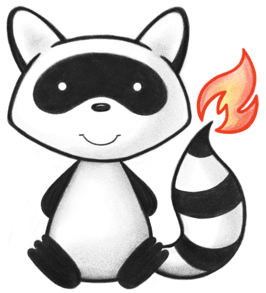
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ContainerCap { 037 038 /** 039 * red cap. 040 */ 041 RED, 042 /** 043 * yellow cap. 044 */ 045 YELLOW, 046 /** 047 * dark yellow cap. 048 */ 049 DARKYELLOW, 050 /** 051 * grey cap. 052 */ 053 GREY, 054 /** 055 * light blue cap. 056 */ 057 LIGHTBLUE, 058 /** 059 * black cap. 060 */ 061 BLACK, 062 /** 063 * green cap. 064 */ 065 GREEN, 066 /** 067 * light green cap. 068 */ 069 LIGHTGREEN, 070 /** 071 * lavender cap. 072 */ 073 LAVENDER, 074 /** 075 * brown cap. 076 */ 077 BROWN, 078 /** 079 * white cap. 080 */ 081 WHITE, 082 /** 083 * pink cap. 084 */ 085 PINK, 086 /** 087 * added to help the parsers 088 */ 089 NULL; 090 091 public static ContainerCap fromCode(String codeString) throws FHIRException { 092 if (codeString == null || "".equals(codeString)) 093 return null; 094 if ("red".equals(codeString)) 095 return RED; 096 if ("yellow".equals(codeString)) 097 return YELLOW; 098 if ("dark-yellow".equals(codeString)) 099 return DARKYELLOW; 100 if ("grey".equals(codeString)) 101 return GREY; 102 if ("light-blue".equals(codeString)) 103 return LIGHTBLUE; 104 if ("black".equals(codeString)) 105 return BLACK; 106 if ("green".equals(codeString)) 107 return GREEN; 108 if ("light-green".equals(codeString)) 109 return LIGHTGREEN; 110 if ("lavender".equals(codeString)) 111 return LAVENDER; 112 if ("brown".equals(codeString)) 113 return BROWN; 114 if ("white".equals(codeString)) 115 return WHITE; 116 if ("pink".equals(codeString)) 117 return PINK; 118 throw new FHIRException("Unknown ContainerCap code '" + codeString + "'"); 119 } 120 121 public String toCode() { 122 switch (this) { 123 case RED: 124 return "red"; 125 case YELLOW: 126 return "yellow"; 127 case DARKYELLOW: 128 return "dark-yellow"; 129 case GREY: 130 return "grey"; 131 case LIGHTBLUE: 132 return "light-blue"; 133 case BLACK: 134 return "black"; 135 case GREEN: 136 return "green"; 137 case LIGHTGREEN: 138 return "light-green"; 139 case LAVENDER: 140 return "lavender"; 141 case BROWN: 142 return "brown"; 143 case WHITE: 144 return "white"; 145 case PINK: 146 return "pink"; 147 case NULL: 148 return null; 149 default: 150 return "?"; 151 } 152 } 153 154 public String getSystem() { 155 return "http://terminology.hl7.org/CodeSystem/container-cap"; 156 } 157 158 public String getDefinition() { 159 switch (this) { 160 case RED: 161 return "red cap."; 162 case YELLOW: 163 return "yellow cap."; 164 case DARKYELLOW: 165 return "dark yellow cap."; 166 case GREY: 167 return "grey cap."; 168 case LIGHTBLUE: 169 return "light blue cap."; 170 case BLACK: 171 return "black cap."; 172 case GREEN: 173 return "green cap."; 174 case LIGHTGREEN: 175 return "light green cap."; 176 case LAVENDER: 177 return "lavender cap."; 178 case BROWN: 179 return "brown cap."; 180 case WHITE: 181 return "white cap."; 182 case PINK: 183 return "pink cap."; 184 case NULL: 185 return null; 186 default: 187 return "?"; 188 } 189 } 190 191 public String getDisplay() { 192 switch (this) { 193 case RED: 194 return "red cap"; 195 case YELLOW: 196 return "yellow cap"; 197 case DARKYELLOW: 198 return "dark yellow cap"; 199 case GREY: 200 return "grey cap"; 201 case LIGHTBLUE: 202 return "light blue cap"; 203 case BLACK: 204 return "black cap"; 205 case GREEN: 206 return "green cap"; 207 case LIGHTGREEN: 208 return "light green cap"; 209 case LAVENDER: 210 return "lavender cap"; 211 case BROWN: 212 return "brown cap"; 213 case WHITE: 214 return "white cap"; 215 case PINK: 216 return "pink cap"; 217 case NULL: 218 return null; 219 default: 220 return "?"; 221 } 222 } 223 224}