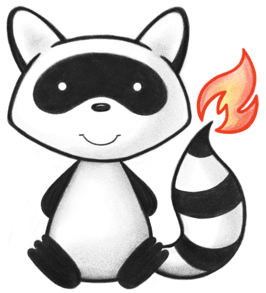
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.DatatypeDef; 046import ca.uhn.fhir.model.api.annotation.Description; 047 048/** 049 * Specifies an event that may occur multiple times. Timing schedules are used 050 * to record when things are planned, expected or requested to occur. The most 051 * common usage is in dosage instructions for medications. They are also used 052 * when planning care of various kinds, and may be used for reporting the 053 * schedule to which past regular activities were carried out. 054 */ 055@DatatypeDef(name = "Timing") 056public class Timing extends BackboneType implements ICompositeType { 057 058 public enum UnitsOfTime { 059 /** 060 * null 061 */ 062 S, 063 /** 064 * null 065 */ 066 MIN, 067 /** 068 * null 069 */ 070 H, 071 /** 072 * null 073 */ 074 D, 075 /** 076 * null 077 */ 078 WK, 079 /** 080 * null 081 */ 082 MO, 083 /** 084 * null 085 */ 086 A, 087 /** 088 * added to help the parsers with the generic types 089 */ 090 NULL; 091 092 public static UnitsOfTime fromCode(String codeString) throws FHIRException { 093 if (codeString == null || "".equals(codeString)) 094 return null; 095 if ("s".equals(codeString)) 096 return S; 097 if ("min".equals(codeString)) 098 return MIN; 099 if ("h".equals(codeString)) 100 return H; 101 if ("d".equals(codeString)) 102 return D; 103 if ("wk".equals(codeString)) 104 return WK; 105 if ("mo".equals(codeString)) 106 return MO; 107 if ("a".equals(codeString)) 108 return A; 109 if (Configuration.isAcceptInvalidEnums()) 110 return null; 111 else 112 throw new FHIRException("Unknown UnitsOfTime code '" + codeString + "'"); 113 } 114 115 public String toCode() { 116 switch (this) { 117 case S: 118 return "s"; 119 case MIN: 120 return "min"; 121 case H: 122 return "h"; 123 case D: 124 return "d"; 125 case WK: 126 return "wk"; 127 case MO: 128 return "mo"; 129 case A: 130 return "a"; 131 case NULL: 132 return null; 133 default: 134 return "?"; 135 } 136 } 137 138 public String getSystem() { 139 switch (this) { 140 case S: 141 return "http://unitsofmeasure.org"; 142 case MIN: 143 return "http://unitsofmeasure.org"; 144 case H: 145 return "http://unitsofmeasure.org"; 146 case D: 147 return "http://unitsofmeasure.org"; 148 case WK: 149 return "http://unitsofmeasure.org"; 150 case MO: 151 return "http://unitsofmeasure.org"; 152 case A: 153 return "http://unitsofmeasure.org"; 154 case NULL: 155 return null; 156 default: 157 return "?"; 158 } 159 } 160 161 public String getDefinition() { 162 switch (this) { 163 case S: 164 return ""; 165 case MIN: 166 return ""; 167 case H: 168 return ""; 169 case D: 170 return ""; 171 case WK: 172 return ""; 173 case MO: 174 return ""; 175 case A: 176 return ""; 177 case NULL: 178 return null; 179 default: 180 return "?"; 181 } 182 } 183 184 public String getDisplay() { 185 switch (this) { 186 case S: 187 return "second"; 188 case MIN: 189 return "minute"; 190 case H: 191 return "hour"; 192 case D: 193 return "day"; 194 case WK: 195 return "week"; 196 case MO: 197 return "month"; 198 case A: 199 return "year"; 200 case NULL: 201 return null; 202 default: 203 return "?"; 204 } 205 } 206 } 207 208 public static class UnitsOfTimeEnumFactory implements EnumFactory<UnitsOfTime> { 209 public UnitsOfTime fromCode(String codeString) throws IllegalArgumentException { 210 if (codeString == null || "".equals(codeString)) 211 if (codeString == null || "".equals(codeString)) 212 return null; 213 if ("s".equals(codeString)) 214 return UnitsOfTime.S; 215 if ("min".equals(codeString)) 216 return UnitsOfTime.MIN; 217 if ("h".equals(codeString)) 218 return UnitsOfTime.H; 219 if ("d".equals(codeString)) 220 return UnitsOfTime.D; 221 if ("wk".equals(codeString)) 222 return UnitsOfTime.WK; 223 if ("mo".equals(codeString)) 224 return UnitsOfTime.MO; 225 if ("a".equals(codeString)) 226 return UnitsOfTime.A; 227 throw new IllegalArgumentException("Unknown UnitsOfTime code '" + codeString + "'"); 228 } 229 230 public Enumeration<UnitsOfTime> fromType(PrimitiveType<?> code) throws FHIRException { 231 if (code == null) 232 return null; 233 if (code.isEmpty()) 234 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.NULL, code); 235 String codeString = code.asStringValue(); 236 if (codeString == null || "".equals(codeString)) 237 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.NULL, code); 238 if ("s".equals(codeString)) 239 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.S, code); 240 if ("min".equals(codeString)) 241 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.MIN, code); 242 if ("h".equals(codeString)) 243 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.H, code); 244 if ("d".equals(codeString)) 245 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.D, code); 246 if ("wk".equals(codeString)) 247 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.WK, code); 248 if ("mo".equals(codeString)) 249 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.MO, code); 250 if ("a".equals(codeString)) 251 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.A, code); 252 throw new FHIRException("Unknown UnitsOfTime code '" + codeString + "'"); 253 } 254 255 public String toCode(UnitsOfTime code) { 256 if (code == UnitsOfTime.S) 257 return "s"; 258 if (code == UnitsOfTime.MIN) 259 return "min"; 260 if (code == UnitsOfTime.H) 261 return "h"; 262 if (code == UnitsOfTime.D) 263 return "d"; 264 if (code == UnitsOfTime.WK) 265 return "wk"; 266 if (code == UnitsOfTime.MO) 267 return "mo"; 268 if (code == UnitsOfTime.A) 269 return "a"; 270 return "?"; 271 } 272 273 public String toSystem(UnitsOfTime code) { 274 return code.getSystem(); 275 } 276 } 277 278 public enum DayOfWeek { 279 /** 280 * Monday. 281 */ 282 MON, 283 /** 284 * Tuesday. 285 */ 286 TUE, 287 /** 288 * Wednesday. 289 */ 290 WED, 291 /** 292 * Thursday. 293 */ 294 THU, 295 /** 296 * Friday. 297 */ 298 FRI, 299 /** 300 * Saturday. 301 */ 302 SAT, 303 /** 304 * Sunday. 305 */ 306 SUN, 307 /** 308 * added to help the parsers with the generic types 309 */ 310 NULL; 311 312 public static DayOfWeek fromCode(String codeString) throws FHIRException { 313 if (codeString == null || "".equals(codeString)) 314 return null; 315 if ("mon".equals(codeString)) 316 return MON; 317 if ("tue".equals(codeString)) 318 return TUE; 319 if ("wed".equals(codeString)) 320 return WED; 321 if ("thu".equals(codeString)) 322 return THU; 323 if ("fri".equals(codeString)) 324 return FRI; 325 if ("sat".equals(codeString)) 326 return SAT; 327 if ("sun".equals(codeString)) 328 return SUN; 329 if (Configuration.isAcceptInvalidEnums()) 330 return null; 331 else 332 throw new FHIRException("Unknown DayOfWeek code '" + codeString + "'"); 333 } 334 335 public String toCode() { 336 switch (this) { 337 case MON: 338 return "mon"; 339 case TUE: 340 return "tue"; 341 case WED: 342 return "wed"; 343 case THU: 344 return "thu"; 345 case FRI: 346 return "fri"; 347 case SAT: 348 return "sat"; 349 case SUN: 350 return "sun"; 351 case NULL: 352 return null; 353 default: 354 return "?"; 355 } 356 } 357 358 public String getSystem() { 359 switch (this) { 360 case MON: 361 return "http://hl7.org/fhir/days-of-week"; 362 case TUE: 363 return "http://hl7.org/fhir/days-of-week"; 364 case WED: 365 return "http://hl7.org/fhir/days-of-week"; 366 case THU: 367 return "http://hl7.org/fhir/days-of-week"; 368 case FRI: 369 return "http://hl7.org/fhir/days-of-week"; 370 case SAT: 371 return "http://hl7.org/fhir/days-of-week"; 372 case SUN: 373 return "http://hl7.org/fhir/days-of-week"; 374 case NULL: 375 return null; 376 default: 377 return "?"; 378 } 379 } 380 381 public String getDefinition() { 382 switch (this) { 383 case MON: 384 return "Monday."; 385 case TUE: 386 return "Tuesday."; 387 case WED: 388 return "Wednesday."; 389 case THU: 390 return "Thursday."; 391 case FRI: 392 return "Friday."; 393 case SAT: 394 return "Saturday."; 395 case SUN: 396 return "Sunday."; 397 case NULL: 398 return null; 399 default: 400 return "?"; 401 } 402 } 403 404 public String getDisplay() { 405 switch (this) { 406 case MON: 407 return "Monday"; 408 case TUE: 409 return "Tuesday"; 410 case WED: 411 return "Wednesday"; 412 case THU: 413 return "Thursday"; 414 case FRI: 415 return "Friday"; 416 case SAT: 417 return "Saturday"; 418 case SUN: 419 return "Sunday"; 420 case NULL: 421 return null; 422 default: 423 return "?"; 424 } 425 } 426 } 427 428 public static class DayOfWeekEnumFactory implements EnumFactory<DayOfWeek> { 429 public DayOfWeek fromCode(String codeString) throws IllegalArgumentException { 430 if (codeString == null || "".equals(codeString)) 431 if (codeString == null || "".equals(codeString)) 432 return null; 433 if ("mon".equals(codeString)) 434 return DayOfWeek.MON; 435 if ("tue".equals(codeString)) 436 return DayOfWeek.TUE; 437 if ("wed".equals(codeString)) 438 return DayOfWeek.WED; 439 if ("thu".equals(codeString)) 440 return DayOfWeek.THU; 441 if ("fri".equals(codeString)) 442 return DayOfWeek.FRI; 443 if ("sat".equals(codeString)) 444 return DayOfWeek.SAT; 445 if ("sun".equals(codeString)) 446 return DayOfWeek.SUN; 447 throw new IllegalArgumentException("Unknown DayOfWeek code '" + codeString + "'"); 448 } 449 450 public Enumeration<DayOfWeek> fromType(PrimitiveType<?> code) throws FHIRException { 451 if (code == null) 452 return null; 453 if (code.isEmpty()) 454 return new Enumeration<DayOfWeek>(this, DayOfWeek.NULL, code); 455 String codeString = code.asStringValue(); 456 if (codeString == null || "".equals(codeString)) 457 return new Enumeration<DayOfWeek>(this, DayOfWeek.NULL, code); 458 if ("mon".equals(codeString)) 459 return new Enumeration<DayOfWeek>(this, DayOfWeek.MON, code); 460 if ("tue".equals(codeString)) 461 return new Enumeration<DayOfWeek>(this, DayOfWeek.TUE, code); 462 if ("wed".equals(codeString)) 463 return new Enumeration<DayOfWeek>(this, DayOfWeek.WED, code); 464 if ("thu".equals(codeString)) 465 return new Enumeration<DayOfWeek>(this, DayOfWeek.THU, code); 466 if ("fri".equals(codeString)) 467 return new Enumeration<DayOfWeek>(this, DayOfWeek.FRI, code); 468 if ("sat".equals(codeString)) 469 return new Enumeration<DayOfWeek>(this, DayOfWeek.SAT, code); 470 if ("sun".equals(codeString)) 471 return new Enumeration<DayOfWeek>(this, DayOfWeek.SUN, code); 472 throw new FHIRException("Unknown DayOfWeek code '" + codeString + "'"); 473 } 474 475 public String toCode(DayOfWeek code) { 476 if (code == DayOfWeek.MON) 477 return "mon"; 478 if (code == DayOfWeek.TUE) 479 return "tue"; 480 if (code == DayOfWeek.WED) 481 return "wed"; 482 if (code == DayOfWeek.THU) 483 return "thu"; 484 if (code == DayOfWeek.FRI) 485 return "fri"; 486 if (code == DayOfWeek.SAT) 487 return "sat"; 488 if (code == DayOfWeek.SUN) 489 return "sun"; 490 return "?"; 491 } 492 493 public String toSystem(DayOfWeek code) { 494 return code.getSystem(); 495 } 496 } 497 498 public enum EventTiming { 499 /** 500 * Event occurs during the morning. The exact time is unspecified and 501 * established by institution convention or patient interpretation. 502 */ 503 MORN, 504 /** 505 * Event occurs during the early morning. The exact time is unspecified and 506 * established by institution convention or patient interpretation. 507 */ 508 MORN_EARLY, 509 /** 510 * Event occurs during the late morning. The exact time is unspecified and 511 * established by institution convention or patient interpretation. 512 */ 513 MORN_LATE, 514 /** 515 * Event occurs around 12:00pm. The exact time is unspecified and established by 516 * institution convention or patient interpretation. 517 */ 518 NOON, 519 /** 520 * Event occurs during the afternoon. The exact time is unspecified and 521 * established by institution convention or patient interpretation. 522 */ 523 AFT, 524 /** 525 * Event occurs during the early afternoon. The exact time is unspecified and 526 * established by institution convention or patient interpretation. 527 */ 528 AFT_EARLY, 529 /** 530 * Event occurs during the late afternoon. The exact time is unspecified and 531 * established by institution convention or patient interpretation. 532 */ 533 AFT_LATE, 534 /** 535 * Event occurs during the evening. The exact time is unspecified and 536 * established by institution convention or patient interpretation. 537 */ 538 EVE, 539 /** 540 * Event occurs during the early evening. The exact time is unspecified and 541 * established by institution convention or patient interpretation. 542 */ 543 EVE_EARLY, 544 /** 545 * Event occurs during the late evening. The exact time is unspecified and 546 * established by institution convention or patient interpretation. 547 */ 548 EVE_LATE, 549 /** 550 * Event occurs during the night. The exact time is unspecified and established 551 * by institution convention or patient interpretation. 552 */ 553 NIGHT, 554 /** 555 * Event occurs [offset] after subject goes to sleep. The exact time is 556 * unspecified and established by institution convention or patient 557 * interpretation. 558 */ 559 PHS, 560 /** 561 * null 562 */ 563 HS, 564 /** 565 * null 566 */ 567 WAKE, 568 /** 569 * null 570 */ 571 C, 572 /** 573 * null 574 */ 575 CM, 576 /** 577 * null 578 */ 579 CD, 580 /** 581 * null 582 */ 583 CV, 584 /** 585 * null 586 */ 587 AC, 588 /** 589 * null 590 */ 591 ACM, 592 /** 593 * null 594 */ 595 ACD, 596 /** 597 * null 598 */ 599 ACV, 600 /** 601 * null 602 */ 603 PC, 604 /** 605 * null 606 */ 607 PCM, 608 /** 609 * null 610 */ 611 PCD, 612 /** 613 * null 614 */ 615 PCV, 616 /** 617 * added to help the parsers with the generic types 618 */ 619 NULL; 620 621 public static EventTiming fromCode(String codeString) throws FHIRException { 622 if (codeString == null || "".equals(codeString)) 623 return null; 624 if ("MORN".equals(codeString)) 625 return MORN; 626 if ("MORN.early".equals(codeString)) 627 return MORN_EARLY; 628 if ("MORN.late".equals(codeString)) 629 return MORN_LATE; 630 if ("NOON".equals(codeString)) 631 return NOON; 632 if ("AFT".equals(codeString)) 633 return AFT; 634 if ("AFT.early".equals(codeString)) 635 return AFT_EARLY; 636 if ("AFT.late".equals(codeString)) 637 return AFT_LATE; 638 if ("EVE".equals(codeString)) 639 return EVE; 640 if ("EVE.early".equals(codeString)) 641 return EVE_EARLY; 642 if ("EVE.late".equals(codeString)) 643 return EVE_LATE; 644 if ("NIGHT".equals(codeString)) 645 return NIGHT; 646 if ("PHS".equals(codeString)) 647 return PHS; 648 if ("HS".equals(codeString)) 649 return HS; 650 if ("WAKE".equals(codeString)) 651 return WAKE; 652 if ("C".equals(codeString)) 653 return C; 654 if ("CM".equals(codeString)) 655 return CM; 656 if ("CD".equals(codeString)) 657 return CD; 658 if ("CV".equals(codeString)) 659 return CV; 660 if ("AC".equals(codeString)) 661 return AC; 662 if ("ACM".equals(codeString)) 663 return ACM; 664 if ("ACD".equals(codeString)) 665 return ACD; 666 if ("ACV".equals(codeString)) 667 return ACV; 668 if ("PC".equals(codeString)) 669 return PC; 670 if ("PCM".equals(codeString)) 671 return PCM; 672 if ("PCD".equals(codeString)) 673 return PCD; 674 if ("PCV".equals(codeString)) 675 return PCV; 676 if (Configuration.isAcceptInvalidEnums()) 677 return null; 678 else 679 throw new FHIRException("Unknown EventTiming code '" + codeString + "'"); 680 } 681 682 public String toCode() { 683 switch (this) { 684 case MORN: 685 return "MORN"; 686 case MORN_EARLY: 687 return "MORN.early"; 688 case MORN_LATE: 689 return "MORN.late"; 690 case NOON: 691 return "NOON"; 692 case AFT: 693 return "AFT"; 694 case AFT_EARLY: 695 return "AFT.early"; 696 case AFT_LATE: 697 return "AFT.late"; 698 case EVE: 699 return "EVE"; 700 case EVE_EARLY: 701 return "EVE.early"; 702 case EVE_LATE: 703 return "EVE.late"; 704 case NIGHT: 705 return "NIGHT"; 706 case PHS: 707 return "PHS"; 708 case HS: 709 return "HS"; 710 case WAKE: 711 return "WAKE"; 712 case C: 713 return "C"; 714 case CM: 715 return "CM"; 716 case CD: 717 return "CD"; 718 case CV: 719 return "CV"; 720 case AC: 721 return "AC"; 722 case ACM: 723 return "ACM"; 724 case ACD: 725 return "ACD"; 726 case ACV: 727 return "ACV"; 728 case PC: 729 return "PC"; 730 case PCM: 731 return "PCM"; 732 case PCD: 733 return "PCD"; 734 case PCV: 735 return "PCV"; 736 case NULL: 737 return null; 738 default: 739 return "?"; 740 } 741 } 742 743 public String getSystem() { 744 switch (this) { 745 case MORN: 746 return "http://hl7.org/fhir/event-timing"; 747 case MORN_EARLY: 748 return "http://hl7.org/fhir/event-timing"; 749 case MORN_LATE: 750 return "http://hl7.org/fhir/event-timing"; 751 case NOON: 752 return "http://hl7.org/fhir/event-timing"; 753 case AFT: 754 return "http://hl7.org/fhir/event-timing"; 755 case AFT_EARLY: 756 return "http://hl7.org/fhir/event-timing"; 757 case AFT_LATE: 758 return "http://hl7.org/fhir/event-timing"; 759 case EVE: 760 return "http://hl7.org/fhir/event-timing"; 761 case EVE_EARLY: 762 return "http://hl7.org/fhir/event-timing"; 763 case EVE_LATE: 764 return "http://hl7.org/fhir/event-timing"; 765 case NIGHT: 766 return "http://hl7.org/fhir/event-timing"; 767 case PHS: 768 return "http://hl7.org/fhir/event-timing"; 769 case HS: 770 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 771 case WAKE: 772 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 773 case C: 774 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 775 case CM: 776 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 777 case CD: 778 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 779 case CV: 780 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 781 case AC: 782 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 783 case ACM: 784 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 785 case ACD: 786 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 787 case ACV: 788 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 789 case PC: 790 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 791 case PCM: 792 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 793 case PCD: 794 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 795 case PCV: 796 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 797 case NULL: 798 return null; 799 default: 800 return "?"; 801 } 802 } 803 804 public String getDefinition() { 805 switch (this) { 806 case MORN: 807 return "Event occurs during the morning. The exact time is unspecified and established by institution convention or patient interpretation."; 808 case MORN_EARLY: 809 return "Event occurs during the early morning. The exact time is unspecified and established by institution convention or patient interpretation."; 810 case MORN_LATE: 811 return "Event occurs during the late morning. The exact time is unspecified and established by institution convention or patient interpretation."; 812 case NOON: 813 return "Event occurs around 12:00pm. The exact time is unspecified and established by institution convention or patient interpretation."; 814 case AFT: 815 return "Event occurs during the afternoon. The exact time is unspecified and established by institution convention or patient interpretation."; 816 case AFT_EARLY: 817 return "Event occurs during the early afternoon. The exact time is unspecified and established by institution convention or patient interpretation."; 818 case AFT_LATE: 819 return "Event occurs during the late afternoon. The exact time is unspecified and established by institution convention or patient interpretation."; 820 case EVE: 821 return "Event occurs during the evening. The exact time is unspecified and established by institution convention or patient interpretation."; 822 case EVE_EARLY: 823 return "Event occurs during the early evening. The exact time is unspecified and established by institution convention or patient interpretation."; 824 case EVE_LATE: 825 return "Event occurs during the late evening. The exact time is unspecified and established by institution convention or patient interpretation."; 826 case NIGHT: 827 return "Event occurs during the night. The exact time is unspecified and established by institution convention or patient interpretation."; 828 case PHS: 829 return "Event occurs [offset] after subject goes to sleep. The exact time is unspecified and established by institution convention or patient interpretation."; 830 case HS: 831 return ""; 832 case WAKE: 833 return ""; 834 case C: 835 return ""; 836 case CM: 837 return ""; 838 case CD: 839 return ""; 840 case CV: 841 return ""; 842 case AC: 843 return ""; 844 case ACM: 845 return ""; 846 case ACD: 847 return ""; 848 case ACV: 849 return ""; 850 case PC: 851 return ""; 852 case PCM: 853 return ""; 854 case PCD: 855 return ""; 856 case PCV: 857 return ""; 858 case NULL: 859 return null; 860 default: 861 return "?"; 862 } 863 } 864 865 public String getDisplay() { 866 switch (this) { 867 case MORN: 868 return "Morning"; 869 case MORN_EARLY: 870 return "Early Morning"; 871 case MORN_LATE: 872 return "Late Morning"; 873 case NOON: 874 return "Noon"; 875 case AFT: 876 return "Afternoon"; 877 case AFT_EARLY: 878 return "Early Afternoon"; 879 case AFT_LATE: 880 return "Late Afternoon"; 881 case EVE: 882 return "Evening"; 883 case EVE_EARLY: 884 return "Early Evening"; 885 case EVE_LATE: 886 return "Late Evening"; 887 case NIGHT: 888 return "Night"; 889 case PHS: 890 return "After Sleep"; 891 case HS: 892 return "HS"; 893 case WAKE: 894 return "WAKE"; 895 case C: 896 return "C"; 897 case CM: 898 return "CM"; 899 case CD: 900 return "CD"; 901 case CV: 902 return "CV"; 903 case AC: 904 return "AC"; 905 case ACM: 906 return "ACM"; 907 case ACD: 908 return "ACD"; 909 case ACV: 910 return "ACV"; 911 case PC: 912 return "PC"; 913 case PCM: 914 return "PCM"; 915 case PCD: 916 return "PCD"; 917 case PCV: 918 return "PCV"; 919 case NULL: 920 return null; 921 default: 922 return "?"; 923 } 924 } 925 } 926 927 public static class EventTimingEnumFactory implements EnumFactory<EventTiming> { 928 public EventTiming fromCode(String codeString) throws IllegalArgumentException { 929 if (codeString == null || "".equals(codeString)) 930 if (codeString == null || "".equals(codeString)) 931 return null; 932 if ("MORN".equals(codeString)) 933 return EventTiming.MORN; 934 if ("MORN.early".equals(codeString)) 935 return EventTiming.MORN_EARLY; 936 if ("MORN.late".equals(codeString)) 937 return EventTiming.MORN_LATE; 938 if ("NOON".equals(codeString)) 939 return EventTiming.NOON; 940 if ("AFT".equals(codeString)) 941 return EventTiming.AFT; 942 if ("AFT.early".equals(codeString)) 943 return EventTiming.AFT_EARLY; 944 if ("AFT.late".equals(codeString)) 945 return EventTiming.AFT_LATE; 946 if ("EVE".equals(codeString)) 947 return EventTiming.EVE; 948 if ("EVE.early".equals(codeString)) 949 return EventTiming.EVE_EARLY; 950 if ("EVE.late".equals(codeString)) 951 return EventTiming.EVE_LATE; 952 if ("NIGHT".equals(codeString)) 953 return EventTiming.NIGHT; 954 if ("PHS".equals(codeString)) 955 return EventTiming.PHS; 956 if ("HS".equals(codeString)) 957 return EventTiming.HS; 958 if ("WAKE".equals(codeString)) 959 return EventTiming.WAKE; 960 if ("C".equals(codeString)) 961 return EventTiming.C; 962 if ("CM".equals(codeString)) 963 return EventTiming.CM; 964 if ("CD".equals(codeString)) 965 return EventTiming.CD; 966 if ("CV".equals(codeString)) 967 return EventTiming.CV; 968 if ("AC".equals(codeString)) 969 return EventTiming.AC; 970 if ("ACM".equals(codeString)) 971 return EventTiming.ACM; 972 if ("ACD".equals(codeString)) 973 return EventTiming.ACD; 974 if ("ACV".equals(codeString)) 975 return EventTiming.ACV; 976 if ("PC".equals(codeString)) 977 return EventTiming.PC; 978 if ("PCM".equals(codeString)) 979 return EventTiming.PCM; 980 if ("PCD".equals(codeString)) 981 return EventTiming.PCD; 982 if ("PCV".equals(codeString)) 983 return EventTiming.PCV; 984 throw new IllegalArgumentException("Unknown EventTiming code '" + codeString + "'"); 985 } 986 987 public Enumeration<EventTiming> fromType(PrimitiveType<?> code) throws FHIRException { 988 if (code == null) 989 return null; 990 if (code.isEmpty()) 991 return new Enumeration<EventTiming>(this, EventTiming.NULL, code); 992 String codeString = code.asStringValue(); 993 if (codeString == null || "".equals(codeString)) 994 return new Enumeration<EventTiming>(this, EventTiming.NULL, code); 995 if ("MORN".equals(codeString)) 996 return new Enumeration<EventTiming>(this, EventTiming.MORN, code); 997 if ("MORN.early".equals(codeString)) 998 return new Enumeration<EventTiming>(this, EventTiming.MORN_EARLY, code); 999 if ("MORN.late".equals(codeString)) 1000 return new Enumeration<EventTiming>(this, EventTiming.MORN_LATE, code); 1001 if ("NOON".equals(codeString)) 1002 return new Enumeration<EventTiming>(this, EventTiming.NOON, code); 1003 if ("AFT".equals(codeString)) 1004 return new Enumeration<EventTiming>(this, EventTiming.AFT, code); 1005 if ("AFT.early".equals(codeString)) 1006 return new Enumeration<EventTiming>(this, EventTiming.AFT_EARLY, code); 1007 if ("AFT.late".equals(codeString)) 1008 return new Enumeration<EventTiming>(this, EventTiming.AFT_LATE, code); 1009 if ("EVE".equals(codeString)) 1010 return new Enumeration<EventTiming>(this, EventTiming.EVE, code); 1011 if ("EVE.early".equals(codeString)) 1012 return new Enumeration<EventTiming>(this, EventTiming.EVE_EARLY, code); 1013 if ("EVE.late".equals(codeString)) 1014 return new Enumeration<EventTiming>(this, EventTiming.EVE_LATE, code); 1015 if ("NIGHT".equals(codeString)) 1016 return new Enumeration<EventTiming>(this, EventTiming.NIGHT, code); 1017 if ("PHS".equals(codeString)) 1018 return new Enumeration<EventTiming>(this, EventTiming.PHS, code); 1019 if ("HS".equals(codeString)) 1020 return new Enumeration<EventTiming>(this, EventTiming.HS, code); 1021 if ("WAKE".equals(codeString)) 1022 return new Enumeration<EventTiming>(this, EventTiming.WAKE, code); 1023 if ("C".equals(codeString)) 1024 return new Enumeration<EventTiming>(this, EventTiming.C, code); 1025 if ("CM".equals(codeString)) 1026 return new Enumeration<EventTiming>(this, EventTiming.CM, code); 1027 if ("CD".equals(codeString)) 1028 return new Enumeration<EventTiming>(this, EventTiming.CD, code); 1029 if ("CV".equals(codeString)) 1030 return new Enumeration<EventTiming>(this, EventTiming.CV, code); 1031 if ("AC".equals(codeString)) 1032 return new Enumeration<EventTiming>(this, EventTiming.AC, code); 1033 if ("ACM".equals(codeString)) 1034 return new Enumeration<EventTiming>(this, EventTiming.ACM, code); 1035 if ("ACD".equals(codeString)) 1036 return new Enumeration<EventTiming>(this, EventTiming.ACD, code); 1037 if ("ACV".equals(codeString)) 1038 return new Enumeration<EventTiming>(this, EventTiming.ACV, code); 1039 if ("PC".equals(codeString)) 1040 return new Enumeration<EventTiming>(this, EventTiming.PC, code); 1041 if ("PCM".equals(codeString)) 1042 return new Enumeration<EventTiming>(this, EventTiming.PCM, code); 1043 if ("PCD".equals(codeString)) 1044 return new Enumeration<EventTiming>(this, EventTiming.PCD, code); 1045 if ("PCV".equals(codeString)) 1046 return new Enumeration<EventTiming>(this, EventTiming.PCV, code); 1047 throw new FHIRException("Unknown EventTiming code '" + codeString + "'"); 1048 } 1049 1050 public String toCode(EventTiming code) { 1051 if (code == EventTiming.MORN) 1052 return "MORN"; 1053 if (code == EventTiming.MORN_EARLY) 1054 return "MORN.early"; 1055 if (code == EventTiming.MORN_LATE) 1056 return "MORN.late"; 1057 if (code == EventTiming.NOON) 1058 return "NOON"; 1059 if (code == EventTiming.AFT) 1060 return "AFT"; 1061 if (code == EventTiming.AFT_EARLY) 1062 return "AFT.early"; 1063 if (code == EventTiming.AFT_LATE) 1064 return "AFT.late"; 1065 if (code == EventTiming.EVE) 1066 return "EVE"; 1067 if (code == EventTiming.EVE_EARLY) 1068 return "EVE.early"; 1069 if (code == EventTiming.EVE_LATE) 1070 return "EVE.late"; 1071 if (code == EventTiming.NIGHT) 1072 return "NIGHT"; 1073 if (code == EventTiming.PHS) 1074 return "PHS"; 1075 if (code == EventTiming.HS) 1076 return "HS"; 1077 if (code == EventTiming.WAKE) 1078 return "WAKE"; 1079 if (code == EventTiming.C) 1080 return "C"; 1081 if (code == EventTiming.CM) 1082 return "CM"; 1083 if (code == EventTiming.CD) 1084 return "CD"; 1085 if (code == EventTiming.CV) 1086 return "CV"; 1087 if (code == EventTiming.AC) 1088 return "AC"; 1089 if (code == EventTiming.ACM) 1090 return "ACM"; 1091 if (code == EventTiming.ACD) 1092 return "ACD"; 1093 if (code == EventTiming.ACV) 1094 return "ACV"; 1095 if (code == EventTiming.PC) 1096 return "PC"; 1097 if (code == EventTiming.PCM) 1098 return "PCM"; 1099 if (code == EventTiming.PCD) 1100 return "PCD"; 1101 if (code == EventTiming.PCV) 1102 return "PCV"; 1103 return "?"; 1104 } 1105 1106 public String toSystem(EventTiming code) { 1107 return code.getSystem(); 1108 } 1109 } 1110 1111 @Block() 1112 public static class TimingRepeatComponent extends Element implements IBaseDatatypeElement { 1113 /** 1114 * Either a duration for the length of the timing schedule, a range of possible 1115 * length, or outer bounds for start and/or end limits of the timing schedule. 1116 */ 1117 @Child(name = "bounds", type = { Duration.class, Range.class, 1118 Period.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1119 @Description(shortDefinition = "Length/Range of lengths, or (Start and/or end) limits", formalDefinition = "Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.") 1120 protected Type bounds; 1121 1122 /** 1123 * A total count of the desired number of repetitions across the duration of the 1124 * entire timing specification. If countMax is present, this element indicates 1125 * the lower bound of the allowed range of count values. 1126 */ 1127 @Child(name = "count", type = { 1128 PositiveIntType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1129 @Description(shortDefinition = "Number of times to repeat", formalDefinition = "A total count of the desired number of repetitions across the duration of the entire timing specification. If countMax is present, this element indicates the lower bound of the allowed range of count values.") 1130 protected PositiveIntType count; 1131 1132 /** 1133 * If present, indicates that the count is a range - so to perform the action 1134 * between [count] and [countMax] times. 1135 */ 1136 @Child(name = "countMax", type = { 1137 PositiveIntType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1138 @Description(shortDefinition = "Maximum number of times to repeat", formalDefinition = "If present, indicates that the count is a range - so to perform the action between [count] and [countMax] times.") 1139 protected PositiveIntType countMax; 1140 1141 /** 1142 * How long this thing happens for when it happens. If durationMax is present, 1143 * this element indicates the lower bound of the allowed range of the duration. 1144 */ 1145 @Child(name = "duration", type = { 1146 DecimalType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1147 @Description(shortDefinition = "How long when it happens", formalDefinition = "How long this thing happens for when it happens. If durationMax is present, this element indicates the lower bound of the allowed range of the duration.") 1148 protected DecimalType duration; 1149 1150 /** 1151 * If present, indicates that the duration is a range - so to perform the action 1152 * between [duration] and [durationMax] time length. 1153 */ 1154 @Child(name = "durationMax", type = { 1155 DecimalType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1156 @Description(shortDefinition = "How long when it happens (Max)", formalDefinition = "If present, indicates that the duration is a range - so to perform the action between [duration] and [durationMax] time length.") 1157 protected DecimalType durationMax; 1158 1159 /** 1160 * The units of time for the duration, in UCUM units. 1161 */ 1162 @Child(name = "durationUnit", type = { 1163 CodeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1164 @Description(shortDefinition = "s | min | h | d | wk | mo | a - unit of time (UCUM)", formalDefinition = "The units of time for the duration, in UCUM units.") 1165 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/units-of-time") 1166 protected Enumeration<UnitsOfTime> durationUnit; 1167 1168 /** 1169 * The number of times to repeat the action within the specified period. If 1170 * frequencyMax is present, this element indicates the lower bound of the 1171 * allowed range of the frequency. 1172 */ 1173 @Child(name = "frequency", type = { 1174 PositiveIntType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1175 @Description(shortDefinition = "Event occurs frequency times per period", formalDefinition = "The number of times to repeat the action within the specified period. If frequencyMax is present, this element indicates the lower bound of the allowed range of the frequency.") 1176 protected PositiveIntType frequency; 1177 1178 /** 1179 * If present, indicates that the frequency is a range - so to repeat between 1180 * [frequency] and [frequencyMax] times within the period or period range. 1181 */ 1182 @Child(name = "frequencyMax", type = { 1183 PositiveIntType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1184 @Description(shortDefinition = "Event occurs up to frequencyMax times per period", formalDefinition = "If present, indicates that the frequency is a range - so to repeat between [frequency] and [frequencyMax] times within the period or period range.") 1185 protected PositiveIntType frequencyMax; 1186 1187 /** 1188 * Indicates the duration of time over which repetitions are to occur; e.g. to 1189 * express "3 times per day", 3 would be the frequency and "1 day" would be the 1190 * period. If periodMax is present, this element indicates the lower bound of 1191 * the allowed range of the period length. 1192 */ 1193 @Child(name = "period", type = { DecimalType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 1194 @Description(shortDefinition = "Event occurs frequency times per period", formalDefinition = "Indicates the duration of time over which repetitions are to occur; e.g. to express \"3 times per day\", 3 would be the frequency and \"1 day\" would be the period. If periodMax is present, this element indicates the lower bound of the allowed range of the period length.") 1195 protected DecimalType period; 1196 1197 /** 1198 * If present, indicates that the period is a range from [period] to 1199 * [periodMax], allowing expressing concepts such as "do this once every 3-5 1200 * days. 1201 */ 1202 @Child(name = "periodMax", type = { 1203 DecimalType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 1204 @Description(shortDefinition = "Upper limit of period (3-4 hours)", formalDefinition = "If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as \"do this once every 3-5 days.") 1205 protected DecimalType periodMax; 1206 1207 /** 1208 * The units of time for the period in UCUM units. 1209 */ 1210 @Child(name = "periodUnit", type = { 1211 CodeType.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 1212 @Description(shortDefinition = "s | min | h | d | wk | mo | a - unit of time (UCUM)", formalDefinition = "The units of time for the period in UCUM units.") 1213 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/units-of-time") 1214 protected Enumeration<UnitsOfTime> periodUnit; 1215 1216 /** 1217 * If one or more days of week is provided, then the action happens only on the 1218 * specified day(s). 1219 */ 1220 @Child(name = "dayOfWeek", type = { 1221 CodeType.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1222 @Description(shortDefinition = "mon | tue | wed | thu | fri | sat | sun", formalDefinition = "If one or more days of week is provided, then the action happens only on the specified day(s).") 1223 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/days-of-week") 1224 protected List<Enumeration<DayOfWeek>> dayOfWeek; 1225 1226 /** 1227 * Specified time of day for action to take place. 1228 */ 1229 @Child(name = "timeOfDay", type = { 1230 TimeType.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1231 @Description(shortDefinition = "Time of day for action", formalDefinition = "Specified time of day for action to take place.") 1232 protected List<TimeType> timeOfDay; 1233 1234 /** 1235 * An approximate time period during the day, potentially linked to an event of 1236 * daily living that indicates when the action should occur. 1237 */ 1238 @Child(name = "when", type = { 1239 CodeType.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1240 @Description(shortDefinition = "Code for time period of occurrence", formalDefinition = "An approximate time period during the day, potentially linked to an event of daily living that indicates when the action should occur.") 1241 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/event-timing") 1242 protected List<Enumeration<EventTiming>> when; 1243 1244 /** 1245 * The number of minutes from the event. If the event code does not indicate 1246 * whether the minutes is before or after the event, then the offset is assumed 1247 * to be after the event. 1248 */ 1249 @Child(name = "offset", type = { 1250 UnsignedIntType.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 1251 @Description(shortDefinition = "Minutes from event (before or after)", formalDefinition = "The number of minutes from the event. If the event code does not indicate whether the minutes is before or after the event, then the offset is assumed to be after the event.") 1252 protected UnsignedIntType offset; 1253 1254 private static final long serialVersionUID = -900253756L; 1255 1256 /** 1257 * Constructor 1258 */ 1259 public TimingRepeatComponent() { 1260 super(); 1261 } 1262 1263 /** 1264 * @return {@link #bounds} (Either a duration for the length of the timing 1265 * schedule, a range of possible length, or outer bounds for start 1266 * and/or end limits of the timing schedule.) 1267 */ 1268 public Type getBounds() { 1269 return this.bounds; 1270 } 1271 1272 /** 1273 * @return {@link #bounds} (Either a duration for the length of the timing 1274 * schedule, a range of possible length, or outer bounds for start 1275 * and/or end limits of the timing schedule.) 1276 */ 1277 public Duration getBoundsDuration() throws FHIRException { 1278 if (this.bounds == null) 1279 this.bounds = new Duration(); 1280 if (!(this.bounds instanceof Duration)) 1281 throw new FHIRException("Type mismatch: the type Duration was expected, but " + this.bounds.getClass().getName() 1282 + " was encountered"); 1283 return (Duration) this.bounds; 1284 } 1285 1286 public boolean hasBoundsDuration() { 1287 return this != null && this.bounds instanceof Duration; 1288 } 1289 1290 /** 1291 * @return {@link #bounds} (Either a duration for the length of the timing 1292 * schedule, a range of possible length, or outer bounds for start 1293 * and/or end limits of the timing schedule.) 1294 */ 1295 public Range getBoundsRange() throws FHIRException { 1296 if (this.bounds == null) 1297 this.bounds = new Range(); 1298 if (!(this.bounds instanceof Range)) 1299 throw new FHIRException( 1300 "Type mismatch: the type Range was expected, but " + this.bounds.getClass().getName() + " was encountered"); 1301 return (Range) this.bounds; 1302 } 1303 1304 public boolean hasBoundsRange() { 1305 return this != null && this.bounds instanceof Range; 1306 } 1307 1308 /** 1309 * @return {@link #bounds} (Either a duration for the length of the timing 1310 * schedule, a range of possible length, or outer bounds for start 1311 * and/or end limits of the timing schedule.) 1312 */ 1313 public Period getBoundsPeriod() throws FHIRException { 1314 if (this.bounds == null) 1315 this.bounds = new Period(); 1316 if (!(this.bounds instanceof Period)) 1317 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.bounds.getClass().getName() 1318 + " was encountered"); 1319 return (Period) this.bounds; 1320 } 1321 1322 public boolean hasBoundsPeriod() { 1323 return this != null && this.bounds instanceof Period; 1324 } 1325 1326 public boolean hasBounds() { 1327 return this.bounds != null && !this.bounds.isEmpty(); 1328 } 1329 1330 /** 1331 * @param value {@link #bounds} (Either a duration for the length of the timing 1332 * schedule, a range of possible length, or outer bounds for start 1333 * and/or end limits of the timing schedule.) 1334 */ 1335 public TimingRepeatComponent setBounds(Type value) { 1336 if (value != null && !(value instanceof Duration || value instanceof Range || value instanceof Period)) 1337 throw new Error("Not the right type for Timing.repeat.bounds[x]: " + value.fhirType()); 1338 this.bounds = value; 1339 return this; 1340 } 1341 1342 /** 1343 * @return {@link #count} (A total count of the desired number of repetitions 1344 * across the duration of the entire timing specification. If countMax 1345 * is present, this element indicates the lower bound of the allowed 1346 * range of count values.). This is the underlying object with id, value 1347 * and extensions. The accessor "getCount" gives direct access to the 1348 * value 1349 */ 1350 public PositiveIntType getCountElement() { 1351 if (this.count == null) 1352 if (Configuration.errorOnAutoCreate()) 1353 throw new Error("Attempt to auto-create TimingRepeatComponent.count"); 1354 else if (Configuration.doAutoCreate()) 1355 this.count = new PositiveIntType(); // bb 1356 return this.count; 1357 } 1358 1359 public boolean hasCountElement() { 1360 return this.count != null && !this.count.isEmpty(); 1361 } 1362 1363 public boolean hasCount() { 1364 return this.count != null && !this.count.isEmpty(); 1365 } 1366 1367 /** 1368 * @param value {@link #count} (A total count of the desired number of 1369 * repetitions across the duration of the entire timing 1370 * specification. If countMax is present, this element indicates 1371 * the lower bound of the allowed range of count values.). This is 1372 * the underlying object with id, value and extensions. The 1373 * accessor "getCount" gives direct access to the value 1374 */ 1375 public TimingRepeatComponent setCountElement(PositiveIntType value) { 1376 this.count = value; 1377 return this; 1378 } 1379 1380 /** 1381 * @return A total count of the desired number of repetitions across the 1382 * duration of the entire timing specification. If countMax is present, 1383 * this element indicates the lower bound of the allowed range of count 1384 * values. 1385 */ 1386 public int getCount() { 1387 return this.count == null || this.count.isEmpty() ? 0 : this.count.getValue(); 1388 } 1389 1390 /** 1391 * @param value A total count of the desired number of repetitions across the 1392 * duration of the entire timing specification. If countMax is 1393 * present, this element indicates the lower bound of the allowed 1394 * range of count values. 1395 */ 1396 public TimingRepeatComponent setCount(int value) { 1397 if (this.count == null) 1398 this.count = new PositiveIntType(); 1399 this.count.setValue(value); 1400 return this; 1401 } 1402 1403 /** 1404 * @return {@link #countMax} (If present, indicates that the count is a range - 1405 * so to perform the action between [count] and [countMax] times.). This 1406 * is the underlying object with id, value and extensions. The accessor 1407 * "getCountMax" gives direct access to the value 1408 */ 1409 public PositiveIntType getCountMaxElement() { 1410 if (this.countMax == null) 1411 if (Configuration.errorOnAutoCreate()) 1412 throw new Error("Attempt to auto-create TimingRepeatComponent.countMax"); 1413 else if (Configuration.doAutoCreate()) 1414 this.countMax = new PositiveIntType(); // bb 1415 return this.countMax; 1416 } 1417 1418 public boolean hasCountMaxElement() { 1419 return this.countMax != null && !this.countMax.isEmpty(); 1420 } 1421 1422 public boolean hasCountMax() { 1423 return this.countMax != null && !this.countMax.isEmpty(); 1424 } 1425 1426 /** 1427 * @param value {@link #countMax} (If present, indicates that the count is a 1428 * range - so to perform the action between [count] and [countMax] 1429 * times.). This is the underlying object with id, value and 1430 * extensions. The accessor "getCountMax" gives direct access to 1431 * the value 1432 */ 1433 public TimingRepeatComponent setCountMaxElement(PositiveIntType value) { 1434 this.countMax = value; 1435 return this; 1436 } 1437 1438 /** 1439 * @return If present, indicates that the count is a range - so to perform the 1440 * action between [count] and [countMax] times. 1441 */ 1442 public int getCountMax() { 1443 return this.countMax == null || this.countMax.isEmpty() ? 0 : this.countMax.getValue(); 1444 } 1445 1446 /** 1447 * @param value If present, indicates that the count is a range - so to perform 1448 * the action between [count] and [countMax] times. 1449 */ 1450 public TimingRepeatComponent setCountMax(int value) { 1451 if (this.countMax == null) 1452 this.countMax = new PositiveIntType(); 1453 this.countMax.setValue(value); 1454 return this; 1455 } 1456 1457 /** 1458 * @return {@link #duration} (How long this thing happens for when it happens. 1459 * If durationMax is present, this element indicates the lower bound of 1460 * the allowed range of the duration.). This is the underlying object 1461 * with id, value and extensions. The accessor "getDuration" gives 1462 * direct access to the value 1463 */ 1464 public DecimalType getDurationElement() { 1465 if (this.duration == null) 1466 if (Configuration.errorOnAutoCreate()) 1467 throw new Error("Attempt to auto-create TimingRepeatComponent.duration"); 1468 else if (Configuration.doAutoCreate()) 1469 this.duration = new DecimalType(); // bb 1470 return this.duration; 1471 } 1472 1473 public boolean hasDurationElement() { 1474 return this.duration != null && !this.duration.isEmpty(); 1475 } 1476 1477 public boolean hasDuration() { 1478 return this.duration != null && !this.duration.isEmpty(); 1479 } 1480 1481 /** 1482 * @param value {@link #duration} (How long this thing happens for when it 1483 * happens. If durationMax is present, this element indicates the 1484 * lower bound of the allowed range of the duration.). This is the 1485 * underlying object with id, value and extensions. The accessor 1486 * "getDuration" gives direct access to the value 1487 */ 1488 public TimingRepeatComponent setDurationElement(DecimalType value) { 1489 this.duration = value; 1490 return this; 1491 } 1492 1493 /** 1494 * @return How long this thing happens for when it happens. If durationMax is 1495 * present, this element indicates the lower bound of the allowed range 1496 * of the duration. 1497 */ 1498 public BigDecimal getDuration() { 1499 return this.duration == null ? null : this.duration.getValue(); 1500 } 1501 1502 /** 1503 * @param value How long this thing happens for when it happens. If durationMax 1504 * is present, this element indicates the lower bound of the 1505 * allowed range of the duration. 1506 */ 1507 public TimingRepeatComponent setDuration(BigDecimal value) { 1508 if (value == null) 1509 this.duration = null; 1510 else { 1511 if (this.duration == null) 1512 this.duration = new DecimalType(); 1513 this.duration.setValue(value); 1514 } 1515 return this; 1516 } 1517 1518 /** 1519 * @param value How long this thing happens for when it happens. If durationMax 1520 * is present, this element indicates the lower bound of the 1521 * allowed range of the duration. 1522 */ 1523 public TimingRepeatComponent setDuration(long value) { 1524 this.duration = new DecimalType(); 1525 this.duration.setValue(value); 1526 return this; 1527 } 1528 1529 /** 1530 * @param value How long this thing happens for when it happens. If durationMax 1531 * is present, this element indicates the lower bound of the 1532 * allowed range of the duration. 1533 */ 1534 public TimingRepeatComponent setDuration(double value) { 1535 this.duration = new DecimalType(); 1536 this.duration.setValue(value); 1537 return this; 1538 } 1539 1540 /** 1541 * @return {@link #durationMax} (If present, indicates that the duration is a 1542 * range - so to perform the action between [duration] and [durationMax] 1543 * time length.). This is the underlying object with id, value and 1544 * extensions. The accessor "getDurationMax" gives direct access to the 1545 * value 1546 */ 1547 public DecimalType getDurationMaxElement() { 1548 if (this.durationMax == null) 1549 if (Configuration.errorOnAutoCreate()) 1550 throw new Error("Attempt to auto-create TimingRepeatComponent.durationMax"); 1551 else if (Configuration.doAutoCreate()) 1552 this.durationMax = new DecimalType(); // bb 1553 return this.durationMax; 1554 } 1555 1556 public boolean hasDurationMaxElement() { 1557 return this.durationMax != null && !this.durationMax.isEmpty(); 1558 } 1559 1560 public boolean hasDurationMax() { 1561 return this.durationMax != null && !this.durationMax.isEmpty(); 1562 } 1563 1564 /** 1565 * @param value {@link #durationMax} (If present, indicates that the duration is 1566 * a range - so to perform the action between [duration] and 1567 * [durationMax] time length.). This is the underlying object with 1568 * id, value and extensions. The accessor "getDurationMax" gives 1569 * direct access to the value 1570 */ 1571 public TimingRepeatComponent setDurationMaxElement(DecimalType value) { 1572 this.durationMax = value; 1573 return this; 1574 } 1575 1576 /** 1577 * @return If present, indicates that the duration is a range - so to perform 1578 * the action between [duration] and [durationMax] time length. 1579 */ 1580 public BigDecimal getDurationMax() { 1581 return this.durationMax == null ? null : this.durationMax.getValue(); 1582 } 1583 1584 /** 1585 * @param value If present, indicates that the duration is a range - so to 1586 * perform the action between [duration] and [durationMax] time 1587 * length. 1588 */ 1589 public TimingRepeatComponent setDurationMax(BigDecimal value) { 1590 if (value == null) 1591 this.durationMax = null; 1592 else { 1593 if (this.durationMax == null) 1594 this.durationMax = new DecimalType(); 1595 this.durationMax.setValue(value); 1596 } 1597 return this; 1598 } 1599 1600 /** 1601 * @param value If present, indicates that the duration is a range - so to 1602 * perform the action between [duration] and [durationMax] time 1603 * length. 1604 */ 1605 public TimingRepeatComponent setDurationMax(long value) { 1606 this.durationMax = new DecimalType(); 1607 this.durationMax.setValue(value); 1608 return this; 1609 } 1610 1611 /** 1612 * @param value If present, indicates that the duration is a range - so to 1613 * perform the action between [duration] and [durationMax] time 1614 * length. 1615 */ 1616 public TimingRepeatComponent setDurationMax(double value) { 1617 this.durationMax = new DecimalType(); 1618 this.durationMax.setValue(value); 1619 return this; 1620 } 1621 1622 /** 1623 * @return {@link #durationUnit} (The units of time for the duration, in UCUM 1624 * units.). This is the underlying object with id, value and extensions. 1625 * The accessor "getDurationUnit" gives direct access to the value 1626 */ 1627 public Enumeration<UnitsOfTime> getDurationUnitElement() { 1628 if (this.durationUnit == null) 1629 if (Configuration.errorOnAutoCreate()) 1630 throw new Error("Attempt to auto-create TimingRepeatComponent.durationUnit"); 1631 else if (Configuration.doAutoCreate()) 1632 this.durationUnit = new Enumeration<UnitsOfTime>(new UnitsOfTimeEnumFactory()); // bb 1633 return this.durationUnit; 1634 } 1635 1636 public boolean hasDurationUnitElement() { 1637 return this.durationUnit != null && !this.durationUnit.isEmpty(); 1638 } 1639 1640 public boolean hasDurationUnit() { 1641 return this.durationUnit != null && !this.durationUnit.isEmpty(); 1642 } 1643 1644 /** 1645 * @param value {@link #durationUnit} (The units of time for the duration, in 1646 * UCUM units.). This is the underlying object with id, value and 1647 * extensions. The accessor "getDurationUnit" gives direct access 1648 * to the value 1649 */ 1650 public TimingRepeatComponent setDurationUnitElement(Enumeration<UnitsOfTime> value) { 1651 this.durationUnit = value; 1652 return this; 1653 } 1654 1655 /** 1656 * @return The units of time for the duration, in UCUM units. 1657 */ 1658 public UnitsOfTime getDurationUnit() { 1659 return this.durationUnit == null ? null : this.durationUnit.getValue(); 1660 } 1661 1662 /** 1663 * @param value The units of time for the duration, in UCUM units. 1664 */ 1665 public TimingRepeatComponent setDurationUnit(UnitsOfTime value) { 1666 if (value == null) 1667 this.durationUnit = null; 1668 else { 1669 if (this.durationUnit == null) 1670 this.durationUnit = new Enumeration<UnitsOfTime>(new UnitsOfTimeEnumFactory()); 1671 this.durationUnit.setValue(value); 1672 } 1673 return this; 1674 } 1675 1676 /** 1677 * @return {@link #frequency} (The number of times to repeat the action within 1678 * the specified period. If frequencyMax is present, this element 1679 * indicates the lower bound of the allowed range of the frequency.). 1680 * This is the underlying object with id, value and extensions. The 1681 * accessor "getFrequency" gives direct access to the value 1682 */ 1683 public PositiveIntType getFrequencyElement() { 1684 if (this.frequency == null) 1685 if (Configuration.errorOnAutoCreate()) 1686 throw new Error("Attempt to auto-create TimingRepeatComponent.frequency"); 1687 else if (Configuration.doAutoCreate()) 1688 this.frequency = new PositiveIntType(); // bb 1689 return this.frequency; 1690 } 1691 1692 public boolean hasFrequencyElement() { 1693 return this.frequency != null && !this.frequency.isEmpty(); 1694 } 1695 1696 public boolean hasFrequency() { 1697 return this.frequency != null && !this.frequency.isEmpty(); 1698 } 1699 1700 /** 1701 * @param value {@link #frequency} (The number of times to repeat the action 1702 * within the specified period. If frequencyMax is present, this 1703 * element indicates the lower bound of the allowed range of the 1704 * frequency.). This is the underlying object with id, value and 1705 * extensions. The accessor "getFrequency" gives direct access to 1706 * the value 1707 */ 1708 public TimingRepeatComponent setFrequencyElement(PositiveIntType value) { 1709 this.frequency = value; 1710 return this; 1711 } 1712 1713 /** 1714 * @return The number of times to repeat the action within the specified period. 1715 * If frequencyMax is present, this element indicates the lower bound of 1716 * the allowed range of the frequency. 1717 */ 1718 public int getFrequency() { 1719 return this.frequency == null || this.frequency.isEmpty() ? 0 : this.frequency.getValue(); 1720 } 1721 1722 /** 1723 * @param value The number of times to repeat the action within the specified 1724 * period. If frequencyMax is present, this element indicates the 1725 * lower bound of the allowed range of the frequency. 1726 */ 1727 public TimingRepeatComponent setFrequency(int value) { 1728 if (this.frequency == null) 1729 this.frequency = new PositiveIntType(); 1730 this.frequency.setValue(value); 1731 return this; 1732 } 1733 1734 /** 1735 * @return {@link #frequencyMax} (If present, indicates that the frequency is a 1736 * range - so to repeat between [frequency] and [frequencyMax] times 1737 * within the period or period range.). This is the underlying object 1738 * with id, value and extensions. The accessor "getFrequencyMax" gives 1739 * direct access to the value 1740 */ 1741 public PositiveIntType getFrequencyMaxElement() { 1742 if (this.frequencyMax == null) 1743 if (Configuration.errorOnAutoCreate()) 1744 throw new Error("Attempt to auto-create TimingRepeatComponent.frequencyMax"); 1745 else if (Configuration.doAutoCreate()) 1746 this.frequencyMax = new PositiveIntType(); // bb 1747 return this.frequencyMax; 1748 } 1749 1750 public boolean hasFrequencyMaxElement() { 1751 return this.frequencyMax != null && !this.frequencyMax.isEmpty(); 1752 } 1753 1754 public boolean hasFrequencyMax() { 1755 return this.frequencyMax != null && !this.frequencyMax.isEmpty(); 1756 } 1757 1758 /** 1759 * @param value {@link #frequencyMax} (If present, indicates that the frequency 1760 * is a range - so to repeat between [frequency] and [frequencyMax] 1761 * times within the period or period range.). This is the 1762 * underlying object with id, value and extensions. The accessor 1763 * "getFrequencyMax" gives direct access to the value 1764 */ 1765 public TimingRepeatComponent setFrequencyMaxElement(PositiveIntType value) { 1766 this.frequencyMax = value; 1767 return this; 1768 } 1769 1770 /** 1771 * @return If present, indicates that the frequency is a range - so to repeat 1772 * between [frequency] and [frequencyMax] times within the period or 1773 * period range. 1774 */ 1775 public int getFrequencyMax() { 1776 return this.frequencyMax == null || this.frequencyMax.isEmpty() ? 0 : this.frequencyMax.getValue(); 1777 } 1778 1779 /** 1780 * @param value If present, indicates that the frequency is a range - so to 1781 * repeat between [frequency] and [frequencyMax] times within the 1782 * period or period range. 1783 */ 1784 public TimingRepeatComponent setFrequencyMax(int value) { 1785 if (this.frequencyMax == null) 1786 this.frequencyMax = new PositiveIntType(); 1787 this.frequencyMax.setValue(value); 1788 return this; 1789 } 1790 1791 /** 1792 * @return {@link #period} (Indicates the duration of time over which 1793 * repetitions are to occur; e.g. to express "3 times per day", 3 would 1794 * be the frequency and "1 day" would be the period. If periodMax is 1795 * present, this element indicates the lower bound of the allowed range 1796 * of the period length.). This is the underlying object with id, value 1797 * and extensions. The accessor "getPeriod" gives direct access to the 1798 * value 1799 */ 1800 public DecimalType getPeriodElement() { 1801 if (this.period == null) 1802 if (Configuration.errorOnAutoCreate()) 1803 throw new Error("Attempt to auto-create TimingRepeatComponent.period"); 1804 else if (Configuration.doAutoCreate()) 1805 this.period = new DecimalType(); // bb 1806 return this.period; 1807 } 1808 1809 public boolean hasPeriodElement() { 1810 return this.period != null && !this.period.isEmpty(); 1811 } 1812 1813 public boolean hasPeriod() { 1814 return this.period != null && !this.period.isEmpty(); 1815 } 1816 1817 /** 1818 * @param value {@link #period} (Indicates the duration of time over which 1819 * repetitions are to occur; e.g. to express "3 times per day", 3 1820 * would be the frequency and "1 day" would be the period. If 1821 * periodMax is present, this element indicates the lower bound of 1822 * the allowed range of the period length.). This is the underlying 1823 * object with id, value and extensions. The accessor "getPeriod" 1824 * gives direct access to the value 1825 */ 1826 public TimingRepeatComponent setPeriodElement(DecimalType value) { 1827 this.period = value; 1828 return this; 1829 } 1830 1831 /** 1832 * @return Indicates the duration of time over which repetitions are to occur; 1833 * e.g. to express "3 times per day", 3 would be the frequency and "1 1834 * day" would be the period. If periodMax is present, this element 1835 * indicates the lower bound of the allowed range of the period length. 1836 */ 1837 public BigDecimal getPeriod() { 1838 return this.period == null ? null : this.period.getValue(); 1839 } 1840 1841 /** 1842 * @param value Indicates the duration of time over which repetitions are to 1843 * occur; e.g. to express "3 times per day", 3 would be the 1844 * frequency and "1 day" would be the period. If periodMax is 1845 * present, this element indicates the lower bound of the allowed 1846 * range of the period length. 1847 */ 1848 public TimingRepeatComponent setPeriod(BigDecimal value) { 1849 if (value == null) 1850 this.period = null; 1851 else { 1852 if (this.period == null) 1853 this.period = new DecimalType(); 1854 this.period.setValue(value); 1855 } 1856 return this; 1857 } 1858 1859 /** 1860 * @param value Indicates the duration of time over which repetitions are to 1861 * occur; e.g. to express "3 times per day", 3 would be the 1862 * frequency and "1 day" would be the period. If periodMax is 1863 * present, this element indicates the lower bound of the allowed 1864 * range of the period length. 1865 */ 1866 public TimingRepeatComponent setPeriod(long value) { 1867 this.period = new DecimalType(); 1868 this.period.setValue(value); 1869 return this; 1870 } 1871 1872 /** 1873 * @param value Indicates the duration of time over which repetitions are to 1874 * occur; e.g. to express "3 times per day", 3 would be the 1875 * frequency and "1 day" would be the period. If periodMax is 1876 * present, this element indicates the lower bound of the allowed 1877 * range of the period length. 1878 */ 1879 public TimingRepeatComponent setPeriod(double value) { 1880 this.period = new DecimalType(); 1881 this.period.setValue(value); 1882 return this; 1883 } 1884 1885 /** 1886 * @return {@link #periodMax} (If present, indicates that the period is a range 1887 * from [period] to [periodMax], allowing expressing concepts such as 1888 * "do this once every 3-5 days.). This is the underlying object with 1889 * id, value and extensions. The accessor "getPeriodMax" gives direct 1890 * access to the value 1891 */ 1892 public DecimalType getPeriodMaxElement() { 1893 if (this.periodMax == null) 1894 if (Configuration.errorOnAutoCreate()) 1895 throw new Error("Attempt to auto-create TimingRepeatComponent.periodMax"); 1896 else if (Configuration.doAutoCreate()) 1897 this.periodMax = new DecimalType(); // bb 1898 return this.periodMax; 1899 } 1900 1901 public boolean hasPeriodMaxElement() { 1902 return this.periodMax != null && !this.periodMax.isEmpty(); 1903 } 1904 1905 public boolean hasPeriodMax() { 1906 return this.periodMax != null && !this.periodMax.isEmpty(); 1907 } 1908 1909 /** 1910 * @param value {@link #periodMax} (If present, indicates that the period is a 1911 * range from [period] to [periodMax], allowing expressing concepts 1912 * such as "do this once every 3-5 days.). This is the underlying 1913 * object with id, value and extensions. The accessor 1914 * "getPeriodMax" gives direct access to the value 1915 */ 1916 public TimingRepeatComponent setPeriodMaxElement(DecimalType value) { 1917 this.periodMax = value; 1918 return this; 1919 } 1920 1921 /** 1922 * @return If present, indicates that the period is a range from [period] to 1923 * [periodMax], allowing expressing concepts such as "do this once every 1924 * 3-5 days. 1925 */ 1926 public BigDecimal getPeriodMax() { 1927 return this.periodMax == null ? null : this.periodMax.getValue(); 1928 } 1929 1930 /** 1931 * @param value If present, indicates that the period is a range from [period] 1932 * to [periodMax], allowing expressing concepts such as "do this 1933 * once every 3-5 days. 1934 */ 1935 public TimingRepeatComponent setPeriodMax(BigDecimal value) { 1936 if (value == null) 1937 this.periodMax = null; 1938 else { 1939 if (this.periodMax == null) 1940 this.periodMax = new DecimalType(); 1941 this.periodMax.setValue(value); 1942 } 1943 return this; 1944 } 1945 1946 /** 1947 * @param value If present, indicates that the period is a range from [period] 1948 * to [periodMax], allowing expressing concepts such as "do this 1949 * once every 3-5 days. 1950 */ 1951 public TimingRepeatComponent setPeriodMax(long value) { 1952 this.periodMax = new DecimalType(); 1953 this.periodMax.setValue(value); 1954 return this; 1955 } 1956 1957 /** 1958 * @param value If present, indicates that the period is a range from [period] 1959 * to [periodMax], allowing expressing concepts such as "do this 1960 * once every 3-5 days. 1961 */ 1962 public TimingRepeatComponent setPeriodMax(double value) { 1963 this.periodMax = new DecimalType(); 1964 this.periodMax.setValue(value); 1965 return this; 1966 } 1967 1968 /** 1969 * @return {@link #periodUnit} (The units of time for the period in UCUM 1970 * units.). This is the underlying object with id, value and extensions. 1971 * The accessor "getPeriodUnit" gives direct access to the value 1972 */ 1973 public Enumeration<UnitsOfTime> getPeriodUnitElement() { 1974 if (this.periodUnit == null) 1975 if (Configuration.errorOnAutoCreate()) 1976 throw new Error("Attempt to auto-create TimingRepeatComponent.periodUnit"); 1977 else if (Configuration.doAutoCreate()) 1978 this.periodUnit = new Enumeration<UnitsOfTime>(new UnitsOfTimeEnumFactory()); // bb 1979 return this.periodUnit; 1980 } 1981 1982 public boolean hasPeriodUnitElement() { 1983 return this.periodUnit != null && !this.periodUnit.isEmpty(); 1984 } 1985 1986 public boolean hasPeriodUnit() { 1987 return this.periodUnit != null && !this.periodUnit.isEmpty(); 1988 } 1989 1990 /** 1991 * @param value {@link #periodUnit} (The units of time for the period in UCUM 1992 * units.). This is the underlying object with id, value and 1993 * extensions. The accessor "getPeriodUnit" gives direct access to 1994 * the value 1995 */ 1996 public TimingRepeatComponent setPeriodUnitElement(Enumeration<UnitsOfTime> value) { 1997 this.periodUnit = value; 1998 return this; 1999 } 2000 2001 /** 2002 * @return The units of time for the period in UCUM units. 2003 */ 2004 public UnitsOfTime getPeriodUnit() { 2005 return this.periodUnit == null ? null : this.periodUnit.getValue(); 2006 } 2007 2008 /** 2009 * @param value The units of time for the period in UCUM units. 2010 */ 2011 public TimingRepeatComponent setPeriodUnit(UnitsOfTime value) { 2012 if (value == null) 2013 this.periodUnit = null; 2014 else { 2015 if (this.periodUnit == null) 2016 this.periodUnit = new Enumeration<UnitsOfTime>(new UnitsOfTimeEnumFactory()); 2017 this.periodUnit.setValue(value); 2018 } 2019 return this; 2020 } 2021 2022 /** 2023 * @return {@link #dayOfWeek} (If one or more days of week is provided, then the 2024 * action happens only on the specified day(s).) 2025 */ 2026 public List<Enumeration<DayOfWeek>> getDayOfWeek() { 2027 if (this.dayOfWeek == null) 2028 this.dayOfWeek = new ArrayList<Enumeration<DayOfWeek>>(); 2029 return this.dayOfWeek; 2030 } 2031 2032 /** 2033 * @return Returns a reference to <code>this</code> for easy method chaining 2034 */ 2035 public TimingRepeatComponent setDayOfWeek(List<Enumeration<DayOfWeek>> theDayOfWeek) { 2036 this.dayOfWeek = theDayOfWeek; 2037 return this; 2038 } 2039 2040 public boolean hasDayOfWeek() { 2041 if (this.dayOfWeek == null) 2042 return false; 2043 for (Enumeration<DayOfWeek> item : this.dayOfWeek) 2044 if (!item.isEmpty()) 2045 return true; 2046 return false; 2047 } 2048 2049 /** 2050 * @return {@link #dayOfWeek} (If one or more days of week is provided, then the 2051 * action happens only on the specified day(s).) 2052 */ 2053 public Enumeration<DayOfWeek> addDayOfWeekElement() {// 2 2054 Enumeration<DayOfWeek> t = new Enumeration<DayOfWeek>(new DayOfWeekEnumFactory()); 2055 if (this.dayOfWeek == null) 2056 this.dayOfWeek = new ArrayList<Enumeration<DayOfWeek>>(); 2057 this.dayOfWeek.add(t); 2058 return t; 2059 } 2060 2061 /** 2062 * @param value {@link #dayOfWeek} (If one or more days of week is provided, 2063 * then the action happens only on the specified day(s).) 2064 */ 2065 public TimingRepeatComponent addDayOfWeek(DayOfWeek value) { // 1 2066 Enumeration<DayOfWeek> t = new Enumeration<DayOfWeek>(new DayOfWeekEnumFactory()); 2067 t.setValue(value); 2068 if (this.dayOfWeek == null) 2069 this.dayOfWeek = new ArrayList<Enumeration<DayOfWeek>>(); 2070 this.dayOfWeek.add(t); 2071 return this; 2072 } 2073 2074 /** 2075 * @param value {@link #dayOfWeek} (If one or more days of week is provided, 2076 * then the action happens only on the specified day(s).) 2077 */ 2078 public boolean hasDayOfWeek(DayOfWeek value) { 2079 if (this.dayOfWeek == null) 2080 return false; 2081 for (Enumeration<DayOfWeek> v : this.dayOfWeek) 2082 if (v.getValue().equals(value)) // code 2083 return true; 2084 return false; 2085 } 2086 2087 /** 2088 * @return {@link #timeOfDay} (Specified time of day for action to take place.) 2089 */ 2090 public List<TimeType> getTimeOfDay() { 2091 if (this.timeOfDay == null) 2092 this.timeOfDay = new ArrayList<TimeType>(); 2093 return this.timeOfDay; 2094 } 2095 2096 /** 2097 * @return Returns a reference to <code>this</code> for easy method chaining 2098 */ 2099 public TimingRepeatComponent setTimeOfDay(List<TimeType> theTimeOfDay) { 2100 this.timeOfDay = theTimeOfDay; 2101 return this; 2102 } 2103 2104 public boolean hasTimeOfDay() { 2105 if (this.timeOfDay == null) 2106 return false; 2107 for (TimeType item : this.timeOfDay) 2108 if (!item.isEmpty()) 2109 return true; 2110 return false; 2111 } 2112 2113 /** 2114 * @return {@link #timeOfDay} (Specified time of day for action to take place.) 2115 */ 2116 public TimeType addTimeOfDayElement() {// 2 2117 TimeType t = new TimeType(); 2118 if (this.timeOfDay == null) 2119 this.timeOfDay = new ArrayList<TimeType>(); 2120 this.timeOfDay.add(t); 2121 return t; 2122 } 2123 2124 /** 2125 * @param value {@link #timeOfDay} (Specified time of day for action to take 2126 * place.) 2127 */ 2128 public TimingRepeatComponent addTimeOfDay(String value) { // 1 2129 TimeType t = new TimeType(); 2130 t.setValue(value); 2131 if (this.timeOfDay == null) 2132 this.timeOfDay = new ArrayList<TimeType>(); 2133 this.timeOfDay.add(t); 2134 return this; 2135 } 2136 2137 /** 2138 * @param value {@link #timeOfDay} (Specified time of day for action to take 2139 * place.) 2140 */ 2141 public boolean hasTimeOfDay(String value) { 2142 if (this.timeOfDay == null) 2143 return false; 2144 for (TimeType v : this.timeOfDay) 2145 if (v.getValue().equals(value)) // time 2146 return true; 2147 return false; 2148 } 2149 2150 /** 2151 * @return {@link #when} (An approximate time period during the day, potentially 2152 * linked to an event of daily living that indicates when the action 2153 * should occur.) 2154 */ 2155 public List<Enumeration<EventTiming>> getWhen() { 2156 if (this.when == null) 2157 this.when = new ArrayList<Enumeration<EventTiming>>(); 2158 return this.when; 2159 } 2160 2161 /** 2162 * @return Returns a reference to <code>this</code> for easy method chaining 2163 */ 2164 public TimingRepeatComponent setWhen(List<Enumeration<EventTiming>> theWhen) { 2165 this.when = theWhen; 2166 return this; 2167 } 2168 2169 public boolean hasWhen() { 2170 if (this.when == null) 2171 return false; 2172 for (Enumeration<EventTiming> item : this.when) 2173 if (!item.isEmpty()) 2174 return true; 2175 return false; 2176 } 2177 2178 /** 2179 * @return {@link #when} (An approximate time period during the day, potentially 2180 * linked to an event of daily living that indicates when the action 2181 * should occur.) 2182 */ 2183 public Enumeration<EventTiming> addWhenElement() {// 2 2184 Enumeration<EventTiming> t = new Enumeration<EventTiming>(new EventTimingEnumFactory()); 2185 if (this.when == null) 2186 this.when = new ArrayList<Enumeration<EventTiming>>(); 2187 this.when.add(t); 2188 return t; 2189 } 2190 2191 /** 2192 * @param value {@link #when} (An approximate time period during the day, 2193 * potentially linked to an event of daily living that indicates 2194 * when the action should occur.) 2195 */ 2196 public TimingRepeatComponent addWhen(EventTiming value) { // 1 2197 Enumeration<EventTiming> t = new Enumeration<EventTiming>(new EventTimingEnumFactory()); 2198 t.setValue(value); 2199 if (this.when == null) 2200 this.when = new ArrayList<Enumeration<EventTiming>>(); 2201 this.when.add(t); 2202 return this; 2203 } 2204 2205 /** 2206 * @param value {@link #when} (An approximate time period during the day, 2207 * potentially linked to an event of daily living that indicates 2208 * when the action should occur.) 2209 */ 2210 public boolean hasWhen(EventTiming value) { 2211 if (this.when == null) 2212 return false; 2213 for (Enumeration<EventTiming> v : this.when) 2214 if (v.getValue().equals(value)) // code 2215 return true; 2216 return false; 2217 } 2218 2219 /** 2220 * @return {@link #offset} (The number of minutes from the event. If the event 2221 * code does not indicate whether the minutes is before or after the 2222 * event, then the offset is assumed to be after the event.). This is 2223 * the underlying object with id, value and extensions. The accessor 2224 * "getOffset" gives direct access to the value 2225 */ 2226 public UnsignedIntType getOffsetElement() { 2227 if (this.offset == null) 2228 if (Configuration.errorOnAutoCreate()) 2229 throw new Error("Attempt to auto-create TimingRepeatComponent.offset"); 2230 else if (Configuration.doAutoCreate()) 2231 this.offset = new UnsignedIntType(); // bb 2232 return this.offset; 2233 } 2234 2235 public boolean hasOffsetElement() { 2236 return this.offset != null && !this.offset.isEmpty(); 2237 } 2238 2239 public boolean hasOffset() { 2240 return this.offset != null && !this.offset.isEmpty(); 2241 } 2242 2243 /** 2244 * @param value {@link #offset} (The number of minutes from the event. If the 2245 * event code does not indicate whether the minutes is before or 2246 * after the event, then the offset is assumed to be after the 2247 * event.). This is the underlying object with id, value and 2248 * extensions. The accessor "getOffset" gives direct access to the 2249 * value 2250 */ 2251 public TimingRepeatComponent setOffsetElement(UnsignedIntType value) { 2252 this.offset = value; 2253 return this; 2254 } 2255 2256 /** 2257 * @return The number of minutes from the event. If the event code does not 2258 * indicate whether the minutes is before or after the event, then the 2259 * offset is assumed to be after the event. 2260 */ 2261 public int getOffset() { 2262 return this.offset == null || this.offset.isEmpty() ? 0 : this.offset.getValue(); 2263 } 2264 2265 /** 2266 * @param value The number of minutes from the event. If the event code does not 2267 * indicate whether the minutes is before or after the event, then 2268 * the offset is assumed to be after the event. 2269 */ 2270 public TimingRepeatComponent setOffset(int value) { 2271 if (this.offset == null) 2272 this.offset = new UnsignedIntType(); 2273 this.offset.setValue(value); 2274 return this; 2275 } 2276 2277 protected void listChildren(List<Property> children) { 2278 super.listChildren(children); 2279 children.add(new Property("bounds[x]", "Duration|Range|Period", 2280 "Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.", 2281 0, 1, bounds)); 2282 children.add(new Property("count", "positiveInt", 2283 "A total count of the desired number of repetitions across the duration of the entire timing specification. If countMax is present, this element indicates the lower bound of the allowed range of count values.", 2284 0, 1, count)); 2285 children.add(new Property("countMax", "positiveInt", 2286 "If present, indicates that the count is a range - so to perform the action between [count] and [countMax] times.", 2287 0, 1, countMax)); 2288 children.add(new Property("duration", "decimal", 2289 "How long this thing happens for when it happens. If durationMax is present, this element indicates the lower bound of the allowed range of the duration.", 2290 0, 1, duration)); 2291 children.add(new Property("durationMax", "decimal", 2292 "If present, indicates that the duration is a range - so to perform the action between [duration] and [durationMax] time length.", 2293 0, 1, durationMax)); 2294 children.add(new Property("durationUnit", "code", "The units of time for the duration, in UCUM units.", 0, 1, 2295 durationUnit)); 2296 children.add(new Property("frequency", "positiveInt", 2297 "The number of times to repeat the action within the specified period. If frequencyMax is present, this element indicates the lower bound of the allowed range of the frequency.", 2298 0, 1, frequency)); 2299 children.add(new Property("frequencyMax", "positiveInt", 2300 "If present, indicates that the frequency is a range - so to repeat between [frequency] and [frequencyMax] times within the period or period range.", 2301 0, 1, frequencyMax)); 2302 children.add(new Property("period", "decimal", 2303 "Indicates the duration of time over which repetitions are to occur; e.g. to express \"3 times per day\", 3 would be the frequency and \"1 day\" would be the period. If periodMax is present, this element indicates the lower bound of the allowed range of the period length.", 2304 0, 1, period)); 2305 children.add(new Property("periodMax", "decimal", 2306 "If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as \"do this once every 3-5 days.", 2307 0, 1, periodMax)); 2308 children 2309 .add(new Property("periodUnit", "code", "The units of time for the period in UCUM units.", 0, 1, periodUnit)); 2310 children.add(new Property("dayOfWeek", "code", 2311 "If one or more days of week is provided, then the action happens only on the specified day(s).", 0, 2312 java.lang.Integer.MAX_VALUE, dayOfWeek)); 2313 children.add(new Property("timeOfDay", "time", "Specified time of day for action to take place.", 0, 2314 java.lang.Integer.MAX_VALUE, timeOfDay)); 2315 children.add(new Property("when", "code", 2316 "An approximate time period during the day, potentially linked to an event of daily living that indicates when the action should occur.", 2317 0, java.lang.Integer.MAX_VALUE, when)); 2318 children.add(new Property("offset", "unsignedInt", 2319 "The number of minutes from the event. If the event code does not indicate whether the minutes is before or after the event, then the offset is assumed to be after the event.", 2320 0, 1, offset)); 2321 } 2322 2323 @Override 2324 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2325 switch (_hash) { 2326 case -1149635157: 2327 /* bounds[x] */ return new Property("bounds[x]", "Duration|Range|Period", 2328 "Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.", 2329 0, 1, bounds); 2330 case -1383205195: 2331 /* bounds */ return new Property("bounds[x]", "Duration|Range|Period", 2332 "Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.", 2333 0, 1, bounds); 2334 case -189193367: 2335 /* boundsDuration */ return new Property("bounds[x]", "Duration|Range|Period", 2336 "Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.", 2337 0, 1, bounds); 2338 case -1001768056: 2339 /* boundsRange */ return new Property("bounds[x]", "Duration|Range|Period", 2340 "Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.", 2341 0, 1, bounds); 2342 case -1043481386: 2343 /* boundsPeriod */ return new Property("bounds[x]", "Duration|Range|Period", 2344 "Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.", 2345 0, 1, bounds); 2346 case 94851343: 2347 /* count */ return new Property("count", "positiveInt", 2348 "A total count of the desired number of repetitions across the duration of the entire timing specification. If countMax is present, this element indicates the lower bound of the allowed range of count values.", 2349 0, 1, count); 2350 case -372044331: 2351 /* countMax */ return new Property("countMax", "positiveInt", 2352 "If present, indicates that the count is a range - so to perform the action between [count] and [countMax] times.", 2353 0, 1, countMax); 2354 case -1992012396: 2355 /* duration */ return new Property("duration", "decimal", 2356 "How long this thing happens for when it happens. If durationMax is present, this element indicates the lower bound of the allowed range of the duration.", 2357 0, 1, duration); 2358 case -478083280: 2359 /* durationMax */ return new Property("durationMax", "decimal", 2360 "If present, indicates that the duration is a range - so to perform the action between [duration] and [durationMax] time length.", 2361 0, 1, durationMax); 2362 case -1935429320: 2363 /* durationUnit */ return new Property("durationUnit", "code", 2364 "The units of time for the duration, in UCUM units.", 0, 1, durationUnit); 2365 case -70023844: 2366 /* frequency */ return new Property("frequency", "positiveInt", 2367 "The number of times to repeat the action within the specified period. If frequencyMax is present, this element indicates the lower bound of the allowed range of the frequency.", 2368 0, 1, frequency); 2369 case 1273846376: 2370 /* frequencyMax */ return new Property("frequencyMax", "positiveInt", 2371 "If present, indicates that the frequency is a range - so to repeat between [frequency] and [frequencyMax] times within the period or period range.", 2372 0, 1, frequencyMax); 2373 case -991726143: 2374 /* period */ return new Property("period", "decimal", 2375 "Indicates the duration of time over which repetitions are to occur; e.g. to express \"3 times per day\", 3 would be the frequency and \"1 day\" would be the period. If periodMax is present, this element indicates the lower bound of the allowed range of the period length.", 2376 0, 1, period); 2377 case 566580195: 2378 /* periodMax */ return new Property("periodMax", "decimal", 2379 "If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as \"do this once every 3-5 days.", 2380 0, 1, periodMax); 2381 case 384367333: 2382 /* periodUnit */ return new Property("periodUnit", "code", "The units of time for the period in UCUM units.", 0, 2383 1, periodUnit); 2384 case -730552025: 2385 /* dayOfWeek */ return new Property("dayOfWeek", "code", 2386 "If one or more days of week is provided, then the action happens only on the specified day(s).", 0, 2387 java.lang.Integer.MAX_VALUE, dayOfWeek); 2388 case 21434232: 2389 /* timeOfDay */ return new Property("timeOfDay", "time", "Specified time of day for action to take place.", 0, 2390 java.lang.Integer.MAX_VALUE, timeOfDay); 2391 case 3648314: 2392 /* when */ return new Property("when", "code", 2393 "An approximate time period during the day, potentially linked to an event of daily living that indicates when the action should occur.", 2394 0, java.lang.Integer.MAX_VALUE, when); 2395 case -1019779949: 2396 /* offset */ return new Property("offset", "unsignedInt", 2397 "The number of minutes from the event. If the event code does not indicate whether the minutes is before or after the event, then the offset is assumed to be after the event.", 2398 0, 1, offset); 2399 default: 2400 return super.getNamedProperty(_hash, _name, _checkValid); 2401 } 2402 2403 } 2404 2405 @Override 2406 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2407 switch (hash) { 2408 case -1383205195: 2409 /* bounds */ return this.bounds == null ? new Base[0] : new Base[] { this.bounds }; // Type 2410 case 94851343: 2411 /* count */ return this.count == null ? new Base[0] : new Base[] { this.count }; // PositiveIntType 2412 case -372044331: 2413 /* countMax */ return this.countMax == null ? new Base[0] : new Base[] { this.countMax }; // PositiveIntType 2414 case -1992012396: 2415 /* duration */ return this.duration == null ? new Base[0] : new Base[] { this.duration }; // DecimalType 2416 case -478083280: 2417 /* durationMax */ return this.durationMax == null ? new Base[0] : new Base[] { this.durationMax }; // DecimalType 2418 case -1935429320: 2419 /* durationUnit */ return this.durationUnit == null ? new Base[0] : new Base[] { this.durationUnit }; // Enumeration<UnitsOfTime> 2420 case -70023844: 2421 /* frequency */ return this.frequency == null ? new Base[0] : new Base[] { this.frequency }; // PositiveIntType 2422 case 1273846376: 2423 /* frequencyMax */ return this.frequencyMax == null ? new Base[0] : new Base[] { this.frequencyMax }; // PositiveIntType 2424 case -991726143: 2425 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // DecimalType 2426 case 566580195: 2427 /* periodMax */ return this.periodMax == null ? new Base[0] : new Base[] { this.periodMax }; // DecimalType 2428 case 384367333: 2429 /* periodUnit */ return this.periodUnit == null ? new Base[0] : new Base[] { this.periodUnit }; // Enumeration<UnitsOfTime> 2430 case -730552025: 2431 /* dayOfWeek */ return this.dayOfWeek == null ? new Base[0] 2432 : this.dayOfWeek.toArray(new Base[this.dayOfWeek.size()]); // Enumeration<DayOfWeek> 2433 case 21434232: 2434 /* timeOfDay */ return this.timeOfDay == null ? new Base[0] 2435 : this.timeOfDay.toArray(new Base[this.timeOfDay.size()]); // TimeType 2436 case 3648314: 2437 /* when */ return this.when == null ? new Base[0] : this.when.toArray(new Base[this.when.size()]); // Enumeration<EventTiming> 2438 case -1019779949: 2439 /* offset */ return this.offset == null ? new Base[0] : new Base[] { this.offset }; // UnsignedIntType 2440 default: 2441 return super.getProperty(hash, name, checkValid); 2442 } 2443 2444 } 2445 2446 @Override 2447 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2448 switch (hash) { 2449 case -1383205195: // bounds 2450 this.bounds = castToType(value); // Type 2451 return value; 2452 case 94851343: // count 2453 this.count = castToPositiveInt(value); // PositiveIntType 2454 return value; 2455 case -372044331: // countMax 2456 this.countMax = castToPositiveInt(value); // PositiveIntType 2457 return value; 2458 case -1992012396: // duration 2459 this.duration = castToDecimal(value); // DecimalType 2460 return value; 2461 case -478083280: // durationMax 2462 this.durationMax = castToDecimal(value); // DecimalType 2463 return value; 2464 case -1935429320: // durationUnit 2465 value = new UnitsOfTimeEnumFactory().fromType(castToCode(value)); 2466 this.durationUnit = (Enumeration) value; // Enumeration<UnitsOfTime> 2467 return value; 2468 case -70023844: // frequency 2469 this.frequency = castToPositiveInt(value); // PositiveIntType 2470 return value; 2471 case 1273846376: // frequencyMax 2472 this.frequencyMax = castToPositiveInt(value); // PositiveIntType 2473 return value; 2474 case -991726143: // period 2475 this.period = castToDecimal(value); // DecimalType 2476 return value; 2477 case 566580195: // periodMax 2478 this.periodMax = castToDecimal(value); // DecimalType 2479 return value; 2480 case 384367333: // periodUnit 2481 value = new UnitsOfTimeEnumFactory().fromType(castToCode(value)); 2482 this.periodUnit = (Enumeration) value; // Enumeration<UnitsOfTime> 2483 return value; 2484 case -730552025: // dayOfWeek 2485 value = new DayOfWeekEnumFactory().fromType(castToCode(value)); 2486 this.getDayOfWeek().add((Enumeration) value); // Enumeration<DayOfWeek> 2487 return value; 2488 case 21434232: // timeOfDay 2489 this.getTimeOfDay().add(castToTime(value)); // TimeType 2490 return value; 2491 case 3648314: // when 2492 value = new EventTimingEnumFactory().fromType(castToCode(value)); 2493 this.getWhen().add((Enumeration) value); // Enumeration<EventTiming> 2494 return value; 2495 case -1019779949: // offset 2496 this.offset = castToUnsignedInt(value); // UnsignedIntType 2497 return value; 2498 default: 2499 return super.setProperty(hash, name, value); 2500 } 2501 2502 } 2503 2504 @Override 2505 public Base setProperty(String name, Base value) throws FHIRException { 2506 if (name.equals("bounds[x]")) { 2507 this.bounds = castToType(value); // Type 2508 } else if (name.equals("count")) { 2509 this.count = castToPositiveInt(value); // PositiveIntType 2510 } else if (name.equals("countMax")) { 2511 this.countMax = castToPositiveInt(value); // PositiveIntType 2512 } else if (name.equals("duration")) { 2513 this.duration = castToDecimal(value); // DecimalType 2514 } else if (name.equals("durationMax")) { 2515 this.durationMax = castToDecimal(value); // DecimalType 2516 } else if (name.equals("durationUnit")) { 2517 value = new UnitsOfTimeEnumFactory().fromType(castToCode(value)); 2518 this.durationUnit = (Enumeration) value; // Enumeration<UnitsOfTime> 2519 } else if (name.equals("frequency")) { 2520 this.frequency = castToPositiveInt(value); // PositiveIntType 2521 } else if (name.equals("frequencyMax")) { 2522 this.frequencyMax = castToPositiveInt(value); // PositiveIntType 2523 } else if (name.equals("period")) { 2524 this.period = castToDecimal(value); // DecimalType 2525 } else if (name.equals("periodMax")) { 2526 this.periodMax = castToDecimal(value); // DecimalType 2527 } else if (name.equals("periodUnit")) { 2528 value = new UnitsOfTimeEnumFactory().fromType(castToCode(value)); 2529 this.periodUnit = (Enumeration) value; // Enumeration<UnitsOfTime> 2530 } else if (name.equals("dayOfWeek")) { 2531 value = new DayOfWeekEnumFactory().fromType(castToCode(value)); 2532 this.getDayOfWeek().add((Enumeration) value); 2533 } else if (name.equals("timeOfDay")) { 2534 this.getTimeOfDay().add(castToTime(value)); 2535 } else if (name.equals("when")) { 2536 value = new EventTimingEnumFactory().fromType(castToCode(value)); 2537 this.getWhen().add((Enumeration) value); 2538 } else if (name.equals("offset")) { 2539 this.offset = castToUnsignedInt(value); // UnsignedIntType 2540 } else 2541 return super.setProperty(name, value); 2542 return value; 2543 } 2544 2545 @Override 2546 public Base makeProperty(int hash, String name) throws FHIRException { 2547 switch (hash) { 2548 case -1149635157: 2549 return getBounds(); 2550 case -1383205195: 2551 return getBounds(); 2552 case 94851343: 2553 return getCountElement(); 2554 case -372044331: 2555 return getCountMaxElement(); 2556 case -1992012396: 2557 return getDurationElement(); 2558 case -478083280: 2559 return getDurationMaxElement(); 2560 case -1935429320: 2561 return getDurationUnitElement(); 2562 case -70023844: 2563 return getFrequencyElement(); 2564 case 1273846376: 2565 return getFrequencyMaxElement(); 2566 case -991726143: 2567 return getPeriodElement(); 2568 case 566580195: 2569 return getPeriodMaxElement(); 2570 case 384367333: 2571 return getPeriodUnitElement(); 2572 case -730552025: 2573 return addDayOfWeekElement(); 2574 case 21434232: 2575 return addTimeOfDayElement(); 2576 case 3648314: 2577 return addWhenElement(); 2578 case -1019779949: 2579 return getOffsetElement(); 2580 default: 2581 return super.makeProperty(hash, name); 2582 } 2583 2584 } 2585 2586 @Override 2587 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2588 switch (hash) { 2589 case -1383205195: 2590 /* bounds */ return new String[] { "Duration", "Range", "Period" }; 2591 case 94851343: 2592 /* count */ return new String[] { "positiveInt" }; 2593 case -372044331: 2594 /* countMax */ return new String[] { "positiveInt" }; 2595 case -1992012396: 2596 /* duration */ return new String[] { "decimal" }; 2597 case -478083280: 2598 /* durationMax */ return new String[] { "decimal" }; 2599 case -1935429320: 2600 /* durationUnit */ return new String[] { "code" }; 2601 case -70023844: 2602 /* frequency */ return new String[] { "positiveInt" }; 2603 case 1273846376: 2604 /* frequencyMax */ return new String[] { "positiveInt" }; 2605 case -991726143: 2606 /* period */ return new String[] { "decimal" }; 2607 case 566580195: 2608 /* periodMax */ return new String[] { "decimal" }; 2609 case 384367333: 2610 /* periodUnit */ return new String[] { "code" }; 2611 case -730552025: 2612 /* dayOfWeek */ return new String[] { "code" }; 2613 case 21434232: 2614 /* timeOfDay */ return new String[] { "time" }; 2615 case 3648314: 2616 /* when */ return new String[] { "code" }; 2617 case -1019779949: 2618 /* offset */ return new String[] { "unsignedInt" }; 2619 default: 2620 return super.getTypesForProperty(hash, name); 2621 } 2622 2623 } 2624 2625 @Override 2626 public Base addChild(String name) throws FHIRException { 2627 if (name.equals("boundsDuration")) { 2628 this.bounds = new Duration(); 2629 return this.bounds; 2630 } else if (name.equals("boundsRange")) { 2631 this.bounds = new Range(); 2632 return this.bounds; 2633 } else if (name.equals("boundsPeriod")) { 2634 this.bounds = new Period(); 2635 return this.bounds; 2636 } else if (name.equals("count")) { 2637 throw new FHIRException("Cannot call addChild on a singleton property Timing.count"); 2638 } else if (name.equals("countMax")) { 2639 throw new FHIRException("Cannot call addChild on a singleton property Timing.countMax"); 2640 } else if (name.equals("duration")) { 2641 throw new FHIRException("Cannot call addChild on a singleton property Timing.duration"); 2642 } else if (name.equals("durationMax")) { 2643 throw new FHIRException("Cannot call addChild on a singleton property Timing.durationMax"); 2644 } else if (name.equals("durationUnit")) { 2645 throw new FHIRException("Cannot call addChild on a singleton property Timing.durationUnit"); 2646 } else if (name.equals("frequency")) { 2647 throw new FHIRException("Cannot call addChild on a singleton property Timing.frequency"); 2648 } else if (name.equals("frequencyMax")) { 2649 throw new FHIRException("Cannot call addChild on a singleton property Timing.frequencyMax"); 2650 } else if (name.equals("period")) { 2651 throw new FHIRException("Cannot call addChild on a singleton property Timing.period"); 2652 } else if (name.equals("periodMax")) { 2653 throw new FHIRException("Cannot call addChild on a singleton property Timing.periodMax"); 2654 } else if (name.equals("periodUnit")) { 2655 throw new FHIRException("Cannot call addChild on a singleton property Timing.periodUnit"); 2656 } else if (name.equals("dayOfWeek")) { 2657 throw new FHIRException("Cannot call addChild on a singleton property Timing.dayOfWeek"); 2658 } else if (name.equals("timeOfDay")) { 2659 throw new FHIRException("Cannot call addChild on a singleton property Timing.timeOfDay"); 2660 } else if (name.equals("when")) { 2661 throw new FHIRException("Cannot call addChild on a singleton property Timing.when"); 2662 } else if (name.equals("offset")) { 2663 throw new FHIRException("Cannot call addChild on a singleton property Timing.offset"); 2664 } else 2665 return super.addChild(name); 2666 } 2667 2668 public TimingRepeatComponent copy() { 2669 TimingRepeatComponent dst = new TimingRepeatComponent(); 2670 copyValues(dst); 2671 return dst; 2672 } 2673 2674 public void copyValues(TimingRepeatComponent dst) { 2675 super.copyValues(dst); 2676 dst.bounds = bounds == null ? null : bounds.copy(); 2677 dst.count = count == null ? null : count.copy(); 2678 dst.countMax = countMax == null ? null : countMax.copy(); 2679 dst.duration = duration == null ? null : duration.copy(); 2680 dst.durationMax = durationMax == null ? null : durationMax.copy(); 2681 dst.durationUnit = durationUnit == null ? null : durationUnit.copy(); 2682 dst.frequency = frequency == null ? null : frequency.copy(); 2683 dst.frequencyMax = frequencyMax == null ? null : frequencyMax.copy(); 2684 dst.period = period == null ? null : period.copy(); 2685 dst.periodMax = periodMax == null ? null : periodMax.copy(); 2686 dst.periodUnit = periodUnit == null ? null : periodUnit.copy(); 2687 if (dayOfWeek != null) { 2688 dst.dayOfWeek = new ArrayList<Enumeration<DayOfWeek>>(); 2689 for (Enumeration<DayOfWeek> i : dayOfWeek) 2690 dst.dayOfWeek.add(i.copy()); 2691 } 2692 ; 2693 if (timeOfDay != null) { 2694 dst.timeOfDay = new ArrayList<TimeType>(); 2695 for (TimeType i : timeOfDay) 2696 dst.timeOfDay.add(i.copy()); 2697 } 2698 ; 2699 if (when != null) { 2700 dst.when = new ArrayList<Enumeration<EventTiming>>(); 2701 for (Enumeration<EventTiming> i : when) 2702 dst.when.add(i.copy()); 2703 } 2704 ; 2705 dst.offset = offset == null ? null : offset.copy(); 2706 } 2707 2708 @Override 2709 public boolean equalsDeep(Base other_) { 2710 if (!super.equalsDeep(other_)) 2711 return false; 2712 if (!(other_ instanceof TimingRepeatComponent)) 2713 return false; 2714 TimingRepeatComponent o = (TimingRepeatComponent) other_; 2715 return compareDeep(bounds, o.bounds, true) && compareDeep(count, o.count, true) 2716 && compareDeep(countMax, o.countMax, true) && compareDeep(duration, o.duration, true) 2717 && compareDeep(durationMax, o.durationMax, true) && compareDeep(durationUnit, o.durationUnit, true) 2718 && compareDeep(frequency, o.frequency, true) && compareDeep(frequencyMax, o.frequencyMax, true) 2719 && compareDeep(period, o.period, true) && compareDeep(periodMax, o.periodMax, true) 2720 && compareDeep(periodUnit, o.periodUnit, true) && compareDeep(dayOfWeek, o.dayOfWeek, true) 2721 && compareDeep(timeOfDay, o.timeOfDay, true) && compareDeep(when, o.when, true) 2722 && compareDeep(offset, o.offset, true); 2723 } 2724 2725 @Override 2726 public boolean equalsShallow(Base other_) { 2727 if (!super.equalsShallow(other_)) 2728 return false; 2729 if (!(other_ instanceof TimingRepeatComponent)) 2730 return false; 2731 TimingRepeatComponent o = (TimingRepeatComponent) other_; 2732 return compareValues(count, o.count, true) && compareValues(countMax, o.countMax, true) 2733 && compareValues(duration, o.duration, true) && compareValues(durationMax, o.durationMax, true) 2734 && compareValues(durationUnit, o.durationUnit, true) && compareValues(frequency, o.frequency, true) 2735 && compareValues(frequencyMax, o.frequencyMax, true) && compareValues(period, o.period, true) 2736 && compareValues(periodMax, o.periodMax, true) && compareValues(periodUnit, o.periodUnit, true) 2737 && compareValues(dayOfWeek, o.dayOfWeek, true) && compareValues(timeOfDay, o.timeOfDay, true) 2738 && compareValues(when, o.when, true) && compareValues(offset, o.offset, true); 2739 } 2740 2741 public boolean isEmpty() { 2742 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(bounds, count, countMax, duration, durationMax, 2743 durationUnit, frequency, frequencyMax, period, periodMax, periodUnit, dayOfWeek, timeOfDay, when, offset); 2744 } 2745 2746 public String fhirType() { 2747 return "Timing.repeat"; 2748 2749 } 2750 2751 } 2752 2753 /** 2754 * Identifies specific times when the event occurs. 2755 */ 2756 @Child(name = "event", type = { 2757 DateTimeType.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2758 @Description(shortDefinition = "When the event occurs", formalDefinition = "Identifies specific times when the event occurs.") 2759 protected List<DateTimeType> event; 2760 2761 /** 2762 * A set of rules that describe when the event is scheduled. 2763 */ 2764 @Child(name = "repeat", type = {}, order = 1, min = 0, max = 1, modifier = false, summary = true) 2765 @Description(shortDefinition = "When the event is to occur", formalDefinition = "A set of rules that describe when the event is scheduled.") 2766 protected TimingRepeatComponent repeat; 2767 2768 /** 2769 * A code for the timing schedule (or just text in code.text). Some codes such 2770 * as BID are ubiquitous, but many institutions define their own additional 2771 * codes. If a code is provided, the code is understood to be a complete 2772 * statement of whatever is specified in the structured timing data, and either 2773 * the code or the data may be used to interpret the Timing, with the exception 2774 * that .repeat.bounds still applies over the code (and is not contained in the 2775 * code). 2776 */ 2777 @Child(name = "code", type = { CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2778 @Description(shortDefinition = "BID | TID | QID | AM | PM | QD | QOD | +", formalDefinition = "A code for the timing schedule (or just text in code.text). Some codes such as BID are ubiquitous, but many institutions define their own additional codes. If a code is provided, the code is understood to be a complete statement of whatever is specified in the structured timing data, and either the code or the data may be used to interpret the Timing, with the exception that .repeat.bounds still applies over the code (and is not contained in the code).") 2779 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/timing-abbreviation") 2780 protected CodeableConcept code; 2781 2782 private static final long serialVersionUID = 791565112L; 2783 2784 /** 2785 * Constructor 2786 */ 2787 public Timing() { 2788 super(); 2789 } 2790 2791 /** 2792 * @return {@link #event} (Identifies specific times when the event occurs.) 2793 */ 2794 public List<DateTimeType> getEvent() { 2795 if (this.event == null) 2796 this.event = new ArrayList<DateTimeType>(); 2797 return this.event; 2798 } 2799 2800 /** 2801 * @return Returns a reference to <code>this</code> for easy method chaining 2802 */ 2803 public Timing setEvent(List<DateTimeType> theEvent) { 2804 this.event = theEvent; 2805 return this; 2806 } 2807 2808 public boolean hasEvent() { 2809 if (this.event == null) 2810 return false; 2811 for (DateTimeType item : this.event) 2812 if (!item.isEmpty()) 2813 return true; 2814 return false; 2815 } 2816 2817 /** 2818 * @return {@link #event} (Identifies specific times when the event occurs.) 2819 */ 2820 public DateTimeType addEventElement() {// 2 2821 DateTimeType t = new DateTimeType(); 2822 if (this.event == null) 2823 this.event = new ArrayList<DateTimeType>(); 2824 this.event.add(t); 2825 return t; 2826 } 2827 2828 /** 2829 * @param value {@link #event} (Identifies specific times when the event 2830 * occurs.) 2831 */ 2832 public Timing addEvent(Date value) { // 1 2833 DateTimeType t = new DateTimeType(); 2834 t.setValue(value); 2835 if (this.event == null) 2836 this.event = new ArrayList<DateTimeType>(); 2837 this.event.add(t); 2838 return this; 2839 } 2840 2841 /** 2842 * @param value {@link #event} (Identifies specific times when the event 2843 * occurs.) 2844 */ 2845 public boolean hasEvent(Date value) { 2846 if (this.event == null) 2847 return false; 2848 for (DateTimeType v : this.event) 2849 if (v.getValue().equals(value)) // dateTime 2850 return true; 2851 return false; 2852 } 2853 2854 /** 2855 * @return {@link #repeat} (A set of rules that describe when the event is 2856 * scheduled.) 2857 */ 2858 public TimingRepeatComponent getRepeat() { 2859 if (this.repeat == null) 2860 if (Configuration.errorOnAutoCreate()) 2861 throw new Error("Attempt to auto-create Timing.repeat"); 2862 else if (Configuration.doAutoCreate()) 2863 this.repeat = new TimingRepeatComponent(); // cc 2864 return this.repeat; 2865 } 2866 2867 public boolean hasRepeat() { 2868 return this.repeat != null && !this.repeat.isEmpty(); 2869 } 2870 2871 /** 2872 * @param value {@link #repeat} (A set of rules that describe when the event is 2873 * scheduled.) 2874 */ 2875 public Timing setRepeat(TimingRepeatComponent value) { 2876 this.repeat = value; 2877 return this; 2878 } 2879 2880 /** 2881 * @return {@link #code} (A code for the timing schedule (or just text in 2882 * code.text). Some codes such as BID are ubiquitous, but many 2883 * institutions define their own additional codes. If a code is 2884 * provided, the code is understood to be a complete statement of 2885 * whatever is specified in the structured timing data, and either the 2886 * code or the data may be used to interpret the Timing, with the 2887 * exception that .repeat.bounds still applies over the code (and is not 2888 * contained in the code).) 2889 */ 2890 public CodeableConcept getCode() { 2891 if (this.code == null) 2892 if (Configuration.errorOnAutoCreate()) 2893 throw new Error("Attempt to auto-create Timing.code"); 2894 else if (Configuration.doAutoCreate()) 2895 this.code = new CodeableConcept(); // cc 2896 return this.code; 2897 } 2898 2899 public boolean hasCode() { 2900 return this.code != null && !this.code.isEmpty(); 2901 } 2902 2903 /** 2904 * @param value {@link #code} (A code for the timing schedule (or just text in 2905 * code.text). Some codes such as BID are ubiquitous, but many 2906 * institutions define their own additional codes. If a code is 2907 * provided, the code is understood to be a complete statement of 2908 * whatever is specified in the structured timing data, and either 2909 * the code or the data may be used to interpret the Timing, with 2910 * the exception that .repeat.bounds still applies over the code 2911 * (and is not contained in the code).) 2912 */ 2913 public Timing setCode(CodeableConcept value) { 2914 this.code = value; 2915 return this; 2916 } 2917 2918 protected void listChildren(List<Property> children) { 2919 super.listChildren(children); 2920 children.add(new Property("event", "dateTime", "Identifies specific times when the event occurs.", 0, 2921 java.lang.Integer.MAX_VALUE, event)); 2922 children.add(new Property("repeat", "", "A set of rules that describe when the event is scheduled.", 0, 1, repeat)); 2923 children.add(new Property("code", "CodeableConcept", 2924 "A code for the timing schedule (or just text in code.text). Some codes such as BID are ubiquitous, but many institutions define their own additional codes. If a code is provided, the code is understood to be a complete statement of whatever is specified in the structured timing data, and either the code or the data may be used to interpret the Timing, with the exception that .repeat.bounds still applies over the code (and is not contained in the code).", 2925 0, 1, code)); 2926 } 2927 2928 @Override 2929 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2930 switch (_hash) { 2931 case 96891546: 2932 /* event */ return new Property("event", "dateTime", "Identifies specific times when the event occurs.", 0, 2933 java.lang.Integer.MAX_VALUE, event); 2934 case -934531685: 2935 /* repeat */ return new Property("repeat", "", "A set of rules that describe when the event is scheduled.", 0, 1, 2936 repeat); 2937 case 3059181: 2938 /* code */ return new Property("code", "CodeableConcept", 2939 "A code for the timing schedule (or just text in code.text). Some codes such as BID are ubiquitous, but many institutions define their own additional codes. If a code is provided, the code is understood to be a complete statement of whatever is specified in the structured timing data, and either the code or the data may be used to interpret the Timing, with the exception that .repeat.bounds still applies over the code (and is not contained in the code).", 2940 0, 1, code); 2941 default: 2942 return super.getNamedProperty(_hash, _name, _checkValid); 2943 } 2944 2945 } 2946 2947 @Override 2948 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2949 switch (hash) { 2950 case 96891546: 2951 /* event */ return this.event == null ? new Base[0] : this.event.toArray(new Base[this.event.size()]); // DateTimeType 2952 case -934531685: 2953 /* repeat */ return this.repeat == null ? new Base[0] : new Base[] { this.repeat }; // TimingRepeatComponent 2954 case 3059181: 2955 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 2956 default: 2957 return super.getProperty(hash, name, checkValid); 2958 } 2959 2960 } 2961 2962 @Override 2963 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2964 switch (hash) { 2965 case 96891546: // event 2966 this.getEvent().add(castToDateTime(value)); // DateTimeType 2967 return value; 2968 case -934531685: // repeat 2969 this.repeat = (TimingRepeatComponent) value; // TimingRepeatComponent 2970 return value; 2971 case 3059181: // code 2972 this.code = castToCodeableConcept(value); // CodeableConcept 2973 return value; 2974 default: 2975 return super.setProperty(hash, name, value); 2976 } 2977 2978 } 2979 2980 @Override 2981 public Base setProperty(String name, Base value) throws FHIRException { 2982 if (name.equals("event")) { 2983 this.getEvent().add(castToDateTime(value)); 2984 } else if (name.equals("repeat")) { 2985 this.repeat = (TimingRepeatComponent) value; // TimingRepeatComponent 2986 } else if (name.equals("code")) { 2987 this.code = castToCodeableConcept(value); // CodeableConcept 2988 } else 2989 return super.setProperty(name, value); 2990 return value; 2991 } 2992 2993 @Override 2994 public Base makeProperty(int hash, String name) throws FHIRException { 2995 switch (hash) { 2996 case 96891546: 2997 return addEventElement(); 2998 case -934531685: 2999 return getRepeat(); 3000 case 3059181: 3001 return getCode(); 3002 default: 3003 return super.makeProperty(hash, name); 3004 } 3005 3006 } 3007 3008 @Override 3009 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3010 switch (hash) { 3011 case 96891546: 3012 /* event */ return new String[] { "dateTime" }; 3013 case -934531685: 3014 /* repeat */ return new String[] {}; 3015 case 3059181: 3016 /* code */ return new String[] { "CodeableConcept" }; 3017 default: 3018 return super.getTypesForProperty(hash, name); 3019 } 3020 3021 } 3022 3023 @Override 3024 public Base addChild(String name) throws FHIRException { 3025 if (name.equals("event")) { 3026 throw new FHIRException("Cannot call addChild on a singleton property Timing.event"); 3027 } else if (name.equals("repeat")) { 3028 this.repeat = new TimingRepeatComponent(); 3029 return this.repeat; 3030 } else if (name.equals("code")) { 3031 this.code = new CodeableConcept(); 3032 return this.code; 3033 } else 3034 return super.addChild(name); 3035 } 3036 3037 public String fhirType() { 3038 return "Timing"; 3039 3040 } 3041 3042 public Timing copy() { 3043 Timing dst = new Timing(); 3044 copyValues(dst); 3045 return dst; 3046 } 3047 3048 public void copyValues(Timing dst) { 3049 super.copyValues(dst); 3050 if (event != null) { 3051 dst.event = new ArrayList<DateTimeType>(); 3052 for (DateTimeType i : event) 3053 dst.event.add(i.copy()); 3054 } 3055 ; 3056 dst.repeat = repeat == null ? null : repeat.copy(); 3057 dst.code = code == null ? null : code.copy(); 3058 } 3059 3060 protected Timing typedCopy() { 3061 return copy(); 3062 } 3063 3064 @Override 3065 public boolean equalsDeep(Base other_) { 3066 if (!super.equalsDeep(other_)) 3067 return false; 3068 if (!(other_ instanceof Timing)) 3069 return false; 3070 Timing o = (Timing) other_; 3071 return compareDeep(event, o.event, true) && compareDeep(repeat, o.repeat, true) && compareDeep(code, o.code, true); 3072 } 3073 3074 @Override 3075 public boolean equalsShallow(Base other_) { 3076 if (!super.equalsShallow(other_)) 3077 return false; 3078 if (!(other_ instanceof Timing)) 3079 return false; 3080 Timing o = (Timing) other_; 3081 return compareValues(event, o.event, true); 3082 } 3083 3084 public boolean isEmpty() { 3085 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(event, repeat, code); 3086 } 3087 3088}