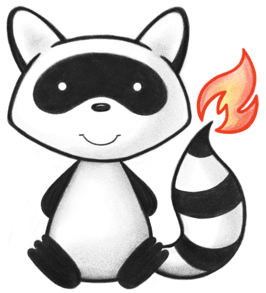
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044 045/** 046 * Record of delivery of what is supplied. 047 */ 048@ResourceDef(name = "SupplyDelivery", profile = "http://hl7.org/fhir/StructureDefinition/SupplyDelivery") 049public class SupplyDelivery extends DomainResource { 050 051 public enum SupplyDeliveryStatus { 052 /** 053 * Supply has been requested, but not delivered. 054 */ 055 INPROGRESS, 056 /** 057 * Supply has been delivered ("completed"). 058 */ 059 COMPLETED, 060 /** 061 * Delivery was not completed. 062 */ 063 ABANDONED, 064 /** 065 * This electronic record should never have existed, though it is possible that 066 * real-world decisions were based on it. (If real-world activity has occurred, 067 * the status should be "abandoned" rather than "entered-in-error".). 068 */ 069 ENTEREDINERROR, 070 /** 071 * added to help the parsers with the generic types 072 */ 073 NULL; 074 075 public static SupplyDeliveryStatus fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("in-progress".equals(codeString)) 079 return INPROGRESS; 080 if ("completed".equals(codeString)) 081 return COMPLETED; 082 if ("abandoned".equals(codeString)) 083 return ABANDONED; 084 if ("entered-in-error".equals(codeString)) 085 return ENTEREDINERROR; 086 if (Configuration.isAcceptInvalidEnums()) 087 return null; 088 else 089 throw new FHIRException("Unknown SupplyDeliveryStatus code '" + codeString + "'"); 090 } 091 092 public String toCode() { 093 switch (this) { 094 case INPROGRESS: 095 return "in-progress"; 096 case COMPLETED: 097 return "completed"; 098 case ABANDONED: 099 return "abandoned"; 100 case ENTEREDINERROR: 101 return "entered-in-error"; 102 case NULL: 103 return null; 104 default: 105 return "?"; 106 } 107 } 108 109 public String getSystem() { 110 switch (this) { 111 case INPROGRESS: 112 return "http://hl7.org/fhir/supplydelivery-status"; 113 case COMPLETED: 114 return "http://hl7.org/fhir/supplydelivery-status"; 115 case ABANDONED: 116 return "http://hl7.org/fhir/supplydelivery-status"; 117 case ENTEREDINERROR: 118 return "http://hl7.org/fhir/supplydelivery-status"; 119 case NULL: 120 return null; 121 default: 122 return "?"; 123 } 124 } 125 126 public String getDefinition() { 127 switch (this) { 128 case INPROGRESS: 129 return "Supply has been requested, but not delivered."; 130 case COMPLETED: 131 return "Supply has been delivered (\"completed\")."; 132 case ABANDONED: 133 return "Delivery was not completed."; 134 case ENTEREDINERROR: 135 return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"abandoned\" rather than \"entered-in-error\".)."; 136 case NULL: 137 return null; 138 default: 139 return "?"; 140 } 141 } 142 143 public String getDisplay() { 144 switch (this) { 145 case INPROGRESS: 146 return "In Progress"; 147 case COMPLETED: 148 return "Delivered"; 149 case ABANDONED: 150 return "Abandoned"; 151 case ENTEREDINERROR: 152 return "Entered In Error"; 153 case NULL: 154 return null; 155 default: 156 return "?"; 157 } 158 } 159 } 160 161 public static class SupplyDeliveryStatusEnumFactory implements EnumFactory<SupplyDeliveryStatus> { 162 public SupplyDeliveryStatus fromCode(String codeString) throws IllegalArgumentException { 163 if (codeString == null || "".equals(codeString)) 164 if (codeString == null || "".equals(codeString)) 165 return null; 166 if ("in-progress".equals(codeString)) 167 return SupplyDeliveryStatus.INPROGRESS; 168 if ("completed".equals(codeString)) 169 return SupplyDeliveryStatus.COMPLETED; 170 if ("abandoned".equals(codeString)) 171 return SupplyDeliveryStatus.ABANDONED; 172 if ("entered-in-error".equals(codeString)) 173 return SupplyDeliveryStatus.ENTEREDINERROR; 174 throw new IllegalArgumentException("Unknown SupplyDeliveryStatus code '" + codeString + "'"); 175 } 176 177 public Enumeration<SupplyDeliveryStatus> fromType(PrimitiveType<?> code) throws FHIRException { 178 if (code == null) 179 return null; 180 if (code.isEmpty()) 181 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.NULL, code); 182 String codeString = code.asStringValue(); 183 if (codeString == null || "".equals(codeString)) 184 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.NULL, code); 185 if ("in-progress".equals(codeString)) 186 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.INPROGRESS, code); 187 if ("completed".equals(codeString)) 188 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.COMPLETED, code); 189 if ("abandoned".equals(codeString)) 190 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.ABANDONED, code); 191 if ("entered-in-error".equals(codeString)) 192 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.ENTEREDINERROR, code); 193 throw new FHIRException("Unknown SupplyDeliveryStatus code '" + codeString + "'"); 194 } 195 196 public String toCode(SupplyDeliveryStatus code) { 197 if (code == SupplyDeliveryStatus.INPROGRESS) 198 return "in-progress"; 199 if (code == SupplyDeliveryStatus.COMPLETED) 200 return "completed"; 201 if (code == SupplyDeliveryStatus.ABANDONED) 202 return "abandoned"; 203 if (code == SupplyDeliveryStatus.ENTEREDINERROR) 204 return "entered-in-error"; 205 return "?"; 206 } 207 208 public String toSystem(SupplyDeliveryStatus code) { 209 return code.getSystem(); 210 } 211 } 212 213 @Block() 214 public static class SupplyDeliverySuppliedItemComponent extends BackboneElement implements IBaseBackboneElement { 215 /** 216 * The amount of supply that has been dispensed. Includes unit of measure. 217 */ 218 @Child(name = "quantity", type = { Quantity.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 219 @Description(shortDefinition = "Amount dispensed", formalDefinition = "The amount of supply that has been dispensed. Includes unit of measure.") 220 protected Quantity quantity; 221 222 /** 223 * Identifies the medication, substance or device being dispensed. This is 224 * either a link to a resource representing the details of the item or a code 225 * that identifies the item from a known list. 226 */ 227 @Child(name = "item", type = { CodeableConcept.class, Medication.class, Substance.class, 228 Device.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 229 @Description(shortDefinition = "Medication, Substance, or Device supplied", formalDefinition = "Identifies the medication, substance or device being dispensed. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.") 230 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/supply-item") 231 protected Type item; 232 233 private static final long serialVersionUID = 1628109307L; 234 235 /** 236 * Constructor 237 */ 238 public SupplyDeliverySuppliedItemComponent() { 239 super(); 240 } 241 242 /** 243 * @return {@link #quantity} (The amount of supply that has been dispensed. 244 * Includes unit of measure.) 245 */ 246 public Quantity getQuantity() { 247 if (this.quantity == null) 248 if (Configuration.errorOnAutoCreate()) 249 throw new Error("Attempt to auto-create SupplyDeliverySuppliedItemComponent.quantity"); 250 else if (Configuration.doAutoCreate()) 251 this.quantity = new Quantity(); // cc 252 return this.quantity; 253 } 254 255 public boolean hasQuantity() { 256 return this.quantity != null && !this.quantity.isEmpty(); 257 } 258 259 /** 260 * @param value {@link #quantity} (The amount of supply that has been dispensed. 261 * Includes unit of measure.) 262 */ 263 public SupplyDeliverySuppliedItemComponent setQuantity(Quantity value) { 264 this.quantity = value; 265 return this; 266 } 267 268 /** 269 * @return {@link #item} (Identifies the medication, substance or device being 270 * dispensed. This is either a link to a resource representing the 271 * details of the item or a code that identifies the item from a known 272 * list.) 273 */ 274 public Type getItem() { 275 return this.item; 276 } 277 278 /** 279 * @return {@link #item} (Identifies the medication, substance or device being 280 * dispensed. This is either a link to a resource representing the 281 * details of the item or a code that identifies the item from a known 282 * list.) 283 */ 284 public CodeableConcept getItemCodeableConcept() throws FHIRException { 285 if (this.item == null) 286 this.item = new CodeableConcept(); 287 if (!(this.item instanceof CodeableConcept)) 288 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 289 + this.item.getClass().getName() + " was encountered"); 290 return (CodeableConcept) this.item; 291 } 292 293 public boolean hasItemCodeableConcept() { 294 return this != null && this.item instanceof CodeableConcept; 295 } 296 297 /** 298 * @return {@link #item} (Identifies the medication, substance or device being 299 * dispensed. This is either a link to a resource representing the 300 * details of the item or a code that identifies the item from a known 301 * list.) 302 */ 303 public Reference getItemReference() throws FHIRException { 304 if (this.item == null) 305 this.item = new Reference(); 306 if (!(this.item instanceof Reference)) 307 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.item.getClass().getName() 308 + " was encountered"); 309 return (Reference) this.item; 310 } 311 312 public boolean hasItemReference() { 313 return this != null && this.item instanceof Reference; 314 } 315 316 public boolean hasItem() { 317 return this.item != null && !this.item.isEmpty(); 318 } 319 320 /** 321 * @param value {@link #item} (Identifies the medication, substance or device 322 * being dispensed. This is either a link to a resource 323 * representing the details of the item or a code that identifies 324 * the item from a known list.) 325 */ 326 public SupplyDeliverySuppliedItemComponent setItem(Type value) { 327 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 328 throw new Error("Not the right type for SupplyDelivery.suppliedItem.item[x]: " + value.fhirType()); 329 this.item = value; 330 return this; 331 } 332 333 protected void listChildren(List<Property> children) { 334 super.listChildren(children); 335 children.add(new Property("quantity", "SimpleQuantity", 336 "The amount of supply that has been dispensed. Includes unit of measure.", 0, 1, quantity)); 337 children.add(new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device)", 338 "Identifies the medication, substance or device being dispensed. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 339 0, 1, item)); 340 } 341 342 @Override 343 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 344 switch (_hash) { 345 case -1285004149: 346 /* quantity */ return new Property("quantity", "SimpleQuantity", 347 "The amount of supply that has been dispensed. Includes unit of measure.", 0, 1, quantity); 348 case 2116201613: 349 /* item[x] */ return new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device)", 350 "Identifies the medication, substance or device being dispensed. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 351 0, 1, item); 352 case 3242771: 353 /* item */ return new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device)", 354 "Identifies the medication, substance or device being dispensed. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 355 0, 1, item); 356 case 106644494: 357 /* itemCodeableConcept */ return new Property("item[x]", 358 "CodeableConcept|Reference(Medication|Substance|Device)", 359 "Identifies the medication, substance or device being dispensed. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 360 0, 1, item); 361 case 1376364920: 362 /* itemReference */ return new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device)", 363 "Identifies the medication, substance or device being dispensed. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 364 0, 1, item); 365 default: 366 return super.getNamedProperty(_hash, _name, _checkValid); 367 } 368 369 } 370 371 @Override 372 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 373 switch (hash) { 374 case -1285004149: 375 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 376 case 3242771: 377 /* item */ return this.item == null ? new Base[0] : new Base[] { this.item }; // Type 378 default: 379 return super.getProperty(hash, name, checkValid); 380 } 381 382 } 383 384 @Override 385 public Base setProperty(int hash, String name, Base value) throws FHIRException { 386 switch (hash) { 387 case -1285004149: // quantity 388 this.quantity = castToQuantity(value); // Quantity 389 return value; 390 case 3242771: // item 391 this.item = castToType(value); // Type 392 return value; 393 default: 394 return super.setProperty(hash, name, value); 395 } 396 397 } 398 399 @Override 400 public Base setProperty(String name, Base value) throws FHIRException { 401 if (name.equals("quantity")) { 402 this.quantity = castToQuantity(value); // Quantity 403 } else if (name.equals("item[x]")) { 404 this.item = castToType(value); // Type 405 } else 406 return super.setProperty(name, value); 407 return value; 408 } 409 410 @Override 411 public Base makeProperty(int hash, String name) throws FHIRException { 412 switch (hash) { 413 case -1285004149: 414 return getQuantity(); 415 case 2116201613: 416 return getItem(); 417 case 3242771: 418 return getItem(); 419 default: 420 return super.makeProperty(hash, name); 421 } 422 423 } 424 425 @Override 426 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 427 switch (hash) { 428 case -1285004149: 429 /* quantity */ return new String[] { "SimpleQuantity" }; 430 case 3242771: 431 /* item */ return new String[] { "CodeableConcept", "Reference" }; 432 default: 433 return super.getTypesForProperty(hash, name); 434 } 435 436 } 437 438 @Override 439 public Base addChild(String name) throws FHIRException { 440 if (name.equals("quantity")) { 441 this.quantity = new Quantity(); 442 return this.quantity; 443 } else if (name.equals("itemCodeableConcept")) { 444 this.item = new CodeableConcept(); 445 return this.item; 446 } else if (name.equals("itemReference")) { 447 this.item = new Reference(); 448 return this.item; 449 } else 450 return super.addChild(name); 451 } 452 453 public SupplyDeliverySuppliedItemComponent copy() { 454 SupplyDeliverySuppliedItemComponent dst = new SupplyDeliverySuppliedItemComponent(); 455 copyValues(dst); 456 return dst; 457 } 458 459 public void copyValues(SupplyDeliverySuppliedItemComponent dst) { 460 super.copyValues(dst); 461 dst.quantity = quantity == null ? null : quantity.copy(); 462 dst.item = item == null ? null : item.copy(); 463 } 464 465 @Override 466 public boolean equalsDeep(Base other_) { 467 if (!super.equalsDeep(other_)) 468 return false; 469 if (!(other_ instanceof SupplyDeliverySuppliedItemComponent)) 470 return false; 471 SupplyDeliverySuppliedItemComponent o = (SupplyDeliverySuppliedItemComponent) other_; 472 return compareDeep(quantity, o.quantity, true) && compareDeep(item, o.item, true); 473 } 474 475 @Override 476 public boolean equalsShallow(Base other_) { 477 if (!super.equalsShallow(other_)) 478 return false; 479 if (!(other_ instanceof SupplyDeliverySuppliedItemComponent)) 480 return false; 481 SupplyDeliverySuppliedItemComponent o = (SupplyDeliverySuppliedItemComponent) other_; 482 return true; 483 } 484 485 public boolean isEmpty() { 486 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(quantity, item); 487 } 488 489 public String fhirType() { 490 return "SupplyDelivery.suppliedItem"; 491 492 } 493 494 } 495 496 /** 497 * Identifier for the supply delivery event that is used to identify it across 498 * multiple disparate systems. 499 */ 500 @Child(name = "identifier", type = { 501 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 502 @Description(shortDefinition = "External identifier", formalDefinition = "Identifier for the supply delivery event that is used to identify it across multiple disparate systems.") 503 protected List<Identifier> identifier; 504 505 /** 506 * A plan, proposal or order that is fulfilled in whole or in part by this 507 * event. 508 */ 509 @Child(name = "basedOn", type = { 510 SupplyRequest.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 511 @Description(shortDefinition = "Fulfills plan, proposal or order", formalDefinition = "A plan, proposal or order that is fulfilled in whole or in part by this event.") 512 protected List<Reference> basedOn; 513 /** 514 * The actual objects that are the target of the reference (A plan, proposal or 515 * order that is fulfilled in whole or in part by this event.) 516 */ 517 protected List<SupplyRequest> basedOnTarget; 518 519 /** 520 * A larger event of which this particular event is a component or step. 521 */ 522 @Child(name = "partOf", type = { SupplyDelivery.class, 523 Contract.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 524 @Description(shortDefinition = "Part of referenced event", formalDefinition = "A larger event of which this particular event is a component or step.") 525 protected List<Reference> partOf; 526 /** 527 * The actual objects that are the target of the reference (A larger event of 528 * which this particular event is a component or step.) 529 */ 530 protected List<Resource> partOfTarget; 531 532 /** 533 * A code specifying the state of the dispense event. 534 */ 535 @Child(name = "status", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = true, summary = true) 536 @Description(shortDefinition = "in-progress | completed | abandoned | entered-in-error", formalDefinition = "A code specifying the state of the dispense event.") 537 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/supplydelivery-status") 538 protected Enumeration<SupplyDeliveryStatus> status; 539 540 /** 541 * A link to a resource representing the person whom the delivered item is for. 542 */ 543 @Child(name = "patient", type = { Patient.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 544 @Description(shortDefinition = "Patient for whom the item is supplied", formalDefinition = "A link to a resource representing the person whom the delivered item is for.") 545 protected Reference patient; 546 547 /** 548 * The actual object that is the target of the reference (A link to a resource 549 * representing the person whom the delivered item is for.) 550 */ 551 protected Patient patientTarget; 552 553 /** 554 * Indicates the type of dispensing event that is performed. Examples include: 555 * Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc. 556 */ 557 @Child(name = "type", type = { 558 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 559 @Description(shortDefinition = "Category of dispense event", formalDefinition = "Indicates the type of dispensing event that is performed. Examples include: Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc.") 560 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/supplydelivery-type") 561 protected CodeableConcept type; 562 563 /** 564 * The item that is being delivered or has been supplied. 565 */ 566 @Child(name = "suppliedItem", type = {}, order = 6, min = 0, max = 1, modifier = false, summary = false) 567 @Description(shortDefinition = "The item that is delivered or supplied", formalDefinition = "The item that is being delivered or has been supplied.") 568 protected SupplyDeliverySuppliedItemComponent suppliedItem; 569 570 /** 571 * The date or time(s) the activity occurred. 572 */ 573 @Child(name = "occurrence", type = { DateTimeType.class, Period.class, 574 Timing.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 575 @Description(shortDefinition = "When event occurred", formalDefinition = "The date or time(s) the activity occurred.") 576 protected Type occurrence; 577 578 /** 579 * The individual responsible for dispensing the medication, supplier or device. 580 */ 581 @Child(name = "supplier", type = { Practitioner.class, PractitionerRole.class, 582 Organization.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 583 @Description(shortDefinition = "Dispenser", formalDefinition = "The individual responsible for dispensing the medication, supplier or device.") 584 protected Reference supplier; 585 586 /** 587 * The actual object that is the target of the reference (The individual 588 * responsible for dispensing the medication, supplier or device.) 589 */ 590 protected Resource supplierTarget; 591 592 /** 593 * Identification of the facility/location where the Supply was shipped to, as 594 * part of the dispense event. 595 */ 596 @Child(name = "destination", type = { 597 Location.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 598 @Description(shortDefinition = "Where the Supply was sent", formalDefinition = "Identification of the facility/location where the Supply was shipped to, as part of the dispense event.") 599 protected Reference destination; 600 601 /** 602 * The actual object that is the target of the reference (Identification of the 603 * facility/location where the Supply was shipped to, as part of the dispense 604 * event.) 605 */ 606 protected Location destinationTarget; 607 608 /** 609 * Identifies the person who picked up the Supply. 610 */ 611 @Child(name = "receiver", type = { Practitioner.class, 612 PractitionerRole.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 613 @Description(shortDefinition = "Who collected the Supply", formalDefinition = "Identifies the person who picked up the Supply.") 614 protected List<Reference> receiver; 615 /** 616 * The actual objects that are the target of the reference (Identifies the 617 * person who picked up the Supply.) 618 */ 619 protected List<Resource> receiverTarget; 620 621 private static final long serialVersionUID = -750389806L; 622 623 /** 624 * Constructor 625 */ 626 public SupplyDelivery() { 627 super(); 628 } 629 630 /** 631 * @return {@link #identifier} (Identifier for the supply delivery event that is 632 * used to identify it across multiple disparate systems.) 633 */ 634 public List<Identifier> getIdentifier() { 635 if (this.identifier == null) 636 this.identifier = new ArrayList<Identifier>(); 637 return this.identifier; 638 } 639 640 /** 641 * @return Returns a reference to <code>this</code> for easy method chaining 642 */ 643 public SupplyDelivery setIdentifier(List<Identifier> theIdentifier) { 644 this.identifier = theIdentifier; 645 return this; 646 } 647 648 public boolean hasIdentifier() { 649 if (this.identifier == null) 650 return false; 651 for (Identifier item : this.identifier) 652 if (!item.isEmpty()) 653 return true; 654 return false; 655 } 656 657 public Identifier addIdentifier() { // 3 658 Identifier t = new Identifier(); 659 if (this.identifier == null) 660 this.identifier = new ArrayList<Identifier>(); 661 this.identifier.add(t); 662 return t; 663 } 664 665 public SupplyDelivery addIdentifier(Identifier t) { // 3 666 if (t == null) 667 return this; 668 if (this.identifier == null) 669 this.identifier = new ArrayList<Identifier>(); 670 this.identifier.add(t); 671 return this; 672 } 673 674 /** 675 * @return The first repetition of repeating field {@link #identifier}, creating 676 * it if it does not already exist 677 */ 678 public Identifier getIdentifierFirstRep() { 679 if (getIdentifier().isEmpty()) { 680 addIdentifier(); 681 } 682 return getIdentifier().get(0); 683 } 684 685 /** 686 * @return {@link #basedOn} (A plan, proposal or order that is fulfilled in 687 * whole or in part by this event.) 688 */ 689 public List<Reference> getBasedOn() { 690 if (this.basedOn == null) 691 this.basedOn = new ArrayList<Reference>(); 692 return this.basedOn; 693 } 694 695 /** 696 * @return Returns a reference to <code>this</code> for easy method chaining 697 */ 698 public SupplyDelivery setBasedOn(List<Reference> theBasedOn) { 699 this.basedOn = theBasedOn; 700 return this; 701 } 702 703 public boolean hasBasedOn() { 704 if (this.basedOn == null) 705 return false; 706 for (Reference item : this.basedOn) 707 if (!item.isEmpty()) 708 return true; 709 return false; 710 } 711 712 public Reference addBasedOn() { // 3 713 Reference t = new Reference(); 714 if (this.basedOn == null) 715 this.basedOn = new ArrayList<Reference>(); 716 this.basedOn.add(t); 717 return t; 718 } 719 720 public SupplyDelivery addBasedOn(Reference t) { // 3 721 if (t == null) 722 return this; 723 if (this.basedOn == null) 724 this.basedOn = new ArrayList<Reference>(); 725 this.basedOn.add(t); 726 return this; 727 } 728 729 /** 730 * @return The first repetition of repeating field {@link #basedOn}, creating it 731 * if it does not already exist 732 */ 733 public Reference getBasedOnFirstRep() { 734 if (getBasedOn().isEmpty()) { 735 addBasedOn(); 736 } 737 return getBasedOn().get(0); 738 } 739 740 /** 741 * @deprecated Use Reference#setResource(IBaseResource) instead 742 */ 743 @Deprecated 744 public List<SupplyRequest> getBasedOnTarget() { 745 if (this.basedOnTarget == null) 746 this.basedOnTarget = new ArrayList<SupplyRequest>(); 747 return this.basedOnTarget; 748 } 749 750 /** 751 * @deprecated Use Reference#setResource(IBaseResource) instead 752 */ 753 @Deprecated 754 public SupplyRequest addBasedOnTarget() { 755 SupplyRequest r = new SupplyRequest(); 756 if (this.basedOnTarget == null) 757 this.basedOnTarget = new ArrayList<SupplyRequest>(); 758 this.basedOnTarget.add(r); 759 return r; 760 } 761 762 /** 763 * @return {@link #partOf} (A larger event of which this particular event is a 764 * component or step.) 765 */ 766 public List<Reference> getPartOf() { 767 if (this.partOf == null) 768 this.partOf = new ArrayList<Reference>(); 769 return this.partOf; 770 } 771 772 /** 773 * @return Returns a reference to <code>this</code> for easy method chaining 774 */ 775 public SupplyDelivery setPartOf(List<Reference> thePartOf) { 776 this.partOf = thePartOf; 777 return this; 778 } 779 780 public boolean hasPartOf() { 781 if (this.partOf == null) 782 return false; 783 for (Reference item : this.partOf) 784 if (!item.isEmpty()) 785 return true; 786 return false; 787 } 788 789 public Reference addPartOf() { // 3 790 Reference t = new Reference(); 791 if (this.partOf == null) 792 this.partOf = new ArrayList<Reference>(); 793 this.partOf.add(t); 794 return t; 795 } 796 797 public SupplyDelivery addPartOf(Reference t) { // 3 798 if (t == null) 799 return this; 800 if (this.partOf == null) 801 this.partOf = new ArrayList<Reference>(); 802 this.partOf.add(t); 803 return this; 804 } 805 806 /** 807 * @return The first repetition of repeating field {@link #partOf}, creating it 808 * if it does not already exist 809 */ 810 public Reference getPartOfFirstRep() { 811 if (getPartOf().isEmpty()) { 812 addPartOf(); 813 } 814 return getPartOf().get(0); 815 } 816 817 /** 818 * @deprecated Use Reference#setResource(IBaseResource) instead 819 */ 820 @Deprecated 821 public List<Resource> getPartOfTarget() { 822 if (this.partOfTarget == null) 823 this.partOfTarget = new ArrayList<Resource>(); 824 return this.partOfTarget; 825 } 826 827 /** 828 * @return {@link #status} (A code specifying the state of the dispense event.). 829 * This is the underlying object with id, value and extensions. The 830 * accessor "getStatus" gives direct access to the value 831 */ 832 public Enumeration<SupplyDeliveryStatus> getStatusElement() { 833 if (this.status == null) 834 if (Configuration.errorOnAutoCreate()) 835 throw new Error("Attempt to auto-create SupplyDelivery.status"); 836 else if (Configuration.doAutoCreate()) 837 this.status = new Enumeration<SupplyDeliveryStatus>(new SupplyDeliveryStatusEnumFactory()); // bb 838 return this.status; 839 } 840 841 public boolean hasStatusElement() { 842 return this.status != null && !this.status.isEmpty(); 843 } 844 845 public boolean hasStatus() { 846 return this.status != null && !this.status.isEmpty(); 847 } 848 849 /** 850 * @param value {@link #status} (A code specifying the state of the dispense 851 * event.). This is the underlying object with id, value and 852 * extensions. The accessor "getStatus" gives direct access to the 853 * value 854 */ 855 public SupplyDelivery setStatusElement(Enumeration<SupplyDeliveryStatus> value) { 856 this.status = value; 857 return this; 858 } 859 860 /** 861 * @return A code specifying the state of the dispense event. 862 */ 863 public SupplyDeliveryStatus getStatus() { 864 return this.status == null ? null : this.status.getValue(); 865 } 866 867 /** 868 * @param value A code specifying the state of the dispense event. 869 */ 870 public SupplyDelivery setStatus(SupplyDeliveryStatus value) { 871 if (value == null) 872 this.status = null; 873 else { 874 if (this.status == null) 875 this.status = new Enumeration<SupplyDeliveryStatus>(new SupplyDeliveryStatusEnumFactory()); 876 this.status.setValue(value); 877 } 878 return this; 879 } 880 881 /** 882 * @return {@link #patient} (A link to a resource representing the person whom 883 * the delivered item is for.) 884 */ 885 public Reference getPatient() { 886 if (this.patient == null) 887 if (Configuration.errorOnAutoCreate()) 888 throw new Error("Attempt to auto-create SupplyDelivery.patient"); 889 else if (Configuration.doAutoCreate()) 890 this.patient = new Reference(); // cc 891 return this.patient; 892 } 893 894 public boolean hasPatient() { 895 return this.patient != null && !this.patient.isEmpty(); 896 } 897 898 /** 899 * @param value {@link #patient} (A link to a resource representing the person 900 * whom the delivered item is for.) 901 */ 902 public SupplyDelivery setPatient(Reference value) { 903 this.patient = value; 904 return this; 905 } 906 907 /** 908 * @return {@link #patient} The actual object that is the target of the 909 * reference. The reference library doesn't populate this, but you can 910 * use it to hold the resource if you resolve it. (A link to a resource 911 * representing the person whom the delivered item is for.) 912 */ 913 public Patient getPatientTarget() { 914 if (this.patientTarget == null) 915 if (Configuration.errorOnAutoCreate()) 916 throw new Error("Attempt to auto-create SupplyDelivery.patient"); 917 else if (Configuration.doAutoCreate()) 918 this.patientTarget = new Patient(); // aa 919 return this.patientTarget; 920 } 921 922 /** 923 * @param value {@link #patient} The actual object that is the target of the 924 * reference. The reference library doesn't use these, but you can 925 * use it to hold the resource if you resolve it. (A link to a 926 * resource representing the person whom the delivered item is 927 * for.) 928 */ 929 public SupplyDelivery setPatientTarget(Patient value) { 930 this.patientTarget = value; 931 return this; 932 } 933 934 /** 935 * @return {@link #type} (Indicates the type of dispensing event that is 936 * performed. Examples include: Trial Fill, Completion of Trial, Partial 937 * Fill, Emergency Fill, Samples, etc.) 938 */ 939 public CodeableConcept getType() { 940 if (this.type == null) 941 if (Configuration.errorOnAutoCreate()) 942 throw new Error("Attempt to auto-create SupplyDelivery.type"); 943 else if (Configuration.doAutoCreate()) 944 this.type = new CodeableConcept(); // cc 945 return this.type; 946 } 947 948 public boolean hasType() { 949 return this.type != null && !this.type.isEmpty(); 950 } 951 952 /** 953 * @param value {@link #type} (Indicates the type of dispensing event that is 954 * performed. Examples include: Trial Fill, Completion of Trial, 955 * Partial Fill, Emergency Fill, Samples, etc.) 956 */ 957 public SupplyDelivery setType(CodeableConcept value) { 958 this.type = value; 959 return this; 960 } 961 962 /** 963 * @return {@link #suppliedItem} (The item that is being delivered or has been 964 * supplied.) 965 */ 966 public SupplyDeliverySuppliedItemComponent getSuppliedItem() { 967 if (this.suppliedItem == null) 968 if (Configuration.errorOnAutoCreate()) 969 throw new Error("Attempt to auto-create SupplyDelivery.suppliedItem"); 970 else if (Configuration.doAutoCreate()) 971 this.suppliedItem = new SupplyDeliverySuppliedItemComponent(); // cc 972 return this.suppliedItem; 973 } 974 975 public boolean hasSuppliedItem() { 976 return this.suppliedItem != null && !this.suppliedItem.isEmpty(); 977 } 978 979 /** 980 * @param value {@link #suppliedItem} (The item that is being delivered or has 981 * been supplied.) 982 */ 983 public SupplyDelivery setSuppliedItem(SupplyDeliverySuppliedItemComponent value) { 984 this.suppliedItem = value; 985 return this; 986 } 987 988 /** 989 * @return {@link #occurrence} (The date or time(s) the activity occurred.) 990 */ 991 public Type getOccurrence() { 992 return this.occurrence; 993 } 994 995 /** 996 * @return {@link #occurrence} (The date or time(s) the activity occurred.) 997 */ 998 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 999 if (this.occurrence == null) 1000 this.occurrence = new DateTimeType(); 1001 if (!(this.occurrence instanceof DateTimeType)) 1002 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1003 + this.occurrence.getClass().getName() + " was encountered"); 1004 return (DateTimeType) this.occurrence; 1005 } 1006 1007 public boolean hasOccurrenceDateTimeType() { 1008 return this != null && this.occurrence instanceof DateTimeType; 1009 } 1010 1011 /** 1012 * @return {@link #occurrence} (The date or time(s) the activity occurred.) 1013 */ 1014 public Period getOccurrencePeriod() throws FHIRException { 1015 if (this.occurrence == null) 1016 this.occurrence = new Period(); 1017 if (!(this.occurrence instanceof Period)) 1018 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.occurrence.getClass().getName() 1019 + " was encountered"); 1020 return (Period) this.occurrence; 1021 } 1022 1023 public boolean hasOccurrencePeriod() { 1024 return this != null && this.occurrence instanceof Period; 1025 } 1026 1027 /** 1028 * @return {@link #occurrence} (The date or time(s) the activity occurred.) 1029 */ 1030 public Timing getOccurrenceTiming() throws FHIRException { 1031 if (this.occurrence == null) 1032 this.occurrence = new Timing(); 1033 if (!(this.occurrence instanceof Timing)) 1034 throw new FHIRException("Type mismatch: the type Timing was expected, but " + this.occurrence.getClass().getName() 1035 + " was encountered"); 1036 return (Timing) this.occurrence; 1037 } 1038 1039 public boolean hasOccurrenceTiming() { 1040 return this != null && this.occurrence instanceof Timing; 1041 } 1042 1043 public boolean hasOccurrence() { 1044 return this.occurrence != null && !this.occurrence.isEmpty(); 1045 } 1046 1047 /** 1048 * @param value {@link #occurrence} (The date or time(s) the activity occurred.) 1049 */ 1050 public SupplyDelivery setOccurrence(Type value) { 1051 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 1052 throw new Error("Not the right type for SupplyDelivery.occurrence[x]: " + value.fhirType()); 1053 this.occurrence = value; 1054 return this; 1055 } 1056 1057 /** 1058 * @return {@link #supplier} (The individual responsible for dispensing the 1059 * medication, supplier or device.) 1060 */ 1061 public Reference getSupplier() { 1062 if (this.supplier == null) 1063 if (Configuration.errorOnAutoCreate()) 1064 throw new Error("Attempt to auto-create SupplyDelivery.supplier"); 1065 else if (Configuration.doAutoCreate()) 1066 this.supplier = new Reference(); // cc 1067 return this.supplier; 1068 } 1069 1070 public boolean hasSupplier() { 1071 return this.supplier != null && !this.supplier.isEmpty(); 1072 } 1073 1074 /** 1075 * @param value {@link #supplier} (The individual responsible for dispensing the 1076 * medication, supplier or device.) 1077 */ 1078 public SupplyDelivery setSupplier(Reference value) { 1079 this.supplier = value; 1080 return this; 1081 } 1082 1083 /** 1084 * @return {@link #supplier} The actual object that is the target of the 1085 * reference. The reference library doesn't populate this, but you can 1086 * use it to hold the resource if you resolve it. (The individual 1087 * responsible for dispensing the medication, supplier or device.) 1088 */ 1089 public Resource getSupplierTarget() { 1090 return this.supplierTarget; 1091 } 1092 1093 /** 1094 * @param value {@link #supplier} The actual object that is the target of the 1095 * reference. The reference library doesn't use these, but you can 1096 * use it to hold the resource if you resolve it. (The individual 1097 * responsible for dispensing the medication, supplier or device.) 1098 */ 1099 public SupplyDelivery setSupplierTarget(Resource value) { 1100 this.supplierTarget = value; 1101 return this; 1102 } 1103 1104 /** 1105 * @return {@link #destination} (Identification of the facility/location where 1106 * the Supply was shipped to, as part of the dispense event.) 1107 */ 1108 public Reference getDestination() { 1109 if (this.destination == null) 1110 if (Configuration.errorOnAutoCreate()) 1111 throw new Error("Attempt to auto-create SupplyDelivery.destination"); 1112 else if (Configuration.doAutoCreate()) 1113 this.destination = new Reference(); // cc 1114 return this.destination; 1115 } 1116 1117 public boolean hasDestination() { 1118 return this.destination != null && !this.destination.isEmpty(); 1119 } 1120 1121 /** 1122 * @param value {@link #destination} (Identification of the facility/location 1123 * where the Supply was shipped to, as part of the dispense event.) 1124 */ 1125 public SupplyDelivery setDestination(Reference value) { 1126 this.destination = value; 1127 return this; 1128 } 1129 1130 /** 1131 * @return {@link #destination} The actual object that is the target of the 1132 * reference. The reference library doesn't populate this, but you can 1133 * use it to hold the resource if you resolve it. (Identification of the 1134 * facility/location where the Supply was shipped to, as part of the 1135 * dispense event.) 1136 */ 1137 public Location getDestinationTarget() { 1138 if (this.destinationTarget == null) 1139 if (Configuration.errorOnAutoCreate()) 1140 throw new Error("Attempt to auto-create SupplyDelivery.destination"); 1141 else if (Configuration.doAutoCreate()) 1142 this.destinationTarget = new Location(); // aa 1143 return this.destinationTarget; 1144 } 1145 1146 /** 1147 * @param value {@link #destination} The actual object that is the target of the 1148 * reference. The reference library doesn't use these, but you can 1149 * use it to hold the resource if you resolve it. (Identification 1150 * of the facility/location where the Supply was shipped to, as 1151 * part of the dispense event.) 1152 */ 1153 public SupplyDelivery setDestinationTarget(Location value) { 1154 this.destinationTarget = value; 1155 return this; 1156 } 1157 1158 /** 1159 * @return {@link #receiver} (Identifies the person who picked up the Supply.) 1160 */ 1161 public List<Reference> getReceiver() { 1162 if (this.receiver == null) 1163 this.receiver = new ArrayList<Reference>(); 1164 return this.receiver; 1165 } 1166 1167 /** 1168 * @return Returns a reference to <code>this</code> for easy method chaining 1169 */ 1170 public SupplyDelivery setReceiver(List<Reference> theReceiver) { 1171 this.receiver = theReceiver; 1172 return this; 1173 } 1174 1175 public boolean hasReceiver() { 1176 if (this.receiver == null) 1177 return false; 1178 for (Reference item : this.receiver) 1179 if (!item.isEmpty()) 1180 return true; 1181 return false; 1182 } 1183 1184 public Reference addReceiver() { // 3 1185 Reference t = new Reference(); 1186 if (this.receiver == null) 1187 this.receiver = new ArrayList<Reference>(); 1188 this.receiver.add(t); 1189 return t; 1190 } 1191 1192 public SupplyDelivery addReceiver(Reference t) { // 3 1193 if (t == null) 1194 return this; 1195 if (this.receiver == null) 1196 this.receiver = new ArrayList<Reference>(); 1197 this.receiver.add(t); 1198 return this; 1199 } 1200 1201 /** 1202 * @return The first repetition of repeating field {@link #receiver}, creating 1203 * it if it does not already exist 1204 */ 1205 public Reference getReceiverFirstRep() { 1206 if (getReceiver().isEmpty()) { 1207 addReceiver(); 1208 } 1209 return getReceiver().get(0); 1210 } 1211 1212 /** 1213 * @deprecated Use Reference#setResource(IBaseResource) instead 1214 */ 1215 @Deprecated 1216 public List<Resource> getReceiverTarget() { 1217 if (this.receiverTarget == null) 1218 this.receiverTarget = new ArrayList<Resource>(); 1219 return this.receiverTarget; 1220 } 1221 1222 protected void listChildren(List<Property> children) { 1223 super.listChildren(children); 1224 children.add(new Property("identifier", "Identifier", 1225 "Identifier for the supply delivery event that is used to identify it across multiple disparate systems.", 0, 1226 java.lang.Integer.MAX_VALUE, identifier)); 1227 children.add(new Property("basedOn", "Reference(SupplyRequest)", 1228 "A plan, proposal or order that is fulfilled in whole or in part by this event.", 0, 1229 java.lang.Integer.MAX_VALUE, basedOn)); 1230 children.add(new Property("partOf", "Reference(SupplyDelivery|Contract)", 1231 "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, 1232 partOf)); 1233 children.add(new Property("status", "code", "A code specifying the state of the dispense event.", 0, 1, status)); 1234 children.add(new Property("patient", "Reference(Patient)", 1235 "A link to a resource representing the person whom the delivered item is for.", 0, 1, patient)); 1236 children.add(new Property("type", "CodeableConcept", 1237 "Indicates the type of dispensing event that is performed. Examples include: Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc.", 1238 0, 1, type)); 1239 children.add( 1240 new Property("suppliedItem", "", "The item that is being delivered or has been supplied.", 0, 1, suppliedItem)); 1241 children.add(new Property("occurrence[x]", "dateTime|Period|Timing", "The date or time(s) the activity occurred.", 1242 0, 1, occurrence)); 1243 children.add(new Property("supplier", "Reference(Practitioner|PractitionerRole|Organization)", 1244 "The individual responsible for dispensing the medication, supplier or device.", 0, 1, supplier)); 1245 children.add(new Property("destination", "Reference(Location)", 1246 "Identification of the facility/location where the Supply was shipped to, as part of the dispense event.", 0, 1, 1247 destination)); 1248 children.add(new Property("receiver", "Reference(Practitioner|PractitionerRole)", 1249 "Identifies the person who picked up the Supply.", 0, java.lang.Integer.MAX_VALUE, receiver)); 1250 } 1251 1252 @Override 1253 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1254 switch (_hash) { 1255 case -1618432855: 1256 /* identifier */ return new Property("identifier", "Identifier", 1257 "Identifier for the supply delivery event that is used to identify it across multiple disparate systems.", 0, 1258 java.lang.Integer.MAX_VALUE, identifier); 1259 case -332612366: 1260 /* basedOn */ return new Property("basedOn", "Reference(SupplyRequest)", 1261 "A plan, proposal or order that is fulfilled in whole or in part by this event.", 0, 1262 java.lang.Integer.MAX_VALUE, basedOn); 1263 case -995410646: 1264 /* partOf */ return new Property("partOf", "Reference(SupplyDelivery|Contract)", 1265 "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, 1266 partOf); 1267 case -892481550: 1268 /* status */ return new Property("status", "code", "A code specifying the state of the dispense event.", 0, 1, 1269 status); 1270 case -791418107: 1271 /* patient */ return new Property("patient", "Reference(Patient)", 1272 "A link to a resource representing the person whom the delivered item is for.", 0, 1, patient); 1273 case 3575610: 1274 /* type */ return new Property("type", "CodeableConcept", 1275 "Indicates the type of dispensing event that is performed. Examples include: Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc.", 1276 0, 1, type); 1277 case 1993333233: 1278 /* suppliedItem */ return new Property("suppliedItem", "", 1279 "The item that is being delivered or has been supplied.", 0, 1, suppliedItem); 1280 case -2022646513: 1281 /* occurrence[x] */ return new Property("occurrence[x]", "dateTime|Period|Timing", 1282 "The date or time(s) the activity occurred.", 0, 1, occurrence); 1283 case 1687874001: 1284 /* occurrence */ return new Property("occurrence[x]", "dateTime|Period|Timing", 1285 "The date or time(s) the activity occurred.", 0, 1, occurrence); 1286 case -298443636: 1287 /* occurrenceDateTime */ return new Property("occurrence[x]", "dateTime|Period|Timing", 1288 "The date or time(s) the activity occurred.", 0, 1, occurrence); 1289 case 1397156594: 1290 /* occurrencePeriod */ return new Property("occurrence[x]", "dateTime|Period|Timing", 1291 "The date or time(s) the activity occurred.", 0, 1, occurrence); 1292 case 1515218299: 1293 /* occurrenceTiming */ return new Property("occurrence[x]", "dateTime|Period|Timing", 1294 "The date or time(s) the activity occurred.", 0, 1, occurrence); 1295 case -1663305268: 1296 /* supplier */ return new Property("supplier", "Reference(Practitioner|PractitionerRole|Organization)", 1297 "The individual responsible for dispensing the medication, supplier or device.", 0, 1, supplier); 1298 case -1429847026: 1299 /* destination */ return new Property("destination", "Reference(Location)", 1300 "Identification of the facility/location where the Supply was shipped to, as part of the dispense event.", 0, 1301 1, destination); 1302 case -808719889: 1303 /* receiver */ return new Property("receiver", "Reference(Practitioner|PractitionerRole)", 1304 "Identifies the person who picked up the Supply.", 0, java.lang.Integer.MAX_VALUE, receiver); 1305 default: 1306 return super.getNamedProperty(_hash, _name, _checkValid); 1307 } 1308 1309 } 1310 1311 @Override 1312 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1313 switch (hash) { 1314 case -1618432855: 1315 /* identifier */ return this.identifier == null ? new Base[0] 1316 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1317 case -332612366: 1318 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1319 case -995410646: 1320 /* partOf */ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 1321 case -892481550: 1322 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<SupplyDeliveryStatus> 1323 case -791418107: 1324 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 1325 case 3575610: 1326 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1327 case 1993333233: 1328 /* suppliedItem */ return this.suppliedItem == null ? new Base[0] : new Base[] { this.suppliedItem }; // SupplyDeliverySuppliedItemComponent 1329 case 1687874001: 1330 /* occurrence */ return this.occurrence == null ? new Base[0] : new Base[] { this.occurrence }; // Type 1331 case -1663305268: 1332 /* supplier */ return this.supplier == null ? new Base[0] : new Base[] { this.supplier }; // Reference 1333 case -1429847026: 1334 /* destination */ return this.destination == null ? new Base[0] : new Base[] { this.destination }; // Reference 1335 case -808719889: 1336 /* receiver */ return this.receiver == null ? new Base[0] : this.receiver.toArray(new Base[this.receiver.size()]); // Reference 1337 default: 1338 return super.getProperty(hash, name, checkValid); 1339 } 1340 1341 } 1342 1343 @Override 1344 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1345 switch (hash) { 1346 case -1618432855: // identifier 1347 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1348 return value; 1349 case -332612366: // basedOn 1350 this.getBasedOn().add(castToReference(value)); // Reference 1351 return value; 1352 case -995410646: // partOf 1353 this.getPartOf().add(castToReference(value)); // Reference 1354 return value; 1355 case -892481550: // status 1356 value = new SupplyDeliveryStatusEnumFactory().fromType(castToCode(value)); 1357 this.status = (Enumeration) value; // Enumeration<SupplyDeliveryStatus> 1358 return value; 1359 case -791418107: // patient 1360 this.patient = castToReference(value); // Reference 1361 return value; 1362 case 3575610: // type 1363 this.type = castToCodeableConcept(value); // CodeableConcept 1364 return value; 1365 case 1993333233: // suppliedItem 1366 this.suppliedItem = (SupplyDeliverySuppliedItemComponent) value; // SupplyDeliverySuppliedItemComponent 1367 return value; 1368 case 1687874001: // occurrence 1369 this.occurrence = castToType(value); // Type 1370 return value; 1371 case -1663305268: // supplier 1372 this.supplier = castToReference(value); // Reference 1373 return value; 1374 case -1429847026: // destination 1375 this.destination = castToReference(value); // Reference 1376 return value; 1377 case -808719889: // receiver 1378 this.getReceiver().add(castToReference(value)); // Reference 1379 return value; 1380 default: 1381 return super.setProperty(hash, name, value); 1382 } 1383 1384 } 1385 1386 @Override 1387 public Base setProperty(String name, Base value) throws FHIRException { 1388 if (name.equals("identifier")) { 1389 this.getIdentifier().add(castToIdentifier(value)); 1390 } else if (name.equals("basedOn")) { 1391 this.getBasedOn().add(castToReference(value)); 1392 } else if (name.equals("partOf")) { 1393 this.getPartOf().add(castToReference(value)); 1394 } else if (name.equals("status")) { 1395 value = new SupplyDeliveryStatusEnumFactory().fromType(castToCode(value)); 1396 this.status = (Enumeration) value; // Enumeration<SupplyDeliveryStatus> 1397 } else if (name.equals("patient")) { 1398 this.patient = castToReference(value); // Reference 1399 } else if (name.equals("type")) { 1400 this.type = castToCodeableConcept(value); // CodeableConcept 1401 } else if (name.equals("suppliedItem")) { 1402 this.suppliedItem = (SupplyDeliverySuppliedItemComponent) value; // SupplyDeliverySuppliedItemComponent 1403 } else if (name.equals("occurrence[x]")) { 1404 this.occurrence = castToType(value); // Type 1405 } else if (name.equals("supplier")) { 1406 this.supplier = castToReference(value); // Reference 1407 } else if (name.equals("destination")) { 1408 this.destination = castToReference(value); // Reference 1409 } else if (name.equals("receiver")) { 1410 this.getReceiver().add(castToReference(value)); 1411 } else 1412 return super.setProperty(name, value); 1413 return value; 1414 } 1415 1416 @Override 1417 public Base makeProperty(int hash, String name) throws FHIRException { 1418 switch (hash) { 1419 case -1618432855: 1420 return addIdentifier(); 1421 case -332612366: 1422 return addBasedOn(); 1423 case -995410646: 1424 return addPartOf(); 1425 case -892481550: 1426 return getStatusElement(); 1427 case -791418107: 1428 return getPatient(); 1429 case 3575610: 1430 return getType(); 1431 case 1993333233: 1432 return getSuppliedItem(); 1433 case -2022646513: 1434 return getOccurrence(); 1435 case 1687874001: 1436 return getOccurrence(); 1437 case -1663305268: 1438 return getSupplier(); 1439 case -1429847026: 1440 return getDestination(); 1441 case -808719889: 1442 return addReceiver(); 1443 default: 1444 return super.makeProperty(hash, name); 1445 } 1446 1447 } 1448 1449 @Override 1450 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1451 switch (hash) { 1452 case -1618432855: 1453 /* identifier */ return new String[] { "Identifier" }; 1454 case -332612366: 1455 /* basedOn */ return new String[] { "Reference" }; 1456 case -995410646: 1457 /* partOf */ return new String[] { "Reference" }; 1458 case -892481550: 1459 /* status */ return new String[] { "code" }; 1460 case -791418107: 1461 /* patient */ return new String[] { "Reference" }; 1462 case 3575610: 1463 /* type */ return new String[] { "CodeableConcept" }; 1464 case 1993333233: 1465 /* suppliedItem */ return new String[] {}; 1466 case 1687874001: 1467 /* occurrence */ return new String[] { "dateTime", "Period", "Timing" }; 1468 case -1663305268: 1469 /* supplier */ return new String[] { "Reference" }; 1470 case -1429847026: 1471 /* destination */ return new String[] { "Reference" }; 1472 case -808719889: 1473 /* receiver */ return new String[] { "Reference" }; 1474 default: 1475 return super.getTypesForProperty(hash, name); 1476 } 1477 1478 } 1479 1480 @Override 1481 public Base addChild(String name) throws FHIRException { 1482 if (name.equals("identifier")) { 1483 return addIdentifier(); 1484 } else if (name.equals("basedOn")) { 1485 return addBasedOn(); 1486 } else if (name.equals("partOf")) { 1487 return addPartOf(); 1488 } else if (name.equals("status")) { 1489 throw new FHIRException("Cannot call addChild on a singleton property SupplyDelivery.status"); 1490 } else if (name.equals("patient")) { 1491 this.patient = new Reference(); 1492 return this.patient; 1493 } else if (name.equals("type")) { 1494 this.type = new CodeableConcept(); 1495 return this.type; 1496 } else if (name.equals("suppliedItem")) { 1497 this.suppliedItem = new SupplyDeliverySuppliedItemComponent(); 1498 return this.suppliedItem; 1499 } else if (name.equals("occurrenceDateTime")) { 1500 this.occurrence = new DateTimeType(); 1501 return this.occurrence; 1502 } else if (name.equals("occurrencePeriod")) { 1503 this.occurrence = new Period(); 1504 return this.occurrence; 1505 } else if (name.equals("occurrenceTiming")) { 1506 this.occurrence = new Timing(); 1507 return this.occurrence; 1508 } else if (name.equals("supplier")) { 1509 this.supplier = new Reference(); 1510 return this.supplier; 1511 } else if (name.equals("destination")) { 1512 this.destination = new Reference(); 1513 return this.destination; 1514 } else if (name.equals("receiver")) { 1515 return addReceiver(); 1516 } else 1517 return super.addChild(name); 1518 } 1519 1520 public String fhirType() { 1521 return "SupplyDelivery"; 1522 1523 } 1524 1525 public SupplyDelivery copy() { 1526 SupplyDelivery dst = new SupplyDelivery(); 1527 copyValues(dst); 1528 return dst; 1529 } 1530 1531 public void copyValues(SupplyDelivery dst) { 1532 super.copyValues(dst); 1533 if (identifier != null) { 1534 dst.identifier = new ArrayList<Identifier>(); 1535 for (Identifier i : identifier) 1536 dst.identifier.add(i.copy()); 1537 } 1538 ; 1539 if (basedOn != null) { 1540 dst.basedOn = new ArrayList<Reference>(); 1541 for (Reference i : basedOn) 1542 dst.basedOn.add(i.copy()); 1543 } 1544 ; 1545 if (partOf != null) { 1546 dst.partOf = new ArrayList<Reference>(); 1547 for (Reference i : partOf) 1548 dst.partOf.add(i.copy()); 1549 } 1550 ; 1551 dst.status = status == null ? null : status.copy(); 1552 dst.patient = patient == null ? null : patient.copy(); 1553 dst.type = type == null ? null : type.copy(); 1554 dst.suppliedItem = suppliedItem == null ? null : suppliedItem.copy(); 1555 dst.occurrence = occurrence == null ? null : occurrence.copy(); 1556 dst.supplier = supplier == null ? null : supplier.copy(); 1557 dst.destination = destination == null ? null : destination.copy(); 1558 if (receiver != null) { 1559 dst.receiver = new ArrayList<Reference>(); 1560 for (Reference i : receiver) 1561 dst.receiver.add(i.copy()); 1562 } 1563 ; 1564 } 1565 1566 protected SupplyDelivery typedCopy() { 1567 return copy(); 1568 } 1569 1570 @Override 1571 public boolean equalsDeep(Base other_) { 1572 if (!super.equalsDeep(other_)) 1573 return false; 1574 if (!(other_ instanceof SupplyDelivery)) 1575 return false; 1576 SupplyDelivery o = (SupplyDelivery) other_; 1577 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) 1578 && compareDeep(partOf, o.partOf, true) && compareDeep(status, o.status, true) 1579 && compareDeep(patient, o.patient, true) && compareDeep(type, o.type, true) 1580 && compareDeep(suppliedItem, o.suppliedItem, true) && compareDeep(occurrence, o.occurrence, true) 1581 && compareDeep(supplier, o.supplier, true) && compareDeep(destination, o.destination, true) 1582 && compareDeep(receiver, o.receiver, true); 1583 } 1584 1585 @Override 1586 public boolean equalsShallow(Base other_) { 1587 if (!super.equalsShallow(other_)) 1588 return false; 1589 if (!(other_ instanceof SupplyDelivery)) 1590 return false; 1591 SupplyDelivery o = (SupplyDelivery) other_; 1592 return compareValues(status, o.status, true); 1593 } 1594 1595 public boolean isEmpty() { 1596 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, partOf, status, patient, type, 1597 suppliedItem, occurrence, supplier, destination, receiver); 1598 } 1599 1600 @Override 1601 public ResourceType getResourceType() { 1602 return ResourceType.SupplyDelivery; 1603 } 1604 1605 /** 1606 * Search parameter: <b>identifier</b> 1607 * <p> 1608 * Description: <b>External identifier</b><br> 1609 * Type: <b>token</b><br> 1610 * Path: <b>SupplyDelivery.identifier</b><br> 1611 * </p> 1612 */ 1613 @SearchParamDefinition(name = "identifier", path = "SupplyDelivery.identifier", description = "External identifier", type = "token") 1614 public static final String SP_IDENTIFIER = "identifier"; 1615 /** 1616 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1617 * <p> 1618 * Description: <b>External identifier</b><br> 1619 * Type: <b>token</b><br> 1620 * Path: <b>SupplyDelivery.identifier</b><br> 1621 * </p> 1622 */ 1623 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1624 SP_IDENTIFIER); 1625 1626 /** 1627 * Search parameter: <b>receiver</b> 1628 * <p> 1629 * Description: <b>Who collected the Supply</b><br> 1630 * Type: <b>reference</b><br> 1631 * Path: <b>SupplyDelivery.receiver</b><br> 1632 * </p> 1633 */ 1634 @SearchParamDefinition(name = "receiver", path = "SupplyDelivery.receiver", description = "Who collected the Supply", type = "reference", providesMembershipIn = { 1635 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Practitioner.class, 1636 PractitionerRole.class }) 1637 public static final String SP_RECEIVER = "receiver"; 1638 /** 1639 * <b>Fluent Client</b> search parameter constant for <b>receiver</b> 1640 * <p> 1641 * Description: <b>Who collected the Supply</b><br> 1642 * Type: <b>reference</b><br> 1643 * Path: <b>SupplyDelivery.receiver</b><br> 1644 * </p> 1645 */ 1646 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECEIVER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1647 SP_RECEIVER); 1648 1649 /** 1650 * Constant for fluent queries to be used to add include statements. Specifies 1651 * the path value of "<b>SupplyDelivery:receiver</b>". 1652 */ 1653 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECEIVER = new ca.uhn.fhir.model.api.Include( 1654 "SupplyDelivery:receiver").toLocked(); 1655 1656 /** 1657 * Search parameter: <b>patient</b> 1658 * <p> 1659 * Description: <b>Patient for whom the item is supplied</b><br> 1660 * Type: <b>reference</b><br> 1661 * Path: <b>SupplyDelivery.patient</b><br> 1662 * </p> 1663 */ 1664 @SearchParamDefinition(name = "patient", path = "SupplyDelivery.patient", description = "Patient for whom the item is supplied", type = "reference", providesMembershipIn = { 1665 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 1666 public static final String SP_PATIENT = "patient"; 1667 /** 1668 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1669 * <p> 1670 * Description: <b>Patient for whom the item is supplied</b><br> 1671 * Type: <b>reference</b><br> 1672 * Path: <b>SupplyDelivery.patient</b><br> 1673 * </p> 1674 */ 1675 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1676 SP_PATIENT); 1677 1678 /** 1679 * Constant for fluent queries to be used to add include statements. Specifies 1680 * the path value of "<b>SupplyDelivery:patient</b>". 1681 */ 1682 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 1683 "SupplyDelivery:patient").toLocked(); 1684 1685 /** 1686 * Search parameter: <b>supplier</b> 1687 * <p> 1688 * Description: <b>Dispenser</b><br> 1689 * Type: <b>reference</b><br> 1690 * Path: <b>SupplyDelivery.supplier</b><br> 1691 * </p> 1692 */ 1693 @SearchParamDefinition(name = "supplier", path = "SupplyDelivery.supplier", description = "Dispenser", type = "reference", providesMembershipIn = { 1694 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Organization.class, 1695 Practitioner.class, PractitionerRole.class }) 1696 public static final String SP_SUPPLIER = "supplier"; 1697 /** 1698 * <b>Fluent Client</b> search parameter constant for <b>supplier</b> 1699 * <p> 1700 * Description: <b>Dispenser</b><br> 1701 * Type: <b>reference</b><br> 1702 * Path: <b>SupplyDelivery.supplier</b><br> 1703 * </p> 1704 */ 1705 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUPPLIER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1706 SP_SUPPLIER); 1707 1708 /** 1709 * Constant for fluent queries to be used to add include statements. Specifies 1710 * the path value of "<b>SupplyDelivery:supplier</b>". 1711 */ 1712 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUPPLIER = new ca.uhn.fhir.model.api.Include( 1713 "SupplyDelivery:supplier").toLocked(); 1714 1715 /** 1716 * Search parameter: <b>status</b> 1717 * <p> 1718 * Description: <b>in-progress | completed | abandoned | 1719 * entered-in-error</b><br> 1720 * Type: <b>token</b><br> 1721 * Path: <b>SupplyDelivery.status</b><br> 1722 * </p> 1723 */ 1724 @SearchParamDefinition(name = "status", path = "SupplyDelivery.status", description = "in-progress | completed | abandoned | entered-in-error", type = "token") 1725 public static final String SP_STATUS = "status"; 1726 /** 1727 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1728 * <p> 1729 * Description: <b>in-progress | completed | abandoned | 1730 * entered-in-error</b><br> 1731 * Type: <b>token</b><br> 1732 * Path: <b>SupplyDelivery.status</b><br> 1733 * </p> 1734 */ 1735 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1736 SP_STATUS); 1737 1738}