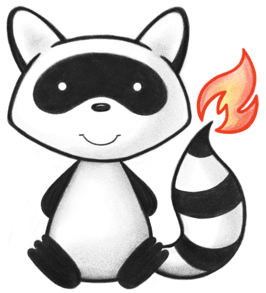
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum CoverageeligibilityresponseExAuthSupport { 037 038 /** 039 * A request or authorization for laboratory diagnostic tests. 040 */ 041 LABORDER, 042 /** 043 * A report on laboratory diagnostic test(s). 044 */ 045 LABREPORT, 046 /** 047 * A request or authorization for diagnostic imaging. 048 */ 049 DIAGNOSTICIMAGEORDER, 050 /** 051 * A report on diagnostic image(s). 052 */ 053 DIAGNOSTICIMAGEREPORT, 054 /** 055 * A report from a licensed professional regarding the siutation, condition or 056 * proposed treatment. 057 */ 058 PROFESSIONALREPORT, 059 /** 060 * A formal accident report as would be filed with police or a simlar official 061 * body. 062 */ 063 ACCIDENTREPORT, 064 /** 065 * A physical model of the affected area. 066 */ 067 MODEL, 068 /** 069 * A photograph of the affected area. 070 */ 071 PICTURE, 072 /** 073 * added to help the parsers 074 */ 075 NULL; 076 077 public static CoverageeligibilityresponseExAuthSupport fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("laborder".equals(codeString)) 081 return LABORDER; 082 if ("labreport".equals(codeString)) 083 return LABREPORT; 084 if ("diagnosticimageorder".equals(codeString)) 085 return DIAGNOSTICIMAGEORDER; 086 if ("diagnosticimagereport".equals(codeString)) 087 return DIAGNOSTICIMAGEREPORT; 088 if ("professionalreport".equals(codeString)) 089 return PROFESSIONALREPORT; 090 if ("accidentreport".equals(codeString)) 091 return ACCIDENTREPORT; 092 if ("model".equals(codeString)) 093 return MODEL; 094 if ("picture".equals(codeString)) 095 return PICTURE; 096 throw new FHIRException("Unknown CoverageeligibilityresponseExAuthSupport code '" + codeString + "'"); 097 } 098 099 public String toCode() { 100 switch (this) { 101 case LABORDER: 102 return "laborder"; 103 case LABREPORT: 104 return "labreport"; 105 case DIAGNOSTICIMAGEORDER: 106 return "diagnosticimageorder"; 107 case DIAGNOSTICIMAGEREPORT: 108 return "diagnosticimagereport"; 109 case PROFESSIONALREPORT: 110 return "professionalreport"; 111 case ACCIDENTREPORT: 112 return "accidentreport"; 113 case MODEL: 114 return "model"; 115 case PICTURE: 116 return "picture"; 117 case NULL: 118 return null; 119 default: 120 return "?"; 121 } 122 } 123 124 public String getSystem() { 125 return "http://terminology.hl7.org/CodeSystem/coverageeligibilityresponse-ex-auth-support"; 126 } 127 128 public String getDefinition() { 129 switch (this) { 130 case LABORDER: 131 return "A request or authorization for laboratory diagnostic tests."; 132 case LABREPORT: 133 return "A report on laboratory diagnostic test(s)."; 134 case DIAGNOSTICIMAGEORDER: 135 return "A request or authorization for diagnostic imaging."; 136 case DIAGNOSTICIMAGEREPORT: 137 return "A report on diagnostic image(s)."; 138 case PROFESSIONALREPORT: 139 return "A report from a licensed professional regarding the siutation, condition or proposed treatment."; 140 case ACCIDENTREPORT: 141 return "A formal accident report as would be filed with police or a simlar official body."; 142 case MODEL: 143 return "A physical model of the affected area."; 144 case PICTURE: 145 return "A photograph of the affected area."; 146 case NULL: 147 return null; 148 default: 149 return "?"; 150 } 151 } 152 153 public String getDisplay() { 154 switch (this) { 155 case LABORDER: 156 return "Lab Order"; 157 case LABREPORT: 158 return "Lab Report"; 159 case DIAGNOSTICIMAGEORDER: 160 return "Diagnostic Image Order"; 161 case DIAGNOSTICIMAGEREPORT: 162 return "Diagnostic Image Report"; 163 case PROFESSIONALREPORT: 164 return "Professional Report"; 165 case ACCIDENTREPORT: 166 return "Accident Report"; 167 case MODEL: 168 return "Model"; 169 case PICTURE: 170 return "Picture"; 171 case NULL: 172 return null; 173 default: 174 return "?"; 175 } 176 } 177 178}