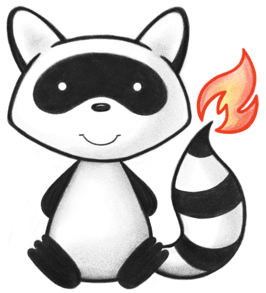
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum DefinitionUse { 037 038 /** 039 * This structure is defined as part of the base FHIR Specification 040 */ 041 FHIRSTRUCTURE, 042 /** 043 * This structure is intended to be treated like a FHIR resource (e.g. on the 044 * FHIR API) 045 */ 046 CUSTOMRESOURCE, 047 /** 048 * This structure captures an analysis of a domain 049 */ 050 DAM, 051 /** 052 * This structure represents and existing structure (e.g. CDA, HL7 v2) 053 */ 054 WIREFORMAT, 055 /** 056 * This structure captures an analysis of a domain 057 */ 058 ARCHETYPE, 059 /** 060 * This structure is a template (n.b: 'template' has many meanings) 061 */ 062 TEMPLATE, 063 /** 064 * added to help the parsers 065 */ 066 NULL; 067 068 public static DefinitionUse fromCode(String codeString) throws FHIRException { 069 if (codeString == null || "".equals(codeString)) 070 return null; 071 if ("fhir-structure".equals(codeString)) 072 return FHIRSTRUCTURE; 073 if ("custom-resource".equals(codeString)) 074 return CUSTOMRESOURCE; 075 if ("dam".equals(codeString)) 076 return DAM; 077 if ("wire-format".equals(codeString)) 078 return WIREFORMAT; 079 if ("archetype".equals(codeString)) 080 return ARCHETYPE; 081 if ("template".equals(codeString)) 082 return TEMPLATE; 083 throw new FHIRException("Unknown DefinitionUse code '" + codeString + "'"); 084 } 085 086 public String toCode() { 087 switch (this) { 088 case FHIRSTRUCTURE: 089 return "fhir-structure"; 090 case CUSTOMRESOURCE: 091 return "custom-resource"; 092 case DAM: 093 return "dam"; 094 case WIREFORMAT: 095 return "wire-format"; 096 case ARCHETYPE: 097 return "archetype"; 098 case TEMPLATE: 099 return "template"; 100 case NULL: 101 return null; 102 default: 103 return "?"; 104 } 105 } 106 107 public String getSystem() { 108 return "http://terminology.hl7.org/CodeSystem/definition-use"; 109 } 110 111 public String getDefinition() { 112 switch (this) { 113 case FHIRSTRUCTURE: 114 return "This structure is defined as part of the base FHIR Specification"; 115 case CUSTOMRESOURCE: 116 return "This structure is intended to be treated like a FHIR resource (e.g. on the FHIR API)"; 117 case DAM: 118 return "This structure captures an analysis of a domain"; 119 case WIREFORMAT: 120 return "This structure represents and existing structure (e.g. CDA, HL7 v2)"; 121 case ARCHETYPE: 122 return "This structure captures an analysis of a domain"; 123 case TEMPLATE: 124 return "This structure is a template (n.b: 'template' has many meanings)"; 125 case NULL: 126 return null; 127 default: 128 return "?"; 129 } 130 } 131 132 public String getDisplay() { 133 switch (this) { 134 case FHIRSTRUCTURE: 135 return "FHIR Structure"; 136 case CUSTOMRESOURCE: 137 return "Custom Resource"; 138 case DAM: 139 return "Domain Analysis Model"; 140 case WIREFORMAT: 141 return "Wire Format"; 142 case ARCHETYPE: 143 return "Domain Analysis Model"; 144 case TEMPLATE: 145 return "Template"; 146 case NULL: 147 return null; 148 default: 149 return "?"; 150 } 151 } 152 153}