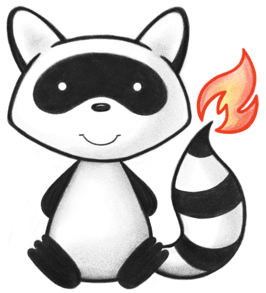
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.utilities.Utilities; 038 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.Description; 041import ca.uhn.fhir.model.api.annotation.ResourceDef; 042import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 043 044/** 045 * A container for slots of time that may be available for booking appointments. 046 */ 047@ResourceDef(name = "Schedule", profile = "http://hl7.org/fhir/StructureDefinition/Schedule") 048public class Schedule extends DomainResource { 049 050 /** 051 * External Ids for this item. 052 */ 053 @Child(name = "identifier", type = { 054 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 055 @Description(shortDefinition = "External Ids for this item", formalDefinition = "External Ids for this item.") 056 protected List<Identifier> identifier; 057 058 /** 059 * Whether this schedule record is in active use or should not be used (such as 060 * was entered in error). 061 */ 062 @Child(name = "active", type = { BooleanType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 063 @Description(shortDefinition = "Whether this schedule is in active use", formalDefinition = "Whether this schedule record is in active use or should not be used (such as was entered in error).") 064 protected BooleanType active; 065 066 /** 067 * A broad categorization of the service that is to be performed during this 068 * appointment. 069 */ 070 @Child(name = "serviceCategory", type = { 071 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 072 @Description(shortDefinition = "High-level category", formalDefinition = "A broad categorization of the service that is to be performed during this appointment.") 073 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-category") 074 protected List<CodeableConcept> serviceCategory; 075 076 /** 077 * The specific service that is to be performed during this appointment. 078 */ 079 @Child(name = "serviceType", type = { 080 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 081 @Description(shortDefinition = "Specific service", formalDefinition = "The specific service that is to be performed during this appointment.") 082 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-type") 083 protected List<CodeableConcept> serviceType; 084 085 /** 086 * The specialty of a practitioner that would be required to perform the service 087 * requested in this appointment. 088 */ 089 @Child(name = "specialty", type = { 090 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 091 @Description(shortDefinition = "Type of specialty needed", formalDefinition = "The specialty of a practitioner that would be required to perform the service requested in this appointment.") 092 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/c80-practice-codes") 093 protected List<CodeableConcept> specialty; 094 095 /** 096 * Slots that reference this schedule resource provide the availability details 097 * to these referenced resource(s). 098 */ 099 @Child(name = "actor", type = { Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, 100 Device.class, HealthcareService.class, 101 Location.class }, order = 5, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 102 @Description(shortDefinition = "Resource(s) that availability information is being provided for", formalDefinition = "Slots that reference this schedule resource provide the availability details to these referenced resource(s).") 103 protected List<Reference> actor; 104 /** 105 * The actual objects that are the target of the reference (Slots that reference 106 * this schedule resource provide the availability details to these referenced 107 * resource(s).) 108 */ 109 protected List<Resource> actorTarget; 110 111 /** 112 * The period of time that the slots that reference this Schedule resource cover 113 * (even if none exist). These cover the amount of time that an organization's 114 * planning horizon; the interval for which they are currently accepting 115 * appointments. This does not define a "template" for planning outside these 116 * dates. 117 */ 118 @Child(name = "planningHorizon", type = { 119 Period.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 120 @Description(shortDefinition = "Period of time covered by schedule", formalDefinition = "The period of time that the slots that reference this Schedule resource cover (even if none exist). These cover the amount of time that an organization's planning horizon; the interval for which they are currently accepting appointments. This does not define a \"template\" for planning outside these dates.") 121 protected Period planningHorizon; 122 123 /** 124 * Comments on the availability to describe any extended information. Such as 125 * custom constraints on the slots that may be associated. 126 */ 127 @Child(name = "comment", type = { StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 128 @Description(shortDefinition = "Comments on availability", formalDefinition = "Comments on the availability to describe any extended information. Such as custom constraints on the slots that may be associated.") 129 protected StringType comment; 130 131 private static final long serialVersionUID = 203182600L; 132 133 /** 134 * Constructor 135 */ 136 public Schedule() { 137 super(); 138 } 139 140 /** 141 * @return {@link #identifier} (External Ids for this item.) 142 */ 143 public List<Identifier> getIdentifier() { 144 if (this.identifier == null) 145 this.identifier = new ArrayList<Identifier>(); 146 return this.identifier; 147 } 148 149 /** 150 * @return Returns a reference to <code>this</code> for easy method chaining 151 */ 152 public Schedule setIdentifier(List<Identifier> theIdentifier) { 153 this.identifier = theIdentifier; 154 return this; 155 } 156 157 public boolean hasIdentifier() { 158 if (this.identifier == null) 159 return false; 160 for (Identifier item : this.identifier) 161 if (!item.isEmpty()) 162 return true; 163 return false; 164 } 165 166 public Identifier addIdentifier() { // 3 167 Identifier t = new Identifier(); 168 if (this.identifier == null) 169 this.identifier = new ArrayList<Identifier>(); 170 this.identifier.add(t); 171 return t; 172 } 173 174 public Schedule addIdentifier(Identifier t) { // 3 175 if (t == null) 176 return this; 177 if (this.identifier == null) 178 this.identifier = new ArrayList<Identifier>(); 179 this.identifier.add(t); 180 return this; 181 } 182 183 /** 184 * @return The first repetition of repeating field {@link #identifier}, creating 185 * it if it does not already exist 186 */ 187 public Identifier getIdentifierFirstRep() { 188 if (getIdentifier().isEmpty()) { 189 addIdentifier(); 190 } 191 return getIdentifier().get(0); 192 } 193 194 /** 195 * @return {@link #active} (Whether this schedule record is in active use or 196 * should not be used (such as was entered in error).). This is the 197 * underlying object with id, value and extensions. The accessor 198 * "getActive" gives direct access to the value 199 */ 200 public BooleanType getActiveElement() { 201 if (this.active == null) 202 if (Configuration.errorOnAutoCreate()) 203 throw new Error("Attempt to auto-create Schedule.active"); 204 else if (Configuration.doAutoCreate()) 205 this.active = new BooleanType(); // bb 206 return this.active; 207 } 208 209 public boolean hasActiveElement() { 210 return this.active != null && !this.active.isEmpty(); 211 } 212 213 public boolean hasActive() { 214 return this.active != null && !this.active.isEmpty(); 215 } 216 217 /** 218 * @param value {@link #active} (Whether this schedule record is in active use 219 * or should not be used (such as was entered in error).). This is 220 * the underlying object with id, value and extensions. The 221 * accessor "getActive" gives direct access to the value 222 */ 223 public Schedule setActiveElement(BooleanType value) { 224 this.active = value; 225 return this; 226 } 227 228 /** 229 * @return Whether this schedule record is in active use or should not be used 230 * (such as was entered in error). 231 */ 232 public boolean getActive() { 233 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 234 } 235 236 /** 237 * @param value Whether this schedule record is in active use or should not be 238 * used (such as was entered in error). 239 */ 240 public Schedule setActive(boolean value) { 241 if (this.active == null) 242 this.active = new BooleanType(); 243 this.active.setValue(value); 244 return this; 245 } 246 247 /** 248 * @return {@link #serviceCategory} (A broad categorization of the service that 249 * is to be performed during this appointment.) 250 */ 251 public List<CodeableConcept> getServiceCategory() { 252 if (this.serviceCategory == null) 253 this.serviceCategory = new ArrayList<CodeableConcept>(); 254 return this.serviceCategory; 255 } 256 257 /** 258 * @return Returns a reference to <code>this</code> for easy method chaining 259 */ 260 public Schedule setServiceCategory(List<CodeableConcept> theServiceCategory) { 261 this.serviceCategory = theServiceCategory; 262 return this; 263 } 264 265 public boolean hasServiceCategory() { 266 if (this.serviceCategory == null) 267 return false; 268 for (CodeableConcept item : this.serviceCategory) 269 if (!item.isEmpty()) 270 return true; 271 return false; 272 } 273 274 public CodeableConcept addServiceCategory() { // 3 275 CodeableConcept t = new CodeableConcept(); 276 if (this.serviceCategory == null) 277 this.serviceCategory = new ArrayList<CodeableConcept>(); 278 this.serviceCategory.add(t); 279 return t; 280 } 281 282 public Schedule addServiceCategory(CodeableConcept t) { // 3 283 if (t == null) 284 return this; 285 if (this.serviceCategory == null) 286 this.serviceCategory = new ArrayList<CodeableConcept>(); 287 this.serviceCategory.add(t); 288 return this; 289 } 290 291 /** 292 * @return The first repetition of repeating field {@link #serviceCategory}, 293 * creating it if it does not already exist 294 */ 295 public CodeableConcept getServiceCategoryFirstRep() { 296 if (getServiceCategory().isEmpty()) { 297 addServiceCategory(); 298 } 299 return getServiceCategory().get(0); 300 } 301 302 /** 303 * @return {@link #serviceType} (The specific service that is to be performed 304 * during this appointment.) 305 */ 306 public List<CodeableConcept> getServiceType() { 307 if (this.serviceType == null) 308 this.serviceType = new ArrayList<CodeableConcept>(); 309 return this.serviceType; 310 } 311 312 /** 313 * @return Returns a reference to <code>this</code> for easy method chaining 314 */ 315 public Schedule setServiceType(List<CodeableConcept> theServiceType) { 316 this.serviceType = theServiceType; 317 return this; 318 } 319 320 public boolean hasServiceType() { 321 if (this.serviceType == null) 322 return false; 323 for (CodeableConcept item : this.serviceType) 324 if (!item.isEmpty()) 325 return true; 326 return false; 327 } 328 329 public CodeableConcept addServiceType() { // 3 330 CodeableConcept t = new CodeableConcept(); 331 if (this.serviceType == null) 332 this.serviceType = new ArrayList<CodeableConcept>(); 333 this.serviceType.add(t); 334 return t; 335 } 336 337 public Schedule addServiceType(CodeableConcept t) { // 3 338 if (t == null) 339 return this; 340 if (this.serviceType == null) 341 this.serviceType = new ArrayList<CodeableConcept>(); 342 this.serviceType.add(t); 343 return this; 344 } 345 346 /** 347 * @return The first repetition of repeating field {@link #serviceType}, 348 * creating it if it does not already exist 349 */ 350 public CodeableConcept getServiceTypeFirstRep() { 351 if (getServiceType().isEmpty()) { 352 addServiceType(); 353 } 354 return getServiceType().get(0); 355 } 356 357 /** 358 * @return {@link #specialty} (The specialty of a practitioner that would be 359 * required to perform the service requested in this appointment.) 360 */ 361 public List<CodeableConcept> getSpecialty() { 362 if (this.specialty == null) 363 this.specialty = new ArrayList<CodeableConcept>(); 364 return this.specialty; 365 } 366 367 /** 368 * @return Returns a reference to <code>this</code> for easy method chaining 369 */ 370 public Schedule setSpecialty(List<CodeableConcept> theSpecialty) { 371 this.specialty = theSpecialty; 372 return this; 373 } 374 375 public boolean hasSpecialty() { 376 if (this.specialty == null) 377 return false; 378 for (CodeableConcept item : this.specialty) 379 if (!item.isEmpty()) 380 return true; 381 return false; 382 } 383 384 public CodeableConcept addSpecialty() { // 3 385 CodeableConcept t = new CodeableConcept(); 386 if (this.specialty == null) 387 this.specialty = new ArrayList<CodeableConcept>(); 388 this.specialty.add(t); 389 return t; 390 } 391 392 public Schedule addSpecialty(CodeableConcept t) { // 3 393 if (t == null) 394 return this; 395 if (this.specialty == null) 396 this.specialty = new ArrayList<CodeableConcept>(); 397 this.specialty.add(t); 398 return this; 399 } 400 401 /** 402 * @return The first repetition of repeating field {@link #specialty}, creating 403 * it if it does not already exist 404 */ 405 public CodeableConcept getSpecialtyFirstRep() { 406 if (getSpecialty().isEmpty()) { 407 addSpecialty(); 408 } 409 return getSpecialty().get(0); 410 } 411 412 /** 413 * @return {@link #actor} (Slots that reference this schedule resource provide 414 * the availability details to these referenced resource(s).) 415 */ 416 public List<Reference> getActor() { 417 if (this.actor == null) 418 this.actor = new ArrayList<Reference>(); 419 return this.actor; 420 } 421 422 /** 423 * @return Returns a reference to <code>this</code> for easy method chaining 424 */ 425 public Schedule setActor(List<Reference> theActor) { 426 this.actor = theActor; 427 return this; 428 } 429 430 public boolean hasActor() { 431 if (this.actor == null) 432 return false; 433 for (Reference item : this.actor) 434 if (!item.isEmpty()) 435 return true; 436 return false; 437 } 438 439 public Reference addActor() { // 3 440 Reference t = new Reference(); 441 if (this.actor == null) 442 this.actor = new ArrayList<Reference>(); 443 this.actor.add(t); 444 return t; 445 } 446 447 public Schedule addActor(Reference t) { // 3 448 if (t == null) 449 return this; 450 if (this.actor == null) 451 this.actor = new ArrayList<Reference>(); 452 this.actor.add(t); 453 return this; 454 } 455 456 /** 457 * @return The first repetition of repeating field {@link #actor}, creating it 458 * if it does not already exist 459 */ 460 public Reference getActorFirstRep() { 461 if (getActor().isEmpty()) { 462 addActor(); 463 } 464 return getActor().get(0); 465 } 466 467 /** 468 * @deprecated Use Reference#setResource(IBaseResource) instead 469 */ 470 @Deprecated 471 public List<Resource> getActorTarget() { 472 if (this.actorTarget == null) 473 this.actorTarget = new ArrayList<Resource>(); 474 return this.actorTarget; 475 } 476 477 /** 478 * @return {@link #planningHorizon} (The period of time that the slots that 479 * reference this Schedule resource cover (even if none exist). These 480 * cover the amount of time that an organization's planning horizon; the 481 * interval for which they are currently accepting appointments. This 482 * does not define a "template" for planning outside these dates.) 483 */ 484 public Period getPlanningHorizon() { 485 if (this.planningHorizon == null) 486 if (Configuration.errorOnAutoCreate()) 487 throw new Error("Attempt to auto-create Schedule.planningHorizon"); 488 else if (Configuration.doAutoCreate()) 489 this.planningHorizon = new Period(); // cc 490 return this.planningHorizon; 491 } 492 493 public boolean hasPlanningHorizon() { 494 return this.planningHorizon != null && !this.planningHorizon.isEmpty(); 495 } 496 497 /** 498 * @param value {@link #planningHorizon} (The period of time that the slots that 499 * reference this Schedule resource cover (even if none exist). 500 * These cover the amount of time that an organization's planning 501 * horizon; the interval for which they are currently accepting 502 * appointments. This does not define a "template" for planning 503 * outside these dates.) 504 */ 505 public Schedule setPlanningHorizon(Period value) { 506 this.planningHorizon = value; 507 return this; 508 } 509 510 /** 511 * @return {@link #comment} (Comments on the availability to describe any 512 * extended information. Such as custom constraints on the slots that 513 * may be associated.). This is the underlying object with id, value and 514 * extensions. The accessor "getComment" gives direct access to the 515 * value 516 */ 517 public StringType getCommentElement() { 518 if (this.comment == null) 519 if (Configuration.errorOnAutoCreate()) 520 throw new Error("Attempt to auto-create Schedule.comment"); 521 else if (Configuration.doAutoCreate()) 522 this.comment = new StringType(); // bb 523 return this.comment; 524 } 525 526 public boolean hasCommentElement() { 527 return this.comment != null && !this.comment.isEmpty(); 528 } 529 530 public boolean hasComment() { 531 return this.comment != null && !this.comment.isEmpty(); 532 } 533 534 /** 535 * @param value {@link #comment} (Comments on the availability to describe any 536 * extended information. Such as custom constraints on the slots 537 * that may be associated.). This is the underlying object with id, 538 * value and extensions. The accessor "getComment" gives direct 539 * access to the value 540 */ 541 public Schedule setCommentElement(StringType value) { 542 this.comment = value; 543 return this; 544 } 545 546 /** 547 * @return Comments on the availability to describe any extended information. 548 * Such as custom constraints on the slots that may be associated. 549 */ 550 public String getComment() { 551 return this.comment == null ? null : this.comment.getValue(); 552 } 553 554 /** 555 * @param value Comments on the availability to describe any extended 556 * information. Such as custom constraints on the slots that may be 557 * associated. 558 */ 559 public Schedule setComment(String value) { 560 if (Utilities.noString(value)) 561 this.comment = null; 562 else { 563 if (this.comment == null) 564 this.comment = new StringType(); 565 this.comment.setValue(value); 566 } 567 return this; 568 } 569 570 protected void listChildren(List<Property> children) { 571 super.listChildren(children); 572 children.add(new Property("identifier", "Identifier", "External Ids for this item.", 0, java.lang.Integer.MAX_VALUE, 573 identifier)); 574 children.add(new Property("active", "boolean", 575 "Whether this schedule record is in active use or should not be used (such as was entered in error).", 0, 1, 576 active)); 577 children.add(new Property("serviceCategory", "CodeableConcept", 578 "A broad categorization of the service that is to be performed during this appointment.", 0, 579 java.lang.Integer.MAX_VALUE, serviceCategory)); 580 children.add(new Property("serviceType", "CodeableConcept", 581 "The specific service that is to be performed during this appointment.", 0, java.lang.Integer.MAX_VALUE, 582 serviceType)); 583 children.add(new Property("specialty", "CodeableConcept", 584 "The specialty of a practitioner that would be required to perform the service requested in this appointment.", 585 0, java.lang.Integer.MAX_VALUE, specialty)); 586 children.add(new Property("actor", 587 "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Device|HealthcareService|Location)", 588 "Slots that reference this schedule resource provide the availability details to these referenced resource(s).", 589 0, java.lang.Integer.MAX_VALUE, actor)); 590 children.add(new Property("planningHorizon", "Period", 591 "The period of time that the slots that reference this Schedule resource cover (even if none exist). These cover the amount of time that an organization's planning horizon; the interval for which they are currently accepting appointments. This does not define a \"template\" for planning outside these dates.", 592 0, 1, planningHorizon)); 593 children.add(new Property("comment", "string", 594 "Comments on the availability to describe any extended information. Such as custom constraints on the slots that may be associated.", 595 0, 1, comment)); 596 } 597 598 @Override 599 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 600 switch (_hash) { 601 case -1618432855: 602 /* identifier */ return new Property("identifier", "Identifier", "External Ids for this item.", 0, 603 java.lang.Integer.MAX_VALUE, identifier); 604 case -1422950650: 605 /* active */ return new Property("active", "boolean", 606 "Whether this schedule record is in active use or should not be used (such as was entered in error).", 0, 1, 607 active); 608 case 1281188563: 609 /* serviceCategory */ return new Property("serviceCategory", "CodeableConcept", 610 "A broad categorization of the service that is to be performed during this appointment.", 0, 611 java.lang.Integer.MAX_VALUE, serviceCategory); 612 case -1928370289: 613 /* serviceType */ return new Property("serviceType", "CodeableConcept", 614 "The specific service that is to be performed during this appointment.", 0, java.lang.Integer.MAX_VALUE, 615 serviceType); 616 case -1694759682: 617 /* specialty */ return new Property("specialty", "CodeableConcept", 618 "The specialty of a practitioner that would be required to perform the service requested in this appointment.", 619 0, java.lang.Integer.MAX_VALUE, specialty); 620 case 92645877: 621 /* actor */ return new Property("actor", 622 "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Device|HealthcareService|Location)", 623 "Slots that reference this schedule resource provide the availability details to these referenced resource(s).", 624 0, java.lang.Integer.MAX_VALUE, actor); 625 case -1718507650: 626 /* planningHorizon */ return new Property("planningHorizon", "Period", 627 "The period of time that the slots that reference this Schedule resource cover (even if none exist). These cover the amount of time that an organization's planning horizon; the interval for which they are currently accepting appointments. This does not define a \"template\" for planning outside these dates.", 628 0, 1, planningHorizon); 629 case 950398559: 630 /* comment */ return new Property("comment", "string", 631 "Comments on the availability to describe any extended information. Such as custom constraints on the slots that may be associated.", 632 0, 1, comment); 633 default: 634 return super.getNamedProperty(_hash, _name, _checkValid); 635 } 636 637 } 638 639 @Override 640 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 641 switch (hash) { 642 case -1618432855: 643 /* identifier */ return this.identifier == null ? new Base[0] 644 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 645 case -1422950650: 646 /* active */ return this.active == null ? new Base[0] : new Base[] { this.active }; // BooleanType 647 case 1281188563: 648 /* serviceCategory */ return this.serviceCategory == null ? new Base[0] 649 : this.serviceCategory.toArray(new Base[this.serviceCategory.size()]); // CodeableConcept 650 case -1928370289: 651 /* serviceType */ return this.serviceType == null ? new Base[0] 652 : this.serviceType.toArray(new Base[this.serviceType.size()]); // CodeableConcept 653 case -1694759682: 654 /* specialty */ return this.specialty == null ? new Base[0] 655 : this.specialty.toArray(new Base[this.specialty.size()]); // CodeableConcept 656 case 92645877: 657 /* actor */ return this.actor == null ? new Base[0] : this.actor.toArray(new Base[this.actor.size()]); // Reference 658 case -1718507650: 659 /* planningHorizon */ return this.planningHorizon == null ? new Base[0] : new Base[] { this.planningHorizon }; // Period 660 case 950398559: 661 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // StringType 662 default: 663 return super.getProperty(hash, name, checkValid); 664 } 665 666 } 667 668 @Override 669 public Base setProperty(int hash, String name, Base value) throws FHIRException { 670 switch (hash) { 671 case -1618432855: // identifier 672 this.getIdentifier().add(castToIdentifier(value)); // Identifier 673 return value; 674 case -1422950650: // active 675 this.active = castToBoolean(value); // BooleanType 676 return value; 677 case 1281188563: // serviceCategory 678 this.getServiceCategory().add(castToCodeableConcept(value)); // CodeableConcept 679 return value; 680 case -1928370289: // serviceType 681 this.getServiceType().add(castToCodeableConcept(value)); // CodeableConcept 682 return value; 683 case -1694759682: // specialty 684 this.getSpecialty().add(castToCodeableConcept(value)); // CodeableConcept 685 return value; 686 case 92645877: // actor 687 this.getActor().add(castToReference(value)); // Reference 688 return value; 689 case -1718507650: // planningHorizon 690 this.planningHorizon = castToPeriod(value); // Period 691 return value; 692 case 950398559: // comment 693 this.comment = castToString(value); // StringType 694 return value; 695 default: 696 return super.setProperty(hash, name, value); 697 } 698 699 } 700 701 @Override 702 public Base setProperty(String name, Base value) throws FHIRException { 703 if (name.equals("identifier")) { 704 this.getIdentifier().add(castToIdentifier(value)); 705 } else if (name.equals("active")) { 706 this.active = castToBoolean(value); // BooleanType 707 } else if (name.equals("serviceCategory")) { 708 this.getServiceCategory().add(castToCodeableConcept(value)); 709 } else if (name.equals("serviceType")) { 710 this.getServiceType().add(castToCodeableConcept(value)); 711 } else if (name.equals("specialty")) { 712 this.getSpecialty().add(castToCodeableConcept(value)); 713 } else if (name.equals("actor")) { 714 this.getActor().add(castToReference(value)); 715 } else if (name.equals("planningHorizon")) { 716 this.planningHorizon = castToPeriod(value); // Period 717 } else if (name.equals("comment")) { 718 this.comment = castToString(value); // StringType 719 } else 720 return super.setProperty(name, value); 721 return value; 722 } 723 724 @Override 725 public void removeChild(String name, Base value) throws FHIRException { 726 if (name.equals("identifier")) { 727 this.getIdentifier().remove(castToIdentifier(value)); 728 } else if (name.equals("active")) { 729 this.active = null; 730 } else if (name.equals("serviceCategory")) { 731 this.getServiceCategory().remove(castToCodeableConcept(value)); 732 } else if (name.equals("serviceType")) { 733 this.getServiceType().remove(castToCodeableConcept(value)); 734 } else if (name.equals("specialty")) { 735 this.getSpecialty().remove(castToCodeableConcept(value)); 736 } else if (name.equals("actor")) { 737 this.getActor().remove(castToReference(value)); 738 } else if (name.equals("planningHorizon")) { 739 this.planningHorizon = null; 740 } else if (name.equals("comment")) { 741 this.comment = null; 742 } else 743 super.removeChild(name, value); 744 745 } 746 747 @Override 748 public Base makeProperty(int hash, String name) throws FHIRException { 749 switch (hash) { 750 case -1618432855: 751 return addIdentifier(); 752 case -1422950650: 753 return getActiveElement(); 754 case 1281188563: 755 return addServiceCategory(); 756 case -1928370289: 757 return addServiceType(); 758 case -1694759682: 759 return addSpecialty(); 760 case 92645877: 761 return addActor(); 762 case -1718507650: 763 return getPlanningHorizon(); 764 case 950398559: 765 return getCommentElement(); 766 default: 767 return super.makeProperty(hash, name); 768 } 769 770 } 771 772 @Override 773 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 774 switch (hash) { 775 case -1618432855: 776 /* identifier */ return new String[] { "Identifier" }; 777 case -1422950650: 778 /* active */ return new String[] { "boolean" }; 779 case 1281188563: 780 /* serviceCategory */ return new String[] { "CodeableConcept" }; 781 case -1928370289: 782 /* serviceType */ return new String[] { "CodeableConcept" }; 783 case -1694759682: 784 /* specialty */ return new String[] { "CodeableConcept" }; 785 case 92645877: 786 /* actor */ return new String[] { "Reference" }; 787 case -1718507650: 788 /* planningHorizon */ return new String[] { "Period" }; 789 case 950398559: 790 /* comment */ return new String[] { "string" }; 791 default: 792 return super.getTypesForProperty(hash, name); 793 } 794 795 } 796 797 @Override 798 public Base addChild(String name) throws FHIRException { 799 if (name.equals("identifier")) { 800 return addIdentifier(); 801 } else if (name.equals("active")) { 802 throw new FHIRException("Cannot call addChild on a singleton property Schedule.active"); 803 } else if (name.equals("serviceCategory")) { 804 return addServiceCategory(); 805 } else if (name.equals("serviceType")) { 806 return addServiceType(); 807 } else if (name.equals("specialty")) { 808 return addSpecialty(); 809 } else if (name.equals("actor")) { 810 return addActor(); 811 } else if (name.equals("planningHorizon")) { 812 this.planningHorizon = new Period(); 813 return this.planningHorizon; 814 } else if (name.equals("comment")) { 815 throw new FHIRException("Cannot call addChild on a singleton property Schedule.comment"); 816 } else 817 return super.addChild(name); 818 } 819 820 public String fhirType() { 821 return "Schedule"; 822 823 } 824 825 public Schedule copy() { 826 Schedule dst = new Schedule(); 827 copyValues(dst); 828 return dst; 829 } 830 831 public void copyValues(Schedule dst) { 832 super.copyValues(dst); 833 if (identifier != null) { 834 dst.identifier = new ArrayList<Identifier>(); 835 for (Identifier i : identifier) 836 dst.identifier.add(i.copy()); 837 } 838 ; 839 dst.active = active == null ? null : active.copy(); 840 if (serviceCategory != null) { 841 dst.serviceCategory = new ArrayList<CodeableConcept>(); 842 for (CodeableConcept i : serviceCategory) 843 dst.serviceCategory.add(i.copy()); 844 } 845 ; 846 if (serviceType != null) { 847 dst.serviceType = new ArrayList<CodeableConcept>(); 848 for (CodeableConcept i : serviceType) 849 dst.serviceType.add(i.copy()); 850 } 851 ; 852 if (specialty != null) { 853 dst.specialty = new ArrayList<CodeableConcept>(); 854 for (CodeableConcept i : specialty) 855 dst.specialty.add(i.copy()); 856 } 857 ; 858 if (actor != null) { 859 dst.actor = new ArrayList<Reference>(); 860 for (Reference i : actor) 861 dst.actor.add(i.copy()); 862 } 863 ; 864 dst.planningHorizon = planningHorizon == null ? null : planningHorizon.copy(); 865 dst.comment = comment == null ? null : comment.copy(); 866 } 867 868 protected Schedule typedCopy() { 869 return copy(); 870 } 871 872 @Override 873 public boolean equalsDeep(Base other_) { 874 if (!super.equalsDeep(other_)) 875 return false; 876 if (!(other_ instanceof Schedule)) 877 return false; 878 Schedule o = (Schedule) other_; 879 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) 880 && compareDeep(serviceCategory, o.serviceCategory, true) && compareDeep(serviceType, o.serviceType, true) 881 && compareDeep(specialty, o.specialty, true) && compareDeep(actor, o.actor, true) 882 && compareDeep(planningHorizon, o.planningHorizon, true) && compareDeep(comment, o.comment, true); 883 } 884 885 @Override 886 public boolean equalsShallow(Base other_) { 887 if (!super.equalsShallow(other_)) 888 return false; 889 if (!(other_ instanceof Schedule)) 890 return false; 891 Schedule o = (Schedule) other_; 892 return compareValues(active, o.active, true) && compareValues(comment, o.comment, true); 893 } 894 895 public boolean isEmpty() { 896 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, serviceCategory, serviceType, 897 specialty, actor, planningHorizon, comment); 898 } 899 900 @Override 901 public ResourceType getResourceType() { 902 return ResourceType.Schedule; 903 } 904 905 /** 906 * Search parameter: <b>actor</b> 907 * <p> 908 * Description: <b>The individual(HealthcareService, Practitioner, Location, 909 * ...) to find a Schedule for</b><br> 910 * Type: <b>reference</b><br> 911 * Path: <b>Schedule.actor</b><br> 912 * </p> 913 */ 914 @SearchParamDefinition(name = "actor", path = "Schedule.actor", description = "The individual(HealthcareService, Practitioner, Location, ...) to find a Schedule for", type = "reference", providesMembershipIn = { 915 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 916 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 917 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 918 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, 919 HealthcareService.class, Location.class, Patient.class, Practitioner.class, PractitionerRole.class, 920 RelatedPerson.class }) 921 public static final String SP_ACTOR = "actor"; 922 /** 923 * <b>Fluent Client</b> search parameter constant for <b>actor</b> 924 * <p> 925 * Description: <b>The individual(HealthcareService, Practitioner, Location, 926 * ...) to find a Schedule for</b><br> 927 * Type: <b>reference</b><br> 928 * Path: <b>Schedule.actor</b><br> 929 * </p> 930 */ 931 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 932 SP_ACTOR); 933 934 /** 935 * Constant for fluent queries to be used to add include statements. Specifies 936 * the path value of "<b>Schedule:actor</b>". 937 */ 938 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACTOR = new ca.uhn.fhir.model.api.Include("Schedule:actor") 939 .toLocked(); 940 941 /** 942 * Search parameter: <b>date</b> 943 * <p> 944 * Description: <b>Search for Schedule resources that have a period that 945 * contains this date specified</b><br> 946 * Type: <b>date</b><br> 947 * Path: <b>Schedule.planningHorizon</b><br> 948 * </p> 949 */ 950 @SearchParamDefinition(name = "date", path = "Schedule.planningHorizon", description = "Search for Schedule resources that have a period that contains this date specified", type = "date") 951 public static final String SP_DATE = "date"; 952 /** 953 * <b>Fluent Client</b> search parameter constant for <b>date</b> 954 * <p> 955 * Description: <b>Search for Schedule resources that have a period that 956 * contains this date specified</b><br> 957 * Type: <b>date</b><br> 958 * Path: <b>Schedule.planningHorizon</b><br> 959 * </p> 960 */ 961 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 962 SP_DATE); 963 964 /** 965 * Search parameter: <b>identifier</b> 966 * <p> 967 * Description: <b>A Schedule Identifier</b><br> 968 * Type: <b>token</b><br> 969 * Path: <b>Schedule.identifier</b><br> 970 * </p> 971 */ 972 @SearchParamDefinition(name = "identifier", path = "Schedule.identifier", description = "A Schedule Identifier", type = "token") 973 public static final String SP_IDENTIFIER = "identifier"; 974 /** 975 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 976 * <p> 977 * Description: <b>A Schedule Identifier</b><br> 978 * Type: <b>token</b><br> 979 * Path: <b>Schedule.identifier</b><br> 980 * </p> 981 */ 982 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 983 SP_IDENTIFIER); 984 985 /** 986 * Search parameter: <b>specialty</b> 987 * <p> 988 * Description: <b>Type of specialty needed</b><br> 989 * Type: <b>token</b><br> 990 * Path: <b>Schedule.specialty</b><br> 991 * </p> 992 */ 993 @SearchParamDefinition(name = "specialty", path = "Schedule.specialty", description = "Type of specialty needed", type = "token") 994 public static final String SP_SPECIALTY = "specialty"; 995 /** 996 * <b>Fluent Client</b> search parameter constant for <b>specialty</b> 997 * <p> 998 * Description: <b>Type of specialty needed</b><br> 999 * Type: <b>token</b><br> 1000 * Path: <b>Schedule.specialty</b><br> 1001 * </p> 1002 */ 1003 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SPECIALTY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1004 SP_SPECIALTY); 1005 1006 /** 1007 * Search parameter: <b>service-category</b> 1008 * <p> 1009 * Description: <b>High-level category</b><br> 1010 * Type: <b>token</b><br> 1011 * Path: <b>Schedule.serviceCategory</b><br> 1012 * </p> 1013 */ 1014 @SearchParamDefinition(name = "service-category", path = "Schedule.serviceCategory", description = "High-level category", type = "token") 1015 public static final String SP_SERVICE_CATEGORY = "service-category"; 1016 /** 1017 * <b>Fluent Client</b> search parameter constant for <b>service-category</b> 1018 * <p> 1019 * Description: <b>High-level category</b><br> 1020 * Type: <b>token</b><br> 1021 * Path: <b>Schedule.serviceCategory</b><br> 1022 * </p> 1023 */ 1024 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERVICE_CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1025 SP_SERVICE_CATEGORY); 1026 1027 /** 1028 * Search parameter: <b>service-type</b> 1029 * <p> 1030 * Description: <b>The type of appointments that can be booked into associated 1031 * slot(s)</b><br> 1032 * Type: <b>token</b><br> 1033 * Path: <b>Schedule.serviceType</b><br> 1034 * </p> 1035 */ 1036 @SearchParamDefinition(name = "service-type", path = "Schedule.serviceType", description = "The type of appointments that can be booked into associated slot(s)", type = "token") 1037 public static final String SP_SERVICE_TYPE = "service-type"; 1038 /** 1039 * <b>Fluent Client</b> search parameter constant for <b>service-type</b> 1040 * <p> 1041 * Description: <b>The type of appointments that can be booked into associated 1042 * slot(s)</b><br> 1043 * Type: <b>token</b><br> 1044 * Path: <b>Schedule.serviceType</b><br> 1045 * </p> 1046 */ 1047 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERVICE_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1048 SP_SERVICE_TYPE); 1049 1050 /** 1051 * Search parameter: <b>active</b> 1052 * <p> 1053 * Description: <b>Is the schedule in active use</b><br> 1054 * Type: <b>token</b><br> 1055 * Path: <b>Schedule.active</b><br> 1056 * </p> 1057 */ 1058 @SearchParamDefinition(name = "active", path = "Schedule.active", description = "Is the schedule in active use", type = "token") 1059 public static final String SP_ACTIVE = "active"; 1060 /** 1061 * <b>Fluent Client</b> search parameter constant for <b>active</b> 1062 * <p> 1063 * Description: <b>Is the schedule in active use</b><br> 1064 * Type: <b>token</b><br> 1065 * Path: <b>Schedule.active</b><br> 1066 * </p> 1067 */ 1068 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1069 SP_ACTIVE); 1070 1071}