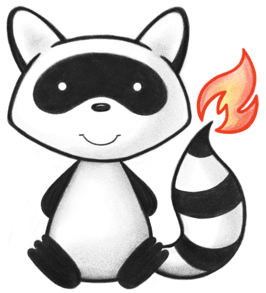
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum DataAbsentReason { 037 038 /** 039 * The value is expected to exist but is not known. 040 */ 041 UNKNOWN, 042 /** 043 * The source was asked but does not know the value. 044 */ 045 ASKEDUNKNOWN, 046 /** 047 * There is reason to expect (from the workflow) that the value may become 048 * known. 049 */ 050 TEMPUNKNOWN, 051 /** 052 * The workflow didn't lead to this value being known. 053 */ 054 NOTASKED, 055 /** 056 * The source was asked but declined to answer. 057 */ 058 ASKEDDECLINED, 059 /** 060 * The information is not available due to security, privacy or related reasons. 061 */ 062 MASKED, 063 /** 064 * There is no proper value for this element (e.g. last menstrual period for a 065 * male). 066 */ 067 NOTAPPLICABLE, 068 /** 069 * The source system wasn't capable of supporting this element. 070 */ 071 UNSUPPORTED, 072 /** 073 * The content of the data is represented in the resource narrative. 074 */ 075 ASTEXT, 076 /** 077 * Some system or workflow process error means that the information is not 078 * available. 079 */ 080 ERROR, 081 /** 082 * The numeric value is undefined or unrepresentable due to a floating point 083 * processing error. 084 */ 085 NOTANUMBER, 086 /** 087 * The numeric value is excessively low and unrepresentable due to a floating 088 * point processing error. 089 */ 090 NEGATIVEINFINITY, 091 /** 092 * The numeric value is excessively high and unrepresentable due to a floating 093 * point processing error. 094 */ 095 POSITIVEINFINITY, 096 /** 097 * The value is not available because the observation procedure (test, etc.) was 098 * not performed. 099 */ 100 NOTPERFORMED, 101 /** 102 * The value is not permitted in this context (e.g. due to profiles, or the base 103 * data types). 104 */ 105 NOTPERMITTED, 106 /** 107 * added to help the parsers 108 */ 109 NULL; 110 111 public static DataAbsentReason fromCode(String codeString) throws FHIRException { 112 if (codeString == null || "".equals(codeString)) 113 return null; 114 if ("unknown".equals(codeString)) 115 return UNKNOWN; 116 if ("asked-unknown".equals(codeString)) 117 return ASKEDUNKNOWN; 118 if ("temp-unknown".equals(codeString)) 119 return TEMPUNKNOWN; 120 if ("not-asked".equals(codeString)) 121 return NOTASKED; 122 if ("asked-declined".equals(codeString)) 123 return ASKEDDECLINED; 124 if ("masked".equals(codeString)) 125 return MASKED; 126 if ("not-applicable".equals(codeString)) 127 return NOTAPPLICABLE; 128 if ("unsupported".equals(codeString)) 129 return UNSUPPORTED; 130 if ("as-text".equals(codeString)) 131 return ASTEXT; 132 if ("error".equals(codeString)) 133 return ERROR; 134 if ("not-a-number".equals(codeString)) 135 return NOTANUMBER; 136 if ("negative-infinity".equals(codeString)) 137 return NEGATIVEINFINITY; 138 if ("positive-infinity".equals(codeString)) 139 return POSITIVEINFINITY; 140 if ("not-performed".equals(codeString)) 141 return NOTPERFORMED; 142 if ("not-permitted".equals(codeString)) 143 return NOTPERMITTED; 144 throw new FHIRException("Unknown DataAbsentReason code '" + codeString + "'"); 145 } 146 147 public String toCode() { 148 switch (this) { 149 case UNKNOWN: 150 return "unknown"; 151 case ASKEDUNKNOWN: 152 return "asked-unknown"; 153 case TEMPUNKNOWN: 154 return "temp-unknown"; 155 case NOTASKED: 156 return "not-asked"; 157 case ASKEDDECLINED: 158 return "asked-declined"; 159 case MASKED: 160 return "masked"; 161 case NOTAPPLICABLE: 162 return "not-applicable"; 163 case UNSUPPORTED: 164 return "unsupported"; 165 case ASTEXT: 166 return "as-text"; 167 case ERROR: 168 return "error"; 169 case NOTANUMBER: 170 return "not-a-number"; 171 case NEGATIVEINFINITY: 172 return "negative-infinity"; 173 case POSITIVEINFINITY: 174 return "positive-infinity"; 175 case NOTPERFORMED: 176 return "not-performed"; 177 case NOTPERMITTED: 178 return "not-permitted"; 179 case NULL: 180 return null; 181 default: 182 return "?"; 183 } 184 } 185 186 public String getSystem() { 187 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 188 } 189 190 public String getDefinition() { 191 switch (this) { 192 case UNKNOWN: 193 return "The value is expected to exist but is not known."; 194 case ASKEDUNKNOWN: 195 return "The source was asked but does not know the value."; 196 case TEMPUNKNOWN: 197 return "There is reason to expect (from the workflow) that the value may become known."; 198 case NOTASKED: 199 return "The workflow didn't lead to this value being known."; 200 case ASKEDDECLINED: 201 return "The source was asked but declined to answer."; 202 case MASKED: 203 return "The information is not available due to security, privacy or related reasons."; 204 case NOTAPPLICABLE: 205 return "There is no proper value for this element (e.g. last menstrual period for a male)."; 206 case UNSUPPORTED: 207 return "The source system wasn't capable of supporting this element."; 208 case ASTEXT: 209 return "The content of the data is represented in the resource narrative."; 210 case ERROR: 211 return "Some system or workflow process error means that the information is not available."; 212 case NOTANUMBER: 213 return "The numeric value is undefined or unrepresentable due to a floating point processing error."; 214 case NEGATIVEINFINITY: 215 return "The numeric value is excessively low and unrepresentable due to a floating point processing error."; 216 case POSITIVEINFINITY: 217 return "The numeric value is excessively high and unrepresentable due to a floating point processing error."; 218 case NOTPERFORMED: 219 return "The value is not available because the observation procedure (test, etc.) was not performed."; 220 case NOTPERMITTED: 221 return "The value is not permitted in this context (e.g. due to profiles, or the base data types)."; 222 case NULL: 223 return null; 224 default: 225 return "?"; 226 } 227 } 228 229 public String getDisplay() { 230 switch (this) { 231 case UNKNOWN: 232 return "Unknown"; 233 case ASKEDUNKNOWN: 234 return "Asked But Unknown"; 235 case TEMPUNKNOWN: 236 return "Temporarily Unknown"; 237 case NOTASKED: 238 return "Not Asked"; 239 case ASKEDDECLINED: 240 return "Asked But Declined"; 241 case MASKED: 242 return "Masked"; 243 case NOTAPPLICABLE: 244 return "Not Applicable"; 245 case UNSUPPORTED: 246 return "Unsupported"; 247 case ASTEXT: 248 return "As Text"; 249 case ERROR: 250 return "Error"; 251 case NOTANUMBER: 252 return "Not a Number (NaN)"; 253 case NEGATIVEINFINITY: 254 return "Negative Infinity (NINF)"; 255 case POSITIVEINFINITY: 256 return "Positive Infinity (PINF)"; 257 case NOTPERFORMED: 258 return "Not Performed"; 259 case NOTPERMITTED: 260 return "Not Permitted"; 261 case NULL: 262 return null; 263 default: 264 return "?"; 265 } 266 } 267 268}