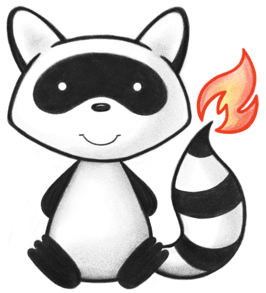
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * A sample to be used for analysis. 049 */ 050@ResourceDef(name = "Specimen", profile = "http://hl7.org/fhir/StructureDefinition/Specimen") 051public class Specimen extends DomainResource { 052 053 public enum SpecimenStatus { 054 /** 055 * The physical specimen is present and in good condition. 056 */ 057 AVAILABLE, 058 /** 059 * There is no physical specimen because it is either lost, destroyed or 060 * consumed. 061 */ 062 UNAVAILABLE, 063 /** 064 * The specimen cannot be used because of a quality issue such as a broken 065 * container, contamination, or too old. 066 */ 067 UNSATISFACTORY, 068 /** 069 * The specimen was entered in error and therefore nullified. 070 */ 071 ENTEREDINERROR, 072 /** 073 * added to help the parsers with the generic types 074 */ 075 NULL; 076 077 public static SpecimenStatus fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("available".equals(codeString)) 081 return AVAILABLE; 082 if ("unavailable".equals(codeString)) 083 return UNAVAILABLE; 084 if ("unsatisfactory".equals(codeString)) 085 return UNSATISFACTORY; 086 if ("entered-in-error".equals(codeString)) 087 return ENTEREDINERROR; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown SpecimenStatus code '" + codeString + "'"); 092 } 093 094 public String toCode() { 095 switch (this) { 096 case AVAILABLE: 097 return "available"; 098 case UNAVAILABLE: 099 return "unavailable"; 100 case UNSATISFACTORY: 101 return "unsatisfactory"; 102 case ENTEREDINERROR: 103 return "entered-in-error"; 104 case NULL: 105 return null; 106 default: 107 return "?"; 108 } 109 } 110 111 public String getSystem() { 112 switch (this) { 113 case AVAILABLE: 114 return "http://hl7.org/fhir/specimen-status"; 115 case UNAVAILABLE: 116 return "http://hl7.org/fhir/specimen-status"; 117 case UNSATISFACTORY: 118 return "http://hl7.org/fhir/specimen-status"; 119 case ENTEREDINERROR: 120 return "http://hl7.org/fhir/specimen-status"; 121 case NULL: 122 return null; 123 default: 124 return "?"; 125 } 126 } 127 128 public String getDefinition() { 129 switch (this) { 130 case AVAILABLE: 131 return "The physical specimen is present and in good condition."; 132 case UNAVAILABLE: 133 return "There is no physical specimen because it is either lost, destroyed or consumed."; 134 case UNSATISFACTORY: 135 return "The specimen cannot be used because of a quality issue such as a broken container, contamination, or too old."; 136 case ENTEREDINERROR: 137 return "The specimen was entered in error and therefore nullified."; 138 case NULL: 139 return null; 140 default: 141 return "?"; 142 } 143 } 144 145 public String getDisplay() { 146 switch (this) { 147 case AVAILABLE: 148 return "Available"; 149 case UNAVAILABLE: 150 return "Unavailable"; 151 case UNSATISFACTORY: 152 return "Unsatisfactory"; 153 case ENTEREDINERROR: 154 return "Entered in Error"; 155 case NULL: 156 return null; 157 default: 158 return "?"; 159 } 160 } 161 } 162 163 public static class SpecimenStatusEnumFactory implements EnumFactory<SpecimenStatus> { 164 public SpecimenStatus fromCode(String codeString) throws IllegalArgumentException { 165 if (codeString == null || "".equals(codeString)) 166 if (codeString == null || "".equals(codeString)) 167 return null; 168 if ("available".equals(codeString)) 169 return SpecimenStatus.AVAILABLE; 170 if ("unavailable".equals(codeString)) 171 return SpecimenStatus.UNAVAILABLE; 172 if ("unsatisfactory".equals(codeString)) 173 return SpecimenStatus.UNSATISFACTORY; 174 if ("entered-in-error".equals(codeString)) 175 return SpecimenStatus.ENTEREDINERROR; 176 throw new IllegalArgumentException("Unknown SpecimenStatus code '" + codeString + "'"); 177 } 178 179 public Enumeration<SpecimenStatus> fromType(PrimitiveType<?> code) throws FHIRException { 180 if (code == null) 181 return null; 182 if (code.isEmpty()) 183 return new Enumeration<SpecimenStatus>(this, SpecimenStatus.NULL, code); 184 String codeString = code.asStringValue(); 185 if (codeString == null || "".equals(codeString)) 186 return new Enumeration<SpecimenStatus>(this, SpecimenStatus.NULL, code); 187 if ("available".equals(codeString)) 188 return new Enumeration<SpecimenStatus>(this, SpecimenStatus.AVAILABLE, code); 189 if ("unavailable".equals(codeString)) 190 return new Enumeration<SpecimenStatus>(this, SpecimenStatus.UNAVAILABLE, code); 191 if ("unsatisfactory".equals(codeString)) 192 return new Enumeration<SpecimenStatus>(this, SpecimenStatus.UNSATISFACTORY, code); 193 if ("entered-in-error".equals(codeString)) 194 return new Enumeration<SpecimenStatus>(this, SpecimenStatus.ENTEREDINERROR, code); 195 throw new FHIRException("Unknown SpecimenStatus code '" + codeString + "'"); 196 } 197 198 public String toCode(SpecimenStatus code) { 199 if (code == SpecimenStatus.NULL) 200 return null; 201 if (code == SpecimenStatus.AVAILABLE) 202 return "available"; 203 if (code == SpecimenStatus.UNAVAILABLE) 204 return "unavailable"; 205 if (code == SpecimenStatus.UNSATISFACTORY) 206 return "unsatisfactory"; 207 if (code == SpecimenStatus.ENTEREDINERROR) 208 return "entered-in-error"; 209 return "?"; 210 } 211 212 public String toSystem(SpecimenStatus code) { 213 return code.getSystem(); 214 } 215 } 216 217 @Block() 218 public static class SpecimenCollectionComponent extends BackboneElement implements IBaseBackboneElement { 219 /** 220 * Person who collected the specimen. 221 */ 222 @Child(name = "collector", type = { Practitioner.class, 223 PractitionerRole.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 224 @Description(shortDefinition = "Who collected the specimen", formalDefinition = "Person who collected the specimen.") 225 protected Reference collector; 226 227 /** 228 * The actual object that is the target of the reference (Person who collected 229 * the specimen.) 230 */ 231 protected Resource collectorTarget; 232 233 /** 234 * Time when specimen was collected from subject - the physiologically relevant 235 * time. 236 */ 237 @Child(name = "collected", type = { DateTimeType.class, 238 Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 239 @Description(shortDefinition = "Collection time", formalDefinition = "Time when specimen was collected from subject - the physiologically relevant time.") 240 protected Type collected; 241 242 /** 243 * The span of time over which the collection of a specimen occurred. 244 */ 245 @Child(name = "duration", type = { Duration.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 246 @Description(shortDefinition = "How long it took to collect specimen", formalDefinition = "The span of time over which the collection of a specimen occurred.") 247 protected Duration duration; 248 249 /** 250 * The quantity of specimen collected; for instance the volume of a blood 251 * sample, or the physical measurement of an anatomic pathology sample. 252 */ 253 @Child(name = "quantity", type = { Quantity.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 254 @Description(shortDefinition = "The quantity of specimen collected", formalDefinition = "The quantity of specimen collected; for instance the volume of a blood sample, or the physical measurement of an anatomic pathology sample.") 255 protected Quantity quantity; 256 257 /** 258 * A coded value specifying the technique that is used to perform the procedure. 259 */ 260 @Child(name = "method", type = { 261 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 262 @Description(shortDefinition = "Technique used to perform collection", formalDefinition = "A coded value specifying the technique that is used to perform the procedure.") 263 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/specimen-collection-method") 264 protected CodeableConcept method; 265 266 /** 267 * Anatomical location from which the specimen was collected (if subject is a 268 * patient). This is the target site. This element is not used for environmental 269 * specimens. 270 */ 271 @Child(name = "bodySite", type = { 272 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 273 @Description(shortDefinition = "Anatomical collection site", formalDefinition = "Anatomical location from which the specimen was collected (if subject is a patient). This is the target site. This element is not used for environmental specimens.") 274 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/body-site") 275 protected CodeableConcept bodySite; 276 277 /** 278 * Abstinence or reduction from some or all food, drink, or both, for a period 279 * of time prior to sample collection. 280 */ 281 @Child(name = "fastingStatus", type = { CodeableConcept.class, 282 Duration.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 283 @Description(shortDefinition = "Whether or how long patient abstained from food and/or drink", formalDefinition = "Abstinence or reduction from some or all food, drink, or both, for a period of time prior to sample collection.") 284 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v2-0916") 285 protected Type fastingStatus; 286 287 private static final long serialVersionUID = -719430195L; 288 289 /** 290 * Constructor 291 */ 292 public SpecimenCollectionComponent() { 293 super(); 294 } 295 296 /** 297 * @return {@link #collector} (Person who collected the specimen.) 298 */ 299 public Reference getCollector() { 300 if (this.collector == null) 301 if (Configuration.errorOnAutoCreate()) 302 throw new Error("Attempt to auto-create SpecimenCollectionComponent.collector"); 303 else if (Configuration.doAutoCreate()) 304 this.collector = new Reference(); // cc 305 return this.collector; 306 } 307 308 public boolean hasCollector() { 309 return this.collector != null && !this.collector.isEmpty(); 310 } 311 312 /** 313 * @param value {@link #collector} (Person who collected the specimen.) 314 */ 315 public SpecimenCollectionComponent setCollector(Reference value) { 316 this.collector = value; 317 return this; 318 } 319 320 /** 321 * @return {@link #collector} The actual object that is the target of the 322 * reference. The reference library doesn't populate this, but you can 323 * use it to hold the resource if you resolve it. (Person who collected 324 * the specimen.) 325 */ 326 public Resource getCollectorTarget() { 327 return this.collectorTarget; 328 } 329 330 /** 331 * @param value {@link #collector} The actual object that is the target of the 332 * reference. The reference library doesn't use these, but you can 333 * use it to hold the resource if you resolve it. (Person who 334 * collected the specimen.) 335 */ 336 public SpecimenCollectionComponent setCollectorTarget(Resource value) { 337 this.collectorTarget = value; 338 return this; 339 } 340 341 /** 342 * @return {@link #collected} (Time when specimen was collected from subject - 343 * the physiologically relevant time.) 344 */ 345 public Type getCollected() { 346 return this.collected; 347 } 348 349 /** 350 * @return {@link #collected} (Time when specimen was collected from subject - 351 * the physiologically relevant time.) 352 */ 353 public DateTimeType getCollectedDateTimeType() throws FHIRException { 354 if (this.collected == null) 355 this.collected = new DateTimeType(); 356 if (!(this.collected instanceof DateTimeType)) 357 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 358 + this.collected.getClass().getName() + " was encountered"); 359 return (DateTimeType) this.collected; 360 } 361 362 public boolean hasCollectedDateTimeType() { 363 return this != null && this.collected instanceof DateTimeType; 364 } 365 366 /** 367 * @return {@link #collected} (Time when specimen was collected from subject - 368 * the physiologically relevant time.) 369 */ 370 public Period getCollectedPeriod() throws FHIRException { 371 if (this.collected == null) 372 this.collected = new Period(); 373 if (!(this.collected instanceof Period)) 374 throw new FHIRException("Type mismatch: the type Period was expected, but " 375 + this.collected.getClass().getName() + " was encountered"); 376 return (Period) this.collected; 377 } 378 379 public boolean hasCollectedPeriod() { 380 return this != null && this.collected instanceof Period; 381 } 382 383 public boolean hasCollected() { 384 return this.collected != null && !this.collected.isEmpty(); 385 } 386 387 /** 388 * @param value {@link #collected} (Time when specimen was collected from 389 * subject - the physiologically relevant time.) 390 */ 391 public SpecimenCollectionComponent setCollected(Type value) { 392 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 393 throw new Error("Not the right type for Specimen.collection.collected[x]: " + value.fhirType()); 394 this.collected = value; 395 return this; 396 } 397 398 /** 399 * @return {@link #duration} (The span of time over which the collection of a 400 * specimen occurred.) 401 */ 402 public Duration getDuration() { 403 if (this.duration == null) 404 if (Configuration.errorOnAutoCreate()) 405 throw new Error("Attempt to auto-create SpecimenCollectionComponent.duration"); 406 else if (Configuration.doAutoCreate()) 407 this.duration = new Duration(); // cc 408 return this.duration; 409 } 410 411 public boolean hasDuration() { 412 return this.duration != null && !this.duration.isEmpty(); 413 } 414 415 /** 416 * @param value {@link #duration} (The span of time over which the collection of 417 * a specimen occurred.) 418 */ 419 public SpecimenCollectionComponent setDuration(Duration value) { 420 this.duration = value; 421 return this; 422 } 423 424 /** 425 * @return {@link #quantity} (The quantity of specimen collected; for instance 426 * the volume of a blood sample, or the physical measurement of an 427 * anatomic pathology sample.) 428 */ 429 public Quantity getQuantity() { 430 if (this.quantity == null) 431 if (Configuration.errorOnAutoCreate()) 432 throw new Error("Attempt to auto-create SpecimenCollectionComponent.quantity"); 433 else if (Configuration.doAutoCreate()) 434 this.quantity = new Quantity(); // cc 435 return this.quantity; 436 } 437 438 public boolean hasQuantity() { 439 return this.quantity != null && !this.quantity.isEmpty(); 440 } 441 442 /** 443 * @param value {@link #quantity} (The quantity of specimen collected; for 444 * instance the volume of a blood sample, or the physical 445 * measurement of an anatomic pathology sample.) 446 */ 447 public SpecimenCollectionComponent setQuantity(Quantity value) { 448 this.quantity = value; 449 return this; 450 } 451 452 /** 453 * @return {@link #method} (A coded value specifying the technique that is used 454 * to perform the procedure.) 455 */ 456 public CodeableConcept getMethod() { 457 if (this.method == null) 458 if (Configuration.errorOnAutoCreate()) 459 throw new Error("Attempt to auto-create SpecimenCollectionComponent.method"); 460 else if (Configuration.doAutoCreate()) 461 this.method = new CodeableConcept(); // cc 462 return this.method; 463 } 464 465 public boolean hasMethod() { 466 return this.method != null && !this.method.isEmpty(); 467 } 468 469 /** 470 * @param value {@link #method} (A coded value specifying the technique that is 471 * used to perform the procedure.) 472 */ 473 public SpecimenCollectionComponent setMethod(CodeableConcept value) { 474 this.method = value; 475 return this; 476 } 477 478 /** 479 * @return {@link #bodySite} (Anatomical location from which the specimen was 480 * collected (if subject is a patient). This is the target site. This 481 * element is not used for environmental specimens.) 482 */ 483 public CodeableConcept getBodySite() { 484 if (this.bodySite == null) 485 if (Configuration.errorOnAutoCreate()) 486 throw new Error("Attempt to auto-create SpecimenCollectionComponent.bodySite"); 487 else if (Configuration.doAutoCreate()) 488 this.bodySite = new CodeableConcept(); // cc 489 return this.bodySite; 490 } 491 492 public boolean hasBodySite() { 493 return this.bodySite != null && !this.bodySite.isEmpty(); 494 } 495 496 /** 497 * @param value {@link #bodySite} (Anatomical location from which the specimen 498 * was collected (if subject is a patient). This is the target 499 * site. This element is not used for environmental specimens.) 500 */ 501 public SpecimenCollectionComponent setBodySite(CodeableConcept value) { 502 this.bodySite = value; 503 return this; 504 } 505 506 /** 507 * @return {@link #fastingStatus} (Abstinence or reduction from some or all 508 * food, drink, or both, for a period of time prior to sample 509 * collection.) 510 */ 511 public Type getFastingStatus() { 512 return this.fastingStatus; 513 } 514 515 /** 516 * @return {@link #fastingStatus} (Abstinence or reduction from some or all 517 * food, drink, or both, for a period of time prior to sample 518 * collection.) 519 */ 520 public CodeableConcept getFastingStatusCodeableConcept() throws FHIRException { 521 if (this.fastingStatus == null) 522 this.fastingStatus = new CodeableConcept(); 523 if (!(this.fastingStatus instanceof CodeableConcept)) 524 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 525 + this.fastingStatus.getClass().getName() + " was encountered"); 526 return (CodeableConcept) this.fastingStatus; 527 } 528 529 public boolean hasFastingStatusCodeableConcept() { 530 return this != null && this.fastingStatus instanceof CodeableConcept; 531 } 532 533 /** 534 * @return {@link #fastingStatus} (Abstinence or reduction from some or all 535 * food, drink, or both, for a period of time prior to sample 536 * collection.) 537 */ 538 public Duration getFastingStatusDuration() throws FHIRException { 539 if (this.fastingStatus == null) 540 this.fastingStatus = new Duration(); 541 if (!(this.fastingStatus instanceof Duration)) 542 throw new FHIRException("Type mismatch: the type Duration was expected, but " 543 + this.fastingStatus.getClass().getName() + " was encountered"); 544 return (Duration) this.fastingStatus; 545 } 546 547 public boolean hasFastingStatusDuration() { 548 return this != null && this.fastingStatus instanceof Duration; 549 } 550 551 public boolean hasFastingStatus() { 552 return this.fastingStatus != null && !this.fastingStatus.isEmpty(); 553 } 554 555 /** 556 * @param value {@link #fastingStatus} (Abstinence or reduction from some or all 557 * food, drink, or both, for a period of time prior to sample 558 * collection.) 559 */ 560 public SpecimenCollectionComponent setFastingStatus(Type value) { 561 if (value != null && !(value instanceof CodeableConcept || value instanceof Duration)) 562 throw new Error("Not the right type for Specimen.collection.fastingStatus[x]: " + value.fhirType()); 563 this.fastingStatus = value; 564 return this; 565 } 566 567 protected void listChildren(List<Property> children) { 568 super.listChildren(children); 569 children.add(new Property("collector", "Reference(Practitioner|PractitionerRole)", 570 "Person who collected the specimen.", 0, 1, collector)); 571 children.add(new Property("collected[x]", "dateTime|Period", 572 "Time when specimen was collected from subject - the physiologically relevant time.", 0, 1, collected)); 573 children.add(new Property("duration", "Duration", 574 "The span of time over which the collection of a specimen occurred.", 0, 1, duration)); 575 children.add(new Property("quantity", "SimpleQuantity", 576 "The quantity of specimen collected; for instance the volume of a blood sample, or the physical measurement of an anatomic pathology sample.", 577 0, 1, quantity)); 578 children.add(new Property("method", "CodeableConcept", 579 "A coded value specifying the technique that is used to perform the procedure.", 0, 1, method)); 580 children.add(new Property("bodySite", "CodeableConcept", 581 "Anatomical location from which the specimen was collected (if subject is a patient). This is the target site. This element is not used for environmental specimens.", 582 0, 1, bodySite)); 583 children.add(new Property("fastingStatus[x]", "CodeableConcept|Duration", 584 "Abstinence or reduction from some or all food, drink, or both, for a period of time prior to sample collection.", 585 0, 1, fastingStatus)); 586 } 587 588 @Override 589 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 590 switch (_hash) { 591 case 1883491469: 592 /* collector */ return new Property("collector", "Reference(Practitioner|PractitionerRole)", 593 "Person who collected the specimen.", 0, 1, collector); 594 case 1632037015: 595 /* collected[x] */ return new Property("collected[x]", "dateTime|Period", 596 "Time when specimen was collected from subject - the physiologically relevant time.", 0, 1, collected); 597 case 1883491145: 598 /* collected */ return new Property("collected[x]", "dateTime|Period", 599 "Time when specimen was collected from subject - the physiologically relevant time.", 0, 1, collected); 600 case 2005009924: 601 /* collectedDateTime */ return new Property("collected[x]", "dateTime|Period", 602 "Time when specimen was collected from subject - the physiologically relevant time.", 0, 1, collected); 603 case 653185642: 604 /* collectedPeriod */ return new Property("collected[x]", "dateTime|Period", 605 "Time when specimen was collected from subject - the physiologically relevant time.", 0, 1, collected); 606 case -1992012396: 607 /* duration */ return new Property("duration", "Duration", 608 "The span of time over which the collection of a specimen occurred.", 0, 1, duration); 609 case -1285004149: 610 /* quantity */ return new Property("quantity", "SimpleQuantity", 611 "The quantity of specimen collected; for instance the volume of a blood sample, or the physical measurement of an anatomic pathology sample.", 612 0, 1, quantity); 613 case -1077554975: 614 /* method */ return new Property("method", "CodeableConcept", 615 "A coded value specifying the technique that is used to perform the procedure.", 0, 1, method); 616 case 1702620169: 617 /* bodySite */ return new Property("bodySite", "CodeableConcept", 618 "Anatomical location from which the specimen was collected (if subject is a patient). This is the target site. This element is not used for environmental specimens.", 619 0, 1, bodySite); 620 case -570577944: 621 /* fastingStatus[x] */ return new Property("fastingStatus[x]", "CodeableConcept|Duration", 622 "Abstinence or reduction from some or all food, drink, or both, for a period of time prior to sample collection.", 623 0, 1, fastingStatus); 624 case -701550184: 625 /* fastingStatus */ return new Property("fastingStatus[x]", "CodeableConcept|Duration", 626 "Abstinence or reduction from some or all food, drink, or both, for a period of time prior to sample collection.", 627 0, 1, fastingStatus); 628 case -1153232151: 629 /* fastingStatusCodeableConcept */ return new Property("fastingStatus[x]", "CodeableConcept|Duration", 630 "Abstinence or reduction from some or all food, drink, or both, for a period of time prior to sample collection.", 631 0, 1, fastingStatus); 632 case -433140916: 633 /* fastingStatusDuration */ return new Property("fastingStatus[x]", "CodeableConcept|Duration", 634 "Abstinence or reduction from some or all food, drink, or both, for a period of time prior to sample collection.", 635 0, 1, fastingStatus); 636 default: 637 return super.getNamedProperty(_hash, _name, _checkValid); 638 } 639 640 } 641 642 @Override 643 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 644 switch (hash) { 645 case 1883491469: 646 /* collector */ return this.collector == null ? new Base[0] : new Base[] { this.collector }; // Reference 647 case 1883491145: 648 /* collected */ return this.collected == null ? new Base[0] : new Base[] { this.collected }; // Type 649 case -1992012396: 650 /* duration */ return this.duration == null ? new Base[0] : new Base[] { this.duration }; // Duration 651 case -1285004149: 652 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 653 case -1077554975: 654 /* method */ return this.method == null ? new Base[0] : new Base[] { this.method }; // CodeableConcept 655 case 1702620169: 656 /* bodySite */ return this.bodySite == null ? new Base[0] : new Base[] { this.bodySite }; // CodeableConcept 657 case -701550184: 658 /* fastingStatus */ return this.fastingStatus == null ? new Base[0] : new Base[] { this.fastingStatus }; // Type 659 default: 660 return super.getProperty(hash, name, checkValid); 661 } 662 663 } 664 665 @Override 666 public Base setProperty(int hash, String name, Base value) throws FHIRException { 667 switch (hash) { 668 case 1883491469: // collector 669 this.collector = castToReference(value); // Reference 670 return value; 671 case 1883491145: // collected 672 this.collected = castToType(value); // Type 673 return value; 674 case -1992012396: // duration 675 this.duration = castToDuration(value); // Duration 676 return value; 677 case -1285004149: // quantity 678 this.quantity = castToQuantity(value); // Quantity 679 return value; 680 case -1077554975: // method 681 this.method = castToCodeableConcept(value); // CodeableConcept 682 return value; 683 case 1702620169: // bodySite 684 this.bodySite = castToCodeableConcept(value); // CodeableConcept 685 return value; 686 case -701550184: // fastingStatus 687 this.fastingStatus = castToType(value); // Type 688 return value; 689 default: 690 return super.setProperty(hash, name, value); 691 } 692 693 } 694 695 @Override 696 public Base setProperty(String name, Base value) throws FHIRException { 697 if (name.equals("collector")) { 698 this.collector = castToReference(value); // Reference 699 } else if (name.equals("collected[x]")) { 700 this.collected = castToType(value); // Type 701 } else if (name.equals("duration")) { 702 this.duration = castToDuration(value); // Duration 703 } else if (name.equals("quantity")) { 704 this.quantity = castToQuantity(value); // Quantity 705 } else if (name.equals("method")) { 706 this.method = castToCodeableConcept(value); // CodeableConcept 707 } else if (name.equals("bodySite")) { 708 this.bodySite = castToCodeableConcept(value); // CodeableConcept 709 } else if (name.equals("fastingStatus[x]")) { 710 this.fastingStatus = castToType(value); // Type 711 } else 712 return super.setProperty(name, value); 713 return value; 714 } 715 716 @Override 717 public void removeChild(String name, Base value) throws FHIRException { 718 if (name.equals("collector")) { 719 this.collector = null; 720 } else if (name.equals("collected[x]")) { 721 this.collected = null; 722 } else if (name.equals("duration")) { 723 this.duration = null; 724 } else if (name.equals("quantity")) { 725 this.quantity = null; 726 } else if (name.equals("method")) { 727 this.method = null; 728 } else if (name.equals("bodySite")) { 729 this.bodySite = null; 730 } else if (name.equals("fastingStatus[x]")) { 731 this.fastingStatus = null; 732 } else 733 super.removeChild(name, value); 734 735 } 736 737 @Override 738 public Base makeProperty(int hash, String name) throws FHIRException { 739 switch (hash) { 740 case 1883491469: 741 return getCollector(); 742 case 1632037015: 743 return getCollected(); 744 case 1883491145: 745 return getCollected(); 746 case -1992012396: 747 return getDuration(); 748 case -1285004149: 749 return getQuantity(); 750 case -1077554975: 751 return getMethod(); 752 case 1702620169: 753 return getBodySite(); 754 case -570577944: 755 return getFastingStatus(); 756 case -701550184: 757 return getFastingStatus(); 758 default: 759 return super.makeProperty(hash, name); 760 } 761 762 } 763 764 @Override 765 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 766 switch (hash) { 767 case 1883491469: 768 /* collector */ return new String[] { "Reference" }; 769 case 1883491145: 770 /* collected */ return new String[] { "dateTime", "Period" }; 771 case -1992012396: 772 /* duration */ return new String[] { "Duration" }; 773 case -1285004149: 774 /* quantity */ return new String[] { "SimpleQuantity" }; 775 case -1077554975: 776 /* method */ return new String[] { "CodeableConcept" }; 777 case 1702620169: 778 /* bodySite */ return new String[] { "CodeableConcept" }; 779 case -701550184: 780 /* fastingStatus */ return new String[] { "CodeableConcept", "Duration" }; 781 default: 782 return super.getTypesForProperty(hash, name); 783 } 784 785 } 786 787 @Override 788 public Base addChild(String name) throws FHIRException { 789 if (name.equals("collector")) { 790 this.collector = new Reference(); 791 return this.collector; 792 } else if (name.equals("collectedDateTime")) { 793 this.collected = new DateTimeType(); 794 return this.collected; 795 } else if (name.equals("collectedPeriod")) { 796 this.collected = new Period(); 797 return this.collected; 798 } else if (name.equals("duration")) { 799 this.duration = new Duration(); 800 return this.duration; 801 } else if (name.equals("quantity")) { 802 this.quantity = new Quantity(); 803 return this.quantity; 804 } else if (name.equals("method")) { 805 this.method = new CodeableConcept(); 806 return this.method; 807 } else if (name.equals("bodySite")) { 808 this.bodySite = new CodeableConcept(); 809 return this.bodySite; 810 } else if (name.equals("fastingStatusCodeableConcept")) { 811 this.fastingStatus = new CodeableConcept(); 812 return this.fastingStatus; 813 } else if (name.equals("fastingStatusDuration")) { 814 this.fastingStatus = new Duration(); 815 return this.fastingStatus; 816 } else 817 return super.addChild(name); 818 } 819 820 public SpecimenCollectionComponent copy() { 821 SpecimenCollectionComponent dst = new SpecimenCollectionComponent(); 822 copyValues(dst); 823 return dst; 824 } 825 826 public void copyValues(SpecimenCollectionComponent dst) { 827 super.copyValues(dst); 828 dst.collector = collector == null ? null : collector.copy(); 829 dst.collected = collected == null ? null : collected.copy(); 830 dst.duration = duration == null ? null : duration.copy(); 831 dst.quantity = quantity == null ? null : quantity.copy(); 832 dst.method = method == null ? null : method.copy(); 833 dst.bodySite = bodySite == null ? null : bodySite.copy(); 834 dst.fastingStatus = fastingStatus == null ? null : fastingStatus.copy(); 835 } 836 837 @Override 838 public boolean equalsDeep(Base other_) { 839 if (!super.equalsDeep(other_)) 840 return false; 841 if (!(other_ instanceof SpecimenCollectionComponent)) 842 return false; 843 SpecimenCollectionComponent o = (SpecimenCollectionComponent) other_; 844 return compareDeep(collector, o.collector, true) && compareDeep(collected, o.collected, true) 845 && compareDeep(duration, o.duration, true) && compareDeep(quantity, o.quantity, true) 846 && compareDeep(method, o.method, true) && compareDeep(bodySite, o.bodySite, true) 847 && compareDeep(fastingStatus, o.fastingStatus, true); 848 } 849 850 @Override 851 public boolean equalsShallow(Base other_) { 852 if (!super.equalsShallow(other_)) 853 return false; 854 if (!(other_ instanceof SpecimenCollectionComponent)) 855 return false; 856 SpecimenCollectionComponent o = (SpecimenCollectionComponent) other_; 857 return true; 858 } 859 860 public boolean isEmpty() { 861 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(collector, collected, duration, quantity, method, 862 bodySite, fastingStatus); 863 } 864 865 public String fhirType() { 866 return "Specimen.collection"; 867 868 } 869 870 } 871 872 @Block() 873 public static class SpecimenProcessingComponent extends BackboneElement implements IBaseBackboneElement { 874 /** 875 * Textual description of procedure. 876 */ 877 @Child(name = "description", type = { 878 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 879 @Description(shortDefinition = "Textual description of procedure", formalDefinition = "Textual description of procedure.") 880 protected StringType description; 881 882 /** 883 * A coded value specifying the procedure used to process the specimen. 884 */ 885 @Child(name = "procedure", type = { 886 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 887 @Description(shortDefinition = "Indicates the treatment step applied to the specimen", formalDefinition = "A coded value specifying the procedure used to process the specimen.") 888 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/specimen-processing-procedure") 889 protected CodeableConcept procedure; 890 891 /** 892 * Material used in the processing step. 893 */ 894 @Child(name = "additive", type = { 895 Substance.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 896 @Description(shortDefinition = "Material used in the processing step", formalDefinition = "Material used in the processing step.") 897 protected List<Reference> additive; 898 /** 899 * The actual objects that are the target of the reference (Material used in the 900 * processing step.) 901 */ 902 protected List<Substance> additiveTarget; 903 904 /** 905 * A record of the time or period when the specimen processing occurred. For 906 * example the time of sample fixation or the period of time the sample was in 907 * formalin. 908 */ 909 @Child(name = "time", type = { DateTimeType.class, 910 Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 911 @Description(shortDefinition = "Date and time of specimen processing", formalDefinition = "A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.") 912 protected Type time; 913 914 private static final long serialVersionUID = 1467214742L; 915 916 /** 917 * Constructor 918 */ 919 public SpecimenProcessingComponent() { 920 super(); 921 } 922 923 /** 924 * @return {@link #description} (Textual description of procedure.). This is the 925 * underlying object with id, value and extensions. The accessor 926 * "getDescription" gives direct access to the value 927 */ 928 public StringType getDescriptionElement() { 929 if (this.description == null) 930 if (Configuration.errorOnAutoCreate()) 931 throw new Error("Attempt to auto-create SpecimenProcessingComponent.description"); 932 else if (Configuration.doAutoCreate()) 933 this.description = new StringType(); // bb 934 return this.description; 935 } 936 937 public boolean hasDescriptionElement() { 938 return this.description != null && !this.description.isEmpty(); 939 } 940 941 public boolean hasDescription() { 942 return this.description != null && !this.description.isEmpty(); 943 } 944 945 /** 946 * @param value {@link #description} (Textual description of procedure.). This 947 * is the underlying object with id, value and extensions. The 948 * accessor "getDescription" gives direct access to the value 949 */ 950 public SpecimenProcessingComponent setDescriptionElement(StringType value) { 951 this.description = value; 952 return this; 953 } 954 955 /** 956 * @return Textual description of procedure. 957 */ 958 public String getDescription() { 959 return this.description == null ? null : this.description.getValue(); 960 } 961 962 /** 963 * @param value Textual description of procedure. 964 */ 965 public SpecimenProcessingComponent setDescription(String value) { 966 if (Utilities.noString(value)) 967 this.description = null; 968 else { 969 if (this.description == null) 970 this.description = new StringType(); 971 this.description.setValue(value); 972 } 973 return this; 974 } 975 976 /** 977 * @return {@link #procedure} (A coded value specifying the procedure used to 978 * process the specimen.) 979 */ 980 public CodeableConcept getProcedure() { 981 if (this.procedure == null) 982 if (Configuration.errorOnAutoCreate()) 983 throw new Error("Attempt to auto-create SpecimenProcessingComponent.procedure"); 984 else if (Configuration.doAutoCreate()) 985 this.procedure = new CodeableConcept(); // cc 986 return this.procedure; 987 } 988 989 public boolean hasProcedure() { 990 return this.procedure != null && !this.procedure.isEmpty(); 991 } 992 993 /** 994 * @param value {@link #procedure} (A coded value specifying the procedure used 995 * to process the specimen.) 996 */ 997 public SpecimenProcessingComponent setProcedure(CodeableConcept value) { 998 this.procedure = value; 999 return this; 1000 } 1001 1002 /** 1003 * @return {@link #additive} (Material used in the processing step.) 1004 */ 1005 public List<Reference> getAdditive() { 1006 if (this.additive == null) 1007 this.additive = new ArrayList<Reference>(); 1008 return this.additive; 1009 } 1010 1011 /** 1012 * @return Returns a reference to <code>this</code> for easy method chaining 1013 */ 1014 public SpecimenProcessingComponent setAdditive(List<Reference> theAdditive) { 1015 this.additive = theAdditive; 1016 return this; 1017 } 1018 1019 public boolean hasAdditive() { 1020 if (this.additive == null) 1021 return false; 1022 for (Reference item : this.additive) 1023 if (!item.isEmpty()) 1024 return true; 1025 return false; 1026 } 1027 1028 public Reference addAdditive() { // 3 1029 Reference t = new Reference(); 1030 if (this.additive == null) 1031 this.additive = new ArrayList<Reference>(); 1032 this.additive.add(t); 1033 return t; 1034 } 1035 1036 public SpecimenProcessingComponent addAdditive(Reference t) { // 3 1037 if (t == null) 1038 return this; 1039 if (this.additive == null) 1040 this.additive = new ArrayList<Reference>(); 1041 this.additive.add(t); 1042 return this; 1043 } 1044 1045 /** 1046 * @return The first repetition of repeating field {@link #additive}, creating 1047 * it if it does not already exist 1048 */ 1049 public Reference getAdditiveFirstRep() { 1050 if (getAdditive().isEmpty()) { 1051 addAdditive(); 1052 } 1053 return getAdditive().get(0); 1054 } 1055 1056 /** 1057 * @deprecated Use Reference#setResource(IBaseResource) instead 1058 */ 1059 @Deprecated 1060 public List<Substance> getAdditiveTarget() { 1061 if (this.additiveTarget == null) 1062 this.additiveTarget = new ArrayList<Substance>(); 1063 return this.additiveTarget; 1064 } 1065 1066 /** 1067 * @deprecated Use Reference#setResource(IBaseResource) instead 1068 */ 1069 @Deprecated 1070 public Substance addAdditiveTarget() { 1071 Substance r = new Substance(); 1072 if (this.additiveTarget == null) 1073 this.additiveTarget = new ArrayList<Substance>(); 1074 this.additiveTarget.add(r); 1075 return r; 1076 } 1077 1078 /** 1079 * @return {@link #time} (A record of the time or period when the specimen 1080 * processing occurred. For example the time of sample fixation or the 1081 * period of time the sample was in formalin.) 1082 */ 1083 public Type getTime() { 1084 return this.time; 1085 } 1086 1087 /** 1088 * @return {@link #time} (A record of the time or period when the specimen 1089 * processing occurred. For example the time of sample fixation or the 1090 * period of time the sample was in formalin.) 1091 */ 1092 public DateTimeType getTimeDateTimeType() throws FHIRException { 1093 if (this.time == null) 1094 this.time = new DateTimeType(); 1095 if (!(this.time instanceof DateTimeType)) 1096 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1097 + this.time.getClass().getName() + " was encountered"); 1098 return (DateTimeType) this.time; 1099 } 1100 1101 public boolean hasTimeDateTimeType() { 1102 return this != null && this.time instanceof DateTimeType; 1103 } 1104 1105 /** 1106 * @return {@link #time} (A record of the time or period when the specimen 1107 * processing occurred. For example the time of sample fixation or the 1108 * period of time the sample was in formalin.) 1109 */ 1110 public Period getTimePeriod() throws FHIRException { 1111 if (this.time == null) 1112 this.time = new Period(); 1113 if (!(this.time instanceof Period)) 1114 throw new FHIRException( 1115 "Type mismatch: the type Period was expected, but " + this.time.getClass().getName() + " was encountered"); 1116 return (Period) this.time; 1117 } 1118 1119 public boolean hasTimePeriod() { 1120 return this != null && this.time instanceof Period; 1121 } 1122 1123 public boolean hasTime() { 1124 return this.time != null && !this.time.isEmpty(); 1125 } 1126 1127 /** 1128 * @param value {@link #time} (A record of the time or period when the specimen 1129 * processing occurred. For example the time of sample fixation or 1130 * the period of time the sample was in formalin.) 1131 */ 1132 public SpecimenProcessingComponent setTime(Type value) { 1133 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1134 throw new Error("Not the right type for Specimen.processing.time[x]: " + value.fhirType()); 1135 this.time = value; 1136 return this; 1137 } 1138 1139 protected void listChildren(List<Property> children) { 1140 super.listChildren(children); 1141 children.add(new Property("description", "string", "Textual description of procedure.", 0, 1, description)); 1142 children.add(new Property("procedure", "CodeableConcept", 1143 "A coded value specifying the procedure used to process the specimen.", 0, 1, procedure)); 1144 children.add(new Property("additive", "Reference(Substance)", "Material used in the processing step.", 0, 1145 java.lang.Integer.MAX_VALUE, additive)); 1146 children.add(new Property("time[x]", "dateTime|Period", 1147 "A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.", 1148 0, 1, time)); 1149 } 1150 1151 @Override 1152 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1153 switch (_hash) { 1154 case -1724546052: 1155 /* description */ return new Property("description", "string", "Textual description of procedure.", 0, 1, 1156 description); 1157 case -1095204141: 1158 /* procedure */ return new Property("procedure", "CodeableConcept", 1159 "A coded value specifying the procedure used to process the specimen.", 0, 1, procedure); 1160 case -1226589236: 1161 /* additive */ return new Property("additive", "Reference(Substance)", "Material used in the processing step.", 1162 0, java.lang.Integer.MAX_VALUE, additive); 1163 case -1313930605: 1164 /* time[x] */ return new Property("time[x]", "dateTime|Period", 1165 "A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.", 1166 0, 1, time); 1167 case 3560141: 1168 /* time */ return new Property("time[x]", "dateTime|Period", 1169 "A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.", 1170 0, 1, time); 1171 case 2135345544: 1172 /* timeDateTime */ return new Property("time[x]", "dateTime|Period", 1173 "A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.", 1174 0, 1, time); 1175 case 693544686: 1176 /* timePeriod */ return new Property("time[x]", "dateTime|Period", 1177 "A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.", 1178 0, 1, time); 1179 default: 1180 return super.getNamedProperty(_hash, _name, _checkValid); 1181 } 1182 1183 } 1184 1185 @Override 1186 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1187 switch (hash) { 1188 case -1724546052: 1189 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1190 case -1095204141: 1191 /* procedure */ return this.procedure == null ? new Base[0] : new Base[] { this.procedure }; // CodeableConcept 1192 case -1226589236: 1193 /* additive */ return this.additive == null ? new Base[0] 1194 : this.additive.toArray(new Base[this.additive.size()]); // Reference 1195 case 3560141: 1196 /* time */ return this.time == null ? new Base[0] : new Base[] { this.time }; // Type 1197 default: 1198 return super.getProperty(hash, name, checkValid); 1199 } 1200 1201 } 1202 1203 @Override 1204 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1205 switch (hash) { 1206 case -1724546052: // description 1207 this.description = castToString(value); // StringType 1208 return value; 1209 case -1095204141: // procedure 1210 this.procedure = castToCodeableConcept(value); // CodeableConcept 1211 return value; 1212 case -1226589236: // additive 1213 this.getAdditive().add(castToReference(value)); // Reference 1214 return value; 1215 case 3560141: // time 1216 this.time = castToType(value); // Type 1217 return value; 1218 default: 1219 return super.setProperty(hash, name, value); 1220 } 1221 1222 } 1223 1224 @Override 1225 public Base setProperty(String name, Base value) throws FHIRException { 1226 if (name.equals("description")) { 1227 this.description = castToString(value); // StringType 1228 } else if (name.equals("procedure")) { 1229 this.procedure = castToCodeableConcept(value); // CodeableConcept 1230 } else if (name.equals("additive")) { 1231 this.getAdditive().add(castToReference(value)); 1232 } else if (name.equals("time[x]")) { 1233 this.time = castToType(value); // Type 1234 } else 1235 return super.setProperty(name, value); 1236 return value; 1237 } 1238 1239 @Override 1240 public void removeChild(String name, Base value) throws FHIRException { 1241 if (name.equals("description")) { 1242 this.description = null; 1243 } else if (name.equals("procedure")) { 1244 this.procedure = null; 1245 } else if (name.equals("additive")) { 1246 this.getAdditive().remove(castToReference(value)); 1247 } else if (name.equals("time[x]")) { 1248 this.time = null; 1249 } else 1250 super.removeChild(name, value); 1251 1252 } 1253 1254 @Override 1255 public Base makeProperty(int hash, String name) throws FHIRException { 1256 switch (hash) { 1257 case -1724546052: 1258 return getDescriptionElement(); 1259 case -1095204141: 1260 return getProcedure(); 1261 case -1226589236: 1262 return addAdditive(); 1263 case -1313930605: 1264 return getTime(); 1265 case 3560141: 1266 return getTime(); 1267 default: 1268 return super.makeProperty(hash, name); 1269 } 1270 1271 } 1272 1273 @Override 1274 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1275 switch (hash) { 1276 case -1724546052: 1277 /* description */ return new String[] { "string" }; 1278 case -1095204141: 1279 /* procedure */ return new String[] { "CodeableConcept" }; 1280 case -1226589236: 1281 /* additive */ return new String[] { "Reference" }; 1282 case 3560141: 1283 /* time */ return new String[] { "dateTime", "Period" }; 1284 default: 1285 return super.getTypesForProperty(hash, name); 1286 } 1287 1288 } 1289 1290 @Override 1291 public Base addChild(String name) throws FHIRException { 1292 if (name.equals("description")) { 1293 throw new FHIRException("Cannot call addChild on a singleton property Specimen.description"); 1294 } else if (name.equals("procedure")) { 1295 this.procedure = new CodeableConcept(); 1296 return this.procedure; 1297 } else if (name.equals("additive")) { 1298 return addAdditive(); 1299 } else if (name.equals("timeDateTime")) { 1300 this.time = new DateTimeType(); 1301 return this.time; 1302 } else if (name.equals("timePeriod")) { 1303 this.time = new Period(); 1304 return this.time; 1305 } else 1306 return super.addChild(name); 1307 } 1308 1309 public SpecimenProcessingComponent copy() { 1310 SpecimenProcessingComponent dst = new SpecimenProcessingComponent(); 1311 copyValues(dst); 1312 return dst; 1313 } 1314 1315 public void copyValues(SpecimenProcessingComponent dst) { 1316 super.copyValues(dst); 1317 dst.description = description == null ? null : description.copy(); 1318 dst.procedure = procedure == null ? null : procedure.copy(); 1319 if (additive != null) { 1320 dst.additive = new ArrayList<Reference>(); 1321 for (Reference i : additive) 1322 dst.additive.add(i.copy()); 1323 } 1324 ; 1325 dst.time = time == null ? null : time.copy(); 1326 } 1327 1328 @Override 1329 public boolean equalsDeep(Base other_) { 1330 if (!super.equalsDeep(other_)) 1331 return false; 1332 if (!(other_ instanceof SpecimenProcessingComponent)) 1333 return false; 1334 SpecimenProcessingComponent o = (SpecimenProcessingComponent) other_; 1335 return compareDeep(description, o.description, true) && compareDeep(procedure, o.procedure, true) 1336 && compareDeep(additive, o.additive, true) && compareDeep(time, o.time, true); 1337 } 1338 1339 @Override 1340 public boolean equalsShallow(Base other_) { 1341 if (!super.equalsShallow(other_)) 1342 return false; 1343 if (!(other_ instanceof SpecimenProcessingComponent)) 1344 return false; 1345 SpecimenProcessingComponent o = (SpecimenProcessingComponent) other_; 1346 return compareValues(description, o.description, true); 1347 } 1348 1349 public boolean isEmpty() { 1350 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, procedure, additive, time); 1351 } 1352 1353 public String fhirType() { 1354 return "Specimen.processing"; 1355 1356 } 1357 1358 } 1359 1360 @Block() 1361 public static class SpecimenContainerComponent extends BackboneElement implements IBaseBackboneElement { 1362 /** 1363 * Id for container. There may be multiple; a manufacturer's bar code, lab 1364 * assigned identifier, etc. The container ID may differ from the specimen id in 1365 * some circumstances. 1366 */ 1367 @Child(name = "identifier", type = { 1368 Identifier.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1369 @Description(shortDefinition = "Id for the container", formalDefinition = "Id for container. There may be multiple; a manufacturer's bar code, lab assigned identifier, etc. The container ID may differ from the specimen id in some circumstances.") 1370 protected List<Identifier> identifier; 1371 1372 /** 1373 * Textual description of the container. 1374 */ 1375 @Child(name = "description", type = { 1376 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1377 @Description(shortDefinition = "Textual description of the container", formalDefinition = "Textual description of the container.") 1378 protected StringType description; 1379 1380 /** 1381 * The type of container associated with the specimen (e.g. slide, aliquot, 1382 * etc.). 1383 */ 1384 @Child(name = "type", type = { 1385 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1386 @Description(shortDefinition = "Kind of container directly associated with specimen", formalDefinition = "The type of container associated with the specimen (e.g. slide, aliquot, etc.).") 1387 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/specimen-container-type") 1388 protected CodeableConcept type; 1389 1390 /** 1391 * The capacity (volume or other measure) the container may contain. 1392 */ 1393 @Child(name = "capacity", type = { Quantity.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1394 @Description(shortDefinition = "Container volume or size", formalDefinition = "The capacity (volume or other measure) the container may contain.") 1395 protected Quantity capacity; 1396 1397 /** 1398 * The quantity of specimen in the container; may be volume, dimensions, or 1399 * other appropriate measurements, depending on the specimen type. 1400 */ 1401 @Child(name = "specimenQuantity", type = { 1402 Quantity.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1403 @Description(shortDefinition = "Quantity of specimen within container", formalDefinition = "The quantity of specimen in the container; may be volume, dimensions, or other appropriate measurements, depending on the specimen type.") 1404 protected Quantity specimenQuantity; 1405 1406 /** 1407 * Introduced substance to preserve, maintain or enhance the specimen. Examples: 1408 * Formalin, Citrate, EDTA. 1409 */ 1410 @Child(name = "additive", type = { CodeableConcept.class, 1411 Substance.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 1412 @Description(shortDefinition = "Additive associated with container", formalDefinition = "Introduced substance to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.") 1413 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v2-0371") 1414 protected Type additive; 1415 1416 private static final long serialVersionUID = -1608132325L; 1417 1418 /** 1419 * Constructor 1420 */ 1421 public SpecimenContainerComponent() { 1422 super(); 1423 } 1424 1425 /** 1426 * @return {@link #identifier} (Id for container. There may be multiple; a 1427 * manufacturer's bar code, lab assigned identifier, etc. The container 1428 * ID may differ from the specimen id in some circumstances.) 1429 */ 1430 public List<Identifier> getIdentifier() { 1431 if (this.identifier == null) 1432 this.identifier = new ArrayList<Identifier>(); 1433 return this.identifier; 1434 } 1435 1436 /** 1437 * @return Returns a reference to <code>this</code> for easy method chaining 1438 */ 1439 public SpecimenContainerComponent setIdentifier(List<Identifier> theIdentifier) { 1440 this.identifier = theIdentifier; 1441 return this; 1442 } 1443 1444 public boolean hasIdentifier() { 1445 if (this.identifier == null) 1446 return false; 1447 for (Identifier item : this.identifier) 1448 if (!item.isEmpty()) 1449 return true; 1450 return false; 1451 } 1452 1453 public Identifier addIdentifier() { // 3 1454 Identifier t = new Identifier(); 1455 if (this.identifier == null) 1456 this.identifier = new ArrayList<Identifier>(); 1457 this.identifier.add(t); 1458 return t; 1459 } 1460 1461 public SpecimenContainerComponent addIdentifier(Identifier t) { // 3 1462 if (t == null) 1463 return this; 1464 if (this.identifier == null) 1465 this.identifier = new ArrayList<Identifier>(); 1466 this.identifier.add(t); 1467 return this; 1468 } 1469 1470 /** 1471 * @return The first repetition of repeating field {@link #identifier}, creating 1472 * it if it does not already exist 1473 */ 1474 public Identifier getIdentifierFirstRep() { 1475 if (getIdentifier().isEmpty()) { 1476 addIdentifier(); 1477 } 1478 return getIdentifier().get(0); 1479 } 1480 1481 /** 1482 * @return {@link #description} (Textual description of the container.). This is 1483 * the underlying object with id, value and extensions. The accessor 1484 * "getDescription" gives direct access to the value 1485 */ 1486 public StringType getDescriptionElement() { 1487 if (this.description == null) 1488 if (Configuration.errorOnAutoCreate()) 1489 throw new Error("Attempt to auto-create SpecimenContainerComponent.description"); 1490 else if (Configuration.doAutoCreate()) 1491 this.description = new StringType(); // bb 1492 return this.description; 1493 } 1494 1495 public boolean hasDescriptionElement() { 1496 return this.description != null && !this.description.isEmpty(); 1497 } 1498 1499 public boolean hasDescription() { 1500 return this.description != null && !this.description.isEmpty(); 1501 } 1502 1503 /** 1504 * @param value {@link #description} (Textual description of the container.). 1505 * This is the underlying object with id, value and extensions. The 1506 * accessor "getDescription" gives direct access to the value 1507 */ 1508 public SpecimenContainerComponent setDescriptionElement(StringType value) { 1509 this.description = value; 1510 return this; 1511 } 1512 1513 /** 1514 * @return Textual description of the container. 1515 */ 1516 public String getDescription() { 1517 return this.description == null ? null : this.description.getValue(); 1518 } 1519 1520 /** 1521 * @param value Textual description of the container. 1522 */ 1523 public SpecimenContainerComponent setDescription(String value) { 1524 if (Utilities.noString(value)) 1525 this.description = null; 1526 else { 1527 if (this.description == null) 1528 this.description = new StringType(); 1529 this.description.setValue(value); 1530 } 1531 return this; 1532 } 1533 1534 /** 1535 * @return {@link #type} (The type of container associated with the specimen 1536 * (e.g. slide, aliquot, etc.).) 1537 */ 1538 public CodeableConcept getType() { 1539 if (this.type == null) 1540 if (Configuration.errorOnAutoCreate()) 1541 throw new Error("Attempt to auto-create SpecimenContainerComponent.type"); 1542 else if (Configuration.doAutoCreate()) 1543 this.type = new CodeableConcept(); // cc 1544 return this.type; 1545 } 1546 1547 public boolean hasType() { 1548 return this.type != null && !this.type.isEmpty(); 1549 } 1550 1551 /** 1552 * @param value {@link #type} (The type of container associated with the 1553 * specimen (e.g. slide, aliquot, etc.).) 1554 */ 1555 public SpecimenContainerComponent setType(CodeableConcept value) { 1556 this.type = value; 1557 return this; 1558 } 1559 1560 /** 1561 * @return {@link #capacity} (The capacity (volume or other measure) the 1562 * container may contain.) 1563 */ 1564 public Quantity getCapacity() { 1565 if (this.capacity == null) 1566 if (Configuration.errorOnAutoCreate()) 1567 throw new Error("Attempt to auto-create SpecimenContainerComponent.capacity"); 1568 else if (Configuration.doAutoCreate()) 1569 this.capacity = new Quantity(); // cc 1570 return this.capacity; 1571 } 1572 1573 public boolean hasCapacity() { 1574 return this.capacity != null && !this.capacity.isEmpty(); 1575 } 1576 1577 /** 1578 * @param value {@link #capacity} (The capacity (volume or other measure) the 1579 * container may contain.) 1580 */ 1581 public SpecimenContainerComponent setCapacity(Quantity value) { 1582 this.capacity = value; 1583 return this; 1584 } 1585 1586 /** 1587 * @return {@link #specimenQuantity} (The quantity of specimen in the container; 1588 * may be volume, dimensions, or other appropriate measurements, 1589 * depending on the specimen type.) 1590 */ 1591 public Quantity getSpecimenQuantity() { 1592 if (this.specimenQuantity == null) 1593 if (Configuration.errorOnAutoCreate()) 1594 throw new Error("Attempt to auto-create SpecimenContainerComponent.specimenQuantity"); 1595 else if (Configuration.doAutoCreate()) 1596 this.specimenQuantity = new Quantity(); // cc 1597 return this.specimenQuantity; 1598 } 1599 1600 public boolean hasSpecimenQuantity() { 1601 return this.specimenQuantity != null && !this.specimenQuantity.isEmpty(); 1602 } 1603 1604 /** 1605 * @param value {@link #specimenQuantity} (The quantity of specimen in the 1606 * container; may be volume, dimensions, or other appropriate 1607 * measurements, depending on the specimen type.) 1608 */ 1609 public SpecimenContainerComponent setSpecimenQuantity(Quantity value) { 1610 this.specimenQuantity = value; 1611 return this; 1612 } 1613 1614 /** 1615 * @return {@link #additive} (Introduced substance to preserve, maintain or 1616 * enhance the specimen. Examples: Formalin, Citrate, EDTA.) 1617 */ 1618 public Type getAdditive() { 1619 return this.additive; 1620 } 1621 1622 /** 1623 * @return {@link #additive} (Introduced substance to preserve, maintain or 1624 * enhance the specimen. Examples: Formalin, Citrate, EDTA.) 1625 */ 1626 public CodeableConcept getAdditiveCodeableConcept() throws FHIRException { 1627 if (this.additive == null) 1628 this.additive = new CodeableConcept(); 1629 if (!(this.additive instanceof CodeableConcept)) 1630 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1631 + this.additive.getClass().getName() + " was encountered"); 1632 return (CodeableConcept) this.additive; 1633 } 1634 1635 public boolean hasAdditiveCodeableConcept() { 1636 return this != null && this.additive instanceof CodeableConcept; 1637 } 1638 1639 /** 1640 * @return {@link #additive} (Introduced substance to preserve, maintain or 1641 * enhance the specimen. Examples: Formalin, Citrate, EDTA.) 1642 */ 1643 public Reference getAdditiveReference() throws FHIRException { 1644 if (this.additive == null) 1645 this.additive = new Reference(); 1646 if (!(this.additive instanceof Reference)) 1647 throw new FHIRException("Type mismatch: the type Reference was expected, but " 1648 + this.additive.getClass().getName() + " was encountered"); 1649 return (Reference) this.additive; 1650 } 1651 1652 public boolean hasAdditiveReference() { 1653 return this != null && this.additive instanceof Reference; 1654 } 1655 1656 public boolean hasAdditive() { 1657 return this.additive != null && !this.additive.isEmpty(); 1658 } 1659 1660 /** 1661 * @param value {@link #additive} (Introduced substance to preserve, maintain or 1662 * enhance the specimen. Examples: Formalin, Citrate, EDTA.) 1663 */ 1664 public SpecimenContainerComponent setAdditive(Type value) { 1665 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1666 throw new Error("Not the right type for Specimen.container.additive[x]: " + value.fhirType()); 1667 this.additive = value; 1668 return this; 1669 } 1670 1671 protected void listChildren(List<Property> children) { 1672 super.listChildren(children); 1673 children.add(new Property("identifier", "Identifier", 1674 "Id for container. There may be multiple; a manufacturer's bar code, lab assigned identifier, etc. The container ID may differ from the specimen id in some circumstances.", 1675 0, java.lang.Integer.MAX_VALUE, identifier)); 1676 children.add(new Property("description", "string", "Textual description of the container.", 0, 1, description)); 1677 children.add(new Property("type", "CodeableConcept", 1678 "The type of container associated with the specimen (e.g. slide, aliquot, etc.).", 0, 1, type)); 1679 children.add(new Property("capacity", "SimpleQuantity", 1680 "The capacity (volume or other measure) the container may contain.", 0, 1, capacity)); 1681 children.add(new Property("specimenQuantity", "SimpleQuantity", 1682 "The quantity of specimen in the container; may be volume, dimensions, or other appropriate measurements, depending on the specimen type.", 1683 0, 1, specimenQuantity)); 1684 children.add(new Property("additive[x]", "CodeableConcept|Reference(Substance)", 1685 "Introduced substance to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 0, 1686 1, additive)); 1687 } 1688 1689 @Override 1690 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1691 switch (_hash) { 1692 case -1618432855: 1693 /* identifier */ return new Property("identifier", "Identifier", 1694 "Id for container. There may be multiple; a manufacturer's bar code, lab assigned identifier, etc. The container ID may differ from the specimen id in some circumstances.", 1695 0, java.lang.Integer.MAX_VALUE, identifier); 1696 case -1724546052: 1697 /* description */ return new Property("description", "string", "Textual description of the container.", 0, 1, 1698 description); 1699 case 3575610: 1700 /* type */ return new Property("type", "CodeableConcept", 1701 "The type of container associated with the specimen (e.g. slide, aliquot, etc.).", 0, 1, type); 1702 case -67824454: 1703 /* capacity */ return new Property("capacity", "SimpleQuantity", 1704 "The capacity (volume or other measure) the container may contain.", 0, 1, capacity); 1705 case 1485980595: 1706 /* specimenQuantity */ return new Property("specimenQuantity", "SimpleQuantity", 1707 "The quantity of specimen in the container; may be volume, dimensions, or other appropriate measurements, depending on the specimen type.", 1708 0, 1, specimenQuantity); 1709 case 261915956: 1710 /* additive[x] */ return new Property("additive[x]", "CodeableConcept|Reference(Substance)", 1711 "Introduced substance to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 0, 1712 1, additive); 1713 case -1226589236: 1714 /* additive */ return new Property("additive[x]", "CodeableConcept|Reference(Substance)", 1715 "Introduced substance to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 0, 1716 1, additive); 1717 case 1330272821: 1718 /* additiveCodeableConcept */ return new Property("additive[x]", "CodeableConcept|Reference(Substance)", 1719 "Introduced substance to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 0, 1720 1, additive); 1721 case -386783009: 1722 /* additiveReference */ return new Property("additive[x]", "CodeableConcept|Reference(Substance)", 1723 "Introduced substance to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 0, 1724 1, additive); 1725 default: 1726 return super.getNamedProperty(_hash, _name, _checkValid); 1727 } 1728 1729 } 1730 1731 @Override 1732 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1733 switch (hash) { 1734 case -1618432855: 1735 /* identifier */ return this.identifier == null ? new Base[0] 1736 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1737 case -1724546052: 1738 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1739 case 3575610: 1740 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1741 case -67824454: 1742 /* capacity */ return this.capacity == null ? new Base[0] : new Base[] { this.capacity }; // Quantity 1743 case 1485980595: 1744 /* specimenQuantity */ return this.specimenQuantity == null ? new Base[0] 1745 : new Base[] { this.specimenQuantity }; // Quantity 1746 case -1226589236: 1747 /* additive */ return this.additive == null ? new Base[0] : new Base[] { this.additive }; // Type 1748 default: 1749 return super.getProperty(hash, name, checkValid); 1750 } 1751 1752 } 1753 1754 @Override 1755 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1756 switch (hash) { 1757 case -1618432855: // identifier 1758 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1759 return value; 1760 case -1724546052: // description 1761 this.description = castToString(value); // StringType 1762 return value; 1763 case 3575610: // type 1764 this.type = castToCodeableConcept(value); // CodeableConcept 1765 return value; 1766 case -67824454: // capacity 1767 this.capacity = castToQuantity(value); // Quantity 1768 return value; 1769 case 1485980595: // specimenQuantity 1770 this.specimenQuantity = castToQuantity(value); // Quantity 1771 return value; 1772 case -1226589236: // additive 1773 this.additive = castToType(value); // Type 1774 return value; 1775 default: 1776 return super.setProperty(hash, name, value); 1777 } 1778 1779 } 1780 1781 @Override 1782 public Base setProperty(String name, Base value) throws FHIRException { 1783 if (name.equals("identifier")) { 1784 this.getIdentifier().add(castToIdentifier(value)); 1785 } else if (name.equals("description")) { 1786 this.description = castToString(value); // StringType 1787 } else if (name.equals("type")) { 1788 this.type = castToCodeableConcept(value); // CodeableConcept 1789 } else if (name.equals("capacity")) { 1790 this.capacity = castToQuantity(value); // Quantity 1791 } else if (name.equals("specimenQuantity")) { 1792 this.specimenQuantity = castToQuantity(value); // Quantity 1793 } else if (name.equals("additive[x]")) { 1794 this.additive = castToType(value); // Type 1795 } else 1796 return super.setProperty(name, value); 1797 return value; 1798 } 1799 1800 @Override 1801 public void removeChild(String name, Base value) throws FHIRException { 1802 if (name.equals("identifier")) { 1803 this.getIdentifier().remove(castToIdentifier(value)); 1804 } else if (name.equals("description")) { 1805 this.description = null; 1806 } else if (name.equals("type")) { 1807 this.type = null; 1808 } else if (name.equals("capacity")) { 1809 this.capacity = null; 1810 } else if (name.equals("specimenQuantity")) { 1811 this.specimenQuantity = null; 1812 } else if (name.equals("additive[x]")) { 1813 this.additive = null; 1814 } else 1815 super.removeChild(name, value); 1816 1817 } 1818 1819 @Override 1820 public Base makeProperty(int hash, String name) throws FHIRException { 1821 switch (hash) { 1822 case -1618432855: 1823 return addIdentifier(); 1824 case -1724546052: 1825 return getDescriptionElement(); 1826 case 3575610: 1827 return getType(); 1828 case -67824454: 1829 return getCapacity(); 1830 case 1485980595: 1831 return getSpecimenQuantity(); 1832 case 261915956: 1833 return getAdditive(); 1834 case -1226589236: 1835 return getAdditive(); 1836 default: 1837 return super.makeProperty(hash, name); 1838 } 1839 1840 } 1841 1842 @Override 1843 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1844 switch (hash) { 1845 case -1618432855: 1846 /* identifier */ return new String[] { "Identifier" }; 1847 case -1724546052: 1848 /* description */ return new String[] { "string" }; 1849 case 3575610: 1850 /* type */ return new String[] { "CodeableConcept" }; 1851 case -67824454: 1852 /* capacity */ return new String[] { "SimpleQuantity" }; 1853 case 1485980595: 1854 /* specimenQuantity */ return new String[] { "SimpleQuantity" }; 1855 case -1226589236: 1856 /* additive */ return new String[] { "CodeableConcept", "Reference" }; 1857 default: 1858 return super.getTypesForProperty(hash, name); 1859 } 1860 1861 } 1862 1863 @Override 1864 public Base addChild(String name) throws FHIRException { 1865 if (name.equals("identifier")) { 1866 return addIdentifier(); 1867 } else if (name.equals("description")) { 1868 throw new FHIRException("Cannot call addChild on a singleton property Specimen.description"); 1869 } else if (name.equals("type")) { 1870 this.type = new CodeableConcept(); 1871 return this.type; 1872 } else if (name.equals("capacity")) { 1873 this.capacity = new Quantity(); 1874 return this.capacity; 1875 } else if (name.equals("specimenQuantity")) { 1876 this.specimenQuantity = new Quantity(); 1877 return this.specimenQuantity; 1878 } else if (name.equals("additiveCodeableConcept")) { 1879 this.additive = new CodeableConcept(); 1880 return this.additive; 1881 } else if (name.equals("additiveReference")) { 1882 this.additive = new Reference(); 1883 return this.additive; 1884 } else 1885 return super.addChild(name); 1886 } 1887 1888 public SpecimenContainerComponent copy() { 1889 SpecimenContainerComponent dst = new SpecimenContainerComponent(); 1890 copyValues(dst); 1891 return dst; 1892 } 1893 1894 public void copyValues(SpecimenContainerComponent dst) { 1895 super.copyValues(dst); 1896 if (identifier != null) { 1897 dst.identifier = new ArrayList<Identifier>(); 1898 for (Identifier i : identifier) 1899 dst.identifier.add(i.copy()); 1900 } 1901 ; 1902 dst.description = description == null ? null : description.copy(); 1903 dst.type = type == null ? null : type.copy(); 1904 dst.capacity = capacity == null ? null : capacity.copy(); 1905 dst.specimenQuantity = specimenQuantity == null ? null : specimenQuantity.copy(); 1906 dst.additive = additive == null ? null : additive.copy(); 1907 } 1908 1909 @Override 1910 public boolean equalsDeep(Base other_) { 1911 if (!super.equalsDeep(other_)) 1912 return false; 1913 if (!(other_ instanceof SpecimenContainerComponent)) 1914 return false; 1915 SpecimenContainerComponent o = (SpecimenContainerComponent) other_; 1916 return compareDeep(identifier, o.identifier, true) && compareDeep(description, o.description, true) 1917 && compareDeep(type, o.type, true) && compareDeep(capacity, o.capacity, true) 1918 && compareDeep(specimenQuantity, o.specimenQuantity, true) && compareDeep(additive, o.additive, true); 1919 } 1920 1921 @Override 1922 public boolean equalsShallow(Base other_) { 1923 if (!super.equalsShallow(other_)) 1924 return false; 1925 if (!(other_ instanceof SpecimenContainerComponent)) 1926 return false; 1927 SpecimenContainerComponent o = (SpecimenContainerComponent) other_; 1928 return compareValues(description, o.description, true); 1929 } 1930 1931 public boolean isEmpty() { 1932 return super.isEmpty() 1933 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, description, type, capacity, specimenQuantity, additive); 1934 } 1935 1936 public String fhirType() { 1937 return "Specimen.container"; 1938 1939 } 1940 1941 } 1942 1943 /** 1944 * Id for specimen. 1945 */ 1946 @Child(name = "identifier", type = { 1947 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1948 @Description(shortDefinition = "External Identifier", formalDefinition = "Id for specimen.") 1949 protected List<Identifier> identifier; 1950 1951 /** 1952 * The identifier assigned by the lab when accessioning specimen(s). This is not 1953 * necessarily the same as the specimen identifier, depending on local lab 1954 * procedures. 1955 */ 1956 @Child(name = "accessionIdentifier", type = { 1957 Identifier.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1958 @Description(shortDefinition = "Identifier assigned by the lab", formalDefinition = "The identifier assigned by the lab when accessioning specimen(s). This is not necessarily the same as the specimen identifier, depending on local lab procedures.") 1959 protected Identifier accessionIdentifier; 1960 1961 /** 1962 * The availability of the specimen. 1963 */ 1964 @Child(name = "status", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = true, summary = true) 1965 @Description(shortDefinition = "available | unavailable | unsatisfactory | entered-in-error", formalDefinition = "The availability of the specimen.") 1966 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/specimen-status") 1967 protected Enumeration<SpecimenStatus> status; 1968 1969 /** 1970 * The kind of material that forms the specimen. 1971 */ 1972 @Child(name = "type", type = { CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1973 @Description(shortDefinition = "Kind of material that forms the specimen", formalDefinition = "The kind of material that forms the specimen.") 1974 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v2-0487") 1975 protected CodeableConcept type; 1976 1977 /** 1978 * Where the specimen came from. This may be from patient(s), from a location 1979 * (e.g., the source of an environmental sample), or a sampling of a substance 1980 * or a device. 1981 */ 1982 @Child(name = "subject", type = { Patient.class, Group.class, Device.class, Substance.class, 1983 Location.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1984 @Description(shortDefinition = "Where the specimen came from. This may be from patient(s), from a location (e.g., the source of an environmental sample), or a sampling of a substance or a device", formalDefinition = "Where the specimen came from. This may be from patient(s), from a location (e.g., the source of an environmental sample), or a sampling of a substance or a device.") 1985 protected Reference subject; 1986 1987 /** 1988 * The actual object that is the target of the reference (Where the specimen 1989 * came from. This may be from patient(s), from a location (e.g., the source of 1990 * an environmental sample), or a sampling of a substance or a device.) 1991 */ 1992 protected Resource subjectTarget; 1993 1994 /** 1995 * Time when specimen was received for processing or testing. 1996 */ 1997 @Child(name = "receivedTime", type = { 1998 DateTimeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1999 @Description(shortDefinition = "The time when specimen was received for processing", formalDefinition = "Time when specimen was received for processing or testing.") 2000 protected DateTimeType receivedTime; 2001 2002 /** 2003 * Reference to the parent (source) specimen which is used when the specimen was 2004 * either derived from or a component of another specimen. 2005 */ 2006 @Child(name = "parent", type = { 2007 Specimen.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2008 @Description(shortDefinition = "Specimen from which this specimen originated", formalDefinition = "Reference to the parent (source) specimen which is used when the specimen was either derived from or a component of another specimen.") 2009 protected List<Reference> parent; 2010 /** 2011 * The actual objects that are the target of the reference (Reference to the 2012 * parent (source) specimen which is used when the specimen was either derived 2013 * from or a component of another specimen.) 2014 */ 2015 protected List<Specimen> parentTarget; 2016 2017 /** 2018 * Details concerning a service request that required a specimen to be 2019 * collected. 2020 */ 2021 @Child(name = "request", type = { 2022 ServiceRequest.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2023 @Description(shortDefinition = "Why the specimen was collected", formalDefinition = "Details concerning a service request that required a specimen to be collected.") 2024 protected List<Reference> request; 2025 /** 2026 * The actual objects that are the target of the reference (Details concerning a 2027 * service request that required a specimen to be collected.) 2028 */ 2029 protected List<ServiceRequest> requestTarget; 2030 2031 /** 2032 * Details concerning the specimen collection. 2033 */ 2034 @Child(name = "collection", type = {}, order = 8, min = 0, max = 1, modifier = false, summary = false) 2035 @Description(shortDefinition = "Collection details", formalDefinition = "Details concerning the specimen collection.") 2036 protected SpecimenCollectionComponent collection; 2037 2038 /** 2039 * Details concerning processing and processing steps for the specimen. 2040 */ 2041 @Child(name = "processing", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2042 @Description(shortDefinition = "Processing and processing step details", formalDefinition = "Details concerning processing and processing steps for the specimen.") 2043 protected List<SpecimenProcessingComponent> processing; 2044 2045 /** 2046 * The container holding the specimen. The recursive nature of containers; i.e. 2047 * blood in tube in tray in rack is not addressed here. 2048 */ 2049 @Child(name = "container", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2050 @Description(shortDefinition = "Direct container of specimen (tube/slide, etc.)", formalDefinition = "The container holding the specimen. The recursive nature of containers; i.e. blood in tube in tray in rack is not addressed here.") 2051 protected List<SpecimenContainerComponent> container; 2052 2053 /** 2054 * A mode or state of being that describes the nature of the specimen. 2055 */ 2056 @Child(name = "condition", type = { 2057 CodeableConcept.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2058 @Description(shortDefinition = "State of the specimen", formalDefinition = "A mode or state of being that describes the nature of the specimen.") 2059 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v2-0493") 2060 protected List<CodeableConcept> condition; 2061 2062 /** 2063 * To communicate any details or issues about the specimen or during the 2064 * specimen collection. (for example: broken vial, sent with patient, frozen). 2065 */ 2066 @Child(name = "note", type = { 2067 Annotation.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2068 @Description(shortDefinition = "Comments", formalDefinition = "To communicate any details or issues about the specimen or during the specimen collection. (for example: broken vial, sent with patient, frozen).") 2069 protected List<Annotation> note; 2070 2071 private static final long serialVersionUID = 1441502239L; 2072 2073 /** 2074 * Constructor 2075 */ 2076 public Specimen() { 2077 super(); 2078 } 2079 2080 /** 2081 * @return {@link #identifier} (Id for specimen.) 2082 */ 2083 public List<Identifier> getIdentifier() { 2084 if (this.identifier == null) 2085 this.identifier = new ArrayList<Identifier>(); 2086 return this.identifier; 2087 } 2088 2089 /** 2090 * @return Returns a reference to <code>this</code> for easy method chaining 2091 */ 2092 public Specimen setIdentifier(List<Identifier> theIdentifier) { 2093 this.identifier = theIdentifier; 2094 return this; 2095 } 2096 2097 public boolean hasIdentifier() { 2098 if (this.identifier == null) 2099 return false; 2100 for (Identifier item : this.identifier) 2101 if (!item.isEmpty()) 2102 return true; 2103 return false; 2104 } 2105 2106 public Identifier addIdentifier() { // 3 2107 Identifier t = new Identifier(); 2108 if (this.identifier == null) 2109 this.identifier = new ArrayList<Identifier>(); 2110 this.identifier.add(t); 2111 return t; 2112 } 2113 2114 public Specimen addIdentifier(Identifier t) { // 3 2115 if (t == null) 2116 return this; 2117 if (this.identifier == null) 2118 this.identifier = new ArrayList<Identifier>(); 2119 this.identifier.add(t); 2120 return this; 2121 } 2122 2123 /** 2124 * @return The first repetition of repeating field {@link #identifier}, creating 2125 * it if it does not already exist 2126 */ 2127 public Identifier getIdentifierFirstRep() { 2128 if (getIdentifier().isEmpty()) { 2129 addIdentifier(); 2130 } 2131 return getIdentifier().get(0); 2132 } 2133 2134 /** 2135 * @return {@link #accessionIdentifier} (The identifier assigned by the lab when 2136 * accessioning specimen(s). This is not necessarily the same as the 2137 * specimen identifier, depending on local lab procedures.) 2138 */ 2139 public Identifier getAccessionIdentifier() { 2140 if (this.accessionIdentifier == null) 2141 if (Configuration.errorOnAutoCreate()) 2142 throw new Error("Attempt to auto-create Specimen.accessionIdentifier"); 2143 else if (Configuration.doAutoCreate()) 2144 this.accessionIdentifier = new Identifier(); // cc 2145 return this.accessionIdentifier; 2146 } 2147 2148 public boolean hasAccessionIdentifier() { 2149 return this.accessionIdentifier != null && !this.accessionIdentifier.isEmpty(); 2150 } 2151 2152 /** 2153 * @param value {@link #accessionIdentifier} (The identifier assigned by the lab 2154 * when accessioning specimen(s). This is not necessarily the same 2155 * as the specimen identifier, depending on local lab procedures.) 2156 */ 2157 public Specimen setAccessionIdentifier(Identifier value) { 2158 this.accessionIdentifier = value; 2159 return this; 2160 } 2161 2162 /** 2163 * @return {@link #status} (The availability of the specimen.). This is the 2164 * underlying object with id, value and extensions. The accessor 2165 * "getStatus" gives direct access to the value 2166 */ 2167 public Enumeration<SpecimenStatus> getStatusElement() { 2168 if (this.status == null) 2169 if (Configuration.errorOnAutoCreate()) 2170 throw new Error("Attempt to auto-create Specimen.status"); 2171 else if (Configuration.doAutoCreate()) 2172 this.status = new Enumeration<SpecimenStatus>(new SpecimenStatusEnumFactory()); // bb 2173 return this.status; 2174 } 2175 2176 public boolean hasStatusElement() { 2177 return this.status != null && !this.status.isEmpty(); 2178 } 2179 2180 public boolean hasStatus() { 2181 return this.status != null && !this.status.isEmpty(); 2182 } 2183 2184 /** 2185 * @param value {@link #status} (The availability of the specimen.). This is the 2186 * underlying object with id, value and extensions. The accessor 2187 * "getStatus" gives direct access to the value 2188 */ 2189 public Specimen setStatusElement(Enumeration<SpecimenStatus> value) { 2190 this.status = value; 2191 return this; 2192 } 2193 2194 /** 2195 * @return The availability of the specimen. 2196 */ 2197 public SpecimenStatus getStatus() { 2198 return this.status == null ? null : this.status.getValue(); 2199 } 2200 2201 /** 2202 * @param value The availability of the specimen. 2203 */ 2204 public Specimen setStatus(SpecimenStatus value) { 2205 if (value == null) 2206 this.status = null; 2207 else { 2208 if (this.status == null) 2209 this.status = new Enumeration<SpecimenStatus>(new SpecimenStatusEnumFactory()); 2210 this.status.setValue(value); 2211 } 2212 return this; 2213 } 2214 2215 /** 2216 * @return {@link #type} (The kind of material that forms the specimen.) 2217 */ 2218 public CodeableConcept getType() { 2219 if (this.type == null) 2220 if (Configuration.errorOnAutoCreate()) 2221 throw new Error("Attempt to auto-create Specimen.type"); 2222 else if (Configuration.doAutoCreate()) 2223 this.type = new CodeableConcept(); // cc 2224 return this.type; 2225 } 2226 2227 public boolean hasType() { 2228 return this.type != null && !this.type.isEmpty(); 2229 } 2230 2231 /** 2232 * @param value {@link #type} (The kind of material that forms the specimen.) 2233 */ 2234 public Specimen setType(CodeableConcept value) { 2235 this.type = value; 2236 return this; 2237 } 2238 2239 /** 2240 * @return {@link #subject} (Where the specimen came from. This may be from 2241 * patient(s), from a location (e.g., the source of an environmental 2242 * sample), or a sampling of a substance or a device.) 2243 */ 2244 public Reference getSubject() { 2245 if (this.subject == null) 2246 if (Configuration.errorOnAutoCreate()) 2247 throw new Error("Attempt to auto-create Specimen.subject"); 2248 else if (Configuration.doAutoCreate()) 2249 this.subject = new Reference(); // cc 2250 return this.subject; 2251 } 2252 2253 public boolean hasSubject() { 2254 return this.subject != null && !this.subject.isEmpty(); 2255 } 2256 2257 /** 2258 * @param value {@link #subject} (Where the specimen came from. This may be from 2259 * patient(s), from a location (e.g., the source of an 2260 * environmental sample), or a sampling of a substance or a 2261 * device.) 2262 */ 2263 public Specimen setSubject(Reference value) { 2264 this.subject = value; 2265 return this; 2266 } 2267 2268 /** 2269 * @return {@link #subject} The actual object that is the target of the 2270 * reference. The reference library doesn't populate this, but you can 2271 * use it to hold the resource if you resolve it. (Where the specimen 2272 * came from. This may be from patient(s), from a location (e.g., the 2273 * source of an environmental sample), or a sampling of a substance or a 2274 * device.) 2275 */ 2276 public Resource getSubjectTarget() { 2277 return this.subjectTarget; 2278 } 2279 2280 /** 2281 * @param value {@link #subject} The actual object that is the target of the 2282 * reference. The reference library doesn't use these, but you can 2283 * use it to hold the resource if you resolve it. (Where the 2284 * specimen came from. This may be from patient(s), from a location 2285 * (e.g., the source of an environmental sample), or a sampling of 2286 * a substance or a device.) 2287 */ 2288 public Specimen setSubjectTarget(Resource value) { 2289 this.subjectTarget = value; 2290 return this; 2291 } 2292 2293 /** 2294 * @return {@link #receivedTime} (Time when specimen was received for processing 2295 * or testing.). This is the underlying object with id, value and 2296 * extensions. The accessor "getReceivedTime" gives direct access to the 2297 * value 2298 */ 2299 public DateTimeType getReceivedTimeElement() { 2300 if (this.receivedTime == null) 2301 if (Configuration.errorOnAutoCreate()) 2302 throw new Error("Attempt to auto-create Specimen.receivedTime"); 2303 else if (Configuration.doAutoCreate()) 2304 this.receivedTime = new DateTimeType(); // bb 2305 return this.receivedTime; 2306 } 2307 2308 public boolean hasReceivedTimeElement() { 2309 return this.receivedTime != null && !this.receivedTime.isEmpty(); 2310 } 2311 2312 public boolean hasReceivedTime() { 2313 return this.receivedTime != null && !this.receivedTime.isEmpty(); 2314 } 2315 2316 /** 2317 * @param value {@link #receivedTime} (Time when specimen was received for 2318 * processing or testing.). This is the underlying object with id, 2319 * value and extensions. The accessor "getReceivedTime" gives 2320 * direct access to the value 2321 */ 2322 public Specimen setReceivedTimeElement(DateTimeType value) { 2323 this.receivedTime = value; 2324 return this; 2325 } 2326 2327 /** 2328 * @return Time when specimen was received for processing or testing. 2329 */ 2330 public Date getReceivedTime() { 2331 return this.receivedTime == null ? null : this.receivedTime.getValue(); 2332 } 2333 2334 /** 2335 * @param value Time when specimen was received for processing or testing. 2336 */ 2337 public Specimen setReceivedTime(Date value) { 2338 if (value == null) 2339 this.receivedTime = null; 2340 else { 2341 if (this.receivedTime == null) 2342 this.receivedTime = new DateTimeType(); 2343 this.receivedTime.setValue(value); 2344 } 2345 return this; 2346 } 2347 2348 /** 2349 * @return {@link #parent} (Reference to the parent (source) specimen which is 2350 * used when the specimen was either derived from or a component of 2351 * another specimen.) 2352 */ 2353 public List<Reference> getParent() { 2354 if (this.parent == null) 2355 this.parent = new ArrayList<Reference>(); 2356 return this.parent; 2357 } 2358 2359 /** 2360 * @return Returns a reference to <code>this</code> for easy method chaining 2361 */ 2362 public Specimen setParent(List<Reference> theParent) { 2363 this.parent = theParent; 2364 return this; 2365 } 2366 2367 public boolean hasParent() { 2368 if (this.parent == null) 2369 return false; 2370 for (Reference item : this.parent) 2371 if (!item.isEmpty()) 2372 return true; 2373 return false; 2374 } 2375 2376 public Reference addParent() { // 3 2377 Reference t = new Reference(); 2378 if (this.parent == null) 2379 this.parent = new ArrayList<Reference>(); 2380 this.parent.add(t); 2381 return t; 2382 } 2383 2384 public Specimen addParent(Reference t) { // 3 2385 if (t == null) 2386 return this; 2387 if (this.parent == null) 2388 this.parent = new ArrayList<Reference>(); 2389 this.parent.add(t); 2390 return this; 2391 } 2392 2393 /** 2394 * @return The first repetition of repeating field {@link #parent}, creating it 2395 * if it does not already exist 2396 */ 2397 public Reference getParentFirstRep() { 2398 if (getParent().isEmpty()) { 2399 addParent(); 2400 } 2401 return getParent().get(0); 2402 } 2403 2404 /** 2405 * @deprecated Use Reference#setResource(IBaseResource) instead 2406 */ 2407 @Deprecated 2408 public List<Specimen> getParentTarget() { 2409 if (this.parentTarget == null) 2410 this.parentTarget = new ArrayList<Specimen>(); 2411 return this.parentTarget; 2412 } 2413 2414 /** 2415 * @deprecated Use Reference#setResource(IBaseResource) instead 2416 */ 2417 @Deprecated 2418 public Specimen addParentTarget() { 2419 Specimen r = new Specimen(); 2420 if (this.parentTarget == null) 2421 this.parentTarget = new ArrayList<Specimen>(); 2422 this.parentTarget.add(r); 2423 return r; 2424 } 2425 2426 /** 2427 * @return {@link #request} (Details concerning a service request that required 2428 * a specimen to be collected.) 2429 */ 2430 public List<Reference> getRequest() { 2431 if (this.request == null) 2432 this.request = new ArrayList<Reference>(); 2433 return this.request; 2434 } 2435 2436 /** 2437 * @return Returns a reference to <code>this</code> for easy method chaining 2438 */ 2439 public Specimen setRequest(List<Reference> theRequest) { 2440 this.request = theRequest; 2441 return this; 2442 } 2443 2444 public boolean hasRequest() { 2445 if (this.request == null) 2446 return false; 2447 for (Reference item : this.request) 2448 if (!item.isEmpty()) 2449 return true; 2450 return false; 2451 } 2452 2453 public Reference addRequest() { // 3 2454 Reference t = new Reference(); 2455 if (this.request == null) 2456 this.request = new ArrayList<Reference>(); 2457 this.request.add(t); 2458 return t; 2459 } 2460 2461 public Specimen addRequest(Reference t) { // 3 2462 if (t == null) 2463 return this; 2464 if (this.request == null) 2465 this.request = new ArrayList<Reference>(); 2466 this.request.add(t); 2467 return this; 2468 } 2469 2470 /** 2471 * @return The first repetition of repeating field {@link #request}, creating it 2472 * if it does not already exist 2473 */ 2474 public Reference getRequestFirstRep() { 2475 if (getRequest().isEmpty()) { 2476 addRequest(); 2477 } 2478 return getRequest().get(0); 2479 } 2480 2481 /** 2482 * @deprecated Use Reference#setResource(IBaseResource) instead 2483 */ 2484 @Deprecated 2485 public List<ServiceRequest> getRequestTarget() { 2486 if (this.requestTarget == null) 2487 this.requestTarget = new ArrayList<ServiceRequest>(); 2488 return this.requestTarget; 2489 } 2490 2491 /** 2492 * @deprecated Use Reference#setResource(IBaseResource) instead 2493 */ 2494 @Deprecated 2495 public ServiceRequest addRequestTarget() { 2496 ServiceRequest r = new ServiceRequest(); 2497 if (this.requestTarget == null) 2498 this.requestTarget = new ArrayList<ServiceRequest>(); 2499 this.requestTarget.add(r); 2500 return r; 2501 } 2502 2503 /** 2504 * @return {@link #collection} (Details concerning the specimen collection.) 2505 */ 2506 public SpecimenCollectionComponent getCollection() { 2507 if (this.collection == null) 2508 if (Configuration.errorOnAutoCreate()) 2509 throw new Error("Attempt to auto-create Specimen.collection"); 2510 else if (Configuration.doAutoCreate()) 2511 this.collection = new SpecimenCollectionComponent(); // cc 2512 return this.collection; 2513 } 2514 2515 public boolean hasCollection() { 2516 return this.collection != null && !this.collection.isEmpty(); 2517 } 2518 2519 /** 2520 * @param value {@link #collection} (Details concerning the specimen 2521 * collection.) 2522 */ 2523 public Specimen setCollection(SpecimenCollectionComponent value) { 2524 this.collection = value; 2525 return this; 2526 } 2527 2528 /** 2529 * @return {@link #processing} (Details concerning processing and processing 2530 * steps for the specimen.) 2531 */ 2532 public List<SpecimenProcessingComponent> getProcessing() { 2533 if (this.processing == null) 2534 this.processing = new ArrayList<SpecimenProcessingComponent>(); 2535 return this.processing; 2536 } 2537 2538 /** 2539 * @return Returns a reference to <code>this</code> for easy method chaining 2540 */ 2541 public Specimen setProcessing(List<SpecimenProcessingComponent> theProcessing) { 2542 this.processing = theProcessing; 2543 return this; 2544 } 2545 2546 public boolean hasProcessing() { 2547 if (this.processing == null) 2548 return false; 2549 for (SpecimenProcessingComponent item : this.processing) 2550 if (!item.isEmpty()) 2551 return true; 2552 return false; 2553 } 2554 2555 public SpecimenProcessingComponent addProcessing() { // 3 2556 SpecimenProcessingComponent t = new SpecimenProcessingComponent(); 2557 if (this.processing == null) 2558 this.processing = new ArrayList<SpecimenProcessingComponent>(); 2559 this.processing.add(t); 2560 return t; 2561 } 2562 2563 public Specimen addProcessing(SpecimenProcessingComponent t) { // 3 2564 if (t == null) 2565 return this; 2566 if (this.processing == null) 2567 this.processing = new ArrayList<SpecimenProcessingComponent>(); 2568 this.processing.add(t); 2569 return this; 2570 } 2571 2572 /** 2573 * @return The first repetition of repeating field {@link #processing}, creating 2574 * it if it does not already exist 2575 */ 2576 public SpecimenProcessingComponent getProcessingFirstRep() { 2577 if (getProcessing().isEmpty()) { 2578 addProcessing(); 2579 } 2580 return getProcessing().get(0); 2581 } 2582 2583 /** 2584 * @return {@link #container} (The container holding the specimen. The recursive 2585 * nature of containers; i.e. blood in tube in tray in rack is not 2586 * addressed here.) 2587 */ 2588 public List<SpecimenContainerComponent> getContainer() { 2589 if (this.container == null) 2590 this.container = new ArrayList<SpecimenContainerComponent>(); 2591 return this.container; 2592 } 2593 2594 /** 2595 * @return Returns a reference to <code>this</code> for easy method chaining 2596 */ 2597 public Specimen setContainer(List<SpecimenContainerComponent> theContainer) { 2598 this.container = theContainer; 2599 return this; 2600 } 2601 2602 public boolean hasContainer() { 2603 if (this.container == null) 2604 return false; 2605 for (SpecimenContainerComponent item : this.container) 2606 if (!item.isEmpty()) 2607 return true; 2608 return false; 2609 } 2610 2611 public SpecimenContainerComponent addContainer() { // 3 2612 SpecimenContainerComponent t = new SpecimenContainerComponent(); 2613 if (this.container == null) 2614 this.container = new ArrayList<SpecimenContainerComponent>(); 2615 this.container.add(t); 2616 return t; 2617 } 2618 2619 public Specimen addContainer(SpecimenContainerComponent t) { // 3 2620 if (t == null) 2621 return this; 2622 if (this.container == null) 2623 this.container = new ArrayList<SpecimenContainerComponent>(); 2624 this.container.add(t); 2625 return this; 2626 } 2627 2628 /** 2629 * @return The first repetition of repeating field {@link #container}, creating 2630 * it if it does not already exist 2631 */ 2632 public SpecimenContainerComponent getContainerFirstRep() { 2633 if (getContainer().isEmpty()) { 2634 addContainer(); 2635 } 2636 return getContainer().get(0); 2637 } 2638 2639 /** 2640 * @return {@link #condition} (A mode or state of being that describes the 2641 * nature of the specimen.) 2642 */ 2643 public List<CodeableConcept> getCondition() { 2644 if (this.condition == null) 2645 this.condition = new ArrayList<CodeableConcept>(); 2646 return this.condition; 2647 } 2648 2649 /** 2650 * @return Returns a reference to <code>this</code> for easy method chaining 2651 */ 2652 public Specimen setCondition(List<CodeableConcept> theCondition) { 2653 this.condition = theCondition; 2654 return this; 2655 } 2656 2657 public boolean hasCondition() { 2658 if (this.condition == null) 2659 return false; 2660 for (CodeableConcept item : this.condition) 2661 if (!item.isEmpty()) 2662 return true; 2663 return false; 2664 } 2665 2666 public CodeableConcept addCondition() { // 3 2667 CodeableConcept t = new CodeableConcept(); 2668 if (this.condition == null) 2669 this.condition = new ArrayList<CodeableConcept>(); 2670 this.condition.add(t); 2671 return t; 2672 } 2673 2674 public Specimen addCondition(CodeableConcept t) { // 3 2675 if (t == null) 2676 return this; 2677 if (this.condition == null) 2678 this.condition = new ArrayList<CodeableConcept>(); 2679 this.condition.add(t); 2680 return this; 2681 } 2682 2683 /** 2684 * @return The first repetition of repeating field {@link #condition}, creating 2685 * it if it does not already exist 2686 */ 2687 public CodeableConcept getConditionFirstRep() { 2688 if (getCondition().isEmpty()) { 2689 addCondition(); 2690 } 2691 return getCondition().get(0); 2692 } 2693 2694 /** 2695 * @return {@link #note} (To communicate any details or issues about the 2696 * specimen or during the specimen collection. (for example: broken 2697 * vial, sent with patient, frozen).) 2698 */ 2699 public List<Annotation> getNote() { 2700 if (this.note == null) 2701 this.note = new ArrayList<Annotation>(); 2702 return this.note; 2703 } 2704 2705 /** 2706 * @return Returns a reference to <code>this</code> for easy method chaining 2707 */ 2708 public Specimen setNote(List<Annotation> theNote) { 2709 this.note = theNote; 2710 return this; 2711 } 2712 2713 public boolean hasNote() { 2714 if (this.note == null) 2715 return false; 2716 for (Annotation item : this.note) 2717 if (!item.isEmpty()) 2718 return true; 2719 return false; 2720 } 2721 2722 public Annotation addNote() { // 3 2723 Annotation t = new Annotation(); 2724 if (this.note == null) 2725 this.note = new ArrayList<Annotation>(); 2726 this.note.add(t); 2727 return t; 2728 } 2729 2730 public Specimen addNote(Annotation t) { // 3 2731 if (t == null) 2732 return this; 2733 if (this.note == null) 2734 this.note = new ArrayList<Annotation>(); 2735 this.note.add(t); 2736 return this; 2737 } 2738 2739 /** 2740 * @return The first repetition of repeating field {@link #note}, creating it if 2741 * it does not already exist 2742 */ 2743 public Annotation getNoteFirstRep() { 2744 if (getNote().isEmpty()) { 2745 addNote(); 2746 } 2747 return getNote().get(0); 2748 } 2749 2750 protected void listChildren(List<Property> children) { 2751 super.listChildren(children); 2752 children 2753 .add(new Property("identifier", "Identifier", "Id for specimen.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2754 children.add(new Property("accessionIdentifier", "Identifier", 2755 "The identifier assigned by the lab when accessioning specimen(s). This is not necessarily the same as the specimen identifier, depending on local lab procedures.", 2756 0, 1, accessionIdentifier)); 2757 children.add(new Property("status", "code", "The availability of the specimen.", 0, 1, status)); 2758 children.add(new Property("type", "CodeableConcept", "The kind of material that forms the specimen.", 0, 1, type)); 2759 children.add(new Property("subject", "Reference(Patient|Group|Device|Substance|Location)", 2760 "Where the specimen came from. This may be from patient(s), from a location (e.g., the source of an environmental sample), or a sampling of a substance or a device.", 2761 0, 1, subject)); 2762 children.add(new Property("receivedTime", "dateTime", "Time when specimen was received for processing or testing.", 2763 0, 1, receivedTime)); 2764 children.add(new Property("parent", "Reference(Specimen)", 2765 "Reference to the parent (source) specimen which is used when the specimen was either derived from or a component of another specimen.", 2766 0, java.lang.Integer.MAX_VALUE, parent)); 2767 children.add(new Property("request", "Reference(ServiceRequest)", 2768 "Details concerning a service request that required a specimen to be collected.", 0, 2769 java.lang.Integer.MAX_VALUE, request)); 2770 children.add(new Property("collection", "", "Details concerning the specimen collection.", 0, 1, collection)); 2771 children.add(new Property("processing", "", "Details concerning processing and processing steps for the specimen.", 2772 0, java.lang.Integer.MAX_VALUE, processing)); 2773 children.add(new Property("container", "", 2774 "The container holding the specimen. The recursive nature of containers; i.e. blood in tube in tray in rack is not addressed here.", 2775 0, java.lang.Integer.MAX_VALUE, container)); 2776 children.add(new Property("condition", "CodeableConcept", 2777 "A mode or state of being that describes the nature of the specimen.", 0, java.lang.Integer.MAX_VALUE, 2778 condition)); 2779 children.add(new Property("note", "Annotation", 2780 "To communicate any details or issues about the specimen or during the specimen collection. (for example: broken vial, sent with patient, frozen).", 2781 0, java.lang.Integer.MAX_VALUE, note)); 2782 } 2783 2784 @Override 2785 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2786 switch (_hash) { 2787 case -1618432855: 2788 /* identifier */ return new Property("identifier", "Identifier", "Id for specimen.", 0, 2789 java.lang.Integer.MAX_VALUE, identifier); 2790 case 818734061: 2791 /* accessionIdentifier */ return new Property("accessionIdentifier", "Identifier", 2792 "The identifier assigned by the lab when accessioning specimen(s). This is not necessarily the same as the specimen identifier, depending on local lab procedures.", 2793 0, 1, accessionIdentifier); 2794 case -892481550: 2795 /* status */ return new Property("status", "code", "The availability of the specimen.", 0, 1, status); 2796 case 3575610: 2797 /* type */ return new Property("type", "CodeableConcept", "The kind of material that forms the specimen.", 0, 1, 2798 type); 2799 case -1867885268: 2800 /* subject */ return new Property("subject", "Reference(Patient|Group|Device|Substance|Location)", 2801 "Where the specimen came from. This may be from patient(s), from a location (e.g., the source of an environmental sample), or a sampling of a substance or a device.", 2802 0, 1, subject); 2803 case -767961010: 2804 /* receivedTime */ return new Property("receivedTime", "dateTime", 2805 "Time when specimen was received for processing or testing.", 0, 1, receivedTime); 2806 case -995424086: 2807 /* parent */ return new Property("parent", "Reference(Specimen)", 2808 "Reference to the parent (source) specimen which is used when the specimen was either derived from or a component of another specimen.", 2809 0, java.lang.Integer.MAX_VALUE, parent); 2810 case 1095692943: 2811 /* request */ return new Property("request", "Reference(ServiceRequest)", 2812 "Details concerning a service request that required a specimen to be collected.", 0, 2813 java.lang.Integer.MAX_VALUE, request); 2814 case -1741312354: 2815 /* collection */ return new Property("collection", "", "Details concerning the specimen collection.", 0, 1, 2816 collection); 2817 case 422194963: 2818 /* processing */ return new Property("processing", "", 2819 "Details concerning processing and processing steps for the specimen.", 0, java.lang.Integer.MAX_VALUE, 2820 processing); 2821 case -410956671: 2822 /* container */ return new Property("container", "", 2823 "The container holding the specimen. The recursive nature of containers; i.e. blood in tube in tray in rack is not addressed here.", 2824 0, java.lang.Integer.MAX_VALUE, container); 2825 case -861311717: 2826 /* condition */ return new Property("condition", "CodeableConcept", 2827 "A mode or state of being that describes the nature of the specimen.", 0, java.lang.Integer.MAX_VALUE, 2828 condition); 2829 case 3387378: 2830 /* note */ return new Property("note", "Annotation", 2831 "To communicate any details or issues about the specimen or during the specimen collection. (for example: broken vial, sent with patient, frozen).", 2832 0, java.lang.Integer.MAX_VALUE, note); 2833 default: 2834 return super.getNamedProperty(_hash, _name, _checkValid); 2835 } 2836 2837 } 2838 2839 @Override 2840 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2841 switch (hash) { 2842 case -1618432855: 2843 /* identifier */ return this.identifier == null ? new Base[0] 2844 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2845 case 818734061: 2846 /* accessionIdentifier */ return this.accessionIdentifier == null ? new Base[0] 2847 : new Base[] { this.accessionIdentifier }; // Identifier 2848 case -892481550: 2849 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<SpecimenStatus> 2850 case 3575610: 2851 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2852 case -1867885268: 2853 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 2854 case -767961010: 2855 /* receivedTime */ return this.receivedTime == null ? new Base[0] : new Base[] { this.receivedTime }; // DateTimeType 2856 case -995424086: 2857 /* parent */ return this.parent == null ? new Base[0] : this.parent.toArray(new Base[this.parent.size()]); // Reference 2858 case 1095692943: 2859 /* request */ return this.request == null ? new Base[0] : this.request.toArray(new Base[this.request.size()]); // Reference 2860 case -1741312354: 2861 /* collection */ return this.collection == null ? new Base[0] : new Base[] { this.collection }; // SpecimenCollectionComponent 2862 case 422194963: 2863 /* processing */ return this.processing == null ? new Base[0] 2864 : this.processing.toArray(new Base[this.processing.size()]); // SpecimenProcessingComponent 2865 case -410956671: 2866 /* container */ return this.container == null ? new Base[0] 2867 : this.container.toArray(new Base[this.container.size()]); // SpecimenContainerComponent 2868 case -861311717: 2869 /* condition */ return this.condition == null ? new Base[0] 2870 : this.condition.toArray(new Base[this.condition.size()]); // CodeableConcept 2871 case 3387378: 2872 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2873 default: 2874 return super.getProperty(hash, name, checkValid); 2875 } 2876 2877 } 2878 2879 @Override 2880 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2881 switch (hash) { 2882 case -1618432855: // identifier 2883 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2884 return value; 2885 case 818734061: // accessionIdentifier 2886 this.accessionIdentifier = castToIdentifier(value); // Identifier 2887 return value; 2888 case -892481550: // status 2889 value = new SpecimenStatusEnumFactory().fromType(castToCode(value)); 2890 this.status = (Enumeration) value; // Enumeration<SpecimenStatus> 2891 return value; 2892 case 3575610: // type 2893 this.type = castToCodeableConcept(value); // CodeableConcept 2894 return value; 2895 case -1867885268: // subject 2896 this.subject = castToReference(value); // Reference 2897 return value; 2898 case -767961010: // receivedTime 2899 this.receivedTime = castToDateTime(value); // DateTimeType 2900 return value; 2901 case -995424086: // parent 2902 this.getParent().add(castToReference(value)); // Reference 2903 return value; 2904 case 1095692943: // request 2905 this.getRequest().add(castToReference(value)); // Reference 2906 return value; 2907 case -1741312354: // collection 2908 this.collection = (SpecimenCollectionComponent) value; // SpecimenCollectionComponent 2909 return value; 2910 case 422194963: // processing 2911 this.getProcessing().add((SpecimenProcessingComponent) value); // SpecimenProcessingComponent 2912 return value; 2913 case -410956671: // container 2914 this.getContainer().add((SpecimenContainerComponent) value); // SpecimenContainerComponent 2915 return value; 2916 case -861311717: // condition 2917 this.getCondition().add(castToCodeableConcept(value)); // CodeableConcept 2918 return value; 2919 case 3387378: // note 2920 this.getNote().add(castToAnnotation(value)); // Annotation 2921 return value; 2922 default: 2923 return super.setProperty(hash, name, value); 2924 } 2925 2926 } 2927 2928 @Override 2929 public Base setProperty(String name, Base value) throws FHIRException { 2930 if (name.equals("identifier")) { 2931 this.getIdentifier().add(castToIdentifier(value)); 2932 } else if (name.equals("accessionIdentifier")) { 2933 this.accessionIdentifier = castToIdentifier(value); // Identifier 2934 } else if (name.equals("status")) { 2935 value = new SpecimenStatusEnumFactory().fromType(castToCode(value)); 2936 this.status = (Enumeration) value; // Enumeration<SpecimenStatus> 2937 } else if (name.equals("type")) { 2938 this.type = castToCodeableConcept(value); // CodeableConcept 2939 } else if (name.equals("subject")) { 2940 this.subject = castToReference(value); // Reference 2941 } else if (name.equals("receivedTime")) { 2942 this.receivedTime = castToDateTime(value); // DateTimeType 2943 } else if (name.equals("parent")) { 2944 this.getParent().add(castToReference(value)); 2945 } else if (name.equals("request")) { 2946 this.getRequest().add(castToReference(value)); 2947 } else if (name.equals("collection")) { 2948 this.collection = (SpecimenCollectionComponent) value; // SpecimenCollectionComponent 2949 } else if (name.equals("processing")) { 2950 this.getProcessing().add((SpecimenProcessingComponent) value); 2951 } else if (name.equals("container")) { 2952 this.getContainer().add((SpecimenContainerComponent) value); 2953 } else if (name.equals("condition")) { 2954 this.getCondition().add(castToCodeableConcept(value)); 2955 } else if (name.equals("note")) { 2956 this.getNote().add(castToAnnotation(value)); 2957 } else 2958 return super.setProperty(name, value); 2959 return value; 2960 } 2961 2962 @Override 2963 public void removeChild(String name, Base value) throws FHIRException { 2964 if (name.equals("identifier")) { 2965 this.getIdentifier().remove(castToIdentifier(value)); 2966 } else if (name.equals("accessionIdentifier")) { 2967 this.accessionIdentifier = null; 2968 } else if (name.equals("status")) { 2969 this.status = null; 2970 } else if (name.equals("type")) { 2971 this.type = null; 2972 } else if (name.equals("subject")) { 2973 this.subject = null; 2974 } else if (name.equals("receivedTime")) { 2975 this.receivedTime = null; 2976 } else if (name.equals("parent")) { 2977 this.getParent().remove(castToReference(value)); 2978 } else if (name.equals("request")) { 2979 this.getRequest().remove(castToReference(value)); 2980 } else if (name.equals("collection")) { 2981 this.collection = (SpecimenCollectionComponent) value; // SpecimenCollectionComponent 2982 } else if (name.equals("processing")) { 2983 this.getProcessing().remove((SpecimenProcessingComponent) value); 2984 } else if (name.equals("container")) { 2985 this.getContainer().remove((SpecimenContainerComponent) value); 2986 } else if (name.equals("condition")) { 2987 this.getCondition().remove(castToCodeableConcept(value)); 2988 } else if (name.equals("note")) { 2989 this.getNote().remove(castToAnnotation(value)); 2990 } else 2991 super.removeChild(name, value); 2992 2993 } 2994 2995 @Override 2996 public Base makeProperty(int hash, String name) throws FHIRException { 2997 switch (hash) { 2998 case -1618432855: 2999 return addIdentifier(); 3000 case 818734061: 3001 return getAccessionIdentifier(); 3002 case -892481550: 3003 return getStatusElement(); 3004 case 3575610: 3005 return getType(); 3006 case -1867885268: 3007 return getSubject(); 3008 case -767961010: 3009 return getReceivedTimeElement(); 3010 case -995424086: 3011 return addParent(); 3012 case 1095692943: 3013 return addRequest(); 3014 case -1741312354: 3015 return getCollection(); 3016 case 422194963: 3017 return addProcessing(); 3018 case -410956671: 3019 return addContainer(); 3020 case -861311717: 3021 return addCondition(); 3022 case 3387378: 3023 return addNote(); 3024 default: 3025 return super.makeProperty(hash, name); 3026 } 3027 3028 } 3029 3030 @Override 3031 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3032 switch (hash) { 3033 case -1618432855: 3034 /* identifier */ return new String[] { "Identifier" }; 3035 case 818734061: 3036 /* accessionIdentifier */ return new String[] { "Identifier" }; 3037 case -892481550: 3038 /* status */ return new String[] { "code" }; 3039 case 3575610: 3040 /* type */ return new String[] { "CodeableConcept" }; 3041 case -1867885268: 3042 /* subject */ return new String[] { "Reference" }; 3043 case -767961010: 3044 /* receivedTime */ return new String[] { "dateTime" }; 3045 case -995424086: 3046 /* parent */ return new String[] { "Reference" }; 3047 case 1095692943: 3048 /* request */ return new String[] { "Reference" }; 3049 case -1741312354: 3050 /* collection */ return new String[] {}; 3051 case 422194963: 3052 /* processing */ return new String[] {}; 3053 case -410956671: 3054 /* container */ return new String[] {}; 3055 case -861311717: 3056 /* condition */ return new String[] { "CodeableConcept" }; 3057 case 3387378: 3058 /* note */ return new String[] { "Annotation" }; 3059 default: 3060 return super.getTypesForProperty(hash, name); 3061 } 3062 3063 } 3064 3065 @Override 3066 public Base addChild(String name) throws FHIRException { 3067 if (name.equals("identifier")) { 3068 return addIdentifier(); 3069 } else if (name.equals("accessionIdentifier")) { 3070 this.accessionIdentifier = new Identifier(); 3071 return this.accessionIdentifier; 3072 } else if (name.equals("status")) { 3073 throw new FHIRException("Cannot call addChild on a singleton property Specimen.status"); 3074 } else if (name.equals("type")) { 3075 this.type = new CodeableConcept(); 3076 return this.type; 3077 } else if (name.equals("subject")) { 3078 this.subject = new Reference(); 3079 return this.subject; 3080 } else if (name.equals("receivedTime")) { 3081 throw new FHIRException("Cannot call addChild on a singleton property Specimen.receivedTime"); 3082 } else if (name.equals("parent")) { 3083 return addParent(); 3084 } else if (name.equals("request")) { 3085 return addRequest(); 3086 } else if (name.equals("collection")) { 3087 this.collection = new SpecimenCollectionComponent(); 3088 return this.collection; 3089 } else if (name.equals("processing")) { 3090 return addProcessing(); 3091 } else if (name.equals("container")) { 3092 return addContainer(); 3093 } else if (name.equals("condition")) { 3094 return addCondition(); 3095 } else if (name.equals("note")) { 3096 return addNote(); 3097 } else 3098 return super.addChild(name); 3099 } 3100 3101 public String fhirType() { 3102 return "Specimen"; 3103 3104 } 3105 3106 public Specimen copy() { 3107 Specimen dst = new Specimen(); 3108 copyValues(dst); 3109 return dst; 3110 } 3111 3112 public void copyValues(Specimen dst) { 3113 super.copyValues(dst); 3114 if (identifier != null) { 3115 dst.identifier = new ArrayList<Identifier>(); 3116 for (Identifier i : identifier) 3117 dst.identifier.add(i.copy()); 3118 } 3119 ; 3120 dst.accessionIdentifier = accessionIdentifier == null ? null : accessionIdentifier.copy(); 3121 dst.status = status == null ? null : status.copy(); 3122 dst.type = type == null ? null : type.copy(); 3123 dst.subject = subject == null ? null : subject.copy(); 3124 dst.receivedTime = receivedTime == null ? null : receivedTime.copy(); 3125 if (parent != null) { 3126 dst.parent = new ArrayList<Reference>(); 3127 for (Reference i : parent) 3128 dst.parent.add(i.copy()); 3129 } 3130 ; 3131 if (request != null) { 3132 dst.request = new ArrayList<Reference>(); 3133 for (Reference i : request) 3134 dst.request.add(i.copy()); 3135 } 3136 ; 3137 dst.collection = collection == null ? null : collection.copy(); 3138 if (processing != null) { 3139 dst.processing = new ArrayList<SpecimenProcessingComponent>(); 3140 for (SpecimenProcessingComponent i : processing) 3141 dst.processing.add(i.copy()); 3142 } 3143 ; 3144 if (container != null) { 3145 dst.container = new ArrayList<SpecimenContainerComponent>(); 3146 for (SpecimenContainerComponent i : container) 3147 dst.container.add(i.copy()); 3148 } 3149 ; 3150 if (condition != null) { 3151 dst.condition = new ArrayList<CodeableConcept>(); 3152 for (CodeableConcept i : condition) 3153 dst.condition.add(i.copy()); 3154 } 3155 ; 3156 if (note != null) { 3157 dst.note = new ArrayList<Annotation>(); 3158 for (Annotation i : note) 3159 dst.note.add(i.copy()); 3160 } 3161 ; 3162 } 3163 3164 protected Specimen typedCopy() { 3165 return copy(); 3166 } 3167 3168 @Override 3169 public boolean equalsDeep(Base other_) { 3170 if (!super.equalsDeep(other_)) 3171 return false; 3172 if (!(other_ instanceof Specimen)) 3173 return false; 3174 Specimen o = (Specimen) other_; 3175 return compareDeep(identifier, o.identifier, true) && compareDeep(accessionIdentifier, o.accessionIdentifier, true) 3176 && compareDeep(status, o.status, true) && compareDeep(type, o.type, true) 3177 && compareDeep(subject, o.subject, true) && compareDeep(receivedTime, o.receivedTime, true) 3178 && compareDeep(parent, o.parent, true) && compareDeep(request, o.request, true) 3179 && compareDeep(collection, o.collection, true) && compareDeep(processing, o.processing, true) 3180 && compareDeep(container, o.container, true) && compareDeep(condition, o.condition, true) 3181 && compareDeep(note, o.note, true); 3182 } 3183 3184 @Override 3185 public boolean equalsShallow(Base other_) { 3186 if (!super.equalsShallow(other_)) 3187 return false; 3188 if (!(other_ instanceof Specimen)) 3189 return false; 3190 Specimen o = (Specimen) other_; 3191 return compareValues(status, o.status, true) && compareValues(receivedTime, o.receivedTime, true); 3192 } 3193 3194 public boolean isEmpty() { 3195 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, accessionIdentifier, status, type, 3196 subject, receivedTime, parent, request, collection, processing, container, condition, note); 3197 } 3198 3199 @Override 3200 public ResourceType getResourceType() { 3201 return ResourceType.Specimen; 3202 } 3203 3204 /** 3205 * Search parameter: <b>container</b> 3206 * <p> 3207 * Description: <b>The kind of specimen container</b><br> 3208 * Type: <b>token</b><br> 3209 * Path: <b>Specimen.container.type</b><br> 3210 * </p> 3211 */ 3212 @SearchParamDefinition(name = "container", path = "Specimen.container.type", description = "The kind of specimen container", type = "token") 3213 public static final String SP_CONTAINER = "container"; 3214 /** 3215 * <b>Fluent Client</b> search parameter constant for <b>container</b> 3216 * <p> 3217 * Description: <b>The kind of specimen container</b><br> 3218 * Type: <b>token</b><br> 3219 * Path: <b>Specimen.container.type</b><br> 3220 * </p> 3221 */ 3222 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTAINER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3223 SP_CONTAINER); 3224 3225 /** 3226 * Search parameter: <b>identifier</b> 3227 * <p> 3228 * Description: <b>The unique identifier associated with the specimen</b><br> 3229 * Type: <b>token</b><br> 3230 * Path: <b>Specimen.identifier</b><br> 3231 * </p> 3232 */ 3233 @SearchParamDefinition(name = "identifier", path = "Specimen.identifier", description = "The unique identifier associated with the specimen", type = "token") 3234 public static final String SP_IDENTIFIER = "identifier"; 3235 /** 3236 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3237 * <p> 3238 * Description: <b>The unique identifier associated with the specimen</b><br> 3239 * Type: <b>token</b><br> 3240 * Path: <b>Specimen.identifier</b><br> 3241 * </p> 3242 */ 3243 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3244 SP_IDENTIFIER); 3245 3246 /** 3247 * Search parameter: <b>parent</b> 3248 * <p> 3249 * Description: <b>The parent of the specimen</b><br> 3250 * Type: <b>reference</b><br> 3251 * Path: <b>Specimen.parent</b><br> 3252 * </p> 3253 */ 3254 @SearchParamDefinition(name = "parent", path = "Specimen.parent", description = "The parent of the specimen", type = "reference", target = { 3255 Specimen.class }) 3256 public static final String SP_PARENT = "parent"; 3257 /** 3258 * <b>Fluent Client</b> search parameter constant for <b>parent</b> 3259 * <p> 3260 * Description: <b>The parent of the specimen</b><br> 3261 * Type: <b>reference</b><br> 3262 * Path: <b>Specimen.parent</b><br> 3263 * </p> 3264 */ 3265 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3266 SP_PARENT); 3267 3268 /** 3269 * Constant for fluent queries to be used to add include statements. Specifies 3270 * the path value of "<b>Specimen:parent</b>". 3271 */ 3272 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARENT = new ca.uhn.fhir.model.api.Include( 3273 "Specimen:parent").toLocked(); 3274 3275 /** 3276 * Search parameter: <b>container-id</b> 3277 * <p> 3278 * Description: <b>The unique identifier associated with the specimen 3279 * container</b><br> 3280 * Type: <b>token</b><br> 3281 * Path: <b>Specimen.container.identifier</b><br> 3282 * </p> 3283 */ 3284 @SearchParamDefinition(name = "container-id", path = "Specimen.container.identifier", description = "The unique identifier associated with the specimen container", type = "token") 3285 public static final String SP_CONTAINER_ID = "container-id"; 3286 /** 3287 * <b>Fluent Client</b> search parameter constant for <b>container-id</b> 3288 * <p> 3289 * Description: <b>The unique identifier associated with the specimen 3290 * container</b><br> 3291 * Type: <b>token</b><br> 3292 * Path: <b>Specimen.container.identifier</b><br> 3293 * </p> 3294 */ 3295 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTAINER_ID = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3296 SP_CONTAINER_ID); 3297 3298 /** 3299 * Search parameter: <b>bodysite</b> 3300 * <p> 3301 * Description: <b>The code for the body site from where the specimen 3302 * originated</b><br> 3303 * Type: <b>token</b><br> 3304 * Path: <b>Specimen.collection.bodySite</b><br> 3305 * </p> 3306 */ 3307 @SearchParamDefinition(name = "bodysite", path = "Specimen.collection.bodySite", description = "The code for the body site from where the specimen originated", type = "token") 3308 public static final String SP_BODYSITE = "bodysite"; 3309 /** 3310 * <b>Fluent Client</b> search parameter constant for <b>bodysite</b> 3311 * <p> 3312 * Description: <b>The code for the body site from where the specimen 3313 * originated</b><br> 3314 * Type: <b>token</b><br> 3315 * Path: <b>Specimen.collection.bodySite</b><br> 3316 * </p> 3317 */ 3318 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BODYSITE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3319 SP_BODYSITE); 3320 3321 /** 3322 * Search parameter: <b>subject</b> 3323 * <p> 3324 * Description: <b>The subject of the specimen</b><br> 3325 * Type: <b>reference</b><br> 3326 * Path: <b>Specimen.subject</b><br> 3327 * </p> 3328 */ 3329 @SearchParamDefinition(name = "subject", path = "Specimen.subject", description = "The subject of the specimen", type = "reference", providesMembershipIn = { 3330 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 3331 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Device.class, Group.class, 3332 Location.class, Patient.class, Substance.class }) 3333 public static final String SP_SUBJECT = "subject"; 3334 /** 3335 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3336 * <p> 3337 * Description: <b>The subject of the specimen</b><br> 3338 * Type: <b>reference</b><br> 3339 * Path: <b>Specimen.subject</b><br> 3340 * </p> 3341 */ 3342 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3343 SP_SUBJECT); 3344 3345 /** 3346 * Constant for fluent queries to be used to add include statements. Specifies 3347 * the path value of "<b>Specimen:subject</b>". 3348 */ 3349 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 3350 "Specimen:subject").toLocked(); 3351 3352 /** 3353 * Search parameter: <b>patient</b> 3354 * <p> 3355 * Description: <b>The patient the specimen comes from</b><br> 3356 * Type: <b>reference</b><br> 3357 * Path: <b>Specimen.subject</b><br> 3358 * </p> 3359 */ 3360 @SearchParamDefinition(name = "patient", path = "Specimen.subject.where(resolve() is Patient)", description = "The patient the specimen comes from", type = "reference", target = { 3361 Patient.class }) 3362 public static final String SP_PATIENT = "patient"; 3363 /** 3364 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3365 * <p> 3366 * Description: <b>The patient the specimen comes from</b><br> 3367 * Type: <b>reference</b><br> 3368 * Path: <b>Specimen.subject</b><br> 3369 * </p> 3370 */ 3371 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3372 SP_PATIENT); 3373 3374 /** 3375 * Constant for fluent queries to be used to add include statements. Specifies 3376 * the path value of "<b>Specimen:patient</b>". 3377 */ 3378 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 3379 "Specimen:patient").toLocked(); 3380 3381 /** 3382 * Search parameter: <b>collected</b> 3383 * <p> 3384 * Description: <b>The date the specimen was collected</b><br> 3385 * Type: <b>date</b><br> 3386 * Path: <b>Specimen.collection.collected[x]</b><br> 3387 * </p> 3388 */ 3389 @SearchParamDefinition(name = "collected", path = "Specimen.collection.collected", description = "The date the specimen was collected", type = "date") 3390 public static final String SP_COLLECTED = "collected"; 3391 /** 3392 * <b>Fluent Client</b> search parameter constant for <b>collected</b> 3393 * <p> 3394 * Description: <b>The date the specimen was collected</b><br> 3395 * Type: <b>date</b><br> 3396 * Path: <b>Specimen.collection.collected[x]</b><br> 3397 * </p> 3398 */ 3399 public static final ca.uhn.fhir.rest.gclient.DateClientParam COLLECTED = new ca.uhn.fhir.rest.gclient.DateClientParam( 3400 SP_COLLECTED); 3401 3402 /** 3403 * Search parameter: <b>accession</b> 3404 * <p> 3405 * Description: <b>The accession number associated with the specimen</b><br> 3406 * Type: <b>token</b><br> 3407 * Path: <b>Specimen.accessionIdentifier</b><br> 3408 * </p> 3409 */ 3410 @SearchParamDefinition(name = "accession", path = "Specimen.accessionIdentifier", description = "The accession number associated with the specimen", type = "token") 3411 public static final String SP_ACCESSION = "accession"; 3412 /** 3413 * <b>Fluent Client</b> search parameter constant for <b>accession</b> 3414 * <p> 3415 * Description: <b>The accession number associated with the specimen</b><br> 3416 * Type: <b>token</b><br> 3417 * Path: <b>Specimen.accessionIdentifier</b><br> 3418 * </p> 3419 */ 3420 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACCESSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3421 SP_ACCESSION); 3422 3423 /** 3424 * Search parameter: <b>type</b> 3425 * <p> 3426 * Description: <b>The specimen type</b><br> 3427 * Type: <b>token</b><br> 3428 * Path: <b>Specimen.type</b><br> 3429 * </p> 3430 */ 3431 @SearchParamDefinition(name = "type", path = "Specimen.type", description = "The specimen type", type = "token") 3432 public static final String SP_TYPE = "type"; 3433 /** 3434 * <b>Fluent Client</b> search parameter constant for <b>type</b> 3435 * <p> 3436 * Description: <b>The specimen type</b><br> 3437 * Type: <b>token</b><br> 3438 * Path: <b>Specimen.type</b><br> 3439 * </p> 3440 */ 3441 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3442 SP_TYPE); 3443 3444 /** 3445 * Search parameter: <b>collector</b> 3446 * <p> 3447 * Description: <b>Who collected the specimen</b><br> 3448 * Type: <b>reference</b><br> 3449 * Path: <b>Specimen.collection.collector</b><br> 3450 * </p> 3451 */ 3452 @SearchParamDefinition(name = "collector", path = "Specimen.collection.collector", description = "Who collected the specimen", type = "reference", providesMembershipIn = { 3453 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Practitioner.class, 3454 PractitionerRole.class }) 3455 public static final String SP_COLLECTOR = "collector"; 3456 /** 3457 * <b>Fluent Client</b> search parameter constant for <b>collector</b> 3458 * <p> 3459 * Description: <b>Who collected the specimen</b><br> 3460 * Type: <b>reference</b><br> 3461 * Path: <b>Specimen.collection.collector</b><br> 3462 * </p> 3463 */ 3464 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COLLECTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3465 SP_COLLECTOR); 3466 3467 /** 3468 * Constant for fluent queries to be used to add include statements. Specifies 3469 * the path value of "<b>Specimen:collector</b>". 3470 */ 3471 public static final ca.uhn.fhir.model.api.Include INCLUDE_COLLECTOR = new ca.uhn.fhir.model.api.Include( 3472 "Specimen:collector").toLocked(); 3473 3474 /** 3475 * Search parameter: <b>status</b> 3476 * <p> 3477 * Description: <b>available | unavailable | unsatisfactory | 3478 * entered-in-error</b><br> 3479 * Type: <b>token</b><br> 3480 * Path: <b>Specimen.status</b><br> 3481 * </p> 3482 */ 3483 @SearchParamDefinition(name = "status", path = "Specimen.status", description = "available | unavailable | unsatisfactory | entered-in-error", type = "token") 3484 public static final String SP_STATUS = "status"; 3485 /** 3486 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3487 * <p> 3488 * Description: <b>available | unavailable | unsatisfactory | 3489 * entered-in-error</b><br> 3490 * Type: <b>token</b><br> 3491 * Path: <b>Specimen.status</b><br> 3492 * </p> 3493 */ 3494 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3495 SP_STATUS); 3496 3497}