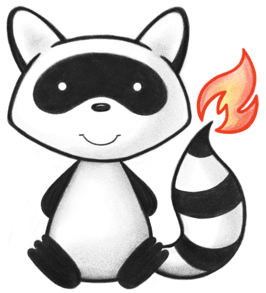
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum DiagnosticReportStatus { 037 038 /** 039 * The existence of the report is registered, but there is nothing yet 040 * available. 041 */ 042 REGISTERED, 043 /** 044 * This is a partial (e.g. initial, interim or preliminary) report: data in the 045 * report may be incomplete or unverified. 046 */ 047 PARTIAL, 048 /** 049 * Verified early results are available, but not all results are final. 050 */ 051 PRELIMINARY, 052 /** 053 * The report is complete and verified by an authorized person. 054 */ 055 FINAL, 056 /** 057 * Subsequent to being final, the report has been modified. This includes any 058 * change in the results, diagnosis, narrative text, or other content of a 059 * report that has been issued. 060 */ 061 AMENDED, 062 /** 063 * Subsequent to being final, the report has been modified to correct an error 064 * in the report or referenced results. 065 */ 066 CORRECTED, 067 /** 068 * Subsequent to being final, the report has been modified by adding new 069 * content. The existing content is unchanged. 070 */ 071 APPENDED, 072 /** 073 * The report is unavailable because the measurement was not started or not 074 * completed (also sometimes called "aborted"). 075 */ 076 CANCELLED, 077 /** 078 * The report has been withdrawn following a previous final release. This 079 * electronic record should never have existed, though it is possible that 080 * real-world decisions were based on it. (If real-world activity has occurred, 081 * the status should be "cancelled" rather than "entered-in-error".). 082 */ 083 ENTEREDINERROR, 084 /** 085 * The authoring/source system does not know which of the status values 086 * currently applies for this observation. Note: This concept is not to be used 087 * for "other" - one of the listed statuses is presumed to apply, but the 088 * authoring/source system does not know which. 089 */ 090 UNKNOWN, 091 /** 092 * added to help the parsers 093 */ 094 NULL; 095 096 public static DiagnosticReportStatus fromCode(String codeString) throws FHIRException { 097 if (codeString == null || "".equals(codeString)) 098 return null; 099 if ("registered".equals(codeString)) 100 return REGISTERED; 101 if ("partial".equals(codeString)) 102 return PARTIAL; 103 if ("preliminary".equals(codeString)) 104 return PRELIMINARY; 105 if ("final".equals(codeString)) 106 return FINAL; 107 if ("amended".equals(codeString)) 108 return AMENDED; 109 if ("corrected".equals(codeString)) 110 return CORRECTED; 111 if ("appended".equals(codeString)) 112 return APPENDED; 113 if ("cancelled".equals(codeString)) 114 return CANCELLED; 115 if ("entered-in-error".equals(codeString)) 116 return ENTEREDINERROR; 117 if ("unknown".equals(codeString)) 118 return UNKNOWN; 119 throw new FHIRException("Unknown DiagnosticReportStatus code '" + codeString + "'"); 120 } 121 122 public String toCode() { 123 switch (this) { 124 case REGISTERED: 125 return "registered"; 126 case PARTIAL: 127 return "partial"; 128 case PRELIMINARY: 129 return "preliminary"; 130 case FINAL: 131 return "final"; 132 case AMENDED: 133 return "amended"; 134 case CORRECTED: 135 return "corrected"; 136 case APPENDED: 137 return "appended"; 138 case CANCELLED: 139 return "cancelled"; 140 case ENTEREDINERROR: 141 return "entered-in-error"; 142 case UNKNOWN: 143 return "unknown"; 144 case NULL: 145 return null; 146 default: 147 return "?"; 148 } 149 } 150 151 public String getSystem() { 152 return "http://hl7.org/fhir/diagnostic-report-status"; 153 } 154 155 public String getDefinition() { 156 switch (this) { 157 case REGISTERED: 158 return "The existence of the report is registered, but there is nothing yet available."; 159 case PARTIAL: 160 return "This is a partial (e.g. initial, interim or preliminary) report: data in the report may be incomplete or unverified."; 161 case PRELIMINARY: 162 return "Verified early results are available, but not all results are final."; 163 case FINAL: 164 return "The report is complete and verified by an authorized person."; 165 case AMENDED: 166 return "Subsequent to being final, the report has been modified. This includes any change in the results, diagnosis, narrative text, or other content of a report that has been issued."; 167 case CORRECTED: 168 return "Subsequent to being final, the report has been modified to correct an error in the report or referenced results."; 169 case APPENDED: 170 return "Subsequent to being final, the report has been modified by adding new content. The existing content is unchanged."; 171 case CANCELLED: 172 return "The report is unavailable because the measurement was not started or not completed (also sometimes called \"aborted\")."; 173 case ENTEREDINERROR: 174 return "The report has been withdrawn following a previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)."; 175 case UNKNOWN: 176 return "The authoring/source system does not know which of the status values currently applies for this observation. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 177 case NULL: 178 return null; 179 default: 180 return "?"; 181 } 182 } 183 184 public String getDisplay() { 185 switch (this) { 186 case REGISTERED: 187 return "Registered"; 188 case PARTIAL: 189 return "Partial"; 190 case PRELIMINARY: 191 return "Preliminary"; 192 case FINAL: 193 return "Final"; 194 case AMENDED: 195 return "Amended"; 196 case CORRECTED: 197 return "Corrected"; 198 case APPENDED: 199 return "Appended"; 200 case CANCELLED: 201 return "Cancelled"; 202 case ENTEREDINERROR: 203 return "Entered in Error"; 204 case UNKNOWN: 205 return "Unknown"; 206 case NULL: 207 return null; 208 default: 209 return "?"; 210 } 211 } 212 213}