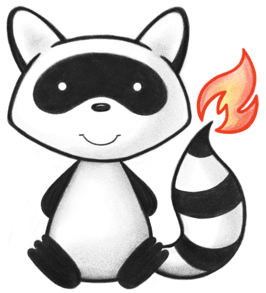
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.FHIRVersion; 040import org.hl7.fhir.r4.model.Enumerations.FHIRVersionEnumFactory; 041import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 042import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.ResourceDef; 050import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 051 052/** 053 * A definition of a FHIR structure. This resource is used to describe the 054 * underlying resources, data types defined in FHIR, and also for describing 055 * extensions and constraints on resources and data types. 056 */ 057@ResourceDef(name = "StructureDefinition", profile = "http://hl7.org/fhir/StructureDefinition/StructureDefinition") 058@ChildOrder(names = { "url", "identifier", "version", "name", "title", "status", "experimental", "date", "publisher", 059 "contact", "description", "useContext", "jurisdiction", "purpose", "copyright", "keyword", "fhirVersion", "mapping", 060 "kind", "abstract", "context", "contextInvariant", "type", "baseDefinition", "derivation", "snapshot", 061 "differential" }) 062public class StructureDefinition extends MetadataResource { 063 064 public enum StructureDefinitionKind { 065 /** 066 * A primitive type that has a value and an extension. These can be used 067 * throughout complex datatype, Resource and extension definitions. Only the 068 * base specification can define primitive types. 069 */ 070 PRIMITIVETYPE, 071 /** 072 * A complex structure that defines a set of data elements that is suitable for 073 * use in 'resources'. The base specification defines a number of complex types, 074 * and other specifications can define additional types. These structures do not 075 * have a maintained identity. 076 */ 077 COMPLEXTYPE, 078 /** 079 * A 'resource' - a directed acyclic graph of elements that aggregrates other 080 * types into an identifiable entity. The base FHIR resources are defined by the 081 * FHIR specification itself but other 'resources' can be defined in additional 082 * specifications (though these will not be recognised as 'resources' by the 083 * FHIR specification (i.e. they do not get end-points etc, or act as the 084 * targets of references in FHIR defined resources - though other specificatiosn 085 * can treat them this way). 086 */ 087 RESOURCE, 088 /** 089 * A pattern or a template that is not intended to be a real resource or complex 090 * type. 091 */ 092 LOGICAL, 093 /** 094 * added to help the parsers with the generic types 095 */ 096 NULL; 097 098 public static StructureDefinitionKind fromCode(String codeString) throws FHIRException { 099 if (codeString == null || "".equals(codeString)) 100 return null; 101 if ("primitive-type".equals(codeString)) 102 return PRIMITIVETYPE; 103 if ("complex-type".equals(codeString)) 104 return COMPLEXTYPE; 105 if ("resource".equals(codeString)) 106 return RESOURCE; 107 if ("logical".equals(codeString)) 108 return LOGICAL; 109 if (Configuration.isAcceptInvalidEnums()) 110 return null; 111 else 112 throw new FHIRException("Unknown StructureDefinitionKind code '" + codeString + "'"); 113 } 114 115 public String toCode() { 116 switch (this) { 117 case PRIMITIVETYPE: 118 return "primitive-type"; 119 case COMPLEXTYPE: 120 return "complex-type"; 121 case RESOURCE: 122 return "resource"; 123 case LOGICAL: 124 return "logical"; 125 case NULL: 126 return null; 127 default: 128 return "?"; 129 } 130 } 131 132 public String getSystem() { 133 switch (this) { 134 case PRIMITIVETYPE: 135 return "http://hl7.org/fhir/structure-definition-kind"; 136 case COMPLEXTYPE: 137 return "http://hl7.org/fhir/structure-definition-kind"; 138 case RESOURCE: 139 return "http://hl7.org/fhir/structure-definition-kind"; 140 case LOGICAL: 141 return "http://hl7.org/fhir/structure-definition-kind"; 142 case NULL: 143 return null; 144 default: 145 return "?"; 146 } 147 } 148 149 public String getDefinition() { 150 switch (this) { 151 case PRIMITIVETYPE: 152 return "A primitive type that has a value and an extension. These can be used throughout complex datatype, Resource and extension definitions. Only the base specification can define primitive types."; 153 case COMPLEXTYPE: 154 return "A complex structure that defines a set of data elements that is suitable for use in 'resources'. The base specification defines a number of complex types, and other specifications can define additional types. These structures do not have a maintained identity."; 155 case RESOURCE: 156 return "A 'resource' - a directed acyclic graph of elements that aggregrates other types into an identifiable entity. The base FHIR resources are defined by the FHIR specification itself but other 'resources' can be defined in additional specifications (though these will not be recognised as 'resources' by the FHIR specification (i.e. they do not get end-points etc, or act as the targets of references in FHIR defined resources - though other specificatiosn can treat them this way)."; 157 case LOGICAL: 158 return "A pattern or a template that is not intended to be a real resource or complex type."; 159 case NULL: 160 return null; 161 default: 162 return "?"; 163 } 164 } 165 166 public String getDisplay() { 167 switch (this) { 168 case PRIMITIVETYPE: 169 return "Primitive Data Type"; 170 case COMPLEXTYPE: 171 return "Complex Data Type"; 172 case RESOURCE: 173 return "Resource"; 174 case LOGICAL: 175 return "Logical"; 176 case NULL: 177 return null; 178 default: 179 return "?"; 180 } 181 } 182 } 183 184 public static class StructureDefinitionKindEnumFactory implements EnumFactory<StructureDefinitionKind> { 185 public StructureDefinitionKind fromCode(String codeString) throws IllegalArgumentException { 186 if (codeString == null || "".equals(codeString)) 187 if (codeString == null || "".equals(codeString)) 188 return null; 189 if ("primitive-type".equals(codeString)) 190 return StructureDefinitionKind.PRIMITIVETYPE; 191 if ("complex-type".equals(codeString)) 192 return StructureDefinitionKind.COMPLEXTYPE; 193 if ("resource".equals(codeString)) 194 return StructureDefinitionKind.RESOURCE; 195 if ("logical".equals(codeString)) 196 return StructureDefinitionKind.LOGICAL; 197 throw new IllegalArgumentException("Unknown StructureDefinitionKind code '" + codeString + "'"); 198 } 199 200 public Enumeration<StructureDefinitionKind> fromType(PrimitiveType<?> code) throws FHIRException { 201 if (code == null) 202 return null; 203 if (code.isEmpty()) 204 return new Enumeration<StructureDefinitionKind>(this, StructureDefinitionKind.NULL, code); 205 String codeString = code.asStringValue(); 206 if (codeString == null || "".equals(codeString)) 207 return new Enumeration<StructureDefinitionKind>(this, StructureDefinitionKind.NULL, code); 208 if ("primitive-type".equals(codeString)) 209 return new Enumeration<StructureDefinitionKind>(this, StructureDefinitionKind.PRIMITIVETYPE, code); 210 if ("complex-type".equals(codeString)) 211 return new Enumeration<StructureDefinitionKind>(this, StructureDefinitionKind.COMPLEXTYPE, code); 212 if ("resource".equals(codeString)) 213 return new Enumeration<StructureDefinitionKind>(this, StructureDefinitionKind.RESOURCE, code); 214 if ("logical".equals(codeString)) 215 return new Enumeration<StructureDefinitionKind>(this, StructureDefinitionKind.LOGICAL, code); 216 throw new FHIRException("Unknown StructureDefinitionKind code '" + codeString + "'"); 217 } 218 219 public String toCode(StructureDefinitionKind code) { 220 if (code == StructureDefinitionKind.NULL) 221 return null; 222 if (code == StructureDefinitionKind.PRIMITIVETYPE) 223 return "primitive-type"; 224 if (code == StructureDefinitionKind.COMPLEXTYPE) 225 return "complex-type"; 226 if (code == StructureDefinitionKind.RESOURCE) 227 return "resource"; 228 if (code == StructureDefinitionKind.LOGICAL) 229 return "logical"; 230 return "?"; 231 } 232 233 public String toSystem(StructureDefinitionKind code) { 234 return code.getSystem(); 235 } 236 } 237 238 public enum ExtensionContextType { 239 /** 240 * The context is all elements that match the FHIRPath query found in the 241 * expression. 242 */ 243 FHIRPATH, 244 /** 245 * The context is any element that has an ElementDefinition.id that matches that 246 * found in the expression. This includes ElementDefinition Ids that have 247 * slicing identifiers. The full path for the element is [url]#[elementid]. If 248 * there is no #, the Element id is one defined in the base specification. 249 */ 250 ELEMENT, 251 /** 252 * The context is a particular extension from a particular StructureDefinition, 253 * and the expression is just a uri that identifies the extension. 254 */ 255 EXTENSION, 256 /** 257 * added to help the parsers with the generic types 258 */ 259 NULL; 260 261 public static ExtensionContextType fromCode(String codeString) throws FHIRException { 262 if (codeString == null || "".equals(codeString)) 263 return null; 264 if ("fhirpath".equals(codeString)) 265 return FHIRPATH; 266 if ("element".equals(codeString)) 267 return ELEMENT; 268 if ("extension".equals(codeString)) 269 return EXTENSION; 270 if (Configuration.isAcceptInvalidEnums()) 271 return null; 272 else 273 throw new FHIRException("Unknown ExtensionContextType code '" + codeString + "'"); 274 } 275 276 public String toCode() { 277 switch (this) { 278 case FHIRPATH: 279 return "fhirpath"; 280 case ELEMENT: 281 return "element"; 282 case EXTENSION: 283 return "extension"; 284 case NULL: 285 return null; 286 default: 287 return "?"; 288 } 289 } 290 291 public String getSystem() { 292 switch (this) { 293 case FHIRPATH: 294 return "http://hl7.org/fhir/extension-context-type"; 295 case ELEMENT: 296 return "http://hl7.org/fhir/extension-context-type"; 297 case EXTENSION: 298 return "http://hl7.org/fhir/extension-context-type"; 299 case NULL: 300 return null; 301 default: 302 return "?"; 303 } 304 } 305 306 public String getDefinition() { 307 switch (this) { 308 case FHIRPATH: 309 return "The context is all elements that match the FHIRPath query found in the expression."; 310 case ELEMENT: 311 return "The context is any element that has an ElementDefinition.id that matches that found in the expression. This includes ElementDefinition Ids that have slicing identifiers. The full path for the element is [url]#[elementid]. If there is no #, the Element id is one defined in the base specification."; 312 case EXTENSION: 313 return "The context is a particular extension from a particular StructureDefinition, and the expression is just a uri that identifies the extension."; 314 case NULL: 315 return null; 316 default: 317 return "?"; 318 } 319 } 320 321 public String getDisplay() { 322 switch (this) { 323 case FHIRPATH: 324 return "FHIRPath"; 325 case ELEMENT: 326 return "Element ID"; 327 case EXTENSION: 328 return "Extension URL"; 329 case NULL: 330 return null; 331 default: 332 return "?"; 333 } 334 } 335 } 336 337 public static class ExtensionContextTypeEnumFactory implements EnumFactory<ExtensionContextType> { 338 public ExtensionContextType fromCode(String codeString) throws IllegalArgumentException { 339 if (codeString == null || "".equals(codeString)) 340 if (codeString == null || "".equals(codeString)) 341 return null; 342 if ("fhirpath".equals(codeString)) 343 return ExtensionContextType.FHIRPATH; 344 if ("element".equals(codeString)) 345 return ExtensionContextType.ELEMENT; 346 if ("extension".equals(codeString)) 347 return ExtensionContextType.EXTENSION; 348 throw new IllegalArgumentException("Unknown ExtensionContextType code '" + codeString + "'"); 349 } 350 351 public Enumeration<ExtensionContextType> fromType(PrimitiveType<?> code) throws FHIRException { 352 if (code == null) 353 return null; 354 if (code.isEmpty()) 355 return new Enumeration<ExtensionContextType>(this, ExtensionContextType.NULL, code); 356 String codeString = code.asStringValue(); 357 if (codeString == null || "".equals(codeString)) 358 return new Enumeration<ExtensionContextType>(this, ExtensionContextType.NULL, code); 359 if ("fhirpath".equals(codeString)) 360 return new Enumeration<ExtensionContextType>(this, ExtensionContextType.FHIRPATH, code); 361 if ("element".equals(codeString)) 362 return new Enumeration<ExtensionContextType>(this, ExtensionContextType.ELEMENT, code); 363 if ("extension".equals(codeString)) 364 return new Enumeration<ExtensionContextType>(this, ExtensionContextType.EXTENSION, code); 365 throw new FHIRException("Unknown ExtensionContextType code '" + codeString + "'"); 366 } 367 368 public String toCode(ExtensionContextType code) { 369 if (code == ExtensionContextType.NULL) 370 return null; 371 if (code == ExtensionContextType.FHIRPATH) 372 return "fhirpath"; 373 if (code == ExtensionContextType.ELEMENT) 374 return "element"; 375 if (code == ExtensionContextType.EXTENSION) 376 return "extension"; 377 return "?"; 378 } 379 380 public String toSystem(ExtensionContextType code) { 381 return code.getSystem(); 382 } 383 } 384 385 public enum TypeDerivationRule { 386 /** 387 * This definition defines a new type that adds additional elements to the base 388 * type. 389 */ 390 SPECIALIZATION, 391 /** 392 * This definition adds additional rules to an existing concrete type. 393 */ 394 CONSTRAINT, 395 /** 396 * added to help the parsers with the generic types 397 */ 398 NULL; 399 400 public static TypeDerivationRule fromCode(String codeString) throws FHIRException { 401 if (codeString == null || "".equals(codeString)) 402 return null; 403 if ("specialization".equals(codeString)) 404 return SPECIALIZATION; 405 if ("constraint".equals(codeString)) 406 return CONSTRAINT; 407 if (Configuration.isAcceptInvalidEnums()) 408 return null; 409 else 410 throw new FHIRException("Unknown TypeDerivationRule code '" + codeString + "'"); 411 } 412 413 public String toCode() { 414 switch (this) { 415 case SPECIALIZATION: 416 return "specialization"; 417 case CONSTRAINT: 418 return "constraint"; 419 case NULL: 420 return null; 421 default: 422 return "?"; 423 } 424 } 425 426 public String getSystem() { 427 switch (this) { 428 case SPECIALIZATION: 429 return "http://hl7.org/fhir/type-derivation-rule"; 430 case CONSTRAINT: 431 return "http://hl7.org/fhir/type-derivation-rule"; 432 case NULL: 433 return null; 434 default: 435 return "?"; 436 } 437 } 438 439 public String getDefinition() { 440 switch (this) { 441 case SPECIALIZATION: 442 return "This definition defines a new type that adds additional elements to the base type."; 443 case CONSTRAINT: 444 return "This definition adds additional rules to an existing concrete type."; 445 case NULL: 446 return null; 447 default: 448 return "?"; 449 } 450 } 451 452 public String getDisplay() { 453 switch (this) { 454 case SPECIALIZATION: 455 return "Specialization"; 456 case CONSTRAINT: 457 return "Constraint"; 458 case NULL: 459 return null; 460 default: 461 return "?"; 462 } 463 } 464 } 465 466 public static class TypeDerivationRuleEnumFactory implements EnumFactory<TypeDerivationRule> { 467 public TypeDerivationRule fromCode(String codeString) throws IllegalArgumentException { 468 if (codeString == null || "".equals(codeString)) 469 if (codeString == null || "".equals(codeString)) 470 return null; 471 if ("specialization".equals(codeString)) 472 return TypeDerivationRule.SPECIALIZATION; 473 if ("constraint".equals(codeString)) 474 return TypeDerivationRule.CONSTRAINT; 475 throw new IllegalArgumentException("Unknown TypeDerivationRule code '" + codeString + "'"); 476 } 477 478 public Enumeration<TypeDerivationRule> fromType(PrimitiveType<?> code) throws FHIRException { 479 if (code == null) 480 return null; 481 if (code.isEmpty()) 482 return new Enumeration<TypeDerivationRule>(this, TypeDerivationRule.NULL, code); 483 String codeString = code.asStringValue(); 484 if (codeString == null || "".equals(codeString)) 485 return new Enumeration<TypeDerivationRule>(this, TypeDerivationRule.NULL, code); 486 if ("specialization".equals(codeString)) 487 return new Enumeration<TypeDerivationRule>(this, TypeDerivationRule.SPECIALIZATION, code); 488 if ("constraint".equals(codeString)) 489 return new Enumeration<TypeDerivationRule>(this, TypeDerivationRule.CONSTRAINT, code); 490 throw new FHIRException("Unknown TypeDerivationRule code '" + codeString + "'"); 491 } 492 493 public String toCode(TypeDerivationRule code) { 494 if (code == TypeDerivationRule.NULL) 495 return null; 496 if (code == TypeDerivationRule.SPECIALIZATION) 497 return "specialization"; 498 if (code == TypeDerivationRule.CONSTRAINT) 499 return "constraint"; 500 return "?"; 501 } 502 503 public String toSystem(TypeDerivationRule code) { 504 return code.getSystem(); 505 } 506 } 507 508 @Block() 509 public static class StructureDefinitionMappingComponent extends BackboneElement implements IBaseBackboneElement { 510 /** 511 * An Internal id that is used to identify this mapping set when specific 512 * mappings are made. 513 */ 514 @Child(name = "identity", type = { IdType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 515 @Description(shortDefinition = "Internal id when this mapping is used", formalDefinition = "An Internal id that is used to identify this mapping set when specific mappings are made.") 516 protected IdType identity; 517 518 /** 519 * An absolute URI that identifies the specification that this mapping is 520 * expressed to. 521 */ 522 @Child(name = "uri", type = { UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 523 @Description(shortDefinition = "Identifies what this mapping refers to", formalDefinition = "An absolute URI that identifies the specification that this mapping is expressed to.") 524 protected UriType uri; 525 526 /** 527 * A name for the specification that is being mapped to. 528 */ 529 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 530 @Description(shortDefinition = "Names what this mapping refers to", formalDefinition = "A name for the specification that is being mapped to.") 531 protected StringType name; 532 533 /** 534 * Comments about this mapping, including version notes, issues, scope 535 * limitations, and other important notes for usage. 536 */ 537 @Child(name = "comment", type = { 538 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 539 @Description(shortDefinition = "Versions, Issues, Scope limitations etc.", formalDefinition = "Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage.") 540 protected StringType comment; 541 542 private static final long serialVersionUID = 9610265L; 543 544 /** 545 * Constructor 546 */ 547 public StructureDefinitionMappingComponent() { 548 super(); 549 } 550 551 /** 552 * Constructor 553 */ 554 public StructureDefinitionMappingComponent(IdType identity) { 555 super(); 556 this.identity = identity; 557 } 558 559 /** 560 * @return {@link #identity} (An Internal id that is used to identify this 561 * mapping set when specific mappings are made.). This is the underlying 562 * object with id, value and extensions. The accessor "getIdentity" 563 * gives direct access to the value 564 */ 565 public IdType getIdentityElement() { 566 if (this.identity == null) 567 if (Configuration.errorOnAutoCreate()) 568 throw new Error("Attempt to auto-create StructureDefinitionMappingComponent.identity"); 569 else if (Configuration.doAutoCreate()) 570 this.identity = new IdType(); // bb 571 return this.identity; 572 } 573 574 public boolean hasIdentityElement() { 575 return this.identity != null && !this.identity.isEmpty(); 576 } 577 578 public boolean hasIdentity() { 579 return this.identity != null && !this.identity.isEmpty(); 580 } 581 582 /** 583 * @param value {@link #identity} (An Internal id that is used to identify this 584 * mapping set when specific mappings are made.). This is the 585 * underlying object with id, value and extensions. The accessor 586 * "getIdentity" gives direct access to the value 587 */ 588 public StructureDefinitionMappingComponent setIdentityElement(IdType value) { 589 this.identity = value; 590 return this; 591 } 592 593 /** 594 * @return An Internal id that is used to identify this mapping set when 595 * specific mappings are made. 596 */ 597 public String getIdentity() { 598 return this.identity == null ? null : this.identity.getValue(); 599 } 600 601 /** 602 * @param value An Internal id that is used to identify this mapping set when 603 * specific mappings are made. 604 */ 605 public StructureDefinitionMappingComponent setIdentity(String value) { 606 if (this.identity == null) 607 this.identity = new IdType(); 608 this.identity.setValue(value); 609 return this; 610 } 611 612 /** 613 * @return {@link #uri} (An absolute URI that identifies the specification that 614 * this mapping is expressed to.). This is the underlying object with 615 * id, value and extensions. The accessor "getUri" gives direct access 616 * to the value 617 */ 618 public UriType getUriElement() { 619 if (this.uri == null) 620 if (Configuration.errorOnAutoCreate()) 621 throw new Error("Attempt to auto-create StructureDefinitionMappingComponent.uri"); 622 else if (Configuration.doAutoCreate()) 623 this.uri = new UriType(); // bb 624 return this.uri; 625 } 626 627 public boolean hasUriElement() { 628 return this.uri != null && !this.uri.isEmpty(); 629 } 630 631 public boolean hasUri() { 632 return this.uri != null && !this.uri.isEmpty(); 633 } 634 635 /** 636 * @param value {@link #uri} (An absolute URI that identifies the specification 637 * that this mapping is expressed to.). This is the underlying 638 * object with id, value and extensions. The accessor "getUri" 639 * gives direct access to the value 640 */ 641 public StructureDefinitionMappingComponent setUriElement(UriType value) { 642 this.uri = value; 643 return this; 644 } 645 646 /** 647 * @return An absolute URI that identifies the specification that this mapping 648 * is expressed to. 649 */ 650 public String getUri() { 651 return this.uri == null ? null : this.uri.getValue(); 652 } 653 654 /** 655 * @param value An absolute URI that identifies the specification that this 656 * mapping is expressed to. 657 */ 658 public StructureDefinitionMappingComponent setUri(String value) { 659 if (Utilities.noString(value)) 660 this.uri = null; 661 else { 662 if (this.uri == null) 663 this.uri = new UriType(); 664 this.uri.setValue(value); 665 } 666 return this; 667 } 668 669 /** 670 * @return {@link #name} (A name for the specification that is being mapped 671 * to.). This is the underlying object with id, value and extensions. 672 * The accessor "getName" gives direct access to the value 673 */ 674 public StringType getNameElement() { 675 if (this.name == null) 676 if (Configuration.errorOnAutoCreate()) 677 throw new Error("Attempt to auto-create StructureDefinitionMappingComponent.name"); 678 else if (Configuration.doAutoCreate()) 679 this.name = new StringType(); // bb 680 return this.name; 681 } 682 683 public boolean hasNameElement() { 684 return this.name != null && !this.name.isEmpty(); 685 } 686 687 public boolean hasName() { 688 return this.name != null && !this.name.isEmpty(); 689 } 690 691 /** 692 * @param value {@link #name} (A name for the specification that is being mapped 693 * to.). This is the underlying object with id, value and 694 * extensions. The accessor "getName" gives direct access to the 695 * value 696 */ 697 public StructureDefinitionMappingComponent setNameElement(StringType value) { 698 this.name = value; 699 return this; 700 } 701 702 /** 703 * @return A name for the specification that is being mapped to. 704 */ 705 public String getName() { 706 return this.name == null ? null : this.name.getValue(); 707 } 708 709 /** 710 * @param value A name for the specification that is being mapped to. 711 */ 712 public StructureDefinitionMappingComponent setName(String value) { 713 if (Utilities.noString(value)) 714 this.name = null; 715 else { 716 if (this.name == null) 717 this.name = new StringType(); 718 this.name.setValue(value); 719 } 720 return this; 721 } 722 723 /** 724 * @return {@link #comment} (Comments about this mapping, including version 725 * notes, issues, scope limitations, and other important notes for 726 * usage.). This is the underlying object with id, value and extensions. 727 * The accessor "getComment" gives direct access to the value 728 */ 729 public StringType getCommentElement() { 730 if (this.comment == null) 731 if (Configuration.errorOnAutoCreate()) 732 throw new Error("Attempt to auto-create StructureDefinitionMappingComponent.comment"); 733 else if (Configuration.doAutoCreate()) 734 this.comment = new StringType(); // bb 735 return this.comment; 736 } 737 738 public boolean hasCommentElement() { 739 return this.comment != null && !this.comment.isEmpty(); 740 } 741 742 public boolean hasComment() { 743 return this.comment != null && !this.comment.isEmpty(); 744 } 745 746 /** 747 * @param value {@link #comment} (Comments about this mapping, including version 748 * notes, issues, scope limitations, and other important notes for 749 * usage.). This is the underlying object with id, value and 750 * extensions. The accessor "getComment" gives direct access to the 751 * value 752 */ 753 public StructureDefinitionMappingComponent setCommentElement(StringType value) { 754 this.comment = value; 755 return this; 756 } 757 758 /** 759 * @return Comments about this mapping, including version notes, issues, scope 760 * limitations, and other important notes for usage. 761 */ 762 public String getComment() { 763 return this.comment == null ? null : this.comment.getValue(); 764 } 765 766 /** 767 * @param value Comments about this mapping, including version notes, issues, 768 * scope limitations, and other important notes for usage. 769 */ 770 public StructureDefinitionMappingComponent setComment(String value) { 771 if (Utilities.noString(value)) 772 this.comment = null; 773 else { 774 if (this.comment == null) 775 this.comment = new StringType(); 776 this.comment.setValue(value); 777 } 778 return this; 779 } 780 781 protected void listChildren(List<Property> children) { 782 super.listChildren(children); 783 children.add(new Property("identity", "id", 784 "An Internal id that is used to identify this mapping set when specific mappings are made.", 0, 1, identity)); 785 children.add(new Property("uri", "uri", 786 "An absolute URI that identifies the specification that this mapping is expressed to.", 0, 1, uri)); 787 children.add(new Property("name", "string", "A name for the specification that is being mapped to.", 0, 1, name)); 788 children.add(new Property("comment", "string", 789 "Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage.", 790 0, 1, comment)); 791 } 792 793 @Override 794 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 795 switch (_hash) { 796 case -135761730: 797 /* identity */ return new Property("identity", "id", 798 "An Internal id that is used to identify this mapping set when specific mappings are made.", 0, 1, 799 identity); 800 case 116076: 801 /* uri */ return new Property("uri", "uri", 802 "An absolute URI that identifies the specification that this mapping is expressed to.", 0, 1, uri); 803 case 3373707: 804 /* name */ return new Property("name", "string", "A name for the specification that is being mapped to.", 0, 1, 805 name); 806 case 950398559: 807 /* comment */ return new Property("comment", "string", 808 "Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage.", 809 0, 1, comment); 810 default: 811 return super.getNamedProperty(_hash, _name, _checkValid); 812 } 813 814 } 815 816 @Override 817 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 818 switch (hash) { 819 case -135761730: 820 /* identity */ return this.identity == null ? new Base[0] : new Base[] { this.identity }; // IdType 821 case 116076: 822 /* uri */ return this.uri == null ? new Base[0] : new Base[] { this.uri }; // UriType 823 case 3373707: 824 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 825 case 950398559: 826 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // StringType 827 default: 828 return super.getProperty(hash, name, checkValid); 829 } 830 831 } 832 833 @Override 834 public Base setProperty(int hash, String name, Base value) throws FHIRException { 835 switch (hash) { 836 case -135761730: // identity 837 this.identity = castToId(value); // IdType 838 return value; 839 case 116076: // uri 840 this.uri = castToUri(value); // UriType 841 return value; 842 case 3373707: // name 843 this.name = castToString(value); // StringType 844 return value; 845 case 950398559: // comment 846 this.comment = castToString(value); // StringType 847 return value; 848 default: 849 return super.setProperty(hash, name, value); 850 } 851 852 } 853 854 @Override 855 public Base setProperty(String name, Base value) throws FHIRException { 856 if (name.equals("identity")) { 857 this.identity = castToId(value); // IdType 858 } else if (name.equals("uri")) { 859 this.uri = castToUri(value); // UriType 860 } else if (name.equals("name")) { 861 this.name = castToString(value); // StringType 862 } else if (name.equals("comment")) { 863 this.comment = castToString(value); // StringType 864 } else 865 return super.setProperty(name, value); 866 return value; 867 } 868 869 @Override 870 public void removeChild(String name, Base value) throws FHIRException { 871 if (name.equals("identity")) { 872 this.identity = null; 873 } else if (name.equals("uri")) { 874 this.uri = null; 875 } else if (name.equals("name")) { 876 this.name = null; 877 } else if (name.equals("comment")) { 878 this.comment = null; 879 } else 880 super.removeChild(name, value); 881 882 } 883 884 @Override 885 public Base makeProperty(int hash, String name) throws FHIRException { 886 switch (hash) { 887 case -135761730: 888 return getIdentityElement(); 889 case 116076: 890 return getUriElement(); 891 case 3373707: 892 return getNameElement(); 893 case 950398559: 894 return getCommentElement(); 895 default: 896 return super.makeProperty(hash, name); 897 } 898 899 } 900 901 @Override 902 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 903 switch (hash) { 904 case -135761730: 905 /* identity */ return new String[] { "id" }; 906 case 116076: 907 /* uri */ return new String[] { "uri" }; 908 case 3373707: 909 /* name */ return new String[] { "string" }; 910 case 950398559: 911 /* comment */ return new String[] { "string" }; 912 default: 913 return super.getTypesForProperty(hash, name); 914 } 915 916 } 917 918 @Override 919 public Base addChild(String name) throws FHIRException { 920 if (name.equals("identity")) { 921 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.identity"); 922 } else if (name.equals("uri")) { 923 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.uri"); 924 } else if (name.equals("name")) { 925 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.name"); 926 } else if (name.equals("comment")) { 927 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.comment"); 928 } else 929 return super.addChild(name); 930 } 931 932 public StructureDefinitionMappingComponent copy() { 933 StructureDefinitionMappingComponent dst = new StructureDefinitionMappingComponent(); 934 copyValues(dst); 935 return dst; 936 } 937 938 public void copyValues(StructureDefinitionMappingComponent dst) { 939 super.copyValues(dst); 940 dst.identity = identity == null ? null : identity.copy(); 941 dst.uri = uri == null ? null : uri.copy(); 942 dst.name = name == null ? null : name.copy(); 943 dst.comment = comment == null ? null : comment.copy(); 944 } 945 946 @Override 947 public boolean equalsDeep(Base other_) { 948 if (!super.equalsDeep(other_)) 949 return false; 950 if (!(other_ instanceof StructureDefinitionMappingComponent)) 951 return false; 952 StructureDefinitionMappingComponent o = (StructureDefinitionMappingComponent) other_; 953 return compareDeep(identity, o.identity, true) && compareDeep(uri, o.uri, true) && compareDeep(name, o.name, true) 954 && compareDeep(comment, o.comment, true); 955 } 956 957 @Override 958 public boolean equalsShallow(Base other_) { 959 if (!super.equalsShallow(other_)) 960 return false; 961 if (!(other_ instanceof StructureDefinitionMappingComponent)) 962 return false; 963 StructureDefinitionMappingComponent o = (StructureDefinitionMappingComponent) other_; 964 return compareValues(identity, o.identity, true) && compareValues(uri, o.uri, true) 965 && compareValues(name, o.name, true) && compareValues(comment, o.comment, true); 966 } 967 968 public boolean isEmpty() { 969 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identity, uri, name, comment); 970 } 971 972 public String fhirType() { 973 return "StructureDefinition.mapping"; 974 975 } 976 977 } 978 979 @Block() 980 public static class StructureDefinitionContextComponent extends BackboneElement implements IBaseBackboneElement { 981 /** 982 * Defines how to interpret the expression that defines what the context of the 983 * extension is. 984 */ 985 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 986 @Description(shortDefinition = "fhirpath | element | extension", formalDefinition = "Defines how to interpret the expression that defines what the context of the extension is.") 987 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/extension-context-type") 988 protected Enumeration<ExtensionContextType> type; 989 990 /** 991 * An expression that defines where an extension can be used in resources. 992 */ 993 @Child(name = "expression", type = { 994 StringType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 995 @Description(shortDefinition = "Where the extension can be used in instances", formalDefinition = "An expression that defines where an extension can be used in resources.") 996 protected StringType expression; 997 998 private static final long serialVersionUID = 1958074856L; 999 1000 /** 1001 * Constructor 1002 */ 1003 public StructureDefinitionContextComponent() { 1004 super(); 1005 } 1006 1007 /** 1008 * Constructor 1009 */ 1010 public StructureDefinitionContextComponent(Enumeration<ExtensionContextType> type, StringType expression) { 1011 super(); 1012 this.type = type; 1013 this.expression = expression; 1014 } 1015 1016 /** 1017 * @return {@link #type} (Defines how to interpret the expression that defines 1018 * what the context of the extension is.). This is the underlying object 1019 * with id, value and extensions. The accessor "getType" gives direct 1020 * access to the value 1021 */ 1022 public Enumeration<ExtensionContextType> getTypeElement() { 1023 if (this.type == null) 1024 if (Configuration.errorOnAutoCreate()) 1025 throw new Error("Attempt to auto-create StructureDefinitionContextComponent.type"); 1026 else if (Configuration.doAutoCreate()) 1027 this.type = new Enumeration<ExtensionContextType>(new ExtensionContextTypeEnumFactory()); // bb 1028 return this.type; 1029 } 1030 1031 public boolean hasTypeElement() { 1032 return this.type != null && !this.type.isEmpty(); 1033 } 1034 1035 public boolean hasType() { 1036 return this.type != null && !this.type.isEmpty(); 1037 } 1038 1039 /** 1040 * @param value {@link #type} (Defines how to interpret the expression that 1041 * defines what the context of the extension is.). This is the 1042 * underlying object with id, value and extensions. The accessor 1043 * "getType" gives direct access to the value 1044 */ 1045 public StructureDefinitionContextComponent setTypeElement(Enumeration<ExtensionContextType> value) { 1046 this.type = value; 1047 return this; 1048 } 1049 1050 /** 1051 * @return Defines how to interpret the expression that defines what the context 1052 * of the extension is. 1053 */ 1054 public ExtensionContextType getType() { 1055 return this.type == null ? null : this.type.getValue(); 1056 } 1057 1058 /** 1059 * @param value Defines how to interpret the expression that defines what the 1060 * context of the extension is. 1061 */ 1062 public StructureDefinitionContextComponent setType(ExtensionContextType value) { 1063 if (this.type == null) 1064 this.type = new Enumeration<ExtensionContextType>(new ExtensionContextTypeEnumFactory()); 1065 this.type.setValue(value); 1066 return this; 1067 } 1068 1069 /** 1070 * @return {@link #expression} (An expression that defines where an extension 1071 * can be used in resources.). This is the underlying object with id, 1072 * value and extensions. The accessor "getExpression" gives direct 1073 * access to the value 1074 */ 1075 public StringType getExpressionElement() { 1076 if (this.expression == null) 1077 if (Configuration.errorOnAutoCreate()) 1078 throw new Error("Attempt to auto-create StructureDefinitionContextComponent.expression"); 1079 else if (Configuration.doAutoCreate()) 1080 this.expression = new StringType(); // bb 1081 return this.expression; 1082 } 1083 1084 public boolean hasExpressionElement() { 1085 return this.expression != null && !this.expression.isEmpty(); 1086 } 1087 1088 public boolean hasExpression() { 1089 return this.expression != null && !this.expression.isEmpty(); 1090 } 1091 1092 /** 1093 * @param value {@link #expression} (An expression that defines where an 1094 * extension can be used in resources.). This is the underlying 1095 * object with id, value and extensions. The accessor 1096 * "getExpression" gives direct access to the value 1097 */ 1098 public StructureDefinitionContextComponent setExpressionElement(StringType value) { 1099 this.expression = value; 1100 return this; 1101 } 1102 1103 /** 1104 * @return An expression that defines where an extension can be used in 1105 * resources. 1106 */ 1107 public String getExpression() { 1108 return this.expression == null ? null : this.expression.getValue(); 1109 } 1110 1111 /** 1112 * @param value An expression that defines where an extension can be used in 1113 * resources. 1114 */ 1115 public StructureDefinitionContextComponent setExpression(String value) { 1116 if (this.expression == null) 1117 this.expression = new StringType(); 1118 this.expression.setValue(value); 1119 return this; 1120 } 1121 1122 protected void listChildren(List<Property> children) { 1123 super.listChildren(children); 1124 children.add(new Property("type", "code", 1125 "Defines how to interpret the expression that defines what the context of the extension is.", 0, 1, type)); 1126 children.add(new Property("expression", "string", 1127 "An expression that defines where an extension can be used in resources.", 0, 1, expression)); 1128 } 1129 1130 @Override 1131 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1132 switch (_hash) { 1133 case 3575610: 1134 /* type */ return new Property("type", "code", 1135 "Defines how to interpret the expression that defines what the context of the extension is.", 0, 1, type); 1136 case -1795452264: 1137 /* expression */ return new Property("expression", "string", 1138 "An expression that defines where an extension can be used in resources.", 0, 1, expression); 1139 default: 1140 return super.getNamedProperty(_hash, _name, _checkValid); 1141 } 1142 1143 } 1144 1145 @Override 1146 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1147 switch (hash) { 1148 case 3575610: 1149 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<ExtensionContextType> 1150 case -1795452264: 1151 /* expression */ return this.expression == null ? new Base[0] : new Base[] { this.expression }; // StringType 1152 default: 1153 return super.getProperty(hash, name, checkValid); 1154 } 1155 1156 } 1157 1158 @Override 1159 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1160 switch (hash) { 1161 case 3575610: // type 1162 value = new ExtensionContextTypeEnumFactory().fromType(castToCode(value)); 1163 this.type = (Enumeration) value; // Enumeration<ExtensionContextType> 1164 return value; 1165 case -1795452264: // expression 1166 this.expression = castToString(value); // StringType 1167 return value; 1168 default: 1169 return super.setProperty(hash, name, value); 1170 } 1171 1172 } 1173 1174 @Override 1175 public Base setProperty(String name, Base value) throws FHIRException { 1176 if (name.equals("type")) { 1177 value = new ExtensionContextTypeEnumFactory().fromType(castToCode(value)); 1178 this.type = (Enumeration) value; // Enumeration<ExtensionContextType> 1179 } else if (name.equals("expression")) { 1180 this.expression = castToString(value); // StringType 1181 } else 1182 return super.setProperty(name, value); 1183 return value; 1184 } 1185 1186 @Override 1187 public void removeChild(String name, Base value) throws FHIRException { 1188 if (name.equals("type")) { 1189 this.type = null; 1190 } else if (name.equals("expression")) { 1191 this.expression = null; 1192 } else 1193 super.removeChild(name, value); 1194 1195 } 1196 1197 @Override 1198 public Base makeProperty(int hash, String name) throws FHIRException { 1199 switch (hash) { 1200 case 3575610: 1201 return getTypeElement(); 1202 case -1795452264: 1203 return getExpressionElement(); 1204 default: 1205 return super.makeProperty(hash, name); 1206 } 1207 1208 } 1209 1210 @Override 1211 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1212 switch (hash) { 1213 case 3575610: 1214 /* type */ return new String[] { "code" }; 1215 case -1795452264: 1216 /* expression */ return new String[] { "string" }; 1217 default: 1218 return super.getTypesForProperty(hash, name); 1219 } 1220 1221 } 1222 1223 @Override 1224 public Base addChild(String name) throws FHIRException { 1225 if (name.equals("type")) { 1226 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.type"); 1227 } else if (name.equals("expression")) { 1228 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.expression"); 1229 } else 1230 return super.addChild(name); 1231 } 1232 1233 public StructureDefinitionContextComponent copy() { 1234 StructureDefinitionContextComponent dst = new StructureDefinitionContextComponent(); 1235 copyValues(dst); 1236 return dst; 1237 } 1238 1239 public void copyValues(StructureDefinitionContextComponent dst) { 1240 super.copyValues(dst); 1241 dst.type = type == null ? null : type.copy(); 1242 dst.expression = expression == null ? null : expression.copy(); 1243 } 1244 1245 @Override 1246 public boolean equalsDeep(Base other_) { 1247 if (!super.equalsDeep(other_)) 1248 return false; 1249 if (!(other_ instanceof StructureDefinitionContextComponent)) 1250 return false; 1251 StructureDefinitionContextComponent o = (StructureDefinitionContextComponent) other_; 1252 return compareDeep(type, o.type, true) && compareDeep(expression, o.expression, true); 1253 } 1254 1255 @Override 1256 public boolean equalsShallow(Base other_) { 1257 if (!super.equalsShallow(other_)) 1258 return false; 1259 if (!(other_ instanceof StructureDefinitionContextComponent)) 1260 return false; 1261 StructureDefinitionContextComponent o = (StructureDefinitionContextComponent) other_; 1262 return compareValues(type, o.type, true) && compareValues(expression, o.expression, true); 1263 } 1264 1265 public boolean isEmpty() { 1266 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, expression); 1267 } 1268 1269 public String fhirType() { 1270 return "StructureDefinition.context"; 1271 1272 } 1273 1274 } 1275 1276 @Block() 1277 public static class StructureDefinitionSnapshotComponent extends BackboneElement implements IBaseBackboneElement { 1278 /** 1279 * Captures constraints on each element within the resource. 1280 */ 1281 @Child(name = "element", type = { 1282 ElementDefinition.class }, order = 1, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1283 @Description(shortDefinition = "Definition of elements in the resource (if no StructureDefinition)", formalDefinition = "Captures constraints on each element within the resource.") 1284 protected List<ElementDefinition> element; 1285 1286 private static final long serialVersionUID = 53896641L; 1287 1288 /** 1289 * Constructor 1290 */ 1291 public StructureDefinitionSnapshotComponent() { 1292 super(); 1293 } 1294 1295 /** 1296 * @return {@link #element} (Captures constraints on each element within the 1297 * resource.) 1298 */ 1299 public List<ElementDefinition> getElement() { 1300 if (this.element == null) 1301 this.element = new ArrayList<ElementDefinition>(); 1302 return this.element; 1303 } 1304 1305 /** 1306 * @return Returns a reference to <code>this</code> for easy method chaining 1307 */ 1308 public StructureDefinitionSnapshotComponent setElement(List<ElementDefinition> theElement) { 1309 this.element = theElement; 1310 return this; 1311 } 1312 1313 public boolean hasElement() { 1314 if (this.element == null) 1315 return false; 1316 for (ElementDefinition item : this.element) 1317 if (!item.isEmpty()) 1318 return true; 1319 return false; 1320 } 1321 1322 public ElementDefinition addElement() { // 3 1323 ElementDefinition t = new ElementDefinition(); 1324 if (this.element == null) 1325 this.element = new ArrayList<ElementDefinition>(); 1326 this.element.add(t); 1327 return t; 1328 } 1329 1330 public StructureDefinitionSnapshotComponent addElement(ElementDefinition t) { // 3 1331 if (t == null) 1332 return this; 1333 if (this.element == null) 1334 this.element = new ArrayList<ElementDefinition>(); 1335 this.element.add(t); 1336 return this; 1337 } 1338 1339 /** 1340 * @return The first repetition of repeating field {@link #element}, creating it 1341 * if it does not already exist 1342 */ 1343 public ElementDefinition getElementFirstRep() { 1344 if (getElement().isEmpty()) { 1345 addElement(); 1346 } 1347 return getElement().get(0); 1348 } 1349 1350 protected void listChildren(List<Property> children) { 1351 super.listChildren(children); 1352 children.add(new Property("element", "ElementDefinition", 1353 "Captures constraints on each element within the resource.", 0, java.lang.Integer.MAX_VALUE, element)); 1354 } 1355 1356 @Override 1357 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1358 switch (_hash) { 1359 case -1662836996: 1360 /* element */ return new Property("element", "ElementDefinition", 1361 "Captures constraints on each element within the resource.", 0, java.lang.Integer.MAX_VALUE, element); 1362 default: 1363 return super.getNamedProperty(_hash, _name, _checkValid); 1364 } 1365 1366 } 1367 1368 @Override 1369 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1370 switch (hash) { 1371 case -1662836996: 1372 /* element */ return this.element == null ? new Base[0] : this.element.toArray(new Base[this.element.size()]); // ElementDefinition 1373 default: 1374 return super.getProperty(hash, name, checkValid); 1375 } 1376 1377 } 1378 1379 @Override 1380 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1381 switch (hash) { 1382 case -1662836996: // element 1383 this.getElement().add(castToElementDefinition(value)); // ElementDefinition 1384 return value; 1385 default: 1386 return super.setProperty(hash, name, value); 1387 } 1388 1389 } 1390 1391 @Override 1392 public Base setProperty(String name, Base value) throws FHIRException { 1393 if (name.equals("element")) { 1394 this.getElement().add(castToElementDefinition(value)); 1395 } else 1396 return super.setProperty(name, value); 1397 return value; 1398 } 1399 1400 @Override 1401 public void removeChild(String name, Base value) throws FHIRException { 1402 if (name.equals("element")) { 1403 this.getElement().remove(castToElementDefinition(value)); 1404 } else 1405 super.removeChild(name, value); 1406 1407 } 1408 1409 @Override 1410 public Base makeProperty(int hash, String name) throws FHIRException { 1411 switch (hash) { 1412 case -1662836996: 1413 return addElement(); 1414 default: 1415 return super.makeProperty(hash, name); 1416 } 1417 1418 } 1419 1420 @Override 1421 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1422 switch (hash) { 1423 case -1662836996: 1424 /* element */ return new String[] { "ElementDefinition" }; 1425 default: 1426 return super.getTypesForProperty(hash, name); 1427 } 1428 1429 } 1430 1431 @Override 1432 public Base addChild(String name) throws FHIRException { 1433 if (name.equals("element")) { 1434 return addElement(); 1435 } else 1436 return super.addChild(name); 1437 } 1438 1439 public StructureDefinitionSnapshotComponent copy() { 1440 StructureDefinitionSnapshotComponent dst = new StructureDefinitionSnapshotComponent(); 1441 copyValues(dst); 1442 return dst; 1443 } 1444 1445 public void copyValues(StructureDefinitionSnapshotComponent dst) { 1446 super.copyValues(dst); 1447 if (element != null) { 1448 dst.element = new ArrayList<ElementDefinition>(); 1449 for (ElementDefinition i : element) 1450 dst.element.add(i.copy()); 1451 } 1452 ; 1453 } 1454 1455 @Override 1456 public boolean equalsDeep(Base other_) { 1457 if (!super.equalsDeep(other_)) 1458 return false; 1459 if (!(other_ instanceof StructureDefinitionSnapshotComponent)) 1460 return false; 1461 StructureDefinitionSnapshotComponent o = (StructureDefinitionSnapshotComponent) other_; 1462 return compareDeep(element, o.element, true); 1463 } 1464 1465 @Override 1466 public boolean equalsShallow(Base other_) { 1467 if (!super.equalsShallow(other_)) 1468 return false; 1469 if (!(other_ instanceof StructureDefinitionSnapshotComponent)) 1470 return false; 1471 StructureDefinitionSnapshotComponent o = (StructureDefinitionSnapshotComponent) other_; 1472 return true; 1473 } 1474 1475 public boolean isEmpty() { 1476 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(element); 1477 } 1478 1479 public String fhirType() { 1480 return "StructureDefinition.snapshot"; 1481 1482 } 1483 1484 public ElementDefinition getElementByPath(String path) { 1485 if (path == null) { 1486 return null; 1487 } 1488 for (ElementDefinition ed : getElement()) { 1489 if (path.equals(ed.getPath()) || (path+"[x]").equals(ed.getPath())) { 1490 return ed; 1491 } 1492 } 1493 return null; 1494 } 1495 1496 1497 public ElementDefinition getElementById(String id) { 1498 if (id == null) { 1499 return null; 1500 } 1501 for (ElementDefinition ed : getElement()) { 1502 if (id.equals(ed.getId())) { 1503 return ed; 1504 } 1505 } 1506 return null; 1507 } 1508 1509 } 1510 1511 @Block() 1512 public static class StructureDefinitionDifferentialComponent extends BackboneElement implements IBaseBackboneElement { 1513 /** 1514 * Captures constraints on each element within the resource. 1515 */ 1516 @Child(name = "element", type = { 1517 ElementDefinition.class }, order = 1, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1518 @Description(shortDefinition = "Definition of elements in the resource (if no StructureDefinition)", formalDefinition = "Captures constraints on each element within the resource.") 1519 protected List<ElementDefinition> element; 1520 1521 private static final long serialVersionUID = 53896641L; 1522 1523 /** 1524 * Constructor 1525 */ 1526 public StructureDefinitionDifferentialComponent() { 1527 super(); 1528 } 1529 1530 /** 1531 * @return {@link #element} (Captures constraints on each element within the 1532 * resource.) 1533 */ 1534 public List<ElementDefinition> getElement() { 1535 if (this.element == null) 1536 this.element = new ArrayList<ElementDefinition>(); 1537 return this.element; 1538 } 1539 1540 /** 1541 * @return Returns a reference to <code>this</code> for easy method chaining 1542 */ 1543 public StructureDefinitionDifferentialComponent setElement(List<ElementDefinition> theElement) { 1544 this.element = theElement; 1545 return this; 1546 } 1547 1548 public boolean hasElement() { 1549 if (this.element == null) 1550 return false; 1551 for (ElementDefinition item : this.element) 1552 if (!item.isEmpty()) 1553 return true; 1554 return false; 1555 } 1556 1557 public ElementDefinition addElement() { // 3 1558 ElementDefinition t = new ElementDefinition(); 1559 if (this.element == null) 1560 this.element = new ArrayList<ElementDefinition>(); 1561 this.element.add(t); 1562 return t; 1563 } 1564 1565 public StructureDefinitionDifferentialComponent addElement(ElementDefinition t) { // 3 1566 if (t == null) 1567 return this; 1568 if (this.element == null) 1569 this.element = new ArrayList<ElementDefinition>(); 1570 this.element.add(t); 1571 return this; 1572 } 1573 1574 /** 1575 * @return The first repetition of repeating field {@link #element}, creating it 1576 * if it does not already exist 1577 */ 1578 public ElementDefinition getElementFirstRep() { 1579 if (getElement().isEmpty()) { 1580 addElement(); 1581 } 1582 return getElement().get(0); 1583 } 1584 1585 protected void listChildren(List<Property> children) { 1586 super.listChildren(children); 1587 children.add(new Property("element", "ElementDefinition", 1588 "Captures constraints on each element within the resource.", 0, java.lang.Integer.MAX_VALUE, element)); 1589 } 1590 1591 @Override 1592 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1593 switch (_hash) { 1594 case -1662836996: 1595 /* element */ return new Property("element", "ElementDefinition", 1596 "Captures constraints on each element within the resource.", 0, java.lang.Integer.MAX_VALUE, element); 1597 default: 1598 return super.getNamedProperty(_hash, _name, _checkValid); 1599 } 1600 1601 } 1602 1603 @Override 1604 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1605 switch (hash) { 1606 case -1662836996: 1607 /* element */ return this.element == null ? new Base[0] : this.element.toArray(new Base[this.element.size()]); // ElementDefinition 1608 default: 1609 return super.getProperty(hash, name, checkValid); 1610 } 1611 1612 } 1613 1614 @Override 1615 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1616 switch (hash) { 1617 case -1662836996: // element 1618 this.getElement().add(castToElementDefinition(value)); // ElementDefinition 1619 return value; 1620 default: 1621 return super.setProperty(hash, name, value); 1622 } 1623 1624 } 1625 1626 @Override 1627 public Base setProperty(String name, Base value) throws FHIRException { 1628 if (name.equals("element")) { 1629 this.getElement().add(castToElementDefinition(value)); 1630 } else 1631 return super.setProperty(name, value); 1632 return value; 1633 } 1634 1635 @Override 1636 public void removeChild(String name, Base value) throws FHIRException { 1637 if (name.equals("element")) { 1638 this.getElement().remove(castToElementDefinition(value)); 1639 } else 1640 super.removeChild(name, value); 1641 1642 } 1643 1644 @Override 1645 public Base makeProperty(int hash, String name) throws FHIRException { 1646 switch (hash) { 1647 case -1662836996: 1648 return addElement(); 1649 default: 1650 return super.makeProperty(hash, name); 1651 } 1652 1653 } 1654 1655 @Override 1656 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1657 switch (hash) { 1658 case -1662836996: 1659 /* element */ return new String[] { "ElementDefinition" }; 1660 default: 1661 return super.getTypesForProperty(hash, name); 1662 } 1663 1664 } 1665 1666 @Override 1667 public Base addChild(String name) throws FHIRException { 1668 if (name.equals("element")) { 1669 return addElement(); 1670 } else 1671 return super.addChild(name); 1672 } 1673 1674 public StructureDefinitionDifferentialComponent copy() { 1675 StructureDefinitionDifferentialComponent dst = new StructureDefinitionDifferentialComponent(); 1676 copyValues(dst); 1677 return dst; 1678 } 1679 1680 public void copyValues(StructureDefinitionDifferentialComponent dst) { 1681 super.copyValues(dst); 1682 if (element != null) { 1683 dst.element = new ArrayList<ElementDefinition>(); 1684 for (ElementDefinition i : element) 1685 dst.element.add(i.copy()); 1686 } 1687 ; 1688 } 1689 1690 @Override 1691 public boolean equalsDeep(Base other_) { 1692 if (!super.equalsDeep(other_)) 1693 return false; 1694 if (!(other_ instanceof StructureDefinitionDifferentialComponent)) 1695 return false; 1696 StructureDefinitionDifferentialComponent o = (StructureDefinitionDifferentialComponent) other_; 1697 return compareDeep(element, o.element, true); 1698 } 1699 1700 @Override 1701 public boolean equalsShallow(Base other_) { 1702 if (!super.equalsShallow(other_)) 1703 return false; 1704 if (!(other_ instanceof StructureDefinitionDifferentialComponent)) 1705 return false; 1706 StructureDefinitionDifferentialComponent o = (StructureDefinitionDifferentialComponent) other_; 1707 return true; 1708 } 1709 1710 public boolean isEmpty() { 1711 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(element); 1712 } 1713 1714 public String fhirType() { 1715 return "StructureDefinition.differential"; 1716 1717 } 1718 1719 } 1720 1721 /** 1722 * A formal identifier that is used to identify this structure definition when 1723 * it is represented in other formats, or referenced in a specification, model, 1724 * design or an instance. 1725 */ 1726 @Child(name = "identifier", type = { 1727 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1728 @Description(shortDefinition = "Additional identifier for the structure definition", formalDefinition = "A formal identifier that is used to identify this structure definition when it is represented in other formats, or referenced in a specification, model, design or an instance.") 1729 protected List<Identifier> identifier; 1730 1731 /** 1732 * Explanation of why this structure definition is needed and why it has been 1733 * designed as it has. 1734 */ 1735 @Child(name = "purpose", type = { 1736 MarkdownType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1737 @Description(shortDefinition = "Why this structure definition is defined", formalDefinition = "Explanation of why this structure definition is needed and why it has been designed as it has.") 1738 protected MarkdownType purpose; 1739 1740 /** 1741 * A copyright statement relating to the structure definition and/or its 1742 * contents. Copyright statements are generally legal restrictions on the use 1743 * and publishing of the structure definition. 1744 */ 1745 @Child(name = "copyright", type = { 1746 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1747 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure definition.") 1748 protected MarkdownType copyright; 1749 1750 /** 1751 * A set of key words or terms from external terminologies that may be used to 1752 * assist with indexing and searching of templates nby describing the use of 1753 * this structure definition, or the content it describes. 1754 */ 1755 @Child(name = "keyword", type = { 1756 Coding.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1757 @Description(shortDefinition = "Assist with indexing and finding", formalDefinition = "A set of key words or terms from external terminologies that may be used to assist with indexing and searching of templates nby describing the use of this structure definition, or the content it describes.") 1758 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/definition-use") 1759 protected List<Coding> keyword; 1760 1761 /** 1762 * The version of the FHIR specification on which this StructureDefinition is 1763 * based - this is the formal version of the specification, without the revision 1764 * number, e.g. [publication].[major].[minor], which is 4.0.1. for this version. 1765 */ 1766 @Child(name = "fhirVersion", type = { CodeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1767 @Description(shortDefinition = "FHIR Version this StructureDefinition targets", formalDefinition = "The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.0.1. for this version.") 1768 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/FHIR-version") 1769 protected Enumeration<FHIRVersion> fhirVersion; 1770 1771 /** 1772 * An external specification that the content is mapped to. 1773 */ 1774 @Child(name = "mapping", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1775 @Description(shortDefinition = "External specification that the content is mapped to", formalDefinition = "An external specification that the content is mapped to.") 1776 protected List<StructureDefinitionMappingComponent> mapping; 1777 1778 /** 1779 * Defines the kind of structure that this definition is describing. 1780 */ 1781 @Child(name = "kind", type = { CodeType.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 1782 @Description(shortDefinition = "primitive-type | complex-type | resource | logical", formalDefinition = "Defines the kind of structure that this definition is describing.") 1783 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/structure-definition-kind") 1784 protected Enumeration<StructureDefinitionKind> kind; 1785 1786 /** 1787 * Whether structure this definition describes is abstract or not - that is, 1788 * whether the structure is not intended to be instantiated. For Resources and 1789 * Data types, abstract types will never be exchanged between systems. 1790 */ 1791 @Child(name = "abstract", type = { BooleanType.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 1792 @Description(shortDefinition = "Whether the structure is abstract", formalDefinition = "Whether structure this definition describes is abstract or not - that is, whether the structure is not intended to be instantiated. For Resources and Data types, abstract types will never be exchanged between systems.") 1793 protected BooleanType abstract_; 1794 1795 /** 1796 * Identifies the types of resource or data type elements to which the extension 1797 * can be applied. 1798 */ 1799 @Child(name = "context", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1800 @Description(shortDefinition = "If an extension, where it can be used in instances", formalDefinition = "Identifies the types of resource or data type elements to which the extension can be applied.") 1801 protected List<StructureDefinitionContextComponent> context; 1802 1803 /** 1804 * A set of rules as FHIRPath Invariants about when the extension can be used 1805 * (e.g. co-occurrence variants for the extension). All the rules must be true. 1806 */ 1807 @Child(name = "contextInvariant", type = { 1808 StringType.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1809 @Description(shortDefinition = "FHIRPath invariants - when the extension can be used", formalDefinition = "A set of rules as FHIRPath Invariants about when the extension can be used (e.g. co-occurrence variants for the extension). All the rules must be true.") 1810 protected List<StringType> contextInvariant; 1811 1812 /** 1813 * The type this structure describes. If the derivation kind is 'specialization' 1814 * then this is the master definition for a type, and there is always one of 1815 * these (a data type, an extension, a resource, including abstract ones). 1816 * Otherwise the structure definition is a constraint on the stated type (and in 1817 * this case, the type cannot be an abstract type). References are URLs that are 1818 * relative to http://hl7.org/fhir/StructureDefinition e.g. "string" is a 1819 * reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs 1820 * are only allowed in logical models. 1821 */ 1822 @Child(name = "type", type = { UriType.class }, order = 10, min = 1, max = 1, modifier = false, summary = true) 1823 @Description(shortDefinition = "Type defined or constrained by this structure", formalDefinition = "The type this structure describes. If the derivation kind is 'specialization' then this is the master definition for a type, and there is always one of these (a data type, an extension, a resource, including abstract ones). Otherwise the structure definition is a constraint on the stated type (and in this case, the type cannot be an abstract type). References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. \"string\" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models.") 1824 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/defined-types") 1825 protected UriType type; 1826 1827 /** 1828 * An absolute URI that is the base structure from which this type is derived, 1829 * either by specialization or constraint. 1830 */ 1831 @Child(name = "baseDefinition", type = { 1832 CanonicalType.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 1833 @Description(shortDefinition = "Definition that this type is constrained/specialized from", formalDefinition = "An absolute URI that is the base structure from which this type is derived, either by specialization or constraint.") 1834 protected CanonicalType baseDefinition; 1835 1836 /** 1837 * How the type relates to the baseDefinition. 1838 */ 1839 @Child(name = "derivation", type = { CodeType.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 1840 @Description(shortDefinition = "specialization | constraint - How relates to base definition", formalDefinition = "How the type relates to the baseDefinition.") 1841 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/type-derivation-rule") 1842 protected Enumeration<TypeDerivationRule> derivation; 1843 1844 /** 1845 * A snapshot view is expressed in a standalone form that can be used and 1846 * interpreted without considering the base StructureDefinition. 1847 */ 1848 @Child(name = "snapshot", type = {}, order = 13, min = 0, max = 1, modifier = false, summary = false) 1849 @Description(shortDefinition = "Snapshot view of the structure", formalDefinition = "A snapshot view is expressed in a standalone form that can be used and interpreted without considering the base StructureDefinition.") 1850 protected StructureDefinitionSnapshotComponent snapshot; 1851 1852 /** 1853 * A differential view is expressed relative to the base StructureDefinition - a 1854 * statement of differences that it applies. 1855 */ 1856 @Child(name = "differential", type = {}, order = 14, min = 0, max = 1, modifier = false, summary = false) 1857 @Description(shortDefinition = "Differential view of the structure", formalDefinition = "A differential view is expressed relative to the base StructureDefinition - a statement of differences that it applies.") 1858 protected StructureDefinitionDifferentialComponent differential; 1859 1860 private static final long serialVersionUID = 316076774L; 1861 1862 /** 1863 * Constructor 1864 */ 1865 public StructureDefinition() { 1866 super(); 1867 } 1868 1869 /** 1870 * Constructor 1871 */ 1872 public StructureDefinition(UriType url, StringType name, Enumeration<PublicationStatus> status, 1873 Enumeration<StructureDefinitionKind> kind, BooleanType abstract_, UriType type) { 1874 super(); 1875 this.url = url; 1876 this.name = name; 1877 this.status = status; 1878 this.kind = kind; 1879 this.abstract_ = abstract_; 1880 this.type = type; 1881 } 1882 1883 /** 1884 * @return {@link #url} (An absolute URI that is used to identify this structure 1885 * definition when it is referenced in a specification, model, design or 1886 * an instance; also called its canonical identifier. This SHOULD be 1887 * globally unique and SHOULD be a literal address at which at which an 1888 * authoritative instance of this structure definition is (or will be) 1889 * published. This URL can be the target of a canonical reference. It 1890 * SHALL remain the same when the structure definition is stored on 1891 * different servers.). This is the underlying object with id, value and 1892 * extensions. The accessor "getUrl" gives direct access to the value 1893 */ 1894 public UriType getUrlElement() { 1895 if (this.url == null) 1896 if (Configuration.errorOnAutoCreate()) 1897 throw new Error("Attempt to auto-create StructureDefinition.url"); 1898 else if (Configuration.doAutoCreate()) 1899 this.url = new UriType(); // bb 1900 return this.url; 1901 } 1902 1903 public boolean hasUrlElement() { 1904 return this.url != null && !this.url.isEmpty(); 1905 } 1906 1907 public boolean hasUrl() { 1908 return this.url != null && !this.url.isEmpty(); 1909 } 1910 1911 /** 1912 * @param value {@link #url} (An absolute URI that is used to identify this 1913 * structure definition when it is referenced in a specification, 1914 * model, design or an instance; also called its canonical 1915 * identifier. This SHOULD be globally unique and SHOULD be a 1916 * literal address at which at which an authoritative instance of 1917 * this structure definition is (or will be) published. This URL 1918 * can be the target of a canonical reference. It SHALL remain the 1919 * same when the structure definition is stored on different 1920 * servers.). This is the underlying object with id, value and 1921 * extensions. The accessor "getUrl" gives direct access to the 1922 * value 1923 */ 1924 public StructureDefinition setUrlElement(UriType value) { 1925 this.url = value; 1926 return this; 1927 } 1928 1929 /** 1930 * @return An absolute URI that is used to identify this structure definition 1931 * when it is referenced in a specification, model, design or an 1932 * instance; also called its canonical identifier. This SHOULD be 1933 * globally unique and SHOULD be a literal address at which at which an 1934 * authoritative instance of this structure definition is (or will be) 1935 * published. This URL can be the target of a canonical reference. It 1936 * SHALL remain the same when the structure definition is stored on 1937 * different servers. 1938 */ 1939 public String getUrl() { 1940 return this.url == null ? null : this.url.getValue(); 1941 } 1942 1943 /** 1944 * @param value An absolute URI that is used to identify this structure 1945 * definition when it is referenced in a specification, model, 1946 * design or an instance; also called its canonical identifier. 1947 * This SHOULD be globally unique and SHOULD be a literal address 1948 * at which at which an authoritative instance of this structure 1949 * definition is (or will be) published. This URL can be the target 1950 * of a canonical reference. It SHALL remain the same when the 1951 * structure definition is stored on different servers. 1952 */ 1953 public StructureDefinition setUrl(String value) { 1954 if (this.url == null) 1955 this.url = new UriType(); 1956 this.url.setValue(value); 1957 return this; 1958 } 1959 1960 /** 1961 * @return {@link #identifier} (A formal identifier that is used to identify 1962 * this structure definition when it is represented in other formats, or 1963 * referenced in a specification, model, design or an instance.) 1964 */ 1965 public List<Identifier> getIdentifier() { 1966 if (this.identifier == null) 1967 this.identifier = new ArrayList<Identifier>(); 1968 return this.identifier; 1969 } 1970 1971 /** 1972 * @return Returns a reference to <code>this</code> for easy method chaining 1973 */ 1974 public StructureDefinition setIdentifier(List<Identifier> theIdentifier) { 1975 this.identifier = theIdentifier; 1976 return this; 1977 } 1978 1979 public boolean hasIdentifier() { 1980 if (this.identifier == null) 1981 return false; 1982 for (Identifier item : this.identifier) 1983 if (!item.isEmpty()) 1984 return true; 1985 return false; 1986 } 1987 1988 public Identifier addIdentifier() { // 3 1989 Identifier t = new Identifier(); 1990 if (this.identifier == null) 1991 this.identifier = new ArrayList<Identifier>(); 1992 this.identifier.add(t); 1993 return t; 1994 } 1995 1996 public StructureDefinition addIdentifier(Identifier t) { // 3 1997 if (t == null) 1998 return this; 1999 if (this.identifier == null) 2000 this.identifier = new ArrayList<Identifier>(); 2001 this.identifier.add(t); 2002 return this; 2003 } 2004 2005 /** 2006 * @return The first repetition of repeating field {@link #identifier}, creating 2007 * it if it does not already exist 2008 */ 2009 public Identifier getIdentifierFirstRep() { 2010 if (getIdentifier().isEmpty()) { 2011 addIdentifier(); 2012 } 2013 return getIdentifier().get(0); 2014 } 2015 2016 /** 2017 * @return {@link #version} (The identifier that is used to identify this 2018 * version of the structure definition when it is referenced in a 2019 * specification, model, design or instance. This is an arbitrary value 2020 * managed by the structure definition author and is not expected to be 2021 * globally unique. For example, it might be a timestamp (e.g. yyyymmdd) 2022 * if a managed version is not available. There is also no expectation 2023 * that versions can be placed in a lexicographical sequence.). This is 2024 * the underlying object with id, value and extensions. The accessor 2025 * "getVersion" gives direct access to the value 2026 */ 2027 public StringType getVersionElement() { 2028 if (this.version == null) 2029 if (Configuration.errorOnAutoCreate()) 2030 throw new Error("Attempt to auto-create StructureDefinition.version"); 2031 else if (Configuration.doAutoCreate()) 2032 this.version = new StringType(); // bb 2033 return this.version; 2034 } 2035 2036 public boolean hasVersionElement() { 2037 return this.version != null && !this.version.isEmpty(); 2038 } 2039 2040 public boolean hasVersion() { 2041 return this.version != null && !this.version.isEmpty(); 2042 } 2043 2044 /** 2045 * @param value {@link #version} (The identifier that is used to identify this 2046 * version of the structure definition when it is referenced in a 2047 * specification, model, design or instance. This is an arbitrary 2048 * value managed by the structure definition author and is not 2049 * expected to be globally unique. For example, it might be a 2050 * timestamp (e.g. yyyymmdd) if a managed version is not available. 2051 * There is also no expectation that versions can be placed in a 2052 * lexicographical sequence.). This is the underlying object with 2053 * id, value and extensions. The accessor "getVersion" gives direct 2054 * access to the value 2055 */ 2056 public StructureDefinition setVersionElement(StringType value) { 2057 this.version = value; 2058 return this; 2059 } 2060 2061 /** 2062 * @return The identifier that is used to identify this version of the structure 2063 * definition when it is referenced in a specification, model, design or 2064 * instance. This is an arbitrary value managed by the structure 2065 * definition author and is not expected to be globally unique. For 2066 * example, it might be a timestamp (e.g. yyyymmdd) if a managed version 2067 * is not available. There is also no expectation that versions can be 2068 * placed in a lexicographical sequence. 2069 */ 2070 public String getVersion() { 2071 return this.version == null ? null : this.version.getValue(); 2072 } 2073 2074 /** 2075 * @param value The identifier that is used to identify this version of the 2076 * structure definition when it is referenced in a specification, 2077 * model, design or instance. This is an arbitrary value managed by 2078 * the structure definition author and is not expected to be 2079 * globally unique. For example, it might be a timestamp (e.g. 2080 * yyyymmdd) if a managed version is not available. There is also 2081 * no expectation that versions can be placed in a lexicographical 2082 * sequence. 2083 */ 2084 public StructureDefinition setVersion(String value) { 2085 if (Utilities.noString(value)) 2086 this.version = null; 2087 else { 2088 if (this.version == null) 2089 this.version = new StringType(); 2090 this.version.setValue(value); 2091 } 2092 return this; 2093 } 2094 2095 /** 2096 * @return {@link #name} (A natural language name identifying the structure 2097 * definition. This name should be usable as an identifier for the 2098 * module by machine processing applications such as code generation.). 2099 * This is the underlying object with id, value and extensions. The 2100 * accessor "getName" gives direct access to the value 2101 */ 2102 public StringType getNameElement() { 2103 if (this.name == null) 2104 if (Configuration.errorOnAutoCreate()) 2105 throw new Error("Attempt to auto-create StructureDefinition.name"); 2106 else if (Configuration.doAutoCreate()) 2107 this.name = new StringType(); // bb 2108 return this.name; 2109 } 2110 2111 public boolean hasNameElement() { 2112 return this.name != null && !this.name.isEmpty(); 2113 } 2114 2115 public boolean hasName() { 2116 return this.name != null && !this.name.isEmpty(); 2117 } 2118 2119 /** 2120 * @param value {@link #name} (A natural language name identifying the structure 2121 * definition. This name should be usable as an identifier for the 2122 * module by machine processing applications such as code 2123 * generation.). This is the underlying object with id, value and 2124 * extensions. The accessor "getName" gives direct access to the 2125 * value 2126 */ 2127 public StructureDefinition setNameElement(StringType value) { 2128 this.name = value; 2129 return this; 2130 } 2131 2132 /** 2133 * @return A natural language name identifying the structure definition. This 2134 * name should be usable as an identifier for the module by machine 2135 * processing applications such as code generation. 2136 */ 2137 public String getName() { 2138 return this.name == null ? null : this.name.getValue(); 2139 } 2140 2141 /** 2142 * @param value A natural language name identifying the structure definition. 2143 * This name should be usable as an identifier for the module by 2144 * machine processing applications such as code generation. 2145 */ 2146 public StructureDefinition setName(String value) { 2147 if (this.name == null) 2148 this.name = new StringType(); 2149 this.name.setValue(value); 2150 return this; 2151 } 2152 2153 /** 2154 * @return {@link #title} (A short, descriptive, user-friendly title for the 2155 * structure definition.). This is the underlying object with id, value 2156 * and extensions. The accessor "getTitle" gives direct access to the 2157 * value 2158 */ 2159 public StringType getTitleElement() { 2160 if (this.title == null) 2161 if (Configuration.errorOnAutoCreate()) 2162 throw new Error("Attempt to auto-create StructureDefinition.title"); 2163 else if (Configuration.doAutoCreate()) 2164 this.title = new StringType(); // bb 2165 return this.title; 2166 } 2167 2168 public boolean hasTitleElement() { 2169 return this.title != null && !this.title.isEmpty(); 2170 } 2171 2172 public boolean hasTitle() { 2173 return this.title != null && !this.title.isEmpty(); 2174 } 2175 2176 /** 2177 * @param value {@link #title} (A short, descriptive, user-friendly title for 2178 * the structure definition.). This is the underlying object with 2179 * id, value and extensions. The accessor "getTitle" gives direct 2180 * access to the value 2181 */ 2182 public StructureDefinition setTitleElement(StringType value) { 2183 this.title = value; 2184 return this; 2185 } 2186 2187 /** 2188 * @return A short, descriptive, user-friendly title for the structure 2189 * definition. 2190 */ 2191 public String getTitle() { 2192 return this.title == null ? null : this.title.getValue(); 2193 } 2194 2195 /** 2196 * @param value A short, descriptive, user-friendly title for the structure 2197 * definition. 2198 */ 2199 public StructureDefinition setTitle(String value) { 2200 if (Utilities.noString(value)) 2201 this.title = null; 2202 else { 2203 if (this.title == null) 2204 this.title = new StringType(); 2205 this.title.setValue(value); 2206 } 2207 return this; 2208 } 2209 2210 /** 2211 * @return {@link #status} (The status of this structure definition. Enables 2212 * tracking the life-cycle of the content.). This is the underlying 2213 * object with id, value and extensions. The accessor "getStatus" gives 2214 * direct access to the value 2215 */ 2216 public Enumeration<PublicationStatus> getStatusElement() { 2217 if (this.status == null) 2218 if (Configuration.errorOnAutoCreate()) 2219 throw new Error("Attempt to auto-create StructureDefinition.status"); 2220 else if (Configuration.doAutoCreate()) 2221 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2222 return this.status; 2223 } 2224 2225 public boolean hasStatusElement() { 2226 return this.status != null && !this.status.isEmpty(); 2227 } 2228 2229 public boolean hasStatus() { 2230 return this.status != null && !this.status.isEmpty(); 2231 } 2232 2233 /** 2234 * @param value {@link #status} (The status of this structure definition. 2235 * Enables tracking the life-cycle of the content.). This is the 2236 * underlying object with id, value and extensions. The accessor 2237 * "getStatus" gives direct access to the value 2238 */ 2239 public StructureDefinition setStatusElement(Enumeration<PublicationStatus> value) { 2240 this.status = value; 2241 return this; 2242 } 2243 2244 /** 2245 * @return The status of this structure definition. Enables tracking the 2246 * life-cycle of the content. 2247 */ 2248 public PublicationStatus getStatus() { 2249 return this.status == null ? null : this.status.getValue(); 2250 } 2251 2252 /** 2253 * @param value The status of this structure definition. Enables tracking the 2254 * life-cycle of the content. 2255 */ 2256 public StructureDefinition setStatus(PublicationStatus value) { 2257 if (this.status == null) 2258 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2259 this.status.setValue(value); 2260 return this; 2261 } 2262 2263 /** 2264 * @return {@link #experimental} (A Boolean value to indicate that this 2265 * structure definition is authored for testing purposes (or 2266 * education/evaluation/marketing) and is not intended to be used for 2267 * genuine usage.). This is the underlying object with id, value and 2268 * extensions. The accessor "getExperimental" gives direct access to the 2269 * value 2270 */ 2271 public BooleanType getExperimentalElement() { 2272 if (this.experimental == null) 2273 if (Configuration.errorOnAutoCreate()) 2274 throw new Error("Attempt to auto-create StructureDefinition.experimental"); 2275 else if (Configuration.doAutoCreate()) 2276 this.experimental = new BooleanType(); // bb 2277 return this.experimental; 2278 } 2279 2280 public boolean hasExperimentalElement() { 2281 return this.experimental != null && !this.experimental.isEmpty(); 2282 } 2283 2284 public boolean hasExperimental() { 2285 return this.experimental != null && !this.experimental.isEmpty(); 2286 } 2287 2288 /** 2289 * @param value {@link #experimental} (A Boolean value to indicate that this 2290 * structure definition is authored for testing purposes (or 2291 * education/evaluation/marketing) and is not intended to be used 2292 * for genuine usage.). This is the underlying object with id, 2293 * value and extensions. The accessor "getExperimental" gives 2294 * direct access to the value 2295 */ 2296 public StructureDefinition setExperimentalElement(BooleanType value) { 2297 this.experimental = value; 2298 return this; 2299 } 2300 2301 /** 2302 * @return A Boolean value to indicate that this structure definition is 2303 * authored for testing purposes (or education/evaluation/marketing) and 2304 * is not intended to be used for genuine usage. 2305 */ 2306 public boolean getExperimental() { 2307 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 2308 } 2309 2310 /** 2311 * @param value A Boolean value to indicate that this structure definition is 2312 * authored for testing purposes (or 2313 * education/evaluation/marketing) and is not intended to be used 2314 * for genuine usage. 2315 */ 2316 public StructureDefinition setExperimental(boolean value) { 2317 if (this.experimental == null) 2318 this.experimental = new BooleanType(); 2319 this.experimental.setValue(value); 2320 return this; 2321 } 2322 2323 /** 2324 * @return {@link #date} (The date (and optionally time) when the structure 2325 * definition was published. The date must change when the business 2326 * version changes and it must change if the status code changes. In 2327 * addition, it should change when the substantive content of the 2328 * structure definition changes.). This is the underlying object with 2329 * id, value and extensions. The accessor "getDate" gives direct access 2330 * to the value 2331 */ 2332 public DateTimeType getDateElement() { 2333 if (this.date == null) 2334 if (Configuration.errorOnAutoCreate()) 2335 throw new Error("Attempt to auto-create StructureDefinition.date"); 2336 else if (Configuration.doAutoCreate()) 2337 this.date = new DateTimeType(); // bb 2338 return this.date; 2339 } 2340 2341 public boolean hasDateElement() { 2342 return this.date != null && !this.date.isEmpty(); 2343 } 2344 2345 public boolean hasDate() { 2346 return this.date != null && !this.date.isEmpty(); 2347 } 2348 2349 /** 2350 * @param value {@link #date} (The date (and optionally time) when the structure 2351 * definition was published. The date must change when the business 2352 * version changes and it must change if the status code changes. 2353 * In addition, it should change when the substantive content of 2354 * the structure definition changes.). This is the underlying 2355 * object with id, value and extensions. The accessor "getDate" 2356 * gives direct access to the value 2357 */ 2358 public StructureDefinition setDateElement(DateTimeType value) { 2359 this.date = value; 2360 return this; 2361 } 2362 2363 /** 2364 * @return The date (and optionally time) when the structure definition was 2365 * published. The date must change when the business version changes and 2366 * it must change if the status code changes. In addition, it should 2367 * change when the substantive content of the structure definition 2368 * changes. 2369 */ 2370 public Date getDate() { 2371 return this.date == null ? null : this.date.getValue(); 2372 } 2373 2374 /** 2375 * @param value The date (and optionally time) when the structure definition was 2376 * published. The date must change when the business version 2377 * changes and it must change if the status code changes. In 2378 * addition, it should change when the substantive content of the 2379 * structure definition changes. 2380 */ 2381 public StructureDefinition setDate(Date value) { 2382 if (value == null) 2383 this.date = null; 2384 else { 2385 if (this.date == null) 2386 this.date = new DateTimeType(); 2387 this.date.setValue(value); 2388 } 2389 return this; 2390 } 2391 2392 /** 2393 * @return {@link #publisher} (The name of the organization or individual that 2394 * published the structure definition.). This is the underlying object 2395 * with id, value and extensions. The accessor "getPublisher" gives 2396 * direct access to the value 2397 */ 2398 public StringType getPublisherElement() { 2399 if (this.publisher == null) 2400 if (Configuration.errorOnAutoCreate()) 2401 throw new Error("Attempt to auto-create StructureDefinition.publisher"); 2402 else if (Configuration.doAutoCreate()) 2403 this.publisher = new StringType(); // bb 2404 return this.publisher; 2405 } 2406 2407 public boolean hasPublisherElement() { 2408 return this.publisher != null && !this.publisher.isEmpty(); 2409 } 2410 2411 public boolean hasPublisher() { 2412 return this.publisher != null && !this.publisher.isEmpty(); 2413 } 2414 2415 /** 2416 * @param value {@link #publisher} (The name of the organization or individual 2417 * that published the structure definition.). This is the 2418 * underlying object with id, value and extensions. The accessor 2419 * "getPublisher" gives direct access to the value 2420 */ 2421 public StructureDefinition setPublisherElement(StringType value) { 2422 this.publisher = value; 2423 return this; 2424 } 2425 2426 /** 2427 * @return The name of the organization or individual that published the 2428 * structure definition. 2429 */ 2430 public String getPublisher() { 2431 return this.publisher == null ? null : this.publisher.getValue(); 2432 } 2433 2434 /** 2435 * @param value The name of the organization or individual that published the 2436 * structure definition. 2437 */ 2438 public StructureDefinition setPublisher(String value) { 2439 if (Utilities.noString(value)) 2440 this.publisher = null; 2441 else { 2442 if (this.publisher == null) 2443 this.publisher = new StringType(); 2444 this.publisher.setValue(value); 2445 } 2446 return this; 2447 } 2448 2449 /** 2450 * @return {@link #contact} (Contact details to assist a user in finding and 2451 * communicating with the publisher.) 2452 */ 2453 public List<ContactDetail> getContact() { 2454 if (this.contact == null) 2455 this.contact = new ArrayList<ContactDetail>(); 2456 return this.contact; 2457 } 2458 2459 /** 2460 * @return Returns a reference to <code>this</code> for easy method chaining 2461 */ 2462 public StructureDefinition setContact(List<ContactDetail> theContact) { 2463 this.contact = theContact; 2464 return this; 2465 } 2466 2467 public boolean hasContact() { 2468 if (this.contact == null) 2469 return false; 2470 for (ContactDetail item : this.contact) 2471 if (!item.isEmpty()) 2472 return true; 2473 return false; 2474 } 2475 2476 public ContactDetail addContact() { // 3 2477 ContactDetail t = new ContactDetail(); 2478 if (this.contact == null) 2479 this.contact = new ArrayList<ContactDetail>(); 2480 this.contact.add(t); 2481 return t; 2482 } 2483 2484 public StructureDefinition addContact(ContactDetail t) { // 3 2485 if (t == null) 2486 return this; 2487 if (this.contact == null) 2488 this.contact = new ArrayList<ContactDetail>(); 2489 this.contact.add(t); 2490 return this; 2491 } 2492 2493 /** 2494 * @return The first repetition of repeating field {@link #contact}, creating it 2495 * if it does not already exist 2496 */ 2497 public ContactDetail getContactFirstRep() { 2498 if (getContact().isEmpty()) { 2499 addContact(); 2500 } 2501 return getContact().get(0); 2502 } 2503 2504 /** 2505 * @return {@link #description} (A free text natural language description of the 2506 * structure definition from a consumer's perspective.). This is the 2507 * underlying object with id, value and extensions. The accessor 2508 * "getDescription" gives direct access to the value 2509 */ 2510 public MarkdownType getDescriptionElement() { 2511 if (this.description == null) 2512 if (Configuration.errorOnAutoCreate()) 2513 throw new Error("Attempt to auto-create StructureDefinition.description"); 2514 else if (Configuration.doAutoCreate()) 2515 this.description = new MarkdownType(); // bb 2516 return this.description; 2517 } 2518 2519 public boolean hasDescriptionElement() { 2520 return this.description != null && !this.description.isEmpty(); 2521 } 2522 2523 public boolean hasDescription() { 2524 return this.description != null && !this.description.isEmpty(); 2525 } 2526 2527 /** 2528 * @param value {@link #description} (A free text natural language description 2529 * of the structure definition from a consumer's perspective.). 2530 * This is the underlying object with id, value and extensions. The 2531 * accessor "getDescription" gives direct access to the value 2532 */ 2533 public StructureDefinition setDescriptionElement(MarkdownType value) { 2534 this.description = value; 2535 return this; 2536 } 2537 2538 /** 2539 * @return A free text natural language description of the structure definition 2540 * from a consumer's perspective. 2541 */ 2542 public String getDescription() { 2543 return this.description == null ? null : this.description.getValue(); 2544 } 2545 2546 /** 2547 * @param value A free text natural language description of the structure 2548 * definition from a consumer's perspective. 2549 */ 2550 public StructureDefinition setDescription(String value) { 2551 if (value == null) 2552 this.description = null; 2553 else { 2554 if (this.description == null) 2555 this.description = new MarkdownType(); 2556 this.description.setValue(value); 2557 } 2558 return this; 2559 } 2560 2561 /** 2562 * @return {@link #useContext} (The content was developed with a focus and 2563 * intent of supporting the contexts that are listed. These contexts may 2564 * be general categories (gender, age, ...) or may be references to 2565 * specific programs (insurance plans, studies, ...) and may be used to 2566 * assist with indexing and searching for appropriate structure 2567 * definition instances.) 2568 */ 2569 public List<UsageContext> getUseContext() { 2570 if (this.useContext == null) 2571 this.useContext = new ArrayList<UsageContext>(); 2572 return this.useContext; 2573 } 2574 2575 /** 2576 * @return Returns a reference to <code>this</code> for easy method chaining 2577 */ 2578 public StructureDefinition setUseContext(List<UsageContext> theUseContext) { 2579 this.useContext = theUseContext; 2580 return this; 2581 } 2582 2583 public boolean hasUseContext() { 2584 if (this.useContext == null) 2585 return false; 2586 for (UsageContext item : this.useContext) 2587 if (!item.isEmpty()) 2588 return true; 2589 return false; 2590 } 2591 2592 public UsageContext addUseContext() { // 3 2593 UsageContext t = new UsageContext(); 2594 if (this.useContext == null) 2595 this.useContext = new ArrayList<UsageContext>(); 2596 this.useContext.add(t); 2597 return t; 2598 } 2599 2600 public StructureDefinition addUseContext(UsageContext t) { // 3 2601 if (t == null) 2602 return this; 2603 if (this.useContext == null) 2604 this.useContext = new ArrayList<UsageContext>(); 2605 this.useContext.add(t); 2606 return this; 2607 } 2608 2609 /** 2610 * @return The first repetition of repeating field {@link #useContext}, creating 2611 * it if it does not already exist 2612 */ 2613 public UsageContext getUseContextFirstRep() { 2614 if (getUseContext().isEmpty()) { 2615 addUseContext(); 2616 } 2617 return getUseContext().get(0); 2618 } 2619 2620 /** 2621 * @return {@link #jurisdiction} (A legal or geographic region in which the 2622 * structure definition is intended to be used.) 2623 */ 2624 public List<CodeableConcept> getJurisdiction() { 2625 if (this.jurisdiction == null) 2626 this.jurisdiction = new ArrayList<CodeableConcept>(); 2627 return this.jurisdiction; 2628 } 2629 2630 /** 2631 * @return Returns a reference to <code>this</code> for easy method chaining 2632 */ 2633 public StructureDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 2634 this.jurisdiction = theJurisdiction; 2635 return this; 2636 } 2637 2638 public boolean hasJurisdiction() { 2639 if (this.jurisdiction == null) 2640 return false; 2641 for (CodeableConcept item : this.jurisdiction) 2642 if (!item.isEmpty()) 2643 return true; 2644 return false; 2645 } 2646 2647 public CodeableConcept addJurisdiction() { // 3 2648 CodeableConcept t = new CodeableConcept(); 2649 if (this.jurisdiction == null) 2650 this.jurisdiction = new ArrayList<CodeableConcept>(); 2651 this.jurisdiction.add(t); 2652 return t; 2653 } 2654 2655 public StructureDefinition addJurisdiction(CodeableConcept t) { // 3 2656 if (t == null) 2657 return this; 2658 if (this.jurisdiction == null) 2659 this.jurisdiction = new ArrayList<CodeableConcept>(); 2660 this.jurisdiction.add(t); 2661 return this; 2662 } 2663 2664 /** 2665 * @return The first repetition of repeating field {@link #jurisdiction}, 2666 * creating it if it does not already exist 2667 */ 2668 public CodeableConcept getJurisdictionFirstRep() { 2669 if (getJurisdiction().isEmpty()) { 2670 addJurisdiction(); 2671 } 2672 return getJurisdiction().get(0); 2673 } 2674 2675 /** 2676 * @return {@link #purpose} (Explanation of why this structure definition is 2677 * needed and why it has been designed as it has.). This is the 2678 * underlying object with id, value and extensions. The accessor 2679 * "getPurpose" gives direct access to the value 2680 */ 2681 public MarkdownType getPurposeElement() { 2682 if (this.purpose == null) 2683 if (Configuration.errorOnAutoCreate()) 2684 throw new Error("Attempt to auto-create StructureDefinition.purpose"); 2685 else if (Configuration.doAutoCreate()) 2686 this.purpose = new MarkdownType(); // bb 2687 return this.purpose; 2688 } 2689 2690 public boolean hasPurposeElement() { 2691 return this.purpose != null && !this.purpose.isEmpty(); 2692 } 2693 2694 public boolean hasPurpose() { 2695 return this.purpose != null && !this.purpose.isEmpty(); 2696 } 2697 2698 /** 2699 * @param value {@link #purpose} (Explanation of why this structure definition 2700 * is needed and why it has been designed as it has.). This is the 2701 * underlying object with id, value and extensions. The accessor 2702 * "getPurpose" gives direct access to the value 2703 */ 2704 public StructureDefinition setPurposeElement(MarkdownType value) { 2705 this.purpose = value; 2706 return this; 2707 } 2708 2709 /** 2710 * @return Explanation of why this structure definition is needed and why it has 2711 * been designed as it has. 2712 */ 2713 public String getPurpose() { 2714 return this.purpose == null ? null : this.purpose.getValue(); 2715 } 2716 2717 /** 2718 * @param value Explanation of why this structure definition is needed and why 2719 * it has been designed as it has. 2720 */ 2721 public StructureDefinition setPurpose(String value) { 2722 if (value == null) 2723 this.purpose = null; 2724 else { 2725 if (this.purpose == null) 2726 this.purpose = new MarkdownType(); 2727 this.purpose.setValue(value); 2728 } 2729 return this; 2730 } 2731 2732 /** 2733 * @return {@link #copyright} (A copyright statement relating to the structure 2734 * definition and/or its contents. Copyright statements are generally 2735 * legal restrictions on the use and publishing of the structure 2736 * definition.). This is the underlying object with id, value and 2737 * extensions. The accessor "getCopyright" gives direct access to the 2738 * value 2739 */ 2740 public MarkdownType getCopyrightElement() { 2741 if (this.copyright == null) 2742 if (Configuration.errorOnAutoCreate()) 2743 throw new Error("Attempt to auto-create StructureDefinition.copyright"); 2744 else if (Configuration.doAutoCreate()) 2745 this.copyright = new MarkdownType(); // bb 2746 return this.copyright; 2747 } 2748 2749 public boolean hasCopyrightElement() { 2750 return this.copyright != null && !this.copyright.isEmpty(); 2751 } 2752 2753 public boolean hasCopyright() { 2754 return this.copyright != null && !this.copyright.isEmpty(); 2755 } 2756 2757 /** 2758 * @param value {@link #copyright} (A copyright statement relating to the 2759 * structure definition and/or its contents. Copyright statements 2760 * are generally legal restrictions on the use and publishing of 2761 * the structure definition.). This is the underlying object with 2762 * id, value and extensions. The accessor "getCopyright" gives 2763 * direct access to the value 2764 */ 2765 public StructureDefinition setCopyrightElement(MarkdownType value) { 2766 this.copyright = value; 2767 return this; 2768 } 2769 2770 /** 2771 * @return A copyright statement relating to the structure definition and/or its 2772 * contents. Copyright statements are generally legal restrictions on 2773 * the use and publishing of the structure definition. 2774 */ 2775 public String getCopyright() { 2776 return this.copyright == null ? null : this.copyright.getValue(); 2777 } 2778 2779 /** 2780 * @param value A copyright statement relating to the structure definition 2781 * and/or its contents. Copyright statements are generally legal 2782 * restrictions on the use and publishing of the structure 2783 * definition. 2784 */ 2785 public StructureDefinition setCopyright(String value) { 2786 if (value == null) 2787 this.copyright = null; 2788 else { 2789 if (this.copyright == null) 2790 this.copyright = new MarkdownType(); 2791 this.copyright.setValue(value); 2792 } 2793 return this; 2794 } 2795 2796 /** 2797 * @return {@link #keyword} (A set of key words or terms from external 2798 * terminologies that may be used to assist with indexing and searching 2799 * of templates nby describing the use of this structure definition, or 2800 * the content it describes.) 2801 */ 2802 public List<Coding> getKeyword() { 2803 if (this.keyword == null) 2804 this.keyword = new ArrayList<Coding>(); 2805 return this.keyword; 2806 } 2807 2808 /** 2809 * @return Returns a reference to <code>this</code> for easy method chaining 2810 */ 2811 public StructureDefinition setKeyword(List<Coding> theKeyword) { 2812 this.keyword = theKeyword; 2813 return this; 2814 } 2815 2816 public boolean hasKeyword() { 2817 if (this.keyword == null) 2818 return false; 2819 for (Coding item : this.keyword) 2820 if (!item.isEmpty()) 2821 return true; 2822 return false; 2823 } 2824 2825 public Coding addKeyword() { // 3 2826 Coding t = new Coding(); 2827 if (this.keyword == null) 2828 this.keyword = new ArrayList<Coding>(); 2829 this.keyword.add(t); 2830 return t; 2831 } 2832 2833 public StructureDefinition addKeyword(Coding t) { // 3 2834 if (t == null) 2835 return this; 2836 if (this.keyword == null) 2837 this.keyword = new ArrayList<Coding>(); 2838 this.keyword.add(t); 2839 return this; 2840 } 2841 2842 /** 2843 * @return The first repetition of repeating field {@link #keyword}, creating it 2844 * if it does not already exist 2845 */ 2846 public Coding getKeywordFirstRep() { 2847 if (getKeyword().isEmpty()) { 2848 addKeyword(); 2849 } 2850 return getKeyword().get(0); 2851 } 2852 2853 /** 2854 * @return {@link #fhirVersion} (The version of the FHIR specification on which 2855 * this StructureDefinition is based - this is the formal version of the 2856 * specification, without the revision number, e.g. 2857 * [publication].[major].[minor], which is 4.0.1. for this version.). 2858 * This is the underlying object with id, value and extensions. The 2859 * accessor "getFhirVersion" gives direct access to the value 2860 */ 2861 public Enumeration<FHIRVersion> getFhirVersionElement() { 2862 if (this.fhirVersion == null) 2863 if (Configuration.errorOnAutoCreate()) 2864 throw new Error("Attempt to auto-create StructureDefinition.fhirVersion"); 2865 else if (Configuration.doAutoCreate()) 2866 this.fhirVersion = new Enumeration<FHIRVersion>(new FHIRVersionEnumFactory()); // bb 2867 return this.fhirVersion; 2868 } 2869 2870 public boolean hasFhirVersionElement() { 2871 return this.fhirVersion != null && !this.fhirVersion.isEmpty(); 2872 } 2873 2874 public boolean hasFhirVersion() { 2875 return this.fhirVersion != null && !this.fhirVersion.isEmpty(); 2876 } 2877 2878 /** 2879 * @param value {@link #fhirVersion} (The version of the FHIR specification on 2880 * which this StructureDefinition is based - this is the formal 2881 * version of the specification, without the revision number, e.g. 2882 * [publication].[major].[minor], which is 4.0.1. for this 2883 * version.). This is the underlying object with id, value and 2884 * extensions. The accessor "getFhirVersion" gives direct access to 2885 * the value 2886 */ 2887 public StructureDefinition setFhirVersionElement(Enumeration<FHIRVersion> value) { 2888 this.fhirVersion = value; 2889 return this; 2890 } 2891 2892 /** 2893 * @return The version of the FHIR specification on which this 2894 * StructureDefinition is based - this is the formal version of the 2895 * specification, without the revision number, e.g. 2896 * [publication].[major].[minor], which is 4.0.1. for this version. 2897 */ 2898 public FHIRVersion getFhirVersion() { 2899 return this.fhirVersion == null ? null : this.fhirVersion.getValue(); 2900 } 2901 2902 /** 2903 * @param value The version of the FHIR specification on which this 2904 * StructureDefinition is based - this is the formal version of the 2905 * specification, without the revision number, e.g. 2906 * [publication].[major].[minor], which is 4.0.1. for this version. 2907 */ 2908 public StructureDefinition setFhirVersion(FHIRVersion value) { 2909 if (value == null) 2910 this.fhirVersion = null; 2911 else { 2912 if (this.fhirVersion == null) 2913 this.fhirVersion = new Enumeration<FHIRVersion>(new FHIRVersionEnumFactory()); 2914 this.fhirVersion.setValue(value); 2915 } 2916 return this; 2917 } 2918 2919 /** 2920 * @return {@link #mapping} (An external specification that the content is 2921 * mapped to.) 2922 */ 2923 public List<StructureDefinitionMappingComponent> getMapping() { 2924 if (this.mapping == null) 2925 this.mapping = new ArrayList<StructureDefinitionMappingComponent>(); 2926 return this.mapping; 2927 } 2928 2929 /** 2930 * @return Returns a reference to <code>this</code> for easy method chaining 2931 */ 2932 public StructureDefinition setMapping(List<StructureDefinitionMappingComponent> theMapping) { 2933 this.mapping = theMapping; 2934 return this; 2935 } 2936 2937 public boolean hasMapping() { 2938 if (this.mapping == null) 2939 return false; 2940 for (StructureDefinitionMappingComponent item : this.mapping) 2941 if (!item.isEmpty()) 2942 return true; 2943 return false; 2944 } 2945 2946 public StructureDefinitionMappingComponent addMapping() { // 3 2947 StructureDefinitionMappingComponent t = new StructureDefinitionMappingComponent(); 2948 if (this.mapping == null) 2949 this.mapping = new ArrayList<StructureDefinitionMappingComponent>(); 2950 this.mapping.add(t); 2951 return t; 2952 } 2953 2954 public StructureDefinition addMapping(StructureDefinitionMappingComponent t) { // 3 2955 if (t == null) 2956 return this; 2957 if (this.mapping == null) 2958 this.mapping = new ArrayList<StructureDefinitionMappingComponent>(); 2959 this.mapping.add(t); 2960 return this; 2961 } 2962 2963 /** 2964 * @return The first repetition of repeating field {@link #mapping}, creating it 2965 * if it does not already exist 2966 */ 2967 public StructureDefinitionMappingComponent getMappingFirstRep() { 2968 if (getMapping().isEmpty()) { 2969 addMapping(); 2970 } 2971 return getMapping().get(0); 2972 } 2973 2974 /** 2975 * @return {@link #kind} (Defines the kind of structure that this definition is 2976 * describing.). This is the underlying object with id, value and 2977 * extensions. The accessor "getKind" gives direct access to the value 2978 */ 2979 public Enumeration<StructureDefinitionKind> getKindElement() { 2980 if (this.kind == null) 2981 if (Configuration.errorOnAutoCreate()) 2982 throw new Error("Attempt to auto-create StructureDefinition.kind"); 2983 else if (Configuration.doAutoCreate()) 2984 this.kind = new Enumeration<StructureDefinitionKind>(new StructureDefinitionKindEnumFactory()); // bb 2985 return this.kind; 2986 } 2987 2988 public boolean hasKindElement() { 2989 return this.kind != null && !this.kind.isEmpty(); 2990 } 2991 2992 public boolean hasKind() { 2993 return this.kind != null && !this.kind.isEmpty(); 2994 } 2995 2996 /** 2997 * @param value {@link #kind} (Defines the kind of structure that this 2998 * definition is describing.). This is the underlying object with 2999 * id, value and extensions. The accessor "getKind" gives direct 3000 * access to the value 3001 */ 3002 public StructureDefinition setKindElement(Enumeration<StructureDefinitionKind> value) { 3003 this.kind = value; 3004 return this; 3005 } 3006 3007 /** 3008 * @return Defines the kind of structure that this definition is describing. 3009 */ 3010 public StructureDefinitionKind getKind() { 3011 return this.kind == null ? null : this.kind.getValue(); 3012 } 3013 3014 /** 3015 * @param value Defines the kind of structure that this definition is 3016 * describing. 3017 */ 3018 public StructureDefinition setKind(StructureDefinitionKind value) { 3019 if (this.kind == null) 3020 this.kind = new Enumeration<StructureDefinitionKind>(new StructureDefinitionKindEnumFactory()); 3021 this.kind.setValue(value); 3022 return this; 3023 } 3024 3025 /** 3026 * @return {@link #abstract_} (Whether structure this definition describes is 3027 * abstract or not - that is, whether the structure is not intended to 3028 * be instantiated. For Resources and Data types, abstract types will 3029 * never be exchanged between systems.). This is the underlying object 3030 * with id, value and extensions. The accessor "getAbstract" gives 3031 * direct access to the value 3032 */ 3033 public BooleanType getAbstractElement() { 3034 if (this.abstract_ == null) 3035 if (Configuration.errorOnAutoCreate()) 3036 throw new Error("Attempt to auto-create StructureDefinition.abstract_"); 3037 else if (Configuration.doAutoCreate()) 3038 this.abstract_ = new BooleanType(); // bb 3039 return this.abstract_; 3040 } 3041 3042 public boolean hasAbstractElement() { 3043 return this.abstract_ != null && !this.abstract_.isEmpty(); 3044 } 3045 3046 public boolean hasAbstract() { 3047 return this.abstract_ != null && !this.abstract_.isEmpty(); 3048 } 3049 3050 /** 3051 * @param value {@link #abstract_} (Whether structure this definition describes 3052 * is abstract or not - that is, whether the structure is not 3053 * intended to be instantiated. For Resources and Data types, 3054 * abstract types will never be exchanged between systems.). This 3055 * is the underlying object with id, value and extensions. The 3056 * accessor "getAbstract" gives direct access to the value 3057 */ 3058 public StructureDefinition setAbstractElement(BooleanType value) { 3059 this.abstract_ = value; 3060 return this; 3061 } 3062 3063 /** 3064 * @return Whether structure this definition describes is abstract or not - that 3065 * is, whether the structure is not intended to be instantiated. For 3066 * Resources and Data types, abstract types will never be exchanged 3067 * between systems. 3068 */ 3069 public boolean getAbstract() { 3070 return this.abstract_ == null || this.abstract_.isEmpty() ? false : this.abstract_.getValue(); 3071 } 3072 3073 /** 3074 * @param value Whether structure this definition describes is abstract or not - 3075 * that is, whether the structure is not intended to be 3076 * instantiated. For Resources and Data types, abstract types will 3077 * never be exchanged between systems. 3078 */ 3079 public StructureDefinition setAbstract(boolean value) { 3080 if (this.abstract_ == null) 3081 this.abstract_ = new BooleanType(); 3082 this.abstract_.setValue(value); 3083 return this; 3084 } 3085 3086 /** 3087 * @return {@link #context} (Identifies the types of resource or data type 3088 * elements to which the extension can be applied.) 3089 */ 3090 public List<StructureDefinitionContextComponent> getContext() { 3091 if (this.context == null) 3092 this.context = new ArrayList<StructureDefinitionContextComponent>(); 3093 return this.context; 3094 } 3095 3096 /** 3097 * @return Returns a reference to <code>this</code> for easy method chaining 3098 */ 3099 public StructureDefinition setContext(List<StructureDefinitionContextComponent> theContext) { 3100 this.context = theContext; 3101 return this; 3102 } 3103 3104 public boolean hasContext() { 3105 if (this.context == null) 3106 return false; 3107 for (StructureDefinitionContextComponent item : this.context) 3108 if (!item.isEmpty()) 3109 return true; 3110 return false; 3111 } 3112 3113 public StructureDefinitionContextComponent addContext() { // 3 3114 StructureDefinitionContextComponent t = new StructureDefinitionContextComponent(); 3115 if (this.context == null) 3116 this.context = new ArrayList<StructureDefinitionContextComponent>(); 3117 this.context.add(t); 3118 return t; 3119 } 3120 3121 public StructureDefinition addContext(StructureDefinitionContextComponent t) { // 3 3122 if (t == null) 3123 return this; 3124 if (this.context == null) 3125 this.context = new ArrayList<StructureDefinitionContextComponent>(); 3126 this.context.add(t); 3127 return this; 3128 } 3129 3130 /** 3131 * @return The first repetition of repeating field {@link #context}, creating it 3132 * if it does not already exist 3133 */ 3134 public StructureDefinitionContextComponent getContextFirstRep() { 3135 if (getContext().isEmpty()) { 3136 addContext(); 3137 } 3138 return getContext().get(0); 3139 } 3140 3141 /** 3142 * @return {@link #contextInvariant} (A set of rules as FHIRPath Invariants 3143 * about when the extension can be used (e.g. co-occurrence variants for 3144 * the extension). All the rules must be true.) 3145 */ 3146 public List<StringType> getContextInvariant() { 3147 if (this.contextInvariant == null) 3148 this.contextInvariant = new ArrayList<StringType>(); 3149 return this.contextInvariant; 3150 } 3151 3152 /** 3153 * @return Returns a reference to <code>this</code> for easy method chaining 3154 */ 3155 public StructureDefinition setContextInvariant(List<StringType> theContextInvariant) { 3156 this.contextInvariant = theContextInvariant; 3157 return this; 3158 } 3159 3160 public boolean hasContextInvariant() { 3161 if (this.contextInvariant == null) 3162 return false; 3163 for (StringType item : this.contextInvariant) 3164 if (!item.isEmpty()) 3165 return true; 3166 return false; 3167 } 3168 3169 /** 3170 * @return {@link #contextInvariant} (A set of rules as FHIRPath Invariants 3171 * about when the extension can be used (e.g. co-occurrence variants for 3172 * the extension). All the rules must be true.) 3173 */ 3174 public StringType addContextInvariantElement() {// 2 3175 StringType t = new StringType(); 3176 if (this.contextInvariant == null) 3177 this.contextInvariant = new ArrayList<StringType>(); 3178 this.contextInvariant.add(t); 3179 return t; 3180 } 3181 3182 /** 3183 * @param value {@link #contextInvariant} (A set of rules as FHIRPath Invariants 3184 * about when the extension can be used (e.g. co-occurrence 3185 * variants for the extension). All the rules must be true.) 3186 */ 3187 public StructureDefinition addContextInvariant(String value) { // 1 3188 StringType t = new StringType(); 3189 t.setValue(value); 3190 if (this.contextInvariant == null) 3191 this.contextInvariant = new ArrayList<StringType>(); 3192 this.contextInvariant.add(t); 3193 return this; 3194 } 3195 3196 /** 3197 * @param value {@link #contextInvariant} (A set of rules as FHIRPath Invariants 3198 * about when the extension can be used (e.g. co-occurrence 3199 * variants for the extension). All the rules must be true.) 3200 */ 3201 public boolean hasContextInvariant(String value) { 3202 if (this.contextInvariant == null) 3203 return false; 3204 for (StringType v : this.contextInvariant) 3205 if (v.getValue().equals(value)) // string 3206 return true; 3207 return false; 3208 } 3209 3210 /** 3211 * @return {@link #type} (The type this structure describes. If the derivation 3212 * kind is 'specialization' then this is the master definition for a 3213 * type, and there is always one of these (a data type, an extension, a 3214 * resource, including abstract ones). Otherwise the structure 3215 * definition is a constraint on the stated type (and in this case, the 3216 * type cannot be an abstract type). References are URLs that are 3217 * relative to http://hl7.org/fhir/StructureDefinition e.g. "string" is 3218 * a reference to http://hl7.org/fhir/StructureDefinition/string. 3219 * Absolute URLs are only allowed in logical models.). This is the 3220 * underlying object with id, value and extensions. The accessor 3221 * "getType" gives direct access to the value 3222 */ 3223 public UriType getTypeElement() { 3224 if (this.type == null) 3225 if (Configuration.errorOnAutoCreate()) 3226 throw new Error("Attempt to auto-create StructureDefinition.type"); 3227 else if (Configuration.doAutoCreate()) 3228 this.type = new UriType(); // bb 3229 return this.type; 3230 } 3231 3232 public boolean hasTypeElement() { 3233 return this.type != null && !this.type.isEmpty(); 3234 } 3235 3236 public boolean hasType() { 3237 return this.type != null && !this.type.isEmpty(); 3238 } 3239 3240 /** 3241 * @param value {@link #type} (The type this structure describes. If the 3242 * derivation kind is 'specialization' then this is the master 3243 * definition for a type, and there is always one of these (a data 3244 * type, an extension, a resource, including abstract ones). 3245 * Otherwise the structure definition is a constraint on the stated 3246 * type (and in this case, the type cannot be an abstract type). 3247 * References are URLs that are relative to 3248 * http://hl7.org/fhir/StructureDefinition e.g. "string" is a 3249 * reference to http://hl7.org/fhir/StructureDefinition/string. 3250 * Absolute URLs are only allowed in logical models.). This is the 3251 * underlying object with id, value and extensions. The accessor 3252 * "getType" gives direct access to the value 3253 */ 3254 public StructureDefinition setTypeElement(UriType value) { 3255 this.type = value; 3256 return this; 3257 } 3258 3259 /** 3260 * @return The type this structure describes. If the derivation kind is 3261 * 'specialization' then this is the master definition for a type, and 3262 * there is always one of these (a data type, an extension, a resource, 3263 * including abstract ones). Otherwise the structure definition is a 3264 * constraint on the stated type (and in this case, the type cannot be 3265 * an abstract type). References are URLs that are relative to 3266 * http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference 3267 * to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are 3268 * only allowed in logical models. 3269 */ 3270 public String getType() { 3271 return this.type == null ? null : this.type.getValue(); 3272 } 3273 3274 /** 3275 * @param value The type this structure describes. If the derivation kind is 3276 * 'specialization' then this is the master definition for a type, 3277 * and there is always one of these (a data type, an extension, a 3278 * resource, including abstract ones). Otherwise the structure 3279 * definition is a constraint on the stated type (and in this case, 3280 * the type cannot be an abstract type). References are URLs that 3281 * are relative to http://hl7.org/fhir/StructureDefinition e.g. 3282 * "string" is a reference to 3283 * http://hl7.org/fhir/StructureDefinition/string. Absolute URLs 3284 * are only allowed in logical models. 3285 */ 3286 public StructureDefinition setType(String value) { 3287 if (this.type == null) 3288 this.type = new UriType(); 3289 this.type.setValue(value); 3290 return this; 3291 } 3292 3293 /** 3294 * @return {@link #baseDefinition} (An absolute URI that is the base structure 3295 * from which this type is derived, either by specialization or 3296 * constraint.). This is the underlying object with id, value and 3297 * extensions. The accessor "getBaseDefinition" gives direct access to 3298 * the value 3299 */ 3300 public CanonicalType getBaseDefinitionElement() { 3301 if (this.baseDefinition == null) 3302 if (Configuration.errorOnAutoCreate()) 3303 throw new Error("Attempt to auto-create StructureDefinition.baseDefinition"); 3304 else if (Configuration.doAutoCreate()) 3305 this.baseDefinition = new CanonicalType(); // bb 3306 return this.baseDefinition; 3307 } 3308 3309 public boolean hasBaseDefinitionElement() { 3310 return this.baseDefinition != null && !this.baseDefinition.isEmpty(); 3311 } 3312 3313 public boolean hasBaseDefinition() { 3314 return this.baseDefinition != null && !this.baseDefinition.isEmpty(); 3315 } 3316 3317 /** 3318 * @param value {@link #baseDefinition} (An absolute URI that is the base 3319 * structure from which this type is derived, either by 3320 * specialization or constraint.). This is the underlying object 3321 * with id, value and extensions. The accessor "getBaseDefinition" 3322 * gives direct access to the value 3323 */ 3324 public StructureDefinition setBaseDefinitionElement(CanonicalType value) { 3325 this.baseDefinition = value; 3326 return this; 3327 } 3328 3329 /** 3330 * @return An absolute URI that is the base structure from which this type is 3331 * derived, either by specialization or constraint. 3332 */ 3333 public String getBaseDefinition() { 3334 return this.baseDefinition == null ? null : this.baseDefinition.getValue(); 3335 } 3336 3337 /** 3338 * @param value An absolute URI that is the base structure from which this type 3339 * is derived, either by specialization or constraint. 3340 */ 3341 public StructureDefinition setBaseDefinition(String value) { 3342 if (Utilities.noString(value)) 3343 this.baseDefinition = null; 3344 else { 3345 if (this.baseDefinition == null) 3346 this.baseDefinition = new CanonicalType(); 3347 this.baseDefinition.setValue(value); 3348 } 3349 return this; 3350 } 3351 3352 /** 3353 * @return {@link #derivation} (How the type relates to the baseDefinition.). 3354 * This is the underlying object with id, value and extensions. The 3355 * accessor "getDerivation" gives direct access to the value 3356 */ 3357 public Enumeration<TypeDerivationRule> getDerivationElement() { 3358 if (this.derivation == null) 3359 if (Configuration.errorOnAutoCreate()) 3360 throw new Error("Attempt to auto-create StructureDefinition.derivation"); 3361 else if (Configuration.doAutoCreate()) 3362 this.derivation = new Enumeration<TypeDerivationRule>(new TypeDerivationRuleEnumFactory()); // bb 3363 return this.derivation; 3364 } 3365 3366 public boolean hasDerivationElement() { 3367 return this.derivation != null && !this.derivation.isEmpty(); 3368 } 3369 3370 public boolean hasDerivation() { 3371 return this.derivation != null && !this.derivation.isEmpty(); 3372 } 3373 3374 /** 3375 * @param value {@link #derivation} (How the type relates to the 3376 * baseDefinition.). This is the underlying object with id, value 3377 * and extensions. The accessor "getDerivation" gives direct access 3378 * to the value 3379 */ 3380 public StructureDefinition setDerivationElement(Enumeration<TypeDerivationRule> value) { 3381 this.derivation = value; 3382 return this; 3383 } 3384 3385 /** 3386 * @return How the type relates to the baseDefinition. 3387 */ 3388 public TypeDerivationRule getDerivation() { 3389 return this.derivation == null ? null : this.derivation.getValue(); 3390 } 3391 3392 /** 3393 * @param value How the type relates to the baseDefinition. 3394 */ 3395 public StructureDefinition setDerivation(TypeDerivationRule value) { 3396 if (value == null) 3397 this.derivation = null; 3398 else { 3399 if (this.derivation == null) 3400 this.derivation = new Enumeration<TypeDerivationRule>(new TypeDerivationRuleEnumFactory()); 3401 this.derivation.setValue(value); 3402 } 3403 return this; 3404 } 3405 3406 /** 3407 * @return {@link #snapshot} (A snapshot view is expressed in a standalone form 3408 * that can be used and interpreted without considering the base 3409 * StructureDefinition.) 3410 */ 3411 public StructureDefinitionSnapshotComponent getSnapshot() { 3412 if (this.snapshot == null) 3413 if (Configuration.errorOnAutoCreate()) 3414 throw new Error("Attempt to auto-create StructureDefinition.snapshot"); 3415 else if (Configuration.doAutoCreate()) 3416 this.snapshot = new StructureDefinitionSnapshotComponent(); // cc 3417 return this.snapshot; 3418 } 3419 3420 public boolean hasSnapshot() { 3421 return this.snapshot != null && !this.snapshot.isEmpty(); 3422 } 3423 3424 /** 3425 * @param value {@link #snapshot} (A snapshot view is expressed in a standalone 3426 * form that can be used and interpreted without considering the 3427 * base StructureDefinition.) 3428 */ 3429 public StructureDefinition setSnapshot(StructureDefinitionSnapshotComponent value) { 3430 this.snapshot = value; 3431 return this; 3432 } 3433 3434 /** 3435 * @return {@link #differential} (A differential view is expressed relative to 3436 * the base StructureDefinition - a statement of differences that it 3437 * applies.) 3438 */ 3439 public StructureDefinitionDifferentialComponent getDifferential() { 3440 if (this.differential == null) 3441 if (Configuration.errorOnAutoCreate()) 3442 throw new Error("Attempt to auto-create StructureDefinition.differential"); 3443 else if (Configuration.doAutoCreate()) 3444 this.differential = new StructureDefinitionDifferentialComponent(); // cc 3445 return this.differential; 3446 } 3447 3448 public boolean hasDifferential() { 3449 return this.differential != null && !this.differential.isEmpty(); 3450 } 3451 3452 /** 3453 * @param value {@link #differential} (A differential view is expressed relative 3454 * to the base StructureDefinition - a statement of differences 3455 * that it applies.) 3456 */ 3457 public StructureDefinition setDifferential(StructureDefinitionDifferentialComponent value) { 3458 this.differential = value; 3459 return this; 3460 } 3461 3462 protected void listChildren(List<Property> children) { 3463 super.listChildren(children); 3464 children.add(new Property("url", "uri", 3465 "An absolute URI that is used to identify this structure definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this structure definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure definition is stored on different servers.", 3466 0, 1, url)); 3467 children.add(new Property("identifier", "Identifier", 3468 "A formal identifier that is used to identify this structure definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 3469 0, java.lang.Integer.MAX_VALUE, identifier)); 3470 children.add(new Property("version", "string", 3471 "The identifier that is used to identify this version of the structure definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 3472 0, 1, version)); 3473 children.add(new Property("name", "string", 3474 "A natural language name identifying the structure definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 3475 0, 1, name)); 3476 children.add(new Property("title", "string", 3477 "A short, descriptive, user-friendly title for the structure definition.", 0, 1, title)); 3478 children.add(new Property("status", "code", 3479 "The status of this structure definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 3480 children.add(new Property("experimental", "boolean", 3481 "A Boolean value to indicate that this structure definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 3482 0, 1, experimental)); 3483 children.add(new Property("date", "dateTime", 3484 "The date (and optionally time) when the structure definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure definition changes.", 3485 0, 1, date)); 3486 children.add(new Property("publisher", "string", 3487 "The name of the organization or individual that published the structure definition.", 0, 1, publisher)); 3488 children.add(new Property("contact", "ContactDetail", 3489 "Contact details to assist a user in finding and communicating with the publisher.", 0, 3490 java.lang.Integer.MAX_VALUE, contact)); 3491 children.add(new Property("description", "markdown", 3492 "A free text natural language description of the structure definition from a consumer's perspective.", 0, 1, 3493 description)); 3494 children.add(new Property("useContext", "UsageContext", 3495 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate structure definition instances.", 3496 0, java.lang.Integer.MAX_VALUE, useContext)); 3497 children.add(new Property("jurisdiction", "CodeableConcept", 3498 "A legal or geographic region in which the structure definition is intended to be used.", 0, 3499 java.lang.Integer.MAX_VALUE, jurisdiction)); 3500 children.add(new Property("purpose", "markdown", 3501 "Explanation of why this structure definition is needed and why it has been designed as it has.", 0, 1, 3502 purpose)); 3503 children.add(new Property("copyright", "markdown", 3504 "A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure definition.", 3505 0, 1, copyright)); 3506 children.add(new Property("keyword", "Coding", 3507 "A set of key words or terms from external terminologies that may be used to assist with indexing and searching of templates nby describing the use of this structure definition, or the content it describes.", 3508 0, java.lang.Integer.MAX_VALUE, keyword)); 3509 children.add(new Property("fhirVersion", "code", 3510 "The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.0.1. for this version.", 3511 0, 1, fhirVersion)); 3512 children.add(new Property("mapping", "", "An external specification that the content is mapped to.", 0, 3513 java.lang.Integer.MAX_VALUE, mapping)); 3514 children.add( 3515 new Property("kind", "code", "Defines the kind of structure that this definition is describing.", 0, 1, kind)); 3516 children.add(new Property("abstract", "boolean", 3517 "Whether structure this definition describes is abstract or not - that is, whether the structure is not intended to be instantiated. For Resources and Data types, abstract types will never be exchanged between systems.", 3518 0, 1, abstract_)); 3519 children.add(new Property("context", "", 3520 "Identifies the types of resource or data type elements to which the extension can be applied.", 0, 3521 java.lang.Integer.MAX_VALUE, context)); 3522 children.add(new Property("contextInvariant", "string", 3523 "A set of rules as FHIRPath Invariants about when the extension can be used (e.g. co-occurrence variants for the extension). All the rules must be true.", 3524 0, java.lang.Integer.MAX_VALUE, contextInvariant)); 3525 children.add(new Property("type", "uri", 3526 "The type this structure describes. If the derivation kind is 'specialization' then this is the master definition for a type, and there is always one of these (a data type, an extension, a resource, including abstract ones). Otherwise the structure definition is a constraint on the stated type (and in this case, the type cannot be an abstract type). References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. \"string\" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models.", 3527 0, 1, type)); 3528 children.add(new Property("baseDefinition", "canonical(StructureDefinition)", 3529 "An absolute URI that is the base structure from which this type is derived, either by specialization or constraint.", 3530 0, 1, baseDefinition)); 3531 children.add(new Property("derivation", "code", "How the type relates to the baseDefinition.", 0, 1, derivation)); 3532 children.add(new Property("snapshot", "", 3533 "A snapshot view is expressed in a standalone form that can be used and interpreted without considering the base StructureDefinition.", 3534 0, 1, snapshot)); 3535 children.add(new Property("differential", "", 3536 "A differential view is expressed relative to the base StructureDefinition - a statement of differences that it applies.", 3537 0, 1, differential)); 3538 } 3539 3540 @Override 3541 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3542 switch (_hash) { 3543 case 116079: 3544 /* url */ return new Property("url", "uri", 3545 "An absolute URI that is used to identify this structure definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this structure definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure definition is stored on different servers.", 3546 0, 1, url); 3547 case -1618432855: 3548 /* identifier */ return new Property("identifier", "Identifier", 3549 "A formal identifier that is used to identify this structure definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 3550 0, java.lang.Integer.MAX_VALUE, identifier); 3551 case 351608024: 3552 /* version */ return new Property("version", "string", 3553 "The identifier that is used to identify this version of the structure definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 3554 0, 1, version); 3555 case 3373707: 3556 /* name */ return new Property("name", "string", 3557 "A natural language name identifying the structure definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 3558 0, 1, name); 3559 case 110371416: 3560 /* title */ return new Property("title", "string", 3561 "A short, descriptive, user-friendly title for the structure definition.", 0, 1, title); 3562 case -892481550: 3563 /* status */ return new Property("status", "code", 3564 "The status of this structure definition. Enables tracking the life-cycle of the content.", 0, 1, status); 3565 case -404562712: 3566 /* experimental */ return new Property("experimental", "boolean", 3567 "A Boolean value to indicate that this structure definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 3568 0, 1, experimental); 3569 case 3076014: 3570 /* date */ return new Property("date", "dateTime", 3571 "The date (and optionally time) when the structure definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure definition changes.", 3572 0, 1, date); 3573 case 1447404028: 3574 /* publisher */ return new Property("publisher", "string", 3575 "The name of the organization or individual that published the structure definition.", 0, 1, publisher); 3576 case 951526432: 3577 /* contact */ return new Property("contact", "ContactDetail", 3578 "Contact details to assist a user in finding and communicating with the publisher.", 0, 3579 java.lang.Integer.MAX_VALUE, contact); 3580 case -1724546052: 3581 /* description */ return new Property("description", "markdown", 3582 "A free text natural language description of the structure definition from a consumer's perspective.", 0, 1, 3583 description); 3584 case -669707736: 3585 /* useContext */ return new Property("useContext", "UsageContext", 3586 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate structure definition instances.", 3587 0, java.lang.Integer.MAX_VALUE, useContext); 3588 case -507075711: 3589 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 3590 "A legal or geographic region in which the structure definition is intended to be used.", 0, 3591 java.lang.Integer.MAX_VALUE, jurisdiction); 3592 case -220463842: 3593 /* purpose */ return new Property("purpose", "markdown", 3594 "Explanation of why this structure definition is needed and why it has been designed as it has.", 0, 1, 3595 purpose); 3596 case 1522889671: 3597 /* copyright */ return new Property("copyright", "markdown", 3598 "A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure definition.", 3599 0, 1, copyright); 3600 case -814408215: 3601 /* keyword */ return new Property("keyword", "Coding", 3602 "A set of key words or terms from external terminologies that may be used to assist with indexing and searching of templates nby describing the use of this structure definition, or the content it describes.", 3603 0, java.lang.Integer.MAX_VALUE, keyword); 3604 case 461006061: 3605 /* fhirVersion */ return new Property("fhirVersion", "code", 3606 "The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.0.1. for this version.", 3607 0, 1, fhirVersion); 3608 case 837556430: 3609 /* mapping */ return new Property("mapping", "", "An external specification that the content is mapped to.", 0, 3610 java.lang.Integer.MAX_VALUE, mapping); 3611 case 3292052: 3612 /* kind */ return new Property("kind", "code", 3613 "Defines the kind of structure that this definition is describing.", 0, 1, kind); 3614 case 1732898850: 3615 /* abstract */ return new Property("abstract", "boolean", 3616 "Whether structure this definition describes is abstract or not - that is, whether the structure is not intended to be instantiated. For Resources and Data types, abstract types will never be exchanged between systems.", 3617 0, 1, abstract_); 3618 case 951530927: 3619 /* context */ return new Property("context", "", 3620 "Identifies the types of resource or data type elements to which the extension can be applied.", 0, 3621 java.lang.Integer.MAX_VALUE, context); 3622 case -802505007: 3623 /* contextInvariant */ return new Property("contextInvariant", "string", 3624 "A set of rules as FHIRPath Invariants about when the extension can be used (e.g. co-occurrence variants for the extension). All the rules must be true.", 3625 0, java.lang.Integer.MAX_VALUE, contextInvariant); 3626 case 3575610: 3627 /* type */ return new Property("type", "uri", 3628 "The type this structure describes. If the derivation kind is 'specialization' then this is the master definition for a type, and there is always one of these (a data type, an extension, a resource, including abstract ones). Otherwise the structure definition is a constraint on the stated type (and in this case, the type cannot be an abstract type). References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. \"string\" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models.", 3629 0, 1, type); 3630 case 1139771140: 3631 /* baseDefinition */ return new Property("baseDefinition", "canonical(StructureDefinition)", 3632 "An absolute URI that is the base structure from which this type is derived, either by specialization or constraint.", 3633 0, 1, baseDefinition); 3634 case -1353885513: 3635 /* derivation */ return new Property("derivation", "code", "How the type relates to the baseDefinition.", 0, 1, 3636 derivation); 3637 case 284874180: 3638 /* snapshot */ return new Property("snapshot", "", 3639 "A snapshot view is expressed in a standalone form that can be used and interpreted without considering the base StructureDefinition.", 3640 0, 1, snapshot); 3641 case -1196150917: 3642 /* differential */ return new Property("differential", "", 3643 "A differential view is expressed relative to the base StructureDefinition - a statement of differences that it applies.", 3644 0, 1, differential); 3645 default: 3646 return super.getNamedProperty(_hash, _name, _checkValid); 3647 } 3648 3649 } 3650 3651 @Override 3652 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3653 switch (hash) { 3654 case 116079: 3655 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 3656 case -1618432855: 3657 /* identifier */ return this.identifier == null ? new Base[0] 3658 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3659 case 351608024: 3660 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 3661 case 3373707: 3662 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 3663 case 110371416: 3664 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 3665 case -892481550: 3666 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 3667 case -404562712: 3668 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 3669 case 3076014: 3670 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 3671 case 1447404028: 3672 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 3673 case 951526432: 3674 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 3675 case -1724546052: 3676 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 3677 case -669707736: 3678 /* useContext */ return this.useContext == null ? new Base[0] 3679 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 3680 case -507075711: 3681 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 3682 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 3683 case -220463842: 3684 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 3685 case 1522889671: 3686 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 3687 case -814408215: 3688 /* keyword */ return this.keyword == null ? new Base[0] : this.keyword.toArray(new Base[this.keyword.size()]); // Coding 3689 case 461006061: 3690 /* fhirVersion */ return this.fhirVersion == null ? new Base[0] : new Base[] { this.fhirVersion }; // Enumeration<FHIRVersion> 3691 case 837556430: 3692 /* mapping */ return this.mapping == null ? new Base[0] : this.mapping.toArray(new Base[this.mapping.size()]); // StructureDefinitionMappingComponent 3693 case 3292052: 3694 /* kind */ return this.kind == null ? new Base[0] : new Base[] { this.kind }; // Enumeration<StructureDefinitionKind> 3695 case 1732898850: 3696 /* abstract */ return this.abstract_ == null ? new Base[0] : new Base[] { this.abstract_ }; // BooleanType 3697 case 951530927: 3698 /* context */ return this.context == null ? new Base[0] : this.context.toArray(new Base[this.context.size()]); // StructureDefinitionContextComponent 3699 case -802505007: 3700 /* contextInvariant */ return this.contextInvariant == null ? new Base[0] 3701 : this.contextInvariant.toArray(new Base[this.contextInvariant.size()]); // StringType 3702 case 3575610: 3703 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // UriType 3704 case 1139771140: 3705 /* baseDefinition */ return this.baseDefinition == null ? new Base[0] : new Base[] { this.baseDefinition }; // CanonicalType 3706 case -1353885513: 3707 /* derivation */ return this.derivation == null ? new Base[0] : new Base[] { this.derivation }; // Enumeration<TypeDerivationRule> 3708 case 284874180: 3709 /* snapshot */ return this.snapshot == null ? new Base[0] : new Base[] { this.snapshot }; // StructureDefinitionSnapshotComponent 3710 case -1196150917: 3711 /* differential */ return this.differential == null ? new Base[0] : new Base[] { this.differential }; // StructureDefinitionDifferentialComponent 3712 default: 3713 return super.getProperty(hash, name, checkValid); 3714 } 3715 3716 } 3717 3718 @Override 3719 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3720 switch (hash) { 3721 case 116079: // url 3722 this.url = castToUri(value); // UriType 3723 return value; 3724 case -1618432855: // identifier 3725 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3726 return value; 3727 case 351608024: // version 3728 this.version = castToString(value); // StringType 3729 return value; 3730 case 3373707: // name 3731 this.name = castToString(value); // StringType 3732 return value; 3733 case 110371416: // title 3734 this.title = castToString(value); // StringType 3735 return value; 3736 case -892481550: // status 3737 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 3738 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3739 return value; 3740 case -404562712: // experimental 3741 this.experimental = castToBoolean(value); // BooleanType 3742 return value; 3743 case 3076014: // date 3744 this.date = castToDateTime(value); // DateTimeType 3745 return value; 3746 case 1447404028: // publisher 3747 this.publisher = castToString(value); // StringType 3748 return value; 3749 case 951526432: // contact 3750 this.getContact().add(castToContactDetail(value)); // ContactDetail 3751 return value; 3752 case -1724546052: // description 3753 this.description = castToMarkdown(value); // MarkdownType 3754 return value; 3755 case -669707736: // useContext 3756 this.getUseContext().add(castToUsageContext(value)); // UsageContext 3757 return value; 3758 case -507075711: // jurisdiction 3759 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 3760 return value; 3761 case -220463842: // purpose 3762 this.purpose = castToMarkdown(value); // MarkdownType 3763 return value; 3764 case 1522889671: // copyright 3765 this.copyright = castToMarkdown(value); // MarkdownType 3766 return value; 3767 case -814408215: // keyword 3768 this.getKeyword().add(castToCoding(value)); // Coding 3769 return value; 3770 case 461006061: // fhirVersion 3771 value = new FHIRVersionEnumFactory().fromType(castToCode(value)); 3772 this.fhirVersion = (Enumeration) value; // Enumeration<FHIRVersion> 3773 return value; 3774 case 837556430: // mapping 3775 this.getMapping().add((StructureDefinitionMappingComponent) value); // StructureDefinitionMappingComponent 3776 return value; 3777 case 3292052: // kind 3778 value = new StructureDefinitionKindEnumFactory().fromType(castToCode(value)); 3779 this.kind = (Enumeration) value; // Enumeration<StructureDefinitionKind> 3780 return value; 3781 case 1732898850: // abstract 3782 this.abstract_ = castToBoolean(value); // BooleanType 3783 return value; 3784 case 951530927: // context 3785 this.getContext().add((StructureDefinitionContextComponent) value); // StructureDefinitionContextComponent 3786 return value; 3787 case -802505007: // contextInvariant 3788 this.getContextInvariant().add(castToString(value)); // StringType 3789 return value; 3790 case 3575610: // type 3791 this.type = castToUri(value); // UriType 3792 return value; 3793 case 1139771140: // baseDefinition 3794 this.baseDefinition = castToCanonical(value); // CanonicalType 3795 return value; 3796 case -1353885513: // derivation 3797 value = new TypeDerivationRuleEnumFactory().fromType(castToCode(value)); 3798 this.derivation = (Enumeration) value; // Enumeration<TypeDerivationRule> 3799 return value; 3800 case 284874180: // snapshot 3801 this.snapshot = (StructureDefinitionSnapshotComponent) value; // StructureDefinitionSnapshotComponent 3802 return value; 3803 case -1196150917: // differential 3804 this.differential = (StructureDefinitionDifferentialComponent) value; // StructureDefinitionDifferentialComponent 3805 return value; 3806 default: 3807 return super.setProperty(hash, name, value); 3808 } 3809 3810 } 3811 3812 @Override 3813 public Base setProperty(String name, Base value) throws FHIRException { 3814 if (name.equals("url")) { 3815 this.url = castToUri(value); // UriType 3816 } else if (name.equals("identifier")) { 3817 this.getIdentifier().add(castToIdentifier(value)); 3818 } else if (name.equals("version")) { 3819 this.version = castToString(value); // StringType 3820 } else if (name.equals("name")) { 3821 this.name = castToString(value); // StringType 3822 } else if (name.equals("title")) { 3823 this.title = castToString(value); // StringType 3824 } else if (name.equals("status")) { 3825 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 3826 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3827 } else if (name.equals("experimental")) { 3828 this.experimental = castToBoolean(value); // BooleanType 3829 } else if (name.equals("date")) { 3830 this.date = castToDateTime(value); // DateTimeType 3831 } else if (name.equals("publisher")) { 3832 this.publisher = castToString(value); // StringType 3833 } else if (name.equals("contact")) { 3834 this.getContact().add(castToContactDetail(value)); 3835 } else if (name.equals("description")) { 3836 this.description = castToMarkdown(value); // MarkdownType 3837 } else if (name.equals("useContext")) { 3838 this.getUseContext().add(castToUsageContext(value)); 3839 } else if (name.equals("jurisdiction")) { 3840 this.getJurisdiction().add(castToCodeableConcept(value)); 3841 } else if (name.equals("purpose")) { 3842 this.purpose = castToMarkdown(value); // MarkdownType 3843 } else if (name.equals("copyright")) { 3844 this.copyright = castToMarkdown(value); // MarkdownType 3845 } else if (name.equals("keyword")) { 3846 this.getKeyword().add(castToCoding(value)); 3847 } else if (name.equals("fhirVersion")) { 3848 value = new FHIRVersionEnumFactory().fromType(castToCode(value)); 3849 this.fhirVersion = (Enumeration) value; // Enumeration<FHIRVersion> 3850 } else if (name.equals("mapping")) { 3851 this.getMapping().add((StructureDefinitionMappingComponent) value); 3852 } else if (name.equals("kind")) { 3853 value = new StructureDefinitionKindEnumFactory().fromType(castToCode(value)); 3854 this.kind = (Enumeration) value; // Enumeration<StructureDefinitionKind> 3855 } else if (name.equals("abstract")) { 3856 this.abstract_ = castToBoolean(value); // BooleanType 3857 } else if (name.equals("context")) { 3858 this.getContext().add((StructureDefinitionContextComponent) value); 3859 } else if (name.equals("contextInvariant")) { 3860 this.getContextInvariant().add(castToString(value)); 3861 } else if (name.equals("type")) { 3862 this.type = castToUri(value); // UriType 3863 } else if (name.equals("baseDefinition")) { 3864 this.baseDefinition = castToCanonical(value); // CanonicalType 3865 } else if (name.equals("derivation")) { 3866 value = new TypeDerivationRuleEnumFactory().fromType(castToCode(value)); 3867 this.derivation = (Enumeration) value; // Enumeration<TypeDerivationRule> 3868 } else if (name.equals("snapshot")) { 3869 this.snapshot = (StructureDefinitionSnapshotComponent) value; // StructureDefinitionSnapshotComponent 3870 } else if (name.equals("differential")) { 3871 this.differential = (StructureDefinitionDifferentialComponent) value; // StructureDefinitionDifferentialComponent 3872 } else 3873 return super.setProperty(name, value); 3874 return value; 3875 } 3876 3877 @Override 3878 public void removeChild(String name, Base value) throws FHIRException { 3879 if (name.equals("url")) { 3880 this.url = null; 3881 } else if (name.equals("identifier")) { 3882 this.getIdentifier().remove(castToIdentifier(value)); 3883 } else if (name.equals("version")) { 3884 this.version = null; 3885 } else if (name.equals("name")) { 3886 this.name = null; 3887 } else if (name.equals("title")) { 3888 this.title = null; 3889 } else if (name.equals("status")) { 3890 this.status = null; 3891 } else if (name.equals("experimental")) { 3892 this.experimental = null; 3893 } else if (name.equals("date")) { 3894 this.date = null; 3895 } else if (name.equals("publisher")) { 3896 this.publisher = null; 3897 } else if (name.equals("contact")) { 3898 this.getContact().remove(castToContactDetail(value)); 3899 } else if (name.equals("description")) { 3900 this.description = null; 3901 } else if (name.equals("useContext")) { 3902 this.getUseContext().remove(castToUsageContext(value)); 3903 } else if (name.equals("jurisdiction")) { 3904 this.getJurisdiction().remove(castToCodeableConcept(value)); 3905 } else if (name.equals("purpose")) { 3906 this.purpose = null; 3907 } else if (name.equals("copyright")) { 3908 this.copyright = null; 3909 } else if (name.equals("keyword")) { 3910 this.getKeyword().remove(castToCoding(value)); 3911 } else if (name.equals("fhirVersion")) { 3912 this.fhirVersion = null; 3913 } else if (name.equals("mapping")) { 3914 this.getMapping().remove((StructureDefinitionMappingComponent) value); 3915 } else if (name.equals("kind")) { 3916 this.kind = null; 3917 } else if (name.equals("abstract")) { 3918 this.abstract_ = null; 3919 } else if (name.equals("context")) { 3920 this.getContext().remove((StructureDefinitionContextComponent) value); 3921 } else if (name.equals("contextInvariant")) { 3922 this.getContextInvariant().remove(castToString(value)); 3923 } else if (name.equals("type")) { 3924 this.type = null; 3925 } else if (name.equals("baseDefinition")) { 3926 this.baseDefinition = null; 3927 } else if (name.equals("derivation")) { 3928 this.derivation = null; 3929 } else if (name.equals("snapshot")) { 3930 this.snapshot = (StructureDefinitionSnapshotComponent) value; // StructureDefinitionSnapshotComponent 3931 } else if (name.equals("differential")) { 3932 this.differential = (StructureDefinitionDifferentialComponent) value; // StructureDefinitionDifferentialComponent 3933 } else 3934 super.removeChild(name, value); 3935 3936 } 3937 3938 @Override 3939 public Base makeProperty(int hash, String name) throws FHIRException { 3940 switch (hash) { 3941 case 116079: 3942 return getUrlElement(); 3943 case -1618432855: 3944 return addIdentifier(); 3945 case 351608024: 3946 return getVersionElement(); 3947 case 3373707: 3948 return getNameElement(); 3949 case 110371416: 3950 return getTitleElement(); 3951 case -892481550: 3952 return getStatusElement(); 3953 case -404562712: 3954 return getExperimentalElement(); 3955 case 3076014: 3956 return getDateElement(); 3957 case 1447404028: 3958 return getPublisherElement(); 3959 case 951526432: 3960 return addContact(); 3961 case -1724546052: 3962 return getDescriptionElement(); 3963 case -669707736: 3964 return addUseContext(); 3965 case -507075711: 3966 return addJurisdiction(); 3967 case -220463842: 3968 return getPurposeElement(); 3969 case 1522889671: 3970 return getCopyrightElement(); 3971 case -814408215: 3972 return addKeyword(); 3973 case 461006061: 3974 return getFhirVersionElement(); 3975 case 837556430: 3976 return addMapping(); 3977 case 3292052: 3978 return getKindElement(); 3979 case 1732898850: 3980 return getAbstractElement(); 3981 case 951530927: 3982 return addContext(); 3983 case -802505007: 3984 return addContextInvariantElement(); 3985 case 3575610: 3986 return getTypeElement(); 3987 case 1139771140: 3988 return getBaseDefinitionElement(); 3989 case -1353885513: 3990 return getDerivationElement(); 3991 case 284874180: 3992 return getSnapshot(); 3993 case -1196150917: 3994 return getDifferential(); 3995 default: 3996 return super.makeProperty(hash, name); 3997 } 3998 3999 } 4000 4001 @Override 4002 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4003 switch (hash) { 4004 case 116079: 4005 /* url */ return new String[] { "uri" }; 4006 case -1618432855: 4007 /* identifier */ return new String[] { "Identifier" }; 4008 case 351608024: 4009 /* version */ return new String[] { "string" }; 4010 case 3373707: 4011 /* name */ return new String[] { "string" }; 4012 case 110371416: 4013 /* title */ return new String[] { "string" }; 4014 case -892481550: 4015 /* status */ return new String[] { "code" }; 4016 case -404562712: 4017 /* experimental */ return new String[] { "boolean" }; 4018 case 3076014: 4019 /* date */ return new String[] { "dateTime" }; 4020 case 1447404028: 4021 /* publisher */ return new String[] { "string" }; 4022 case 951526432: 4023 /* contact */ return new String[] { "ContactDetail" }; 4024 case -1724546052: 4025 /* description */ return new String[] { "markdown" }; 4026 case -669707736: 4027 /* useContext */ return new String[] { "UsageContext" }; 4028 case -507075711: 4029 /* jurisdiction */ return new String[] { "CodeableConcept" }; 4030 case -220463842: 4031 /* purpose */ return new String[] { "markdown" }; 4032 case 1522889671: 4033 /* copyright */ return new String[] { "markdown" }; 4034 case -814408215: 4035 /* keyword */ return new String[] { "Coding" }; 4036 case 461006061: 4037 /* fhirVersion */ return new String[] { "code" }; 4038 case 837556430: 4039 /* mapping */ return new String[] {}; 4040 case 3292052: 4041 /* kind */ return new String[] { "code" }; 4042 case 1732898850: 4043 /* abstract */ return new String[] { "boolean" }; 4044 case 951530927: 4045 /* context */ return new String[] {}; 4046 case -802505007: 4047 /* contextInvariant */ return new String[] { "string" }; 4048 case 3575610: 4049 /* type */ return new String[] { "uri" }; 4050 case 1139771140: 4051 /* baseDefinition */ return new String[] { "canonical" }; 4052 case -1353885513: 4053 /* derivation */ return new String[] { "code" }; 4054 case 284874180: 4055 /* snapshot */ return new String[] {}; 4056 case -1196150917: 4057 /* differential */ return new String[] {}; 4058 default: 4059 return super.getTypesForProperty(hash, name); 4060 } 4061 4062 } 4063 4064 @Override 4065 public Base addChild(String name) throws FHIRException { 4066 if (name.equals("url")) { 4067 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.url"); 4068 } else if (name.equals("identifier")) { 4069 return addIdentifier(); 4070 } else if (name.equals("version")) { 4071 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.version"); 4072 } else if (name.equals("name")) { 4073 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.name"); 4074 } else if (name.equals("title")) { 4075 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.title"); 4076 } else if (name.equals("status")) { 4077 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.status"); 4078 } else if (name.equals("experimental")) { 4079 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.experimental"); 4080 } else if (name.equals("date")) { 4081 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.date"); 4082 } else if (name.equals("publisher")) { 4083 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.publisher"); 4084 } else if (name.equals("contact")) { 4085 return addContact(); 4086 } else if (name.equals("description")) { 4087 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.description"); 4088 } else if (name.equals("useContext")) { 4089 return addUseContext(); 4090 } else if (name.equals("jurisdiction")) { 4091 return addJurisdiction(); 4092 } else if (name.equals("purpose")) { 4093 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.purpose"); 4094 } else if (name.equals("copyright")) { 4095 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.copyright"); 4096 } else if (name.equals("keyword")) { 4097 return addKeyword(); 4098 } else if (name.equals("fhirVersion")) { 4099 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.fhirVersion"); 4100 } else if (name.equals("mapping")) { 4101 return addMapping(); 4102 } else if (name.equals("kind")) { 4103 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.kind"); 4104 } else if (name.equals("abstract")) { 4105 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.abstract"); 4106 } else if (name.equals("context")) { 4107 return addContext(); 4108 } else if (name.equals("contextInvariant")) { 4109 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.contextInvariant"); 4110 } else if (name.equals("type")) { 4111 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.type"); 4112 } else if (name.equals("baseDefinition")) { 4113 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.baseDefinition"); 4114 } else if (name.equals("derivation")) { 4115 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.derivation"); 4116 } else if (name.equals("snapshot")) { 4117 this.snapshot = new StructureDefinitionSnapshotComponent(); 4118 return this.snapshot; 4119 } else if (name.equals("differential")) { 4120 this.differential = new StructureDefinitionDifferentialComponent(); 4121 return this.differential; 4122 } else 4123 return super.addChild(name); 4124 } 4125 4126 public String fhirType() { 4127 return "StructureDefinition"; 4128 4129 } 4130 4131 public StructureDefinition copy() { 4132 StructureDefinition dst = new StructureDefinition(); 4133 copyValues(dst); 4134 return dst; 4135 } 4136 4137 public void copyValues(StructureDefinition dst) { 4138 super.copyValues(dst); 4139 dst.url = url == null ? null : url.copy(); 4140 if (identifier != null) { 4141 dst.identifier = new ArrayList<Identifier>(); 4142 for (Identifier i : identifier) 4143 dst.identifier.add(i.copy()); 4144 } 4145 ; 4146 dst.version = version == null ? null : version.copy(); 4147 dst.name = name == null ? null : name.copy(); 4148 dst.title = title == null ? null : title.copy(); 4149 dst.status = status == null ? null : status.copy(); 4150 dst.experimental = experimental == null ? null : experimental.copy(); 4151 dst.date = date == null ? null : date.copy(); 4152 dst.publisher = publisher == null ? null : publisher.copy(); 4153 if (contact != null) { 4154 dst.contact = new ArrayList<ContactDetail>(); 4155 for (ContactDetail i : contact) 4156 dst.contact.add(i.copy()); 4157 } 4158 ; 4159 dst.description = description == null ? null : description.copy(); 4160 if (useContext != null) { 4161 dst.useContext = new ArrayList<UsageContext>(); 4162 for (UsageContext i : useContext) 4163 dst.useContext.add(i.copy()); 4164 } 4165 ; 4166 if (jurisdiction != null) { 4167 dst.jurisdiction = new ArrayList<CodeableConcept>(); 4168 for (CodeableConcept i : jurisdiction) 4169 dst.jurisdiction.add(i.copy()); 4170 } 4171 ; 4172 dst.purpose = purpose == null ? null : purpose.copy(); 4173 dst.copyright = copyright == null ? null : copyright.copy(); 4174 if (keyword != null) { 4175 dst.keyword = new ArrayList<Coding>(); 4176 for (Coding i : keyword) 4177 dst.keyword.add(i.copy()); 4178 } 4179 ; 4180 dst.fhirVersion = fhirVersion == null ? null : fhirVersion.copy(); 4181 if (mapping != null) { 4182 dst.mapping = new ArrayList<StructureDefinitionMappingComponent>(); 4183 for (StructureDefinitionMappingComponent i : mapping) 4184 dst.mapping.add(i.copy()); 4185 } 4186 ; 4187 dst.kind = kind == null ? null : kind.copy(); 4188 dst.abstract_ = abstract_ == null ? null : abstract_.copy(); 4189 if (context != null) { 4190 dst.context = new ArrayList<StructureDefinitionContextComponent>(); 4191 for (StructureDefinitionContextComponent i : context) 4192 dst.context.add(i.copy()); 4193 } 4194 ; 4195 if (contextInvariant != null) { 4196 dst.contextInvariant = new ArrayList<StringType>(); 4197 for (StringType i : contextInvariant) 4198 dst.contextInvariant.add(i.copy()); 4199 } 4200 ; 4201 dst.type = type == null ? null : type.copy(); 4202 dst.baseDefinition = baseDefinition == null ? null : baseDefinition.copy(); 4203 dst.derivation = derivation == null ? null : derivation.copy(); 4204 dst.snapshot = snapshot == null ? null : snapshot.copy(); 4205 dst.differential = differential == null ? null : differential.copy(); 4206 } 4207 4208 protected StructureDefinition typedCopy() { 4209 return copy(); 4210 } 4211 4212 @Override 4213 public boolean equalsDeep(Base other_) { 4214 if (!super.equalsDeep(other_)) 4215 return false; 4216 if (!(other_ instanceof StructureDefinition)) 4217 return false; 4218 StructureDefinition o = (StructureDefinition) other_; 4219 return compareDeep(identifier, o.identifier, true) && compareDeep(purpose, o.purpose, true) 4220 && compareDeep(copyright, o.copyright, true) && compareDeep(keyword, o.keyword, true) 4221 && compareDeep(fhirVersion, o.fhirVersion, true) && compareDeep(mapping, o.mapping, true) 4222 && compareDeep(kind, o.kind, true) && compareDeep(abstract_, o.abstract_, true) 4223 && compareDeep(context, o.context, true) && compareDeep(contextInvariant, o.contextInvariant, true) 4224 && compareDeep(type, o.type, true) && compareDeep(baseDefinition, o.baseDefinition, true) 4225 && compareDeep(derivation, o.derivation, true) && compareDeep(snapshot, o.snapshot, true) 4226 && compareDeep(differential, o.differential, true); 4227 } 4228 4229 @Override 4230 public boolean equalsShallow(Base other_) { 4231 if (!super.equalsShallow(other_)) 4232 return false; 4233 if (!(other_ instanceof StructureDefinition)) 4234 return false; 4235 StructureDefinition o = (StructureDefinition) other_; 4236 return compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) 4237 && compareValues(fhirVersion, o.fhirVersion, true) && compareValues(kind, o.kind, true) 4238 && compareValues(abstract_, o.abstract_, true) && compareValues(contextInvariant, o.contextInvariant, true) 4239 && compareValues(type, o.type, true) && compareValues(derivation, o.derivation, true); 4240 } 4241 4242 public boolean isEmpty() { 4243 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, purpose, copyright, keyword, fhirVersion, 4244 mapping, kind, abstract_, context, contextInvariant, type, baseDefinition, derivation, snapshot, differential); 4245 } 4246 4247 @Override 4248 public ResourceType getResourceType() { 4249 return ResourceType.StructureDefinition; 4250 } 4251 4252 /** 4253 * Search parameter: <b>date</b> 4254 * <p> 4255 * Description: <b>The structure definition publication date</b><br> 4256 * Type: <b>date</b><br> 4257 * Path: <b>StructureDefinition.date</b><br> 4258 * </p> 4259 */ 4260 @SearchParamDefinition(name = "date", path = "StructureDefinition.date", description = "The structure definition publication date", type = "date") 4261 public static final String SP_DATE = "date"; 4262 /** 4263 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4264 * <p> 4265 * Description: <b>The structure definition publication date</b><br> 4266 * Type: <b>date</b><br> 4267 * Path: <b>StructureDefinition.date</b><br> 4268 * </p> 4269 */ 4270 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 4271 SP_DATE); 4272 4273 /** 4274 * Search parameter: <b>context-type-value</b> 4275 * <p> 4276 * Description: <b>A use context type and value assigned to the structure 4277 * definition</b><br> 4278 * Type: <b>composite</b><br> 4279 * Path: <b></b><br> 4280 * </p> 4281 */ 4282 @SearchParamDefinition(name = "context-type-value", path = "StructureDefinition.useContext", description = "A use context type and value assigned to the structure definition", type = "composite", compositeOf = { 4283 "context-type", "context" }) 4284 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 4285 /** 4286 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 4287 * <p> 4288 * Description: <b>A use context type and value assigned to the structure 4289 * definition</b><br> 4290 * Type: <b>composite</b><br> 4291 * Path: <b></b><br> 4292 * </p> 4293 */ 4294 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 4295 SP_CONTEXT_TYPE_VALUE); 4296 4297 /** 4298 * Search parameter: <b>jurisdiction</b> 4299 * <p> 4300 * Description: <b>Intended jurisdiction for the structure definition</b><br> 4301 * Type: <b>token</b><br> 4302 * Path: <b>StructureDefinition.jurisdiction</b><br> 4303 * </p> 4304 */ 4305 @SearchParamDefinition(name = "jurisdiction", path = "StructureDefinition.jurisdiction", description = "Intended jurisdiction for the structure definition", type = "token") 4306 public static final String SP_JURISDICTION = "jurisdiction"; 4307 /** 4308 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 4309 * <p> 4310 * Description: <b>Intended jurisdiction for the structure definition</b><br> 4311 * Type: <b>token</b><br> 4312 * Path: <b>StructureDefinition.jurisdiction</b><br> 4313 * </p> 4314 */ 4315 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4316 SP_JURISDICTION); 4317 4318 /** 4319 * Search parameter: <b>description</b> 4320 * <p> 4321 * Description: <b>The description of the structure definition</b><br> 4322 * Type: <b>string</b><br> 4323 * Path: <b>StructureDefinition.description</b><br> 4324 * </p> 4325 */ 4326 @SearchParamDefinition(name = "description", path = "StructureDefinition.description", description = "The description of the structure definition", type = "string") 4327 public static final String SP_DESCRIPTION = "description"; 4328 /** 4329 * <b>Fluent Client</b> search parameter constant for <b>description</b> 4330 * <p> 4331 * Description: <b>The description of the structure definition</b><br> 4332 * Type: <b>string</b><br> 4333 * Path: <b>StructureDefinition.description</b><br> 4334 * </p> 4335 */ 4336 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 4337 SP_DESCRIPTION); 4338 4339 /** 4340 * Search parameter: <b>context-type</b> 4341 * <p> 4342 * Description: <b>A type of use context assigned to the structure 4343 * definition</b><br> 4344 * Type: <b>token</b><br> 4345 * Path: <b>StructureDefinition.useContext.code</b><br> 4346 * </p> 4347 */ 4348 @SearchParamDefinition(name = "context-type", path = "StructureDefinition.useContext.code", description = "A type of use context assigned to the structure definition", type = "token") 4349 public static final String SP_CONTEXT_TYPE = "context-type"; 4350 /** 4351 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 4352 * <p> 4353 * Description: <b>A type of use context assigned to the structure 4354 * definition</b><br> 4355 * Type: <b>token</b><br> 4356 * Path: <b>StructureDefinition.useContext.code</b><br> 4357 * </p> 4358 */ 4359 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4360 SP_CONTEXT_TYPE); 4361 4362 /** 4363 * Search parameter: <b>experimental</b> 4364 * <p> 4365 * Description: <b>For testing purposes, not real usage</b><br> 4366 * Type: <b>token</b><br> 4367 * Path: <b>StructureDefinition.experimental</b><br> 4368 * </p> 4369 */ 4370 @SearchParamDefinition(name = "experimental", path = "StructureDefinition.experimental", description = "For testing purposes, not real usage", type = "token") 4371 public static final String SP_EXPERIMENTAL = "experimental"; 4372 /** 4373 * <b>Fluent Client</b> search parameter constant for <b>experimental</b> 4374 * <p> 4375 * Description: <b>For testing purposes, not real usage</b><br> 4376 * Type: <b>token</b><br> 4377 * Path: <b>StructureDefinition.experimental</b><br> 4378 * </p> 4379 */ 4380 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EXPERIMENTAL = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4381 SP_EXPERIMENTAL); 4382 4383 /** 4384 * Search parameter: <b>title</b> 4385 * <p> 4386 * Description: <b>The human-friendly name of the structure definition</b><br> 4387 * Type: <b>string</b><br> 4388 * Path: <b>StructureDefinition.title</b><br> 4389 * </p> 4390 */ 4391 @SearchParamDefinition(name = "title", path = "StructureDefinition.title", description = "The human-friendly name of the structure definition", type = "string") 4392 public static final String SP_TITLE = "title"; 4393 /** 4394 * <b>Fluent Client</b> search parameter constant for <b>title</b> 4395 * <p> 4396 * Description: <b>The human-friendly name of the structure definition</b><br> 4397 * Type: <b>string</b><br> 4398 * Path: <b>StructureDefinition.title</b><br> 4399 * </p> 4400 */ 4401 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 4402 SP_TITLE); 4403 4404 /** 4405 * Search parameter: <b>type</b> 4406 * <p> 4407 * Description: <b>Type defined or constrained by this structure</b><br> 4408 * Type: <b>uri</b><br> 4409 * Path: <b>StructureDefinition.type</b><br> 4410 * </p> 4411 */ 4412 @SearchParamDefinition(name = "type", path = "StructureDefinition.type", description = "Type defined or constrained by this structure", type = "uri") 4413 public static final String SP_TYPE = "type"; 4414 /** 4415 * <b>Fluent Client</b> search parameter constant for <b>type</b> 4416 * <p> 4417 * Description: <b>Type defined or constrained by this structure</b><br> 4418 * Type: <b>uri</b><br> 4419 * Path: <b>StructureDefinition.type</b><br> 4420 * </p> 4421 */ 4422 public static final ca.uhn.fhir.rest.gclient.UriClientParam TYPE = new ca.uhn.fhir.rest.gclient.UriClientParam( 4423 SP_TYPE); 4424 4425 /** 4426 * Search parameter: <b>context-quantity</b> 4427 * <p> 4428 * Description: <b>A quantity- or range-valued use context assigned to the 4429 * structure definition</b><br> 4430 * Type: <b>quantity</b><br> 4431 * Path: <b>StructureDefinition.useContext.valueQuantity, 4432 * StructureDefinition.useContext.valueRange</b><br> 4433 * </p> 4434 */ 4435 @SearchParamDefinition(name = "context-quantity", path = "(StructureDefinition.useContext.value as Quantity) | (StructureDefinition.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the structure definition", type = "quantity") 4436 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 4437 /** 4438 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 4439 * <p> 4440 * Description: <b>A quantity- or range-valued use context assigned to the 4441 * structure definition</b><br> 4442 * Type: <b>quantity</b><br> 4443 * Path: <b>StructureDefinition.useContext.valueQuantity, 4444 * StructureDefinition.useContext.valueRange</b><br> 4445 * </p> 4446 */ 4447 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 4448 SP_CONTEXT_QUANTITY); 4449 4450 /** 4451 * Search parameter: <b>path</b> 4452 * <p> 4453 * Description: <b>A path that is constrained in the StructureDefinition</b><br> 4454 * Type: <b>token</b><br> 4455 * Path: <b>StructureDefinition.snapshot.element.path, 4456 * StructureDefinition.differential.element.path</b><br> 4457 * </p> 4458 */ 4459 @SearchParamDefinition(name = "path", path = "StructureDefinition.snapshot.element.path | StructureDefinition.differential.element.path", description = "A path that is constrained in the StructureDefinition", type = "token") 4460 public static final String SP_PATH = "path"; 4461 /** 4462 * <b>Fluent Client</b> search parameter constant for <b>path</b> 4463 * <p> 4464 * Description: <b>A path that is constrained in the StructureDefinition</b><br> 4465 * Type: <b>token</b><br> 4466 * Path: <b>StructureDefinition.snapshot.element.path, 4467 * StructureDefinition.differential.element.path</b><br> 4468 * </p> 4469 */ 4470 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PATH = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4471 SP_PATH); 4472 4473 /** 4474 * Search parameter: <b>context</b> 4475 * <p> 4476 * Description: <b>A use context assigned to the structure definition</b><br> 4477 * Type: <b>token</b><br> 4478 * Path: <b>StructureDefinition.useContext.valueCodeableConcept</b><br> 4479 * </p> 4480 */ 4481 @SearchParamDefinition(name = "context", path = "(StructureDefinition.useContext.value as CodeableConcept)", description = "A use context assigned to the structure definition", type = "token") 4482 public static final String SP_CONTEXT = "context"; 4483 /** 4484 * <b>Fluent Client</b> search parameter constant for <b>context</b> 4485 * <p> 4486 * Description: <b>A use context assigned to the structure definition</b><br> 4487 * Type: <b>token</b><br> 4488 * Path: <b>StructureDefinition.useContext.valueCodeableConcept</b><br> 4489 * </p> 4490 */ 4491 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4492 SP_CONTEXT); 4493 4494 /** 4495 * Search parameter: <b>base-path</b> 4496 * <p> 4497 * Description: <b>Path that identifies the base element</b><br> 4498 * Type: <b>token</b><br> 4499 * Path: <b>StructureDefinition.snapshot.element.base.path, 4500 * StructureDefinition.differential.element.base.path</b><br> 4501 * </p> 4502 */ 4503 @SearchParamDefinition(name = "base-path", path = "StructureDefinition.snapshot.element.base.path | StructureDefinition.differential.element.base.path", description = "Path that identifies the base element", type = "token") 4504 public static final String SP_BASE_PATH = "base-path"; 4505 /** 4506 * <b>Fluent Client</b> search parameter constant for <b>base-path</b> 4507 * <p> 4508 * Description: <b>Path that identifies the base element</b><br> 4509 * Type: <b>token</b><br> 4510 * Path: <b>StructureDefinition.snapshot.element.base.path, 4511 * StructureDefinition.differential.element.base.path</b><br> 4512 * </p> 4513 */ 4514 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BASE_PATH = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4515 SP_BASE_PATH); 4516 4517 /** 4518 * Search parameter: <b>keyword</b> 4519 * <p> 4520 * Description: <b>A code for the StructureDefinition</b><br> 4521 * Type: <b>token</b><br> 4522 * Path: <b>StructureDefinition.keyword</b><br> 4523 * </p> 4524 */ 4525 @SearchParamDefinition(name = "keyword", path = "StructureDefinition.keyword", description = "A code for the StructureDefinition", type = "token") 4526 public static final String SP_KEYWORD = "keyword"; 4527 /** 4528 * <b>Fluent Client</b> search parameter constant for <b>keyword</b> 4529 * <p> 4530 * Description: <b>A code for the StructureDefinition</b><br> 4531 * Type: <b>token</b><br> 4532 * Path: <b>StructureDefinition.keyword</b><br> 4533 * </p> 4534 */ 4535 public static final ca.uhn.fhir.rest.gclient.TokenClientParam KEYWORD = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4536 SP_KEYWORD); 4537 4538 /** 4539 * Search parameter: <b>context-type-quantity</b> 4540 * <p> 4541 * Description: <b>A use context type and quantity- or range-based value 4542 * assigned to the structure definition</b><br> 4543 * Type: <b>composite</b><br> 4544 * Path: <b></b><br> 4545 * </p> 4546 */ 4547 @SearchParamDefinition(name = "context-type-quantity", path = "StructureDefinition.useContext", description = "A use context type and quantity- or range-based value assigned to the structure definition", type = "composite", compositeOf = { 4548 "context-type", "context-quantity" }) 4549 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 4550 /** 4551 * <b>Fluent Client</b> search parameter constant for 4552 * <b>context-type-quantity</b> 4553 * <p> 4554 * Description: <b>A use context type and quantity- or range-based value 4555 * assigned to the structure definition</b><br> 4556 * Type: <b>composite</b><br> 4557 * Path: <b></b><br> 4558 * </p> 4559 */ 4560 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 4561 SP_CONTEXT_TYPE_QUANTITY); 4562 4563 /** 4564 * Search parameter: <b>identifier</b> 4565 * <p> 4566 * Description: <b>External identifier for the structure definition</b><br> 4567 * Type: <b>token</b><br> 4568 * Path: <b>StructureDefinition.identifier</b><br> 4569 * </p> 4570 */ 4571 @SearchParamDefinition(name = "identifier", path = "StructureDefinition.identifier", description = "External identifier for the structure definition", type = "token") 4572 public static final String SP_IDENTIFIER = "identifier"; 4573 /** 4574 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4575 * <p> 4576 * Description: <b>External identifier for the structure definition</b><br> 4577 * Type: <b>token</b><br> 4578 * Path: <b>StructureDefinition.identifier</b><br> 4579 * </p> 4580 */ 4581 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4582 SP_IDENTIFIER); 4583 4584 /** 4585 * Search parameter: <b>valueset</b> 4586 * <p> 4587 * Description: <b>A vocabulary binding reference</b><br> 4588 * Type: <b>reference</b><br> 4589 * Path: <b>StructureDefinition.snapshot.element.binding.valueSet</b><br> 4590 * </p> 4591 */ 4592 @SearchParamDefinition(name = "valueset", path = "StructureDefinition.snapshot.element.binding.valueSet", description = "A vocabulary binding reference", type = "reference", target = { 4593 ValueSet.class }) 4594 public static final String SP_VALUESET = "valueset"; 4595 /** 4596 * <b>Fluent Client</b> search parameter constant for <b>valueset</b> 4597 * <p> 4598 * Description: <b>A vocabulary binding reference</b><br> 4599 * Type: <b>reference</b><br> 4600 * Path: <b>StructureDefinition.snapshot.element.binding.valueSet</b><br> 4601 * </p> 4602 */ 4603 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam VALUESET = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4604 SP_VALUESET); 4605 4606 /** 4607 * Constant for fluent queries to be used to add include statements. Specifies 4608 * the path value of "<b>StructureDefinition:valueset</b>". 4609 */ 4610 public static final ca.uhn.fhir.model.api.Include INCLUDE_VALUESET = new ca.uhn.fhir.model.api.Include( 4611 "StructureDefinition:valueset").toLocked(); 4612 4613 /** 4614 * Search parameter: <b>kind</b> 4615 * <p> 4616 * Description: <b>primitive-type | complex-type | resource | logical</b><br> 4617 * Type: <b>token</b><br> 4618 * Path: <b>StructureDefinition.kind</b><br> 4619 * </p> 4620 */ 4621 @SearchParamDefinition(name = "kind", path = "StructureDefinition.kind", description = "primitive-type | complex-type | resource | logical", type = "token") 4622 public static final String SP_KIND = "kind"; 4623 /** 4624 * <b>Fluent Client</b> search parameter constant for <b>kind</b> 4625 * <p> 4626 * Description: <b>primitive-type | complex-type | resource | logical</b><br> 4627 * Type: <b>token</b><br> 4628 * Path: <b>StructureDefinition.kind</b><br> 4629 * </p> 4630 */ 4631 public static final ca.uhn.fhir.rest.gclient.TokenClientParam KIND = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4632 SP_KIND); 4633 4634 /** 4635 * Search parameter: <b>abstract</b> 4636 * <p> 4637 * Description: <b>Whether the structure is abstract</b><br> 4638 * Type: <b>token</b><br> 4639 * Path: <b>StructureDefinition.abstract</b><br> 4640 * </p> 4641 */ 4642 @SearchParamDefinition(name = "abstract", path = "StructureDefinition.abstract", description = "Whether the structure is abstract", type = "token") 4643 public static final String SP_ABSTRACT = "abstract"; 4644 /** 4645 * <b>Fluent Client</b> search parameter constant for <b>abstract</b> 4646 * <p> 4647 * Description: <b>Whether the structure is abstract</b><br> 4648 * Type: <b>token</b><br> 4649 * Path: <b>StructureDefinition.abstract</b><br> 4650 * </p> 4651 */ 4652 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ABSTRACT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4653 SP_ABSTRACT); 4654 4655 /** 4656 * Search parameter: <b>version</b> 4657 * <p> 4658 * Description: <b>The business version of the structure definition</b><br> 4659 * Type: <b>token</b><br> 4660 * Path: <b>StructureDefinition.version</b><br> 4661 * </p> 4662 */ 4663 @SearchParamDefinition(name = "version", path = "StructureDefinition.version", description = "The business version of the structure definition", type = "token") 4664 public static final String SP_VERSION = "version"; 4665 /** 4666 * <b>Fluent Client</b> search parameter constant for <b>version</b> 4667 * <p> 4668 * Description: <b>The business version of the structure definition</b><br> 4669 * Type: <b>token</b><br> 4670 * Path: <b>StructureDefinition.version</b><br> 4671 * </p> 4672 */ 4673 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4674 SP_VERSION); 4675 4676 /** 4677 * Search parameter: <b>url</b> 4678 * <p> 4679 * Description: <b>The uri that identifies the structure definition</b><br> 4680 * Type: <b>uri</b><br> 4681 * Path: <b>StructureDefinition.url</b><br> 4682 * </p> 4683 */ 4684 @SearchParamDefinition(name = "url", path = "StructureDefinition.url", description = "The uri that identifies the structure definition", type = "uri") 4685 public static final String SP_URL = "url"; 4686 /** 4687 * <b>Fluent Client</b> search parameter constant for <b>url</b> 4688 * <p> 4689 * Description: <b>The uri that identifies the structure definition</b><br> 4690 * Type: <b>uri</b><br> 4691 * Path: <b>StructureDefinition.url</b><br> 4692 * </p> 4693 */ 4694 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 4695 4696 /** 4697 * Search parameter: <b>ext-context</b> 4698 * <p> 4699 * Description: <b>The system is the URL for the context-type: e.g. 4700 * http://hl7.org/fhir/extension-context-type#element|CodeableConcept.text</b><br> 4701 * Type: <b>token</b><br> 4702 * Path: <b>StructureDefinition.context.type</b><br> 4703 * </p> 4704 */ 4705 @SearchParamDefinition(name = "ext-context", path = "StructureDefinition.context.type", description = "The system is the URL for the context-type: e.g. http://hl7.org/fhir/extension-context-type#element|CodeableConcept.text", type = "token") 4706 public static final String SP_EXT_CONTEXT = "ext-context"; 4707 /** 4708 * <b>Fluent Client</b> search parameter constant for <b>ext-context</b> 4709 * <p> 4710 * Description: <b>The system is the URL for the context-type: e.g. 4711 * http://hl7.org/fhir/extension-context-type#element|CodeableConcept.text</b><br> 4712 * Type: <b>token</b><br> 4713 * Path: <b>StructureDefinition.context.type</b><br> 4714 * </p> 4715 */ 4716 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EXT_CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4717 SP_EXT_CONTEXT); 4718 4719 /** 4720 * Search parameter: <b>name</b> 4721 * <p> 4722 * Description: <b>Computationally friendly name of the structure 4723 * definition</b><br> 4724 * Type: <b>string</b><br> 4725 * Path: <b>StructureDefinition.name</b><br> 4726 * </p> 4727 */ 4728 @SearchParamDefinition(name = "name", path = "StructureDefinition.name", description = "Computationally friendly name of the structure definition", type = "string") 4729 public static final String SP_NAME = "name"; 4730 /** 4731 * <b>Fluent Client</b> search parameter constant for <b>name</b> 4732 * <p> 4733 * Description: <b>Computationally friendly name of the structure 4734 * definition</b><br> 4735 * Type: <b>string</b><br> 4736 * Path: <b>StructureDefinition.name</b><br> 4737 * </p> 4738 */ 4739 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 4740 SP_NAME); 4741 4742 /** 4743 * Search parameter: <b>publisher</b> 4744 * <p> 4745 * Description: <b>Name of the publisher of the structure definition</b><br> 4746 * Type: <b>string</b><br> 4747 * Path: <b>StructureDefinition.publisher</b><br> 4748 * </p> 4749 */ 4750 @SearchParamDefinition(name = "publisher", path = "StructureDefinition.publisher", description = "Name of the publisher of the structure definition", type = "string") 4751 public static final String SP_PUBLISHER = "publisher"; 4752 /** 4753 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 4754 * <p> 4755 * Description: <b>Name of the publisher of the structure definition</b><br> 4756 * Type: <b>string</b><br> 4757 * Path: <b>StructureDefinition.publisher</b><br> 4758 * </p> 4759 */ 4760 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 4761 SP_PUBLISHER); 4762 4763 /** 4764 * Search parameter: <b>derivation</b> 4765 * <p> 4766 * Description: <b>specialization | constraint - How relates to base 4767 * definition</b><br> 4768 * Type: <b>token</b><br> 4769 * Path: <b>StructureDefinition.derivation</b><br> 4770 * </p> 4771 */ 4772 @SearchParamDefinition(name = "derivation", path = "StructureDefinition.derivation", description = "specialization | constraint - How relates to base definition", type = "token") 4773 public static final String SP_DERIVATION = "derivation"; 4774 /** 4775 * <b>Fluent Client</b> search parameter constant for <b>derivation</b> 4776 * <p> 4777 * Description: <b>specialization | constraint - How relates to base 4778 * definition</b><br> 4779 * Type: <b>token</b><br> 4780 * Path: <b>StructureDefinition.derivation</b><br> 4781 * </p> 4782 */ 4783 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DERIVATION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4784 SP_DERIVATION); 4785 4786 /** 4787 * Search parameter: <b>status</b> 4788 * <p> 4789 * Description: <b>The current status of the structure definition</b><br> 4790 * Type: <b>token</b><br> 4791 * Path: <b>StructureDefinition.status</b><br> 4792 * </p> 4793 */ 4794 @SearchParamDefinition(name = "status", path = "StructureDefinition.status", description = "The current status of the structure definition", type = "token") 4795 public static final String SP_STATUS = "status"; 4796 /** 4797 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4798 * <p> 4799 * Description: <b>The current status of the structure definition</b><br> 4800 * Type: <b>token</b><br> 4801 * Path: <b>StructureDefinition.status</b><br> 4802 * </p> 4803 */ 4804 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4805 SP_STATUS); 4806 4807 /** 4808 * Search parameter: <b>base</b> 4809 * <p> 4810 * Description: <b>Definition that this type is constrained/specialized 4811 * from</b><br> 4812 * Type: <b>reference</b><br> 4813 * Path: <b>StructureDefinition.baseDefinition</b><br> 4814 * </p> 4815 */ 4816 @SearchParamDefinition(name = "base", path = "StructureDefinition.baseDefinition", description = "Definition that this type is constrained/specialized from", type = "reference", target = { 4817 StructureDefinition.class }) 4818 public static final String SP_BASE = "base"; 4819 /** 4820 * <b>Fluent Client</b> search parameter constant for <b>base</b> 4821 * <p> 4822 * Description: <b>Definition that this type is constrained/specialized 4823 * from</b><br> 4824 * Type: <b>reference</b><br> 4825 * Path: <b>StructureDefinition.baseDefinition</b><br> 4826 * </p> 4827 */ 4828 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4829 SP_BASE); 4830 4831 /** 4832 * Constant for fluent queries to be used to add include statements. Specifies 4833 * the path value of "<b>StructureDefinition:base</b>". 4834 */ 4835 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASE = new ca.uhn.fhir.model.api.Include( 4836 "StructureDefinition:base").toLocked(); 4837 4838 public String getVersionedUrl() { 4839 return hasVersion() ? getUrl()+"|"+getVersion() : getUrl(); 4840 } 4841 4842}