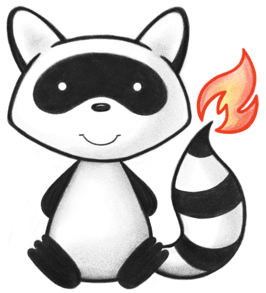
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ContractStatus { 037 038 /** 039 * Contract is augmented with additional information to correct errors in a 040 * predecessor or to updated values in a predecessor. Usage: Contract altered 041 * within effective time. Precedence Order = 9. Comparable FHIR and v.3 status 042 * codes: revised; replaced. 043 */ 044 AMENDED, 045 /** 046 * Contract is augmented with additional information that was missing from a 047 * predecessor Contract. Usage: Contract altered within effective time. 048 * Precedence Order = 9. Comparable FHIR and v.3 status codes: updated, 049 * replaced. 050 */ 051 APPENDED, 052 /** 053 * Contract is terminated due to failure of the Grantor and/or the Grantee to 054 * fulfil one or more contract provisions. Usage: Abnormal contract termination. 055 * Precedence Order = 10. Comparable FHIR and v.3 status codes: stopped; failed; 056 * aborted. 057 */ 058 CANCELLED, 059 /** 060 * Contract is pended to rectify failure of the Grantor or the Grantee to fulfil 061 * contract provision(s). E.g., Grantee complaint about Grantor's failure to 062 * comply with contract provisions. Usage: Contract pended. Precedence Order = 063 * 7. Comparable FHIR and v.3 status codes: on hold; pended; suspended. 064 */ 065 DISPUTED, 066 /** 067 * Contract was created in error. No Precedence Order. Status may be applied to 068 * a Contract with any status. 069 */ 070 ENTEREDINERROR, 071 /** 072 * Contract execution pending; may be executed when either the Grantor or the 073 * Grantee accepts the contract provisions by signing. I.e., where either the 074 * Grantor or the Grantee has signed, but not both. E.g., when an insurance 075 * applicant signs the insurers application, which references the policy. Usage: 076 * Optional first step of contract execution activity. May be skipped and 077 * contracting activity moves directly to executed state. Precedence Order = 3. 078 * Comparable FHIR and v.3 status codes: draft; preliminary; planned; intended; 079 * active. 080 */ 081 EXECUTABLE, 082 /** 083 * Contract is activated for period stipulated when both the Grantor and Grantee 084 * have signed it. Usage: Required state for normal completion of contracting 085 * activity. Precedence Order = 6. Comparable FHIR and v.3 status codes: 086 * accepted; completed. 087 */ 088 EXECUTED, 089 /** 090 * Contract execution is suspended while either or both the Grantor and Grantee 091 * propose and consider new or revised contract provisions. I.e., where the 092 * party which has not signed proposes changes to the terms. E .g., a life 093 * insurer declines to agree to the signed application because the life insurer 094 * has evidence that the applicant, who asserted to being younger or a 095 * non-smoker to get a lower premium rate - but offers instead to agree to a 096 * higher premium based on the applicants actual age or smoking status. Usage: 097 * Optional contract activity between executable and executed state. Precedence 098 * Order = 4. Comparable FHIR and v.3 status codes: in progress; review; held. 099 */ 100 NEGOTIABLE, 101 /** 102 * Contract is a proposal by either the Grantor or the Grantee. Aka - A Contract 103 * hard copy or electronic 'template', 'form' or 'application'. E.g., health 104 * insurance application; consent directive form. Usage: Beginning of contract 105 * negotiation, which may have been completed as a precondition because used for 106 * 0..* contracts. Precedence Order = 2. Comparable FHIR and v.3 status codes: 107 * requested; new. 108 */ 109 OFFERED, 110 /** 111 * Contract template is available as the basis for an application or offer by 112 * the Grantor or Grantee. E.g., health insurance policy; consent directive 113 * policy. Usage: Required initial contract activity, which may have been 114 * completed as a precondition because used for 0..* contracts. Precedence Order 115 * = 1. Comparable FHIR and v.3 status codes: proposed; intended. 116 */ 117 POLICY, 118 /** 119 * Execution of the Contract is not completed because either or both the Grantor 120 * and Grantee decline to accept some or all of the contract provisions. Usage: 121 * Optional contract activity between executable and abnormal termination. 122 * Precedence Order = 5. Comparable FHIR and v.3 status codes: stopped; 123 * cancelled. 124 */ 125 REJECTED, 126 /** 127 * Beginning of a successor Contract at the termination of predecessor Contract 128 * lifecycle. Usage: Follows termination of a preceding Contract that has 129 * reached its expiry date. Precedence Order = 13. Comparable FHIR and v.3 130 * status codes: superseded. 131 */ 132 RENEWED, 133 /** 134 * A Contract that is rescinded. May be required prior to replacing with an 135 * updated Contract. Comparable FHIR and v.3 status codes: nullified. 136 */ 137 REVOKED, 138 /** 139 * Contract is reactivated after being pended because of faulty execution. 140 * *E.g., competency of the signer(s), or where the policy is substantially 141 * different from and did not accompany the application/form so that the 142 * applicant could not compare them. Aka - ''reactivated''. Usage: Optional 143 * stage where a pended contract is reactivated. Precedence Order = 8. 144 * Comparable FHIR and v.3 status codes: reactivated. 145 */ 146 RESOLVED, 147 /** 148 * Contract reaches its expiry date. It might or might not be renewed or 149 * renegotiated. Usage: Normal end of contract period. Precedence Order = 12. 150 * Comparable FHIR and v.3 status codes: Obsoleted. 151 */ 152 TERMINATED, 153 /** 154 * added to help the parsers 155 */ 156 NULL; 157 158 public static ContractStatus fromCode(String codeString) throws FHIRException { 159 if (codeString == null || "".equals(codeString)) 160 return null; 161 if ("amended".equals(codeString)) 162 return AMENDED; 163 if ("appended".equals(codeString)) 164 return APPENDED; 165 if ("cancelled".equals(codeString)) 166 return CANCELLED; 167 if ("disputed".equals(codeString)) 168 return DISPUTED; 169 if ("entered-in-error".equals(codeString)) 170 return ENTEREDINERROR; 171 if ("executable".equals(codeString)) 172 return EXECUTABLE; 173 if ("executed".equals(codeString)) 174 return EXECUTED; 175 if ("negotiable".equals(codeString)) 176 return NEGOTIABLE; 177 if ("offered".equals(codeString)) 178 return OFFERED; 179 if ("policy".equals(codeString)) 180 return POLICY; 181 if ("rejected".equals(codeString)) 182 return REJECTED; 183 if ("renewed".equals(codeString)) 184 return RENEWED; 185 if ("revoked".equals(codeString)) 186 return REVOKED; 187 if ("resolved".equals(codeString)) 188 return RESOLVED; 189 if ("terminated".equals(codeString)) 190 return TERMINATED; 191 throw new FHIRException("Unknown ContractStatus code '" + codeString + "'"); 192 } 193 194 public String toCode() { 195 switch (this) { 196 case AMENDED: 197 return "amended"; 198 case APPENDED: 199 return "appended"; 200 case CANCELLED: 201 return "cancelled"; 202 case DISPUTED: 203 return "disputed"; 204 case ENTEREDINERROR: 205 return "entered-in-error"; 206 case EXECUTABLE: 207 return "executable"; 208 case EXECUTED: 209 return "executed"; 210 case NEGOTIABLE: 211 return "negotiable"; 212 case OFFERED: 213 return "offered"; 214 case POLICY: 215 return "policy"; 216 case REJECTED: 217 return "rejected"; 218 case RENEWED: 219 return "renewed"; 220 case REVOKED: 221 return "revoked"; 222 case RESOLVED: 223 return "resolved"; 224 case TERMINATED: 225 return "terminated"; 226 case NULL: 227 return null; 228 default: 229 return "?"; 230 } 231 } 232 233 public String getSystem() { 234 return "http://hl7.org/fhir/contract-status"; 235 } 236 237 public String getDefinition() { 238 switch (this) { 239 case AMENDED: 240 return "Contract is augmented with additional information to correct errors in a predecessor or to updated values in a predecessor. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: revised; replaced."; 241 case APPENDED: 242 return "Contract is augmented with additional information that was missing from a predecessor Contract. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: updated, replaced."; 243 case CANCELLED: 244 return "Contract is terminated due to failure of the Grantor and/or the Grantee to fulfil one or more contract provisions. Usage: Abnormal contract termination. Precedence Order = 10. Comparable FHIR and v.3 status codes: stopped; failed; aborted."; 245 case DISPUTED: 246 return "Contract is pended to rectify failure of the Grantor or the Grantee to fulfil contract provision(s). E.g., Grantee complaint about Grantor's failure to comply with contract provisions. Usage: Contract pended. Precedence Order = 7. Comparable FHIR and v.3 status codes: on hold; pended; suspended."; 247 case ENTEREDINERROR: 248 return "Contract was created in error. No Precedence Order. Status may be applied to a Contract with any status."; 249 case EXECUTABLE: 250 return "Contract execution pending; may be executed when either the Grantor or the Grantee accepts the contract provisions by signing. I.e., where either the Grantor or the Grantee has signed, but not both. E.g., when an insurance applicant signs the insurers application, which references the policy. Usage: Optional first step of contract execution activity. May be skipped and contracting activity moves directly to executed state. Precedence Order = 3. Comparable FHIR and v.3 status codes: draft; preliminary; planned; intended; active."; 251 case EXECUTED: 252 return "Contract is activated for period stipulated when both the Grantor and Grantee have signed it. Usage: Required state for normal completion of contracting activity. Precedence Order = 6. Comparable FHIR and v.3 status codes: accepted; completed."; 253 case NEGOTIABLE: 254 return "Contract execution is suspended while either or both the Grantor and Grantee propose and consider new or revised contract provisions. I.e., where the party which has not signed proposes changes to the terms. E .g., a life insurer declines to agree to the signed application because the life insurer has evidence that the applicant, who asserted to being younger or a non-smoker to get a lower premium rate - but offers instead to agree to a higher premium based on the applicants actual age or smoking status. Usage: Optional contract activity between executable and executed state. Precedence Order = 4. Comparable FHIR and v.3 status codes: in progress; review; held."; 255 case OFFERED: 256 return "Contract is a proposal by either the Grantor or the Grantee. Aka - A Contract hard copy or electronic 'template', 'form' or 'application'. E.g., health insurance application; consent directive form. Usage: Beginning of contract negotiation, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 2. Comparable FHIR and v.3 status codes: requested; new."; 257 case POLICY: 258 return "Contract template is available as the basis for an application or offer by the Grantor or Grantee. E.g., health insurance policy; consent directive policy. Usage: Required initial contract activity, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 1. Comparable FHIR and v.3 status codes: proposed; intended."; 259 case REJECTED: 260 return " Execution of the Contract is not completed because either or both the Grantor and Grantee decline to accept some or all of the contract provisions. Usage: Optional contract activity between executable and abnormal termination. Precedence Order = 5. Comparable FHIR and v.3 status codes: stopped; cancelled."; 261 case RENEWED: 262 return "Beginning of a successor Contract at the termination of predecessor Contract lifecycle. Usage: Follows termination of a preceding Contract that has reached its expiry date. Precedence Order = 13. Comparable FHIR and v.3 status codes: superseded."; 263 case REVOKED: 264 return "A Contract that is rescinded. May be required prior to replacing with an updated Contract. Comparable FHIR and v.3 status codes: nullified."; 265 case RESOLVED: 266 return "Contract is reactivated after being pended because of faulty execution. *E.g., competency of the signer(s), or where the policy is substantially different from and did not accompany the application/form so that the applicant could not compare them. Aka - ''reactivated''. Usage: Optional stage where a pended contract is reactivated. Precedence Order = 8. Comparable FHIR and v.3 status codes: reactivated."; 267 case TERMINATED: 268 return "Contract reaches its expiry date. It might or might not be renewed or renegotiated. Usage: Normal end of contract period. Precedence Order = 12. Comparable FHIR and v.3 status codes: Obsoleted."; 269 case NULL: 270 return null; 271 default: 272 return "?"; 273 } 274 } 275 276 public String getDisplay() { 277 switch (this) { 278 case AMENDED: 279 return "Amended"; 280 case APPENDED: 281 return "Appended"; 282 case CANCELLED: 283 return "Cancelled"; 284 case DISPUTED: 285 return "Disputed"; 286 case ENTEREDINERROR: 287 return "Entered in Error"; 288 case EXECUTABLE: 289 return "Executable"; 290 case EXECUTED: 291 return "Executed"; 292 case NEGOTIABLE: 293 return "Negotiable"; 294 case OFFERED: 295 return "Offered"; 296 case POLICY: 297 return "Policy"; 298 case REJECTED: 299 return "Rejected"; 300 case RENEWED: 301 return "Renewed"; 302 case REVOKED: 303 return "Revoked"; 304 case RESOLVED: 305 return "Resolved"; 306 case TERMINATED: 307 return "Terminated"; 308 case NULL: 309 return null; 310 default: 311 return "?"; 312 } 313 } 314 315}