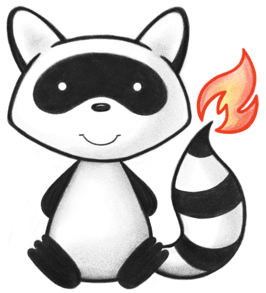
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum MedicationdispenseStatusReason { 037 038 /** 039 * The order has been stopped by the prescriber but this fact has not 040 * necessarily captured electronically. Example: A verbal stop, a fax, etc. 041 */ 042 FRR01, 043 /** 044 * Order has not been fulfilled within a reasonable amount of time, and might 045 * not be current. 046 */ 047 FRR02, 048 /** 049 * Data needed to safely act on the order which was expected to become available 050 * independent of the order is not yet available. Example: Lab results, 051 * diagnostic imaging, etc. 052 */ 053 FRR03, 054 /** 055 * Product not available or manufactured. Cannot supply. 056 */ 057 FRR04, 058 /** 059 * The dispenser has ethical, religious or moral objections to fulfilling the 060 * order/dispensing the product. 061 */ 062 FRR05, 063 /** 064 * Fulfiller not able to provide appropriate care associated with fulfilling the 065 * order. Example: Therapy requires ongoing monitoring by fulfiller and 066 * fulfiller will be ending practice, leaving town, unable to schedule necessary 067 * time, etc. 068 */ 069 FRR06, 070 /** 071 * This therapy has been ordered as a backup to a preferred therapy. This order 072 * will be released when and if the preferred therapy is unsuccessful. 073 */ 074 ALTCHOICE, 075 /** 076 * Clarification is required before the order can be acted upon. 077 */ 078 CLARIF, 079 /** 080 * The current level of the medication in the patient's system is too high. The 081 * medication is suspended to allow the level to subside to a safer level. 082 */ 083 DRUGHIGH, 084 /** 085 * The patient has been admitted to a care facility and their community 086 * medications are suspended until hospital discharge. 087 */ 088 HOSPADM, 089 /** 090 * The therapy would interfere with a planned lab test and the therapy is being 091 * withdrawn until the test is completed. 092 */ 093 LABINT, 094 /** 095 * Patient not available for a period of time due to a scheduled therapy, leave 096 * of absence or other reason. 097 */ 098 NONAVAIL, 099 /** 100 * The patient is pregnant or breast feeding. The therapy will be resumed when 101 * the pregnancy is complete and the patient is no longer breastfeeding. 102 */ 103 PREG, 104 /** 105 * The patient is believed to be allergic to a substance that is part of the 106 * therapy and the therapy is being temporarily withdrawn to confirm. 107 */ 108 SAIG, 109 /** 110 * The drug interacts with a short-term treatment that is more urgently 111 * required. This order will be resumed when the short-term treatment is 112 * complete. 113 */ 114 SDDI, 115 /** 116 * Another short-term co-occurring therapy fulfills the same purpose as this 117 * therapy. This therapy will be resumed when the co-occuring therapy is 118 * complete. 119 */ 120 SDUPTHER, 121 /** 122 * The patient is believed to have an intolerance to a substance that is part of 123 * the therapy and the therapy is being temporarily withdrawn to confirm. 124 */ 125 SINTOL, 126 /** 127 * The drug is contraindicated for patients receiving surgery and the patient is 128 * scheduled to be admitted for surgery in the near future. The drug will be 129 * resumed when the patient has sufficiently recovered from the surgery. 130 */ 131 SURG, 132 /** 133 * The patient was previously receiving a medication contraindicated with the 134 * current medication. The current medication will remain on hold until the 135 * prior medication has been cleansed from their system. 136 */ 137 WASHOUT, 138 /** 139 * Drug out of stock. Cannot supply. 140 */ 141 OUTOFSTOCK, 142 /** 143 * Drug no longer marketed Cannot supply. 144 */ 145 OFFMARKET, 146 /** 147 * added to help the parsers 148 */ 149 NULL; 150 151 public static MedicationdispenseStatusReason fromCode(String codeString) throws FHIRException { 152 if (codeString == null || "".equals(codeString)) 153 return null; 154 if ("frr01".equals(codeString)) 155 return FRR01; 156 if ("frr02".equals(codeString)) 157 return FRR02; 158 if ("frr03".equals(codeString)) 159 return FRR03; 160 if ("frr04".equals(codeString)) 161 return FRR04; 162 if ("frr05".equals(codeString)) 163 return FRR05; 164 if ("frr06".equals(codeString)) 165 return FRR06; 166 if ("altchoice".equals(codeString)) 167 return ALTCHOICE; 168 if ("clarif".equals(codeString)) 169 return CLARIF; 170 if ("drughigh".equals(codeString)) 171 return DRUGHIGH; 172 if ("hospadm".equals(codeString)) 173 return HOSPADM; 174 if ("labint".equals(codeString)) 175 return LABINT; 176 if ("non-avail".equals(codeString)) 177 return NONAVAIL; 178 if ("preg".equals(codeString)) 179 return PREG; 180 if ("saig".equals(codeString)) 181 return SAIG; 182 if ("sddi".equals(codeString)) 183 return SDDI; 184 if ("sdupther".equals(codeString)) 185 return SDUPTHER; 186 if ("sintol".equals(codeString)) 187 return SINTOL; 188 if ("surg".equals(codeString)) 189 return SURG; 190 if ("washout".equals(codeString)) 191 return WASHOUT; 192 if ("outofstock".equals(codeString)) 193 return OUTOFSTOCK; 194 if ("offmarket".equals(codeString)) 195 return OFFMARKET; 196 throw new FHIRException("Unknown MedicationdispenseStatusReason code '" + codeString + "'"); 197 } 198 199 public String toCode() { 200 switch (this) { 201 case FRR01: 202 return "frr01"; 203 case FRR02: 204 return "frr02"; 205 case FRR03: 206 return "frr03"; 207 case FRR04: 208 return "frr04"; 209 case FRR05: 210 return "frr05"; 211 case FRR06: 212 return "frr06"; 213 case ALTCHOICE: 214 return "altchoice"; 215 case CLARIF: 216 return "clarif"; 217 case DRUGHIGH: 218 return "drughigh"; 219 case HOSPADM: 220 return "hospadm"; 221 case LABINT: 222 return "labint"; 223 case NONAVAIL: 224 return "non-avail"; 225 case PREG: 226 return "preg"; 227 case SAIG: 228 return "saig"; 229 case SDDI: 230 return "sddi"; 231 case SDUPTHER: 232 return "sdupther"; 233 case SINTOL: 234 return "sintol"; 235 case SURG: 236 return "surg"; 237 case WASHOUT: 238 return "washout"; 239 case OUTOFSTOCK: 240 return "outofstock"; 241 case OFFMARKET: 242 return "offmarket"; 243 case NULL: 244 return null; 245 default: 246 return "?"; 247 } 248 } 249 250 public String getSystem() { 251 return "http://terminology.hl7.org/fhir/CodeSystem/medicationdispense-status-reason"; 252 } 253 254 public String getDefinition() { 255 switch (this) { 256 case FRR01: 257 return "The order has been stopped by the prescriber but this fact has not necessarily captured electronically. Example: A verbal stop, a fax, etc."; 258 case FRR02: 259 return "Order has not been fulfilled within a reasonable amount of time, and might not be current."; 260 case FRR03: 261 return "Data needed to safely act on the order which was expected to become available independent of the order is not yet available. Example: Lab results, diagnostic imaging, etc."; 262 case FRR04: 263 return "Product not available or manufactured. Cannot supply."; 264 case FRR05: 265 return "The dispenser has ethical, religious or moral objections to fulfilling the order/dispensing the product."; 266 case FRR06: 267 return "Fulfiller not able to provide appropriate care associated with fulfilling the order. Example: Therapy requires ongoing monitoring by fulfiller and fulfiller will be ending practice, leaving town, unable to schedule necessary time, etc."; 268 case ALTCHOICE: 269 return "This therapy has been ordered as a backup to a preferred therapy. This order will be released when and if the preferred therapy is unsuccessful."; 270 case CLARIF: 271 return "Clarification is required before the order can be acted upon."; 272 case DRUGHIGH: 273 return "The current level of the medication in the patient's system is too high. The medication is suspended to allow the level to subside to a safer level."; 274 case HOSPADM: 275 return "The patient has been admitted to a care facility and their community medications are suspended until hospital discharge."; 276 case LABINT: 277 return "The therapy would interfere with a planned lab test and the therapy is being withdrawn until the test is completed."; 278 case NONAVAIL: 279 return "Patient not available for a period of time due to a scheduled therapy, leave of absence or other reason."; 280 case PREG: 281 return "The patient is pregnant or breast feeding. The therapy will be resumed when the pregnancy is complete and the patient is no longer breastfeeding."; 282 case SAIG: 283 return "The patient is believed to be allergic to a substance that is part of the therapy and the therapy is being temporarily withdrawn to confirm."; 284 case SDDI: 285 return "The drug interacts with a short-term treatment that is more urgently required. This order will be resumed when the short-term treatment is complete."; 286 case SDUPTHER: 287 return "Another short-term co-occurring therapy fulfills the same purpose as this therapy. This therapy will be resumed when the co-occuring therapy is complete."; 288 case SINTOL: 289 return "The patient is believed to have an intolerance to a substance that is part of the therapy and the therapy is being temporarily withdrawn to confirm."; 290 case SURG: 291 return "The drug is contraindicated for patients receiving surgery and the patient is scheduled to be admitted for surgery in the near future. The drug will be resumed when the patient has sufficiently recovered from the surgery."; 292 case WASHOUT: 293 return "The patient was previously receiving a medication contraindicated with the current medication. The current medication will remain on hold until the prior medication has been cleansed from their system."; 294 case OUTOFSTOCK: 295 return "Drug out of stock. Cannot supply."; 296 case OFFMARKET: 297 return "Drug no longer marketed Cannot supply."; 298 case NULL: 299 return null; 300 default: 301 return "?"; 302 } 303 } 304 305 public String getDisplay() { 306 switch (this) { 307 case FRR01: 308 return "Order Stopped"; 309 case FRR02: 310 return "Stale-dated Order"; 311 case FRR03: 312 return "Incomplete data"; 313 case FRR04: 314 return "Product unavailable"; 315 case FRR05: 316 return "Ethical/religious"; 317 case FRR06: 318 return "Unable to provide care"; 319 case ALTCHOICE: 320 return "Try another treatment first"; 321 case CLARIF: 322 return "Prescription/Request requires clarification"; 323 case DRUGHIGH: 324 return "Drug level too high"; 325 case HOSPADM: 326 return "Admission to hospital"; 327 case LABINT: 328 return "Lab interference issues"; 329 case NONAVAIL: 330 return "Patient not available"; 331 case PREG: 332 return "Patient is pregnant or breastfeeding"; 333 case SAIG: 334 return "Allergy"; 335 case SDDI: 336 return "Drug interacts with another drug"; 337 case SDUPTHER: 338 return "Duplicate therapy"; 339 case SINTOL: 340 return "Suspected intolerance"; 341 case SURG: 342 return "Patient scheduled for surgery"; 343 case WASHOUT: 344 return "Washout"; 345 case OUTOFSTOCK: 346 return "Drug not available - out of stock"; 347 case OFFMARKET: 348 return "Drug not available - off market"; 349 case NULL: 350 return null; 351 default: 352 return "?"; 353 } 354 } 355 356}