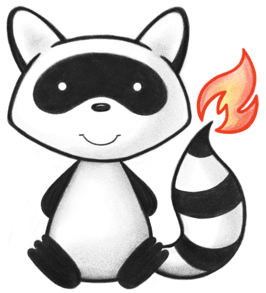
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum NameUse { 037 038 /** 039 * Known as/conventional/the one you normally use. 040 */ 041 USUAL, 042 /** 043 * The formal name as registered in an official (government) registry, but which 044 * name might not be commonly used. May be called "legal name". 045 */ 046 OFFICIAL, 047 /** 048 * A temporary name. Name.period can provide more detailed information. This may 049 * also be used for temporary names assigned at birth or in emergency 050 * situations. 051 */ 052 TEMP, 053 /** 054 * A name that is used to address the person in an informal manner, but is not 055 * part of their formal or usual name. 056 */ 057 NICKNAME, 058 /** 059 * Anonymous assigned name, alias, or pseudonym (used to protect a person's 060 * identity for privacy reasons). 061 */ 062 ANONYMOUS, 063 /** 064 * This name is no longer in use (or was never correct, but retained for 065 * records). 066 */ 067 OLD, 068 /** 069 * A name used prior to changing name because of marriage. This name use is for 070 * use by applications that collect and store names that were used prior to a 071 * marriage. Marriage naming customs vary greatly around the world, and are 072 * constantly changing. This term is not gender specific. The use of this term 073 * does not imply any particular history for a person's name. 074 */ 075 MAIDEN, 076 /** 077 * added to help the parsers 078 */ 079 NULL; 080 081 public static NameUse fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("usual".equals(codeString)) 085 return USUAL; 086 if ("official".equals(codeString)) 087 return OFFICIAL; 088 if ("temp".equals(codeString)) 089 return TEMP; 090 if ("nickname".equals(codeString)) 091 return NICKNAME; 092 if ("anonymous".equals(codeString)) 093 return ANONYMOUS; 094 if ("old".equals(codeString)) 095 return OLD; 096 if ("maiden".equals(codeString)) 097 return MAIDEN; 098 throw new FHIRException("Unknown NameUse code '" + codeString + "'"); 099 } 100 101 public String toCode() { 102 switch (this) { 103 case USUAL: 104 return "usual"; 105 case OFFICIAL: 106 return "official"; 107 case TEMP: 108 return "temp"; 109 case NICKNAME: 110 return "nickname"; 111 case ANONYMOUS: 112 return "anonymous"; 113 case OLD: 114 return "old"; 115 case MAIDEN: 116 return "maiden"; 117 case NULL: 118 return null; 119 default: 120 return "?"; 121 } 122 } 123 124 public String getSystem() { 125 return "http://hl7.org/fhir/name-use"; 126 } 127 128 public String getDefinition() { 129 switch (this) { 130 case USUAL: 131 return "Known as/conventional/the one you normally use."; 132 case OFFICIAL: 133 return "The formal name as registered in an official (government) registry, but which name might not be commonly used. May be called \"legal name\"."; 134 case TEMP: 135 return "A temporary name. Name.period can provide more detailed information. This may also be used for temporary names assigned at birth or in emergency situations."; 136 case NICKNAME: 137 return "A name that is used to address the person in an informal manner, but is not part of their formal or usual name."; 138 case ANONYMOUS: 139 return "Anonymous assigned name, alias, or pseudonym (used to protect a person's identity for privacy reasons)."; 140 case OLD: 141 return "This name is no longer in use (or was never correct, but retained for records)."; 142 case MAIDEN: 143 return "A name used prior to changing name because of marriage. This name use is for use by applications that collect and store names that were used prior to a marriage. Marriage naming customs vary greatly around the world, and are constantly changing. This term is not gender specific. The use of this term does not imply any particular history for a person's name."; 144 case NULL: 145 return null; 146 default: 147 return "?"; 148 } 149 } 150 151 public String getDisplay() { 152 switch (this) { 153 case USUAL: 154 return "Usual"; 155 case OFFICIAL: 156 return "Official"; 157 case TEMP: 158 return "Temp"; 159 case NICKNAME: 160 return "Nickname"; 161 case ANONYMOUS: 162 return "Anonymous"; 163 case OLD: 164 return "Old"; 165 case MAIDEN: 166 return "Name changed for Marriage"; 167 case NULL: 168 return null; 169 default: 170 return "?"; 171 } 172 } 173 174}