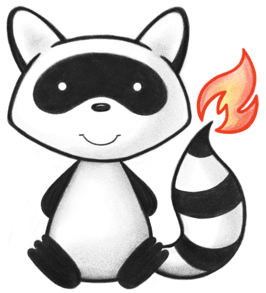
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum Adjudication { 037 038 /** 039 * The total submitted amount for the claim or group or line item. 040 */ 041 SUBMITTED, 042 /** 043 * Patient Co-Payment 044 */ 045 COPAY, 046 /** 047 * Amount of the change which is considered for adjudication. 048 */ 049 ELIGIBLE, 050 /** 051 * Amount deducted from the eligible amount prior to adjudication. 052 */ 053 DEDUCTIBLE, 054 /** 055 * The amount of deductible which could not allocated to other line items. 056 */ 057 UNALLOCDEDUCT, 058 /** 059 * Eligible Percentage. 060 */ 061 ELIGPERCENT, 062 /** 063 * The amount of tax. 064 */ 065 TAX, 066 /** 067 * Amount payable under the coverage 068 */ 069 BENEFIT, 070 /** 071 * added to help the parsers 072 */ 073 NULL; 074 075 public static Adjudication fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("submitted".equals(codeString)) 079 return SUBMITTED; 080 if ("copay".equals(codeString)) 081 return COPAY; 082 if ("eligible".equals(codeString)) 083 return ELIGIBLE; 084 if ("deductible".equals(codeString)) 085 return DEDUCTIBLE; 086 if ("unallocdeduct".equals(codeString)) 087 return UNALLOCDEDUCT; 088 if ("eligpercent".equals(codeString)) 089 return ELIGPERCENT; 090 if ("tax".equals(codeString)) 091 return TAX; 092 if ("benefit".equals(codeString)) 093 return BENEFIT; 094 throw new FHIRException("Unknown Adjudication code '" + codeString + "'"); 095 } 096 097 public String toCode() { 098 switch (this) { 099 case SUBMITTED: 100 return "submitted"; 101 case COPAY: 102 return "copay"; 103 case ELIGIBLE: 104 return "eligible"; 105 case DEDUCTIBLE: 106 return "deductible"; 107 case UNALLOCDEDUCT: 108 return "unallocdeduct"; 109 case ELIGPERCENT: 110 return "eligpercent"; 111 case TAX: 112 return "tax"; 113 case BENEFIT: 114 return "benefit"; 115 case NULL: 116 return null; 117 default: 118 return "?"; 119 } 120 } 121 122 public String getSystem() { 123 return "http://terminology.hl7.org/CodeSystem/adjudication"; 124 } 125 126 public String getDefinition() { 127 switch (this) { 128 case SUBMITTED: 129 return "The total submitted amount for the claim or group or line item."; 130 case COPAY: 131 return "Patient Co-Payment"; 132 case ELIGIBLE: 133 return "Amount of the change which is considered for adjudication."; 134 case DEDUCTIBLE: 135 return "Amount deducted from the eligible amount prior to adjudication."; 136 case UNALLOCDEDUCT: 137 return "The amount of deductible which could not allocated to other line items."; 138 case ELIGPERCENT: 139 return "Eligible Percentage."; 140 case TAX: 141 return "The amount of tax."; 142 case BENEFIT: 143 return "Amount payable under the coverage"; 144 case NULL: 145 return null; 146 default: 147 return "?"; 148 } 149 } 150 151 public String getDisplay() { 152 switch (this) { 153 case SUBMITTED: 154 return "Submitted Amount"; 155 case COPAY: 156 return "CoPay"; 157 case ELIGIBLE: 158 return "Eligible Amount"; 159 case DEDUCTIBLE: 160 return "Deductible"; 161 case UNALLOCDEDUCT: 162 return "Unallocated Deductible"; 163 case ELIGPERCENT: 164 return "Eligible %"; 165 case TAX: 166 return "Tax"; 167 case BENEFIT: 168 return "Benefit Amount"; 169 case NULL: 170 return null; 171 default: 172 return "?"; 173 } 174 } 175 176}