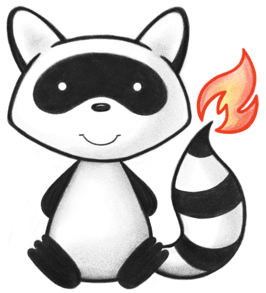
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ObjectRole { 037 038 /** 039 * This object is the patient that is the subject of care related to this event. 040 * It is identifiable by patient ID or equivalent. The patient may be either 041 * human or animal. 042 */ 043 _1, 044 /** 045 * This is a location identified as related to the event. This is usually the 046 * location where the event took place. Note that for shipping, the usual events 047 * are arrival at a location or departure from a location. 048 */ 049 _2, 050 /** 051 * This object is any kind of persistent document created as a result of the 052 * event. This could be a paper report, film, electronic report, DICOM Study, 053 * etc. Issues related to medical records life cycle management are conveyed 054 * elsewhere. 055 */ 056 _3, 057 /** 058 * A logical object related to a health record event. This is any healthcare 059 * specific resource (object) not restricted to FHIR defined Resources. 060 */ 061 _4, 062 /** 063 * This is any configurable file used to control creation of documents. Examples 064 * include the objects maintained by the HL7 Master File transactions, Value 065 * Sets, etc. 066 */ 067 _5, 068 /** 069 * A human participant not otherwise identified by some other category. 070 */ 071 _6, 072 /** 073 * (deprecated). 074 */ 075 _7, 076 /** 077 * Typically, a licensed person who is providing or performing care related to 078 * the event, generally a physician. The key distinction between doctor and 079 * practitioner is with regards to their role, not the licensing. The doctor is 080 * the human who actually performed the work. The practitioner is the human or 081 * organization that is responsible for the work. 082 */ 083 _8, 084 /** 085 * A person or system that is being notified as part of the event. This is 086 * relevant in situations where automated systems provide notifications to other 087 * parties when an event took place. 088 */ 089 _9, 090 /** 091 * Insurance company, or any other organization who accepts responsibility for 092 * paying for the healthcare event. 093 */ 094 _10, 095 /** 096 * A person or active system object involved in the event with a security role. 097 */ 098 _11, 099 /** 100 * A person or system object involved in the event with the authority to modify 101 * security roles of other objects. 102 */ 103 _12, 104 /** 105 * A passive object, such as a role table, that is relevant to the event. 106 */ 107 _13, 108 /** 109 * (deprecated) Relevant to certain RBAC security methodologies. 110 */ 111 _14, 112 /** 113 * Any person or organization responsible for providing care. This encompasses 114 * all forms of care, licensed or otherwise, and all sorts of teams and care 115 * groups. Note the distinction between practitioner and the doctor that 116 * actually provided the care to the patient. 117 */ 118 _15, 119 /** 120 * The source or destination for data transfer, when it does not match some 121 * other role. 122 */ 123 _16, 124 /** 125 * A source or destination for data transfer that acts as an archive, database, 126 * or similar role. 127 */ 128 _17, 129 /** 130 * An object that holds schedule information. This could be an appointment book, 131 * availability information, etc. 132 */ 133 _18, 134 /** 135 * An organization or person that is the recipient of services. This could be an 136 * organization that is buying services for a patient, or a person that is 137 * buying services for an animal. 138 */ 139 _19, 140 /** 141 * An order, task, work item, procedure step, or other description of work to be 142 * performed; e.g. a particular instance of an MPPS. 143 */ 144 _20, 145 /** 146 * A list of jobs or a system that provides lists of jobs; e.g. an MWL SCP. 147 */ 148 _21, 149 /** 150 * (Deprecated). 151 */ 152 _22, 153 /** 154 * An object that specifies or controls the routing or delivery of items. For 155 * example, a distribution list is the routing criteria for mail. The items 156 * delivered may be documents, jobs, or other objects. 157 */ 158 _23, 159 /** 160 * The contents of a query. This is used to capture the contents of any kind of 161 * query. For security surveillance purposes knowing the queries being made is 162 * very important. 163 */ 164 _24, 165 /** 166 * added to help the parsers 167 */ 168 NULL; 169 170 public static ObjectRole fromCode(String codeString) throws FHIRException { 171 if (codeString == null || "".equals(codeString)) 172 return null; 173 if ("1".equals(codeString)) 174 return _1; 175 if ("2".equals(codeString)) 176 return _2; 177 if ("3".equals(codeString)) 178 return _3; 179 if ("4".equals(codeString)) 180 return _4; 181 if ("5".equals(codeString)) 182 return _5; 183 if ("6".equals(codeString)) 184 return _6; 185 if ("7".equals(codeString)) 186 return _7; 187 if ("8".equals(codeString)) 188 return _8; 189 if ("9".equals(codeString)) 190 return _9; 191 if ("10".equals(codeString)) 192 return _10; 193 if ("11".equals(codeString)) 194 return _11; 195 if ("12".equals(codeString)) 196 return _12; 197 if ("13".equals(codeString)) 198 return _13; 199 if ("14".equals(codeString)) 200 return _14; 201 if ("15".equals(codeString)) 202 return _15; 203 if ("16".equals(codeString)) 204 return _16; 205 if ("17".equals(codeString)) 206 return _17; 207 if ("18".equals(codeString)) 208 return _18; 209 if ("19".equals(codeString)) 210 return _19; 211 if ("20".equals(codeString)) 212 return _20; 213 if ("21".equals(codeString)) 214 return _21; 215 if ("22".equals(codeString)) 216 return _22; 217 if ("23".equals(codeString)) 218 return _23; 219 if ("24".equals(codeString)) 220 return _24; 221 throw new FHIRException("Unknown ObjectRole code '" + codeString + "'"); 222 } 223 224 public String toCode() { 225 switch (this) { 226 case _1: 227 return "1"; 228 case _2: 229 return "2"; 230 case _3: 231 return "3"; 232 case _4: 233 return "4"; 234 case _5: 235 return "5"; 236 case _6: 237 return "6"; 238 case _7: 239 return "7"; 240 case _8: 241 return "8"; 242 case _9: 243 return "9"; 244 case _10: 245 return "10"; 246 case _11: 247 return "11"; 248 case _12: 249 return "12"; 250 case _13: 251 return "13"; 252 case _14: 253 return "14"; 254 case _15: 255 return "15"; 256 case _16: 257 return "16"; 258 case _17: 259 return "17"; 260 case _18: 261 return "18"; 262 case _19: 263 return "19"; 264 case _20: 265 return "20"; 266 case _21: 267 return "21"; 268 case _22: 269 return "22"; 270 case _23: 271 return "23"; 272 case _24: 273 return "24"; 274 case NULL: 275 return null; 276 default: 277 return "?"; 278 } 279 } 280 281 public String getSystem() { 282 return "http://terminology.hl7.org/CodeSystem/object-role"; 283 } 284 285 public String getDefinition() { 286 switch (this) { 287 case _1: 288 return "This object is the patient that is the subject of care related to this event. It is identifiable by patient ID or equivalent. The patient may be either human or animal."; 289 case _2: 290 return "This is a location identified as related to the event. This is usually the location where the event took place. Note that for shipping, the usual events are arrival at a location or departure from a location."; 291 case _3: 292 return "This object is any kind of persistent document created as a result of the event. This could be a paper report, film, electronic report, DICOM Study, etc. Issues related to medical records life cycle management are conveyed elsewhere."; 293 case _4: 294 return "A logical object related to a health record event. This is any healthcare specific resource (object) not restricted to FHIR defined Resources."; 295 case _5: 296 return "This is any configurable file used to control creation of documents. Examples include the objects maintained by the HL7 Master File transactions, Value Sets, etc."; 297 case _6: 298 return "A human participant not otherwise identified by some other category."; 299 case _7: 300 return "(deprecated)."; 301 case _8: 302 return "Typically, a licensed person who is providing or performing care related to the event, generally a physician. The key distinction between doctor and practitioner is with regards to their role, not the licensing. The doctor is the human who actually performed the work. The practitioner is the human or organization that is responsible for the work."; 303 case _9: 304 return "A person or system that is being notified as part of the event. This is relevant in situations where automated systems provide notifications to other parties when an event took place."; 305 case _10: 306 return "Insurance company, or any other organization who accepts responsibility for paying for the healthcare event."; 307 case _11: 308 return "A person or active system object involved in the event with a security role."; 309 case _12: 310 return "A person or system object involved in the event with the authority to modify security roles of other objects."; 311 case _13: 312 return "A passive object, such as a role table, that is relevant to the event."; 313 case _14: 314 return "(deprecated) Relevant to certain RBAC security methodologies."; 315 case _15: 316 return "Any person or organization responsible for providing care. This encompasses all forms of care, licensed or otherwise, and all sorts of teams and care groups. Note the distinction between practitioner and the doctor that actually provided the care to the patient."; 317 case _16: 318 return "The source or destination for data transfer, when it does not match some other role."; 319 case _17: 320 return "A source or destination for data transfer that acts as an archive, database, or similar role."; 321 case _18: 322 return "An object that holds schedule information. This could be an appointment book, availability information, etc."; 323 case _19: 324 return "An organization or person that is the recipient of services. This could be an organization that is buying services for a patient, or a person that is buying services for an animal."; 325 case _20: 326 return "An order, task, work item, procedure step, or other description of work to be performed; e.g. a particular instance of an MPPS."; 327 case _21: 328 return "A list of jobs or a system that provides lists of jobs; e.g. an MWL SCP."; 329 case _22: 330 return "(Deprecated)."; 331 case _23: 332 return "An object that specifies or controls the routing or delivery of items. For example, a distribution list is the routing criteria for mail. The items delivered may be documents, jobs, or other objects."; 333 case _24: 334 return "The contents of a query. This is used to capture the contents of any kind of query. For security surveillance purposes knowing the queries being made is very important."; 335 case NULL: 336 return null; 337 default: 338 return "?"; 339 } 340 } 341 342 public String getDisplay() { 343 switch (this) { 344 case _1: 345 return "Patient"; 346 case _2: 347 return "Location"; 348 case _3: 349 return "Report"; 350 case _4: 351 return "Domain Resource"; 352 case _5: 353 return "Master file"; 354 case _6: 355 return "User"; 356 case _7: 357 return "List"; 358 case _8: 359 return "Doctor"; 360 case _9: 361 return "Subscriber"; 362 case _10: 363 return "Guarantor"; 364 case _11: 365 return "Security User Entity"; 366 case _12: 367 return "Security User Group"; 368 case _13: 369 return "Security Resource"; 370 case _14: 371 return "Security Granularity Definition"; 372 case _15: 373 return "Practitioner"; 374 case _16: 375 return "Data Destination"; 376 case _17: 377 return "Data Repository"; 378 case _18: 379 return "Schedule"; 380 case _19: 381 return "Customer"; 382 case _20: 383 return "Job"; 384 case _21: 385 return "Job Stream"; 386 case _22: 387 return "Table"; 388 case _23: 389 return "Routing Criteria"; 390 case _24: 391 return "Query"; 392 case NULL: 393 return null; 394 default: 395 return "?"; 396 } 397 } 398 399}