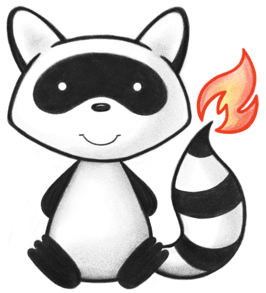
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * The subscription resource is used to define a push-based subscription from a 049 * server to another system. Once a subscription is registered with the server, 050 * the server checks every resource that is created or updated, and if the 051 * resource matches the given criteria, it sends a message on the defined 052 * "channel" so that another system can take an appropriate action. 053 */ 054@ResourceDef(name = "Subscription", profile = "http://hl7.org/fhir/StructureDefinition/Subscription") 055public class Subscription extends DomainResource { 056 057 public enum SubscriptionStatus { 058 /** 059 * The client has requested the subscription, and the server has not yet set it 060 * up. 061 */ 062 REQUESTED, 063 /** 064 * The subscription is active. 065 */ 066 ACTIVE, 067 /** 068 * The server has an error executing the notification. 069 */ 070 ERROR, 071 /** 072 * Too many errors have occurred or the subscription has expired. 073 */ 074 OFF, 075 /** 076 * added to help the parsers with the generic types 077 */ 078 NULL; 079 080 public static SubscriptionStatus fromCode(String codeString) throws FHIRException { 081 if (codeString == null || "".equals(codeString)) 082 return null; 083 if ("requested".equals(codeString)) 084 return REQUESTED; 085 if ("active".equals(codeString)) 086 return ACTIVE; 087 if ("error".equals(codeString)) 088 return ERROR; 089 if ("off".equals(codeString)) 090 return OFF; 091 if (Configuration.isAcceptInvalidEnums()) 092 return null; 093 else 094 throw new FHIRException("Unknown SubscriptionStatus code '" + codeString + "'"); 095 } 096 097 public String toCode() { 098 switch (this) { 099 case REQUESTED: 100 return "requested"; 101 case ACTIVE: 102 return "active"; 103 case ERROR: 104 return "error"; 105 case OFF: 106 return "off"; 107 case NULL: 108 return null; 109 default: 110 return "?"; 111 } 112 } 113 114 public String getSystem() { 115 switch (this) { 116 case REQUESTED: 117 return "http://hl7.org/fhir/subscription-status"; 118 case ACTIVE: 119 return "http://hl7.org/fhir/subscription-status"; 120 case ERROR: 121 return "http://hl7.org/fhir/subscription-status"; 122 case OFF: 123 return "http://hl7.org/fhir/subscription-status"; 124 case NULL: 125 return null; 126 default: 127 return "?"; 128 } 129 } 130 131 public String getDefinition() { 132 switch (this) { 133 case REQUESTED: 134 return "The client has requested the subscription, and the server has not yet set it up."; 135 case ACTIVE: 136 return "The subscription is active."; 137 case ERROR: 138 return "The server has an error executing the notification."; 139 case OFF: 140 return "Too many errors have occurred or the subscription has expired."; 141 case NULL: 142 return null; 143 default: 144 return "?"; 145 } 146 } 147 148 public String getDisplay() { 149 switch (this) { 150 case REQUESTED: 151 return "Requested"; 152 case ACTIVE: 153 return "Active"; 154 case ERROR: 155 return "Error"; 156 case OFF: 157 return "Off"; 158 case NULL: 159 return null; 160 default: 161 return "?"; 162 } 163 } 164 } 165 166 public static class SubscriptionStatusEnumFactory implements EnumFactory<SubscriptionStatus> { 167 public SubscriptionStatus fromCode(String codeString) throws IllegalArgumentException { 168 if (codeString == null || "".equals(codeString)) 169 if (codeString == null || "".equals(codeString)) 170 return null; 171 if ("requested".equals(codeString)) 172 return SubscriptionStatus.REQUESTED; 173 if ("active".equals(codeString)) 174 return SubscriptionStatus.ACTIVE; 175 if ("error".equals(codeString)) 176 return SubscriptionStatus.ERROR; 177 if ("off".equals(codeString)) 178 return SubscriptionStatus.OFF; 179 throw new IllegalArgumentException("Unknown SubscriptionStatus code '" + codeString + "'"); 180 } 181 182 public Enumeration<SubscriptionStatus> fromType(PrimitiveType<?> code) throws FHIRException { 183 if (code == null) 184 return null; 185 if (code.isEmpty()) 186 return new Enumeration<SubscriptionStatus>(this, SubscriptionStatus.NULL, code); 187 String codeString = code.asStringValue(); 188 if (codeString == null || "".equals(codeString)) 189 return new Enumeration<SubscriptionStatus>(this, SubscriptionStatus.NULL, code); 190 if ("requested".equals(codeString)) 191 return new Enumeration<SubscriptionStatus>(this, SubscriptionStatus.REQUESTED, code); 192 if ("active".equals(codeString)) 193 return new Enumeration<SubscriptionStatus>(this, SubscriptionStatus.ACTIVE, code); 194 if ("error".equals(codeString)) 195 return new Enumeration<SubscriptionStatus>(this, SubscriptionStatus.ERROR, code); 196 if ("off".equals(codeString)) 197 return new Enumeration<SubscriptionStatus>(this, SubscriptionStatus.OFF, code); 198 throw new FHIRException("Unknown SubscriptionStatus code '" + codeString + "'"); 199 } 200 201 public String toCode(SubscriptionStatus code) { 202 if (code == SubscriptionStatus.NULL) 203 return null; 204 if (code == SubscriptionStatus.REQUESTED) 205 return "requested"; 206 if (code == SubscriptionStatus.ACTIVE) 207 return "active"; 208 if (code == SubscriptionStatus.ERROR) 209 return "error"; 210 if (code == SubscriptionStatus.OFF) 211 return "off"; 212 return "?"; 213 } 214 215 public String toSystem(SubscriptionStatus code) { 216 return code.getSystem(); 217 } 218 } 219 220 public enum SubscriptionChannelType { 221 /** 222 * The channel is executed by making a post to the URI. If a payload is 223 * included, the URL is interpreted as the service base, and an update (PUT) is 224 * made. 225 */ 226 RESTHOOK, 227 /** 228 * The channel is executed by sending a packet across a web socket connection 229 * maintained by the client. The URL identifies the websocket, and the client 230 * binds to this URL. 231 */ 232 WEBSOCKET, 233 /** 234 * The channel is executed by sending an email to the email addressed in the URI 235 * (which must be a mailto:). 236 */ 237 EMAIL, 238 /** 239 * The channel is executed by sending an SMS message to the phone number 240 * identified in the URL (tel:). 241 */ 242 SMS, 243 /** 244 * The channel is executed by sending a message (e.g. a Bundle with a 245 * MessageHeader resource etc.) to the application identified in the URI. 246 */ 247 MESSAGE, 248 /** 249 * added to help the parsers with the generic types 250 */ 251 NULL; 252 253 public static SubscriptionChannelType fromCode(String codeString) throws FHIRException { 254 if (codeString == null || "".equals(codeString)) 255 return null; 256 if ("rest-hook".equals(codeString)) 257 return RESTHOOK; 258 if ("websocket".equals(codeString)) 259 return WEBSOCKET; 260 if ("email".equals(codeString)) 261 return EMAIL; 262 if ("sms".equals(codeString)) 263 return SMS; 264 if ("message".equals(codeString)) 265 return MESSAGE; 266 if (Configuration.isAcceptInvalidEnums()) 267 return null; 268 else 269 throw new FHIRException("Unknown SubscriptionChannelType code '" + codeString + "'"); 270 } 271 272 public String toCode() { 273 switch (this) { 274 case RESTHOOK: 275 return "rest-hook"; 276 case WEBSOCKET: 277 return "websocket"; 278 case EMAIL: 279 return "email"; 280 case SMS: 281 return "sms"; 282 case MESSAGE: 283 return "message"; 284 case NULL: 285 return null; 286 default: 287 return "?"; 288 } 289 } 290 291 public String getSystem() { 292 switch (this) { 293 case RESTHOOK: 294 return "http://hl7.org/fhir/subscription-channel-type"; 295 case WEBSOCKET: 296 return "http://hl7.org/fhir/subscription-channel-type"; 297 case EMAIL: 298 return "http://hl7.org/fhir/subscription-channel-type"; 299 case SMS: 300 return "http://hl7.org/fhir/subscription-channel-type"; 301 case MESSAGE: 302 return "http://hl7.org/fhir/subscription-channel-type"; 303 case NULL: 304 return null; 305 default: 306 return "?"; 307 } 308 } 309 310 public String getDefinition() { 311 switch (this) { 312 case RESTHOOK: 313 return "The channel is executed by making a post to the URI. If a payload is included, the URL is interpreted as the service base, and an update (PUT) is made."; 314 case WEBSOCKET: 315 return "The channel is executed by sending a packet across a web socket connection maintained by the client. The URL identifies the websocket, and the client binds to this URL."; 316 case EMAIL: 317 return "The channel is executed by sending an email to the email addressed in the URI (which must be a mailto:)."; 318 case SMS: 319 return "The channel is executed by sending an SMS message to the phone number identified in the URL (tel:)."; 320 case MESSAGE: 321 return "The channel is executed by sending a message (e.g. a Bundle with a MessageHeader resource etc.) to the application identified in the URI."; 322 case NULL: 323 return null; 324 default: 325 return "?"; 326 } 327 } 328 329 public String getDisplay() { 330 switch (this) { 331 case RESTHOOK: 332 return "Rest Hook"; 333 case WEBSOCKET: 334 return "Websocket"; 335 case EMAIL: 336 return "Email"; 337 case SMS: 338 return "SMS"; 339 case MESSAGE: 340 return "Message"; 341 case NULL: 342 return null; 343 default: 344 return "?"; 345 } 346 } 347 } 348 349 public static class SubscriptionChannelTypeEnumFactory implements EnumFactory<SubscriptionChannelType> { 350 public SubscriptionChannelType fromCode(String codeString) throws IllegalArgumentException { 351 if (codeString == null || "".equals(codeString)) 352 if (codeString == null || "".equals(codeString)) 353 return null; 354 if ("rest-hook".equals(codeString)) 355 return SubscriptionChannelType.RESTHOOK; 356 if ("websocket".equals(codeString)) 357 return SubscriptionChannelType.WEBSOCKET; 358 if ("email".equals(codeString)) 359 return SubscriptionChannelType.EMAIL; 360 if ("sms".equals(codeString)) 361 return SubscriptionChannelType.SMS; 362 if ("message".equals(codeString)) 363 return SubscriptionChannelType.MESSAGE; 364 throw new IllegalArgumentException("Unknown SubscriptionChannelType code '" + codeString + "'"); 365 } 366 367 public Enumeration<SubscriptionChannelType> fromType(PrimitiveType<?> code) throws FHIRException { 368 if (code == null) 369 return null; 370 if (code.isEmpty()) 371 return new Enumeration<SubscriptionChannelType>(this, SubscriptionChannelType.NULL, code); 372 String codeString = code.asStringValue(); 373 if (codeString == null || "".equals(codeString)) 374 return new Enumeration<SubscriptionChannelType>(this, SubscriptionChannelType.NULL, code); 375 if ("rest-hook".equals(codeString)) 376 return new Enumeration<SubscriptionChannelType>(this, SubscriptionChannelType.RESTHOOK, code); 377 if ("websocket".equals(codeString)) 378 return new Enumeration<SubscriptionChannelType>(this, SubscriptionChannelType.WEBSOCKET, code); 379 if ("email".equals(codeString)) 380 return new Enumeration<SubscriptionChannelType>(this, SubscriptionChannelType.EMAIL, code); 381 if ("sms".equals(codeString)) 382 return new Enumeration<SubscriptionChannelType>(this, SubscriptionChannelType.SMS, code); 383 if ("message".equals(codeString)) 384 return new Enumeration<SubscriptionChannelType>(this, SubscriptionChannelType.MESSAGE, code); 385 throw new FHIRException("Unknown SubscriptionChannelType code '" + codeString + "'"); 386 } 387 388 public String toCode(SubscriptionChannelType code) { 389 if (code == SubscriptionChannelType.NULL) 390 return null; 391 if (code == SubscriptionChannelType.RESTHOOK) 392 return "rest-hook"; 393 if (code == SubscriptionChannelType.WEBSOCKET) 394 return "websocket"; 395 if (code == SubscriptionChannelType.EMAIL) 396 return "email"; 397 if (code == SubscriptionChannelType.SMS) 398 return "sms"; 399 if (code == SubscriptionChannelType.MESSAGE) 400 return "message"; 401 return "?"; 402 } 403 404 public String toSystem(SubscriptionChannelType code) { 405 return code.getSystem(); 406 } 407 } 408 409 @Block() 410 public static class SubscriptionChannelComponent extends BackboneElement implements IBaseBackboneElement { 411 /** 412 * The type of channel to send notifications on. 413 */ 414 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 415 @Description(shortDefinition = "rest-hook | websocket | email | sms | message", formalDefinition = "The type of channel to send notifications on.") 416 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/subscription-channel-type") 417 protected Enumeration<SubscriptionChannelType> type; 418 419 /** 420 * The url that describes the actual end-point to send messages to. 421 */ 422 @Child(name = "endpoint", type = { UrlType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 423 @Description(shortDefinition = "Where the channel points to", formalDefinition = "The url that describes the actual end-point to send messages to.") 424 protected UrlType endpoint; 425 426 /** 427 * The mime type to send the payload in - either application/fhir+xml, or 428 * application/fhir+json. If the payload is not present, then there is no 429 * payload in the notification, just a notification. The mime type "text/plain" 430 * may also be used for Email and SMS subscriptions. 431 */ 432 @Child(name = "payload", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 433 @Description(shortDefinition = "MIME type to send, or omit for no payload", formalDefinition = "The mime type to send the payload in - either application/fhir+xml, or application/fhir+json. If the payload is not present, then there is no payload in the notification, just a notification. The mime type \"text/plain\" may also be used for Email and SMS subscriptions.") 434 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/mimetypes") 435 protected CodeType payload; 436 437 /** 438 * Additional headers / information to send as part of the notification. 439 */ 440 @Child(name = "header", type = { 441 StringType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 442 @Description(shortDefinition = "Usage depends on the channel type", formalDefinition = "Additional headers / information to send as part of the notification.") 443 protected List<StringType> header; 444 445 private static final long serialVersionUID = -771044852L; 446 447 /** 448 * Constructor 449 */ 450 public SubscriptionChannelComponent() { 451 super(); 452 } 453 454 /** 455 * Constructor 456 */ 457 public SubscriptionChannelComponent(Enumeration<SubscriptionChannelType> type) { 458 super(); 459 this.type = type; 460 } 461 462 /** 463 * @return {@link #type} (The type of channel to send notifications on.). This 464 * is the underlying object with id, value and extensions. The accessor 465 * "getType" gives direct access to the value 466 */ 467 public Enumeration<SubscriptionChannelType> getTypeElement() { 468 if (this.type == null) 469 if (Configuration.errorOnAutoCreate()) 470 throw new Error("Attempt to auto-create SubscriptionChannelComponent.type"); 471 else if (Configuration.doAutoCreate()) 472 this.type = new Enumeration<SubscriptionChannelType>(new SubscriptionChannelTypeEnumFactory()); // bb 473 return this.type; 474 } 475 476 public boolean hasTypeElement() { 477 return this.type != null && !this.type.isEmpty(); 478 } 479 480 public boolean hasType() { 481 return this.type != null && !this.type.isEmpty(); 482 } 483 484 /** 485 * @param value {@link #type} (The type of channel to send notifications on.). 486 * This is the underlying object with id, value and extensions. The 487 * accessor "getType" gives direct access to the value 488 */ 489 public SubscriptionChannelComponent setTypeElement(Enumeration<SubscriptionChannelType> value) { 490 this.type = value; 491 return this; 492 } 493 494 /** 495 * @return The type of channel to send notifications on. 496 */ 497 public SubscriptionChannelType getType() { 498 return this.type == null ? null : this.type.getValue(); 499 } 500 501 /** 502 * @param value The type of channel to send notifications on. 503 */ 504 public SubscriptionChannelComponent setType(SubscriptionChannelType value) { 505 if (this.type == null) 506 this.type = new Enumeration<SubscriptionChannelType>(new SubscriptionChannelTypeEnumFactory()); 507 this.type.setValue(value); 508 return this; 509 } 510 511 /** 512 * @return {@link #endpoint} (The url that describes the actual end-point to 513 * send messages to.). This is the underlying object with id, value and 514 * extensions. The accessor "getEndpoint" gives direct access to the 515 * value 516 */ 517 public UrlType getEndpointElement() { 518 if (this.endpoint == null) 519 if (Configuration.errorOnAutoCreate()) 520 throw new Error("Attempt to auto-create SubscriptionChannelComponent.endpoint"); 521 else if (Configuration.doAutoCreate()) 522 this.endpoint = new UrlType(); // bb 523 return this.endpoint; 524 } 525 526 public boolean hasEndpointElement() { 527 return this.endpoint != null && !this.endpoint.isEmpty(); 528 } 529 530 public boolean hasEndpoint() { 531 return this.endpoint != null && !this.endpoint.isEmpty(); 532 } 533 534 /** 535 * @param value {@link #endpoint} (The url that describes the actual end-point 536 * to send messages to.). This is the underlying object with id, 537 * value and extensions. The accessor "getEndpoint" gives direct 538 * access to the value 539 */ 540 public SubscriptionChannelComponent setEndpointElement(UrlType value) { 541 this.endpoint = value; 542 return this; 543 } 544 545 /** 546 * @return The url that describes the actual end-point to send messages to. 547 */ 548 public String getEndpoint() { 549 return this.endpoint == null ? null : this.endpoint.getValue(); 550 } 551 552 /** 553 * @param value The url that describes the actual end-point to send messages to. 554 */ 555 public SubscriptionChannelComponent setEndpoint(String value) { 556 if (Utilities.noString(value)) 557 this.endpoint = null; 558 else { 559 if (this.endpoint == null) 560 this.endpoint = new UrlType(); 561 this.endpoint.setValue(value); 562 } 563 return this; 564 } 565 566 /** 567 * @return {@link #payload} (The mime type to send the payload in - either 568 * application/fhir+xml, or application/fhir+json. If the payload is not 569 * present, then there is no payload in the notification, just a 570 * notification. The mime type "text/plain" may also be used for Email 571 * and SMS subscriptions.). This is the underlying object with id, value 572 * and extensions. The accessor "getPayload" gives direct access to the 573 * value 574 */ 575 public CodeType getPayloadElement() { 576 if (this.payload == null) 577 if (Configuration.errorOnAutoCreate()) 578 throw new Error("Attempt to auto-create SubscriptionChannelComponent.payload"); 579 else if (Configuration.doAutoCreate()) 580 this.payload = new CodeType(); // bb 581 return this.payload; 582 } 583 584 public boolean hasPayloadElement() { 585 return this.payload != null && !this.payload.isEmpty(); 586 } 587 588 public boolean hasPayload() { 589 return this.payload != null && !this.payload.isEmpty(); 590 } 591 592 /** 593 * @param value {@link #payload} (The mime type to send the payload in - either 594 * application/fhir+xml, or application/fhir+json. If the payload 595 * is not present, then there is no payload in the notification, 596 * just a notification. The mime type "text/plain" may also be used 597 * for Email and SMS subscriptions.). This is the underlying object 598 * with id, value and extensions. The accessor "getPayload" gives 599 * direct access to the value 600 */ 601 public SubscriptionChannelComponent setPayloadElement(CodeType value) { 602 this.payload = value; 603 return this; 604 } 605 606 /** 607 * @return The mime type to send the payload in - either application/fhir+xml, 608 * or application/fhir+json. If the payload is not present, then there 609 * is no payload in the notification, just a notification. The mime type 610 * "text/plain" may also be used for Email and SMS subscriptions. 611 */ 612 public String getPayload() { 613 return this.payload == null ? null : this.payload.getValue(); 614 } 615 616 /** 617 * @param value The mime type to send the payload in - either 618 * application/fhir+xml, or application/fhir+json. If the payload 619 * is not present, then there is no payload in the notification, 620 * just a notification. The mime type "text/plain" may also be used 621 * for Email and SMS subscriptions. 622 */ 623 public SubscriptionChannelComponent setPayload(String value) { 624 if (Utilities.noString(value)) 625 this.payload = null; 626 else { 627 if (this.payload == null) 628 this.payload = new CodeType(); 629 this.payload.setValue(value); 630 } 631 return this; 632 } 633 634 /** 635 * @return {@link #header} (Additional headers / information to send as part of 636 * the notification.) 637 */ 638 public List<StringType> getHeader() { 639 if (this.header == null) 640 this.header = new ArrayList<StringType>(); 641 return this.header; 642 } 643 644 /** 645 * @return Returns a reference to <code>this</code> for easy method chaining 646 */ 647 public SubscriptionChannelComponent setHeader(List<StringType> theHeader) { 648 this.header = theHeader; 649 return this; 650 } 651 652 public boolean hasHeader() { 653 if (this.header == null) 654 return false; 655 for (StringType item : this.header) 656 if (!item.isEmpty()) 657 return true; 658 return false; 659 } 660 661 /** 662 * @return {@link #header} (Additional headers / information to send as part of 663 * the notification.) 664 */ 665 public StringType addHeaderElement() {// 2 666 StringType t = new StringType(); 667 if (this.header == null) 668 this.header = new ArrayList<StringType>(); 669 this.header.add(t); 670 return t; 671 } 672 673 /** 674 * @param value {@link #header} (Additional headers / information to send as 675 * part of the notification.) 676 */ 677 public SubscriptionChannelComponent addHeader(String value) { // 1 678 StringType t = new StringType(); 679 t.setValue(value); 680 if (this.header == null) 681 this.header = new ArrayList<StringType>(); 682 this.header.add(t); 683 return this; 684 } 685 686 /** 687 * @param value {@link #header} (Additional headers / information to send as 688 * part of the notification.) 689 */ 690 public boolean hasHeader(String value) { 691 if (this.header == null) 692 return false; 693 for (StringType v : this.header) 694 if (v.getValue().equals(value)) // string 695 return true; 696 return false; 697 } 698 699 protected void listChildren(List<Property> children) { 700 super.listChildren(children); 701 children.add(new Property("type", "code", "The type of channel to send notifications on.", 0, 1, type)); 702 children.add(new Property("endpoint", "url", "The url that describes the actual end-point to send messages to.", 703 0, 1, endpoint)); 704 children.add(new Property("payload", "code", 705 "The mime type to send the payload in - either application/fhir+xml, or application/fhir+json. If the payload is not present, then there is no payload in the notification, just a notification. The mime type \"text/plain\" may also be used for Email and SMS subscriptions.", 706 0, 1, payload)); 707 children 708 .add(new Property("header", "string", "Additional headers / information to send as part of the notification.", 709 0, java.lang.Integer.MAX_VALUE, header)); 710 } 711 712 @Override 713 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 714 switch (_hash) { 715 case 3575610: 716 /* type */ return new Property("type", "code", "The type of channel to send notifications on.", 0, 1, type); 717 case 1741102485: 718 /* endpoint */ return new Property("endpoint", "url", 719 "The url that describes the actual end-point to send messages to.", 0, 1, endpoint); 720 case -786701938: 721 /* payload */ return new Property("payload", "code", 722 "The mime type to send the payload in - either application/fhir+xml, or application/fhir+json. If the payload is not present, then there is no payload in the notification, just a notification. The mime type \"text/plain\" may also be used for Email and SMS subscriptions.", 723 0, 1, payload); 724 case -1221270899: 725 /* header */ return new Property("header", "string", 726 "Additional headers / information to send as part of the notification.", 0, java.lang.Integer.MAX_VALUE, 727 header); 728 default: 729 return super.getNamedProperty(_hash, _name, _checkValid); 730 } 731 732 } 733 734 @Override 735 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 736 switch (hash) { 737 case 3575610: 738 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<SubscriptionChannelType> 739 case 1741102485: 740 /* endpoint */ return this.endpoint == null ? new Base[0] : new Base[] { this.endpoint }; // UrlType 741 case -786701938: 742 /* payload */ return this.payload == null ? new Base[0] : new Base[] { this.payload }; // CodeType 743 case -1221270899: 744 /* header */ return this.header == null ? new Base[0] : this.header.toArray(new Base[this.header.size()]); // StringType 745 default: 746 return super.getProperty(hash, name, checkValid); 747 } 748 749 } 750 751 @Override 752 public Base setProperty(int hash, String name, Base value) throws FHIRException { 753 switch (hash) { 754 case 3575610: // type 755 value = new SubscriptionChannelTypeEnumFactory().fromType(castToCode(value)); 756 this.type = (Enumeration) value; // Enumeration<SubscriptionChannelType> 757 return value; 758 case 1741102485: // endpoint 759 this.endpoint = castToUrl(value); // UrlType 760 return value; 761 case -786701938: // payload 762 this.payload = castToCode(value); // CodeType 763 return value; 764 case -1221270899: // header 765 this.getHeader().add(castToString(value)); // StringType 766 return value; 767 default: 768 return super.setProperty(hash, name, value); 769 } 770 771 } 772 773 @Override 774 public Base setProperty(String name, Base value) throws FHIRException { 775 if (name.equals("type")) { 776 value = new SubscriptionChannelTypeEnumFactory().fromType(castToCode(value)); 777 this.type = (Enumeration) value; // Enumeration<SubscriptionChannelType> 778 } else if (name.equals("endpoint")) { 779 this.endpoint = castToUrl(value); // UrlType 780 } else if (name.equals("payload")) { 781 this.payload = castToCode(value); // CodeType 782 } else if (name.equals("header")) { 783 this.getHeader().add(castToString(value)); 784 } else 785 return super.setProperty(name, value); 786 return value; 787 } 788 789 @Override 790 public void removeChild(String name, Base value) throws FHIRException { 791 if (name.equals("type")) { 792 this.type = null; 793 } else if (name.equals("endpoint")) { 794 this.endpoint = null; 795 } else if (name.equals("payload")) { 796 this.payload = null; 797 } else if (name.equals("header")) { 798 this.getHeader().remove(castToString(value)); 799 } else 800 super.removeChild(name, value); 801 802 } 803 804 @Override 805 public Base makeProperty(int hash, String name) throws FHIRException { 806 switch (hash) { 807 case 3575610: 808 return getTypeElement(); 809 case 1741102485: 810 return getEndpointElement(); 811 case -786701938: 812 return getPayloadElement(); 813 case -1221270899: 814 return addHeaderElement(); 815 default: 816 return super.makeProperty(hash, name); 817 } 818 819 } 820 821 @Override 822 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 823 switch (hash) { 824 case 3575610: 825 /* type */ return new String[] { "code" }; 826 case 1741102485: 827 /* endpoint */ return new String[] { "url" }; 828 case -786701938: 829 /* payload */ return new String[] { "code" }; 830 case -1221270899: 831 /* header */ return new String[] { "string" }; 832 default: 833 return super.getTypesForProperty(hash, name); 834 } 835 836 } 837 838 @Override 839 public Base addChild(String name) throws FHIRException { 840 if (name.equals("type")) { 841 throw new FHIRException("Cannot call addChild on a singleton property Subscription.type"); 842 } else if (name.equals("endpoint")) { 843 throw new FHIRException("Cannot call addChild on a singleton property Subscription.endpoint"); 844 } else if (name.equals("payload")) { 845 throw new FHIRException("Cannot call addChild on a singleton property Subscription.payload"); 846 } else if (name.equals("header")) { 847 throw new FHIRException("Cannot call addChild on a singleton property Subscription.header"); 848 } else 849 return super.addChild(name); 850 } 851 852 public SubscriptionChannelComponent copy() { 853 SubscriptionChannelComponent dst = new SubscriptionChannelComponent(); 854 copyValues(dst); 855 return dst; 856 } 857 858 public void copyValues(SubscriptionChannelComponent dst) { 859 super.copyValues(dst); 860 dst.type = type == null ? null : type.copy(); 861 dst.endpoint = endpoint == null ? null : endpoint.copy(); 862 dst.payload = payload == null ? null : payload.copy(); 863 if (header != null) { 864 dst.header = new ArrayList<StringType>(); 865 for (StringType i : header) 866 dst.header.add(i.copy()); 867 } 868 ; 869 } 870 871 @Override 872 public boolean equalsDeep(Base other_) { 873 if (!super.equalsDeep(other_)) 874 return false; 875 if (!(other_ instanceof SubscriptionChannelComponent)) 876 return false; 877 SubscriptionChannelComponent o = (SubscriptionChannelComponent) other_; 878 return compareDeep(type, o.type, true) && compareDeep(endpoint, o.endpoint, true) 879 && compareDeep(payload, o.payload, true) && compareDeep(header, o.header, true); 880 } 881 882 @Override 883 public boolean equalsShallow(Base other_) { 884 if (!super.equalsShallow(other_)) 885 return false; 886 if (!(other_ instanceof SubscriptionChannelComponent)) 887 return false; 888 SubscriptionChannelComponent o = (SubscriptionChannelComponent) other_; 889 return compareValues(type, o.type, true) && compareValues(endpoint, o.endpoint, true) 890 && compareValues(payload, o.payload, true) && compareValues(header, o.header, true); 891 } 892 893 public boolean isEmpty() { 894 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, endpoint, payload, header); 895 } 896 897 public String fhirType() { 898 return "Subscription.channel"; 899 900 } 901 902 } 903 904 /** 905 * The status of the subscription, which marks the server state for managing the 906 * subscription. 907 */ 908 @Child(name = "status", type = { CodeType.class }, order = 0, min = 1, max = 1, modifier = true, summary = true) 909 @Description(shortDefinition = "requested | active | error | off", formalDefinition = "The status of the subscription, which marks the server state for managing the subscription.") 910 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/subscription-status") 911 protected Enumeration<SubscriptionStatus> status; 912 913 /** 914 * Contact details for a human to contact about the subscription. The primary 915 * use of this for system administrator troubleshooting. 916 */ 917 @Child(name = "contact", type = { 918 ContactPoint.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 919 @Description(shortDefinition = "Contact details for source (e.g. troubleshooting)", formalDefinition = "Contact details for a human to contact about the subscription. The primary use of this for system administrator troubleshooting.") 920 protected List<ContactPoint> contact; 921 922 /** 923 * The time for the server to turn the subscription off. 924 */ 925 @Child(name = "end", type = { InstantType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 926 @Description(shortDefinition = "When to automatically delete the subscription", formalDefinition = "The time for the server to turn the subscription off.") 927 protected InstantType end; 928 929 /** 930 * A description of why this subscription is defined. 931 */ 932 @Child(name = "reason", type = { StringType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 933 @Description(shortDefinition = "Description of why this subscription was created", formalDefinition = "A description of why this subscription is defined.") 934 protected StringType reason; 935 936 /** 937 * The rules that the server should use to determine when to generate 938 * notifications for this subscription. 939 */ 940 @Child(name = "criteria", type = { StringType.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 941 @Description(shortDefinition = "Rule for server push", formalDefinition = "The rules that the server should use to determine when to generate notifications for this subscription.") 942 protected StringType criteria; 943 944 /** 945 * A record of the last error that occurred when the server processed a 946 * notification. 947 */ 948 @Child(name = "error", type = { StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 949 @Description(shortDefinition = "Latest error note", formalDefinition = "A record of the last error that occurred when the server processed a notification.") 950 protected StringType error; 951 952 /** 953 * Details where to send notifications when resources are received that meet the 954 * criteria. 955 */ 956 @Child(name = "channel", type = {}, order = 6, min = 1, max = 1, modifier = false, summary = true) 957 @Description(shortDefinition = "The channel on which to report matches to the criteria", formalDefinition = "Details where to send notifications when resources are received that meet the criteria.") 958 protected SubscriptionChannelComponent channel; 959 960 private static final long serialVersionUID = 1072504988L; 961 962 /** 963 * Constructor 964 */ 965 public Subscription() { 966 super(); 967 } 968 969 /** 970 * Constructor 971 */ 972 public Subscription(Enumeration<SubscriptionStatus> status, StringType reason, StringType criteria, 973 SubscriptionChannelComponent channel) { 974 super(); 975 this.status = status; 976 this.reason = reason; 977 this.criteria = criteria; 978 this.channel = channel; 979 } 980 981 /** 982 * @return {@link #status} (The status of the subscription, which marks the 983 * server state for managing the subscription.). This is the underlying 984 * object with id, value and extensions. The accessor "getStatus" gives 985 * direct access to the value 986 */ 987 public Enumeration<SubscriptionStatus> getStatusElement() { 988 if (this.status == null) 989 if (Configuration.errorOnAutoCreate()) 990 throw new Error("Attempt to auto-create Subscription.status"); 991 else if (Configuration.doAutoCreate()) 992 this.status = new Enumeration<SubscriptionStatus>(new SubscriptionStatusEnumFactory()); // bb 993 return this.status; 994 } 995 996 public boolean hasStatusElement() { 997 return this.status != null && !this.status.isEmpty(); 998 } 999 1000 public boolean hasStatus() { 1001 return this.status != null && !this.status.isEmpty(); 1002 } 1003 1004 /** 1005 * @param value {@link #status} (The status of the subscription, which marks the 1006 * server state for managing the subscription.). This is the 1007 * underlying object with id, value and extensions. The accessor 1008 * "getStatus" gives direct access to the value 1009 */ 1010 public Subscription setStatusElement(Enumeration<SubscriptionStatus> value) { 1011 this.status = value; 1012 return this; 1013 } 1014 1015 /** 1016 * @return The status of the subscription, which marks the server state for 1017 * managing the subscription. 1018 */ 1019 public SubscriptionStatus getStatus() { 1020 return this.status == null ? null : this.status.getValue(); 1021 } 1022 1023 /** 1024 * @param value The status of the subscription, which marks the server state for 1025 * managing the subscription. 1026 */ 1027 public Subscription setStatus(SubscriptionStatus value) { 1028 if (this.status == null) 1029 this.status = new Enumeration<SubscriptionStatus>(new SubscriptionStatusEnumFactory()); 1030 this.status.setValue(value); 1031 return this; 1032 } 1033 1034 /** 1035 * @return {@link #contact} (Contact details for a human to contact about the 1036 * subscription. The primary use of this for system administrator 1037 * troubleshooting.) 1038 */ 1039 public List<ContactPoint> getContact() { 1040 if (this.contact == null) 1041 this.contact = new ArrayList<ContactPoint>(); 1042 return this.contact; 1043 } 1044 1045 /** 1046 * @return Returns a reference to <code>this</code> for easy method chaining 1047 */ 1048 public Subscription setContact(List<ContactPoint> theContact) { 1049 this.contact = theContact; 1050 return this; 1051 } 1052 1053 public boolean hasContact() { 1054 if (this.contact == null) 1055 return false; 1056 for (ContactPoint item : this.contact) 1057 if (!item.isEmpty()) 1058 return true; 1059 return false; 1060 } 1061 1062 public ContactPoint addContact() { // 3 1063 ContactPoint t = new ContactPoint(); 1064 if (this.contact == null) 1065 this.contact = new ArrayList<ContactPoint>(); 1066 this.contact.add(t); 1067 return t; 1068 } 1069 1070 public Subscription addContact(ContactPoint t) { // 3 1071 if (t == null) 1072 return this; 1073 if (this.contact == null) 1074 this.contact = new ArrayList<ContactPoint>(); 1075 this.contact.add(t); 1076 return this; 1077 } 1078 1079 /** 1080 * @return The first repetition of repeating field {@link #contact}, creating it 1081 * if it does not already exist 1082 */ 1083 public ContactPoint getContactFirstRep() { 1084 if (getContact().isEmpty()) { 1085 addContact(); 1086 } 1087 return getContact().get(0); 1088 } 1089 1090 /** 1091 * @return {@link #end} (The time for the server to turn the subscription off.). 1092 * This is the underlying object with id, value and extensions. The 1093 * accessor "getEnd" gives direct access to the value 1094 */ 1095 public InstantType getEndElement() { 1096 if (this.end == null) 1097 if (Configuration.errorOnAutoCreate()) 1098 throw new Error("Attempt to auto-create Subscription.end"); 1099 else if (Configuration.doAutoCreate()) 1100 this.end = new InstantType(); // bb 1101 return this.end; 1102 } 1103 1104 public boolean hasEndElement() { 1105 return this.end != null && !this.end.isEmpty(); 1106 } 1107 1108 public boolean hasEnd() { 1109 return this.end != null && !this.end.isEmpty(); 1110 } 1111 1112 /** 1113 * @param value {@link #end} (The time for the server to turn the subscription 1114 * off.). This is the underlying object with id, value and 1115 * extensions. The accessor "getEnd" gives direct access to the 1116 * value 1117 */ 1118 public Subscription setEndElement(InstantType value) { 1119 this.end = value; 1120 return this; 1121 } 1122 1123 /** 1124 * @return The time for the server to turn the subscription off. 1125 */ 1126 public Date getEnd() { 1127 return this.end == null ? null : this.end.getValue(); 1128 } 1129 1130 /** 1131 * @param value The time for the server to turn the subscription off. 1132 */ 1133 public Subscription setEnd(Date value) { 1134 if (value == null) 1135 this.end = null; 1136 else { 1137 if (this.end == null) 1138 this.end = new InstantType(); 1139 this.end.setValue(value); 1140 } 1141 return this; 1142 } 1143 1144 /** 1145 * @return {@link #reason} (A description of why this subscription is defined.). 1146 * This is the underlying object with id, value and extensions. The 1147 * accessor "getReason" gives direct access to the value 1148 */ 1149 public StringType getReasonElement() { 1150 if (this.reason == null) 1151 if (Configuration.errorOnAutoCreate()) 1152 throw new Error("Attempt to auto-create Subscription.reason"); 1153 else if (Configuration.doAutoCreate()) 1154 this.reason = new StringType(); // bb 1155 return this.reason; 1156 } 1157 1158 public boolean hasReasonElement() { 1159 return this.reason != null && !this.reason.isEmpty(); 1160 } 1161 1162 public boolean hasReason() { 1163 return this.reason != null && !this.reason.isEmpty(); 1164 } 1165 1166 /** 1167 * @param value {@link #reason} (A description of why this subscription is 1168 * defined.). This is the underlying object with id, value and 1169 * extensions. The accessor "getReason" gives direct access to the 1170 * value 1171 */ 1172 public Subscription setReasonElement(StringType value) { 1173 this.reason = value; 1174 return this; 1175 } 1176 1177 /** 1178 * @return A description of why this subscription is defined. 1179 */ 1180 public String getReason() { 1181 return this.reason == null ? null : this.reason.getValue(); 1182 } 1183 1184 /** 1185 * @param value A description of why this subscription is defined. 1186 */ 1187 public Subscription setReason(String value) { 1188 if (this.reason == null) 1189 this.reason = new StringType(); 1190 this.reason.setValue(value); 1191 return this; 1192 } 1193 1194 /** 1195 * @return {@link #criteria} (The rules that the server should use to determine 1196 * when to generate notifications for this subscription.). This is the 1197 * underlying object with id, value and extensions. The accessor 1198 * "getCriteria" gives direct access to the value 1199 */ 1200 public StringType getCriteriaElement() { 1201 if (this.criteria == null) 1202 if (Configuration.errorOnAutoCreate()) 1203 throw new Error("Attempt to auto-create Subscription.criteria"); 1204 else if (Configuration.doAutoCreate()) 1205 this.criteria = new StringType(); // bb 1206 return this.criteria; 1207 } 1208 1209 public boolean hasCriteriaElement() { 1210 return this.criteria != null && !this.criteria.isEmpty(); 1211 } 1212 1213 public boolean hasCriteria() { 1214 return this.criteria != null && !this.criteria.isEmpty(); 1215 } 1216 1217 /** 1218 * @param value {@link #criteria} (The rules that the server should use to 1219 * determine when to generate notifications for this 1220 * subscription.). This is the underlying object with id, value and 1221 * extensions. The accessor "getCriteria" gives direct access to 1222 * the value 1223 */ 1224 public Subscription setCriteriaElement(StringType value) { 1225 this.criteria = value; 1226 return this; 1227 } 1228 1229 /** 1230 * @return The rules that the server should use to determine when to generate 1231 * notifications for this subscription. 1232 */ 1233 public String getCriteria() { 1234 return this.criteria == null ? null : this.criteria.getValue(); 1235 } 1236 1237 /** 1238 * @param value The rules that the server should use to determine when to 1239 * generate notifications for this subscription. 1240 */ 1241 public Subscription setCriteria(String value) { 1242 if (this.criteria == null) 1243 this.criteria = new StringType(); 1244 this.criteria.setValue(value); 1245 return this; 1246 } 1247 1248 /** 1249 * @return {@link #error} (A record of the last error that occurred when the 1250 * server processed a notification.). This is the underlying object with 1251 * id, value and extensions. The accessor "getError" gives direct access 1252 * to the value 1253 */ 1254 public StringType getErrorElement() { 1255 if (this.error == null) 1256 if (Configuration.errorOnAutoCreate()) 1257 throw new Error("Attempt to auto-create Subscription.error"); 1258 else if (Configuration.doAutoCreate()) 1259 this.error = new StringType(); // bb 1260 return this.error; 1261 } 1262 1263 public boolean hasErrorElement() { 1264 return this.error != null && !this.error.isEmpty(); 1265 } 1266 1267 public boolean hasError() { 1268 return this.error != null && !this.error.isEmpty(); 1269 } 1270 1271 /** 1272 * @param value {@link #error} (A record of the last error that occurred when 1273 * the server processed a notification.). This is the underlying 1274 * object with id, value and extensions. The accessor "getError" 1275 * gives direct access to the value 1276 */ 1277 public Subscription setErrorElement(StringType value) { 1278 this.error = value; 1279 return this; 1280 } 1281 1282 /** 1283 * @return A record of the last error that occurred when the server processed a 1284 * notification. 1285 */ 1286 public String getError() { 1287 return this.error == null ? null : this.error.getValue(); 1288 } 1289 1290 /** 1291 * @param value A record of the last error that occurred when the server 1292 * processed a notification. 1293 */ 1294 public Subscription setError(String value) { 1295 if (Utilities.noString(value)) 1296 this.error = null; 1297 else { 1298 if (this.error == null) 1299 this.error = new StringType(); 1300 this.error.setValue(value); 1301 } 1302 return this; 1303 } 1304 1305 /** 1306 * @return {@link #channel} (Details where to send notifications when resources 1307 * are received that meet the criteria.) 1308 */ 1309 public SubscriptionChannelComponent getChannel() { 1310 if (this.channel == null) 1311 if (Configuration.errorOnAutoCreate()) 1312 throw new Error("Attempt to auto-create Subscription.channel"); 1313 else if (Configuration.doAutoCreate()) 1314 this.channel = new SubscriptionChannelComponent(); // cc 1315 return this.channel; 1316 } 1317 1318 public boolean hasChannel() { 1319 return this.channel != null && !this.channel.isEmpty(); 1320 } 1321 1322 /** 1323 * @param value {@link #channel} (Details where to send notifications when 1324 * resources are received that meet the criteria.) 1325 */ 1326 public Subscription setChannel(SubscriptionChannelComponent value) { 1327 this.channel = value; 1328 return this; 1329 } 1330 1331 protected void listChildren(List<Property> children) { 1332 super.listChildren(children); 1333 children.add(new Property("status", "code", 1334 "The status of the subscription, which marks the server state for managing the subscription.", 0, 1, status)); 1335 children.add(new Property("contact", "ContactPoint", 1336 "Contact details for a human to contact about the subscription. The primary use of this for system administrator troubleshooting.", 1337 0, java.lang.Integer.MAX_VALUE, contact)); 1338 children.add(new Property("end", "instant", "The time for the server to turn the subscription off.", 0, 1, end)); 1339 children.add(new Property("reason", "string", "A description of why this subscription is defined.", 0, 1, reason)); 1340 children.add(new Property("criteria", "string", 1341 "The rules that the server should use to determine when to generate notifications for this subscription.", 0, 1, 1342 criteria)); 1343 children.add(new Property("error", "string", 1344 "A record of the last error that occurred when the server processed a notification.", 0, 1, error)); 1345 children.add(new Property("channel", "", 1346 "Details where to send notifications when resources are received that meet the criteria.", 0, 1, channel)); 1347 } 1348 1349 @Override 1350 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1351 switch (_hash) { 1352 case -892481550: 1353 /* status */ return new Property("status", "code", 1354 "The status of the subscription, which marks the server state for managing the subscription.", 0, 1, status); 1355 case 951526432: 1356 /* contact */ return new Property("contact", "ContactPoint", 1357 "Contact details for a human to contact about the subscription. The primary use of this for system administrator troubleshooting.", 1358 0, java.lang.Integer.MAX_VALUE, contact); 1359 case 100571: 1360 /* end */ return new Property("end", "instant", "The time for the server to turn the subscription off.", 0, 1, 1361 end); 1362 case -934964668: 1363 /* reason */ return new Property("reason", "string", "A description of why this subscription is defined.", 0, 1, 1364 reason); 1365 case 1952046943: 1366 /* criteria */ return new Property("criteria", "string", 1367 "The rules that the server should use to determine when to generate notifications for this subscription.", 0, 1368 1, criteria); 1369 case 96784904: 1370 /* error */ return new Property("error", "string", 1371 "A record of the last error that occurred when the server processed a notification.", 0, 1, error); 1372 case 738950403: 1373 /* channel */ return new Property("channel", "", 1374 "Details where to send notifications when resources are received that meet the criteria.", 0, 1, channel); 1375 default: 1376 return super.getNamedProperty(_hash, _name, _checkValid); 1377 } 1378 1379 } 1380 1381 @Override 1382 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1383 switch (hash) { 1384 case -892481550: 1385 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<SubscriptionStatus> 1386 case 951526432: 1387 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactPoint 1388 case 100571: 1389 /* end */ return this.end == null ? new Base[0] : new Base[] { this.end }; // InstantType 1390 case -934964668: 1391 /* reason */ return this.reason == null ? new Base[0] : new Base[] { this.reason }; // StringType 1392 case 1952046943: 1393 /* criteria */ return this.criteria == null ? new Base[0] : new Base[] { this.criteria }; // StringType 1394 case 96784904: 1395 /* error */ return this.error == null ? new Base[0] : new Base[] { this.error }; // StringType 1396 case 738950403: 1397 /* channel */ return this.channel == null ? new Base[0] : new Base[] { this.channel }; // SubscriptionChannelComponent 1398 default: 1399 return super.getProperty(hash, name, checkValid); 1400 } 1401 1402 } 1403 1404 @Override 1405 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1406 switch (hash) { 1407 case -892481550: // status 1408 value = new SubscriptionStatusEnumFactory().fromType(castToCode(value)); 1409 this.status = (Enumeration) value; // Enumeration<SubscriptionStatus> 1410 return value; 1411 case 951526432: // contact 1412 this.getContact().add(castToContactPoint(value)); // ContactPoint 1413 return value; 1414 case 100571: // end 1415 this.end = castToInstant(value); // InstantType 1416 return value; 1417 case -934964668: // reason 1418 this.reason = castToString(value); // StringType 1419 return value; 1420 case 1952046943: // criteria 1421 this.criteria = castToString(value); // StringType 1422 return value; 1423 case 96784904: // error 1424 this.error = castToString(value); // StringType 1425 return value; 1426 case 738950403: // channel 1427 this.channel = (SubscriptionChannelComponent) value; // SubscriptionChannelComponent 1428 return value; 1429 default: 1430 return super.setProperty(hash, name, value); 1431 } 1432 1433 } 1434 1435 @Override 1436 public Base setProperty(String name, Base value) throws FHIRException { 1437 if (name.equals("status")) { 1438 value = new SubscriptionStatusEnumFactory().fromType(castToCode(value)); 1439 this.status = (Enumeration) value; // Enumeration<SubscriptionStatus> 1440 } else if (name.equals("contact")) { 1441 this.getContact().add(castToContactPoint(value)); 1442 } else if (name.equals("end")) { 1443 this.end = castToInstant(value); // InstantType 1444 } else if (name.equals("reason")) { 1445 this.reason = castToString(value); // StringType 1446 } else if (name.equals("criteria")) { 1447 this.criteria = castToString(value); // StringType 1448 } else if (name.equals("error")) { 1449 this.error = castToString(value); // StringType 1450 } else if (name.equals("channel")) { 1451 this.channel = (SubscriptionChannelComponent) value; // SubscriptionChannelComponent 1452 } else 1453 return super.setProperty(name, value); 1454 return value; 1455 } 1456 1457 @Override 1458 public void removeChild(String name, Base value) throws FHIRException { 1459 if (name.equals("status")) { 1460 this.status = null; 1461 } else if (name.equals("contact")) { 1462 this.getContact().remove(castToContactPoint(value)); 1463 } else if (name.equals("end")) { 1464 this.end = null; 1465 } else if (name.equals("reason")) { 1466 this.reason = null; 1467 } else if (name.equals("criteria")) { 1468 this.criteria = null; 1469 } else if (name.equals("error")) { 1470 this.error = null; 1471 } else if (name.equals("channel")) { 1472 this.channel = (SubscriptionChannelComponent) value; // SubscriptionChannelComponent 1473 } else 1474 super.removeChild(name, value); 1475 1476 } 1477 1478 @Override 1479 public Base makeProperty(int hash, String name) throws FHIRException { 1480 switch (hash) { 1481 case -892481550: 1482 return getStatusElement(); 1483 case 951526432: 1484 return addContact(); 1485 case 100571: 1486 return getEndElement(); 1487 case -934964668: 1488 return getReasonElement(); 1489 case 1952046943: 1490 return getCriteriaElement(); 1491 case 96784904: 1492 return getErrorElement(); 1493 case 738950403: 1494 return getChannel(); 1495 default: 1496 return super.makeProperty(hash, name); 1497 } 1498 1499 } 1500 1501 @Override 1502 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1503 switch (hash) { 1504 case -892481550: 1505 /* status */ return new String[] { "code" }; 1506 case 951526432: 1507 /* contact */ return new String[] { "ContactPoint" }; 1508 case 100571: 1509 /* end */ return new String[] { "instant" }; 1510 case -934964668: 1511 /* reason */ return new String[] { "string" }; 1512 case 1952046943: 1513 /* criteria */ return new String[] { "string" }; 1514 case 96784904: 1515 /* error */ return new String[] { "string" }; 1516 case 738950403: 1517 /* channel */ return new String[] {}; 1518 default: 1519 return super.getTypesForProperty(hash, name); 1520 } 1521 1522 } 1523 1524 @Override 1525 public Base addChild(String name) throws FHIRException { 1526 if (name.equals("status")) { 1527 throw new FHIRException("Cannot call addChild on a singleton property Subscription.status"); 1528 } else if (name.equals("contact")) { 1529 return addContact(); 1530 } else if (name.equals("end")) { 1531 throw new FHIRException("Cannot call addChild on a singleton property Subscription.end"); 1532 } else if (name.equals("reason")) { 1533 throw new FHIRException("Cannot call addChild on a singleton property Subscription.reason"); 1534 } else if (name.equals("criteria")) { 1535 throw new FHIRException("Cannot call addChild on a singleton property Subscription.criteria"); 1536 } else if (name.equals("error")) { 1537 throw new FHIRException("Cannot call addChild on a singleton property Subscription.error"); 1538 } else if (name.equals("channel")) { 1539 this.channel = new SubscriptionChannelComponent(); 1540 return this.channel; 1541 } else 1542 return super.addChild(name); 1543 } 1544 1545 public String fhirType() { 1546 return "Subscription"; 1547 1548 } 1549 1550 public Subscription copy() { 1551 Subscription dst = new Subscription(); 1552 copyValues(dst); 1553 return dst; 1554 } 1555 1556 public void copyValues(Subscription dst) { 1557 super.copyValues(dst); 1558 dst.status = status == null ? null : status.copy(); 1559 if (contact != null) { 1560 dst.contact = new ArrayList<ContactPoint>(); 1561 for (ContactPoint i : contact) 1562 dst.contact.add(i.copy()); 1563 } 1564 ; 1565 dst.end = end == null ? null : end.copy(); 1566 dst.reason = reason == null ? null : reason.copy(); 1567 dst.criteria = criteria == null ? null : criteria.copy(); 1568 dst.error = error == null ? null : error.copy(); 1569 dst.channel = channel == null ? null : channel.copy(); 1570 } 1571 1572 protected Subscription typedCopy() { 1573 return copy(); 1574 } 1575 1576 @Override 1577 public boolean equalsDeep(Base other_) { 1578 if (!super.equalsDeep(other_)) 1579 return false; 1580 if (!(other_ instanceof Subscription)) 1581 return false; 1582 Subscription o = (Subscription) other_; 1583 return compareDeep(status, o.status, true) && compareDeep(contact, o.contact, true) && compareDeep(end, o.end, true) 1584 && compareDeep(reason, o.reason, true) && compareDeep(criteria, o.criteria, true) 1585 && compareDeep(error, o.error, true) && compareDeep(channel, o.channel, true); 1586 } 1587 1588 @Override 1589 public boolean equalsShallow(Base other_) { 1590 if (!super.equalsShallow(other_)) 1591 return false; 1592 if (!(other_ instanceof Subscription)) 1593 return false; 1594 Subscription o = (Subscription) other_; 1595 return compareValues(status, o.status, true) && compareValues(end, o.end, true) 1596 && compareValues(reason, o.reason, true) && compareValues(criteria, o.criteria, true) 1597 && compareValues(error, o.error, true); 1598 } 1599 1600 public boolean isEmpty() { 1601 return super.isEmpty() 1602 && ca.uhn.fhir.util.ElementUtil.isEmpty(status, contact, end, reason, criteria, error, channel); 1603 } 1604 1605 @Override 1606 public ResourceType getResourceType() { 1607 return ResourceType.Subscription; 1608 } 1609 1610 /** 1611 * Search parameter: <b>payload</b> 1612 * <p> 1613 * Description: <b>The mime-type of the notification payload</b><br> 1614 * Type: <b>token</b><br> 1615 * Path: <b>Subscription.channel.payload</b><br> 1616 * </p> 1617 */ 1618 @SearchParamDefinition(name = "payload", path = "Subscription.channel.payload", description = "The mime-type of the notification payload", type = "token") 1619 public static final String SP_PAYLOAD = "payload"; 1620 /** 1621 * <b>Fluent Client</b> search parameter constant for <b>payload</b> 1622 * <p> 1623 * Description: <b>The mime-type of the notification payload</b><br> 1624 * Type: <b>token</b><br> 1625 * Path: <b>Subscription.channel.payload</b><br> 1626 * </p> 1627 */ 1628 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PAYLOAD = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1629 SP_PAYLOAD); 1630 1631 /** 1632 * Search parameter: <b>criteria</b> 1633 * <p> 1634 * Description: <b>The search rules used to determine when to send a 1635 * notification</b><br> 1636 * Type: <b>string</b><br> 1637 * Path: <b>Subscription.criteria</b><br> 1638 * </p> 1639 */ 1640 @SearchParamDefinition(name = "criteria", path = "Subscription.criteria", description = "The search rules used to determine when to send a notification", type = "string") 1641 public static final String SP_CRITERIA = "criteria"; 1642 /** 1643 * <b>Fluent Client</b> search parameter constant for <b>criteria</b> 1644 * <p> 1645 * Description: <b>The search rules used to determine when to send a 1646 * notification</b><br> 1647 * Type: <b>string</b><br> 1648 * Path: <b>Subscription.criteria</b><br> 1649 * </p> 1650 */ 1651 public static final ca.uhn.fhir.rest.gclient.StringClientParam CRITERIA = new ca.uhn.fhir.rest.gclient.StringClientParam( 1652 SP_CRITERIA); 1653 1654 /** 1655 * Search parameter: <b>contact</b> 1656 * <p> 1657 * Description: <b>Contact details for the subscription</b><br> 1658 * Type: <b>token</b><br> 1659 * Path: <b>Subscription.contact</b><br> 1660 * </p> 1661 */ 1662 @SearchParamDefinition(name = "contact", path = "Subscription.contact", description = "Contact details for the subscription", type = "token") 1663 public static final String SP_CONTACT = "contact"; 1664 /** 1665 * <b>Fluent Client</b> search parameter constant for <b>contact</b> 1666 * <p> 1667 * Description: <b>Contact details for the subscription</b><br> 1668 * Type: <b>token</b><br> 1669 * Path: <b>Subscription.contact</b><br> 1670 * </p> 1671 */ 1672 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTACT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1673 SP_CONTACT); 1674 1675 /** 1676 * Search parameter: <b>type</b> 1677 * <p> 1678 * Description: <b>The type of channel for the sent notifications</b><br> 1679 * Type: <b>token</b><br> 1680 * Path: <b>Subscription.channel.type</b><br> 1681 * </p> 1682 */ 1683 @SearchParamDefinition(name = "type", path = "Subscription.channel.type", description = "The type of channel for the sent notifications", type = "token") 1684 public static final String SP_TYPE = "type"; 1685 /** 1686 * <b>Fluent Client</b> search parameter constant for <b>type</b> 1687 * <p> 1688 * Description: <b>The type of channel for the sent notifications</b><br> 1689 * Type: <b>token</b><br> 1690 * Path: <b>Subscription.channel.type</b><br> 1691 * </p> 1692 */ 1693 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1694 SP_TYPE); 1695 1696 /** 1697 * Search parameter: <b>url</b> 1698 * <p> 1699 * Description: <b>The uri that will receive the notifications</b><br> 1700 * Type: <b>uri</b><br> 1701 * Path: <b>Subscription.channel.endpoint</b><br> 1702 * </p> 1703 */ 1704 @SearchParamDefinition(name = "url", path = "Subscription.channel.endpoint", description = "The uri that will receive the notifications", type = "uri") 1705 public static final String SP_URL = "url"; 1706 /** 1707 * <b>Fluent Client</b> search parameter constant for <b>url</b> 1708 * <p> 1709 * Description: <b>The uri that will receive the notifications</b><br> 1710 * Type: <b>uri</b><br> 1711 * Path: <b>Subscription.channel.endpoint</b><br> 1712 * </p> 1713 */ 1714 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 1715 1716 /** 1717 * Search parameter: <b>status</b> 1718 * <p> 1719 * Description: <b>The current state of the subscription</b><br> 1720 * Type: <b>token</b><br> 1721 * Path: <b>Subscription.status</b><br> 1722 * </p> 1723 */ 1724 @SearchParamDefinition(name = "status", path = "Subscription.status", description = "The current state of the subscription", type = "token") 1725 public static final String SP_STATUS = "status"; 1726 /** 1727 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1728 * <p> 1729 * Description: <b>The current state of the subscription</b><br> 1730 * Type: <b>token</b><br> 1731 * Path: <b>Subscription.status</b><br> 1732 * </p> 1733 */ 1734 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1735 SP_STATUS); 1736 1737}