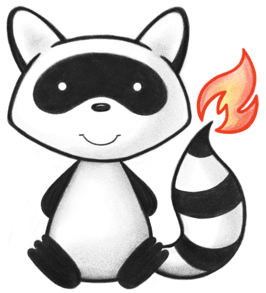
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * A sample to be used for analysis. 049 */ 050@ResourceDef(name = "Specimen", profile = "http://hl7.org/fhir/StructureDefinition/Specimen") 051public class Specimen extends DomainResource { 052 053 public enum SpecimenStatus { 054 /** 055 * The physical specimen is present and in good condition. 056 */ 057 AVAILABLE, 058 /** 059 * There is no physical specimen because it is either lost, destroyed or 060 * consumed. 061 */ 062 UNAVAILABLE, 063 /** 064 * The specimen cannot be used because of a quality issue such as a broken 065 * container, contamination, or too old. 066 */ 067 UNSATISFACTORY, 068 /** 069 * The specimen was entered in error and therefore nullified. 070 */ 071 ENTEREDINERROR, 072 /** 073 * added to help the parsers with the generic types 074 */ 075 NULL; 076 077 public static SpecimenStatus fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("available".equals(codeString)) 081 return AVAILABLE; 082 if ("unavailable".equals(codeString)) 083 return UNAVAILABLE; 084 if ("unsatisfactory".equals(codeString)) 085 return UNSATISFACTORY; 086 if ("entered-in-error".equals(codeString)) 087 return ENTEREDINERROR; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown SpecimenStatus code '" + codeString + "'"); 092 } 093 094 public String toCode() { 095 switch (this) { 096 case AVAILABLE: 097 return "available"; 098 case UNAVAILABLE: 099 return "unavailable"; 100 case UNSATISFACTORY: 101 return "unsatisfactory"; 102 case ENTEREDINERROR: 103 return "entered-in-error"; 104 case NULL: 105 return null; 106 default: 107 return "?"; 108 } 109 } 110 111 public String getSystem() { 112 switch (this) { 113 case AVAILABLE: 114 return "http://hl7.org/fhir/specimen-status"; 115 case UNAVAILABLE: 116 return "http://hl7.org/fhir/specimen-status"; 117 case UNSATISFACTORY: 118 return "http://hl7.org/fhir/specimen-status"; 119 case ENTEREDINERROR: 120 return "http://hl7.org/fhir/specimen-status"; 121 case NULL: 122 return null; 123 default: 124 return "?"; 125 } 126 } 127 128 public String getDefinition() { 129 switch (this) { 130 case AVAILABLE: 131 return "The physical specimen is present and in good condition."; 132 case UNAVAILABLE: 133 return "There is no physical specimen because it is either lost, destroyed or consumed."; 134 case UNSATISFACTORY: 135 return "The specimen cannot be used because of a quality issue such as a broken container, contamination, or too old."; 136 case ENTEREDINERROR: 137 return "The specimen was entered in error and therefore nullified."; 138 case NULL: 139 return null; 140 default: 141 return "?"; 142 } 143 } 144 145 public String getDisplay() { 146 switch (this) { 147 case AVAILABLE: 148 return "Available"; 149 case UNAVAILABLE: 150 return "Unavailable"; 151 case UNSATISFACTORY: 152 return "Unsatisfactory"; 153 case ENTEREDINERROR: 154 return "Entered in Error"; 155 case NULL: 156 return null; 157 default: 158 return "?"; 159 } 160 } 161 } 162 163 public static class SpecimenStatusEnumFactory implements EnumFactory<SpecimenStatus> { 164 public SpecimenStatus fromCode(String codeString) throws IllegalArgumentException { 165 if (codeString == null || "".equals(codeString)) 166 if (codeString == null || "".equals(codeString)) 167 return null; 168 if ("available".equals(codeString)) 169 return SpecimenStatus.AVAILABLE; 170 if ("unavailable".equals(codeString)) 171 return SpecimenStatus.UNAVAILABLE; 172 if ("unsatisfactory".equals(codeString)) 173 return SpecimenStatus.UNSATISFACTORY; 174 if ("entered-in-error".equals(codeString)) 175 return SpecimenStatus.ENTEREDINERROR; 176 throw new IllegalArgumentException("Unknown SpecimenStatus code '" + codeString + "'"); 177 } 178 179 public Enumeration<SpecimenStatus> fromType(PrimitiveType<?> code) throws FHIRException { 180 if (code == null) 181 return null; 182 if (code.isEmpty()) 183 return new Enumeration<SpecimenStatus>(this, SpecimenStatus.NULL, code); 184 String codeString = code.asStringValue(); 185 if (codeString == null || "".equals(codeString)) 186 return new Enumeration<SpecimenStatus>(this, SpecimenStatus.NULL, code); 187 if ("available".equals(codeString)) 188 return new Enumeration<SpecimenStatus>(this, SpecimenStatus.AVAILABLE, code); 189 if ("unavailable".equals(codeString)) 190 return new Enumeration<SpecimenStatus>(this, SpecimenStatus.UNAVAILABLE, code); 191 if ("unsatisfactory".equals(codeString)) 192 return new Enumeration<SpecimenStatus>(this, SpecimenStatus.UNSATISFACTORY, code); 193 if ("entered-in-error".equals(codeString)) 194 return new Enumeration<SpecimenStatus>(this, SpecimenStatus.ENTEREDINERROR, code); 195 throw new FHIRException("Unknown SpecimenStatus code '" + codeString + "'"); 196 } 197 198 public String toCode(SpecimenStatus code) { 199 if (code == SpecimenStatus.AVAILABLE) 200 return "available"; 201 if (code == SpecimenStatus.UNAVAILABLE) 202 return "unavailable"; 203 if (code == SpecimenStatus.UNSATISFACTORY) 204 return "unsatisfactory"; 205 if (code == SpecimenStatus.ENTEREDINERROR) 206 return "entered-in-error"; 207 return "?"; 208 } 209 210 public String toSystem(SpecimenStatus code) { 211 return code.getSystem(); 212 } 213 } 214 215 @Block() 216 public static class SpecimenCollectionComponent extends BackboneElement implements IBaseBackboneElement { 217 /** 218 * Person who collected the specimen. 219 */ 220 @Child(name = "collector", type = { Practitioner.class, 221 PractitionerRole.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 222 @Description(shortDefinition = "Who collected the specimen", formalDefinition = "Person who collected the specimen.") 223 protected Reference collector; 224 225 /** 226 * The actual object that is the target of the reference (Person who collected 227 * the specimen.) 228 */ 229 protected Resource collectorTarget; 230 231 /** 232 * Time when specimen was collected from subject - the physiologically relevant 233 * time. 234 */ 235 @Child(name = "collected", type = { DateTimeType.class, 236 Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 237 @Description(shortDefinition = "Collection time", formalDefinition = "Time when specimen was collected from subject - the physiologically relevant time.") 238 protected Type collected; 239 240 /** 241 * The span of time over which the collection of a specimen occurred. 242 */ 243 @Child(name = "duration", type = { Duration.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 244 @Description(shortDefinition = "How long it took to collect specimen", formalDefinition = "The span of time over which the collection of a specimen occurred.") 245 protected Duration duration; 246 247 /** 248 * The quantity of specimen collected; for instance the volume of a blood 249 * sample, or the physical measurement of an anatomic pathology sample. 250 */ 251 @Child(name = "quantity", type = { Quantity.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 252 @Description(shortDefinition = "The quantity of specimen collected", formalDefinition = "The quantity of specimen collected; for instance the volume of a blood sample, or the physical measurement of an anatomic pathology sample.") 253 protected Quantity quantity; 254 255 /** 256 * A coded value specifying the technique that is used to perform the procedure. 257 */ 258 @Child(name = "method", type = { 259 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 260 @Description(shortDefinition = "Technique used to perform collection", formalDefinition = "A coded value specifying the technique that is used to perform the procedure.") 261 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/specimen-collection-method") 262 protected CodeableConcept method; 263 264 /** 265 * Anatomical location from which the specimen was collected (if subject is a 266 * patient). This is the target site. This element is not used for environmental 267 * specimens. 268 */ 269 @Child(name = "bodySite", type = { 270 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 271 @Description(shortDefinition = "Anatomical collection site", formalDefinition = "Anatomical location from which the specimen was collected (if subject is a patient). This is the target site. This element is not used for environmental specimens.") 272 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/body-site") 273 protected CodeableConcept bodySite; 274 275 /** 276 * Abstinence or reduction from some or all food, drink, or both, for a period 277 * of time prior to sample collection. 278 */ 279 @Child(name = "fastingStatus", type = { CodeableConcept.class, 280 Duration.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 281 @Description(shortDefinition = "Whether or how long patient abstained from food and/or drink", formalDefinition = "Abstinence or reduction from some or all food, drink, or both, for a period of time prior to sample collection.") 282 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v2-0916") 283 protected Type fastingStatus; 284 285 private static final long serialVersionUID = -719430195L; 286 287 /** 288 * Constructor 289 */ 290 public SpecimenCollectionComponent() { 291 super(); 292 } 293 294 /** 295 * @return {@link #collector} (Person who collected the specimen.) 296 */ 297 public Reference getCollector() { 298 if (this.collector == null) 299 if (Configuration.errorOnAutoCreate()) 300 throw new Error("Attempt to auto-create SpecimenCollectionComponent.collector"); 301 else if (Configuration.doAutoCreate()) 302 this.collector = new Reference(); // cc 303 return this.collector; 304 } 305 306 public boolean hasCollector() { 307 return this.collector != null && !this.collector.isEmpty(); 308 } 309 310 /** 311 * @param value {@link #collector} (Person who collected the specimen.) 312 */ 313 public SpecimenCollectionComponent setCollector(Reference value) { 314 this.collector = value; 315 return this; 316 } 317 318 /** 319 * @return {@link #collector} The actual object that is the target of the 320 * reference. The reference library doesn't populate this, but you can 321 * use it to hold the resource if you resolve it. (Person who collected 322 * the specimen.) 323 */ 324 public Resource getCollectorTarget() { 325 return this.collectorTarget; 326 } 327 328 /** 329 * @param value {@link #collector} The actual object that is the target of the 330 * reference. The reference library doesn't use these, but you can 331 * use it to hold the resource if you resolve it. (Person who 332 * collected the specimen.) 333 */ 334 public SpecimenCollectionComponent setCollectorTarget(Resource value) { 335 this.collectorTarget = value; 336 return this; 337 } 338 339 /** 340 * @return {@link #collected} (Time when specimen was collected from subject - 341 * the physiologically relevant time.) 342 */ 343 public Type getCollected() { 344 return this.collected; 345 } 346 347 /** 348 * @return {@link #collected} (Time when specimen was collected from subject - 349 * the physiologically relevant time.) 350 */ 351 public DateTimeType getCollectedDateTimeType() throws FHIRException { 352 if (this.collected == null) 353 this.collected = new DateTimeType(); 354 if (!(this.collected instanceof DateTimeType)) 355 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 356 + this.collected.getClass().getName() + " was encountered"); 357 return (DateTimeType) this.collected; 358 } 359 360 public boolean hasCollectedDateTimeType() { 361 return this != null && this.collected instanceof DateTimeType; 362 } 363 364 /** 365 * @return {@link #collected} (Time when specimen was collected from subject - 366 * the physiologically relevant time.) 367 */ 368 public Period getCollectedPeriod() throws FHIRException { 369 if (this.collected == null) 370 this.collected = new Period(); 371 if (!(this.collected instanceof Period)) 372 throw new FHIRException("Type mismatch: the type Period was expected, but " 373 + this.collected.getClass().getName() + " was encountered"); 374 return (Period) this.collected; 375 } 376 377 public boolean hasCollectedPeriod() { 378 return this != null && this.collected instanceof Period; 379 } 380 381 public boolean hasCollected() { 382 return this.collected != null && !this.collected.isEmpty(); 383 } 384 385 /** 386 * @param value {@link #collected} (Time when specimen was collected from 387 * subject - the physiologically relevant time.) 388 */ 389 public SpecimenCollectionComponent setCollected(Type value) { 390 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 391 throw new Error("Not the right type for Specimen.collection.collected[x]: " + value.fhirType()); 392 this.collected = value; 393 return this; 394 } 395 396 /** 397 * @return {@link #duration} (The span of time over which the collection of a 398 * specimen occurred.) 399 */ 400 public Duration getDuration() { 401 if (this.duration == null) 402 if (Configuration.errorOnAutoCreate()) 403 throw new Error("Attempt to auto-create SpecimenCollectionComponent.duration"); 404 else if (Configuration.doAutoCreate()) 405 this.duration = new Duration(); // cc 406 return this.duration; 407 } 408 409 public boolean hasDuration() { 410 return this.duration != null && !this.duration.isEmpty(); 411 } 412 413 /** 414 * @param value {@link #duration} (The span of time over which the collection of 415 * a specimen occurred.) 416 */ 417 public SpecimenCollectionComponent setDuration(Duration value) { 418 this.duration = value; 419 return this; 420 } 421 422 /** 423 * @return {@link #quantity} (The quantity of specimen collected; for instance 424 * the volume of a blood sample, or the physical measurement of an 425 * anatomic pathology sample.) 426 */ 427 public Quantity getQuantity() { 428 if (this.quantity == null) 429 if (Configuration.errorOnAutoCreate()) 430 throw new Error("Attempt to auto-create SpecimenCollectionComponent.quantity"); 431 else if (Configuration.doAutoCreate()) 432 this.quantity = new Quantity(); // cc 433 return this.quantity; 434 } 435 436 public boolean hasQuantity() { 437 return this.quantity != null && !this.quantity.isEmpty(); 438 } 439 440 /** 441 * @param value {@link #quantity} (The quantity of specimen collected; for 442 * instance the volume of a blood sample, or the physical 443 * measurement of an anatomic pathology sample.) 444 */ 445 public SpecimenCollectionComponent setQuantity(Quantity value) { 446 this.quantity = value; 447 return this; 448 } 449 450 /** 451 * @return {@link #method} (A coded value specifying the technique that is used 452 * to perform the procedure.) 453 */ 454 public CodeableConcept getMethod() { 455 if (this.method == null) 456 if (Configuration.errorOnAutoCreate()) 457 throw new Error("Attempt to auto-create SpecimenCollectionComponent.method"); 458 else if (Configuration.doAutoCreate()) 459 this.method = new CodeableConcept(); // cc 460 return this.method; 461 } 462 463 public boolean hasMethod() { 464 return this.method != null && !this.method.isEmpty(); 465 } 466 467 /** 468 * @param value {@link #method} (A coded value specifying the technique that is 469 * used to perform the procedure.) 470 */ 471 public SpecimenCollectionComponent setMethod(CodeableConcept value) { 472 this.method = value; 473 return this; 474 } 475 476 /** 477 * @return {@link #bodySite} (Anatomical location from which the specimen was 478 * collected (if subject is a patient). This is the target site. This 479 * element is not used for environmental specimens.) 480 */ 481 public CodeableConcept getBodySite() { 482 if (this.bodySite == null) 483 if (Configuration.errorOnAutoCreate()) 484 throw new Error("Attempt to auto-create SpecimenCollectionComponent.bodySite"); 485 else if (Configuration.doAutoCreate()) 486 this.bodySite = new CodeableConcept(); // cc 487 return this.bodySite; 488 } 489 490 public boolean hasBodySite() { 491 return this.bodySite != null && !this.bodySite.isEmpty(); 492 } 493 494 /** 495 * @param value {@link #bodySite} (Anatomical location from which the specimen 496 * was collected (if subject is a patient). This is the target 497 * site. This element is not used for environmental specimens.) 498 */ 499 public SpecimenCollectionComponent setBodySite(CodeableConcept value) { 500 this.bodySite = value; 501 return this; 502 } 503 504 /** 505 * @return {@link #fastingStatus} (Abstinence or reduction from some or all 506 * food, drink, or both, for a period of time prior to sample 507 * collection.) 508 */ 509 public Type getFastingStatus() { 510 return this.fastingStatus; 511 } 512 513 /** 514 * @return {@link #fastingStatus} (Abstinence or reduction from some or all 515 * food, drink, or both, for a period of time prior to sample 516 * collection.) 517 */ 518 public CodeableConcept getFastingStatusCodeableConcept() throws FHIRException { 519 if (this.fastingStatus == null) 520 this.fastingStatus = new CodeableConcept(); 521 if (!(this.fastingStatus instanceof CodeableConcept)) 522 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 523 + this.fastingStatus.getClass().getName() + " was encountered"); 524 return (CodeableConcept) this.fastingStatus; 525 } 526 527 public boolean hasFastingStatusCodeableConcept() { 528 return this != null && this.fastingStatus instanceof CodeableConcept; 529 } 530 531 /** 532 * @return {@link #fastingStatus} (Abstinence or reduction from some or all 533 * food, drink, or both, for a period of time prior to sample 534 * collection.) 535 */ 536 public Duration getFastingStatusDuration() throws FHIRException { 537 if (this.fastingStatus == null) 538 this.fastingStatus = new Duration(); 539 if (!(this.fastingStatus instanceof Duration)) 540 throw new FHIRException("Type mismatch: the type Duration was expected, but " 541 + this.fastingStatus.getClass().getName() + " was encountered"); 542 return (Duration) this.fastingStatus; 543 } 544 545 public boolean hasFastingStatusDuration() { 546 return this != null && this.fastingStatus instanceof Duration; 547 } 548 549 public boolean hasFastingStatus() { 550 return this.fastingStatus != null && !this.fastingStatus.isEmpty(); 551 } 552 553 /** 554 * @param value {@link #fastingStatus} (Abstinence or reduction from some or all 555 * food, drink, or both, for a period of time prior to sample 556 * collection.) 557 */ 558 public SpecimenCollectionComponent setFastingStatus(Type value) { 559 if (value != null && !(value instanceof CodeableConcept || value instanceof Duration)) 560 throw new Error("Not the right type for Specimen.collection.fastingStatus[x]: " + value.fhirType()); 561 this.fastingStatus = value; 562 return this; 563 } 564 565 protected void listChildren(List<Property> children) { 566 super.listChildren(children); 567 children.add(new Property("collector", "Reference(Practitioner|PractitionerRole)", 568 "Person who collected the specimen.", 0, 1, collector)); 569 children.add(new Property("collected[x]", "dateTime|Period", 570 "Time when specimen was collected from subject - the physiologically relevant time.", 0, 1, collected)); 571 children.add(new Property("duration", "Duration", 572 "The span of time over which the collection of a specimen occurred.", 0, 1, duration)); 573 children.add(new Property("quantity", "SimpleQuantity", 574 "The quantity of specimen collected; for instance the volume of a blood sample, or the physical measurement of an anatomic pathology sample.", 575 0, 1, quantity)); 576 children.add(new Property("method", "CodeableConcept", 577 "A coded value specifying the technique that is used to perform the procedure.", 0, 1, method)); 578 children.add(new Property("bodySite", "CodeableConcept", 579 "Anatomical location from which the specimen was collected (if subject is a patient). This is the target site. This element is not used for environmental specimens.", 580 0, 1, bodySite)); 581 children.add(new Property("fastingStatus[x]", "CodeableConcept|Duration", 582 "Abstinence or reduction from some or all food, drink, or both, for a period of time prior to sample collection.", 583 0, 1, fastingStatus)); 584 } 585 586 @Override 587 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 588 switch (_hash) { 589 case 1883491469: 590 /* collector */ return new Property("collector", "Reference(Practitioner|PractitionerRole)", 591 "Person who collected the specimen.", 0, 1, collector); 592 case 1632037015: 593 /* collected[x] */ return new Property("collected[x]", "dateTime|Period", 594 "Time when specimen was collected from subject - the physiologically relevant time.", 0, 1, collected); 595 case 1883491145: 596 /* collected */ return new Property("collected[x]", "dateTime|Period", 597 "Time when specimen was collected from subject - the physiologically relevant time.", 0, 1, collected); 598 case 2005009924: 599 /* collectedDateTime */ return new Property("collected[x]", "dateTime|Period", 600 "Time when specimen was collected from subject - the physiologically relevant time.", 0, 1, collected); 601 case 653185642: 602 /* collectedPeriod */ return new Property("collected[x]", "dateTime|Period", 603 "Time when specimen was collected from subject - the physiologically relevant time.", 0, 1, collected); 604 case -1992012396: 605 /* duration */ return new Property("duration", "Duration", 606 "The span of time over which the collection of a specimen occurred.", 0, 1, duration); 607 case -1285004149: 608 /* quantity */ return new Property("quantity", "SimpleQuantity", 609 "The quantity of specimen collected; for instance the volume of a blood sample, or the physical measurement of an anatomic pathology sample.", 610 0, 1, quantity); 611 case -1077554975: 612 /* method */ return new Property("method", "CodeableConcept", 613 "A coded value specifying the technique that is used to perform the procedure.", 0, 1, method); 614 case 1702620169: 615 /* bodySite */ return new Property("bodySite", "CodeableConcept", 616 "Anatomical location from which the specimen was collected (if subject is a patient). This is the target site. This element is not used for environmental specimens.", 617 0, 1, bodySite); 618 case -570577944: 619 /* fastingStatus[x] */ return new Property("fastingStatus[x]", "CodeableConcept|Duration", 620 "Abstinence or reduction from some or all food, drink, or both, for a period of time prior to sample collection.", 621 0, 1, fastingStatus); 622 case -701550184: 623 /* fastingStatus */ return new Property("fastingStatus[x]", "CodeableConcept|Duration", 624 "Abstinence or reduction from some or all food, drink, or both, for a period of time prior to sample collection.", 625 0, 1, fastingStatus); 626 case -1153232151: 627 /* fastingStatusCodeableConcept */ return new Property("fastingStatus[x]", "CodeableConcept|Duration", 628 "Abstinence or reduction from some or all food, drink, or both, for a period of time prior to sample collection.", 629 0, 1, fastingStatus); 630 case -433140916: 631 /* fastingStatusDuration */ return new Property("fastingStatus[x]", "CodeableConcept|Duration", 632 "Abstinence or reduction from some or all food, drink, or both, for a period of time prior to sample collection.", 633 0, 1, fastingStatus); 634 default: 635 return super.getNamedProperty(_hash, _name, _checkValid); 636 } 637 638 } 639 640 @Override 641 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 642 switch (hash) { 643 case 1883491469: 644 /* collector */ return this.collector == null ? new Base[0] : new Base[] { this.collector }; // Reference 645 case 1883491145: 646 /* collected */ return this.collected == null ? new Base[0] : new Base[] { this.collected }; // Type 647 case -1992012396: 648 /* duration */ return this.duration == null ? new Base[0] : new Base[] { this.duration }; // Duration 649 case -1285004149: 650 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 651 case -1077554975: 652 /* method */ return this.method == null ? new Base[0] : new Base[] { this.method }; // CodeableConcept 653 case 1702620169: 654 /* bodySite */ return this.bodySite == null ? new Base[0] : new Base[] { this.bodySite }; // CodeableConcept 655 case -701550184: 656 /* fastingStatus */ return this.fastingStatus == null ? new Base[0] : new Base[] { this.fastingStatus }; // Type 657 default: 658 return super.getProperty(hash, name, checkValid); 659 } 660 661 } 662 663 @Override 664 public Base setProperty(int hash, String name, Base value) throws FHIRException { 665 switch (hash) { 666 case 1883491469: // collector 667 this.collector = castToReference(value); // Reference 668 return value; 669 case 1883491145: // collected 670 this.collected = castToType(value); // Type 671 return value; 672 case -1992012396: // duration 673 this.duration = castToDuration(value); // Duration 674 return value; 675 case -1285004149: // quantity 676 this.quantity = castToQuantity(value); // Quantity 677 return value; 678 case -1077554975: // method 679 this.method = castToCodeableConcept(value); // CodeableConcept 680 return value; 681 case 1702620169: // bodySite 682 this.bodySite = castToCodeableConcept(value); // CodeableConcept 683 return value; 684 case -701550184: // fastingStatus 685 this.fastingStatus = castToType(value); // Type 686 return value; 687 default: 688 return super.setProperty(hash, name, value); 689 } 690 691 } 692 693 @Override 694 public Base setProperty(String name, Base value) throws FHIRException { 695 if (name.equals("collector")) { 696 this.collector = castToReference(value); // Reference 697 } else if (name.equals("collected[x]")) { 698 this.collected = castToType(value); // Type 699 } else if (name.equals("duration")) { 700 this.duration = castToDuration(value); // Duration 701 } else if (name.equals("quantity")) { 702 this.quantity = castToQuantity(value); // Quantity 703 } else if (name.equals("method")) { 704 this.method = castToCodeableConcept(value); // CodeableConcept 705 } else if (name.equals("bodySite")) { 706 this.bodySite = castToCodeableConcept(value); // CodeableConcept 707 } else if (name.equals("fastingStatus[x]")) { 708 this.fastingStatus = castToType(value); // Type 709 } else 710 return super.setProperty(name, value); 711 return value; 712 } 713 714 @Override 715 public Base makeProperty(int hash, String name) throws FHIRException { 716 switch (hash) { 717 case 1883491469: 718 return getCollector(); 719 case 1632037015: 720 return getCollected(); 721 case 1883491145: 722 return getCollected(); 723 case -1992012396: 724 return getDuration(); 725 case -1285004149: 726 return getQuantity(); 727 case -1077554975: 728 return getMethod(); 729 case 1702620169: 730 return getBodySite(); 731 case -570577944: 732 return getFastingStatus(); 733 case -701550184: 734 return getFastingStatus(); 735 default: 736 return super.makeProperty(hash, name); 737 } 738 739 } 740 741 @Override 742 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 743 switch (hash) { 744 case 1883491469: 745 /* collector */ return new String[] { "Reference" }; 746 case 1883491145: 747 /* collected */ return new String[] { "dateTime", "Period" }; 748 case -1992012396: 749 /* duration */ return new String[] { "Duration" }; 750 case -1285004149: 751 /* quantity */ return new String[] { "SimpleQuantity" }; 752 case -1077554975: 753 /* method */ return new String[] { "CodeableConcept" }; 754 case 1702620169: 755 /* bodySite */ return new String[] { "CodeableConcept" }; 756 case -701550184: 757 /* fastingStatus */ return new String[] { "CodeableConcept", "Duration" }; 758 default: 759 return super.getTypesForProperty(hash, name); 760 } 761 762 } 763 764 @Override 765 public Base addChild(String name) throws FHIRException { 766 if (name.equals("collector")) { 767 this.collector = new Reference(); 768 return this.collector; 769 } else if (name.equals("collectedDateTime")) { 770 this.collected = new DateTimeType(); 771 return this.collected; 772 } else if (name.equals("collectedPeriod")) { 773 this.collected = new Period(); 774 return this.collected; 775 } else if (name.equals("duration")) { 776 this.duration = new Duration(); 777 return this.duration; 778 } else if (name.equals("quantity")) { 779 this.quantity = new Quantity(); 780 return this.quantity; 781 } else if (name.equals("method")) { 782 this.method = new CodeableConcept(); 783 return this.method; 784 } else if (name.equals("bodySite")) { 785 this.bodySite = new CodeableConcept(); 786 return this.bodySite; 787 } else if (name.equals("fastingStatusCodeableConcept")) { 788 this.fastingStatus = new CodeableConcept(); 789 return this.fastingStatus; 790 } else if (name.equals("fastingStatusDuration")) { 791 this.fastingStatus = new Duration(); 792 return this.fastingStatus; 793 } else 794 return super.addChild(name); 795 } 796 797 public SpecimenCollectionComponent copy() { 798 SpecimenCollectionComponent dst = new SpecimenCollectionComponent(); 799 copyValues(dst); 800 return dst; 801 } 802 803 public void copyValues(SpecimenCollectionComponent dst) { 804 super.copyValues(dst); 805 dst.collector = collector == null ? null : collector.copy(); 806 dst.collected = collected == null ? null : collected.copy(); 807 dst.duration = duration == null ? null : duration.copy(); 808 dst.quantity = quantity == null ? null : quantity.copy(); 809 dst.method = method == null ? null : method.copy(); 810 dst.bodySite = bodySite == null ? null : bodySite.copy(); 811 dst.fastingStatus = fastingStatus == null ? null : fastingStatus.copy(); 812 } 813 814 @Override 815 public boolean equalsDeep(Base other_) { 816 if (!super.equalsDeep(other_)) 817 return false; 818 if (!(other_ instanceof SpecimenCollectionComponent)) 819 return false; 820 SpecimenCollectionComponent o = (SpecimenCollectionComponent) other_; 821 return compareDeep(collector, o.collector, true) && compareDeep(collected, o.collected, true) 822 && compareDeep(duration, o.duration, true) && compareDeep(quantity, o.quantity, true) 823 && compareDeep(method, o.method, true) && compareDeep(bodySite, o.bodySite, true) 824 && compareDeep(fastingStatus, o.fastingStatus, true); 825 } 826 827 @Override 828 public boolean equalsShallow(Base other_) { 829 if (!super.equalsShallow(other_)) 830 return false; 831 if (!(other_ instanceof SpecimenCollectionComponent)) 832 return false; 833 SpecimenCollectionComponent o = (SpecimenCollectionComponent) other_; 834 return true; 835 } 836 837 public boolean isEmpty() { 838 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(collector, collected, duration, quantity, method, 839 bodySite, fastingStatus); 840 } 841 842 public String fhirType() { 843 return "Specimen.collection"; 844 845 } 846 847 } 848 849 @Block() 850 public static class SpecimenProcessingComponent extends BackboneElement implements IBaseBackboneElement { 851 /** 852 * Textual description of procedure. 853 */ 854 @Child(name = "description", type = { 855 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 856 @Description(shortDefinition = "Textual description of procedure", formalDefinition = "Textual description of procedure.") 857 protected StringType description; 858 859 /** 860 * A coded value specifying the procedure used to process the specimen. 861 */ 862 @Child(name = "procedure", type = { 863 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 864 @Description(shortDefinition = "Indicates the treatment step applied to the specimen", formalDefinition = "A coded value specifying the procedure used to process the specimen.") 865 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/specimen-processing-procedure") 866 protected CodeableConcept procedure; 867 868 /** 869 * Material used in the processing step. 870 */ 871 @Child(name = "additive", type = { 872 Substance.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 873 @Description(shortDefinition = "Material used in the processing step", formalDefinition = "Material used in the processing step.") 874 protected List<Reference> additive; 875 /** 876 * The actual objects that are the target of the reference (Material used in the 877 * processing step.) 878 */ 879 protected List<Substance> additiveTarget; 880 881 /** 882 * A record of the time or period when the specimen processing occurred. For 883 * example the time of sample fixation or the period of time the sample was in 884 * formalin. 885 */ 886 @Child(name = "time", type = { DateTimeType.class, 887 Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 888 @Description(shortDefinition = "Date and time of specimen processing", formalDefinition = "A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.") 889 protected Type time; 890 891 private static final long serialVersionUID = 1467214742L; 892 893 /** 894 * Constructor 895 */ 896 public SpecimenProcessingComponent() { 897 super(); 898 } 899 900 /** 901 * @return {@link #description} (Textual description of procedure.). This is the 902 * underlying object with id, value and extensions. The accessor 903 * "getDescription" gives direct access to the value 904 */ 905 public StringType getDescriptionElement() { 906 if (this.description == null) 907 if (Configuration.errorOnAutoCreate()) 908 throw new Error("Attempt to auto-create SpecimenProcessingComponent.description"); 909 else if (Configuration.doAutoCreate()) 910 this.description = new StringType(); // bb 911 return this.description; 912 } 913 914 public boolean hasDescriptionElement() { 915 return this.description != null && !this.description.isEmpty(); 916 } 917 918 public boolean hasDescription() { 919 return this.description != null && !this.description.isEmpty(); 920 } 921 922 /** 923 * @param value {@link #description} (Textual description of procedure.). This 924 * is the underlying object with id, value and extensions. The 925 * accessor "getDescription" gives direct access to the value 926 */ 927 public SpecimenProcessingComponent setDescriptionElement(StringType value) { 928 this.description = value; 929 return this; 930 } 931 932 /** 933 * @return Textual description of procedure. 934 */ 935 public String getDescription() { 936 return this.description == null ? null : this.description.getValue(); 937 } 938 939 /** 940 * @param value Textual description of procedure. 941 */ 942 public SpecimenProcessingComponent setDescription(String value) { 943 if (Utilities.noString(value)) 944 this.description = null; 945 else { 946 if (this.description == null) 947 this.description = new StringType(); 948 this.description.setValue(value); 949 } 950 return this; 951 } 952 953 /** 954 * @return {@link #procedure} (A coded value specifying the procedure used to 955 * process the specimen.) 956 */ 957 public CodeableConcept getProcedure() { 958 if (this.procedure == null) 959 if (Configuration.errorOnAutoCreate()) 960 throw new Error("Attempt to auto-create SpecimenProcessingComponent.procedure"); 961 else if (Configuration.doAutoCreate()) 962 this.procedure = new CodeableConcept(); // cc 963 return this.procedure; 964 } 965 966 public boolean hasProcedure() { 967 return this.procedure != null && !this.procedure.isEmpty(); 968 } 969 970 /** 971 * @param value {@link #procedure} (A coded value specifying the procedure used 972 * to process the specimen.) 973 */ 974 public SpecimenProcessingComponent setProcedure(CodeableConcept value) { 975 this.procedure = value; 976 return this; 977 } 978 979 /** 980 * @return {@link #additive} (Material used in the processing step.) 981 */ 982 public List<Reference> getAdditive() { 983 if (this.additive == null) 984 this.additive = new ArrayList<Reference>(); 985 return this.additive; 986 } 987 988 /** 989 * @return Returns a reference to <code>this</code> for easy method chaining 990 */ 991 public SpecimenProcessingComponent setAdditive(List<Reference> theAdditive) { 992 this.additive = theAdditive; 993 return this; 994 } 995 996 public boolean hasAdditive() { 997 if (this.additive == null) 998 return false; 999 for (Reference item : this.additive) 1000 if (!item.isEmpty()) 1001 return true; 1002 return false; 1003 } 1004 1005 public Reference addAdditive() { // 3 1006 Reference t = new Reference(); 1007 if (this.additive == null) 1008 this.additive = new ArrayList<Reference>(); 1009 this.additive.add(t); 1010 return t; 1011 } 1012 1013 public SpecimenProcessingComponent addAdditive(Reference t) { // 3 1014 if (t == null) 1015 return this; 1016 if (this.additive == null) 1017 this.additive = new ArrayList<Reference>(); 1018 this.additive.add(t); 1019 return this; 1020 } 1021 1022 /** 1023 * @return The first repetition of repeating field {@link #additive}, creating 1024 * it if it does not already exist 1025 */ 1026 public Reference getAdditiveFirstRep() { 1027 if (getAdditive().isEmpty()) { 1028 addAdditive(); 1029 } 1030 return getAdditive().get(0); 1031 } 1032 1033 /** 1034 * @deprecated Use Reference#setResource(IBaseResource) instead 1035 */ 1036 @Deprecated 1037 public List<Substance> getAdditiveTarget() { 1038 if (this.additiveTarget == null) 1039 this.additiveTarget = new ArrayList<Substance>(); 1040 return this.additiveTarget; 1041 } 1042 1043 /** 1044 * @deprecated Use Reference#setResource(IBaseResource) instead 1045 */ 1046 @Deprecated 1047 public Substance addAdditiveTarget() { 1048 Substance r = new Substance(); 1049 if (this.additiveTarget == null) 1050 this.additiveTarget = new ArrayList<Substance>(); 1051 this.additiveTarget.add(r); 1052 return r; 1053 } 1054 1055 /** 1056 * @return {@link #time} (A record of the time or period when the specimen 1057 * processing occurred. For example the time of sample fixation or the 1058 * period of time the sample was in formalin.) 1059 */ 1060 public Type getTime() { 1061 return this.time; 1062 } 1063 1064 /** 1065 * @return {@link #time} (A record of the time or period when the specimen 1066 * processing occurred. For example the time of sample fixation or the 1067 * period of time the sample was in formalin.) 1068 */ 1069 public DateTimeType getTimeDateTimeType() throws FHIRException { 1070 if (this.time == null) 1071 this.time = new DateTimeType(); 1072 if (!(this.time instanceof DateTimeType)) 1073 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1074 + this.time.getClass().getName() + " was encountered"); 1075 return (DateTimeType) this.time; 1076 } 1077 1078 public boolean hasTimeDateTimeType() { 1079 return this != null && this.time instanceof DateTimeType; 1080 } 1081 1082 /** 1083 * @return {@link #time} (A record of the time or period when the specimen 1084 * processing occurred. For example the time of sample fixation or the 1085 * period of time the sample was in formalin.) 1086 */ 1087 public Period getTimePeriod() throws FHIRException { 1088 if (this.time == null) 1089 this.time = new Period(); 1090 if (!(this.time instanceof Period)) 1091 throw new FHIRException( 1092 "Type mismatch: the type Period was expected, but " + this.time.getClass().getName() + " was encountered"); 1093 return (Period) this.time; 1094 } 1095 1096 public boolean hasTimePeriod() { 1097 return this != null && this.time instanceof Period; 1098 } 1099 1100 public boolean hasTime() { 1101 return this.time != null && !this.time.isEmpty(); 1102 } 1103 1104 /** 1105 * @param value {@link #time} (A record of the time or period when the specimen 1106 * processing occurred. For example the time of sample fixation or 1107 * the period of time the sample was in formalin.) 1108 */ 1109 public SpecimenProcessingComponent setTime(Type value) { 1110 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1111 throw new Error("Not the right type for Specimen.processing.time[x]: " + value.fhirType()); 1112 this.time = value; 1113 return this; 1114 } 1115 1116 protected void listChildren(List<Property> children) { 1117 super.listChildren(children); 1118 children.add(new Property("description", "string", "Textual description of procedure.", 0, 1, description)); 1119 children.add(new Property("procedure", "CodeableConcept", 1120 "A coded value specifying the procedure used to process the specimen.", 0, 1, procedure)); 1121 children.add(new Property("additive", "Reference(Substance)", "Material used in the processing step.", 0, 1122 java.lang.Integer.MAX_VALUE, additive)); 1123 children.add(new Property("time[x]", "dateTime|Period", 1124 "A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.", 1125 0, 1, time)); 1126 } 1127 1128 @Override 1129 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1130 switch (_hash) { 1131 case -1724546052: 1132 /* description */ return new Property("description", "string", "Textual description of procedure.", 0, 1, 1133 description); 1134 case -1095204141: 1135 /* procedure */ return new Property("procedure", "CodeableConcept", 1136 "A coded value specifying the procedure used to process the specimen.", 0, 1, procedure); 1137 case -1226589236: 1138 /* additive */ return new Property("additive", "Reference(Substance)", "Material used in the processing step.", 1139 0, java.lang.Integer.MAX_VALUE, additive); 1140 case -1313930605: 1141 /* time[x] */ return new Property("time[x]", "dateTime|Period", 1142 "A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.", 1143 0, 1, time); 1144 case 3560141: 1145 /* time */ return new Property("time[x]", "dateTime|Period", 1146 "A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.", 1147 0, 1, time); 1148 case 2135345544: 1149 /* timeDateTime */ return new Property("time[x]", "dateTime|Period", 1150 "A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.", 1151 0, 1, time); 1152 case 693544686: 1153 /* timePeriod */ return new Property("time[x]", "dateTime|Period", 1154 "A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.", 1155 0, 1, time); 1156 default: 1157 return super.getNamedProperty(_hash, _name, _checkValid); 1158 } 1159 1160 } 1161 1162 @Override 1163 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1164 switch (hash) { 1165 case -1724546052: 1166 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1167 case -1095204141: 1168 /* procedure */ return this.procedure == null ? new Base[0] : new Base[] { this.procedure }; // CodeableConcept 1169 case -1226589236: 1170 /* additive */ return this.additive == null ? new Base[0] 1171 : this.additive.toArray(new Base[this.additive.size()]); // Reference 1172 case 3560141: 1173 /* time */ return this.time == null ? new Base[0] : new Base[] { this.time }; // Type 1174 default: 1175 return super.getProperty(hash, name, checkValid); 1176 } 1177 1178 } 1179 1180 @Override 1181 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1182 switch (hash) { 1183 case -1724546052: // description 1184 this.description = castToString(value); // StringType 1185 return value; 1186 case -1095204141: // procedure 1187 this.procedure = castToCodeableConcept(value); // CodeableConcept 1188 return value; 1189 case -1226589236: // additive 1190 this.getAdditive().add(castToReference(value)); // Reference 1191 return value; 1192 case 3560141: // time 1193 this.time = castToType(value); // Type 1194 return value; 1195 default: 1196 return super.setProperty(hash, name, value); 1197 } 1198 1199 } 1200 1201 @Override 1202 public Base setProperty(String name, Base value) throws FHIRException { 1203 if (name.equals("description")) { 1204 this.description = castToString(value); // StringType 1205 } else if (name.equals("procedure")) { 1206 this.procedure = castToCodeableConcept(value); // CodeableConcept 1207 } else if (name.equals("additive")) { 1208 this.getAdditive().add(castToReference(value)); 1209 } else if (name.equals("time[x]")) { 1210 this.time = castToType(value); // Type 1211 } else 1212 return super.setProperty(name, value); 1213 return value; 1214 } 1215 1216 @Override 1217 public Base makeProperty(int hash, String name) throws FHIRException { 1218 switch (hash) { 1219 case -1724546052: 1220 return getDescriptionElement(); 1221 case -1095204141: 1222 return getProcedure(); 1223 case -1226589236: 1224 return addAdditive(); 1225 case -1313930605: 1226 return getTime(); 1227 case 3560141: 1228 return getTime(); 1229 default: 1230 return super.makeProperty(hash, name); 1231 } 1232 1233 } 1234 1235 @Override 1236 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1237 switch (hash) { 1238 case -1724546052: 1239 /* description */ return new String[] { "string" }; 1240 case -1095204141: 1241 /* procedure */ return new String[] { "CodeableConcept" }; 1242 case -1226589236: 1243 /* additive */ return new String[] { "Reference" }; 1244 case 3560141: 1245 /* time */ return new String[] { "dateTime", "Period" }; 1246 default: 1247 return super.getTypesForProperty(hash, name); 1248 } 1249 1250 } 1251 1252 @Override 1253 public Base addChild(String name) throws FHIRException { 1254 if (name.equals("description")) { 1255 throw new FHIRException("Cannot call addChild on a singleton property Specimen.description"); 1256 } else if (name.equals("procedure")) { 1257 this.procedure = new CodeableConcept(); 1258 return this.procedure; 1259 } else if (name.equals("additive")) { 1260 return addAdditive(); 1261 } else if (name.equals("timeDateTime")) { 1262 this.time = new DateTimeType(); 1263 return this.time; 1264 } else if (name.equals("timePeriod")) { 1265 this.time = new Period(); 1266 return this.time; 1267 } else 1268 return super.addChild(name); 1269 } 1270 1271 public SpecimenProcessingComponent copy() { 1272 SpecimenProcessingComponent dst = new SpecimenProcessingComponent(); 1273 copyValues(dst); 1274 return dst; 1275 } 1276 1277 public void copyValues(SpecimenProcessingComponent dst) { 1278 super.copyValues(dst); 1279 dst.description = description == null ? null : description.copy(); 1280 dst.procedure = procedure == null ? null : procedure.copy(); 1281 if (additive != null) { 1282 dst.additive = new ArrayList<Reference>(); 1283 for (Reference i : additive) 1284 dst.additive.add(i.copy()); 1285 } 1286 ; 1287 dst.time = time == null ? null : time.copy(); 1288 } 1289 1290 @Override 1291 public boolean equalsDeep(Base other_) { 1292 if (!super.equalsDeep(other_)) 1293 return false; 1294 if (!(other_ instanceof SpecimenProcessingComponent)) 1295 return false; 1296 SpecimenProcessingComponent o = (SpecimenProcessingComponent) other_; 1297 return compareDeep(description, o.description, true) && compareDeep(procedure, o.procedure, true) 1298 && compareDeep(additive, o.additive, true) && compareDeep(time, o.time, true); 1299 } 1300 1301 @Override 1302 public boolean equalsShallow(Base other_) { 1303 if (!super.equalsShallow(other_)) 1304 return false; 1305 if (!(other_ instanceof SpecimenProcessingComponent)) 1306 return false; 1307 SpecimenProcessingComponent o = (SpecimenProcessingComponent) other_; 1308 return compareValues(description, o.description, true); 1309 } 1310 1311 public boolean isEmpty() { 1312 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, procedure, additive, time); 1313 } 1314 1315 public String fhirType() { 1316 return "Specimen.processing"; 1317 1318 } 1319 1320 } 1321 1322 @Block() 1323 public static class SpecimenContainerComponent extends BackboneElement implements IBaseBackboneElement { 1324 /** 1325 * Id for container. There may be multiple; a manufacturer's bar code, lab 1326 * assigned identifier, etc. The container ID may differ from the specimen id in 1327 * some circumstances. 1328 */ 1329 @Child(name = "identifier", type = { 1330 Identifier.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1331 @Description(shortDefinition = "Id for the container", formalDefinition = "Id for container. There may be multiple; a manufacturer's bar code, lab assigned identifier, etc. The container ID may differ from the specimen id in some circumstances.") 1332 protected List<Identifier> identifier; 1333 1334 /** 1335 * Textual description of the container. 1336 */ 1337 @Child(name = "description", type = { 1338 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1339 @Description(shortDefinition = "Textual description of the container", formalDefinition = "Textual description of the container.") 1340 protected StringType description; 1341 1342 /** 1343 * The type of container associated with the specimen (e.g. slide, aliquot, 1344 * etc.). 1345 */ 1346 @Child(name = "type", type = { 1347 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1348 @Description(shortDefinition = "Kind of container directly associated with specimen", formalDefinition = "The type of container associated with the specimen (e.g. slide, aliquot, etc.).") 1349 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/specimen-container-type") 1350 protected CodeableConcept type; 1351 1352 /** 1353 * The capacity (volume or other measure) the container may contain. 1354 */ 1355 @Child(name = "capacity", type = { Quantity.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1356 @Description(shortDefinition = "Container volume or size", formalDefinition = "The capacity (volume or other measure) the container may contain.") 1357 protected Quantity capacity; 1358 1359 /** 1360 * The quantity of specimen in the container; may be volume, dimensions, or 1361 * other appropriate measurements, depending on the specimen type. 1362 */ 1363 @Child(name = "specimenQuantity", type = { 1364 Quantity.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1365 @Description(shortDefinition = "Quantity of specimen within container", formalDefinition = "The quantity of specimen in the container; may be volume, dimensions, or other appropriate measurements, depending on the specimen type.") 1366 protected Quantity specimenQuantity; 1367 1368 /** 1369 * Introduced substance to preserve, maintain or enhance the specimen. Examples: 1370 * Formalin, Citrate, EDTA. 1371 */ 1372 @Child(name = "additive", type = { CodeableConcept.class, 1373 Substance.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 1374 @Description(shortDefinition = "Additive associated with container", formalDefinition = "Introduced substance to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.") 1375 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v2-0371") 1376 protected Type additive; 1377 1378 private static final long serialVersionUID = -1608132325L; 1379 1380 /** 1381 * Constructor 1382 */ 1383 public SpecimenContainerComponent() { 1384 super(); 1385 } 1386 1387 /** 1388 * @return {@link #identifier} (Id for container. There may be multiple; a 1389 * manufacturer's bar code, lab assigned identifier, etc. The container 1390 * ID may differ from the specimen id in some circumstances.) 1391 */ 1392 public List<Identifier> getIdentifier() { 1393 if (this.identifier == null) 1394 this.identifier = new ArrayList<Identifier>(); 1395 return this.identifier; 1396 } 1397 1398 /** 1399 * @return Returns a reference to <code>this</code> for easy method chaining 1400 */ 1401 public SpecimenContainerComponent setIdentifier(List<Identifier> theIdentifier) { 1402 this.identifier = theIdentifier; 1403 return this; 1404 } 1405 1406 public boolean hasIdentifier() { 1407 if (this.identifier == null) 1408 return false; 1409 for (Identifier item : this.identifier) 1410 if (!item.isEmpty()) 1411 return true; 1412 return false; 1413 } 1414 1415 public Identifier addIdentifier() { // 3 1416 Identifier t = new Identifier(); 1417 if (this.identifier == null) 1418 this.identifier = new ArrayList<Identifier>(); 1419 this.identifier.add(t); 1420 return t; 1421 } 1422 1423 public SpecimenContainerComponent addIdentifier(Identifier t) { // 3 1424 if (t == null) 1425 return this; 1426 if (this.identifier == null) 1427 this.identifier = new ArrayList<Identifier>(); 1428 this.identifier.add(t); 1429 return this; 1430 } 1431 1432 /** 1433 * @return The first repetition of repeating field {@link #identifier}, creating 1434 * it if it does not already exist 1435 */ 1436 public Identifier getIdentifierFirstRep() { 1437 if (getIdentifier().isEmpty()) { 1438 addIdentifier(); 1439 } 1440 return getIdentifier().get(0); 1441 } 1442 1443 /** 1444 * @return {@link #description} (Textual description of the container.). This is 1445 * the underlying object with id, value and extensions. The accessor 1446 * "getDescription" gives direct access to the value 1447 */ 1448 public StringType getDescriptionElement() { 1449 if (this.description == null) 1450 if (Configuration.errorOnAutoCreate()) 1451 throw new Error("Attempt to auto-create SpecimenContainerComponent.description"); 1452 else if (Configuration.doAutoCreate()) 1453 this.description = new StringType(); // bb 1454 return this.description; 1455 } 1456 1457 public boolean hasDescriptionElement() { 1458 return this.description != null && !this.description.isEmpty(); 1459 } 1460 1461 public boolean hasDescription() { 1462 return this.description != null && !this.description.isEmpty(); 1463 } 1464 1465 /** 1466 * @param value {@link #description} (Textual description of the container.). 1467 * This is the underlying object with id, value and extensions. The 1468 * accessor "getDescription" gives direct access to the value 1469 */ 1470 public SpecimenContainerComponent setDescriptionElement(StringType value) { 1471 this.description = value; 1472 return this; 1473 } 1474 1475 /** 1476 * @return Textual description of the container. 1477 */ 1478 public String getDescription() { 1479 return this.description == null ? null : this.description.getValue(); 1480 } 1481 1482 /** 1483 * @param value Textual description of the container. 1484 */ 1485 public SpecimenContainerComponent setDescription(String value) { 1486 if (Utilities.noString(value)) 1487 this.description = null; 1488 else { 1489 if (this.description == null) 1490 this.description = new StringType(); 1491 this.description.setValue(value); 1492 } 1493 return this; 1494 } 1495 1496 /** 1497 * @return {@link #type} (The type of container associated with the specimen 1498 * (e.g. slide, aliquot, etc.).) 1499 */ 1500 public CodeableConcept getType() { 1501 if (this.type == null) 1502 if (Configuration.errorOnAutoCreate()) 1503 throw new Error("Attempt to auto-create SpecimenContainerComponent.type"); 1504 else if (Configuration.doAutoCreate()) 1505 this.type = new CodeableConcept(); // cc 1506 return this.type; 1507 } 1508 1509 public boolean hasType() { 1510 return this.type != null && !this.type.isEmpty(); 1511 } 1512 1513 /** 1514 * @param value {@link #type} (The type of container associated with the 1515 * specimen (e.g. slide, aliquot, etc.).) 1516 */ 1517 public SpecimenContainerComponent setType(CodeableConcept value) { 1518 this.type = value; 1519 return this; 1520 } 1521 1522 /** 1523 * @return {@link #capacity} (The capacity (volume or other measure) the 1524 * container may contain.) 1525 */ 1526 public Quantity getCapacity() { 1527 if (this.capacity == null) 1528 if (Configuration.errorOnAutoCreate()) 1529 throw new Error("Attempt to auto-create SpecimenContainerComponent.capacity"); 1530 else if (Configuration.doAutoCreate()) 1531 this.capacity = new Quantity(); // cc 1532 return this.capacity; 1533 } 1534 1535 public boolean hasCapacity() { 1536 return this.capacity != null && !this.capacity.isEmpty(); 1537 } 1538 1539 /** 1540 * @param value {@link #capacity} (The capacity (volume or other measure) the 1541 * container may contain.) 1542 */ 1543 public SpecimenContainerComponent setCapacity(Quantity value) { 1544 this.capacity = value; 1545 return this; 1546 } 1547 1548 /** 1549 * @return {@link #specimenQuantity} (The quantity of specimen in the container; 1550 * may be volume, dimensions, or other appropriate measurements, 1551 * depending on the specimen type.) 1552 */ 1553 public Quantity getSpecimenQuantity() { 1554 if (this.specimenQuantity == null) 1555 if (Configuration.errorOnAutoCreate()) 1556 throw new Error("Attempt to auto-create SpecimenContainerComponent.specimenQuantity"); 1557 else if (Configuration.doAutoCreate()) 1558 this.specimenQuantity = new Quantity(); // cc 1559 return this.specimenQuantity; 1560 } 1561 1562 public boolean hasSpecimenQuantity() { 1563 return this.specimenQuantity != null && !this.specimenQuantity.isEmpty(); 1564 } 1565 1566 /** 1567 * @param value {@link #specimenQuantity} (The quantity of specimen in the 1568 * container; may be volume, dimensions, or other appropriate 1569 * measurements, depending on the specimen type.) 1570 */ 1571 public SpecimenContainerComponent setSpecimenQuantity(Quantity value) { 1572 this.specimenQuantity = value; 1573 return this; 1574 } 1575 1576 /** 1577 * @return {@link #additive} (Introduced substance to preserve, maintain or 1578 * enhance the specimen. Examples: Formalin, Citrate, EDTA.) 1579 */ 1580 public Type getAdditive() { 1581 return this.additive; 1582 } 1583 1584 /** 1585 * @return {@link #additive} (Introduced substance to preserve, maintain or 1586 * enhance the specimen. Examples: Formalin, Citrate, EDTA.) 1587 */ 1588 public CodeableConcept getAdditiveCodeableConcept() throws FHIRException { 1589 if (this.additive == null) 1590 this.additive = new CodeableConcept(); 1591 if (!(this.additive instanceof CodeableConcept)) 1592 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1593 + this.additive.getClass().getName() + " was encountered"); 1594 return (CodeableConcept) this.additive; 1595 } 1596 1597 public boolean hasAdditiveCodeableConcept() { 1598 return this != null && this.additive instanceof CodeableConcept; 1599 } 1600 1601 /** 1602 * @return {@link #additive} (Introduced substance to preserve, maintain or 1603 * enhance the specimen. Examples: Formalin, Citrate, EDTA.) 1604 */ 1605 public Reference getAdditiveReference() throws FHIRException { 1606 if (this.additive == null) 1607 this.additive = new Reference(); 1608 if (!(this.additive instanceof Reference)) 1609 throw new FHIRException("Type mismatch: the type Reference was expected, but " 1610 + this.additive.getClass().getName() + " was encountered"); 1611 return (Reference) this.additive; 1612 } 1613 1614 public boolean hasAdditiveReference() { 1615 return this != null && this.additive instanceof Reference; 1616 } 1617 1618 public boolean hasAdditive() { 1619 return this.additive != null && !this.additive.isEmpty(); 1620 } 1621 1622 /** 1623 * @param value {@link #additive} (Introduced substance to preserve, maintain or 1624 * enhance the specimen. Examples: Formalin, Citrate, EDTA.) 1625 */ 1626 public SpecimenContainerComponent setAdditive(Type value) { 1627 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1628 throw new Error("Not the right type for Specimen.container.additive[x]: " + value.fhirType()); 1629 this.additive = value; 1630 return this; 1631 } 1632 1633 protected void listChildren(List<Property> children) { 1634 super.listChildren(children); 1635 children.add(new Property("identifier", "Identifier", 1636 "Id for container. There may be multiple; a manufacturer's bar code, lab assigned identifier, etc. The container ID may differ from the specimen id in some circumstances.", 1637 0, java.lang.Integer.MAX_VALUE, identifier)); 1638 children.add(new Property("description", "string", "Textual description of the container.", 0, 1, description)); 1639 children.add(new Property("type", "CodeableConcept", 1640 "The type of container associated with the specimen (e.g. slide, aliquot, etc.).", 0, 1, type)); 1641 children.add(new Property("capacity", "SimpleQuantity", 1642 "The capacity (volume or other measure) the container may contain.", 0, 1, capacity)); 1643 children.add(new Property("specimenQuantity", "SimpleQuantity", 1644 "The quantity of specimen in the container; may be volume, dimensions, or other appropriate measurements, depending on the specimen type.", 1645 0, 1, specimenQuantity)); 1646 children.add(new Property("additive[x]", "CodeableConcept|Reference(Substance)", 1647 "Introduced substance to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 0, 1648 1, additive)); 1649 } 1650 1651 @Override 1652 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1653 switch (_hash) { 1654 case -1618432855: 1655 /* identifier */ return new Property("identifier", "Identifier", 1656 "Id for container. There may be multiple; a manufacturer's bar code, lab assigned identifier, etc. The container ID may differ from the specimen id in some circumstances.", 1657 0, java.lang.Integer.MAX_VALUE, identifier); 1658 case -1724546052: 1659 /* description */ return new Property("description", "string", "Textual description of the container.", 0, 1, 1660 description); 1661 case 3575610: 1662 /* type */ return new Property("type", "CodeableConcept", 1663 "The type of container associated with the specimen (e.g. slide, aliquot, etc.).", 0, 1, type); 1664 case -67824454: 1665 /* capacity */ return new Property("capacity", "SimpleQuantity", 1666 "The capacity (volume or other measure) the container may contain.", 0, 1, capacity); 1667 case 1485980595: 1668 /* specimenQuantity */ return new Property("specimenQuantity", "SimpleQuantity", 1669 "The quantity of specimen in the container; may be volume, dimensions, or other appropriate measurements, depending on the specimen type.", 1670 0, 1, specimenQuantity); 1671 case 261915956: 1672 /* additive[x] */ return new Property("additive[x]", "CodeableConcept|Reference(Substance)", 1673 "Introduced substance to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 0, 1674 1, additive); 1675 case -1226589236: 1676 /* additive */ return new Property("additive[x]", "CodeableConcept|Reference(Substance)", 1677 "Introduced substance to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 0, 1678 1, additive); 1679 case 1330272821: 1680 /* additiveCodeableConcept */ return new Property("additive[x]", "CodeableConcept|Reference(Substance)", 1681 "Introduced substance to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 0, 1682 1, additive); 1683 case -386783009: 1684 /* additiveReference */ return new Property("additive[x]", "CodeableConcept|Reference(Substance)", 1685 "Introduced substance to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 0, 1686 1, additive); 1687 default: 1688 return super.getNamedProperty(_hash, _name, _checkValid); 1689 } 1690 1691 } 1692 1693 @Override 1694 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1695 switch (hash) { 1696 case -1618432855: 1697 /* identifier */ return this.identifier == null ? new Base[0] 1698 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1699 case -1724546052: 1700 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1701 case 3575610: 1702 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1703 case -67824454: 1704 /* capacity */ return this.capacity == null ? new Base[0] : new Base[] { this.capacity }; // Quantity 1705 case 1485980595: 1706 /* specimenQuantity */ return this.specimenQuantity == null ? new Base[0] 1707 : new Base[] { this.specimenQuantity }; // Quantity 1708 case -1226589236: 1709 /* additive */ return this.additive == null ? new Base[0] : new Base[] { this.additive }; // Type 1710 default: 1711 return super.getProperty(hash, name, checkValid); 1712 } 1713 1714 } 1715 1716 @Override 1717 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1718 switch (hash) { 1719 case -1618432855: // identifier 1720 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1721 return value; 1722 case -1724546052: // description 1723 this.description = castToString(value); // StringType 1724 return value; 1725 case 3575610: // type 1726 this.type = castToCodeableConcept(value); // CodeableConcept 1727 return value; 1728 case -67824454: // capacity 1729 this.capacity = castToQuantity(value); // Quantity 1730 return value; 1731 case 1485980595: // specimenQuantity 1732 this.specimenQuantity = castToQuantity(value); // Quantity 1733 return value; 1734 case -1226589236: // additive 1735 this.additive = castToType(value); // Type 1736 return value; 1737 default: 1738 return super.setProperty(hash, name, value); 1739 } 1740 1741 } 1742 1743 @Override 1744 public Base setProperty(String name, Base value) throws FHIRException { 1745 if (name.equals("identifier")) { 1746 this.getIdentifier().add(castToIdentifier(value)); 1747 } else if (name.equals("description")) { 1748 this.description = castToString(value); // StringType 1749 } else if (name.equals("type")) { 1750 this.type = castToCodeableConcept(value); // CodeableConcept 1751 } else if (name.equals("capacity")) { 1752 this.capacity = castToQuantity(value); // Quantity 1753 } else if (name.equals("specimenQuantity")) { 1754 this.specimenQuantity = castToQuantity(value); // Quantity 1755 } else if (name.equals("additive[x]")) { 1756 this.additive = castToType(value); // Type 1757 } else 1758 return super.setProperty(name, value); 1759 return value; 1760 } 1761 1762 @Override 1763 public Base makeProperty(int hash, String name) throws FHIRException { 1764 switch (hash) { 1765 case -1618432855: 1766 return addIdentifier(); 1767 case -1724546052: 1768 return getDescriptionElement(); 1769 case 3575610: 1770 return getType(); 1771 case -67824454: 1772 return getCapacity(); 1773 case 1485980595: 1774 return getSpecimenQuantity(); 1775 case 261915956: 1776 return getAdditive(); 1777 case -1226589236: 1778 return getAdditive(); 1779 default: 1780 return super.makeProperty(hash, name); 1781 } 1782 1783 } 1784 1785 @Override 1786 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1787 switch (hash) { 1788 case -1618432855: 1789 /* identifier */ return new String[] { "Identifier" }; 1790 case -1724546052: 1791 /* description */ return new String[] { "string" }; 1792 case 3575610: 1793 /* type */ return new String[] { "CodeableConcept" }; 1794 case -67824454: 1795 /* capacity */ return new String[] { "SimpleQuantity" }; 1796 case 1485980595: 1797 /* specimenQuantity */ return new String[] { "SimpleQuantity" }; 1798 case -1226589236: 1799 /* additive */ return new String[] { "CodeableConcept", "Reference" }; 1800 default: 1801 return super.getTypesForProperty(hash, name); 1802 } 1803 1804 } 1805 1806 @Override 1807 public Base addChild(String name) throws FHIRException { 1808 if (name.equals("identifier")) { 1809 return addIdentifier(); 1810 } else if (name.equals("description")) { 1811 throw new FHIRException("Cannot call addChild on a singleton property Specimen.description"); 1812 } else if (name.equals("type")) { 1813 this.type = new CodeableConcept(); 1814 return this.type; 1815 } else if (name.equals("capacity")) { 1816 this.capacity = new Quantity(); 1817 return this.capacity; 1818 } else if (name.equals("specimenQuantity")) { 1819 this.specimenQuantity = new Quantity(); 1820 return this.specimenQuantity; 1821 } else if (name.equals("additiveCodeableConcept")) { 1822 this.additive = new CodeableConcept(); 1823 return this.additive; 1824 } else if (name.equals("additiveReference")) { 1825 this.additive = new Reference(); 1826 return this.additive; 1827 } else 1828 return super.addChild(name); 1829 } 1830 1831 public SpecimenContainerComponent copy() { 1832 SpecimenContainerComponent dst = new SpecimenContainerComponent(); 1833 copyValues(dst); 1834 return dst; 1835 } 1836 1837 public void copyValues(SpecimenContainerComponent dst) { 1838 super.copyValues(dst); 1839 if (identifier != null) { 1840 dst.identifier = new ArrayList<Identifier>(); 1841 for (Identifier i : identifier) 1842 dst.identifier.add(i.copy()); 1843 } 1844 ; 1845 dst.description = description == null ? null : description.copy(); 1846 dst.type = type == null ? null : type.copy(); 1847 dst.capacity = capacity == null ? null : capacity.copy(); 1848 dst.specimenQuantity = specimenQuantity == null ? null : specimenQuantity.copy(); 1849 dst.additive = additive == null ? null : additive.copy(); 1850 } 1851 1852 @Override 1853 public boolean equalsDeep(Base other_) { 1854 if (!super.equalsDeep(other_)) 1855 return false; 1856 if (!(other_ instanceof SpecimenContainerComponent)) 1857 return false; 1858 SpecimenContainerComponent o = (SpecimenContainerComponent) other_; 1859 return compareDeep(identifier, o.identifier, true) && compareDeep(description, o.description, true) 1860 && compareDeep(type, o.type, true) && compareDeep(capacity, o.capacity, true) 1861 && compareDeep(specimenQuantity, o.specimenQuantity, true) && compareDeep(additive, o.additive, true); 1862 } 1863 1864 @Override 1865 public boolean equalsShallow(Base other_) { 1866 if (!super.equalsShallow(other_)) 1867 return false; 1868 if (!(other_ instanceof SpecimenContainerComponent)) 1869 return false; 1870 SpecimenContainerComponent o = (SpecimenContainerComponent) other_; 1871 return compareValues(description, o.description, true); 1872 } 1873 1874 public boolean isEmpty() { 1875 return super.isEmpty() 1876 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, description, type, capacity, specimenQuantity, additive); 1877 } 1878 1879 public String fhirType() { 1880 return "Specimen.container"; 1881 1882 } 1883 1884 } 1885 1886 /** 1887 * Id for specimen. 1888 */ 1889 @Child(name = "identifier", type = { 1890 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1891 @Description(shortDefinition = "External Identifier", formalDefinition = "Id for specimen.") 1892 protected List<Identifier> identifier; 1893 1894 /** 1895 * The identifier assigned by the lab when accessioning specimen(s). This is not 1896 * necessarily the same as the specimen identifier, depending on local lab 1897 * procedures. 1898 */ 1899 @Child(name = "accessionIdentifier", type = { 1900 Identifier.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1901 @Description(shortDefinition = "Identifier assigned by the lab", formalDefinition = "The identifier assigned by the lab when accessioning specimen(s). This is not necessarily the same as the specimen identifier, depending on local lab procedures.") 1902 protected Identifier accessionIdentifier; 1903 1904 /** 1905 * The availability of the specimen. 1906 */ 1907 @Child(name = "status", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = true, summary = true) 1908 @Description(shortDefinition = "available | unavailable | unsatisfactory | entered-in-error", formalDefinition = "The availability of the specimen.") 1909 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/specimen-status") 1910 protected Enumeration<SpecimenStatus> status; 1911 1912 /** 1913 * The kind of material that forms the specimen. 1914 */ 1915 @Child(name = "type", type = { CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1916 @Description(shortDefinition = "Kind of material that forms the specimen", formalDefinition = "The kind of material that forms the specimen.") 1917 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v2-0487") 1918 protected CodeableConcept type; 1919 1920 /** 1921 * Where the specimen came from. This may be from patient(s), from a location 1922 * (e.g., the source of an environmental sample), or a sampling of a substance 1923 * or a device. 1924 */ 1925 @Child(name = "subject", type = { Patient.class, Group.class, Device.class, Substance.class, 1926 Location.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1927 @Description(shortDefinition = "Where the specimen came from. This may be from patient(s), from a location (e.g., the source of an environmental sample), or a sampling of a substance or a device", formalDefinition = "Where the specimen came from. This may be from patient(s), from a location (e.g., the source of an environmental sample), or a sampling of a substance or a device.") 1928 protected Reference subject; 1929 1930 /** 1931 * The actual object that is the target of the reference (Where the specimen 1932 * came from. This may be from patient(s), from a location (e.g., the source of 1933 * an environmental sample), or a sampling of a substance or a device.) 1934 */ 1935 protected Resource subjectTarget; 1936 1937 /** 1938 * Time when specimen was received for processing or testing. 1939 */ 1940 @Child(name = "receivedTime", type = { 1941 DateTimeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1942 @Description(shortDefinition = "The time when specimen was received for processing", formalDefinition = "Time when specimen was received for processing or testing.") 1943 protected DateTimeType receivedTime; 1944 1945 /** 1946 * Reference to the parent (source) specimen which is used when the specimen was 1947 * either derived from or a component of another specimen. 1948 */ 1949 @Child(name = "parent", type = { 1950 Specimen.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1951 @Description(shortDefinition = "Specimen from which this specimen originated", formalDefinition = "Reference to the parent (source) specimen which is used when the specimen was either derived from or a component of another specimen.") 1952 protected List<Reference> parent; 1953 /** 1954 * The actual objects that are the target of the reference (Reference to the 1955 * parent (source) specimen which is used when the specimen was either derived 1956 * from or a component of another specimen.) 1957 */ 1958 protected List<Specimen> parentTarget; 1959 1960 /** 1961 * Details concerning a service request that required a specimen to be 1962 * collected. 1963 */ 1964 @Child(name = "request", type = { 1965 ServiceRequest.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1966 @Description(shortDefinition = "Why the specimen was collected", formalDefinition = "Details concerning a service request that required a specimen to be collected.") 1967 protected List<Reference> request; 1968 /** 1969 * The actual objects that are the target of the reference (Details concerning a 1970 * service request that required a specimen to be collected.) 1971 */ 1972 protected List<ServiceRequest> requestTarget; 1973 1974 /** 1975 * Details concerning the specimen collection. 1976 */ 1977 @Child(name = "collection", type = {}, order = 8, min = 0, max = 1, modifier = false, summary = false) 1978 @Description(shortDefinition = "Collection details", formalDefinition = "Details concerning the specimen collection.") 1979 protected SpecimenCollectionComponent collection; 1980 1981 /** 1982 * Details concerning processing and processing steps for the specimen. 1983 */ 1984 @Child(name = "processing", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1985 @Description(shortDefinition = "Processing and processing step details", formalDefinition = "Details concerning processing and processing steps for the specimen.") 1986 protected List<SpecimenProcessingComponent> processing; 1987 1988 /** 1989 * The container holding the specimen. The recursive nature of containers; i.e. 1990 * blood in tube in tray in rack is not addressed here. 1991 */ 1992 @Child(name = "container", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1993 @Description(shortDefinition = "Direct container of specimen (tube/slide, etc.)", formalDefinition = "The container holding the specimen. The recursive nature of containers; i.e. blood in tube in tray in rack is not addressed here.") 1994 protected List<SpecimenContainerComponent> container; 1995 1996 /** 1997 * A mode or state of being that describes the nature of the specimen. 1998 */ 1999 @Child(name = "condition", type = { 2000 CodeableConcept.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2001 @Description(shortDefinition = "State of the specimen", formalDefinition = "A mode or state of being that describes the nature of the specimen.") 2002 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v2-0493") 2003 protected List<CodeableConcept> condition; 2004 2005 /** 2006 * To communicate any details or issues about the specimen or during the 2007 * specimen collection. (for example: broken vial, sent with patient, frozen). 2008 */ 2009 @Child(name = "note", type = { 2010 Annotation.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2011 @Description(shortDefinition = "Comments", formalDefinition = "To communicate any details or issues about the specimen or during the specimen collection. (for example: broken vial, sent with patient, frozen).") 2012 protected List<Annotation> note; 2013 2014 private static final long serialVersionUID = 1441502239L; 2015 2016 /** 2017 * Constructor 2018 */ 2019 public Specimen() { 2020 super(); 2021 } 2022 2023 /** 2024 * @return {@link #identifier} (Id for specimen.) 2025 */ 2026 public List<Identifier> getIdentifier() { 2027 if (this.identifier == null) 2028 this.identifier = new ArrayList<Identifier>(); 2029 return this.identifier; 2030 } 2031 2032 /** 2033 * @return Returns a reference to <code>this</code> for easy method chaining 2034 */ 2035 public Specimen setIdentifier(List<Identifier> theIdentifier) { 2036 this.identifier = theIdentifier; 2037 return this; 2038 } 2039 2040 public boolean hasIdentifier() { 2041 if (this.identifier == null) 2042 return false; 2043 for (Identifier item : this.identifier) 2044 if (!item.isEmpty()) 2045 return true; 2046 return false; 2047 } 2048 2049 public Identifier addIdentifier() { // 3 2050 Identifier t = new Identifier(); 2051 if (this.identifier == null) 2052 this.identifier = new ArrayList<Identifier>(); 2053 this.identifier.add(t); 2054 return t; 2055 } 2056 2057 public Specimen addIdentifier(Identifier t) { // 3 2058 if (t == null) 2059 return this; 2060 if (this.identifier == null) 2061 this.identifier = new ArrayList<Identifier>(); 2062 this.identifier.add(t); 2063 return this; 2064 } 2065 2066 /** 2067 * @return The first repetition of repeating field {@link #identifier}, creating 2068 * it if it does not already exist 2069 */ 2070 public Identifier getIdentifierFirstRep() { 2071 if (getIdentifier().isEmpty()) { 2072 addIdentifier(); 2073 } 2074 return getIdentifier().get(0); 2075 } 2076 2077 /** 2078 * @return {@link #accessionIdentifier} (The identifier assigned by the lab when 2079 * accessioning specimen(s). This is not necessarily the same as the 2080 * specimen identifier, depending on local lab procedures.) 2081 */ 2082 public Identifier getAccessionIdentifier() { 2083 if (this.accessionIdentifier == null) 2084 if (Configuration.errorOnAutoCreate()) 2085 throw new Error("Attempt to auto-create Specimen.accessionIdentifier"); 2086 else if (Configuration.doAutoCreate()) 2087 this.accessionIdentifier = new Identifier(); // cc 2088 return this.accessionIdentifier; 2089 } 2090 2091 public boolean hasAccessionIdentifier() { 2092 return this.accessionIdentifier != null && !this.accessionIdentifier.isEmpty(); 2093 } 2094 2095 /** 2096 * @param value {@link #accessionIdentifier} (The identifier assigned by the lab 2097 * when accessioning specimen(s). This is not necessarily the same 2098 * as the specimen identifier, depending on local lab procedures.) 2099 */ 2100 public Specimen setAccessionIdentifier(Identifier value) { 2101 this.accessionIdentifier = value; 2102 return this; 2103 } 2104 2105 /** 2106 * @return {@link #status} (The availability of the specimen.). This is the 2107 * underlying object with id, value and extensions. The accessor 2108 * "getStatus" gives direct access to the value 2109 */ 2110 public Enumeration<SpecimenStatus> getStatusElement() { 2111 if (this.status == null) 2112 if (Configuration.errorOnAutoCreate()) 2113 throw new Error("Attempt to auto-create Specimen.status"); 2114 else if (Configuration.doAutoCreate()) 2115 this.status = new Enumeration<SpecimenStatus>(new SpecimenStatusEnumFactory()); // bb 2116 return this.status; 2117 } 2118 2119 public boolean hasStatusElement() { 2120 return this.status != null && !this.status.isEmpty(); 2121 } 2122 2123 public boolean hasStatus() { 2124 return this.status != null && !this.status.isEmpty(); 2125 } 2126 2127 /** 2128 * @param value {@link #status} (The availability of the specimen.). This is the 2129 * underlying object with id, value and extensions. The accessor 2130 * "getStatus" gives direct access to the value 2131 */ 2132 public Specimen setStatusElement(Enumeration<SpecimenStatus> value) { 2133 this.status = value; 2134 return this; 2135 } 2136 2137 /** 2138 * @return The availability of the specimen. 2139 */ 2140 public SpecimenStatus getStatus() { 2141 return this.status == null ? null : this.status.getValue(); 2142 } 2143 2144 /** 2145 * @param value The availability of the specimen. 2146 */ 2147 public Specimen setStatus(SpecimenStatus value) { 2148 if (value == null) 2149 this.status = null; 2150 else { 2151 if (this.status == null) 2152 this.status = new Enumeration<SpecimenStatus>(new SpecimenStatusEnumFactory()); 2153 this.status.setValue(value); 2154 } 2155 return this; 2156 } 2157 2158 /** 2159 * @return {@link #type} (The kind of material that forms the specimen.) 2160 */ 2161 public CodeableConcept getType() { 2162 if (this.type == null) 2163 if (Configuration.errorOnAutoCreate()) 2164 throw new Error("Attempt to auto-create Specimen.type"); 2165 else if (Configuration.doAutoCreate()) 2166 this.type = new CodeableConcept(); // cc 2167 return this.type; 2168 } 2169 2170 public boolean hasType() { 2171 return this.type != null && !this.type.isEmpty(); 2172 } 2173 2174 /** 2175 * @param value {@link #type} (The kind of material that forms the specimen.) 2176 */ 2177 public Specimen setType(CodeableConcept value) { 2178 this.type = value; 2179 return this; 2180 } 2181 2182 /** 2183 * @return {@link #subject} (Where the specimen came from. This may be from 2184 * patient(s), from a location (e.g., the source of an environmental 2185 * sample), or a sampling of a substance or a device.) 2186 */ 2187 public Reference getSubject() { 2188 if (this.subject == null) 2189 if (Configuration.errorOnAutoCreate()) 2190 throw new Error("Attempt to auto-create Specimen.subject"); 2191 else if (Configuration.doAutoCreate()) 2192 this.subject = new Reference(); // cc 2193 return this.subject; 2194 } 2195 2196 public boolean hasSubject() { 2197 return this.subject != null && !this.subject.isEmpty(); 2198 } 2199 2200 /** 2201 * @param value {@link #subject} (Where the specimen came from. This may be from 2202 * patient(s), from a location (e.g., the source of an 2203 * environmental sample), or a sampling of a substance or a 2204 * device.) 2205 */ 2206 public Specimen setSubject(Reference value) { 2207 this.subject = value; 2208 return this; 2209 } 2210 2211 /** 2212 * @return {@link #subject} The actual object that is the target of the 2213 * reference. The reference library doesn't populate this, but you can 2214 * use it to hold the resource if you resolve it. (Where the specimen 2215 * came from. This may be from patient(s), from a location (e.g., the 2216 * source of an environmental sample), or a sampling of a substance or a 2217 * device.) 2218 */ 2219 public Resource getSubjectTarget() { 2220 return this.subjectTarget; 2221 } 2222 2223 /** 2224 * @param value {@link #subject} The actual object that is the target of the 2225 * reference. The reference library doesn't use these, but you can 2226 * use it to hold the resource if you resolve it. (Where the 2227 * specimen came from. This may be from patient(s), from a location 2228 * (e.g., the source of an environmental sample), or a sampling of 2229 * a substance or a device.) 2230 */ 2231 public Specimen setSubjectTarget(Resource value) { 2232 this.subjectTarget = value; 2233 return this; 2234 } 2235 2236 /** 2237 * @return {@link #receivedTime} (Time when specimen was received for processing 2238 * or testing.). This is the underlying object with id, value and 2239 * extensions. The accessor "getReceivedTime" gives direct access to the 2240 * value 2241 */ 2242 public DateTimeType getReceivedTimeElement() { 2243 if (this.receivedTime == null) 2244 if (Configuration.errorOnAutoCreate()) 2245 throw new Error("Attempt to auto-create Specimen.receivedTime"); 2246 else if (Configuration.doAutoCreate()) 2247 this.receivedTime = new DateTimeType(); // bb 2248 return this.receivedTime; 2249 } 2250 2251 public boolean hasReceivedTimeElement() { 2252 return this.receivedTime != null && !this.receivedTime.isEmpty(); 2253 } 2254 2255 public boolean hasReceivedTime() { 2256 return this.receivedTime != null && !this.receivedTime.isEmpty(); 2257 } 2258 2259 /** 2260 * @param value {@link #receivedTime} (Time when specimen was received for 2261 * processing or testing.). This is the underlying object with id, 2262 * value and extensions. The accessor "getReceivedTime" gives 2263 * direct access to the value 2264 */ 2265 public Specimen setReceivedTimeElement(DateTimeType value) { 2266 this.receivedTime = value; 2267 return this; 2268 } 2269 2270 /** 2271 * @return Time when specimen was received for processing or testing. 2272 */ 2273 public Date getReceivedTime() { 2274 return this.receivedTime == null ? null : this.receivedTime.getValue(); 2275 } 2276 2277 /** 2278 * @param value Time when specimen was received for processing or testing. 2279 */ 2280 public Specimen setReceivedTime(Date value) { 2281 if (value == null) 2282 this.receivedTime = null; 2283 else { 2284 if (this.receivedTime == null) 2285 this.receivedTime = new DateTimeType(); 2286 this.receivedTime.setValue(value); 2287 } 2288 return this; 2289 } 2290 2291 /** 2292 * @return {@link #parent} (Reference to the parent (source) specimen which is 2293 * used when the specimen was either derived from or a component of 2294 * another specimen.) 2295 */ 2296 public List<Reference> getParent() { 2297 if (this.parent == null) 2298 this.parent = new ArrayList<Reference>(); 2299 return this.parent; 2300 } 2301 2302 /** 2303 * @return Returns a reference to <code>this</code> for easy method chaining 2304 */ 2305 public Specimen setParent(List<Reference> theParent) { 2306 this.parent = theParent; 2307 return this; 2308 } 2309 2310 public boolean hasParent() { 2311 if (this.parent == null) 2312 return false; 2313 for (Reference item : this.parent) 2314 if (!item.isEmpty()) 2315 return true; 2316 return false; 2317 } 2318 2319 public Reference addParent() { // 3 2320 Reference t = new Reference(); 2321 if (this.parent == null) 2322 this.parent = new ArrayList<Reference>(); 2323 this.parent.add(t); 2324 return t; 2325 } 2326 2327 public Specimen addParent(Reference t) { // 3 2328 if (t == null) 2329 return this; 2330 if (this.parent == null) 2331 this.parent = new ArrayList<Reference>(); 2332 this.parent.add(t); 2333 return this; 2334 } 2335 2336 /** 2337 * @return The first repetition of repeating field {@link #parent}, creating it 2338 * if it does not already exist 2339 */ 2340 public Reference getParentFirstRep() { 2341 if (getParent().isEmpty()) { 2342 addParent(); 2343 } 2344 return getParent().get(0); 2345 } 2346 2347 /** 2348 * @deprecated Use Reference#setResource(IBaseResource) instead 2349 */ 2350 @Deprecated 2351 public List<Specimen> getParentTarget() { 2352 if (this.parentTarget == null) 2353 this.parentTarget = new ArrayList<Specimen>(); 2354 return this.parentTarget; 2355 } 2356 2357 /** 2358 * @deprecated Use Reference#setResource(IBaseResource) instead 2359 */ 2360 @Deprecated 2361 public Specimen addParentTarget() { 2362 Specimen r = new Specimen(); 2363 if (this.parentTarget == null) 2364 this.parentTarget = new ArrayList<Specimen>(); 2365 this.parentTarget.add(r); 2366 return r; 2367 } 2368 2369 /** 2370 * @return {@link #request} (Details concerning a service request that required 2371 * a specimen to be collected.) 2372 */ 2373 public List<Reference> getRequest() { 2374 if (this.request == null) 2375 this.request = new ArrayList<Reference>(); 2376 return this.request; 2377 } 2378 2379 /** 2380 * @return Returns a reference to <code>this</code> for easy method chaining 2381 */ 2382 public Specimen setRequest(List<Reference> theRequest) { 2383 this.request = theRequest; 2384 return this; 2385 } 2386 2387 public boolean hasRequest() { 2388 if (this.request == null) 2389 return false; 2390 for (Reference item : this.request) 2391 if (!item.isEmpty()) 2392 return true; 2393 return false; 2394 } 2395 2396 public Reference addRequest() { // 3 2397 Reference t = new Reference(); 2398 if (this.request == null) 2399 this.request = new ArrayList<Reference>(); 2400 this.request.add(t); 2401 return t; 2402 } 2403 2404 public Specimen addRequest(Reference t) { // 3 2405 if (t == null) 2406 return this; 2407 if (this.request == null) 2408 this.request = new ArrayList<Reference>(); 2409 this.request.add(t); 2410 return this; 2411 } 2412 2413 /** 2414 * @return The first repetition of repeating field {@link #request}, creating it 2415 * if it does not already exist 2416 */ 2417 public Reference getRequestFirstRep() { 2418 if (getRequest().isEmpty()) { 2419 addRequest(); 2420 } 2421 return getRequest().get(0); 2422 } 2423 2424 /** 2425 * @deprecated Use Reference#setResource(IBaseResource) instead 2426 */ 2427 @Deprecated 2428 public List<ServiceRequest> getRequestTarget() { 2429 if (this.requestTarget == null) 2430 this.requestTarget = new ArrayList<ServiceRequest>(); 2431 return this.requestTarget; 2432 } 2433 2434 /** 2435 * @deprecated Use Reference#setResource(IBaseResource) instead 2436 */ 2437 @Deprecated 2438 public ServiceRequest addRequestTarget() { 2439 ServiceRequest r = new ServiceRequest(); 2440 if (this.requestTarget == null) 2441 this.requestTarget = new ArrayList<ServiceRequest>(); 2442 this.requestTarget.add(r); 2443 return r; 2444 } 2445 2446 /** 2447 * @return {@link #collection} (Details concerning the specimen collection.) 2448 */ 2449 public SpecimenCollectionComponent getCollection() { 2450 if (this.collection == null) 2451 if (Configuration.errorOnAutoCreate()) 2452 throw new Error("Attempt to auto-create Specimen.collection"); 2453 else if (Configuration.doAutoCreate()) 2454 this.collection = new SpecimenCollectionComponent(); // cc 2455 return this.collection; 2456 } 2457 2458 public boolean hasCollection() { 2459 return this.collection != null && !this.collection.isEmpty(); 2460 } 2461 2462 /** 2463 * @param value {@link #collection} (Details concerning the specimen 2464 * collection.) 2465 */ 2466 public Specimen setCollection(SpecimenCollectionComponent value) { 2467 this.collection = value; 2468 return this; 2469 } 2470 2471 /** 2472 * @return {@link #processing} (Details concerning processing and processing 2473 * steps for the specimen.) 2474 */ 2475 public List<SpecimenProcessingComponent> getProcessing() { 2476 if (this.processing == null) 2477 this.processing = new ArrayList<SpecimenProcessingComponent>(); 2478 return this.processing; 2479 } 2480 2481 /** 2482 * @return Returns a reference to <code>this</code> for easy method chaining 2483 */ 2484 public Specimen setProcessing(List<SpecimenProcessingComponent> theProcessing) { 2485 this.processing = theProcessing; 2486 return this; 2487 } 2488 2489 public boolean hasProcessing() { 2490 if (this.processing == null) 2491 return false; 2492 for (SpecimenProcessingComponent item : this.processing) 2493 if (!item.isEmpty()) 2494 return true; 2495 return false; 2496 } 2497 2498 public SpecimenProcessingComponent addProcessing() { // 3 2499 SpecimenProcessingComponent t = new SpecimenProcessingComponent(); 2500 if (this.processing == null) 2501 this.processing = new ArrayList<SpecimenProcessingComponent>(); 2502 this.processing.add(t); 2503 return t; 2504 } 2505 2506 public Specimen addProcessing(SpecimenProcessingComponent t) { // 3 2507 if (t == null) 2508 return this; 2509 if (this.processing == null) 2510 this.processing = new ArrayList<SpecimenProcessingComponent>(); 2511 this.processing.add(t); 2512 return this; 2513 } 2514 2515 /** 2516 * @return The first repetition of repeating field {@link #processing}, creating 2517 * it if it does not already exist 2518 */ 2519 public SpecimenProcessingComponent getProcessingFirstRep() { 2520 if (getProcessing().isEmpty()) { 2521 addProcessing(); 2522 } 2523 return getProcessing().get(0); 2524 } 2525 2526 /** 2527 * @return {@link #container} (The container holding the specimen. The recursive 2528 * nature of containers; i.e. blood in tube in tray in rack is not 2529 * addressed here.) 2530 */ 2531 public List<SpecimenContainerComponent> getContainer() { 2532 if (this.container == null) 2533 this.container = new ArrayList<SpecimenContainerComponent>(); 2534 return this.container; 2535 } 2536 2537 /** 2538 * @return Returns a reference to <code>this</code> for easy method chaining 2539 */ 2540 public Specimen setContainer(List<SpecimenContainerComponent> theContainer) { 2541 this.container = theContainer; 2542 return this; 2543 } 2544 2545 public boolean hasContainer() { 2546 if (this.container == null) 2547 return false; 2548 for (SpecimenContainerComponent item : this.container) 2549 if (!item.isEmpty()) 2550 return true; 2551 return false; 2552 } 2553 2554 public SpecimenContainerComponent addContainer() { // 3 2555 SpecimenContainerComponent t = new SpecimenContainerComponent(); 2556 if (this.container == null) 2557 this.container = new ArrayList<SpecimenContainerComponent>(); 2558 this.container.add(t); 2559 return t; 2560 } 2561 2562 public Specimen addContainer(SpecimenContainerComponent t) { // 3 2563 if (t == null) 2564 return this; 2565 if (this.container == null) 2566 this.container = new ArrayList<SpecimenContainerComponent>(); 2567 this.container.add(t); 2568 return this; 2569 } 2570 2571 /** 2572 * @return The first repetition of repeating field {@link #container}, creating 2573 * it if it does not already exist 2574 */ 2575 public SpecimenContainerComponent getContainerFirstRep() { 2576 if (getContainer().isEmpty()) { 2577 addContainer(); 2578 } 2579 return getContainer().get(0); 2580 } 2581 2582 /** 2583 * @return {@link #condition} (A mode or state of being that describes the 2584 * nature of the specimen.) 2585 */ 2586 public List<CodeableConcept> getCondition() { 2587 if (this.condition == null) 2588 this.condition = new ArrayList<CodeableConcept>(); 2589 return this.condition; 2590 } 2591 2592 /** 2593 * @return Returns a reference to <code>this</code> for easy method chaining 2594 */ 2595 public Specimen setCondition(List<CodeableConcept> theCondition) { 2596 this.condition = theCondition; 2597 return this; 2598 } 2599 2600 public boolean hasCondition() { 2601 if (this.condition == null) 2602 return false; 2603 for (CodeableConcept item : this.condition) 2604 if (!item.isEmpty()) 2605 return true; 2606 return false; 2607 } 2608 2609 public CodeableConcept addCondition() { // 3 2610 CodeableConcept t = new CodeableConcept(); 2611 if (this.condition == null) 2612 this.condition = new ArrayList<CodeableConcept>(); 2613 this.condition.add(t); 2614 return t; 2615 } 2616 2617 public Specimen addCondition(CodeableConcept t) { // 3 2618 if (t == null) 2619 return this; 2620 if (this.condition == null) 2621 this.condition = new ArrayList<CodeableConcept>(); 2622 this.condition.add(t); 2623 return this; 2624 } 2625 2626 /** 2627 * @return The first repetition of repeating field {@link #condition}, creating 2628 * it if it does not already exist 2629 */ 2630 public CodeableConcept getConditionFirstRep() { 2631 if (getCondition().isEmpty()) { 2632 addCondition(); 2633 } 2634 return getCondition().get(0); 2635 } 2636 2637 /** 2638 * @return {@link #note} (To communicate any details or issues about the 2639 * specimen or during the specimen collection. (for example: broken 2640 * vial, sent with patient, frozen).) 2641 */ 2642 public List<Annotation> getNote() { 2643 if (this.note == null) 2644 this.note = new ArrayList<Annotation>(); 2645 return this.note; 2646 } 2647 2648 /** 2649 * @return Returns a reference to <code>this</code> for easy method chaining 2650 */ 2651 public Specimen setNote(List<Annotation> theNote) { 2652 this.note = theNote; 2653 return this; 2654 } 2655 2656 public boolean hasNote() { 2657 if (this.note == null) 2658 return false; 2659 for (Annotation item : this.note) 2660 if (!item.isEmpty()) 2661 return true; 2662 return false; 2663 } 2664 2665 public Annotation addNote() { // 3 2666 Annotation t = new Annotation(); 2667 if (this.note == null) 2668 this.note = new ArrayList<Annotation>(); 2669 this.note.add(t); 2670 return t; 2671 } 2672 2673 public Specimen addNote(Annotation t) { // 3 2674 if (t == null) 2675 return this; 2676 if (this.note == null) 2677 this.note = new ArrayList<Annotation>(); 2678 this.note.add(t); 2679 return this; 2680 } 2681 2682 /** 2683 * @return The first repetition of repeating field {@link #note}, creating it if 2684 * it does not already exist 2685 */ 2686 public Annotation getNoteFirstRep() { 2687 if (getNote().isEmpty()) { 2688 addNote(); 2689 } 2690 return getNote().get(0); 2691 } 2692 2693 protected void listChildren(List<Property> children) { 2694 super.listChildren(children); 2695 children 2696 .add(new Property("identifier", "Identifier", "Id for specimen.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2697 children.add(new Property("accessionIdentifier", "Identifier", 2698 "The identifier assigned by the lab when accessioning specimen(s). This is not necessarily the same as the specimen identifier, depending on local lab procedures.", 2699 0, 1, accessionIdentifier)); 2700 children.add(new Property("status", "code", "The availability of the specimen.", 0, 1, status)); 2701 children.add(new Property("type", "CodeableConcept", "The kind of material that forms the specimen.", 0, 1, type)); 2702 children.add(new Property("subject", "Reference(Patient|Group|Device|Substance|Location)", 2703 "Where the specimen came from. This may be from patient(s), from a location (e.g., the source of an environmental sample), or a sampling of a substance or a device.", 2704 0, 1, subject)); 2705 children.add(new Property("receivedTime", "dateTime", "Time when specimen was received for processing or testing.", 2706 0, 1, receivedTime)); 2707 children.add(new Property("parent", "Reference(Specimen)", 2708 "Reference to the parent (source) specimen which is used when the specimen was either derived from or a component of another specimen.", 2709 0, java.lang.Integer.MAX_VALUE, parent)); 2710 children.add(new Property("request", "Reference(ServiceRequest)", 2711 "Details concerning a service request that required a specimen to be collected.", 0, 2712 java.lang.Integer.MAX_VALUE, request)); 2713 children.add(new Property("collection", "", "Details concerning the specimen collection.", 0, 1, collection)); 2714 children.add(new Property("processing", "", "Details concerning processing and processing steps for the specimen.", 2715 0, java.lang.Integer.MAX_VALUE, processing)); 2716 children.add(new Property("container", "", 2717 "The container holding the specimen. The recursive nature of containers; i.e. blood in tube in tray in rack is not addressed here.", 2718 0, java.lang.Integer.MAX_VALUE, container)); 2719 children.add(new Property("condition", "CodeableConcept", 2720 "A mode or state of being that describes the nature of the specimen.", 0, java.lang.Integer.MAX_VALUE, 2721 condition)); 2722 children.add(new Property("note", "Annotation", 2723 "To communicate any details or issues about the specimen or during the specimen collection. (for example: broken vial, sent with patient, frozen).", 2724 0, java.lang.Integer.MAX_VALUE, note)); 2725 } 2726 2727 @Override 2728 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2729 switch (_hash) { 2730 case -1618432855: 2731 /* identifier */ return new Property("identifier", "Identifier", "Id for specimen.", 0, 2732 java.lang.Integer.MAX_VALUE, identifier); 2733 case 818734061: 2734 /* accessionIdentifier */ return new Property("accessionIdentifier", "Identifier", 2735 "The identifier assigned by the lab when accessioning specimen(s). This is not necessarily the same as the specimen identifier, depending on local lab procedures.", 2736 0, 1, accessionIdentifier); 2737 case -892481550: 2738 /* status */ return new Property("status", "code", "The availability of the specimen.", 0, 1, status); 2739 case 3575610: 2740 /* type */ return new Property("type", "CodeableConcept", "The kind of material that forms the specimen.", 0, 1, 2741 type); 2742 case -1867885268: 2743 /* subject */ return new Property("subject", "Reference(Patient|Group|Device|Substance|Location)", 2744 "Where the specimen came from. This may be from patient(s), from a location (e.g., the source of an environmental sample), or a sampling of a substance or a device.", 2745 0, 1, subject); 2746 case -767961010: 2747 /* receivedTime */ return new Property("receivedTime", "dateTime", 2748 "Time when specimen was received for processing or testing.", 0, 1, receivedTime); 2749 case -995424086: 2750 /* parent */ return new Property("parent", "Reference(Specimen)", 2751 "Reference to the parent (source) specimen which is used when the specimen was either derived from or a component of another specimen.", 2752 0, java.lang.Integer.MAX_VALUE, parent); 2753 case 1095692943: 2754 /* request */ return new Property("request", "Reference(ServiceRequest)", 2755 "Details concerning a service request that required a specimen to be collected.", 0, 2756 java.lang.Integer.MAX_VALUE, request); 2757 case -1741312354: 2758 /* collection */ return new Property("collection", "", "Details concerning the specimen collection.", 0, 1, 2759 collection); 2760 case 422194963: 2761 /* processing */ return new Property("processing", "", 2762 "Details concerning processing and processing steps for the specimen.", 0, java.lang.Integer.MAX_VALUE, 2763 processing); 2764 case -410956671: 2765 /* container */ return new Property("container", "", 2766 "The container holding the specimen. The recursive nature of containers; i.e. blood in tube in tray in rack is not addressed here.", 2767 0, java.lang.Integer.MAX_VALUE, container); 2768 case -861311717: 2769 /* condition */ return new Property("condition", "CodeableConcept", 2770 "A mode or state of being that describes the nature of the specimen.", 0, java.lang.Integer.MAX_VALUE, 2771 condition); 2772 case 3387378: 2773 /* note */ return new Property("note", "Annotation", 2774 "To communicate any details or issues about the specimen or during the specimen collection. (for example: broken vial, sent with patient, frozen).", 2775 0, java.lang.Integer.MAX_VALUE, note); 2776 default: 2777 return super.getNamedProperty(_hash, _name, _checkValid); 2778 } 2779 2780 } 2781 2782 @Override 2783 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2784 switch (hash) { 2785 case -1618432855: 2786 /* identifier */ return this.identifier == null ? new Base[0] 2787 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2788 case 818734061: 2789 /* accessionIdentifier */ return this.accessionIdentifier == null ? new Base[0] 2790 : new Base[] { this.accessionIdentifier }; // Identifier 2791 case -892481550: 2792 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<SpecimenStatus> 2793 case 3575610: 2794 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2795 case -1867885268: 2796 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 2797 case -767961010: 2798 /* receivedTime */ return this.receivedTime == null ? new Base[0] : new Base[] { this.receivedTime }; // DateTimeType 2799 case -995424086: 2800 /* parent */ return this.parent == null ? new Base[0] : this.parent.toArray(new Base[this.parent.size()]); // Reference 2801 case 1095692943: 2802 /* request */ return this.request == null ? new Base[0] : this.request.toArray(new Base[this.request.size()]); // Reference 2803 case -1741312354: 2804 /* collection */ return this.collection == null ? new Base[0] : new Base[] { this.collection }; // SpecimenCollectionComponent 2805 case 422194963: 2806 /* processing */ return this.processing == null ? new Base[0] 2807 : this.processing.toArray(new Base[this.processing.size()]); // SpecimenProcessingComponent 2808 case -410956671: 2809 /* container */ return this.container == null ? new Base[0] 2810 : this.container.toArray(new Base[this.container.size()]); // SpecimenContainerComponent 2811 case -861311717: 2812 /* condition */ return this.condition == null ? new Base[0] 2813 : this.condition.toArray(new Base[this.condition.size()]); // CodeableConcept 2814 case 3387378: 2815 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2816 default: 2817 return super.getProperty(hash, name, checkValid); 2818 } 2819 2820 } 2821 2822 @Override 2823 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2824 switch (hash) { 2825 case -1618432855: // identifier 2826 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2827 return value; 2828 case 818734061: // accessionIdentifier 2829 this.accessionIdentifier = castToIdentifier(value); // Identifier 2830 return value; 2831 case -892481550: // status 2832 value = new SpecimenStatusEnumFactory().fromType(castToCode(value)); 2833 this.status = (Enumeration) value; // Enumeration<SpecimenStatus> 2834 return value; 2835 case 3575610: // type 2836 this.type = castToCodeableConcept(value); // CodeableConcept 2837 return value; 2838 case -1867885268: // subject 2839 this.subject = castToReference(value); // Reference 2840 return value; 2841 case -767961010: // receivedTime 2842 this.receivedTime = castToDateTime(value); // DateTimeType 2843 return value; 2844 case -995424086: // parent 2845 this.getParent().add(castToReference(value)); // Reference 2846 return value; 2847 case 1095692943: // request 2848 this.getRequest().add(castToReference(value)); // Reference 2849 return value; 2850 case -1741312354: // collection 2851 this.collection = (SpecimenCollectionComponent) value; // SpecimenCollectionComponent 2852 return value; 2853 case 422194963: // processing 2854 this.getProcessing().add((SpecimenProcessingComponent) value); // SpecimenProcessingComponent 2855 return value; 2856 case -410956671: // container 2857 this.getContainer().add((SpecimenContainerComponent) value); // SpecimenContainerComponent 2858 return value; 2859 case -861311717: // condition 2860 this.getCondition().add(castToCodeableConcept(value)); // CodeableConcept 2861 return value; 2862 case 3387378: // note 2863 this.getNote().add(castToAnnotation(value)); // Annotation 2864 return value; 2865 default: 2866 return super.setProperty(hash, name, value); 2867 } 2868 2869 } 2870 2871 @Override 2872 public Base setProperty(String name, Base value) throws FHIRException { 2873 if (name.equals("identifier")) { 2874 this.getIdentifier().add(castToIdentifier(value)); 2875 } else if (name.equals("accessionIdentifier")) { 2876 this.accessionIdentifier = castToIdentifier(value); // Identifier 2877 } else if (name.equals("status")) { 2878 value = new SpecimenStatusEnumFactory().fromType(castToCode(value)); 2879 this.status = (Enumeration) value; // Enumeration<SpecimenStatus> 2880 } else if (name.equals("type")) { 2881 this.type = castToCodeableConcept(value); // CodeableConcept 2882 } else if (name.equals("subject")) { 2883 this.subject = castToReference(value); // Reference 2884 } else if (name.equals("receivedTime")) { 2885 this.receivedTime = castToDateTime(value); // DateTimeType 2886 } else if (name.equals("parent")) { 2887 this.getParent().add(castToReference(value)); 2888 } else if (name.equals("request")) { 2889 this.getRequest().add(castToReference(value)); 2890 } else if (name.equals("collection")) { 2891 this.collection = (SpecimenCollectionComponent) value; // SpecimenCollectionComponent 2892 } else if (name.equals("processing")) { 2893 this.getProcessing().add((SpecimenProcessingComponent) value); 2894 } else if (name.equals("container")) { 2895 this.getContainer().add((SpecimenContainerComponent) value); 2896 } else if (name.equals("condition")) { 2897 this.getCondition().add(castToCodeableConcept(value)); 2898 } else if (name.equals("note")) { 2899 this.getNote().add(castToAnnotation(value)); 2900 } else 2901 return super.setProperty(name, value); 2902 return value; 2903 } 2904 2905 @Override 2906 public Base makeProperty(int hash, String name) throws FHIRException { 2907 switch (hash) { 2908 case -1618432855: 2909 return addIdentifier(); 2910 case 818734061: 2911 return getAccessionIdentifier(); 2912 case -892481550: 2913 return getStatusElement(); 2914 case 3575610: 2915 return getType(); 2916 case -1867885268: 2917 return getSubject(); 2918 case -767961010: 2919 return getReceivedTimeElement(); 2920 case -995424086: 2921 return addParent(); 2922 case 1095692943: 2923 return addRequest(); 2924 case -1741312354: 2925 return getCollection(); 2926 case 422194963: 2927 return addProcessing(); 2928 case -410956671: 2929 return addContainer(); 2930 case -861311717: 2931 return addCondition(); 2932 case 3387378: 2933 return addNote(); 2934 default: 2935 return super.makeProperty(hash, name); 2936 } 2937 2938 } 2939 2940 @Override 2941 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2942 switch (hash) { 2943 case -1618432855: 2944 /* identifier */ return new String[] { "Identifier" }; 2945 case 818734061: 2946 /* accessionIdentifier */ return new String[] { "Identifier" }; 2947 case -892481550: 2948 /* status */ return new String[] { "code" }; 2949 case 3575610: 2950 /* type */ return new String[] { "CodeableConcept" }; 2951 case -1867885268: 2952 /* subject */ return new String[] { "Reference" }; 2953 case -767961010: 2954 /* receivedTime */ return new String[] { "dateTime" }; 2955 case -995424086: 2956 /* parent */ return new String[] { "Reference" }; 2957 case 1095692943: 2958 /* request */ return new String[] { "Reference" }; 2959 case -1741312354: 2960 /* collection */ return new String[] {}; 2961 case 422194963: 2962 /* processing */ return new String[] {}; 2963 case -410956671: 2964 /* container */ return new String[] {}; 2965 case -861311717: 2966 /* condition */ return new String[] { "CodeableConcept" }; 2967 case 3387378: 2968 /* note */ return new String[] { "Annotation" }; 2969 default: 2970 return super.getTypesForProperty(hash, name); 2971 } 2972 2973 } 2974 2975 @Override 2976 public Base addChild(String name) throws FHIRException { 2977 if (name.equals("identifier")) { 2978 return addIdentifier(); 2979 } else if (name.equals("accessionIdentifier")) { 2980 this.accessionIdentifier = new Identifier(); 2981 return this.accessionIdentifier; 2982 } else if (name.equals("status")) { 2983 throw new FHIRException("Cannot call addChild on a singleton property Specimen.status"); 2984 } else if (name.equals("type")) { 2985 this.type = new CodeableConcept(); 2986 return this.type; 2987 } else if (name.equals("subject")) { 2988 this.subject = new Reference(); 2989 return this.subject; 2990 } else if (name.equals("receivedTime")) { 2991 throw new FHIRException("Cannot call addChild on a singleton property Specimen.receivedTime"); 2992 } else if (name.equals("parent")) { 2993 return addParent(); 2994 } else if (name.equals("request")) { 2995 return addRequest(); 2996 } else if (name.equals("collection")) { 2997 this.collection = new SpecimenCollectionComponent(); 2998 return this.collection; 2999 } else if (name.equals("processing")) { 3000 return addProcessing(); 3001 } else if (name.equals("container")) { 3002 return addContainer(); 3003 } else if (name.equals("condition")) { 3004 return addCondition(); 3005 } else if (name.equals("note")) { 3006 return addNote(); 3007 } else 3008 return super.addChild(name); 3009 } 3010 3011 public String fhirType() { 3012 return "Specimen"; 3013 3014 } 3015 3016 public Specimen copy() { 3017 Specimen dst = new Specimen(); 3018 copyValues(dst); 3019 return dst; 3020 } 3021 3022 public void copyValues(Specimen dst) { 3023 super.copyValues(dst); 3024 if (identifier != null) { 3025 dst.identifier = new ArrayList<Identifier>(); 3026 for (Identifier i : identifier) 3027 dst.identifier.add(i.copy()); 3028 } 3029 ; 3030 dst.accessionIdentifier = accessionIdentifier == null ? null : accessionIdentifier.copy(); 3031 dst.status = status == null ? null : status.copy(); 3032 dst.type = type == null ? null : type.copy(); 3033 dst.subject = subject == null ? null : subject.copy(); 3034 dst.receivedTime = receivedTime == null ? null : receivedTime.copy(); 3035 if (parent != null) { 3036 dst.parent = new ArrayList<Reference>(); 3037 for (Reference i : parent) 3038 dst.parent.add(i.copy()); 3039 } 3040 ; 3041 if (request != null) { 3042 dst.request = new ArrayList<Reference>(); 3043 for (Reference i : request) 3044 dst.request.add(i.copy()); 3045 } 3046 ; 3047 dst.collection = collection == null ? null : collection.copy(); 3048 if (processing != null) { 3049 dst.processing = new ArrayList<SpecimenProcessingComponent>(); 3050 for (SpecimenProcessingComponent i : processing) 3051 dst.processing.add(i.copy()); 3052 } 3053 ; 3054 if (container != null) { 3055 dst.container = new ArrayList<SpecimenContainerComponent>(); 3056 for (SpecimenContainerComponent i : container) 3057 dst.container.add(i.copy()); 3058 } 3059 ; 3060 if (condition != null) { 3061 dst.condition = new ArrayList<CodeableConcept>(); 3062 for (CodeableConcept i : condition) 3063 dst.condition.add(i.copy()); 3064 } 3065 ; 3066 if (note != null) { 3067 dst.note = new ArrayList<Annotation>(); 3068 for (Annotation i : note) 3069 dst.note.add(i.copy()); 3070 } 3071 ; 3072 } 3073 3074 protected Specimen typedCopy() { 3075 return copy(); 3076 } 3077 3078 @Override 3079 public boolean equalsDeep(Base other_) { 3080 if (!super.equalsDeep(other_)) 3081 return false; 3082 if (!(other_ instanceof Specimen)) 3083 return false; 3084 Specimen o = (Specimen) other_; 3085 return compareDeep(identifier, o.identifier, true) && compareDeep(accessionIdentifier, o.accessionIdentifier, true) 3086 && compareDeep(status, o.status, true) && compareDeep(type, o.type, true) 3087 && compareDeep(subject, o.subject, true) && compareDeep(receivedTime, o.receivedTime, true) 3088 && compareDeep(parent, o.parent, true) && compareDeep(request, o.request, true) 3089 && compareDeep(collection, o.collection, true) && compareDeep(processing, o.processing, true) 3090 && compareDeep(container, o.container, true) && compareDeep(condition, o.condition, true) 3091 && compareDeep(note, o.note, true); 3092 } 3093 3094 @Override 3095 public boolean equalsShallow(Base other_) { 3096 if (!super.equalsShallow(other_)) 3097 return false; 3098 if (!(other_ instanceof Specimen)) 3099 return false; 3100 Specimen o = (Specimen) other_; 3101 return compareValues(status, o.status, true) && compareValues(receivedTime, o.receivedTime, true); 3102 } 3103 3104 public boolean isEmpty() { 3105 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, accessionIdentifier, status, type, 3106 subject, receivedTime, parent, request, collection, processing, container, condition, note); 3107 } 3108 3109 @Override 3110 public ResourceType getResourceType() { 3111 return ResourceType.Specimen; 3112 } 3113 3114 /** 3115 * Search parameter: <b>container</b> 3116 * <p> 3117 * Description: <b>The kind of specimen container</b><br> 3118 * Type: <b>token</b><br> 3119 * Path: <b>Specimen.container.type</b><br> 3120 * </p> 3121 */ 3122 @SearchParamDefinition(name = "container", path = "Specimen.container.type", description = "The kind of specimen container", type = "token") 3123 public static final String SP_CONTAINER = "container"; 3124 /** 3125 * <b>Fluent Client</b> search parameter constant for <b>container</b> 3126 * <p> 3127 * Description: <b>The kind of specimen container</b><br> 3128 * Type: <b>token</b><br> 3129 * Path: <b>Specimen.container.type</b><br> 3130 * </p> 3131 */ 3132 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTAINER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3133 SP_CONTAINER); 3134 3135 /** 3136 * Search parameter: <b>identifier</b> 3137 * <p> 3138 * Description: <b>The unique identifier associated with the specimen</b><br> 3139 * Type: <b>token</b><br> 3140 * Path: <b>Specimen.identifier</b><br> 3141 * </p> 3142 */ 3143 @SearchParamDefinition(name = "identifier", path = "Specimen.identifier", description = "The unique identifier associated with the specimen", type = "token") 3144 public static final String SP_IDENTIFIER = "identifier"; 3145 /** 3146 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3147 * <p> 3148 * Description: <b>The unique identifier associated with the specimen</b><br> 3149 * Type: <b>token</b><br> 3150 * Path: <b>Specimen.identifier</b><br> 3151 * </p> 3152 */ 3153 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3154 SP_IDENTIFIER); 3155 3156 /** 3157 * Search parameter: <b>parent</b> 3158 * <p> 3159 * Description: <b>The parent of the specimen</b><br> 3160 * Type: <b>reference</b><br> 3161 * Path: <b>Specimen.parent</b><br> 3162 * </p> 3163 */ 3164 @SearchParamDefinition(name = "parent", path = "Specimen.parent", description = "The parent of the specimen", type = "reference", target = { 3165 Specimen.class }) 3166 public static final String SP_PARENT = "parent"; 3167 /** 3168 * <b>Fluent Client</b> search parameter constant for <b>parent</b> 3169 * <p> 3170 * Description: <b>The parent of the specimen</b><br> 3171 * Type: <b>reference</b><br> 3172 * Path: <b>Specimen.parent</b><br> 3173 * </p> 3174 */ 3175 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3176 SP_PARENT); 3177 3178 /** 3179 * Constant for fluent queries to be used to add include statements. Specifies 3180 * the path value of "<b>Specimen:parent</b>". 3181 */ 3182 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARENT = new ca.uhn.fhir.model.api.Include( 3183 "Specimen:parent").toLocked(); 3184 3185 /** 3186 * Search parameter: <b>container-id</b> 3187 * <p> 3188 * Description: <b>The unique identifier associated with the specimen 3189 * container</b><br> 3190 * Type: <b>token</b><br> 3191 * Path: <b>Specimen.container.identifier</b><br> 3192 * </p> 3193 */ 3194 @SearchParamDefinition(name = "container-id", path = "Specimen.container.identifier", description = "The unique identifier associated with the specimen container", type = "token") 3195 public static final String SP_CONTAINER_ID = "container-id"; 3196 /** 3197 * <b>Fluent Client</b> search parameter constant for <b>container-id</b> 3198 * <p> 3199 * Description: <b>The unique identifier associated with the specimen 3200 * container</b><br> 3201 * Type: <b>token</b><br> 3202 * Path: <b>Specimen.container.identifier</b><br> 3203 * </p> 3204 */ 3205 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTAINER_ID = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3206 SP_CONTAINER_ID); 3207 3208 /** 3209 * Search parameter: <b>bodysite</b> 3210 * <p> 3211 * Description: <b>The code for the body site from where the specimen 3212 * originated</b><br> 3213 * Type: <b>token</b><br> 3214 * Path: <b>Specimen.collection.bodySite</b><br> 3215 * </p> 3216 */ 3217 @SearchParamDefinition(name = "bodysite", path = "Specimen.collection.bodySite", description = "The code for the body site from where the specimen originated", type = "token") 3218 public static final String SP_BODYSITE = "bodysite"; 3219 /** 3220 * <b>Fluent Client</b> search parameter constant for <b>bodysite</b> 3221 * <p> 3222 * Description: <b>The code for the body site from where the specimen 3223 * originated</b><br> 3224 * Type: <b>token</b><br> 3225 * Path: <b>Specimen.collection.bodySite</b><br> 3226 * </p> 3227 */ 3228 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BODYSITE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3229 SP_BODYSITE); 3230 3231 /** 3232 * Search parameter: <b>subject</b> 3233 * <p> 3234 * Description: <b>The subject of the specimen</b><br> 3235 * Type: <b>reference</b><br> 3236 * Path: <b>Specimen.subject</b><br> 3237 * </p> 3238 */ 3239 @SearchParamDefinition(name = "subject", path = "Specimen.subject", description = "The subject of the specimen", type = "reference", providesMembershipIn = { 3240 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 3241 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Device.class, Group.class, 3242 Location.class, Patient.class, Substance.class }) 3243 public static final String SP_SUBJECT = "subject"; 3244 /** 3245 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3246 * <p> 3247 * Description: <b>The subject of the specimen</b><br> 3248 * Type: <b>reference</b><br> 3249 * Path: <b>Specimen.subject</b><br> 3250 * </p> 3251 */ 3252 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3253 SP_SUBJECT); 3254 3255 /** 3256 * Constant for fluent queries to be used to add include statements. Specifies 3257 * the path value of "<b>Specimen:subject</b>". 3258 */ 3259 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 3260 "Specimen:subject").toLocked(); 3261 3262 /** 3263 * Search parameter: <b>patient</b> 3264 * <p> 3265 * Description: <b>The patient the specimen comes from</b><br> 3266 * Type: <b>reference</b><br> 3267 * Path: <b>Specimen.subject</b><br> 3268 * </p> 3269 */ 3270 @SearchParamDefinition(name = "patient", path = "Specimen.subject.where(resolve() is Patient)", description = "The patient the specimen comes from", type = "reference", target = { 3271 Patient.class }) 3272 public static final String SP_PATIENT = "patient"; 3273 /** 3274 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3275 * <p> 3276 * Description: <b>The patient the specimen comes from</b><br> 3277 * Type: <b>reference</b><br> 3278 * Path: <b>Specimen.subject</b><br> 3279 * </p> 3280 */ 3281 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3282 SP_PATIENT); 3283 3284 /** 3285 * Constant for fluent queries to be used to add include statements. Specifies 3286 * the path value of "<b>Specimen:patient</b>". 3287 */ 3288 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 3289 "Specimen:patient").toLocked(); 3290 3291 /** 3292 * Search parameter: <b>collected</b> 3293 * <p> 3294 * Description: <b>The date the specimen was collected</b><br> 3295 * Type: <b>date</b><br> 3296 * Path: <b>Specimen.collection.collected[x]</b><br> 3297 * </p> 3298 */ 3299 @SearchParamDefinition(name = "collected", path = "Specimen.collection.collected", description = "The date the specimen was collected", type = "date") 3300 public static final String SP_COLLECTED = "collected"; 3301 /** 3302 * <b>Fluent Client</b> search parameter constant for <b>collected</b> 3303 * <p> 3304 * Description: <b>The date the specimen was collected</b><br> 3305 * Type: <b>date</b><br> 3306 * Path: <b>Specimen.collection.collected[x]</b><br> 3307 * </p> 3308 */ 3309 public static final ca.uhn.fhir.rest.gclient.DateClientParam COLLECTED = new ca.uhn.fhir.rest.gclient.DateClientParam( 3310 SP_COLLECTED); 3311 3312 /** 3313 * Search parameter: <b>accession</b> 3314 * <p> 3315 * Description: <b>The accession number associated with the specimen</b><br> 3316 * Type: <b>token</b><br> 3317 * Path: <b>Specimen.accessionIdentifier</b><br> 3318 * </p> 3319 */ 3320 @SearchParamDefinition(name = "accession", path = "Specimen.accessionIdentifier", description = "The accession number associated with the specimen", type = "token") 3321 public static final String SP_ACCESSION = "accession"; 3322 /** 3323 * <b>Fluent Client</b> search parameter constant for <b>accession</b> 3324 * <p> 3325 * Description: <b>The accession number associated with the specimen</b><br> 3326 * Type: <b>token</b><br> 3327 * Path: <b>Specimen.accessionIdentifier</b><br> 3328 * </p> 3329 */ 3330 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACCESSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3331 SP_ACCESSION); 3332 3333 /** 3334 * Search parameter: <b>type</b> 3335 * <p> 3336 * Description: <b>The specimen type</b><br> 3337 * Type: <b>token</b><br> 3338 * Path: <b>Specimen.type</b><br> 3339 * </p> 3340 */ 3341 @SearchParamDefinition(name = "type", path = "Specimen.type", description = "The specimen type", type = "token") 3342 public static final String SP_TYPE = "type"; 3343 /** 3344 * <b>Fluent Client</b> search parameter constant for <b>type</b> 3345 * <p> 3346 * Description: <b>The specimen type</b><br> 3347 * Type: <b>token</b><br> 3348 * Path: <b>Specimen.type</b><br> 3349 * </p> 3350 */ 3351 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3352 SP_TYPE); 3353 3354 /** 3355 * Search parameter: <b>collector</b> 3356 * <p> 3357 * Description: <b>Who collected the specimen</b><br> 3358 * Type: <b>reference</b><br> 3359 * Path: <b>Specimen.collection.collector</b><br> 3360 * </p> 3361 */ 3362 @SearchParamDefinition(name = "collector", path = "Specimen.collection.collector", description = "Who collected the specimen", type = "reference", providesMembershipIn = { 3363 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Practitioner.class, 3364 PractitionerRole.class }) 3365 public static final String SP_COLLECTOR = "collector"; 3366 /** 3367 * <b>Fluent Client</b> search parameter constant for <b>collector</b> 3368 * <p> 3369 * Description: <b>Who collected the specimen</b><br> 3370 * Type: <b>reference</b><br> 3371 * Path: <b>Specimen.collection.collector</b><br> 3372 * </p> 3373 */ 3374 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COLLECTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3375 SP_COLLECTOR); 3376 3377 /** 3378 * Constant for fluent queries to be used to add include statements. Specifies 3379 * the path value of "<b>Specimen:collector</b>". 3380 */ 3381 public static final ca.uhn.fhir.model.api.Include INCLUDE_COLLECTOR = new ca.uhn.fhir.model.api.Include( 3382 "Specimen:collector").toLocked(); 3383 3384 /** 3385 * Search parameter: <b>status</b> 3386 * <p> 3387 * Description: <b>available | unavailable | unsatisfactory | 3388 * entered-in-error</b><br> 3389 * Type: <b>token</b><br> 3390 * Path: <b>Specimen.status</b><br> 3391 * </p> 3392 */ 3393 @SearchParamDefinition(name = "status", path = "Specimen.status", description = "available | unavailable | unsatisfactory | entered-in-error", type = "token") 3394 public static final String SP_STATUS = "status"; 3395 /** 3396 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3397 * <p> 3398 * Description: <b>available | unavailable | unsatisfactory | 3399 * entered-in-error</b><br> 3400 * Type: <b>token</b><br> 3401 * Path: <b>Specimen.status</b><br> 3402 * </p> 3403 */ 3404 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3405 SP_STATUS); 3406 3407}