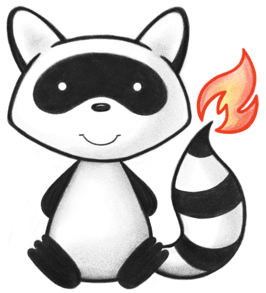
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044 045/** 046 * Todo. 047 */ 048@ResourceDef(name = "SubstanceReferenceInformation", profile = "http://hl7.org/fhir/StructureDefinition/SubstanceReferenceInformation") 049public class SubstanceReferenceInformation extends DomainResource { 050 051 @Block() 052 public static class SubstanceReferenceInformationGeneComponent extends BackboneElement 053 implements IBaseBackboneElement { 054 /** 055 * Todo. 056 */ 057 @Child(name = "geneSequenceOrigin", type = { 058 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 059 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 060 protected CodeableConcept geneSequenceOrigin; 061 062 /** 063 * Todo. 064 */ 065 @Child(name = "gene", type = { 066 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 067 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 068 protected CodeableConcept gene; 069 070 /** 071 * Todo. 072 */ 073 @Child(name = "source", type = { 074 DocumentReference.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 075 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 076 protected List<Reference> source; 077 /** 078 * The actual objects that are the target of the reference (Todo.) 079 */ 080 protected List<DocumentReference> sourceTarget; 081 082 private static final long serialVersionUID = 1615185105L; 083 084 /** 085 * Constructor 086 */ 087 public SubstanceReferenceInformationGeneComponent() { 088 super(); 089 } 090 091 /** 092 * @return {@link #geneSequenceOrigin} (Todo.) 093 */ 094 public CodeableConcept getGeneSequenceOrigin() { 095 if (this.geneSequenceOrigin == null) 096 if (Configuration.errorOnAutoCreate()) 097 throw new Error("Attempt to auto-create SubstanceReferenceInformationGeneComponent.geneSequenceOrigin"); 098 else if (Configuration.doAutoCreate()) 099 this.geneSequenceOrigin = new CodeableConcept(); // cc 100 return this.geneSequenceOrigin; 101 } 102 103 public boolean hasGeneSequenceOrigin() { 104 return this.geneSequenceOrigin != null && !this.geneSequenceOrigin.isEmpty(); 105 } 106 107 /** 108 * @param value {@link #geneSequenceOrigin} (Todo.) 109 */ 110 public SubstanceReferenceInformationGeneComponent setGeneSequenceOrigin(CodeableConcept value) { 111 this.geneSequenceOrigin = value; 112 return this; 113 } 114 115 /** 116 * @return {@link #gene} (Todo.) 117 */ 118 public CodeableConcept getGene() { 119 if (this.gene == null) 120 if (Configuration.errorOnAutoCreate()) 121 throw new Error("Attempt to auto-create SubstanceReferenceInformationGeneComponent.gene"); 122 else if (Configuration.doAutoCreate()) 123 this.gene = new CodeableConcept(); // cc 124 return this.gene; 125 } 126 127 public boolean hasGene() { 128 return this.gene != null && !this.gene.isEmpty(); 129 } 130 131 /** 132 * @param value {@link #gene} (Todo.) 133 */ 134 public SubstanceReferenceInformationGeneComponent setGene(CodeableConcept value) { 135 this.gene = value; 136 return this; 137 } 138 139 /** 140 * @return {@link #source} (Todo.) 141 */ 142 public List<Reference> getSource() { 143 if (this.source == null) 144 this.source = new ArrayList<Reference>(); 145 return this.source; 146 } 147 148 /** 149 * @return Returns a reference to <code>this</code> for easy method chaining 150 */ 151 public SubstanceReferenceInformationGeneComponent setSource(List<Reference> theSource) { 152 this.source = theSource; 153 return this; 154 } 155 156 public boolean hasSource() { 157 if (this.source == null) 158 return false; 159 for (Reference item : this.source) 160 if (!item.isEmpty()) 161 return true; 162 return false; 163 } 164 165 public Reference addSource() { // 3 166 Reference t = new Reference(); 167 if (this.source == null) 168 this.source = new ArrayList<Reference>(); 169 this.source.add(t); 170 return t; 171 } 172 173 public SubstanceReferenceInformationGeneComponent addSource(Reference t) { // 3 174 if (t == null) 175 return this; 176 if (this.source == null) 177 this.source = new ArrayList<Reference>(); 178 this.source.add(t); 179 return this; 180 } 181 182 /** 183 * @return The first repetition of repeating field {@link #source}, creating it 184 * if it does not already exist 185 */ 186 public Reference getSourceFirstRep() { 187 if (getSource().isEmpty()) { 188 addSource(); 189 } 190 return getSource().get(0); 191 } 192 193 protected void listChildren(List<Property> children) { 194 super.listChildren(children); 195 children.add(new Property("geneSequenceOrigin", "CodeableConcept", "Todo.", 0, 1, geneSequenceOrigin)); 196 children.add(new Property("gene", "CodeableConcept", "Todo.", 0, 1, gene)); 197 children 198 .add(new Property("source", "Reference(DocumentReference)", "Todo.", 0, java.lang.Integer.MAX_VALUE, source)); 199 } 200 201 @Override 202 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 203 switch (_hash) { 204 case -1089463108: 205 /* geneSequenceOrigin */ return new Property("geneSequenceOrigin", "CodeableConcept", "Todo.", 0, 1, 206 geneSequenceOrigin); 207 case 3169045: 208 /* gene */ return new Property("gene", "CodeableConcept", "Todo.", 0, 1, gene); 209 case -896505829: 210 /* source */ return new Property("source", "Reference(DocumentReference)", "Todo.", 0, 211 java.lang.Integer.MAX_VALUE, source); 212 default: 213 return super.getNamedProperty(_hash, _name, _checkValid); 214 } 215 216 } 217 218 @Override 219 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 220 switch (hash) { 221 case -1089463108: 222 /* geneSequenceOrigin */ return this.geneSequenceOrigin == null ? new Base[0] 223 : new Base[] { this.geneSequenceOrigin }; // CodeableConcept 224 case 3169045: 225 /* gene */ return this.gene == null ? new Base[0] : new Base[] { this.gene }; // CodeableConcept 226 case -896505829: 227 /* source */ return this.source == null ? new Base[0] : this.source.toArray(new Base[this.source.size()]); // Reference 228 default: 229 return super.getProperty(hash, name, checkValid); 230 } 231 232 } 233 234 @Override 235 public Base setProperty(int hash, String name, Base value) throws FHIRException { 236 switch (hash) { 237 case -1089463108: // geneSequenceOrigin 238 this.geneSequenceOrigin = castToCodeableConcept(value); // CodeableConcept 239 return value; 240 case 3169045: // gene 241 this.gene = castToCodeableConcept(value); // CodeableConcept 242 return value; 243 case -896505829: // source 244 this.getSource().add(castToReference(value)); // Reference 245 return value; 246 default: 247 return super.setProperty(hash, name, value); 248 } 249 250 } 251 252 @Override 253 public Base setProperty(String name, Base value) throws FHIRException { 254 if (name.equals("geneSequenceOrigin")) { 255 this.geneSequenceOrigin = castToCodeableConcept(value); // CodeableConcept 256 } else if (name.equals("gene")) { 257 this.gene = castToCodeableConcept(value); // CodeableConcept 258 } else if (name.equals("source")) { 259 this.getSource().add(castToReference(value)); 260 } else 261 return super.setProperty(name, value); 262 return value; 263 } 264 265 @Override 266 public void removeChild(String name, Base value) throws FHIRException { 267 if (name.equals("geneSequenceOrigin")) { 268 this.geneSequenceOrigin = null; 269 } else if (name.equals("gene")) { 270 this.gene = null; 271 } else if (name.equals("source")) { 272 this.getSource().remove(castToReference(value)); 273 } else 274 super.removeChild(name, value); 275 276 } 277 278 @Override 279 public Base makeProperty(int hash, String name) throws FHIRException { 280 switch (hash) { 281 case -1089463108: 282 return getGeneSequenceOrigin(); 283 case 3169045: 284 return getGene(); 285 case -896505829: 286 return addSource(); 287 default: 288 return super.makeProperty(hash, name); 289 } 290 291 } 292 293 @Override 294 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 295 switch (hash) { 296 case -1089463108: 297 /* geneSequenceOrigin */ return new String[] { "CodeableConcept" }; 298 case 3169045: 299 /* gene */ return new String[] { "CodeableConcept" }; 300 case -896505829: 301 /* source */ return new String[] { "Reference" }; 302 default: 303 return super.getTypesForProperty(hash, name); 304 } 305 306 } 307 308 @Override 309 public Base addChild(String name) throws FHIRException { 310 if (name.equals("geneSequenceOrigin")) { 311 this.geneSequenceOrigin = new CodeableConcept(); 312 return this.geneSequenceOrigin; 313 } else if (name.equals("gene")) { 314 this.gene = new CodeableConcept(); 315 return this.gene; 316 } else if (name.equals("source")) { 317 return addSource(); 318 } else 319 return super.addChild(name); 320 } 321 322 public SubstanceReferenceInformationGeneComponent copy() { 323 SubstanceReferenceInformationGeneComponent dst = new SubstanceReferenceInformationGeneComponent(); 324 copyValues(dst); 325 return dst; 326 } 327 328 public void copyValues(SubstanceReferenceInformationGeneComponent dst) { 329 super.copyValues(dst); 330 dst.geneSequenceOrigin = geneSequenceOrigin == null ? null : geneSequenceOrigin.copy(); 331 dst.gene = gene == null ? null : gene.copy(); 332 if (source != null) { 333 dst.source = new ArrayList<Reference>(); 334 for (Reference i : source) 335 dst.source.add(i.copy()); 336 } 337 ; 338 } 339 340 @Override 341 public boolean equalsDeep(Base other_) { 342 if (!super.equalsDeep(other_)) 343 return false; 344 if (!(other_ instanceof SubstanceReferenceInformationGeneComponent)) 345 return false; 346 SubstanceReferenceInformationGeneComponent o = (SubstanceReferenceInformationGeneComponent) other_; 347 return compareDeep(geneSequenceOrigin, o.geneSequenceOrigin, true) && compareDeep(gene, o.gene, true) 348 && compareDeep(source, o.source, true); 349 } 350 351 @Override 352 public boolean equalsShallow(Base other_) { 353 if (!super.equalsShallow(other_)) 354 return false; 355 if (!(other_ instanceof SubstanceReferenceInformationGeneComponent)) 356 return false; 357 SubstanceReferenceInformationGeneComponent o = (SubstanceReferenceInformationGeneComponent) other_; 358 return true; 359 } 360 361 public boolean isEmpty() { 362 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(geneSequenceOrigin, gene, source); 363 } 364 365 public String fhirType() { 366 return "SubstanceReferenceInformation.gene"; 367 368 } 369 370 } 371 372 @Block() 373 public static class SubstanceReferenceInformationGeneElementComponent extends BackboneElement 374 implements IBaseBackboneElement { 375 /** 376 * Todo. 377 */ 378 @Child(name = "type", type = { 379 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 380 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 381 protected CodeableConcept type; 382 383 /** 384 * Todo. 385 */ 386 @Child(name = "element", type = { Identifier.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 387 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 388 protected Identifier element; 389 390 /** 391 * Todo. 392 */ 393 @Child(name = "source", type = { 394 DocumentReference.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 395 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 396 protected List<Reference> source; 397 /** 398 * The actual objects that are the target of the reference (Todo.) 399 */ 400 protected List<DocumentReference> sourceTarget; 401 402 private static final long serialVersionUID = 2055145950L; 403 404 /** 405 * Constructor 406 */ 407 public SubstanceReferenceInformationGeneElementComponent() { 408 super(); 409 } 410 411 /** 412 * @return {@link #type} (Todo.) 413 */ 414 public CodeableConcept getType() { 415 if (this.type == null) 416 if (Configuration.errorOnAutoCreate()) 417 throw new Error("Attempt to auto-create SubstanceReferenceInformationGeneElementComponent.type"); 418 else if (Configuration.doAutoCreate()) 419 this.type = new CodeableConcept(); // cc 420 return this.type; 421 } 422 423 public boolean hasType() { 424 return this.type != null && !this.type.isEmpty(); 425 } 426 427 /** 428 * @param value {@link #type} (Todo.) 429 */ 430 public SubstanceReferenceInformationGeneElementComponent setType(CodeableConcept value) { 431 this.type = value; 432 return this; 433 } 434 435 /** 436 * @return {@link #element} (Todo.) 437 */ 438 public Identifier getElement() { 439 if (this.element == null) 440 if (Configuration.errorOnAutoCreate()) 441 throw new Error("Attempt to auto-create SubstanceReferenceInformationGeneElementComponent.element"); 442 else if (Configuration.doAutoCreate()) 443 this.element = new Identifier(); // cc 444 return this.element; 445 } 446 447 public boolean hasElement() { 448 return this.element != null && !this.element.isEmpty(); 449 } 450 451 /** 452 * @param value {@link #element} (Todo.) 453 */ 454 public SubstanceReferenceInformationGeneElementComponent setElement(Identifier value) { 455 this.element = value; 456 return this; 457 } 458 459 /** 460 * @return {@link #source} (Todo.) 461 */ 462 public List<Reference> getSource() { 463 if (this.source == null) 464 this.source = new ArrayList<Reference>(); 465 return this.source; 466 } 467 468 /** 469 * @return Returns a reference to <code>this</code> for easy method chaining 470 */ 471 public SubstanceReferenceInformationGeneElementComponent setSource(List<Reference> theSource) { 472 this.source = theSource; 473 return this; 474 } 475 476 public boolean hasSource() { 477 if (this.source == null) 478 return false; 479 for (Reference item : this.source) 480 if (!item.isEmpty()) 481 return true; 482 return false; 483 } 484 485 public Reference addSource() { // 3 486 Reference t = new Reference(); 487 if (this.source == null) 488 this.source = new ArrayList<Reference>(); 489 this.source.add(t); 490 return t; 491 } 492 493 public SubstanceReferenceInformationGeneElementComponent addSource(Reference t) { // 3 494 if (t == null) 495 return this; 496 if (this.source == null) 497 this.source = new ArrayList<Reference>(); 498 this.source.add(t); 499 return this; 500 } 501 502 /** 503 * @return The first repetition of repeating field {@link #source}, creating it 504 * if it does not already exist 505 */ 506 public Reference getSourceFirstRep() { 507 if (getSource().isEmpty()) { 508 addSource(); 509 } 510 return getSource().get(0); 511 } 512 513 protected void listChildren(List<Property> children) { 514 super.listChildren(children); 515 children.add(new Property("type", "CodeableConcept", "Todo.", 0, 1, type)); 516 children.add(new Property("element", "Identifier", "Todo.", 0, 1, element)); 517 children 518 .add(new Property("source", "Reference(DocumentReference)", "Todo.", 0, java.lang.Integer.MAX_VALUE, source)); 519 } 520 521 @Override 522 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 523 switch (_hash) { 524 case 3575610: 525 /* type */ return new Property("type", "CodeableConcept", "Todo.", 0, 1, type); 526 case -1662836996: 527 /* element */ return new Property("element", "Identifier", "Todo.", 0, 1, element); 528 case -896505829: 529 /* source */ return new Property("source", "Reference(DocumentReference)", "Todo.", 0, 530 java.lang.Integer.MAX_VALUE, source); 531 default: 532 return super.getNamedProperty(_hash, _name, _checkValid); 533 } 534 535 } 536 537 @Override 538 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 539 switch (hash) { 540 case 3575610: 541 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 542 case -1662836996: 543 /* element */ return this.element == null ? new Base[0] : new Base[] { this.element }; // Identifier 544 case -896505829: 545 /* source */ return this.source == null ? new Base[0] : this.source.toArray(new Base[this.source.size()]); // Reference 546 default: 547 return super.getProperty(hash, name, checkValid); 548 } 549 550 } 551 552 @Override 553 public Base setProperty(int hash, String name, Base value) throws FHIRException { 554 switch (hash) { 555 case 3575610: // type 556 this.type = castToCodeableConcept(value); // CodeableConcept 557 return value; 558 case -1662836996: // element 559 this.element = castToIdentifier(value); // Identifier 560 return value; 561 case -896505829: // source 562 this.getSource().add(castToReference(value)); // Reference 563 return value; 564 default: 565 return super.setProperty(hash, name, value); 566 } 567 568 } 569 570 @Override 571 public Base setProperty(String name, Base value) throws FHIRException { 572 if (name.equals("type")) { 573 this.type = castToCodeableConcept(value); // CodeableConcept 574 } else if (name.equals("element")) { 575 this.element = castToIdentifier(value); // Identifier 576 } else if (name.equals("source")) { 577 this.getSource().add(castToReference(value)); 578 } else 579 return super.setProperty(name, value); 580 return value; 581 } 582 583 @Override 584 public void removeChild(String name, Base value) throws FHIRException { 585 if (name.equals("type")) { 586 this.type = null; 587 } else if (name.equals("element")) { 588 this.element = null; 589 } else if (name.equals("source")) { 590 this.getSource().remove(castToReference(value)); 591 } else 592 super.removeChild(name, value); 593 594 } 595 596 @Override 597 public Base makeProperty(int hash, String name) throws FHIRException { 598 switch (hash) { 599 case 3575610: 600 return getType(); 601 case -1662836996: 602 return getElement(); 603 case -896505829: 604 return addSource(); 605 default: 606 return super.makeProperty(hash, name); 607 } 608 609 } 610 611 @Override 612 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 613 switch (hash) { 614 case 3575610: 615 /* type */ return new String[] { "CodeableConcept" }; 616 case -1662836996: 617 /* element */ return new String[] { "Identifier" }; 618 case -896505829: 619 /* source */ return new String[] { "Reference" }; 620 default: 621 return super.getTypesForProperty(hash, name); 622 } 623 624 } 625 626 @Override 627 public Base addChild(String name) throws FHIRException { 628 if (name.equals("type")) { 629 this.type = new CodeableConcept(); 630 return this.type; 631 } else if (name.equals("element")) { 632 this.element = new Identifier(); 633 return this.element; 634 } else if (name.equals("source")) { 635 return addSource(); 636 } else 637 return super.addChild(name); 638 } 639 640 public SubstanceReferenceInformationGeneElementComponent copy() { 641 SubstanceReferenceInformationGeneElementComponent dst = new SubstanceReferenceInformationGeneElementComponent(); 642 copyValues(dst); 643 return dst; 644 } 645 646 public void copyValues(SubstanceReferenceInformationGeneElementComponent dst) { 647 super.copyValues(dst); 648 dst.type = type == null ? null : type.copy(); 649 dst.element = element == null ? null : element.copy(); 650 if (source != null) { 651 dst.source = new ArrayList<Reference>(); 652 for (Reference i : source) 653 dst.source.add(i.copy()); 654 } 655 ; 656 } 657 658 @Override 659 public boolean equalsDeep(Base other_) { 660 if (!super.equalsDeep(other_)) 661 return false; 662 if (!(other_ instanceof SubstanceReferenceInformationGeneElementComponent)) 663 return false; 664 SubstanceReferenceInformationGeneElementComponent o = (SubstanceReferenceInformationGeneElementComponent) other_; 665 return compareDeep(type, o.type, true) && compareDeep(element, o.element, true) 666 && compareDeep(source, o.source, true); 667 } 668 669 @Override 670 public boolean equalsShallow(Base other_) { 671 if (!super.equalsShallow(other_)) 672 return false; 673 if (!(other_ instanceof SubstanceReferenceInformationGeneElementComponent)) 674 return false; 675 SubstanceReferenceInformationGeneElementComponent o = (SubstanceReferenceInformationGeneElementComponent) other_; 676 return true; 677 } 678 679 public boolean isEmpty() { 680 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, element, source); 681 } 682 683 public String fhirType() { 684 return "SubstanceReferenceInformation.geneElement"; 685 686 } 687 688 } 689 690 @Block() 691 public static class SubstanceReferenceInformationClassificationComponent extends BackboneElement 692 implements IBaseBackboneElement { 693 /** 694 * Todo. 695 */ 696 @Child(name = "domain", type = { 697 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 698 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 699 protected CodeableConcept domain; 700 701 /** 702 * Todo. 703 */ 704 @Child(name = "classification", type = { 705 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 706 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 707 protected CodeableConcept classification; 708 709 /** 710 * Todo. 711 */ 712 @Child(name = "subtype", type = { 713 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 714 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 715 protected List<CodeableConcept> subtype; 716 717 /** 718 * Todo. 719 */ 720 @Child(name = "source", type = { 721 DocumentReference.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 722 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 723 protected List<Reference> source; 724 /** 725 * The actual objects that are the target of the reference (Todo.) 726 */ 727 protected List<DocumentReference> sourceTarget; 728 729 private static final long serialVersionUID = -430084579L; 730 731 /** 732 * Constructor 733 */ 734 public SubstanceReferenceInformationClassificationComponent() { 735 super(); 736 } 737 738 /** 739 * @return {@link #domain} (Todo.) 740 */ 741 public CodeableConcept getDomain() { 742 if (this.domain == null) 743 if (Configuration.errorOnAutoCreate()) 744 throw new Error("Attempt to auto-create SubstanceReferenceInformationClassificationComponent.domain"); 745 else if (Configuration.doAutoCreate()) 746 this.domain = new CodeableConcept(); // cc 747 return this.domain; 748 } 749 750 public boolean hasDomain() { 751 return this.domain != null && !this.domain.isEmpty(); 752 } 753 754 /** 755 * @param value {@link #domain} (Todo.) 756 */ 757 public SubstanceReferenceInformationClassificationComponent setDomain(CodeableConcept value) { 758 this.domain = value; 759 return this; 760 } 761 762 /** 763 * @return {@link #classification} (Todo.) 764 */ 765 public CodeableConcept getClassification() { 766 if (this.classification == null) 767 if (Configuration.errorOnAutoCreate()) 768 throw new Error("Attempt to auto-create SubstanceReferenceInformationClassificationComponent.classification"); 769 else if (Configuration.doAutoCreate()) 770 this.classification = new CodeableConcept(); // cc 771 return this.classification; 772 } 773 774 public boolean hasClassification() { 775 return this.classification != null && !this.classification.isEmpty(); 776 } 777 778 /** 779 * @param value {@link #classification} (Todo.) 780 */ 781 public SubstanceReferenceInformationClassificationComponent setClassification(CodeableConcept value) { 782 this.classification = value; 783 return this; 784 } 785 786 /** 787 * @return {@link #subtype} (Todo.) 788 */ 789 public List<CodeableConcept> getSubtype() { 790 if (this.subtype == null) 791 this.subtype = new ArrayList<CodeableConcept>(); 792 return this.subtype; 793 } 794 795 /** 796 * @return Returns a reference to <code>this</code> for easy method chaining 797 */ 798 public SubstanceReferenceInformationClassificationComponent setSubtype(List<CodeableConcept> theSubtype) { 799 this.subtype = theSubtype; 800 return this; 801 } 802 803 public boolean hasSubtype() { 804 if (this.subtype == null) 805 return false; 806 for (CodeableConcept item : this.subtype) 807 if (!item.isEmpty()) 808 return true; 809 return false; 810 } 811 812 public CodeableConcept addSubtype() { // 3 813 CodeableConcept t = new CodeableConcept(); 814 if (this.subtype == null) 815 this.subtype = new ArrayList<CodeableConcept>(); 816 this.subtype.add(t); 817 return t; 818 } 819 820 public SubstanceReferenceInformationClassificationComponent addSubtype(CodeableConcept t) { // 3 821 if (t == null) 822 return this; 823 if (this.subtype == null) 824 this.subtype = new ArrayList<CodeableConcept>(); 825 this.subtype.add(t); 826 return this; 827 } 828 829 /** 830 * @return The first repetition of repeating field {@link #subtype}, creating it 831 * if it does not already exist 832 */ 833 public CodeableConcept getSubtypeFirstRep() { 834 if (getSubtype().isEmpty()) { 835 addSubtype(); 836 } 837 return getSubtype().get(0); 838 } 839 840 /** 841 * @return {@link #source} (Todo.) 842 */ 843 public List<Reference> getSource() { 844 if (this.source == null) 845 this.source = new ArrayList<Reference>(); 846 return this.source; 847 } 848 849 /** 850 * @return Returns a reference to <code>this</code> for easy method chaining 851 */ 852 public SubstanceReferenceInformationClassificationComponent setSource(List<Reference> theSource) { 853 this.source = theSource; 854 return this; 855 } 856 857 public boolean hasSource() { 858 if (this.source == null) 859 return false; 860 for (Reference item : this.source) 861 if (!item.isEmpty()) 862 return true; 863 return false; 864 } 865 866 public Reference addSource() { // 3 867 Reference t = new Reference(); 868 if (this.source == null) 869 this.source = new ArrayList<Reference>(); 870 this.source.add(t); 871 return t; 872 } 873 874 public SubstanceReferenceInformationClassificationComponent addSource(Reference t) { // 3 875 if (t == null) 876 return this; 877 if (this.source == null) 878 this.source = new ArrayList<Reference>(); 879 this.source.add(t); 880 return this; 881 } 882 883 /** 884 * @return The first repetition of repeating field {@link #source}, creating it 885 * if it does not already exist 886 */ 887 public Reference getSourceFirstRep() { 888 if (getSource().isEmpty()) { 889 addSource(); 890 } 891 return getSource().get(0); 892 } 893 894 protected void listChildren(List<Property> children) { 895 super.listChildren(children); 896 children.add(new Property("domain", "CodeableConcept", "Todo.", 0, 1, domain)); 897 children.add(new Property("classification", "CodeableConcept", "Todo.", 0, 1, classification)); 898 children.add(new Property("subtype", "CodeableConcept", "Todo.", 0, java.lang.Integer.MAX_VALUE, subtype)); 899 children 900 .add(new Property("source", "Reference(DocumentReference)", "Todo.", 0, java.lang.Integer.MAX_VALUE, source)); 901 } 902 903 @Override 904 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 905 switch (_hash) { 906 case -1326197564: 907 /* domain */ return new Property("domain", "CodeableConcept", "Todo.", 0, 1, domain); 908 case 382350310: 909 /* classification */ return new Property("classification", "CodeableConcept", "Todo.", 0, 1, classification); 910 case -1867567750: 911 /* subtype */ return new Property("subtype", "CodeableConcept", "Todo.", 0, java.lang.Integer.MAX_VALUE, 912 subtype); 913 case -896505829: 914 /* source */ return new Property("source", "Reference(DocumentReference)", "Todo.", 0, 915 java.lang.Integer.MAX_VALUE, source); 916 default: 917 return super.getNamedProperty(_hash, _name, _checkValid); 918 } 919 920 } 921 922 @Override 923 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 924 switch (hash) { 925 case -1326197564: 926 /* domain */ return this.domain == null ? new Base[0] : new Base[] { this.domain }; // CodeableConcept 927 case 382350310: 928 /* classification */ return this.classification == null ? new Base[0] : new Base[] { this.classification }; // CodeableConcept 929 case -1867567750: 930 /* subtype */ return this.subtype == null ? new Base[0] : this.subtype.toArray(new Base[this.subtype.size()]); // CodeableConcept 931 case -896505829: 932 /* source */ return this.source == null ? new Base[0] : this.source.toArray(new Base[this.source.size()]); // Reference 933 default: 934 return super.getProperty(hash, name, checkValid); 935 } 936 937 } 938 939 @Override 940 public Base setProperty(int hash, String name, Base value) throws FHIRException { 941 switch (hash) { 942 case -1326197564: // domain 943 this.domain = castToCodeableConcept(value); // CodeableConcept 944 return value; 945 case 382350310: // classification 946 this.classification = castToCodeableConcept(value); // CodeableConcept 947 return value; 948 case -1867567750: // subtype 949 this.getSubtype().add(castToCodeableConcept(value)); // CodeableConcept 950 return value; 951 case -896505829: // source 952 this.getSource().add(castToReference(value)); // Reference 953 return value; 954 default: 955 return super.setProperty(hash, name, value); 956 } 957 958 } 959 960 @Override 961 public Base setProperty(String name, Base value) throws FHIRException { 962 if (name.equals("domain")) { 963 this.domain = castToCodeableConcept(value); // CodeableConcept 964 } else if (name.equals("classification")) { 965 this.classification = castToCodeableConcept(value); // CodeableConcept 966 } else if (name.equals("subtype")) { 967 this.getSubtype().add(castToCodeableConcept(value)); 968 } else if (name.equals("source")) { 969 this.getSource().add(castToReference(value)); 970 } else 971 return super.setProperty(name, value); 972 return value; 973 } 974 975 @Override 976 public void removeChild(String name, Base value) throws FHIRException { 977 if (name.equals("domain")) { 978 this.domain = null; 979 } else if (name.equals("classification")) { 980 this.classification = null; 981 } else if (name.equals("subtype")) { 982 this.getSubtype().remove(castToCodeableConcept(value)); 983 } else if (name.equals("source")) { 984 this.getSource().remove(castToReference(value)); 985 } else 986 super.removeChild(name, value); 987 988 } 989 990 @Override 991 public Base makeProperty(int hash, String name) throws FHIRException { 992 switch (hash) { 993 case -1326197564: 994 return getDomain(); 995 case 382350310: 996 return getClassification(); 997 case -1867567750: 998 return addSubtype(); 999 case -896505829: 1000 return addSource(); 1001 default: 1002 return super.makeProperty(hash, name); 1003 } 1004 1005 } 1006 1007 @Override 1008 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1009 switch (hash) { 1010 case -1326197564: 1011 /* domain */ return new String[] { "CodeableConcept" }; 1012 case 382350310: 1013 /* classification */ return new String[] { "CodeableConcept" }; 1014 case -1867567750: 1015 /* subtype */ return new String[] { "CodeableConcept" }; 1016 case -896505829: 1017 /* source */ return new String[] { "Reference" }; 1018 default: 1019 return super.getTypesForProperty(hash, name); 1020 } 1021 1022 } 1023 1024 @Override 1025 public Base addChild(String name) throws FHIRException { 1026 if (name.equals("domain")) { 1027 this.domain = new CodeableConcept(); 1028 return this.domain; 1029 } else if (name.equals("classification")) { 1030 this.classification = new CodeableConcept(); 1031 return this.classification; 1032 } else if (name.equals("subtype")) { 1033 return addSubtype(); 1034 } else if (name.equals("source")) { 1035 return addSource(); 1036 } else 1037 return super.addChild(name); 1038 } 1039 1040 public SubstanceReferenceInformationClassificationComponent copy() { 1041 SubstanceReferenceInformationClassificationComponent dst = new SubstanceReferenceInformationClassificationComponent(); 1042 copyValues(dst); 1043 return dst; 1044 } 1045 1046 public void copyValues(SubstanceReferenceInformationClassificationComponent dst) { 1047 super.copyValues(dst); 1048 dst.domain = domain == null ? null : domain.copy(); 1049 dst.classification = classification == null ? null : classification.copy(); 1050 if (subtype != null) { 1051 dst.subtype = new ArrayList<CodeableConcept>(); 1052 for (CodeableConcept i : subtype) 1053 dst.subtype.add(i.copy()); 1054 } 1055 ; 1056 if (source != null) { 1057 dst.source = new ArrayList<Reference>(); 1058 for (Reference i : source) 1059 dst.source.add(i.copy()); 1060 } 1061 ; 1062 } 1063 1064 @Override 1065 public boolean equalsDeep(Base other_) { 1066 if (!super.equalsDeep(other_)) 1067 return false; 1068 if (!(other_ instanceof SubstanceReferenceInformationClassificationComponent)) 1069 return false; 1070 SubstanceReferenceInformationClassificationComponent o = (SubstanceReferenceInformationClassificationComponent) other_; 1071 return compareDeep(domain, o.domain, true) && compareDeep(classification, o.classification, true) 1072 && compareDeep(subtype, o.subtype, true) && compareDeep(source, o.source, true); 1073 } 1074 1075 @Override 1076 public boolean equalsShallow(Base other_) { 1077 if (!super.equalsShallow(other_)) 1078 return false; 1079 if (!(other_ instanceof SubstanceReferenceInformationClassificationComponent)) 1080 return false; 1081 SubstanceReferenceInformationClassificationComponent o = (SubstanceReferenceInformationClassificationComponent) other_; 1082 return true; 1083 } 1084 1085 public boolean isEmpty() { 1086 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(domain, classification, subtype, source); 1087 } 1088 1089 public String fhirType() { 1090 return "SubstanceReferenceInformation.classification"; 1091 1092 } 1093 1094 } 1095 1096 @Block() 1097 public static class SubstanceReferenceInformationTargetComponent extends BackboneElement 1098 implements IBaseBackboneElement { 1099 /** 1100 * Todo. 1101 */ 1102 @Child(name = "target", type = { Identifier.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1103 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1104 protected Identifier target; 1105 1106 /** 1107 * Todo. 1108 */ 1109 @Child(name = "type", type = { 1110 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1111 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1112 protected CodeableConcept type; 1113 1114 /** 1115 * Todo. 1116 */ 1117 @Child(name = "interaction", type = { 1118 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1119 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1120 protected CodeableConcept interaction; 1121 1122 /** 1123 * Todo. 1124 */ 1125 @Child(name = "organism", type = { 1126 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1127 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1128 protected CodeableConcept organism; 1129 1130 /** 1131 * Todo. 1132 */ 1133 @Child(name = "organismType", type = { 1134 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1135 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1136 protected CodeableConcept organismType; 1137 1138 /** 1139 * Todo. 1140 */ 1141 @Child(name = "amount", type = { Quantity.class, Range.class, 1142 StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1143 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1144 protected Type amount; 1145 1146 /** 1147 * Todo. 1148 */ 1149 @Child(name = "amountType", type = { 1150 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1151 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1152 protected CodeableConcept amountType; 1153 1154 /** 1155 * Todo. 1156 */ 1157 @Child(name = "source", type = { 1158 DocumentReference.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1159 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1160 protected List<Reference> source; 1161 /** 1162 * The actual objects that are the target of the reference (Todo.) 1163 */ 1164 protected List<DocumentReference> sourceTarget; 1165 1166 private static final long serialVersionUID = -1682270197L; 1167 1168 /** 1169 * Constructor 1170 */ 1171 public SubstanceReferenceInformationTargetComponent() { 1172 super(); 1173 } 1174 1175 /** 1176 * @return {@link #target} (Todo.) 1177 */ 1178 public Identifier getTarget() { 1179 if (this.target == null) 1180 if (Configuration.errorOnAutoCreate()) 1181 throw new Error("Attempt to auto-create SubstanceReferenceInformationTargetComponent.target"); 1182 else if (Configuration.doAutoCreate()) 1183 this.target = new Identifier(); // cc 1184 return this.target; 1185 } 1186 1187 public boolean hasTarget() { 1188 return this.target != null && !this.target.isEmpty(); 1189 } 1190 1191 /** 1192 * @param value {@link #target} (Todo.) 1193 */ 1194 public SubstanceReferenceInformationTargetComponent setTarget(Identifier value) { 1195 this.target = value; 1196 return this; 1197 } 1198 1199 /** 1200 * @return {@link #type} (Todo.) 1201 */ 1202 public CodeableConcept getType() { 1203 if (this.type == null) 1204 if (Configuration.errorOnAutoCreate()) 1205 throw new Error("Attempt to auto-create SubstanceReferenceInformationTargetComponent.type"); 1206 else if (Configuration.doAutoCreate()) 1207 this.type = new CodeableConcept(); // cc 1208 return this.type; 1209 } 1210 1211 public boolean hasType() { 1212 return this.type != null && !this.type.isEmpty(); 1213 } 1214 1215 /** 1216 * @param value {@link #type} (Todo.) 1217 */ 1218 public SubstanceReferenceInformationTargetComponent setType(CodeableConcept value) { 1219 this.type = value; 1220 return this; 1221 } 1222 1223 /** 1224 * @return {@link #interaction} (Todo.) 1225 */ 1226 public CodeableConcept getInteraction() { 1227 if (this.interaction == null) 1228 if (Configuration.errorOnAutoCreate()) 1229 throw new Error("Attempt to auto-create SubstanceReferenceInformationTargetComponent.interaction"); 1230 else if (Configuration.doAutoCreate()) 1231 this.interaction = new CodeableConcept(); // cc 1232 return this.interaction; 1233 } 1234 1235 public boolean hasInteraction() { 1236 return this.interaction != null && !this.interaction.isEmpty(); 1237 } 1238 1239 /** 1240 * @param value {@link #interaction} (Todo.) 1241 */ 1242 public SubstanceReferenceInformationTargetComponent setInteraction(CodeableConcept value) { 1243 this.interaction = value; 1244 return this; 1245 } 1246 1247 /** 1248 * @return {@link #organism} (Todo.) 1249 */ 1250 public CodeableConcept getOrganism() { 1251 if (this.organism == null) 1252 if (Configuration.errorOnAutoCreate()) 1253 throw new Error("Attempt to auto-create SubstanceReferenceInformationTargetComponent.organism"); 1254 else if (Configuration.doAutoCreate()) 1255 this.organism = new CodeableConcept(); // cc 1256 return this.organism; 1257 } 1258 1259 public boolean hasOrganism() { 1260 return this.organism != null && !this.organism.isEmpty(); 1261 } 1262 1263 /** 1264 * @param value {@link #organism} (Todo.) 1265 */ 1266 public SubstanceReferenceInformationTargetComponent setOrganism(CodeableConcept value) { 1267 this.organism = value; 1268 return this; 1269 } 1270 1271 /** 1272 * @return {@link #organismType} (Todo.) 1273 */ 1274 public CodeableConcept getOrganismType() { 1275 if (this.organismType == null) 1276 if (Configuration.errorOnAutoCreate()) 1277 throw new Error("Attempt to auto-create SubstanceReferenceInformationTargetComponent.organismType"); 1278 else if (Configuration.doAutoCreate()) 1279 this.organismType = new CodeableConcept(); // cc 1280 return this.organismType; 1281 } 1282 1283 public boolean hasOrganismType() { 1284 return this.organismType != null && !this.organismType.isEmpty(); 1285 } 1286 1287 /** 1288 * @param value {@link #organismType} (Todo.) 1289 */ 1290 public SubstanceReferenceInformationTargetComponent setOrganismType(CodeableConcept value) { 1291 this.organismType = value; 1292 return this; 1293 } 1294 1295 /** 1296 * @return {@link #amount} (Todo.) 1297 */ 1298 public Type getAmount() { 1299 return this.amount; 1300 } 1301 1302 /** 1303 * @return {@link #amount} (Todo.) 1304 */ 1305 public Quantity getAmountQuantity() throws FHIRException { 1306 if (this.amount == null) 1307 this.amount = new Quantity(); 1308 if (!(this.amount instanceof Quantity)) 1309 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.amount.getClass().getName() 1310 + " was encountered"); 1311 return (Quantity) this.amount; 1312 } 1313 1314 public boolean hasAmountQuantity() { 1315 return this.amount instanceof Quantity; 1316 } 1317 1318 /** 1319 * @return {@link #amount} (Todo.) 1320 */ 1321 public Range getAmountRange() throws FHIRException { 1322 if (this.amount == null) 1323 this.amount = new Range(); 1324 if (!(this.amount instanceof Range)) 1325 throw new FHIRException( 1326 "Type mismatch: the type Range was expected, but " + this.amount.getClass().getName() + " was encountered"); 1327 return (Range) this.amount; 1328 } 1329 1330 public boolean hasAmountRange() { 1331 return this.amount instanceof Range; 1332 } 1333 1334 /** 1335 * @return {@link #amount} (Todo.) 1336 */ 1337 public StringType getAmountStringType() throws FHIRException { 1338 if (this.amount == null) 1339 this.amount = new StringType(); 1340 if (!(this.amount instanceof StringType)) 1341 throw new FHIRException("Type mismatch: the type StringType was expected, but " 1342 + this.amount.getClass().getName() + " was encountered"); 1343 return (StringType) this.amount; 1344 } 1345 1346 public boolean hasAmountStringType() { 1347 return this.amount instanceof StringType; 1348 } 1349 1350 public boolean hasAmount() { 1351 return this.amount != null && !this.amount.isEmpty(); 1352 } 1353 1354 /** 1355 * @param value {@link #amount} (Todo.) 1356 */ 1357 public SubstanceReferenceInformationTargetComponent setAmount(Type value) { 1358 if (value != null && !(value instanceof Quantity || value instanceof Range || value instanceof StringType)) 1359 throw new Error("Not the right type for SubstanceReferenceInformation.target.amount[x]: " + value.fhirType()); 1360 this.amount = value; 1361 return this; 1362 } 1363 1364 /** 1365 * @return {@link #amountType} (Todo.) 1366 */ 1367 public CodeableConcept getAmountType() { 1368 if (this.amountType == null) 1369 if (Configuration.errorOnAutoCreate()) 1370 throw new Error("Attempt to auto-create SubstanceReferenceInformationTargetComponent.amountType"); 1371 else if (Configuration.doAutoCreate()) 1372 this.amountType = new CodeableConcept(); // cc 1373 return this.amountType; 1374 } 1375 1376 public boolean hasAmountType() { 1377 return this.amountType != null && !this.amountType.isEmpty(); 1378 } 1379 1380 /** 1381 * @param value {@link #amountType} (Todo.) 1382 */ 1383 public SubstanceReferenceInformationTargetComponent setAmountType(CodeableConcept value) { 1384 this.amountType = value; 1385 return this; 1386 } 1387 1388 /** 1389 * @return {@link #source} (Todo.) 1390 */ 1391 public List<Reference> getSource() { 1392 if (this.source == null) 1393 this.source = new ArrayList<Reference>(); 1394 return this.source; 1395 } 1396 1397 /** 1398 * @return Returns a reference to <code>this</code> for easy method chaining 1399 */ 1400 public SubstanceReferenceInformationTargetComponent setSource(List<Reference> theSource) { 1401 this.source = theSource; 1402 return this; 1403 } 1404 1405 public boolean hasSource() { 1406 if (this.source == null) 1407 return false; 1408 for (Reference item : this.source) 1409 if (!item.isEmpty()) 1410 return true; 1411 return false; 1412 } 1413 1414 public Reference addSource() { // 3 1415 Reference t = new Reference(); 1416 if (this.source == null) 1417 this.source = new ArrayList<Reference>(); 1418 this.source.add(t); 1419 return t; 1420 } 1421 1422 public SubstanceReferenceInformationTargetComponent addSource(Reference t) { // 3 1423 if (t == null) 1424 return this; 1425 if (this.source == null) 1426 this.source = new ArrayList<Reference>(); 1427 this.source.add(t); 1428 return this; 1429 } 1430 1431 /** 1432 * @return The first repetition of repeating field {@link #source}, creating it 1433 * if it does not already exist 1434 */ 1435 public Reference getSourceFirstRep() { 1436 if (getSource().isEmpty()) { 1437 addSource(); 1438 } 1439 return getSource().get(0); 1440 } 1441 1442 protected void listChildren(List<Property> children) { 1443 super.listChildren(children); 1444 children.add(new Property("target", "Identifier", "Todo.", 0, 1, target)); 1445 children.add(new Property("type", "CodeableConcept", "Todo.", 0, 1, type)); 1446 children.add(new Property("interaction", "CodeableConcept", "Todo.", 0, 1, interaction)); 1447 children.add(new Property("organism", "CodeableConcept", "Todo.", 0, 1, organism)); 1448 children.add(new Property("organismType", "CodeableConcept", "Todo.", 0, 1, organismType)); 1449 children.add(new Property("amount[x]", "Quantity|Range|string", "Todo.", 0, 1, amount)); 1450 children.add(new Property("amountType", "CodeableConcept", "Todo.", 0, 1, amountType)); 1451 children 1452 .add(new Property("source", "Reference(DocumentReference)", "Todo.", 0, java.lang.Integer.MAX_VALUE, source)); 1453 } 1454 1455 @Override 1456 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1457 switch (_hash) { 1458 case -880905839: 1459 /* target */ return new Property("target", "Identifier", "Todo.", 0, 1, target); 1460 case 3575610: 1461 /* type */ return new Property("type", "CodeableConcept", "Todo.", 0, 1, type); 1462 case 1844104722: 1463 /* interaction */ return new Property("interaction", "CodeableConcept", "Todo.", 0, 1, interaction); 1464 case 1316389074: 1465 /* organism */ return new Property("organism", "CodeableConcept", "Todo.", 0, 1, organism); 1466 case 988662572: 1467 /* organismType */ return new Property("organismType", "CodeableConcept", "Todo.", 0, 1, organismType); 1468 case 646780200: 1469 /* amount[x] */ return new Property("amount[x]", "Quantity|Range|string", "Todo.", 0, 1, amount); 1470 case -1413853096: 1471 /* amount */ return new Property("amount[x]", "Quantity|Range|string", "Todo.", 0, 1, amount); 1472 case 1664303363: 1473 /* amountQuantity */ return new Property("amount[x]", "Quantity|Range|string", "Todo.", 0, 1, amount); 1474 case -1223462971: 1475 /* amountRange */ return new Property("amount[x]", "Quantity|Range|string", "Todo.", 0, 1, amount); 1476 case 773651081: 1477 /* amountString */ return new Property("amount[x]", "Quantity|Range|string", "Todo.", 0, 1, amount); 1478 case -1424857166: 1479 /* amountType */ return new Property("amountType", "CodeableConcept", "Todo.", 0, 1, amountType); 1480 case -896505829: 1481 /* source */ return new Property("source", "Reference(DocumentReference)", "Todo.", 0, 1482 java.lang.Integer.MAX_VALUE, source); 1483 default: 1484 return super.getNamedProperty(_hash, _name, _checkValid); 1485 } 1486 1487 } 1488 1489 @Override 1490 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1491 switch (hash) { 1492 case -880905839: 1493 /* target */ return this.target == null ? new Base[0] : new Base[] { this.target }; // Identifier 1494 case 3575610: 1495 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1496 case 1844104722: 1497 /* interaction */ return this.interaction == null ? new Base[0] : new Base[] { this.interaction }; // CodeableConcept 1498 case 1316389074: 1499 /* organism */ return this.organism == null ? new Base[0] : new Base[] { this.organism }; // CodeableConcept 1500 case 988662572: 1501 /* organismType */ return this.organismType == null ? new Base[0] : new Base[] { this.organismType }; // CodeableConcept 1502 case -1413853096: 1503 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // Type 1504 case -1424857166: 1505 /* amountType */ return this.amountType == null ? new Base[0] : new Base[] { this.amountType }; // CodeableConcept 1506 case -896505829: 1507 /* source */ return this.source == null ? new Base[0] : this.source.toArray(new Base[this.source.size()]); // Reference 1508 default: 1509 return super.getProperty(hash, name, checkValid); 1510 } 1511 1512 } 1513 1514 @Override 1515 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1516 switch (hash) { 1517 case -880905839: // target 1518 this.target = castToIdentifier(value); // Identifier 1519 return value; 1520 case 3575610: // type 1521 this.type = castToCodeableConcept(value); // CodeableConcept 1522 return value; 1523 case 1844104722: // interaction 1524 this.interaction = castToCodeableConcept(value); // CodeableConcept 1525 return value; 1526 case 1316389074: // organism 1527 this.organism = castToCodeableConcept(value); // CodeableConcept 1528 return value; 1529 case 988662572: // organismType 1530 this.organismType = castToCodeableConcept(value); // CodeableConcept 1531 return value; 1532 case -1413853096: // amount 1533 this.amount = castToType(value); // Type 1534 return value; 1535 case -1424857166: // amountType 1536 this.amountType = castToCodeableConcept(value); // CodeableConcept 1537 return value; 1538 case -896505829: // source 1539 this.getSource().add(castToReference(value)); // Reference 1540 return value; 1541 default: 1542 return super.setProperty(hash, name, value); 1543 } 1544 1545 } 1546 1547 @Override 1548 public Base setProperty(String name, Base value) throws FHIRException { 1549 if (name.equals("target")) { 1550 this.target = castToIdentifier(value); // Identifier 1551 } else if (name.equals("type")) { 1552 this.type = castToCodeableConcept(value); // CodeableConcept 1553 } else if (name.equals("interaction")) { 1554 this.interaction = castToCodeableConcept(value); // CodeableConcept 1555 } else if (name.equals("organism")) { 1556 this.organism = castToCodeableConcept(value); // CodeableConcept 1557 } else if (name.equals("organismType")) { 1558 this.organismType = castToCodeableConcept(value); // CodeableConcept 1559 } else if (name.equals("amount[x]")) { 1560 this.amount = castToType(value); // Type 1561 } else if (name.equals("amountType")) { 1562 this.amountType = castToCodeableConcept(value); // CodeableConcept 1563 } else if (name.equals("source")) { 1564 this.getSource().add(castToReference(value)); 1565 } else 1566 return super.setProperty(name, value); 1567 return value; 1568 } 1569 1570 @Override 1571 public void removeChild(String name, Base value) throws FHIRException { 1572 if (name.equals("target")) { 1573 this.target = null; 1574 } else if (name.equals("type")) { 1575 this.type = null; 1576 } else if (name.equals("interaction")) { 1577 this.interaction = null; 1578 } else if (name.equals("organism")) { 1579 this.organism = null; 1580 } else if (name.equals("organismType")) { 1581 this.organismType = null; 1582 } else if (name.equals("amount[x]")) { 1583 this.amount = null; 1584 } else if (name.equals("amountType")) { 1585 this.amountType = null; 1586 } else if (name.equals("source")) { 1587 this.getSource().remove(castToReference(value)); 1588 } else 1589 super.removeChild(name, value); 1590 1591 } 1592 1593 @Override 1594 public Base makeProperty(int hash, String name) throws FHIRException { 1595 switch (hash) { 1596 case -880905839: 1597 return getTarget(); 1598 case 3575610: 1599 return getType(); 1600 case 1844104722: 1601 return getInteraction(); 1602 case 1316389074: 1603 return getOrganism(); 1604 case 988662572: 1605 return getOrganismType(); 1606 case 646780200: 1607 return getAmount(); 1608 case -1413853096: 1609 return getAmount(); 1610 case -1424857166: 1611 return getAmountType(); 1612 case -896505829: 1613 return addSource(); 1614 default: 1615 return super.makeProperty(hash, name); 1616 } 1617 1618 } 1619 1620 @Override 1621 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1622 switch (hash) { 1623 case -880905839: 1624 /* target */ return new String[] { "Identifier" }; 1625 case 3575610: 1626 /* type */ return new String[] { "CodeableConcept" }; 1627 case 1844104722: 1628 /* interaction */ return new String[] { "CodeableConcept" }; 1629 case 1316389074: 1630 /* organism */ return new String[] { "CodeableConcept" }; 1631 case 988662572: 1632 /* organismType */ return new String[] { "CodeableConcept" }; 1633 case -1413853096: 1634 /* amount */ return new String[] { "Quantity", "Range", "string" }; 1635 case -1424857166: 1636 /* amountType */ return new String[] { "CodeableConcept" }; 1637 case -896505829: 1638 /* source */ return new String[] { "Reference" }; 1639 default: 1640 return super.getTypesForProperty(hash, name); 1641 } 1642 1643 } 1644 1645 @Override 1646 public Base addChild(String name) throws FHIRException { 1647 if (name.equals("target")) { 1648 this.target = new Identifier(); 1649 return this.target; 1650 } else if (name.equals("type")) { 1651 this.type = new CodeableConcept(); 1652 return this.type; 1653 } else if (name.equals("interaction")) { 1654 this.interaction = new CodeableConcept(); 1655 return this.interaction; 1656 } else if (name.equals("organism")) { 1657 this.organism = new CodeableConcept(); 1658 return this.organism; 1659 } else if (name.equals("organismType")) { 1660 this.organismType = new CodeableConcept(); 1661 return this.organismType; 1662 } else if (name.equals("amountQuantity")) { 1663 this.amount = new Quantity(); 1664 return this.amount; 1665 } else if (name.equals("amountRange")) { 1666 this.amount = new Range(); 1667 return this.amount; 1668 } else if (name.equals("amountString")) { 1669 this.amount = new StringType(); 1670 return this.amount; 1671 } else if (name.equals("amountType")) { 1672 this.amountType = new CodeableConcept(); 1673 return this.amountType; 1674 } else if (name.equals("source")) { 1675 return addSource(); 1676 } else 1677 return super.addChild(name); 1678 } 1679 1680 public SubstanceReferenceInformationTargetComponent copy() { 1681 SubstanceReferenceInformationTargetComponent dst = new SubstanceReferenceInformationTargetComponent(); 1682 copyValues(dst); 1683 return dst; 1684 } 1685 1686 public void copyValues(SubstanceReferenceInformationTargetComponent dst) { 1687 super.copyValues(dst); 1688 dst.target = target == null ? null : target.copy(); 1689 dst.type = type == null ? null : type.copy(); 1690 dst.interaction = interaction == null ? null : interaction.copy(); 1691 dst.organism = organism == null ? null : organism.copy(); 1692 dst.organismType = organismType == null ? null : organismType.copy(); 1693 dst.amount = amount == null ? null : amount.copy(); 1694 dst.amountType = amountType == null ? null : amountType.copy(); 1695 if (source != null) { 1696 dst.source = new ArrayList<Reference>(); 1697 for (Reference i : source) 1698 dst.source.add(i.copy()); 1699 } 1700 ; 1701 } 1702 1703 @Override 1704 public boolean equalsDeep(Base other_) { 1705 if (!super.equalsDeep(other_)) 1706 return false; 1707 if (!(other_ instanceof SubstanceReferenceInformationTargetComponent)) 1708 return false; 1709 SubstanceReferenceInformationTargetComponent o = (SubstanceReferenceInformationTargetComponent) other_; 1710 return compareDeep(target, o.target, true) && compareDeep(type, o.type, true) 1711 && compareDeep(interaction, o.interaction, true) && compareDeep(organism, o.organism, true) 1712 && compareDeep(organismType, o.organismType, true) && compareDeep(amount, o.amount, true) 1713 && compareDeep(amountType, o.amountType, true) && compareDeep(source, o.source, true); 1714 } 1715 1716 @Override 1717 public boolean equalsShallow(Base other_) { 1718 if (!super.equalsShallow(other_)) 1719 return false; 1720 if (!(other_ instanceof SubstanceReferenceInformationTargetComponent)) 1721 return false; 1722 SubstanceReferenceInformationTargetComponent o = (SubstanceReferenceInformationTargetComponent) other_; 1723 return true; 1724 } 1725 1726 public boolean isEmpty() { 1727 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(target, type, interaction, organism, organismType, 1728 amount, amountType, source); 1729 } 1730 1731 public String fhirType() { 1732 return "SubstanceReferenceInformation.target"; 1733 1734 } 1735 1736 } 1737 1738 /** 1739 * Todo. 1740 */ 1741 @Child(name = "comment", type = { StringType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 1742 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1743 protected StringType comment; 1744 1745 /** 1746 * Todo. 1747 */ 1748 @Child(name = "gene", type = {}, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1749 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1750 protected List<SubstanceReferenceInformationGeneComponent> gene; 1751 1752 /** 1753 * Todo. 1754 */ 1755 @Child(name = "geneElement", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1756 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1757 protected List<SubstanceReferenceInformationGeneElementComponent> geneElement; 1758 1759 /** 1760 * Todo. 1761 */ 1762 @Child(name = "classification", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1763 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1764 protected List<SubstanceReferenceInformationClassificationComponent> classification; 1765 1766 /** 1767 * Todo. 1768 */ 1769 @Child(name = "target", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1770 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1771 protected List<SubstanceReferenceInformationTargetComponent> target; 1772 1773 private static final long serialVersionUID = 890303332L; 1774 1775 /** 1776 * Constructor 1777 */ 1778 public SubstanceReferenceInformation() { 1779 super(); 1780 } 1781 1782 /** 1783 * @return {@link #comment} (Todo.). This is the underlying object with id, 1784 * value and extensions. The accessor "getComment" gives direct access 1785 * to the value 1786 */ 1787 public StringType getCommentElement() { 1788 if (this.comment == null) 1789 if (Configuration.errorOnAutoCreate()) 1790 throw new Error("Attempt to auto-create SubstanceReferenceInformation.comment"); 1791 else if (Configuration.doAutoCreate()) 1792 this.comment = new StringType(); // bb 1793 return this.comment; 1794 } 1795 1796 public boolean hasCommentElement() { 1797 return this.comment != null && !this.comment.isEmpty(); 1798 } 1799 1800 public boolean hasComment() { 1801 return this.comment != null && !this.comment.isEmpty(); 1802 } 1803 1804 /** 1805 * @param value {@link #comment} (Todo.). This is the underlying object with id, 1806 * value and extensions. The accessor "getComment" gives direct 1807 * access to the value 1808 */ 1809 public SubstanceReferenceInformation setCommentElement(StringType value) { 1810 this.comment = value; 1811 return this; 1812 } 1813 1814 /** 1815 * @return Todo. 1816 */ 1817 public String getComment() { 1818 return this.comment == null ? null : this.comment.getValue(); 1819 } 1820 1821 /** 1822 * @param value Todo. 1823 */ 1824 public SubstanceReferenceInformation setComment(String value) { 1825 if (Utilities.noString(value)) 1826 this.comment = null; 1827 else { 1828 if (this.comment == null) 1829 this.comment = new StringType(); 1830 this.comment.setValue(value); 1831 } 1832 return this; 1833 } 1834 1835 /** 1836 * @return {@link #gene} (Todo.) 1837 */ 1838 public List<SubstanceReferenceInformationGeneComponent> getGene() { 1839 if (this.gene == null) 1840 this.gene = new ArrayList<SubstanceReferenceInformationGeneComponent>(); 1841 return this.gene; 1842 } 1843 1844 /** 1845 * @return Returns a reference to <code>this</code> for easy method chaining 1846 */ 1847 public SubstanceReferenceInformation setGene(List<SubstanceReferenceInformationGeneComponent> theGene) { 1848 this.gene = theGene; 1849 return this; 1850 } 1851 1852 public boolean hasGene() { 1853 if (this.gene == null) 1854 return false; 1855 for (SubstanceReferenceInformationGeneComponent item : this.gene) 1856 if (!item.isEmpty()) 1857 return true; 1858 return false; 1859 } 1860 1861 public SubstanceReferenceInformationGeneComponent addGene() { // 3 1862 SubstanceReferenceInformationGeneComponent t = new SubstanceReferenceInformationGeneComponent(); 1863 if (this.gene == null) 1864 this.gene = new ArrayList<SubstanceReferenceInformationGeneComponent>(); 1865 this.gene.add(t); 1866 return t; 1867 } 1868 1869 public SubstanceReferenceInformation addGene(SubstanceReferenceInformationGeneComponent t) { // 3 1870 if (t == null) 1871 return this; 1872 if (this.gene == null) 1873 this.gene = new ArrayList<SubstanceReferenceInformationGeneComponent>(); 1874 this.gene.add(t); 1875 return this; 1876 } 1877 1878 /** 1879 * @return The first repetition of repeating field {@link #gene}, creating it if 1880 * it does not already exist 1881 */ 1882 public SubstanceReferenceInformationGeneComponent getGeneFirstRep() { 1883 if (getGene().isEmpty()) { 1884 addGene(); 1885 } 1886 return getGene().get(0); 1887 } 1888 1889 /** 1890 * @return {@link #geneElement} (Todo.) 1891 */ 1892 public List<SubstanceReferenceInformationGeneElementComponent> getGeneElement() { 1893 if (this.geneElement == null) 1894 this.geneElement = new ArrayList<SubstanceReferenceInformationGeneElementComponent>(); 1895 return this.geneElement; 1896 } 1897 1898 /** 1899 * @return Returns a reference to <code>this</code> for easy method chaining 1900 */ 1901 public SubstanceReferenceInformation setGeneElement( 1902 List<SubstanceReferenceInformationGeneElementComponent> theGeneElement) { 1903 this.geneElement = theGeneElement; 1904 return this; 1905 } 1906 1907 public boolean hasGeneElement() { 1908 if (this.geneElement == null) 1909 return false; 1910 for (SubstanceReferenceInformationGeneElementComponent item : this.geneElement) 1911 if (!item.isEmpty()) 1912 return true; 1913 return false; 1914 } 1915 1916 public SubstanceReferenceInformationGeneElementComponent addGeneElement() { // 3 1917 SubstanceReferenceInformationGeneElementComponent t = new SubstanceReferenceInformationGeneElementComponent(); 1918 if (this.geneElement == null) 1919 this.geneElement = new ArrayList<SubstanceReferenceInformationGeneElementComponent>(); 1920 this.geneElement.add(t); 1921 return t; 1922 } 1923 1924 public SubstanceReferenceInformation addGeneElement(SubstanceReferenceInformationGeneElementComponent t) { // 3 1925 if (t == null) 1926 return this; 1927 if (this.geneElement == null) 1928 this.geneElement = new ArrayList<SubstanceReferenceInformationGeneElementComponent>(); 1929 this.geneElement.add(t); 1930 return this; 1931 } 1932 1933 /** 1934 * @return The first repetition of repeating field {@link #geneElement}, 1935 * creating it if it does not already exist 1936 */ 1937 public SubstanceReferenceInformationGeneElementComponent getGeneElementFirstRep() { 1938 if (getGeneElement().isEmpty()) { 1939 addGeneElement(); 1940 } 1941 return getGeneElement().get(0); 1942 } 1943 1944 /** 1945 * @return {@link #classification} (Todo.) 1946 */ 1947 public List<SubstanceReferenceInformationClassificationComponent> getClassification() { 1948 if (this.classification == null) 1949 this.classification = new ArrayList<SubstanceReferenceInformationClassificationComponent>(); 1950 return this.classification; 1951 } 1952 1953 /** 1954 * @return Returns a reference to <code>this</code> for easy method chaining 1955 */ 1956 public SubstanceReferenceInformation setClassification( 1957 List<SubstanceReferenceInformationClassificationComponent> theClassification) { 1958 this.classification = theClassification; 1959 return this; 1960 } 1961 1962 public boolean hasClassification() { 1963 if (this.classification == null) 1964 return false; 1965 for (SubstanceReferenceInformationClassificationComponent item : this.classification) 1966 if (!item.isEmpty()) 1967 return true; 1968 return false; 1969 } 1970 1971 public SubstanceReferenceInformationClassificationComponent addClassification() { // 3 1972 SubstanceReferenceInformationClassificationComponent t = new SubstanceReferenceInformationClassificationComponent(); 1973 if (this.classification == null) 1974 this.classification = new ArrayList<SubstanceReferenceInformationClassificationComponent>(); 1975 this.classification.add(t); 1976 return t; 1977 } 1978 1979 public SubstanceReferenceInformation addClassification(SubstanceReferenceInformationClassificationComponent t) { // 3 1980 if (t == null) 1981 return this; 1982 if (this.classification == null) 1983 this.classification = new ArrayList<SubstanceReferenceInformationClassificationComponent>(); 1984 this.classification.add(t); 1985 return this; 1986 } 1987 1988 /** 1989 * @return The first repetition of repeating field {@link #classification}, 1990 * creating it if it does not already exist 1991 */ 1992 public SubstanceReferenceInformationClassificationComponent getClassificationFirstRep() { 1993 if (getClassification().isEmpty()) { 1994 addClassification(); 1995 } 1996 return getClassification().get(0); 1997 } 1998 1999 /** 2000 * @return {@link #target} (Todo.) 2001 */ 2002 public List<SubstanceReferenceInformationTargetComponent> getTarget() { 2003 if (this.target == null) 2004 this.target = new ArrayList<SubstanceReferenceInformationTargetComponent>(); 2005 return this.target; 2006 } 2007 2008 /** 2009 * @return Returns a reference to <code>this</code> for easy method chaining 2010 */ 2011 public SubstanceReferenceInformation setTarget(List<SubstanceReferenceInformationTargetComponent> theTarget) { 2012 this.target = theTarget; 2013 return this; 2014 } 2015 2016 public boolean hasTarget() { 2017 if (this.target == null) 2018 return false; 2019 for (SubstanceReferenceInformationTargetComponent item : this.target) 2020 if (!item.isEmpty()) 2021 return true; 2022 return false; 2023 } 2024 2025 public SubstanceReferenceInformationTargetComponent addTarget() { // 3 2026 SubstanceReferenceInformationTargetComponent t = new SubstanceReferenceInformationTargetComponent(); 2027 if (this.target == null) 2028 this.target = new ArrayList<SubstanceReferenceInformationTargetComponent>(); 2029 this.target.add(t); 2030 return t; 2031 } 2032 2033 public SubstanceReferenceInformation addTarget(SubstanceReferenceInformationTargetComponent t) { // 3 2034 if (t == null) 2035 return this; 2036 if (this.target == null) 2037 this.target = new ArrayList<SubstanceReferenceInformationTargetComponent>(); 2038 this.target.add(t); 2039 return this; 2040 } 2041 2042 /** 2043 * @return The first repetition of repeating field {@link #target}, creating it 2044 * if it does not already exist 2045 */ 2046 public SubstanceReferenceInformationTargetComponent getTargetFirstRep() { 2047 if (getTarget().isEmpty()) { 2048 addTarget(); 2049 } 2050 return getTarget().get(0); 2051 } 2052 2053 protected void listChildren(List<Property> children) { 2054 super.listChildren(children); 2055 children.add(new Property("comment", "string", "Todo.", 0, 1, comment)); 2056 children.add(new Property("gene", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, gene)); 2057 children.add(new Property("geneElement", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, geneElement)); 2058 children.add(new Property("classification", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, classification)); 2059 children.add(new Property("target", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, target)); 2060 } 2061 2062 @Override 2063 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2064 switch (_hash) { 2065 case 950398559: 2066 /* comment */ return new Property("comment", "string", "Todo.", 0, 1, comment); 2067 case 3169045: 2068 /* gene */ return new Property("gene", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, gene); 2069 case -94918105: 2070 /* geneElement */ return new Property("geneElement", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, geneElement); 2071 case 382350310: 2072 /* classification */ return new Property("classification", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, 2073 classification); 2074 case -880905839: 2075 /* target */ return new Property("target", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, target); 2076 default: 2077 return super.getNamedProperty(_hash, _name, _checkValid); 2078 } 2079 2080 } 2081 2082 @Override 2083 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2084 switch (hash) { 2085 case 950398559: 2086 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // StringType 2087 case 3169045: 2088 /* gene */ return this.gene == null ? new Base[0] : this.gene.toArray(new Base[this.gene.size()]); // SubstanceReferenceInformationGeneComponent 2089 case -94918105: 2090 /* geneElement */ return this.geneElement == null ? new Base[0] 2091 : this.geneElement.toArray(new Base[this.geneElement.size()]); // SubstanceReferenceInformationGeneElementComponent 2092 case 382350310: 2093 /* classification */ return this.classification == null ? new Base[0] 2094 : this.classification.toArray(new Base[this.classification.size()]); // SubstanceReferenceInformationClassificationComponent 2095 case -880905839: 2096 /* target */ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // SubstanceReferenceInformationTargetComponent 2097 default: 2098 return super.getProperty(hash, name, checkValid); 2099 } 2100 2101 } 2102 2103 @Override 2104 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2105 switch (hash) { 2106 case 950398559: // comment 2107 this.comment = castToString(value); // StringType 2108 return value; 2109 case 3169045: // gene 2110 this.getGene().add((SubstanceReferenceInformationGeneComponent) value); // SubstanceReferenceInformationGeneComponent 2111 return value; 2112 case -94918105: // geneElement 2113 this.getGeneElement().add((SubstanceReferenceInformationGeneElementComponent) value); // SubstanceReferenceInformationGeneElementComponent 2114 return value; 2115 case 382350310: // classification 2116 this.getClassification().add((SubstanceReferenceInformationClassificationComponent) value); // SubstanceReferenceInformationClassificationComponent 2117 return value; 2118 case -880905839: // target 2119 this.getTarget().add((SubstanceReferenceInformationTargetComponent) value); // SubstanceReferenceInformationTargetComponent 2120 return value; 2121 default: 2122 return super.setProperty(hash, name, value); 2123 } 2124 2125 } 2126 2127 @Override 2128 public Base setProperty(String name, Base value) throws FHIRException { 2129 if (name.equals("comment")) { 2130 this.comment = castToString(value); // StringType 2131 } else if (name.equals("gene")) { 2132 this.getGene().add((SubstanceReferenceInformationGeneComponent) value); 2133 } else if (name.equals("geneElement")) { 2134 this.getGeneElement().add((SubstanceReferenceInformationGeneElementComponent) value); 2135 } else if (name.equals("classification")) { 2136 this.getClassification().add((SubstanceReferenceInformationClassificationComponent) value); 2137 } else if (name.equals("target")) { 2138 this.getTarget().add((SubstanceReferenceInformationTargetComponent) value); 2139 } else 2140 return super.setProperty(name, value); 2141 return value; 2142 } 2143 2144 @Override 2145 public void removeChild(String name, Base value) throws FHIRException { 2146 if (name.equals("comment")) { 2147 this.comment = null; 2148 } else if (name.equals("gene")) { 2149 this.getGene().remove((SubstanceReferenceInformationGeneComponent) value); 2150 } else if (name.equals("geneElement")) { 2151 this.getGeneElement().remove((SubstanceReferenceInformationGeneElementComponent) value); 2152 } else if (name.equals("classification")) { 2153 this.getClassification().remove((SubstanceReferenceInformationClassificationComponent) value); 2154 } else if (name.equals("target")) { 2155 this.getTarget().remove((SubstanceReferenceInformationTargetComponent) value); 2156 } else 2157 super.removeChild(name, value); 2158 2159 } 2160 2161 @Override 2162 public Base makeProperty(int hash, String name) throws FHIRException { 2163 switch (hash) { 2164 case 950398559: 2165 return getCommentElement(); 2166 case 3169045: 2167 return addGene(); 2168 case -94918105: 2169 return addGeneElement(); 2170 case 382350310: 2171 return addClassification(); 2172 case -880905839: 2173 return addTarget(); 2174 default: 2175 return super.makeProperty(hash, name); 2176 } 2177 2178 } 2179 2180 @Override 2181 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2182 switch (hash) { 2183 case 950398559: 2184 /* comment */ return new String[] { "string" }; 2185 case 3169045: 2186 /* gene */ return new String[] {}; 2187 case -94918105: 2188 /* geneElement */ return new String[] {}; 2189 case 382350310: 2190 /* classification */ return new String[] {}; 2191 case -880905839: 2192 /* target */ return new String[] {}; 2193 default: 2194 return super.getTypesForProperty(hash, name); 2195 } 2196 2197 } 2198 2199 @Override 2200 public Base addChild(String name) throws FHIRException { 2201 if (name.equals("comment")) { 2202 throw new FHIRException("Cannot call addChild on a singleton property SubstanceReferenceInformation.comment"); 2203 } else if (name.equals("gene")) { 2204 return addGene(); 2205 } else if (name.equals("geneElement")) { 2206 return addGeneElement(); 2207 } else if (name.equals("classification")) { 2208 return addClassification(); 2209 } else if (name.equals("target")) { 2210 return addTarget(); 2211 } else 2212 return super.addChild(name); 2213 } 2214 2215 public String fhirType() { 2216 return "SubstanceReferenceInformation"; 2217 2218 } 2219 2220 public SubstanceReferenceInformation copy() { 2221 SubstanceReferenceInformation dst = new SubstanceReferenceInformation(); 2222 copyValues(dst); 2223 return dst; 2224 } 2225 2226 public void copyValues(SubstanceReferenceInformation dst) { 2227 super.copyValues(dst); 2228 dst.comment = comment == null ? null : comment.copy(); 2229 if (gene != null) { 2230 dst.gene = new ArrayList<SubstanceReferenceInformationGeneComponent>(); 2231 for (SubstanceReferenceInformationGeneComponent i : gene) 2232 dst.gene.add(i.copy()); 2233 } 2234 ; 2235 if (geneElement != null) { 2236 dst.geneElement = new ArrayList<SubstanceReferenceInformationGeneElementComponent>(); 2237 for (SubstanceReferenceInformationGeneElementComponent i : geneElement) 2238 dst.geneElement.add(i.copy()); 2239 } 2240 ; 2241 if (classification != null) { 2242 dst.classification = new ArrayList<SubstanceReferenceInformationClassificationComponent>(); 2243 for (SubstanceReferenceInformationClassificationComponent i : classification) 2244 dst.classification.add(i.copy()); 2245 } 2246 ; 2247 if (target != null) { 2248 dst.target = new ArrayList<SubstanceReferenceInformationTargetComponent>(); 2249 for (SubstanceReferenceInformationTargetComponent i : target) 2250 dst.target.add(i.copy()); 2251 } 2252 ; 2253 } 2254 2255 protected SubstanceReferenceInformation typedCopy() { 2256 return copy(); 2257 } 2258 2259 @Override 2260 public boolean equalsDeep(Base other_) { 2261 if (!super.equalsDeep(other_)) 2262 return false; 2263 if (!(other_ instanceof SubstanceReferenceInformation)) 2264 return false; 2265 SubstanceReferenceInformation o = (SubstanceReferenceInformation) other_; 2266 return compareDeep(comment, o.comment, true) && compareDeep(gene, o.gene, true) 2267 && compareDeep(geneElement, o.geneElement, true) && compareDeep(classification, o.classification, true) 2268 && compareDeep(target, o.target, true); 2269 } 2270 2271 @Override 2272 public boolean equalsShallow(Base other_) { 2273 if (!super.equalsShallow(other_)) 2274 return false; 2275 if (!(other_ instanceof SubstanceReferenceInformation)) 2276 return false; 2277 SubstanceReferenceInformation o = (SubstanceReferenceInformation) other_; 2278 return compareValues(comment, o.comment, true); 2279 } 2280 2281 public boolean isEmpty() { 2282 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(comment, gene, geneElement, classification, target); 2283 } 2284 2285 @Override 2286 public ResourceType getResourceType() { 2287 return ResourceType.SubstanceReferenceInformation; 2288 } 2289 2290}