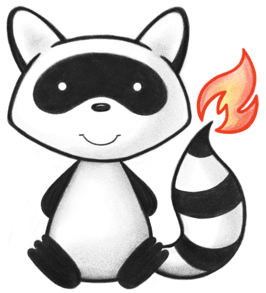
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * A record of a request for a medication, substance or device used in the 048 * healthcare setting. 049 */ 050@ResourceDef(name = "SupplyRequest", profile = "http://hl7.org/fhir/StructureDefinition/SupplyRequest") 051public class SupplyRequest extends DomainResource { 052 053 public enum SupplyRequestStatus { 054 /** 055 * The request has been created but is not yet complete or ready for action. 056 */ 057 DRAFT, 058 /** 059 * The request is ready to be acted upon. 060 */ 061 ACTIVE, 062 /** 063 * The authorization/request to act has been temporarily withdrawn but is 064 * expected to resume in the future. 065 */ 066 SUSPENDED, 067 /** 068 * The authorization/request to act has been terminated prior to the full 069 * completion of the intended actions. No further activity should occur. 070 */ 071 CANCELLED, 072 /** 073 * Activity against the request has been sufficiently completed to the 074 * satisfaction of the requester. 075 */ 076 COMPLETED, 077 /** 078 * This electronic record should never have existed, though it is possible that 079 * real-world decisions were based on it. (If real-world activity has occurred, 080 * the status should be "cancelled" rather than "entered-in-error".). 081 */ 082 ENTEREDINERROR, 083 /** 084 * The authoring/source system does not know which of the status values 085 * currently applies for this observation. Note: This concept is not to be used 086 * for "other" - one of the listed statuses is presumed to apply, but the 087 * authoring/source system does not know which. 088 */ 089 UNKNOWN, 090 /** 091 * added to help the parsers with the generic types 092 */ 093 NULL; 094 095 public static SupplyRequestStatus fromCode(String codeString) throws FHIRException { 096 if (codeString == null || "".equals(codeString)) 097 return null; 098 if ("draft".equals(codeString)) 099 return DRAFT; 100 if ("active".equals(codeString)) 101 return ACTIVE; 102 if ("suspended".equals(codeString)) 103 return SUSPENDED; 104 if ("cancelled".equals(codeString)) 105 return CANCELLED; 106 if ("completed".equals(codeString)) 107 return COMPLETED; 108 if ("entered-in-error".equals(codeString)) 109 return ENTEREDINERROR; 110 if ("unknown".equals(codeString)) 111 return UNKNOWN; 112 if (Configuration.isAcceptInvalidEnums()) 113 return null; 114 else 115 throw new FHIRException("Unknown SupplyRequestStatus code '" + codeString + "'"); 116 } 117 118 public String toCode() { 119 switch (this) { 120 case DRAFT: 121 return "draft"; 122 case ACTIVE: 123 return "active"; 124 case SUSPENDED: 125 return "suspended"; 126 case CANCELLED: 127 return "cancelled"; 128 case COMPLETED: 129 return "completed"; 130 case ENTEREDINERROR: 131 return "entered-in-error"; 132 case UNKNOWN: 133 return "unknown"; 134 case NULL: 135 return null; 136 default: 137 return "?"; 138 } 139 } 140 141 public String getSystem() { 142 switch (this) { 143 case DRAFT: 144 return "http://hl7.org/fhir/supplyrequest-status"; 145 case ACTIVE: 146 return "http://hl7.org/fhir/supplyrequest-status"; 147 case SUSPENDED: 148 return "http://hl7.org/fhir/supplyrequest-status"; 149 case CANCELLED: 150 return "http://hl7.org/fhir/supplyrequest-status"; 151 case COMPLETED: 152 return "http://hl7.org/fhir/supplyrequest-status"; 153 case ENTEREDINERROR: 154 return "http://hl7.org/fhir/supplyrequest-status"; 155 case UNKNOWN: 156 return "http://hl7.org/fhir/supplyrequest-status"; 157 case NULL: 158 return null; 159 default: 160 return "?"; 161 } 162 } 163 164 public String getDefinition() { 165 switch (this) { 166 case DRAFT: 167 return "The request has been created but is not yet complete or ready for action."; 168 case ACTIVE: 169 return "The request is ready to be acted upon."; 170 case SUSPENDED: 171 return "The authorization/request to act has been temporarily withdrawn but is expected to resume in the future."; 172 case CANCELLED: 173 return "The authorization/request to act has been terminated prior to the full completion of the intended actions. No further activity should occur."; 174 case COMPLETED: 175 return "Activity against the request has been sufficiently completed to the satisfaction of the requester."; 176 case ENTEREDINERROR: 177 return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)."; 178 case UNKNOWN: 179 return "The authoring/source system does not know which of the status values currently applies for this observation. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 180 case NULL: 181 return null; 182 default: 183 return "?"; 184 } 185 } 186 187 public String getDisplay() { 188 switch (this) { 189 case DRAFT: 190 return "Draft"; 191 case ACTIVE: 192 return "Active"; 193 case SUSPENDED: 194 return "Suspended"; 195 case CANCELLED: 196 return "Cancelled"; 197 case COMPLETED: 198 return "Completed"; 199 case ENTEREDINERROR: 200 return "Entered in Error"; 201 case UNKNOWN: 202 return "Unknown"; 203 case NULL: 204 return null; 205 default: 206 return "?"; 207 } 208 } 209 } 210 211 public static class SupplyRequestStatusEnumFactory implements EnumFactory<SupplyRequestStatus> { 212 public SupplyRequestStatus fromCode(String codeString) throws IllegalArgumentException { 213 if (codeString == null || "".equals(codeString)) 214 if (codeString == null || "".equals(codeString)) 215 return null; 216 if ("draft".equals(codeString)) 217 return SupplyRequestStatus.DRAFT; 218 if ("active".equals(codeString)) 219 return SupplyRequestStatus.ACTIVE; 220 if ("suspended".equals(codeString)) 221 return SupplyRequestStatus.SUSPENDED; 222 if ("cancelled".equals(codeString)) 223 return SupplyRequestStatus.CANCELLED; 224 if ("completed".equals(codeString)) 225 return SupplyRequestStatus.COMPLETED; 226 if ("entered-in-error".equals(codeString)) 227 return SupplyRequestStatus.ENTEREDINERROR; 228 if ("unknown".equals(codeString)) 229 return SupplyRequestStatus.UNKNOWN; 230 throw new IllegalArgumentException("Unknown SupplyRequestStatus code '" + codeString + "'"); 231 } 232 233 public Enumeration<SupplyRequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 234 if (code == null) 235 return null; 236 if (code.isEmpty()) 237 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.NULL, code); 238 String codeString = code.asStringValue(); 239 if (codeString == null || "".equals(codeString)) 240 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.NULL, code); 241 if ("draft".equals(codeString)) 242 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.DRAFT, code); 243 if ("active".equals(codeString)) 244 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.ACTIVE, code); 245 if ("suspended".equals(codeString)) 246 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.SUSPENDED, code); 247 if ("cancelled".equals(codeString)) 248 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.CANCELLED, code); 249 if ("completed".equals(codeString)) 250 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.COMPLETED, code); 251 if ("entered-in-error".equals(codeString)) 252 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.ENTEREDINERROR, code); 253 if ("unknown".equals(codeString)) 254 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.UNKNOWN, code); 255 throw new FHIRException("Unknown SupplyRequestStatus code '" + codeString + "'"); 256 } 257 258 public String toCode(SupplyRequestStatus code) { 259 if (code == SupplyRequestStatus.NULL) 260 return null; 261 if (code == SupplyRequestStatus.DRAFT) 262 return "draft"; 263 if (code == SupplyRequestStatus.ACTIVE) 264 return "active"; 265 if (code == SupplyRequestStatus.SUSPENDED) 266 return "suspended"; 267 if (code == SupplyRequestStatus.CANCELLED) 268 return "cancelled"; 269 if (code == SupplyRequestStatus.COMPLETED) 270 return "completed"; 271 if (code == SupplyRequestStatus.ENTEREDINERROR) 272 return "entered-in-error"; 273 if (code == SupplyRequestStatus.UNKNOWN) 274 return "unknown"; 275 return "?"; 276 } 277 278 public String toSystem(SupplyRequestStatus code) { 279 return code.getSystem(); 280 } 281 } 282 283 public enum RequestPriority { 284 /** 285 * The request has normal priority. 286 */ 287 ROUTINE, 288 /** 289 * The request should be actioned promptly - higher priority than routine. 290 */ 291 URGENT, 292 /** 293 * The request should be actioned as soon as possible - higher priority than 294 * urgent. 295 */ 296 ASAP, 297 /** 298 * The request should be actioned immediately - highest possible priority. E.g. 299 * an emergency. 300 */ 301 STAT, 302 /** 303 * added to help the parsers with the generic types 304 */ 305 NULL; 306 307 public static RequestPriority fromCode(String codeString) throws FHIRException { 308 if (codeString == null || "".equals(codeString)) 309 return null; 310 if ("routine".equals(codeString)) 311 return ROUTINE; 312 if ("urgent".equals(codeString)) 313 return URGENT; 314 if ("asap".equals(codeString)) 315 return ASAP; 316 if ("stat".equals(codeString)) 317 return STAT; 318 if (Configuration.isAcceptInvalidEnums()) 319 return null; 320 else 321 throw new FHIRException("Unknown RequestPriority code '" + codeString + "'"); 322 } 323 324 public String toCode() { 325 switch (this) { 326 case ROUTINE: 327 return "routine"; 328 case URGENT: 329 return "urgent"; 330 case ASAP: 331 return "asap"; 332 case STAT: 333 return "stat"; 334 case NULL: 335 return null; 336 default: 337 return "?"; 338 } 339 } 340 341 public String getSystem() { 342 switch (this) { 343 case ROUTINE: 344 return "http://hl7.org/fhir/request-priority"; 345 case URGENT: 346 return "http://hl7.org/fhir/request-priority"; 347 case ASAP: 348 return "http://hl7.org/fhir/request-priority"; 349 case STAT: 350 return "http://hl7.org/fhir/request-priority"; 351 case NULL: 352 return null; 353 default: 354 return "?"; 355 } 356 } 357 358 public String getDefinition() { 359 switch (this) { 360 case ROUTINE: 361 return "The request has normal priority."; 362 case URGENT: 363 return "The request should be actioned promptly - higher priority than routine."; 364 case ASAP: 365 return "The request should be actioned as soon as possible - higher priority than urgent."; 366 case STAT: 367 return "The request should be actioned immediately - highest possible priority. E.g. an emergency."; 368 case NULL: 369 return null; 370 default: 371 return "?"; 372 } 373 } 374 375 public String getDisplay() { 376 switch (this) { 377 case ROUTINE: 378 return "Routine"; 379 case URGENT: 380 return "Urgent"; 381 case ASAP: 382 return "ASAP"; 383 case STAT: 384 return "STAT"; 385 case NULL: 386 return null; 387 default: 388 return "?"; 389 } 390 } 391 } 392 393 public static class RequestPriorityEnumFactory implements EnumFactory<RequestPriority> { 394 public RequestPriority fromCode(String codeString) throws IllegalArgumentException { 395 if (codeString == null || "".equals(codeString)) 396 if (codeString == null || "".equals(codeString)) 397 return null; 398 if ("routine".equals(codeString)) 399 return RequestPriority.ROUTINE; 400 if ("urgent".equals(codeString)) 401 return RequestPriority.URGENT; 402 if ("asap".equals(codeString)) 403 return RequestPriority.ASAP; 404 if ("stat".equals(codeString)) 405 return RequestPriority.STAT; 406 throw new IllegalArgumentException("Unknown RequestPriority code '" + codeString + "'"); 407 } 408 409 public Enumeration<RequestPriority> fromType(PrimitiveType<?> code) throws FHIRException { 410 if (code == null) 411 return null; 412 if (code.isEmpty()) 413 return new Enumeration<RequestPriority>(this, RequestPriority.NULL, code); 414 String codeString = code.asStringValue(); 415 if (codeString == null || "".equals(codeString)) 416 return new Enumeration<RequestPriority>(this, RequestPriority.NULL, code); 417 if ("routine".equals(codeString)) 418 return new Enumeration<RequestPriority>(this, RequestPriority.ROUTINE, code); 419 if ("urgent".equals(codeString)) 420 return new Enumeration<RequestPriority>(this, RequestPriority.URGENT, code); 421 if ("asap".equals(codeString)) 422 return new Enumeration<RequestPriority>(this, RequestPriority.ASAP, code); 423 if ("stat".equals(codeString)) 424 return new Enumeration<RequestPriority>(this, RequestPriority.STAT, code); 425 throw new FHIRException("Unknown RequestPriority code '" + codeString + "'"); 426 } 427 428 public String toCode(RequestPriority code) { 429 if (code == RequestPriority.NULL) 430 return null; 431 if (code == RequestPriority.ROUTINE) 432 return "routine"; 433 if (code == RequestPriority.URGENT) 434 return "urgent"; 435 if (code == RequestPriority.ASAP) 436 return "asap"; 437 if (code == RequestPriority.STAT) 438 return "stat"; 439 return "?"; 440 } 441 442 public String toSystem(RequestPriority code) { 443 return code.getSystem(); 444 } 445 } 446 447 @Block() 448 public static class SupplyRequestParameterComponent extends BackboneElement implements IBaseBackboneElement { 449 /** 450 * A code or string that identifies the device detail being asserted. 451 */ 452 @Child(name = "code", type = { 453 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 454 @Description(shortDefinition = "Item detail", formalDefinition = "A code or string that identifies the device detail being asserted.") 455 protected CodeableConcept code; 456 457 /** 458 * The value of the device detail. 459 */ 460 @Child(name = "value", type = { CodeableConcept.class, Quantity.class, Range.class, 461 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 462 @Description(shortDefinition = "Value of detail", formalDefinition = "The value of the device detail.") 463 protected Type value; 464 465 private static final long serialVersionUID = 884525025L; 466 467 /** 468 * Constructor 469 */ 470 public SupplyRequestParameterComponent() { 471 super(); 472 } 473 474 /** 475 * @return {@link #code} (A code or string that identifies the device detail 476 * being asserted.) 477 */ 478 public CodeableConcept getCode() { 479 if (this.code == null) 480 if (Configuration.errorOnAutoCreate()) 481 throw new Error("Attempt to auto-create SupplyRequestParameterComponent.code"); 482 else if (Configuration.doAutoCreate()) 483 this.code = new CodeableConcept(); // cc 484 return this.code; 485 } 486 487 public boolean hasCode() { 488 return this.code != null && !this.code.isEmpty(); 489 } 490 491 /** 492 * @param value {@link #code} (A code or string that identifies the device 493 * detail being asserted.) 494 */ 495 public SupplyRequestParameterComponent setCode(CodeableConcept value) { 496 this.code = value; 497 return this; 498 } 499 500 /** 501 * @return {@link #value} (The value of the device detail.) 502 */ 503 public Type getValue() { 504 return this.value; 505 } 506 507 /** 508 * @return {@link #value} (The value of the device detail.) 509 */ 510 public CodeableConcept getValueCodeableConcept() throws FHIRException { 511 if (this.value == null) 512 this.value = new CodeableConcept(); 513 if (!(this.value instanceof CodeableConcept)) 514 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 515 + this.value.getClass().getName() + " was encountered"); 516 return (CodeableConcept) this.value; 517 } 518 519 public boolean hasValueCodeableConcept() { 520 return this.value instanceof CodeableConcept; 521 } 522 523 /** 524 * @return {@link #value} (The value of the device detail.) 525 */ 526 public Quantity getValueQuantity() throws FHIRException { 527 if (this.value == null) 528 this.value = new Quantity(); 529 if (!(this.value instanceof Quantity)) 530 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.value.getClass().getName() 531 + " was encountered"); 532 return (Quantity) this.value; 533 } 534 535 public boolean hasValueQuantity() { 536 return this.value instanceof Quantity; 537 } 538 539 /** 540 * @return {@link #value} (The value of the device detail.) 541 */ 542 public Range getValueRange() throws FHIRException { 543 if (this.value == null) 544 this.value = new Range(); 545 if (!(this.value instanceof Range)) 546 throw new FHIRException( 547 "Type mismatch: the type Range was expected, but " + this.value.getClass().getName() + " was encountered"); 548 return (Range) this.value; 549 } 550 551 public boolean hasValueRange() { 552 return this.value instanceof Range; 553 } 554 555 /** 556 * @return {@link #value} (The value of the device detail.) 557 */ 558 public BooleanType getValueBooleanType() throws FHIRException { 559 if (this.value == null) 560 this.value = new BooleanType(); 561 if (!(this.value instanceof BooleanType)) 562 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 563 + this.value.getClass().getName() + " was encountered"); 564 return (BooleanType) this.value; 565 } 566 567 public boolean hasValueBooleanType() { 568 return this.value instanceof BooleanType; 569 } 570 571 public boolean hasValue() { 572 return this.value != null && !this.value.isEmpty(); 573 } 574 575 /** 576 * @param value {@link #value} (The value of the device detail.) 577 */ 578 public SupplyRequestParameterComponent setValue(Type value) { 579 if (value != null && !(value instanceof CodeableConcept || value instanceof Quantity || value instanceof Range 580 || value instanceof BooleanType)) 581 throw new Error("Not the right type for SupplyRequest.parameter.value[x]: " + value.fhirType()); 582 this.value = value; 583 return this; 584 } 585 586 protected void listChildren(List<Property> children) { 587 super.listChildren(children); 588 children.add(new Property("code", "CodeableConcept", 589 "A code or string that identifies the device detail being asserted.", 0, 1, code)); 590 children.add(new Property("value[x]", "CodeableConcept|Quantity|Range|boolean", "The value of the device detail.", 591 0, 1, value)); 592 } 593 594 @Override 595 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 596 switch (_hash) { 597 case 3059181: 598 /* code */ return new Property("code", "CodeableConcept", 599 "A code or string that identifies the device detail being asserted.", 0, 1, code); 600 case -1410166417: 601 /* value[x] */ return new Property("value[x]", "CodeableConcept|Quantity|Range|boolean", 602 "The value of the device detail.", 0, 1, value); 603 case 111972721: 604 /* value */ return new Property("value[x]", "CodeableConcept|Quantity|Range|boolean", 605 "The value of the device detail.", 0, 1, value); 606 case 924902896: 607 /* valueCodeableConcept */ return new Property("value[x]", "CodeableConcept|Quantity|Range|boolean", 608 "The value of the device detail.", 0, 1, value); 609 case -2029823716: 610 /* valueQuantity */ return new Property("value[x]", "CodeableConcept|Quantity|Range|boolean", 611 "The value of the device detail.", 0, 1, value); 612 case 2030761548: 613 /* valueRange */ return new Property("value[x]", "CodeableConcept|Quantity|Range|boolean", 614 "The value of the device detail.", 0, 1, value); 615 case 733421943: 616 /* valueBoolean */ return new Property("value[x]", "CodeableConcept|Quantity|Range|boolean", 617 "The value of the device detail.", 0, 1, value); 618 default: 619 return super.getNamedProperty(_hash, _name, _checkValid); 620 } 621 622 } 623 624 @Override 625 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 626 switch (hash) { 627 case 3059181: 628 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 629 case 111972721: 630 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 631 default: 632 return super.getProperty(hash, name, checkValid); 633 } 634 635 } 636 637 @Override 638 public Base setProperty(int hash, String name, Base value) throws FHIRException { 639 switch (hash) { 640 case 3059181: // code 641 this.code = castToCodeableConcept(value); // CodeableConcept 642 return value; 643 case 111972721: // value 644 this.value = castToType(value); // Type 645 return value; 646 default: 647 return super.setProperty(hash, name, value); 648 } 649 650 } 651 652 @Override 653 public Base setProperty(String name, Base value) throws FHIRException { 654 if (name.equals("code")) { 655 this.code = castToCodeableConcept(value); // CodeableConcept 656 } else if (name.equals("value[x]")) { 657 this.value = castToType(value); // Type 658 } else 659 return super.setProperty(name, value); 660 return value; 661 } 662 663 @Override 664 public void removeChild(String name, Base value) throws FHIRException { 665 if (name.equals("code")) { 666 this.code = null; 667 } else if (name.equals("value[x]")) { 668 this.value = null; 669 } else 670 super.removeChild(name, value); 671 672 } 673 674 @Override 675 public Base makeProperty(int hash, String name) throws FHIRException { 676 switch (hash) { 677 case 3059181: 678 return getCode(); 679 case -1410166417: 680 return getValue(); 681 case 111972721: 682 return getValue(); 683 default: 684 return super.makeProperty(hash, name); 685 } 686 687 } 688 689 @Override 690 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 691 switch (hash) { 692 case 3059181: 693 /* code */ return new String[] { "CodeableConcept" }; 694 case 111972721: 695 /* value */ return new String[] { "CodeableConcept", "Quantity", "Range", "boolean" }; 696 default: 697 return super.getTypesForProperty(hash, name); 698 } 699 700 } 701 702 @Override 703 public Base addChild(String name) throws FHIRException { 704 if (name.equals("code")) { 705 this.code = new CodeableConcept(); 706 return this.code; 707 } else if (name.equals("valueCodeableConcept")) { 708 this.value = new CodeableConcept(); 709 return this.value; 710 } else if (name.equals("valueQuantity")) { 711 this.value = new Quantity(); 712 return this.value; 713 } else if (name.equals("valueRange")) { 714 this.value = new Range(); 715 return this.value; 716 } else if (name.equals("valueBoolean")) { 717 this.value = new BooleanType(); 718 return this.value; 719 } else 720 return super.addChild(name); 721 } 722 723 public SupplyRequestParameterComponent copy() { 724 SupplyRequestParameterComponent dst = new SupplyRequestParameterComponent(); 725 copyValues(dst); 726 return dst; 727 } 728 729 public void copyValues(SupplyRequestParameterComponent dst) { 730 super.copyValues(dst); 731 dst.code = code == null ? null : code.copy(); 732 dst.value = value == null ? null : value.copy(); 733 } 734 735 @Override 736 public boolean equalsDeep(Base other_) { 737 if (!super.equalsDeep(other_)) 738 return false; 739 if (!(other_ instanceof SupplyRequestParameterComponent)) 740 return false; 741 SupplyRequestParameterComponent o = (SupplyRequestParameterComponent) other_; 742 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true); 743 } 744 745 @Override 746 public boolean equalsShallow(Base other_) { 747 if (!super.equalsShallow(other_)) 748 return false; 749 if (!(other_ instanceof SupplyRequestParameterComponent)) 750 return false; 751 SupplyRequestParameterComponent o = (SupplyRequestParameterComponent) other_; 752 return true; 753 } 754 755 public boolean isEmpty() { 756 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value); 757 } 758 759 public String fhirType() { 760 return "SupplyRequest.parameter"; 761 762 } 763 764 } 765 766 /** 767 * Business identifiers assigned to this SupplyRequest by the author and/or 768 * other systems. These identifiers remain constant as the resource is updated 769 * and propagates from server to server. 770 */ 771 @Child(name = "identifier", type = { 772 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 773 @Description(shortDefinition = "Business Identifier for SupplyRequest", formalDefinition = "Business identifiers assigned to this SupplyRequest by the author and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.") 774 protected List<Identifier> identifier; 775 776 /** 777 * Status of the supply request. 778 */ 779 @Child(name = "status", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 780 @Description(shortDefinition = "draft | active | suspended +", formalDefinition = "Status of the supply request.") 781 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/supplyrequest-status") 782 protected Enumeration<SupplyRequestStatus> status; 783 784 /** 785 * Category of supply, e.g. central, non-stock, etc. This is used to support 786 * work flows associated with the supply process. 787 */ 788 @Child(name = "category", type = { 789 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 790 @Description(shortDefinition = "The kind of supply (central, non-stock, etc.)", formalDefinition = "Category of supply, e.g. central, non-stock, etc. This is used to support work flows associated with the supply process.") 791 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/supplyrequest-kind") 792 protected CodeableConcept category; 793 794 /** 795 * Indicates how quickly this SupplyRequest should be addressed with respect to 796 * other requests. 797 */ 798 @Child(name = "priority", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 799 @Description(shortDefinition = "routine | urgent | asap | stat", formalDefinition = "Indicates how quickly this SupplyRequest should be addressed with respect to other requests.") 800 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-priority") 801 protected Enumeration<RequestPriority> priority; 802 803 /** 804 * The item that is requested to be supplied. This is either a link to a 805 * resource representing the details of the item or a code that identifies the 806 * item from a known list. 807 */ 808 @Child(name = "item", type = { CodeableConcept.class, Medication.class, Substance.class, 809 Device.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 810 @Description(shortDefinition = "Medication, Substance, or Device requested to be supplied", formalDefinition = "The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.") 811 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/supply-item") 812 protected Type item; 813 814 /** 815 * The amount that is being ordered of the indicated item. 816 */ 817 @Child(name = "quantity", type = { Quantity.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 818 @Description(shortDefinition = "The requested amount of the item indicated", formalDefinition = "The amount that is being ordered of the indicated item.") 819 protected Quantity quantity; 820 821 /** 822 * Specific parameters for the ordered item. For example, the size of the 823 * indicated item. 824 */ 825 @Child(name = "parameter", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 826 @Description(shortDefinition = "Ordered item details", formalDefinition = "Specific parameters for the ordered item. For example, the size of the indicated item.") 827 protected List<SupplyRequestParameterComponent> parameter; 828 829 /** 830 * When the request should be fulfilled. 831 */ 832 @Child(name = "occurrence", type = { DateTimeType.class, Period.class, 833 Timing.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 834 @Description(shortDefinition = "When the request should be fulfilled", formalDefinition = "When the request should be fulfilled.") 835 protected Type occurrence; 836 837 /** 838 * When the request was made. 839 */ 840 @Child(name = "authoredOn", type = { 841 DateTimeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 842 @Description(shortDefinition = "When the request was made", formalDefinition = "When the request was made.") 843 protected DateTimeType authoredOn; 844 845 /** 846 * The device, practitioner, etc. who initiated the request. 847 */ 848 @Child(name = "requester", type = { Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, 849 RelatedPerson.class, Device.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 850 @Description(shortDefinition = "Individual making the request", formalDefinition = "The device, practitioner, etc. who initiated the request.") 851 protected Reference requester; 852 853 /** 854 * The actual object that is the target of the reference (The device, 855 * practitioner, etc. who initiated the request.) 856 */ 857 protected Resource requesterTarget; 858 859 /** 860 * Who is intended to fulfill the request. 861 */ 862 @Child(name = "supplier", type = { Organization.class, 863 HealthcareService.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 864 @Description(shortDefinition = "Who is intended to fulfill the request", formalDefinition = "Who is intended to fulfill the request.") 865 protected List<Reference> supplier; 866 /** 867 * The actual objects that are the target of the reference (Who is intended to 868 * fulfill the request.) 869 */ 870 protected List<Resource> supplierTarget; 871 872 /** 873 * The reason why the supply item was requested. 874 */ 875 @Child(name = "reasonCode", type = { 876 CodeableConcept.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 877 @Description(shortDefinition = "The reason why the supply item was requested", formalDefinition = "The reason why the supply item was requested.") 878 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/supplyrequest-reason") 879 protected List<CodeableConcept> reasonCode; 880 881 /** 882 * The reason why the supply item was requested. 883 */ 884 @Child(name = "reasonReference", type = { Condition.class, Observation.class, DiagnosticReport.class, 885 DocumentReference.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 886 @Description(shortDefinition = "The reason why the supply item was requested", formalDefinition = "The reason why the supply item was requested.") 887 protected List<Reference> reasonReference; 888 /** 889 * The actual objects that are the target of the reference (The reason why the 890 * supply item was requested.) 891 */ 892 protected List<Resource> reasonReferenceTarget; 893 894 /** 895 * Where the supply is expected to come from. 896 */ 897 @Child(name = "deliverFrom", type = { Organization.class, 898 Location.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 899 @Description(shortDefinition = "The origin of the supply", formalDefinition = "Where the supply is expected to come from.") 900 protected Reference deliverFrom; 901 902 /** 903 * The actual object that is the target of the reference (Where the supply is 904 * expected to come from.) 905 */ 906 protected Resource deliverFromTarget; 907 908 /** 909 * Where the supply is destined to go. 910 */ 911 @Child(name = "deliverTo", type = { Organization.class, Location.class, 912 Patient.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 913 @Description(shortDefinition = "The destination of the supply", formalDefinition = "Where the supply is destined to go.") 914 protected Reference deliverTo; 915 916 /** 917 * The actual object that is the target of the reference (Where the supply is 918 * destined to go.) 919 */ 920 protected Resource deliverToTarget; 921 922 private static final long serialVersionUID = 1456312151L; 923 924 /** 925 * Constructor 926 */ 927 public SupplyRequest() { 928 super(); 929 } 930 931 /** 932 * Constructor 933 */ 934 public SupplyRequest(Type item, Quantity quantity) { 935 super(); 936 this.item = item; 937 this.quantity = quantity; 938 } 939 940 /** 941 * @return {@link #identifier} (Business identifiers assigned to this 942 * SupplyRequest by the author and/or other systems. These identifiers 943 * remain constant as the resource is updated and propagates from server 944 * to server.) 945 */ 946 public List<Identifier> getIdentifier() { 947 if (this.identifier == null) 948 this.identifier = new ArrayList<Identifier>(); 949 return this.identifier; 950 } 951 952 /** 953 * @return Returns a reference to <code>this</code> for easy method chaining 954 */ 955 public SupplyRequest setIdentifier(List<Identifier> theIdentifier) { 956 this.identifier = theIdentifier; 957 return this; 958 } 959 960 public boolean hasIdentifier() { 961 if (this.identifier == null) 962 return false; 963 for (Identifier item : this.identifier) 964 if (!item.isEmpty()) 965 return true; 966 return false; 967 } 968 969 public Identifier addIdentifier() { // 3 970 Identifier t = new Identifier(); 971 if (this.identifier == null) 972 this.identifier = new ArrayList<Identifier>(); 973 this.identifier.add(t); 974 return t; 975 } 976 977 public SupplyRequest addIdentifier(Identifier t) { // 3 978 if (t == null) 979 return this; 980 if (this.identifier == null) 981 this.identifier = new ArrayList<Identifier>(); 982 this.identifier.add(t); 983 return this; 984 } 985 986 /** 987 * @return The first repetition of repeating field {@link #identifier}, creating 988 * it if it does not already exist 989 */ 990 public Identifier getIdentifierFirstRep() { 991 if (getIdentifier().isEmpty()) { 992 addIdentifier(); 993 } 994 return getIdentifier().get(0); 995 } 996 997 /** 998 * @return {@link #status} (Status of the supply request.). This is the 999 * underlying object with id, value and extensions. The accessor 1000 * "getStatus" gives direct access to the value 1001 */ 1002 public Enumeration<SupplyRequestStatus> getStatusElement() { 1003 if (this.status == null) 1004 if (Configuration.errorOnAutoCreate()) 1005 throw new Error("Attempt to auto-create SupplyRequest.status"); 1006 else if (Configuration.doAutoCreate()) 1007 this.status = new Enumeration<SupplyRequestStatus>(new SupplyRequestStatusEnumFactory()); // bb 1008 return this.status; 1009 } 1010 1011 public boolean hasStatusElement() { 1012 return this.status != null && !this.status.isEmpty(); 1013 } 1014 1015 public boolean hasStatus() { 1016 return this.status != null && !this.status.isEmpty(); 1017 } 1018 1019 /** 1020 * @param value {@link #status} (Status of the supply request.). This is the 1021 * underlying object with id, value and extensions. The accessor 1022 * "getStatus" gives direct access to the value 1023 */ 1024 public SupplyRequest setStatusElement(Enumeration<SupplyRequestStatus> value) { 1025 this.status = value; 1026 return this; 1027 } 1028 1029 /** 1030 * @return Status of the supply request. 1031 */ 1032 public SupplyRequestStatus getStatus() { 1033 return this.status == null ? null : this.status.getValue(); 1034 } 1035 1036 /** 1037 * @param value Status of the supply request. 1038 */ 1039 public SupplyRequest setStatus(SupplyRequestStatus value) { 1040 if (value == null) 1041 this.status = null; 1042 else { 1043 if (this.status == null) 1044 this.status = new Enumeration<SupplyRequestStatus>(new SupplyRequestStatusEnumFactory()); 1045 this.status.setValue(value); 1046 } 1047 return this; 1048 } 1049 1050 /** 1051 * @return {@link #category} (Category of supply, e.g. central, non-stock, etc. 1052 * This is used to support work flows associated with the supply 1053 * process.) 1054 */ 1055 public CodeableConcept getCategory() { 1056 if (this.category == null) 1057 if (Configuration.errorOnAutoCreate()) 1058 throw new Error("Attempt to auto-create SupplyRequest.category"); 1059 else if (Configuration.doAutoCreate()) 1060 this.category = new CodeableConcept(); // cc 1061 return this.category; 1062 } 1063 1064 public boolean hasCategory() { 1065 return this.category != null && !this.category.isEmpty(); 1066 } 1067 1068 /** 1069 * @param value {@link #category} (Category of supply, e.g. central, non-stock, 1070 * etc. This is used to support work flows associated with the 1071 * supply process.) 1072 */ 1073 public SupplyRequest setCategory(CodeableConcept value) { 1074 this.category = value; 1075 return this; 1076 } 1077 1078 /** 1079 * @return {@link #priority} (Indicates how quickly this SupplyRequest should be 1080 * addressed with respect to other requests.). This is the underlying 1081 * object with id, value and extensions. The accessor "getPriority" 1082 * gives direct access to the value 1083 */ 1084 public Enumeration<RequestPriority> getPriorityElement() { 1085 if (this.priority == null) 1086 if (Configuration.errorOnAutoCreate()) 1087 throw new Error("Attempt to auto-create SupplyRequest.priority"); 1088 else if (Configuration.doAutoCreate()) 1089 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 1090 return this.priority; 1091 } 1092 1093 public boolean hasPriorityElement() { 1094 return this.priority != null && !this.priority.isEmpty(); 1095 } 1096 1097 public boolean hasPriority() { 1098 return this.priority != null && !this.priority.isEmpty(); 1099 } 1100 1101 /** 1102 * @param value {@link #priority} (Indicates how quickly this SupplyRequest 1103 * should be addressed with respect to other requests.). This is 1104 * the underlying object with id, value and extensions. The 1105 * accessor "getPriority" gives direct access to the value 1106 */ 1107 public SupplyRequest setPriorityElement(Enumeration<RequestPriority> value) { 1108 this.priority = value; 1109 return this; 1110 } 1111 1112 /** 1113 * @return Indicates how quickly this SupplyRequest should be addressed with 1114 * respect to other requests. 1115 */ 1116 public RequestPriority getPriority() { 1117 return this.priority == null ? null : this.priority.getValue(); 1118 } 1119 1120 /** 1121 * @param value Indicates how quickly this SupplyRequest should be addressed 1122 * with respect to other requests. 1123 */ 1124 public SupplyRequest setPriority(RequestPriority value) { 1125 if (value == null) 1126 this.priority = null; 1127 else { 1128 if (this.priority == null) 1129 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 1130 this.priority.setValue(value); 1131 } 1132 return this; 1133 } 1134 1135 /** 1136 * @return {@link #item} (The item that is requested to be supplied. This is 1137 * either a link to a resource representing the details of the item or a 1138 * code that identifies the item from a known list.) 1139 */ 1140 public Type getItem() { 1141 return this.item; 1142 } 1143 1144 /** 1145 * @return {@link #item} (The item that is requested to be supplied. This is 1146 * either a link to a resource representing the details of the item or a 1147 * code that identifies the item from a known list.) 1148 */ 1149 public CodeableConcept getItemCodeableConcept() throws FHIRException { 1150 if (this.item == null) 1151 this.item = new CodeableConcept(); 1152 if (!(this.item instanceof CodeableConcept)) 1153 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1154 + this.item.getClass().getName() + " was encountered"); 1155 return (CodeableConcept) this.item; 1156 } 1157 1158 public boolean hasItemCodeableConcept() { 1159 return this.item instanceof CodeableConcept; 1160 } 1161 1162 /** 1163 * @return {@link #item} (The item that is requested to be supplied. This is 1164 * either a link to a resource representing the details of the item or a 1165 * code that identifies the item from a known list.) 1166 */ 1167 public Reference getItemReference() throws FHIRException { 1168 if (this.item == null) 1169 this.item = new Reference(); 1170 if (!(this.item instanceof Reference)) 1171 throw new FHIRException( 1172 "Type mismatch: the type Reference was expected, but " + this.item.getClass().getName() + " was encountered"); 1173 return (Reference) this.item; 1174 } 1175 1176 public boolean hasItemReference() { 1177 return this.item instanceof Reference; 1178 } 1179 1180 public boolean hasItem() { 1181 return this.item != null && !this.item.isEmpty(); 1182 } 1183 1184 /** 1185 * @param value {@link #item} (The item that is requested to be supplied. This 1186 * is either a link to a resource representing the details of the 1187 * item or a code that identifies the item from a known list.) 1188 */ 1189 public SupplyRequest setItem(Type value) { 1190 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1191 throw new Error("Not the right type for SupplyRequest.item[x]: " + value.fhirType()); 1192 this.item = value; 1193 return this; 1194 } 1195 1196 /** 1197 * @return {@link #quantity} (The amount that is being ordered of the indicated 1198 * item.) 1199 */ 1200 public Quantity getQuantity() { 1201 if (this.quantity == null) 1202 if (Configuration.errorOnAutoCreate()) 1203 throw new Error("Attempt to auto-create SupplyRequest.quantity"); 1204 else if (Configuration.doAutoCreate()) 1205 this.quantity = new Quantity(); // cc 1206 return this.quantity; 1207 } 1208 1209 public boolean hasQuantity() { 1210 return this.quantity != null && !this.quantity.isEmpty(); 1211 } 1212 1213 /** 1214 * @param value {@link #quantity} (The amount that is being ordered of the 1215 * indicated item.) 1216 */ 1217 public SupplyRequest setQuantity(Quantity value) { 1218 this.quantity = value; 1219 return this; 1220 } 1221 1222 /** 1223 * @return {@link #parameter} (Specific parameters for the ordered item. For 1224 * example, the size of the indicated item.) 1225 */ 1226 public List<SupplyRequestParameterComponent> getParameter() { 1227 if (this.parameter == null) 1228 this.parameter = new ArrayList<SupplyRequestParameterComponent>(); 1229 return this.parameter; 1230 } 1231 1232 /** 1233 * @return Returns a reference to <code>this</code> for easy method chaining 1234 */ 1235 public SupplyRequest setParameter(List<SupplyRequestParameterComponent> theParameter) { 1236 this.parameter = theParameter; 1237 return this; 1238 } 1239 1240 public boolean hasParameter() { 1241 if (this.parameter == null) 1242 return false; 1243 for (SupplyRequestParameterComponent item : this.parameter) 1244 if (!item.isEmpty()) 1245 return true; 1246 return false; 1247 } 1248 1249 public SupplyRequestParameterComponent addParameter() { // 3 1250 SupplyRequestParameterComponent t = new SupplyRequestParameterComponent(); 1251 if (this.parameter == null) 1252 this.parameter = new ArrayList<SupplyRequestParameterComponent>(); 1253 this.parameter.add(t); 1254 return t; 1255 } 1256 1257 public SupplyRequest addParameter(SupplyRequestParameterComponent t) { // 3 1258 if (t == null) 1259 return this; 1260 if (this.parameter == null) 1261 this.parameter = new ArrayList<SupplyRequestParameterComponent>(); 1262 this.parameter.add(t); 1263 return this; 1264 } 1265 1266 /** 1267 * @return The first repetition of repeating field {@link #parameter}, creating 1268 * it if it does not already exist 1269 */ 1270 public SupplyRequestParameterComponent getParameterFirstRep() { 1271 if (getParameter().isEmpty()) { 1272 addParameter(); 1273 } 1274 return getParameter().get(0); 1275 } 1276 1277 /** 1278 * @return {@link #occurrence} (When the request should be fulfilled.) 1279 */ 1280 public Type getOccurrence() { 1281 return this.occurrence; 1282 } 1283 1284 /** 1285 * @return {@link #occurrence} (When the request should be fulfilled.) 1286 */ 1287 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 1288 if (this.occurrence == null) 1289 this.occurrence = new DateTimeType(); 1290 if (!(this.occurrence instanceof DateTimeType)) 1291 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1292 + this.occurrence.getClass().getName() + " was encountered"); 1293 return (DateTimeType) this.occurrence; 1294 } 1295 1296 public boolean hasOccurrenceDateTimeType() { 1297 return this.occurrence instanceof DateTimeType; 1298 } 1299 1300 /** 1301 * @return {@link #occurrence} (When the request should be fulfilled.) 1302 */ 1303 public Period getOccurrencePeriod() throws FHIRException { 1304 if (this.occurrence == null) 1305 this.occurrence = new Period(); 1306 if (!(this.occurrence instanceof Period)) 1307 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.occurrence.getClass().getName() 1308 + " was encountered"); 1309 return (Period) this.occurrence; 1310 } 1311 1312 public boolean hasOccurrencePeriod() { 1313 return this.occurrence instanceof Period; 1314 } 1315 1316 /** 1317 * @return {@link #occurrence} (When the request should be fulfilled.) 1318 */ 1319 public Timing getOccurrenceTiming() throws FHIRException { 1320 if (this.occurrence == null) 1321 this.occurrence = new Timing(); 1322 if (!(this.occurrence instanceof Timing)) 1323 throw new FHIRException("Type mismatch: the type Timing was expected, but " + this.occurrence.getClass().getName() 1324 + " was encountered"); 1325 return (Timing) this.occurrence; 1326 } 1327 1328 public boolean hasOccurrenceTiming() { 1329 return this.occurrence instanceof Timing; 1330 } 1331 1332 public boolean hasOccurrence() { 1333 return this.occurrence != null && !this.occurrence.isEmpty(); 1334 } 1335 1336 /** 1337 * @param value {@link #occurrence} (When the request should be fulfilled.) 1338 */ 1339 public SupplyRequest setOccurrence(Type value) { 1340 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 1341 throw new Error("Not the right type for SupplyRequest.occurrence[x]: " + value.fhirType()); 1342 this.occurrence = value; 1343 return this; 1344 } 1345 1346 /** 1347 * @return {@link #authoredOn} (When the request was made.). This is the 1348 * underlying object with id, value and extensions. The accessor 1349 * "getAuthoredOn" gives direct access to the value 1350 */ 1351 public DateTimeType getAuthoredOnElement() { 1352 if (this.authoredOn == null) 1353 if (Configuration.errorOnAutoCreate()) 1354 throw new Error("Attempt to auto-create SupplyRequest.authoredOn"); 1355 else if (Configuration.doAutoCreate()) 1356 this.authoredOn = new DateTimeType(); // bb 1357 return this.authoredOn; 1358 } 1359 1360 public boolean hasAuthoredOnElement() { 1361 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1362 } 1363 1364 public boolean hasAuthoredOn() { 1365 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1366 } 1367 1368 /** 1369 * @param value {@link #authoredOn} (When the request was made.). This is the 1370 * underlying object with id, value and extensions. The accessor 1371 * "getAuthoredOn" gives direct access to the value 1372 */ 1373 public SupplyRequest setAuthoredOnElement(DateTimeType value) { 1374 this.authoredOn = value; 1375 return this; 1376 } 1377 1378 /** 1379 * @return When the request was made. 1380 */ 1381 public Date getAuthoredOn() { 1382 return this.authoredOn == null ? null : this.authoredOn.getValue(); 1383 } 1384 1385 /** 1386 * @param value When the request was made. 1387 */ 1388 public SupplyRequest setAuthoredOn(Date value) { 1389 if (value == null) 1390 this.authoredOn = null; 1391 else { 1392 if (this.authoredOn == null) 1393 this.authoredOn = new DateTimeType(); 1394 this.authoredOn.setValue(value); 1395 } 1396 return this; 1397 } 1398 1399 /** 1400 * @return {@link #requester} (The device, practitioner, etc. who initiated the 1401 * request.) 1402 */ 1403 public Reference getRequester() { 1404 if (this.requester == null) 1405 if (Configuration.errorOnAutoCreate()) 1406 throw new Error("Attempt to auto-create SupplyRequest.requester"); 1407 else if (Configuration.doAutoCreate()) 1408 this.requester = new Reference(); // cc 1409 return this.requester; 1410 } 1411 1412 public boolean hasRequester() { 1413 return this.requester != null && !this.requester.isEmpty(); 1414 } 1415 1416 /** 1417 * @param value {@link #requester} (The device, practitioner, etc. who initiated 1418 * the request.) 1419 */ 1420 public SupplyRequest setRequester(Reference value) { 1421 this.requester = value; 1422 return this; 1423 } 1424 1425 /** 1426 * @return {@link #requester} The actual object that is the target of the 1427 * reference. The reference library doesn't populate this, but you can 1428 * use it to hold the resource if you resolve it. (The device, 1429 * practitioner, etc. who initiated the request.) 1430 */ 1431 public Resource getRequesterTarget() { 1432 return this.requesterTarget; 1433 } 1434 1435 /** 1436 * @param value {@link #requester} The actual object that is the target of the 1437 * reference. The reference library doesn't use these, but you can 1438 * use it to hold the resource if you resolve it. (The device, 1439 * practitioner, etc. who initiated the request.) 1440 */ 1441 public SupplyRequest setRequesterTarget(Resource value) { 1442 this.requesterTarget = value; 1443 return this; 1444 } 1445 1446 /** 1447 * @return {@link #supplier} (Who is intended to fulfill the request.) 1448 */ 1449 public List<Reference> getSupplier() { 1450 if (this.supplier == null) 1451 this.supplier = new ArrayList<Reference>(); 1452 return this.supplier; 1453 } 1454 1455 /** 1456 * @return Returns a reference to <code>this</code> for easy method chaining 1457 */ 1458 public SupplyRequest setSupplier(List<Reference> theSupplier) { 1459 this.supplier = theSupplier; 1460 return this; 1461 } 1462 1463 public boolean hasSupplier() { 1464 if (this.supplier == null) 1465 return false; 1466 for (Reference item : this.supplier) 1467 if (!item.isEmpty()) 1468 return true; 1469 return false; 1470 } 1471 1472 public Reference addSupplier() { // 3 1473 Reference t = new Reference(); 1474 if (this.supplier == null) 1475 this.supplier = new ArrayList<Reference>(); 1476 this.supplier.add(t); 1477 return t; 1478 } 1479 1480 public SupplyRequest addSupplier(Reference t) { // 3 1481 if (t == null) 1482 return this; 1483 if (this.supplier == null) 1484 this.supplier = new ArrayList<Reference>(); 1485 this.supplier.add(t); 1486 return this; 1487 } 1488 1489 /** 1490 * @return The first repetition of repeating field {@link #supplier}, creating 1491 * it if it does not already exist 1492 */ 1493 public Reference getSupplierFirstRep() { 1494 if (getSupplier().isEmpty()) { 1495 addSupplier(); 1496 } 1497 return getSupplier().get(0); 1498 } 1499 1500 /** 1501 * @deprecated Use Reference#setResource(IBaseResource) instead 1502 */ 1503 @Deprecated 1504 public List<Resource> getSupplierTarget() { 1505 if (this.supplierTarget == null) 1506 this.supplierTarget = new ArrayList<Resource>(); 1507 return this.supplierTarget; 1508 } 1509 1510 /** 1511 * @return {@link #reasonCode} (The reason why the supply item was requested.) 1512 */ 1513 public List<CodeableConcept> getReasonCode() { 1514 if (this.reasonCode == null) 1515 this.reasonCode = new ArrayList<CodeableConcept>(); 1516 return this.reasonCode; 1517 } 1518 1519 /** 1520 * @return Returns a reference to <code>this</code> for easy method chaining 1521 */ 1522 public SupplyRequest setReasonCode(List<CodeableConcept> theReasonCode) { 1523 this.reasonCode = theReasonCode; 1524 return this; 1525 } 1526 1527 public boolean hasReasonCode() { 1528 if (this.reasonCode == null) 1529 return false; 1530 for (CodeableConcept item : this.reasonCode) 1531 if (!item.isEmpty()) 1532 return true; 1533 return false; 1534 } 1535 1536 public CodeableConcept addReasonCode() { // 3 1537 CodeableConcept t = new CodeableConcept(); 1538 if (this.reasonCode == null) 1539 this.reasonCode = new ArrayList<CodeableConcept>(); 1540 this.reasonCode.add(t); 1541 return t; 1542 } 1543 1544 public SupplyRequest addReasonCode(CodeableConcept t) { // 3 1545 if (t == null) 1546 return this; 1547 if (this.reasonCode == null) 1548 this.reasonCode = new ArrayList<CodeableConcept>(); 1549 this.reasonCode.add(t); 1550 return this; 1551 } 1552 1553 /** 1554 * @return The first repetition of repeating field {@link #reasonCode}, creating 1555 * it if it does not already exist 1556 */ 1557 public CodeableConcept getReasonCodeFirstRep() { 1558 if (getReasonCode().isEmpty()) { 1559 addReasonCode(); 1560 } 1561 return getReasonCode().get(0); 1562 } 1563 1564 /** 1565 * @return {@link #reasonReference} (The reason why the supply item was 1566 * requested.) 1567 */ 1568 public List<Reference> getReasonReference() { 1569 if (this.reasonReference == null) 1570 this.reasonReference = new ArrayList<Reference>(); 1571 return this.reasonReference; 1572 } 1573 1574 /** 1575 * @return Returns a reference to <code>this</code> for easy method chaining 1576 */ 1577 public SupplyRequest setReasonReference(List<Reference> theReasonReference) { 1578 this.reasonReference = theReasonReference; 1579 return this; 1580 } 1581 1582 public boolean hasReasonReference() { 1583 if (this.reasonReference == null) 1584 return false; 1585 for (Reference item : this.reasonReference) 1586 if (!item.isEmpty()) 1587 return true; 1588 return false; 1589 } 1590 1591 public Reference addReasonReference() { // 3 1592 Reference t = new Reference(); 1593 if (this.reasonReference == null) 1594 this.reasonReference = new ArrayList<Reference>(); 1595 this.reasonReference.add(t); 1596 return t; 1597 } 1598 1599 public SupplyRequest addReasonReference(Reference t) { // 3 1600 if (t == null) 1601 return this; 1602 if (this.reasonReference == null) 1603 this.reasonReference = new ArrayList<Reference>(); 1604 this.reasonReference.add(t); 1605 return this; 1606 } 1607 1608 /** 1609 * @return The first repetition of repeating field {@link #reasonReference}, 1610 * creating it if it does not already exist 1611 */ 1612 public Reference getReasonReferenceFirstRep() { 1613 if (getReasonReference().isEmpty()) { 1614 addReasonReference(); 1615 } 1616 return getReasonReference().get(0); 1617 } 1618 1619 /** 1620 * @deprecated Use Reference#setResource(IBaseResource) instead 1621 */ 1622 @Deprecated 1623 public List<Resource> getReasonReferenceTarget() { 1624 if (this.reasonReferenceTarget == null) 1625 this.reasonReferenceTarget = new ArrayList<Resource>(); 1626 return this.reasonReferenceTarget; 1627 } 1628 1629 /** 1630 * @return {@link #deliverFrom} (Where the supply is expected to come from.) 1631 */ 1632 public Reference getDeliverFrom() { 1633 if (this.deliverFrom == null) 1634 if (Configuration.errorOnAutoCreate()) 1635 throw new Error("Attempt to auto-create SupplyRequest.deliverFrom"); 1636 else if (Configuration.doAutoCreate()) 1637 this.deliverFrom = new Reference(); // cc 1638 return this.deliverFrom; 1639 } 1640 1641 public boolean hasDeliverFrom() { 1642 return this.deliverFrom != null && !this.deliverFrom.isEmpty(); 1643 } 1644 1645 /** 1646 * @param value {@link #deliverFrom} (Where the supply is expected to come 1647 * from.) 1648 */ 1649 public SupplyRequest setDeliverFrom(Reference value) { 1650 this.deliverFrom = value; 1651 return this; 1652 } 1653 1654 /** 1655 * @return {@link #deliverFrom} The actual object that is the target of the 1656 * reference. The reference library doesn't populate this, but you can 1657 * use it to hold the resource if you resolve it. (Where the supply is 1658 * expected to come from.) 1659 */ 1660 public Resource getDeliverFromTarget() { 1661 return this.deliverFromTarget; 1662 } 1663 1664 /** 1665 * @param value {@link #deliverFrom} The actual object that is the target of the 1666 * reference. The reference library doesn't use these, but you can 1667 * use it to hold the resource if you resolve it. (Where the supply 1668 * is expected to come from.) 1669 */ 1670 public SupplyRequest setDeliverFromTarget(Resource value) { 1671 this.deliverFromTarget = value; 1672 return this; 1673 } 1674 1675 /** 1676 * @return {@link #deliverTo} (Where the supply is destined to go.) 1677 */ 1678 public Reference getDeliverTo() { 1679 if (this.deliverTo == null) 1680 if (Configuration.errorOnAutoCreate()) 1681 throw new Error("Attempt to auto-create SupplyRequest.deliverTo"); 1682 else if (Configuration.doAutoCreate()) 1683 this.deliverTo = new Reference(); // cc 1684 return this.deliverTo; 1685 } 1686 1687 public boolean hasDeliverTo() { 1688 return this.deliverTo != null && !this.deliverTo.isEmpty(); 1689 } 1690 1691 /** 1692 * @param value {@link #deliverTo} (Where the supply is destined to go.) 1693 */ 1694 public SupplyRequest setDeliverTo(Reference value) { 1695 this.deliverTo = value; 1696 return this; 1697 } 1698 1699 /** 1700 * @return {@link #deliverTo} The actual object that is the target of the 1701 * reference. The reference library doesn't populate this, but you can 1702 * use it to hold the resource if you resolve it. (Where the supply is 1703 * destined to go.) 1704 */ 1705 public Resource getDeliverToTarget() { 1706 return this.deliverToTarget; 1707 } 1708 1709 /** 1710 * @param value {@link #deliverTo} The actual object that is the target of the 1711 * reference. The reference library doesn't use these, but you can 1712 * use it to hold the resource if you resolve it. (Where the supply 1713 * is destined to go.) 1714 */ 1715 public SupplyRequest setDeliverToTarget(Resource value) { 1716 this.deliverToTarget = value; 1717 return this; 1718 } 1719 1720 protected void listChildren(List<Property> children) { 1721 super.listChildren(children); 1722 children.add(new Property("identifier", "Identifier", 1723 "Business identifiers assigned to this SupplyRequest by the author and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.", 1724 0, java.lang.Integer.MAX_VALUE, identifier)); 1725 children.add(new Property("status", "code", "Status of the supply request.", 0, 1, status)); 1726 children.add(new Property("category", "CodeableConcept", 1727 "Category of supply, e.g. central, non-stock, etc. This is used to support work flows associated with the supply process.", 1728 0, 1, category)); 1729 children.add(new Property("priority", "code", 1730 "Indicates how quickly this SupplyRequest should be addressed with respect to other requests.", 0, 1, 1731 priority)); 1732 children.add(new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device)", 1733 "The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 1734 0, 1, item)); 1735 children.add(new Property("quantity", "Quantity", "The amount that is being ordered of the indicated item.", 0, 1, 1736 quantity)); 1737 children.add(new Property("parameter", "", 1738 "Specific parameters for the ordered item. For example, the size of the indicated item.", 0, 1739 java.lang.Integer.MAX_VALUE, parameter)); 1740 children.add(new Property("occurrence[x]", "dateTime|Period|Timing", "When the request should be fulfilled.", 0, 1, 1741 occurrence)); 1742 children.add(new Property("authoredOn", "dateTime", "When the request was made.", 0, 1, authoredOn)); 1743 children.add( 1744 new Property("requester", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson|Device)", 1745 "The device, practitioner, etc. who initiated the request.", 0, 1, requester)); 1746 children.add(new Property("supplier", "Reference(Organization|HealthcareService)", 1747 "Who is intended to fulfill the request.", 0, java.lang.Integer.MAX_VALUE, supplier)); 1748 children.add(new Property("reasonCode", "CodeableConcept", "The reason why the supply item was requested.", 0, 1749 java.lang.Integer.MAX_VALUE, reasonCode)); 1750 children.add(new Property("reasonReference", "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 1751 "The reason why the supply item was requested.", 0, java.lang.Integer.MAX_VALUE, reasonReference)); 1752 children.add(new Property("deliverFrom", "Reference(Organization|Location)", 1753 "Where the supply is expected to come from.", 0, 1, deliverFrom)); 1754 children.add(new Property("deliverTo", "Reference(Organization|Location|Patient)", 1755 "Where the supply is destined to go.", 0, 1, deliverTo)); 1756 } 1757 1758 @Override 1759 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1760 switch (_hash) { 1761 case -1618432855: 1762 /* identifier */ return new Property("identifier", "Identifier", 1763 "Business identifiers assigned to this SupplyRequest by the author and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.", 1764 0, java.lang.Integer.MAX_VALUE, identifier); 1765 case -892481550: 1766 /* status */ return new Property("status", "code", "Status of the supply request.", 0, 1, status); 1767 case 50511102: 1768 /* category */ return new Property("category", "CodeableConcept", 1769 "Category of supply, e.g. central, non-stock, etc. This is used to support work flows associated with the supply process.", 1770 0, 1, category); 1771 case -1165461084: 1772 /* priority */ return new Property("priority", "code", 1773 "Indicates how quickly this SupplyRequest should be addressed with respect to other requests.", 0, 1, 1774 priority); 1775 case 2116201613: 1776 /* item[x] */ return new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device)", 1777 "The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 1778 0, 1, item); 1779 case 3242771: 1780 /* item */ return new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device)", 1781 "The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 1782 0, 1, item); 1783 case 106644494: 1784 /* itemCodeableConcept */ return new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device)", 1785 "The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 1786 0, 1, item); 1787 case 1376364920: 1788 /* itemReference */ return new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device)", 1789 "The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 1790 0, 1, item); 1791 case -1285004149: 1792 /* quantity */ return new Property("quantity", "Quantity", 1793 "The amount that is being ordered of the indicated item.", 0, 1, quantity); 1794 case 1954460585: 1795 /* parameter */ return new Property("parameter", "", 1796 "Specific parameters for the ordered item. For example, the size of the indicated item.", 0, 1797 java.lang.Integer.MAX_VALUE, parameter); 1798 case -2022646513: 1799 /* occurrence[x] */ return new Property("occurrence[x]", "dateTime|Period|Timing", 1800 "When the request should be fulfilled.", 0, 1, occurrence); 1801 case 1687874001: 1802 /* occurrence */ return new Property("occurrence[x]", "dateTime|Period|Timing", 1803 "When the request should be fulfilled.", 0, 1, occurrence); 1804 case -298443636: 1805 /* occurrenceDateTime */ return new Property("occurrence[x]", "dateTime|Period|Timing", 1806 "When the request should be fulfilled.", 0, 1, occurrence); 1807 case 1397156594: 1808 /* occurrencePeriod */ return new Property("occurrence[x]", "dateTime|Period|Timing", 1809 "When the request should be fulfilled.", 0, 1, occurrence); 1810 case 1515218299: 1811 /* occurrenceTiming */ return new Property("occurrence[x]", "dateTime|Period|Timing", 1812 "When the request should be fulfilled.", 0, 1, occurrence); 1813 case -1500852503: 1814 /* authoredOn */ return new Property("authoredOn", "dateTime", "When the request was made.", 0, 1, authoredOn); 1815 case 693933948: 1816 /* requester */ return new Property("requester", 1817 "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson|Device)", 1818 "The device, practitioner, etc. who initiated the request.", 0, 1, requester); 1819 case -1663305268: 1820 /* supplier */ return new Property("supplier", "Reference(Organization|HealthcareService)", 1821 "Who is intended to fulfill the request.", 0, java.lang.Integer.MAX_VALUE, supplier); 1822 case 722137681: 1823 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 1824 "The reason why the supply item was requested.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 1825 case -1146218137: 1826 /* reasonReference */ return new Property("reasonReference", 1827 "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 1828 "The reason why the supply item was requested.", 0, java.lang.Integer.MAX_VALUE, reasonReference); 1829 case -949323153: 1830 /* deliverFrom */ return new Property("deliverFrom", "Reference(Organization|Location)", 1831 "Where the supply is expected to come from.", 0, 1, deliverFrom); 1832 case -242327936: 1833 /* deliverTo */ return new Property("deliverTo", "Reference(Organization|Location|Patient)", 1834 "Where the supply is destined to go.", 0, 1, deliverTo); 1835 default: 1836 return super.getNamedProperty(_hash, _name, _checkValid); 1837 } 1838 1839 } 1840 1841 @Override 1842 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1843 switch (hash) { 1844 case -1618432855: 1845 /* identifier */ return this.identifier == null ? new Base[0] 1846 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1847 case -892481550: 1848 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<SupplyRequestStatus> 1849 case 50511102: 1850 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 1851 case -1165461084: 1852 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // Enumeration<RequestPriority> 1853 case 3242771: 1854 /* item */ return this.item == null ? new Base[0] : new Base[] { this.item }; // Type 1855 case -1285004149: 1856 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 1857 case 1954460585: 1858 /* parameter */ return this.parameter == null ? new Base[0] 1859 : this.parameter.toArray(new Base[this.parameter.size()]); // SupplyRequestParameterComponent 1860 case 1687874001: 1861 /* occurrence */ return this.occurrence == null ? new Base[0] : new Base[] { this.occurrence }; // Type 1862 case -1500852503: 1863 /* authoredOn */ return this.authoredOn == null ? new Base[0] : new Base[] { this.authoredOn }; // DateTimeType 1864 case 693933948: 1865 /* requester */ return this.requester == null ? new Base[0] : new Base[] { this.requester }; // Reference 1866 case -1663305268: 1867 /* supplier */ return this.supplier == null ? new Base[0] : this.supplier.toArray(new Base[this.supplier.size()]); // Reference 1868 case 722137681: 1869 /* reasonCode */ return this.reasonCode == null ? new Base[0] 1870 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 1871 case -1146218137: 1872 /* reasonReference */ return this.reasonReference == null ? new Base[0] 1873 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 1874 case -949323153: 1875 /* deliverFrom */ return this.deliverFrom == null ? new Base[0] : new Base[] { this.deliverFrom }; // Reference 1876 case -242327936: 1877 /* deliverTo */ return this.deliverTo == null ? new Base[0] : new Base[] { this.deliverTo }; // Reference 1878 default: 1879 return super.getProperty(hash, name, checkValid); 1880 } 1881 1882 } 1883 1884 @Override 1885 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1886 switch (hash) { 1887 case -1618432855: // identifier 1888 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1889 return value; 1890 case -892481550: // status 1891 value = new SupplyRequestStatusEnumFactory().fromType(castToCode(value)); 1892 this.status = (Enumeration) value; // Enumeration<SupplyRequestStatus> 1893 return value; 1894 case 50511102: // category 1895 this.category = castToCodeableConcept(value); // CodeableConcept 1896 return value; 1897 case -1165461084: // priority 1898 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 1899 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 1900 return value; 1901 case 3242771: // item 1902 this.item = castToType(value); // Type 1903 return value; 1904 case -1285004149: // quantity 1905 this.quantity = castToQuantity(value); // Quantity 1906 return value; 1907 case 1954460585: // parameter 1908 this.getParameter().add((SupplyRequestParameterComponent) value); // SupplyRequestParameterComponent 1909 return value; 1910 case 1687874001: // occurrence 1911 this.occurrence = castToType(value); // Type 1912 return value; 1913 case -1500852503: // authoredOn 1914 this.authoredOn = castToDateTime(value); // DateTimeType 1915 return value; 1916 case 693933948: // requester 1917 this.requester = castToReference(value); // Reference 1918 return value; 1919 case -1663305268: // supplier 1920 this.getSupplier().add(castToReference(value)); // Reference 1921 return value; 1922 case 722137681: // reasonCode 1923 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 1924 return value; 1925 case -1146218137: // reasonReference 1926 this.getReasonReference().add(castToReference(value)); // Reference 1927 return value; 1928 case -949323153: // deliverFrom 1929 this.deliverFrom = castToReference(value); // Reference 1930 return value; 1931 case -242327936: // deliverTo 1932 this.deliverTo = castToReference(value); // Reference 1933 return value; 1934 default: 1935 return super.setProperty(hash, name, value); 1936 } 1937 1938 } 1939 1940 @Override 1941 public Base setProperty(String name, Base value) throws FHIRException { 1942 if (name.equals("identifier")) { 1943 this.getIdentifier().add(castToIdentifier(value)); 1944 } else if (name.equals("status")) { 1945 value = new SupplyRequestStatusEnumFactory().fromType(castToCode(value)); 1946 this.status = (Enumeration) value; // Enumeration<SupplyRequestStatus> 1947 } else if (name.equals("category")) { 1948 this.category = castToCodeableConcept(value); // CodeableConcept 1949 } else if (name.equals("priority")) { 1950 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 1951 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 1952 } else if (name.equals("item[x]")) { 1953 this.item = castToType(value); // Type 1954 } else if (name.equals("quantity")) { 1955 this.quantity = castToQuantity(value); // Quantity 1956 } else if (name.equals("parameter")) { 1957 this.getParameter().add((SupplyRequestParameterComponent) value); 1958 } else if (name.equals("occurrence[x]")) { 1959 this.occurrence = castToType(value); // Type 1960 } else if (name.equals("authoredOn")) { 1961 this.authoredOn = castToDateTime(value); // DateTimeType 1962 } else if (name.equals("requester")) { 1963 this.requester = castToReference(value); // Reference 1964 } else if (name.equals("supplier")) { 1965 this.getSupplier().add(castToReference(value)); 1966 } else if (name.equals("reasonCode")) { 1967 this.getReasonCode().add(castToCodeableConcept(value)); 1968 } else if (name.equals("reasonReference")) { 1969 this.getReasonReference().add(castToReference(value)); 1970 } else if (name.equals("deliverFrom")) { 1971 this.deliverFrom = castToReference(value); // Reference 1972 } else if (name.equals("deliverTo")) { 1973 this.deliverTo = castToReference(value); // Reference 1974 } else 1975 return super.setProperty(name, value); 1976 return value; 1977 } 1978 1979 @Override 1980 public void removeChild(String name, Base value) throws FHIRException { 1981 if (name.equals("identifier")) { 1982 this.getIdentifier().remove(castToIdentifier(value)); 1983 } else if (name.equals("status")) { 1984 this.status = null; 1985 } else if (name.equals("category")) { 1986 this.category = null; 1987 } else if (name.equals("priority")) { 1988 this.priority = null; 1989 } else if (name.equals("item[x]")) { 1990 this.item = null; 1991 } else if (name.equals("quantity")) { 1992 this.quantity = null; 1993 } else if (name.equals("parameter")) { 1994 this.getParameter().remove((SupplyRequestParameterComponent) value); 1995 } else if (name.equals("occurrence[x]")) { 1996 this.occurrence = null; 1997 } else if (name.equals("authoredOn")) { 1998 this.authoredOn = null; 1999 } else if (name.equals("requester")) { 2000 this.requester = null; 2001 } else if (name.equals("supplier")) { 2002 this.getSupplier().remove(castToReference(value)); 2003 } else if (name.equals("reasonCode")) { 2004 this.getReasonCode().remove(castToCodeableConcept(value)); 2005 } else if (name.equals("reasonReference")) { 2006 this.getReasonReference().remove(castToReference(value)); 2007 } else if (name.equals("deliverFrom")) { 2008 this.deliverFrom = null; 2009 } else if (name.equals("deliverTo")) { 2010 this.deliverTo = null; 2011 } else 2012 super.removeChild(name, value); 2013 2014 } 2015 2016 @Override 2017 public Base makeProperty(int hash, String name) throws FHIRException { 2018 switch (hash) { 2019 case -1618432855: 2020 return addIdentifier(); 2021 case -892481550: 2022 return getStatusElement(); 2023 case 50511102: 2024 return getCategory(); 2025 case -1165461084: 2026 return getPriorityElement(); 2027 case 2116201613: 2028 return getItem(); 2029 case 3242771: 2030 return getItem(); 2031 case -1285004149: 2032 return getQuantity(); 2033 case 1954460585: 2034 return addParameter(); 2035 case -2022646513: 2036 return getOccurrence(); 2037 case 1687874001: 2038 return getOccurrence(); 2039 case -1500852503: 2040 return getAuthoredOnElement(); 2041 case 693933948: 2042 return getRequester(); 2043 case -1663305268: 2044 return addSupplier(); 2045 case 722137681: 2046 return addReasonCode(); 2047 case -1146218137: 2048 return addReasonReference(); 2049 case -949323153: 2050 return getDeliverFrom(); 2051 case -242327936: 2052 return getDeliverTo(); 2053 default: 2054 return super.makeProperty(hash, name); 2055 } 2056 2057 } 2058 2059 @Override 2060 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2061 switch (hash) { 2062 case -1618432855: 2063 /* identifier */ return new String[] { "Identifier" }; 2064 case -892481550: 2065 /* status */ return new String[] { "code" }; 2066 case 50511102: 2067 /* category */ return new String[] { "CodeableConcept" }; 2068 case -1165461084: 2069 /* priority */ return new String[] { "code" }; 2070 case 3242771: 2071 /* item */ return new String[] { "CodeableConcept", "Reference" }; 2072 case -1285004149: 2073 /* quantity */ return new String[] { "Quantity" }; 2074 case 1954460585: 2075 /* parameter */ return new String[] {}; 2076 case 1687874001: 2077 /* occurrence */ return new String[] { "dateTime", "Period", "Timing" }; 2078 case -1500852503: 2079 /* authoredOn */ return new String[] { "dateTime" }; 2080 case 693933948: 2081 /* requester */ return new String[] { "Reference" }; 2082 case -1663305268: 2083 /* supplier */ return new String[] { "Reference" }; 2084 case 722137681: 2085 /* reasonCode */ return new String[] { "CodeableConcept" }; 2086 case -1146218137: 2087 /* reasonReference */ return new String[] { "Reference" }; 2088 case -949323153: 2089 /* deliverFrom */ return new String[] { "Reference" }; 2090 case -242327936: 2091 /* deliverTo */ return new String[] { "Reference" }; 2092 default: 2093 return super.getTypesForProperty(hash, name); 2094 } 2095 2096 } 2097 2098 @Override 2099 public Base addChild(String name) throws FHIRException { 2100 if (name.equals("identifier")) { 2101 return addIdentifier(); 2102 } else if (name.equals("status")) { 2103 throw new FHIRException("Cannot call addChild on a singleton property SupplyRequest.status"); 2104 } else if (name.equals("category")) { 2105 this.category = new CodeableConcept(); 2106 return this.category; 2107 } else if (name.equals("priority")) { 2108 throw new FHIRException("Cannot call addChild on a singleton property SupplyRequest.priority"); 2109 } else if (name.equals("itemCodeableConcept")) { 2110 this.item = new CodeableConcept(); 2111 return this.item; 2112 } else if (name.equals("itemReference")) { 2113 this.item = new Reference(); 2114 return this.item; 2115 } else if (name.equals("quantity")) { 2116 this.quantity = new Quantity(); 2117 return this.quantity; 2118 } else if (name.equals("parameter")) { 2119 return addParameter(); 2120 } else if (name.equals("occurrenceDateTime")) { 2121 this.occurrence = new DateTimeType(); 2122 return this.occurrence; 2123 } else if (name.equals("occurrencePeriod")) { 2124 this.occurrence = new Period(); 2125 return this.occurrence; 2126 } else if (name.equals("occurrenceTiming")) { 2127 this.occurrence = new Timing(); 2128 return this.occurrence; 2129 } else if (name.equals("authoredOn")) { 2130 throw new FHIRException("Cannot call addChild on a singleton property SupplyRequest.authoredOn"); 2131 } else if (name.equals("requester")) { 2132 this.requester = new Reference(); 2133 return this.requester; 2134 } else if (name.equals("supplier")) { 2135 return addSupplier(); 2136 } else if (name.equals("reasonCode")) { 2137 return addReasonCode(); 2138 } else if (name.equals("reasonReference")) { 2139 return addReasonReference(); 2140 } else if (name.equals("deliverFrom")) { 2141 this.deliverFrom = new Reference(); 2142 return this.deliverFrom; 2143 } else if (name.equals("deliverTo")) { 2144 this.deliverTo = new Reference(); 2145 return this.deliverTo; 2146 } else 2147 return super.addChild(name); 2148 } 2149 2150 public String fhirType() { 2151 return "SupplyRequest"; 2152 2153 } 2154 2155 public SupplyRequest copy() { 2156 SupplyRequest dst = new SupplyRequest(); 2157 copyValues(dst); 2158 return dst; 2159 } 2160 2161 public void copyValues(SupplyRequest dst) { 2162 super.copyValues(dst); 2163 if (identifier != null) { 2164 dst.identifier = new ArrayList<Identifier>(); 2165 for (Identifier i : identifier) 2166 dst.identifier.add(i.copy()); 2167 } 2168 ; 2169 dst.status = status == null ? null : status.copy(); 2170 dst.category = category == null ? null : category.copy(); 2171 dst.priority = priority == null ? null : priority.copy(); 2172 dst.item = item == null ? null : item.copy(); 2173 dst.quantity = quantity == null ? null : quantity.copy(); 2174 if (parameter != null) { 2175 dst.parameter = new ArrayList<SupplyRequestParameterComponent>(); 2176 for (SupplyRequestParameterComponent i : parameter) 2177 dst.parameter.add(i.copy()); 2178 } 2179 ; 2180 dst.occurrence = occurrence == null ? null : occurrence.copy(); 2181 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 2182 dst.requester = requester == null ? null : requester.copy(); 2183 if (supplier != null) { 2184 dst.supplier = new ArrayList<Reference>(); 2185 for (Reference i : supplier) 2186 dst.supplier.add(i.copy()); 2187 } 2188 ; 2189 if (reasonCode != null) { 2190 dst.reasonCode = new ArrayList<CodeableConcept>(); 2191 for (CodeableConcept i : reasonCode) 2192 dst.reasonCode.add(i.copy()); 2193 } 2194 ; 2195 if (reasonReference != null) { 2196 dst.reasonReference = new ArrayList<Reference>(); 2197 for (Reference i : reasonReference) 2198 dst.reasonReference.add(i.copy()); 2199 } 2200 ; 2201 dst.deliverFrom = deliverFrom == null ? null : deliverFrom.copy(); 2202 dst.deliverTo = deliverTo == null ? null : deliverTo.copy(); 2203 } 2204 2205 protected SupplyRequest typedCopy() { 2206 return copy(); 2207 } 2208 2209 @Override 2210 public boolean equalsDeep(Base other_) { 2211 if (!super.equalsDeep(other_)) 2212 return false; 2213 if (!(other_ instanceof SupplyRequest)) 2214 return false; 2215 SupplyRequest o = (SupplyRequest) other_; 2216 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 2217 && compareDeep(category, o.category, true) && compareDeep(priority, o.priority, true) 2218 && compareDeep(item, o.item, true) && compareDeep(quantity, o.quantity, true) 2219 && compareDeep(parameter, o.parameter, true) && compareDeep(occurrence, o.occurrence, true) 2220 && compareDeep(authoredOn, o.authoredOn, true) && compareDeep(requester, o.requester, true) 2221 && compareDeep(supplier, o.supplier, true) && compareDeep(reasonCode, o.reasonCode, true) 2222 && compareDeep(reasonReference, o.reasonReference, true) && compareDeep(deliverFrom, o.deliverFrom, true) 2223 && compareDeep(deliverTo, o.deliverTo, true); 2224 } 2225 2226 @Override 2227 public boolean equalsShallow(Base other_) { 2228 if (!super.equalsShallow(other_)) 2229 return false; 2230 if (!(other_ instanceof SupplyRequest)) 2231 return false; 2232 SupplyRequest o = (SupplyRequest) other_; 2233 return compareValues(status, o.status, true) && compareValues(priority, o.priority, true) 2234 && compareValues(authoredOn, o.authoredOn, true); 2235 } 2236 2237 public boolean isEmpty() { 2238 return super.isEmpty() 2239 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, category, priority, item, quantity, parameter, 2240 occurrence, authoredOn, requester, supplier, reasonCode, reasonReference, deliverFrom, deliverTo); 2241 } 2242 2243 @Override 2244 public ResourceType getResourceType() { 2245 return ResourceType.SupplyRequest; 2246 } 2247 2248 /** 2249 * Search parameter: <b>requester</b> 2250 * <p> 2251 * Description: <b>Individual making the request</b><br> 2252 * Type: <b>reference</b><br> 2253 * Path: <b>SupplyRequest.requester</b><br> 2254 * </p> 2255 */ 2256 @SearchParamDefinition(name = "requester", path = "SupplyRequest.requester", description = "Individual making the request", type = "reference", providesMembershipIn = { 2257 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 2258 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 2259 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, 2260 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 2261 public static final String SP_REQUESTER = "requester"; 2262 /** 2263 * <b>Fluent Client</b> search parameter constant for <b>requester</b> 2264 * <p> 2265 * Description: <b>Individual making the request</b><br> 2266 * Type: <b>reference</b><br> 2267 * Path: <b>SupplyRequest.requester</b><br> 2268 * </p> 2269 */ 2270 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2271 SP_REQUESTER); 2272 2273 /** 2274 * Constant for fluent queries to be used to add include statements. Specifies 2275 * the path value of "<b>SupplyRequest:requester</b>". 2276 */ 2277 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTER = new ca.uhn.fhir.model.api.Include( 2278 "SupplyRequest:requester").toLocked(); 2279 2280 /** 2281 * Search parameter: <b>date</b> 2282 * <p> 2283 * Description: <b>When the request was made</b><br> 2284 * Type: <b>date</b><br> 2285 * Path: <b>SupplyRequest.authoredOn</b><br> 2286 * </p> 2287 */ 2288 @SearchParamDefinition(name = "date", path = "SupplyRequest.authoredOn", description = "When the request was made", type = "date") 2289 public static final String SP_DATE = "date"; 2290 /** 2291 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2292 * <p> 2293 * Description: <b>When the request was made</b><br> 2294 * Type: <b>date</b><br> 2295 * Path: <b>SupplyRequest.authoredOn</b><br> 2296 * </p> 2297 */ 2298 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2299 SP_DATE); 2300 2301 /** 2302 * Search parameter: <b>identifier</b> 2303 * <p> 2304 * Description: <b>Business Identifier for SupplyRequest</b><br> 2305 * Type: <b>token</b><br> 2306 * Path: <b>SupplyRequest.identifier</b><br> 2307 * </p> 2308 */ 2309 @SearchParamDefinition(name = "identifier", path = "SupplyRequest.identifier", description = "Business Identifier for SupplyRequest", type = "token") 2310 public static final String SP_IDENTIFIER = "identifier"; 2311 /** 2312 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2313 * <p> 2314 * Description: <b>Business Identifier for SupplyRequest</b><br> 2315 * Type: <b>token</b><br> 2316 * Path: <b>SupplyRequest.identifier</b><br> 2317 * </p> 2318 */ 2319 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2320 SP_IDENTIFIER); 2321 2322 /** 2323 * Search parameter: <b>subject</b> 2324 * <p> 2325 * Description: <b>The destination of the supply</b><br> 2326 * Type: <b>reference</b><br> 2327 * Path: <b>SupplyRequest.deliverTo</b><br> 2328 * </p> 2329 */ 2330 @SearchParamDefinition(name = "subject", path = "SupplyRequest.deliverTo", description = "The destination of the supply", type = "reference", providesMembershipIn = { 2331 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Location.class, Organization.class, 2332 Patient.class }) 2333 public static final String SP_SUBJECT = "subject"; 2334 /** 2335 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2336 * <p> 2337 * Description: <b>The destination of the supply</b><br> 2338 * Type: <b>reference</b><br> 2339 * Path: <b>SupplyRequest.deliverTo</b><br> 2340 * </p> 2341 */ 2342 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2343 SP_SUBJECT); 2344 2345 /** 2346 * Constant for fluent queries to be used to add include statements. Specifies 2347 * the path value of "<b>SupplyRequest:subject</b>". 2348 */ 2349 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 2350 "SupplyRequest:subject").toLocked(); 2351 2352 /** 2353 * Search parameter: <b>supplier</b> 2354 * <p> 2355 * Description: <b>Who is intended to fulfill the request</b><br> 2356 * Type: <b>reference</b><br> 2357 * Path: <b>SupplyRequest.supplier</b><br> 2358 * </p> 2359 */ 2360 @SearchParamDefinition(name = "supplier", path = "SupplyRequest.supplier", description = "Who is intended to fulfill the request", type = "reference", target = { 2361 HealthcareService.class, Organization.class }) 2362 public static final String SP_SUPPLIER = "supplier"; 2363 /** 2364 * <b>Fluent Client</b> search parameter constant for <b>supplier</b> 2365 * <p> 2366 * Description: <b>Who is intended to fulfill the request</b><br> 2367 * Type: <b>reference</b><br> 2368 * Path: <b>SupplyRequest.supplier</b><br> 2369 * </p> 2370 */ 2371 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUPPLIER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2372 SP_SUPPLIER); 2373 2374 /** 2375 * Constant for fluent queries to be used to add include statements. Specifies 2376 * the path value of "<b>SupplyRequest:supplier</b>". 2377 */ 2378 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUPPLIER = new ca.uhn.fhir.model.api.Include( 2379 "SupplyRequest:supplier").toLocked(); 2380 2381 /** 2382 * Search parameter: <b>category</b> 2383 * <p> 2384 * Description: <b>The kind of supply (central, non-stock, etc.)</b><br> 2385 * Type: <b>token</b><br> 2386 * Path: <b>SupplyRequest.category</b><br> 2387 * </p> 2388 */ 2389 @SearchParamDefinition(name = "category", path = "SupplyRequest.category", description = "The kind of supply (central, non-stock, etc.)", type = "token") 2390 public static final String SP_CATEGORY = "category"; 2391 /** 2392 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2393 * <p> 2394 * Description: <b>The kind of supply (central, non-stock, etc.)</b><br> 2395 * Type: <b>token</b><br> 2396 * Path: <b>SupplyRequest.category</b><br> 2397 * </p> 2398 */ 2399 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2400 SP_CATEGORY); 2401 2402 /** 2403 * Search parameter: <b>status</b> 2404 * <p> 2405 * Description: <b>draft | active | suspended +</b><br> 2406 * Type: <b>token</b><br> 2407 * Path: <b>SupplyRequest.status</b><br> 2408 * </p> 2409 */ 2410 @SearchParamDefinition(name = "status", path = "SupplyRequest.status", description = "draft | active | suspended +", type = "token") 2411 public static final String SP_STATUS = "status"; 2412 /** 2413 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2414 * <p> 2415 * Description: <b>draft | active | suspended +</b><br> 2416 * Type: <b>token</b><br> 2417 * Path: <b>SupplyRequest.status</b><br> 2418 * </p> 2419 */ 2420 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2421 SP_STATUS); 2422 2423}