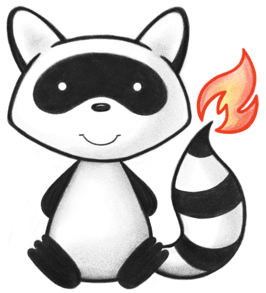
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum MapTransform { 037 038 /** 039 * create(type : string) - type is passed through to the application on the 040 * standard API, and must be known by it. 041 */ 042 CREATE, 043 /** 044 * copy(source). 045 */ 046 COPY, 047 /** 048 * truncate(source, length) - source must be stringy type. 049 */ 050 TRUNCATE, 051 /** 052 * escape(source, fmt1, fmt2) - change source from one kind of escaping to 053 * another (plain, java, xml, json). note that this is for when the string 054 * itself is escaped. 055 */ 056 ESCAPE, 057 /** 058 * cast(source, type?) - case source from one type to another. target type can 059 * be left as implicit if there is one and only one target type known. 060 */ 061 CAST, 062 /** 063 * append(source...) - source is element or string. 064 */ 065 APPEND, 066 /** 067 * translate(source, uri_of_map) - use the translate operation. 068 */ 069 TRANSLATE, 070 /** 071 * reference(source : object) - return a string that references the provided 072 * tree properly. 073 */ 074 REFERENCE, 075 /** 076 * Perform a date operation. *Parameters to be documented*. 077 */ 078 DATEOP, 079 /** 080 * Generate a random UUID (in lowercase). No Parameters. 081 */ 082 UUID, 083 /** 084 * Return the appropriate string to put in a reference that refers to the 085 * resource provided as a parameter. 086 */ 087 POINTER, 088 /** 089 * Execute the supplied FHIRPath expression and use the value returned by that. 090 */ 091 EVALUATE, 092 /** 093 * Create a CodeableConcept. Parameters = (text) or (system. Code[, display]). 094 */ 095 CC, 096 /** 097 * Create a Coding. Parameters = (system. Code[, display]). 098 */ 099 C, 100 /** 101 * Create a quantity. Parameters = (text) or (value, unit, [system, code]) where 102 * text is the natural representation e.g. [comparator]value[space]unit. 103 */ 104 QTY, 105 /** 106 * Create an identifier. Parameters = (system, value[, type]) where type is a 107 * code from the identifier type value set. 108 */ 109 ID, 110 /** 111 * Create a contact details. Parameters = (value) or (system, value). If no 112 * system is provided, the system should be inferred from the content of the 113 * value. 114 */ 115 CP, 116 /** 117 * added to help the parsers 118 */ 119 NULL; 120 121 public static MapTransform fromCode(String codeString) throws FHIRException { 122 if (codeString == null || "".equals(codeString)) 123 return null; 124 if ("create".equals(codeString)) 125 return CREATE; 126 if ("copy".equals(codeString)) 127 return COPY; 128 if ("truncate".equals(codeString)) 129 return TRUNCATE; 130 if ("escape".equals(codeString)) 131 return ESCAPE; 132 if ("cast".equals(codeString)) 133 return CAST; 134 if ("append".equals(codeString)) 135 return APPEND; 136 if ("translate".equals(codeString)) 137 return TRANSLATE; 138 if ("reference".equals(codeString)) 139 return REFERENCE; 140 if ("dateOp".equals(codeString)) 141 return DATEOP; 142 if ("uuid".equals(codeString)) 143 return UUID; 144 if ("pointer".equals(codeString)) 145 return POINTER; 146 if ("evaluate".equals(codeString)) 147 return EVALUATE; 148 if ("cc".equals(codeString)) 149 return CC; 150 if ("c".equals(codeString)) 151 return C; 152 if ("qty".equals(codeString)) 153 return QTY; 154 if ("id".equals(codeString)) 155 return ID; 156 if ("cp".equals(codeString)) 157 return CP; 158 throw new FHIRException("Unknown MapTransform code '" + codeString + "'"); 159 } 160 161 public String toCode() { 162 switch (this) { 163 case CREATE: 164 return "create"; 165 case COPY: 166 return "copy"; 167 case TRUNCATE: 168 return "truncate"; 169 case ESCAPE: 170 return "escape"; 171 case CAST: 172 return "cast"; 173 case APPEND: 174 return "append"; 175 case TRANSLATE: 176 return "translate"; 177 case REFERENCE: 178 return "reference"; 179 case DATEOP: 180 return "dateOp"; 181 case UUID: 182 return "uuid"; 183 case POINTER: 184 return "pointer"; 185 case EVALUATE: 186 return "evaluate"; 187 case CC: 188 return "cc"; 189 case C: 190 return "c"; 191 case QTY: 192 return "qty"; 193 case ID: 194 return "id"; 195 case CP: 196 return "cp"; 197 case NULL: 198 return null; 199 default: 200 return "?"; 201 } 202 } 203 204 public String getSystem() { 205 return "http://hl7.org/fhir/map-transform"; 206 } 207 208 public String getDefinition() { 209 switch (this) { 210 case CREATE: 211 return "create(type : string) - type is passed through to the application on the standard API, and must be known by it."; 212 case COPY: 213 return "copy(source)."; 214 case TRUNCATE: 215 return "truncate(source, length) - source must be stringy type."; 216 case ESCAPE: 217 return "escape(source, fmt1, fmt2) - change source from one kind of escaping to another (plain, java, xml, json). note that this is for when the string itself is escaped."; 218 case CAST: 219 return "cast(source, type?) - case source from one type to another. target type can be left as implicit if there is one and only one target type known."; 220 case APPEND: 221 return "append(source...) - source is element or string."; 222 case TRANSLATE: 223 return "translate(source, uri_of_map) - use the translate operation."; 224 case REFERENCE: 225 return "reference(source : object) - return a string that references the provided tree properly."; 226 case DATEOP: 227 return "Perform a date operation. *Parameters to be documented*."; 228 case UUID: 229 return "Generate a random UUID (in lowercase). No Parameters."; 230 case POINTER: 231 return "Return the appropriate string to put in a reference that refers to the resource provided as a parameter."; 232 case EVALUATE: 233 return "Execute the supplied FHIRPath expression and use the value returned by that."; 234 case CC: 235 return "Create a CodeableConcept. Parameters = (text) or (system. Code[, display])."; 236 case C: 237 return "Create a Coding. Parameters = (system. Code[, display])."; 238 case QTY: 239 return "Create a quantity. Parameters = (text) or (value, unit, [system, code]) where text is the natural representation e.g. [comparator]value[space]unit."; 240 case ID: 241 return "Create an identifier. Parameters = (system, value[, type]) where type is a code from the identifier type value set."; 242 case CP: 243 return "Create a contact details. Parameters = (value) or (system, value). If no system is provided, the system should be inferred from the content of the value."; 244 case NULL: 245 return null; 246 default: 247 return "?"; 248 } 249 } 250 251 public String getDisplay() { 252 switch (this) { 253 case CREATE: 254 return "create"; 255 case COPY: 256 return "copy"; 257 case TRUNCATE: 258 return "truncate"; 259 case ESCAPE: 260 return "escape"; 261 case CAST: 262 return "cast"; 263 case APPEND: 264 return "append"; 265 case TRANSLATE: 266 return "translate"; 267 case REFERENCE: 268 return "reference"; 269 case DATEOP: 270 return "dateOp"; 271 case UUID: 272 return "uuid"; 273 case POINTER: 274 return "pointer"; 275 case EVALUATE: 276 return "evaluate"; 277 case CC: 278 return "cc"; 279 case C: 280 return "c"; 281 case QTY: 282 return "qty"; 283 case ID: 284 return "id"; 285 case CP: 286 return "cp"; 287 case NULL: 288 return null; 289 default: 290 return "?"; 291 } 292 } 293 294}