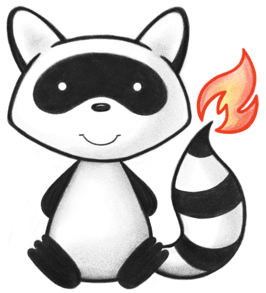
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ExpansionParameterSource { 037 038 /** 039 * The parameter was supplied by the client in the $expand request. 040 */ 041 INPUT, 042 /** 043 * The parameter was added by the expansion engine on the server. 044 */ 045 SERVER, 046 /** 047 * The parameter was added from one the code systems used in the $expand 048 * operation. 049 */ 050 CODESYSTEM, 051 /** 052 * added to help the parsers 053 */ 054 NULL; 055 056 public static ExpansionParameterSource fromCode(String codeString) throws FHIRException { 057 if (codeString == null || "".equals(codeString)) 058 return null; 059 if ("input".equals(codeString)) 060 return INPUT; 061 if ("server".equals(codeString)) 062 return SERVER; 063 if ("codesystem".equals(codeString)) 064 return CODESYSTEM; 065 throw new FHIRException("Unknown ExpansionParameterSource code '" + codeString + "'"); 066 } 067 068 public String toCode() { 069 switch (this) { 070 case INPUT: 071 return "input"; 072 case SERVER: 073 return "server"; 074 case CODESYSTEM: 075 return "codesystem"; 076 case NULL: 077 return null; 078 default: 079 return "?"; 080 } 081 } 082 083 public String getSystem() { 084 return "http://terminology.hl7.org/CodeSystem/expansion-parameter-source"; 085 } 086 087 public String getDefinition() { 088 switch (this) { 089 case INPUT: 090 return "The parameter was supplied by the client in the $expand request."; 091 case SERVER: 092 return "The parameter was added by the expansion engine on the server."; 093 case CODESYSTEM: 094 return "The parameter was added from one the code systems used in the $expand operation."; 095 case NULL: 096 return null; 097 default: 098 return "?"; 099 } 100 } 101 102 public String getDisplay() { 103 switch (this) { 104 case INPUT: 105 return "Client Input"; 106 case SERVER: 107 return "Server Engine"; 108 case CODESYSTEM: 109 return "Code System"; 110 case NULL: 111 return null; 112 default: 113 return "?"; 114 } 115 } 116 117}