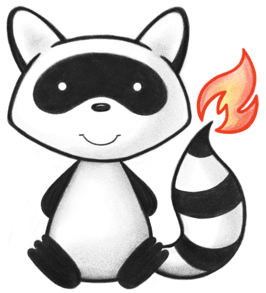
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * A kind of specimen with associated set of requirements. 048 */ 049@ResourceDef(name = "SpecimenDefinition", profile = "http://hl7.org/fhir/StructureDefinition/SpecimenDefinition") 050public class SpecimenDefinition extends DomainResource { 051 052 public enum SpecimenContainedPreference { 053 /** 054 * This type of contained specimen is preferred to collect this kind of 055 * specimen. 056 */ 057 PREFERRED, 058 /** 059 * This type of conditioned specimen is an alternate. 060 */ 061 ALTERNATE, 062 /** 063 * added to help the parsers with the generic types 064 */ 065 NULL; 066 067 public static SpecimenContainedPreference fromCode(String codeString) throws FHIRException { 068 if (codeString == null || "".equals(codeString)) 069 return null; 070 if ("preferred".equals(codeString)) 071 return PREFERRED; 072 if ("alternate".equals(codeString)) 073 return ALTERNATE; 074 if (Configuration.isAcceptInvalidEnums()) 075 return null; 076 else 077 throw new FHIRException("Unknown SpecimenContainedPreference code '" + codeString + "'"); 078 } 079 080 public String toCode() { 081 switch (this) { 082 case PREFERRED: 083 return "preferred"; 084 case ALTERNATE: 085 return "alternate"; 086 case NULL: 087 return null; 088 default: 089 return "?"; 090 } 091 } 092 093 public String getSystem() { 094 switch (this) { 095 case PREFERRED: 096 return "http://hl7.org/fhir/specimen-contained-preference"; 097 case ALTERNATE: 098 return "http://hl7.org/fhir/specimen-contained-preference"; 099 case NULL: 100 return null; 101 default: 102 return "?"; 103 } 104 } 105 106 public String getDefinition() { 107 switch (this) { 108 case PREFERRED: 109 return "This type of contained specimen is preferred to collect this kind of specimen."; 110 case ALTERNATE: 111 return "This type of conditioned specimen is an alternate."; 112 case NULL: 113 return null; 114 default: 115 return "?"; 116 } 117 } 118 119 public String getDisplay() { 120 switch (this) { 121 case PREFERRED: 122 return "Preferred"; 123 case ALTERNATE: 124 return "Alternate"; 125 case NULL: 126 return null; 127 default: 128 return "?"; 129 } 130 } 131 } 132 133 public static class SpecimenContainedPreferenceEnumFactory implements EnumFactory<SpecimenContainedPreference> { 134 public SpecimenContainedPreference fromCode(String codeString) throws IllegalArgumentException { 135 if (codeString == null || "".equals(codeString)) 136 if (codeString == null || "".equals(codeString)) 137 return null; 138 if ("preferred".equals(codeString)) 139 return SpecimenContainedPreference.PREFERRED; 140 if ("alternate".equals(codeString)) 141 return SpecimenContainedPreference.ALTERNATE; 142 throw new IllegalArgumentException("Unknown SpecimenContainedPreference code '" + codeString + "'"); 143 } 144 145 public Enumeration<SpecimenContainedPreference> fromType(PrimitiveType<?> code) throws FHIRException { 146 if (code == null) 147 return null; 148 if (code.isEmpty()) 149 return new Enumeration<SpecimenContainedPreference>(this, SpecimenContainedPreference.NULL, code); 150 String codeString = code.asStringValue(); 151 if (codeString == null || "".equals(codeString)) 152 return new Enumeration<SpecimenContainedPreference>(this, SpecimenContainedPreference.NULL, code); 153 if ("preferred".equals(codeString)) 154 return new Enumeration<SpecimenContainedPreference>(this, SpecimenContainedPreference.PREFERRED, code); 155 if ("alternate".equals(codeString)) 156 return new Enumeration<SpecimenContainedPreference>(this, SpecimenContainedPreference.ALTERNATE, code); 157 throw new FHIRException("Unknown SpecimenContainedPreference code '" + codeString + "'"); 158 } 159 160 public String toCode(SpecimenContainedPreference code) { 161 if (code == SpecimenContainedPreference.NULL) 162 return null; 163 if (code == SpecimenContainedPreference.PREFERRED) 164 return "preferred"; 165 if (code == SpecimenContainedPreference.ALTERNATE) 166 return "alternate"; 167 return "?"; 168 } 169 170 public String toSystem(SpecimenContainedPreference code) { 171 return code.getSystem(); 172 } 173 } 174 175 @Block() 176 public static class SpecimenDefinitionTypeTestedComponent extends BackboneElement implements IBaseBackboneElement { 177 /** 178 * Primary of secondary specimen. 179 */ 180 @Child(name = "isDerived", type = { 181 BooleanType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 182 @Description(shortDefinition = "Primary or secondary specimen", formalDefinition = "Primary of secondary specimen.") 183 protected BooleanType isDerived; 184 185 /** 186 * The kind of specimen conditioned for testing expected by lab. 187 */ 188 @Child(name = "type", type = { 189 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 190 @Description(shortDefinition = "Type of intended specimen", formalDefinition = "The kind of specimen conditioned for testing expected by lab.") 191 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v2-0487") 192 protected CodeableConcept type; 193 194 /** 195 * The preference for this type of conditioned specimen. 196 */ 197 @Child(name = "preference", type = { 198 CodeType.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 199 @Description(shortDefinition = "preferred | alternate", formalDefinition = "The preference for this type of conditioned specimen.") 200 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/specimen-contained-preference") 201 protected Enumeration<SpecimenContainedPreference> preference; 202 203 /** 204 * The specimen's container. 205 */ 206 @Child(name = "container", type = {}, order = 4, min = 0, max = 1, modifier = false, summary = false) 207 @Description(shortDefinition = "The specimen's container", formalDefinition = "The specimen's container.") 208 protected SpecimenDefinitionTypeTestedContainerComponent container; 209 210 /** 211 * Requirements for delivery and special handling of this kind of conditioned 212 * specimen. 213 */ 214 @Child(name = "requirement", type = { 215 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 216 @Description(shortDefinition = "Specimen requirements", formalDefinition = "Requirements for delivery and special handling of this kind of conditioned specimen.") 217 protected StringType requirement; 218 219 /** 220 * The usual time that a specimen of this kind is retained after the ordered 221 * tests are completed, for the purpose of additional testing. 222 */ 223 @Child(name = "retentionTime", type = { 224 Duration.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 225 @Description(shortDefinition = "Specimen retention time", formalDefinition = "The usual time that a specimen of this kind is retained after the ordered tests are completed, for the purpose of additional testing.") 226 protected Duration retentionTime; 227 228 /** 229 * Criterion for rejection of the specimen in its container by the laboratory. 230 */ 231 @Child(name = "rejectionCriterion", type = { 232 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 233 @Description(shortDefinition = "Rejection criterion", formalDefinition = "Criterion for rejection of the specimen in its container by the laboratory.") 234 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/rejection-criteria") 235 protected List<CodeableConcept> rejectionCriterion; 236 237 /** 238 * Set of instructions for preservation/transport of the specimen at a defined 239 * temperature interval, prior the testing process. 240 */ 241 @Child(name = "handling", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 242 @Description(shortDefinition = "Specimen handling before testing", formalDefinition = "Set of instructions for preservation/transport of the specimen at a defined temperature interval, prior the testing process.") 243 protected List<SpecimenDefinitionTypeTestedHandlingComponent> handling; 244 245 private static final long serialVersionUID = 308313920L; 246 247 /** 248 * Constructor 249 */ 250 public SpecimenDefinitionTypeTestedComponent() { 251 super(); 252 } 253 254 /** 255 * Constructor 256 */ 257 public SpecimenDefinitionTypeTestedComponent(Enumeration<SpecimenContainedPreference> preference) { 258 super(); 259 this.preference = preference; 260 } 261 262 /** 263 * @return {@link #isDerived} (Primary of secondary specimen.). This is the 264 * underlying object with id, value and extensions. The accessor 265 * "getIsDerived" gives direct access to the value 266 */ 267 public BooleanType getIsDerivedElement() { 268 if (this.isDerived == null) 269 if (Configuration.errorOnAutoCreate()) 270 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedComponent.isDerived"); 271 else if (Configuration.doAutoCreate()) 272 this.isDerived = new BooleanType(); // bb 273 return this.isDerived; 274 } 275 276 public boolean hasIsDerivedElement() { 277 return this.isDerived != null && !this.isDerived.isEmpty(); 278 } 279 280 public boolean hasIsDerived() { 281 return this.isDerived != null && !this.isDerived.isEmpty(); 282 } 283 284 /** 285 * @param value {@link #isDerived} (Primary of secondary specimen.). This is the 286 * underlying object with id, value and extensions. The accessor 287 * "getIsDerived" gives direct access to the value 288 */ 289 public SpecimenDefinitionTypeTestedComponent setIsDerivedElement(BooleanType value) { 290 this.isDerived = value; 291 return this; 292 } 293 294 /** 295 * @return Primary of secondary specimen. 296 */ 297 public boolean getIsDerived() { 298 return this.isDerived == null || this.isDerived.isEmpty() ? false : this.isDerived.getValue(); 299 } 300 301 /** 302 * @param value Primary of secondary specimen. 303 */ 304 public SpecimenDefinitionTypeTestedComponent setIsDerived(boolean value) { 305 if (this.isDerived == null) 306 this.isDerived = new BooleanType(); 307 this.isDerived.setValue(value); 308 return this; 309 } 310 311 /** 312 * @return {@link #type} (The kind of specimen conditioned for testing expected 313 * by lab.) 314 */ 315 public CodeableConcept getType() { 316 if (this.type == null) 317 if (Configuration.errorOnAutoCreate()) 318 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedComponent.type"); 319 else if (Configuration.doAutoCreate()) 320 this.type = new CodeableConcept(); // cc 321 return this.type; 322 } 323 324 public boolean hasType() { 325 return this.type != null && !this.type.isEmpty(); 326 } 327 328 /** 329 * @param value {@link #type} (The kind of specimen conditioned for testing 330 * expected by lab.) 331 */ 332 public SpecimenDefinitionTypeTestedComponent setType(CodeableConcept value) { 333 this.type = value; 334 return this; 335 } 336 337 /** 338 * @return {@link #preference} (The preference for this type of conditioned 339 * specimen.). This is the underlying object with id, value and 340 * extensions. The accessor "getPreference" gives direct access to the 341 * value 342 */ 343 public Enumeration<SpecimenContainedPreference> getPreferenceElement() { 344 if (this.preference == null) 345 if (Configuration.errorOnAutoCreate()) 346 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedComponent.preference"); 347 else if (Configuration.doAutoCreate()) 348 this.preference = new Enumeration<SpecimenContainedPreference>(new SpecimenContainedPreferenceEnumFactory()); // bb 349 return this.preference; 350 } 351 352 public boolean hasPreferenceElement() { 353 return this.preference != null && !this.preference.isEmpty(); 354 } 355 356 public boolean hasPreference() { 357 return this.preference != null && !this.preference.isEmpty(); 358 } 359 360 /** 361 * @param value {@link #preference} (The preference for this type of conditioned 362 * specimen.). This is the underlying object with id, value and 363 * extensions. The accessor "getPreference" gives direct access to 364 * the value 365 */ 366 public SpecimenDefinitionTypeTestedComponent setPreferenceElement(Enumeration<SpecimenContainedPreference> value) { 367 this.preference = value; 368 return this; 369 } 370 371 /** 372 * @return The preference for this type of conditioned specimen. 373 */ 374 public SpecimenContainedPreference getPreference() { 375 return this.preference == null ? null : this.preference.getValue(); 376 } 377 378 /** 379 * @param value The preference for this type of conditioned specimen. 380 */ 381 public SpecimenDefinitionTypeTestedComponent setPreference(SpecimenContainedPreference value) { 382 if (this.preference == null) 383 this.preference = new Enumeration<SpecimenContainedPreference>(new SpecimenContainedPreferenceEnumFactory()); 384 this.preference.setValue(value); 385 return this; 386 } 387 388 /** 389 * @return {@link #container} (The specimen's container.) 390 */ 391 public SpecimenDefinitionTypeTestedContainerComponent getContainer() { 392 if (this.container == null) 393 if (Configuration.errorOnAutoCreate()) 394 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedComponent.container"); 395 else if (Configuration.doAutoCreate()) 396 this.container = new SpecimenDefinitionTypeTestedContainerComponent(); // cc 397 return this.container; 398 } 399 400 public boolean hasContainer() { 401 return this.container != null && !this.container.isEmpty(); 402 } 403 404 /** 405 * @param value {@link #container} (The specimen's container.) 406 */ 407 public SpecimenDefinitionTypeTestedComponent setContainer(SpecimenDefinitionTypeTestedContainerComponent value) { 408 this.container = value; 409 return this; 410 } 411 412 /** 413 * @return {@link #requirement} (Requirements for delivery and special handling 414 * of this kind of conditioned specimen.). This is the underlying object 415 * with id, value and extensions. The accessor "getRequirement" gives 416 * direct access to the value 417 */ 418 public StringType getRequirementElement() { 419 if (this.requirement == null) 420 if (Configuration.errorOnAutoCreate()) 421 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedComponent.requirement"); 422 else if (Configuration.doAutoCreate()) 423 this.requirement = new StringType(); // bb 424 return this.requirement; 425 } 426 427 public boolean hasRequirementElement() { 428 return this.requirement != null && !this.requirement.isEmpty(); 429 } 430 431 public boolean hasRequirement() { 432 return this.requirement != null && !this.requirement.isEmpty(); 433 } 434 435 /** 436 * @param value {@link #requirement} (Requirements for delivery and special 437 * handling of this kind of conditioned specimen.). This is the 438 * underlying object with id, value and extensions. The accessor 439 * "getRequirement" gives direct access to the value 440 */ 441 public SpecimenDefinitionTypeTestedComponent setRequirementElement(StringType value) { 442 this.requirement = value; 443 return this; 444 } 445 446 /** 447 * @return Requirements for delivery and special handling of this kind of 448 * conditioned specimen. 449 */ 450 public String getRequirement() { 451 return this.requirement == null ? null : this.requirement.getValue(); 452 } 453 454 /** 455 * @param value Requirements for delivery and special handling of this kind of 456 * conditioned specimen. 457 */ 458 public SpecimenDefinitionTypeTestedComponent setRequirement(String value) { 459 if (Utilities.noString(value)) 460 this.requirement = null; 461 else { 462 if (this.requirement == null) 463 this.requirement = new StringType(); 464 this.requirement.setValue(value); 465 } 466 return this; 467 } 468 469 /** 470 * @return {@link #retentionTime} (The usual time that a specimen of this kind 471 * is retained after the ordered tests are completed, for the purpose of 472 * additional testing.) 473 */ 474 public Duration getRetentionTime() { 475 if (this.retentionTime == null) 476 if (Configuration.errorOnAutoCreate()) 477 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedComponent.retentionTime"); 478 else if (Configuration.doAutoCreate()) 479 this.retentionTime = new Duration(); // cc 480 return this.retentionTime; 481 } 482 483 public boolean hasRetentionTime() { 484 return this.retentionTime != null && !this.retentionTime.isEmpty(); 485 } 486 487 /** 488 * @param value {@link #retentionTime} (The usual time that a specimen of this 489 * kind is retained after the ordered tests are completed, for the 490 * purpose of additional testing.) 491 */ 492 public SpecimenDefinitionTypeTestedComponent setRetentionTime(Duration value) { 493 this.retentionTime = value; 494 return this; 495 } 496 497 /** 498 * @return {@link #rejectionCriterion} (Criterion for rejection of the specimen 499 * in its container by the laboratory.) 500 */ 501 public List<CodeableConcept> getRejectionCriterion() { 502 if (this.rejectionCriterion == null) 503 this.rejectionCriterion = new ArrayList<CodeableConcept>(); 504 return this.rejectionCriterion; 505 } 506 507 /** 508 * @return Returns a reference to <code>this</code> for easy method chaining 509 */ 510 public SpecimenDefinitionTypeTestedComponent setRejectionCriterion(List<CodeableConcept> theRejectionCriterion) { 511 this.rejectionCriterion = theRejectionCriterion; 512 return this; 513 } 514 515 public boolean hasRejectionCriterion() { 516 if (this.rejectionCriterion == null) 517 return false; 518 for (CodeableConcept item : this.rejectionCriterion) 519 if (!item.isEmpty()) 520 return true; 521 return false; 522 } 523 524 public CodeableConcept addRejectionCriterion() { // 3 525 CodeableConcept t = new CodeableConcept(); 526 if (this.rejectionCriterion == null) 527 this.rejectionCriterion = new ArrayList<CodeableConcept>(); 528 this.rejectionCriterion.add(t); 529 return t; 530 } 531 532 public SpecimenDefinitionTypeTestedComponent addRejectionCriterion(CodeableConcept t) { // 3 533 if (t == null) 534 return this; 535 if (this.rejectionCriterion == null) 536 this.rejectionCriterion = new ArrayList<CodeableConcept>(); 537 this.rejectionCriterion.add(t); 538 return this; 539 } 540 541 /** 542 * @return The first repetition of repeating field {@link #rejectionCriterion}, 543 * creating it if it does not already exist 544 */ 545 public CodeableConcept getRejectionCriterionFirstRep() { 546 if (getRejectionCriterion().isEmpty()) { 547 addRejectionCriterion(); 548 } 549 return getRejectionCriterion().get(0); 550 } 551 552 /** 553 * @return {@link #handling} (Set of instructions for preservation/transport of 554 * the specimen at a defined temperature interval, prior the testing 555 * process.) 556 */ 557 public List<SpecimenDefinitionTypeTestedHandlingComponent> getHandling() { 558 if (this.handling == null) 559 this.handling = new ArrayList<SpecimenDefinitionTypeTestedHandlingComponent>(); 560 return this.handling; 561 } 562 563 /** 564 * @return Returns a reference to <code>this</code> for easy method chaining 565 */ 566 public SpecimenDefinitionTypeTestedComponent setHandling( 567 List<SpecimenDefinitionTypeTestedHandlingComponent> theHandling) { 568 this.handling = theHandling; 569 return this; 570 } 571 572 public boolean hasHandling() { 573 if (this.handling == null) 574 return false; 575 for (SpecimenDefinitionTypeTestedHandlingComponent item : this.handling) 576 if (!item.isEmpty()) 577 return true; 578 return false; 579 } 580 581 public SpecimenDefinitionTypeTestedHandlingComponent addHandling() { // 3 582 SpecimenDefinitionTypeTestedHandlingComponent t = new SpecimenDefinitionTypeTestedHandlingComponent(); 583 if (this.handling == null) 584 this.handling = new ArrayList<SpecimenDefinitionTypeTestedHandlingComponent>(); 585 this.handling.add(t); 586 return t; 587 } 588 589 public SpecimenDefinitionTypeTestedComponent addHandling(SpecimenDefinitionTypeTestedHandlingComponent t) { // 3 590 if (t == null) 591 return this; 592 if (this.handling == null) 593 this.handling = new ArrayList<SpecimenDefinitionTypeTestedHandlingComponent>(); 594 this.handling.add(t); 595 return this; 596 } 597 598 /** 599 * @return The first repetition of repeating field {@link #handling}, creating 600 * it if it does not already exist 601 */ 602 public SpecimenDefinitionTypeTestedHandlingComponent getHandlingFirstRep() { 603 if (getHandling().isEmpty()) { 604 addHandling(); 605 } 606 return getHandling().get(0); 607 } 608 609 protected void listChildren(List<Property> children) { 610 super.listChildren(children); 611 children.add(new Property("isDerived", "boolean", "Primary of secondary specimen.", 0, 1, isDerived)); 612 children.add(new Property("type", "CodeableConcept", 613 "The kind of specimen conditioned for testing expected by lab.", 0, 1, type)); 614 children.add(new Property("preference", "code", "The preference for this type of conditioned specimen.", 0, 1, 615 preference)); 616 children.add(new Property("container", "", "The specimen's container.", 0, 1, container)); 617 children.add(new Property("requirement", "string", 618 "Requirements for delivery and special handling of this kind of conditioned specimen.", 0, 1, requirement)); 619 children.add(new Property("retentionTime", "Duration", 620 "The usual time that a specimen of this kind is retained after the ordered tests are completed, for the purpose of additional testing.", 621 0, 1, retentionTime)); 622 children.add(new Property("rejectionCriterion", "CodeableConcept", 623 "Criterion for rejection of the specimen in its container by the laboratory.", 0, java.lang.Integer.MAX_VALUE, 624 rejectionCriterion)); 625 children.add(new Property("handling", "", 626 "Set of instructions for preservation/transport of the specimen at a defined temperature interval, prior the testing process.", 627 0, java.lang.Integer.MAX_VALUE, handling)); 628 } 629 630 @Override 631 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 632 switch (_hash) { 633 case 976346515: 634 /* isDerived */ return new Property("isDerived", "boolean", "Primary of secondary specimen.", 0, 1, isDerived); 635 case 3575610: 636 /* type */ return new Property("type", "CodeableConcept", 637 "The kind of specimen conditioned for testing expected by lab.", 0, 1, type); 638 case -1459831589: 639 /* preference */ return new Property("preference", "code", 640 "The preference for this type of conditioned specimen.", 0, 1, preference); 641 case -410956671: 642 /* container */ return new Property("container", "", "The specimen's container.", 0, 1, container); 643 case 363387971: 644 /* requirement */ return new Property("requirement", "string", 645 "Requirements for delivery and special handling of this kind of conditioned specimen.", 0, 1, requirement); 646 case 1434969867: 647 /* retentionTime */ return new Property("retentionTime", "Duration", 648 "The usual time that a specimen of this kind is retained after the ordered tests are completed, for the purpose of additional testing.", 649 0, 1, retentionTime); 650 case -553706344: 651 /* rejectionCriterion */ return new Property("rejectionCriterion", "CodeableConcept", 652 "Criterion for rejection of the specimen in its container by the laboratory.", 0, 653 java.lang.Integer.MAX_VALUE, rejectionCriterion); 654 case 2072805: 655 /* handling */ return new Property("handling", "", 656 "Set of instructions for preservation/transport of the specimen at a defined temperature interval, prior the testing process.", 657 0, java.lang.Integer.MAX_VALUE, handling); 658 default: 659 return super.getNamedProperty(_hash, _name, _checkValid); 660 } 661 662 } 663 664 @Override 665 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 666 switch (hash) { 667 case 976346515: 668 /* isDerived */ return this.isDerived == null ? new Base[0] : new Base[] { this.isDerived }; // BooleanType 669 case 3575610: 670 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 671 case -1459831589: 672 /* preference */ return this.preference == null ? new Base[0] : new Base[] { this.preference }; // Enumeration<SpecimenContainedPreference> 673 case -410956671: 674 /* container */ return this.container == null ? new Base[0] : new Base[] { this.container }; // SpecimenDefinitionTypeTestedContainerComponent 675 case 363387971: 676 /* requirement */ return this.requirement == null ? new Base[0] : new Base[] { this.requirement }; // StringType 677 case 1434969867: 678 /* retentionTime */ return this.retentionTime == null ? new Base[0] : new Base[] { this.retentionTime }; // Duration 679 case -553706344: 680 /* rejectionCriterion */ return this.rejectionCriterion == null ? new Base[0] 681 : this.rejectionCriterion.toArray(new Base[this.rejectionCriterion.size()]); // CodeableConcept 682 case 2072805: 683 /* handling */ return this.handling == null ? new Base[0] 684 : this.handling.toArray(new Base[this.handling.size()]); // SpecimenDefinitionTypeTestedHandlingComponent 685 default: 686 return super.getProperty(hash, name, checkValid); 687 } 688 689 } 690 691 @Override 692 public Base setProperty(int hash, String name, Base value) throws FHIRException { 693 switch (hash) { 694 case 976346515: // isDerived 695 this.isDerived = castToBoolean(value); // BooleanType 696 return value; 697 case 3575610: // type 698 this.type = castToCodeableConcept(value); // CodeableConcept 699 return value; 700 case -1459831589: // preference 701 value = new SpecimenContainedPreferenceEnumFactory().fromType(castToCode(value)); 702 this.preference = (Enumeration) value; // Enumeration<SpecimenContainedPreference> 703 return value; 704 case -410956671: // container 705 this.container = (SpecimenDefinitionTypeTestedContainerComponent) value; // SpecimenDefinitionTypeTestedContainerComponent 706 return value; 707 case 363387971: // requirement 708 this.requirement = castToString(value); // StringType 709 return value; 710 case 1434969867: // retentionTime 711 this.retentionTime = castToDuration(value); // Duration 712 return value; 713 case -553706344: // rejectionCriterion 714 this.getRejectionCriterion().add(castToCodeableConcept(value)); // CodeableConcept 715 return value; 716 case 2072805: // handling 717 this.getHandling().add((SpecimenDefinitionTypeTestedHandlingComponent) value); // SpecimenDefinitionTypeTestedHandlingComponent 718 return value; 719 default: 720 return super.setProperty(hash, name, value); 721 } 722 723 } 724 725 @Override 726 public Base setProperty(String name, Base value) throws FHIRException { 727 if (name.equals("isDerived")) { 728 this.isDerived = castToBoolean(value); // BooleanType 729 } else if (name.equals("type")) { 730 this.type = castToCodeableConcept(value); // CodeableConcept 731 } else if (name.equals("preference")) { 732 value = new SpecimenContainedPreferenceEnumFactory().fromType(castToCode(value)); 733 this.preference = (Enumeration) value; // Enumeration<SpecimenContainedPreference> 734 } else if (name.equals("container")) { 735 this.container = (SpecimenDefinitionTypeTestedContainerComponent) value; // SpecimenDefinitionTypeTestedContainerComponent 736 } else if (name.equals("requirement")) { 737 this.requirement = castToString(value); // StringType 738 } else if (name.equals("retentionTime")) { 739 this.retentionTime = castToDuration(value); // Duration 740 } else if (name.equals("rejectionCriterion")) { 741 this.getRejectionCriterion().add(castToCodeableConcept(value)); 742 } else if (name.equals("handling")) { 743 this.getHandling().add((SpecimenDefinitionTypeTestedHandlingComponent) value); 744 } else 745 return super.setProperty(name, value); 746 return value; 747 } 748 749 @Override 750 public void removeChild(String name, Base value) throws FHIRException { 751 if (name.equals("isDerived")) { 752 this.isDerived = null; 753 } else if (name.equals("type")) { 754 this.type = null; 755 } else if (name.equals("preference")) { 756 this.preference = null; 757 } else if (name.equals("container")) { 758 this.container = (SpecimenDefinitionTypeTestedContainerComponent) value; // SpecimenDefinitionTypeTestedContainerComponent 759 } else if (name.equals("requirement")) { 760 this.requirement = null; 761 } else if (name.equals("retentionTime")) { 762 this.retentionTime = null; 763 } else if (name.equals("rejectionCriterion")) { 764 this.getRejectionCriterion().remove(castToCodeableConcept(value)); 765 } else if (name.equals("handling")) { 766 this.getHandling().remove((SpecimenDefinitionTypeTestedHandlingComponent) value); 767 } else 768 super.removeChild(name, value); 769 770 } 771 772 @Override 773 public Base makeProperty(int hash, String name) throws FHIRException { 774 switch (hash) { 775 case 976346515: 776 return getIsDerivedElement(); 777 case 3575610: 778 return getType(); 779 case -1459831589: 780 return getPreferenceElement(); 781 case -410956671: 782 return getContainer(); 783 case 363387971: 784 return getRequirementElement(); 785 case 1434969867: 786 return getRetentionTime(); 787 case -553706344: 788 return addRejectionCriterion(); 789 case 2072805: 790 return addHandling(); 791 default: 792 return super.makeProperty(hash, name); 793 } 794 795 } 796 797 @Override 798 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 799 switch (hash) { 800 case 976346515: 801 /* isDerived */ return new String[] { "boolean" }; 802 case 3575610: 803 /* type */ return new String[] { "CodeableConcept" }; 804 case -1459831589: 805 /* preference */ return new String[] { "code" }; 806 case -410956671: 807 /* container */ return new String[] {}; 808 case 363387971: 809 /* requirement */ return new String[] { "string" }; 810 case 1434969867: 811 /* retentionTime */ return new String[] { "Duration" }; 812 case -553706344: 813 /* rejectionCriterion */ return new String[] { "CodeableConcept" }; 814 case 2072805: 815 /* handling */ return new String[] {}; 816 default: 817 return super.getTypesForProperty(hash, name); 818 } 819 820 } 821 822 @Override 823 public Base addChild(String name) throws FHIRException { 824 if (name.equals("isDerived")) { 825 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.isDerived"); 826 } else if (name.equals("type")) { 827 this.type = new CodeableConcept(); 828 return this.type; 829 } else if (name.equals("preference")) { 830 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.preference"); 831 } else if (name.equals("container")) { 832 this.container = new SpecimenDefinitionTypeTestedContainerComponent(); 833 return this.container; 834 } else if (name.equals("requirement")) { 835 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.requirement"); 836 } else if (name.equals("retentionTime")) { 837 this.retentionTime = new Duration(); 838 return this.retentionTime; 839 } else if (name.equals("rejectionCriterion")) { 840 return addRejectionCriterion(); 841 } else if (name.equals("handling")) { 842 return addHandling(); 843 } else 844 return super.addChild(name); 845 } 846 847 public SpecimenDefinitionTypeTestedComponent copy() { 848 SpecimenDefinitionTypeTestedComponent dst = new SpecimenDefinitionTypeTestedComponent(); 849 copyValues(dst); 850 return dst; 851 } 852 853 public void copyValues(SpecimenDefinitionTypeTestedComponent dst) { 854 super.copyValues(dst); 855 dst.isDerived = isDerived == null ? null : isDerived.copy(); 856 dst.type = type == null ? null : type.copy(); 857 dst.preference = preference == null ? null : preference.copy(); 858 dst.container = container == null ? null : container.copy(); 859 dst.requirement = requirement == null ? null : requirement.copy(); 860 dst.retentionTime = retentionTime == null ? null : retentionTime.copy(); 861 if (rejectionCriterion != null) { 862 dst.rejectionCriterion = new ArrayList<CodeableConcept>(); 863 for (CodeableConcept i : rejectionCriterion) 864 dst.rejectionCriterion.add(i.copy()); 865 } 866 ; 867 if (handling != null) { 868 dst.handling = new ArrayList<SpecimenDefinitionTypeTestedHandlingComponent>(); 869 for (SpecimenDefinitionTypeTestedHandlingComponent i : handling) 870 dst.handling.add(i.copy()); 871 } 872 ; 873 } 874 875 @Override 876 public boolean equalsDeep(Base other_) { 877 if (!super.equalsDeep(other_)) 878 return false; 879 if (!(other_ instanceof SpecimenDefinitionTypeTestedComponent)) 880 return false; 881 SpecimenDefinitionTypeTestedComponent o = (SpecimenDefinitionTypeTestedComponent) other_; 882 return compareDeep(isDerived, o.isDerived, true) && compareDeep(type, o.type, true) 883 && compareDeep(preference, o.preference, true) && compareDeep(container, o.container, true) 884 && compareDeep(requirement, o.requirement, true) && compareDeep(retentionTime, o.retentionTime, true) 885 && compareDeep(rejectionCriterion, o.rejectionCriterion, true) && compareDeep(handling, o.handling, true); 886 } 887 888 @Override 889 public boolean equalsShallow(Base other_) { 890 if (!super.equalsShallow(other_)) 891 return false; 892 if (!(other_ instanceof SpecimenDefinitionTypeTestedComponent)) 893 return false; 894 SpecimenDefinitionTypeTestedComponent o = (SpecimenDefinitionTypeTestedComponent) other_; 895 return compareValues(isDerived, o.isDerived, true) && compareValues(preference, o.preference, true) 896 && compareValues(requirement, o.requirement, true); 897 } 898 899 public boolean isEmpty() { 900 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(isDerived, type, preference, container, 901 requirement, retentionTime, rejectionCriterion, handling); 902 } 903 904 public String fhirType() { 905 return "SpecimenDefinition.typeTested"; 906 907 } 908 909 } 910 911 @Block() 912 public static class SpecimenDefinitionTypeTestedContainerComponent extends BackboneElement 913 implements IBaseBackboneElement { 914 /** 915 * The type of material of the container. 916 */ 917 @Child(name = "material", type = { 918 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 919 @Description(shortDefinition = "Container material", formalDefinition = "The type of material of the container.") 920 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/container-material") 921 protected CodeableConcept material; 922 923 /** 924 * The type of container used to contain this kind of specimen. 925 */ 926 @Child(name = "type", type = { 927 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 928 @Description(shortDefinition = "Kind of container associated with the kind of specimen", formalDefinition = "The type of container used to contain this kind of specimen.") 929 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/specimen-container-type") 930 protected CodeableConcept type; 931 932 /** 933 * Color of container cap. 934 */ 935 @Child(name = "cap", type = { 936 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 937 @Description(shortDefinition = "Color of container cap", formalDefinition = "Color of container cap.") 938 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/container-cap") 939 protected CodeableConcept cap; 940 941 /** 942 * The textual description of the kind of container. 943 */ 944 @Child(name = "description", type = { 945 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 946 @Description(shortDefinition = "Container description", formalDefinition = "The textual description of the kind of container.") 947 protected StringType description; 948 949 /** 950 * The capacity (volume or other measure) of this kind of container. 951 */ 952 @Child(name = "capacity", type = { Quantity.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 953 @Description(shortDefinition = "Container capacity", formalDefinition = "The capacity (volume or other measure) of this kind of container.") 954 protected Quantity capacity; 955 956 /** 957 * The minimum volume to be conditioned in the container. 958 */ 959 @Child(name = "minimumVolume", type = { Quantity.class, 960 StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 961 @Description(shortDefinition = "Minimum volume", formalDefinition = "The minimum volume to be conditioned in the container.") 962 protected Type minimumVolume; 963 964 /** 965 * Substance introduced in the kind of container to preserve, maintain or 966 * enhance the specimen. Examples: Formalin, Citrate, EDTA. 967 */ 968 @Child(name = "additive", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 969 @Description(shortDefinition = "Additive associated with container", formalDefinition = "Substance introduced in the kind of container to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.") 970 protected List<SpecimenDefinitionTypeTestedContainerAdditiveComponent> additive; 971 972 /** 973 * Special processing that should be applied to the container for this kind of 974 * specimen. 975 */ 976 @Child(name = "preparation", type = { 977 StringType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 978 @Description(shortDefinition = "Specimen container preparation", formalDefinition = "Special processing that should be applied to the container for this kind of specimen.") 979 protected StringType preparation; 980 981 private static final long serialVersionUID = 175789710L; 982 983 /** 984 * Constructor 985 */ 986 public SpecimenDefinitionTypeTestedContainerComponent() { 987 super(); 988 } 989 990 /** 991 * @return {@link #material} (The type of material of the container.) 992 */ 993 public CodeableConcept getMaterial() { 994 if (this.material == null) 995 if (Configuration.errorOnAutoCreate()) 996 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedContainerComponent.material"); 997 else if (Configuration.doAutoCreate()) 998 this.material = new CodeableConcept(); // cc 999 return this.material; 1000 } 1001 1002 public boolean hasMaterial() { 1003 return this.material != null && !this.material.isEmpty(); 1004 } 1005 1006 /** 1007 * @param value {@link #material} (The type of material of the container.) 1008 */ 1009 public SpecimenDefinitionTypeTestedContainerComponent setMaterial(CodeableConcept value) { 1010 this.material = value; 1011 return this; 1012 } 1013 1014 /** 1015 * @return {@link #type} (The type of container used to contain this kind of 1016 * specimen.) 1017 */ 1018 public CodeableConcept getType() { 1019 if (this.type == null) 1020 if (Configuration.errorOnAutoCreate()) 1021 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedContainerComponent.type"); 1022 else if (Configuration.doAutoCreate()) 1023 this.type = new CodeableConcept(); // cc 1024 return this.type; 1025 } 1026 1027 public boolean hasType() { 1028 return this.type != null && !this.type.isEmpty(); 1029 } 1030 1031 /** 1032 * @param value {@link #type} (The type of container used to contain this kind 1033 * of specimen.) 1034 */ 1035 public SpecimenDefinitionTypeTestedContainerComponent setType(CodeableConcept value) { 1036 this.type = value; 1037 return this; 1038 } 1039 1040 /** 1041 * @return {@link #cap} (Color of container cap.) 1042 */ 1043 public CodeableConcept getCap() { 1044 if (this.cap == null) 1045 if (Configuration.errorOnAutoCreate()) 1046 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedContainerComponent.cap"); 1047 else if (Configuration.doAutoCreate()) 1048 this.cap = new CodeableConcept(); // cc 1049 return this.cap; 1050 } 1051 1052 public boolean hasCap() { 1053 return this.cap != null && !this.cap.isEmpty(); 1054 } 1055 1056 /** 1057 * @param value {@link #cap} (Color of container cap.) 1058 */ 1059 public SpecimenDefinitionTypeTestedContainerComponent setCap(CodeableConcept value) { 1060 this.cap = value; 1061 return this; 1062 } 1063 1064 /** 1065 * @return {@link #description} (The textual description of the kind of 1066 * container.). This is the underlying object with id, value and 1067 * extensions. The accessor "getDescription" gives direct access to the 1068 * value 1069 */ 1070 public StringType getDescriptionElement() { 1071 if (this.description == null) 1072 if (Configuration.errorOnAutoCreate()) 1073 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedContainerComponent.description"); 1074 else if (Configuration.doAutoCreate()) 1075 this.description = new StringType(); // bb 1076 return this.description; 1077 } 1078 1079 public boolean hasDescriptionElement() { 1080 return this.description != null && !this.description.isEmpty(); 1081 } 1082 1083 public boolean hasDescription() { 1084 return this.description != null && !this.description.isEmpty(); 1085 } 1086 1087 /** 1088 * @param value {@link #description} (The textual description of the kind of 1089 * container.). This is the underlying object with id, value and 1090 * extensions. The accessor "getDescription" gives direct access to 1091 * the value 1092 */ 1093 public SpecimenDefinitionTypeTestedContainerComponent setDescriptionElement(StringType value) { 1094 this.description = value; 1095 return this; 1096 } 1097 1098 /** 1099 * @return The textual description of the kind of container. 1100 */ 1101 public String getDescription() { 1102 return this.description == null ? null : this.description.getValue(); 1103 } 1104 1105 /** 1106 * @param value The textual description of the kind of container. 1107 */ 1108 public SpecimenDefinitionTypeTestedContainerComponent setDescription(String value) { 1109 if (Utilities.noString(value)) 1110 this.description = null; 1111 else { 1112 if (this.description == null) 1113 this.description = new StringType(); 1114 this.description.setValue(value); 1115 } 1116 return this; 1117 } 1118 1119 /** 1120 * @return {@link #capacity} (The capacity (volume or other measure) of this 1121 * kind of container.) 1122 */ 1123 public Quantity getCapacity() { 1124 if (this.capacity == null) 1125 if (Configuration.errorOnAutoCreate()) 1126 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedContainerComponent.capacity"); 1127 else if (Configuration.doAutoCreate()) 1128 this.capacity = new Quantity(); // cc 1129 return this.capacity; 1130 } 1131 1132 public boolean hasCapacity() { 1133 return this.capacity != null && !this.capacity.isEmpty(); 1134 } 1135 1136 /** 1137 * @param value {@link #capacity} (The capacity (volume or other measure) of 1138 * this kind of container.) 1139 */ 1140 public SpecimenDefinitionTypeTestedContainerComponent setCapacity(Quantity value) { 1141 this.capacity = value; 1142 return this; 1143 } 1144 1145 /** 1146 * @return {@link #minimumVolume} (The minimum volume to be conditioned in the 1147 * container.) 1148 */ 1149 public Type getMinimumVolume() { 1150 return this.minimumVolume; 1151 } 1152 1153 /** 1154 * @return {@link #minimumVolume} (The minimum volume to be conditioned in the 1155 * container.) 1156 */ 1157 public Quantity getMinimumVolumeQuantity() throws FHIRException { 1158 if (this.minimumVolume == null) 1159 this.minimumVolume = new Quantity(); 1160 if (!(this.minimumVolume instanceof Quantity)) 1161 throw new FHIRException("Type mismatch: the type Quantity was expected, but " 1162 + this.minimumVolume.getClass().getName() + " was encountered"); 1163 return (Quantity) this.minimumVolume; 1164 } 1165 1166 public boolean hasMinimumVolumeQuantity() { 1167 return this != null && this.minimumVolume instanceof Quantity; 1168 } 1169 1170 /** 1171 * @return {@link #minimumVolume} (The minimum volume to be conditioned in the 1172 * container.) 1173 */ 1174 public StringType getMinimumVolumeStringType() throws FHIRException { 1175 if (this.minimumVolume == null) 1176 this.minimumVolume = new StringType(); 1177 if (!(this.minimumVolume instanceof StringType)) 1178 throw new FHIRException("Type mismatch: the type StringType was expected, but " 1179 + this.minimumVolume.getClass().getName() + " was encountered"); 1180 return (StringType) this.minimumVolume; 1181 } 1182 1183 public boolean hasMinimumVolumeStringType() { 1184 return this != null && this.minimumVolume instanceof StringType; 1185 } 1186 1187 public boolean hasMinimumVolume() { 1188 return this.minimumVolume != null && !this.minimumVolume.isEmpty(); 1189 } 1190 1191 /** 1192 * @param value {@link #minimumVolume} (The minimum volume to be conditioned in 1193 * the container.) 1194 */ 1195 public SpecimenDefinitionTypeTestedContainerComponent setMinimumVolume(Type value) { 1196 if (value != null && !(value instanceof Quantity || value instanceof StringType)) 1197 throw new Error( 1198 "Not the right type for SpecimenDefinition.typeTested.container.minimumVolume[x]: " + value.fhirType()); 1199 this.minimumVolume = value; 1200 return this; 1201 } 1202 1203 /** 1204 * @return {@link #additive} (Substance introduced in the kind of container to 1205 * preserve, maintain or enhance the specimen. Examples: Formalin, 1206 * Citrate, EDTA.) 1207 */ 1208 public List<SpecimenDefinitionTypeTestedContainerAdditiveComponent> getAdditive() { 1209 if (this.additive == null) 1210 this.additive = new ArrayList<SpecimenDefinitionTypeTestedContainerAdditiveComponent>(); 1211 return this.additive; 1212 } 1213 1214 /** 1215 * @return Returns a reference to <code>this</code> for easy method chaining 1216 */ 1217 public SpecimenDefinitionTypeTestedContainerComponent setAdditive( 1218 List<SpecimenDefinitionTypeTestedContainerAdditiveComponent> theAdditive) { 1219 this.additive = theAdditive; 1220 return this; 1221 } 1222 1223 public boolean hasAdditive() { 1224 if (this.additive == null) 1225 return false; 1226 for (SpecimenDefinitionTypeTestedContainerAdditiveComponent item : this.additive) 1227 if (!item.isEmpty()) 1228 return true; 1229 return false; 1230 } 1231 1232 public SpecimenDefinitionTypeTestedContainerAdditiveComponent addAdditive() { // 3 1233 SpecimenDefinitionTypeTestedContainerAdditiveComponent t = new SpecimenDefinitionTypeTestedContainerAdditiveComponent(); 1234 if (this.additive == null) 1235 this.additive = new ArrayList<SpecimenDefinitionTypeTestedContainerAdditiveComponent>(); 1236 this.additive.add(t); 1237 return t; 1238 } 1239 1240 public SpecimenDefinitionTypeTestedContainerComponent addAdditive( 1241 SpecimenDefinitionTypeTestedContainerAdditiveComponent t) { // 3 1242 if (t == null) 1243 return this; 1244 if (this.additive == null) 1245 this.additive = new ArrayList<SpecimenDefinitionTypeTestedContainerAdditiveComponent>(); 1246 this.additive.add(t); 1247 return this; 1248 } 1249 1250 /** 1251 * @return The first repetition of repeating field {@link #additive}, creating 1252 * it if it does not already exist 1253 */ 1254 public SpecimenDefinitionTypeTestedContainerAdditiveComponent getAdditiveFirstRep() { 1255 if (getAdditive().isEmpty()) { 1256 addAdditive(); 1257 } 1258 return getAdditive().get(0); 1259 } 1260 1261 /** 1262 * @return {@link #preparation} (Special processing that should be applied to 1263 * the container for this kind of specimen.). This is the underlying 1264 * object with id, value and extensions. The accessor "getPreparation" 1265 * gives direct access to the value 1266 */ 1267 public StringType getPreparationElement() { 1268 if (this.preparation == null) 1269 if (Configuration.errorOnAutoCreate()) 1270 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedContainerComponent.preparation"); 1271 else if (Configuration.doAutoCreate()) 1272 this.preparation = new StringType(); // bb 1273 return this.preparation; 1274 } 1275 1276 public boolean hasPreparationElement() { 1277 return this.preparation != null && !this.preparation.isEmpty(); 1278 } 1279 1280 public boolean hasPreparation() { 1281 return this.preparation != null && !this.preparation.isEmpty(); 1282 } 1283 1284 /** 1285 * @param value {@link #preparation} (Special processing that should be applied 1286 * to the container for this kind of specimen.). This is the 1287 * underlying object with id, value and extensions. The accessor 1288 * "getPreparation" gives direct access to the value 1289 */ 1290 public SpecimenDefinitionTypeTestedContainerComponent setPreparationElement(StringType value) { 1291 this.preparation = value; 1292 return this; 1293 } 1294 1295 /** 1296 * @return Special processing that should be applied to the container for this 1297 * kind of specimen. 1298 */ 1299 public String getPreparation() { 1300 return this.preparation == null ? null : this.preparation.getValue(); 1301 } 1302 1303 /** 1304 * @param value Special processing that should be applied to the container for 1305 * this kind of specimen. 1306 */ 1307 public SpecimenDefinitionTypeTestedContainerComponent setPreparation(String value) { 1308 if (Utilities.noString(value)) 1309 this.preparation = null; 1310 else { 1311 if (this.preparation == null) 1312 this.preparation = new StringType(); 1313 this.preparation.setValue(value); 1314 } 1315 return this; 1316 } 1317 1318 protected void listChildren(List<Property> children) { 1319 super.listChildren(children); 1320 children 1321 .add(new Property("material", "CodeableConcept", "The type of material of the container.", 0, 1, material)); 1322 children.add(new Property("type", "CodeableConcept", 1323 "The type of container used to contain this kind of specimen.", 0, 1, type)); 1324 children.add(new Property("cap", "CodeableConcept", "Color of container cap.", 0, 1, cap)); 1325 children.add(new Property("description", "string", "The textual description of the kind of container.", 0, 1, 1326 description)); 1327 children.add(new Property("capacity", "SimpleQuantity", 1328 "The capacity (volume or other measure) of this kind of container.", 0, 1, capacity)); 1329 children.add(new Property("minimumVolume[x]", "SimpleQuantity|string", 1330 "The minimum volume to be conditioned in the container.", 0, 1, minimumVolume)); 1331 children.add(new Property("additive", "", 1332 "Substance introduced in the kind of container to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 1333 0, java.lang.Integer.MAX_VALUE, additive)); 1334 children.add(new Property("preparation", "string", 1335 "Special processing that should be applied to the container for this kind of specimen.", 0, 1, preparation)); 1336 } 1337 1338 @Override 1339 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1340 switch (_hash) { 1341 case 299066663: 1342 /* material */ return new Property("material", "CodeableConcept", "The type of material of the container.", 0, 1343 1, material); 1344 case 3575610: 1345 /* type */ return new Property("type", "CodeableConcept", 1346 "The type of container used to contain this kind of specimen.", 0, 1, type); 1347 case 98258: 1348 /* cap */ return new Property("cap", "CodeableConcept", "Color of container cap.", 0, 1, cap); 1349 case -1724546052: 1350 /* description */ return new Property("description", "string", 1351 "The textual description of the kind of container.", 0, 1, description); 1352 case -67824454: 1353 /* capacity */ return new Property("capacity", "SimpleQuantity", 1354 "The capacity (volume or other measure) of this kind of container.", 0, 1, capacity); 1355 case 371830456: 1356 /* minimumVolume[x] */ return new Property("minimumVolume[x]", "SimpleQuantity|string", 1357 "The minimum volume to be conditioned in the container.", 0, 1, minimumVolume); 1358 case -1674665784: 1359 /* minimumVolume */ return new Property("minimumVolume[x]", "SimpleQuantity|string", 1360 "The minimum volume to be conditioned in the container.", 0, 1, minimumVolume); 1361 case -532143757: 1362 /* minimumVolumeQuantity */ return new Property("minimumVolume[x]", "SimpleQuantity|string", 1363 "The minimum volume to be conditioned in the container.", 0, 1, minimumVolume); 1364 case 248461049: 1365 /* minimumVolumeString */ return new Property("minimumVolume[x]", "SimpleQuantity|string", 1366 "The minimum volume to be conditioned in the container.", 0, 1, minimumVolume); 1367 case -1226589236: 1368 /* additive */ return new Property("additive", "", 1369 "Substance introduced in the kind of container to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 1370 0, java.lang.Integer.MAX_VALUE, additive); 1371 case -1315428713: 1372 /* preparation */ return new Property("preparation", "string", 1373 "Special processing that should be applied to the container for this kind of specimen.", 0, 1, preparation); 1374 default: 1375 return super.getNamedProperty(_hash, _name, _checkValid); 1376 } 1377 1378 } 1379 1380 @Override 1381 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1382 switch (hash) { 1383 case 299066663: 1384 /* material */ return this.material == null ? new Base[0] : new Base[] { this.material }; // CodeableConcept 1385 case 3575610: 1386 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1387 case 98258: 1388 /* cap */ return this.cap == null ? new Base[0] : new Base[] { this.cap }; // CodeableConcept 1389 case -1724546052: 1390 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1391 case -67824454: 1392 /* capacity */ return this.capacity == null ? new Base[0] : new Base[] { this.capacity }; // Quantity 1393 case -1674665784: 1394 /* minimumVolume */ return this.minimumVolume == null ? new Base[0] : new Base[] { this.minimumVolume }; // Type 1395 case -1226589236: 1396 /* additive */ return this.additive == null ? new Base[0] 1397 : this.additive.toArray(new Base[this.additive.size()]); // SpecimenDefinitionTypeTestedContainerAdditiveComponent 1398 case -1315428713: 1399 /* preparation */ return this.preparation == null ? new Base[0] : new Base[] { this.preparation }; // StringType 1400 default: 1401 return super.getProperty(hash, name, checkValid); 1402 } 1403 1404 } 1405 1406 @Override 1407 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1408 switch (hash) { 1409 case 299066663: // material 1410 this.material = castToCodeableConcept(value); // CodeableConcept 1411 return value; 1412 case 3575610: // type 1413 this.type = castToCodeableConcept(value); // CodeableConcept 1414 return value; 1415 case 98258: // cap 1416 this.cap = castToCodeableConcept(value); // CodeableConcept 1417 return value; 1418 case -1724546052: // description 1419 this.description = castToString(value); // StringType 1420 return value; 1421 case -67824454: // capacity 1422 this.capacity = castToQuantity(value); // Quantity 1423 return value; 1424 case -1674665784: // minimumVolume 1425 this.minimumVolume = castToType(value); // Type 1426 return value; 1427 case -1226589236: // additive 1428 this.getAdditive().add((SpecimenDefinitionTypeTestedContainerAdditiveComponent) value); // SpecimenDefinitionTypeTestedContainerAdditiveComponent 1429 return value; 1430 case -1315428713: // preparation 1431 this.preparation = castToString(value); // StringType 1432 return value; 1433 default: 1434 return super.setProperty(hash, name, value); 1435 } 1436 1437 } 1438 1439 @Override 1440 public Base setProperty(String name, Base value) throws FHIRException { 1441 if (name.equals("material")) { 1442 this.material = castToCodeableConcept(value); // CodeableConcept 1443 } else if (name.equals("type")) { 1444 this.type = castToCodeableConcept(value); // CodeableConcept 1445 } else if (name.equals("cap")) { 1446 this.cap = castToCodeableConcept(value); // CodeableConcept 1447 } else if (name.equals("description")) { 1448 this.description = castToString(value); // StringType 1449 } else if (name.equals("capacity")) { 1450 this.capacity = castToQuantity(value); // Quantity 1451 } else if (name.equals("minimumVolume[x]")) { 1452 this.minimumVolume = castToType(value); // Type 1453 } else if (name.equals("additive")) { 1454 this.getAdditive().add((SpecimenDefinitionTypeTestedContainerAdditiveComponent) value); 1455 } else if (name.equals("preparation")) { 1456 this.preparation = castToString(value); // StringType 1457 } else 1458 return super.setProperty(name, value); 1459 return value; 1460 } 1461 1462 @Override 1463 public void removeChild(String name, Base value) throws FHIRException { 1464 if (name.equals("material")) { 1465 this.material = null; 1466 } else if (name.equals("type")) { 1467 this.type = null; 1468 } else if (name.equals("cap")) { 1469 this.cap = null; 1470 } else if (name.equals("description")) { 1471 this.description = null; 1472 } else if (name.equals("capacity")) { 1473 this.capacity = null; 1474 } else if (name.equals("minimumVolume[x]")) { 1475 this.minimumVolume = null; 1476 } else if (name.equals("additive")) { 1477 this.getAdditive().remove((SpecimenDefinitionTypeTestedContainerAdditiveComponent) value); 1478 } else if (name.equals("preparation")) { 1479 this.preparation = null; 1480 } else 1481 super.removeChild(name, value); 1482 1483 } 1484 1485 @Override 1486 public Base makeProperty(int hash, String name) throws FHIRException { 1487 switch (hash) { 1488 case 299066663: 1489 return getMaterial(); 1490 case 3575610: 1491 return getType(); 1492 case 98258: 1493 return getCap(); 1494 case -1724546052: 1495 return getDescriptionElement(); 1496 case -67824454: 1497 return getCapacity(); 1498 case 371830456: 1499 return getMinimumVolume(); 1500 case -1674665784: 1501 return getMinimumVolume(); 1502 case -1226589236: 1503 return addAdditive(); 1504 case -1315428713: 1505 return getPreparationElement(); 1506 default: 1507 return super.makeProperty(hash, name); 1508 } 1509 1510 } 1511 1512 @Override 1513 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1514 switch (hash) { 1515 case 299066663: 1516 /* material */ return new String[] { "CodeableConcept" }; 1517 case 3575610: 1518 /* type */ return new String[] { "CodeableConcept" }; 1519 case 98258: 1520 /* cap */ return new String[] { "CodeableConcept" }; 1521 case -1724546052: 1522 /* description */ return new String[] { "string" }; 1523 case -67824454: 1524 /* capacity */ return new String[] { "SimpleQuantity" }; 1525 case -1674665784: 1526 /* minimumVolume */ return new String[] { "SimpleQuantity", "string" }; 1527 case -1226589236: 1528 /* additive */ return new String[] {}; 1529 case -1315428713: 1530 /* preparation */ return new String[] { "string" }; 1531 default: 1532 return super.getTypesForProperty(hash, name); 1533 } 1534 1535 } 1536 1537 @Override 1538 public Base addChild(String name) throws FHIRException { 1539 if (name.equals("material")) { 1540 this.material = new CodeableConcept(); 1541 return this.material; 1542 } else if (name.equals("type")) { 1543 this.type = new CodeableConcept(); 1544 return this.type; 1545 } else if (name.equals("cap")) { 1546 this.cap = new CodeableConcept(); 1547 return this.cap; 1548 } else if (name.equals("description")) { 1549 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.description"); 1550 } else if (name.equals("capacity")) { 1551 this.capacity = new Quantity(); 1552 return this.capacity; 1553 } else if (name.equals("minimumVolumeQuantity")) { 1554 this.minimumVolume = new Quantity(); 1555 return this.minimumVolume; 1556 } else if (name.equals("minimumVolumeString")) { 1557 this.minimumVolume = new StringType(); 1558 return this.minimumVolume; 1559 } else if (name.equals("additive")) { 1560 return addAdditive(); 1561 } else if (name.equals("preparation")) { 1562 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.preparation"); 1563 } else 1564 return super.addChild(name); 1565 } 1566 1567 public SpecimenDefinitionTypeTestedContainerComponent copy() { 1568 SpecimenDefinitionTypeTestedContainerComponent dst = new SpecimenDefinitionTypeTestedContainerComponent(); 1569 copyValues(dst); 1570 return dst; 1571 } 1572 1573 public void copyValues(SpecimenDefinitionTypeTestedContainerComponent dst) { 1574 super.copyValues(dst); 1575 dst.material = material == null ? null : material.copy(); 1576 dst.type = type == null ? null : type.copy(); 1577 dst.cap = cap == null ? null : cap.copy(); 1578 dst.description = description == null ? null : description.copy(); 1579 dst.capacity = capacity == null ? null : capacity.copy(); 1580 dst.minimumVolume = minimumVolume == null ? null : minimumVolume.copy(); 1581 if (additive != null) { 1582 dst.additive = new ArrayList<SpecimenDefinitionTypeTestedContainerAdditiveComponent>(); 1583 for (SpecimenDefinitionTypeTestedContainerAdditiveComponent i : additive) 1584 dst.additive.add(i.copy()); 1585 } 1586 ; 1587 dst.preparation = preparation == null ? null : preparation.copy(); 1588 } 1589 1590 @Override 1591 public boolean equalsDeep(Base other_) { 1592 if (!super.equalsDeep(other_)) 1593 return false; 1594 if (!(other_ instanceof SpecimenDefinitionTypeTestedContainerComponent)) 1595 return false; 1596 SpecimenDefinitionTypeTestedContainerComponent o = (SpecimenDefinitionTypeTestedContainerComponent) other_; 1597 return compareDeep(material, o.material, true) && compareDeep(type, o.type, true) && compareDeep(cap, o.cap, true) 1598 && compareDeep(description, o.description, true) && compareDeep(capacity, o.capacity, true) 1599 && compareDeep(minimumVolume, o.minimumVolume, true) && compareDeep(additive, o.additive, true) 1600 && compareDeep(preparation, o.preparation, true); 1601 } 1602 1603 @Override 1604 public boolean equalsShallow(Base other_) { 1605 if (!super.equalsShallow(other_)) 1606 return false; 1607 if (!(other_ instanceof SpecimenDefinitionTypeTestedContainerComponent)) 1608 return false; 1609 SpecimenDefinitionTypeTestedContainerComponent o = (SpecimenDefinitionTypeTestedContainerComponent) other_; 1610 return compareValues(description, o.description, true) && compareValues(preparation, o.preparation, true); 1611 } 1612 1613 public boolean isEmpty() { 1614 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(material, type, cap, description, capacity, 1615 minimumVolume, additive, preparation); 1616 } 1617 1618 public String fhirType() { 1619 return "SpecimenDefinition.typeTested.container"; 1620 1621 } 1622 1623 } 1624 1625 @Block() 1626 public static class SpecimenDefinitionTypeTestedContainerAdditiveComponent extends BackboneElement 1627 implements IBaseBackboneElement { 1628 /** 1629 * Substance introduced in the kind of container to preserve, maintain or 1630 * enhance the specimen. Examples: Formalin, Citrate, EDTA. 1631 */ 1632 @Child(name = "additive", type = { CodeableConcept.class, 1633 Substance.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1634 @Description(shortDefinition = "Additive associated with container", formalDefinition = "Substance introduced in the kind of container to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.") 1635 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v2-0371") 1636 protected Type additive; 1637 1638 private static final long serialVersionUID = 1819209272L; 1639 1640 /** 1641 * Constructor 1642 */ 1643 public SpecimenDefinitionTypeTestedContainerAdditiveComponent() { 1644 super(); 1645 } 1646 1647 /** 1648 * Constructor 1649 */ 1650 public SpecimenDefinitionTypeTestedContainerAdditiveComponent(Type additive) { 1651 super(); 1652 this.additive = additive; 1653 } 1654 1655 /** 1656 * @return {@link #additive} (Substance introduced in the kind of container to 1657 * preserve, maintain or enhance the specimen. Examples: Formalin, 1658 * Citrate, EDTA.) 1659 */ 1660 public Type getAdditive() { 1661 return this.additive; 1662 } 1663 1664 /** 1665 * @return {@link #additive} (Substance introduced in the kind of container to 1666 * preserve, maintain or enhance the specimen. Examples: Formalin, 1667 * Citrate, EDTA.) 1668 */ 1669 public CodeableConcept getAdditiveCodeableConcept() throws FHIRException { 1670 if (this.additive == null) 1671 this.additive = new CodeableConcept(); 1672 if (!(this.additive instanceof CodeableConcept)) 1673 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1674 + this.additive.getClass().getName() + " was encountered"); 1675 return (CodeableConcept) this.additive; 1676 } 1677 1678 public boolean hasAdditiveCodeableConcept() { 1679 return this != null && this.additive instanceof CodeableConcept; 1680 } 1681 1682 /** 1683 * @return {@link #additive} (Substance introduced in the kind of container to 1684 * preserve, maintain or enhance the specimen. Examples: Formalin, 1685 * Citrate, EDTA.) 1686 */ 1687 public Reference getAdditiveReference() throws FHIRException { 1688 if (this.additive == null) 1689 this.additive = new Reference(); 1690 if (!(this.additive instanceof Reference)) 1691 throw new FHIRException("Type mismatch: the type Reference was expected, but " 1692 + this.additive.getClass().getName() + " was encountered"); 1693 return (Reference) this.additive; 1694 } 1695 1696 public boolean hasAdditiveReference() { 1697 return this != null && this.additive instanceof Reference; 1698 } 1699 1700 public boolean hasAdditive() { 1701 return this.additive != null && !this.additive.isEmpty(); 1702 } 1703 1704 /** 1705 * @param value {@link #additive} (Substance introduced in the kind of container 1706 * to preserve, maintain or enhance the specimen. Examples: 1707 * Formalin, Citrate, EDTA.) 1708 */ 1709 public SpecimenDefinitionTypeTestedContainerAdditiveComponent setAdditive(Type value) { 1710 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1711 throw new Error( 1712 "Not the right type for SpecimenDefinition.typeTested.container.additive.additive[x]: " + value.fhirType()); 1713 this.additive = value; 1714 return this; 1715 } 1716 1717 protected void listChildren(List<Property> children) { 1718 super.listChildren(children); 1719 children.add(new Property("additive[x]", "CodeableConcept|Reference(Substance)", 1720 "Substance introduced in the kind of container to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 1721 0, 1, additive)); 1722 } 1723 1724 @Override 1725 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1726 switch (_hash) { 1727 case 261915956: 1728 /* additive[x] */ return new Property("additive[x]", "CodeableConcept|Reference(Substance)", 1729 "Substance introduced in the kind of container to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 1730 0, 1, additive); 1731 case -1226589236: 1732 /* additive */ return new Property("additive[x]", "CodeableConcept|Reference(Substance)", 1733 "Substance introduced in the kind of container to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 1734 0, 1, additive); 1735 case 1330272821: 1736 /* additiveCodeableConcept */ return new Property("additive[x]", "CodeableConcept|Reference(Substance)", 1737 "Substance introduced in the kind of container to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 1738 0, 1, additive); 1739 case -386783009: 1740 /* additiveReference */ return new Property("additive[x]", "CodeableConcept|Reference(Substance)", 1741 "Substance introduced in the kind of container to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 1742 0, 1, additive); 1743 default: 1744 return super.getNamedProperty(_hash, _name, _checkValid); 1745 } 1746 1747 } 1748 1749 @Override 1750 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1751 switch (hash) { 1752 case -1226589236: 1753 /* additive */ return this.additive == null ? new Base[0] : new Base[] { this.additive }; // Type 1754 default: 1755 return super.getProperty(hash, name, checkValid); 1756 } 1757 1758 } 1759 1760 @Override 1761 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1762 switch (hash) { 1763 case -1226589236: // additive 1764 this.additive = castToType(value); // Type 1765 return value; 1766 default: 1767 return super.setProperty(hash, name, value); 1768 } 1769 1770 } 1771 1772 @Override 1773 public Base setProperty(String name, Base value) throws FHIRException { 1774 if (name.equals("additive[x]")) { 1775 this.additive = castToType(value); // Type 1776 } else 1777 return super.setProperty(name, value); 1778 return value; 1779 } 1780 1781 @Override 1782 public void removeChild(String name, Base value) throws FHIRException { 1783 if (name.equals("additive[x]")) { 1784 this.additive = null; 1785 } else 1786 super.removeChild(name, value); 1787 1788 } 1789 1790 @Override 1791 public Base makeProperty(int hash, String name) throws FHIRException { 1792 switch (hash) { 1793 case 261915956: 1794 return getAdditive(); 1795 case -1226589236: 1796 return getAdditive(); 1797 default: 1798 return super.makeProperty(hash, name); 1799 } 1800 1801 } 1802 1803 @Override 1804 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1805 switch (hash) { 1806 case -1226589236: 1807 /* additive */ return new String[] { "CodeableConcept", "Reference" }; 1808 default: 1809 return super.getTypesForProperty(hash, name); 1810 } 1811 1812 } 1813 1814 @Override 1815 public Base addChild(String name) throws FHIRException { 1816 if (name.equals("additiveCodeableConcept")) { 1817 this.additive = new CodeableConcept(); 1818 return this.additive; 1819 } else if (name.equals("additiveReference")) { 1820 this.additive = new Reference(); 1821 return this.additive; 1822 } else 1823 return super.addChild(name); 1824 } 1825 1826 public SpecimenDefinitionTypeTestedContainerAdditiveComponent copy() { 1827 SpecimenDefinitionTypeTestedContainerAdditiveComponent dst = new SpecimenDefinitionTypeTestedContainerAdditiveComponent(); 1828 copyValues(dst); 1829 return dst; 1830 } 1831 1832 public void copyValues(SpecimenDefinitionTypeTestedContainerAdditiveComponent dst) { 1833 super.copyValues(dst); 1834 dst.additive = additive == null ? null : additive.copy(); 1835 } 1836 1837 @Override 1838 public boolean equalsDeep(Base other_) { 1839 if (!super.equalsDeep(other_)) 1840 return false; 1841 if (!(other_ instanceof SpecimenDefinitionTypeTestedContainerAdditiveComponent)) 1842 return false; 1843 SpecimenDefinitionTypeTestedContainerAdditiveComponent o = (SpecimenDefinitionTypeTestedContainerAdditiveComponent) other_; 1844 return compareDeep(additive, o.additive, true); 1845 } 1846 1847 @Override 1848 public boolean equalsShallow(Base other_) { 1849 if (!super.equalsShallow(other_)) 1850 return false; 1851 if (!(other_ instanceof SpecimenDefinitionTypeTestedContainerAdditiveComponent)) 1852 return false; 1853 SpecimenDefinitionTypeTestedContainerAdditiveComponent o = (SpecimenDefinitionTypeTestedContainerAdditiveComponent) other_; 1854 return true; 1855 } 1856 1857 public boolean isEmpty() { 1858 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(additive); 1859 } 1860 1861 public String fhirType() { 1862 return "SpecimenDefinition.typeTested.container.additive"; 1863 1864 } 1865 1866 } 1867 1868 @Block() 1869 public static class SpecimenDefinitionTypeTestedHandlingComponent extends BackboneElement 1870 implements IBaseBackboneElement { 1871 /** 1872 * It qualifies the interval of temperature, which characterizes an occurrence 1873 * of handling. Conditions that are not related to temperature may be handled in 1874 * the instruction element. 1875 */ 1876 @Child(name = "temperatureQualifier", type = { 1877 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1878 @Description(shortDefinition = "Temperature qualifier", formalDefinition = "It qualifies the interval of temperature, which characterizes an occurrence of handling. Conditions that are not related to temperature may be handled in the instruction element.") 1879 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/handling-condition") 1880 protected CodeableConcept temperatureQualifier; 1881 1882 /** 1883 * The temperature interval for this set of handling instructions. 1884 */ 1885 @Child(name = "temperatureRange", type = { 1886 Range.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1887 @Description(shortDefinition = "Temperature range", formalDefinition = "The temperature interval for this set of handling instructions.") 1888 protected Range temperatureRange; 1889 1890 /** 1891 * The maximum time interval of preservation of the specimen with these 1892 * conditions. 1893 */ 1894 @Child(name = "maxDuration", type = { 1895 Duration.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1896 @Description(shortDefinition = "Maximum preservation time", formalDefinition = "The maximum time interval of preservation of the specimen with these conditions.") 1897 protected Duration maxDuration; 1898 1899 /** 1900 * Additional textual instructions for the preservation or transport of the 1901 * specimen. For instance, 'Protect from light exposure'. 1902 */ 1903 @Child(name = "instruction", type = { 1904 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1905 @Description(shortDefinition = "Preservation instruction", formalDefinition = "Additional textual instructions for the preservation or transport of the specimen. For instance, 'Protect from light exposure'.") 1906 protected StringType instruction; 1907 1908 private static final long serialVersionUID = 2130906844L; 1909 1910 /** 1911 * Constructor 1912 */ 1913 public SpecimenDefinitionTypeTestedHandlingComponent() { 1914 super(); 1915 } 1916 1917 /** 1918 * @return {@link #temperatureQualifier} (It qualifies the interval of 1919 * temperature, which characterizes an occurrence of handling. 1920 * Conditions that are not related to temperature may be handled in the 1921 * instruction element.) 1922 */ 1923 public CodeableConcept getTemperatureQualifier() { 1924 if (this.temperatureQualifier == null) 1925 if (Configuration.errorOnAutoCreate()) 1926 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedHandlingComponent.temperatureQualifier"); 1927 else if (Configuration.doAutoCreate()) 1928 this.temperatureQualifier = new CodeableConcept(); // cc 1929 return this.temperatureQualifier; 1930 } 1931 1932 public boolean hasTemperatureQualifier() { 1933 return this.temperatureQualifier != null && !this.temperatureQualifier.isEmpty(); 1934 } 1935 1936 /** 1937 * @param value {@link #temperatureQualifier} (It qualifies the interval of 1938 * temperature, which characterizes an occurrence of handling. 1939 * Conditions that are not related to temperature may be handled in 1940 * the instruction element.) 1941 */ 1942 public SpecimenDefinitionTypeTestedHandlingComponent setTemperatureQualifier(CodeableConcept value) { 1943 this.temperatureQualifier = value; 1944 return this; 1945 } 1946 1947 /** 1948 * @return {@link #temperatureRange} (The temperature interval for this set of 1949 * handling instructions.) 1950 */ 1951 public Range getTemperatureRange() { 1952 if (this.temperatureRange == null) 1953 if (Configuration.errorOnAutoCreate()) 1954 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedHandlingComponent.temperatureRange"); 1955 else if (Configuration.doAutoCreate()) 1956 this.temperatureRange = new Range(); // cc 1957 return this.temperatureRange; 1958 } 1959 1960 public boolean hasTemperatureRange() { 1961 return this.temperatureRange != null && !this.temperatureRange.isEmpty(); 1962 } 1963 1964 /** 1965 * @param value {@link #temperatureRange} (The temperature interval for this set 1966 * of handling instructions.) 1967 */ 1968 public SpecimenDefinitionTypeTestedHandlingComponent setTemperatureRange(Range value) { 1969 this.temperatureRange = value; 1970 return this; 1971 } 1972 1973 /** 1974 * @return {@link #maxDuration} (The maximum time interval of preservation of 1975 * the specimen with these conditions.) 1976 */ 1977 public Duration getMaxDuration() { 1978 if (this.maxDuration == null) 1979 if (Configuration.errorOnAutoCreate()) 1980 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedHandlingComponent.maxDuration"); 1981 else if (Configuration.doAutoCreate()) 1982 this.maxDuration = new Duration(); // cc 1983 return this.maxDuration; 1984 } 1985 1986 public boolean hasMaxDuration() { 1987 return this.maxDuration != null && !this.maxDuration.isEmpty(); 1988 } 1989 1990 /** 1991 * @param value {@link #maxDuration} (The maximum time interval of preservation 1992 * of the specimen with these conditions.) 1993 */ 1994 public SpecimenDefinitionTypeTestedHandlingComponent setMaxDuration(Duration value) { 1995 this.maxDuration = value; 1996 return this; 1997 } 1998 1999 /** 2000 * @return {@link #instruction} (Additional textual instructions for the 2001 * preservation or transport of the specimen. For instance, 'Protect 2002 * from light exposure'.). This is the underlying object with id, value 2003 * and extensions. The accessor "getInstruction" gives direct access to 2004 * the value 2005 */ 2006 public StringType getInstructionElement() { 2007 if (this.instruction == null) 2008 if (Configuration.errorOnAutoCreate()) 2009 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedHandlingComponent.instruction"); 2010 else if (Configuration.doAutoCreate()) 2011 this.instruction = new StringType(); // bb 2012 return this.instruction; 2013 } 2014 2015 public boolean hasInstructionElement() { 2016 return this.instruction != null && !this.instruction.isEmpty(); 2017 } 2018 2019 public boolean hasInstruction() { 2020 return this.instruction != null && !this.instruction.isEmpty(); 2021 } 2022 2023 /** 2024 * @param value {@link #instruction} (Additional textual instructions for the 2025 * preservation or transport of the specimen. For instance, 2026 * 'Protect from light exposure'.). This is the underlying object 2027 * with id, value and extensions. The accessor "getInstruction" 2028 * gives direct access to the value 2029 */ 2030 public SpecimenDefinitionTypeTestedHandlingComponent setInstructionElement(StringType value) { 2031 this.instruction = value; 2032 return this; 2033 } 2034 2035 /** 2036 * @return Additional textual instructions for the preservation or transport of 2037 * the specimen. For instance, 'Protect from light exposure'. 2038 */ 2039 public String getInstruction() { 2040 return this.instruction == null ? null : this.instruction.getValue(); 2041 } 2042 2043 /** 2044 * @param value Additional textual instructions for the preservation or 2045 * transport of the specimen. For instance, 'Protect from light 2046 * exposure'. 2047 */ 2048 public SpecimenDefinitionTypeTestedHandlingComponent setInstruction(String value) { 2049 if (Utilities.noString(value)) 2050 this.instruction = null; 2051 else { 2052 if (this.instruction == null) 2053 this.instruction = new StringType(); 2054 this.instruction.setValue(value); 2055 } 2056 return this; 2057 } 2058 2059 protected void listChildren(List<Property> children) { 2060 super.listChildren(children); 2061 children.add(new Property("temperatureQualifier", "CodeableConcept", 2062 "It qualifies the interval of temperature, which characterizes an occurrence of handling. Conditions that are not related to temperature may be handled in the instruction element.", 2063 0, 1, temperatureQualifier)); 2064 children.add(new Property("temperatureRange", "Range", 2065 "The temperature interval for this set of handling instructions.", 0, 1, temperatureRange)); 2066 children.add(new Property("maxDuration", "Duration", 2067 "The maximum time interval of preservation of the specimen with these conditions.", 0, 1, maxDuration)); 2068 children.add(new Property("instruction", "string", 2069 "Additional textual instructions for the preservation or transport of the specimen. For instance, 'Protect from light exposure'.", 2070 0, 1, instruction)); 2071 } 2072 2073 @Override 2074 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2075 switch (_hash) { 2076 case 548941206: 2077 /* temperatureQualifier */ return new Property("temperatureQualifier", "CodeableConcept", 2078 "It qualifies the interval of temperature, which characterizes an occurrence of handling. Conditions that are not related to temperature may be handled in the instruction element.", 2079 0, 1, temperatureQualifier); 2080 case -39203799: 2081 /* temperatureRange */ return new Property("temperatureRange", "Range", 2082 "The temperature interval for this set of handling instructions.", 0, 1, temperatureRange); 2083 case 40284952: 2084 /* maxDuration */ return new Property("maxDuration", "Duration", 2085 "The maximum time interval of preservation of the specimen with these conditions.", 0, 1, maxDuration); 2086 case 301526158: 2087 /* instruction */ return new Property("instruction", "string", 2088 "Additional textual instructions for the preservation or transport of the specimen. For instance, 'Protect from light exposure'.", 2089 0, 1, instruction); 2090 default: 2091 return super.getNamedProperty(_hash, _name, _checkValid); 2092 } 2093 2094 } 2095 2096 @Override 2097 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2098 switch (hash) { 2099 case 548941206: 2100 /* temperatureQualifier */ return this.temperatureQualifier == null ? new Base[0] 2101 : new Base[] { this.temperatureQualifier }; // CodeableConcept 2102 case -39203799: 2103 /* temperatureRange */ return this.temperatureRange == null ? new Base[0] 2104 : new Base[] { this.temperatureRange }; // Range 2105 case 40284952: 2106 /* maxDuration */ return this.maxDuration == null ? new Base[0] : new Base[] { this.maxDuration }; // Duration 2107 case 301526158: 2108 /* instruction */ return this.instruction == null ? new Base[0] : new Base[] { this.instruction }; // StringType 2109 default: 2110 return super.getProperty(hash, name, checkValid); 2111 } 2112 2113 } 2114 2115 @Override 2116 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2117 switch (hash) { 2118 case 548941206: // temperatureQualifier 2119 this.temperatureQualifier = castToCodeableConcept(value); // CodeableConcept 2120 return value; 2121 case -39203799: // temperatureRange 2122 this.temperatureRange = castToRange(value); // Range 2123 return value; 2124 case 40284952: // maxDuration 2125 this.maxDuration = castToDuration(value); // Duration 2126 return value; 2127 case 301526158: // instruction 2128 this.instruction = castToString(value); // StringType 2129 return value; 2130 default: 2131 return super.setProperty(hash, name, value); 2132 } 2133 2134 } 2135 2136 @Override 2137 public Base setProperty(String name, Base value) throws FHIRException { 2138 if (name.equals("temperatureQualifier")) { 2139 this.temperatureQualifier = castToCodeableConcept(value); // CodeableConcept 2140 } else if (name.equals("temperatureRange")) { 2141 this.temperatureRange = castToRange(value); // Range 2142 } else if (name.equals("maxDuration")) { 2143 this.maxDuration = castToDuration(value); // Duration 2144 } else if (name.equals("instruction")) { 2145 this.instruction = castToString(value); // StringType 2146 } else 2147 return super.setProperty(name, value); 2148 return value; 2149 } 2150 2151 @Override 2152 public void removeChild(String name, Base value) throws FHIRException { 2153 if (name.equals("temperatureQualifier")) { 2154 this.temperatureQualifier = null; 2155 } else if (name.equals("temperatureRange")) { 2156 this.temperatureRange = null; 2157 } else if (name.equals("maxDuration")) { 2158 this.maxDuration = null; 2159 } else if (name.equals("instruction")) { 2160 this.instruction = null; 2161 } else 2162 super.removeChild(name, value); 2163 2164 } 2165 2166 @Override 2167 public Base makeProperty(int hash, String name) throws FHIRException { 2168 switch (hash) { 2169 case 548941206: 2170 return getTemperatureQualifier(); 2171 case -39203799: 2172 return getTemperatureRange(); 2173 case 40284952: 2174 return getMaxDuration(); 2175 case 301526158: 2176 return getInstructionElement(); 2177 default: 2178 return super.makeProperty(hash, name); 2179 } 2180 2181 } 2182 2183 @Override 2184 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2185 switch (hash) { 2186 case 548941206: 2187 /* temperatureQualifier */ return new String[] { "CodeableConcept" }; 2188 case -39203799: 2189 /* temperatureRange */ return new String[] { "Range" }; 2190 case 40284952: 2191 /* maxDuration */ return new String[] { "Duration" }; 2192 case 301526158: 2193 /* instruction */ return new String[] { "string" }; 2194 default: 2195 return super.getTypesForProperty(hash, name); 2196 } 2197 2198 } 2199 2200 @Override 2201 public Base addChild(String name) throws FHIRException { 2202 if (name.equals("temperatureQualifier")) { 2203 this.temperatureQualifier = new CodeableConcept(); 2204 return this.temperatureQualifier; 2205 } else if (name.equals("temperatureRange")) { 2206 this.temperatureRange = new Range(); 2207 return this.temperatureRange; 2208 } else if (name.equals("maxDuration")) { 2209 this.maxDuration = new Duration(); 2210 return this.maxDuration; 2211 } else if (name.equals("instruction")) { 2212 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.instruction"); 2213 } else 2214 return super.addChild(name); 2215 } 2216 2217 public SpecimenDefinitionTypeTestedHandlingComponent copy() { 2218 SpecimenDefinitionTypeTestedHandlingComponent dst = new SpecimenDefinitionTypeTestedHandlingComponent(); 2219 copyValues(dst); 2220 return dst; 2221 } 2222 2223 public void copyValues(SpecimenDefinitionTypeTestedHandlingComponent dst) { 2224 super.copyValues(dst); 2225 dst.temperatureQualifier = temperatureQualifier == null ? null : temperatureQualifier.copy(); 2226 dst.temperatureRange = temperatureRange == null ? null : temperatureRange.copy(); 2227 dst.maxDuration = maxDuration == null ? null : maxDuration.copy(); 2228 dst.instruction = instruction == null ? null : instruction.copy(); 2229 } 2230 2231 @Override 2232 public boolean equalsDeep(Base other_) { 2233 if (!super.equalsDeep(other_)) 2234 return false; 2235 if (!(other_ instanceof SpecimenDefinitionTypeTestedHandlingComponent)) 2236 return false; 2237 SpecimenDefinitionTypeTestedHandlingComponent o = (SpecimenDefinitionTypeTestedHandlingComponent) other_; 2238 return compareDeep(temperatureQualifier, o.temperatureQualifier, true) 2239 && compareDeep(temperatureRange, o.temperatureRange, true) && compareDeep(maxDuration, o.maxDuration, true) 2240 && compareDeep(instruction, o.instruction, true); 2241 } 2242 2243 @Override 2244 public boolean equalsShallow(Base other_) { 2245 if (!super.equalsShallow(other_)) 2246 return false; 2247 if (!(other_ instanceof SpecimenDefinitionTypeTestedHandlingComponent)) 2248 return false; 2249 SpecimenDefinitionTypeTestedHandlingComponent o = (SpecimenDefinitionTypeTestedHandlingComponent) other_; 2250 return compareValues(instruction, o.instruction, true); 2251 } 2252 2253 public boolean isEmpty() { 2254 return super.isEmpty() 2255 && ca.uhn.fhir.util.ElementUtil.isEmpty(temperatureQualifier, temperatureRange, maxDuration, instruction); 2256 } 2257 2258 public String fhirType() { 2259 return "SpecimenDefinition.typeTested.handling"; 2260 2261 } 2262 2263 } 2264 2265 /** 2266 * A business identifier associated with the kind of specimen. 2267 */ 2268 @Child(name = "identifier", type = { 2269 Identifier.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 2270 @Description(shortDefinition = "Business identifier of a kind of specimen", formalDefinition = "A business identifier associated with the kind of specimen.") 2271 protected Identifier identifier; 2272 2273 /** 2274 * The kind of material to be collected. 2275 */ 2276 @Child(name = "typeCollected", type = { 2277 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 2278 @Description(shortDefinition = "Kind of material to collect", formalDefinition = "The kind of material to be collected.") 2279 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v2-0487") 2280 protected CodeableConcept typeCollected; 2281 2282 /** 2283 * Preparation of the patient for specimen collection. 2284 */ 2285 @Child(name = "patientPreparation", type = { 2286 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2287 @Description(shortDefinition = "Patient preparation for collection", formalDefinition = "Preparation of the patient for specimen collection.") 2288 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/prepare-patient-prior-specimen-collection") 2289 protected List<CodeableConcept> patientPreparation; 2290 2291 /** 2292 * Time aspect of specimen collection (duration or offset). 2293 */ 2294 @Child(name = "timeAspect", type = { 2295 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 2296 @Description(shortDefinition = "Time aspect for collection", formalDefinition = "Time aspect of specimen collection (duration or offset).") 2297 protected StringType timeAspect; 2298 2299 /** 2300 * The action to be performed for collecting the specimen. 2301 */ 2302 @Child(name = "collection", type = { 2303 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2304 @Description(shortDefinition = "Specimen collection procedure", formalDefinition = "The action to be performed for collecting the specimen.") 2305 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/specimen-collection") 2306 protected List<CodeableConcept> collection; 2307 2308 /** 2309 * Specimen conditioned in a container as expected by the testing laboratory. 2310 */ 2311 @Child(name = "typeTested", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2312 @Description(shortDefinition = "Specimen in container intended for testing by lab", formalDefinition = "Specimen conditioned in a container as expected by the testing laboratory.") 2313 protected List<SpecimenDefinitionTypeTestedComponent> typeTested; 2314 2315 private static final long serialVersionUID = -330188872L; 2316 2317 /** 2318 * Constructor 2319 */ 2320 public SpecimenDefinition() { 2321 super(); 2322 } 2323 2324 /** 2325 * @return {@link #identifier} (A business identifier associated with the kind 2326 * of specimen.) 2327 */ 2328 public Identifier getIdentifier() { 2329 if (this.identifier == null) 2330 if (Configuration.errorOnAutoCreate()) 2331 throw new Error("Attempt to auto-create SpecimenDefinition.identifier"); 2332 else if (Configuration.doAutoCreate()) 2333 this.identifier = new Identifier(); // cc 2334 return this.identifier; 2335 } 2336 2337 public boolean hasIdentifier() { 2338 return this.identifier != null && !this.identifier.isEmpty(); 2339 } 2340 2341 /** 2342 * @param value {@link #identifier} (A business identifier associated with the 2343 * kind of specimen.) 2344 */ 2345 public SpecimenDefinition setIdentifier(Identifier value) { 2346 this.identifier = value; 2347 return this; 2348 } 2349 2350 /** 2351 * @return {@link #typeCollected} (The kind of material to be collected.) 2352 */ 2353 public CodeableConcept getTypeCollected() { 2354 if (this.typeCollected == null) 2355 if (Configuration.errorOnAutoCreate()) 2356 throw new Error("Attempt to auto-create SpecimenDefinition.typeCollected"); 2357 else if (Configuration.doAutoCreate()) 2358 this.typeCollected = new CodeableConcept(); // cc 2359 return this.typeCollected; 2360 } 2361 2362 public boolean hasTypeCollected() { 2363 return this.typeCollected != null && !this.typeCollected.isEmpty(); 2364 } 2365 2366 /** 2367 * @param value {@link #typeCollected} (The kind of material to be collected.) 2368 */ 2369 public SpecimenDefinition setTypeCollected(CodeableConcept value) { 2370 this.typeCollected = value; 2371 return this; 2372 } 2373 2374 /** 2375 * @return {@link #patientPreparation} (Preparation of the patient for specimen 2376 * collection.) 2377 */ 2378 public List<CodeableConcept> getPatientPreparation() { 2379 if (this.patientPreparation == null) 2380 this.patientPreparation = new ArrayList<CodeableConcept>(); 2381 return this.patientPreparation; 2382 } 2383 2384 /** 2385 * @return Returns a reference to <code>this</code> for easy method chaining 2386 */ 2387 public SpecimenDefinition setPatientPreparation(List<CodeableConcept> thePatientPreparation) { 2388 this.patientPreparation = thePatientPreparation; 2389 return this; 2390 } 2391 2392 public boolean hasPatientPreparation() { 2393 if (this.patientPreparation == null) 2394 return false; 2395 for (CodeableConcept item : this.patientPreparation) 2396 if (!item.isEmpty()) 2397 return true; 2398 return false; 2399 } 2400 2401 public CodeableConcept addPatientPreparation() { // 3 2402 CodeableConcept t = new CodeableConcept(); 2403 if (this.patientPreparation == null) 2404 this.patientPreparation = new ArrayList<CodeableConcept>(); 2405 this.patientPreparation.add(t); 2406 return t; 2407 } 2408 2409 public SpecimenDefinition addPatientPreparation(CodeableConcept t) { // 3 2410 if (t == null) 2411 return this; 2412 if (this.patientPreparation == null) 2413 this.patientPreparation = new ArrayList<CodeableConcept>(); 2414 this.patientPreparation.add(t); 2415 return this; 2416 } 2417 2418 /** 2419 * @return The first repetition of repeating field {@link #patientPreparation}, 2420 * creating it if it does not already exist 2421 */ 2422 public CodeableConcept getPatientPreparationFirstRep() { 2423 if (getPatientPreparation().isEmpty()) { 2424 addPatientPreparation(); 2425 } 2426 return getPatientPreparation().get(0); 2427 } 2428 2429 /** 2430 * @return {@link #timeAspect} (Time aspect of specimen collection (duration or 2431 * offset).). This is the underlying object with id, value and 2432 * extensions. The accessor "getTimeAspect" gives direct access to the 2433 * value 2434 */ 2435 public StringType getTimeAspectElement() { 2436 if (this.timeAspect == null) 2437 if (Configuration.errorOnAutoCreate()) 2438 throw new Error("Attempt to auto-create SpecimenDefinition.timeAspect"); 2439 else if (Configuration.doAutoCreate()) 2440 this.timeAspect = new StringType(); // bb 2441 return this.timeAspect; 2442 } 2443 2444 public boolean hasTimeAspectElement() { 2445 return this.timeAspect != null && !this.timeAspect.isEmpty(); 2446 } 2447 2448 public boolean hasTimeAspect() { 2449 return this.timeAspect != null && !this.timeAspect.isEmpty(); 2450 } 2451 2452 /** 2453 * @param value {@link #timeAspect} (Time aspect of specimen collection 2454 * (duration or offset).). This is the underlying object with id, 2455 * value and extensions. The accessor "getTimeAspect" gives direct 2456 * access to the value 2457 */ 2458 public SpecimenDefinition setTimeAspectElement(StringType value) { 2459 this.timeAspect = value; 2460 return this; 2461 } 2462 2463 /** 2464 * @return Time aspect of specimen collection (duration or offset). 2465 */ 2466 public String getTimeAspect() { 2467 return this.timeAspect == null ? null : this.timeAspect.getValue(); 2468 } 2469 2470 /** 2471 * @param value Time aspect of specimen collection (duration or offset). 2472 */ 2473 public SpecimenDefinition setTimeAspect(String value) { 2474 if (Utilities.noString(value)) 2475 this.timeAspect = null; 2476 else { 2477 if (this.timeAspect == null) 2478 this.timeAspect = new StringType(); 2479 this.timeAspect.setValue(value); 2480 } 2481 return this; 2482 } 2483 2484 /** 2485 * @return {@link #collection} (The action to be performed for collecting the 2486 * specimen.) 2487 */ 2488 public List<CodeableConcept> getCollection() { 2489 if (this.collection == null) 2490 this.collection = new ArrayList<CodeableConcept>(); 2491 return this.collection; 2492 } 2493 2494 /** 2495 * @return Returns a reference to <code>this</code> for easy method chaining 2496 */ 2497 public SpecimenDefinition setCollection(List<CodeableConcept> theCollection) { 2498 this.collection = theCollection; 2499 return this; 2500 } 2501 2502 public boolean hasCollection() { 2503 if (this.collection == null) 2504 return false; 2505 for (CodeableConcept item : this.collection) 2506 if (!item.isEmpty()) 2507 return true; 2508 return false; 2509 } 2510 2511 public CodeableConcept addCollection() { // 3 2512 CodeableConcept t = new CodeableConcept(); 2513 if (this.collection == null) 2514 this.collection = new ArrayList<CodeableConcept>(); 2515 this.collection.add(t); 2516 return t; 2517 } 2518 2519 public SpecimenDefinition addCollection(CodeableConcept t) { // 3 2520 if (t == null) 2521 return this; 2522 if (this.collection == null) 2523 this.collection = new ArrayList<CodeableConcept>(); 2524 this.collection.add(t); 2525 return this; 2526 } 2527 2528 /** 2529 * @return The first repetition of repeating field {@link #collection}, creating 2530 * it if it does not already exist 2531 */ 2532 public CodeableConcept getCollectionFirstRep() { 2533 if (getCollection().isEmpty()) { 2534 addCollection(); 2535 } 2536 return getCollection().get(0); 2537 } 2538 2539 /** 2540 * @return {@link #typeTested} (Specimen conditioned in a container as expected 2541 * by the testing laboratory.) 2542 */ 2543 public List<SpecimenDefinitionTypeTestedComponent> getTypeTested() { 2544 if (this.typeTested == null) 2545 this.typeTested = new ArrayList<SpecimenDefinitionTypeTestedComponent>(); 2546 return this.typeTested; 2547 } 2548 2549 /** 2550 * @return Returns a reference to <code>this</code> for easy method chaining 2551 */ 2552 public SpecimenDefinition setTypeTested(List<SpecimenDefinitionTypeTestedComponent> theTypeTested) { 2553 this.typeTested = theTypeTested; 2554 return this; 2555 } 2556 2557 public boolean hasTypeTested() { 2558 if (this.typeTested == null) 2559 return false; 2560 for (SpecimenDefinitionTypeTestedComponent item : this.typeTested) 2561 if (!item.isEmpty()) 2562 return true; 2563 return false; 2564 } 2565 2566 public SpecimenDefinitionTypeTestedComponent addTypeTested() { // 3 2567 SpecimenDefinitionTypeTestedComponent t = new SpecimenDefinitionTypeTestedComponent(); 2568 if (this.typeTested == null) 2569 this.typeTested = new ArrayList<SpecimenDefinitionTypeTestedComponent>(); 2570 this.typeTested.add(t); 2571 return t; 2572 } 2573 2574 public SpecimenDefinition addTypeTested(SpecimenDefinitionTypeTestedComponent t) { // 3 2575 if (t == null) 2576 return this; 2577 if (this.typeTested == null) 2578 this.typeTested = new ArrayList<SpecimenDefinitionTypeTestedComponent>(); 2579 this.typeTested.add(t); 2580 return this; 2581 } 2582 2583 /** 2584 * @return The first repetition of repeating field {@link #typeTested}, creating 2585 * it if it does not already exist 2586 */ 2587 public SpecimenDefinitionTypeTestedComponent getTypeTestedFirstRep() { 2588 if (getTypeTested().isEmpty()) { 2589 addTypeTested(); 2590 } 2591 return getTypeTested().get(0); 2592 } 2593 2594 protected void listChildren(List<Property> children) { 2595 super.listChildren(children); 2596 children.add(new Property("identifier", "Identifier", "A business identifier associated with the kind of specimen.", 2597 0, 1, identifier)); 2598 children.add( 2599 new Property("typeCollected", "CodeableConcept", "The kind of material to be collected.", 0, 1, typeCollected)); 2600 children.add(new Property("patientPreparation", "CodeableConcept", 2601 "Preparation of the patient for specimen collection.", 0, java.lang.Integer.MAX_VALUE, patientPreparation)); 2602 children.add(new Property("timeAspect", "string", "Time aspect of specimen collection (duration or offset).", 0, 1, 2603 timeAspect)); 2604 children.add(new Property("collection", "CodeableConcept", 2605 "The action to be performed for collecting the specimen.", 0, java.lang.Integer.MAX_VALUE, collection)); 2606 children.add( 2607 new Property("typeTested", "", "Specimen conditioned in a container as expected by the testing laboratory.", 0, 2608 java.lang.Integer.MAX_VALUE, typeTested)); 2609 } 2610 2611 @Override 2612 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2613 switch (_hash) { 2614 case -1618432855: 2615 /* identifier */ return new Property("identifier", "Identifier", 2616 "A business identifier associated with the kind of specimen.", 0, 1, identifier); 2617 case 588504367: 2618 /* typeCollected */ return new Property("typeCollected", "CodeableConcept", 2619 "The kind of material to be collected.", 0, 1, typeCollected); 2620 case -879411630: 2621 /* patientPreparation */ return new Property("patientPreparation", "CodeableConcept", 2622 "Preparation of the patient for specimen collection.", 0, java.lang.Integer.MAX_VALUE, patientPreparation); 2623 case 276972933: 2624 /* timeAspect */ return new Property("timeAspect", "string", 2625 "Time aspect of specimen collection (duration or offset).", 0, 1, timeAspect); 2626 case -1741312354: 2627 /* collection */ return new Property("collection", "CodeableConcept", 2628 "The action to be performed for collecting the specimen.", 0, java.lang.Integer.MAX_VALUE, collection); 2629 case -1407902581: 2630 /* typeTested */ return new Property("typeTested", "", 2631 "Specimen conditioned in a container as expected by the testing laboratory.", 0, java.lang.Integer.MAX_VALUE, 2632 typeTested); 2633 default: 2634 return super.getNamedProperty(_hash, _name, _checkValid); 2635 } 2636 2637 } 2638 2639 @Override 2640 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2641 switch (hash) { 2642 case -1618432855: 2643 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 2644 case 588504367: 2645 /* typeCollected */ return this.typeCollected == null ? new Base[0] : new Base[] { this.typeCollected }; // CodeableConcept 2646 case -879411630: 2647 /* patientPreparation */ return this.patientPreparation == null ? new Base[0] 2648 : this.patientPreparation.toArray(new Base[this.patientPreparation.size()]); // CodeableConcept 2649 case 276972933: 2650 /* timeAspect */ return this.timeAspect == null ? new Base[0] : new Base[] { this.timeAspect }; // StringType 2651 case -1741312354: 2652 /* collection */ return this.collection == null ? new Base[0] 2653 : this.collection.toArray(new Base[this.collection.size()]); // CodeableConcept 2654 case -1407902581: 2655 /* typeTested */ return this.typeTested == null ? new Base[0] 2656 : this.typeTested.toArray(new Base[this.typeTested.size()]); // SpecimenDefinitionTypeTestedComponent 2657 default: 2658 return super.getProperty(hash, name, checkValid); 2659 } 2660 2661 } 2662 2663 @Override 2664 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2665 switch (hash) { 2666 case -1618432855: // identifier 2667 this.identifier = castToIdentifier(value); // Identifier 2668 return value; 2669 case 588504367: // typeCollected 2670 this.typeCollected = castToCodeableConcept(value); // CodeableConcept 2671 return value; 2672 case -879411630: // patientPreparation 2673 this.getPatientPreparation().add(castToCodeableConcept(value)); // CodeableConcept 2674 return value; 2675 case 276972933: // timeAspect 2676 this.timeAspect = castToString(value); // StringType 2677 return value; 2678 case -1741312354: // collection 2679 this.getCollection().add(castToCodeableConcept(value)); // CodeableConcept 2680 return value; 2681 case -1407902581: // typeTested 2682 this.getTypeTested().add((SpecimenDefinitionTypeTestedComponent) value); // SpecimenDefinitionTypeTestedComponent 2683 return value; 2684 default: 2685 return super.setProperty(hash, name, value); 2686 } 2687 2688 } 2689 2690 @Override 2691 public Base setProperty(String name, Base value) throws FHIRException { 2692 if (name.equals("identifier")) { 2693 this.identifier = castToIdentifier(value); // Identifier 2694 } else if (name.equals("typeCollected")) { 2695 this.typeCollected = castToCodeableConcept(value); // CodeableConcept 2696 } else if (name.equals("patientPreparation")) { 2697 this.getPatientPreparation().add(castToCodeableConcept(value)); 2698 } else if (name.equals("timeAspect")) { 2699 this.timeAspect = castToString(value); // StringType 2700 } else if (name.equals("collection")) { 2701 this.getCollection().add(castToCodeableConcept(value)); 2702 } else if (name.equals("typeTested")) { 2703 this.getTypeTested().add((SpecimenDefinitionTypeTestedComponent) value); 2704 } else 2705 return super.setProperty(name, value); 2706 return value; 2707 } 2708 2709 @Override 2710 public void removeChild(String name, Base value) throws FHIRException { 2711 if (name.equals("identifier")) { 2712 this.identifier = null; 2713 } else if (name.equals("typeCollected")) { 2714 this.typeCollected = null; 2715 } else if (name.equals("patientPreparation")) { 2716 this.getPatientPreparation().remove(castToCodeableConcept(value)); 2717 } else if (name.equals("timeAspect")) { 2718 this.timeAspect = null; 2719 } else if (name.equals("collection")) { 2720 this.getCollection().remove(castToCodeableConcept(value)); 2721 } else if (name.equals("typeTested")) { 2722 this.getTypeTested().remove((SpecimenDefinitionTypeTestedComponent) value); 2723 } else 2724 super.removeChild(name, value); 2725 2726 } 2727 2728 @Override 2729 public Base makeProperty(int hash, String name) throws FHIRException { 2730 switch (hash) { 2731 case -1618432855: 2732 return getIdentifier(); 2733 case 588504367: 2734 return getTypeCollected(); 2735 case -879411630: 2736 return addPatientPreparation(); 2737 case 276972933: 2738 return getTimeAspectElement(); 2739 case -1741312354: 2740 return addCollection(); 2741 case -1407902581: 2742 return addTypeTested(); 2743 default: 2744 return super.makeProperty(hash, name); 2745 } 2746 2747 } 2748 2749 @Override 2750 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2751 switch (hash) { 2752 case -1618432855: 2753 /* identifier */ return new String[] { "Identifier" }; 2754 case 588504367: 2755 /* typeCollected */ return new String[] { "CodeableConcept" }; 2756 case -879411630: 2757 /* patientPreparation */ return new String[] { "CodeableConcept" }; 2758 case 276972933: 2759 /* timeAspect */ return new String[] { "string" }; 2760 case -1741312354: 2761 /* collection */ return new String[] { "CodeableConcept" }; 2762 case -1407902581: 2763 /* typeTested */ return new String[] {}; 2764 default: 2765 return super.getTypesForProperty(hash, name); 2766 } 2767 2768 } 2769 2770 @Override 2771 public Base addChild(String name) throws FHIRException { 2772 if (name.equals("identifier")) { 2773 this.identifier = new Identifier(); 2774 return this.identifier; 2775 } else if (name.equals("typeCollected")) { 2776 this.typeCollected = new CodeableConcept(); 2777 return this.typeCollected; 2778 } else if (name.equals("patientPreparation")) { 2779 return addPatientPreparation(); 2780 } else if (name.equals("timeAspect")) { 2781 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.timeAspect"); 2782 } else if (name.equals("collection")) { 2783 return addCollection(); 2784 } else if (name.equals("typeTested")) { 2785 return addTypeTested(); 2786 } else 2787 return super.addChild(name); 2788 } 2789 2790 public String fhirType() { 2791 return "SpecimenDefinition"; 2792 2793 } 2794 2795 public SpecimenDefinition copy() { 2796 SpecimenDefinition dst = new SpecimenDefinition(); 2797 copyValues(dst); 2798 return dst; 2799 } 2800 2801 public void copyValues(SpecimenDefinition dst) { 2802 super.copyValues(dst); 2803 dst.identifier = identifier == null ? null : identifier.copy(); 2804 dst.typeCollected = typeCollected == null ? null : typeCollected.copy(); 2805 if (patientPreparation != null) { 2806 dst.patientPreparation = new ArrayList<CodeableConcept>(); 2807 for (CodeableConcept i : patientPreparation) 2808 dst.patientPreparation.add(i.copy()); 2809 } 2810 ; 2811 dst.timeAspect = timeAspect == null ? null : timeAspect.copy(); 2812 if (collection != null) { 2813 dst.collection = new ArrayList<CodeableConcept>(); 2814 for (CodeableConcept i : collection) 2815 dst.collection.add(i.copy()); 2816 } 2817 ; 2818 if (typeTested != null) { 2819 dst.typeTested = new ArrayList<SpecimenDefinitionTypeTestedComponent>(); 2820 for (SpecimenDefinitionTypeTestedComponent i : typeTested) 2821 dst.typeTested.add(i.copy()); 2822 } 2823 ; 2824 } 2825 2826 protected SpecimenDefinition typedCopy() { 2827 return copy(); 2828 } 2829 2830 @Override 2831 public boolean equalsDeep(Base other_) { 2832 if (!super.equalsDeep(other_)) 2833 return false; 2834 if (!(other_ instanceof SpecimenDefinition)) 2835 return false; 2836 SpecimenDefinition o = (SpecimenDefinition) other_; 2837 return compareDeep(identifier, o.identifier, true) && compareDeep(typeCollected, o.typeCollected, true) 2838 && compareDeep(patientPreparation, o.patientPreparation, true) && compareDeep(timeAspect, o.timeAspect, true) 2839 && compareDeep(collection, o.collection, true) && compareDeep(typeTested, o.typeTested, true); 2840 } 2841 2842 @Override 2843 public boolean equalsShallow(Base other_) { 2844 if (!super.equalsShallow(other_)) 2845 return false; 2846 if (!(other_ instanceof SpecimenDefinition)) 2847 return false; 2848 SpecimenDefinition o = (SpecimenDefinition) other_; 2849 return compareValues(timeAspect, o.timeAspect, true); 2850 } 2851 2852 public boolean isEmpty() { 2853 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, typeCollected, patientPreparation, 2854 timeAspect, collection, typeTested); 2855 } 2856 2857 @Override 2858 public ResourceType getResourceType() { 2859 return ResourceType.SpecimenDefinition; 2860 } 2861 2862 /** 2863 * Search parameter: <b>container</b> 2864 * <p> 2865 * Description: <b>The type of specimen conditioned in container expected by the 2866 * lab</b><br> 2867 * Type: <b>token</b><br> 2868 * Path: <b>SpecimenDefinition.typeTested.container.type</b><br> 2869 * </p> 2870 */ 2871 @SearchParamDefinition(name = "container", path = "SpecimenDefinition.typeTested.container.type", description = "The type of specimen conditioned in container expected by the lab", type = "token") 2872 public static final String SP_CONTAINER = "container"; 2873 /** 2874 * <b>Fluent Client</b> search parameter constant for <b>container</b> 2875 * <p> 2876 * Description: <b>The type of specimen conditioned in container expected by the 2877 * lab</b><br> 2878 * Type: <b>token</b><br> 2879 * Path: <b>SpecimenDefinition.typeTested.container.type</b><br> 2880 * </p> 2881 */ 2882 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTAINER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2883 SP_CONTAINER); 2884 2885 /** 2886 * Search parameter: <b>identifier</b> 2887 * <p> 2888 * Description: <b>The unique identifier associated with the specimen</b><br> 2889 * Type: <b>token</b><br> 2890 * Path: <b>SpecimenDefinition.identifier</b><br> 2891 * </p> 2892 */ 2893 @SearchParamDefinition(name = "identifier", path = "SpecimenDefinition.identifier", description = "The unique identifier associated with the specimen", type = "token") 2894 public static final String SP_IDENTIFIER = "identifier"; 2895 /** 2896 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2897 * <p> 2898 * Description: <b>The unique identifier associated with the specimen</b><br> 2899 * Type: <b>token</b><br> 2900 * Path: <b>SpecimenDefinition.identifier</b><br> 2901 * </p> 2902 */ 2903 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2904 SP_IDENTIFIER); 2905 2906 /** 2907 * Search parameter: <b>type</b> 2908 * <p> 2909 * Description: <b>The type of collected specimen</b><br> 2910 * Type: <b>token</b><br> 2911 * Path: <b>SpecimenDefinition.typeCollected</b><br> 2912 * </p> 2913 */ 2914 @SearchParamDefinition(name = "type", path = "SpecimenDefinition.typeCollected", description = "The type of collected specimen", type = "token") 2915 public static final String SP_TYPE = "type"; 2916 /** 2917 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2918 * <p> 2919 * Description: <b>The type of collected specimen</b><br> 2920 * Type: <b>token</b><br> 2921 * Path: <b>SpecimenDefinition.typeCollected</b><br> 2922 * </p> 2923 */ 2924 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2925 SP_TYPE); 2926 2927}