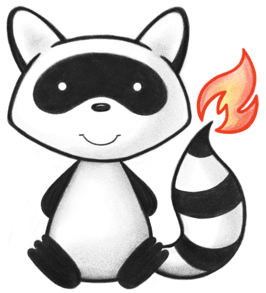
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048 049/** 050 * An authorization for the provision of glasses and/or contact lenses to a 051 * patient. 052 */ 053@ResourceDef(name = "VisionPrescription", profile = "http://hl7.org/fhir/StructureDefinition/VisionPrescription") 054public class VisionPrescription extends DomainResource { 055 056 public enum VisionStatus { 057 /** 058 * The instance is currently in-force. 059 */ 060 ACTIVE, 061 /** 062 * The instance is withdrawn, rescinded or reversed. 063 */ 064 CANCELLED, 065 /** 066 * A new instance the contents of which is not complete. 067 */ 068 DRAFT, 069 /** 070 * The instance was entered in error. 071 */ 072 ENTEREDINERROR, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 078 public static VisionStatus fromCode(String codeString) throws FHIRException { 079 if (codeString == null || "".equals(codeString)) 080 return null; 081 if ("active".equals(codeString)) 082 return ACTIVE; 083 if ("cancelled".equals(codeString)) 084 return CANCELLED; 085 if ("draft".equals(codeString)) 086 return DRAFT; 087 if ("entered-in-error".equals(codeString)) 088 return ENTEREDINERROR; 089 if (Configuration.isAcceptInvalidEnums()) 090 return null; 091 else 092 throw new FHIRException("Unknown VisionStatus code '" + codeString + "'"); 093 } 094 095 public String toCode() { 096 switch (this) { 097 case ACTIVE: 098 return "active"; 099 case CANCELLED: 100 return "cancelled"; 101 case DRAFT: 102 return "draft"; 103 case ENTEREDINERROR: 104 return "entered-in-error"; 105 case NULL: 106 return null; 107 default: 108 return "?"; 109 } 110 } 111 112 public String getSystem() { 113 switch (this) { 114 case ACTIVE: 115 return "http://hl7.org/fhir/fm-status"; 116 case CANCELLED: 117 return "http://hl7.org/fhir/fm-status"; 118 case DRAFT: 119 return "http://hl7.org/fhir/fm-status"; 120 case ENTEREDINERROR: 121 return "http://hl7.org/fhir/fm-status"; 122 case NULL: 123 return null; 124 default: 125 return "?"; 126 } 127 } 128 129 public String getDefinition() { 130 switch (this) { 131 case ACTIVE: 132 return "The instance is currently in-force."; 133 case CANCELLED: 134 return "The instance is withdrawn, rescinded or reversed."; 135 case DRAFT: 136 return "A new instance the contents of which is not complete."; 137 case ENTEREDINERROR: 138 return "The instance was entered in error."; 139 case NULL: 140 return null; 141 default: 142 return "?"; 143 } 144 } 145 146 public String getDisplay() { 147 switch (this) { 148 case ACTIVE: 149 return "Active"; 150 case CANCELLED: 151 return "Cancelled"; 152 case DRAFT: 153 return "Draft"; 154 case ENTEREDINERROR: 155 return "Entered in Error"; 156 case NULL: 157 return null; 158 default: 159 return "?"; 160 } 161 } 162 } 163 164 public static class VisionStatusEnumFactory implements EnumFactory<VisionStatus> { 165 public VisionStatus fromCode(String codeString) throws IllegalArgumentException { 166 if (codeString == null || "".equals(codeString)) 167 if (codeString == null || "".equals(codeString)) 168 return null; 169 if ("active".equals(codeString)) 170 return VisionStatus.ACTIVE; 171 if ("cancelled".equals(codeString)) 172 return VisionStatus.CANCELLED; 173 if ("draft".equals(codeString)) 174 return VisionStatus.DRAFT; 175 if ("entered-in-error".equals(codeString)) 176 return VisionStatus.ENTEREDINERROR; 177 throw new IllegalArgumentException("Unknown VisionStatus code '" + codeString + "'"); 178 } 179 180 public Enumeration<VisionStatus> fromType(PrimitiveType<?> code) throws FHIRException { 181 if (code == null) 182 return null; 183 if (code.isEmpty()) 184 return new Enumeration<VisionStatus>(this, VisionStatus.NULL, code); 185 String codeString = code.asStringValue(); 186 if (codeString == null || "".equals(codeString)) 187 return new Enumeration<VisionStatus>(this, VisionStatus.NULL, code); 188 if ("active".equals(codeString)) 189 return new Enumeration<VisionStatus>(this, VisionStatus.ACTIVE, code); 190 if ("cancelled".equals(codeString)) 191 return new Enumeration<VisionStatus>(this, VisionStatus.CANCELLED, code); 192 if ("draft".equals(codeString)) 193 return new Enumeration<VisionStatus>(this, VisionStatus.DRAFT, code); 194 if ("entered-in-error".equals(codeString)) 195 return new Enumeration<VisionStatus>(this, VisionStatus.ENTEREDINERROR, code); 196 throw new FHIRException("Unknown VisionStatus code '" + codeString + "'"); 197 } 198 199 public String toCode(VisionStatus code) { 200 if (code == VisionStatus.ACTIVE) 201 return "active"; 202 if (code == VisionStatus.CANCELLED) 203 return "cancelled"; 204 if (code == VisionStatus.DRAFT) 205 return "draft"; 206 if (code == VisionStatus.ENTEREDINERROR) 207 return "entered-in-error"; 208 return "?"; 209 } 210 211 public String toSystem(VisionStatus code) { 212 return code.getSystem(); 213 } 214 } 215 216 public enum VisionEyes { 217 /** 218 * Right Eye. 219 */ 220 RIGHT, 221 /** 222 * Left Eye. 223 */ 224 LEFT, 225 /** 226 * added to help the parsers with the generic types 227 */ 228 NULL; 229 230 public static VisionEyes fromCode(String codeString) throws FHIRException { 231 if (codeString == null || "".equals(codeString)) 232 return null; 233 if ("right".equals(codeString)) 234 return RIGHT; 235 if ("left".equals(codeString)) 236 return LEFT; 237 if (Configuration.isAcceptInvalidEnums()) 238 return null; 239 else 240 throw new FHIRException("Unknown VisionEyes code '" + codeString + "'"); 241 } 242 243 public String toCode() { 244 switch (this) { 245 case RIGHT: 246 return "right"; 247 case LEFT: 248 return "left"; 249 case NULL: 250 return null; 251 default: 252 return "?"; 253 } 254 } 255 256 public String getSystem() { 257 switch (this) { 258 case RIGHT: 259 return "http://hl7.org/fhir/vision-eye-codes"; 260 case LEFT: 261 return "http://hl7.org/fhir/vision-eye-codes"; 262 case NULL: 263 return null; 264 default: 265 return "?"; 266 } 267 } 268 269 public String getDefinition() { 270 switch (this) { 271 case RIGHT: 272 return "Right Eye."; 273 case LEFT: 274 return "Left Eye."; 275 case NULL: 276 return null; 277 default: 278 return "?"; 279 } 280 } 281 282 public String getDisplay() { 283 switch (this) { 284 case RIGHT: 285 return "Right Eye"; 286 case LEFT: 287 return "Left Eye"; 288 case NULL: 289 return null; 290 default: 291 return "?"; 292 } 293 } 294 } 295 296 public static class VisionEyesEnumFactory implements EnumFactory<VisionEyes> { 297 public VisionEyes fromCode(String codeString) throws IllegalArgumentException { 298 if (codeString == null || "".equals(codeString)) 299 if (codeString == null || "".equals(codeString)) 300 return null; 301 if ("right".equals(codeString)) 302 return VisionEyes.RIGHT; 303 if ("left".equals(codeString)) 304 return VisionEyes.LEFT; 305 throw new IllegalArgumentException("Unknown VisionEyes code '" + codeString + "'"); 306 } 307 308 public Enumeration<VisionEyes> fromType(PrimitiveType<?> code) throws FHIRException { 309 if (code == null) 310 return null; 311 if (code.isEmpty()) 312 return new Enumeration<VisionEyes>(this, VisionEyes.NULL, code); 313 String codeString = code.asStringValue(); 314 if (codeString == null || "".equals(codeString)) 315 return new Enumeration<VisionEyes>(this, VisionEyes.NULL, code); 316 if ("right".equals(codeString)) 317 return new Enumeration<VisionEyes>(this, VisionEyes.RIGHT, code); 318 if ("left".equals(codeString)) 319 return new Enumeration<VisionEyes>(this, VisionEyes.LEFT, code); 320 throw new FHIRException("Unknown VisionEyes code '" + codeString + "'"); 321 } 322 323 public String toCode(VisionEyes code) { 324 if (code == VisionEyes.RIGHT) 325 return "right"; 326 if (code == VisionEyes.LEFT) 327 return "left"; 328 return "?"; 329 } 330 331 public String toSystem(VisionEyes code) { 332 return code.getSystem(); 333 } 334 } 335 336 public enum VisionBase { 337 /** 338 * top. 339 */ 340 UP, 341 /** 342 * bottom. 343 */ 344 DOWN, 345 /** 346 * inner edge. 347 */ 348 IN, 349 /** 350 * outer edge. 351 */ 352 OUT, 353 /** 354 * added to help the parsers with the generic types 355 */ 356 NULL; 357 358 public static VisionBase fromCode(String codeString) throws FHIRException { 359 if (codeString == null || "".equals(codeString)) 360 return null; 361 if ("up".equals(codeString)) 362 return UP; 363 if ("down".equals(codeString)) 364 return DOWN; 365 if ("in".equals(codeString)) 366 return IN; 367 if ("out".equals(codeString)) 368 return OUT; 369 if (Configuration.isAcceptInvalidEnums()) 370 return null; 371 else 372 throw new FHIRException("Unknown VisionBase code '" + codeString + "'"); 373 } 374 375 public String toCode() { 376 switch (this) { 377 case UP: 378 return "up"; 379 case DOWN: 380 return "down"; 381 case IN: 382 return "in"; 383 case OUT: 384 return "out"; 385 case NULL: 386 return null; 387 default: 388 return "?"; 389 } 390 } 391 392 public String getSystem() { 393 switch (this) { 394 case UP: 395 return "http://hl7.org/fhir/vision-base-codes"; 396 case DOWN: 397 return "http://hl7.org/fhir/vision-base-codes"; 398 case IN: 399 return "http://hl7.org/fhir/vision-base-codes"; 400 case OUT: 401 return "http://hl7.org/fhir/vision-base-codes"; 402 case NULL: 403 return null; 404 default: 405 return "?"; 406 } 407 } 408 409 public String getDefinition() { 410 switch (this) { 411 case UP: 412 return "top."; 413 case DOWN: 414 return "bottom."; 415 case IN: 416 return "inner edge."; 417 case OUT: 418 return "outer edge."; 419 case NULL: 420 return null; 421 default: 422 return "?"; 423 } 424 } 425 426 public String getDisplay() { 427 switch (this) { 428 case UP: 429 return "Up"; 430 case DOWN: 431 return "Down"; 432 case IN: 433 return "In"; 434 case OUT: 435 return "Out"; 436 case NULL: 437 return null; 438 default: 439 return "?"; 440 } 441 } 442 } 443 444 public static class VisionBaseEnumFactory implements EnumFactory<VisionBase> { 445 public VisionBase fromCode(String codeString) throws IllegalArgumentException { 446 if (codeString == null || "".equals(codeString)) 447 if (codeString == null || "".equals(codeString)) 448 return null; 449 if ("up".equals(codeString)) 450 return VisionBase.UP; 451 if ("down".equals(codeString)) 452 return VisionBase.DOWN; 453 if ("in".equals(codeString)) 454 return VisionBase.IN; 455 if ("out".equals(codeString)) 456 return VisionBase.OUT; 457 throw new IllegalArgumentException("Unknown VisionBase code '" + codeString + "'"); 458 } 459 460 public Enumeration<VisionBase> fromType(PrimitiveType<?> code) throws FHIRException { 461 if (code == null) 462 return null; 463 if (code.isEmpty()) 464 return new Enumeration<VisionBase>(this, VisionBase.NULL, code); 465 String codeString = code.asStringValue(); 466 if (codeString == null || "".equals(codeString)) 467 return new Enumeration<VisionBase>(this, VisionBase.NULL, code); 468 if ("up".equals(codeString)) 469 return new Enumeration<VisionBase>(this, VisionBase.UP, code); 470 if ("down".equals(codeString)) 471 return new Enumeration<VisionBase>(this, VisionBase.DOWN, code); 472 if ("in".equals(codeString)) 473 return new Enumeration<VisionBase>(this, VisionBase.IN, code); 474 if ("out".equals(codeString)) 475 return new Enumeration<VisionBase>(this, VisionBase.OUT, code); 476 throw new FHIRException("Unknown VisionBase code '" + codeString + "'"); 477 } 478 479 public String toCode(VisionBase code) { 480 if (code == VisionBase.UP) 481 return "up"; 482 if (code == VisionBase.DOWN) 483 return "down"; 484 if (code == VisionBase.IN) 485 return "in"; 486 if (code == VisionBase.OUT) 487 return "out"; 488 return "?"; 489 } 490 491 public String toSystem(VisionBase code) { 492 return code.getSystem(); 493 } 494 } 495 496 @Block() 497 public static class VisionPrescriptionLensSpecificationComponent extends BackboneElement 498 implements IBaseBackboneElement { 499 /** 500 * Identifies the type of vision correction product which is required for the 501 * patient. 502 */ 503 @Child(name = "product", type = { 504 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 505 @Description(shortDefinition = "Product to be supplied", formalDefinition = "Identifies the type of vision correction product which is required for the patient.") 506 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/vision-product") 507 protected CodeableConcept product; 508 509 /** 510 * The eye for which the lens specification applies. 511 */ 512 @Child(name = "eye", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 513 @Description(shortDefinition = "right | left", formalDefinition = "The eye for which the lens specification applies.") 514 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/vision-eye-codes") 515 protected Enumeration<VisionEyes> eye; 516 517 /** 518 * Lens power measured in dioptres (0.25 units). 519 */ 520 @Child(name = "sphere", type = { 521 DecimalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 522 @Description(shortDefinition = "Power of the lens", formalDefinition = "Lens power measured in dioptres (0.25 units).") 523 protected DecimalType sphere; 524 525 /** 526 * Power adjustment for astigmatism measured in dioptres (0.25 units). 527 */ 528 @Child(name = "cylinder", type = { 529 DecimalType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 530 @Description(shortDefinition = "Lens power for astigmatism", formalDefinition = "Power adjustment for astigmatism measured in dioptres (0.25 units).") 531 protected DecimalType cylinder; 532 533 /** 534 * Adjustment for astigmatism measured in integer degrees. 535 */ 536 @Child(name = "axis", type = { IntegerType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 537 @Description(shortDefinition = "Lens meridian which contain no power for astigmatism", formalDefinition = "Adjustment for astigmatism measured in integer degrees.") 538 protected IntegerType axis; 539 540 /** 541 * Allows for adjustment on two axis. 542 */ 543 @Child(name = "prism", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 544 @Description(shortDefinition = "Eye alignment compensation", formalDefinition = "Allows for adjustment on two axis.") 545 protected List<PrismComponent> prism; 546 547 /** 548 * Power adjustment for multifocal lenses measured in dioptres (0.25 units). 549 */ 550 @Child(name = "add", type = { DecimalType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 551 @Description(shortDefinition = "Added power for multifocal levels", formalDefinition = "Power adjustment for multifocal lenses measured in dioptres (0.25 units).") 552 protected DecimalType add; 553 554 /** 555 * Contact lens power measured in dioptres (0.25 units). 556 */ 557 @Child(name = "power", type = { DecimalType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 558 @Description(shortDefinition = "Contact lens power", formalDefinition = "Contact lens power measured in dioptres (0.25 units).") 559 protected DecimalType power; 560 561 /** 562 * Back curvature measured in millimetres. 563 */ 564 @Child(name = "backCurve", type = { 565 DecimalType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 566 @Description(shortDefinition = "Contact lens back curvature", formalDefinition = "Back curvature measured in millimetres.") 567 protected DecimalType backCurve; 568 569 /** 570 * Contact lens diameter measured in millimetres. 571 */ 572 @Child(name = "diameter", type = { 573 DecimalType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 574 @Description(shortDefinition = "Contact lens diameter", formalDefinition = "Contact lens diameter measured in millimetres.") 575 protected DecimalType diameter; 576 577 /** 578 * The recommended maximum wear period for the lens. 579 */ 580 @Child(name = "duration", type = { 581 Quantity.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 582 @Description(shortDefinition = "Lens wear duration", formalDefinition = "The recommended maximum wear period for the lens.") 583 protected Quantity duration; 584 585 /** 586 * Special color or pattern. 587 */ 588 @Child(name = "color", type = { StringType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 589 @Description(shortDefinition = "Color required", formalDefinition = "Special color or pattern.") 590 protected StringType color; 591 592 /** 593 * Brand recommendations or restrictions. 594 */ 595 @Child(name = "brand", type = { StringType.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 596 @Description(shortDefinition = "Brand required", formalDefinition = "Brand recommendations or restrictions.") 597 protected StringType brand; 598 599 /** 600 * Notes for special requirements such as coatings and lens materials. 601 */ 602 @Child(name = "note", type = { 603 Annotation.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 604 @Description(shortDefinition = "Notes for coatings", formalDefinition = "Notes for special requirements such as coatings and lens materials.") 605 protected List<Annotation> note; 606 607 private static final long serialVersionUID = 688924460L; 608 609 /** 610 * Constructor 611 */ 612 public VisionPrescriptionLensSpecificationComponent() { 613 super(); 614 } 615 616 /** 617 * Constructor 618 */ 619 public VisionPrescriptionLensSpecificationComponent(CodeableConcept product, Enumeration<VisionEyes> eye) { 620 super(); 621 this.product = product; 622 this.eye = eye; 623 } 624 625 /** 626 * @return {@link #product} (Identifies the type of vision correction product 627 * which is required for the patient.) 628 */ 629 public CodeableConcept getProduct() { 630 if (this.product == null) 631 if (Configuration.errorOnAutoCreate()) 632 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.product"); 633 else if (Configuration.doAutoCreate()) 634 this.product = new CodeableConcept(); // cc 635 return this.product; 636 } 637 638 public boolean hasProduct() { 639 return this.product != null && !this.product.isEmpty(); 640 } 641 642 /** 643 * @param value {@link #product} (Identifies the type of vision correction 644 * product which is required for the patient.) 645 */ 646 public VisionPrescriptionLensSpecificationComponent setProduct(CodeableConcept value) { 647 this.product = value; 648 return this; 649 } 650 651 /** 652 * @return {@link #eye} (The eye for which the lens specification applies.). 653 * This is the underlying object with id, value and extensions. The 654 * accessor "getEye" gives direct access to the value 655 */ 656 public Enumeration<VisionEyes> getEyeElement() { 657 if (this.eye == null) 658 if (Configuration.errorOnAutoCreate()) 659 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.eye"); 660 else if (Configuration.doAutoCreate()) 661 this.eye = new Enumeration<VisionEyes>(new VisionEyesEnumFactory()); // bb 662 return this.eye; 663 } 664 665 public boolean hasEyeElement() { 666 return this.eye != null && !this.eye.isEmpty(); 667 } 668 669 public boolean hasEye() { 670 return this.eye != null && !this.eye.isEmpty(); 671 } 672 673 /** 674 * @param value {@link #eye} (The eye for which the lens specification 675 * applies.). This is the underlying object with id, value and 676 * extensions. The accessor "getEye" gives direct access to the 677 * value 678 */ 679 public VisionPrescriptionLensSpecificationComponent setEyeElement(Enumeration<VisionEyes> value) { 680 this.eye = value; 681 return this; 682 } 683 684 /** 685 * @return The eye for which the lens specification applies. 686 */ 687 public VisionEyes getEye() { 688 return this.eye == null ? null : this.eye.getValue(); 689 } 690 691 /** 692 * @param value The eye for which the lens specification applies. 693 */ 694 public VisionPrescriptionLensSpecificationComponent setEye(VisionEyes value) { 695 if (this.eye == null) 696 this.eye = new Enumeration<VisionEyes>(new VisionEyesEnumFactory()); 697 this.eye.setValue(value); 698 return this; 699 } 700 701 /** 702 * @return {@link #sphere} (Lens power measured in dioptres (0.25 units).). This 703 * is the underlying object with id, value and extensions. The accessor 704 * "getSphere" gives direct access to the value 705 */ 706 public DecimalType getSphereElement() { 707 if (this.sphere == null) 708 if (Configuration.errorOnAutoCreate()) 709 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.sphere"); 710 else if (Configuration.doAutoCreate()) 711 this.sphere = new DecimalType(); // bb 712 return this.sphere; 713 } 714 715 public boolean hasSphereElement() { 716 return this.sphere != null && !this.sphere.isEmpty(); 717 } 718 719 public boolean hasSphere() { 720 return this.sphere != null && !this.sphere.isEmpty(); 721 } 722 723 /** 724 * @param value {@link #sphere} (Lens power measured in dioptres (0.25 units).). 725 * This is the underlying object with id, value and extensions. The 726 * accessor "getSphere" gives direct access to the value 727 */ 728 public VisionPrescriptionLensSpecificationComponent setSphereElement(DecimalType value) { 729 this.sphere = value; 730 return this; 731 } 732 733 /** 734 * @return Lens power measured in dioptres (0.25 units). 735 */ 736 public BigDecimal getSphere() { 737 return this.sphere == null ? null : this.sphere.getValue(); 738 } 739 740 /** 741 * @param value Lens power measured in dioptres (0.25 units). 742 */ 743 public VisionPrescriptionLensSpecificationComponent setSphere(BigDecimal value) { 744 if (value == null) 745 this.sphere = null; 746 else { 747 if (this.sphere == null) 748 this.sphere = new DecimalType(); 749 this.sphere.setValue(value); 750 } 751 return this; 752 } 753 754 /** 755 * @param value Lens power measured in dioptres (0.25 units). 756 */ 757 public VisionPrescriptionLensSpecificationComponent setSphere(long value) { 758 this.sphere = new DecimalType(); 759 this.sphere.setValue(value); 760 return this; 761 } 762 763 /** 764 * @param value Lens power measured in dioptres (0.25 units). 765 */ 766 public VisionPrescriptionLensSpecificationComponent setSphere(double value) { 767 this.sphere = new DecimalType(); 768 this.sphere.setValue(value); 769 return this; 770 } 771 772 /** 773 * @return {@link #cylinder} (Power adjustment for astigmatism measured in 774 * dioptres (0.25 units).). This is the underlying object with id, value 775 * and extensions. The accessor "getCylinder" gives direct access to the 776 * value 777 */ 778 public DecimalType getCylinderElement() { 779 if (this.cylinder == null) 780 if (Configuration.errorOnAutoCreate()) 781 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.cylinder"); 782 else if (Configuration.doAutoCreate()) 783 this.cylinder = new DecimalType(); // bb 784 return this.cylinder; 785 } 786 787 public boolean hasCylinderElement() { 788 return this.cylinder != null && !this.cylinder.isEmpty(); 789 } 790 791 public boolean hasCylinder() { 792 return this.cylinder != null && !this.cylinder.isEmpty(); 793 } 794 795 /** 796 * @param value {@link #cylinder} (Power adjustment for astigmatism measured in 797 * dioptres (0.25 units).). This is the underlying object with id, 798 * value and extensions. The accessor "getCylinder" gives direct 799 * access to the value 800 */ 801 public VisionPrescriptionLensSpecificationComponent setCylinderElement(DecimalType value) { 802 this.cylinder = value; 803 return this; 804 } 805 806 /** 807 * @return Power adjustment for astigmatism measured in dioptres (0.25 units). 808 */ 809 public BigDecimal getCylinder() { 810 return this.cylinder == null ? null : this.cylinder.getValue(); 811 } 812 813 /** 814 * @param value Power adjustment for astigmatism measured in dioptres (0.25 815 * units). 816 */ 817 public VisionPrescriptionLensSpecificationComponent setCylinder(BigDecimal value) { 818 if (value == null) 819 this.cylinder = null; 820 else { 821 if (this.cylinder == null) 822 this.cylinder = new DecimalType(); 823 this.cylinder.setValue(value); 824 } 825 return this; 826 } 827 828 /** 829 * @param value Power adjustment for astigmatism measured in dioptres (0.25 830 * units). 831 */ 832 public VisionPrescriptionLensSpecificationComponent setCylinder(long value) { 833 this.cylinder = new DecimalType(); 834 this.cylinder.setValue(value); 835 return this; 836 } 837 838 /** 839 * @param value Power adjustment for astigmatism measured in dioptres (0.25 840 * units). 841 */ 842 public VisionPrescriptionLensSpecificationComponent setCylinder(double value) { 843 this.cylinder = new DecimalType(); 844 this.cylinder.setValue(value); 845 return this; 846 } 847 848 /** 849 * @return {@link #axis} (Adjustment for astigmatism measured in integer 850 * degrees.). This is the underlying object with id, value and 851 * extensions. The accessor "getAxis" gives direct access to the value 852 */ 853 public IntegerType getAxisElement() { 854 if (this.axis == null) 855 if (Configuration.errorOnAutoCreate()) 856 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.axis"); 857 else if (Configuration.doAutoCreate()) 858 this.axis = new IntegerType(); // bb 859 return this.axis; 860 } 861 862 public boolean hasAxisElement() { 863 return this.axis != null && !this.axis.isEmpty(); 864 } 865 866 public boolean hasAxis() { 867 return this.axis != null && !this.axis.isEmpty(); 868 } 869 870 /** 871 * @param value {@link #axis} (Adjustment for astigmatism measured in integer 872 * degrees.). This is the underlying object with id, value and 873 * extensions. The accessor "getAxis" gives direct access to the 874 * value 875 */ 876 public VisionPrescriptionLensSpecificationComponent setAxisElement(IntegerType value) { 877 this.axis = value; 878 return this; 879 } 880 881 /** 882 * @return Adjustment for astigmatism measured in integer degrees. 883 */ 884 public int getAxis() { 885 return this.axis == null || this.axis.isEmpty() ? 0 : this.axis.getValue(); 886 } 887 888 /** 889 * @param value Adjustment for astigmatism measured in integer degrees. 890 */ 891 public VisionPrescriptionLensSpecificationComponent setAxis(int value) { 892 if (this.axis == null) 893 this.axis = new IntegerType(); 894 this.axis.setValue(value); 895 return this; 896 } 897 898 /** 899 * @return {@link #prism} (Allows for adjustment on two axis.) 900 */ 901 public List<PrismComponent> getPrism() { 902 if (this.prism == null) 903 this.prism = new ArrayList<PrismComponent>(); 904 return this.prism; 905 } 906 907 /** 908 * @return Returns a reference to <code>this</code> for easy method chaining 909 */ 910 public VisionPrescriptionLensSpecificationComponent setPrism(List<PrismComponent> thePrism) { 911 this.prism = thePrism; 912 return this; 913 } 914 915 public boolean hasPrism() { 916 if (this.prism == null) 917 return false; 918 for (PrismComponent item : this.prism) 919 if (!item.isEmpty()) 920 return true; 921 return false; 922 } 923 924 public PrismComponent addPrism() { // 3 925 PrismComponent t = new PrismComponent(); 926 if (this.prism == null) 927 this.prism = new ArrayList<PrismComponent>(); 928 this.prism.add(t); 929 return t; 930 } 931 932 public VisionPrescriptionLensSpecificationComponent addPrism(PrismComponent t) { // 3 933 if (t == null) 934 return this; 935 if (this.prism == null) 936 this.prism = new ArrayList<PrismComponent>(); 937 this.prism.add(t); 938 return this; 939 } 940 941 /** 942 * @return The first repetition of repeating field {@link #prism}, creating it 943 * if it does not already exist 944 */ 945 public PrismComponent getPrismFirstRep() { 946 if (getPrism().isEmpty()) { 947 addPrism(); 948 } 949 return getPrism().get(0); 950 } 951 952 /** 953 * @return {@link #add} (Power adjustment for multifocal lenses measured in 954 * dioptres (0.25 units).). This is the underlying object with id, value 955 * and extensions. The accessor "getAdd" gives direct access to the 956 * value 957 */ 958 public DecimalType getAddElement() { 959 if (this.add == null) 960 if (Configuration.errorOnAutoCreate()) 961 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.add"); 962 else if (Configuration.doAutoCreate()) 963 this.add = new DecimalType(); // bb 964 return this.add; 965 } 966 967 public boolean hasAddElement() { 968 return this.add != null && !this.add.isEmpty(); 969 } 970 971 public boolean hasAdd() { 972 return this.add != null && !this.add.isEmpty(); 973 } 974 975 /** 976 * @param value {@link #add} (Power adjustment for multifocal lenses measured in 977 * dioptres (0.25 units).). This is the underlying object with id, 978 * value and extensions. The accessor "getAdd" gives direct access 979 * to the value 980 */ 981 public VisionPrescriptionLensSpecificationComponent setAddElement(DecimalType value) { 982 this.add = value; 983 return this; 984 } 985 986 /** 987 * @return Power adjustment for multifocal lenses measured in dioptres (0.25 988 * units). 989 */ 990 public BigDecimal getAdd() { 991 return this.add == null ? null : this.add.getValue(); 992 } 993 994 /** 995 * @param value Power adjustment for multifocal lenses measured in dioptres 996 * (0.25 units). 997 */ 998 public VisionPrescriptionLensSpecificationComponent setAdd(BigDecimal value) { 999 if (value == null) 1000 this.add = null; 1001 else { 1002 if (this.add == null) 1003 this.add = new DecimalType(); 1004 this.add.setValue(value); 1005 } 1006 return this; 1007 } 1008 1009 /** 1010 * @param value Power adjustment for multifocal lenses measured in dioptres 1011 * (0.25 units). 1012 */ 1013 public VisionPrescriptionLensSpecificationComponent setAdd(long value) { 1014 this.add = new DecimalType(); 1015 this.add.setValue(value); 1016 return this; 1017 } 1018 1019 /** 1020 * @param value Power adjustment for multifocal lenses measured in dioptres 1021 * (0.25 units). 1022 */ 1023 public VisionPrescriptionLensSpecificationComponent setAdd(double value) { 1024 this.add = new DecimalType(); 1025 this.add.setValue(value); 1026 return this; 1027 } 1028 1029 /** 1030 * @return {@link #power} (Contact lens power measured in dioptres (0.25 1031 * units).). This is the underlying object with id, value and 1032 * extensions. The accessor "getPower" gives direct access to the value 1033 */ 1034 public DecimalType getPowerElement() { 1035 if (this.power == null) 1036 if (Configuration.errorOnAutoCreate()) 1037 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.power"); 1038 else if (Configuration.doAutoCreate()) 1039 this.power = new DecimalType(); // bb 1040 return this.power; 1041 } 1042 1043 public boolean hasPowerElement() { 1044 return this.power != null && !this.power.isEmpty(); 1045 } 1046 1047 public boolean hasPower() { 1048 return this.power != null && !this.power.isEmpty(); 1049 } 1050 1051 /** 1052 * @param value {@link #power} (Contact lens power measured in dioptres (0.25 1053 * units).). This is the underlying object with id, value and 1054 * extensions. The accessor "getPower" gives direct access to the 1055 * value 1056 */ 1057 public VisionPrescriptionLensSpecificationComponent setPowerElement(DecimalType value) { 1058 this.power = value; 1059 return this; 1060 } 1061 1062 /** 1063 * @return Contact lens power measured in dioptres (0.25 units). 1064 */ 1065 public BigDecimal getPower() { 1066 return this.power == null ? null : this.power.getValue(); 1067 } 1068 1069 /** 1070 * @param value Contact lens power measured in dioptres (0.25 units). 1071 */ 1072 public VisionPrescriptionLensSpecificationComponent setPower(BigDecimal value) { 1073 if (value == null) 1074 this.power = null; 1075 else { 1076 if (this.power == null) 1077 this.power = new DecimalType(); 1078 this.power.setValue(value); 1079 } 1080 return this; 1081 } 1082 1083 /** 1084 * @param value Contact lens power measured in dioptres (0.25 units). 1085 */ 1086 public VisionPrescriptionLensSpecificationComponent setPower(long value) { 1087 this.power = new DecimalType(); 1088 this.power.setValue(value); 1089 return this; 1090 } 1091 1092 /** 1093 * @param value Contact lens power measured in dioptres (0.25 units). 1094 */ 1095 public VisionPrescriptionLensSpecificationComponent setPower(double value) { 1096 this.power = new DecimalType(); 1097 this.power.setValue(value); 1098 return this; 1099 } 1100 1101 /** 1102 * @return {@link #backCurve} (Back curvature measured in millimetres.). This is 1103 * the underlying object with id, value and extensions. The accessor 1104 * "getBackCurve" gives direct access to the value 1105 */ 1106 public DecimalType getBackCurveElement() { 1107 if (this.backCurve == null) 1108 if (Configuration.errorOnAutoCreate()) 1109 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.backCurve"); 1110 else if (Configuration.doAutoCreate()) 1111 this.backCurve = new DecimalType(); // bb 1112 return this.backCurve; 1113 } 1114 1115 public boolean hasBackCurveElement() { 1116 return this.backCurve != null && !this.backCurve.isEmpty(); 1117 } 1118 1119 public boolean hasBackCurve() { 1120 return this.backCurve != null && !this.backCurve.isEmpty(); 1121 } 1122 1123 /** 1124 * @param value {@link #backCurve} (Back curvature measured in millimetres.). 1125 * This is the underlying object with id, value and extensions. The 1126 * accessor "getBackCurve" gives direct access to the value 1127 */ 1128 public VisionPrescriptionLensSpecificationComponent setBackCurveElement(DecimalType value) { 1129 this.backCurve = value; 1130 return this; 1131 } 1132 1133 /** 1134 * @return Back curvature measured in millimetres. 1135 */ 1136 public BigDecimal getBackCurve() { 1137 return this.backCurve == null ? null : this.backCurve.getValue(); 1138 } 1139 1140 /** 1141 * @param value Back curvature measured in millimetres. 1142 */ 1143 public VisionPrescriptionLensSpecificationComponent setBackCurve(BigDecimal value) { 1144 if (value == null) 1145 this.backCurve = null; 1146 else { 1147 if (this.backCurve == null) 1148 this.backCurve = new DecimalType(); 1149 this.backCurve.setValue(value); 1150 } 1151 return this; 1152 } 1153 1154 /** 1155 * @param value Back curvature measured in millimetres. 1156 */ 1157 public VisionPrescriptionLensSpecificationComponent setBackCurve(long value) { 1158 this.backCurve = new DecimalType(); 1159 this.backCurve.setValue(value); 1160 return this; 1161 } 1162 1163 /** 1164 * @param value Back curvature measured in millimetres. 1165 */ 1166 public VisionPrescriptionLensSpecificationComponent setBackCurve(double value) { 1167 this.backCurve = new DecimalType(); 1168 this.backCurve.setValue(value); 1169 return this; 1170 } 1171 1172 /** 1173 * @return {@link #diameter} (Contact lens diameter measured in millimetres.). 1174 * This is the underlying object with id, value and extensions. The 1175 * accessor "getDiameter" gives direct access to the value 1176 */ 1177 public DecimalType getDiameterElement() { 1178 if (this.diameter == null) 1179 if (Configuration.errorOnAutoCreate()) 1180 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.diameter"); 1181 else if (Configuration.doAutoCreate()) 1182 this.diameter = new DecimalType(); // bb 1183 return this.diameter; 1184 } 1185 1186 public boolean hasDiameterElement() { 1187 return this.diameter != null && !this.diameter.isEmpty(); 1188 } 1189 1190 public boolean hasDiameter() { 1191 return this.diameter != null && !this.diameter.isEmpty(); 1192 } 1193 1194 /** 1195 * @param value {@link #diameter} (Contact lens diameter measured in 1196 * millimetres.). This is the underlying object with id, value and 1197 * extensions. The accessor "getDiameter" gives direct access to 1198 * the value 1199 */ 1200 public VisionPrescriptionLensSpecificationComponent setDiameterElement(DecimalType value) { 1201 this.diameter = value; 1202 return this; 1203 } 1204 1205 /** 1206 * @return Contact lens diameter measured in millimetres. 1207 */ 1208 public BigDecimal getDiameter() { 1209 return this.diameter == null ? null : this.diameter.getValue(); 1210 } 1211 1212 /** 1213 * @param value Contact lens diameter measured in millimetres. 1214 */ 1215 public VisionPrescriptionLensSpecificationComponent setDiameter(BigDecimal value) { 1216 if (value == null) 1217 this.diameter = null; 1218 else { 1219 if (this.diameter == null) 1220 this.diameter = new DecimalType(); 1221 this.diameter.setValue(value); 1222 } 1223 return this; 1224 } 1225 1226 /** 1227 * @param value Contact lens diameter measured in millimetres. 1228 */ 1229 public VisionPrescriptionLensSpecificationComponent setDiameter(long value) { 1230 this.diameter = new DecimalType(); 1231 this.diameter.setValue(value); 1232 return this; 1233 } 1234 1235 /** 1236 * @param value Contact lens diameter measured in millimetres. 1237 */ 1238 public VisionPrescriptionLensSpecificationComponent setDiameter(double value) { 1239 this.diameter = new DecimalType(); 1240 this.diameter.setValue(value); 1241 return this; 1242 } 1243 1244 /** 1245 * @return {@link #duration} (The recommended maximum wear period for the lens.) 1246 */ 1247 public Quantity getDuration() { 1248 if (this.duration == null) 1249 if (Configuration.errorOnAutoCreate()) 1250 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.duration"); 1251 else if (Configuration.doAutoCreate()) 1252 this.duration = new Quantity(); // cc 1253 return this.duration; 1254 } 1255 1256 public boolean hasDuration() { 1257 return this.duration != null && !this.duration.isEmpty(); 1258 } 1259 1260 /** 1261 * @param value {@link #duration} (The recommended maximum wear period for the 1262 * lens.) 1263 */ 1264 public VisionPrescriptionLensSpecificationComponent setDuration(Quantity value) { 1265 this.duration = value; 1266 return this; 1267 } 1268 1269 /** 1270 * @return {@link #color} (Special color or pattern.). This is the underlying 1271 * object with id, value and extensions. The accessor "getColor" gives 1272 * direct access to the value 1273 */ 1274 public StringType getColorElement() { 1275 if (this.color == null) 1276 if (Configuration.errorOnAutoCreate()) 1277 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.color"); 1278 else if (Configuration.doAutoCreate()) 1279 this.color = new StringType(); // bb 1280 return this.color; 1281 } 1282 1283 public boolean hasColorElement() { 1284 return this.color != null && !this.color.isEmpty(); 1285 } 1286 1287 public boolean hasColor() { 1288 return this.color != null && !this.color.isEmpty(); 1289 } 1290 1291 /** 1292 * @param value {@link #color} (Special color or pattern.). This is the 1293 * underlying object with id, value and extensions. The accessor 1294 * "getColor" gives direct access to the value 1295 */ 1296 public VisionPrescriptionLensSpecificationComponent setColorElement(StringType value) { 1297 this.color = value; 1298 return this; 1299 } 1300 1301 /** 1302 * @return Special color or pattern. 1303 */ 1304 public String getColor() { 1305 return this.color == null ? null : this.color.getValue(); 1306 } 1307 1308 /** 1309 * @param value Special color or pattern. 1310 */ 1311 public VisionPrescriptionLensSpecificationComponent setColor(String value) { 1312 if (Utilities.noString(value)) 1313 this.color = null; 1314 else { 1315 if (this.color == null) 1316 this.color = new StringType(); 1317 this.color.setValue(value); 1318 } 1319 return this; 1320 } 1321 1322 /** 1323 * @return {@link #brand} (Brand recommendations or restrictions.). This is the 1324 * underlying object with id, value and extensions. The accessor 1325 * "getBrand" gives direct access to the value 1326 */ 1327 public StringType getBrandElement() { 1328 if (this.brand == null) 1329 if (Configuration.errorOnAutoCreate()) 1330 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.brand"); 1331 else if (Configuration.doAutoCreate()) 1332 this.brand = new StringType(); // bb 1333 return this.brand; 1334 } 1335 1336 public boolean hasBrandElement() { 1337 return this.brand != null && !this.brand.isEmpty(); 1338 } 1339 1340 public boolean hasBrand() { 1341 return this.brand != null && !this.brand.isEmpty(); 1342 } 1343 1344 /** 1345 * @param value {@link #brand} (Brand recommendations or restrictions.). This is 1346 * the underlying object with id, value and extensions. The 1347 * accessor "getBrand" gives direct access to the value 1348 */ 1349 public VisionPrescriptionLensSpecificationComponent setBrandElement(StringType value) { 1350 this.brand = value; 1351 return this; 1352 } 1353 1354 /** 1355 * @return Brand recommendations or restrictions. 1356 */ 1357 public String getBrand() { 1358 return this.brand == null ? null : this.brand.getValue(); 1359 } 1360 1361 /** 1362 * @param value Brand recommendations or restrictions. 1363 */ 1364 public VisionPrescriptionLensSpecificationComponent setBrand(String value) { 1365 if (Utilities.noString(value)) 1366 this.brand = null; 1367 else { 1368 if (this.brand == null) 1369 this.brand = new StringType(); 1370 this.brand.setValue(value); 1371 } 1372 return this; 1373 } 1374 1375 /** 1376 * @return {@link #note} (Notes for special requirements such as coatings and 1377 * lens materials.) 1378 */ 1379 public List<Annotation> getNote() { 1380 if (this.note == null) 1381 this.note = new ArrayList<Annotation>(); 1382 return this.note; 1383 } 1384 1385 /** 1386 * @return Returns a reference to <code>this</code> for easy method chaining 1387 */ 1388 public VisionPrescriptionLensSpecificationComponent setNote(List<Annotation> theNote) { 1389 this.note = theNote; 1390 return this; 1391 } 1392 1393 public boolean hasNote() { 1394 if (this.note == null) 1395 return false; 1396 for (Annotation item : this.note) 1397 if (!item.isEmpty()) 1398 return true; 1399 return false; 1400 } 1401 1402 public Annotation addNote() { // 3 1403 Annotation t = new Annotation(); 1404 if (this.note == null) 1405 this.note = new ArrayList<Annotation>(); 1406 this.note.add(t); 1407 return t; 1408 } 1409 1410 public VisionPrescriptionLensSpecificationComponent addNote(Annotation t) { // 3 1411 if (t == null) 1412 return this; 1413 if (this.note == null) 1414 this.note = new ArrayList<Annotation>(); 1415 this.note.add(t); 1416 return this; 1417 } 1418 1419 /** 1420 * @return The first repetition of repeating field {@link #note}, creating it if 1421 * it does not already exist 1422 */ 1423 public Annotation getNoteFirstRep() { 1424 if (getNote().isEmpty()) { 1425 addNote(); 1426 } 1427 return getNote().get(0); 1428 } 1429 1430 protected void listChildren(List<Property> children) { 1431 super.listChildren(children); 1432 children.add(new Property("product", "CodeableConcept", 1433 "Identifies the type of vision correction product which is required for the patient.", 0, 1, product)); 1434 children.add(new Property("eye", "code", "The eye for which the lens specification applies.", 0, 1, eye)); 1435 children.add(new Property("sphere", "decimal", "Lens power measured in dioptres (0.25 units).", 0, 1, sphere)); 1436 children.add(new Property("cylinder", "decimal", 1437 "Power adjustment for astigmatism measured in dioptres (0.25 units).", 0, 1, cylinder)); 1438 children 1439 .add(new Property("axis", "integer", "Adjustment for astigmatism measured in integer degrees.", 0, 1, axis)); 1440 children 1441 .add(new Property("prism", "", "Allows for adjustment on two axis.", 0, java.lang.Integer.MAX_VALUE, prism)); 1442 children.add(new Property("add", "decimal", 1443 "Power adjustment for multifocal lenses measured in dioptres (0.25 units).", 0, 1, add)); 1444 children 1445 .add(new Property("power", "decimal", "Contact lens power measured in dioptres (0.25 units).", 0, 1, power)); 1446 children.add(new Property("backCurve", "decimal", "Back curvature measured in millimetres.", 0, 1, backCurve)); 1447 children 1448 .add(new Property("diameter", "decimal", "Contact lens diameter measured in millimetres.", 0, 1, diameter)); 1449 children.add(new Property("duration", "SimpleQuantity", "The recommended maximum wear period for the lens.", 0, 1, 1450 duration)); 1451 children.add(new Property("color", "string", "Special color or pattern.", 0, 1, color)); 1452 children.add(new Property("brand", "string", "Brand recommendations or restrictions.", 0, 1, brand)); 1453 children.add(new Property("note", "Annotation", 1454 "Notes for special requirements such as coatings and lens materials.", 0, java.lang.Integer.MAX_VALUE, note)); 1455 } 1456 1457 @Override 1458 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1459 switch (_hash) { 1460 case -309474065: 1461 /* product */ return new Property("product", "CodeableConcept", 1462 "Identifies the type of vision correction product which is required for the patient.", 0, 1, product); 1463 case 100913: 1464 /* eye */ return new Property("eye", "code", "The eye for which the lens specification applies.", 0, 1, eye); 1465 case -895981619: 1466 /* sphere */ return new Property("sphere", "decimal", "Lens power measured in dioptres (0.25 units).", 0, 1, 1467 sphere); 1468 case -349378602: 1469 /* cylinder */ return new Property("cylinder", "decimal", 1470 "Power adjustment for astigmatism measured in dioptres (0.25 units).", 0, 1, cylinder); 1471 case 3008417: 1472 /* axis */ return new Property("axis", "integer", "Adjustment for astigmatism measured in integer degrees.", 0, 1473 1, axis); 1474 case 106935105: 1475 /* prism */ return new Property("prism", "", "Allows for adjustment on two axis.", 0, 1476 java.lang.Integer.MAX_VALUE, prism); 1477 case 96417: 1478 /* add */ return new Property("add", "decimal", 1479 "Power adjustment for multifocal lenses measured in dioptres (0.25 units).", 0, 1, add); 1480 case 106858757: 1481 /* power */ return new Property("power", "decimal", "Contact lens power measured in dioptres (0.25 units).", 0, 1482 1, power); 1483 case 1309344840: 1484 /* backCurve */ return new Property("backCurve", "decimal", "Back curvature measured in millimetres.", 0, 1, 1485 backCurve); 1486 case -233204595: 1487 /* diameter */ return new Property("diameter", "decimal", "Contact lens diameter measured in millimetres.", 0, 1488 1, diameter); 1489 case -1992012396: 1490 /* duration */ return new Property("duration", "SimpleQuantity", 1491 "The recommended maximum wear period for the lens.", 0, 1, duration); 1492 case 94842723: 1493 /* color */ return new Property("color", "string", "Special color or pattern.", 0, 1, color); 1494 case 93997959: 1495 /* brand */ return new Property("brand", "string", "Brand recommendations or restrictions.", 0, 1, brand); 1496 case 3387378: 1497 /* note */ return new Property("note", "Annotation", 1498 "Notes for special requirements such as coatings and lens materials.", 0, java.lang.Integer.MAX_VALUE, 1499 note); 1500 default: 1501 return super.getNamedProperty(_hash, _name, _checkValid); 1502 } 1503 1504 } 1505 1506 @Override 1507 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1508 switch (hash) { 1509 case -309474065: 1510 /* product */ return this.product == null ? new Base[0] : new Base[] { this.product }; // CodeableConcept 1511 case 100913: 1512 /* eye */ return this.eye == null ? new Base[0] : new Base[] { this.eye }; // Enumeration<VisionEyes> 1513 case -895981619: 1514 /* sphere */ return this.sphere == null ? new Base[0] : new Base[] { this.sphere }; // DecimalType 1515 case -349378602: 1516 /* cylinder */ return this.cylinder == null ? new Base[0] : new Base[] { this.cylinder }; // DecimalType 1517 case 3008417: 1518 /* axis */ return this.axis == null ? new Base[0] : new Base[] { this.axis }; // IntegerType 1519 case 106935105: 1520 /* prism */ return this.prism == null ? new Base[0] : this.prism.toArray(new Base[this.prism.size()]); // PrismComponent 1521 case 96417: 1522 /* add */ return this.add == null ? new Base[0] : new Base[] { this.add }; // DecimalType 1523 case 106858757: 1524 /* power */ return this.power == null ? new Base[0] : new Base[] { this.power }; // DecimalType 1525 case 1309344840: 1526 /* backCurve */ return this.backCurve == null ? new Base[0] : new Base[] { this.backCurve }; // DecimalType 1527 case -233204595: 1528 /* diameter */ return this.diameter == null ? new Base[0] : new Base[] { this.diameter }; // DecimalType 1529 case -1992012396: 1530 /* duration */ return this.duration == null ? new Base[0] : new Base[] { this.duration }; // Quantity 1531 case 94842723: 1532 /* color */ return this.color == null ? new Base[0] : new Base[] { this.color }; // StringType 1533 case 93997959: 1534 /* brand */ return this.brand == null ? new Base[0] : new Base[] { this.brand }; // StringType 1535 case 3387378: 1536 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1537 default: 1538 return super.getProperty(hash, name, checkValid); 1539 } 1540 1541 } 1542 1543 @Override 1544 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1545 switch (hash) { 1546 case -309474065: // product 1547 this.product = castToCodeableConcept(value); // CodeableConcept 1548 return value; 1549 case 100913: // eye 1550 value = new VisionEyesEnumFactory().fromType(castToCode(value)); 1551 this.eye = (Enumeration) value; // Enumeration<VisionEyes> 1552 return value; 1553 case -895981619: // sphere 1554 this.sphere = castToDecimal(value); // DecimalType 1555 return value; 1556 case -349378602: // cylinder 1557 this.cylinder = castToDecimal(value); // DecimalType 1558 return value; 1559 case 3008417: // axis 1560 this.axis = castToInteger(value); // IntegerType 1561 return value; 1562 case 106935105: // prism 1563 this.getPrism().add((PrismComponent) value); // PrismComponent 1564 return value; 1565 case 96417: // add 1566 this.add = castToDecimal(value); // DecimalType 1567 return value; 1568 case 106858757: // power 1569 this.power = castToDecimal(value); // DecimalType 1570 return value; 1571 case 1309344840: // backCurve 1572 this.backCurve = castToDecimal(value); // DecimalType 1573 return value; 1574 case -233204595: // diameter 1575 this.diameter = castToDecimal(value); // DecimalType 1576 return value; 1577 case -1992012396: // duration 1578 this.duration = castToQuantity(value); // Quantity 1579 return value; 1580 case 94842723: // color 1581 this.color = castToString(value); // StringType 1582 return value; 1583 case 93997959: // brand 1584 this.brand = castToString(value); // StringType 1585 return value; 1586 case 3387378: // note 1587 this.getNote().add(castToAnnotation(value)); // Annotation 1588 return value; 1589 default: 1590 return super.setProperty(hash, name, value); 1591 } 1592 1593 } 1594 1595 @Override 1596 public Base setProperty(String name, Base value) throws FHIRException { 1597 if (name.equals("product")) { 1598 this.product = castToCodeableConcept(value); // CodeableConcept 1599 } else if (name.equals("eye")) { 1600 value = new VisionEyesEnumFactory().fromType(castToCode(value)); 1601 this.eye = (Enumeration) value; // Enumeration<VisionEyes> 1602 } else if (name.equals("sphere")) { 1603 this.sphere = castToDecimal(value); // DecimalType 1604 } else if (name.equals("cylinder")) { 1605 this.cylinder = castToDecimal(value); // DecimalType 1606 } else if (name.equals("axis")) { 1607 this.axis = castToInteger(value); // IntegerType 1608 } else if (name.equals("prism")) { 1609 this.getPrism().add((PrismComponent) value); 1610 } else if (name.equals("add")) { 1611 this.add = castToDecimal(value); // DecimalType 1612 } else if (name.equals("power")) { 1613 this.power = castToDecimal(value); // DecimalType 1614 } else if (name.equals("backCurve")) { 1615 this.backCurve = castToDecimal(value); // DecimalType 1616 } else if (name.equals("diameter")) { 1617 this.diameter = castToDecimal(value); // DecimalType 1618 } else if (name.equals("duration")) { 1619 this.duration = castToQuantity(value); // Quantity 1620 } else if (name.equals("color")) { 1621 this.color = castToString(value); // StringType 1622 } else if (name.equals("brand")) { 1623 this.brand = castToString(value); // StringType 1624 } else if (name.equals("note")) { 1625 this.getNote().add(castToAnnotation(value)); 1626 } else 1627 return super.setProperty(name, value); 1628 return value; 1629 } 1630 1631 @Override 1632 public Base makeProperty(int hash, String name) throws FHIRException { 1633 switch (hash) { 1634 case -309474065: 1635 return getProduct(); 1636 case 100913: 1637 return getEyeElement(); 1638 case -895981619: 1639 return getSphereElement(); 1640 case -349378602: 1641 return getCylinderElement(); 1642 case 3008417: 1643 return getAxisElement(); 1644 case 106935105: 1645 return addPrism(); 1646 case 96417: 1647 return getAddElement(); 1648 case 106858757: 1649 return getPowerElement(); 1650 case 1309344840: 1651 return getBackCurveElement(); 1652 case -233204595: 1653 return getDiameterElement(); 1654 case -1992012396: 1655 return getDuration(); 1656 case 94842723: 1657 return getColorElement(); 1658 case 93997959: 1659 return getBrandElement(); 1660 case 3387378: 1661 return addNote(); 1662 default: 1663 return super.makeProperty(hash, name); 1664 } 1665 1666 } 1667 1668 @Override 1669 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1670 switch (hash) { 1671 case -309474065: 1672 /* product */ return new String[] { "CodeableConcept" }; 1673 case 100913: 1674 /* eye */ return new String[] { "code" }; 1675 case -895981619: 1676 /* sphere */ return new String[] { "decimal" }; 1677 case -349378602: 1678 /* cylinder */ return new String[] { "decimal" }; 1679 case 3008417: 1680 /* axis */ return new String[] { "integer" }; 1681 case 106935105: 1682 /* prism */ return new String[] {}; 1683 case 96417: 1684 /* add */ return new String[] { "decimal" }; 1685 case 106858757: 1686 /* power */ return new String[] { "decimal" }; 1687 case 1309344840: 1688 /* backCurve */ return new String[] { "decimal" }; 1689 case -233204595: 1690 /* diameter */ return new String[] { "decimal" }; 1691 case -1992012396: 1692 /* duration */ return new String[] { "SimpleQuantity" }; 1693 case 94842723: 1694 /* color */ return new String[] { "string" }; 1695 case 93997959: 1696 /* brand */ return new String[] { "string" }; 1697 case 3387378: 1698 /* note */ return new String[] { "Annotation" }; 1699 default: 1700 return super.getTypesForProperty(hash, name); 1701 } 1702 1703 } 1704 1705 @Override 1706 public Base addChild(String name) throws FHIRException { 1707 if (name.equals("product")) { 1708 this.product = new CodeableConcept(); 1709 return this.product; 1710 } else if (name.equals("eye")) { 1711 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.eye"); 1712 } else if (name.equals("sphere")) { 1713 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.sphere"); 1714 } else if (name.equals("cylinder")) { 1715 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.cylinder"); 1716 } else if (name.equals("axis")) { 1717 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.axis"); 1718 } else if (name.equals("prism")) { 1719 return addPrism(); 1720 } else if (name.equals("add")) { 1721 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.add"); 1722 } else if (name.equals("power")) { 1723 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.power"); 1724 } else if (name.equals("backCurve")) { 1725 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.backCurve"); 1726 } else if (name.equals("diameter")) { 1727 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.diameter"); 1728 } else if (name.equals("duration")) { 1729 this.duration = new Quantity(); 1730 return this.duration; 1731 } else if (name.equals("color")) { 1732 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.color"); 1733 } else if (name.equals("brand")) { 1734 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.brand"); 1735 } else if (name.equals("note")) { 1736 return addNote(); 1737 } else 1738 return super.addChild(name); 1739 } 1740 1741 public VisionPrescriptionLensSpecificationComponent copy() { 1742 VisionPrescriptionLensSpecificationComponent dst = new VisionPrescriptionLensSpecificationComponent(); 1743 copyValues(dst); 1744 return dst; 1745 } 1746 1747 public void copyValues(VisionPrescriptionLensSpecificationComponent dst) { 1748 super.copyValues(dst); 1749 dst.product = product == null ? null : product.copy(); 1750 dst.eye = eye == null ? null : eye.copy(); 1751 dst.sphere = sphere == null ? null : sphere.copy(); 1752 dst.cylinder = cylinder == null ? null : cylinder.copy(); 1753 dst.axis = axis == null ? null : axis.copy(); 1754 if (prism != null) { 1755 dst.prism = new ArrayList<PrismComponent>(); 1756 for (PrismComponent i : prism) 1757 dst.prism.add(i.copy()); 1758 } 1759 ; 1760 dst.add = add == null ? null : add.copy(); 1761 dst.power = power == null ? null : power.copy(); 1762 dst.backCurve = backCurve == null ? null : backCurve.copy(); 1763 dst.diameter = diameter == null ? null : diameter.copy(); 1764 dst.duration = duration == null ? null : duration.copy(); 1765 dst.color = color == null ? null : color.copy(); 1766 dst.brand = brand == null ? null : brand.copy(); 1767 if (note != null) { 1768 dst.note = new ArrayList<Annotation>(); 1769 for (Annotation i : note) 1770 dst.note.add(i.copy()); 1771 } 1772 ; 1773 } 1774 1775 @Override 1776 public boolean equalsDeep(Base other_) { 1777 if (!super.equalsDeep(other_)) 1778 return false; 1779 if (!(other_ instanceof VisionPrescriptionLensSpecificationComponent)) 1780 return false; 1781 VisionPrescriptionLensSpecificationComponent o = (VisionPrescriptionLensSpecificationComponent) other_; 1782 return compareDeep(product, o.product, true) && compareDeep(eye, o.eye, true) 1783 && compareDeep(sphere, o.sphere, true) && compareDeep(cylinder, o.cylinder, true) 1784 && compareDeep(axis, o.axis, true) && compareDeep(prism, o.prism, true) && compareDeep(add, o.add, true) 1785 && compareDeep(power, o.power, true) && compareDeep(backCurve, o.backCurve, true) 1786 && compareDeep(diameter, o.diameter, true) && compareDeep(duration, o.duration, true) 1787 && compareDeep(color, o.color, true) && compareDeep(brand, o.brand, true) && compareDeep(note, o.note, true); 1788 } 1789 1790 @Override 1791 public boolean equalsShallow(Base other_) { 1792 if (!super.equalsShallow(other_)) 1793 return false; 1794 if (!(other_ instanceof VisionPrescriptionLensSpecificationComponent)) 1795 return false; 1796 VisionPrescriptionLensSpecificationComponent o = (VisionPrescriptionLensSpecificationComponent) other_; 1797 return compareValues(eye, o.eye, true) && compareValues(sphere, o.sphere, true) 1798 && compareValues(cylinder, o.cylinder, true) && compareValues(axis, o.axis, true) 1799 && compareValues(add, o.add, true) && compareValues(power, o.power, true) 1800 && compareValues(backCurve, o.backCurve, true) && compareValues(diameter, o.diameter, true) 1801 && compareValues(color, o.color, true) && compareValues(brand, o.brand, true); 1802 } 1803 1804 public boolean isEmpty() { 1805 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(product, eye, sphere, cylinder, axis, prism, add, 1806 power, backCurve, diameter, duration, color, brand, note); 1807 } 1808 1809 public String fhirType() { 1810 return "VisionPrescription.lensSpecification"; 1811 1812 } 1813 1814 } 1815 1816 @Block() 1817 public static class PrismComponent extends BackboneElement implements IBaseBackboneElement { 1818 /** 1819 * Amount of prism to compensate for eye alignment in fractional units. 1820 */ 1821 @Child(name = "amount", type = { 1822 DecimalType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1823 @Description(shortDefinition = "Amount of adjustment", formalDefinition = "Amount of prism to compensate for eye alignment in fractional units.") 1824 protected DecimalType amount; 1825 1826 /** 1827 * The relative base, or reference lens edge, for the prism. 1828 */ 1829 @Child(name = "base", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 1830 @Description(shortDefinition = "up | down | in | out", formalDefinition = "The relative base, or reference lens edge, for the prism.") 1831 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/vision-base-codes") 1832 protected Enumeration<VisionBase> base; 1833 1834 private static final long serialVersionUID = 1677247628L; 1835 1836 /** 1837 * Constructor 1838 */ 1839 public PrismComponent() { 1840 super(); 1841 } 1842 1843 /** 1844 * Constructor 1845 */ 1846 public PrismComponent(DecimalType amount, Enumeration<VisionBase> base) { 1847 super(); 1848 this.amount = amount; 1849 this.base = base; 1850 } 1851 1852 /** 1853 * @return {@link #amount} (Amount of prism to compensate for eye alignment in 1854 * fractional units.). This is the underlying object with id, value and 1855 * extensions. The accessor "getAmount" gives direct access to the value 1856 */ 1857 public DecimalType getAmountElement() { 1858 if (this.amount == null) 1859 if (Configuration.errorOnAutoCreate()) 1860 throw new Error("Attempt to auto-create PrismComponent.amount"); 1861 else if (Configuration.doAutoCreate()) 1862 this.amount = new DecimalType(); // bb 1863 return this.amount; 1864 } 1865 1866 public boolean hasAmountElement() { 1867 return this.amount != null && !this.amount.isEmpty(); 1868 } 1869 1870 public boolean hasAmount() { 1871 return this.amount != null && !this.amount.isEmpty(); 1872 } 1873 1874 /** 1875 * @param value {@link #amount} (Amount of prism to compensate for eye alignment 1876 * in fractional units.). This is the underlying object with id, 1877 * value and extensions. The accessor "getAmount" gives direct 1878 * access to the value 1879 */ 1880 public PrismComponent setAmountElement(DecimalType value) { 1881 this.amount = value; 1882 return this; 1883 } 1884 1885 /** 1886 * @return Amount of prism to compensate for eye alignment in fractional units. 1887 */ 1888 public BigDecimal getAmount() { 1889 return this.amount == null ? null : this.amount.getValue(); 1890 } 1891 1892 /** 1893 * @param value Amount of prism to compensate for eye alignment in fractional 1894 * units. 1895 */ 1896 public PrismComponent setAmount(BigDecimal value) { 1897 if (this.amount == null) 1898 this.amount = new DecimalType(); 1899 this.amount.setValue(value); 1900 return this; 1901 } 1902 1903 /** 1904 * @param value Amount of prism to compensate for eye alignment in fractional 1905 * units. 1906 */ 1907 public PrismComponent setAmount(long value) { 1908 this.amount = new DecimalType(); 1909 this.amount.setValue(value); 1910 return this; 1911 } 1912 1913 /** 1914 * @param value Amount of prism to compensate for eye alignment in fractional 1915 * units. 1916 */ 1917 public PrismComponent setAmount(double value) { 1918 this.amount = new DecimalType(); 1919 this.amount.setValue(value); 1920 return this; 1921 } 1922 1923 /** 1924 * @return {@link #base} (The relative base, or reference lens edge, for the 1925 * prism.). This is the underlying object with id, value and extensions. 1926 * The accessor "getBase" gives direct access to the value 1927 */ 1928 public Enumeration<VisionBase> getBaseElement() { 1929 if (this.base == null) 1930 if (Configuration.errorOnAutoCreate()) 1931 throw new Error("Attempt to auto-create PrismComponent.base"); 1932 else if (Configuration.doAutoCreate()) 1933 this.base = new Enumeration<VisionBase>(new VisionBaseEnumFactory()); // bb 1934 return this.base; 1935 } 1936 1937 public boolean hasBaseElement() { 1938 return this.base != null && !this.base.isEmpty(); 1939 } 1940 1941 public boolean hasBase() { 1942 return this.base != null && !this.base.isEmpty(); 1943 } 1944 1945 /** 1946 * @param value {@link #base} (The relative base, or reference lens edge, for 1947 * the prism.). This is the underlying object with id, value and 1948 * extensions. The accessor "getBase" gives direct access to the 1949 * value 1950 */ 1951 public PrismComponent setBaseElement(Enumeration<VisionBase> value) { 1952 this.base = value; 1953 return this; 1954 } 1955 1956 /** 1957 * @return The relative base, or reference lens edge, for the prism. 1958 */ 1959 public VisionBase getBase() { 1960 return this.base == null ? null : this.base.getValue(); 1961 } 1962 1963 /** 1964 * @param value The relative base, or reference lens edge, for the prism. 1965 */ 1966 public PrismComponent setBase(VisionBase value) { 1967 if (this.base == null) 1968 this.base = new Enumeration<VisionBase>(new VisionBaseEnumFactory()); 1969 this.base.setValue(value); 1970 return this; 1971 } 1972 1973 protected void listChildren(List<Property> children) { 1974 super.listChildren(children); 1975 children.add(new Property("amount", "decimal", 1976 "Amount of prism to compensate for eye alignment in fractional units.", 0, 1, amount)); 1977 children 1978 .add(new Property("base", "code", "The relative base, or reference lens edge, for the prism.", 0, 1, base)); 1979 } 1980 1981 @Override 1982 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1983 switch (_hash) { 1984 case -1413853096: 1985 /* amount */ return new Property("amount", "decimal", 1986 "Amount of prism to compensate for eye alignment in fractional units.", 0, 1, amount); 1987 case 3016401: 1988 /* base */ return new Property("base", "code", "The relative base, or reference lens edge, for the prism.", 0, 1989 1, base); 1990 default: 1991 return super.getNamedProperty(_hash, _name, _checkValid); 1992 } 1993 1994 } 1995 1996 @Override 1997 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1998 switch (hash) { 1999 case -1413853096: 2000 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // DecimalType 2001 case 3016401: 2002 /* base */ return this.base == null ? new Base[0] : new Base[] { this.base }; // Enumeration<VisionBase> 2003 default: 2004 return super.getProperty(hash, name, checkValid); 2005 } 2006 2007 } 2008 2009 @Override 2010 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2011 switch (hash) { 2012 case -1413853096: // amount 2013 this.amount = castToDecimal(value); // DecimalType 2014 return value; 2015 case 3016401: // base 2016 value = new VisionBaseEnumFactory().fromType(castToCode(value)); 2017 this.base = (Enumeration) value; // Enumeration<VisionBase> 2018 return value; 2019 default: 2020 return super.setProperty(hash, name, value); 2021 } 2022 2023 } 2024 2025 @Override 2026 public Base setProperty(String name, Base value) throws FHIRException { 2027 if (name.equals("amount")) { 2028 this.amount = castToDecimal(value); // DecimalType 2029 } else if (name.equals("base")) { 2030 value = new VisionBaseEnumFactory().fromType(castToCode(value)); 2031 this.base = (Enumeration) value; // Enumeration<VisionBase> 2032 } else 2033 return super.setProperty(name, value); 2034 return value; 2035 } 2036 2037 @Override 2038 public Base makeProperty(int hash, String name) throws FHIRException { 2039 switch (hash) { 2040 case -1413853096: 2041 return getAmountElement(); 2042 case 3016401: 2043 return getBaseElement(); 2044 default: 2045 return super.makeProperty(hash, name); 2046 } 2047 2048 } 2049 2050 @Override 2051 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2052 switch (hash) { 2053 case -1413853096: 2054 /* amount */ return new String[] { "decimal" }; 2055 case 3016401: 2056 /* base */ return new String[] { "code" }; 2057 default: 2058 return super.getTypesForProperty(hash, name); 2059 } 2060 2061 } 2062 2063 @Override 2064 public Base addChild(String name) throws FHIRException { 2065 if (name.equals("amount")) { 2066 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.amount"); 2067 } else if (name.equals("base")) { 2068 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.base"); 2069 } else 2070 return super.addChild(name); 2071 } 2072 2073 public PrismComponent copy() { 2074 PrismComponent dst = new PrismComponent(); 2075 copyValues(dst); 2076 return dst; 2077 } 2078 2079 public void copyValues(PrismComponent dst) { 2080 super.copyValues(dst); 2081 dst.amount = amount == null ? null : amount.copy(); 2082 dst.base = base == null ? null : base.copy(); 2083 } 2084 2085 @Override 2086 public boolean equalsDeep(Base other_) { 2087 if (!super.equalsDeep(other_)) 2088 return false; 2089 if (!(other_ instanceof PrismComponent)) 2090 return false; 2091 PrismComponent o = (PrismComponent) other_; 2092 return compareDeep(amount, o.amount, true) && compareDeep(base, o.base, true); 2093 } 2094 2095 @Override 2096 public boolean equalsShallow(Base other_) { 2097 if (!super.equalsShallow(other_)) 2098 return false; 2099 if (!(other_ instanceof PrismComponent)) 2100 return false; 2101 PrismComponent o = (PrismComponent) other_; 2102 return compareValues(amount, o.amount, true) && compareValues(base, o.base, true); 2103 } 2104 2105 public boolean isEmpty() { 2106 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(amount, base); 2107 } 2108 2109 public String fhirType() { 2110 return "VisionPrescription.lensSpecification.prism"; 2111 2112 } 2113 2114 } 2115 2116 /** 2117 * A unique identifier assigned to this vision prescription. 2118 */ 2119 @Child(name = "identifier", type = { 2120 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2121 @Description(shortDefinition = "Business Identifier for vision prescription", formalDefinition = "A unique identifier assigned to this vision prescription.") 2122 protected List<Identifier> identifier; 2123 2124 /** 2125 * The status of the resource instance. 2126 */ 2127 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 2128 @Description(shortDefinition = "active | cancelled | draft | entered-in-error", formalDefinition = "The status of the resource instance.") 2129 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/fm-status") 2130 protected Enumeration<VisionStatus> status; 2131 2132 /** 2133 * The date this resource was created. 2134 */ 2135 @Child(name = "created", type = { DateTimeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 2136 @Description(shortDefinition = "Response creation date", formalDefinition = "The date this resource was created.") 2137 protected DateTimeType created; 2138 2139 /** 2140 * A resource reference to the person to whom the vision prescription applies. 2141 */ 2142 @Child(name = "patient", type = { Patient.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 2143 @Description(shortDefinition = "Who prescription is for", formalDefinition = "A resource reference to the person to whom the vision prescription applies.") 2144 protected Reference patient; 2145 2146 /** 2147 * The actual object that is the target of the reference (A resource reference 2148 * to the person to whom the vision prescription applies.) 2149 */ 2150 protected Patient patientTarget; 2151 2152 /** 2153 * A reference to a resource that identifies the particular occurrence of 2154 * contact between patient and health care provider during which the 2155 * prescription was issued. 2156 */ 2157 @Child(name = "encounter", type = { Encounter.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2158 @Description(shortDefinition = "Created during encounter / admission / stay", formalDefinition = "A reference to a resource that identifies the particular occurrence of contact between patient and health care provider during which the prescription was issued.") 2159 protected Reference encounter; 2160 2161 /** 2162 * The actual object that is the target of the reference (A reference to a 2163 * resource that identifies the particular occurrence of contact between patient 2164 * and health care provider during which the prescription was issued.) 2165 */ 2166 protected Encounter encounterTarget; 2167 2168 /** 2169 * The date (and perhaps time) when the prescription was written. 2170 */ 2171 @Child(name = "dateWritten", type = { 2172 DateTimeType.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 2173 @Description(shortDefinition = "When prescription was authorized", formalDefinition = "The date (and perhaps time) when the prescription was written.") 2174 protected DateTimeType dateWritten; 2175 2176 /** 2177 * The healthcare professional responsible for authorizing the prescription. 2178 */ 2179 @Child(name = "prescriber", type = { Practitioner.class, 2180 PractitionerRole.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 2181 @Description(shortDefinition = "Who authorized the vision prescription", formalDefinition = "The healthcare professional responsible for authorizing the prescription.") 2182 protected Reference prescriber; 2183 2184 /** 2185 * The actual object that is the target of the reference (The healthcare 2186 * professional responsible for authorizing the prescription.) 2187 */ 2188 protected Resource prescriberTarget; 2189 2190 /** 2191 * Contain the details of the individual lens specifications and serves as the 2192 * authorization for the fullfillment by certified professionals. 2193 */ 2194 @Child(name = "lensSpecification", type = {}, order = 7, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2195 @Description(shortDefinition = "Vision lens authorization", formalDefinition = "Contain the details of the individual lens specifications and serves as the authorization for the fullfillment by certified professionals.") 2196 protected List<VisionPrescriptionLensSpecificationComponent> lensSpecification; 2197 2198 private static final long serialVersionUID = 988021071L; 2199 2200 /** 2201 * Constructor 2202 */ 2203 public VisionPrescription() { 2204 super(); 2205 } 2206 2207 /** 2208 * Constructor 2209 */ 2210 public VisionPrescription(Enumeration<VisionStatus> status, DateTimeType created, Reference patient, 2211 DateTimeType dateWritten, Reference prescriber) { 2212 super(); 2213 this.status = status; 2214 this.created = created; 2215 this.patient = patient; 2216 this.dateWritten = dateWritten; 2217 this.prescriber = prescriber; 2218 } 2219 2220 /** 2221 * @return {@link #identifier} (A unique identifier assigned to this vision 2222 * prescription.) 2223 */ 2224 public List<Identifier> getIdentifier() { 2225 if (this.identifier == null) 2226 this.identifier = new ArrayList<Identifier>(); 2227 return this.identifier; 2228 } 2229 2230 /** 2231 * @return Returns a reference to <code>this</code> for easy method chaining 2232 */ 2233 public VisionPrescription setIdentifier(List<Identifier> theIdentifier) { 2234 this.identifier = theIdentifier; 2235 return this; 2236 } 2237 2238 public boolean hasIdentifier() { 2239 if (this.identifier == null) 2240 return false; 2241 for (Identifier item : this.identifier) 2242 if (!item.isEmpty()) 2243 return true; 2244 return false; 2245 } 2246 2247 public Identifier addIdentifier() { // 3 2248 Identifier t = new Identifier(); 2249 if (this.identifier == null) 2250 this.identifier = new ArrayList<Identifier>(); 2251 this.identifier.add(t); 2252 return t; 2253 } 2254 2255 public VisionPrescription addIdentifier(Identifier t) { // 3 2256 if (t == null) 2257 return this; 2258 if (this.identifier == null) 2259 this.identifier = new ArrayList<Identifier>(); 2260 this.identifier.add(t); 2261 return this; 2262 } 2263 2264 /** 2265 * @return The first repetition of repeating field {@link #identifier}, creating 2266 * it if it does not already exist 2267 */ 2268 public Identifier getIdentifierFirstRep() { 2269 if (getIdentifier().isEmpty()) { 2270 addIdentifier(); 2271 } 2272 return getIdentifier().get(0); 2273 } 2274 2275 /** 2276 * @return {@link #status} (The status of the resource instance.). This is the 2277 * underlying object with id, value and extensions. The accessor 2278 * "getStatus" gives direct access to the value 2279 */ 2280 public Enumeration<VisionStatus> getStatusElement() { 2281 if (this.status == null) 2282 if (Configuration.errorOnAutoCreate()) 2283 throw new Error("Attempt to auto-create VisionPrescription.status"); 2284 else if (Configuration.doAutoCreate()) 2285 this.status = new Enumeration<VisionStatus>(new VisionStatusEnumFactory()); // bb 2286 return this.status; 2287 } 2288 2289 public boolean hasStatusElement() { 2290 return this.status != null && !this.status.isEmpty(); 2291 } 2292 2293 public boolean hasStatus() { 2294 return this.status != null && !this.status.isEmpty(); 2295 } 2296 2297 /** 2298 * @param value {@link #status} (The status of the resource instance.). This is 2299 * the underlying object with id, value and extensions. The 2300 * accessor "getStatus" gives direct access to the value 2301 */ 2302 public VisionPrescription setStatusElement(Enumeration<VisionStatus> value) { 2303 this.status = value; 2304 return this; 2305 } 2306 2307 /** 2308 * @return The status of the resource instance. 2309 */ 2310 public VisionStatus getStatus() { 2311 return this.status == null ? null : this.status.getValue(); 2312 } 2313 2314 /** 2315 * @param value The status of the resource instance. 2316 */ 2317 public VisionPrescription setStatus(VisionStatus value) { 2318 if (this.status == null) 2319 this.status = new Enumeration<VisionStatus>(new VisionStatusEnumFactory()); 2320 this.status.setValue(value); 2321 return this; 2322 } 2323 2324 /** 2325 * @return {@link #created} (The date this resource was created.). This is the 2326 * underlying object with id, value and extensions. The accessor 2327 * "getCreated" gives direct access to the value 2328 */ 2329 public DateTimeType getCreatedElement() { 2330 if (this.created == null) 2331 if (Configuration.errorOnAutoCreate()) 2332 throw new Error("Attempt to auto-create VisionPrescription.created"); 2333 else if (Configuration.doAutoCreate()) 2334 this.created = new DateTimeType(); // bb 2335 return this.created; 2336 } 2337 2338 public boolean hasCreatedElement() { 2339 return this.created != null && !this.created.isEmpty(); 2340 } 2341 2342 public boolean hasCreated() { 2343 return this.created != null && !this.created.isEmpty(); 2344 } 2345 2346 /** 2347 * @param value {@link #created} (The date this resource was created.). This is 2348 * the underlying object with id, value and extensions. The 2349 * accessor "getCreated" gives direct access to the value 2350 */ 2351 public VisionPrescription setCreatedElement(DateTimeType value) { 2352 this.created = value; 2353 return this; 2354 } 2355 2356 /** 2357 * @return The date this resource was created. 2358 */ 2359 public Date getCreated() { 2360 return this.created == null ? null : this.created.getValue(); 2361 } 2362 2363 /** 2364 * @param value The date this resource was created. 2365 */ 2366 public VisionPrescription setCreated(Date value) { 2367 if (this.created == null) 2368 this.created = new DateTimeType(); 2369 this.created.setValue(value); 2370 return this; 2371 } 2372 2373 /** 2374 * @return {@link #patient} (A resource reference to the person to whom the 2375 * vision prescription applies.) 2376 */ 2377 public Reference getPatient() { 2378 if (this.patient == null) 2379 if (Configuration.errorOnAutoCreate()) 2380 throw new Error("Attempt to auto-create VisionPrescription.patient"); 2381 else if (Configuration.doAutoCreate()) 2382 this.patient = new Reference(); // cc 2383 return this.patient; 2384 } 2385 2386 public boolean hasPatient() { 2387 return this.patient != null && !this.patient.isEmpty(); 2388 } 2389 2390 /** 2391 * @param value {@link #patient} (A resource reference to the person to whom the 2392 * vision prescription applies.) 2393 */ 2394 public VisionPrescription setPatient(Reference value) { 2395 this.patient = value; 2396 return this; 2397 } 2398 2399 /** 2400 * @return {@link #patient} The actual object that is the target of the 2401 * reference. The reference library doesn't populate this, but you can 2402 * use it to hold the resource if you resolve it. (A resource reference 2403 * to the person to whom the vision prescription applies.) 2404 */ 2405 public Patient getPatientTarget() { 2406 if (this.patientTarget == null) 2407 if (Configuration.errorOnAutoCreate()) 2408 throw new Error("Attempt to auto-create VisionPrescription.patient"); 2409 else if (Configuration.doAutoCreate()) 2410 this.patientTarget = new Patient(); // aa 2411 return this.patientTarget; 2412 } 2413 2414 /** 2415 * @param value {@link #patient} The actual object that is the target of the 2416 * reference. The reference library doesn't use these, but you can 2417 * use it to hold the resource if you resolve it. (A resource 2418 * reference to the person to whom the vision prescription 2419 * applies.) 2420 */ 2421 public VisionPrescription setPatientTarget(Patient value) { 2422 this.patientTarget = value; 2423 return this; 2424 } 2425 2426 /** 2427 * @return {@link #encounter} (A reference to a resource that identifies the 2428 * particular occurrence of contact between patient and health care 2429 * provider during which the prescription was issued.) 2430 */ 2431 public Reference getEncounter() { 2432 if (this.encounter == null) 2433 if (Configuration.errorOnAutoCreate()) 2434 throw new Error("Attempt to auto-create VisionPrescription.encounter"); 2435 else if (Configuration.doAutoCreate()) 2436 this.encounter = new Reference(); // cc 2437 return this.encounter; 2438 } 2439 2440 public boolean hasEncounter() { 2441 return this.encounter != null && !this.encounter.isEmpty(); 2442 } 2443 2444 /** 2445 * @param value {@link #encounter} (A reference to a resource that identifies 2446 * the particular occurrence of contact between patient and health 2447 * care provider during which the prescription was issued.) 2448 */ 2449 public VisionPrescription setEncounter(Reference value) { 2450 this.encounter = value; 2451 return this; 2452 } 2453 2454 /** 2455 * @return {@link #encounter} The actual object that is the target of the 2456 * reference. The reference library doesn't populate this, but you can 2457 * use it to hold the resource if you resolve it. (A reference to a 2458 * resource that identifies the particular occurrence of contact between 2459 * patient and health care provider during which the prescription was 2460 * issued.) 2461 */ 2462 public Encounter getEncounterTarget() { 2463 if (this.encounterTarget == null) 2464 if (Configuration.errorOnAutoCreate()) 2465 throw new Error("Attempt to auto-create VisionPrescription.encounter"); 2466 else if (Configuration.doAutoCreate()) 2467 this.encounterTarget = new Encounter(); // aa 2468 return this.encounterTarget; 2469 } 2470 2471 /** 2472 * @param value {@link #encounter} The actual object that is the target of the 2473 * reference. The reference library doesn't use these, but you can 2474 * use it to hold the resource if you resolve it. (A reference to a 2475 * resource that identifies the particular occurrence of contact 2476 * between patient and health care provider during which the 2477 * prescription was issued.) 2478 */ 2479 public VisionPrescription setEncounterTarget(Encounter value) { 2480 this.encounterTarget = value; 2481 return this; 2482 } 2483 2484 /** 2485 * @return {@link #dateWritten} (The date (and perhaps time) when the 2486 * prescription was written.). This is the underlying object with id, 2487 * value and extensions. The accessor "getDateWritten" gives direct 2488 * access to the value 2489 */ 2490 public DateTimeType getDateWrittenElement() { 2491 if (this.dateWritten == null) 2492 if (Configuration.errorOnAutoCreate()) 2493 throw new Error("Attempt to auto-create VisionPrescription.dateWritten"); 2494 else if (Configuration.doAutoCreate()) 2495 this.dateWritten = new DateTimeType(); // bb 2496 return this.dateWritten; 2497 } 2498 2499 public boolean hasDateWrittenElement() { 2500 return this.dateWritten != null && !this.dateWritten.isEmpty(); 2501 } 2502 2503 public boolean hasDateWritten() { 2504 return this.dateWritten != null && !this.dateWritten.isEmpty(); 2505 } 2506 2507 /** 2508 * @param value {@link #dateWritten} (The date (and perhaps time) when the 2509 * prescription was written.). This is the underlying object with 2510 * id, value and extensions. The accessor "getDateWritten" gives 2511 * direct access to the value 2512 */ 2513 public VisionPrescription setDateWrittenElement(DateTimeType value) { 2514 this.dateWritten = value; 2515 return this; 2516 } 2517 2518 /** 2519 * @return The date (and perhaps time) when the prescription was written. 2520 */ 2521 public Date getDateWritten() { 2522 return this.dateWritten == null ? null : this.dateWritten.getValue(); 2523 } 2524 2525 /** 2526 * @param value The date (and perhaps time) when the prescription was written. 2527 */ 2528 public VisionPrescription setDateWritten(Date value) { 2529 if (this.dateWritten == null) 2530 this.dateWritten = new DateTimeType(); 2531 this.dateWritten.setValue(value); 2532 return this; 2533 } 2534 2535 /** 2536 * @return {@link #prescriber} (The healthcare professional responsible for 2537 * authorizing the prescription.) 2538 */ 2539 public Reference getPrescriber() { 2540 if (this.prescriber == null) 2541 if (Configuration.errorOnAutoCreate()) 2542 throw new Error("Attempt to auto-create VisionPrescription.prescriber"); 2543 else if (Configuration.doAutoCreate()) 2544 this.prescriber = new Reference(); // cc 2545 return this.prescriber; 2546 } 2547 2548 public boolean hasPrescriber() { 2549 return this.prescriber != null && !this.prescriber.isEmpty(); 2550 } 2551 2552 /** 2553 * @param value {@link #prescriber} (The healthcare professional responsible for 2554 * authorizing the prescription.) 2555 */ 2556 public VisionPrescription setPrescriber(Reference value) { 2557 this.prescriber = value; 2558 return this; 2559 } 2560 2561 /** 2562 * @return {@link #prescriber} The actual object that is the target of the 2563 * reference. The reference library doesn't populate this, but you can 2564 * use it to hold the resource if you resolve it. (The healthcare 2565 * professional responsible for authorizing the prescription.) 2566 */ 2567 public Resource getPrescriberTarget() { 2568 return this.prescriberTarget; 2569 } 2570 2571 /** 2572 * @param value {@link #prescriber} The actual object that is the target of the 2573 * reference. The reference library doesn't use these, but you can 2574 * use it to hold the resource if you resolve it. (The healthcare 2575 * professional responsible for authorizing the prescription.) 2576 */ 2577 public VisionPrescription setPrescriberTarget(Resource value) { 2578 this.prescriberTarget = value; 2579 return this; 2580 } 2581 2582 /** 2583 * @return {@link #lensSpecification} (Contain the details of the individual 2584 * lens specifications and serves as the authorization for the 2585 * fullfillment by certified professionals.) 2586 */ 2587 public List<VisionPrescriptionLensSpecificationComponent> getLensSpecification() { 2588 if (this.lensSpecification == null) 2589 this.lensSpecification = new ArrayList<VisionPrescriptionLensSpecificationComponent>(); 2590 return this.lensSpecification; 2591 } 2592 2593 /** 2594 * @return Returns a reference to <code>this</code> for easy method chaining 2595 */ 2596 public VisionPrescription setLensSpecification( 2597 List<VisionPrescriptionLensSpecificationComponent> theLensSpecification) { 2598 this.lensSpecification = theLensSpecification; 2599 return this; 2600 } 2601 2602 public boolean hasLensSpecification() { 2603 if (this.lensSpecification == null) 2604 return false; 2605 for (VisionPrescriptionLensSpecificationComponent item : this.lensSpecification) 2606 if (!item.isEmpty()) 2607 return true; 2608 return false; 2609 } 2610 2611 public VisionPrescriptionLensSpecificationComponent addLensSpecification() { // 3 2612 VisionPrescriptionLensSpecificationComponent t = new VisionPrescriptionLensSpecificationComponent(); 2613 if (this.lensSpecification == null) 2614 this.lensSpecification = new ArrayList<VisionPrescriptionLensSpecificationComponent>(); 2615 this.lensSpecification.add(t); 2616 return t; 2617 } 2618 2619 public VisionPrescription addLensSpecification(VisionPrescriptionLensSpecificationComponent t) { // 3 2620 if (t == null) 2621 return this; 2622 if (this.lensSpecification == null) 2623 this.lensSpecification = new ArrayList<VisionPrescriptionLensSpecificationComponent>(); 2624 this.lensSpecification.add(t); 2625 return this; 2626 } 2627 2628 /** 2629 * @return The first repetition of repeating field {@link #lensSpecification}, 2630 * creating it if it does not already exist 2631 */ 2632 public VisionPrescriptionLensSpecificationComponent getLensSpecificationFirstRep() { 2633 if (getLensSpecification().isEmpty()) { 2634 addLensSpecification(); 2635 } 2636 return getLensSpecification().get(0); 2637 } 2638 2639 protected void listChildren(List<Property> children) { 2640 super.listChildren(children); 2641 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this vision prescription.", 2642 0, java.lang.Integer.MAX_VALUE, identifier)); 2643 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 2644 children.add(new Property("created", "dateTime", "The date this resource was created.", 0, 1, created)); 2645 children.add(new Property("patient", "Reference(Patient)", 2646 "A resource reference to the person to whom the vision prescription applies.", 0, 1, patient)); 2647 children.add(new Property("encounter", "Reference(Encounter)", 2648 "A reference to a resource that identifies the particular occurrence of contact between patient and health care provider during which the prescription was issued.", 2649 0, 1, encounter)); 2650 children.add(new Property("dateWritten", "dateTime", 2651 "The date (and perhaps time) when the prescription was written.", 0, 1, dateWritten)); 2652 children.add(new Property("prescriber", "Reference(Practitioner|PractitionerRole)", 2653 "The healthcare professional responsible for authorizing the prescription.", 0, 1, prescriber)); 2654 children.add(new Property("lensSpecification", "", 2655 "Contain the details of the individual lens specifications and serves as the authorization for the fullfillment by certified professionals.", 2656 0, java.lang.Integer.MAX_VALUE, lensSpecification)); 2657 } 2658 2659 @Override 2660 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2661 switch (_hash) { 2662 case -1618432855: 2663 /* identifier */ return new Property("identifier", "Identifier", 2664 "A unique identifier assigned to this vision prescription.", 0, java.lang.Integer.MAX_VALUE, identifier); 2665 case -892481550: 2666 /* status */ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 2667 case 1028554472: 2668 /* created */ return new Property("created", "dateTime", "The date this resource was created.", 0, 1, created); 2669 case -791418107: 2670 /* patient */ return new Property("patient", "Reference(Patient)", 2671 "A resource reference to the person to whom the vision prescription applies.", 0, 1, patient); 2672 case 1524132147: 2673 /* encounter */ return new Property("encounter", "Reference(Encounter)", 2674 "A reference to a resource that identifies the particular occurrence of contact between patient and health care provider during which the prescription was issued.", 2675 0, 1, encounter); 2676 case -1496880759: 2677 /* dateWritten */ return new Property("dateWritten", "dateTime", 2678 "The date (and perhaps time) when the prescription was written.", 0, 1, dateWritten); 2679 case 1430631077: 2680 /* prescriber */ return new Property("prescriber", "Reference(Practitioner|PractitionerRole)", 2681 "The healthcare professional responsible for authorizing the prescription.", 0, 1, prescriber); 2682 case -1767318363: 2683 /* lensSpecification */ return new Property("lensSpecification", "", 2684 "Contain the details of the individual lens specifications and serves as the authorization for the fullfillment by certified professionals.", 2685 0, java.lang.Integer.MAX_VALUE, lensSpecification); 2686 default: 2687 return super.getNamedProperty(_hash, _name, _checkValid); 2688 } 2689 2690 } 2691 2692 @Override 2693 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2694 switch (hash) { 2695 case -1618432855: 2696 /* identifier */ return this.identifier == null ? new Base[0] 2697 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2698 case -892481550: 2699 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<VisionStatus> 2700 case 1028554472: 2701 /* created */ return this.created == null ? new Base[0] : new Base[] { this.created }; // DateTimeType 2702 case -791418107: 2703 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 2704 case 1524132147: 2705 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 2706 case -1496880759: 2707 /* dateWritten */ return this.dateWritten == null ? new Base[0] : new Base[] { this.dateWritten }; // DateTimeType 2708 case 1430631077: 2709 /* prescriber */ return this.prescriber == null ? new Base[0] : new Base[] { this.prescriber }; // Reference 2710 case -1767318363: 2711 /* lensSpecification */ return this.lensSpecification == null ? new Base[0] 2712 : this.lensSpecification.toArray(new Base[this.lensSpecification.size()]); // VisionPrescriptionLensSpecificationComponent 2713 default: 2714 return super.getProperty(hash, name, checkValid); 2715 } 2716 2717 } 2718 2719 @Override 2720 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2721 switch (hash) { 2722 case -1618432855: // identifier 2723 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2724 return value; 2725 case -892481550: // status 2726 value = new VisionStatusEnumFactory().fromType(castToCode(value)); 2727 this.status = (Enumeration) value; // Enumeration<VisionStatus> 2728 return value; 2729 case 1028554472: // created 2730 this.created = castToDateTime(value); // DateTimeType 2731 return value; 2732 case -791418107: // patient 2733 this.patient = castToReference(value); // Reference 2734 return value; 2735 case 1524132147: // encounter 2736 this.encounter = castToReference(value); // Reference 2737 return value; 2738 case -1496880759: // dateWritten 2739 this.dateWritten = castToDateTime(value); // DateTimeType 2740 return value; 2741 case 1430631077: // prescriber 2742 this.prescriber = castToReference(value); // Reference 2743 return value; 2744 case -1767318363: // lensSpecification 2745 this.getLensSpecification().add((VisionPrescriptionLensSpecificationComponent) value); // VisionPrescriptionLensSpecificationComponent 2746 return value; 2747 default: 2748 return super.setProperty(hash, name, value); 2749 } 2750 2751 } 2752 2753 @Override 2754 public Base setProperty(String name, Base value) throws FHIRException { 2755 if (name.equals("identifier")) { 2756 this.getIdentifier().add(castToIdentifier(value)); 2757 } else if (name.equals("status")) { 2758 value = new VisionStatusEnumFactory().fromType(castToCode(value)); 2759 this.status = (Enumeration) value; // Enumeration<VisionStatus> 2760 } else if (name.equals("created")) { 2761 this.created = castToDateTime(value); // DateTimeType 2762 } else if (name.equals("patient")) { 2763 this.patient = castToReference(value); // Reference 2764 } else if (name.equals("encounter")) { 2765 this.encounter = castToReference(value); // Reference 2766 } else if (name.equals("dateWritten")) { 2767 this.dateWritten = castToDateTime(value); // DateTimeType 2768 } else if (name.equals("prescriber")) { 2769 this.prescriber = castToReference(value); // Reference 2770 } else if (name.equals("lensSpecification")) { 2771 this.getLensSpecification().add((VisionPrescriptionLensSpecificationComponent) value); 2772 } else 2773 return super.setProperty(name, value); 2774 return value; 2775 } 2776 2777 @Override 2778 public Base makeProperty(int hash, String name) throws FHIRException { 2779 switch (hash) { 2780 case -1618432855: 2781 return addIdentifier(); 2782 case -892481550: 2783 return getStatusElement(); 2784 case 1028554472: 2785 return getCreatedElement(); 2786 case -791418107: 2787 return getPatient(); 2788 case 1524132147: 2789 return getEncounter(); 2790 case -1496880759: 2791 return getDateWrittenElement(); 2792 case 1430631077: 2793 return getPrescriber(); 2794 case -1767318363: 2795 return addLensSpecification(); 2796 default: 2797 return super.makeProperty(hash, name); 2798 } 2799 2800 } 2801 2802 @Override 2803 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2804 switch (hash) { 2805 case -1618432855: 2806 /* identifier */ return new String[] { "Identifier" }; 2807 case -892481550: 2808 /* status */ return new String[] { "code" }; 2809 case 1028554472: 2810 /* created */ return new String[] { "dateTime" }; 2811 case -791418107: 2812 /* patient */ return new String[] { "Reference" }; 2813 case 1524132147: 2814 /* encounter */ return new String[] { "Reference" }; 2815 case -1496880759: 2816 /* dateWritten */ return new String[] { "dateTime" }; 2817 case 1430631077: 2818 /* prescriber */ return new String[] { "Reference" }; 2819 case -1767318363: 2820 /* lensSpecification */ return new String[] {}; 2821 default: 2822 return super.getTypesForProperty(hash, name); 2823 } 2824 2825 } 2826 2827 @Override 2828 public Base addChild(String name) throws FHIRException { 2829 if (name.equals("identifier")) { 2830 return addIdentifier(); 2831 } else if (name.equals("status")) { 2832 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.status"); 2833 } else if (name.equals("created")) { 2834 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.created"); 2835 } else if (name.equals("patient")) { 2836 this.patient = new Reference(); 2837 return this.patient; 2838 } else if (name.equals("encounter")) { 2839 this.encounter = new Reference(); 2840 return this.encounter; 2841 } else if (name.equals("dateWritten")) { 2842 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.dateWritten"); 2843 } else if (name.equals("prescriber")) { 2844 this.prescriber = new Reference(); 2845 return this.prescriber; 2846 } else if (name.equals("lensSpecification")) { 2847 return addLensSpecification(); 2848 } else 2849 return super.addChild(name); 2850 } 2851 2852 public String fhirType() { 2853 return "VisionPrescription"; 2854 2855 } 2856 2857 public VisionPrescription copy() { 2858 VisionPrescription dst = new VisionPrescription(); 2859 copyValues(dst); 2860 return dst; 2861 } 2862 2863 public void copyValues(VisionPrescription dst) { 2864 super.copyValues(dst); 2865 if (identifier != null) { 2866 dst.identifier = new ArrayList<Identifier>(); 2867 for (Identifier i : identifier) 2868 dst.identifier.add(i.copy()); 2869 } 2870 ; 2871 dst.status = status == null ? null : status.copy(); 2872 dst.created = created == null ? null : created.copy(); 2873 dst.patient = patient == null ? null : patient.copy(); 2874 dst.encounter = encounter == null ? null : encounter.copy(); 2875 dst.dateWritten = dateWritten == null ? null : dateWritten.copy(); 2876 dst.prescriber = prescriber == null ? null : prescriber.copy(); 2877 if (lensSpecification != null) { 2878 dst.lensSpecification = new ArrayList<VisionPrescriptionLensSpecificationComponent>(); 2879 for (VisionPrescriptionLensSpecificationComponent i : lensSpecification) 2880 dst.lensSpecification.add(i.copy()); 2881 } 2882 ; 2883 } 2884 2885 protected VisionPrescription typedCopy() { 2886 return copy(); 2887 } 2888 2889 @Override 2890 public boolean equalsDeep(Base other_) { 2891 if (!super.equalsDeep(other_)) 2892 return false; 2893 if (!(other_ instanceof VisionPrescription)) 2894 return false; 2895 VisionPrescription o = (VisionPrescription) other_; 2896 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 2897 && compareDeep(created, o.created, true) && compareDeep(patient, o.patient, true) 2898 && compareDeep(encounter, o.encounter, true) && compareDeep(dateWritten, o.dateWritten, true) 2899 && compareDeep(prescriber, o.prescriber, true) && compareDeep(lensSpecification, o.lensSpecification, true); 2900 } 2901 2902 @Override 2903 public boolean equalsShallow(Base other_) { 2904 if (!super.equalsShallow(other_)) 2905 return false; 2906 if (!(other_ instanceof VisionPrescription)) 2907 return false; 2908 VisionPrescription o = (VisionPrescription) other_; 2909 return compareValues(status, o.status, true) && compareValues(created, o.created, true) 2910 && compareValues(dateWritten, o.dateWritten, true); 2911 } 2912 2913 public boolean isEmpty() { 2914 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, created, patient, encounter, 2915 dateWritten, prescriber, lensSpecification); 2916 } 2917 2918 @Override 2919 public ResourceType getResourceType() { 2920 return ResourceType.VisionPrescription; 2921 } 2922 2923 /** 2924 * Search parameter: <b>prescriber</b> 2925 * <p> 2926 * Description: <b>Who authorized the vision prescription</b><br> 2927 * Type: <b>reference</b><br> 2928 * Path: <b>VisionPrescription.prescriber</b><br> 2929 * </p> 2930 */ 2931 @SearchParamDefinition(name = "prescriber", path = "VisionPrescription.prescriber", description = "Who authorized the vision prescription", type = "reference", providesMembershipIn = { 2932 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Practitioner.class, 2933 PractitionerRole.class }) 2934 public static final String SP_PRESCRIBER = "prescriber"; 2935 /** 2936 * <b>Fluent Client</b> search parameter constant for <b>prescriber</b> 2937 * <p> 2938 * Description: <b>Who authorized the vision prescription</b><br> 2939 * Type: <b>reference</b><br> 2940 * Path: <b>VisionPrescription.prescriber</b><br> 2941 * </p> 2942 */ 2943 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRESCRIBER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2944 SP_PRESCRIBER); 2945 2946 /** 2947 * Constant for fluent queries to be used to add include statements. Specifies 2948 * the path value of "<b>VisionPrescription:prescriber</b>". 2949 */ 2950 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRESCRIBER = new ca.uhn.fhir.model.api.Include( 2951 "VisionPrescription:prescriber").toLocked(); 2952 2953 /** 2954 * Search parameter: <b>identifier</b> 2955 * <p> 2956 * Description: <b>Return prescriptions with this external identifier</b><br> 2957 * Type: <b>token</b><br> 2958 * Path: <b>VisionPrescription.identifier</b><br> 2959 * </p> 2960 */ 2961 @SearchParamDefinition(name = "identifier", path = "VisionPrescription.identifier", description = "Return prescriptions with this external identifier", type = "token") 2962 public static final String SP_IDENTIFIER = "identifier"; 2963 /** 2964 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2965 * <p> 2966 * Description: <b>Return prescriptions with this external identifier</b><br> 2967 * Type: <b>token</b><br> 2968 * Path: <b>VisionPrescription.identifier</b><br> 2969 * </p> 2970 */ 2971 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2972 SP_IDENTIFIER); 2973 2974 /** 2975 * Search parameter: <b>patient</b> 2976 * <p> 2977 * Description: <b>The identity of a patient to list dispenses for</b><br> 2978 * Type: <b>reference</b><br> 2979 * Path: <b>VisionPrescription.patient</b><br> 2980 * </p> 2981 */ 2982 @SearchParamDefinition(name = "patient", path = "VisionPrescription.patient", description = "The identity of a patient to list dispenses for", type = "reference", providesMembershipIn = { 2983 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 2984 public static final String SP_PATIENT = "patient"; 2985 /** 2986 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2987 * <p> 2988 * Description: <b>The identity of a patient to list dispenses for</b><br> 2989 * Type: <b>reference</b><br> 2990 * Path: <b>VisionPrescription.patient</b><br> 2991 * </p> 2992 */ 2993 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2994 SP_PATIENT); 2995 2996 /** 2997 * Constant for fluent queries to be used to add include statements. Specifies 2998 * the path value of "<b>VisionPrescription:patient</b>". 2999 */ 3000 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 3001 "VisionPrescription:patient").toLocked(); 3002 3003 /** 3004 * Search parameter: <b>datewritten</b> 3005 * <p> 3006 * Description: <b>Return prescriptions written on this date</b><br> 3007 * Type: <b>date</b><br> 3008 * Path: <b>VisionPrescription.dateWritten</b><br> 3009 * </p> 3010 */ 3011 @SearchParamDefinition(name = "datewritten", path = "VisionPrescription.dateWritten", description = "Return prescriptions written on this date", type = "date") 3012 public static final String SP_DATEWRITTEN = "datewritten"; 3013 /** 3014 * <b>Fluent Client</b> search parameter constant for <b>datewritten</b> 3015 * <p> 3016 * Description: <b>Return prescriptions written on this date</b><br> 3017 * Type: <b>date</b><br> 3018 * Path: <b>VisionPrescription.dateWritten</b><br> 3019 * </p> 3020 */ 3021 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATEWRITTEN = new ca.uhn.fhir.rest.gclient.DateClientParam( 3022 SP_DATEWRITTEN); 3023 3024 /** 3025 * Search parameter: <b>encounter</b> 3026 * <p> 3027 * Description: <b>Return prescriptions with this encounter identifier</b><br> 3028 * Type: <b>reference</b><br> 3029 * Path: <b>VisionPrescription.encounter</b><br> 3030 * </p> 3031 */ 3032 @SearchParamDefinition(name = "encounter", path = "VisionPrescription.encounter", description = "Return prescriptions with this encounter identifier", type = "reference", providesMembershipIn = { 3033 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 3034 public static final String SP_ENCOUNTER = "encounter"; 3035 /** 3036 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 3037 * <p> 3038 * Description: <b>Return prescriptions with this encounter identifier</b><br> 3039 * Type: <b>reference</b><br> 3040 * Path: <b>VisionPrescription.encounter</b><br> 3041 * </p> 3042 */ 3043 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3044 SP_ENCOUNTER); 3045 3046 /** 3047 * Constant for fluent queries to be used to add include statements. Specifies 3048 * the path value of "<b>VisionPrescription:encounter</b>". 3049 */ 3050 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 3051 "VisionPrescription:encounter").toLocked(); 3052 3053 /** 3054 * Search parameter: <b>status</b> 3055 * <p> 3056 * Description: <b>The status of the vision prescription</b><br> 3057 * Type: <b>token</b><br> 3058 * Path: <b>VisionPrescription.status</b><br> 3059 * </p> 3060 */ 3061 @SearchParamDefinition(name = "status", path = "VisionPrescription.status", description = "The status of the vision prescription", type = "token") 3062 public static final String SP_STATUS = "status"; 3063 /** 3064 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3065 * <p> 3066 * Description: <b>The status of the vision prescription</b><br> 3067 * Type: <b>token</b><br> 3068 * Path: <b>VisionPrescription.status</b><br> 3069 * </p> 3070 */ 3071 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3072 SP_STATUS); 3073 3074}