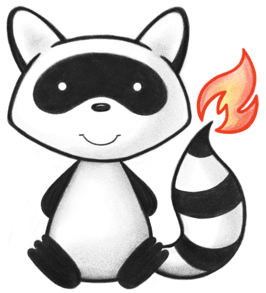
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ConceptProperties { 037 038 /** 039 * True if the concept is not considered active - e.g. not a valid concept any 040 * more. Property type is boolean, default value is false 041 */ 042 INACTIVE, 043 /** 044 * The date at which a concept was deprecated. Concepts that are deprecated but 045 * not inactive can still be used, but their use is discouraged, and they should 046 * be expected to be made inactive in a future release. Property type is 047 * dateTime 048 */ 049 DEPRECATED, 050 /** 051 * The concept is not intended to be chosen by the user - only intended to be 052 * used as a selector for other concepts. Note, though, that the interpretation 053 * of this is highly contextual; all concepts are selectable in some context. 054 * Property type is boolean 055 */ 056 NOTSELECTABLE, 057 /** 058 * The concept identified in this property is a parent of the concept on which 059 * it is a property. The property type will be 'code'. The meaning of 'parent' 060 * is defined by the hierarchyMeaning attribute 061 */ 062 PARENT, 063 /** 064 * The concept identified in this property is a child of the concept on which it 065 * is a property. The property type will be 'code'. The meaning of 'child' is 066 * defined by the hierarchyMeaning attribute 067 */ 068 CHILD, 069 /** 070 * added to help the parsers 071 */ 072 NULL; 073 074 public static ConceptProperties fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("inactive".equals(codeString)) 078 return INACTIVE; 079 if ("deprecated".equals(codeString)) 080 return DEPRECATED; 081 if ("notSelectable".equals(codeString)) 082 return NOTSELECTABLE; 083 if ("parent".equals(codeString)) 084 return PARENT; 085 if ("child".equals(codeString)) 086 return CHILD; 087 throw new FHIRException("Unknown ConceptProperties code '" + codeString + "'"); 088 } 089 090 public String toCode() { 091 switch (this) { 092 case INACTIVE: 093 return "inactive"; 094 case DEPRECATED: 095 return "deprecated"; 096 case NOTSELECTABLE: 097 return "notSelectable"; 098 case PARENT: 099 return "parent"; 100 case CHILD: 101 return "child"; 102 case NULL: 103 return null; 104 default: 105 return "?"; 106 } 107 } 108 109 public String getSystem() { 110 return "http://hl7.org/fhir/concept-properties"; 111 } 112 113 public String getDefinition() { 114 switch (this) { 115 case INACTIVE: 116 return "True if the concept is not considered active - e.g. not a valid concept any more. Property type is boolean, default value is false"; 117 case DEPRECATED: 118 return "The date at which a concept was deprecated. Concepts that are deprecated but not inactive can still be used, but their use is discouraged, and they should be expected to be made inactive in a future release. Property type is dateTime"; 119 case NOTSELECTABLE: 120 return "The concept is not intended to be chosen by the user - only intended to be used as a selector for other concepts. Note, though, that the interpretation of this is highly contextual; all concepts are selectable in some context. Property type is boolean"; 121 case PARENT: 122 return "The concept identified in this property is a parent of the concept on which it is a property. The property type will be 'code'. The meaning of 'parent' is defined by the hierarchyMeaning attribute"; 123 case CHILD: 124 return "The concept identified in this property is a child of the concept on which it is a property. The property type will be 'code'. The meaning of 'child' is defined by the hierarchyMeaning attribute"; 125 case NULL: 126 return null; 127 default: 128 return "?"; 129 } 130 } 131 132 public String getDisplay() { 133 switch (this) { 134 case INACTIVE: 135 return "Inactive"; 136 case DEPRECATED: 137 return "Deprecated"; 138 case NOTSELECTABLE: 139 return "Not Selectable"; 140 case PARENT: 141 return "Parent"; 142 case CHILD: 143 return "Child"; 144 case NULL: 145 return null; 146 default: 147 return "?"; 148 } 149 } 150 151}