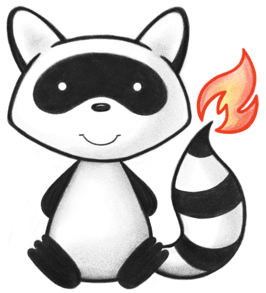
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ProcedureProgressStatusCodes { 037 038 /** 039 * A patient is in the Operating Room. 040 */ 041 INOPERATINGROOM, 042 /** 043 * The patient is prepared for a procedure. 044 */ 045 PREPARED, 046 /** 047 * The patient is under anesthesia. 048 */ 049 ANESTHESIAINDUCED, 050 /** 051 * The patient has open incision(s). 052 */ 053 OPENINCISION, 054 /** 055 * The patient has incision(s) closed. 056 */ 057 CLOSEDINCISION, 058 /** 059 * The patient is in the recovery room. 060 */ 061 INRECOVERYROOM, 062 /** 063 * added to help the parsers 064 */ 065 NULL; 066 067 public static ProcedureProgressStatusCodes fromCode(String codeString) throws FHIRException { 068 if (codeString == null || "".equals(codeString)) 069 return null; 070 if ("in-operating-room".equals(codeString)) 071 return INOPERATINGROOM; 072 if ("prepared".equals(codeString)) 073 return PREPARED; 074 if ("anesthesia-induced".equals(codeString)) 075 return ANESTHESIAINDUCED; 076 if ("open-incision".equals(codeString)) 077 return OPENINCISION; 078 if ("closed-incision".equals(codeString)) 079 return CLOSEDINCISION; 080 if ("in-recovery-room".equals(codeString)) 081 return INRECOVERYROOM; 082 throw new FHIRException("Unknown ProcedureProgressStatusCodes code '" + codeString + "'"); 083 } 084 085 public String toCode() { 086 switch (this) { 087 case INOPERATINGROOM: 088 return "in-operating-room"; 089 case PREPARED: 090 return "prepared"; 091 case ANESTHESIAINDUCED: 092 return "anesthesia-induced"; 093 case OPENINCISION: 094 return "open-incision"; 095 case CLOSEDINCISION: 096 return "closed-incision"; 097 case INRECOVERYROOM: 098 return "in-recovery-room"; 099 case NULL: 100 return null; 101 default: 102 return "?"; 103 } 104 } 105 106 public String getSystem() { 107 return "http://hl7.org/fhir/procedure-progress-status-code"; 108 } 109 110 public String getDefinition() { 111 switch (this) { 112 case INOPERATINGROOM: 113 return "A patient is in the Operating Room."; 114 case PREPARED: 115 return "The patient is prepared for a procedure."; 116 case ANESTHESIAINDUCED: 117 return "The patient is under anesthesia."; 118 case OPENINCISION: 119 return "The patient has open incision(s)."; 120 case CLOSEDINCISION: 121 return "The patient has incision(s) closed."; 122 case INRECOVERYROOM: 123 return "The patient is in the recovery room."; 124 case NULL: 125 return null; 126 default: 127 return "?"; 128 } 129 } 130 131 public String getDisplay() { 132 switch (this) { 133 case INOPERATINGROOM: 134 return "In Operating Room"; 135 case PREPARED: 136 return "Prepared"; 137 case ANESTHESIAINDUCED: 138 return "Anesthesia Induced"; 139 case OPENINCISION: 140 return "Open Incision"; 141 case CLOSEDINCISION: 142 return "Closed Incision"; 143 case INRECOVERYROOM: 144 return "In Recovery Room"; 145 case NULL: 146 return null; 147 default: 148 return "?"; 149 } 150 } 151 152}