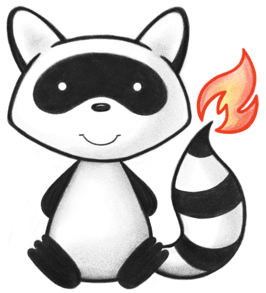
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum MeasurePopulation { 037 038 /** 039 * The initial population refers to all patients or events to be evaluated by a 040 * quality measure involving patients who share a common set of specified 041 * characterstics. All patients or events counted (for example, as numerator, as 042 * denominator) are drawn from the initial population. 043 */ 044 INITIALPOPULATION, 045 /** 046 * The upper portion of a fraction used to calculate a rate, proportion, or 047 * ratio. Also called the measure focus, it is the target process, condition, 048 * event, or outcome. Numerator criteria are the processes or outcomes expected 049 * for each patient, or event defined in the denominator. A numerator statement 050 * describes the clinical action that satisfies the conditions of the measure. 051 */ 052 NUMERATOR, 053 /** 054 * Numerator exclusion criteria define patients or events to be removed from the 055 * numerator. Numerator exclusions are used in proportion and ratio measures to 056 * help narrow the numerator (for inverted measures). 057 */ 058 NUMERATOREXCLUSION, 059 /** 060 * The lower portion of a fraction used to calculate a rate, proportion, or 061 * ratio. The denominator can be the same as the initial population, or a subset 062 * of the initial population to further constrain the population for the purpose 063 * of the measure. 064 */ 065 DENOMINATOR, 066 /** 067 * Denominator exclusion criteria define patients or events that should be 068 * removed from the denominator before determining if numerator criteria are 069 * met. Denominator exclusions are used in proportion and ratio measures to help 070 * narrow the denominator. For example, patients with bilateral lower extremity 071 * amputations would be listed as a denominator exclusion for a measure 072 * requiring foot exams. 073 */ 074 DENOMINATOREXCLUSION, 075 /** 076 * Denominator exceptions are conditions that should remove a patient or event 077 * from the denominator of a measure only if the numerator criteria are not met. 078 * Denominator exception allows for adjustment of the calculated score for those 079 * providers with higher risk populations. Denominator exception criteria are 080 * only used in proportion measures. 081 */ 082 DENOMINATOREXCEPTION, 083 /** 084 * Measure population criteria define the patients or events for which the 085 * individual observation for the measure should be taken. Measure populations 086 * are used for continuous variable measures rather than numerator and 087 * denominator criteria. 088 */ 089 MEASUREPOPULATION, 090 /** 091 * Measure population criteria define the patients or events that should be 092 * removed from the measure population before determining the outcome of one or 093 * more continuous variables defined for the measure observation. Measure 094 * population exclusion criteria are used within continuous variable measures to 095 * help narrow the measure population. 096 */ 097 MEASUREPOPULATIONEXCLUSION, 098 /** 099 * Defines the individual observation to be performed for each patient or event 100 * in the measure population. Measure observations for each case in the 101 * population are aggregated to determine the overall measure score for the 102 * population. 103 */ 104 MEASUREOBSERVATION, 105 /** 106 * added to help the parsers 107 */ 108 NULL; 109 110 public static MeasurePopulation fromCode(String codeString) throws FHIRException { 111 if (codeString == null || "".equals(codeString)) 112 return null; 113 if ("initial-population".equals(codeString)) 114 return INITIALPOPULATION; 115 if ("numerator".equals(codeString)) 116 return NUMERATOR; 117 if ("numerator-exclusion".equals(codeString)) 118 return NUMERATOREXCLUSION; 119 if ("denominator".equals(codeString)) 120 return DENOMINATOR; 121 if ("denominator-exclusion".equals(codeString)) 122 return DENOMINATOREXCLUSION; 123 if ("denominator-exception".equals(codeString)) 124 return DENOMINATOREXCEPTION; 125 if ("measure-population".equals(codeString)) 126 return MEASUREPOPULATION; 127 if ("measure-population-exclusion".equals(codeString)) 128 return MEASUREPOPULATIONEXCLUSION; 129 if ("measure-observation".equals(codeString)) 130 return MEASUREOBSERVATION; 131 throw new FHIRException("Unknown MeasurePopulation code '" + codeString + "'"); 132 } 133 134 public String toCode() { 135 switch (this) { 136 case INITIALPOPULATION: 137 return "initial-population"; 138 case NUMERATOR: 139 return "numerator"; 140 case NUMERATOREXCLUSION: 141 return "numerator-exclusion"; 142 case DENOMINATOR: 143 return "denominator"; 144 case DENOMINATOREXCLUSION: 145 return "denominator-exclusion"; 146 case DENOMINATOREXCEPTION: 147 return "denominator-exception"; 148 case MEASUREPOPULATION: 149 return "measure-population"; 150 case MEASUREPOPULATIONEXCLUSION: 151 return "measure-population-exclusion"; 152 case MEASUREOBSERVATION: 153 return "measure-observation"; 154 case NULL: 155 return null; 156 default: 157 return "?"; 158 } 159 } 160 161 public String getSystem() { 162 return "http://terminology.hl7.org/CodeSystem/measure-population"; 163 } 164 165 public String getDefinition() { 166 switch (this) { 167 case INITIALPOPULATION: 168 return "The initial population refers to all patients or events to be evaluated by a quality measure involving patients who share a common set of specified characterstics. All patients or events counted (for example, as numerator, as denominator) are drawn from the initial population."; 169 case NUMERATOR: 170 return "The upper portion of a fraction used to calculate a rate, proportion, or ratio. Also called the measure focus, it is the target process, condition, event, or outcome. Numerator criteria are the processes or outcomes expected for each patient, or event defined in the denominator. A numerator statement describes the clinical action that satisfies the conditions of the measure."; 171 case NUMERATOREXCLUSION: 172 return "Numerator exclusion criteria define patients or events to be removed from the numerator. Numerator exclusions are used in proportion and ratio measures to help narrow the numerator (for inverted measures)."; 173 case DENOMINATOR: 174 return "The lower portion of a fraction used to calculate a rate, proportion, or ratio. The denominator can be the same as the initial population, or a subset of the initial population to further constrain the population for the purpose of the measure."; 175 case DENOMINATOREXCLUSION: 176 return "Denominator exclusion criteria define patients or events that should be removed from the denominator before determining if numerator criteria are met. Denominator exclusions are used in proportion and ratio measures to help narrow the denominator. For example, patients with bilateral lower extremity amputations would be listed as a denominator exclusion for a measure requiring foot exams."; 177 case DENOMINATOREXCEPTION: 178 return "Denominator exceptions are conditions that should remove a patient or event from the denominator of a measure only if the numerator criteria are not met. Denominator exception allows for adjustment of the calculated score for those providers with higher risk populations. Denominator exception criteria are only used in proportion measures."; 179 case MEASUREPOPULATION: 180 return "Measure population criteria define the patients or events for which the individual observation for the measure should be taken. Measure populations are used for continuous variable measures rather than numerator and denominator criteria."; 181 case MEASUREPOPULATIONEXCLUSION: 182 return "Measure population criteria define the patients or events that should be removed from the measure population before determining the outcome of one or more continuous variables defined for the measure observation. Measure population exclusion criteria are used within continuous variable measures to help narrow the measure population."; 183 case MEASUREOBSERVATION: 184 return "Defines the individual observation to be performed for each patient or event in the measure population. Measure observations for each case in the population are aggregated to determine the overall measure score for the population."; 185 case NULL: 186 return null; 187 default: 188 return "?"; 189 } 190 } 191 192 public String getDisplay() { 193 switch (this) { 194 case INITIALPOPULATION: 195 return "Initial Population"; 196 case NUMERATOR: 197 return "Numerator"; 198 case NUMERATOREXCLUSION: 199 return "Numerator Exclusion"; 200 case DENOMINATOR: 201 return "Denominator"; 202 case DENOMINATOREXCLUSION: 203 return "Denominator Exclusion"; 204 case DENOMINATOREXCEPTION: 205 return "Denominator Exception"; 206 case MEASUREPOPULATION: 207 return "Measure Population"; 208 case MEASUREPOPULATIONEXCLUSION: 209 return "Measure Population Exclusion"; 210 case MEASUREOBSERVATION: 211 return "Measure Observation"; 212 case NULL: 213 return null; 214 default: 215 return "?"; 216 } 217 } 218 219}