @return The first repetition of repeating field {@link #reviewer}, creating 3763 * it if it does not already exist 3764 */ 3765 public ContactDetail getReviewerFirstRep() { 3766 if (getReviewer().isEmpty()) { 3767 addReviewer(); 3768 } 3769 return getReviewer().get(0); 3770 } 3771 3772 /** 3773 * @return {@link #endorser} (An individual or organization responsible for 3774 * officially endorsing the content for use in some setting.) 3775 */ 3776 public List<ContactDetail> getEndorser() { 3777 if (this.endorser == null) 3778 this.endorser = new ArrayList<ContactDetail>(); 3779 return this.endorser; 3780 } 3781 3782 /** 3783 * @return Returns a reference to <code>this</code> for easy method chaining 3784 */ 3785 public RiskEvidenceSynthesis setEndorser(List<ContactDetail> theEndorser) { 3786 this.endorser = theEndorser; 3787 return this; 3788 } 3789 3790 public boolean hasEndorser() { 3791 if (this.endorser == null) 3792 return false; 3793 for (ContactDetail item : this.endorser) 3794 if (!item.isEmpty()) 3795 return true; 3796 return false; 3797 } 3798 3799 public ContactDetail addEndorser() { // 3 3800 ContactDetail t = new ContactDetail(); 3801 if (this.endorser == null) 3802 this.endorser = new ArrayList<ContactDetail>(); 3803 this.endorser.add(t); 3804 return t; 3805 } 3806 3807 public RiskEvidenceSynthesis addEndorser(ContactDetail t) { // 3 3808 if (t == null) 3809 return this; 3810 if (this.endorser == null) 3811 this.endorser = new ArrayList<ContactDetail>(); 3812 this.endorser.add(t); 3813 return this; 3814 } 3815 3816 /** 3817 * @return The first repetition of repeating field {@link #endorser}, creating 3818 * it if it does not already exist 3819 */ 3820 public ContactDetail getEndorserFirstRep() { 3821 if (getEndorser().isEmpty()) { 3822 addEndorser(); 3823 } 3824 return getEndorser().get(0); 3825 } 3826 3827 /** 3828 * @return {@link #relatedArtifact} (Related artifacts such as additional 3829 * documentation, justification, or bibliographic references.) 3830 */ 3831 public List<RelatedArtifact> getRelatedArtifact() { 3832 if (this.relatedArtifact == null) 3833 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3834 return this.relatedArtifact; 3835 } 3836 3837 /** 3838 * @return Returns a reference to <code>this</code> for easy method chaining 3839 */ 3840 public RiskEvidenceSynthesis setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 3841 this.relatedArtifact = theRelatedArtifact; 3842 return this; 3843 } 3844 3845 public boolean hasRelatedArtifact() { 3846 if (this.relatedArtifact == null) 3847 return false; 3848 for (RelatedArtifact item : this.relatedArtifact) 3849 if (!item.isEmpty()) 3850 return true; 3851 return false; 3852 } 3853 3854 public RelatedArtifact addRelatedArtifact() { // 3 3855 RelatedArtifact t = new RelatedArtifact(); 3856 if (this.relatedArtifact == null) 3857 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3858 this.relatedArtifact.add(t); 3859 return t; 3860 } 3861 3862 public RiskEvidenceSynthesis addRelatedArtifact(RelatedArtifact t) { // 3 3863 if (t == null) 3864 return this; 3865 if (this.relatedArtifact == null) 3866 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3867 this.relatedArtifact.add(t); 3868 return this; 3869 } 3870 3871 /** 3872 * @return The first repetition of repeating field {@link #relatedArtifact}, 3873 * creating it if it does not already exist 3874 */ 3875 public RelatedArtifact getRelatedArtifactFirstRep() { 3876 if (getRelatedArtifact().isEmpty()) { 3877 addRelatedArtifact(); 3878 } 3879 return getRelatedArtifact().get(0); 3880 } 3881 3882 /** 3883 * @return {@link #synthesisType} (Type of synthesis eg meta-analysis.) 3884 */ 3885 public CodeableConcept getSynthesisType() { 3886 if (this.synthesisType == null) 3887 if (Configuration.errorOnAutoCreate()) 3888 throw new Error("Attempt to auto-create RiskEvidenceSynthesis.synthesisType"); 3889 else if (Configuration.doAutoCreate()) 3890 this.synthesisType = new CodeableConcept(); // cc 3891 return this.synthesisType; 3892 } 3893 3894 public boolean hasSynthesisType() { 3895 return this.synthesisType != null && !this.synthesisType.isEmpty(); 3896 } 3897 3898 /** 3899 * @param value {@link #synthesisType} (Type of synthesis eg meta-analysis.) 3900 */ 3901 public RiskEvidenceSynthesis setSynthesisType(CodeableConcept value) { 3902 this.synthesisType = value; 3903 return this; 3904 } 3905 3906 /** 3907 * @return {@link #studyType} (Type of study eg randomized trial.) 3908 */ 3909 public CodeableConcept getStudyType() { 3910 if (this.studyType == null) 3911 if (Configuration.errorOnAutoCreate()) 3912 throw new Error("Attempt to auto-create RiskEvidenceSynthesis.studyType"); 3913 else if (Configuration.doAutoCreate()) 3914 this.studyType = new CodeableConcept(); // cc 3915 return this.studyType; 3916 } 3917 3918 public boolean hasStudyType() { 3919 return this.studyType != null && !this.studyType.isEmpty(); 3920 } 3921 3922 /** 3923 * @param value {@link #studyType} (Type of study eg randomized trial.) 3924 */ 3925 public RiskEvidenceSynthesis setStudyType(CodeableConcept value) { 3926 this.studyType = value; 3927 return this; 3928 } 3929 3930 /** 3931 * @return {@link #population} (A reference to a EvidenceVariable resource that 3932 * defines the population for the research.) 3933 */ 3934 public Reference getPopulation() { 3935 if (this.population == null) 3936 if (Configuration.errorOnAutoCreate()) 3937 throw new Error("Attempt to auto-create RiskEvidenceSynthesis.population"); 3938 else if (Configuration.doAutoCreate()) 3939 this.population = new Reference(); // cc 3940 return this.population; 3941 } 3942 3943 public boolean hasPopulation() { 3944 return this.population != null && !this.population.isEmpty(); 3945 } 3946 3947 /** 3948 * @param value {@link #population} (A reference to a EvidenceVariable resource 3949 * that defines the population for the research.) 3950 */ 3951 public RiskEvidenceSynthesis setPopulation(Reference value) { 3952 this.population = value; 3953 return this; 3954 } 3955 3956 /** 3957 * @return {@link #population} The actual object that is the target of the 3958 * reference. The reference library doesn't populate this, but you can 3959 * use it to hold the resource if you resolve it. (A reference to a 3960 * EvidenceVariable resource that defines the population for the 3961 * research.) 3962 */ 3963 public EvidenceVariable getPopulationTarget() { 3964 if (this.populationTarget == null) 3965 if (Configuration.errorOnAutoCreate()) 3966 throw new Error("Attempt to auto-create RiskEvidenceSynthesis.population"); 3967 else if (Configuration.doAutoCreate()) 3968 this.populationTarget = new EvidenceVariable(); // aa 3969 return this.populationTarget; 3970 } 3971 3972 /** 3973 * @param value {@link #population} The actual object that is the target of the 3974 * reference. The reference library doesn't use these, but you can 3975 * use it to hold the resource if you resolve it. (A reference to a 3976 * EvidenceVariable resource that defines the population for the 3977 * research.) 3978 */ 3979 public RiskEvidenceSynthesis setPopulationTarget(EvidenceVariable value) { 3980 this.populationTarget = value; 3981 return this; 3982 } 3983 3984 /** 3985 * @return {@link #exposure} (A reference to a EvidenceVariable resource that 3986 * defines the exposure for the research.) 3987 */ 3988 public Reference getExposure() { 3989 if (this.exposure == null) 3990 if (Configuration.errorOnAutoCreate()) 3991 throw new Error("Attempt to auto-create RiskEvidenceSynthesis.exposure"); 3992 else if (Configuration.doAutoCreate()) 3993 this.exposure = new Reference(); // cc 3994 return this.exposure; 3995 } 3996 3997 public boolean hasExposure() { 3998 return this.exposure != null && !this.exposure.isEmpty(); 3999 } 4000 4001 /** 4002 * @param value {@link #exposure} (A reference to a EvidenceVariable resource 4003 * that defines the exposure for the research.) 4004 */ 4005 public RiskEvidenceSynthesis setExposure(Reference value) { 4006 this.exposure = value; 4007 return this; 4008 } 4009 4010 /** 4011 * @return {@link #exposure} The actual object that is the target of the 4012 * reference. The reference library doesn't populate this, but you can 4013 * use it to hold the resource if you resolve it. (A reference to a 4014 * EvidenceVariable resource that defines the exposure for the 4015 * research.) 4016 */ 4017 public EvidenceVariable getExposureTarget() { 4018 if (this.exposureTarget == null) 4019 if (Configuration.errorOnAutoCreate()) 4020 throw new Error("Attempt to auto-create RiskEvidenceSynthesis.exposure"); 4021 else if (Configuration.doAutoCreate()) 4022 this.exposureTarget = new EvidenceVariable(); // aa 4023 return this.exposureTarget; 4024 } 4025 4026 /** 4027 * @param value {@link #exposure} The actual object that is the target of the 4028 * reference. The reference library doesn't use these, but you can 4029 * use it to hold the resource if you resolve it. (A reference to a 4030 * EvidenceVariable resource that defines the exposure for the 4031 * research.) 4032 */ 4033 public RiskEvidenceSynthesis setExposureTarget(EvidenceVariable value) { 4034 this.exposureTarget = value; 4035 return this; 4036 } 4037 4038 /** 4039 * @return {@link #outcome} (A reference to a EvidenceVariable resomece that 4040 * defines the outcome for the research.) 4041 */ 4042 public Reference getOutcome() { 4043 if (this.outcome == null) 4044 if (Configuration.errorOnAutoCreate()) 4045 throw new Error("Attempt to auto-create RiskEvidenceSynthesis.outcome"); 4046 else if (Configuration.doAutoCreate()) 4047 this.outcome = new Reference(); // cc 4048 return this.outcome; 4049 } 4050 4051 public boolean hasOutcome() { 4052 return this.outcome != null && !this.outcome.isEmpty(); 4053 } 4054 4055 /** 4056 * @param value {@link #outcome} (A reference to a EvidenceVariable resomece 4057 * that defines the outcome for the research.) 4058 */ 4059 public RiskEvidenceSynthesis setOutcome(Reference value) { 4060 this.outcome = value; 4061 return this; 4062 } 4063 4064 /** 4065 * @return {@link #outcome} The actual object that is the target of the 4066 * reference. The reference library doesn't populate this, but you can 4067 * use it to hold the resource if you resolve it. (A reference to a 4068 * EvidenceVariable resomece that defines the outcome for the research.) 4069 */ 4070 public EvidenceVariable getOutcomeTarget() { 4071 if (this.outcomeTarget == null) 4072 if (Configuration.errorOnAutoCreate()) 4073 throw new Error("Attempt to auto-create RiskEvidenceSynthesis.outcome"); 4074 else if (Configuration.doAutoCreate()) 4075 this.outcomeTarget = new EvidenceVariable(); // aa 4076 return this.outcomeTarget; 4077 } 4078 4079 /** 4080 * @param value {@link #outcome} The actual object that is the target of the 4081 * reference. The reference library doesn't use these, but you can 4082 * use it to hold the resource if you resolve it. (A reference to a 4083 * EvidenceVariable resomece that defines the outcome for the 4084 * research.) 4085 */ 4086 public RiskEvidenceSynthesis setOutcomeTarget(EvidenceVariable value) { 4087 this.outcomeTarget = value; 4088 return this; 4089 } 4090 4091 /** 4092 * @return {@link #sampleSize} (A description of the size of the sample involved 4093 * in the synthesis.) 4094 */ 4095 public RiskEvidenceSynthesisSampleSizeComponent getSampleSize() { 4096 if (this.sampleSize == null) 4097 if (Configuration.errorOnAutoCreate()) 4098 throw new Error("Attempt to auto-create RiskEvidenceSynthesis.sampleSize"); 4099 else if (Configuration.doAutoCreate()) 4100 this.sampleSize = new RiskEvidenceSynthesisSampleSizeComponent(); // cc 4101 return this.sampleSize; 4102 } 4103 4104 public boolean hasSampleSize() { 4105 return this.sampleSize != null && !this.sampleSize.isEmpty(); 4106 } 4107 4108 /** 4109 * @param value {@link #sampleSize} (A description of the size of the sample 4110 * involved in the synthesis.) 4111 */ 4112 public RiskEvidenceSynthesis setSampleSize(RiskEvidenceSynthesisSampleSizeComponent value) { 4113 this.sampleSize = value; 4114 return this; 4115 } 4116 4117 /** 4118 * @return {@link #riskEstimate} (The estimated risk of the outcome.) 4119 */ 4120 public RiskEvidenceSynthesisRiskEstimateComponent getRiskEstimate() { 4121 if (this.riskEstimate == null) 4122 if (Configuration.errorOnAutoCreate()) 4123 throw new Error("Attempt to auto-create RiskEvidenceSynthesis.riskEstimate"); 4124 else if (Configuration.doAutoCreate()) 4125 this.riskEstimate = new RiskEvidenceSynthesisRiskEstimateComponent(); // cc 4126 return this.riskEstimate; 4127 } 4128 4129 public boolean hasRiskEstimate() { 4130 return this.riskEstimate != null && !this.riskEstimate.isEmpty(); 4131 } 4132 4133 /** 4134 * @param value {@link #riskEstimate} (The estimated risk of the outcome.) 4135 */ 4136 public RiskEvidenceSynthesis setRiskEstimate(RiskEvidenceSynthesisRiskEstimateComponent value) { 4137 this.riskEstimate = value; 4138 return this; 4139 } 4140 4141 /** 4142 * @return {@link #certainty} (A description of the certainty of the risk 4143 * estimate.) 4144 */ 4145 public List<RiskEvidenceSynthesisCertaintyComponent> getCertainty() { 4146 if (this.certainty == null) 4147 this.certainty = new ArrayList<RiskEvidenceSynthesisCertaintyComponent>(); 4148 return this.certainty; 4149 } 4150 4151 /** 4152 * @return Returns a reference to <code>this</code> for easy method chaining 4153 */ 4154 public RiskEvidenceSynthesis setCertainty(List<RiskEvidenceSynthesisCertaintyComponent> theCertainty) { 4155 this.certainty = theCertainty; 4156 return this; 4157 } 4158 4159 public boolean hasCertainty() { 4160 if (this.certainty == null) 4161 return false; 4162 for (RiskEvidenceSynthesisCertaintyComponent item : this.certainty) 4163 if (!item.isEmpty()) 4164 return true; 4165 return false; 4166 } 4167 4168 public RiskEvidenceSynthesisCertaintyComponent addCertainty() { // 3 4169 RiskEvidenceSynthesisCertaintyComponent t = new RiskEvidenceSynthesisCertaintyComponent(); 4170 if (this.certainty == null) 4171 this.certainty = new ArrayList<RiskEvidenceSynthesisCertaintyComponent>(); 4172 this.certainty.add(t); 4173 return t; 4174 } 4175 4176 public RiskEvidenceSynthesis addCertainty(RiskEvidenceSynthesisCertaintyComponent t) { // 3 4177 if (t == null) 4178 return this; 4179 if (this.certainty == null) 4180 this.certainty = new ArrayList<RiskEvidenceSynthesisCertaintyComponent>(); 4181 this.certainty.add(t); 4182 return this; 4183 } 4184 4185 /** 4186 * @return The first repetition of repeating field {@link #certainty}, creating 4187 * it if it does not already exist 4188 */ 4189 public RiskEvidenceSynthesisCertaintyComponent getCertaintyFirstRep() { 4190 if (getCertainty().isEmpty()) { 4191 addCertainty(); 4192 } 4193 return getCertainty().get(0); 4194 } 4195 4196 protected void listChildren(List<Property> children) { 4197 super.listChildren(children); 4198 children.add(new Property("url", "uri", 4199 "An absolute URI that is used to identify this risk evidence synthesis when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this risk evidence synthesis is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the risk evidence synthesis is stored on different servers.", 4200 0, 1, url)); 4201 children.add(new Property("identifier", "Identifier", 4202 "A formal identifier that is used to identify this risk evidence synthesis when it is represented in other formats, or referenced in a specification, model, design or an instance.", 4203 0, java.lang.Integer.MAX_VALUE, identifier)); 4204 children.add(new Property("version", "string", 4205 "The identifier that is used to identify this version of the risk evidence synthesis when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the risk evidence synthesis author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 4206 0, 1, version)); 4207 children.add(new Property("name", "string", 4208 "A natural language name identifying the risk evidence synthesis. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 4209 0, 1, name)); 4210 children.add(new Property("title", "string", 4211 "A short, descriptive, user-friendly title for the risk evidence synthesis.", 0, 1, title)); 4212 children.add(new Property("status", "code", 4213 "The status of this risk evidence synthesis. Enables tracking the life-cycle of the content.", 0, 1, status)); 4214 children.add(new Property("date", "dateTime", 4215 "The date (and optionally time) when the risk evidence synthesis was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the risk evidence synthesis changes.", 4216 0, 1, date)); 4217 children.add(new Property("publisher", "string", 4218 "The name of the organization or individual that published the risk evidence synthesis.", 0, 1, publisher)); 4219 children.add(new Property("contact", "ContactDetail", 4220 "Contact details to assist a user in finding and communicating with the publisher.", 0, 4221 java.lang.Integer.MAX_VALUE, contact)); 4222 children.add(new Property("description", "markdown", 4223 "A free text natural language description of the risk evidence synthesis from a consumer's perspective.", 0, 1, 4224 description)); 4225 children.add( 4226 new Property("note", "Annotation", "A human-readable string to clarify or explain concepts about the resource.", 4227 0, java.lang.Integer.MAX_VALUE, note)); 4228 children.add(new Property("useContext", "UsageContext", 4229 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate risk evidence synthesis instances.", 4230 0, java.lang.Integer.MAX_VALUE, useContext)); 4231 children.add(new Property("jurisdiction", "CodeableConcept", 4232 "A legal or geographic region in which the risk evidence synthesis is intended to be used.", 0, 4233 java.lang.Integer.MAX_VALUE, jurisdiction)); 4234 children.add(new Property("copyright", "markdown", 4235 "A copyright statement relating to the risk evidence synthesis and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the risk evidence synthesis.", 4236 0, 1, copyright)); 4237 children.add(new Property("approvalDate", "date", 4238 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 4239 0, 1, approvalDate)); 4240 children.add(new Property("lastReviewDate", "date", 4241 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 4242 0, 1, lastReviewDate)); 4243 children.add(new Property("effectivePeriod", "Period", 4244 "The period during which the risk evidence synthesis content was or is planned to be in active use.", 0, 1, 4245 effectivePeriod)); 4246 children.add(new Property("topic", "CodeableConcept", 4247 "Descriptive topics related to the content of the RiskEvidenceSynthesis. Topics provide a high-level categorization grouping types of EffectEvidenceSynthesiss that can be useful for filtering and searching.", 4248 0, java.lang.Integer.MAX_VALUE, topic)); 4249 children.add(new Property("author", "ContactDetail", 4250 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 4251 java.lang.Integer.MAX_VALUE, author)); 4252 children.add(new Property("editor", "ContactDetail", 4253 "An individual or organization primarily responsible for internal coherence of the content.", 0, 4254 java.lang.Integer.MAX_VALUE, editor)); 4255 children.add(new Property("reviewer", "ContactDetail", 4256 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 4257 java.lang.Integer.MAX_VALUE, reviewer)); 4258 children.add(new Property("endorser", "ContactDetail", 4259 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 4260 java.lang.Integer.MAX_VALUE, endorser)); 4261 children.add(new Property("relatedArtifact", "RelatedArtifact", 4262 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 4263 java.lang.Integer.MAX_VALUE, relatedArtifact)); 4264 children.add( 4265 new Property("synthesisType", "CodeableConcept", "Type of synthesis eg meta-analysis.", 0, 1, synthesisType)); 4266 children.add(new Property("studyType", "CodeableConcept", "Type of study eg randomized trial.", 0, 1, studyType)); 4267 children.add(new Property("population", "Reference(EvidenceVariable)", 4268 "A reference to a EvidenceVariable resource that defines the population for the research.", 0, 1, population)); 4269 children.add(new Property("exposure", "Reference(EvidenceVariable)", 4270 "A reference to a EvidenceVariable resource that defines the exposure for the research.", 0, 1, exposure)); 4271 children.add(new Property("outcome", "Reference(EvidenceVariable)", 4272 "A reference to a EvidenceVariable resomece that defines the outcome for the research.", 0, 1, outcome)); 4273 children.add(new Property("sampleSize", "", "A description of the size of the sample involved in the synthesis.", 0, 4274 1, sampleSize)); 4275 children.add(new Property("riskEstimate", "", "The estimated risk of the outcome.", 0, 1, riskEstimate)); 4276 children.add(new Property("certainty", "", "A description of the certainty of the risk estimate.", 0, 4277 java.lang.Integer.MAX_VALUE, certainty)); 4278 } 4279 4280 @Override 4281 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4282 switch (_hash) { 4283 case 116079: 4284 /* url */ return new Property("url", "uri", 4285 "An absolute URI that is used to identify this risk evidence synthesis when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this risk evidence synthesis is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the risk evidence synthesis is stored on different servers.", 4286 0, 1, url); 4287 case -1618432855: 4288 /* identifier */ return new Property("identifier", "Identifier", 4289 "A formal identifier that is used to identify this risk evidence synthesis when it is represented in other formats, or referenced in a specification, model, design or an instance.", 4290 0, java.lang.Integer.MAX_VALUE, identifier); 4291 case 351608024: 4292 /* version */ return new Property("version", "string", 4293 "The identifier that is used to identify this version of the risk evidence synthesis when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the risk evidence synthesis author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 4294 0, 1, version); 4295 case 3373707: 4296 /* name */ return new Property("name", "string", 4297 "A natural language name identifying the risk evidence synthesis. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 4298 0, 1, name); 4299 case 110371416: 4300 /* title */ return new Property("title", "string", 4301 "A short, descriptive, user-friendly title for the risk evidence synthesis.", 0, 1, title); 4302 case -892481550: 4303 /* status */ return new Property("status", "code", 4304 "The status of this risk evidence synthesis. Enables tracking the life-cycle of the content.", 0, 1, status); 4305 case 3076014: 4306 /* date */ return new Property("date", "dateTime", 4307 "The date (and optionally time) when the risk evidence synthesis was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the risk evidence synthesis changes.", 4308 0, 1, date); 4309 case 1447404028: 4310 /* publisher */ return new Property("publisher", "string", 4311 "The name of the organization or individual that published the risk evidence synthesis.", 0, 1, publisher); 4312 case 951526432: 4313 /* contact */ return new Property("contact", "ContactDetail", 4314 "Contact details to assist a user in finding and communicating with the publisher.", 0, 4315 java.lang.Integer.MAX_VALUE, contact); 4316 case -1724546052: 4317 /* description */ return new Property("description", "markdown", 4318 "A free text natural language description of the risk evidence synthesis from a consumer's perspective.", 0, 4319 1, description); 4320 case 3387378: 4321 /* note */ return new Property("note", "Annotation", 4322 "A human-readable string to clarify or explain concepts about the resource.", 0, java.lang.Integer.MAX_VALUE, 4323 note); 4324 case -669707736: 4325 /* useContext */ return new Property("useContext", "UsageContext", 4326 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate risk evidence synthesis instances.", 4327 0, java.lang.Integer.MAX_VALUE, useContext); 4328 case -507075711: 4329 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 4330 "A legal or geographic region in which the risk evidence synthesis is intended to be used.", 0, 4331 java.lang.Integer.MAX_VALUE, jurisdiction); 4332 case 1522889671: 4333 /* copyright */ return new Property("copyright", "markdown", 4334 "A copyright statement relating to the risk evidence synthesis and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the risk evidence synthesis.", 4335 0, 1, copyright); 4336 case 223539345: 4337 /* approvalDate */ return new Property("approvalDate", "date", 4338 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 4339 0, 1, approvalDate); 4340 case -1687512484: 4341 /* lastReviewDate */ return new Property("lastReviewDate", "date", 4342 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 4343 0, 1, lastReviewDate); 4344 case -403934648: 4345 /* effectivePeriod */ return new Property("effectivePeriod", "Period", 4346 "The period during which the risk evidence synthesis content was or is planned to be in active use.", 0, 1, 4347 effectivePeriod); 4348 case 110546223: 4349 /* topic */ return new Property("topic", "CodeableConcept", 4350 "Descriptive topics related to the content of the RiskEvidenceSynthesis. Topics provide a high-level categorization grouping types of EffectEvidenceSynthesiss that can be useful for filtering and searching.", 4351 0, java.lang.Integer.MAX_VALUE, topic); 4352 case -1406328437: 4353 /* author */ return new Property("author", "ContactDetail", 4354 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 4355 java.lang.Integer.MAX_VALUE, author); 4356 case -1307827859: 4357 /* editor */ return new Property("editor", "ContactDetail", 4358 "An individual or organization primarily responsible for internal coherence of the content.", 0, 4359 java.lang.Integer.MAX_VALUE, editor); 4360 case -261190139: 4361 /* reviewer */ return new Property("reviewer", "ContactDetail", 4362 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 4363 java.lang.Integer.MAX_VALUE, reviewer); 4364 case 1740277666: 4365 /* endorser */ return new Property("endorser", "ContactDetail", 4366 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 4367 java.lang.Integer.MAX_VALUE, endorser); 4368 case 666807069: 4369 /* relatedArtifact */ return new Property("relatedArtifact", "RelatedArtifact", 4370 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 4371 java.lang.Integer.MAX_VALUE, relatedArtifact); 4372 case 672726254: 4373 /* synthesisType */ return new Property("synthesisType", "CodeableConcept", "Type of synthesis eg meta-analysis.", 4374 0, 1, synthesisType); 4375 case -1955265373: 4376 /* studyType */ return new Property("studyType", "CodeableConcept", "Type of study eg randomized trial.", 0, 1, 4377 studyType); 4378 case -2023558323: 4379 /* population */ return new Property("population", "Reference(EvidenceVariable)", 4380 "A reference to a EvidenceVariable resource that defines the population for the research.", 0, 1, population); 4381 case -1926005497: 4382 /* exposure */ return new Property("exposure", "Reference(EvidenceVariable)", 4383 "A reference to a EvidenceVariable resource that defines the exposure for the research.", 0, 1, exposure); 4384 case -1106507950: 4385 /* outcome */ return new Property("outcome", "Reference(EvidenceVariable)", 4386 "A reference to a EvidenceVariable resomece that defines the outcome for the research.", 0, 1, outcome); 4387 case 143123659: 4388 /* sampleSize */ return new Property("sampleSize", "", 4389 "A description of the size of the sample involved in the synthesis.", 0, 1, sampleSize); 4390 case -1014254313: 4391 /* riskEstimate */ return new Property("riskEstimate", "", "The estimated risk of the outcome.", 0, 1, 4392 riskEstimate); 4393 case -1404142937: 4394 /* certainty */ return new Property("certainty", "", "A description of the certainty of the risk estimate.", 0, 4395 java.lang.Integer.MAX_VALUE, certainty); 4396 default: 4397 return super.getNamedProperty(_hash, _name, _checkValid); 4398 } 4399 4400 } 4401 4402 @Override 4403 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4404 switch (hash) { 4405 case 116079: 4406 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 4407 case -1618432855: 4408 /* identifier */ return this.identifier == null ? new Base[0] 4409 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4410 case 351608024: 4411 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 4412 case 3373707: 4413 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 4414 case 110371416: 4415 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 4416 case -892481550: 4417 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 4418 case 3076014: 4419 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 4420 case 1447404028: 4421 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 4422 case 951526432: 4423 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 4424 case -1724546052: 4425 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 4426 case 3387378: 4427 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 4428 case -669707736: 4429 /* useContext */ return this.useContext == null ? new Base[0] 4430 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 4431 case -507075711: 4432 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 4433 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 4434 case 1522889671: 4435 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 4436 case 223539345: 4437 /* approvalDate */ return this.approvalDate == null ? new Base[0] : new Base[] { this.approvalDate }; // DateType 4438 case -1687512484: 4439 /* lastReviewDate */ return this.lastReviewDate == null ? new Base[0] : new Base[] { this.lastReviewDate }; // DateType 4440 case -403934648: 4441 /* effectivePeriod */ return this.effectivePeriod == null ? new Base[0] : new Base[] { this.effectivePeriod }; // Period 4442 case 110546223: 4443 /* topic */ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 4444 case -1406328437: 4445 /* author */ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 4446 case -1307827859: 4447 /* editor */ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 4448 case -261190139: 4449 /* reviewer */ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 4450 case 1740277666: 4451 /* endorser */ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 4452 case 666807069: 4453 /* relatedArtifact */ return this.relatedArtifact == null ? new Base[0] 4454 : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 4455 case 672726254: 4456 /* synthesisType */ return this.synthesisType == null ? new Base[0] : new Base[] { this.synthesisType }; // CodeableConcept 4457 case -1955265373: 4458 /* studyType */ return this.studyType == null ? new Base[0] : new Base[] { this.studyType }; // CodeableConcept 4459 case -2023558323: 4460 /* population */ return this.population == null ? new Base[0] : new Base[] { this.population }; // Reference 4461 case -1926005497: 4462 /* exposure */ return this.exposure == null ? new Base[0] : new Base[] { this.exposure }; // Reference 4463 case -1106507950: 4464 /* outcome */ return this.outcome == null ? new Base[0] : new Base[] { this.outcome }; // Reference 4465 case 143123659: 4466 /* sampleSize */ return this.sampleSize == null ? new Base[0] : new Base[] { this.sampleSize }; // RiskEvidenceSynthesisSampleSizeComponent 4467 case -1014254313: 4468 /* riskEstimate */ return this.riskEstimate == null ? new Base[0] : new Base[] { this.riskEstimate }; // RiskEvidenceSynthesisRiskEstimateComponent 4469 case -1404142937: 4470 /* certainty */ return this.certainty == null ? new Base[0] 4471 : this.certainty.toArray(new Base[this.certainty.size()]); // RiskEvidenceSynthesisCertaintyComponent 4472 default: 4473 return super.getProperty(hash, name, checkValid); 4474 } 4475 4476 } 4477 4478 @Override 4479 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4480 switch (hash) { 4481 case 116079: // url 4482 this.url = castToUri(value); // UriType 4483 return value; 4484 case -1618432855: // identifier 4485 this.getIdentifier().add(castToIdentifier(value)); // Identifier 4486 return value; 4487 case 351608024: // version 4488 this.version = castToString(value); // StringType 4489 return value; 4490 case 3373707: // name 4491 this.name = castToString(value); // StringType 4492 return value; 4493 case 110371416: // title 4494 this.title = castToString(value); // StringType 4495 return value; 4496 case -892481550: // status 4497 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 4498 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4499 return value; 4500 case 3076014: // date 4501 this.date = castToDateTime(value); // DateTimeType 4502 return value; 4503 case 1447404028: // publisher 4504 this.publisher = castToString(value); // StringType 4505 return value; 4506 case 951526432: // contact 4507 this.getContact().add(castToContactDetail(value)); // ContactDetail 4508 return value; 4509 case -1724546052: // description 4510 this.description = castToMarkdown(value); // MarkdownType 4511 return value; 4512 case 3387378: // note 4513 this.getNote().add(castToAnnotation(value)); // Annotation 4514 return value; 4515 case -669707736: // useContext 4516 this.getUseContext().add(castToUsageContext(value)); // UsageContext 4517 return value; 4518 case -507075711: // jurisdiction 4519 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 4520 return value; 4521 case 1522889671: // copyright 4522 this.copyright = castToMarkdown(value); // MarkdownType 4523 return value; 4524 case 223539345: // approvalDate 4525 this.approvalDate = castToDate(value); // DateType 4526 return value; 4527 case -1687512484: // lastReviewDate 4528 this.lastReviewDate = castToDate(value); // DateType 4529 return value; 4530 case -403934648: // effectivePeriod 4531 this.effectivePeriod = castToPeriod(value); // Period 4532 return value; 4533 case 110546223: // topic 4534 this.getTopic().add(castToCodeableConcept(value)); // CodeableConcept 4535 return value; 4536 case -1406328437: // author 4537 this.getAuthor().add(castToContactDetail(value)); // ContactDetail 4538 return value; 4539 case -1307827859: // editor 4540 this.getEditor().add(castToContactDetail(value)); // ContactDetail 4541 return value; 4542 case -261190139: // reviewer 4543 this.getReviewer().add(castToContactDetail(value)); // ContactDetail 4544 return value; 4545 case 1740277666: // endorser 4546 this.getEndorser().add(castToContactDetail(value)); // ContactDetail 4547 return value; 4548 case 666807069: // relatedArtifact 4549 this.getRelatedArtifact().add(castToRelatedArtifact(value)); // RelatedArtifact 4550 return value; 4551 case 672726254: // synthesisType 4552 this.synthesisType = castToCodeableConcept(value); // CodeableConcept 4553 return value; 4554 case -1955265373: // studyType 4555 this.studyType = castToCodeableConcept(value); // CodeableConcept 4556 return value; 4557 case -2023558323: // population 4558 this.population = castToReference(value); // Reference 4559 return value; 4560 case -1926005497: // exposure 4561 this.exposure = castToReference(value); // Reference 4562 return value; 4563 case -1106507950: // outcome 4564 this.outcome = castToReference(value); // Reference 4565 return value; 4566 case 143123659: // sampleSize 4567 this.sampleSize = (RiskEvidenceSynthesisSampleSizeComponent) value; // RiskEvidenceSynthesisSampleSizeComponent 4568 return value; 4569 case -1014254313: // riskEstimate 4570 this.riskEstimate = (RiskEvidenceSynthesisRiskEstimateComponent) value; // RiskEvidenceSynthesisRiskEstimateComponent 4571 return value; 4572 case -1404142937: // certainty 4573 this.getCertainty().add((RiskEvidenceSynthesisCertaintyComponent) value); // RiskEvidenceSynthesisCertaintyComponent 4574 return value; 4575 default: 4576 return super.setProperty(hash, name, value); 4577 } 4578 4579 } 4580 4581 @Override 4582 public Base setProperty(String name, Base value) throws FHIRException { 4583 if (name.equals("url")) { 4584 this.url = castToUri(value); // UriType 4585 } else if (name.equals("identifier")) { 4586 this.getIdentifier().add(castToIdentifier(value)); 4587 } else if (name.equals("version")) { 4588 this.version = castToString(value); // StringType 4589 } else if (name.equals("name")) { 4590 this.name = castToString(value); // StringType 4591 } else if (name.equals("title")) { 4592 this.title = castToString(value); // StringType 4593 } else if (name.equals("status")) { 4594 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 4595 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4596 } else if (name.equals("date")) { 4597 this.date = castToDateTime(value); // DateTimeType 4598 } else if (name.equals("publisher")) { 4599 this.publisher = castToString(value); // StringType 4600 } else if (name.equals("contact")) { 4601 this.getContact().add(castToContactDetail(value)); 4602 } else if (name.equals("description")) { 4603 this.description = castToMarkdown(value); // MarkdownType 4604 } else if (name.equals("note")) { 4605 this.getNote().add(castToAnnotation(value)); 4606 } else if (name.equals("useContext")) { 4607 this.getUseContext().add(castToUsageContext(value)); 4608 } else if (name.equals("jurisdiction")) { 4609 this.getJurisdiction().add(castToCodeableConcept(value)); 4610 } else if (name.equals("copyright")) { 4611 this.copyright = castToMarkdown(value); // MarkdownType 4612 } else if (name.equals("approvalDate")) { 4613 this.approvalDate = castToDate(value); // DateType 4614 } else if (name.equals("lastReviewDate")) { 4615 this.lastReviewDate = castToDate(value); // DateType 4616 } else if (name.equals("effectivePeriod")) { 4617 this.effectivePeriod = castToPeriod(value); // Period 4618 } else if (name.equals("topic")) { 4619 this.getTopic().add(castToCodeableConcept(value)); 4620 } else if (name.equals("author")) { 4621 this.getAuthor().add(castToContactDetail(value)); 4622 } else if (name.equals("editor")) { 4623 this.getEditor().add(castToContactDetail(value)); 4624 } else if (name.equals("reviewer")) { 4625 this.getReviewer().add(castToContactDetail(value)); 4626 } else if (name.equals("endorser")) { 4627 this.getEndorser().add(castToContactDetail(value)); 4628 } else if (name.equals("relatedArtifact")) { 4629 this.getRelatedArtifact().add(castToRelatedArtifact(value)); 4630 } else if (name.equals("synthesisType")) { 4631 this.synthesisType = castToCodeableConcept(value); // CodeableConcept 4632 } else if (name.equals("studyType")) { 4633 this.studyType = castToCodeableConcept(value); // CodeableConcept 4634 } else if (name.equals("population")) { 4635 this.population = castToReference(value); // Reference 4636 } else if (name.equals("exposure")) { 4637 this.exposure = castToReference(value); // Reference 4638 } else if (name.equals("outcome")) { 4639 this.outcome = castToReference(value); // Reference 4640 } else if (name.equals("sampleSize")) { 4641 this.sampleSize = (RiskEvidenceSynthesisSampleSizeComponent) value; // RiskEvidenceSynthesisSampleSizeComponent 4642 } else if (name.equals("riskEstimate")) { 4643 this.riskEstimate = (RiskEvidenceSynthesisRiskEstimateComponent) value; // RiskEvidenceSynthesisRiskEstimateComponent 4644 } else if (name.equals("certainty")) { 4645 this.getCertainty().add((RiskEvidenceSynthesisCertaintyComponent) value); 4646 } else 4647 return super.setProperty(name, value); 4648 return value; 4649 } 4650 4651 @Override 4652 public void removeChild(String name, Base value) throws FHIRException { 4653 if (name.equals("url")) { 4654 this.url = null; 4655 } else if (name.equals("identifier")) { 4656 this.getIdentifier().remove(castToIdentifier(value)); 4657 } else if (name.equals("version")) { 4658 this.version = null; 4659 } else if (name.equals("name")) { 4660 this.name = null; 4661 } else if (name.equals("title")) { 4662 this.title = null; 4663 } else if (name.equals("status")) { 4664 this.status = null; 4665 } else if (name.equals("date")) { 4666 this.date = null; 4667 } else if (name.equals("publisher")) { 4668 this.publisher = null; 4669 } else if (name.equals("contact")) { 4670 this.getContact().remove(castToContactDetail(value)); 4671 } else if (name.equals("description")) { 4672 this.description = null; 4673 } else if (name.equals("note")) { 4674 this.getNote().remove(castToAnnotation(value)); 4675 } else if (name.equals("useContext")) { 4676 this.getUseContext().remove(castToUsageContext(value)); 4677 } else if (name.equals("jurisdiction")) { 4678 this.getJurisdiction().remove(castToCodeableConcept(value)); 4679 } else if (name.equals("copyright")) { 4680 this.copyright = null; 4681 } else if (name.equals("approvalDate")) { 4682 this.approvalDate = null; 4683 } else if (name.equals("lastReviewDate")) { 4684 this.lastReviewDate = null; 4685 } else if (name.equals("effectivePeriod")) { 4686 this.effectivePeriod = null; 4687 } else if (name.equals("topic")) { 4688 this.getTopic().remove(castToCodeableConcept(value)); 4689 } else if (name.equals("author")) { 4690 this.getAuthor().remove(castToContactDetail(value)); 4691 } else if (name.equals("editor")) { 4692 this.getEditor().remove(castToContactDetail(value)); 4693 } else if (name.equals("reviewer")) { 4694 this.getReviewer().remove(castToContactDetail(value)); 4695 } else if (name.equals("endorser")) { 4696 this.getEndorser().remove(castToContactDetail(value)); 4697 } else if (name.equals("relatedArtifact")) { 4698 this.getRelatedArtifact().remove(castToRelatedArtifact(value)); 4699 } else if (name.equals("synthesisType")) { 4700 this.synthesisType = null; 4701 } else if (name.equals("studyType")) { 4702 this.studyType = null; 4703 } else if (name.equals("population")) { 4704 this.population = null; 4705 } else if (name.equals("exposure")) { 4706 this.exposure = null; 4707 } else if (name.equals("outcome")) { 4708 this.outcome = null; 4709 } else if (name.equals("sampleSize")) { 4710 this.sampleSize = (RiskEvidenceSynthesisSampleSizeComponent) value; // RiskEvidenceSynthesisSampleSizeComponent 4711 } else if (name.equals("riskEstimate")) { 4712 this.riskEstimate = (RiskEvidenceSynthesisRiskEstimateComponent) value; // RiskEvidenceSynthesisRiskEstimateComponent 4713 } else if (name.equals("certainty")) { 4714 this.getCertainty().remove((RiskEvidenceSynthesisCertaintyComponent) value); 4715 } else 4716 super.removeChild(name, value); 4717 4718 } 4719 4720 @Override 4721 public Base makeProperty(int hash, String name) throws FHIRException { 4722 switch (hash) { 4723 case 116079: 4724 return getUrlElement(); 4725 case -1618432855: 4726 return addIdentifier(); 4727 case 351608024: 4728 return getVersionElement(); 4729 case 3373707: 4730 return getNameElement(); 4731 case 110371416: 4732 return getTitleElement(); 4733 case -892481550: 4734 return getStatusElement(); 4735 case 3076014: 4736 return getDateElement(); 4737 case 1447404028: 4738 return getPublisherElement(); 4739 case 951526432: 4740 return addContact(); 4741 case -1724546052: 4742 return getDescriptionElement(); 4743 case 3387378: 4744 return addNote(); 4745 case -669707736: 4746 return addUseContext(); 4747 case -507075711: 4748 return addJurisdiction(); 4749 case 1522889671: 4750 return getCopyrightElement(); 4751 case 223539345: 4752 return getApprovalDateElement(); 4753 case -1687512484: 4754 return getLastReviewDateElement(); 4755 case -403934648: 4756 return getEffectivePeriod(); 4757 case 110546223: 4758 return addTopic(); 4759 case -1406328437: 4760 return addAuthor(); 4761 case -1307827859: 4762 return addEditor(); 4763 case -261190139: 4764 return addReviewer(); 4765 case 1740277666: 4766 return addEndorser(); 4767 case 666807069: 4768 return addRelatedArtifact(); 4769 case 672726254: 4770 return getSynthesisType(); 4771 case -1955265373: 4772 return getStudyType(); 4773 case -2023558323: 4774 return getPopulation(); 4775 case -1926005497: 4776 return getExposure(); 4777 case -1106507950: 4778 return getOutcome(); 4779 case 143123659: 4780 return getSampleSize(); 4781 case -1014254313: 4782 return getRiskEstimate(); 4783 case -1404142937: 4784 return addCertainty(); 4785 default: 4786 return super.makeProperty(hash, name); 4787 } 4788 4789 } 4790 4791 @Override 4792 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4793 switch (hash) { 4794 case 116079: 4795 /* url */ return new String[] { "uri" }; 4796 case -1618432855: 4797 /* identifier */ return new String[] { "Identifier" }; 4798 case 351608024: 4799 /* version */ return new String[] { "string" }; 4800 case 3373707: 4801 /* name */ return new String[] { "string" }; 4802 case 110371416: 4803 /* title */ return new String[] { "string" }; 4804 case -892481550: 4805 /* status */ return new String[] { "code" }; 4806 case 3076014: 4807 /* date */ return new String[] { "dateTime" }; 4808 case 1447404028: 4809 /* publisher */ return new String[] { "string" }; 4810 case 951526432: 4811 /* contact */ return new String[] { "ContactDetail" }; 4812 case -1724546052: 4813 /* description */ return new String[] { "markdown" }; 4814 case 3387378: 4815 /* note */ return new String[] { "Annotation" }; 4816 case -669707736: 4817 /* useContext */ return new String[] { "UsageContext" }; 4818 case -507075711: 4819 /* jurisdiction */ return new String[] { "CodeableConcept" }; 4820 case 1522889671: 4821 /* copyright */ return new String[] { "markdown" }; 4822 case 223539345: 4823 /* approvalDate */ return new String[] { "date" }; 4824 case -1687512484: 4825 /* lastReviewDate */ return new String[] { "date" }; 4826 case -403934648: 4827 /* effectivePeriod */ return new String[] { "Period" }; 4828 case 110546223: 4829 /* topic */ return new String[] { "CodeableConcept" }; 4830 case -1406328437: 4831 /* author */ return new String[] { "ContactDetail" }; 4832 case -1307827859: 4833 /* editor */ return new String[] { "ContactDetail" }; 4834 case -261190139: 4835 /* reviewer */ return new String[] { "ContactDetail" }; 4836 case 1740277666: 4837 /* endorser */ return new String[] { "ContactDetail" }; 4838 case 666807069: 4839 /* relatedArtifact */ return new String[] { "RelatedArtifact" }; 4840 case 672726254: 4841 /* synthesisType */ return new String[] { "CodeableConcept" }; 4842 case -1955265373: 4843 /* studyType */ return new String[] { "CodeableConcept" }; 4844 case -2023558323: 4845 /* population */ return new String[] { "Reference" }; 4846 case -1926005497: 4847 /* exposure */ return new String[] { "Reference" }; 4848 case -1106507950: 4849 /* outcome */ return new String[] { "Reference" }; 4850 case 143123659: 4851 /* sampleSize */ return new String[] {}; 4852 case -1014254313: 4853 /* riskEstimate */ return new String[] {}; 4854 case -1404142937: 4855 /* certainty */ return new String[] {}; 4856 default: 4857 return super.getTypesForProperty(hash, name); 4858 } 4859 4860 } 4861 4862 @Override 4863 public Base addChild(String name) throws FHIRException { 4864 if (name.equals("url")) { 4865 throw new FHIRException("Cannot call addChild on a singleton property RiskEvidenceSynthesis.url"); 4866 } else if (name.equals("identifier")) { 4867 return addIdentifier(); 4868 } else if (name.equals("version")) { 4869 throw new FHIRException("Cannot call addChild on a singleton property RiskEvidenceSynthesis.version"); 4870 } else if (name.equals("name")) { 4871 throw new FHIRException("Cannot call addChild on a singleton property RiskEvidenceSynthesis.name"); 4872 } else if (name.equals("title")) { 4873 throw new FHIRException("Cannot call addChild on a singleton property RiskEvidenceSynthesis.title"); 4874 } else if (name.equals("status")) { 4875 throw new FHIRException("Cannot call addChild on a singleton property RiskEvidenceSynthesis.status"); 4876 } else if (name.equals("date")) { 4877 throw new FHIRException("Cannot call addChild on a singleton property RiskEvidenceSynthesis.date"); 4878 } else if (name.equals("publisher")) { 4879 throw new FHIRException("Cannot call addChild on a singleton property RiskEvidenceSynthesis.publisher"); 4880 } else if (name.equals("contact")) { 4881 return addContact(); 4882 } else if (name.equals("description")) { 4883 throw new FHIRException("Cannot call addChild on a singleton property RiskEvidenceSynthesis.description"); 4884 } else if (name.equals("note")) { 4885 return addNote(); 4886 } else if (name.equals("useContext")) { 4887 return addUseContext(); 4888 } else if (name.equals("jurisdiction")) { 4889 return addJurisdiction(); 4890 } else if (name.equals("copyright")) { 4891 throw new FHIRException("Cannot call addChild on a singleton property RiskEvidenceSynthesis.copyright"); 4892 } else if (name.equals("approvalDate")) { 4893 throw new FHIRException("Cannot call addChild on a singleton property RiskEvidenceSynthesis.approvalDate"); 4894 } else if (name.equals("lastReviewDate")) { 4895 throw new FHIRException("Cannot call addChild on a singleton property RiskEvidenceSynthesis.lastReviewDate"); 4896 } else if (name.equals("effectivePeriod")) { 4897 this.effectivePeriod = new Period(); 4898 return this.effectivePeriod; 4899 } else if (name.equals("topic")) { 4900 return addTopic(); 4901 } else if (name.equals("author")) { 4902 return addAuthor(); 4903 } else if (name.equals("editor")) { 4904 return addEditor(); 4905 } else if (name.equals("reviewer")) { 4906 return addReviewer(); 4907 } else if (name.equals("endorser")) { 4908 return addEndorser(); 4909 } else if (name.equals("relatedArtifact")) { 4910 return addRelatedArtifact(); 4911 } else if (name.equals("synthesisType")) { 4912 this.synthesisType = new CodeableConcept(); 4913 return this.synthesisType; 4914 } else if (name.equals("studyType")) { 4915 this.studyType = new CodeableConcept(); 4916 return this.studyType; 4917 } else if (name.equals("population")) { 4918 this.population = new Reference(); 4919 return this.population; 4920 } else if (name.equals("exposure")) { 4921 this.exposure = new Reference(); 4922 return this.exposure; 4923 } else if (name.equals("outcome")) { 4924 this.outcome = new Reference(); 4925 return this.outcome; 4926 } else if (name.equals("sampleSize")) { 4927 this.sampleSize = new RiskEvidenceSynthesisSampleSizeComponent(); 4928 return this.sampleSize; 4929 } else if (name.equals("riskEstimate")) { 4930 this.riskEstimate = new RiskEvidenceSynthesisRiskEstimateComponent(); 4931 return this.riskEstimate; 4932 } else if (name.equals("certainty")) { 4933 return addCertainty(); 4934 } else 4935 return super.addChild(name); 4936 } 4937 4938 public String fhirType() { 4939 return "RiskEvidenceSynthesis"; 4940 4941 } 4942 4943 public RiskEvidenceSynthesis copy() { 4944 RiskEvidenceSynthesis dst = new RiskEvidenceSynthesis(); 4945 copyValues(dst); 4946 return dst; 4947 } 4948 4949 public void copyValues(RiskEvidenceSynthesis dst) { 4950 super.copyValues(dst); 4951 dst.url = url == null ? null : url.copy(); 4952 if (identifier != null) { 4953 dst.identifier = new ArrayList<Identifier>(); 4954 for (Identifier i : identifier) 4955 dst.identifier.add(i.copy()); 4956 } 4957 ; 4958 dst.version = version == null ? null : version.copy(); 4959 dst.name = name == null ? null : name.copy(); 4960 dst.title = title == null ? null : title.copy(); 4961 dst.status = status == null ? null : status.copy(); 4962 dst.date = date == null ? null : date.copy(); 4963 dst.publisher = publisher == null ? null : publisher.copy(); 4964 if (contact != null) { 4965 dst.contact = new ArrayList<ContactDetail>(); 4966 for (ContactDetail i : contact) 4967 dst.contact.add(i.copy()); 4968 } 4969 ; 4970 dst.description = description == null ? null : description.copy(); 4971 if (note != null) { 4972 dst.note = new ArrayList<Annotation>(); 4973 for (Annotation i : note) 4974 dst.note.add(i.copy()); 4975 } 4976 ; 4977 if (useContext != null) { 4978 dst.useContext = new ArrayList<UsageContext>(); 4979 for (UsageContext i : useContext) 4980 dst.useContext.add(i.copy()); 4981 } 4982 ; 4983 if (jurisdiction != null) { 4984 dst.jurisdiction = new ArrayList<CodeableConcept>(); 4985 for (CodeableConcept i : jurisdiction) 4986 dst.jurisdiction.add(i.copy()); 4987 } 4988 ; 4989 dst.copyright = copyright == null ? null : copyright.copy(); 4990 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 4991 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 4992 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 4993 if (topic != null) { 4994 dst.topic = new ArrayList<CodeableConcept>(); 4995 for (CodeableConcept i : topic) 4996 dst.topic.add(i.copy()); 4997 } 4998 ; 4999 if (author != null) { 5000 dst.author = new ArrayList<ContactDetail>(); 5001 for (ContactDetail i : author) 5002 dst.author.add(i.copy()); 5003 } 5004 ; 5005 if (editor != null) { 5006 dst.editor = new ArrayList<ContactDetail>(); 5007 for (ContactDetail i : editor) 5008 dst.editor.add(i.copy()); 5009 } 5010 ; 5011 if (reviewer != null) { 5012 dst.reviewer = new ArrayList<ContactDetail>(); 5013 for (ContactDetail i : reviewer) 5014 dst.reviewer.add(i.copy()); 5015 } 5016 ; 5017 if (endorser != null) { 5018 dst.endorser = new ArrayList<ContactDetail>(); 5019 for (ContactDetail i : endorser) 5020 dst.endorser.add(i.copy()); 5021 } 5022 ; 5023 if (relatedArtifact != null) { 5024 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 5025 for (RelatedArtifact i : relatedArtifact) 5026 dst.relatedArtifact.add(i.copy()); 5027 } 5028 ; 5029 dst.synthesisType = synthesisType == null ? null : synthesisType.copy(); 5030 dst.studyType = studyType == null ? null : studyType.copy(); 5031 dst.population = population == null ? null : population.copy(); 5032 dst.exposure = exposure == null ? null : exposure.copy(); 5033 dst.outcome = outcome == null ? null : outcome.copy(); 5034 dst.sampleSize = sampleSize == null ? null : sampleSize.copy(); 5035 dst.riskEstimate = riskEstimate == null ? null : riskEstimate.copy(); 5036 if (certainty != null) { 5037 dst.certainty = new ArrayList<RiskEvidenceSynthesisCertaintyComponent>(); 5038 for (RiskEvidenceSynthesisCertaintyComponent i : certainty) 5039 dst.certainty.add(i.copy()); 5040 } 5041 ; 5042 } 5043 5044 protected RiskEvidenceSynthesis typedCopy() { 5045 return copy(); 5046 } 5047 5048 @Override 5049 public boolean equalsDeep(Base other_) { 5050 if (!super.equalsDeep(other_)) 5051 return false; 5052 if (!(other_ instanceof RiskEvidenceSynthesis)) 5053 return false; 5054 RiskEvidenceSynthesis o = (RiskEvidenceSynthesis) other_; 5055 return compareDeep(identifier, o.identifier, true) && compareDeep(note, o.note, true) 5056 && compareDeep(copyright, o.copyright, true) && compareDeep(approvalDate, o.approvalDate, true) 5057 && compareDeep(lastReviewDate, o.lastReviewDate, true) && compareDeep(effectivePeriod, o.effectivePeriod, true) 5058 && compareDeep(topic, o.topic, true) && compareDeep(author, o.author, true) 5059 && compareDeep(editor, o.editor, true) && compareDeep(reviewer, o.reviewer, true) 5060 && compareDeep(endorser, o.endorser, true) && compareDeep(relatedArtifact, o.relatedArtifact, true) 5061 && compareDeep(synthesisType, o.synthesisType, true) && compareDeep(studyType, o.studyType, true) 5062 && compareDeep(population, o.population, true) && compareDeep(exposure, o.exposure, true) 5063 && compareDeep(outcome, o.outcome, true) && compareDeep(sampleSize, o.sampleSize, true) 5064 && compareDeep(riskEstimate, o.riskEstimate, true) && compareDeep(certainty, o.certainty, true); 5065 } 5066 5067 @Override 5068 public boolean equalsShallow(Base other_) { 5069 if (!super.equalsShallow(other_)) 5070 return false; 5071 if (!(other_ instanceof RiskEvidenceSynthesis)) 5072 return false; 5073 RiskEvidenceSynthesis o = (RiskEvidenceSynthesis) other_; 5074 return compareValues(copyright, o.copyright, true) && compareValues(approvalDate, o.approvalDate, true) 5075 && compareValues(lastReviewDate, o.lastReviewDate, true); 5076 } 5077 5078 public boolean isEmpty() { 5079 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, note, copyright, approvalDate, 5080 lastReviewDate, effectivePeriod, topic, author, editor, reviewer, endorser, relatedArtifact, synthesisType, 5081 studyType, population, exposure, outcome, sampleSize, riskEstimate, certainty); 5082 } 5083 5084 @Override 5085 public ResourceType getResourceType() { 5086 return ResourceType.RiskEvidenceSynthesis; 5087 } 5088 5089 /** 5090 * Search parameter: <b>date</b> 5091 * <p> 5092 * Description: <b>The risk evidence synthesis publication date</b><br> 5093 * Type: <b>date</b><br> 5094 * Path: <b>RiskEvidenceSynthesis.date</b><br> 5095 * </p> 5096 */ 5097 @SearchParamDefinition(name = "date", path = "RiskEvidenceSynthesis.date", description = "The risk evidence synthesis publication date", type = "date") 5098 public static final String SP_DATE = "date"; 5099 /** 5100 * <b>Fluent Client</b> search parameter constant for <b>date</b> 5101 * <p> 5102 * Description: <b>The risk evidence synthesis publication date</b><br> 5103 * Type: <b>date</b><br> 5104 * Path: <b>RiskEvidenceSynthesis.date</b><br> 5105 * </p> 5106 */ 5107 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 5108 SP_DATE); 5109 5110 /** 5111 * Search parameter: <b>identifier</b> 5112 * <p> 5113 * Description: <b>External identifier for the risk evidence synthesis</b><br> 5114 * Type: <b>token</b><br> 5115 * Path: <b>RiskEvidenceSynthesis.identifier</b><br> 5116 * </p> 5117 */ 5118 @SearchParamDefinition(name = "identifier", path = "RiskEvidenceSynthesis.identifier", description = "External identifier for the risk evidence synthesis", type = "token") 5119 public static final String SP_IDENTIFIER = "identifier"; 5120 /** 5121 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 5122 * <p> 5123 * Description: <b>External identifier for the risk evidence synthesis</b><br> 5124 * Type: <b>token</b><br> 5125 * Path: <b>RiskEvidenceSynthesis.identifier</b><br> 5126 * </p> 5127 */ 5128 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5129 SP_IDENTIFIER); 5130 5131 /** 5132 * Search parameter: <b>context-type-value</b> 5133 * <p> 5134 * Description: <b>A use context type and value assigned to the risk evidence 5135 * synthesis</b><br> 5136 * Type: <b>composite</b><br> 5137 * Path: <b></b><br> 5138 * </p> 5139 */ 5140 @SearchParamDefinition(name = "context-type-value", path = "RiskEvidenceSynthesis.useContext", description = "A use context type and value assigned to the risk evidence synthesis", type = "composite", compositeOf = { 5141 "context-type", "context" }) 5142 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 5143 /** 5144 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 5145 * <p> 5146 * Description: <b>A use context type and value assigned to the risk evidence 5147 * synthesis</b><br> 5148 * Type: <b>composite</b><br> 5149 * Path: <b></b><br> 5150 * </p> 5151 */ 5152 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 5153 SP_CONTEXT_TYPE_VALUE); 5154 5155 /** 5156 * Search parameter: <b>jurisdiction</b> 5157 * <p> 5158 * Description: <b>Intended jurisdiction for the risk evidence synthesis</b><br> 5159 * Type: <b>token</b><br> 5160 * Path: <b>RiskEvidenceSynthesis.jurisdiction</b><br> 5161 * </p> 5162 */ 5163 @SearchParamDefinition(name = "jurisdiction", path = "RiskEvidenceSynthesis.jurisdiction", description = "Intended jurisdiction for the risk evidence synthesis", type = "token") 5164 public static final String SP_JURISDICTION = "jurisdiction"; 5165 /** 5166 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 5167 * <p> 5168 * Description: <b>Intended jurisdiction for the risk evidence synthesis</b><br> 5169 * Type: <b>token</b><br> 5170 * Path: <b>RiskEvidenceSynthesis.jurisdiction</b><br> 5171 * </p> 5172 */ 5173 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5174 SP_JURISDICTION); 5175 5176 /** 5177 * Search parameter: <b>description</b> 5178 * <p> 5179 * Description: <b>The description of the risk evidence synthesis</b><br> 5180 * Type: <b>string</b><br> 5181 * Path: <b>RiskEvidenceSynthesis.description</b><br> 5182 * </p> 5183 */ 5184 @SearchParamDefinition(name = "description", path = "RiskEvidenceSynthesis.description", description = "The description of the risk evidence synthesis", type = "string") 5185 public static final String SP_DESCRIPTION = "description"; 5186 /** 5187 * <b>Fluent Client</b> search parameter constant for <b>description</b> 5188 * <p> 5189 * Description: <b>The description of the risk evidence synthesis</b><br> 5190 * Type: <b>string</b><br> 5191 * Path: <b>RiskEvidenceSynthesis.description</b><br> 5192 * </p> 5193 */ 5194 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 5195 SP_DESCRIPTION); 5196 5197 /** 5198 * Search parameter: <b>context-type</b> 5199 * <p> 5200 * Description: <b>A type of use context assigned to the risk evidence 5201 * synthesis</b><br> 5202 * Type: <b>token</b><br> 5203 * Path: <b>RiskEvidenceSynthesis.useContext.code</b><br> 5204 * </p> 5205 */ 5206 @SearchParamDefinition(name = "context-type", path = "RiskEvidenceSynthesis.useContext.code", description = "A type of use context assigned to the risk evidence synthesis", type = "token") 5207 public static final String SP_CONTEXT_TYPE = "context-type"; 5208 /** 5209 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 5210 * <p> 5211 * Description: <b>A type of use context assigned to the risk evidence 5212 * synthesis</b><br> 5213 * Type: <b>token</b><br> 5214 * Path: <b>RiskEvidenceSynthesis.useContext.code</b><br> 5215 * </p> 5216 */ 5217 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5218 SP_CONTEXT_TYPE); 5219 5220 /** 5221 * Search parameter: <b>title</b> 5222 * <p> 5223 * Description: <b>The human-friendly name of the risk evidence 5224 * synthesis</b><br> 5225 * Type: <b>string</b><br> 5226 * Path: <b>RiskEvidenceSynthesis.title</b><br> 5227 * </p> 5228 */ 5229 @SearchParamDefinition(name = "title", path = "RiskEvidenceSynthesis.title", description = "The human-friendly name of the risk evidence synthesis", type = "string") 5230 public static final String SP_TITLE = "title"; 5231 /** 5232 * <b>Fluent Client</b> search parameter constant for <b>title</b> 5233 * <p> 5234 * Description: <b>The human-friendly name of the risk evidence 5235 * synthesis</b><br> 5236 * Type: <b>string</b><br> 5237 * Path: <b>RiskEvidenceSynthesis.title</b><br> 5238 * </p> 5239 */ 5240 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 5241 SP_TITLE); 5242 5243 /** 5244 * Search parameter: <b>version</b> 5245 * <p> 5246 * Description: <b>The business version of the risk evidence synthesis</b><br> 5247 * Type: <b>token</b><br> 5248 * Path: <b>RiskEvidenceSynthesis.version</b><br> 5249 * </p> 5250 */ 5251 @SearchParamDefinition(name = "version", path = "RiskEvidenceSynthesis.version", description = "The business version of the risk evidence synthesis", type = "token") 5252 public static final String SP_VERSION = "version"; 5253 /** 5254 * <b>Fluent Client</b> search parameter constant for <b>version</b> 5255 * <p> 5256 * Description: <b>The business version of the risk evidence synthesis</b><br> 5257 * Type: <b>token</b><br> 5258 * Path: <b>RiskEvidenceSynthesis.version</b><br> 5259 * </p> 5260 */ 5261 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5262 SP_VERSION); 5263 5264 /** 5265 * Search parameter: <b>url</b> 5266 * <p> 5267 * Description: <b>The uri that identifies the risk evidence synthesis</b><br> 5268 * Type: <b>uri</b><br> 5269 * Path: <b>RiskEvidenceSynthesis.url</b><br> 5270 * </p> 5271 */ 5272 @SearchParamDefinition(name = "url", path = "RiskEvidenceSynthesis.url", description = "The uri that identifies the risk evidence synthesis", type = "uri") 5273 public static final String SP_URL = "url"; 5274 /** 5275 * <b>Fluent Client</b> search parameter constant for <b>url</b> 5276 * <p> 5277 * Description: <b>The uri that identifies the risk evidence synthesis</b><br> 5278 * Type: <b>uri</b><br> 5279 * Path: <b>RiskEvidenceSynthesis.url</b><br> 5280 * </p> 5281 */ 5282 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 5283 5284 /** 5285 * Search parameter: <b>context-quantity</b> 5286 * <p> 5287 * Description: <b>A quantity- or range-valued use context assigned to the risk 5288 * evidence synthesis</b><br> 5289 * Type: <b>quantity</b><br> 5290 * Path: <b>RiskEvidenceSynthesis.useContext.valueQuantity, 5291 * RiskEvidenceSynthesis.useContext.valueRange</b><br> 5292 * </p> 5293 */ 5294 @SearchParamDefinition(name = "context-quantity", path = "(RiskEvidenceSynthesis.useContext.value as Quantity) | (RiskEvidenceSynthesis.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the risk evidence synthesis", type = "quantity") 5295 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 5296 /** 5297 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 5298 * <p> 5299 * Description: <b>A quantity- or range-valued use context assigned to the risk 5300 * evidence synthesis</b><br> 5301 * Type: <b>quantity</b><br> 5302 * Path: <b>RiskEvidenceSynthesis.useContext.valueQuantity, 5303 * RiskEvidenceSynthesis.useContext.valueRange</b><br> 5304 * </p> 5305 */ 5306 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 5307 SP_CONTEXT_QUANTITY); 5308 5309 /** 5310 * Search parameter: <b>effective</b> 5311 * <p> 5312 * Description: <b>The time during which the risk evidence synthesis is intended 5313 * to be in use</b><br> 5314 * Type: <b>date</b><br> 5315 * Path: <b>RiskEvidenceSynthesis.effectivePeriod</b><br> 5316 * </p> 5317 */ 5318 @SearchParamDefinition(name = "effective", path = "RiskEvidenceSynthesis.effectivePeriod", description = "The time during which the risk evidence synthesis is intended to be in use", type = "date") 5319 public static final String SP_EFFECTIVE = "effective"; 5320 /** 5321 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 5322 * <p> 5323 * Description: <b>The time during which the risk evidence synthesis is intended 5324 * to be in use</b><br> 5325 * Type: <b>date</b><br> 5326 * Path: <b>RiskEvidenceSynthesis.effectivePeriod</b><br> 5327 * </p> 5328 */ 5329 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam( 5330 SP_EFFECTIVE); 5331 5332 /** 5333 * Search parameter: <b>name</b> 5334 * <p> 5335 * Description: <b>Computationally friendly name of the risk evidence 5336 * synthesis</b><br> 5337 * Type: <b>string</b><br> 5338 * Path: <b>RiskEvidenceSynthesis.name</b><br> 5339 * </p> 5340 */ 5341 @SearchParamDefinition(name = "name", path = "RiskEvidenceSynthesis.name", description = "Computationally friendly name of the risk evidence synthesis", type = "string") 5342 public static final String SP_NAME = "name"; 5343 /** 5344 * <b>Fluent Client</b> search parameter constant for <b>name</b> 5345 * <p> 5346 * Description: <b>Computationally friendly name of the risk evidence 5347 * synthesis</b><br> 5348 * Type: <b>string</b><br> 5349 * Path: <b>RiskEvidenceSynthesis.name</b><br> 5350 * </p> 5351 */ 5352 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 5353 SP_NAME); 5354 5355 /** 5356 * Search parameter: <b>context</b> 5357 * <p> 5358 * Description: <b>A use context assigned to the risk evidence synthesis</b><br> 5359 * Type: <b>token</b><br> 5360 * Path: <b>RiskEvidenceSynthesis.useContext.valueCodeableConcept</b><br> 5361 * </p> 5362 */ 5363 @SearchParamDefinition(name = "context", path = "(RiskEvidenceSynthesis.useContext.value as CodeableConcept)", description = "A use context assigned to the risk evidence synthesis", type = "token") 5364 public static final String SP_CONTEXT = "context"; 5365 /** 5366 * <b>Fluent Client</b> search parameter constant for <b>context</b> 5367 * <p> 5368 * Description: <b>A use context assigned to the risk evidence synthesis</b><br> 5369 * Type: <b>token</b><br> 5370 * Path: <b>RiskEvidenceSynthesis.useContext.valueCodeableConcept</b><br> 5371 * </p> 5372 */ 5373 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5374 SP_CONTEXT); 5375 5376 /** 5377 * Search parameter: <b>publisher</b> 5378 * <p> 5379 * Description: <b>Name of the publisher of the risk evidence synthesis</b><br> 5380 * Type: <b>string</b><br> 5381 * Path: <b>RiskEvidenceSynthesis.publisher</b><br> 5382 * </p> 5383 */ 5384 @SearchParamDefinition(name = "publisher", path = "RiskEvidenceSynthesis.publisher", description = "Name of the publisher of the risk evidence synthesis", type = "string") 5385 public static final String SP_PUBLISHER = "publisher"; 5386 /** 5387 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 5388 * <p> 5389 * Description: <b>Name of the publisher of the risk evidence synthesis</b><br> 5390 * Type: <b>string</b><br> 5391 * Path: <b>RiskEvidenceSynthesis.publisher</b><br> 5392 * </p> 5393 */ 5394 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 5395 SP_PUBLISHER); 5396 5397 /** 5398 * Search parameter: <b>context-type-quantity</b> 5399 * <p> 5400 * Description: <b>A use context type and quantity- or range-based value 5401 * assigned to the risk evidence synthesis</b><br> 5402 * Type: <b>composite</b><br> 5403 * Path: <b></b><br> 5404 * </p> 5405 */ 5406 @SearchParamDefinition(name = "context-type-quantity", path = "RiskEvidenceSynthesis.useContext", description = "A use context type and quantity- or range-based value assigned to the risk evidence synthesis", type = "composite", compositeOf = { 5407 "context-type", "context-quantity" }) 5408 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 5409 /** 5410 * <b>Fluent Client</b> search parameter constant for 5411 * <b>context-type-quantity</b> 5412 * <p> 5413 * Description: <b>A use context type and quantity- or range-based value 5414 * assigned to the risk evidence synthesis</b><br> 5415 * Type: <b>composite</b><br> 5416 * Path: <b></b><br> 5417 * </p> 5418 */ 5419 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 5420 SP_CONTEXT_TYPE_QUANTITY); 5421 5422 /** 5423 * Search parameter: <b>status</b> 5424 * <p> 5425 * Description: <b>The current status of the risk evidence synthesis</b><br> 5426 * Type: <b>token</b><br> 5427 * Path: <b>RiskEvidenceSynthesis.status</b><br> 5428 * </p> 5429 */ 5430 @SearchParamDefinition(name = "status", path = "RiskEvidenceSynthesis.status", description = "The current status of the risk evidence synthesis", type = "token") 5431 public static final String SP_STATUS = "status"; 5432 /** 5433 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5434 * <p> 5435 * Description: <b>The current status of the risk evidence synthesis</b><br> 5436 * Type: <b>token</b><br> 5437 * Path: <b>RiskEvidenceSynthesis.status</b><br> 5438 * </p> 5439 */ 5440 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5441 SP_STATUS); 5442 5443}