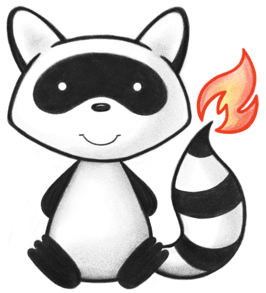
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.r4.model.Enumerations.SearchParamType; 042import org.hl7.fhir.r4.model.Enumerations.SearchParamTypeEnumFactory; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.ResourceDef; 050import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 051 052/** 053 * A search parameter that defines a named search item that can be used to 054 * search/filter on a resource. 055 */ 056@ResourceDef(name = "SearchParameter", profile = "http://hl7.org/fhir/StructureDefinition/SearchParameter") 057@ChildOrder(names = { "url", "version", "name", "derivedFrom", "status", "experimental", "date", "publisher", "contact", 058 "description", "useContext", "jurisdiction", "purpose", "code", "base", "type", "expression", "xpath", "xpathUsage", 059 "target", "multipleOr", "multipleAnd", "comparator", "modifier", "chain", "component" }) 060public class SearchParameter extends MetadataResource { 061 062 public enum XPathUsageType { 063 /** 064 * The search parameter is derived directly from the selected nodes based on the 065 * type definitions. 066 */ 067 NORMAL, 068 /** 069 * The search parameter is derived by a phonetic transform from the selected 070 * nodes. 071 */ 072 PHONETIC, 073 /** 074 * The search parameter is based on a spatial transform of the selected nodes. 075 */ 076 NEARBY, 077 /** 078 * The search parameter is based on a spatial transform of the selected nodes, 079 * using physical distance from the middle. 080 */ 081 DISTANCE, 082 /** 083 * The interpretation of the xpath statement is unknown (and can't be 084 * automated). 085 */ 086 OTHER, 087 /** 088 * added to help the parsers with the generic types 089 */ 090 NULL; 091 092 public static XPathUsageType fromCode(String codeString) throws FHIRException { 093 if (codeString == null || "".equals(codeString)) 094 return null; 095 if ("normal".equals(codeString)) 096 return NORMAL; 097 if ("phonetic".equals(codeString)) 098 return PHONETIC; 099 if ("nearby".equals(codeString)) 100 return NEARBY; 101 if ("distance".equals(codeString)) 102 return DISTANCE; 103 if ("other".equals(codeString)) 104 return OTHER; 105 if (Configuration.isAcceptInvalidEnums()) 106 return null; 107 else 108 throw new FHIRException("Unknown XPathUsageType code '" + codeString + "'"); 109 } 110 111 public String toCode() { 112 switch (this) { 113 case NORMAL: 114 return "normal"; 115 case PHONETIC: 116 return "phonetic"; 117 case NEARBY: 118 return "nearby"; 119 case DISTANCE: 120 return "distance"; 121 case OTHER: 122 return "other"; 123 case NULL: 124 return null; 125 default: 126 return "?"; 127 } 128 } 129 130 public String getSystem() { 131 switch (this) { 132 case NORMAL: 133 return "http://hl7.org/fhir/search-xpath-usage"; 134 case PHONETIC: 135 return "http://hl7.org/fhir/search-xpath-usage"; 136 case NEARBY: 137 return "http://hl7.org/fhir/search-xpath-usage"; 138 case DISTANCE: 139 return "http://hl7.org/fhir/search-xpath-usage"; 140 case OTHER: 141 return "http://hl7.org/fhir/search-xpath-usage"; 142 case NULL: 143 return null; 144 default: 145 return "?"; 146 } 147 } 148 149 public String getDefinition() { 150 switch (this) { 151 case NORMAL: 152 return "The search parameter is derived directly from the selected nodes based on the type definitions."; 153 case PHONETIC: 154 return "The search parameter is derived by a phonetic transform from the selected nodes."; 155 case NEARBY: 156 return "The search parameter is based on a spatial transform of the selected nodes."; 157 case DISTANCE: 158 return "The search parameter is based on a spatial transform of the selected nodes, using physical distance from the middle."; 159 case OTHER: 160 return "The interpretation of the xpath statement is unknown (and can't be automated)."; 161 case NULL: 162 return null; 163 default: 164 return "?"; 165 } 166 } 167 168 public String getDisplay() { 169 switch (this) { 170 case NORMAL: 171 return "Normal"; 172 case PHONETIC: 173 return "Phonetic"; 174 case NEARBY: 175 return "Nearby"; 176 case DISTANCE: 177 return "Distance"; 178 case OTHER: 179 return "Other"; 180 case NULL: 181 return null; 182 default: 183 return "?"; 184 } 185 } 186 } 187 188 public static class XPathUsageTypeEnumFactory implements EnumFactory<XPathUsageType> { 189 public XPathUsageType fromCode(String codeString) throws IllegalArgumentException { 190 if (codeString == null || "".equals(codeString)) 191 if (codeString == null || "".equals(codeString)) 192 return null; 193 if ("normal".equals(codeString)) 194 return XPathUsageType.NORMAL; 195 if ("phonetic".equals(codeString)) 196 return XPathUsageType.PHONETIC; 197 if ("nearby".equals(codeString)) 198 return XPathUsageType.NEARBY; 199 if ("distance".equals(codeString)) 200 return XPathUsageType.DISTANCE; 201 if ("other".equals(codeString)) 202 return XPathUsageType.OTHER; 203 throw new IllegalArgumentException("Unknown XPathUsageType code '" + codeString + "'"); 204 } 205 206 public Enumeration<XPathUsageType> fromType(PrimitiveType<?> code) throws FHIRException { 207 if (code == null) 208 return null; 209 if (code.isEmpty()) 210 return new Enumeration<XPathUsageType>(this, XPathUsageType.NULL, code); 211 String codeString = code.asStringValue(); 212 if (codeString == null || "".equals(codeString)) 213 return new Enumeration<XPathUsageType>(this, XPathUsageType.NULL, code); 214 if ("normal".equals(codeString)) 215 return new Enumeration<XPathUsageType>(this, XPathUsageType.NORMAL, code); 216 if ("phonetic".equals(codeString)) 217 return new Enumeration<XPathUsageType>(this, XPathUsageType.PHONETIC, code); 218 if ("nearby".equals(codeString)) 219 return new Enumeration<XPathUsageType>(this, XPathUsageType.NEARBY, code); 220 if ("distance".equals(codeString)) 221 return new Enumeration<XPathUsageType>(this, XPathUsageType.DISTANCE, code); 222 if ("other".equals(codeString)) 223 return new Enumeration<XPathUsageType>(this, XPathUsageType.OTHER, code); 224 throw new FHIRException("Unknown XPathUsageType code '" + codeString + "'"); 225 } 226 227 public String toCode(XPathUsageType code) { 228 if (code == XPathUsageType.NORMAL) 229 return "normal"; 230 if (code == XPathUsageType.PHONETIC) 231 return "phonetic"; 232 if (code == XPathUsageType.NEARBY) 233 return "nearby"; 234 if (code == XPathUsageType.DISTANCE) 235 return "distance"; 236 if (code == XPathUsageType.OTHER) 237 return "other"; 238 return "?"; 239 } 240 241 public String toSystem(XPathUsageType code) { 242 return code.getSystem(); 243 } 244 } 245 246 public enum SearchComparator { 247 /** 248 * the value for the parameter in the resource is equal to the provided value. 249 */ 250 EQ, 251 /** 252 * the value for the parameter in the resource is not equal to the provided 253 * value. 254 */ 255 NE, 256 /** 257 * the value for the parameter in the resource is greater than the provided 258 * value. 259 */ 260 GT, 261 /** 262 * the value for the parameter in the resource is less than the provided value. 263 */ 264 LT, 265 /** 266 * the value for the parameter in the resource is greater or equal to the 267 * provided value. 268 */ 269 GE, 270 /** 271 * the value for the parameter in the resource is less or equal to the provided 272 * value. 273 */ 274 LE, 275 /** 276 * the value for the parameter in the resource starts after the provided value. 277 */ 278 SA, 279 /** 280 * the value for the parameter in the resource ends before the provided value. 281 */ 282 EB, 283 /** 284 * the value for the parameter in the resource is approximately the same to the 285 * provided value. 286 */ 287 AP, 288 /** 289 * added to help the parsers with the generic types 290 */ 291 NULL; 292 293 public static SearchComparator fromCode(String codeString) throws FHIRException { 294 if (codeString == null || "".equals(codeString)) 295 return null; 296 if ("eq".equals(codeString)) 297 return EQ; 298 if ("ne".equals(codeString)) 299 return NE; 300 if ("gt".equals(codeString)) 301 return GT; 302 if ("lt".equals(codeString)) 303 return LT; 304 if ("ge".equals(codeString)) 305 return GE; 306 if ("le".equals(codeString)) 307 return LE; 308 if ("sa".equals(codeString)) 309 return SA; 310 if ("eb".equals(codeString)) 311 return EB; 312 if ("ap".equals(codeString)) 313 return AP; 314 if (Configuration.isAcceptInvalidEnums()) 315 return null; 316 else 317 throw new FHIRException("Unknown SearchComparator code '" + codeString + "'"); 318 } 319 320 public String toCode() { 321 switch (this) { 322 case EQ: 323 return "eq"; 324 case NE: 325 return "ne"; 326 case GT: 327 return "gt"; 328 case LT: 329 return "lt"; 330 case GE: 331 return "ge"; 332 case LE: 333 return "le"; 334 case SA: 335 return "sa"; 336 case EB: 337 return "eb"; 338 case AP: 339 return "ap"; 340 case NULL: 341 return null; 342 default: 343 return "?"; 344 } 345 } 346 347 public String getSystem() { 348 switch (this) { 349 case EQ: 350 return "http://hl7.org/fhir/search-comparator"; 351 case NE: 352 return "http://hl7.org/fhir/search-comparator"; 353 case GT: 354 return "http://hl7.org/fhir/search-comparator"; 355 case LT: 356 return "http://hl7.org/fhir/search-comparator"; 357 case GE: 358 return "http://hl7.org/fhir/search-comparator"; 359 case LE: 360 return "http://hl7.org/fhir/search-comparator"; 361 case SA: 362 return "http://hl7.org/fhir/search-comparator"; 363 case EB: 364 return "http://hl7.org/fhir/search-comparator"; 365 case AP: 366 return "http://hl7.org/fhir/search-comparator"; 367 case NULL: 368 return null; 369 default: 370 return "?"; 371 } 372 } 373 374 public String getDefinition() { 375 switch (this) { 376 case EQ: 377 return "the value for the parameter in the resource is equal to the provided value."; 378 case NE: 379 return "the value for the parameter in the resource is not equal to the provided value."; 380 case GT: 381 return "the value for the parameter in the resource is greater than the provided value."; 382 case LT: 383 return "the value for the parameter in the resource is less than the provided value."; 384 case GE: 385 return "the value for the parameter in the resource is greater or equal to the provided value."; 386 case LE: 387 return "the value for the parameter in the resource is less or equal to the provided value."; 388 case SA: 389 return "the value for the parameter in the resource starts after the provided value."; 390 case EB: 391 return "the value for the parameter in the resource ends before the provided value."; 392 case AP: 393 return "the value for the parameter in the resource is approximately the same to the provided value."; 394 case NULL: 395 return null; 396 default: 397 return "?"; 398 } 399 } 400 401 public String getDisplay() { 402 switch (this) { 403 case EQ: 404 return "Equals"; 405 case NE: 406 return "Not Equals"; 407 case GT: 408 return "Greater Than"; 409 case LT: 410 return "Less Than"; 411 case GE: 412 return "Greater or Equals"; 413 case LE: 414 return "Less of Equal"; 415 case SA: 416 return "Starts After"; 417 case EB: 418 return "Ends Before"; 419 case AP: 420 return "Approximately"; 421 case NULL: 422 return null; 423 default: 424 return "?"; 425 } 426 } 427 } 428 429 public static class SearchComparatorEnumFactory implements EnumFactory<SearchComparator> { 430 public SearchComparator fromCode(String codeString) throws IllegalArgumentException { 431 if (codeString == null || "".equals(codeString)) 432 if (codeString == null || "".equals(codeString)) 433 return null; 434 if ("eq".equals(codeString)) 435 return SearchComparator.EQ; 436 if ("ne".equals(codeString)) 437 return SearchComparator.NE; 438 if ("gt".equals(codeString)) 439 return SearchComparator.GT; 440 if ("lt".equals(codeString)) 441 return SearchComparator.LT; 442 if ("ge".equals(codeString)) 443 return SearchComparator.GE; 444 if ("le".equals(codeString)) 445 return SearchComparator.LE; 446 if ("sa".equals(codeString)) 447 return SearchComparator.SA; 448 if ("eb".equals(codeString)) 449 return SearchComparator.EB; 450 if ("ap".equals(codeString)) 451 return SearchComparator.AP; 452 throw new IllegalArgumentException("Unknown SearchComparator code '" + codeString + "'"); 453 } 454 455 public Enumeration<SearchComparator> fromType(PrimitiveType<?> code) throws FHIRException { 456 if (code == null) 457 return null; 458 if (code.isEmpty()) 459 return new Enumeration<SearchComparator>(this, SearchComparator.NULL, code); 460 String codeString = code.asStringValue(); 461 if (codeString == null || "".equals(codeString)) 462 return new Enumeration<SearchComparator>(this, SearchComparator.NULL, code); 463 if ("eq".equals(codeString)) 464 return new Enumeration<SearchComparator>(this, SearchComparator.EQ, code); 465 if ("ne".equals(codeString)) 466 return new Enumeration<SearchComparator>(this, SearchComparator.NE, code); 467 if ("gt".equals(codeString)) 468 return new Enumeration<SearchComparator>(this, SearchComparator.GT, code); 469 if ("lt".equals(codeString)) 470 return new Enumeration<SearchComparator>(this, SearchComparator.LT, code); 471 if ("ge".equals(codeString)) 472 return new Enumeration<SearchComparator>(this, SearchComparator.GE, code); 473 if ("le".equals(codeString)) 474 return new Enumeration<SearchComparator>(this, SearchComparator.LE, code); 475 if ("sa".equals(codeString)) 476 return new Enumeration<SearchComparator>(this, SearchComparator.SA, code); 477 if ("eb".equals(codeString)) 478 return new Enumeration<SearchComparator>(this, SearchComparator.EB, code); 479 if ("ap".equals(codeString)) 480 return new Enumeration<SearchComparator>(this, SearchComparator.AP, code); 481 throw new FHIRException("Unknown SearchComparator code '" + codeString + "'"); 482 } 483 484 public String toCode(SearchComparator code) { 485 if (code == SearchComparator.EQ) 486 return "eq"; 487 if (code == SearchComparator.NE) 488 return "ne"; 489 if (code == SearchComparator.GT) 490 return "gt"; 491 if (code == SearchComparator.LT) 492 return "lt"; 493 if (code == SearchComparator.GE) 494 return "ge"; 495 if (code == SearchComparator.LE) 496 return "le"; 497 if (code == SearchComparator.SA) 498 return "sa"; 499 if (code == SearchComparator.EB) 500 return "eb"; 501 if (code == SearchComparator.AP) 502 return "ap"; 503 return "?"; 504 } 505 506 public String toSystem(SearchComparator code) { 507 return code.getSystem(); 508 } 509 } 510 511 public enum SearchModifierCode { 512 /** 513 * The search parameter returns resources that have a value or not. 514 */ 515 MISSING, 516 /** 517 * The search parameter returns resources that have a value that exactly matches 518 * the supplied parameter (the whole string, including casing and accents). 519 */ 520 EXACT, 521 /** 522 * The search parameter returns resources that include the supplied parameter 523 * value anywhere within the field being searched. 524 */ 525 CONTAINS, 526 /** 527 * The search parameter returns resources that do not contain a match. 528 */ 529 NOT, 530 /** 531 * The search parameter is processed as a string that searches text associated 532 * with the code/value - either CodeableConcept.text, Coding.display, or 533 * Identifier.type.text. 534 */ 535 TEXT, 536 /** 537 * The search parameter is a URI (relative or absolute) that identifies a value 538 * set, and the search parameter tests whether the coding is in the specified 539 * value set. 540 */ 541 IN, 542 /** 543 * The search parameter is a URI (relative or absolute) that identifies a value 544 * set, and the search parameter tests whether the coding is not in the 545 * specified value set. 546 */ 547 NOTIN, 548 /** 549 * The search parameter tests whether the value in a resource is subsumed by the 550 * specified value (is-a, or hierarchical relationships). 551 */ 552 BELOW, 553 /** 554 * The search parameter tests whether the value in a resource subsumes the 555 * specified value (is-a, or hierarchical relationships). 556 */ 557 ABOVE, 558 /** 559 * The search parameter only applies to the Resource Type specified as a 560 * modifier (e.g. the modifier is not actually :type, but :Patient etc.). 561 */ 562 TYPE, 563 /** 564 * The search parameter applies to the identifier on the resource, not the 565 * reference. 566 */ 567 IDENTIFIER, 568 /** 569 * The search parameter has the format system|code|value, where the system and 570 * code refer to an Identifier.type.coding.system and .code, and match if any of 571 * the type codes match. All 3 parts must be present. 572 */ 573 OFTYPE, 574 /** 575 * added to help the parsers with the generic types 576 */ 577 NULL; 578 579 public static SearchModifierCode fromCode(String codeString) throws FHIRException { 580 if (codeString == null || "".equals(codeString)) 581 return null; 582 if ("missing".equals(codeString)) 583 return MISSING; 584 if ("exact".equals(codeString)) 585 return EXACT; 586 if ("contains".equals(codeString)) 587 return CONTAINS; 588 if ("not".equals(codeString)) 589 return NOT; 590 if ("text".equals(codeString)) 591 return TEXT; 592 if ("in".equals(codeString)) 593 return IN; 594 if ("not-in".equals(codeString)) 595 return NOTIN; 596 if ("below".equals(codeString)) 597 return BELOW; 598 if ("above".equals(codeString)) 599 return ABOVE; 600 if ("type".equals(codeString)) 601 return TYPE; 602 if ("identifier".equals(codeString)) 603 return IDENTIFIER; 604 if ("ofType".equals(codeString)) 605 return OFTYPE; 606 if (Configuration.isAcceptInvalidEnums()) 607 return null; 608 else 609 throw new FHIRException("Unknown SearchModifierCode code '" + codeString + "'"); 610 } 611 612 public String toCode() { 613 switch (this) { 614 case MISSING: 615 return "missing"; 616 case EXACT: 617 return "exact"; 618 case CONTAINS: 619 return "contains"; 620 case NOT: 621 return "not"; 622 case TEXT: 623 return "text"; 624 case IN: 625 return "in"; 626 case NOTIN: 627 return "not-in"; 628 case BELOW: 629 return "below"; 630 case ABOVE: 631 return "above"; 632 case TYPE: 633 return "type"; 634 case IDENTIFIER: 635 return "identifier"; 636 case OFTYPE: 637 return "ofType"; 638 case NULL: 639 return null; 640 default: 641 return "?"; 642 } 643 } 644 645 public String getSystem() { 646 switch (this) { 647 case MISSING: 648 return "http://hl7.org/fhir/search-modifier-code"; 649 case EXACT: 650 return "http://hl7.org/fhir/search-modifier-code"; 651 case CONTAINS: 652 return "http://hl7.org/fhir/search-modifier-code"; 653 case NOT: 654 return "http://hl7.org/fhir/search-modifier-code"; 655 case TEXT: 656 return "http://hl7.org/fhir/search-modifier-code"; 657 case IN: 658 return "http://hl7.org/fhir/search-modifier-code"; 659 case NOTIN: 660 return "http://hl7.org/fhir/search-modifier-code"; 661 case BELOW: 662 return "http://hl7.org/fhir/search-modifier-code"; 663 case ABOVE: 664 return "http://hl7.org/fhir/search-modifier-code"; 665 case TYPE: 666 return "http://hl7.org/fhir/search-modifier-code"; 667 case IDENTIFIER: 668 return "http://hl7.org/fhir/search-modifier-code"; 669 case OFTYPE: 670 return "http://hl7.org/fhir/search-modifier-code"; 671 case NULL: 672 return null; 673 default: 674 return "?"; 675 } 676 } 677 678 public String getDefinition() { 679 switch (this) { 680 case MISSING: 681 return "The search parameter returns resources that have a value or not."; 682 case EXACT: 683 return "The search parameter returns resources that have a value that exactly matches the supplied parameter (the whole string, including casing and accents)."; 684 case CONTAINS: 685 return "The search parameter returns resources that include the supplied parameter value anywhere within the field being searched."; 686 case NOT: 687 return "The search parameter returns resources that do not contain a match."; 688 case TEXT: 689 return "The search parameter is processed as a string that searches text associated with the code/value - either CodeableConcept.text, Coding.display, or Identifier.type.text."; 690 case IN: 691 return "The search parameter is a URI (relative or absolute) that identifies a value set, and the search parameter tests whether the coding is in the specified value set."; 692 case NOTIN: 693 return "The search parameter is a URI (relative or absolute) that identifies a value set, and the search parameter tests whether the coding is not in the specified value set."; 694 case BELOW: 695 return "The search parameter tests whether the value in a resource is subsumed by the specified value (is-a, or hierarchical relationships)."; 696 case ABOVE: 697 return "The search parameter tests whether the value in a resource subsumes the specified value (is-a, or hierarchical relationships)."; 698 case TYPE: 699 return "The search parameter only applies to the Resource Type specified as a modifier (e.g. the modifier is not actually :type, but :Patient etc.)."; 700 case IDENTIFIER: 701 return "The search parameter applies to the identifier on the resource, not the reference."; 702 case OFTYPE: 703 return "The search parameter has the format system|code|value, where the system and code refer to an Identifier.type.coding.system and .code, and match if any of the type codes match. All 3 parts must be present."; 704 case NULL: 705 return null; 706 default: 707 return "?"; 708 } 709 } 710 711 public String getDisplay() { 712 switch (this) { 713 case MISSING: 714 return "Missing"; 715 case EXACT: 716 return "Exact"; 717 case CONTAINS: 718 return "Contains"; 719 case NOT: 720 return "Not"; 721 case TEXT: 722 return "Text"; 723 case IN: 724 return "In"; 725 case NOTIN: 726 return "Not In"; 727 case BELOW: 728 return "Below"; 729 case ABOVE: 730 return "Above"; 731 case TYPE: 732 return "Type"; 733 case IDENTIFIER: 734 return "Identifier"; 735 case OFTYPE: 736 return "Of Type"; 737 case NULL: 738 return null; 739 default: 740 return "?"; 741 } 742 } 743 } 744 745 public static class SearchModifierCodeEnumFactory implements EnumFactory<SearchModifierCode> { 746 public SearchModifierCode fromCode(String codeString) throws IllegalArgumentException { 747 if (codeString == null || "".equals(codeString)) 748 if (codeString == null || "".equals(codeString)) 749 return null; 750 if ("missing".equals(codeString)) 751 return SearchModifierCode.MISSING; 752 if ("exact".equals(codeString)) 753 return SearchModifierCode.EXACT; 754 if ("contains".equals(codeString)) 755 return SearchModifierCode.CONTAINS; 756 if ("not".equals(codeString)) 757 return SearchModifierCode.NOT; 758 if ("text".equals(codeString)) 759 return SearchModifierCode.TEXT; 760 if ("in".equals(codeString)) 761 return SearchModifierCode.IN; 762 if ("not-in".equals(codeString)) 763 return SearchModifierCode.NOTIN; 764 if ("below".equals(codeString)) 765 return SearchModifierCode.BELOW; 766 if ("above".equals(codeString)) 767 return SearchModifierCode.ABOVE; 768 if ("type".equals(codeString)) 769 return SearchModifierCode.TYPE; 770 if ("identifier".equals(codeString)) 771 return SearchModifierCode.IDENTIFIER; 772 if ("ofType".equals(codeString)) 773 return SearchModifierCode.OFTYPE; 774 throw new IllegalArgumentException("Unknown SearchModifierCode code '" + codeString + "'"); 775 } 776 777 public Enumeration<SearchModifierCode> fromType(PrimitiveType<?> code) throws FHIRException { 778 if (code == null) 779 return null; 780 if (code.isEmpty()) 781 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.NULL, code); 782 String codeString = code.asStringValue(); 783 if (codeString == null || "".equals(codeString)) 784 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.NULL, code); 785 if ("missing".equals(codeString)) 786 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.MISSING, code); 787 if ("exact".equals(codeString)) 788 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.EXACT, code); 789 if ("contains".equals(codeString)) 790 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.CONTAINS, code); 791 if ("not".equals(codeString)) 792 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.NOT, code); 793 if ("text".equals(codeString)) 794 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.TEXT, code); 795 if ("in".equals(codeString)) 796 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.IN, code); 797 if ("not-in".equals(codeString)) 798 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.NOTIN, code); 799 if ("below".equals(codeString)) 800 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.BELOW, code); 801 if ("above".equals(codeString)) 802 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.ABOVE, code); 803 if ("type".equals(codeString)) 804 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.TYPE, code); 805 if ("identifier".equals(codeString)) 806 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.IDENTIFIER, code); 807 if ("ofType".equals(codeString)) 808 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.OFTYPE, code); 809 throw new FHIRException("Unknown SearchModifierCode code '" + codeString + "'"); 810 } 811 812 public String toCode(SearchModifierCode code) { 813 if (code == SearchModifierCode.MISSING) 814 return "missing"; 815 if (code == SearchModifierCode.EXACT) 816 return "exact"; 817 if (code == SearchModifierCode.CONTAINS) 818 return "contains"; 819 if (code == SearchModifierCode.NOT) 820 return "not"; 821 if (code == SearchModifierCode.TEXT) 822 return "text"; 823 if (code == SearchModifierCode.IN) 824 return "in"; 825 if (code == SearchModifierCode.NOTIN) 826 return "not-in"; 827 if (code == SearchModifierCode.BELOW) 828 return "below"; 829 if (code == SearchModifierCode.ABOVE) 830 return "above"; 831 if (code == SearchModifierCode.TYPE) 832 return "type"; 833 if (code == SearchModifierCode.IDENTIFIER) 834 return "identifier"; 835 if (code == SearchModifierCode.OFTYPE) 836 return "ofType"; 837 return "?"; 838 } 839 840 public String toSystem(SearchModifierCode code) { 841 return code.getSystem(); 842 } 843 } 844 845 @Block() 846 public static class SearchParameterComponentComponent extends BackboneElement implements IBaseBackboneElement { 847 /** 848 * The definition of the search parameter that describes this part. 849 */ 850 @Child(name = "definition", type = { 851 CanonicalType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 852 @Description(shortDefinition = "Defines how the part works", formalDefinition = "The definition of the search parameter that describes this part.") 853 protected CanonicalType definition; 854 855 /** 856 * A sub-expression that defines how to extract values for this component from 857 * the output of the main SearchParameter.expression. 858 */ 859 @Child(name = "expression", type = { 860 StringType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 861 @Description(shortDefinition = "Subexpression relative to main expression", formalDefinition = "A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression.") 862 protected StringType expression; 863 864 private static final long serialVersionUID = -1469435618L; 865 866 /** 867 * Constructor 868 */ 869 public SearchParameterComponentComponent() { 870 super(); 871 } 872 873 /** 874 * Constructor 875 */ 876 public SearchParameterComponentComponent(CanonicalType definition, StringType expression) { 877 super(); 878 this.definition = definition; 879 this.expression = expression; 880 } 881 882 /** 883 * @return {@link #definition} (The definition of the search parameter that 884 * describes this part.). This is the underlying object with id, value 885 * and extensions. The accessor "getDefinition" gives direct access to 886 * the value 887 */ 888 public CanonicalType getDefinitionElement() { 889 if (this.definition == null) 890 if (Configuration.errorOnAutoCreate()) 891 throw new Error("Attempt to auto-create SearchParameterComponentComponent.definition"); 892 else if (Configuration.doAutoCreate()) 893 this.definition = new CanonicalType(); // bb 894 return this.definition; 895 } 896 897 public boolean hasDefinitionElement() { 898 return this.definition != null && !this.definition.isEmpty(); 899 } 900 901 public boolean hasDefinition() { 902 return this.definition != null && !this.definition.isEmpty(); 903 } 904 905 /** 906 * @param value {@link #definition} (The definition of the search parameter that 907 * describes this part.). This is the underlying object with id, 908 * value and extensions. The accessor "getDefinition" gives direct 909 * access to the value 910 */ 911 public SearchParameterComponentComponent setDefinitionElement(CanonicalType value) { 912 this.definition = value; 913 return this; 914 } 915 916 /** 917 * @return The definition of the search parameter that describes this part. 918 */ 919 public String getDefinition() { 920 return this.definition == null ? null : this.definition.getValue(); 921 } 922 923 /** 924 * @param value The definition of the search parameter that describes this part. 925 */ 926 public SearchParameterComponentComponent setDefinition(String value) { 927 if (this.definition == null) 928 this.definition = new CanonicalType(); 929 this.definition.setValue(value); 930 return this; 931 } 932 933 /** 934 * @return {@link #expression} (A sub-expression that defines how to extract 935 * values for this component from the output of the main 936 * SearchParameter.expression.). This is the underlying object with id, 937 * value and extensions. The accessor "getExpression" gives direct 938 * access to the value 939 */ 940 public StringType getExpressionElement() { 941 if (this.expression == null) 942 if (Configuration.errorOnAutoCreate()) 943 throw new Error("Attempt to auto-create SearchParameterComponentComponent.expression"); 944 else if (Configuration.doAutoCreate()) 945 this.expression = new StringType(); // bb 946 return this.expression; 947 } 948 949 public boolean hasExpressionElement() { 950 return this.expression != null && !this.expression.isEmpty(); 951 } 952 953 public boolean hasExpression() { 954 return this.expression != null && !this.expression.isEmpty(); 955 } 956 957 /** 958 * @param value {@link #expression} (A sub-expression that defines how to 959 * extract values for this component from the output of the main 960 * SearchParameter.expression.). This is the underlying object with 961 * id, value and extensions. The accessor "getExpression" gives 962 * direct access to the value 963 */ 964 public SearchParameterComponentComponent setExpressionElement(StringType value) { 965 this.expression = value; 966 return this; 967 } 968 969 /** 970 * @return A sub-expression that defines how to extract values for this 971 * component from the output of the main SearchParameter.expression. 972 */ 973 public String getExpression() { 974 return this.expression == null ? null : this.expression.getValue(); 975 } 976 977 /** 978 * @param value A sub-expression that defines how to extract values for this 979 * component from the output of the main 980 * SearchParameter.expression. 981 */ 982 public SearchParameterComponentComponent setExpression(String value) { 983 if (this.expression == null) 984 this.expression = new StringType(); 985 this.expression.setValue(value); 986 return this; 987 } 988 989 protected void listChildren(List<Property> children) { 990 super.listChildren(children); 991 children.add(new Property("definition", "canonical(SearchParameter)", 992 "The definition of the search parameter that describes this part.", 0, 1, definition)); 993 children.add(new Property("expression", "string", 994 "A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression.", 995 0, 1, expression)); 996 } 997 998 @Override 999 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1000 switch (_hash) { 1001 case -1014418093: 1002 /* definition */ return new Property("definition", "canonical(SearchParameter)", 1003 "The definition of the search parameter that describes this part.", 0, 1, definition); 1004 case -1795452264: 1005 /* expression */ return new Property("expression", "string", 1006 "A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression.", 1007 0, 1, expression); 1008 default: 1009 return super.getNamedProperty(_hash, _name, _checkValid); 1010 } 1011 1012 } 1013 1014 @Override 1015 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1016 switch (hash) { 1017 case -1014418093: 1018 /* definition */ return this.definition == null ? new Base[0] : new Base[] { this.definition }; // CanonicalType 1019 case -1795452264: 1020 /* expression */ return this.expression == null ? new Base[0] : new Base[] { this.expression }; // StringType 1021 default: 1022 return super.getProperty(hash, name, checkValid); 1023 } 1024 1025 } 1026 1027 @Override 1028 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1029 switch (hash) { 1030 case -1014418093: // definition 1031 this.definition = castToCanonical(value); // CanonicalType 1032 return value; 1033 case -1795452264: // expression 1034 this.expression = castToString(value); // StringType 1035 return value; 1036 default: 1037 return super.setProperty(hash, name, value); 1038 } 1039 1040 } 1041 1042 @Override 1043 public Base setProperty(String name, Base value) throws FHIRException { 1044 if (name.equals("definition")) { 1045 this.definition = castToCanonical(value); // CanonicalType 1046 } else if (name.equals("expression")) { 1047 this.expression = castToString(value); // StringType 1048 } else 1049 return super.setProperty(name, value); 1050 return value; 1051 } 1052 1053 @Override 1054 public Base makeProperty(int hash, String name) throws FHIRException { 1055 switch (hash) { 1056 case -1014418093: 1057 return getDefinitionElement(); 1058 case -1795452264: 1059 return getExpressionElement(); 1060 default: 1061 return super.makeProperty(hash, name); 1062 } 1063 1064 } 1065 1066 @Override 1067 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1068 switch (hash) { 1069 case -1014418093: 1070 /* definition */ return new String[] { "canonical" }; 1071 case -1795452264: 1072 /* expression */ return new String[] { "string" }; 1073 default: 1074 return super.getTypesForProperty(hash, name); 1075 } 1076 1077 } 1078 1079 @Override 1080 public Base addChild(String name) throws FHIRException { 1081 if (name.equals("definition")) { 1082 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.definition"); 1083 } else if (name.equals("expression")) { 1084 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.expression"); 1085 } else 1086 return super.addChild(name); 1087 } 1088 1089 public SearchParameterComponentComponent copy() { 1090 SearchParameterComponentComponent dst = new SearchParameterComponentComponent(); 1091 copyValues(dst); 1092 return dst; 1093 } 1094 1095 public void copyValues(SearchParameterComponentComponent dst) { 1096 super.copyValues(dst); 1097 dst.definition = definition == null ? null : definition.copy(); 1098 dst.expression = expression == null ? null : expression.copy(); 1099 } 1100 1101 @Override 1102 public boolean equalsDeep(Base other_) { 1103 if (!super.equalsDeep(other_)) 1104 return false; 1105 if (!(other_ instanceof SearchParameterComponentComponent)) 1106 return false; 1107 SearchParameterComponentComponent o = (SearchParameterComponentComponent) other_; 1108 return compareDeep(definition, o.definition, true) && compareDeep(expression, o.expression, true); 1109 } 1110 1111 @Override 1112 public boolean equalsShallow(Base other_) { 1113 if (!super.equalsShallow(other_)) 1114 return false; 1115 if (!(other_ instanceof SearchParameterComponentComponent)) 1116 return false; 1117 SearchParameterComponentComponent o = (SearchParameterComponentComponent) other_; 1118 return compareValues(expression, o.expression, true); 1119 } 1120 1121 public boolean isEmpty() { 1122 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(definition, expression); 1123 } 1124 1125 public String fhirType() { 1126 return "SearchParameter.component"; 1127 1128 } 1129 1130 } 1131 1132 /** 1133 * Where this search parameter is originally defined. If a derivedFrom is 1134 * provided, then the details in the search parameter must be consistent with 1135 * the definition from which it is defined. i.e. the parameter should have the 1136 * same meaning, and (usually) the functionality should be a proper subset of 1137 * the underlying search parameter. 1138 */ 1139 @Child(name = "derivedFrom", type = { 1140 CanonicalType.class }, order = 0, min = 0, max = 1, modifier = false, summary = false) 1141 @Description(shortDefinition = "Original definition for the search parameter", formalDefinition = "Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. i.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter.") 1142 protected CanonicalType derivedFrom; 1143 1144 /** 1145 * Explanation of why this search parameter is needed and why it has been 1146 * designed as it has. 1147 */ 1148 @Child(name = "purpose", type = { 1149 MarkdownType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1150 @Description(shortDefinition = "Why this search parameter is defined", formalDefinition = "Explanation of why this search parameter is needed and why it has been designed as it has.") 1151 protected MarkdownType purpose; 1152 1153 /** 1154 * The code used in the URL or the parameter name in a parameters resource for 1155 * this search parameter. 1156 */ 1157 @Child(name = "code", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1158 @Description(shortDefinition = "Code used in URL", formalDefinition = "The code used in the URL or the parameter name in a parameters resource for this search parameter.") 1159 protected CodeType code; 1160 1161 /** 1162 * The base resource type(s) that this search parameter can be used against. 1163 */ 1164 @Child(name = "base", type = { 1165 CodeType.class }, order = 3, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1166 @Description(shortDefinition = "The resource type(s) this search parameter applies to", formalDefinition = "The base resource type(s) that this search parameter can be used against.") 1167 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/resource-types") 1168 protected List<CodeType> base; 1169 1170 /** 1171 * The type of value that a search parameter may contain, and how the content is 1172 * interpreted. 1173 */ 1174 @Child(name = "type", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 1175 @Description(shortDefinition = "number | date | string | token | reference | composite | quantity | uri | special", formalDefinition = "The type of value that a search parameter may contain, and how the content is interpreted.") 1176 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/search-param-type") 1177 protected Enumeration<SearchParamType> type; 1178 1179 /** 1180 * A FHIRPath expression that returns a set of elements for the search 1181 * parameter. 1182 */ 1183 @Child(name = "expression", type = { 1184 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1185 @Description(shortDefinition = "FHIRPath expression that extracts the values", formalDefinition = "A FHIRPath expression that returns a set of elements for the search parameter.") 1186 protected StringType expression; 1187 1188 /** 1189 * An XPath expression that returns a set of elements for the search parameter. 1190 */ 1191 @Child(name = "xpath", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 1192 @Description(shortDefinition = "XPath that extracts the values", formalDefinition = "An XPath expression that returns a set of elements for the search parameter.") 1193 protected StringType xpath; 1194 1195 /** 1196 * How the search parameter relates to the set of elements returned by 1197 * evaluating the xpath query. 1198 */ 1199 @Child(name = "xpathUsage", type = { CodeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 1200 @Description(shortDefinition = "normal | phonetic | nearby | distance | other", formalDefinition = "How the search parameter relates to the set of elements returned by evaluating the xpath query.") 1201 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/search-xpath-usage") 1202 protected Enumeration<XPathUsageType> xpathUsage; 1203 1204 /** 1205 * Types of resource (if a resource is referenced). 1206 */ 1207 @Child(name = "target", type = { 1208 CodeType.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1209 @Description(shortDefinition = "Types of resource (if a resource reference)", formalDefinition = "Types of resource (if a resource is referenced).") 1210 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/resource-types") 1211 protected List<CodeType> target; 1212 1213 /** 1214 * Whether multiple values are allowed for each time the parameter exists. 1215 * Values are separated by commas, and the parameter matches if any of the 1216 * values match. 1217 */ 1218 @Child(name = "multipleOr", type = { 1219 BooleanType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1220 @Description(shortDefinition = "Allow multiple values per parameter (or)", formalDefinition = "Whether multiple values are allowed for each time the parameter exists. Values are separated by commas, and the parameter matches if any of the values match.") 1221 protected BooleanType multipleOr; 1222 1223 /** 1224 * Whether multiple parameters are allowed - e.g. more than one parameter with 1225 * the same name. The search matches if all the parameters match. 1226 */ 1227 @Child(name = "multipleAnd", type = { 1228 BooleanType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 1229 @Description(shortDefinition = "Allow multiple parameters (and)", formalDefinition = "Whether multiple parameters are allowed - e.g. more than one parameter with the same name. The search matches if all the parameters match.") 1230 protected BooleanType multipleAnd; 1231 1232 /** 1233 * Comparators supported for the search parameter. 1234 */ 1235 @Child(name = "comparator", type = { 1236 CodeType.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1237 @Description(shortDefinition = "eq | ne | gt | lt | ge | le | sa | eb | ap", formalDefinition = "Comparators supported for the search parameter.") 1238 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/search-comparator") 1239 protected List<Enumeration<SearchComparator>> comparator; 1240 1241 /** 1242 * A modifier supported for the search parameter. 1243 */ 1244 @Child(name = "modifier", type = { 1245 CodeType.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1246 @Description(shortDefinition = "missing | exact | contains | not | text | in | not-in | below | above | type | identifier | ofType", formalDefinition = "A modifier supported for the search parameter.") 1247 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/search-modifier-code") 1248 protected List<Enumeration<SearchModifierCode>> modifier; 1249 1250 /** 1251 * Contains the names of any search parameters which may be chained to the 1252 * containing search parameter. Chained parameters may be added to search 1253 * parameters of type reference and specify that resources will only be returned 1254 * if they contain a reference to a resource which matches the chained parameter 1255 * value. Values for this field should be drawn from SearchParameter.code for a 1256 * parameter on the target resource type. 1257 */ 1258 @Child(name = "chain", type = { 1259 StringType.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1260 @Description(shortDefinition = "Chained names supported", formalDefinition = "Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.") 1261 protected List<StringType> chain; 1262 1263 /** 1264 * Used to define the parts of a composite search parameter. 1265 */ 1266 @Child(name = "component", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1267 @Description(shortDefinition = "For Composite resources to define the parts", formalDefinition = "Used to define the parts of a composite search parameter.") 1268 protected List<SearchParameterComponentComponent> component; 1269 1270 private static final long serialVersionUID = -533803519L; 1271 1272 /** 1273 * Constructor 1274 */ 1275 public SearchParameter() { 1276 super(); 1277 } 1278 1279 /** 1280 * Constructor 1281 */ 1282 public SearchParameter(UriType url, StringType name, Enumeration<PublicationStatus> status, MarkdownType description, 1283 CodeType code, Enumeration<SearchParamType> type) { 1284 super(); 1285 this.url = url; 1286 this.name = name; 1287 this.status = status; 1288 this.description = description; 1289 this.code = code; 1290 this.type = type; 1291 } 1292 1293 /** 1294 * @return {@link #url} (An absolute URI that is used to identify this search 1295 * parameter when it is referenced in a specification, model, design or 1296 * an instance; also called its canonical identifier. This SHOULD be 1297 * globally unique and SHOULD be a literal address at which at which an 1298 * authoritative instance of this search parameter is (or will be) 1299 * published. This URL can be the target of a canonical reference. It 1300 * SHALL remain the same when the search parameter is stored on 1301 * different servers.). This is the underlying object with id, value and 1302 * extensions. The accessor "getUrl" gives direct access to the value 1303 */ 1304 public UriType getUrlElement() { 1305 if (this.url == null) 1306 if (Configuration.errorOnAutoCreate()) 1307 throw new Error("Attempt to auto-create SearchParameter.url"); 1308 else if (Configuration.doAutoCreate()) 1309 this.url = new UriType(); // bb 1310 return this.url; 1311 } 1312 1313 public boolean hasUrlElement() { 1314 return this.url != null && !this.url.isEmpty(); 1315 } 1316 1317 public boolean hasUrl() { 1318 return this.url != null && !this.url.isEmpty(); 1319 } 1320 1321 /** 1322 * @param value {@link #url} (An absolute URI that is used to identify this 1323 * search parameter when it is referenced in a specification, 1324 * model, design or an instance; also called its canonical 1325 * identifier. This SHOULD be globally unique and SHOULD be a 1326 * literal address at which at which an authoritative instance of 1327 * this search parameter is (or will be) published. This URL can be 1328 * the target of a canonical reference. It SHALL remain the same 1329 * when the search parameter is stored on different servers.). This 1330 * is the underlying object with id, value and extensions. The 1331 * accessor "getUrl" gives direct access to the value 1332 */ 1333 public SearchParameter setUrlElement(UriType value) { 1334 this.url = value; 1335 return this; 1336 } 1337 1338 /** 1339 * @return An absolute URI that is used to identify this search parameter when 1340 * it is referenced in a specification, model, design or an instance; 1341 * also called its canonical identifier. This SHOULD be globally unique 1342 * and SHOULD be a literal address at which at which an authoritative 1343 * instance of this search parameter is (or will be) published. This URL 1344 * can be the target of a canonical reference. It SHALL remain the same 1345 * when the search parameter is stored on different servers. 1346 */ 1347 public String getUrl() { 1348 return this.url == null ? null : this.url.getValue(); 1349 } 1350 1351 /** 1352 * @param value An absolute URI that is used to identify this search parameter 1353 * when it is referenced in a specification, model, design or an 1354 * instance; also called its canonical identifier. This SHOULD be 1355 * globally unique and SHOULD be a literal address at which at 1356 * which an authoritative instance of this search parameter is (or 1357 * will be) published. This URL can be the target of a canonical 1358 * reference. It SHALL remain the same when the search parameter is 1359 * stored on different servers. 1360 */ 1361 public SearchParameter setUrl(String value) { 1362 if (this.url == null) 1363 this.url = new UriType(); 1364 this.url.setValue(value); 1365 return this; 1366 } 1367 1368 /** 1369 * @return {@link #version} (The identifier that is used to identify this 1370 * version of the search parameter when it is referenced in a 1371 * specification, model, design or instance. This is an arbitrary value 1372 * managed by the search parameter author and is not expected to be 1373 * globally unique. For example, it might be a timestamp (e.g. yyyymmdd) 1374 * if a managed version is not available. There is also no expectation 1375 * that versions can be placed in a lexicographical sequence.). This is 1376 * the underlying object with id, value and extensions. The accessor 1377 * "getVersion" gives direct access to the value 1378 */ 1379 public StringType getVersionElement() { 1380 if (this.version == null) 1381 if (Configuration.errorOnAutoCreate()) 1382 throw new Error("Attempt to auto-create SearchParameter.version"); 1383 else if (Configuration.doAutoCreate()) 1384 this.version = new StringType(); // bb 1385 return this.version; 1386 } 1387 1388 public boolean hasVersionElement() { 1389 return this.version != null && !this.version.isEmpty(); 1390 } 1391 1392 public boolean hasVersion() { 1393 return this.version != null && !this.version.isEmpty(); 1394 } 1395 1396 /** 1397 * @param value {@link #version} (The identifier that is used to identify this 1398 * version of the search parameter when it is referenced in a 1399 * specification, model, design or instance. This is an arbitrary 1400 * value managed by the search parameter author and is not expected 1401 * to be globally unique. For example, it might be a timestamp 1402 * (e.g. yyyymmdd) if a managed version is not available. There is 1403 * also no expectation that versions can be placed in a 1404 * lexicographical sequence.). This is the underlying object with 1405 * id, value and extensions. The accessor "getVersion" gives direct 1406 * access to the value 1407 */ 1408 public SearchParameter setVersionElement(StringType value) { 1409 this.version = value; 1410 return this; 1411 } 1412 1413 /** 1414 * @return The identifier that is used to identify this version of the search 1415 * parameter when it is referenced in a specification, model, design or 1416 * instance. This is an arbitrary value managed by the search parameter 1417 * author and is not expected to be globally unique. For example, it 1418 * might be a timestamp (e.g. yyyymmdd) if a managed version is not 1419 * available. There is also no expectation that versions can be placed 1420 * in a lexicographical sequence. 1421 */ 1422 public String getVersion() { 1423 return this.version == null ? null : this.version.getValue(); 1424 } 1425 1426 /** 1427 * @param value The identifier that is used to identify this version of the 1428 * search parameter when it is referenced in a specification, 1429 * model, design or instance. This is an arbitrary value managed by 1430 * the search parameter author and is not expected to be globally 1431 * unique. For example, it might be a timestamp (e.g. yyyymmdd) if 1432 * a managed version is not available. There is also no expectation 1433 * that versions can be placed in a lexicographical sequence. 1434 */ 1435 public SearchParameter setVersion(String value) { 1436 if (Utilities.noString(value)) 1437 this.version = null; 1438 else { 1439 if (this.version == null) 1440 this.version = new StringType(); 1441 this.version.setValue(value); 1442 } 1443 return this; 1444 } 1445 1446 /** 1447 * @return {@link #name} (A natural language name identifying the search 1448 * parameter. This name should be usable as an identifier for the module 1449 * by machine processing applications such as code generation.). This is 1450 * the underlying object with id, value and extensions. The accessor 1451 * "getName" gives direct access to the value 1452 */ 1453 public StringType getNameElement() { 1454 if (this.name == null) 1455 if (Configuration.errorOnAutoCreate()) 1456 throw new Error("Attempt to auto-create SearchParameter.name"); 1457 else if (Configuration.doAutoCreate()) 1458 this.name = new StringType(); // bb 1459 return this.name; 1460 } 1461 1462 public boolean hasNameElement() { 1463 return this.name != null && !this.name.isEmpty(); 1464 } 1465 1466 public boolean hasName() { 1467 return this.name != null && !this.name.isEmpty(); 1468 } 1469 1470 /** 1471 * @param value {@link #name} (A natural language name identifying the search 1472 * parameter. This name should be usable as an identifier for the 1473 * module by machine processing applications such as code 1474 * generation.). This is the underlying object with id, value and 1475 * extensions. The accessor "getName" gives direct access to the 1476 * value 1477 */ 1478 public SearchParameter setNameElement(StringType value) { 1479 this.name = value; 1480 return this; 1481 } 1482 1483 /** 1484 * @return A natural language name identifying the search parameter. This name 1485 * should be usable as an identifier for the module by machine 1486 * processing applications such as code generation. 1487 */ 1488 public String getName() { 1489 return this.name == null ? null : this.name.getValue(); 1490 } 1491 1492 /** 1493 * @param value A natural language name identifying the search parameter. This 1494 * name should be usable as an identifier for the module by machine 1495 * processing applications such as code generation. 1496 */ 1497 public SearchParameter setName(String value) { 1498 if (this.name == null) 1499 this.name = new StringType(); 1500 this.name.setValue(value); 1501 return this; 1502 } 1503 1504 /** 1505 * @return {@link #derivedFrom} (Where this search parameter is originally 1506 * defined. If a derivedFrom is provided, then the details in the search 1507 * parameter must be consistent with the definition from which it is 1508 * defined. i.e. the parameter should have the same meaning, and 1509 * (usually) the functionality should be a proper subset of the 1510 * underlying search parameter.). This is the underlying object with id, 1511 * value and extensions. The accessor "getDerivedFrom" gives direct 1512 * access to the value 1513 */ 1514 public CanonicalType getDerivedFromElement() { 1515 if (this.derivedFrom == null) 1516 if (Configuration.errorOnAutoCreate()) 1517 throw new Error("Attempt to auto-create SearchParameter.derivedFrom"); 1518 else if (Configuration.doAutoCreate()) 1519 this.derivedFrom = new CanonicalType(); // bb 1520 return this.derivedFrom; 1521 } 1522 1523 public boolean hasDerivedFromElement() { 1524 return this.derivedFrom != null && !this.derivedFrom.isEmpty(); 1525 } 1526 1527 public boolean hasDerivedFrom() { 1528 return this.derivedFrom != null && !this.derivedFrom.isEmpty(); 1529 } 1530 1531 /** 1532 * @param value {@link #derivedFrom} (Where this search parameter is originally 1533 * defined. If a derivedFrom is provided, then the details in the 1534 * search parameter must be consistent with the definition from 1535 * which it is defined. i.e. the parameter should have the same 1536 * meaning, and (usually) the functionality should be a proper 1537 * subset of the underlying search parameter.). This is the 1538 * underlying object with id, value and extensions. The accessor 1539 * "getDerivedFrom" gives direct access to the value 1540 */ 1541 public SearchParameter setDerivedFromElement(CanonicalType value) { 1542 this.derivedFrom = value; 1543 return this; 1544 } 1545 1546 /** 1547 * @return Where this search parameter is originally defined. If a derivedFrom 1548 * is provided, then the details in the search parameter must be 1549 * consistent with the definition from which it is defined. i.e. the 1550 * parameter should have the same meaning, and (usually) the 1551 * functionality should be a proper subset of the underlying search 1552 * parameter. 1553 */ 1554 public String getDerivedFrom() { 1555 return this.derivedFrom == null ? null : this.derivedFrom.getValue(); 1556 } 1557 1558 /** 1559 * @param value Where this search parameter is originally defined. If a 1560 * derivedFrom is provided, then the details in the search 1561 * parameter must be consistent with the definition from which it 1562 * is defined. i.e. the parameter should have the same meaning, and 1563 * (usually) the functionality should be a proper subset of the 1564 * underlying search parameter. 1565 */ 1566 public SearchParameter setDerivedFrom(String value) { 1567 if (Utilities.noString(value)) 1568 this.derivedFrom = null; 1569 else { 1570 if (this.derivedFrom == null) 1571 this.derivedFrom = new CanonicalType(); 1572 this.derivedFrom.setValue(value); 1573 } 1574 return this; 1575 } 1576 1577 /** 1578 * @return {@link #status} (The status of this search parameter. Enables 1579 * tracking the life-cycle of the content.). This is the underlying 1580 * object with id, value and extensions. The accessor "getStatus" gives 1581 * direct access to the value 1582 */ 1583 public Enumeration<PublicationStatus> getStatusElement() { 1584 if (this.status == null) 1585 if (Configuration.errorOnAutoCreate()) 1586 throw new Error("Attempt to auto-create SearchParameter.status"); 1587 else if (Configuration.doAutoCreate()) 1588 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 1589 return this.status; 1590 } 1591 1592 public boolean hasStatusElement() { 1593 return this.status != null && !this.status.isEmpty(); 1594 } 1595 1596 public boolean hasStatus() { 1597 return this.status != null && !this.status.isEmpty(); 1598 } 1599 1600 /** 1601 * @param value {@link #status} (The status of this search parameter. Enables 1602 * tracking the life-cycle of the content.). This is the underlying 1603 * object with id, value and extensions. The accessor "getStatus" 1604 * gives direct access to the value 1605 */ 1606 public SearchParameter setStatusElement(Enumeration<PublicationStatus> value) { 1607 this.status = value; 1608 return this; 1609 } 1610 1611 /** 1612 * @return The status of this search parameter. Enables tracking the life-cycle 1613 * of the content. 1614 */ 1615 public PublicationStatus getStatus() { 1616 return this.status == null ? null : this.status.getValue(); 1617 } 1618 1619 /** 1620 * @param value The status of this search parameter. Enables tracking the 1621 * life-cycle of the content. 1622 */ 1623 public SearchParameter setStatus(PublicationStatus value) { 1624 if (this.status == null) 1625 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 1626 this.status.setValue(value); 1627 return this; 1628 } 1629 1630 /** 1631 * @return {@link #experimental} (A Boolean value to indicate that this search 1632 * parameter is authored for testing purposes (or 1633 * education/evaluation/marketing) and is not intended to be used for 1634 * genuine usage.). This is the underlying object with id, value and 1635 * extensions. The accessor "getExperimental" gives direct access to the 1636 * value 1637 */ 1638 public BooleanType getExperimentalElement() { 1639 if (this.experimental == null) 1640 if (Configuration.errorOnAutoCreate()) 1641 throw new Error("Attempt to auto-create SearchParameter.experimental"); 1642 else if (Configuration.doAutoCreate()) 1643 this.experimental = new BooleanType(); // bb 1644 return this.experimental; 1645 } 1646 1647 public boolean hasExperimentalElement() { 1648 return this.experimental != null && !this.experimental.isEmpty(); 1649 } 1650 1651 public boolean hasExperimental() { 1652 return this.experimental != null && !this.experimental.isEmpty(); 1653 } 1654 1655 /** 1656 * @param value {@link #experimental} (A Boolean value to indicate that this 1657 * search parameter is authored for testing purposes (or 1658 * education/evaluation/marketing) and is not intended to be used 1659 * for genuine usage.). This is the underlying object with id, 1660 * value and extensions. The accessor "getExperimental" gives 1661 * direct access to the value 1662 */ 1663 public SearchParameter setExperimentalElement(BooleanType value) { 1664 this.experimental = value; 1665 return this; 1666 } 1667 1668 /** 1669 * @return A Boolean value to indicate that this search parameter is authored 1670 * for testing purposes (or education/evaluation/marketing) and is not 1671 * intended to be used for genuine usage. 1672 */ 1673 public boolean getExperimental() { 1674 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1675 } 1676 1677 /** 1678 * @param value A Boolean value to indicate that this search parameter is 1679 * authored for testing purposes (or 1680 * education/evaluation/marketing) and is not intended to be used 1681 * for genuine usage. 1682 */ 1683 public SearchParameter setExperimental(boolean value) { 1684 if (this.experimental == null) 1685 this.experimental = new BooleanType(); 1686 this.experimental.setValue(value); 1687 return this; 1688 } 1689 1690 /** 1691 * @return {@link #date} (The date (and optionally time) when the search 1692 * parameter was published. The date must change when the business 1693 * version changes and it must change if the status code changes. In 1694 * addition, it should change when the substantive content of the search 1695 * parameter changes.). This is the underlying object with id, value and 1696 * extensions. The accessor "getDate" gives direct access to the value 1697 */ 1698 public DateTimeType getDateElement() { 1699 if (this.date == null) 1700 if (Configuration.errorOnAutoCreate()) 1701 throw new Error("Attempt to auto-create SearchParameter.date"); 1702 else if (Configuration.doAutoCreate()) 1703 this.date = new DateTimeType(); // bb 1704 return this.date; 1705 } 1706 1707 public boolean hasDateElement() { 1708 return this.date != null && !this.date.isEmpty(); 1709 } 1710 1711 public boolean hasDate() { 1712 return this.date != null && !this.date.isEmpty(); 1713 } 1714 1715 /** 1716 * @param value {@link #date} (The date (and optionally time) when the search 1717 * parameter was published. The date must change when the business 1718 * version changes and it must change if the status code changes. 1719 * In addition, it should change when the substantive content of 1720 * the search parameter changes.). This is the underlying object 1721 * with id, value and extensions. The accessor "getDate" gives 1722 * direct access to the value 1723 */ 1724 public SearchParameter setDateElement(DateTimeType value) { 1725 this.date = value; 1726 return this; 1727 } 1728 1729 /** 1730 * @return The date (and optionally time) when the search parameter was 1731 * published. The date must change when the business version changes and 1732 * it must change if the status code changes. In addition, it should 1733 * change when the substantive content of the search parameter changes. 1734 */ 1735 public Date getDate() { 1736 return this.date == null ? null : this.date.getValue(); 1737 } 1738 1739 /** 1740 * @param value The date (and optionally time) when the search parameter was 1741 * published. The date must change when the business version 1742 * changes and it must change if the status code changes. In 1743 * addition, it should change when the substantive content of the 1744 * search parameter changes. 1745 */ 1746 public SearchParameter setDate(Date value) { 1747 if (value == null) 1748 this.date = null; 1749 else { 1750 if (this.date == null) 1751 this.date = new DateTimeType(); 1752 this.date.setValue(value); 1753 } 1754 return this; 1755 } 1756 1757 /** 1758 * @return {@link #publisher} (The name of the organization or individual that 1759 * published the search parameter.). This is the underlying object with 1760 * id, value and extensions. The accessor "getPublisher" gives direct 1761 * access to the value 1762 */ 1763 public StringType getPublisherElement() { 1764 if (this.publisher == null) 1765 if (Configuration.errorOnAutoCreate()) 1766 throw new Error("Attempt to auto-create SearchParameter.publisher"); 1767 else if (Configuration.doAutoCreate()) 1768 this.publisher = new StringType(); // bb 1769 return this.publisher; 1770 } 1771 1772 public boolean hasPublisherElement() { 1773 return this.publisher != null && !this.publisher.isEmpty(); 1774 } 1775 1776 public boolean hasPublisher() { 1777 return this.publisher != null && !this.publisher.isEmpty(); 1778 } 1779 1780 /** 1781 * @param value {@link #publisher} (The name of the organization or individual 1782 * that published the search parameter.). This is the underlying 1783 * object with id, value and extensions. The accessor 1784 * "getPublisher" gives direct access to the value 1785 */ 1786 public SearchParameter setPublisherElement(StringType value) { 1787 this.publisher = value; 1788 return this; 1789 } 1790 1791 /** 1792 * @return The name of the organization or individual that published the search 1793 * parameter. 1794 */ 1795 public String getPublisher() { 1796 return this.publisher == null ? null : this.publisher.getValue(); 1797 } 1798 1799 /** 1800 * @param value The name of the organization or individual that published the 1801 * search parameter. 1802 */ 1803 public SearchParameter setPublisher(String value) { 1804 if (Utilities.noString(value)) 1805 this.publisher = null; 1806 else { 1807 if (this.publisher == null) 1808 this.publisher = new StringType(); 1809 this.publisher.setValue(value); 1810 } 1811 return this; 1812 } 1813 1814 /** 1815 * @return {@link #contact} (Contact details to assist a user in finding and 1816 * communicating with the publisher.) 1817 */ 1818 public List<ContactDetail> getContact() { 1819 if (this.contact == null) 1820 this.contact = new ArrayList<ContactDetail>(); 1821 return this.contact; 1822 } 1823 1824 /** 1825 * @return Returns a reference to <code>this</code> for easy method chaining 1826 */ 1827 public SearchParameter setContact(List<ContactDetail> theContact) { 1828 this.contact = theContact; 1829 return this; 1830 } 1831 1832 public boolean hasContact() { 1833 if (this.contact == null) 1834 return false; 1835 for (ContactDetail item : this.contact) 1836 if (!item.isEmpty()) 1837 return true; 1838 return false; 1839 } 1840 1841 public ContactDetail addContact() { // 3 1842 ContactDetail t = new ContactDetail(); 1843 if (this.contact == null) 1844 this.contact = new ArrayList<ContactDetail>(); 1845 this.contact.add(t); 1846 return t; 1847 } 1848 1849 public SearchParameter addContact(ContactDetail t) { // 3 1850 if (t == null) 1851 return this; 1852 if (this.contact == null) 1853 this.contact = new ArrayList<ContactDetail>(); 1854 this.contact.add(t); 1855 return this; 1856 } 1857 1858 /** 1859 * @return The first repetition of repeating field {@link #contact}, creating it 1860 * if it does not already exist 1861 */ 1862 public ContactDetail getContactFirstRep() { 1863 if (getContact().isEmpty()) { 1864 addContact(); 1865 } 1866 return getContact().get(0); 1867 } 1868 1869 /** 1870 * @return {@link #description} (And how it used.). This is the underlying 1871 * object with id, value and extensions. The accessor "getDescription" 1872 * gives direct access to the value 1873 */ 1874 public MarkdownType getDescriptionElement() { 1875 if (this.description == null) 1876 if (Configuration.errorOnAutoCreate()) 1877 throw new Error("Attempt to auto-create SearchParameter.description"); 1878 else if (Configuration.doAutoCreate()) 1879 this.description = new MarkdownType(); // bb 1880 return this.description; 1881 } 1882 1883 public boolean hasDescriptionElement() { 1884 return this.description != null && !this.description.isEmpty(); 1885 } 1886 1887 public boolean hasDescription() { 1888 return this.description != null && !this.description.isEmpty(); 1889 } 1890 1891 /** 1892 * @param value {@link #description} (And how it used.). This is the underlying 1893 * object with id, value and extensions. The accessor 1894 * "getDescription" gives direct access to the value 1895 */ 1896 public SearchParameter setDescriptionElement(MarkdownType value) { 1897 this.description = value; 1898 return this; 1899 } 1900 1901 /** 1902 * @return And how it used. 1903 */ 1904 public String getDescription() { 1905 return this.description == null ? null : this.description.getValue(); 1906 } 1907 1908 /** 1909 * @param value And how it used. 1910 */ 1911 public SearchParameter setDescription(String value) { 1912 if (this.description == null) 1913 this.description = new MarkdownType(); 1914 this.description.setValue(value); 1915 return this; 1916 } 1917 1918 /** 1919 * @return {@link #useContext} (The content was developed with a focus and 1920 * intent of supporting the contexts that are listed. These contexts may 1921 * be general categories (gender, age, ...) or may be references to 1922 * specific programs (insurance plans, studies, ...) and may be used to 1923 * assist with indexing and searching for appropriate search parameter 1924 * instances.) 1925 */ 1926 public List<UsageContext> getUseContext() { 1927 if (this.useContext == null) 1928 this.useContext = new ArrayList<UsageContext>(); 1929 return this.useContext; 1930 } 1931 1932 /** 1933 * @return Returns a reference to <code>this</code> for easy method chaining 1934 */ 1935 public SearchParameter setUseContext(List<UsageContext> theUseContext) { 1936 this.useContext = theUseContext; 1937 return this; 1938 } 1939 1940 public boolean hasUseContext() { 1941 if (this.useContext == null) 1942 return false; 1943 for (UsageContext item : this.useContext) 1944 if (!item.isEmpty()) 1945 return true; 1946 return false; 1947 } 1948 1949 public UsageContext addUseContext() { // 3 1950 UsageContext t = new UsageContext(); 1951 if (this.useContext == null) 1952 this.useContext = new ArrayList<UsageContext>(); 1953 this.useContext.add(t); 1954 return t; 1955 } 1956 1957 public SearchParameter addUseContext(UsageContext t) { // 3 1958 if (t == null) 1959 return this; 1960 if (this.useContext == null) 1961 this.useContext = new ArrayList<UsageContext>(); 1962 this.useContext.add(t); 1963 return this; 1964 } 1965 1966 /** 1967 * @return The first repetition of repeating field {@link #useContext}, creating 1968 * it if it does not already exist 1969 */ 1970 public UsageContext getUseContextFirstRep() { 1971 if (getUseContext().isEmpty()) { 1972 addUseContext(); 1973 } 1974 return getUseContext().get(0); 1975 } 1976 1977 /** 1978 * @return {@link #jurisdiction} (A legal or geographic region in which the 1979 * search parameter is intended to be used.) 1980 */ 1981 public List<CodeableConcept> getJurisdiction() { 1982 if (this.jurisdiction == null) 1983 this.jurisdiction = new ArrayList<CodeableConcept>(); 1984 return this.jurisdiction; 1985 } 1986 1987 /** 1988 * @return Returns a reference to <code>this</code> for easy method chaining 1989 */ 1990 public SearchParameter setJurisdiction(List<CodeableConcept> theJurisdiction) { 1991 this.jurisdiction = theJurisdiction; 1992 return this; 1993 } 1994 1995 public boolean hasJurisdiction() { 1996 if (this.jurisdiction == null) 1997 return false; 1998 for (CodeableConcept item : this.jurisdiction) 1999 if (!item.isEmpty()) 2000 return true; 2001 return false; 2002 } 2003 2004 public CodeableConcept addJurisdiction() { // 3 2005 CodeableConcept t = new CodeableConcept(); 2006 if (this.jurisdiction == null) 2007 this.jurisdiction = new ArrayList<CodeableConcept>(); 2008 this.jurisdiction.add(t); 2009 return t; 2010 } 2011 2012 public SearchParameter addJurisdiction(CodeableConcept t) { // 3 2013 if (t == null) 2014 return this; 2015 if (this.jurisdiction == null) 2016 this.jurisdiction = new ArrayList<CodeableConcept>(); 2017 this.jurisdiction.add(t); 2018 return this; 2019 } 2020 2021 /** 2022 * @return The first repetition of repeating field {@link #jurisdiction}, 2023 * creating it if it does not already exist 2024 */ 2025 public CodeableConcept getJurisdictionFirstRep() { 2026 if (getJurisdiction().isEmpty()) { 2027 addJurisdiction(); 2028 } 2029 return getJurisdiction().get(0); 2030 } 2031 2032 /** 2033 * @return {@link #purpose} (Explanation of why this search parameter is needed 2034 * and why it has been designed as it has.). This is the underlying 2035 * object with id, value and extensions. The accessor "getPurpose" gives 2036 * direct access to the value 2037 */ 2038 public MarkdownType getPurposeElement() { 2039 if (this.purpose == null) 2040 if (Configuration.errorOnAutoCreate()) 2041 throw new Error("Attempt to auto-create SearchParameter.purpose"); 2042 else if (Configuration.doAutoCreate()) 2043 this.purpose = new MarkdownType(); // bb 2044 return this.purpose; 2045 } 2046 2047 public boolean hasPurposeElement() { 2048 return this.purpose != null && !this.purpose.isEmpty(); 2049 } 2050 2051 public boolean hasPurpose() { 2052 return this.purpose != null && !this.purpose.isEmpty(); 2053 } 2054 2055 /** 2056 * @param value {@link #purpose} (Explanation of why this search parameter is 2057 * needed and why it has been designed as it has.). This is the 2058 * underlying object with id, value and extensions. The accessor 2059 * "getPurpose" gives direct access to the value 2060 */ 2061 public SearchParameter setPurposeElement(MarkdownType value) { 2062 this.purpose = value; 2063 return this; 2064 } 2065 2066 /** 2067 * @return Explanation of why this search parameter is needed and why it has 2068 * been designed as it has. 2069 */ 2070 public String getPurpose() { 2071 return this.purpose == null ? null : this.purpose.getValue(); 2072 } 2073 2074 /** 2075 * @param value Explanation of why this search parameter is needed and why it 2076 * has been designed as it has. 2077 */ 2078 public SearchParameter setPurpose(String value) { 2079 if (value == null) 2080 this.purpose = null; 2081 else { 2082 if (this.purpose == null) 2083 this.purpose = new MarkdownType(); 2084 this.purpose.setValue(value); 2085 } 2086 return this; 2087 } 2088 2089 /** 2090 * @return {@link #code} (The code used in the URL or the parameter name in a 2091 * parameters resource for this search parameter.). This is the 2092 * underlying object with id, value and extensions. The accessor 2093 * "getCode" gives direct access to the value 2094 */ 2095 public CodeType getCodeElement() { 2096 if (this.code == null) 2097 if (Configuration.errorOnAutoCreate()) 2098 throw new Error("Attempt to auto-create SearchParameter.code"); 2099 else if (Configuration.doAutoCreate()) 2100 this.code = new CodeType(); // bb 2101 return this.code; 2102 } 2103 2104 public boolean hasCodeElement() { 2105 return this.code != null && !this.code.isEmpty(); 2106 } 2107 2108 public boolean hasCode() { 2109 return this.code != null && !this.code.isEmpty(); 2110 } 2111 2112 /** 2113 * @param value {@link #code} (The code used in the URL or the parameter name in 2114 * a parameters resource for this search parameter.). This is the 2115 * underlying object with id, value and extensions. The accessor 2116 * "getCode" gives direct access to the value 2117 */ 2118 public SearchParameter setCodeElement(CodeType value) { 2119 this.code = value; 2120 return this; 2121 } 2122 2123 /** 2124 * @return The code used in the URL or the parameter name in a parameters 2125 * resource for this search parameter. 2126 */ 2127 public String getCode() { 2128 return this.code == null ? null : this.code.getValue(); 2129 } 2130 2131 /** 2132 * @param value The code used in the URL or the parameter name in a parameters 2133 * resource for this search parameter. 2134 */ 2135 public SearchParameter setCode(String value) { 2136 if (this.code == null) 2137 this.code = new CodeType(); 2138 this.code.setValue(value); 2139 return this; 2140 } 2141 2142 /** 2143 * @return {@link #base} (The base resource type(s) that this search parameter 2144 * can be used against.) 2145 */ 2146 public List<CodeType> getBase() { 2147 if (this.base == null) 2148 this.base = new ArrayList<CodeType>(); 2149 return this.base; 2150 } 2151 2152 /** 2153 * @return Returns a reference to <code>this</code> for easy method chaining 2154 */ 2155 public SearchParameter setBase(List<CodeType> theBase) { 2156 this.base = theBase; 2157 return this; 2158 } 2159 2160 public boolean hasBase() { 2161 if (this.base == null) 2162 return false; 2163 for (CodeType item : this.base) 2164 if (!item.isEmpty()) 2165 return true; 2166 return false; 2167 } 2168 2169 /** 2170 * @return {@link #base} (The base resource type(s) that this search parameter 2171 * can be used against.) 2172 */ 2173 public CodeType addBaseElement() {// 2 2174 CodeType t = new CodeType(); 2175 if (this.base == null) 2176 this.base = new ArrayList<CodeType>(); 2177 this.base.add(t); 2178 return t; 2179 } 2180 2181 /** 2182 * @param value {@link #base} (The base resource type(s) that this search 2183 * parameter can be used against.) 2184 */ 2185 public SearchParameter addBase(String value) { // 1 2186 CodeType t = new CodeType(); 2187 t.setValue(value); 2188 if (this.base == null) 2189 this.base = new ArrayList<CodeType>(); 2190 this.base.add(t); 2191 return this; 2192 } 2193 2194 /** 2195 * @param value {@link #base} (The base resource type(s) that this search 2196 * parameter can be used against.) 2197 */ 2198 public boolean hasBase(String value) { 2199 if (this.base == null) 2200 return false; 2201 for (CodeType v : this.base) 2202 if (v.getValue().equals(value)) // code 2203 return true; 2204 return false; 2205 } 2206 2207 /** 2208 * @return {@link #type} (The type of value that a search parameter may contain, 2209 * and how the content is interpreted.). This is the underlying object 2210 * with id, value and extensions. The accessor "getType" gives direct 2211 * access to the value 2212 */ 2213 public Enumeration<SearchParamType> getTypeElement() { 2214 if (this.type == null) 2215 if (Configuration.errorOnAutoCreate()) 2216 throw new Error("Attempt to auto-create SearchParameter.type"); 2217 else if (Configuration.doAutoCreate()) 2218 this.type = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); // bb 2219 return this.type; 2220 } 2221 2222 public boolean hasTypeElement() { 2223 return this.type != null && !this.type.isEmpty(); 2224 } 2225 2226 public boolean hasType() { 2227 return this.type != null && !this.type.isEmpty(); 2228 } 2229 2230 /** 2231 * @param value {@link #type} (The type of value that a search parameter may 2232 * contain, and how the content is interpreted.). This is the 2233 * underlying object with id, value and extensions. The accessor 2234 * "getType" gives direct access to the value 2235 */ 2236 public SearchParameter setTypeElement(Enumeration<SearchParamType> value) { 2237 this.type = value; 2238 return this; 2239 } 2240 2241 /** 2242 * @return The type of value that a search parameter may contain, and how the 2243 * content is interpreted. 2244 */ 2245 public SearchParamType getType() { 2246 return this.type == null ? null : this.type.getValue(); 2247 } 2248 2249 /** 2250 * @param value The type of value that a search parameter may contain, and how 2251 * the content is interpreted. 2252 */ 2253 public SearchParameter setType(SearchParamType value) { 2254 if (this.type == null) 2255 this.type = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); 2256 this.type.setValue(value); 2257 return this; 2258 } 2259 2260 /** 2261 * @return {@link #expression} (A FHIRPath expression that returns a set of 2262 * elements for the search parameter.). This is the underlying object 2263 * with id, value and extensions. The accessor "getExpression" gives 2264 * direct access to the value 2265 */ 2266 public StringType getExpressionElement() { 2267 if (this.expression == null) 2268 if (Configuration.errorOnAutoCreate()) 2269 throw new Error("Attempt to auto-create SearchParameter.expression"); 2270 else if (Configuration.doAutoCreate()) 2271 this.expression = new StringType(); // bb 2272 return this.expression; 2273 } 2274 2275 public boolean hasExpressionElement() { 2276 return this.expression != null && !this.expression.isEmpty(); 2277 } 2278 2279 public boolean hasExpression() { 2280 return this.expression != null && !this.expression.isEmpty(); 2281 } 2282 2283 /** 2284 * @param value {@link #expression} (A FHIRPath expression that returns a set of 2285 * elements for the search parameter.). This is the underlying 2286 * object with id, value and extensions. The accessor 2287 * "getExpression" gives direct access to the value 2288 */ 2289 public SearchParameter setExpressionElement(StringType value) { 2290 this.expression = value; 2291 return this; 2292 } 2293 2294 /** 2295 * @return A FHIRPath expression that returns a set of elements for the search 2296 * parameter. 2297 */ 2298 public String getExpression() { 2299 return this.expression == null ? null : this.expression.getValue(); 2300 } 2301 2302 /** 2303 * @param value A FHIRPath expression that returns a set of elements for the 2304 * search parameter. 2305 */ 2306 public SearchParameter setExpression(String value) { 2307 if (Utilities.noString(value)) 2308 this.expression = null; 2309 else { 2310 if (this.expression == null) 2311 this.expression = new StringType(); 2312 this.expression.setValue(value); 2313 } 2314 return this; 2315 } 2316 2317 /** 2318 * @return {@link #xpath} (An XPath expression that returns a set of elements 2319 * for the search parameter.). This is the underlying object with id, 2320 * value and extensions. The accessor "getXpath" gives direct access to 2321 * the value 2322 */ 2323 public StringType getXpathElement() { 2324 if (this.xpath == null) 2325 if (Configuration.errorOnAutoCreate()) 2326 throw new Error("Attempt to auto-create SearchParameter.xpath"); 2327 else if (Configuration.doAutoCreate()) 2328 this.xpath = new StringType(); // bb 2329 return this.xpath; 2330 } 2331 2332 public boolean hasXpathElement() { 2333 return this.xpath != null && !this.xpath.isEmpty(); 2334 } 2335 2336 public boolean hasXpath() { 2337 return this.xpath != null && !this.xpath.isEmpty(); 2338 } 2339 2340 /** 2341 * @param value {@link #xpath} (An XPath expression that returns a set of 2342 * elements for the search parameter.). This is the underlying 2343 * object with id, value and extensions. The accessor "getXpath" 2344 * gives direct access to the value 2345 */ 2346 public SearchParameter setXpathElement(StringType value) { 2347 this.xpath = value; 2348 return this; 2349 } 2350 2351 /** 2352 * @return An XPath expression that returns a set of elements for the search 2353 * parameter. 2354 */ 2355 public String getXpath() { 2356 return this.xpath == null ? null : this.xpath.getValue(); 2357 } 2358 2359 /** 2360 * @param value An XPath expression that returns a set of elements for the 2361 * search parameter. 2362 */ 2363 public SearchParameter setXpath(String value) { 2364 if (Utilities.noString(value)) 2365 this.xpath = null; 2366 else { 2367 if (this.xpath == null) 2368 this.xpath = new StringType(); 2369 this.xpath.setValue(value); 2370 } 2371 return this; 2372 } 2373 2374 /** 2375 * @return {@link #xpathUsage} (How the search parameter relates to the set of 2376 * elements returned by evaluating the xpath query.). This is the 2377 * underlying object with id, value and extensions. The accessor 2378 * "getXpathUsage" gives direct access to the value 2379 */ 2380 public Enumeration<XPathUsageType> getXpathUsageElement() { 2381 if (this.xpathUsage == null) 2382 if (Configuration.errorOnAutoCreate()) 2383 throw new Error("Attempt to auto-create SearchParameter.xpathUsage"); 2384 else if (Configuration.doAutoCreate()) 2385 this.xpathUsage = new Enumeration<XPathUsageType>(new XPathUsageTypeEnumFactory()); // bb 2386 return this.xpathUsage; 2387 } 2388 2389 public boolean hasXpathUsageElement() { 2390 return this.xpathUsage != null && !this.xpathUsage.isEmpty(); 2391 } 2392 2393 public boolean hasXpathUsage() { 2394 return this.xpathUsage != null && !this.xpathUsage.isEmpty(); 2395 } 2396 2397 /** 2398 * @param value {@link #xpathUsage} (How the search parameter relates to the set 2399 * of elements returned by evaluating the xpath query.). This is 2400 * the underlying object with id, value and extensions. The 2401 * accessor "getXpathUsage" gives direct access to the value 2402 */ 2403 public SearchParameter setXpathUsageElement(Enumeration<XPathUsageType> value) { 2404 this.xpathUsage = value; 2405 return this; 2406 } 2407 2408 /** 2409 * @return How the search parameter relates to the set of elements returned by 2410 * evaluating the xpath query. 2411 */ 2412 public XPathUsageType getXpathUsage() { 2413 return this.xpathUsage == null ? null : this.xpathUsage.getValue(); 2414 } 2415 2416 /** 2417 * @param value How the search parameter relates to the set of elements returned 2418 * by evaluating the xpath query. 2419 */ 2420 public SearchParameter setXpathUsage(XPathUsageType value) { 2421 if (value == null) 2422 this.xpathUsage = null; 2423 else { 2424 if (this.xpathUsage == null) 2425 this.xpathUsage = new Enumeration<XPathUsageType>(new XPathUsageTypeEnumFactory()); 2426 this.xpathUsage.setValue(value); 2427 } 2428 return this; 2429 } 2430 2431 /** 2432 * @return {@link #target} (Types of resource (if a resource is referenced).) 2433 */ 2434 public List<CodeType> getTarget() { 2435 if (this.target == null) 2436 this.target = new ArrayList<CodeType>(); 2437 return this.target; 2438 } 2439 2440 /** 2441 * @return Returns a reference to <code>this</code> for easy method chaining 2442 */ 2443 public SearchParameter setTarget(List<CodeType> theTarget) { 2444 this.target = theTarget; 2445 return this; 2446 } 2447 2448 public boolean hasTarget() { 2449 if (this.target == null) 2450 return false; 2451 for (CodeType item : this.target) 2452 if (!item.isEmpty()) 2453 return true; 2454 return false; 2455 } 2456 2457 /** 2458 * @return {@link #target} (Types of resource (if a resource is referenced).) 2459 */ 2460 public CodeType addTargetElement() {// 2 2461 CodeType t = new CodeType(); 2462 if (this.target == null) 2463 this.target = new ArrayList<CodeType>(); 2464 this.target.add(t); 2465 return t; 2466 } 2467 2468 /** 2469 * @param value {@link #target} (Types of resource (if a resource is 2470 * referenced).) 2471 */ 2472 public SearchParameter addTarget(String value) { // 1 2473 CodeType t = new CodeType(); 2474 t.setValue(value); 2475 if (this.target == null) 2476 this.target = new ArrayList<CodeType>(); 2477 this.target.add(t); 2478 return this; 2479 } 2480 2481 /** 2482 * @param value {@link #target} (Types of resource (if a resource is 2483 * referenced).) 2484 */ 2485 public boolean hasTarget(String value) { 2486 if (this.target == null) 2487 return false; 2488 for (CodeType v : this.target) 2489 if (v.getValue().equals(value)) // code 2490 return true; 2491 return false; 2492 } 2493 2494 /** 2495 * @return {@link #multipleOr} (Whether multiple values are allowed for each 2496 * time the parameter exists. Values are separated by commas, and the 2497 * parameter matches if any of the values match.). This is the 2498 * underlying object with id, value and extensions. The accessor 2499 * "getMultipleOr" gives direct access to the value 2500 */ 2501 public BooleanType getMultipleOrElement() { 2502 if (this.multipleOr == null) 2503 if (Configuration.errorOnAutoCreate()) 2504 throw new Error("Attempt to auto-create SearchParameter.multipleOr"); 2505 else if (Configuration.doAutoCreate()) 2506 this.multipleOr = new BooleanType(); // bb 2507 return this.multipleOr; 2508 } 2509 2510 public boolean hasMultipleOrElement() { 2511 return this.multipleOr != null && !this.multipleOr.isEmpty(); 2512 } 2513 2514 public boolean hasMultipleOr() { 2515 return this.multipleOr != null && !this.multipleOr.isEmpty(); 2516 } 2517 2518 /** 2519 * @param value {@link #multipleOr} (Whether multiple values are allowed for 2520 * each time the parameter exists. Values are separated by commas, 2521 * and the parameter matches if any of the values match.). This is 2522 * the underlying object with id, value and extensions. The 2523 * accessor "getMultipleOr" gives direct access to the value 2524 */ 2525 public SearchParameter setMultipleOrElement(BooleanType value) { 2526 this.multipleOr = value; 2527 return this; 2528 } 2529 2530 /** 2531 * @return Whether multiple values are allowed for each time the parameter 2532 * exists. Values are separated by commas, and the parameter matches if 2533 * any of the values match. 2534 */ 2535 public boolean getMultipleOr() { 2536 return this.multipleOr == null || this.multipleOr.isEmpty() ? false : this.multipleOr.getValue(); 2537 } 2538 2539 /** 2540 * @param value Whether multiple values are allowed for each time the parameter 2541 * exists. Values are separated by commas, and the parameter 2542 * matches if any of the values match. 2543 */ 2544 public SearchParameter setMultipleOr(boolean value) { 2545 if (this.multipleOr == null) 2546 this.multipleOr = new BooleanType(); 2547 this.multipleOr.setValue(value); 2548 return this; 2549 } 2550 2551 /** 2552 * @return {@link #multipleAnd} (Whether multiple parameters are allowed - e.g. 2553 * more than one parameter with the same name. The search matches if all 2554 * the parameters match.). This is the underlying object with id, value 2555 * and extensions. The accessor "getMultipleAnd" gives direct access to 2556 * the value 2557 */ 2558 public BooleanType getMultipleAndElement() { 2559 if (this.multipleAnd == null) 2560 if (Configuration.errorOnAutoCreate()) 2561 throw new Error("Attempt to auto-create SearchParameter.multipleAnd"); 2562 else if (Configuration.doAutoCreate()) 2563 this.multipleAnd = new BooleanType(); // bb 2564 return this.multipleAnd; 2565 } 2566 2567 public boolean hasMultipleAndElement() { 2568 return this.multipleAnd != null && !this.multipleAnd.isEmpty(); 2569 } 2570 2571 public boolean hasMultipleAnd() { 2572 return this.multipleAnd != null && !this.multipleAnd.isEmpty(); 2573 } 2574 2575 /** 2576 * @param value {@link #multipleAnd} (Whether multiple parameters are allowed - 2577 * e.g. more than one parameter with the same name. The search 2578 * matches if all the parameters match.). This is the underlying 2579 * object with id, value and extensions. The accessor 2580 * "getMultipleAnd" gives direct access to the value 2581 */ 2582 public SearchParameter setMultipleAndElement(BooleanType value) { 2583 this.multipleAnd = value; 2584 return this; 2585 } 2586 2587 /** 2588 * @return Whether multiple parameters are allowed - e.g. more than one 2589 * parameter with the same name. The search matches if all the 2590 * parameters match. 2591 */ 2592 public boolean getMultipleAnd() { 2593 return this.multipleAnd == null || this.multipleAnd.isEmpty() ? false : this.multipleAnd.getValue(); 2594 } 2595 2596 /** 2597 * @param value Whether multiple parameters are allowed - e.g. more than one 2598 * parameter with the same name. The search matches if all the 2599 * parameters match. 2600 */ 2601 public SearchParameter setMultipleAnd(boolean value) { 2602 if (this.multipleAnd == null) 2603 this.multipleAnd = new BooleanType(); 2604 this.multipleAnd.setValue(value); 2605 return this; 2606 } 2607 2608 /** 2609 * @return {@link #comparator} (Comparators supported for the search parameter.) 2610 */ 2611 public List<Enumeration<SearchComparator>> getComparator() { 2612 if (this.comparator == null) 2613 this.comparator = new ArrayList<Enumeration<SearchComparator>>(); 2614 return this.comparator; 2615 } 2616 2617 /** 2618 * @return Returns a reference to <code>this</code> for easy method chaining 2619 */ 2620 public SearchParameter setComparator(List<Enumeration<SearchComparator>> theComparator) { 2621 this.comparator = theComparator; 2622 return this; 2623 } 2624 2625 public boolean hasComparator() { 2626 if (this.comparator == null) 2627 return false; 2628 for (Enumeration<SearchComparator> item : this.comparator) 2629 if (!item.isEmpty()) 2630 return true; 2631 return false; 2632 } 2633 2634 /** 2635 * @return {@link #comparator} (Comparators supported for the search parameter.) 2636 */ 2637 public Enumeration<SearchComparator> addComparatorElement() {// 2 2638 Enumeration<SearchComparator> t = new Enumeration<SearchComparator>(new SearchComparatorEnumFactory()); 2639 if (this.comparator == null) 2640 this.comparator = new ArrayList<Enumeration<SearchComparator>>(); 2641 this.comparator.add(t); 2642 return t; 2643 } 2644 2645 /** 2646 * @param value {@link #comparator} (Comparators supported for the search 2647 * parameter.) 2648 */ 2649 public SearchParameter addComparator(SearchComparator value) { // 1 2650 Enumeration<SearchComparator> t = new Enumeration<SearchComparator>(new SearchComparatorEnumFactory()); 2651 t.setValue(value); 2652 if (this.comparator == null) 2653 this.comparator = new ArrayList<Enumeration<SearchComparator>>(); 2654 this.comparator.add(t); 2655 return this; 2656 } 2657 2658 /** 2659 * @param value {@link #comparator} (Comparators supported for the search 2660 * parameter.) 2661 */ 2662 public boolean hasComparator(SearchComparator value) { 2663 if (this.comparator == null) 2664 return false; 2665 for (Enumeration<SearchComparator> v : this.comparator) 2666 if (v.getValue().equals(value)) // code 2667 return true; 2668 return false; 2669 } 2670 2671 /** 2672 * @return {@link #modifier} (A modifier supported for the search parameter.) 2673 */ 2674 public List<Enumeration<SearchModifierCode>> getModifier() { 2675 if (this.modifier == null) 2676 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 2677 return this.modifier; 2678 } 2679 2680 /** 2681 * @return Returns a reference to <code>this</code> for easy method chaining 2682 */ 2683 public SearchParameter setModifier(List<Enumeration<SearchModifierCode>> theModifier) { 2684 this.modifier = theModifier; 2685 return this; 2686 } 2687 2688 public boolean hasModifier() { 2689 if (this.modifier == null) 2690 return false; 2691 for (Enumeration<SearchModifierCode> item : this.modifier) 2692 if (!item.isEmpty()) 2693 return true; 2694 return false; 2695 } 2696 2697 /** 2698 * @return {@link #modifier} (A modifier supported for the search parameter.) 2699 */ 2700 public Enumeration<SearchModifierCode> addModifierElement() {// 2 2701 Enumeration<SearchModifierCode> t = new Enumeration<SearchModifierCode>(new SearchModifierCodeEnumFactory()); 2702 if (this.modifier == null) 2703 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 2704 this.modifier.add(t); 2705 return t; 2706 } 2707 2708 /** 2709 * @param value {@link #modifier} (A modifier supported for the search 2710 * parameter.) 2711 */ 2712 public SearchParameter addModifier(SearchModifierCode value) { // 1 2713 Enumeration<SearchModifierCode> t = new Enumeration<SearchModifierCode>(new SearchModifierCodeEnumFactory()); 2714 t.setValue(value); 2715 if (this.modifier == null) 2716 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 2717 this.modifier.add(t); 2718 return this; 2719 } 2720 2721 /** 2722 * @param value {@link #modifier} (A modifier supported for the search 2723 * parameter.) 2724 */ 2725 public boolean hasModifier(SearchModifierCode value) { 2726 if (this.modifier == null) 2727 return false; 2728 for (Enumeration<SearchModifierCode> v : this.modifier) 2729 if (v.getValue().equals(value)) // code 2730 return true; 2731 return false; 2732 } 2733 2734 /** 2735 * @return {@link #chain} (Contains the names of any search parameters which may 2736 * be chained to the containing search parameter. Chained parameters may 2737 * be added to search parameters of type reference and specify that 2738 * resources will only be returned if they contain a reference to a 2739 * resource which matches the chained parameter value. Values for this 2740 * field should be drawn from SearchParameter.code for a parameter on 2741 * the target resource type.) 2742 */ 2743 public List<StringType> getChain() { 2744 if (this.chain == null) 2745 this.chain = new ArrayList<StringType>(); 2746 return this.chain; 2747 } 2748 2749 /** 2750 * @return Returns a reference to <code>this</code> for easy method chaining 2751 */ 2752 public SearchParameter setChain(List<StringType> theChain) { 2753 this.chain = theChain; 2754 return this; 2755 } 2756 2757 public boolean hasChain() { 2758 if (this.chain == null) 2759 return false; 2760 for (StringType item : this.chain) 2761 if (!item.isEmpty()) 2762 return true; 2763 return false; 2764 } 2765 2766 /** 2767 * @return {@link #chain} (Contains the names of any search parameters which may 2768 * be chained to the containing search parameter. Chained parameters may 2769 * be added to search parameters of type reference and specify that 2770 * resources will only be returned if they contain a reference to a 2771 * resource which matches the chained parameter value. Values for this 2772 * field should be drawn from SearchParameter.code for a parameter on 2773 * the target resource type.) 2774 */ 2775 public StringType addChainElement() {// 2 2776 StringType t = new StringType(); 2777 if (this.chain == null) 2778 this.chain = new ArrayList<StringType>(); 2779 this.chain.add(t); 2780 return t; 2781 } 2782 2783 /** 2784 * @param value {@link #chain} (Contains the names of any search parameters 2785 * which may be chained to the containing search parameter. Chained 2786 * parameters may be added to search parameters of type reference 2787 * and specify that resources will only be returned if they contain 2788 * a reference to a resource which matches the chained parameter 2789 * value. Values for this field should be drawn from 2790 * SearchParameter.code for a parameter on the target resource 2791 * type.) 2792 */ 2793 public SearchParameter addChain(String value) { // 1 2794 StringType t = new StringType(); 2795 t.setValue(value); 2796 if (this.chain == null) 2797 this.chain = new ArrayList<StringType>(); 2798 this.chain.add(t); 2799 return this; 2800 } 2801 2802 /** 2803 * @param value {@link #chain} (Contains the names of any search parameters 2804 * which may be chained to the containing search parameter. Chained 2805 * parameters may be added to search parameters of type reference 2806 * and specify that resources will only be returned if they contain 2807 * a reference to a resource which matches the chained parameter 2808 * value. Values for this field should be drawn from 2809 * SearchParameter.code for a parameter on the target resource 2810 * type.) 2811 */ 2812 public boolean hasChain(String value) { 2813 if (this.chain == null) 2814 return false; 2815 for (StringType v : this.chain) 2816 if (v.getValue().equals(value)) // string 2817 return true; 2818 return false; 2819 } 2820 2821 /** 2822 * @return {@link #component} (Used to define the parts of a composite search 2823 * parameter.) 2824 */ 2825 public List<SearchParameterComponentComponent> getComponent() { 2826 if (this.component == null) 2827 this.component = new ArrayList<SearchParameterComponentComponent>(); 2828 return this.component; 2829 } 2830 2831 /** 2832 * @return Returns a reference to <code>this</code> for easy method chaining 2833 */ 2834 public SearchParameter setComponent(List<SearchParameterComponentComponent> theComponent) { 2835 this.component = theComponent; 2836 return this; 2837 } 2838 2839 public boolean hasComponent() { 2840 if (this.component == null) 2841 return false; 2842 for (SearchParameterComponentComponent item : this.component) 2843 if (!item.isEmpty()) 2844 return true; 2845 return false; 2846 } 2847 2848 public SearchParameterComponentComponent addComponent() { // 3 2849 SearchParameterComponentComponent t = new SearchParameterComponentComponent(); 2850 if (this.component == null) 2851 this.component = new ArrayList<SearchParameterComponentComponent>(); 2852 this.component.add(t); 2853 return t; 2854 } 2855 2856 public SearchParameter addComponent(SearchParameterComponentComponent t) { // 3 2857 if (t == null) 2858 return this; 2859 if (this.component == null) 2860 this.component = new ArrayList<SearchParameterComponentComponent>(); 2861 this.component.add(t); 2862 return this; 2863 } 2864 2865 /** 2866 * @return The first repetition of repeating field {@link #component}, creating 2867 * it if it does not already exist 2868 */ 2869 public SearchParameterComponentComponent getComponentFirstRep() { 2870 if (getComponent().isEmpty()) { 2871 addComponent(); 2872 } 2873 return getComponent().get(0); 2874 } 2875 2876 protected void listChildren(List<Property> children) { 2877 super.listChildren(children); 2878 children.add(new Property("url", "uri", 2879 "An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this search parameter is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the search parameter is stored on different servers.", 2880 0, 1, url)); 2881 children.add(new Property("version", "string", 2882 "The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 2883 0, 1, version)); 2884 children.add(new Property("name", "string", 2885 "A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 2886 0, 1, name)); 2887 children.add(new Property("derivedFrom", "canonical(SearchParameter)", 2888 "Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. i.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter.", 2889 0, 1, derivedFrom)); 2890 children.add(new Property("status", "code", 2891 "The status of this search parameter. Enables tracking the life-cycle of the content.", 0, 1, status)); 2892 children.add(new Property("experimental", "boolean", 2893 "A Boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 2894 0, 1, experimental)); 2895 children.add(new Property("date", "dateTime", 2896 "The date (and optionally time) when the search parameter was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes.", 2897 0, 1, date)); 2898 children.add(new Property("publisher", "string", 2899 "The name of the organization or individual that published the search parameter.", 0, 1, publisher)); 2900 children.add(new Property("contact", "ContactDetail", 2901 "Contact details to assist a user in finding and communicating with the publisher.", 0, 2902 java.lang.Integer.MAX_VALUE, contact)); 2903 children.add(new Property("description", "markdown", "And how it used.", 0, 1, description)); 2904 children.add(new Property("useContext", "UsageContext", 2905 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate search parameter instances.", 2906 0, java.lang.Integer.MAX_VALUE, useContext)); 2907 children.add(new Property("jurisdiction", "CodeableConcept", 2908 "A legal or geographic region in which the search parameter is intended to be used.", 0, 2909 java.lang.Integer.MAX_VALUE, jurisdiction)); 2910 children.add(new Property("purpose", "markdown", 2911 "Explanation of why this search parameter is needed and why it has been designed as it has.", 0, 1, purpose)); 2912 children.add(new Property("code", "code", 2913 "The code used in the URL or the parameter name in a parameters resource for this search parameter.", 0, 1, 2914 code)); 2915 children 2916 .add(new Property("base", "code", "The base resource type(s) that this search parameter can be used against.", 2917 0, java.lang.Integer.MAX_VALUE, base)); 2918 children.add(new Property("type", "code", 2919 "The type of value that a search parameter may contain, and how the content is interpreted.", 0, 1, type)); 2920 children.add(new Property("expression", "string", 2921 "A FHIRPath expression that returns a set of elements for the search parameter.", 0, 1, expression)); 2922 children.add(new Property("xpath", "string", 2923 "An XPath expression that returns a set of elements for the search parameter.", 0, 1, xpath)); 2924 children.add(new Property("xpathUsage", "code", 2925 "How the search parameter relates to the set of elements returned by evaluating the xpath query.", 0, 1, 2926 xpathUsage)); 2927 children.add(new Property("target", "code", "Types of resource (if a resource is referenced).", 0, 2928 java.lang.Integer.MAX_VALUE, target)); 2929 children.add(new Property("multipleOr", "boolean", 2930 "Whether multiple values are allowed for each time the parameter exists. Values are separated by commas, and the parameter matches if any of the values match.", 2931 0, 1, multipleOr)); 2932 children.add(new Property("multipleAnd", "boolean", 2933 "Whether multiple parameters are allowed - e.g. more than one parameter with the same name. The search matches if all the parameters match.", 2934 0, 1, multipleAnd)); 2935 children.add(new Property("comparator", "code", "Comparators supported for the search parameter.", 0, 2936 java.lang.Integer.MAX_VALUE, comparator)); 2937 children.add(new Property("modifier", "code", "A modifier supported for the search parameter.", 0, 2938 java.lang.Integer.MAX_VALUE, modifier)); 2939 children.add(new Property("chain", "string", 2940 "Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.", 2941 0, java.lang.Integer.MAX_VALUE, chain)); 2942 children.add(new Property("component", "", "Used to define the parts of a composite search parameter.", 0, 2943 java.lang.Integer.MAX_VALUE, component)); 2944 } 2945 2946 @Override 2947 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2948 switch (_hash) { 2949 case 116079: 2950 /* url */ return new Property("url", "uri", 2951 "An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this search parameter is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the search parameter is stored on different servers.", 2952 0, 1, url); 2953 case 351608024: 2954 /* version */ return new Property("version", "string", 2955 "The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 2956 0, 1, version); 2957 case 3373707: 2958 /* name */ return new Property("name", "string", 2959 "A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 2960 0, 1, name); 2961 case 1077922663: 2962 /* derivedFrom */ return new Property("derivedFrom", "canonical(SearchParameter)", 2963 "Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. i.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter.", 2964 0, 1, derivedFrom); 2965 case -892481550: 2966 /* status */ return new Property("status", "code", 2967 "The status of this search parameter. Enables tracking the life-cycle of the content.", 0, 1, status); 2968 case -404562712: 2969 /* experimental */ return new Property("experimental", "boolean", 2970 "A Boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 2971 0, 1, experimental); 2972 case 3076014: 2973 /* date */ return new Property("date", "dateTime", 2974 "The date (and optionally time) when the search parameter was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes.", 2975 0, 1, date); 2976 case 1447404028: 2977 /* publisher */ return new Property("publisher", "string", 2978 "The name of the organization or individual that published the search parameter.", 0, 1, publisher); 2979 case 951526432: 2980 /* contact */ return new Property("contact", "ContactDetail", 2981 "Contact details to assist a user in finding and communicating with the publisher.", 0, 2982 java.lang.Integer.MAX_VALUE, contact); 2983 case -1724546052: 2984 /* description */ return new Property("description", "markdown", "And how it used.", 0, 1, description); 2985 case -669707736: 2986 /* useContext */ return new Property("useContext", "UsageContext", 2987 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate search parameter instances.", 2988 0, java.lang.Integer.MAX_VALUE, useContext); 2989 case -507075711: 2990 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 2991 "A legal or geographic region in which the search parameter is intended to be used.", 0, 2992 java.lang.Integer.MAX_VALUE, jurisdiction); 2993 case -220463842: 2994 /* purpose */ return new Property("purpose", "markdown", 2995 "Explanation of why this search parameter is needed and why it has been designed as it has.", 0, 1, purpose); 2996 case 3059181: 2997 /* code */ return new Property("code", "code", 2998 "The code used in the URL or the parameter name in a parameters resource for this search parameter.", 0, 1, 2999 code); 3000 case 3016401: 3001 /* base */ return new Property("base", "code", 3002 "The base resource type(s) that this search parameter can be used against.", 0, java.lang.Integer.MAX_VALUE, 3003 base); 3004 case 3575610: 3005 /* type */ return new Property("type", "code", 3006 "The type of value that a search parameter may contain, and how the content is interpreted.", 0, 1, type); 3007 case -1795452264: 3008 /* expression */ return new Property("expression", "string", 3009 "A FHIRPath expression that returns a set of elements for the search parameter.", 0, 1, expression); 3010 case 114256029: 3011 /* xpath */ return new Property("xpath", "string", 3012 "An XPath expression that returns a set of elements for the search parameter.", 0, 1, xpath); 3013 case 1801322244: 3014 /* xpathUsage */ return new Property("xpathUsage", "code", 3015 "How the search parameter relates to the set of elements returned by evaluating the xpath query.", 0, 1, 3016 xpathUsage); 3017 case -880905839: 3018 /* target */ return new Property("target", "code", "Types of resource (if a resource is referenced).", 0, 3019 java.lang.Integer.MAX_VALUE, target); 3020 case 1265069075: 3021 /* multipleOr */ return new Property("multipleOr", "boolean", 3022 "Whether multiple values are allowed for each time the parameter exists. Values are separated by commas, and the parameter matches if any of the values match.", 3023 0, 1, multipleOr); 3024 case 562422183: 3025 /* multipleAnd */ return new Property("multipleAnd", "boolean", 3026 "Whether multiple parameters are allowed - e.g. more than one parameter with the same name. The search matches if all the parameters match.", 3027 0, 1, multipleAnd); 3028 case -844673834: 3029 /* comparator */ return new Property("comparator", "code", "Comparators supported for the search parameter.", 0, 3030 java.lang.Integer.MAX_VALUE, comparator); 3031 case -615513385: 3032 /* modifier */ return new Property("modifier", "code", "A modifier supported for the search parameter.", 0, 3033 java.lang.Integer.MAX_VALUE, modifier); 3034 case 94623425: 3035 /* chain */ return new Property("chain", "string", 3036 "Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.", 3037 0, java.lang.Integer.MAX_VALUE, chain); 3038 case -1399907075: 3039 /* component */ return new Property("component", "", "Used to define the parts of a composite search parameter.", 3040 0, java.lang.Integer.MAX_VALUE, component); 3041 default: 3042 return super.getNamedProperty(_hash, _name, _checkValid); 3043 } 3044 3045 } 3046 3047 @Override 3048 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3049 switch (hash) { 3050 case 116079: 3051 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 3052 case 351608024: 3053 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 3054 case 3373707: 3055 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 3056 case 1077922663: 3057 /* derivedFrom */ return this.derivedFrom == null ? new Base[0] : new Base[] { this.derivedFrom }; // CanonicalType 3058 case -892481550: 3059 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 3060 case -404562712: 3061 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 3062 case 3076014: 3063 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 3064 case 1447404028: 3065 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 3066 case 951526432: 3067 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 3068 case -1724546052: 3069 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 3070 case -669707736: 3071 /* useContext */ return this.useContext == null ? new Base[0] 3072 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 3073 case -507075711: 3074 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 3075 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 3076 case -220463842: 3077 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 3078 case 3059181: 3079 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeType 3080 case 3016401: 3081 /* base */ return this.base == null ? new Base[0] : this.base.toArray(new Base[this.base.size()]); // CodeType 3082 case 3575610: 3083 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<SearchParamType> 3084 case -1795452264: 3085 /* expression */ return this.expression == null ? new Base[0] : new Base[] { this.expression }; // StringType 3086 case 114256029: 3087 /* xpath */ return this.xpath == null ? new Base[0] : new Base[] { this.xpath }; // StringType 3088 case 1801322244: 3089 /* xpathUsage */ return this.xpathUsage == null ? new Base[0] : new Base[] { this.xpathUsage }; // Enumeration<XPathUsageType> 3090 case -880905839: 3091 /* target */ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // CodeType 3092 case 1265069075: 3093 /* multipleOr */ return this.multipleOr == null ? new Base[0] : new Base[] { this.multipleOr }; // BooleanType 3094 case 562422183: 3095 /* multipleAnd */ return this.multipleAnd == null ? new Base[0] : new Base[] { this.multipleAnd }; // BooleanType 3096 case -844673834: 3097 /* comparator */ return this.comparator == null ? new Base[0] 3098 : this.comparator.toArray(new Base[this.comparator.size()]); // Enumeration<SearchComparator> 3099 case -615513385: 3100 /* modifier */ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // Enumeration<SearchModifierCode> 3101 case 94623425: 3102 /* chain */ return this.chain == null ? new Base[0] : this.chain.toArray(new Base[this.chain.size()]); // StringType 3103 case -1399907075: 3104 /* component */ return this.component == null ? new Base[0] 3105 : this.component.toArray(new Base[this.component.size()]); // SearchParameterComponentComponent 3106 default: 3107 return super.getProperty(hash, name, checkValid); 3108 } 3109 3110 } 3111 3112 @Override 3113 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3114 switch (hash) { 3115 case 116079: // url 3116 this.url = castToUri(value); // UriType 3117 return value; 3118 case 351608024: // version 3119 this.version = castToString(value); // StringType 3120 return value; 3121 case 3373707: // name 3122 this.name = castToString(value); // StringType 3123 return value; 3124 case 1077922663: // derivedFrom 3125 this.derivedFrom = castToCanonical(value); // CanonicalType 3126 return value; 3127 case -892481550: // status 3128 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 3129 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3130 return value; 3131 case -404562712: // experimental 3132 this.experimental = castToBoolean(value); // BooleanType 3133 return value; 3134 case 3076014: // date 3135 this.date = castToDateTime(value); // DateTimeType 3136 return value; 3137 case 1447404028: // publisher 3138 this.publisher = castToString(value); // StringType 3139 return value; 3140 case 951526432: // contact 3141 this.getContact().add(castToContactDetail(value)); // ContactDetail 3142 return value; 3143 case -1724546052: // description 3144 this.description = castToMarkdown(value); // MarkdownType 3145 return value; 3146 case -669707736: // useContext 3147 this.getUseContext().add(castToUsageContext(value)); // UsageContext 3148 return value; 3149 case -507075711: // jurisdiction 3150 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 3151 return value; 3152 case -220463842: // purpose 3153 this.purpose = castToMarkdown(value); // MarkdownType 3154 return value; 3155 case 3059181: // code 3156 this.code = castToCode(value); // CodeType 3157 return value; 3158 case 3016401: // base 3159 this.getBase().add(castToCode(value)); // CodeType 3160 return value; 3161 case 3575610: // type 3162 value = new SearchParamTypeEnumFactory().fromType(castToCode(value)); 3163 this.type = (Enumeration) value; // Enumeration<SearchParamType> 3164 return value; 3165 case -1795452264: // expression 3166 this.expression = castToString(value); // StringType 3167 return value; 3168 case 114256029: // xpath 3169 this.xpath = castToString(value); // StringType 3170 return value; 3171 case 1801322244: // xpathUsage 3172 value = new XPathUsageTypeEnumFactory().fromType(castToCode(value)); 3173 this.xpathUsage = (Enumeration) value; // Enumeration<XPathUsageType> 3174 return value; 3175 case -880905839: // target 3176 this.getTarget().add(castToCode(value)); // CodeType 3177 return value; 3178 case 1265069075: // multipleOr 3179 this.multipleOr = castToBoolean(value); // BooleanType 3180 return value; 3181 case 562422183: // multipleAnd 3182 this.multipleAnd = castToBoolean(value); // BooleanType 3183 return value; 3184 case -844673834: // comparator 3185 value = new SearchComparatorEnumFactory().fromType(castToCode(value)); 3186 this.getComparator().add((Enumeration) value); // Enumeration<SearchComparator> 3187 return value; 3188 case -615513385: // modifier 3189 value = new SearchModifierCodeEnumFactory().fromType(castToCode(value)); 3190 this.getModifier().add((Enumeration) value); // Enumeration<SearchModifierCode> 3191 return value; 3192 case 94623425: // chain 3193 this.getChain().add(castToString(value)); // StringType 3194 return value; 3195 case -1399907075: // component 3196 this.getComponent().add((SearchParameterComponentComponent) value); // SearchParameterComponentComponent 3197 return value; 3198 default: 3199 return super.setProperty(hash, name, value); 3200 } 3201 3202 } 3203 3204 @Override 3205 public Base setProperty(String name, Base value) throws FHIRException { 3206 if (name.equals("url")) { 3207 this.url = castToUri(value); // UriType 3208 } else if (name.equals("version")) { 3209 this.version = castToString(value); // StringType 3210 } else if (name.equals("name")) { 3211 this.name = castToString(value); // StringType 3212 } else if (name.equals("derivedFrom")) { 3213 this.derivedFrom = castToCanonical(value); // CanonicalType 3214 } else if (name.equals("status")) { 3215 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 3216 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3217 } else if (name.equals("experimental")) { 3218 this.experimental = castToBoolean(value); // BooleanType 3219 } else if (name.equals("date")) { 3220 this.date = castToDateTime(value); // DateTimeType 3221 } else if (name.equals("publisher")) { 3222 this.publisher = castToString(value); // StringType 3223 } else if (name.equals("contact")) { 3224 this.getContact().add(castToContactDetail(value)); 3225 } else if (name.equals("description")) { 3226 this.description = castToMarkdown(value); // MarkdownType 3227 } else if (name.equals("useContext")) { 3228 this.getUseContext().add(castToUsageContext(value)); 3229 } else if (name.equals("jurisdiction")) { 3230 this.getJurisdiction().add(castToCodeableConcept(value)); 3231 } else if (name.equals("purpose")) { 3232 this.purpose = castToMarkdown(value); // MarkdownType 3233 } else if (name.equals("code")) { 3234 this.code = castToCode(value); // CodeType 3235 } else if (name.equals("base")) { 3236 this.getBase().add(castToCode(value)); 3237 } else if (name.equals("type")) { 3238 value = new SearchParamTypeEnumFactory().fromType(castToCode(value)); 3239 this.type = (Enumeration) value; // Enumeration<SearchParamType> 3240 } else if (name.equals("expression")) { 3241 this.expression = castToString(value); // StringType 3242 } else if (name.equals("xpath")) { 3243 this.xpath = castToString(value); // StringType 3244 } else if (name.equals("xpathUsage")) { 3245 value = new XPathUsageTypeEnumFactory().fromType(castToCode(value)); 3246 this.xpathUsage = (Enumeration) value; // Enumeration<XPathUsageType> 3247 } else if (name.equals("target")) { 3248 this.getTarget().add(castToCode(value)); 3249 } else if (name.equals("multipleOr")) { 3250 this.multipleOr = castToBoolean(value); // BooleanType 3251 } else if (name.equals("multipleAnd")) { 3252 this.multipleAnd = castToBoolean(value); // BooleanType 3253 } else if (name.equals("comparator")) { 3254 value = new SearchComparatorEnumFactory().fromType(castToCode(value)); 3255 this.getComparator().add((Enumeration) value); 3256 } else if (name.equals("modifier")) { 3257 value = new SearchModifierCodeEnumFactory().fromType(castToCode(value)); 3258 this.getModifier().add((Enumeration) value); 3259 } else if (name.equals("chain")) { 3260 this.getChain().add(castToString(value)); 3261 } else if (name.equals("component")) { 3262 this.getComponent().add((SearchParameterComponentComponent) value); 3263 } else 3264 return super.setProperty(name, value); 3265 return value; 3266 } 3267 3268 @Override 3269 public Base makeProperty(int hash, String name) throws FHIRException { 3270 switch (hash) { 3271 case 116079: 3272 return getUrlElement(); 3273 case 351608024: 3274 return getVersionElement(); 3275 case 3373707: 3276 return getNameElement(); 3277 case 1077922663: 3278 return getDerivedFromElement(); 3279 case -892481550: 3280 return getStatusElement(); 3281 case -404562712: 3282 return getExperimentalElement(); 3283 case 3076014: 3284 return getDateElement(); 3285 case 1447404028: 3286 return getPublisherElement(); 3287 case 951526432: 3288 return addContact(); 3289 case -1724546052: 3290 return getDescriptionElement(); 3291 case -669707736: 3292 return addUseContext(); 3293 case -507075711: 3294 return addJurisdiction(); 3295 case -220463842: 3296 return getPurposeElement(); 3297 case 3059181: 3298 return getCodeElement(); 3299 case 3016401: 3300 return addBaseElement(); 3301 case 3575610: 3302 return getTypeElement(); 3303 case -1795452264: 3304 return getExpressionElement(); 3305 case 114256029: 3306 return getXpathElement(); 3307 case 1801322244: 3308 return getXpathUsageElement(); 3309 case -880905839: 3310 return addTargetElement(); 3311 case 1265069075: 3312 return getMultipleOrElement(); 3313 case 562422183: 3314 return getMultipleAndElement(); 3315 case -844673834: 3316 return addComparatorElement(); 3317 case -615513385: 3318 return addModifierElement(); 3319 case 94623425: 3320 return addChainElement(); 3321 case -1399907075: 3322 return addComponent(); 3323 default: 3324 return super.makeProperty(hash, name); 3325 } 3326 3327 } 3328 3329 @Override 3330 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3331 switch (hash) { 3332 case 116079: 3333 /* url */ return new String[] { "uri" }; 3334 case 351608024: 3335 /* version */ return new String[] { "string" }; 3336 case 3373707: 3337 /* name */ return new String[] { "string" }; 3338 case 1077922663: 3339 /* derivedFrom */ return new String[] { "canonical" }; 3340 case -892481550: 3341 /* status */ return new String[] { "code" }; 3342 case -404562712: 3343 /* experimental */ return new String[] { "boolean" }; 3344 case 3076014: 3345 /* date */ return new String[] { "dateTime" }; 3346 case 1447404028: 3347 /* publisher */ return new String[] { "string" }; 3348 case 951526432: 3349 /* contact */ return new String[] { "ContactDetail" }; 3350 case -1724546052: 3351 /* description */ return new String[] { "markdown" }; 3352 case -669707736: 3353 /* useContext */ return new String[] { "UsageContext" }; 3354 case -507075711: 3355 /* jurisdiction */ return new String[] { "CodeableConcept" }; 3356 case -220463842: 3357 /* purpose */ return new String[] { "markdown" }; 3358 case 3059181: 3359 /* code */ return new String[] { "code" }; 3360 case 3016401: 3361 /* base */ return new String[] { "code" }; 3362 case 3575610: 3363 /* type */ return new String[] { "code" }; 3364 case -1795452264: 3365 /* expression */ return new String[] { "string" }; 3366 case 114256029: 3367 /* xpath */ return new String[] { "string" }; 3368 case 1801322244: 3369 /* xpathUsage */ return new String[] { "code" }; 3370 case -880905839: 3371 /* target */ return new String[] { "code" }; 3372 case 1265069075: 3373 /* multipleOr */ return new String[] { "boolean" }; 3374 case 562422183: 3375 /* multipleAnd */ return new String[] { "boolean" }; 3376 case -844673834: 3377 /* comparator */ return new String[] { "code" }; 3378 case -615513385: 3379 /* modifier */ return new String[] { "code" }; 3380 case 94623425: 3381 /* chain */ return new String[] { "string" }; 3382 case -1399907075: 3383 /* component */ return new String[] {}; 3384 default: 3385 return super.getTypesForProperty(hash, name); 3386 } 3387 3388 } 3389 3390 @Override 3391 public Base addChild(String name) throws FHIRException { 3392 if (name.equals("url")) { 3393 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.url"); 3394 } else if (name.equals("version")) { 3395 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.version"); 3396 } else if (name.equals("name")) { 3397 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.name"); 3398 } else if (name.equals("derivedFrom")) { 3399 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.derivedFrom"); 3400 } else if (name.equals("status")) { 3401 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.status"); 3402 } else if (name.equals("experimental")) { 3403 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.experimental"); 3404 } else if (name.equals("date")) { 3405 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.date"); 3406 } else if (name.equals("publisher")) { 3407 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.publisher"); 3408 } else if (name.equals("contact")) { 3409 return addContact(); 3410 } else if (name.equals("description")) { 3411 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.description"); 3412 } else if (name.equals("useContext")) { 3413 return addUseContext(); 3414 } else if (name.equals("jurisdiction")) { 3415 return addJurisdiction(); 3416 } else if (name.equals("purpose")) { 3417 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.purpose"); 3418 } else if (name.equals("code")) { 3419 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.code"); 3420 } else if (name.equals("base")) { 3421 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.base"); 3422 } else if (name.equals("type")) { 3423 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.type"); 3424 } else if (name.equals("expression")) { 3425 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.expression"); 3426 } else if (name.equals("xpath")) { 3427 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.xpath"); 3428 } else if (name.equals("xpathUsage")) { 3429 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.xpathUsage"); 3430 } else if (name.equals("target")) { 3431 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.target"); 3432 } else if (name.equals("multipleOr")) { 3433 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.multipleOr"); 3434 } else if (name.equals("multipleAnd")) { 3435 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.multipleAnd"); 3436 } else if (name.equals("comparator")) { 3437 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.comparator"); 3438 } else if (name.equals("modifier")) { 3439 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.modifier"); 3440 } else if (name.equals("chain")) { 3441 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.chain"); 3442 } else if (name.equals("component")) { 3443 return addComponent(); 3444 } else 3445 return super.addChild(name); 3446 } 3447 3448 public String fhirType() { 3449 return "SearchParameter"; 3450 3451 } 3452 3453 public SearchParameter copy() { 3454 SearchParameter dst = new SearchParameter(); 3455 copyValues(dst); 3456 return dst; 3457 } 3458 3459 public void copyValues(SearchParameter dst) { 3460 super.copyValues(dst); 3461 dst.url = url == null ? null : url.copy(); 3462 dst.version = version == null ? null : version.copy(); 3463 dst.name = name == null ? null : name.copy(); 3464 dst.derivedFrom = derivedFrom == null ? null : derivedFrom.copy(); 3465 dst.status = status == null ? null : status.copy(); 3466 dst.experimental = experimental == null ? null : experimental.copy(); 3467 dst.date = date == null ? null : date.copy(); 3468 dst.publisher = publisher == null ? null : publisher.copy(); 3469 if (contact != null) { 3470 dst.contact = new ArrayList<ContactDetail>(); 3471 for (ContactDetail i : contact) 3472 dst.contact.add(i.copy()); 3473 } 3474 ; 3475 dst.description = description == null ? null : description.copy(); 3476 if (useContext != null) { 3477 dst.useContext = new ArrayList<UsageContext>(); 3478 for (UsageContext i : useContext) 3479 dst.useContext.add(i.copy()); 3480 } 3481 ; 3482 if (jurisdiction != null) { 3483 dst.jurisdiction = new ArrayList<CodeableConcept>(); 3484 for (CodeableConcept i : jurisdiction) 3485 dst.jurisdiction.add(i.copy()); 3486 } 3487 ; 3488 dst.purpose = purpose == null ? null : purpose.copy(); 3489 dst.code = code == null ? null : code.copy(); 3490 if (base != null) { 3491 dst.base = new ArrayList<CodeType>(); 3492 for (CodeType i : base) 3493 dst.base.add(i.copy()); 3494 } 3495 ; 3496 dst.type = type == null ? null : type.copy(); 3497 dst.expression = expression == null ? null : expression.copy(); 3498 dst.xpath = xpath == null ? null : xpath.copy(); 3499 dst.xpathUsage = xpathUsage == null ? null : xpathUsage.copy(); 3500 if (target != null) { 3501 dst.target = new ArrayList<CodeType>(); 3502 for (CodeType i : target) 3503 dst.target.add(i.copy()); 3504 } 3505 ; 3506 dst.multipleOr = multipleOr == null ? null : multipleOr.copy(); 3507 dst.multipleAnd = multipleAnd == null ? null : multipleAnd.copy(); 3508 if (comparator != null) { 3509 dst.comparator = new ArrayList<Enumeration<SearchComparator>>(); 3510 for (Enumeration<SearchComparator> i : comparator) 3511 dst.comparator.add(i.copy()); 3512 } 3513 ; 3514 if (modifier != null) { 3515 dst.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 3516 for (Enumeration<SearchModifierCode> i : modifier) 3517 dst.modifier.add(i.copy()); 3518 } 3519 ; 3520 if (chain != null) { 3521 dst.chain = new ArrayList<StringType>(); 3522 for (StringType i : chain) 3523 dst.chain.add(i.copy()); 3524 } 3525 ; 3526 if (component != null) { 3527 dst.component = new ArrayList<SearchParameterComponentComponent>(); 3528 for (SearchParameterComponentComponent i : component) 3529 dst.component.add(i.copy()); 3530 } 3531 ; 3532 } 3533 3534 protected SearchParameter typedCopy() { 3535 return copy(); 3536 } 3537 3538 @Override 3539 public boolean equalsDeep(Base other_) { 3540 if (!super.equalsDeep(other_)) 3541 return false; 3542 if (!(other_ instanceof SearchParameter)) 3543 return false; 3544 SearchParameter o = (SearchParameter) other_; 3545 return compareDeep(derivedFrom, o.derivedFrom, true) && compareDeep(purpose, o.purpose, true) 3546 && compareDeep(code, o.code, true) && compareDeep(base, o.base, true) && compareDeep(type, o.type, true) 3547 && compareDeep(expression, o.expression, true) && compareDeep(xpath, o.xpath, true) 3548 && compareDeep(xpathUsage, o.xpathUsage, true) && compareDeep(target, o.target, true) 3549 && compareDeep(multipleOr, o.multipleOr, true) && compareDeep(multipleAnd, o.multipleAnd, true) 3550 && compareDeep(comparator, o.comparator, true) && compareDeep(modifier, o.modifier, true) 3551 && compareDeep(chain, o.chain, true) && compareDeep(component, o.component, true); 3552 } 3553 3554 @Override 3555 public boolean equalsShallow(Base other_) { 3556 if (!super.equalsShallow(other_)) 3557 return false; 3558 if (!(other_ instanceof SearchParameter)) 3559 return false; 3560 SearchParameter o = (SearchParameter) other_; 3561 return compareValues(purpose, o.purpose, true) && compareValues(code, o.code, true) 3562 && compareValues(base, o.base, true) && compareValues(type, o.type, true) 3563 && compareValues(expression, o.expression, true) && compareValues(xpath, o.xpath, true) 3564 && compareValues(xpathUsage, o.xpathUsage, true) && compareValues(target, o.target, true) 3565 && compareValues(multipleOr, o.multipleOr, true) && compareValues(multipleAnd, o.multipleAnd, true) 3566 && compareValues(comparator, o.comparator, true) && compareValues(modifier, o.modifier, true) 3567 && compareValues(chain, o.chain, true); 3568 } 3569 3570 public boolean isEmpty() { 3571 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(derivedFrom, purpose, code, base, type, expression, 3572 xpath, xpathUsage, target, multipleOr, multipleAnd, comparator, modifier, chain, component); 3573 } 3574 3575 @Override 3576 public ResourceType getResourceType() { 3577 return ResourceType.SearchParameter; 3578 } 3579 3580 /** 3581 * Search parameter: <b>date</b> 3582 * <p> 3583 * Description: <b>The search parameter publication date</b><br> 3584 * Type: <b>date</b><br> 3585 * Path: <b>SearchParameter.date</b><br> 3586 * </p> 3587 */ 3588 @SearchParamDefinition(name = "date", path = "SearchParameter.date", description = "The search parameter publication date", type = "date") 3589 public static final String SP_DATE = "date"; 3590 /** 3591 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3592 * <p> 3593 * Description: <b>The search parameter publication date</b><br> 3594 * Type: <b>date</b><br> 3595 * Path: <b>SearchParameter.date</b><br> 3596 * </p> 3597 */ 3598 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3599 SP_DATE); 3600 3601 /** 3602 * Search parameter: <b>code</b> 3603 * <p> 3604 * Description: <b>Code used in URL</b><br> 3605 * Type: <b>token</b><br> 3606 * Path: <b>SearchParameter.code</b><br> 3607 * </p> 3608 */ 3609 @SearchParamDefinition(name = "code", path = "SearchParameter.code", description = "Code used in URL", type = "token") 3610 public static final String SP_CODE = "code"; 3611 /** 3612 * <b>Fluent Client</b> search parameter constant for <b>code</b> 3613 * <p> 3614 * Description: <b>Code used in URL</b><br> 3615 * Type: <b>token</b><br> 3616 * Path: <b>SearchParameter.code</b><br> 3617 * </p> 3618 */ 3619 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3620 SP_CODE); 3621 3622 /** 3623 * Search parameter: <b>context-type-value</b> 3624 * <p> 3625 * Description: <b>A use context type and value assigned to the search 3626 * parameter</b><br> 3627 * Type: <b>composite</b><br> 3628 * Path: <b></b><br> 3629 * </p> 3630 */ 3631 @SearchParamDefinition(name = "context-type-value", path = "SearchParameter.useContext", description = "A use context type and value assigned to the search parameter", type = "composite", compositeOf = { 3632 "context-type", "context" }) 3633 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 3634 /** 3635 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 3636 * <p> 3637 * Description: <b>A use context type and value assigned to the search 3638 * parameter</b><br> 3639 * Type: <b>composite</b><br> 3640 * Path: <b></b><br> 3641 * </p> 3642 */ 3643 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 3644 SP_CONTEXT_TYPE_VALUE); 3645 3646 /** 3647 * Search parameter: <b>jurisdiction</b> 3648 * <p> 3649 * Description: <b>Intended jurisdiction for the search parameter</b><br> 3650 * Type: <b>token</b><br> 3651 * Path: <b>SearchParameter.jurisdiction</b><br> 3652 * </p> 3653 */ 3654 @SearchParamDefinition(name = "jurisdiction", path = "SearchParameter.jurisdiction", description = "Intended jurisdiction for the search parameter", type = "token") 3655 public static final String SP_JURISDICTION = "jurisdiction"; 3656 /** 3657 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 3658 * <p> 3659 * Description: <b>Intended jurisdiction for the search parameter</b><br> 3660 * Type: <b>token</b><br> 3661 * Path: <b>SearchParameter.jurisdiction</b><br> 3662 * </p> 3663 */ 3664 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3665 SP_JURISDICTION); 3666 3667 /** 3668 * Search parameter: <b>description</b> 3669 * <p> 3670 * Description: <b>The description of the search parameter</b><br> 3671 * Type: <b>string</b><br> 3672 * Path: <b>SearchParameter.description</b><br> 3673 * </p> 3674 */ 3675 @SearchParamDefinition(name = "description", path = "SearchParameter.description", description = "The description of the search parameter", type = "string") 3676 public static final String SP_DESCRIPTION = "description"; 3677 /** 3678 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3679 * <p> 3680 * Description: <b>The description of the search parameter</b><br> 3681 * Type: <b>string</b><br> 3682 * Path: <b>SearchParameter.description</b><br> 3683 * </p> 3684 */ 3685 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 3686 SP_DESCRIPTION); 3687 3688 /** 3689 * Search parameter: <b>derived-from</b> 3690 * <p> 3691 * Description: <b>Original definition for the search parameter</b><br> 3692 * Type: <b>reference</b><br> 3693 * Path: <b>SearchParameter.derivedFrom</b><br> 3694 * </p> 3695 */ 3696 @SearchParamDefinition(name = "derived-from", path = "SearchParameter.derivedFrom", description = "Original definition for the search parameter", type = "reference", target = { 3697 SearchParameter.class }) 3698 public static final String SP_DERIVED_FROM = "derived-from"; 3699 /** 3700 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 3701 * <p> 3702 * Description: <b>Original definition for the search parameter</b><br> 3703 * Type: <b>reference</b><br> 3704 * Path: <b>SearchParameter.derivedFrom</b><br> 3705 * </p> 3706 */ 3707 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3708 SP_DERIVED_FROM); 3709 3710 /** 3711 * Constant for fluent queries to be used to add include statements. Specifies 3712 * the path value of "<b>SearchParameter:derived-from</b>". 3713 */ 3714 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include( 3715 "SearchParameter:derived-from").toLocked(); 3716 3717 /** 3718 * Search parameter: <b>context-type</b> 3719 * <p> 3720 * Description: <b>A type of use context assigned to the search 3721 * parameter</b><br> 3722 * Type: <b>token</b><br> 3723 * Path: <b>SearchParameter.useContext.code</b><br> 3724 * </p> 3725 */ 3726 @SearchParamDefinition(name = "context-type", path = "SearchParameter.useContext.code", description = "A type of use context assigned to the search parameter", type = "token") 3727 public static final String SP_CONTEXT_TYPE = "context-type"; 3728 /** 3729 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 3730 * <p> 3731 * Description: <b>A type of use context assigned to the search 3732 * parameter</b><br> 3733 * Type: <b>token</b><br> 3734 * Path: <b>SearchParameter.useContext.code</b><br> 3735 * </p> 3736 */ 3737 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3738 SP_CONTEXT_TYPE); 3739 3740 /** 3741 * Search parameter: <b>type</b> 3742 * <p> 3743 * Description: <b>number | date | string | token | reference | composite | 3744 * quantity | uri | special</b><br> 3745 * Type: <b>token</b><br> 3746 * Path: <b>SearchParameter.type</b><br> 3747 * </p> 3748 */ 3749 @SearchParamDefinition(name = "type", path = "SearchParameter.type", description = "number | date | string | token | reference | composite | quantity | uri | special", type = "token") 3750 public static final String SP_TYPE = "type"; 3751 /** 3752 * <b>Fluent Client</b> search parameter constant for <b>type</b> 3753 * <p> 3754 * Description: <b>number | date | string | token | reference | composite | 3755 * quantity | uri | special</b><br> 3756 * Type: <b>token</b><br> 3757 * Path: <b>SearchParameter.type</b><br> 3758 * </p> 3759 */ 3760 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3761 SP_TYPE); 3762 3763 /** 3764 * Search parameter: <b>version</b> 3765 * <p> 3766 * Description: <b>The business version of the search parameter</b><br> 3767 * Type: <b>token</b><br> 3768 * Path: <b>SearchParameter.version</b><br> 3769 * </p> 3770 */ 3771 @SearchParamDefinition(name = "version", path = "SearchParameter.version", description = "The business version of the search parameter", type = "token") 3772 public static final String SP_VERSION = "version"; 3773 /** 3774 * <b>Fluent Client</b> search parameter constant for <b>version</b> 3775 * <p> 3776 * Description: <b>The business version of the search parameter</b><br> 3777 * Type: <b>token</b><br> 3778 * Path: <b>SearchParameter.version</b><br> 3779 * </p> 3780 */ 3781 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3782 SP_VERSION); 3783 3784 /** 3785 * Search parameter: <b>url</b> 3786 * <p> 3787 * Description: <b>The uri that identifies the search parameter</b><br> 3788 * Type: <b>uri</b><br> 3789 * Path: <b>SearchParameter.url</b><br> 3790 * </p> 3791 */ 3792 @SearchParamDefinition(name = "url", path = "SearchParameter.url", description = "The uri that identifies the search parameter", type = "uri") 3793 public static final String SP_URL = "url"; 3794 /** 3795 * <b>Fluent Client</b> search parameter constant for <b>url</b> 3796 * <p> 3797 * Description: <b>The uri that identifies the search parameter</b><br> 3798 * Type: <b>uri</b><br> 3799 * Path: <b>SearchParameter.url</b><br> 3800 * </p> 3801 */ 3802 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 3803 3804 /** 3805 * Search parameter: <b>target</b> 3806 * <p> 3807 * Description: <b>Types of resource (if a resource reference)</b><br> 3808 * Type: <b>token</b><br> 3809 * Path: <b>SearchParameter.target</b><br> 3810 * </p> 3811 */ 3812 @SearchParamDefinition(name = "target", path = "SearchParameter.target", description = "Types of resource (if a resource reference)", type = "token") 3813 public static final String SP_TARGET = "target"; 3814 /** 3815 * <b>Fluent Client</b> search parameter constant for <b>target</b> 3816 * <p> 3817 * Description: <b>Types of resource (if a resource reference)</b><br> 3818 * Type: <b>token</b><br> 3819 * Path: <b>SearchParameter.target</b><br> 3820 * </p> 3821 */ 3822 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TARGET = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3823 SP_TARGET); 3824 3825 /** 3826 * Search parameter: <b>context-quantity</b> 3827 * <p> 3828 * Description: <b>A quantity- or range-valued use context assigned to the 3829 * search parameter</b><br> 3830 * Type: <b>quantity</b><br> 3831 * Path: <b>SearchParameter.useContext.valueQuantity, 3832 * SearchParameter.useContext.valueRange</b><br> 3833 * </p> 3834 */ 3835 @SearchParamDefinition(name = "context-quantity", path = "(SearchParameter.useContext.value as Quantity) | (SearchParameter.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the search parameter", type = "quantity") 3836 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 3837 /** 3838 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 3839 * <p> 3840 * Description: <b>A quantity- or range-valued use context assigned to the 3841 * search parameter</b><br> 3842 * Type: <b>quantity</b><br> 3843 * Path: <b>SearchParameter.useContext.valueQuantity, 3844 * SearchParameter.useContext.valueRange</b><br> 3845 * </p> 3846 */ 3847 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 3848 SP_CONTEXT_QUANTITY); 3849 3850 /** 3851 * Search parameter: <b>component</b> 3852 * <p> 3853 * Description: <b>Defines how the part works</b><br> 3854 * Type: <b>reference</b><br> 3855 * Path: <b>SearchParameter.component.definition</b><br> 3856 * </p> 3857 */ 3858 @SearchParamDefinition(name = "component", path = "SearchParameter.component.definition", description = "Defines how the part works", type = "reference", target = { 3859 SearchParameter.class }) 3860 public static final String SP_COMPONENT = "component"; 3861 /** 3862 * <b>Fluent Client</b> search parameter constant for <b>component</b> 3863 * <p> 3864 * Description: <b>Defines how the part works</b><br> 3865 * Type: <b>reference</b><br> 3866 * Path: <b>SearchParameter.component.definition</b><br> 3867 * </p> 3868 */ 3869 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPONENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3870 SP_COMPONENT); 3871 3872 /** 3873 * Constant for fluent queries to be used to add include statements. Specifies 3874 * the path value of "<b>SearchParameter:component</b>". 3875 */ 3876 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPONENT = new ca.uhn.fhir.model.api.Include( 3877 "SearchParameter:component").toLocked(); 3878 3879 /** 3880 * Search parameter: <b>name</b> 3881 * <p> 3882 * Description: <b>Computationally friendly name of the search parameter</b><br> 3883 * Type: <b>string</b><br> 3884 * Path: <b>SearchParameter.name</b><br> 3885 * </p> 3886 */ 3887 @SearchParamDefinition(name = "name", path = "SearchParameter.name", description = "Computationally friendly name of the search parameter", type = "string") 3888 public static final String SP_NAME = "name"; 3889 /** 3890 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3891 * <p> 3892 * Description: <b>Computationally friendly name of the search parameter</b><br> 3893 * Type: <b>string</b><br> 3894 * Path: <b>SearchParameter.name</b><br> 3895 * </p> 3896 */ 3897 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 3898 SP_NAME); 3899 3900 /** 3901 * Search parameter: <b>context</b> 3902 * <p> 3903 * Description: <b>A use context assigned to the search parameter</b><br> 3904 * Type: <b>token</b><br> 3905 * Path: <b>SearchParameter.useContext.valueCodeableConcept</b><br> 3906 * </p> 3907 */ 3908 @SearchParamDefinition(name = "context", path = "(SearchParameter.useContext.value as CodeableConcept)", description = "A use context assigned to the search parameter", type = "token") 3909 public static final String SP_CONTEXT = "context"; 3910 /** 3911 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3912 * <p> 3913 * Description: <b>A use context assigned to the search parameter</b><br> 3914 * Type: <b>token</b><br> 3915 * Path: <b>SearchParameter.useContext.valueCodeableConcept</b><br> 3916 * </p> 3917 */ 3918 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3919 SP_CONTEXT); 3920 3921 /** 3922 * Search parameter: <b>publisher</b> 3923 * <p> 3924 * Description: <b>Name of the publisher of the search parameter</b><br> 3925 * Type: <b>string</b><br> 3926 * Path: <b>SearchParameter.publisher</b><br> 3927 * </p> 3928 */ 3929 @SearchParamDefinition(name = "publisher", path = "SearchParameter.publisher", description = "Name of the publisher of the search parameter", type = "string") 3930 public static final String SP_PUBLISHER = "publisher"; 3931 /** 3932 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 3933 * <p> 3934 * Description: <b>Name of the publisher of the search parameter</b><br> 3935 * Type: <b>string</b><br> 3936 * Path: <b>SearchParameter.publisher</b><br> 3937 * </p> 3938 */ 3939 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 3940 SP_PUBLISHER); 3941 3942 /** 3943 * Search parameter: <b>context-type-quantity</b> 3944 * <p> 3945 * Description: <b>A use context type and quantity- or range-based value 3946 * assigned to the search parameter</b><br> 3947 * Type: <b>composite</b><br> 3948 * Path: <b></b><br> 3949 * </p> 3950 */ 3951 @SearchParamDefinition(name = "context-type-quantity", path = "SearchParameter.useContext", description = "A use context type and quantity- or range-based value assigned to the search parameter", type = "composite", compositeOf = { 3952 "context-type", "context-quantity" }) 3953 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 3954 /** 3955 * <b>Fluent Client</b> search parameter constant for 3956 * <b>context-type-quantity</b> 3957 * <p> 3958 * Description: <b>A use context type and quantity- or range-based value 3959 * assigned to the search parameter</b><br> 3960 * Type: <b>composite</b><br> 3961 * Path: <b></b><br> 3962 * </p> 3963 */ 3964 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 3965 SP_CONTEXT_TYPE_QUANTITY); 3966 3967 /** 3968 * Search parameter: <b>status</b> 3969 * <p> 3970 * Description: <b>The current status of the search parameter</b><br> 3971 * Type: <b>token</b><br> 3972 * Path: <b>SearchParameter.status</b><br> 3973 * </p> 3974 */ 3975 @SearchParamDefinition(name = "status", path = "SearchParameter.status", description = "The current status of the search parameter", type = "token") 3976 public static final String SP_STATUS = "status"; 3977 /** 3978 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3979 * <p> 3980 * Description: <b>The current status of the search parameter</b><br> 3981 * Type: <b>token</b><br> 3982 * Path: <b>SearchParameter.status</b><br> 3983 * </p> 3984 */ 3985 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3986 SP_STATUS); 3987 3988 /** 3989 * Search parameter: <b>base</b> 3990 * <p> 3991 * Description: <b>The resource type(s) this search parameter applies to</b><br> 3992 * Type: <b>token</b><br> 3993 * Path: <b>SearchParameter.base</b><br> 3994 * </p> 3995 */ 3996 @SearchParamDefinition(name = "base", path = "SearchParameter.base", description = "The resource type(s) this search parameter applies to", type = "token") 3997 public static final String SP_BASE = "base"; 3998 /** 3999 * <b>Fluent Client</b> search parameter constant for <b>base</b> 4000 * <p> 4001 * Description: <b>The resource type(s) this search parameter applies to</b><br> 4002 * Type: <b>token</b><br> 4003 * Path: <b>SearchParameter.base</b><br> 4004 * </p> 4005 */ 4006 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BASE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4007 SP_BASE); 4008 4009}