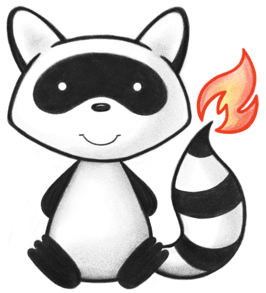
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum AdverseEventCausalityAssess { 037 038 /** 039 * i) Event or laboratory test abnormality, with plausible time relationship to 040 * drug intake; ii) Cannot be explained by disease or other drugs; iii) Response 041 * to withdrawal plausible (pharmacologically, pathologically); iv) Event 042 * definitive pharmacologically or phenomenologically (i.e. an objective and 043 * specific medical disorder or a recognized pharmacological phenomenon); or v) 044 * Re-challenge satisfactory, if necessary. 045 */ 046 CERTAIN, 047 /** 048 * i) Event or laboratory test abnormality, with reasonable time relationship to 049 * drug intake; ii) Unlikely to be attributed to disease or other drugs; iii) 050 * Response to withdrawal clinically reasonable; or iv) Re-challenge not 051 * required. 052 */ 053 PROBABLYLIKELY, 054 /** 055 * i) Event or laboratory test abnormality, with reasonable time relationship to 056 * drug intake; ii) Could also be explained by disease or other drugs; or iii) 057 * Information on drug withdrawal may be lacking or unclear. 058 */ 059 POSSIBLE, 060 /** 061 * i) Event or laboratory test abnormality, with a time to drug intake that 062 * makes a relationship improbable (but not impossible); or ii) Disease or other 063 * drugs provide plausible explanations. 064 */ 065 UNLIKELY, 066 /** 067 * i) Event or laboratory test abnormality; ii) More data for proper assessment 068 * needed; or iii) Additional data under examination. 069 */ 070 CONDITIONALCLASSIFIED, 071 /** 072 * i) Report suggesting an adverse reaction; ii) Cannot be judged because 073 * information is insufficient or contradictory; or iii) Data cannot be 074 * supplemented or verified. 075 */ 076 UNASSESSABLEUNCLASSIFIABLE, 077 /** 078 * added to help the parsers 079 */ 080 NULL; 081 082 public static AdverseEventCausalityAssess fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("Certain".equals(codeString)) 086 return CERTAIN; 087 if ("Probably-Likely".equals(codeString)) 088 return PROBABLYLIKELY; 089 if ("Possible".equals(codeString)) 090 return POSSIBLE; 091 if ("Unlikely".equals(codeString)) 092 return UNLIKELY; 093 if ("Conditional-Classified".equals(codeString)) 094 return CONDITIONALCLASSIFIED; 095 if ("Unassessable-Unclassifiable".equals(codeString)) 096 return UNASSESSABLEUNCLASSIFIABLE; 097 throw new FHIRException("Unknown AdverseEventCausalityAssess code '" + codeString + "'"); 098 } 099 100 public String toCode() { 101 switch (this) { 102 case CERTAIN: 103 return "Certain"; 104 case PROBABLYLIKELY: 105 return "Probably-Likely"; 106 case POSSIBLE: 107 return "Possible"; 108 case UNLIKELY: 109 return "Unlikely"; 110 case CONDITIONALCLASSIFIED: 111 return "Conditional-Classified"; 112 case UNASSESSABLEUNCLASSIFIABLE: 113 return "Unassessable-Unclassifiable"; 114 case NULL: 115 return null; 116 default: 117 return "?"; 118 } 119 } 120 121 public String getSystem() { 122 return "http://terminology.hl7.org/CodeSystem/adverse-event-causality-assess"; 123 } 124 125 public String getDefinition() { 126 switch (this) { 127 case CERTAIN: 128 return "i) Event or laboratory test abnormality, with plausible time relationship to drug intake; ii) Cannot be explained by disease or other drugs; iii) Response to withdrawal plausible (pharmacologically, pathologically); iv) Event definitive pharmacologically or phenomenologically (i.e. an objective and specific medical disorder or a recognized pharmacological phenomenon); or v) Re-challenge satisfactory, if necessary."; 129 case PROBABLYLIKELY: 130 return "i) Event or laboratory test abnormality, with reasonable time relationship to drug intake; ii) Unlikely to be attributed to disease or other drugs; iii) Response to withdrawal clinically reasonable; or iv) Re-challenge not required."; 131 case POSSIBLE: 132 return "i) Event or laboratory test abnormality, with reasonable time relationship to drug intake; ii) Could also be explained by disease or other drugs; or iii) Information on drug withdrawal may be lacking or unclear."; 133 case UNLIKELY: 134 return "i) Event or laboratory test abnormality, with a time to drug intake that makes a relationship improbable (but not impossible); or ii) Disease or other drugs provide plausible explanations."; 135 case CONDITIONALCLASSIFIED: 136 return "i) Event or laboratory test abnormality; ii) More data for proper assessment needed; or iii) Additional data under examination."; 137 case UNASSESSABLEUNCLASSIFIABLE: 138 return "i) Report suggesting an adverse reaction; ii) Cannot be judged because information is insufficient or contradictory; or iii) Data cannot be supplemented or verified."; 139 case NULL: 140 return null; 141 default: 142 return "?"; 143 } 144 } 145 146 public String getDisplay() { 147 switch (this) { 148 case CERTAIN: 149 return "Certain"; 150 case PROBABLYLIKELY: 151 return "Probably/Likely"; 152 case POSSIBLE: 153 return "Possible"; 154 case UNLIKELY: 155 return "Unlikely"; 156 case CONDITIONALCLASSIFIED: 157 return "Conditional/Classified"; 158 case UNASSESSABLEUNCLASSIFIABLE: 159 return "Unassessable/Unclassifiable"; 160 case NULL: 161 return null; 162 default: 163 return "?"; 164 } 165 } 166 167}